diff --git a/css/styles-projects.css b/css/styles-projects.css index 05586da82d10f010f66eb0d58c7905702af0cb1d..fdb878626125223ada48911af9c19148cc8bf3ef 100644 --- a/css/styles-projects.css +++ b/css/styles-projects.css @@ -7,6 +7,13 @@ font-weight: normal; font-style: normal; } + + @font-face { + font-family: "GinesoCondMedium"; + src: url("../fonts/Gineso/GinesoCondMedium.otf") format("opentype"); + font-weight: normal; + font-style: normal; + } /* --------------------------------------- 2) Global Reset & Body Styles @@ -128,15 +135,36 @@ } .project-description { + font-family: "GinesoCondMedium"; flex: 1 1 55%; } .project-description h2 { + font-family: "GinesoCondMedium"; font-size: clamp(1.5rem, 3vw, 2rem); margin-bottom: 0.5rem; } .project-description p { + font-family: "GinesoCondMedium"; font-size: clamp(1rem, 2.5vw, 1.2rem); } - \ No newline at end of file + + /* --------------------------------------- + 7) SVJ CONTAINERS + --------------------------------------- */ + + .svg-container{ + position: absolute; + top: 0; + left: 0; + display: flex; + align-items: center; + justify-content: center; + width: 100%; + height: 6473px; + } + + svg{ + height: 100%; + } diff --git a/js/projects.js b/js/projects.js index 3466f1ee90177f105c7245ae515af02279e7a80a..912aa90c3bd8eccb26c21990daed2bcf0f5cc8f2 100644 --- a/js/projects.js +++ b/js/projects.js @@ -1,22 +1,31 @@ -$(document).ready(function () { - // Initial position of the line - var $line = $(".vertical-line"); - var $window = $(window); +// Register GSAP Plugins +gsap.registerPlugin(ScrollTrigger); - // Function to update the line position on scroll - function updateLinePosition() { - var scrollPercentage = - ($window.scrollTop() / ($("body").height() - $window.height())) * 100; - var newHeight = (scrollPercentage / 100) * ($window.height() * 3.44); +// Select the SVG path +let svg = document.querySelector("svg"); +let path = svg.querySelector("path"); - $line.css("height", newHeight + 20 + "px"); - } +// Get the total path length +const pathLength = path.getTotalLength(); + +console.log("Path Length:", pathLength); // Debugging line to check length - // Update the line position on page load - updateLinePosition(); +// Set initial strokeDashArray and strokeDashOffset +gsap.set(path, { strokeDasharray: pathLength, strokeDashoffset: pathLength }); - // Update the line position on scroll - $window.scroll(function () { - updateLinePosition(); - }); -}); \ No newline at end of file +// Create the scroll-based animation +gsap.fromTo( + path, + { strokeDashoffset: pathLength }, + { + strokeDashoffset: 0, + duration: 5, + ease: "none", + scrollTrigger: { + trigger: ".svg-container", // Make sure this is the right selector + start: "top top", + end: "bottom bottom", + scrub: 1, + } + } +); diff --git a/node_modules/.package-lock.json b/node_modules/.package-lock.json new file mode 100644 index 0000000000000000000000000000000000000000..d7a88fdee0b12661d72fc1a47ded6c7aa924377d --- /dev/null +++ b/node_modules/.package-lock.json @@ -0,0 +1,13 @@ +{ + "name": "Personal-Portfolio", + "lockfileVersion": 3, + "requires": true, + "packages": { + "node_modules/gsap": { + "version": "3.12.7", + "resolved": "https://registry.npmjs.org/gsap/-/gsap-3.12.7.tgz", + "integrity": "sha512-V4GsyVamhmKefvcAKaoy0h6si0xX7ogwBoBSs2CTJwt7luW0oZzC0LhdkyuKV8PJAXr7Yaj8pMjCKD4GJ+eEMg==", + "license": "Standard 'no charge' license: https://gsap.com/standard-license. Club GSAP members get more: https://gsap.com/licensing/. Why GreenSock doesn't employ an MIT license: https://gsap.com/why-license/" + } + } +} diff --git a/node_modules/gsap/CSSPlugin.js b/node_modules/gsap/CSSPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..20a8f3fd42de6b489bddb3288c13dec8fb063232 --- /dev/null +++ b/node_modules/gsap/CSSPlugin.js @@ -0,0 +1,1573 @@ +/*! + * CSSPlugin 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +import { gsap, _getProperty, _numExp, _numWithUnitExp, getUnit, _isString, _isUndefined, _renderComplexString, _relExp, _forEachName, _sortPropTweensByPriority, _colorStringFilter, _checkPlugin, _replaceRandom, _plugins, GSCache, PropTween, _config, _ticker, _round, _missingPlugin, _getSetter, _getCache, _colorExp, _parseRelative, _setDefaults, _removeLinkedListItem //for the commented-out className feature. +} from "./gsap-core.js"; + +var _win, + _doc, + _docElement, + _pluginInitted, + _tempDiv, + _tempDivStyler, + _recentSetterPlugin, + _reverting, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _transformProps = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _atan2 = Math.atan2, + _bigNum = 1e8, + _capsExp = /([A-Z])/g, + _horizontalExp = /(left|right|width|margin|padding|x)/i, + _complexExp = /[\s,\(]\S/, + _propertyAliases = { + autoAlpha: "opacity,visibility", + scale: "scaleX,scaleY", + alpha: "opacity" +}, + _renderCSSProp = function _renderCSSProp(ratio, data) { + return data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u, data); +}, + _renderPropWithEnd = function _renderPropWithEnd(ratio, data) { + return data.set(data.t, data.p, ratio === 1 ? data.e : Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u, data); +}, + _renderCSSPropWithBeginning = function _renderCSSPropWithBeginning(ratio, data) { + return data.set(data.t, data.p, ratio ? Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u : data.b, data); +}, + //if units change, we need a way to render the original unit/value when the tween goes all the way back to the beginning (ratio:0) +_renderRoundedCSSProp = function _renderRoundedCSSProp(ratio, data) { + var value = data.s + data.c * ratio; + data.set(data.t, data.p, ~~(value + (value < 0 ? -.5 : .5)) + data.u, data); +}, + _renderNonTweeningValue = function _renderNonTweeningValue(ratio, data) { + return data.set(data.t, data.p, ratio ? data.e : data.b, data); +}, + _renderNonTweeningValueOnlyAtEnd = function _renderNonTweeningValueOnlyAtEnd(ratio, data) { + return data.set(data.t, data.p, ratio !== 1 ? data.b : data.e, data); +}, + _setterCSSStyle = function _setterCSSStyle(target, property, value) { + return target.style[property] = value; +}, + _setterCSSProp = function _setterCSSProp(target, property, value) { + return target.style.setProperty(property, value); +}, + _setterTransform = function _setterTransform(target, property, value) { + return target._gsap[property] = value; +}, + _setterScale = function _setterScale(target, property, value) { + return target._gsap.scaleX = target._gsap.scaleY = value; +}, + _setterScaleWithRender = function _setterScaleWithRender(target, property, value, data, ratio) { + var cache = target._gsap; + cache.scaleX = cache.scaleY = value; + cache.renderTransform(ratio, cache); +}, + _setterTransformWithRender = function _setterTransformWithRender(target, property, value, data, ratio) { + var cache = target._gsap; + cache[property] = value; + cache.renderTransform(ratio, cache); +}, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _saveStyle = function _saveStyle(property, isNotCSS) { + var _this = this; + + var target = this.target, + style = target.style, + cache = target._gsap; + + if (property in _transformProps && style) { + this.tfm = this.tfm || {}; + + if (property !== "transform") { + property = _propertyAliases[property] || property; + ~property.indexOf(",") ? property.split(",").forEach(function (a) { + return _this.tfm[a] = _get(target, a); + }) : this.tfm[property] = cache.x ? cache[property] : _get(target, property); // note: scale would map to "scaleX,scaleY", thus we loop and apply them both. + + property === _transformOriginProp && (this.tfm.zOrigin = cache.zOrigin); + } else { + return _propertyAliases.transform.split(",").forEach(function (p) { + return _saveStyle.call(_this, p, isNotCSS); + }); + } + + if (this.props.indexOf(_transformProp) >= 0) { + return; + } + + if (cache.svg) { + this.svgo = target.getAttribute("data-svg-origin"); + this.props.push(_transformOriginProp, isNotCSS, ""); + } + + property = _transformProp; + } + + (style || isNotCSS) && this.props.push(property, isNotCSS, style[property]); +}, + _removeIndependentTransforms = function _removeIndependentTransforms(style) { + if (style.translate) { + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } +}, + _revertStyle = function _revertStyle() { + var props = this.props, + target = this.target, + style = target.style, + cache = target._gsap, + i, + p; + + for (i = 0; i < props.length; i += 3) { + // stored like this: property, isNotCSS, value + if (!props[i + 1]) { + props[i + 2] ? style[props[i]] = props[i + 2] : style.removeProperty(props[i].substr(0, 2) === "--" ? props[i] : props[i].replace(_capsExp, "-$1").toLowerCase()); + } else if (props[i + 1] === 2) { + // non-CSS value (function-based) + target[props[i]](props[i + 2]); + } else { + // non-CSS value (not function-based) + target[props[i]] = props[i + 2]; + } + } + + if (this.tfm) { + for (p in this.tfm) { + cache[p] = this.tfm[p]; + } + + if (cache.svg) { + cache.renderTransform(); + target.setAttribute("data-svg-origin", this.svgo || ""); + } + + i = _reverting(); + + if ((!i || !i.isStart) && !style[_transformProp]) { + _removeIndependentTransforms(style); + + if (cache.zOrigin && style[_transformOriginProp]) { + style[_transformOriginProp] += " " + cache.zOrigin + "px"; // since we're uncaching, we must put the zOrigin back into the transformOrigin so that we can pull it out accurately when we parse again. Otherwise, we'd lose the z portion of the origin since we extract it to protect from Safari bugs. + + cache.zOrigin = 0; + cache.renderTransform(); + } + + cache.uncache = 1; // if it's a startAt that's being reverted in the _initTween() of the core, we don't need to uncache transforms. This is purely a performance optimization. + } + } +}, + _getStyleSaver = function _getStyleSaver(target, properties) { + var saver = { + target: target, + props: [], + revert: _revertStyle, + save: _saveStyle + }; + target._gsap || gsap.core.getCache(target); // just make sure there's a _gsap cache defined because we read from it in _saveStyle() and it's more efficient to just check it here once. + + properties && target.style && target.nodeType && properties.split(",").forEach(function (p) { + return saver.save(p); + }); // make sure it's a DOM node too. + + return saver; +}, + _supports3D, + _createElement = function _createElement(type, ns) { + var e = _doc.createElementNS ? _doc.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc.createElement(type); //some servers swap in https for http in the namespace which can break things, making "style" inaccessible. + + return e && e.style ? e : _doc.createElement(type); //some environments won't allow access to the element's style when created with a namespace in which case we default to the standard createElement() to work around the issue. Also note that when GSAP is embedded directly inside an SVG file, createElement() won't allow access to the style object in Firefox (see https://gsap.com/forums/topic/20215-problem-using-tweenmax-in-standalone-self-containing-svg-file-err-cannot-set-property-csstext-of-undefined/). +}, + _getComputedProperty = function _getComputedProperty(target, property, skipPrefixFallback) { + var cs = getComputedStyle(target); + return cs[property] || cs.getPropertyValue(property.replace(_capsExp, "-$1").toLowerCase()) || cs.getPropertyValue(property) || !skipPrefixFallback && _getComputedProperty(target, _checkPropPrefix(property) || property, 1) || ""; //css variables may not need caps swapped out for dashes and lowercase. +}, + _prefixes = "O,Moz,ms,Ms,Webkit".split(","), + _checkPropPrefix = function _checkPropPrefix(property, element, preferPrefix) { + var e = element || _tempDiv, + s = e.style, + i = 5; + + if (property in s && !preferPrefix) { + return property; + } + + property = property.charAt(0).toUpperCase() + property.substr(1); + + while (i-- && !(_prefixes[i] + property in s)) {} + + return i < 0 ? null : (i === 3 ? "ms" : i >= 0 ? _prefixes[i] : "") + property; +}, + _initCore = function _initCore() { + if (_windowExists() && window.document) { + _win = window; + _doc = _win.document; + _docElement = _doc.documentElement; + _tempDiv = _createElement("div") || { + style: {} + }; + _tempDivStyler = _createElement("div"); + _transformProp = _checkPropPrefix(_transformProp); + _transformOriginProp = _transformProp + "Origin"; + _tempDiv.style.cssText = "border-width:0;line-height:0;position:absolute;padding:0"; //make sure to override certain properties that may contaminate measurements, in case the user has overreaching style sheets. + + _supports3D = !!_checkPropPrefix("perspective"); + _reverting = gsap.core.reverting; + _pluginInitted = 1; + } +}, + _getReparentedCloneBBox = function _getReparentedCloneBBox(target) { + //works around issues in some browsers (like Firefox) that don't correctly report getBBox() on SVG elements inside a <defs> element and/or <mask>. We try creating an SVG, adding it to the documentElement and toss the element in there so that it's definitely part of the rendering tree, then grab the bbox and if it works, we actually swap out the original getBBox() method for our own that does these extra steps whenever getBBox is needed. This helps ensure that performance is optimal (only do all these extra steps when absolutely necessary...most elements don't need it). + var owner = target.ownerSVGElement, + svg = _createElement("svg", owner && owner.getAttribute("xmlns") || "http://www.w3.org/2000/svg"), + clone = target.cloneNode(true), + bbox; + + clone.style.display = "block"; + svg.appendChild(clone); + + _docElement.appendChild(svg); + + try { + bbox = clone.getBBox(); + } catch (e) {} + + svg.removeChild(clone); + + _docElement.removeChild(svg); + + return bbox; +}, + _getAttributeFallbacks = function _getAttributeFallbacks(target, attributesArray) { + var i = attributesArray.length; + + while (i--) { + if (target.hasAttribute(attributesArray[i])) { + return target.getAttribute(attributesArray[i]); + } + } +}, + _getBBox = function _getBBox(target) { + var bounds, cloned; + + try { + bounds = target.getBBox(); //Firefox throws errors if you try calling getBBox() on an SVG element that's not rendered (like in a <symbol> or <defs>). https://bugzilla.mozilla.org/show_bug.cgi?id=612118 + } catch (error) { + bounds = _getReparentedCloneBBox(target); + cloned = 1; + } + + bounds && (bounds.width || bounds.height) || cloned || (bounds = _getReparentedCloneBBox(target)); //some browsers (like Firefox) misreport the bounds if the element has zero width and height (it just assumes it's at x:0, y:0), thus we need to manually grab the position in that case. + + return bounds && !bounds.width && !bounds.x && !bounds.y ? { + x: +_getAttributeFallbacks(target, ["x", "cx", "x1"]) || 0, + y: +_getAttributeFallbacks(target, ["y", "cy", "y1"]) || 0, + width: 0, + height: 0 + } : bounds; +}, + _isSVG = function _isSVG(e) { + return !!(e.getCTM && (!e.parentNode || e.ownerSVGElement) && _getBBox(e)); +}, + //reports if the element is an SVG on which getBBox() actually works +_removeProperty = function _removeProperty(target, property) { + if (property) { + var style = target.style, + first2Chars; + + if (property in _transformProps && property !== _transformOriginProp) { + property = _transformProp; + } + + if (style.removeProperty) { + first2Chars = property.substr(0, 2); + + if (first2Chars === "ms" || property.substr(0, 6) === "webkit") { + //Microsoft and some Webkit browsers don't conform to the standard of capitalizing the first prefix character, so we adjust so that when we prefix the caps with a dash, it's correct (otherwise it'd be "ms-transform" instead of "-ms-transform" for IE9, for example) + property = "-" + property; + } + + style.removeProperty(first2Chars === "--" ? property : property.replace(_capsExp, "-$1").toLowerCase()); + } else { + //note: old versions of IE use "removeAttribute()" instead of "removeProperty()" + style.removeAttribute(property); + } + } +}, + _addNonTweeningPT = function _addNonTweeningPT(plugin, target, property, beginning, end, onlySetAtEnd) { + var pt = new PropTween(plugin._pt, target, property, 0, 1, onlySetAtEnd ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue); + plugin._pt = pt; + pt.b = beginning; + pt.e = end; + + plugin._props.push(property); + + return pt; +}, + _nonConvertibleUnits = { + deg: 1, + rad: 1, + turn: 1 +}, + _nonStandardLayouts = { + grid: 1, + flex: 1 +}, + //takes a single value like 20px and converts it to the unit specified, like "%", returning only the numeric amount. +_convertToUnit = function _convertToUnit(target, property, value, unit) { + var curValue = parseFloat(value) || 0, + curUnit = (value + "").trim().substr((curValue + "").length) || "px", + // some browsers leave extra whitespace at the beginning of CSS variables, hence the need to trim() + style = _tempDiv.style, + horizontal = _horizontalExp.test(property), + isRootSVG = target.tagName.toLowerCase() === "svg", + measureProperty = (isRootSVG ? "client" : "offset") + (horizontal ? "Width" : "Height"), + amount = 100, + toPixels = unit === "px", + toPercent = unit === "%", + px, + parent, + cache, + isSVG; + + if (unit === curUnit || !curValue || _nonConvertibleUnits[unit] || _nonConvertibleUnits[curUnit]) { + return curValue; + } + + curUnit !== "px" && !toPixels && (curValue = _convertToUnit(target, property, value, "px")); + isSVG = target.getCTM && _isSVG(target); + + if ((toPercent || curUnit === "%") && (_transformProps[property] || ~property.indexOf("adius"))) { + px = isSVG ? target.getBBox()[horizontal ? "width" : "height"] : target[measureProperty]; + return _round(toPercent ? curValue / px * amount : curValue / 100 * px); + } + + style[horizontal ? "width" : "height"] = amount + (toPixels ? curUnit : unit); + parent = unit !== "rem" && ~property.indexOf("adius") || unit === "em" && target.appendChild && !isRootSVG ? target : target.parentNode; + + if (isSVG) { + parent = (target.ownerSVGElement || {}).parentNode; + } + + if (!parent || parent === _doc || !parent.appendChild) { + parent = _doc.body; + } + + cache = parent._gsap; + + if (cache && toPercent && cache.width && horizontal && cache.time === _ticker.time && !cache.uncache) { + return _round(curValue / cache.width * amount); + } else { + if (toPercent && (property === "height" || property === "width")) { + // if we're dealing with width/height that's inside a container with padding and/or it's a flexbox/grid container, we must apply it to the target itself rather than the _tempDiv in order to ensure complete accuracy, factoring in the parent's padding. + var v = target.style[property]; + target.style[property] = amount + unit; + px = target[measureProperty]; + v ? target.style[property] = v : _removeProperty(target, property); + } else { + (toPercent || curUnit === "%") && !_nonStandardLayouts[_getComputedProperty(parent, "display")] && (style.position = _getComputedProperty(target, "position")); + parent === target && (style.position = "static"); // like for borderRadius, if it's a % we must have it relative to the target itself but that may not have position: relative or position: absolute in which case it'd go up the chain until it finds its offsetParent (bad). position: static protects against that. + + parent.appendChild(_tempDiv); + px = _tempDiv[measureProperty]; + parent.removeChild(_tempDiv); + style.position = "absolute"; + } + + if (horizontal && toPercent) { + cache = _getCache(parent); + cache.time = _ticker.time; + cache.width = parent[measureProperty]; + } + } + + return _round(toPixels ? px * curValue / amount : px && curValue ? amount / px * curValue : 0); +}, + _get = function _get(target, property, unit, uncache) { + var value; + _pluginInitted || _initCore(); + + if (property in _propertyAliases && property !== "transform") { + property = _propertyAliases[property]; + + if (~property.indexOf(",")) { + property = property.split(",")[0]; + } + } + + if (_transformProps[property] && property !== "transform") { + value = _parseTransform(target, uncache); + value = property !== "transformOrigin" ? value[property] : value.svg ? value.origin : _firstTwoOnly(_getComputedProperty(target, _transformOriginProp)) + " " + value.zOrigin + "px"; + } else { + value = target.style[property]; + + if (!value || value === "auto" || uncache || ~(value + "").indexOf("calc(")) { + value = _specialProps[property] && _specialProps[property](target, property, unit) || _getComputedProperty(target, property) || _getProperty(target, property) || (property === "opacity" ? 1 : 0); // note: some browsers, like Firefox, don't report borderRadius correctly! Instead, it only reports every corner like borderTopLeftRadius + } + } + + return unit && !~(value + "").trim().indexOf(" ") ? _convertToUnit(target, property, value, unit) + unit : value; +}, + _tweenComplexCSSString = function _tweenComplexCSSString(target, prop, start, end) { + // note: we call _tweenComplexCSSString.call(pluginInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus "this" would refer to the plugin. + if (!start || start === "none") { + // some browsers like Safari actually PREFER the prefixed property and mis-report the unprefixed value like clipPath (BUG). In other words, even though clipPath exists in the style ("clipPath" in target.style) and it's set in the CSS properly (along with -webkit-clip-path), Safari reports clipPath as "none" whereas WebkitClipPath reports accurately like "ellipse(100% 0% at 50% 0%)", so in this case we must SWITCH to using the prefixed property instead. See https://gsap.com/forums/topic/18310-clippath-doesnt-work-on-ios/ + var p = _checkPropPrefix(prop, target, 1), + s = p && _getComputedProperty(target, p, 1); + + if (s && s !== start) { + prop = p; + start = s; + } else if (prop === "borderColor") { + start = _getComputedProperty(target, "borderTopColor"); // Firefox bug: always reports "borderColor" as "", so we must fall back to borderTopColor. See https://gsap.com/forums/topic/24583-how-to-return-colors-that-i-had-after-reverse/ + } + } + + var pt = new PropTween(this._pt, target.style, prop, 0, 1, _renderComplexString), + index = 0, + matchIndex = 0, + a, + result, + startValues, + startNum, + color, + startValue, + endValue, + endNum, + chunk, + endUnit, + startUnit, + endValues; + pt.b = start; + pt.e = end; + start += ""; // ensure values are strings + + end += ""; + + if (end === "auto") { + startValue = target.style[prop]; + target.style[prop] = end; + end = _getComputedProperty(target, prop) || end; + startValue ? target.style[prop] = startValue : _removeProperty(target, prop); + } + + a = [start, end]; + + _colorStringFilter(a); // pass an array with the starting and ending values and let the filter do whatever it needs to the values. If colors are found, it returns true and then we must match where the color shows up order-wise because for things like boxShadow, sometimes the browser provides the computed values with the color FIRST, but the user provides it with the color LAST, so flip them if necessary. Same for drop-shadow(). + + + start = a[0]; + end = a[1]; + startValues = start.match(_numWithUnitExp) || []; + endValues = end.match(_numWithUnitExp) || []; + + if (endValues.length) { + while (result = _numWithUnitExp.exec(end)) { + endValue = result[0]; + chunk = end.substring(index, result.index); + + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(" || chunk.substr(-5) === "hsla(") { + color = 1; + } + + if (endValue !== (startValue = startValues[matchIndex++] || "")) { + startNum = parseFloat(startValue) || 0; + startUnit = startValue.substr((startNum + "").length); + endValue.charAt(1) === "=" && (endValue = _parseRelative(startNum, endValue) + startUnit); + endNum = parseFloat(endValue); + endUnit = endValue.substr((endNum + "").length); + index = _numWithUnitExp.lastIndex - endUnit.length; + + if (!endUnit) { + //if something like "perspective:300" is passed in and we must add a unit to the end + endUnit = endUnit || _config.units[prop] || startUnit; + + if (index === end.length) { + end += endUnit; + pt.e += endUnit; + } + } + + if (startUnit !== endUnit) { + startNum = _convertToUnit(target, prop, startValue, endUnit) || 0; + } // these nested PropTweens are handled in a special way - we'll never actually call a render or setter method on them. We'll just loop through them in the parent complex string PropTween's render method. + + + pt._pt = { + _next: pt._pt, + p: chunk || matchIndex === 1 ? chunk : ",", + //note: SVG spec allows omission of comma/space when a negative sign is wedged between two numbers, like 2.5-5.3 instead of 2.5,-5.3 but when tweening, the negative value may switch to positive, so we insert the comma just in case. + s: startNum, + c: endNum - startNum, + m: color && color < 4 || prop === "zIndex" ? Math.round : 0 + }; + } + } + + pt.c = index < end.length ? end.substring(index, end.length) : ""; //we use the "c" of the PropTween to store the final part of the string (after the last number) + } else { + pt.r = prop === "display" && end === "none" ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue; + } + + _relExp.test(end) && (pt.e = 0); //if the end string contains relative values or dynamic random(...) values, delete the end it so that on the final render we don't actually set it to the string with += or -= characters (forces it to use the calculated value). + + this._pt = pt; //start the linked list with this new PropTween. Remember, we call _tweenComplexCSSString.call(pluginInstance...) to ensure that it's scoped properly. We may call it from within another plugin too, thus "this" would refer to the plugin. + + return pt; +}, + _keywordToPercent = { + top: "0%", + bottom: "100%", + left: "0%", + right: "100%", + center: "50%" +}, + _convertKeywordsToPercentages = function _convertKeywordsToPercentages(value) { + var split = value.split(" "), + x = split[0], + y = split[1] || "50%"; + + if (x === "top" || x === "bottom" || y === "left" || y === "right") { + //the user provided them in the wrong order, so flip them + value = x; + x = y; + y = value; + } + + split[0] = _keywordToPercent[x] || x; + split[1] = _keywordToPercent[y] || y; + return split.join(" "); +}, + _renderClearProps = function _renderClearProps(ratio, data) { + if (data.tween && data.tween._time === data.tween._dur) { + var target = data.t, + style = target.style, + props = data.u, + cache = target._gsap, + prop, + clearTransforms, + i; + + if (props === "all" || props === true) { + style.cssText = ""; + clearTransforms = 1; + } else { + props = props.split(","); + i = props.length; + + while (--i > -1) { + prop = props[i]; + + if (_transformProps[prop]) { + clearTransforms = 1; + prop = prop === "transformOrigin" ? _transformOriginProp : _transformProp; + } + + _removeProperty(target, prop); + } + } + + if (clearTransforms) { + _removeProperty(target, _transformProp); + + if (cache) { + cache.svg && target.removeAttribute("transform"); + style.scale = style.rotate = style.translate = "none"; + + _parseTransform(target, 1); // force all the cached values back to "normal"/identity, otherwise if there's another tween that's already set to render transforms on this element, it could display the wrong values. + + + cache.uncache = 1; + + _removeIndependentTransforms(style); + } + } + } +}, + // note: specialProps should return 1 if (and only if) they have a non-zero priority. It indicates we need to sort the linked list. +_specialProps = { + clearProps: function clearProps(plugin, target, property, endValue, tween) { + if (tween.data !== "isFromStart") { + var pt = plugin._pt = new PropTween(plugin._pt, target, property, 0, 0, _renderClearProps); + pt.u = endValue; + pt.pr = -10; + pt.tween = tween; + + plugin._props.push(property); + + return 1; + } + } + /* className feature (about 0.4kb gzipped). + , className(plugin, target, property, endValue, tween) { + let _renderClassName = (ratio, data) => { + data.css.render(ratio, data.css); + if (!ratio || ratio === 1) { + let inline = data.rmv, + target = data.t, + p; + target.setAttribute("class", ratio ? data.e : data.b); + for (p in inline) { + _removeProperty(target, p); + } + } + }, + _getAllStyles = (target) => { + let styles = {}, + computed = getComputedStyle(target), + p; + for (p in computed) { + if (isNaN(p) && p !== "cssText" && p !== "length") { + styles[p] = computed[p]; + } + } + _setDefaults(styles, _parseTransform(target, 1)); + return styles; + }, + startClassList = target.getAttribute("class"), + style = target.style, + cssText = style.cssText, + cache = target._gsap, + classPT = cache.classPT, + inlineToRemoveAtEnd = {}, + data = {t:target, plugin:plugin, rmv:inlineToRemoveAtEnd, b:startClassList, e:(endValue.charAt(1) !== "=") ? endValue : startClassList.replace(new RegExp("(?:\\s|^)" + endValue.substr(2) + "(?![\\w-])"), "") + ((endValue.charAt(0) === "+") ? " " + endValue.substr(2) : "")}, + changingVars = {}, + startVars = _getAllStyles(target), + transformRelated = /(transform|perspective)/i, + endVars, p; + if (classPT) { + classPT.r(1, classPT.d); + _removeLinkedListItem(classPT.d.plugin, classPT, "_pt"); + } + target.setAttribute("class", data.e); + endVars = _getAllStyles(target, true); + target.setAttribute("class", startClassList); + for (p in endVars) { + if (endVars[p] !== startVars[p] && !transformRelated.test(p)) { + changingVars[p] = endVars[p]; + if (!style[p] && style[p] !== "0") { + inlineToRemoveAtEnd[p] = 1; + } + } + } + cache.classPT = plugin._pt = new PropTween(plugin._pt, target, "className", 0, 0, _renderClassName, data, 0, -11); + if (style.cssText !== cssText) { //only apply if things change. Otherwise, in cases like a background-image that's pulled dynamically, it could cause a refresh. See https://gsap.com/forums/topic/20368-possible-gsap-bug-switching-classnames-in-chrome/. + style.cssText = cssText; //we recorded cssText before we swapped classes and ran _getAllStyles() because in cases when a className tween is overwritten, we remove all the related tweening properties from that class change (otherwise class-specific stuff can't override properties we've directly set on the target's style object due to specificity). + } + _parseTransform(target, true); //to clear the caching of transforms + data.css = new gsap.plugins.css(); + data.css.init(target, changingVars, tween); + plugin._props.push(...data.css._props); + return 1; + } + */ + +}, + +/* + * -------------------------------------------------------------------------------------- + * TRANSFORMS + * -------------------------------------------------------------------------------------- + */ +_identity2DMatrix = [1, 0, 0, 1, 0, 0], + _rotationalProperties = {}, + _isNullTransform = function _isNullTransform(value) { + return value === "matrix(1, 0, 0, 1, 0, 0)" || value === "none" || !value; +}, + _getComputedTransformMatrixAsArray = function _getComputedTransformMatrixAsArray(target) { + var matrixString = _getComputedProperty(target, _transformProp); + + return _isNullTransform(matrixString) ? _identity2DMatrix : matrixString.substr(7).match(_numExp).map(_round); +}, + _getMatrix = function _getMatrix(target, force2D) { + var cache = target._gsap || _getCache(target), + style = target.style, + matrix = _getComputedTransformMatrixAsArray(target), + parent, + nextSibling, + temp, + addedToDOM; + + if (cache.svg && target.getAttribute("transform")) { + temp = target.transform.baseVal.consolidate().matrix; //ensures that even complex values like "translate(50,60) rotate(135,0,0)" are parsed because it mashes it into a matrix. + + matrix = [temp.a, temp.b, temp.c, temp.d, temp.e, temp.f]; + return matrix.join(",") === "1,0,0,1,0,0" ? _identity2DMatrix : matrix; + } else if (matrix === _identity2DMatrix && !target.offsetParent && target !== _docElement && !cache.svg) { + //note: if offsetParent is null, that means the element isn't in the normal document flow, like if it has display:none or one of its ancestors has display:none). Firefox returns null for getComputedStyle() if the element is in an iframe that has display:none. https://bugzilla.mozilla.org/show_bug.cgi?id=548397 + //browsers don't report transforms accurately unless the element is in the DOM and has a display value that's not "none". Firefox and Microsoft browsers have a partial bug where they'll report transforms even if display:none BUT not any percentage-based values like translate(-50%, 8px) will be reported as if it's translate(0, 8px). + temp = style.display; + style.display = "block"; + parent = target.parentNode; + + if (!parent || !target.offsetParent && !target.getBoundingClientRect().width) { + // note: in 3.3.0 we switched target.offsetParent to _doc.body.contains(target) to avoid [sometimes unnecessary] MutationObserver calls but that wasn't adequate because there are edge cases where nested position: fixed elements need to get reparented to accurately sense transforms. See https://github.com/greensock/GSAP/issues/388 and https://github.com/greensock/GSAP/issues/375. Note: position: fixed elements report a null offsetParent but they could also be invisible because they're in an ancestor with display: none, so we check getBoundingClientRect(). We only want to alter the DOM if we absolutely have to because it can cause iframe content to reload, like a Vimeo video. + addedToDOM = 1; //flag + + nextSibling = target.nextElementSibling; + + _docElement.appendChild(target); //we must add it to the DOM in order to get values properly + + } + + matrix = _getComputedTransformMatrixAsArray(target); + temp ? style.display = temp : _removeProperty(target, "display"); + + if (addedToDOM) { + nextSibling ? parent.insertBefore(target, nextSibling) : parent ? parent.appendChild(target) : _docElement.removeChild(target); + } + } + + return force2D && matrix.length > 6 ? [matrix[0], matrix[1], matrix[4], matrix[5], matrix[12], matrix[13]] : matrix; +}, + _applySVGOrigin = function _applySVGOrigin(target, origin, originIsAbsolute, smooth, matrixArray, pluginToAddPropTweensTo) { + var cache = target._gsap, + matrix = matrixArray || _getMatrix(target, true), + xOriginOld = cache.xOrigin || 0, + yOriginOld = cache.yOrigin || 0, + xOffsetOld = cache.xOffset || 0, + yOffsetOld = cache.yOffset || 0, + a = matrix[0], + b = matrix[1], + c = matrix[2], + d = matrix[3], + tx = matrix[4], + ty = matrix[5], + originSplit = origin.split(" "), + xOrigin = parseFloat(originSplit[0]) || 0, + yOrigin = parseFloat(originSplit[1]) || 0, + bounds, + determinant, + x, + y; + + if (!originIsAbsolute) { + bounds = _getBBox(target); + xOrigin = bounds.x + (~originSplit[0].indexOf("%") ? xOrigin / 100 * bounds.width : xOrigin); + yOrigin = bounds.y + (~(originSplit[1] || originSplit[0]).indexOf("%") ? yOrigin / 100 * bounds.height : yOrigin); // if (!("xOrigin" in cache) && (xOrigin || yOrigin)) { // added in 3.12.3, reverted in 3.12.4; requires more exploration + // xOrigin -= bounds.x; + // yOrigin -= bounds.y; + // } + } else if (matrix !== _identity2DMatrix && (determinant = a * d - b * c)) { + //if it's zero (like if scaleX and scaleY are zero), skip it to avoid errors with dividing by zero. + x = xOrigin * (d / determinant) + yOrigin * (-c / determinant) + (c * ty - d * tx) / determinant; + y = xOrigin * (-b / determinant) + yOrigin * (a / determinant) - (a * ty - b * tx) / determinant; + xOrigin = x; + yOrigin = y; // theory: we only had to do this for smoothing and it assumes that the previous one was not originIsAbsolute. + } + + if (smooth || smooth !== false && cache.smooth) { + tx = xOrigin - xOriginOld; + ty = yOrigin - yOriginOld; + cache.xOffset = xOffsetOld + (tx * a + ty * c) - tx; + cache.yOffset = yOffsetOld + (tx * b + ty * d) - ty; + } else { + cache.xOffset = cache.yOffset = 0; + } + + cache.xOrigin = xOrigin; + cache.yOrigin = yOrigin; + cache.smooth = !!smooth; + cache.origin = origin; + cache.originIsAbsolute = !!originIsAbsolute; + target.style[_transformOriginProp] = "0px 0px"; //otherwise, if someone sets an origin via CSS, it will likely interfere with the SVG transform attribute ones (because remember, we're baking the origin into the matrix() value). + + if (pluginToAddPropTweensTo) { + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOrigin", xOriginOld, xOrigin); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOrigin", yOriginOld, yOrigin); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOffset", xOffsetOld, cache.xOffset); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOffset", yOffsetOld, cache.yOffset); + } + + target.setAttribute("data-svg-origin", xOrigin + " " + yOrigin); +}, + _parseTransform = function _parseTransform(target, uncache) { + var cache = target._gsap || new GSCache(target); + + if ("x" in cache && !uncache && !cache.uncache) { + return cache; + } + + var style = target.style, + invertedScaleX = cache.scaleX < 0, + px = "px", + deg = "deg", + cs = getComputedStyle(target), + origin = _getComputedProperty(target, _transformOriginProp) || "0", + x, + y, + z, + scaleX, + scaleY, + rotation, + rotationX, + rotationY, + skewX, + skewY, + perspective, + xOrigin, + yOrigin, + matrix, + angle, + cos, + sin, + a, + b, + c, + d, + a12, + a22, + t1, + t2, + t3, + a13, + a23, + a33, + a42, + a43, + a32; + x = y = z = rotation = rotationX = rotationY = skewX = skewY = perspective = 0; + scaleX = scaleY = 1; + cache.svg = !!(target.getCTM && _isSVG(target)); + + if (cs.translate) { + // accommodate independent transforms by combining them into normal ones. + if (cs.translate !== "none" || cs.scale !== "none" || cs.rotate !== "none") { + style[_transformProp] = (cs.translate !== "none" ? "translate3d(" + (cs.translate + " 0 0").split(" ").slice(0, 3).join(", ") + ") " : "") + (cs.rotate !== "none" ? "rotate(" + cs.rotate + ") " : "") + (cs.scale !== "none" ? "scale(" + cs.scale.split(" ").join(",") + ") " : "") + (cs[_transformProp] !== "none" ? cs[_transformProp] : ""); + } + + style.scale = style.rotate = style.translate = "none"; + } + + matrix = _getMatrix(target, cache.svg); + + if (cache.svg) { + if (cache.uncache) { + // if cache.uncache is true (and maybe if origin is 0,0), we need to set element.style.transformOrigin = (cache.xOrigin - bbox.x) + "px " + (cache.yOrigin - bbox.y) + "px". Previously we let the data-svg-origin stay instead, but when introducing revert(), it complicated things. + t2 = target.getBBox(); + origin = cache.xOrigin - t2.x + "px " + (cache.yOrigin - t2.y) + "px"; + t1 = ""; + } else { + t1 = !uncache && target.getAttribute("data-svg-origin"); // Remember, to work around browser inconsistencies we always force SVG elements' transformOrigin to 0,0 and offset the translation accordingly. + } + + _applySVGOrigin(target, t1 || origin, !!t1 || cache.originIsAbsolute, cache.smooth !== false, matrix); + } + + xOrigin = cache.xOrigin || 0; + yOrigin = cache.yOrigin || 0; + + if (matrix !== _identity2DMatrix) { + a = matrix[0]; //a11 + + b = matrix[1]; //a21 + + c = matrix[2]; //a31 + + d = matrix[3]; //a41 + + x = a12 = matrix[4]; + y = a22 = matrix[5]; //2D matrix + + if (matrix.length === 6) { + scaleX = Math.sqrt(a * a + b * b); + scaleY = Math.sqrt(d * d + c * c); + rotation = a || b ? _atan2(b, a) * _RAD2DEG : 0; //note: if scaleX is 0, we cannot accurately measure rotation. Same for skewX with a scaleY of 0. Therefore, we default to the previously recorded value (or zero if that doesn't exist). + + skewX = c || d ? _atan2(c, d) * _RAD2DEG + rotation : 0; + skewX && (scaleY *= Math.abs(Math.cos(skewX * _DEG2RAD))); + + if (cache.svg) { + x -= xOrigin - (xOrigin * a + yOrigin * c); + y -= yOrigin - (xOrigin * b + yOrigin * d); + } //3D matrix + + } else { + a32 = matrix[6]; + a42 = matrix[7]; + a13 = matrix[8]; + a23 = matrix[9]; + a33 = matrix[10]; + a43 = matrix[11]; + x = matrix[12]; + y = matrix[13]; + z = matrix[14]; + angle = _atan2(a32, a33); + rotationX = angle * _RAD2DEG; //rotationX + + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a12 * cos + a13 * sin; + t2 = a22 * cos + a23 * sin; + t3 = a32 * cos + a33 * sin; + a13 = a12 * -sin + a13 * cos; + a23 = a22 * -sin + a23 * cos; + a33 = a32 * -sin + a33 * cos; + a43 = a42 * -sin + a43 * cos; + a12 = t1; + a22 = t2; + a32 = t3; + } //rotationY + + + angle = _atan2(-c, a33); + rotationY = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a * cos - a13 * sin; + t2 = b * cos - a23 * sin; + t3 = c * cos - a33 * sin; + a43 = d * sin + a43 * cos; + a = t1; + b = t2; + c = t3; + } //rotationZ + + + angle = _atan2(b, a); + rotation = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(angle); + sin = Math.sin(angle); + t1 = a * cos + b * sin; + t2 = a12 * cos + a22 * sin; + b = b * cos - a * sin; + a22 = a22 * cos - a12 * sin; + a = t1; + a12 = t2; + } + + if (rotationX && Math.abs(rotationX) + Math.abs(rotation) > 359.9) { + //when rotationY is set, it will often be parsed as 180 degrees different than it should be, and rotationX and rotation both being 180 (it looks the same), so we adjust for that here. + rotationX = rotation = 0; + rotationY = 180 - rotationY; + } + + scaleX = _round(Math.sqrt(a * a + b * b + c * c)); + scaleY = _round(Math.sqrt(a22 * a22 + a32 * a32)); + angle = _atan2(a12, a22); + skewX = Math.abs(angle) > 0.0002 ? angle * _RAD2DEG : 0; + perspective = a43 ? 1 / (a43 < 0 ? -a43 : a43) : 0; + } + + if (cache.svg) { + //sense if there are CSS transforms applied on an SVG element in which case we must overwrite them when rendering. The transform attribute is more reliable cross-browser, but we can't just remove the CSS ones because they may be applied in a CSS rule somewhere (not just inline). + t1 = target.getAttribute("transform"); + cache.forceCSS = target.setAttribute("transform", "") || !_isNullTransform(_getComputedProperty(target, _transformProp)); + t1 && target.setAttribute("transform", t1); + } + } + + if (Math.abs(skewX) > 90 && Math.abs(skewX) < 270) { + if (invertedScaleX) { + scaleX *= -1; + skewX += rotation <= 0 ? 180 : -180; + rotation += rotation <= 0 ? 180 : -180; + } else { + scaleY *= -1; + skewX += skewX <= 0 ? 180 : -180; + } + } + + uncache = uncache || cache.uncache; + cache.x = x - ((cache.xPercent = x && (!uncache && cache.xPercent || (Math.round(target.offsetWidth / 2) === Math.round(-x) ? -50 : 0))) ? target.offsetWidth * cache.xPercent / 100 : 0) + px; + cache.y = y - ((cache.yPercent = y && (!uncache && cache.yPercent || (Math.round(target.offsetHeight / 2) === Math.round(-y) ? -50 : 0))) ? target.offsetHeight * cache.yPercent / 100 : 0) + px; + cache.z = z + px; + cache.scaleX = _round(scaleX); + cache.scaleY = _round(scaleY); + cache.rotation = _round(rotation) + deg; + cache.rotationX = _round(rotationX) + deg; + cache.rotationY = _round(rotationY) + deg; + cache.skewX = skewX + deg; + cache.skewY = skewY + deg; + cache.transformPerspective = perspective + px; + + if (cache.zOrigin = parseFloat(origin.split(" ")[2]) || !uncache && cache.zOrigin || 0) { + style[_transformOriginProp] = _firstTwoOnly(origin); + } + + cache.xOffset = cache.yOffset = 0; + cache.force3D = _config.force3D; + cache.renderTransform = cache.svg ? _renderSVGTransforms : _supports3D ? _renderCSSTransforms : _renderNon3DTransforms; + cache.uncache = 0; + return cache; +}, + _firstTwoOnly = function _firstTwoOnly(value) { + return (value = value.split(" "))[0] + " " + value[1]; +}, + //for handling transformOrigin values, stripping out the 3rd dimension +_addPxTranslate = function _addPxTranslate(target, start, value) { + var unit = getUnit(start); + return _round(parseFloat(start) + parseFloat(_convertToUnit(target, "x", value + "px", unit))) + unit; +}, + _renderNon3DTransforms = function _renderNon3DTransforms(ratio, cache) { + cache.z = "0px"; + cache.rotationY = cache.rotationX = "0deg"; + cache.force3D = 0; + + _renderCSSTransforms(ratio, cache); +}, + _zeroDeg = "0deg", + _zeroPx = "0px", + _endParenthesis = ") ", + _renderCSSTransforms = function _renderCSSTransforms(ratio, cache) { + var _ref = cache || this, + xPercent = _ref.xPercent, + yPercent = _ref.yPercent, + x = _ref.x, + y = _ref.y, + z = _ref.z, + rotation = _ref.rotation, + rotationY = _ref.rotationY, + rotationX = _ref.rotationX, + skewX = _ref.skewX, + skewY = _ref.skewY, + scaleX = _ref.scaleX, + scaleY = _ref.scaleY, + transformPerspective = _ref.transformPerspective, + force3D = _ref.force3D, + target = _ref.target, + zOrigin = _ref.zOrigin, + transforms = "", + use3D = force3D === "auto" && ratio && ratio !== 1 || force3D === true; // Safari has a bug that causes it not to render 3D transform-origin values properly, so we force the z origin to 0, record it in the cache, and then do the math here to offset the translate values accordingly (basically do the 3D transform-origin part manually) + + + if (zOrigin && (rotationX !== _zeroDeg || rotationY !== _zeroDeg)) { + var angle = parseFloat(rotationY) * _DEG2RAD, + a13 = Math.sin(angle), + a33 = Math.cos(angle), + cos; + + angle = parseFloat(rotationX) * _DEG2RAD; + cos = Math.cos(angle); + x = _addPxTranslate(target, x, a13 * cos * -zOrigin); + y = _addPxTranslate(target, y, -Math.sin(angle) * -zOrigin); + z = _addPxTranslate(target, z, a33 * cos * -zOrigin + zOrigin); + } + + if (transformPerspective !== _zeroPx) { + transforms += "perspective(" + transformPerspective + _endParenthesis; + } + + if (xPercent || yPercent) { + transforms += "translate(" + xPercent + "%, " + yPercent + "%) "; + } + + if (use3D || x !== _zeroPx || y !== _zeroPx || z !== _zeroPx) { + transforms += z !== _zeroPx || use3D ? "translate3d(" + x + ", " + y + ", " + z + ") " : "translate(" + x + ", " + y + _endParenthesis; + } + + if (rotation !== _zeroDeg) { + transforms += "rotate(" + rotation + _endParenthesis; + } + + if (rotationY !== _zeroDeg) { + transforms += "rotateY(" + rotationY + _endParenthesis; + } + + if (rotationX !== _zeroDeg) { + transforms += "rotateX(" + rotationX + _endParenthesis; + } + + if (skewX !== _zeroDeg || skewY !== _zeroDeg) { + transforms += "skew(" + skewX + ", " + skewY + _endParenthesis; + } + + if (scaleX !== 1 || scaleY !== 1) { + transforms += "scale(" + scaleX + ", " + scaleY + _endParenthesis; + } + + target.style[_transformProp] = transforms || "translate(0, 0)"; +}, + _renderSVGTransforms = function _renderSVGTransforms(ratio, cache) { + var _ref2 = cache || this, + xPercent = _ref2.xPercent, + yPercent = _ref2.yPercent, + x = _ref2.x, + y = _ref2.y, + rotation = _ref2.rotation, + skewX = _ref2.skewX, + skewY = _ref2.skewY, + scaleX = _ref2.scaleX, + scaleY = _ref2.scaleY, + target = _ref2.target, + xOrigin = _ref2.xOrigin, + yOrigin = _ref2.yOrigin, + xOffset = _ref2.xOffset, + yOffset = _ref2.yOffset, + forceCSS = _ref2.forceCSS, + tx = parseFloat(x), + ty = parseFloat(y), + a11, + a21, + a12, + a22, + temp; + + rotation = parseFloat(rotation); + skewX = parseFloat(skewX); + skewY = parseFloat(skewY); + + if (skewY) { + //for performance reasons, we combine all skewing into the skewX and rotation values. Remember, a skewY of 10 degrees looks the same as a rotation of 10 degrees plus a skewX of 10 degrees. + skewY = parseFloat(skewY); + skewX += skewY; + rotation += skewY; + } + + if (rotation || skewX) { + rotation *= _DEG2RAD; + skewX *= _DEG2RAD; + a11 = Math.cos(rotation) * scaleX; + a21 = Math.sin(rotation) * scaleX; + a12 = Math.sin(rotation - skewX) * -scaleY; + a22 = Math.cos(rotation - skewX) * scaleY; + + if (skewX) { + skewY *= _DEG2RAD; + temp = Math.tan(skewX - skewY); + temp = Math.sqrt(1 + temp * temp); + a12 *= temp; + a22 *= temp; + + if (skewY) { + temp = Math.tan(skewY); + temp = Math.sqrt(1 + temp * temp); + a11 *= temp; + a21 *= temp; + } + } + + a11 = _round(a11); + a21 = _round(a21); + a12 = _round(a12); + a22 = _round(a22); + } else { + a11 = scaleX; + a22 = scaleY; + a21 = a12 = 0; + } + + if (tx && !~(x + "").indexOf("px") || ty && !~(y + "").indexOf("px")) { + tx = _convertToUnit(target, "x", x, "px"); + ty = _convertToUnit(target, "y", y, "px"); + } + + if (xOrigin || yOrigin || xOffset || yOffset) { + tx = _round(tx + xOrigin - (xOrigin * a11 + yOrigin * a12) + xOffset); + ty = _round(ty + yOrigin - (xOrigin * a21 + yOrigin * a22) + yOffset); + } + + if (xPercent || yPercent) { + //The SVG spec doesn't support percentage-based translation in the "transform" attribute, so we merge it into the translation to simulate it. + temp = target.getBBox(); + tx = _round(tx + xPercent / 100 * temp.width); + ty = _round(ty + yPercent / 100 * temp.height); + } + + temp = "matrix(" + a11 + "," + a21 + "," + a12 + "," + a22 + "," + tx + "," + ty + ")"; + target.setAttribute("transform", temp); + forceCSS && (target.style[_transformProp] = temp); //some browsers prioritize CSS transforms over the transform attribute. When we sense that the user has CSS transforms applied, we must overwrite them this way (otherwise some browser simply won't render the transform attribute changes!) +}, + _addRotationalPropTween = function _addRotationalPropTween(plugin, target, property, startNum, endValue) { + var cap = 360, + isString = _isString(endValue), + endNum = parseFloat(endValue) * (isString && ~endValue.indexOf("rad") ? _RAD2DEG : 1), + change = endNum - startNum, + finalValue = startNum + change + "deg", + direction, + pt; + + if (isString) { + direction = endValue.split("_")[1]; + + if (direction === "short") { + change %= cap; + + if (change !== change % (cap / 2)) { + change += change < 0 ? cap : -cap; + } + } + + if (direction === "cw" && change < 0) { + change = (change + cap * _bigNum) % cap - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = (change - cap * _bigNum) % cap - ~~(change / cap) * cap; + } + } + + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + pt.u = "deg"; + + plugin._props.push(property); + + return pt; +}, + _assign = function _assign(target, source) { + // Internet Explorer doesn't have Object.assign(), so we recreate it here. + for (var p in source) { + target[p] = source[p]; + } + + return target; +}, + _addRawTransformPTs = function _addRawTransformPTs(plugin, transforms, target) { + //for handling cases where someone passes in a whole transform string, like transform: "scale(2, 3) rotate(20deg) translateY(30em)" + var startCache = _assign({}, target._gsap), + exclude = "perspective,force3D,transformOrigin,svgOrigin", + style = target.style, + endCache, + p, + startValue, + endValue, + startNum, + endNum, + startUnit, + endUnit; + + if (startCache.svg) { + startValue = target.getAttribute("transform"); + target.setAttribute("transform", ""); + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + + _removeProperty(target, _transformProp); + + target.setAttribute("transform", startValue); + } else { + startValue = getComputedStyle(target)[_transformProp]; + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + style[_transformProp] = startValue; + } + + for (p in _transformProps) { + startValue = startCache[p]; + endValue = endCache[p]; + + if (startValue !== endValue && exclude.indexOf(p) < 0) { + //tweening to no perspective gives very unintuitive results - just keep the same perspective in that case. + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + startNum = startUnit !== endUnit ? _convertToUnit(target, p, startValue, endUnit) : parseFloat(startValue); + endNum = parseFloat(endValue); + plugin._pt = new PropTween(plugin._pt, endCache, p, startNum, endNum - startNum, _renderCSSProp); + plugin._pt.u = endUnit || 0; + + plugin._props.push(p); + } + } + + _assign(endCache, startCache); +}; // handle splitting apart padding, margin, borderWidth, and borderRadius into their 4 components. Firefox, for example, won't report borderRadius correctly - it will only do borderTopLeftRadius and the other corners. We also want to handle paddingTop, marginLeft, borderRightWidth, etc. + + +_forEachName("padding,margin,Width,Radius", function (name, index) { + var t = "Top", + r = "Right", + b = "Bottom", + l = "Left", + props = (index < 3 ? [t, r, b, l] : [t + l, t + r, b + r, b + l]).map(function (side) { + return index < 2 ? name + side : "border" + side + name; + }); + + _specialProps[index > 1 ? "border" + name : name] = function (plugin, target, property, endValue, tween) { + var a, vars; + + if (arguments.length < 4) { + // getter, passed target, property, and unit (from _get()) + a = props.map(function (prop) { + return _get(plugin, prop, property); + }); + vars = a.join(" "); + return vars.split(a[0]).length === 5 ? a[0] : vars; + } + + a = (endValue + "").split(" "); + vars = {}; + props.forEach(function (prop, i) { + return vars[prop] = a[i] = a[i] || a[(i - 1) / 2 | 0]; + }); + plugin.init(target, vars, tween); + }; +}); + +export var CSSPlugin = { + name: "css", + register: _initCore, + targetTest: function targetTest(target) { + return target.style && target.nodeType; + }, + init: function init(target, vars, tween, index, targets) { + var props = this._props, + style = target.style, + startAt = tween.vars.startAt, + startValue, + endValue, + endNum, + startNum, + type, + specialProp, + p, + startUnit, + endUnit, + relative, + isTransformRelated, + transformPropTween, + cache, + smooth, + hasPriority, + inlineProps; + _pluginInitted || _initCore(); // we may call init() multiple times on the same plugin instance, like when adding special properties, so make sure we don't overwrite the revert data or inlineProps + + this.styles = this.styles || _getStyleSaver(target); + inlineProps = this.styles.props; + this.tween = tween; + + for (p in vars) { + if (p === "autoRound") { + continue; + } + + endValue = vars[p]; + + if (_plugins[p] && _checkPlugin(p, vars, tween, index, target, targets)) { + // plugins + continue; + } + + type = typeof endValue; + specialProp = _specialProps[p]; + + if (type === "function") { + endValue = endValue.call(tween, index, target, targets); + type = typeof endValue; + } + + if (type === "string" && ~endValue.indexOf("random(")) { + endValue = _replaceRandom(endValue); + } + + if (specialProp) { + specialProp(this, target, p, endValue, tween) && (hasPriority = 1); + } else if (p.substr(0, 2) === "--") { + //CSS variable + startValue = (getComputedStyle(target).getPropertyValue(p) + "").trim(); + endValue += ""; + _colorExp.lastIndex = 0; + + if (!_colorExp.test(startValue)) { + // colors don't have units + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + } + + endUnit ? startUnit !== endUnit && (startValue = _convertToUnit(target, p, startValue, endUnit) + endUnit) : startUnit && (endValue += startUnit); + this.add(style, "setProperty", startValue, endValue, index, targets, 0, 0, p); + props.push(p); + inlineProps.push(p, 0, style[p]); + } else if (type !== "undefined") { + if (startAt && p in startAt) { + // in case someone hard-codes a complex value as the start, like top: "calc(2vh / 2)". Without this, it'd use the computed value (always in px) + startValue = typeof startAt[p] === "function" ? startAt[p].call(tween, index, target, targets) : startAt[p]; + _isString(startValue) && ~startValue.indexOf("random(") && (startValue = _replaceRandom(startValue)); + getUnit(startValue + "") || startValue === "auto" || (startValue += _config.units[p] || getUnit(_get(target, p)) || ""); // for cases when someone passes in a unitless value like {x: 100}; if we try setting translate(100, 0px) it won't work. + + (startValue + "").charAt(1) === "=" && (startValue = _get(target, p)); // can't work with relative values + } else { + startValue = _get(target, p); + } + + startNum = parseFloat(startValue); + relative = type === "string" && endValue.charAt(1) === "=" && endValue.substr(0, 2); + relative && (endValue = endValue.substr(2)); + endNum = parseFloat(endValue); + + if (p in _propertyAliases) { + if (p === "autoAlpha") { + //special case where we control the visibility along with opacity. We still allow the opacity value to pass through and get tweened. + if (startNum === 1 && _get(target, "visibility") === "hidden" && endNum) { + //if visibility is initially set to "hidden", we should interpret that as intent to make opacity 0 (a convenience) + startNum = 0; + } + + inlineProps.push("visibility", 0, style.visibility); + + _addNonTweeningPT(this, style, "visibility", startNum ? "inherit" : "hidden", endNum ? "inherit" : "hidden", !endNum); + } + + if (p !== "scale" && p !== "transform") { + p = _propertyAliases[p]; + ~p.indexOf(",") && (p = p.split(",")[0]); + } + } + + isTransformRelated = p in _transformProps; //--- TRANSFORM-RELATED --- + + if (isTransformRelated) { + this.styles.save(p); + + if (!transformPropTween) { + cache = target._gsap; + cache.renderTransform && !vars.parseTransform || _parseTransform(target, vars.parseTransform); // if, for example, gsap.set(... {transform:"translateX(50vw)"}), the _get() call doesn't parse the transform, thus cache.renderTransform won't be set yet so force the parsing of the transform here. + + smooth = vars.smoothOrigin !== false && cache.smooth; + transformPropTween = this._pt = new PropTween(this._pt, style, _transformProp, 0, 1, cache.renderTransform, cache, 0, -1); //the first time through, create the rendering PropTween so that it runs LAST (in the linked list, we keep adding to the beginning) + + transformPropTween.dep = 1; //flag it as dependent so that if things get killed/overwritten and this is the only PropTween left, we can safely kill the whole tween. + } + + if (p === "scale") { + this._pt = new PropTween(this._pt, cache, "scaleY", cache.scaleY, (relative ? _parseRelative(cache.scaleY, relative + endNum) : endNum) - cache.scaleY || 0, _renderCSSProp); + this._pt.u = 0; + props.push("scaleY", p); + p += "X"; + } else if (p === "transformOrigin") { + inlineProps.push(_transformOriginProp, 0, style[_transformOriginProp]); + endValue = _convertKeywordsToPercentages(endValue); //in case something like "left top" or "bottom right" is passed in. Convert to percentages. + + if (cache.svg) { + _applySVGOrigin(target, endValue, 0, smooth, 0, this); + } else { + endUnit = parseFloat(endValue.split(" ")[2]) || 0; //handle the zOrigin separately! + + endUnit !== cache.zOrigin && _addNonTweeningPT(this, cache, "zOrigin", cache.zOrigin, endUnit); + + _addNonTweeningPT(this, style, p, _firstTwoOnly(startValue), _firstTwoOnly(endValue)); + } + + continue; + } else if (p === "svgOrigin") { + _applySVGOrigin(target, endValue, 1, smooth, 0, this); + + continue; + } else if (p in _rotationalProperties) { + _addRotationalPropTween(this, cache, p, startNum, relative ? _parseRelative(startNum, relative + endValue) : endValue); + + continue; + } else if (p === "smoothOrigin") { + _addNonTweeningPT(this, cache, "smooth", cache.smooth, endValue); + + continue; + } else if (p === "force3D") { + cache[p] = endValue; + continue; + } else if (p === "transform") { + _addRawTransformPTs(this, endValue, target); + + continue; + } + } else if (!(p in style)) { + p = _checkPropPrefix(p) || p; + } + + if (isTransformRelated || (endNum || endNum === 0) && (startNum || startNum === 0) && !_complexExp.test(endValue) && p in style) { + startUnit = (startValue + "").substr((startNum + "").length); + endNum || (endNum = 0); // protect against NaN + + endUnit = getUnit(endValue) || (p in _config.units ? _config.units[p] : startUnit); + startUnit !== endUnit && (startNum = _convertToUnit(target, p, startValue, endUnit)); + this._pt = new PropTween(this._pt, isTransformRelated ? cache : style, p, startNum, (relative ? _parseRelative(startNum, relative + endNum) : endNum) - startNum, !isTransformRelated && (endUnit === "px" || p === "zIndex") && vars.autoRound !== false ? _renderRoundedCSSProp : _renderCSSProp); + this._pt.u = endUnit || 0; + + if (startUnit !== endUnit && endUnit !== "%") { + //when the tween goes all the way back to the beginning, we need to revert it to the OLD/ORIGINAL value (with those units). We record that as a "b" (beginning) property and point to a render method that handles that. (performance optimization) + this._pt.b = startValue; + this._pt.r = _renderCSSPropWithBeginning; + } + } else if (!(p in style)) { + if (p in target) { + //maybe it's not a style - it could be a property added directly to an element in which case we'll try to animate that. + this.add(target, p, startValue || target[p], relative ? relative + endValue : endValue, index, targets); + } else if (p !== "parseTransform") { + _missingPlugin(p, endValue); + + continue; + } + } else { + _tweenComplexCSSString.call(this, target, p, startValue, relative ? relative + endValue : endValue); + } + + isTransformRelated || (p in style ? inlineProps.push(p, 0, style[p]) : typeof target[p] === "function" ? inlineProps.push(p, 2, target[p]()) : inlineProps.push(p, 1, startValue || target[p])); + props.push(p); + } + } + + hasPriority && _sortPropTweensByPriority(this); + }, + render: function render(ratio, data) { + if (data.tween._time || !_reverting()) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + } else { + data.styles.revert(); + } + }, + get: _get, + aliases: _propertyAliases, + getSetter: function getSetter(target, property, plugin) { + //returns a setter function that accepts target, property, value and applies it accordingly. Remember, properties like "x" aren't as simple as target.style.property = value because they've got to be applied to a proxy object and then merged into a transform string in a renderer. + var p = _propertyAliases[property]; + p && p.indexOf(",") < 0 && (property = p); + return property in _transformProps && property !== _transformOriginProp && (target._gsap.x || _get(target, "x")) ? plugin && _recentSetterPlugin === plugin ? property === "scale" ? _setterScale : _setterTransform : (_recentSetterPlugin = plugin || {}) && (property === "scale" ? _setterScaleWithRender : _setterTransformWithRender) : target.style && !_isUndefined(target.style[property]) ? _setterCSSStyle : ~property.indexOf("-") ? _setterCSSProp : _getSetter(target, property); + }, + core: { + _removeProperty: _removeProperty, + _getMatrix: _getMatrix + } +}; +gsap.utils.checkPrefix = _checkPropPrefix; +gsap.core.getStyleSaver = _getStyleSaver; + +(function (positionAndScale, rotation, others, aliases) { + var all = _forEachName(positionAndScale + "," + rotation + "," + others, function (name) { + _transformProps[name] = 1; + }); + + _forEachName(rotation, function (name) { + _config.units[name] = "deg"; + _rotationalProperties[name] = 1; + }); + + _propertyAliases[all[13]] = positionAndScale + "," + rotation; + + _forEachName(aliases, function (name) { + var split = name.split(":"); + _propertyAliases[split[1]] = all[split[0]]; + }); +})("x,y,z,scale,scaleX,scaleY,xPercent,yPercent", "rotation,rotationX,rotationY,skewX,skewY", "transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective", "0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY"); + +_forEachName("x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective", function (name) { + _config.units[name] = "px"; +}); + +gsap.registerPlugin(CSSPlugin); +export { CSSPlugin as default, _getBBox, _createElement, _checkPropPrefix as checkPrefix }; \ No newline at end of file diff --git a/node_modules/gsap/CSSRulePlugin.js b/node_modules/gsap/CSSRulePlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..035eb6b64aca782443f6edf8bab7e345e75cb9d0 --- /dev/null +++ b/node_modules/gsap/CSSRulePlugin.js @@ -0,0 +1,134 @@ +/*! + * CSSRulePlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var gsap, + _coreInitted, + _win, + _doc, + CSSPlugin, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _checkRegister = function _checkRegister() { + if (!_coreInitted) { + _initCore(); + + if (!CSSPlugin) { + console.warn("Please gsap.registerPlugin(CSSPlugin, CSSRulePlugin)"); + } + } + + return _coreInitted; +}, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (_windowExists()) { + _win = window; + _doc = document; + } + + if (gsap) { + CSSPlugin = gsap.plugins.css; + + if (CSSPlugin) { + _coreInitted = 1; + } + } +}; + +export var CSSRulePlugin = { + version: "3.12.7", + name: "cssRule", + init: function init(target, value, tween, index, targets) { + if (!_checkRegister() || typeof target.cssText === "undefined") { + return false; + } + + var div = target._gsProxy = target._gsProxy || _doc.createElement("div"); + + this.ss = target; + this.style = div.style; + div.style.cssText = target.cssText; + CSSPlugin.prototype.init.call(this, div, value, tween, index, targets); //we just offload all the work to the regular CSSPlugin and then copy the cssText back over to the rule in the render() method. This allows us to have all of the updates to CSSPlugin automatically flow through to CSSRulePlugin instead of having to maintain both + }, + render: function render(ratio, data) { + var pt = data._pt, + style = data.style, + ss = data.ss, + i; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + i = style.length; + + while (--i > -1) { + ss[style[i]] = style[style[i]]; + } + }, + getRule: function getRule(selector) { + _checkRegister(); + + var ruleProp = _doc.all ? "rules" : "cssRules", + styleSheets = _doc.styleSheets, + i = styleSheets.length, + pseudo = selector.charAt(0) === ":", + j, + curSS, + cs, + a; + selector = (pseudo ? "" : ",") + selector.split("::").join(":").toLowerCase() + ","; //note: old versions of IE report tag name selectors as upper case, so we just change everything to lowercase. + + if (pseudo) { + a = []; + } + + while (i--) { + //Firefox may throw insecure operation errors when css is loaded from other domains, so try/catch. + try { + curSS = styleSheets[i][ruleProp]; + + if (!curSS) { + continue; + } + + j = curSS.length; + } catch (e) { + console.warn(e); + continue; + } + + while (--j > -1) { + cs = curSS[j]; + + if (cs.selectorText && ("," + cs.selectorText.split("::").join(":").toLowerCase() + ",").indexOf(selector) !== -1) { + //note: IE adds an extra ":" to pseudo selectors, so .myClass:after becomes .myClass::after, so we need to strip the extra one out. + if (pseudo) { + a.push(cs.style); + } else { + return cs.style; + } + } + } + } + + return a; + }, + register: _initCore +}; +_getGSAP() && gsap.registerPlugin(CSSRulePlugin); +export { CSSRulePlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/CustomEase.js b/node_modules/gsap/CustomEase.js new file mode 100644 index 0000000000000000000000000000000000000000..a48aa2d8ffb1fbecb9c7bd8d93a2636878df4d7d --- /dev/null +++ b/node_modules/gsap/CustomEase.js @@ -0,0 +1,374 @@ +/*! + * CustomEase 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +import { stringToRawPath, rawPathToString, transformRawPath } from "./utils/paths.js"; + +var gsap, + _coreInitted, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _initCore = function _initCore() { + gsap = _getGSAP(); + + if (gsap) { + gsap.registerEase("_CE", CustomEase.create); + _coreInitted = 1; + } else { + console.warn("Please gsap.registerPlugin(CustomEase)"); + } +}, + _bigNum = 1e20, + _round = function _round(value) { + return ~~(value * 1000 + (value < 0 ? -.5 : .5)) / 1000; +}, + _bonusValidated = 1, + //<name>CustomEase</name> +_numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/gi, + //finds any numbers, including ones that start with += or -=, negative numbers, and ones in scientific notation like 1e-8. +_needsParsingExp = /[cLlsSaAhHvVtTqQ]/g, + _findMinimum = function _findMinimum(values) { + var l = values.length, + min = _bigNum, + i; + + for (i = 1; i < l; i += 6) { + +values[i] < min && (min = +values[i]); + } + + return min; +}, + //takes all the points and translates/scales them so that the x starts at 0 and ends at 1. +_normalize = function _normalize(values, height, originY) { + if (!originY && originY !== 0) { + originY = Math.max(+values[values.length - 1], +values[1]); + } + + var tx = +values[0] * -1, + ty = -originY, + l = values.length, + sx = 1 / (+values[l - 2] + tx), + sy = -height || (Math.abs(+values[l - 1] - +values[1]) < 0.01 * (+values[l - 2] - +values[0]) ? _findMinimum(values) + ty : +values[l - 1] + ty), + i; + + if (sy) { + //typically y ends at 1 (so that the end values are reached) + sy = 1 / sy; + } else { + //in case the ease returns to its beginning value, scale everything proportionally + sy = -sx; + } + + for (i = 0; i < l; i += 2) { + values[i] = (+values[i] + tx) * sx; + values[i + 1] = (+values[i + 1] + ty) * sy; + } +}, + //note that this function returns point objects like {x, y} rather than working with segments which are arrays with alternating x, y values as in the similar function in paths.js +_bezierToPoints = function _bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) { + var x12 = (x1 + x2) / 2, + y12 = (y1 + y2) / 2, + x23 = (x2 + x3) / 2, + y23 = (y2 + y3) / 2, + x34 = (x3 + x4) / 2, + y34 = (y3 + y4) / 2, + x123 = (x12 + x23) / 2, + y123 = (y12 + y23) / 2, + x234 = (x23 + x34) / 2, + y234 = (y23 + y34) / 2, + x1234 = (x123 + x234) / 2, + y1234 = (y123 + y234) / 2, + dx = x4 - x1, + dy = y4 - y1, + d2 = Math.abs((x2 - x4) * dy - (y2 - y4) * dx), + d3 = Math.abs((x3 - x4) * dy - (y3 - y4) * dx), + length; + + if (!points) { + points = [{ + x: x1, + y: y1 + }, { + x: x4, + y: y4 + }]; + index = 1; + } + + points.splice(index || points.length - 1, 0, { + x: x1234, + y: y1234 + }); + + if ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) { + length = points.length; + + _bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index); + + _bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 1 + (points.length - length)); + } + + return points; +}; + +export var CustomEase = /*#__PURE__*/function () { + function CustomEase(id, data, config) { + _coreInitted || _initCore(); + this.id = id; + _bonusValidated && this.setData(data, config); + } + + var _proto = CustomEase.prototype; + + _proto.setData = function setData(data, config) { + config = config || {}; + data = data || "0,0,1,1"; + var values = data.match(_numExp), + closest = 1, + points = [], + lookup = [], + precision = config.precision || 1, + fast = precision <= 1, + l, + a1, + a2, + i, + inc, + j, + point, + prevPoint, + p; + this.data = data; + + if (_needsParsingExp.test(data) || ~data.indexOf("M") && data.indexOf("C") < 0) { + values = stringToRawPath(data)[0]; + } + + l = values.length; + + if (l === 4) { + values.unshift(0, 0); + values.push(1, 1); + l = 8; + } else if ((l - 2) % 6) { + throw "Invalid CustomEase"; + } + + if (+values[0] !== 0 || +values[l - 2] !== 1) { + _normalize(values, config.height, config.originY); + } + + this.segment = values; + + for (i = 2; i < l; i += 6) { + a1 = { + x: +values[i - 2], + y: +values[i - 1] + }; + a2 = { + x: +values[i + 4], + y: +values[i + 5] + }; + points.push(a1, a2); + + _bezierToPoints(a1.x, a1.y, +values[i], +values[i + 1], +values[i + 2], +values[i + 3], a2.x, a2.y, 1 / (precision * 200000), points, points.length - 1); + } + + l = points.length; + + for (i = 0; i < l; i++) { + point = points[i]; + prevPoint = points[i - 1] || point; + + if ((point.x > prevPoint.x || prevPoint.y !== point.y && prevPoint.x === point.x || point === prevPoint) && point.x <= 1) { + //if a point goes BACKWARD in time or is a duplicate, just drop it. Also it shouldn't go past 1 on the x axis, as could happen in a string like "M0,0 C0,0 0.12,0.68 0.18,0.788 0.195,0.845 0.308,1 0.32,1 0.403,1.005 0.398,1 0.5,1 0.602,1 0.816,1.005 0.9,1 0.91,1 0.948,0.69 0.962,0.615 1.003,0.376 1,0 1,0". + prevPoint.cx = point.x - prevPoint.x; //change in x between this point and the next point (performance optimization) + + prevPoint.cy = point.y - prevPoint.y; + prevPoint.n = point; + prevPoint.nx = point.x; //next point's x value (performance optimization, making lookups faster in getRatio()). Remember, the lookup will always land on a spot where it's either this point or the very next one (never beyond that) + + if (fast && i > 1 && Math.abs(prevPoint.cy / prevPoint.cx - points[i - 2].cy / points[i - 2].cx) > 2) { + //if there's a sudden change in direction, prioritize accuracy over speed. Like a bounce ease - you don't want to risk the sampling chunks landing on each side of the bounce anchor and having it clipped off. + fast = 0; + } + + if (prevPoint.cx < closest) { + if (!prevPoint.cx) { + prevPoint.cx = 0.001; //avoids math problems in getRatio() (dividing by zero) + + if (i === l - 1) { + //in case the final segment goes vertical RIGHT at the end, make sure we end at the end. + prevPoint.x -= 0.001; + closest = Math.min(closest, 0.001); + fast = 0; + } + } else { + closest = prevPoint.cx; + } + } + } else { + points.splice(i--, 1); + l--; + } + } + + l = 1 / closest + 1 | 0; + inc = 1 / l; + j = 0; + point = points[0]; + + if (fast) { + for (i = 0; i < l; i++) { + //for fastest lookups, we just sample along the path at equal x (time) distance. Uses more memory and is slightly less accurate for anchors that don't land on the sampling points, but for the vast majority of eases it's excellent (and fast). + p = i * inc; + + if (point.nx < p) { + point = points[++j]; + } + + a1 = point.y + (p - point.x) / point.cx * point.cy; + lookup[i] = { + x: p, + cx: inc, + y: a1, + cy: 0, + nx: 9 + }; + + if (i) { + lookup[i - 1].cy = a1 - lookup[i - 1].y; + } + } + + j = points[points.length - 1]; + lookup[l - 1].cy = j.y - a1; + lookup[l - 1].cx = j.x - lookup[lookup.length - 1].x; //make sure it lands EXACTLY where it should. Otherwise, it might be something like 0.9999999999 instead of 1. + } else { + //this option is more accurate, ensuring that EVERY anchor is hit perfectly. Clipping across a bounce, for example, would never happen. + for (i = 0; i < l; i++) { + //build a lookup table based on the smallest distance so that we can instantly find the appropriate point (well, it'll either be that point or the very next one). We'll look up based on the linear progress. So it's it's 0.5 and the lookup table has 100 elements, it'd be like lookup[Math.floor(0.5 * 100)] + if (point.nx < i * inc) { + point = points[++j]; + } + + lookup[i] = point; + } + + if (j < points.length - 1) { + lookup[i - 1] = points[points.length - 2]; + } + } //this._calcEnd = (points[points.length-1].y !== 1 || points[0].y !== 0); //ensures that we don't run into floating point errors. As long as we're starting at 0 and ending at 1, tell GSAP to skip the final calculation and use 0/1 as the factor. + + + this.ease = function (p) { + var point = lookup[p * l | 0] || lookup[l - 1]; + + if (point.nx < p) { + point = point.n; + } + + return point.y + (p - point.x) / point.cx * point.cy; + }; + + this.ease.custom = this; + this.id && gsap && gsap.registerEase(this.id, this.ease); + return this; + }; + + _proto.getSVGData = function getSVGData(config) { + return CustomEase.getSVGData(this, config); + }; + + CustomEase.create = function create(id, data, config) { + return new CustomEase(id, data, config).ease; + }; + + CustomEase.register = function register(core) { + gsap = core; + + _initCore(); + }; + + CustomEase.get = function get(id) { + return gsap.parseEase(id); + }; + + CustomEase.getSVGData = function getSVGData(ease, config) { + config = config || {}; + var width = config.width || 100, + height = config.height || 100, + x = config.x || 0, + y = (config.y || 0) + height, + e = gsap.utils.toArray(config.path)[0], + a, + slope, + i, + inc, + tx, + ty, + precision, + threshold, + prevX, + prevY; + + if (config.invert) { + height = -height; + y = 0; + } + + if (typeof ease === "string") { + ease = gsap.parseEase(ease); + } + + if (ease.custom) { + ease = ease.custom; + } + + if (ease instanceof CustomEase) { + a = rawPathToString(transformRawPath([ease.segment], width, 0, 0, -height, x, y)); + } else { + a = [x, y]; + precision = Math.max(5, (config.precision || 1) * 200); + inc = 1 / precision; + precision += 2; + threshold = 5 / precision; + prevX = _round(x + inc * width); + prevY = _round(y + ease(inc) * -height); + slope = (prevY - y) / (prevX - x); + + for (i = 2; i < precision; i++) { + tx = _round(x + i * inc * width); + ty = _round(y + ease(i * inc) * -height); + + if (Math.abs((ty - prevY) / (tx - prevX) - slope) > threshold || i === precision - 1) { + //only add points when the slope changes beyond the threshold + a.push(prevX, prevY); + slope = (ty - prevY) / (tx - prevX); + } + + prevX = tx; + prevY = ty; + } + + a = "M" + a.join(","); + } + + e && e.setAttribute("d", a); + return a; + }; + + return CustomEase; +}(); +CustomEase.version = "3.12.7"; +CustomEase.headless = true; +_getGSAP() && gsap.registerPlugin(CustomEase); +export { CustomEase as default }; \ No newline at end of file diff --git a/node_modules/gsap/Draggable.js b/node_modules/gsap/Draggable.js new file mode 100644 index 0000000000000000000000000000000000000000..4314d42d0c11a7651d6ade5869a698a7f1ed30da --- /dev/null +++ b/node_modules/gsap/Draggable.js @@ -0,0 +1,2699 @@ +function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } + +function _inheritsLoose(subClass, superClass) { subClass.prototype = Object.create(superClass.prototype); subClass.prototype.constructor = subClass; subClass.__proto__ = superClass; } + +/*! + * Draggable 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +/* eslint-disable */ +import { getGlobalMatrix, Matrix2D } from "./utils/matrix.js"; + +var gsap, + _win, + _doc, + _docElement, + _body, + _tempDiv, + _placeholderDiv, + _coreInitted, + _checkPrefix, + _toArray, + _supportsPassive, + _isTouchDevice, + _touchEventLookup, + _isMultiTouching, + _isAndroid, + InertiaPlugin, + _defaultCursor, + _supportsPointer, + _context, + _getStyleSaver, + _dragCount = 0, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _isFunction = function _isFunction(value) { + return typeof value === "function"; +}, + _isObject = function _isObject(value) { + return typeof value === "object"; +}, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; +}, + _emptyFunc = function _emptyFunc() { + return false; +}, + _transformProp = "transform", + _transformOriginProp = "transformOrigin", + _round = function _round(value) { + return Math.round(value * 10000) / 10000; +}, + _isArray = Array.isArray, + _createElement = function _createElement(type, ns) { + var e = _doc.createElementNS ? _doc.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc.createElement(type); //some servers swap in https for http in the namespace which can break things, making "style" inaccessible. + + return e.style ? e : _doc.createElement(type); //some environments won't allow access to the element's style when created with a namespace in which case we default to the standard createElement() to work around the issue. Also note that when GSAP is embedded directly inside an SVG file, createElement() won't allow access to the style object in Firefox (see https://gsap.com/forums/topic/20215-problem-using-tweenmax-in-standalone-self-containing-svg-file-err-cannot-set-property-csstext-of-undefined/). +}, + _RAD2DEG = 180 / Math.PI, + _bigNum = 1e20, + _identityMatrix = new Matrix2D(), + _getTime = Date.now || function () { + return new Date().getTime(); +}, + _renderQueue = [], + _lookup = {}, + //when a Draggable is created, the target gets a unique _gsDragID property that allows gets associated with the Draggable instance for quick lookups in Draggable.get(). This avoids circular references that could cause gc problems. +_lookupCount = 0, + _clickableTagExp = /^(?:a|input|textarea|button|select)$/i, + _lastDragTime = 0, + _temp1 = {}, + // a simple object we reuse and populate (usually x/y properties) to conserve memory and improve performance. +_windowProxy = {}, + //memory/performance optimization - we reuse this object during autoScroll to store window-related bounds/offsets. +_copy = function _copy(obj, factor) { + var copy = {}, + p; + + for (p in obj) { + copy[p] = factor ? obj[p] * factor : obj[p]; + } + + return copy; +}, + _extend = function _extend(obj, defaults) { + for (var p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + + return obj; +}, + _setTouchActionForAllDescendants = function _setTouchActionForAllDescendants(elements, value) { + var i = elements.length, + children; + + while (i--) { + value ? elements[i].style.touchAction = value : elements[i].style.removeProperty("touch-action"); + children = elements[i].children; + children && children.length && _setTouchActionForAllDescendants(children, value); + } +}, + _renderQueueTick = function _renderQueueTick() { + return _renderQueue.forEach(function (func) { + return func(); + }); +}, + _addToRenderQueue = function _addToRenderQueue(func) { + _renderQueue.push(func); + + if (_renderQueue.length === 1) { + gsap.ticker.add(_renderQueueTick); + } +}, + _renderQueueTimeout = function _renderQueueTimeout() { + return !_renderQueue.length && gsap.ticker.remove(_renderQueueTick); +}, + _removeFromRenderQueue = function _removeFromRenderQueue(func) { + var i = _renderQueue.length; + + while (i--) { + if (_renderQueue[i] === func) { + _renderQueue.splice(i, 1); + } + } + + gsap.to(_renderQueueTimeout, { + overwrite: true, + delay: 15, + duration: 0, + onComplete: _renderQueueTimeout, + data: "_draggable" + }); //remove the "tick" listener only after the render queue is empty for 15 seconds (to improve performance). Adding/removing it constantly for every click/touch wouldn't deliver optimal speed, and we also don't want the ticker to keep calling the render method when things are idle for long periods of time (we want to improve battery life on mobile devices). +}, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + + return obj; +}, + _addListener = function _addListener(element, type, func, capture) { + if (element.addEventListener) { + var touchType = _touchEventLookup[type]; + capture = capture || (_supportsPassive ? { + passive: false + } : null); + element.addEventListener(touchType || type, func, capture); + touchType && type !== touchType && element.addEventListener(type, func, capture); //some browsers actually support both, so must we. But pointer events cover all. + } +}, + _removeListener = function _removeListener(element, type, func, capture) { + if (element.removeEventListener) { + var touchType = _touchEventLookup[type]; + element.removeEventListener(touchType || type, func, capture); + touchType && type !== touchType && element.removeEventListener(type, func, capture); + } +}, + _preventDefault = function _preventDefault(event) { + event.preventDefault && event.preventDefault(); + event.preventManipulation && event.preventManipulation(); //for some Microsoft browsers +}, + _hasTouchID = function _hasTouchID(list, ID) { + var i = list.length; + + while (i--) { + if (list[i].identifier === ID) { + return true; + } + } +}, + _onMultiTouchDocumentEnd = function _onMultiTouchDocumentEnd(event) { + _isMultiTouching = event.touches && _dragCount < event.touches.length; + + _removeListener(event.target, "touchend", _onMultiTouchDocumentEnd); +}, + _onMultiTouchDocument = function _onMultiTouchDocument(event) { + _isMultiTouching = event.touches && _dragCount < event.touches.length; + + _addListener(event.target, "touchend", _onMultiTouchDocumentEnd); +}, + _getDocScrollTop = function _getDocScrollTop(doc) { + return _win.pageYOffset || doc.scrollTop || doc.documentElement.scrollTop || doc.body.scrollTop || 0; +}, + _getDocScrollLeft = function _getDocScrollLeft(doc) { + return _win.pageXOffset || doc.scrollLeft || doc.documentElement.scrollLeft || doc.body.scrollLeft || 0; +}, + _addScrollListener = function _addScrollListener(e, callback) { + _addListener(e, "scroll", callback); + + if (!_isRoot(e.parentNode)) { + _addScrollListener(e.parentNode, callback); + } +}, + _removeScrollListener = function _removeScrollListener(e, callback) { + _removeListener(e, "scroll", callback); + + if (!_isRoot(e.parentNode)) { + _removeScrollListener(e.parentNode, callback); + } +}, + _isRoot = function _isRoot(e) { + return !!(!e || e === _docElement || e.nodeType === 9 || e === _doc.body || e === _win || !e.nodeType || !e.parentNode); +}, + _getMaxScroll = function _getMaxScroll(element, axis) { + var dim = axis === "x" ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return Math.max(0, _isRoot(element) ? Math.max(_docElement[scroll], _body[scroll]) - (_win["inner" + dim] || _docElement[client] || _body[client]) : element[scroll] - element[client]); +}, + _recordMaxScrolls = function _recordMaxScrolls(e, skipCurrent) { + //records _gsMaxScrollX and _gsMaxScrollY properties for the element and all ancestors up the chain so that we can cap it, otherwise dragging beyond the edges with autoScroll on can endlessly scroll. + var x = _getMaxScroll(e, "x"), + y = _getMaxScroll(e, "y"); + + if (_isRoot(e)) { + e = _windowProxy; + } else { + _recordMaxScrolls(e.parentNode, skipCurrent); + } + + e._gsMaxScrollX = x; + e._gsMaxScrollY = y; + + if (!skipCurrent) { + e._gsScrollX = e.scrollLeft || 0; + e._gsScrollY = e.scrollTop || 0; + } +}, + _setStyle = function _setStyle(element, property, value) { + var style = element.style; + + if (!style) { + return; + } + + if (_isUndefined(style[property])) { + property = _checkPrefix(property, element) || property; + } + + if (value == null) { + style.removeProperty && style.removeProperty(property.replace(/([A-Z])/g, "-$1").toLowerCase()); + } else { + style[property] = value; + } +}, + _getComputedStyle = function _getComputedStyle(element) { + return _win.getComputedStyle(element instanceof Element ? element : element.host || (element.parentNode || {}).host || element); +}, + //the "host" stuff helps to accommodate ShadowDom objects. +_tempRect = {}, + //reuse to reduce garbage collection tasks +_parseRect = function _parseRect(e) { + //accepts a DOM element, a mouse event, or a rectangle object and returns the corresponding rectangle with left, right, width, height, top, and bottom properties + if (e === _win) { + _tempRect.left = _tempRect.top = 0; + _tempRect.width = _tempRect.right = _docElement.clientWidth || e.innerWidth || _body.clientWidth || 0; + _tempRect.height = _tempRect.bottom = (e.innerHeight || 0) - 20 < _docElement.clientHeight ? _docElement.clientHeight : e.innerHeight || _body.clientHeight || 0; + return _tempRect; + } + + var doc = e.ownerDocument || _doc, + r = !_isUndefined(e.pageX) ? { + left: e.pageX - _getDocScrollLeft(doc), + top: e.pageY - _getDocScrollTop(doc), + right: e.pageX - _getDocScrollLeft(doc) + 1, + bottom: e.pageY - _getDocScrollTop(doc) + 1 + } : !e.nodeType && !_isUndefined(e.left) && !_isUndefined(e.top) ? e : _toArray(e)[0].getBoundingClientRect(); + + if (_isUndefined(r.right) && !_isUndefined(r.width)) { + r.right = r.left + r.width; + r.bottom = r.top + r.height; + } else if (_isUndefined(r.width)) { + //some browsers don't include width and height properties. We can't just set them directly on r because some browsers throw errors, so create a new generic object. + r = { + width: r.right - r.left, + height: r.bottom - r.top, + right: r.right, + left: r.left, + bottom: r.bottom, + top: r.top + }; + } + + return r; +}, + _dispatchEvent = function _dispatchEvent(target, type, callbackName) { + var vars = target.vars, + callback = vars[callbackName], + listeners = target._listeners[type], + result; + + if (_isFunction(callback)) { + result = callback.apply(vars.callbackScope || target, vars[callbackName + "Params"] || [target.pointerEvent]); + } + + if (listeners && target.dispatchEvent(type) === false) { + result = false; + } + + return result; +}, + _getBounds = function _getBounds(target, context) { + //accepts any of the following: a DOM element, jQuery object, selector text, or an object defining bounds as {top, left, width, height} or {minX, maxX, minY, maxY}. Returns an object with left, top, width, and height properties. + var e = _toArray(target)[0], + top, + left, + offset; + + if (!e.nodeType && e !== _win) { + if (!_isUndefined(target.left)) { + offset = { + x: 0, + y: 0 + }; //_getOffsetTransformOrigin(context); //the bounds should be relative to the origin + + return { + left: target.left - offset.x, + top: target.top - offset.y, + width: target.width, + height: target.height + }; + } + + left = target.min || target.minX || target.minRotation || 0; + top = target.min || target.minY || 0; + return { + left: left, + top: top, + width: (target.max || target.maxX || target.maxRotation || 0) - left, + height: (target.max || target.maxY || 0) - top + }; + } + + return _getElementBounds(e, context); +}, + _point1 = {}, + //we reuse to minimize garbage collection tasks. +_getElementBounds = function _getElementBounds(element, context) { + context = _toArray(context)[0]; + var isSVG = element.getBBox && element.ownerSVGElement, + doc = element.ownerDocument || _doc, + left, + right, + top, + bottom, + matrix, + p1, + p2, + p3, + p4, + bbox, + width, + height, + cs; + + if (element === _win) { + top = _getDocScrollTop(doc); + left = _getDocScrollLeft(doc); + right = left + (doc.documentElement.clientWidth || element.innerWidth || doc.body.clientWidth || 0); + bottom = top + ((element.innerHeight || 0) - 20 < doc.documentElement.clientHeight ? doc.documentElement.clientHeight : element.innerHeight || doc.body.clientHeight || 0); //some browsers (like Firefox) ignore absolutely positioned elements, and collapse the height of the documentElement, so it could be 8px, for example, if you have just an absolutely positioned div. In that case, we use the innerHeight to resolve this. + } else if (context === _win || _isUndefined(context)) { + return element.getBoundingClientRect(); + } else { + left = top = 0; + + if (isSVG) { + bbox = element.getBBox(); + width = bbox.width; + height = bbox.height; + } else { + if (element.viewBox && (bbox = element.viewBox.baseVal)) { + left = bbox.x || 0; + top = bbox.y || 0; + width = bbox.width; + height = bbox.height; + } + + if (!width) { + cs = _getComputedStyle(element); + bbox = cs.boxSizing === "border-box"; + width = (parseFloat(cs.width) || element.clientWidth || 0) + (bbox ? 0 : parseFloat(cs.borderLeftWidth) + parseFloat(cs.borderRightWidth)); + height = (parseFloat(cs.height) || element.clientHeight || 0) + (bbox ? 0 : parseFloat(cs.borderTopWidth) + parseFloat(cs.borderBottomWidth)); + } + } + + right = width; + bottom = height; + } + + if (element === context) { + return { + left: left, + top: top, + width: right - left, + height: bottom - top + }; + } + + matrix = getGlobalMatrix(context, true).multiply(getGlobalMatrix(element)); + p1 = matrix.apply({ + x: left, + y: top + }); + p2 = matrix.apply({ + x: right, + y: top + }); + p3 = matrix.apply({ + x: right, + y: bottom + }); + p4 = matrix.apply({ + x: left, + y: bottom + }); + left = Math.min(p1.x, p2.x, p3.x, p4.x); + top = Math.min(p1.y, p2.y, p3.y, p4.y); + return { + left: left, + top: top, + width: Math.max(p1.x, p2.x, p3.x, p4.x) - left, + height: Math.max(p1.y, p2.y, p3.y, p4.y) - top + }; +}, + _parseInertia = function _parseInertia(draggable, snap, max, min, factor, forceZeroVelocity) { + var vars = {}, + a, + i, + l; + + if (snap) { + if (factor !== 1 && snap instanceof Array) { + //some data must be altered to make sense, like if the user passes in an array of rotational values in degrees, we must convert it to radians. Or for scrollLeft and scrollTop, we invert the values. + vars.end = a = []; + l = snap.length; + + if (_isObject(snap[0])) { + //if the array is populated with objects, like points ({x:100, y:200}), make copies before multiplying by the factor, otherwise we'll mess up the originals and the user may reuse it elsewhere. + for (i = 0; i < l; i++) { + a[i] = _copy(snap[i], factor); + } + } else { + for (i = 0; i < l; i++) { + a[i] = snap[i] * factor; + } + } + + max += 1.1; //allow 1.1 pixels of wiggle room when snapping in order to work around some browser inconsistencies in the way bounds are reported which can make them roughly a pixel off. For example, if "snap:[-$('#menu').width(), 0]" was defined and #menu had a wrapper that was used as the bounds, some browsers would be one pixel off, making the minimum -752 for example when snap was [-753,0], thus instead of snapping to -753, it would snap to 0 since -753 was below the minimum. + + min -= 1.1; + } else if (_isFunction(snap)) { + vars.end = function (value) { + var result = snap.call(draggable, value), + copy, + p; + + if (factor !== 1) { + if (_isObject(result)) { + copy = {}; + + for (p in result) { + copy[p] = result[p] * factor; + } + + result = copy; + } else { + result *= factor; + } + } + + return result; //we need to ensure that we can scope the function call to the Draggable instance itself so that users can access important values like maxX, minX, maxY, minY, x, and y from within that function. + }; + } else { + vars.end = snap; + } + } + + if (max || max === 0) { + vars.max = max; + } + + if (min || min === 0) { + vars.min = min; + } + + if (forceZeroVelocity) { + vars.velocity = 0; + } + + return vars; +}, + _isClickable = function _isClickable(element) { + //sometimes it's convenient to mark an element as clickable by adding a data-clickable="true" attribute (in which case we won't preventDefault() the mouse/touch event). This method checks if the element is an <a>, <input>, or <button> or has the data-clickable or contentEditable attribute set to true (or any of its parent elements). + var data; + return !element || !element.getAttribute || element === _body ? false : (data = element.getAttribute("data-clickable")) === "true" || data !== "false" && (_clickableTagExp.test(element.nodeName + "") || element.getAttribute("contentEditable") === "true") ? true : _isClickable(element.parentNode); +}, + _setSelectable = function _setSelectable(elements, selectable) { + var i = elements.length, + e; + + while (i--) { + e = elements[i]; + e.ondragstart = e.onselectstart = selectable ? null : _emptyFunc; + gsap.set(e, { + lazy: true, + userSelect: selectable ? "text" : "none" + }); + } +}, + _isFixed = function _isFixed(element) { + if (_getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + // avoid document fragments which will throw an error. + return _isFixed(element); + } +}, + _supports3D, + _addPaddingBR, + //The ScrollProxy class wraps an element's contents into another div (we call it "content") that we either add padding when necessary or apply a translate3d() transform in order to overscroll (scroll past the boundaries). This allows us to simply set the scrollTop/scrollLeft (or top/left for easier reverse-axis orientation, which is what we do in Draggable) and it'll do all the work for us. For example, if we tried setting scrollTop to -100 on a normal DOM element, it wouldn't work - it'd look the same as setting it to 0, but if we set scrollTop of a ScrollProxy to -100, it'll give the correct appearance by either setting paddingTop of the wrapper to 100 or applying a 100-pixel translateY. +ScrollProxy = function ScrollProxy(element, vars) { + element = gsap.utils.toArray(element)[0]; + vars = vars || {}; + var content = document.createElement("div"), + style = content.style, + node = element.firstChild, + offsetTop = 0, + offsetLeft = 0, + prevTop = element.scrollTop, + prevLeft = element.scrollLeft, + scrollWidth = element.scrollWidth, + scrollHeight = element.scrollHeight, + extraPadRight = 0, + maxLeft = 0, + maxTop = 0, + elementWidth, + elementHeight, + contentHeight, + nextNode, + transformStart, + transformEnd; + + if (_supports3D && vars.force3D !== false) { + transformStart = "translate3d("; + transformEnd = "px,0px)"; + } else if (_transformProp) { + transformStart = "translate("; + transformEnd = "px)"; + } + + this.scrollTop = function (value, force) { + if (!arguments.length) { + return -this.top(); + } + + this.top(-value, force); + }; + + this.scrollLeft = function (value, force) { + if (!arguments.length) { + return -this.left(); + } + + this.left(-value, force); + }; + + this.left = function (value, force) { + if (!arguments.length) { + return -(element.scrollLeft + offsetLeft); + } + + var dif = element.scrollLeft - prevLeft, + oldOffset = offsetLeft; + + if ((dif > 2 || dif < -2) && !force) { + //if the user interacts with the scrollbar (or something else scrolls it, like the mouse wheel), we should kill any tweens of the ScrollProxy. + prevLeft = element.scrollLeft; + gsap.killTweensOf(this, { + left: 1, + scrollLeft: 1 + }); + this.left(-prevLeft); + + if (vars.onKill) { + vars.onKill(); + } + + return; + } + + value = -value; //invert because scrolling works in the opposite direction + + if (value < 0) { + offsetLeft = value - 0.5 | 0; + value = 0; + } else if (value > maxLeft) { + offsetLeft = value - maxLeft | 0; + value = maxLeft; + } else { + offsetLeft = 0; + } + + if (offsetLeft || oldOffset) { + if (!this._skip) { + style[_transformProp] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + + if (offsetLeft + extraPadRight >= 0) { + style.paddingRight = offsetLeft + extraPadRight + "px"; + } + } + + element.scrollLeft = value | 0; + prevLeft = element.scrollLeft; //don't merge this with the line above because some browsers adjust the scrollLeft after it's set, so in order to be 100% accurate in tracking it, we need to ask the browser to report it. + }; + + this.top = function (value, force) { + if (!arguments.length) { + return -(element.scrollTop + offsetTop); + } + + var dif = element.scrollTop - prevTop, + oldOffset = offsetTop; + + if ((dif > 2 || dif < -2) && !force) { + //if the user interacts with the scrollbar (or something else scrolls it, like the mouse wheel), we should kill any tweens of the ScrollProxy. + prevTop = element.scrollTop; + gsap.killTweensOf(this, { + top: 1, + scrollTop: 1 + }); + this.top(-prevTop); + + if (vars.onKill) { + vars.onKill(); + } + + return; + } + + value = -value; //invert because scrolling works in the opposite direction + + if (value < 0) { + offsetTop = value - 0.5 | 0; + value = 0; + } else if (value > maxTop) { + offsetTop = value - maxTop | 0; + value = maxTop; + } else { + offsetTop = 0; + } + + if (offsetTop || oldOffset) { + if (!this._skip) { + style[_transformProp] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + } + + element.scrollTop = value | 0; + prevTop = element.scrollTop; + }; + + this.maxScrollTop = function () { + return maxTop; + }; + + this.maxScrollLeft = function () { + return maxLeft; + }; + + this.disable = function () { + node = content.firstChild; + + while (node) { + nextNode = node.nextSibling; + element.appendChild(node); + node = nextNode; + } + + if (element === content.parentNode) { + //in case disable() is called when it's already disabled. + element.removeChild(content); + } + }; + + this.enable = function () { + node = element.firstChild; + + if (node === content) { + return; + } + + while (node) { + nextNode = node.nextSibling; + content.appendChild(node); + node = nextNode; + } + + element.appendChild(content); + this.calibrate(); + }; + + this.calibrate = function (force) { + var widthMatches = element.clientWidth === elementWidth, + cs, + x, + y; + prevTop = element.scrollTop; + prevLeft = element.scrollLeft; + + if (widthMatches && element.clientHeight === elementHeight && content.offsetHeight === contentHeight && scrollWidth === element.scrollWidth && scrollHeight === element.scrollHeight && !force) { + return; //no need to recalculate things if the width and height haven't changed. + } + + if (offsetTop || offsetLeft) { + x = this.left(); + y = this.top(); + this.left(-element.scrollLeft); + this.top(-element.scrollTop); + } + + cs = _getComputedStyle(element); //first, we need to remove any width constraints to see how the content naturally flows so that we can see if it's wider than the containing element. If so, we've got to record the amount of overage so that we can apply that as padding in order for browsers to correctly handle things. Then we switch back to a width of 100% (without that, some browsers don't flow the content correctly) + + if (!widthMatches || force) { + style.display = "block"; + style.width = "auto"; + style.paddingRight = "0px"; + extraPadRight = Math.max(0, element.scrollWidth - element.clientWidth); //if the content is wider than the container, we need to add the paddingLeft and paddingRight in order for things to behave correctly. + + if (extraPadRight) { + extraPadRight += parseFloat(cs.paddingLeft) + (_addPaddingBR ? parseFloat(cs.paddingRight) : 0); + } + } + + style.display = "inline-block"; + style.position = "relative"; + style.overflow = "visible"; + style.verticalAlign = "top"; + style.boxSizing = "content-box"; + style.width = "100%"; + style.paddingRight = extraPadRight + "px"; //some browsers neglect to factor in the bottom padding when calculating the scrollHeight, so we need to add that padding to the content when that happens. Allow a 2px margin for error + + if (_addPaddingBR) { + style.paddingBottom = cs.paddingBottom; + } + + elementWidth = element.clientWidth; + elementHeight = element.clientHeight; + scrollWidth = element.scrollWidth; + scrollHeight = element.scrollHeight; + maxLeft = element.scrollWidth - elementWidth; + maxTop = element.scrollHeight - elementHeight; + contentHeight = content.offsetHeight; + style.display = "block"; + + if (x || y) { + this.left(x); + this.top(y); + } + }; + + this.content = content; + this.element = element; + this._skip = false; + this.enable(); +}, + _initCore = function _initCore(required) { + if (_windowExists() && document.body) { + var nav = window && window.navigator; + _win = window; + _doc = document; + _docElement = _doc.documentElement; + _body = _doc.body; + _tempDiv = _createElement("div"); + _supportsPointer = !!window.PointerEvent; + _placeholderDiv = _createElement("div"); + _placeholderDiv.style.cssText = "visibility:hidden;height:1px;top:-1px;pointer-events:none;position:relative;clear:both;cursor:grab"; + _defaultCursor = _placeholderDiv.style.cursor === "grab" ? "grab" : "move"; + _isAndroid = nav && nav.userAgent.toLowerCase().indexOf("android") !== -1; //Android handles touch events in an odd way and it's virtually impossible to "feature test" so we resort to UA sniffing + + _isTouchDevice = "ontouchstart" in _docElement && "orientation" in _win || nav && (nav.MaxTouchPoints > 0 || nav.msMaxTouchPoints > 0); + + _addPaddingBR = function () { + //this function is in charge of analyzing browser behavior related to padding. It sets the _addPaddingBR to true if the browser doesn't normally factor in the bottom or right padding on the element inside the scrolling area, and it sets _addPaddingLeft to true if it's a browser that requires the extra offset (offsetLeft) to be added to the paddingRight (like Opera). + var div = _createElement("div"), + child = _createElement("div"), + childStyle = child.style, + parent = _body, + val; + + childStyle.display = "inline-block"; + childStyle.position = "relative"; + div.style.cssText = "width:90px;height:40px;padding:10px;overflow:auto;visibility:hidden"; + div.appendChild(child); + parent.appendChild(div); + val = child.offsetHeight + 18 > div.scrollHeight; //div.scrollHeight should be child.offsetHeight + 20 because of the 10px of padding on each side, but some browsers ignore one side. We allow a 2px margin of error. + + parent.removeChild(div); + return val; + }(); + + _touchEventLookup = function (types) { + //we create an object that makes it easy to translate touch event types into their "pointer" counterparts if we're in a browser that uses those instead. Like IE10 uses "MSPointerDown" instead of "touchstart", for example. + var standard = types.split(","), + converted = ("onpointerdown" in _tempDiv ? "pointerdown,pointermove,pointerup,pointercancel" : "onmspointerdown" in _tempDiv ? "MSPointerDown,MSPointerMove,MSPointerUp,MSPointerCancel" : types).split(","), + obj = {}, + i = 4; + + while (--i > -1) { + obj[standard[i]] = converted[i]; + obj[converted[i]] = standard[i]; + } //to avoid problems in iOS 9, test to see if the browser supports the "passive" option on addEventListener(). + + + try { + _docElement.addEventListener("test", null, Object.defineProperty({}, "passive", { + get: function get() { + _supportsPassive = 1; + } + })); + } catch (e) {} + + return obj; + }("touchstart,touchmove,touchend,touchcancel"); + + _addListener(_doc, "touchcancel", _emptyFunc); //some older Android devices intermittently stop dispatching "touchmove" events if we don't listen for "touchcancel" on the document. Very strange indeed. + + + _addListener(_win, "touchmove", _emptyFunc); //works around Safari bugs that still allow the page to scroll even when we preventDefault() on the touchmove event. + + + _body && _body.addEventListener("touchstart", _emptyFunc); //works around Safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/ + + _addListener(_doc, "contextmenu", function () { + for (var p in _lookup) { + if (_lookup[p].isPressed) { + _lookup[p].endDrag(); + } + } + }); + + gsap = _coreInitted = _getGSAP(); + } + + if (gsap) { + InertiaPlugin = gsap.plugins.inertia; + + _context = gsap.core.context || function () {}; + + _checkPrefix = gsap.utils.checkPrefix; + _transformProp = _checkPrefix(_transformProp); + _transformOriginProp = _checkPrefix(_transformOriginProp); + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + _supports3D = !!_checkPrefix("perspective"); + } else if (required) { + console.warn("Please gsap.registerPlugin(Draggable)"); + } +}; + +var EventDispatcher = /*#__PURE__*/function () { + function EventDispatcher(target) { + this._listeners = {}; + this.target = target || this; + } + + var _proto = EventDispatcher.prototype; + + _proto.addEventListener = function addEventListener(type, callback) { + var list = this._listeners[type] || (this._listeners[type] = []); + + if (!~list.indexOf(callback)) { + list.push(callback); + } + }; + + _proto.removeEventListener = function removeEventListener(type, callback) { + var list = this._listeners[type], + i = list && list.indexOf(callback); + i >= 0 && list.splice(i, 1); + }; + + _proto.dispatchEvent = function dispatchEvent(type) { + var _this = this; + + var result; + (this._listeners[type] || []).forEach(function (callback) { + return callback.call(_this, { + type: type, + target: _this.target + }) === false && (result = false); + }); + return result; //if any of the callbacks return false, pass that along. + }; + + return EventDispatcher; +}(); + +export var Draggable = /*#__PURE__*/function (_EventDispatcher) { + _inheritsLoose(Draggable, _EventDispatcher); + + function Draggable(target, vars) { + var _this2; + + _this2 = _EventDispatcher.call(this) || this; + _coreInitted || _initCore(1); + target = _toArray(target)[0]; //in case the target is a selector object or selector text + + _this2.styles = _getStyleSaver && _getStyleSaver(target, "transform,left,top"); + + if (!InertiaPlugin) { + InertiaPlugin = gsap.plugins.inertia; + } + + _this2.vars = vars = _copy(vars || {}); + _this2.target = target; + _this2.x = _this2.y = _this2.rotation = 0; + _this2.dragResistance = parseFloat(vars.dragResistance) || 0; + _this2.edgeResistance = isNaN(vars.edgeResistance) ? 1 : parseFloat(vars.edgeResistance) || 0; + _this2.lockAxis = vars.lockAxis; + _this2.autoScroll = vars.autoScroll || 0; + _this2.lockedAxis = null; + _this2.allowEventDefault = !!vars.allowEventDefault; + gsap.getProperty(target, "x"); // to ensure that transforms are instantiated. + + var type = (vars.type || "x,y").toLowerCase(), + xyMode = ~type.indexOf("x") || ~type.indexOf("y"), + rotationMode = type.indexOf("rotation") !== -1, + xProp = rotationMode ? "rotation" : xyMode ? "x" : "left", + yProp = xyMode ? "y" : "top", + allowX = !!(~type.indexOf("x") || ~type.indexOf("left") || type === "scroll"), + allowY = !!(~type.indexOf("y") || ~type.indexOf("top") || type === "scroll"), + minimumMovement = vars.minimumMovement || 2, + self = _assertThisInitialized(_this2), + triggers = _toArray(vars.trigger || vars.handle || target), + killProps = {}, + dragEndTime = 0, + checkAutoScrollBounds = false, + autoScrollMarginTop = vars.autoScrollMarginTop || 40, + autoScrollMarginRight = vars.autoScrollMarginRight || 40, + autoScrollMarginBottom = vars.autoScrollMarginBottom || 40, + autoScrollMarginLeft = vars.autoScrollMarginLeft || 40, + isClickable = vars.clickableTest || _isClickable, + clickTime = 0, + gsCache = target._gsap || gsap.core.getCache(target), + isFixed = _isFixed(target), + getPropAsNum = function getPropAsNum(property, unit) { + return parseFloat(gsCache.get(target, property, unit)); + }, + ownerDoc = target.ownerDocument || _doc, + enabled, + scrollProxy, + startPointerX, + startPointerY, + startElementX, + startElementY, + hasBounds, + hasDragCallback, + hasMoveCallback, + maxX, + minX, + maxY, + minY, + touch, + touchID, + rotationOrigin, + dirty, + old, + snapX, + snapY, + snapXY, + isClicking, + touchEventTarget, + matrix, + interrupted, + allowNativeTouchScrolling, + touchDragAxis, + isDispatching, + clickDispatch, + trustedClickDispatch, + isPreventingDefault, + innerMatrix, + dragged, + onContextMenu = function onContextMenu(e) { + //used to prevent long-touch from triggering a context menu. + // (self.isPressed && e.which < 2) && self.endDrag() // previously ended drag when context menu was triggered, but instead we should just stop propagation and prevent the default event behavior. + _preventDefault(e); + + e.stopImmediatePropagation && e.stopImmediatePropagation(); + return false; + }, + //this method gets called on every tick of TweenLite.ticker which allows us to synchronize the renders to the core engine (which is typically synchronized with the display refresh via requestAnimationFrame). This is an optimization - it's better than applying the values inside the "mousemove" or "touchmove" event handler which may get called many times inbetween refreshes. + render = function render(suppressEvents) { + if (self.autoScroll && self.isDragging && (checkAutoScrollBounds || dirty)) { + var e = target, + autoScrollFactor = self.autoScroll * 15, + //multiplying by 15 just gives us a better "feel" speed-wise. + parent, + isRoot, + rect, + pointerX, + pointerY, + changeX, + changeY, + gap; + checkAutoScrollBounds = false; + _windowProxy.scrollTop = _win.pageYOffset != null ? _win.pageYOffset : ownerDoc.documentElement.scrollTop != null ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop; + _windowProxy.scrollLeft = _win.pageXOffset != null ? _win.pageXOffset : ownerDoc.documentElement.scrollLeft != null ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft; + pointerX = self.pointerX - _windowProxy.scrollLeft; + pointerY = self.pointerY - _windowProxy.scrollTop; + + while (e && !isRoot) { + //walk up the chain and sense wherever the pointer is within 40px of an edge that's scrollable. + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + rect = isRoot ? { + bottom: Math.max(_docElement.clientHeight, _win.innerHeight || 0), + right: Math.max(_docElement.clientWidth, _win.innerWidth || 0), + left: 0, + top: 0 + } : parent.getBoundingClientRect(); + changeX = changeY = 0; + + if (allowY) { + gap = parent._gsMaxScrollY - parent.scrollTop; + + if (gap < 0) { + changeY = gap; + } else if (pointerY > rect.bottom - autoScrollMarginBottom && gap) { + checkAutoScrollBounds = true; + changeY = Math.min(gap, autoScrollFactor * (1 - Math.max(0, rect.bottom - pointerY) / autoScrollMarginBottom) | 0); + } else if (pointerY < rect.top + autoScrollMarginTop && parent.scrollTop) { + checkAutoScrollBounds = true; + changeY = -Math.min(parent.scrollTop, autoScrollFactor * (1 - Math.max(0, pointerY - rect.top) / autoScrollMarginTop) | 0); + } + + if (changeY) { + parent.scrollTop += changeY; + } + } + + if (allowX) { + gap = parent._gsMaxScrollX - parent.scrollLeft; + + if (gap < 0) { + changeX = gap; + } else if (pointerX > rect.right - autoScrollMarginRight && gap) { + checkAutoScrollBounds = true; + changeX = Math.min(gap, autoScrollFactor * (1 - Math.max(0, rect.right - pointerX) / autoScrollMarginRight) | 0); + } else if (pointerX < rect.left + autoScrollMarginLeft && parent.scrollLeft) { + checkAutoScrollBounds = true; + changeX = -Math.min(parent.scrollLeft, autoScrollFactor * (1 - Math.max(0, pointerX - rect.left) / autoScrollMarginLeft) | 0); + } + + if (changeX) { + parent.scrollLeft += changeX; + } + } + + if (isRoot && (changeX || changeY)) { + _win.scrollTo(parent.scrollLeft, parent.scrollTop); + + setPointerPosition(self.pointerX + changeX, self.pointerY + changeY); + } + + e = parent; + } + } + + if (dirty) { + var x = self.x, + y = self.y; + + if (rotationMode) { + self.deltaX = x - parseFloat(gsCache.rotation); + self.rotation = x; + gsCache.rotation = x + "deg"; + gsCache.renderTransform(1, gsCache); + } else { + if (scrollProxy) { + if (allowY) { + self.deltaY = y - scrollProxy.top(); + scrollProxy.top(y); + } + + if (allowX) { + self.deltaX = x - scrollProxy.left(); + scrollProxy.left(x); + } + } else if (xyMode) { + if (allowY) { + self.deltaY = y - parseFloat(gsCache.y); + gsCache.y = y + "px"; + } + + if (allowX) { + self.deltaX = x - parseFloat(gsCache.x); + gsCache.x = x + "px"; + } + + gsCache.renderTransform(1, gsCache); + } else { + if (allowY) { + self.deltaY = y - parseFloat(target.style.top || 0); + target.style.top = y + "px"; + } + + if (allowX) { + self.deltaX = x - parseFloat(target.style.left || 0); + target.style.left = x + "px"; + } + } + } + + if (hasDragCallback && !suppressEvents && !isDispatching) { + isDispatching = true; //in case onDrag has an update() call (avoid endless loop) + + if (_dispatchEvent(self, "drag", "onDrag") === false) { + if (allowX) { + self.x -= self.deltaX; + } + + if (allowY) { + self.y -= self.deltaY; + } + + render(true); + } + + isDispatching = false; + } + } + + dirty = false; + }, + //copies the x/y from the element (whether that be transforms, top/left, or ScrollProxy's top/left) to the Draggable's x and y (and rotation if necessary) properties so that they reflect reality and it also (optionally) applies any snapping necessary. This is used by the InertiaPlugin tween in an onUpdate to ensure things are synced and snapped. + syncXY = function syncXY(skipOnUpdate, skipSnap) { + var x = self.x, + y = self.y, + snappedValue, + cs; + + if (!target._gsap) { + //just in case the _gsap cache got wiped, like if the user called clearProps on the transform or something (very rare). + gsCache = gsap.core.getCache(target); + } + + gsCache.uncache && gsap.getProperty(target, "x"); // trigger a re-cache + + if (xyMode) { + self.x = parseFloat(gsCache.x); + self.y = parseFloat(gsCache.y); + } else if (rotationMode) { + self.x = self.rotation = parseFloat(gsCache.rotation); + } else if (scrollProxy) { + self.y = scrollProxy.top(); + self.x = scrollProxy.left(); + } else { + self.y = parseFloat(target.style.top || (cs = _getComputedStyle(target)) && cs.top) || 0; + self.x = parseFloat(target.style.left || (cs || {}).left) || 0; + } + + if ((snapX || snapY || snapXY) && !skipSnap && (self.isDragging || self.isThrowing)) { + if (snapXY) { + _temp1.x = self.x; + _temp1.y = self.y; + snappedValue = snapXY(_temp1); + + if (snappedValue.x !== self.x) { + self.x = snappedValue.x; + dirty = true; + } + + if (snappedValue.y !== self.y) { + self.y = snappedValue.y; + dirty = true; + } + } + + if (snapX) { + snappedValue = snapX(self.x); + + if (snappedValue !== self.x) { + self.x = snappedValue; + + if (rotationMode) { + self.rotation = snappedValue; + } + + dirty = true; + } + } + + if (snapY) { + snappedValue = snapY(self.y); + + if (snappedValue !== self.y) { + self.y = snappedValue; + } + + dirty = true; + } + } + + dirty && render(true); + + if (!skipOnUpdate) { + self.deltaX = self.x - x; + self.deltaY = self.y - y; + + _dispatchEvent(self, "throwupdate", "onThrowUpdate"); + } + }, + buildSnapFunc = function buildSnapFunc(snap, min, max, factor) { + if (min == null) { + min = -_bigNum; + } + + if (max == null) { + max = _bigNum; + } + + if (_isFunction(snap)) { + return function (n) { + var edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance; //if we're tweening, disable the edgeTolerance because it's already factored into the tweening values (we don't want to apply it multiple times) + + return snap.call(self, (n > max ? max + (n - max) * edgeTolerance : n < min ? min + (n - min) * edgeTolerance : n) * factor) * factor; + }; + } + + if (_isArray(snap)) { + return function (n) { + var i = snap.length, + closest = 0, + absDif = _bigNum, + val, + dif; + + while (--i > -1) { + val = snap[i]; + dif = val - n; + + if (dif < 0) { + dif = -dif; + } + + if (dif < absDif && val >= min && val <= max) { + closest = i; + absDif = dif; + } + } + + return snap[closest]; + }; + } + + return isNaN(snap) ? function (n) { + return n; + } : function () { + return snap * factor; + }; + }, + buildPointSnapFunc = function buildPointSnapFunc(snap, minX, maxX, minY, maxY, radius, factor) { + radius = radius && radius < _bigNum ? radius * radius : _bigNum; //so we don't have to Math.sqrt() in the functions. Performance optimization. + + if (_isFunction(snap)) { + return function (point) { + var edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance, + x = point.x, + y = point.y, + result, + dx, + dy; //if we're tweening, disable the edgeTolerance because it's already factored into the tweening values (we don't want to apply it multiple times) + + point.x = x = x > maxX ? maxX + (x - maxX) * edgeTolerance : x < minX ? minX + (x - minX) * edgeTolerance : x; + point.y = y = y > maxY ? maxY + (y - maxY) * edgeTolerance : y < minY ? minY + (y - minY) * edgeTolerance : y; + result = snap.call(self, point); + + if (result !== point) { + point.x = result.x; + point.y = result.y; + } + + if (factor !== 1) { + point.x *= factor; + point.y *= factor; + } + + if (radius < _bigNum) { + dx = point.x - x; + dy = point.y - y; + + if (dx * dx + dy * dy > radius) { + point.x = x; + point.y = y; + } + } + + return point; + }; + } + + if (_isArray(snap)) { + return function (p) { + var i = snap.length, + closest = 0, + minDist = _bigNum, + x, + y, + point, + dist; + + while (--i > -1) { + point = snap[i]; + x = point.x - p.x; + y = point.y - p.y; + dist = x * x + y * y; + + if (dist < minDist) { + closest = i; + minDist = dist; + } + } + + return minDist <= radius ? snap[closest] : p; + }; + } + + return function (n) { + return n; + }; + }, + calculateBounds = function calculateBounds() { + var bounds, targetBounds, snap, snapIsRaw; + hasBounds = false; + + if (scrollProxy) { + scrollProxy.calibrate(); + self.minX = minX = -scrollProxy.maxScrollLeft(); + self.minY = minY = -scrollProxy.maxScrollTop(); + self.maxX = maxX = self.maxY = maxY = 0; + hasBounds = true; + } else if (!!vars.bounds) { + bounds = _getBounds(vars.bounds, target.parentNode); //could be a selector/jQuery object or a DOM element or a generic object like {top:0, left:100, width:1000, height:800} or {minX:100, maxX:1100, minY:0, maxY:800} + + if (rotationMode) { + self.minX = minX = bounds.left; + self.maxX = maxX = bounds.left + bounds.width; + self.minY = minY = self.maxY = maxY = 0; + } else if (!_isUndefined(vars.bounds.maxX) || !_isUndefined(vars.bounds.maxY)) { + bounds = vars.bounds; + self.minX = minX = bounds.minX; + self.minY = minY = bounds.minY; + self.maxX = maxX = bounds.maxX; + self.maxY = maxY = bounds.maxY; + } else { + targetBounds = _getBounds(target, target.parentNode); + self.minX = minX = Math.round(getPropAsNum(xProp, "px") + bounds.left - targetBounds.left); + self.minY = minY = Math.round(getPropAsNum(yProp, "px") + bounds.top - targetBounds.top); + self.maxX = maxX = Math.round(minX + (bounds.width - targetBounds.width)); + self.maxY = maxY = Math.round(minY + (bounds.height - targetBounds.height)); + } + + if (minX > maxX) { + self.minX = maxX; + self.maxX = maxX = minX; + minX = self.minX; + } + + if (minY > maxY) { + self.minY = maxY; + self.maxY = maxY = minY; + minY = self.minY; + } + + if (rotationMode) { + self.minRotation = minX; + self.maxRotation = maxX; + } + + hasBounds = true; + } + + if (vars.liveSnap) { + snap = vars.liveSnap === true ? vars.snap || {} : vars.liveSnap; + snapIsRaw = _isArray(snap) || _isFunction(snap); + + if (rotationMode) { + snapX = buildSnapFunc(snapIsRaw ? snap : snap.rotation, minX, maxX, 1); + snapY = null; + } else { + if (snap.points) { + snapXY = buildPointSnapFunc(snapIsRaw ? snap : snap.points, minX, maxX, minY, maxY, snap.radius, scrollProxy ? -1 : 1); + } else { + if (allowX) { + snapX = buildSnapFunc(snapIsRaw ? snap : snap.x || snap.left || snap.scrollLeft, minX, maxX, scrollProxy ? -1 : 1); + } + + if (allowY) { + snapY = buildSnapFunc(snapIsRaw ? snap : snap.y || snap.top || snap.scrollTop, minY, maxY, scrollProxy ? -1 : 1); + } + } + } + } + }, + onThrowComplete = function onThrowComplete() { + self.isThrowing = false; + + _dispatchEvent(self, "throwcomplete", "onThrowComplete"); + }, + onThrowInterrupt = function onThrowInterrupt() { + self.isThrowing = false; + }, + animate = function animate(inertia, forceZeroVelocity) { + var snap, snapIsRaw, tween, overshootTolerance; + + if (inertia && InertiaPlugin) { + if (inertia === true) { + snap = vars.snap || vars.liveSnap || {}; + snapIsRaw = _isArray(snap) || _isFunction(snap); + inertia = { + resistance: (vars.throwResistance || vars.resistance || 1000) / (rotationMode ? 10 : 1) + }; + + if (rotationMode) { + inertia.rotation = _parseInertia(self, snapIsRaw ? snap : snap.rotation, maxX, minX, 1, forceZeroVelocity); + } else { + if (allowX) { + inertia[xProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.x || snap.left, maxX, minX, scrollProxy ? -1 : 1, forceZeroVelocity || self.lockedAxis === "x"); + } + + if (allowY) { + inertia[yProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.y || snap.top, maxY, minY, scrollProxy ? -1 : 1, forceZeroVelocity || self.lockedAxis === "y"); + } + + if (snap.points || _isArray(snap) && _isObject(snap[0])) { + inertia.linkedProps = xProp + "," + yProp; + inertia.radius = snap.radius; //note: we also disable liveSnapping while throwing if there's a "radius" defined, otherwise it looks weird to have the item thrown past a snapping point but live-snapping mid-tween. We do this by altering the onUpdateParams so that "skipSnap" parameter is true for syncXY. + } + } + } + + self.isThrowing = true; + overshootTolerance = !isNaN(vars.overshootTolerance) ? vars.overshootTolerance : vars.edgeResistance === 1 ? 0 : 1 - self.edgeResistance + 0.2; + + if (!inertia.duration) { + inertia.duration = { + max: Math.max(vars.minDuration || 0, "maxDuration" in vars ? vars.maxDuration : 2), + min: !isNaN(vars.minDuration) ? vars.minDuration : overshootTolerance === 0 || _isObject(inertia) && inertia.resistance > 1000 ? 0 : 0.5, + overshoot: overshootTolerance + }; + } + + self.tween = tween = gsap.to(scrollProxy || target, { + inertia: inertia, + data: "_draggable", + inherit: false, + onComplete: onThrowComplete, + onInterrupt: onThrowInterrupt, + onUpdate: vars.fastMode ? _dispatchEvent : syncXY, + onUpdateParams: vars.fastMode ? [self, "onthrowupdate", "onThrowUpdate"] : snap && snap.radius ? [false, true] : [] + }); + + if (!vars.fastMode) { + if (scrollProxy) { + scrollProxy._skip = true; // Microsoft browsers have a bug that causes them to briefly render the position incorrectly (it flashes to the end state when we seek() the tween even though we jump right back to the current position, and this only seems to happen when we're affecting both top and left), so we set a _suspendTransforms flag to prevent it from actually applying the values in the ScrollProxy. + } + + tween.render(1e9, true, true); // force to the end. Remember, the duration will likely change upon initting because that's when InertiaPlugin calculates it. + + syncXY(true, true); + self.endX = self.x; + self.endY = self.y; + + if (rotationMode) { + self.endRotation = self.x; + } + + tween.play(0); + syncXY(true, true); + + if (scrollProxy) { + scrollProxy._skip = false; //Microsoft browsers have a bug that causes them to briefly render the position incorrectly (it flashes to the end state when we seek() the tween even though we jump right back to the current position, and this only seems to happen when we're affecting both top and left), so we set a _suspendTransforms flag to prevent it from actually applying the values in the ScrollProxy. + } + } + } else if (hasBounds) { + self.applyBounds(); + } + }, + updateMatrix = function updateMatrix(shiftStart) { + var start = matrix, + p; + matrix = getGlobalMatrix(target.parentNode, true); + + if (shiftStart && self.isPressed && !matrix.equals(start || new Matrix2D())) { + //if the matrix changes WHILE the element is pressed, we must adjust the startPointerX and startPointerY accordingly, so we invert the original matrix and figure out where the pointerX and pointerY were in the global space, then apply the new matrix to get the updated coordinates. + p = start.inverse().apply({ + x: startPointerX, + y: startPointerY + }); + matrix.apply(p, p); + startPointerX = p.x; + startPointerY = p.y; + } + + if (matrix.equals(_identityMatrix)) { + //if there are no transforms, we can optimize performance by not factoring in the matrix + matrix = null; + } + }, + recordStartPositions = function recordStartPositions() { + var edgeTolerance = 1 - self.edgeResistance, + offsetX = isFixed ? _getDocScrollLeft(ownerDoc) : 0, + offsetY = isFixed ? _getDocScrollTop(ownerDoc) : 0, + parsedOrigin, + x, + y; + + if (xyMode) { + // in case the user set it as a different unit, like animating the x to "100%". We must convert it back to px! + gsCache.x = getPropAsNum(xProp, "px") + "px"; + gsCache.y = getPropAsNum(yProp, "px") + "px"; + gsCache.renderTransform(); + } + + updateMatrix(false); + _point1.x = self.pointerX - offsetX; + _point1.y = self.pointerY - offsetY; + matrix && matrix.apply(_point1, _point1); + startPointerX = _point1.x; //translate to local coordinate system + + startPointerY = _point1.y; + + if (dirty) { + setPointerPosition(self.pointerX, self.pointerY); + render(true); + } + + innerMatrix = getGlobalMatrix(target); + + if (scrollProxy) { + calculateBounds(); + startElementY = scrollProxy.top(); + startElementX = scrollProxy.left(); + } else { + //if the element is in the process of tweening, don't force snapping to occur because it could make it jump. Imagine the user throwing, then before it's done, clicking on the element in its inbetween state. + if (isTweening()) { + syncXY(true, true); + calculateBounds(); + } else { + self.applyBounds(); + } + + if (rotationMode) { + parsedOrigin = target.ownerSVGElement ? [gsCache.xOrigin - target.getBBox().x, gsCache.yOrigin - target.getBBox().y] : (_getComputedStyle(target)[_transformOriginProp] || "0 0").split(" "); + rotationOrigin = self.rotationOrigin = getGlobalMatrix(target).apply({ + x: parseFloat(parsedOrigin[0]) || 0, + y: parseFloat(parsedOrigin[1]) || 0 + }); + syncXY(true, true); + x = self.pointerX - rotationOrigin.x - offsetX; + y = rotationOrigin.y - self.pointerY + offsetY; + startElementX = self.x; //starting rotation (x always refers to rotation in type:"rotation", measured in degrees) + + startElementY = self.y = Math.atan2(y, x) * _RAD2DEG; + } else { + //parent = !isFixed && target.parentNode; + //startScrollTop = parent ? parent.scrollTop || 0 : 0; + //startScrollLeft = parent ? parent.scrollLeft || 0 : 0; + startElementY = getPropAsNum(yProp, "px"); //record the starting top and left values so that we can just add the mouse's movement to them later. + + startElementX = getPropAsNum(xProp, "px"); + } + } + + if (hasBounds && edgeTolerance) { + if (startElementX > maxX) { + startElementX = maxX + (startElementX - maxX) / edgeTolerance; + } else if (startElementX < minX) { + startElementX = minX - (minX - startElementX) / edgeTolerance; + } + + if (!rotationMode) { + if (startElementY > maxY) { + startElementY = maxY + (startElementY - maxY) / edgeTolerance; + } else if (startElementY < minY) { + startElementY = minY - (minY - startElementY) / edgeTolerance; + } + } + } + + self.startX = startElementX = _round(startElementX); + self.startY = startElementY = _round(startElementY); + }, + isTweening = function isTweening() { + return self.tween && self.tween.isActive(); + }, + removePlaceholder = function removePlaceholder() { + if (_placeholderDiv.parentNode && !isTweening() && !self.isDragging) { + //_placeholderDiv just props open auto-scrolling containers so they don't collapse as the user drags left/up. We remove it after dragging (and throwing, if necessary) finishes. + _placeholderDiv.parentNode.removeChild(_placeholderDiv); + } + }, + //called when the mouse is pressed (or touch starts) + onPress = function onPress(e, force) { + var i; + + if (!enabled || self.isPressed || !e || (e.type === "mousedown" || e.type === "pointerdown") && !force && _getTime() - clickTime < 30 && _touchEventLookup[self.pointerEvent.type]) { + //when we DON'T preventDefault() in order to accommodate touch-scrolling and the user just taps, many browsers also fire a mousedown/mouseup sequence AFTER the touchstart/touchend sequence, thus it'd result in two quick "click" events being dispatched. This line senses that condition and halts it on the subsequent mousedown. + isPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchstart, pointerdown, mousedown. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary. + + return; + } + + interrupted = isTweening(); + dragged = false; // we need to track whether or not it was dragged in this interaction so that if, for example, the user calls .endDrag() to FORCE it to stop and then they keep the mouse pressed down and eventually release, that would normally cause an onClick but we have to skip it in that case if there was dragging that occurred. + + self.pointerEvent = e; + + if (_touchEventLookup[e.type]) { + //note: on iOS, BOTH touchmove and mousemove are dispatched, but the mousemove has pageY and pageX of 0 which would mess up the calculations and needlessly hurt performance. + touchEventTarget = ~e.type.indexOf("touch") ? e.currentTarget || e.target : ownerDoc; //pointer-based touches (for Microsoft browsers) don't remain locked to the original target like other browsers, so we must use the document instead. The event type would be "MSPointerDown" or "pointerdown". + + _addListener(touchEventTarget, "touchend", onRelease); + + _addListener(touchEventTarget, "touchmove", onMove); // possible future change if PointerEvents are more standardized: https://developer.mozilla.org/en-US/docs/Web/API/Element/setPointerCapture + + + _addListener(touchEventTarget, "touchcancel", onRelease); + + _addListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + touchEventTarget = null; + + _addListener(ownerDoc, "mousemove", onMove); //attach these to the document instead of the box itself so that if the user's mouse moves too quickly (and off of the box), things still work. + + } + + touchDragAxis = null; + + if (!_supportsPointer || !touchEventTarget) { + _addListener(ownerDoc, "mouseup", onRelease); + + e && e.target && _addListener(e.target, "mouseup", onRelease); //we also have to listen directly on the element because some browsers don't bubble up the event to the _doc on elements with contentEditable="true" + } + + isClicking = isClickable.call(self, e.target) && vars.dragClickables === false && !force; + + if (isClicking) { + _addListener(e.target, "change", onRelease); //in some browsers, when you mousedown on a <select> element, no mouseup gets dispatched! So we listen for a "change" event instead. + + + _dispatchEvent(self, "pressInit", "onPressInit"); + + _dispatchEvent(self, "press", "onPress"); + + _setSelectable(triggers, true); //accommodates things like inputs and elements with contentEditable="true" (otherwise user couldn't drag to select text) + + + isPreventingDefault = false; + return; + } + + allowNativeTouchScrolling = !touchEventTarget || allowX === allowY || self.vars.allowNativeTouchScrolling === false || self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2) ? false : allowX ? "y" : "x"; //note: in Chrome, right-clicking (for a context menu) fires onPress and it doesn't have the event.which set properly, so we must look for event.ctrlKey. If the user wants to allow context menus we should of course sense it here and not allow native touch scrolling. + + isPreventingDefault = !allowNativeTouchScrolling && !self.allowEventDefault; + + if (isPreventingDefault) { + _preventDefault(e); + + _addListener(_win, "touchforcechange", _preventDefault); //works around safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/ + + } + + if (e.changedTouches) { + //touch events store the data slightly differently + e = touch = e.changedTouches[0]; + touchID = e.identifier; + } else if (e.pointerId) { + touchID = e.pointerId; //for some Microsoft browsers + } else { + touch = touchID = null; + } + + _dragCount++; + + _addToRenderQueue(render); //causes the Draggable to render on each "tick" of gsap.ticker (performance optimization - updating values in a mousemove can cause them to happen too frequently, like multiple times between frame redraws which is wasteful, and it also prevents values from updating properly in IE8) + + + startPointerY = self.pointerY = e.pageY; //record the starting x and y so that we can calculate the movement from the original in _onMouseMove + + startPointerX = self.pointerX = e.pageX; + + _dispatchEvent(self, "pressInit", "onPressInit"); + + if (allowNativeTouchScrolling || self.autoScroll) { + _recordMaxScrolls(target.parentNode); + } + + if (target.parentNode && self.autoScroll && !scrollProxy && !rotationMode && target.parentNode._gsMaxScrollX && !_placeholderDiv.parentNode && !target.getBBox) { + //add a placeholder div to prevent the parent container from collapsing when the user drags the element left. + _placeholderDiv.style.width = target.parentNode.scrollWidth + "px"; + target.parentNode.appendChild(_placeholderDiv); + } + + recordStartPositions(); + self.tween && self.tween.kill(); + self.isThrowing = false; + gsap.killTweensOf(scrollProxy || target, killProps, true); //in case the user tries to drag it before the last tween is done. + + scrollProxy && gsap.killTweensOf(target, { + scrollTo: 1 + }, true); //just in case the original target's scroll position is being tweened somewhere else. + + self.tween = self.lockedAxis = null; + + if (vars.zIndexBoost || !rotationMode && !scrollProxy && vars.zIndexBoost !== false) { + target.style.zIndex = Draggable.zIndex++; + } + + self.isPressed = true; + hasDragCallback = !!(vars.onDrag || self._listeners.drag); + hasMoveCallback = !!(vars.onMove || self._listeners.move); + + if (vars.cursor !== false || vars.activeCursor) { + i = triggers.length; + + while (--i > -1) { + gsap.set(triggers[i], { + cursor: vars.activeCursor || vars.cursor || (_defaultCursor === "grab" ? "grabbing" : _defaultCursor) + }); + } + } + + _dispatchEvent(self, "press", "onPress"); + }, + //called every time the mouse/touch moves + onMove = function onMove(e) { + var originalEvent = e, + touches, + pointerX, + pointerY, + i, + dx, + dy; + + if (!enabled || _isMultiTouching || !self.isPressed || !e) { + isPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchmove, pointermove, mousemove. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary. + + return; + } + + self.pointerEvent = e; + touches = e.changedTouches; + + if (touches) { + //touch events store the data slightly differently + e = touches[0]; + + if (e !== touch && e.identifier !== touchID) { + //Usually changedTouches[0] will be what we're looking for, but in case it's not, look through the rest of the array...(and Android browsers don't reuse the event like iOS) + i = touches.length; + + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} // Some Android devices dispatch a touchstart AND pointerdown initially, and then only pointermove thus the touchID may not match because it was grabbed from the touchstart event whereas the pointer event is the one that the browser dispatches for move, so if the event target matches this Draggable's target, let it through. + + + if (i < 0) { + return; + } + } + } else if (e.pointerId && touchID && e.pointerId !== touchID) { + //for some Microsoft browsers, we must attach the listener to the doc rather than the trigger so that when the finger moves outside the bounds of the trigger, things still work. So if the event we're receiving has a pointerId that doesn't match the touchID, ignore it (for multi-touch) + return; + } + + if (touchEventTarget && allowNativeTouchScrolling && !touchDragAxis) { + //Android browsers force us to decide on the first "touchmove" event if we should allow the default (scrolling) behavior or preventDefault(). Otherwise, a "touchcancel" will be fired and then no "touchmove" or "touchend" will fire during the scrolling (no good). + _point1.x = e.pageX - (isFixed ? _getDocScrollLeft(ownerDoc) : 0); + _point1.y = e.pageY - (isFixed ? _getDocScrollTop(ownerDoc) : 0); + matrix && matrix.apply(_point1, _point1); + pointerX = _point1.x; + pointerY = _point1.y; + dx = Math.abs(pointerX - startPointerX); + dy = Math.abs(pointerY - startPointerY); + + if (dx !== dy && (dx > minimumMovement || dy > minimumMovement) || _isAndroid && allowNativeTouchScrolling === touchDragAxis) { + touchDragAxis = dx > dy && allowX ? "x" : "y"; + + if (allowNativeTouchScrolling && touchDragAxis !== allowNativeTouchScrolling) { + _addListener(_win, "touchforcechange", _preventDefault); // prevents native touch scrolling from taking over if the user started dragging in the other direction in iOS Safari + + } + + if (self.vars.lockAxisOnTouchScroll !== false && allowX && allowY) { + self.lockedAxis = touchDragAxis === "x" ? "y" : "x"; + _isFunction(self.vars.onLockAxis) && self.vars.onLockAxis.call(self, originalEvent); + } + + if (_isAndroid && allowNativeTouchScrolling === touchDragAxis) { + onRelease(originalEvent); + return; + } + } + } + + if (!self.allowEventDefault && (!allowNativeTouchScrolling || touchDragAxis && allowNativeTouchScrolling !== touchDragAxis) && originalEvent.cancelable !== false) { + _preventDefault(originalEvent); + + isPreventingDefault = true; + } else if (isPreventingDefault) { + isPreventingDefault = false; + } + + if (self.autoScroll) { + checkAutoScrollBounds = true; + } + + setPointerPosition(e.pageX, e.pageY, hasMoveCallback); + }, + setPointerPosition = function setPointerPosition(pointerX, pointerY, invokeOnMove) { + var dragTolerance = 1 - self.dragResistance, + edgeTolerance = 1 - self.edgeResistance, + prevPointerX = self.pointerX, + prevPointerY = self.pointerY, + prevStartElementY = startElementY, + prevX = self.x, + prevY = self.y, + prevEndX = self.endX, + prevEndY = self.endY, + prevEndRotation = self.endRotation, + prevDirty = dirty, + xChange, + yChange, + x, + y, + dif, + temp; + self.pointerX = pointerX; + self.pointerY = pointerY; + + if (isFixed) { + pointerX -= _getDocScrollLeft(ownerDoc); + pointerY -= _getDocScrollTop(ownerDoc); + } + + if (rotationMode) { + y = Math.atan2(rotationOrigin.y - pointerY, pointerX - rotationOrigin.x) * _RAD2DEG; + dif = self.y - y; + + if (dif > 180) { + startElementY -= 360; + self.y = y; + } else if (dif < -180) { + startElementY += 360; + self.y = y; + } + + if (self.x !== startElementX || Math.max(Math.abs(startPointerX - pointerX), Math.abs(startPointerY - pointerY)) > minimumMovement) { + self.y = y; + x = startElementX + (startElementY - y) * dragTolerance; + } else { + x = startElementX; + } + } else { + if (matrix) { + temp = pointerX * matrix.a + pointerY * matrix.c + matrix.e; + pointerY = pointerX * matrix.b + pointerY * matrix.d + matrix.f; + pointerX = temp; + } + + yChange = pointerY - startPointerY; + xChange = pointerX - startPointerX; + + if (yChange < minimumMovement && yChange > -minimumMovement) { + yChange = 0; + } + + if (xChange < minimumMovement && xChange > -minimumMovement) { + xChange = 0; + } + + if ((self.lockAxis || self.lockedAxis) && (xChange || yChange)) { + temp = self.lockedAxis; + + if (!temp) { + self.lockedAxis = temp = allowX && Math.abs(xChange) > Math.abs(yChange) ? "y" : allowY ? "x" : null; + + if (temp && _isFunction(self.vars.onLockAxis)) { + self.vars.onLockAxis.call(self, self.pointerEvent); + } + } + + if (temp === "y") { + yChange = 0; + } else if (temp === "x") { + xChange = 0; + } + } + + x = _round(startElementX + xChange * dragTolerance); + y = _round(startElementY + yChange * dragTolerance); + } + + if ((snapX || snapY || snapXY) && (self.x !== x || self.y !== y && !rotationMode)) { + if (snapXY) { + _temp1.x = x; + _temp1.y = y; + temp = snapXY(_temp1); + x = _round(temp.x); + y = _round(temp.y); + } + + if (snapX) { + x = _round(snapX(x)); + } + + if (snapY) { + y = _round(snapY(y)); + } + } + + if (hasBounds) { + if (x > maxX) { + x = maxX + Math.round((x - maxX) * edgeTolerance); + } else if (x < minX) { + x = minX + Math.round((x - minX) * edgeTolerance); + } + + if (!rotationMode) { + if (y > maxY) { + y = Math.round(maxY + (y - maxY) * edgeTolerance); + } else if (y < minY) { + y = Math.round(minY + (y - minY) * edgeTolerance); + } + } + } + + if (self.x !== x || self.y !== y && !rotationMode) { + if (rotationMode) { + self.endRotation = self.x = self.endX = x; + dirty = true; + } else { + if (allowY) { + self.y = self.endY = y; + dirty = true; //a flag that indicates we need to render the target next time the TweenLite.ticker dispatches a "tick" event (typically on a requestAnimationFrame) - this is a performance optimization (we shouldn't render on every move because sometimes many move events can get dispatched between screen refreshes, and that'd be wasteful to render every time) + } + + if (allowX) { + self.x = self.endX = x; + dirty = true; + } + } + + if (!invokeOnMove || _dispatchEvent(self, "move", "onMove") !== false) { + if (!self.isDragging && self.isPressed) { + self.isDragging = dragged = true; + + _dispatchEvent(self, "dragstart", "onDragStart"); + } + } else { + //revert because the onMove returned false! + self.pointerX = prevPointerX; + self.pointerY = prevPointerY; + startElementY = prevStartElementY; + self.x = prevX; + self.y = prevY; + self.endX = prevEndX; + self.endY = prevEndY; + self.endRotation = prevEndRotation; + dirty = prevDirty; + } + } + }, + //called when the mouse/touch is released + onRelease = function onRelease(e, force) { + if (!enabled || !self.isPressed || e && touchID != null && !force && (e.pointerId && e.pointerId !== touchID && e.target !== target || e.changedTouches && !_hasTouchID(e.changedTouches, touchID))) { + //for some Microsoft browsers, we must attach the listener to the doc rather than the trigger so that when the finger moves outside the bounds of the trigger, things still work. So if the event we're receiving has a pointerId that doesn't match the touchID, ignore it (for multi-touch) + isPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchend, pointerup, mouseup. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary. + + return; + } + + self.isPressed = false; + var originalEvent = e, + wasDragging = self.isDragging, + isContextMenuRelease = self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2), + placeholderDelayedCall = gsap.delayedCall(0.001, removePlaceholder), + touches, + i, + syntheticEvent, + eventTarget, + syntheticClick; + + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchend", onRelease); + + _removeListener(touchEventTarget, "touchmove", onMove); + + _removeListener(touchEventTarget, "touchcancel", onRelease); + + _removeListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + _removeListener(ownerDoc, "mousemove", onMove); + } + + _removeListener(_win, "touchforcechange", _preventDefault); + + if (!_supportsPointer || !touchEventTarget) { + _removeListener(ownerDoc, "mouseup", onRelease); + + e && e.target && _removeListener(e.target, "mouseup", onRelease); + } + + dirty = false; + + if (wasDragging) { + dragEndTime = _lastDragTime = _getTime(); + self.isDragging = false; + } + + _removeFromRenderQueue(render); + + if (isClicking && !isContextMenuRelease) { + if (e) { + _removeListener(e.target, "change", onRelease); + + self.pointerEvent = originalEvent; + } + + _setSelectable(triggers, false); + + _dispatchEvent(self, "release", "onRelease"); + + _dispatchEvent(self, "click", "onClick"); + + isClicking = false; + return; + } + + i = triggers.length; + + while (--i > -1) { + _setStyle(triggers[i], "cursor", vars.cursor || (vars.cursor !== false ? _defaultCursor : null)); + } + + _dragCount--; + + if (e) { + touches = e.changedTouches; + + if (touches) { + //touch events store the data slightly differently + e = touches[0]; + + if (e !== touch && e.identifier !== touchID) { + //Usually changedTouches[0] will be what we're looking for, but in case it's not, look through the rest of the array...(and Android browsers don't reuse the event like iOS) + i = touches.length; + + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} + + if (i < 0 && !force) { + return; + } + } + } + + self.pointerEvent = originalEvent; + self.pointerX = e.pageX; + self.pointerY = e.pageY; + } + + if (isContextMenuRelease && originalEvent) { + _preventDefault(originalEvent); + + isPreventingDefault = true; + + _dispatchEvent(self, "release", "onRelease"); + } else if (originalEvent && !wasDragging) { + isPreventingDefault = false; + + if (interrupted && (vars.snap || vars.bounds)) { + //otherwise, if the user clicks on the object while it's animating to a snapped position, and then releases without moving 3 pixels, it will just stay there (it should animate/snap) + animate(vars.inertia || vars.throwProps); + } + + _dispatchEvent(self, "release", "onRelease"); + + if ((!_isAndroid || originalEvent.type !== "touchmove") && originalEvent.type.indexOf("cancel") === -1) { + //to accommodate native scrolling on Android devices, we have to immediately call onRelease() on the first touchmove event, but that shouldn't trigger a "click". + _dispatchEvent(self, "click", "onClick"); + + if (_getTime() - clickTime < 300) { + _dispatchEvent(self, "doubleclick", "onDoubleClick"); + } + + eventTarget = originalEvent.target || target; //old IE uses srcElement + + clickTime = _getTime(); + + syntheticClick = function syntheticClick() { + // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular "click" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the "real"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the "real" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched. + if (clickTime !== clickDispatch && self.enabled() && !self.isPressed && !originalEvent.defaultPrevented) { + if (eventTarget.click) { + //some browsers (like mobile Safari) don't properly trigger the click event + eventTarget.click(); + } else if (ownerDoc.createEvent) { + syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win, 1, self.pointerEvent.screenX, self.pointerEvent.screenY, self.pointerX, self.pointerY, false, false, false, false, 0, null); + eventTarget.dispatchEvent(syntheticEvent); + } + } + }; + + if (!_isAndroid && !originalEvent.defaultPrevented) { + //iOS Safari requires the synthetic click to happen immediately or else it simply won't work, but Android doesn't play nice. + gsap.delayedCall(0.05, syntheticClick); //in addition to the iOS bug workaround, there's a Firefox issue with clicking on things like a video to play, so we must fake a click event in a slightly delayed fashion. Previously, we listened for the "click" event with "capture" false which solved the video-click-to-play issue, but it would allow the "click" event to be dispatched twice like if you were using a jQuery.click() because that was handled in the capture phase, thus we had to switch to the capture phase to avoid the double-dispatching, but do the delayed synthetic click. Don't fire it too fast (like 0.00001) because we want to give the native event a chance to fire first as it's "trusted". + } + } + } else { + animate(vars.inertia || vars.throwProps); //will skip if inertia/throwProps isn't defined or InertiaPlugin isn't loaded. + + if (!self.allowEventDefault && originalEvent && (vars.dragClickables !== false || !isClickable.call(self, originalEvent.target)) && wasDragging && (!allowNativeTouchScrolling || touchDragAxis && allowNativeTouchScrolling === touchDragAxis) && originalEvent.cancelable !== false) { + isPreventingDefault = true; + + _preventDefault(originalEvent); + } else { + isPreventingDefault = false; + } + + _dispatchEvent(self, "release", "onRelease"); + } + + isTweening() && placeholderDelayedCall.duration(self.tween.duration()); //sync the timing so that the placeholder DIV gets + + wasDragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return true; + }, + updateScroll = function updateScroll(e) { + if (e && self.isDragging && !scrollProxy) { + var parent = e.target || target.parentNode, + deltaX = parent.scrollLeft - parent._gsScrollX, + deltaY = parent.scrollTop - parent._gsScrollY; + + if (deltaX || deltaY) { + if (matrix) { + startPointerX -= deltaX * matrix.a + deltaY * matrix.c; + startPointerY -= deltaY * matrix.d + deltaX * matrix.b; + } else { + startPointerX -= deltaX; + startPointerY -= deltaY; + } + + parent._gsScrollX += deltaX; + parent._gsScrollY += deltaY; + setPointerPosition(self.pointerX, self.pointerY); + } + } + }, + onClick = function onClick(e) { + //this was a huge pain in the neck to align all the various browsers and their behaviors. Chrome, Firefox, Safari, Opera, Android, and Microsoft Edge all handle events differently! Some will only trigger native behavior (like checkbox toggling) from trusted events. Others don't even support isTrusted, but require 2 events to flow through before triggering native behavior. Edge treats everything as trusted but also mandates that 2 flow through to trigger the correct native behavior. + var time = _getTime(), + recentlyClicked = time - clickTime < 100, + recentlyDragged = time - dragEndTime < 50, + alreadyDispatched = recentlyClicked && clickDispatch === clickTime, + defaultPrevented = self.pointerEvent && self.pointerEvent.defaultPrevented, + alreadyDispatchedTrusted = recentlyClicked && trustedClickDispatch === clickTime, + trusted = e.isTrusted || e.isTrusted == null && recentlyClicked && alreadyDispatched; //note: Safari doesn't support isTrusted, and it won't properly execute native behavior (like toggling checkboxes) on the first synthetic "click" event - we must wait for the 2nd and treat it as trusted (but stop propagation at that point). Confusing, I know. Don't you love cross-browser compatibility challenges? + + + if ((alreadyDispatched || recentlyDragged && self.vars.suppressClickOnDrag !== false) && e.stopImmediatePropagation) { + e.stopImmediatePropagation(); + } + + if (recentlyClicked && !(self.pointerEvent && self.pointerEvent.defaultPrevented) && (!alreadyDispatched || trusted && !alreadyDispatchedTrusted)) { + //let the first click pass through unhindered. Let the next one only if it's trusted, then no more (stop quick-succession ones) + if (trusted && alreadyDispatched) { + trustedClickDispatch = clickTime; + } + + clickDispatch = clickTime; + return; + } + + if (self.isPressed || recentlyDragged || recentlyClicked) { + if (!trusted || !e.detail || !recentlyClicked || defaultPrevented) { + _preventDefault(e); + } + } + + if (!recentlyClicked && !recentlyDragged && !dragged) { + // for script-triggered event dispatches, like element.click() + e && e.target && (self.pointerEvent = e); + + _dispatchEvent(self, "click", "onClick"); + } + }, + localizePoint = function localizePoint(p) { + return matrix ? { + x: p.x * matrix.a + p.y * matrix.c + matrix.e, + y: p.x * matrix.b + p.y * matrix.d + matrix.f + } : { + x: p.x, + y: p.y + }; + }; + + old = Draggable.get(target); + old && old.kill(); // avoids duplicates (an element can only be controlled by one Draggable) + //give the user access to start/stop dragging... + + _this2.startDrag = function (event, align) { + var r1, r2, p1, p2; + onPress(event || self.pointerEvent, true); //if the pointer isn't on top of the element, adjust things accordingly + + if (align && !self.hitTest(event || self.pointerEvent)) { + r1 = _parseRect(event || self.pointerEvent); + r2 = _parseRect(target); + p1 = localizePoint({ + x: r1.left + r1.width / 2, + y: r1.top + r1.height / 2 + }); + p2 = localizePoint({ + x: r2.left + r2.width / 2, + y: r2.top + r2.height / 2 + }); + startPointerX -= p1.x - p2.x; + startPointerY -= p1.y - p2.y; + } + + if (!self.isDragging) { + self.isDragging = dragged = true; + + _dispatchEvent(self, "dragstart", "onDragStart"); + } + }; + + _this2.drag = onMove; + + _this2.endDrag = function (e) { + return onRelease(e || self.pointerEvent, true); + }; + + _this2.timeSinceDrag = function () { + return self.isDragging ? 0 : (_getTime() - dragEndTime) / 1000; + }; + + _this2.timeSinceClick = function () { + return (_getTime() - clickTime) / 1000; + }; + + _this2.hitTest = function (target, threshold) { + return Draggable.hitTest(self.target, target, threshold); + }; + + _this2.getDirection = function (from, diagonalThreshold) { + //from can be "start" (default), "velocity", or an element + var mode = from === "velocity" && InertiaPlugin ? from : _isObject(from) && !rotationMode ? "element" : "start", + xChange, + yChange, + ratio, + direction, + r1, + r2; + + if (mode === "element") { + r1 = _parseRect(self.target); + r2 = _parseRect(from); + } + + xChange = mode === "start" ? self.x - startElementX : mode === "velocity" ? InertiaPlugin.getVelocity(target, xProp) : r1.left + r1.width / 2 - (r2.left + r2.width / 2); + + if (rotationMode) { + return xChange < 0 ? "counter-clockwise" : "clockwise"; + } else { + diagonalThreshold = diagonalThreshold || 2; + yChange = mode === "start" ? self.y - startElementY : mode === "velocity" ? InertiaPlugin.getVelocity(target, yProp) : r1.top + r1.height / 2 - (r2.top + r2.height / 2); + ratio = Math.abs(xChange / yChange); + direction = ratio < 1 / diagonalThreshold ? "" : xChange < 0 ? "left" : "right"; + + if (ratio < diagonalThreshold) { + if (direction !== "") { + direction += "-"; + } + + direction += yChange < 0 ? "up" : "down"; + } + } + + return direction; + }; + + _this2.applyBounds = function (newBounds, sticky) { + var x, y, forceZeroVelocity, e, parent, isRoot; + + if (newBounds && vars.bounds !== newBounds) { + vars.bounds = newBounds; + return self.update(true, sticky); + } + + syncXY(true); + calculateBounds(); + + if (hasBounds && !isTweening()) { + x = self.x; + y = self.y; + + if (x > maxX) { + x = maxX; + } else if (x < minX) { + x = minX; + } + + if (y > maxY) { + y = maxY; + } else if (y < minY) { + y = minY; + } + + if (self.x !== x || self.y !== y) { + forceZeroVelocity = true; + self.x = self.endX = x; + + if (rotationMode) { + self.endRotation = x; + } else { + self.y = self.endY = y; + } + + dirty = true; + render(true); + + if (self.autoScroll && !self.isDragging) { + _recordMaxScrolls(target.parentNode); + + e = target; + _windowProxy.scrollTop = _win.pageYOffset != null ? _win.pageYOffset : ownerDoc.documentElement.scrollTop != null ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop; + _windowProxy.scrollLeft = _win.pageXOffset != null ? _win.pageXOffset : ownerDoc.documentElement.scrollLeft != null ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft; + + while (e && !isRoot) { + //walk up the chain and sense wherever the scrollTop/scrollLeft exceeds the maximum. + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + + if (allowY && parent.scrollTop > parent._gsMaxScrollY) { + parent.scrollTop = parent._gsMaxScrollY; + } + + if (allowX && parent.scrollLeft > parent._gsMaxScrollX) { + parent.scrollLeft = parent._gsMaxScrollX; + } + + e = parent; + } + } + } + + if (self.isThrowing && (forceZeroVelocity || self.endX > maxX || self.endX < minX || self.endY > maxY || self.endY < minY)) { + animate(vars.inertia || vars.throwProps, forceZeroVelocity); + } + } + + return self; + }; + + _this2.update = function (applyBounds, sticky, ignoreExternalChanges) { + if (sticky && self.isPressed) { + // in case the element was repositioned in the document flow, thus its x/y may be identical but its position is actually quite different. + var m = getGlobalMatrix(target), + p = innerMatrix.apply({ + x: self.x - startElementX, + y: self.y - startElementY + }), + m2 = getGlobalMatrix(target.parentNode, true); + m2.apply({ + x: m.e - p.x, + y: m.f - p.y + }, p); + self.x -= p.x - m2.e; + self.y -= p.y - m2.f; + render(true); + recordStartPositions(); + } + + var x = self.x, + y = self.y; + updateMatrix(!sticky); + + if (applyBounds) { + self.applyBounds(); + } else { + dirty && ignoreExternalChanges && render(true); + syncXY(true); + } + + if (sticky) { + setPointerPosition(self.pointerX, self.pointerY); + dirty && render(true); + } + + if (self.isPressed && !sticky && (allowX && Math.abs(x - self.x) > 0.01 || allowY && Math.abs(y - self.y) > 0.01 && !rotationMode)) { + recordStartPositions(); + } + + if (self.autoScroll) { + _recordMaxScrolls(target.parentNode, self.isDragging); + + checkAutoScrollBounds = self.isDragging; + render(true); //in case reparenting occurred. + + _removeScrollListener(target, updateScroll); + + _addScrollListener(target, updateScroll); + } + + return self; + }; + + _this2.enable = function (type) { + var setVars = { + lazy: true + }, + id, + i, + trigger; + + if (vars.cursor !== false) { + setVars.cursor = vars.cursor || _defaultCursor; + } + + if (gsap.utils.checkPrefix("touchCallout")) { + setVars.touchCallout = "none"; + } + + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, allowX === allowY ? "none" : vars.allowNativeTouchScrolling && target.scrollHeight === target.clientHeight === (target.scrollWidth === target.clientHeight) || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x"); // Some browsers like Internet Explorer will fire a pointercancel event when the user attempts to drag when touchAction is "manipulate" because it's perceived as a pan. If the element has scrollable content in only one direction, we should use pan-x or pan-y accordingly so that the pointercancel doesn't prevent dragging. + + + i = triggers.length; + + while (--i > -1) { + trigger = triggers[i]; + _supportsPointer || _addListener(trigger, "mousedown", onPress); + + _addListener(trigger, "touchstart", onPress); + + _addListener(trigger, "click", onClick, true); // note: used to pass true for capture but it prevented click-to-play-video functionality in Firefox. + + + gsap.set(trigger, setVars); + + if (trigger.getBBox && trigger.ownerSVGElement && allowX !== allowY) { + // a bug in chrome doesn't respect touch-action on SVG elements - it only works if we set it on the parent SVG. + gsap.set(trigger.ownerSVGElement, { + touchAction: vars.allowNativeTouchScrolling || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x" + }); + } + + vars.allowContextMenu || _addListener(trigger, "contextmenu", onContextMenu); + } + + _setSelectable(triggers, false); + } + + _addScrollListener(target, updateScroll); + + enabled = true; + + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.track(scrollProxy || target, xyMode ? "x,y" : rotationMode ? "rotation" : "top,left"); + } + + target._gsDragID = id = target._gsDragID || "d" + _lookupCount++; + _lookup[id] = self; + + if (scrollProxy) { + scrollProxy.enable(); + scrollProxy.element._gsDragID = id; + } + + (vars.bounds || rotationMode) && recordStartPositions(); + vars.bounds && self.applyBounds(); + return self; + }; + + _this2.disable = function (type) { + var dragging = self.isDragging, + i = triggers.length, + trigger; + + while (--i > -1) { + _setStyle(triggers[i], "cursor", null); + } + + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, null); + + i = triggers.length; + + while (--i > -1) { + trigger = triggers[i]; + + _setStyle(trigger, "touchCallout", null); + + _removeListener(trigger, "mousedown", onPress); + + _removeListener(trigger, "touchstart", onPress); + + _removeListener(trigger, "click", onClick, true); + + _removeListener(trigger, "contextmenu", onContextMenu); + } + + _setSelectable(triggers, true); + + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchcancel", onRelease); + + _removeListener(touchEventTarget, "touchend", onRelease); + + _removeListener(touchEventTarget, "touchmove", onMove); + } + + _removeListener(ownerDoc, "mouseup", onRelease); + + _removeListener(ownerDoc, "mousemove", onMove); + } + + _removeScrollListener(target, updateScroll); + + enabled = false; + + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.untrack(scrollProxy || target, xyMode ? "x,y" : rotationMode ? "rotation" : "top,left"); + self.tween && self.tween.kill(); + } + + scrollProxy && scrollProxy.disable(); + + _removeFromRenderQueue(render); + + self.isDragging = self.isPressed = isClicking = false; + dragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return self; + }; + + _this2.enabled = function (value, type) { + return arguments.length ? value ? self.enable(type) : self.disable(type) : enabled; + }; + + _this2.kill = function () { + self.isThrowing = false; + self.tween && self.tween.kill(); + self.disable(); + gsap.set(triggers, { + clearProps: "userSelect" + }); + delete _lookup[target._gsDragID]; + return self; + }; + + _this2.revert = function () { + this.kill(); + this.styles && this.styles.revert(); + }; + + if (~type.indexOf("scroll")) { + scrollProxy = _this2.scrollProxy = new ScrollProxy(target, _extend({ + onKill: function onKill() { + //ScrollProxy's onKill() gets called if/when the ScrollProxy senses that the user interacted with the scroll position manually (like using the scrollbar). IE9 doesn't fire the "mouseup" properly when users drag the scrollbar of an element, so this works around that issue. + self.isPressed && onRelease(null); + } + }, vars)); //a bug in many Android devices' stock browser causes scrollTop to get forced back to 0 after it is altered via JS, so we set overflow to "hidden" on mobile/touch devices (they hide the scroll bar anyway). That works around the bug. (This bug is discussed at https://code.google.com/p/android/issues/detail?id=19625) + + target.style.overflowY = allowY && !_isTouchDevice ? "auto" : "hidden"; + target.style.overflowX = allowX && !_isTouchDevice ? "auto" : "hidden"; + target = scrollProxy.content; + } + + if (rotationMode) { + killProps.rotation = 1; + } else { + if (allowX) { + killProps[xProp] = 1; + } + + if (allowY) { + killProps[yProp] = 1; + } + } + + gsCache.force3D = "force3D" in vars ? vars.force3D : true; //otherwise, normal dragging would be in 2D and then as soon as it's released and there's an inertia tween, it'd jump to 3D which can create an initial jump due to the work the browser must to do layerize it. + + _context(_assertThisInitialized(_this2)); + + _this2.enable(); + + return _this2; + } + + Draggable.register = function register(core) { + gsap = core; + + _initCore(); + }; + + Draggable.create = function create(targets, vars) { + _coreInitted || _initCore(true); + return _toArray(targets).map(function (target) { + return new Draggable(target, vars); + }); + }; + + Draggable.get = function get(target) { + return _lookup[(_toArray(target)[0] || {})._gsDragID]; + }; + + Draggable.timeSinceDrag = function timeSinceDrag() { + return (_getTime() - _lastDragTime) / 1000; + }; + + Draggable.hitTest = function hitTest(obj1, obj2, threshold) { + if (obj1 === obj2) { + return false; + } + + var r1 = _parseRect(obj1), + r2 = _parseRect(obj2), + top = r1.top, + left = r1.left, + right = r1.right, + bottom = r1.bottom, + width = r1.width, + height = r1.height, + isOutside = r2.left > right || r2.right < left || r2.top > bottom || r2.bottom < top, + overlap, + area, + isRatio; + + if (isOutside || !threshold) { + return !isOutside; + } + + isRatio = (threshold + "").indexOf("%") !== -1; + threshold = parseFloat(threshold) || 0; + overlap = { + left: Math.max(left, r2.left), + top: Math.max(top, r2.top) + }; + overlap.width = Math.min(right, r2.right) - overlap.left; + overlap.height = Math.min(bottom, r2.bottom) - overlap.top; + + if (overlap.width < 0 || overlap.height < 0) { + return false; + } + + if (isRatio) { + threshold *= 0.01; + area = overlap.width * overlap.height; + return area >= width * height * threshold || area >= r2.width * r2.height * threshold; + } + + return overlap.width > threshold && overlap.height > threshold; + }; + + return Draggable; +}(EventDispatcher); + +_setDefaults(Draggable.prototype, { + pointerX: 0, + pointerY: 0, + startX: 0, + startY: 0, + deltaX: 0, + deltaY: 0, + isDragging: false, + isPressed: false +}); + +Draggable.zIndex = 1000; +Draggable.version = "3.12.7"; +_getGSAP() && gsap.registerPlugin(Draggable); +export { Draggable as default }; \ No newline at end of file diff --git a/node_modules/gsap/EasePack.js b/node_modules/gsap/EasePack.js new file mode 100644 index 0000000000000000000000000000000000000000..9a8771485d24d3e3b3a72b793739d904f4dad03a --- /dev/null +++ b/node_modules/gsap/EasePack.js @@ -0,0 +1,212 @@ +/*! + * EasePack 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var gsap, + _coreInitted, + _registerEase, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _boolean = function _boolean(value, defaultValue) { + return !!(typeof value === "undefined" ? defaultValue : value && !~(value + "").indexOf("false")); +}, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (gsap) { + _registerEase = gsap.registerEase; //add weighted ease capabilities to standard eases so users can do "power2.inOut(0.8)" for example to push everything toward the "out", or (-0.8) to push it toward the "in" (0 is neutral) + + var eases = gsap.parseEase(), + createConfig = function createConfig(ease) { + return function (ratio) { + var y = 0.5 + ratio / 2; + + ease.config = function (p) { + return ease(2 * (1 - p) * p * y + p * p); + }; + }; + }, + p; + + for (p in eases) { + if (!eases[p].config) { + createConfig(eases[p]); + } + } + + _registerEase("slow", SlowMo); + + _registerEase("expoScale", ExpoScaleEase); + + _registerEase("rough", RoughEase); + + for (p in EasePack) { + p !== "version" && gsap.core.globals(p, EasePack[p]); + } + + _coreInitted = 1; + } +}, + _createSlowMo = function _createSlowMo(linearRatio, power, yoyoMode) { + linearRatio = Math.min(1, linearRatio || 0.7); + + var pow = linearRatio < 1 ? power || power === 0 ? power : 0.7 : 0, + p1 = (1 - linearRatio) / 2, + p3 = p1 + linearRatio, + calcEnd = _boolean(yoyoMode); + + return function (p) { + var r = p + (0.5 - p) * pow; + return p < p1 ? calcEnd ? 1 - (p = 1 - p / p1) * p : r - (p = 1 - p / p1) * p * p * p * r : p > p3 ? calcEnd ? p === 1 ? 0 : 1 - (p = (p - p3) / p1) * p : r + (p - r) * (p = (p - p3) / p1) * p * p * p : calcEnd ? 1 : r; + }; +}, + _createExpoScale = function _createExpoScale(start, end, ease) { + var p1 = Math.log(end / start), + p2 = end - start; + ease && (ease = gsap.parseEase(ease)); + return function (p) { + return (start * Math.exp(p1 * (ease ? ease(p) : p)) - start) / p2; + }; +}, + EasePoint = function EasePoint(time, value, next) { + this.t = time; + this.v = value; + + if (next) { + this.next = next; + next.prev = this; + this.c = next.v - value; + this.gap = next.t - time; + } +}, + _createRoughEase = function _createRoughEase(vars) { + if (typeof vars !== "object") { + //users may pass in via a string, like "rough(30)" + vars = { + points: +vars || 20 + }; + } + + var taper = vars.taper || "none", + a = [], + cnt = 0, + points = (+vars.points || 20) | 0, + i = points, + randomize = _boolean(vars.randomize, true), + clamp = _boolean(vars.clamp), + template = gsap ? gsap.parseEase(vars.template) : 0, + strength = (+vars.strength || 1) * 0.4, + x, + y, + bump, + invX, + obj, + pnt, + recent; + + while (--i > -1) { + x = randomize ? Math.random() : 1 / points * i; + y = template ? template(x) : x; + + if (taper === "none") { + bump = strength; + } else if (taper === "out") { + invX = 1 - x; + bump = invX * invX * strength; + } else if (taper === "in") { + bump = x * x * strength; + } else if (x < 0.5) { + //"both" (start) + invX = x * 2; + bump = invX * invX * 0.5 * strength; + } else { + //"both" (end) + invX = (1 - x) * 2; + bump = invX * invX * 0.5 * strength; + } + + if (randomize) { + y += Math.random() * bump - bump * 0.5; + } else if (i % 2) { + y += bump * 0.5; + } else { + y -= bump * 0.5; + } + + if (clamp) { + if (y > 1) { + y = 1; + } else if (y < 0) { + y = 0; + } + } + + a[cnt++] = { + x: x, + y: y + }; + } + + a.sort(function (a, b) { + return a.x - b.x; + }); + pnt = new EasePoint(1, 1, null); + i = points; + + while (i--) { + obj = a[i]; + pnt = new EasePoint(obj.x, obj.y, pnt); + } + + recent = new EasePoint(0, 0, pnt.t ? pnt : pnt.next); + return function (p) { + var pnt = recent; + + if (p > pnt.t) { + while (pnt.next && p >= pnt.t) { + pnt = pnt.next; + } + + pnt = pnt.prev; + } else { + while (pnt.prev && p <= pnt.t) { + pnt = pnt.prev; + } + } + + recent = pnt; + return pnt.v + (p - pnt.t) / pnt.gap * pnt.c; + }; +}; + +export var SlowMo = _createSlowMo(0.7); +SlowMo.ease = SlowMo; //for backward compatibility + +SlowMo.config = _createSlowMo; +export var ExpoScaleEase = _createExpoScale(1, 2); +ExpoScaleEase.config = _createExpoScale; +export var RoughEase = _createRoughEase(); +RoughEase.ease = RoughEase; //for backward compatibility + +RoughEase.config = _createRoughEase; +export var EasePack = { + SlowMo: SlowMo, + RoughEase: RoughEase, + ExpoScaleEase: ExpoScaleEase +}; + +for (var p in EasePack) { + EasePack[p].register = _initCore; + EasePack[p].version = "3.12.7"; +} + +_getGSAP() && gsap.registerPlugin(SlowMo); +export { EasePack as default }; \ No newline at end of file diff --git a/node_modules/gsap/EaselPlugin.js b/node_modules/gsap/EaselPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..86217f3c1c96ad2e64b246a7c2f18fe32b9f5702 --- /dev/null +++ b/node_modules/gsap/EaselPlugin.js @@ -0,0 +1,341 @@ +/*! + * EaselPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var gsap, + _coreInitted, + _win, + _createJS, + _ColorFilter, + _ColorMatrixFilter, + _colorProps = "redMultiplier,greenMultiplier,blueMultiplier,alphaMultiplier,redOffset,greenOffset,blueOffset,alphaOffset".split(","), + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _getCreateJS = function _getCreateJS() { + return _createJS || _win && _win.createjs || _win || {}; +}, + _warn = function _warn(message) { + return console.warn(message); +}, + _cache = function _cache(target) { + var b = target.getBounds && target.getBounds(); + + if (!b) { + b = target.nominalBounds || { + x: 0, + y: 0, + width: 100, + height: 100 + }; + target.setBounds && target.setBounds(b.x, b.y, b.width, b.height); + } + + target.cache && target.cache(b.x, b.y, b.width, b.height); + + _warn("EaselPlugin: for filters to display in EaselJS, you must call the object's cache() method first. GSAP attempted to use the target's getBounds() for the cache but that may not be completely accurate. " + target); +}, + _parseColorFilter = function _parseColorFilter(target, v, plugin) { + if (!_ColorFilter) { + _ColorFilter = _getCreateJS().ColorFilter; + + if (!_ColorFilter) { + _warn("EaselPlugin error: The EaselJS ColorFilter JavaScript file wasn't loaded."); + } + } + + var filters = target.filters || [], + i = filters.length, + c, + s, + e, + a, + p, + pt; + + while (i--) { + if (filters[i] instanceof _ColorFilter) { + s = filters[i]; + break; + } + } + + if (!s) { + s = new _ColorFilter(); + filters.push(s); + target.filters = filters; + } + + e = s.clone(); + + if (v.tint != null) { + c = gsap.utils.splitColor(v.tint); + a = v.tintAmount != null ? +v.tintAmount : 1; + e.redOffset = +c[0] * a; + e.greenOffset = +c[1] * a; + e.blueOffset = +c[2] * a; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - a; + } else { + for (p in v) { + if (p !== "exposure") if (p !== "brightness") { + e[p] = +v[p]; + } + } + } + + if (v.exposure != null) { + e.redOffset = e.greenOffset = e.blueOffset = 255 * (+v.exposure - 1); + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1; + } else if (v.brightness != null) { + a = +v.brightness - 1; + e.redOffset = e.greenOffset = e.blueOffset = a > 0 ? a * 255 : 0; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - Math.abs(a); + } + + i = 8; + + while (i--) { + p = _colorProps[i]; + + if (s[p] !== e[p]) { + pt = plugin.add(s, p, s[p], e[p], 0, 0, 0, 0, 0, 1); + + if (pt) { + pt.op = "easel_colorFilter"; + } + } + } + + plugin._props.push("easel_colorFilter"); + + if (!target.cacheID) { + _cache(target); + } +}, + _idMatrix = [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + _applyMatrix = function _applyMatrix(m, m2) { + if (!(m instanceof Array) || !(m2 instanceof Array)) { + return m2; + } + + var temp = [], + i = 0, + z = 0, + y, + x; + + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = x === 4 ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i + 1] * m2[x + 5] + m[i + 2] * m2[x + 10] + m[i + 3] * m2[x + 15] + z; + } + + i += 5; + } + + return temp; +}, + _setSaturation = function _setSaturation(m, n) { + if (isNaN(n)) { + return m; + } + + var inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); +}, + _colorize = function _colorize(m, color, amount) { + if (isNaN(amount)) { + amount = 1; + } + + var c = gsap.utils.splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); +}, + _setHue = function _setHue(m, n) { + if (isNaN(n)) { + return m; + } + + n *= Math.PI / 180; + var c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([_lumR + c * (1 - _lumR) + s * -_lumR, _lumG + c * -_lumG + s * -_lumG, _lumB + c * -_lumB + s * (1 - _lumB), 0, 0, _lumR + c * -_lumR + s * 0.143, _lumG + c * (1 - _lumG) + s * 0.14, _lumB + c * -_lumB + s * -0.283, 0, 0, _lumR + c * -_lumR + s * -(1 - _lumR), _lumG + c * -_lumG + s * _lumG, _lumB + c * (1 - _lumB) + s * _lumB, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); +}, + _setContrast = function _setContrast(m, n) { + if (isNaN(n)) { + return m; + } + + n += 0.01; + return _applyMatrix([n, 0, 0, 0, 128 * (1 - n), 0, n, 0, 0, 128 * (1 - n), 0, 0, n, 0, 128 * (1 - n), 0, 0, 0, 1, 0], m); +}, + _parseColorMatrixFilter = function _parseColorMatrixFilter(target, v, plugin) { + if (!_ColorMatrixFilter) { + _ColorMatrixFilter = _getCreateJS().ColorMatrixFilter; + + if (!_ColorMatrixFilter) { + _warn("EaselPlugin: The EaselJS ColorMatrixFilter JavaScript file wasn't loaded."); + } + } + + var filters = target.filters || [], + i = filters.length, + matrix, + startMatrix, + s, + pg; + + while (--i > -1) { + if (filters[i] instanceof _ColorMatrixFilter) { + s = filters[i]; + break; + } + } + + if (!s) { + s = new _ColorMatrixFilter(_idMatrix.slice()); + filters.push(s); + target.filters = filters; + } + + startMatrix = s.matrix; + matrix = _idMatrix.slice(); + + if (v.colorize != null) { + matrix = _colorize(matrix, v.colorize, Number(v.colorizeAmount)); + } + + if (v.contrast != null) { + matrix = _setContrast(matrix, Number(v.contrast)); + } + + if (v.hue != null) { + matrix = _setHue(matrix, Number(v.hue)); + } + + if (v.saturation != null) { + matrix = _setSaturation(matrix, Number(v.saturation)); + } + + i = matrix.length; + + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg = plugin.add(startMatrix, i, startMatrix[i], matrix[i], 0, 0, 0, 0, 0, 1); + + if (pg) { + pg.op = "easel_colorMatrixFilter"; + } + } + } + + plugin._props.push("easel_colorMatrixFilter"); + + if (!target.cacheID) { + _cache(); + } + + plugin._matrix = startMatrix; +}, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (_windowExists()) { + _win = window; + } + + if (gsap) { + _coreInitted = 1; + } +}; + +export var EaselPlugin = { + version: "3.12.7", + name: "easel", + init: function init(target, value, tween, index, targets) { + if (!_coreInitted) { + _initCore(); + + if (!gsap) { + _warn("Please gsap.registerPlugin(EaselPlugin)"); + } + } + + this.target = target; + var p, pt, tint, colorMatrix, end, labels, i; + + for (p in value) { + end = value[p]; + + if (p === "colorFilter" || p === "tint" || p === "tintAmount" || p === "exposure" || p === "brightness") { + if (!tint) { + _parseColorFilter(target, value.colorFilter || value, this); + + tint = true; + } + } else if (p === "saturation" || p === "contrast" || p === "hue" || p === "colorize" || p === "colorizeAmount") { + if (!colorMatrix) { + _parseColorMatrixFilter(target, value.colorMatrixFilter || value, this); + + colorMatrix = true; + } + } else if (p === "frame") { + if (typeof end === "string" && end.charAt(1) !== "=" && (labels = target.labels)) { + for (i = 0; i < labels.length; i++) { + if (labels[i].label === end) { + end = labels[i].position; + } + } + } + + pt = this.add(target, "gotoAndStop", target.currentFrame, end, index, targets, Math.round, 0, 0, 1); + + if (pt) { + pt.op = p; + } + } else if (target[p] != null) { + this.add(target, p, "get", end); + } + } + }, + render: function render(ratio, data) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + if (data.target.cacheID) { + data.target.updateCache(); + } + }, + register: _initCore +}; + +EaselPlugin.registerCreateJS = function (createjs) { + _createJS = createjs; +}; + +_getGSAP() && gsap.registerPlugin(EaselPlugin); +export { EaselPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/Flip.js b/node_modules/gsap/Flip.js new file mode 100644 index 0000000000000000000000000000000000000000..3061cc237c2ac8a96b538b402e0cba1e27cc7f65 --- /dev/null +++ b/node_modules/gsap/Flip.js @@ -0,0 +1,1519 @@ +/*! + * Flip 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +import { getGlobalMatrix, _getDocScrollTop, _getDocScrollLeft, Matrix2D, _setDoc, _getCTM } from "./utils/matrix.js"; + +var _id = 1, + _toArray, + gsap, + _batch, + _batchAction, + _body, + _closestTenth, + _getStyleSaver, + _forEachBatch = function _forEachBatch(batch, name) { + return batch.actions.forEach(function (a) { + return a.vars[name] && a.vars[name](a); + }); +}, + _batchLookup = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _emptyObj = {}, + _dashedNameLookup = {}, + _memoizedRemoveProps = {}, + _listToArray = function _listToArray(list) { + return typeof list === "string" ? list.split(" ").join("").split(",") : list; +}, + // removes extra spaces contaminating the names, returns an Array. +_callbacks = _listToArray("onStart,onUpdate,onComplete,onReverseComplete,onInterrupt"), + _removeProps = _listToArray("transform,transformOrigin,width,height,position,top,left,opacity,zIndex,maxWidth,maxHeight,minWidth,minHeight"), + _getEl = function _getEl(target) { + return _toArray(target)[0] || console.warn("Element not found:", target); +}, + _round = function _round(value) { + return Math.round(value * 10000) / 10000 || 0; +}, + _toggleClass = function _toggleClass(targets, className, action) { + return targets.forEach(function (el) { + return el.classList[action](className); + }); +}, + _reserved = { + zIndex: 1, + kill: 1, + simple: 1, + spin: 1, + clearProps: 1, + targets: 1, + toggleClass: 1, + onComplete: 1, + onUpdate: 1, + onInterrupt: 1, + onStart: 1, + delay: 1, + repeat: 1, + repeatDelay: 1, + yoyo: 1, + scale: 1, + fade: 1, + absolute: 1, + props: 1, + onEnter: 1, + onLeave: 1, + custom: 1, + paused: 1, + nested: 1, + prune: 1, + absoluteOnLeave: 1 +}, + _fitReserved = { + zIndex: 1, + simple: 1, + clearProps: 1, + scale: 1, + absolute: 1, + fitChild: 1, + getVars: 1, + props: 1 +}, + _camelToDashed = function _camelToDashed(p) { + return p.replace(/([A-Z])/g, "-$1").toLowerCase(); +}, + _copy = function _copy(obj, exclude) { + var result = {}, + p; + + for (p in obj) { + exclude[p] || (result[p] = obj[p]); + } + + return result; +}, + _memoizedProps = {}, + _memoizeProps = function _memoizeProps(props) { + var p = _memoizedProps[props] = _listToArray(props); + + _memoizedRemoveProps[props] = p.concat(_removeProps); + return p; +}, + _getInverseGlobalMatrix = function _getInverseGlobalMatrix(el) { + // integrates caching for improved performance + var cache = el._gsap || gsap.core.getCache(el); + + if (cache.gmCache === gsap.ticker.frame) { + return cache.gMatrix; + } + + cache.gmCache = gsap.ticker.frame; + return cache.gMatrix = getGlobalMatrix(el, true, false, true); +}, + _getDOMDepth = function _getDOMDepth(el, invert, level) { + if (level === void 0) { + level = 0; + } + + // In invert is true, the sibling depth is increments of 1, and parent/nesting depth is increments of 1000. This lets us order elements in an Array to reflect document flow. + var parent = el.parentNode, + inc = 1000 * Math.pow(10, level) * (invert ? -1 : 1), + l = invert ? -inc * 900 : 0; + + while (el) { + l += inc; + el = el.previousSibling; + } + + return parent ? l + _getDOMDepth(parent, invert, level + 1) : l; +}, + _orderByDOMDepth = function _orderByDOMDepth(comps, invert, isElStates) { + comps.forEach(function (comp) { + return comp.d = _getDOMDepth(isElStates ? comp.element : comp.t, invert); + }); + comps.sort(function (c1, c2) { + return c1.d - c2.d; + }); + return comps; +}, + _recordInlineStyles = function _recordInlineStyles(elState, props) { + // records the current inline CSS properties into an Array in alternating name/value pairs that's stored in a "css" property on the state object so that we can revert later. + var style = elState.element.style, + a = elState.css = elState.css || [], + i = props.length, + p, + v; + + while (i--) { + p = props[i]; + v = style[p] || style.getPropertyValue(p); + a.push(v ? p : _dashedNameLookup[p] || (_dashedNameLookup[p] = _camelToDashed(p)), v); + } + + return style; +}, + _applyInlineStyles = function _applyInlineStyles(state) { + var css = state.css, + style = state.element.style, + i = 0; + state.cache.uncache = 1; + + for (; i < css.length; i += 2) { + css[i + 1] ? style[css[i]] = css[i + 1] : style.removeProperty(css[i]); + } + + if (!css[css.indexOf("transform") + 1] && style.translate) { + // CSSPlugin adds scale, translate, and rotate inline CSS as "none" in order to keep CSS rules from contaminating transforms. + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } +}, + _setFinalStates = function _setFinalStates(comps, onlyTransforms) { + comps.forEach(function (c) { + return c.a.cache.uncache = 1; + }); + onlyTransforms || comps.finalStates.forEach(_applyInlineStyles); +}, + _absoluteProps = "paddingTop,paddingRight,paddingBottom,paddingLeft,gridArea,transition".split(","), + // properties that we must record just +_makeAbsolute = function _makeAbsolute(elState, fallbackNode, ignoreBatch) { + var element = elState.element, + width = elState.width, + height = elState.height, + uncache = elState.uncache, + getProp = elState.getProp, + style = element.style, + i = 4, + result, + displayIsNone, + cs; + typeof fallbackNode !== "object" && (fallbackNode = elState); + + if (_batch && ignoreBatch !== 1) { + _batch._abs.push({ + t: element, + b: elState, + a: elState, + sd: 0 + }); + + _batch._final.push(function () { + return (elState.cache.uncache = 1) && _applyInlineStyles(elState); + }); + + return element; + } + + displayIsNone = getProp("display") === "none"; + + if (!elState.isVisible || displayIsNone) { + displayIsNone && (_recordInlineStyles(elState, ["display"]).display = fallbackNode.display); + elState.matrix = fallbackNode.matrix; + elState.width = width = elState.width || fallbackNode.width; + elState.height = height = elState.height || fallbackNode.height; + } + + _recordInlineStyles(elState, _absoluteProps); + + cs = window.getComputedStyle(element); + + while (i--) { + style[_absoluteProps[i]] = cs[_absoluteProps[i]]; // record paddings as px-based because if removed from grid, percentage-based ones could be altered. + } + + style.gridArea = "1 / 1 / 1 / 1"; + style.transition = "none"; + style.position = "absolute"; + style.width = width + "px"; + style.height = height + "px"; + style.top || (style.top = "0px"); + style.left || (style.left = "0px"); + + if (uncache) { + result = new ElementState(element); + } else { + // better performance + result = _copy(elState, _emptyObj); + result.position = "absolute"; + + if (elState.simple) { + var bounds = element.getBoundingClientRect(); + result.matrix = new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()); + } else { + result.matrix = getGlobalMatrix(element, false, false, true); + } + } + + result = _fit(result, elState, true); + elState.x = _closestTenth(result.x, 0.01); + elState.y = _closestTenth(result.y, 0.01); + return element; +}, + _filterComps = function _filterComps(comps, targets) { + if (targets !== true) { + targets = _toArray(targets); + comps = comps.filter(function (c) { + if (targets.indexOf((c.sd < 0 ? c.b : c.a).element) !== -1) { + return true; + } else { + c.t._gsap.renderTransform(1); // we must force transforms to render on anything that isn't being made position: absolute, otherwise the absolute position happens and then when animation begins it applies transforms which can create a new stacking context, throwing off positioning! + + + if (c.b.isVisible) { + c.t.style.width = c.b.width + "px"; // otherwise things can collapse when contents are made position: absolute. + + c.t.style.height = c.b.height + "px"; + } + } + }); + } + + return comps; +}, + _makeCompsAbsolute = function _makeCompsAbsolute(comps) { + return _orderByDOMDepth(comps, true).forEach(function (c) { + return (c.a.isVisible || c.b.isVisible) && _makeAbsolute(c.sd < 0 ? c.b : c.a, c.b, 1); + }); +}, + _findElStateInState = function _findElStateInState(state, other) { + return other && state.idLookup[_parseElementState(other).id] || state.elementStates[0]; +}, + _parseElementState = function _parseElementState(elOrNode, props, simple, other) { + return elOrNode instanceof ElementState ? elOrNode : elOrNode instanceof FlipState ? _findElStateInState(elOrNode, other) : new ElementState(typeof elOrNode === "string" ? _getEl(elOrNode) || console.warn(elOrNode + " not found") : elOrNode, props, simple); +}, + _recordProps = function _recordProps(elState, props) { + var getProp = gsap.getProperty(elState.element, null, "native"), + obj = elState.props = {}, + i = props.length; + + while (i--) { + obj[props[i]] = (getProp(props[i]) + "").trim(); + } + + obj.zIndex && (obj.zIndex = parseFloat(obj.zIndex) || 0); + return elState; +}, + _applyProps = function _applyProps(element, props) { + var style = element.style || element, + // could pass in a vars object. + p; + + for (p in props) { + style[p] = props[p]; + } +}, + _getID = function _getID(el) { + var id = el.getAttribute("data-flip-id"); + id || el.setAttribute("data-flip-id", id = "auto-" + _id++); + return id; +}, + _elementsFromElementStates = function _elementsFromElementStates(elStates) { + return elStates.map(function (elState) { + return elState.element; + }); +}, + _handleCallback = function _handleCallback(callback, elStates, tl) { + return callback && elStates.length && tl.add(callback(_elementsFromElementStates(elStates), tl, new FlipState(elStates, 0, true)), 0); +}, + _fit = function _fit(fromState, toState, scale, applyProps, fitChild, vars) { + var element = fromState.element, + cache = fromState.cache, + parent = fromState.parent, + x = fromState.x, + y = fromState.y, + width = toState.width, + height = toState.height, + scaleX = toState.scaleX, + scaleY = toState.scaleY, + rotation = toState.rotation, + bounds = toState.bounds, + styles = vars && _getStyleSaver && _getStyleSaver(element, "transform,width,height"), + dimensionState = fromState, + _toState$matrix = toState.matrix, + e = _toState$matrix.e, + f = _toState$matrix.f, + deep = fromState.bounds.width !== bounds.width || fromState.bounds.height !== bounds.height || fromState.scaleX !== scaleX || fromState.scaleY !== scaleY || fromState.rotation !== rotation, + simple = !deep && fromState.simple && toState.simple && !fitChild, + skewX, + fromPoint, + toPoint, + getProp, + parentMatrix, + matrix, + bbox; + + if (simple || !parent) { + scaleX = scaleY = 1; + rotation = skewX = 0; + } else { + parentMatrix = _getInverseGlobalMatrix(parent); + matrix = parentMatrix.clone().multiply(toState.ctm ? toState.matrix.clone().multiply(toState.ctm) : toState.matrix); // root SVG elements have a ctm that we must factor out (for example, viewBox:"0 0 94 94" with a width of 200px would scale the internals by 2.127 but when we're matching the size of the root <svg> element itself, that scaling shouldn't factor in!) + + rotation = _round(Math.atan2(matrix.b, matrix.a) * _RAD2DEG); + skewX = _round(Math.atan2(matrix.c, matrix.d) * _RAD2DEG + rotation) % 360; // in very rare cases, minor rounding might end up with 360 which should be 0. + + scaleX = Math.sqrt(Math.pow(matrix.a, 2) + Math.pow(matrix.b, 2)); + scaleY = Math.sqrt(Math.pow(matrix.c, 2) + Math.pow(matrix.d, 2)) * Math.cos(skewX * _DEG2RAD); + + if (fitChild) { + fitChild = _toArray(fitChild)[0]; + getProp = gsap.getProperty(fitChild); + bbox = fitChild.getBBox && typeof fitChild.getBBox === "function" && fitChild.getBBox(); + dimensionState = { + scaleX: getProp("scaleX"), + scaleY: getProp("scaleY"), + width: bbox ? bbox.width : Math.ceil(parseFloat(getProp("width", "px"))), + height: bbox ? bbox.height : parseFloat(getProp("height", "px")) + }; + } + + cache.rotation = rotation + "deg"; + cache.skewX = skewX + "deg"; + } + + if (scale) { + scaleX *= width === dimensionState.width || !dimensionState.width ? 1 : width / dimensionState.width; // note if widths are both 0, we should make scaleX 1 - some elements have box-sizing that incorporates padding, etc. and we don't want it to collapse in that case. + + scaleY *= height === dimensionState.height || !dimensionState.height ? 1 : height / dimensionState.height; + cache.scaleX = scaleX; + cache.scaleY = scaleY; + } else { + width = _closestTenth(width * scaleX / dimensionState.scaleX, 0); + height = _closestTenth(height * scaleY / dimensionState.scaleY, 0); + element.style.width = width + "px"; + element.style.height = height + "px"; + } // if (fromState.isFixed) { // commented out because it's now taken care of in getGlobalMatrix() with a flag at the end. + // e -= _getDocScrollLeft(); + // f -= _getDocScrollTop(); + // } + + + applyProps && _applyProps(element, toState.props); + + if (simple || !parent) { + x += e - fromState.matrix.e; + y += f - fromState.matrix.f; + } else if (deep || parent !== toState.parent) { + cache.renderTransform(1, cache); + matrix = getGlobalMatrix(fitChild || element, false, false, true); + fromPoint = parentMatrix.apply({ + x: matrix.e, + y: matrix.f + }); + toPoint = parentMatrix.apply({ + x: e, + y: f + }); + x += toPoint.x - fromPoint.x; + y += toPoint.y - fromPoint.y; + } else { + // use a faster/cheaper algorithm if we're just moving x/y + parentMatrix.e = parentMatrix.f = 0; + toPoint = parentMatrix.apply({ + x: e - fromState.matrix.e, + y: f - fromState.matrix.f + }); + x += toPoint.x; + y += toPoint.y; + } + + x = _closestTenth(x, 0.02); + y = _closestTenth(y, 0.02); + + if (vars && !(vars instanceof ElementState)) { + // revert + styles && styles.revert(); + } else { + // or apply the transform immediately + cache.x = x + "px"; + cache.y = y + "px"; + cache.renderTransform(1, cache); + } + + if (vars) { + vars.x = x; + vars.y = y; + vars.rotation = rotation; + vars.skewX = skewX; + + if (scale) { + vars.scaleX = scaleX; + vars.scaleY = scaleY; + } else { + vars.width = width; + vars.height = height; + } + } + + return vars || cache; +}, + _parseState = function _parseState(targetsOrState, vars) { + return targetsOrState instanceof FlipState ? targetsOrState : new FlipState(targetsOrState, vars); +}, + _getChangingElState = function _getChangingElState(toState, fromState, id) { + var to1 = toState.idLookup[id], + to2 = toState.alt[id]; + return to2.isVisible && (!(fromState.getElementState(to2.element) || to2).isVisible || !to1.isVisible) ? to2 : to1; +}, + _bodyMetrics = [], + _bodyProps = "width,height,overflowX,overflowY".split(","), + _bodyLocked, + _lockBodyScroll = function _lockBodyScroll(lock) { + // if there's no scrollbar, we should lock that so that measurements don't get affected by temporary repositioning, like if something is centered in the window. + if (lock !== _bodyLocked) { + var s = _body.style, + w = _body.clientWidth === window.outerWidth, + h = _body.clientHeight === window.outerHeight, + i = 4; + + if (lock && (w || h)) { + while (i--) { + _bodyMetrics[i] = s[_bodyProps[i]]; + } + + if (w) { + s.width = _body.clientWidth + "px"; + s.overflowY = "hidden"; + } + + if (h) { + s.height = _body.clientHeight + "px"; + s.overflowX = "hidden"; + } + + _bodyLocked = lock; + } else if (_bodyLocked) { + while (i--) { + _bodyMetrics[i] ? s[_bodyProps[i]] = _bodyMetrics[i] : s.removeProperty(_camelToDashed(_bodyProps[i])); + } + + _bodyLocked = lock; + } + } +}, + _fromTo = function _fromTo(fromState, toState, vars, relative) { + // relative is -1 if "from()", and 1 if "to()" + fromState instanceof FlipState && toState instanceof FlipState || console.warn("Not a valid state object."); + vars = vars || {}; + + var _vars = vars, + clearProps = _vars.clearProps, + onEnter = _vars.onEnter, + onLeave = _vars.onLeave, + absolute = _vars.absolute, + absoluteOnLeave = _vars.absoluteOnLeave, + custom = _vars.custom, + delay = _vars.delay, + paused = _vars.paused, + repeat = _vars.repeat, + repeatDelay = _vars.repeatDelay, + yoyo = _vars.yoyo, + toggleClass = _vars.toggleClass, + nested = _vars.nested, + _zIndex = _vars.zIndex, + scale = _vars.scale, + fade = _vars.fade, + stagger = _vars.stagger, + spin = _vars.spin, + prune = _vars.prune, + props = ("props" in vars ? vars : fromState).props, + tweenVars = _copy(vars, _reserved), + animation = gsap.timeline({ + delay: delay, + paused: paused, + repeat: repeat, + repeatDelay: repeatDelay, + yoyo: yoyo, + data: "isFlip" + }), + remainingProps = tweenVars, + entering = [], + leaving = [], + comps = [], + swapOutTargets = [], + spinNum = spin === true ? 1 : spin || 0, + spinFunc = typeof spin === "function" ? spin : function () { + return spinNum; + }, + interrupted = fromState.interrupted || toState.interrupted, + addFunc = animation[relative !== 1 ? "to" : "from"], + v, + p, + endTime, + i, + el, + comp, + state, + targets, + finalStates, + fromNode, + toNode, + run, + a, + b; //relative || (toState = (new FlipState(toState.targets, {props: props})).fit(toState, scale)); + + + for (p in toState.idLookup) { + toNode = !toState.alt[p] ? toState.idLookup[p] : _getChangingElState(toState, fromState, p); + el = toNode.element; + fromNode = fromState.idLookup[p]; + fromState.alt[p] && el === fromNode.element && (fromState.alt[p].isVisible || !toNode.isVisible) && (fromNode = fromState.alt[p]); + + if (fromNode) { + comp = { + t: el, + b: fromNode, + a: toNode, + sd: fromNode.element === el ? 0 : toNode.isVisible ? 1 : -1 + }; + comps.push(comp); + + if (comp.sd) { + if (comp.sd < 0) { + comp.b = toNode; + comp.a = fromNode; + } // for swapping elements that got interrupted, we must re-record the inline styles to ensure they're not tainted. Remember, .batch() permits getState() not to force in-progress flips to their end state. + + + interrupted && _recordInlineStyles(comp.b, props ? _memoizedRemoveProps[props] : _removeProps); + fade && comps.push(comp.swap = { + t: fromNode.element, + b: comp.b, + a: comp.a, + sd: -comp.sd, + swap: comp + }); + } + + el._flip = fromNode.element._flip = _batch ? _batch.timeline : animation; + } else if (toNode.isVisible) { + comps.push({ + t: el, + b: _copy(toNode, { + isVisible: 1 + }), + a: toNode, + sd: 0, + entering: 1 + }); // to include it in the "entering" Array and do absolute positioning if necessary + + el._flip = _batch ? _batch.timeline : animation; + } + } + + props && (_memoizedProps[props] || _memoizeProps(props)).forEach(function (p) { + return tweenVars[p] = function (i) { + return comps[i].a.props[p]; + }; + }); + comps.finalStates = finalStates = []; + + run = function run() { + _orderByDOMDepth(comps); + + _lockBodyScroll(true); // otherwise, measurements may get thrown off when things get fit. + // TODO: cache the matrix, especially for parent because it'll probably get reused quite a bit, but lock it to a particular cycle(?). + + + for (i = 0; i < comps.length; i++) { + comp = comps[i]; + a = comp.a; + b = comp.b; + + if (prune && !a.isDifferent(b) && !comp.entering) { + // only flip if things changed! Don't omit it from comps initially because that'd prevent the element from being positioned absolutely (if necessary) + comps.splice(i--, 1); + } else { + el = comp.t; + nested && !(comp.sd < 0) && i && (a.matrix = getGlobalMatrix(el, false, false, true)); // moving a parent affects the position of children + + if (b.isVisible && a.isVisible) { + if (comp.sd < 0) { + // swapping OUT (swap direction of -1 is out) + state = new ElementState(el, props, fromState.simple); + + _fit(state, a, scale, 0, 0, state); + + state.matrix = getGlobalMatrix(el, false, false, true); + state.css = comp.b.css; + comp.a = a = state; + fade && (el.style.opacity = interrupted ? b.opacity : a.opacity); + stagger && swapOutTargets.push(el); + } else if (comp.sd > 0 && fade) { + // swapping IN (swap direction of 1 is in) + el.style.opacity = interrupted ? a.opacity - b.opacity : "0"; + } + + _fit(a, b, scale, props); + } else if (b.isVisible !== a.isVisible) { + // either entering or leaving (one side is invisible) + if (!b.isVisible) { + // entering + a.isVisible && entering.push(a); + comps.splice(i--, 1); + } else if (!a.isVisible) { + // leaving + b.css = a.css; + leaving.push(b); + comps.splice(i--, 1); + absolute && nested && _fit(a, b, scale, props); + } + } + + if (!scale) { + el.style.maxWidth = Math.max(a.width, b.width) + "px"; + el.style.maxHeight = Math.max(a.height, b.height) + "px"; + el.style.minWidth = Math.min(a.width, b.width) + "px"; + el.style.minHeight = Math.min(a.height, b.height) + "px"; + } + + nested && toggleClass && el.classList.add(toggleClass); + } + + finalStates.push(a); + } + + var classTargets; + + if (toggleClass) { + classTargets = finalStates.map(function (s) { + return s.element; + }); + nested && classTargets.forEach(function (e) { + return e.classList.remove(toggleClass); + }); // there could be a delay, so don't leave the classes applied (we'll do it in a timeline callback) + } + + _lockBodyScroll(false); + + if (scale) { + tweenVars.scaleX = function (i) { + return comps[i].a.scaleX; + }; + + tweenVars.scaleY = function (i) { + return comps[i].a.scaleY; + }; + } else { + tweenVars.width = function (i) { + return comps[i].a.width + "px"; + }; + + tweenVars.height = function (i) { + return comps[i].a.height + "px"; + }; + + tweenVars.autoRound = vars.autoRound || false; + } + + tweenVars.x = function (i) { + return comps[i].a.x + "px"; + }; + + tweenVars.y = function (i) { + return comps[i].a.y + "px"; + }; + + tweenVars.rotation = function (i) { + return comps[i].a.rotation + (spin ? spinFunc(i, targets[i], targets) * 360 : 0); + }; + + tweenVars.skewX = function (i) { + return comps[i].a.skewX; + }; + + targets = comps.map(function (c) { + return c.t; + }); + + if (_zIndex || _zIndex === 0) { + tweenVars.modifiers = { + zIndex: function zIndex() { + return _zIndex; + } + }; + tweenVars.zIndex = _zIndex; + tweenVars.immediateRender = vars.immediateRender !== false; + } + + fade && (tweenVars.opacity = function (i) { + return comps[i].sd < 0 ? 0 : comps[i].sd > 0 ? comps[i].a.opacity : "+=0"; + }); + + if (swapOutTargets.length) { + stagger = gsap.utils.distribute(stagger); + var dummyArray = targets.slice(swapOutTargets.length); + + tweenVars.stagger = function (i, el) { + return stagger(~swapOutTargets.indexOf(el) ? targets.indexOf(comps[i].swap.t) : i, el, dummyArray); + }; + } // // for testing... + // gsap.delayedCall(vars.data ? 50 : 1, function() { + // animation.eventCallback("onComplete", () => _setFinalStates(comps, !clearProps)); + // addFunc.call(animation, targets, tweenVars, 0).play(); + // }); + // return; + + + _callbacks.forEach(function (name) { + return vars[name] && animation.eventCallback(name, vars[name], vars[name + "Params"]); + }); // apply callbacks to the timeline, not tweens (because "custom" timing can make multiple tweens) + + + if (custom && targets.length) { + // bust out the custom properties as their own tweens so they can use different eases, durations, etc. + remainingProps = _copy(tweenVars, _reserved); + + if ("scale" in custom) { + custom.scaleX = custom.scaleY = custom.scale; + delete custom.scale; + } + + for (p in custom) { + v = _copy(custom[p], _fitReserved); + v[p] = tweenVars[p]; + !("duration" in v) && "duration" in tweenVars && (v.duration = tweenVars.duration); + v.stagger = tweenVars.stagger; + addFunc.call(animation, targets, v, 0); + delete remainingProps[p]; + } + } + + if (targets.length || leaving.length || entering.length) { + toggleClass && animation.add(function () { + return _toggleClass(classTargets, toggleClass, animation._zTime < 0 ? "remove" : "add"); + }, 0) && !paused && _toggleClass(classTargets, toggleClass, "add"); + targets.length && addFunc.call(animation, targets, remainingProps, 0); + } + + _handleCallback(onEnter, entering, animation); + + _handleCallback(onLeave, leaving, animation); + + var batchTl = _batch && _batch.timeline; + + if (batchTl) { + batchTl.add(animation, 0); + + _batch._final.push(function () { + return _setFinalStates(comps, !clearProps); + }); + } + + endTime = animation.duration(); + animation.call(function () { + var forward = animation.time() >= endTime; + forward && !batchTl && _setFinalStates(comps, !clearProps); + toggleClass && _toggleClass(classTargets, toggleClass, forward ? "remove" : "add"); + }); + }; + + absoluteOnLeave && (absolute = comps.filter(function (comp) { + return !comp.sd && !comp.a.isVisible && comp.b.isVisible; + }).map(function (comp) { + return comp.a.element; + })); + + if (_batch) { + var _batch$_abs; + + absolute && (_batch$_abs = _batch._abs).push.apply(_batch$_abs, _filterComps(comps, absolute)); + + _batch._run.push(run); + } else { + absolute && _makeCompsAbsolute(_filterComps(comps, absolute)); // when making absolute, we must go in a very particular order so that document flow changes don't affect things. Don't make it visible if both the before and after states are invisible! There's no point, and it could make things appear visible during the flip that shouldn't be. + + run(); + } + + var anim = _batch ? _batch.timeline : animation; + + anim.revert = function () { + return _killFlip(anim, 1, 1); + }; // a Flip timeline should behave very different when reverting - it should actually jump to the end so that styles get cleared out. + + + return anim; +}, + _interrupt = function _interrupt(tl) { + tl.vars.onInterrupt && tl.vars.onInterrupt.apply(tl, tl.vars.onInterruptParams || []); + tl.getChildren(true, false, true).forEach(_interrupt); +}, + _killFlip = function _killFlip(tl, action, force) { + // action: 0 = nothing, 1 = complete, 2 = only kill (don't complete) + if (tl && tl.progress() < 1 && (!tl.paused() || force)) { + if (action) { + _interrupt(tl); + + action < 2 && tl.progress(1); // we should also kill it in case it was added to a parent timeline. + + tl.kill(); + } + + return true; + } +}, + _createLookup = function _createLookup(state) { + var lookup = state.idLookup = {}, + alt = state.alt = {}, + elStates = state.elementStates, + i = elStates.length, + elState; + + while (i--) { + elState = elStates[i]; + lookup[elState.id] ? alt[elState.id] = elState : lookup[elState.id] = elState; + } +}; + +var FlipState = /*#__PURE__*/function () { + function FlipState(targets, vars, targetsAreElementStates) { + this.props = vars && vars.props; + this.simple = !!(vars && vars.simple); + + if (targetsAreElementStates) { + this.targets = _elementsFromElementStates(targets); + this.elementStates = targets; + + _createLookup(this); + } else { + this.targets = _toArray(targets); + var soft = vars && (vars.kill === false || vars.batch && !vars.kill); + _batch && !soft && _batch._kill.push(this); + this.update(soft || !!_batch); // when batching, don't force in-progress flips to their end; we need to do that AFTER all getStates() are called. + } + } + + var _proto = FlipState.prototype; + + _proto.update = function update(soft) { + var _this = this; + + this.elementStates = this.targets.map(function (el) { + return new ElementState(el, _this.props, _this.simple); + }); + + _createLookup(this); + + this.interrupt(soft); + this.recordInlineStyles(); + return this; + }; + + _proto.clear = function clear() { + this.targets.length = this.elementStates.length = 0; + + _createLookup(this); + + return this; + }; + + _proto.fit = function fit(state, scale, nested) { + var elStatesInOrder = _orderByDOMDepth(this.elementStates.slice(0), false, true), + toElStates = (state || this).idLookup, + i = 0, + fromNode, + toNode; + + for (; i < elStatesInOrder.length; i++) { + fromNode = elStatesInOrder[i]; + nested && (fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true)); // moving a parent affects the position of children + + toNode = toElStates[fromNode.id]; + toNode && _fit(fromNode, toNode, scale, true, 0, fromNode); + fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true); + } + + return this; + }; + + _proto.getProperty = function getProperty(element, property) { + var es = this.getElementState(element) || _emptyObj; + + return (property in es ? es : es.props || _emptyObj)[property]; + }; + + _proto.add = function add(state) { + var i = state.targets.length, + lookup = this.idLookup, + alt = this.alt, + index, + es, + es2; + + while (i--) { + es = state.elementStates[i]; + es2 = lookup[es.id]; + + if (es2 && (es.element === es2.element || alt[es.id] && alt[es.id].element === es.element)) { + // if the flip id is already in this FlipState, replace it! + index = this.elementStates.indexOf(es.element === es2.element ? es2 : alt[es.id]); + this.targets.splice(index, 1, state.targets[i]); + this.elementStates.splice(index, 1, es); + } else { + this.targets.push(state.targets[i]); + this.elementStates.push(es); + } + } + + state.interrupted && (this.interrupted = true); + state.simple || (this.simple = false); + + _createLookup(this); + + return this; + }; + + _proto.compare = function compare(state) { + var l1 = state.idLookup, + l2 = this.idLookup, + unchanged = [], + changed = [], + enter = [], + leave = [], + targets = [], + a1 = state.alt, + a2 = this.alt, + place = function place(s1, s2, el) { + return (s1.isVisible !== s2.isVisible ? s1.isVisible ? enter : leave : s1.isVisible ? changed : unchanged).push(el) && targets.push(el); + }, + placeIfDoesNotExist = function placeIfDoesNotExist(s1, s2, el) { + return targets.indexOf(el) < 0 && place(s1, s2, el); + }, + s1, + s2, + p, + el, + s1Alt, + s2Alt, + c1, + c2; + + for (p in l1) { + s1Alt = a1[p]; + s2Alt = a2[p]; + s1 = !s1Alt ? l1[p] : _getChangingElState(state, this, p); + el = s1.element; + s2 = l2[p]; + + if (s2Alt) { + c2 = s2.isVisible || !s2Alt.isVisible && el === s2.element ? s2 : s2Alt; + c1 = s1Alt && !s1.isVisible && !s1Alt.isVisible && c2.element === s1Alt.element ? s1Alt : s1; //c1.element !== c2.element && c1.element === s2.element && (c2 = s2); + + if (c1.isVisible && c2.isVisible && c1.element !== c2.element) { + // swapping, so force into "changed" array + (c1.isDifferent(c2) ? changed : unchanged).push(c1.element, c2.element); + targets.push(c1.element, c2.element); + } else { + place(c1, c2, c1.element); + } + + s1Alt && c1.element === s1Alt.element && (s1Alt = l1[p]); + placeIfDoesNotExist(c1.element !== s2.element && s1Alt ? s1Alt : c1, s2, s2.element); + placeIfDoesNotExist(s1Alt && s1Alt.element === s2Alt.element ? s1Alt : c1, s2Alt, s2Alt.element); + s1Alt && placeIfDoesNotExist(s1Alt, s2Alt.element === s1Alt.element ? s2Alt : s2, s1Alt.element); + } else { + !s2 ? enter.push(el) : !s2.isDifferent(s1) ? unchanged.push(el) : place(s1, s2, el); + s1Alt && placeIfDoesNotExist(s1Alt, s2, s1Alt.element); + } + } + + for (p in l2) { + if (!l1[p]) { + leave.push(l2[p].element); + a2[p] && leave.push(a2[p].element); + } + } + + return { + changed: changed, + unchanged: unchanged, + enter: enter, + leave: leave + }; + }; + + _proto.recordInlineStyles = function recordInlineStyles() { + var props = _memoizedRemoveProps[this.props] || _removeProps, + i = this.elementStates.length; + + while (i--) { + _recordInlineStyles(this.elementStates[i], props); + } + }; + + _proto.interrupt = function interrupt(soft) { + var _this2 = this; + + // soft = DON'T force in-progress flip animations to completion (like when running a batch, we can't immediately kill flips when getting states because it could contaminate positioning and other .getState() calls that will run in the batch (we kill AFTER all the .getState() calls complete). + var timelines = []; + this.targets.forEach(function (t) { + var tl = t._flip, + foundInProgress = _killFlip(tl, soft ? 0 : 1); + + soft && foundInProgress && timelines.indexOf(tl) < 0 && tl.add(function () { + return _this2.updateVisibility(); + }); + foundInProgress && timelines.push(tl); + }); + !soft && timelines.length && this.updateVisibility(); // if we found an in-progress Flip animation, we must record all the values in their current state at that point BUT we should update the isVisible value AFTER pushing that flip to completion so that elements that are entering or leaving will populate those Arrays properly. + + this.interrupted || (this.interrupted = !!timelines.length); + }; + + _proto.updateVisibility = function updateVisibility() { + this.elementStates.forEach(function (es) { + var b = es.element.getBoundingClientRect(); + es.isVisible = !!(b.width || b.height || b.top || b.left); + es.uncache = 1; + }); + }; + + _proto.getElementState = function getElementState(element) { + return this.elementStates[this.targets.indexOf(_getEl(element))]; + }; + + _proto.makeAbsolute = function makeAbsolute() { + return _orderByDOMDepth(this.elementStates.slice(0), true, true).map(_makeAbsolute); + }; + + return FlipState; +}(); + +var ElementState = /*#__PURE__*/function () { + function ElementState(element, props, simple) { + this.element = element; + this.update(props, simple); + } + + var _proto2 = ElementState.prototype; + + _proto2.isDifferent = function isDifferent(state) { + var b1 = this.bounds, + b2 = state.bounds; + return b1.top !== b2.top || b1.left !== b2.left || b1.width !== b2.width || b1.height !== b2.height || !this.matrix.equals(state.matrix) || this.opacity !== state.opacity || this.props && state.props && JSON.stringify(this.props) !== JSON.stringify(state.props); + }; + + _proto2.update = function update(props, simple) { + var self = this, + element = self.element, + getProp = gsap.getProperty(element), + cache = gsap.core.getCache(element), + bounds = element.getBoundingClientRect(), + bbox = element.getBBox && typeof element.getBBox === "function" && element.nodeName.toLowerCase() !== "svg" && element.getBBox(), + m = simple ? new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()) : getGlobalMatrix(element, false, false, true); + self.getProp = getProp; + self.element = element; + self.id = _getID(element); + self.matrix = m; + self.cache = cache; + self.bounds = bounds; + self.isVisible = !!(bounds.width || bounds.height || bounds.left || bounds.top); + self.display = getProp("display"); + self.position = getProp("position"); + self.parent = element.parentNode; + self.x = getProp("x"); + self.y = getProp("y"); + self.scaleX = cache.scaleX; + self.scaleY = cache.scaleY; + self.rotation = getProp("rotation"); + self.skewX = getProp("skewX"); + self.opacity = getProp("opacity"); + self.width = bbox ? bbox.width : _closestTenth(getProp("width", "px"), 0.04); // round up to the closest 0.1 so that text doesn't wrap. + + self.height = bbox ? bbox.height : _closestTenth(getProp("height", "px"), 0.04); + props && _recordProps(self, _memoizedProps[props] || _memoizeProps(props)); + self.ctm = element.getCTM && element.nodeName.toLowerCase() === "svg" && _getCTM(element).inverse(); + self.simple = simple || _round(m.a) === 1 && !_round(m.b) && !_round(m.c) && _round(m.d) === 1; // allows us to speed through some other tasks if it's not scale/rotated + + self.uncache = 0; + }; + + return ElementState; +}(); + +var FlipAction = /*#__PURE__*/function () { + function FlipAction(vars, batch) { + this.vars = vars; + this.batch = batch; + this.states = []; + this.timeline = batch.timeline; + } + + var _proto3 = FlipAction.prototype; + + _proto3.getStateById = function getStateById(id) { + var i = this.states.length; + + while (i--) { + if (this.states[i].idLookup[id]) { + return this.states[i]; + } + } + }; + + _proto3.kill = function kill() { + this.batch.remove(this); + }; + + return FlipAction; +}(); + +var FlipBatch = /*#__PURE__*/function () { + function FlipBatch(id) { + this.id = id; + this.actions = []; + this._kill = []; + this._final = []; + this._abs = []; + this._run = []; + this.data = {}; + this.state = new FlipState(); + this.timeline = gsap.timeline(); + } + + var _proto4 = FlipBatch.prototype; + + _proto4.add = function add(config) { + var result = this.actions.filter(function (action) { + return action.vars === config; + }); + + if (result.length) { + return result[0]; + } + + result = new FlipAction(typeof config === "function" ? { + animate: config + } : config, this); + this.actions.push(result); + return result; + }; + + _proto4.remove = function remove(action) { + var i = this.actions.indexOf(action); + i >= 0 && this.actions.splice(i, 1); + return this; + }; + + _proto4.getState = function getState(merge) { + var _this3 = this; + + var prevBatch = _batch, + prevAction = _batchAction; + _batch = this; + this.state.clear(); + this._kill.length = 0; + this.actions.forEach(function (action) { + if (action.vars.getState) { + action.states.length = 0; + _batchAction = action; + action.state = action.vars.getState(action); + } + + merge && action.states.forEach(function (s) { + return _this3.state.add(s); + }); + }); + _batchAction = prevAction; + _batch = prevBatch; + this.killConflicts(); + return this; + }; + + _proto4.animate = function animate() { + var _this4 = this; + + var prevBatch = _batch, + tl = this.timeline, + i = this.actions.length, + finalStates, + endTime; + _batch = this; + tl.clear(); + this._abs.length = this._final.length = this._run.length = 0; + this.actions.forEach(function (a) { + a.vars.animate && a.vars.animate(a); + var onEnter = a.vars.onEnter, + onLeave = a.vars.onLeave, + targets = a.targets, + s, + result; + + if (targets && targets.length && (onEnter || onLeave)) { + s = new FlipState(); + a.states.forEach(function (state) { + return s.add(state); + }); + result = s.compare(Flip.getState(targets)); + result.enter.length && onEnter && onEnter(result.enter); + result.leave.length && onLeave && onLeave(result.leave); + } + }); + + _makeCompsAbsolute(this._abs); + + this._run.forEach(function (f) { + return f(); + }); + + endTime = tl.duration(); + finalStates = this._final.slice(0); + tl.add(function () { + if (endTime <= tl.time()) { + // only call if moving forward in the timeline (in case it's nested in a timeline that gets reversed) + finalStates.forEach(function (f) { + return f(); + }); + + _forEachBatch(_this4, "onComplete"); + } + }); + _batch = prevBatch; + + while (i--) { + this.actions[i].vars.once && this.actions[i].kill(); + } + + _forEachBatch(this, "onStart"); + + tl.restart(); + return this; + }; + + _proto4.loadState = function loadState(done) { + done || (done = function done() { + return 0; + }); + var queue = []; + this.actions.forEach(function (c) { + if (c.vars.loadState) { + var i, + f = function f(targets) { + targets && (c.targets = targets); + i = queue.indexOf(f); + + if (~i) { + queue.splice(i, 1); + queue.length || done(); + } + }; + + queue.push(f); + c.vars.loadState(f); + } + }); + queue.length || done(); + return this; + }; + + _proto4.setState = function setState() { + this.actions.forEach(function (c) { + return c.targets = c.vars.setState && c.vars.setState(c); + }); + return this; + }; + + _proto4.killConflicts = function killConflicts(soft) { + this.state.interrupt(soft); + + this._kill.forEach(function (state) { + return state.interrupt(soft); + }); + + return this; + }; + + _proto4.run = function run(skipGetState, merge) { + var _this5 = this; + + if (this !== _batch) { + skipGetState || this.getState(merge); + this.loadState(function () { + if (!_this5._killed) { + _this5.setState(); + + _this5.animate(); + } + }); + } + + return this; + }; + + _proto4.clear = function clear(stateOnly) { + this.state.clear(); + stateOnly || (this.actions.length = 0); + }; + + _proto4.getStateById = function getStateById(id) { + var i = this.actions.length, + s; + + while (i--) { + s = this.actions[i].getStateById(id); + + if (s) { + return s; + } + } + + return this.state.idLookup[id] && this.state; + }; + + _proto4.kill = function kill() { + this._killed = 1; + this.clear(); + delete _batchLookup[this.id]; + }; + + return FlipBatch; +}(); + +export var Flip = /*#__PURE__*/function () { + function Flip() {} + + Flip.getState = function getState(targets, vars) { + var state = _parseState(targets, vars); + + _batchAction && _batchAction.states.push(state); + vars && vars.batch && Flip.batch(vars.batch).state.add(state); + return state; + }; + + Flip.from = function from(state, vars) { + vars = vars || {}; + "clearProps" in vars || (vars.clearProps = true); + return _fromTo(state, _parseState(vars.targets || state.targets, { + props: vars.props || state.props, + simple: vars.simple, + kill: !!vars.kill + }), vars, -1); + }; + + Flip.to = function to(state, vars) { + return _fromTo(state, _parseState(vars.targets || state.targets, { + props: vars.props || state.props, + simple: vars.simple, + kill: !!vars.kill + }), vars, 1); + }; + + Flip.fromTo = function fromTo(fromState, toState, vars) { + return _fromTo(fromState, toState, vars); + }; + + Flip.fit = function fit(fromEl, toEl, vars) { + var v = vars ? _copy(vars, _fitReserved) : {}, + _ref = vars || v, + absolute = _ref.absolute, + scale = _ref.scale, + getVars = _ref.getVars, + props = _ref.props, + runBackwards = _ref.runBackwards, + onComplete = _ref.onComplete, + simple = _ref.simple, + fitChild = vars && vars.fitChild && _getEl(vars.fitChild), + before = _parseElementState(toEl, props, simple, fromEl), + after = _parseElementState(fromEl, 0, simple, before), + inlineProps = props ? _memoizedRemoveProps[props] : _removeProps, + ctx = gsap.context(); + + props && _applyProps(v, before.props); + + _recordInlineStyles(after, inlineProps); + + if (runBackwards) { + "immediateRender" in v || (v.immediateRender = true); + + v.onComplete = function () { + _applyInlineStyles(after); + + onComplete && onComplete.apply(this, arguments); + }; + } + + absolute && _makeAbsolute(after, before); + v = _fit(after, before, scale || fitChild, props, fitChild, v.duration || getVars ? v : 0); + typeof vars === "object" && "zIndex" in vars && (v.zIndex = vars.zIndex); + ctx && !getVars && ctx.add(function () { + return function () { + return _applyInlineStyles(after); + }; + }); + return getVars ? v : v.duration ? gsap.to(after.element, v) : null; + }; + + Flip.makeAbsolute = function makeAbsolute(targetsOrStates, vars) { + return (targetsOrStates instanceof FlipState ? targetsOrStates : new FlipState(targetsOrStates, vars)).makeAbsolute(); + }; + + Flip.batch = function batch(id) { + id || (id = "default"); + return _batchLookup[id] || (_batchLookup[id] = new FlipBatch(id)); + }; + + Flip.killFlipsOf = function killFlipsOf(targets, complete) { + (targets instanceof FlipState ? targets.targets : _toArray(targets)).forEach(function (t) { + return t && _killFlip(t._flip, complete !== false ? 1 : 2); + }); + }; + + Flip.isFlipping = function isFlipping(target) { + var f = Flip.getByTarget(target); + return !!f && f.isActive(); + }; + + Flip.getByTarget = function getByTarget(target) { + return (_getEl(target) || _emptyObj)._flip; + }; + + Flip.getElementState = function getElementState(target, props) { + return new ElementState(_getEl(target), props); + }; + + Flip.convertCoordinates = function convertCoordinates(fromElement, toElement, point) { + var m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }; + + Flip.register = function register(core) { + _body = typeof document !== "undefined" && document.body; + + if (_body) { + gsap = core; + + _setDoc(_body); + + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + var snap = gsap.utils.snap(0.1); + + _closestTenth = function _closestTenth(value, add) { + return snap(parseFloat(value) + add); + }; + } + }; + + return Flip; +}(); +Flip.version = "3.12.7"; // function whenImagesLoad(el, func) { +// let pending = [], +// onLoad = e => { +// pending.splice(pending.indexOf(e.target), 1); +// e.target.removeEventListener("load", onLoad); +// pending.length || func(); +// }; +// gsap.utils.toArray(el.tagName.toLowerCase() === "img" ? el : el.querySelectorAll("img")).forEach(img => img.complete || img.addEventListener("load", onLoad) || pending.push(img)); +// pending.length || func(); +// } + +typeof window !== "undefined" && window.gsap && window.gsap.registerPlugin(Flip); +export { Flip as default }; \ No newline at end of file diff --git a/node_modules/gsap/MotionPathPlugin.js b/node_modules/gsap/MotionPathPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..4874e494c1fb15e7d6a75fd81026264e88c1f0a8 --- /dev/null +++ b/node_modules/gsap/MotionPathPlugin.js @@ -0,0 +1,370 @@ +/*! + * MotionPathPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +import { getRawPath, cacheRawPathMeasurements, getPositionOnPath, pointsToSegment, flatPointsToSegment, sliceRawPath, stringToRawPath, rawPathToString, transformRawPath, convertToPath as _convertToPath } from "./utils/paths.js"; +import { getGlobalMatrix } from "./utils/matrix.js"; + +var _xProps = "x,translateX,left,marginLeft,xPercent".split(","), + _yProps = "y,translateY,top,marginTop,yPercent".split(","), + _DEG2RAD = Math.PI / 180, + gsap, + PropTween, + _getUnit, + _toArray, + _getStyleSaver, + _reverting, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _populateSegmentFromArray = function _populateSegmentFromArray(segment, values, property, mode) { + //mode: 0 = x but don't fill y yet, 1 = y, 2 = x and fill y with 0. + var l = values.length, + si = mode === 2 ? 0 : mode, + i = 0, + v; + + for (; i < l; i++) { + segment[si] = v = parseFloat(values[i][property]); + mode === 2 && (segment[si + 1] = 0); + si += 2; + } + + return segment; +}, + _getPropNum = function _getPropNum(target, prop, unit) { + return parseFloat(target._gsap.get(target, prop, unit || "px")) || 0; +}, + _relativize = function _relativize(segment) { + var x = segment[0], + y = segment[1], + i; + + for (i = 2; i < segment.length; i += 2) { + x = segment[i] += x; + y = segment[i + 1] += y; + } +}, + // feed in an array of quadratic bezier points like [{x: 0, y: 0}, ...] and it'll convert it to cubic bezier +// _quadToCubic = points => { +// let cubic = [], +// l = points.length - 1, +// i = 1, +// a, b, c; +// for (; i < l; i+=2) { +// a = points[i-1]; +// b = points[i]; +// c = points[i+1]; +// cubic.push(a, {x: (2 * b.x + a.x) / 3, y: (2 * b.y + a.y) / 3}, {x: (2 * b.x + c.x) / 3, y: (2 * b.y + c.y) / 3}); +// } +// cubic.push(points[l]); +// return cubic; +// }, +_segmentToRawPath = function _segmentToRawPath(plugin, segment, target, x, y, slicer, vars, unitX, unitY) { + if (vars.type === "cubic") { + segment = [segment]; + } else { + vars.fromCurrent !== false && segment.unshift(_getPropNum(target, x, unitX), y ? _getPropNum(target, y, unitY) : 0); + vars.relative && _relativize(segment); + var pointFunc = y ? pointsToSegment : flatPointsToSegment; + segment = [pointFunc(segment, vars.curviness)]; + } + + segment = slicer(_align(segment, target, vars)); + + _addDimensionalPropTween(plugin, target, x, segment, "x", unitX); + + y && _addDimensionalPropTween(plugin, target, y, segment, "y", unitY); + return cacheRawPathMeasurements(segment, vars.resolution || (vars.curviness === 0 ? 20 : 12)); //when curviness is 0, it creates control points right on top of the anchors which makes it more sensitive to resolution, thus we change the default accordingly. +}, + _emptyFunc = function _emptyFunc(v) { + return v; +}, + _numExp = /[-+\.]*\d+\.?(?:e-|e\+)?\d*/g, + _originToPoint = function _originToPoint(element, origin, parentMatrix) { + // origin is an array of normalized values (0-1) in relation to the width/height, so [0.5, 0.5] would be the center. It can also be "auto" in which case it will be the top left unless it's a <path>, when it will start at the beginning of the path itself. + var m = getGlobalMatrix(element), + x = 0, + y = 0, + svg; + + if ((element.tagName + "").toLowerCase() === "svg") { + svg = element.viewBox.baseVal; + svg.width || (svg = { + width: +element.getAttribute("width"), + height: +element.getAttribute("height") + }); + } else { + svg = origin && element.getBBox && element.getBBox(); + } + + if (origin && origin !== "auto") { + x = origin.push ? origin[0] * (svg ? svg.width : element.offsetWidth || 0) : origin.x; + y = origin.push ? origin[1] * (svg ? svg.height : element.offsetHeight || 0) : origin.y; + } + + return parentMatrix.apply(x || y ? m.apply({ + x: x, + y: y + }) : { + x: m.e, + y: m.f + }); +}, + _getAlignMatrix = function _getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin) { + var parentMatrix = getGlobalMatrix(fromElement.parentNode, true, true), + m = parentMatrix.clone().multiply(getGlobalMatrix(toElement)), + fromPoint = _originToPoint(fromElement, fromOrigin, parentMatrix), + _originToPoint2 = _originToPoint(toElement, toOrigin, parentMatrix), + x = _originToPoint2.x, + y = _originToPoint2.y, + p; + + m.e = m.f = 0; + + if (toOrigin === "auto" && toElement.getTotalLength && toElement.tagName.toLowerCase() === "path") { + p = toElement.getAttribute("d").match(_numExp) || []; + p = m.apply({ + x: +p[0], + y: +p[1] + }); + x += p.x; + y += p.y; + } //if (p || (toElement.getBBox && fromElement.getBBox && toElement.ownerSVGElement === fromElement.ownerSVGElement)) { + + + if (p) { + p = m.apply(toElement.getBBox()); + x -= p.x; + y -= p.y; + } + + m.e = x - fromPoint.x; + m.f = y - fromPoint.y; + return m; +}, + _align = function _align(rawPath, target, _ref) { + var align = _ref.align, + matrix = _ref.matrix, + offsetX = _ref.offsetX, + offsetY = _ref.offsetY, + alignOrigin = _ref.alignOrigin; + + var x = rawPath[0][0], + y = rawPath[0][1], + curX = _getPropNum(target, "x"), + curY = _getPropNum(target, "y"), + alignTarget, + m, + p; + + if (!rawPath || !rawPath.length) { + return getRawPath("M0,0L0,0"); + } + + if (align) { + if (align === "self" || (alignTarget = _toArray(align)[0] || target) === target) { + transformRawPath(rawPath, 1, 0, 0, 1, curX - x, curY - y); + } else { + if (alignOrigin && alignOrigin[2] !== false) { + gsap.set(target, { + transformOrigin: alignOrigin[0] * 100 + "% " + alignOrigin[1] * 100 + "%" + }); + } else { + alignOrigin = [_getPropNum(target, "xPercent") / -100, _getPropNum(target, "yPercent") / -100]; + } + + m = _getAlignMatrix(target, alignTarget, alignOrigin, "auto"); + p = m.apply({ + x: x, + y: y + }); + transformRawPath(rawPath, m.a, m.b, m.c, m.d, curX + m.e - (p.x - m.e), curY + m.f - (p.y - m.f)); + } + } + + if (matrix) { + transformRawPath(rawPath, matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f); + } else if (offsetX || offsetY) { + transformRawPath(rawPath, 1, 0, 0, 1, offsetX || 0, offsetY || 0); + } + + return rawPath; +}, + _addDimensionalPropTween = function _addDimensionalPropTween(plugin, target, property, rawPath, pathProperty, forceUnit) { + var cache = target._gsap, + harness = cache.harness, + alias = harness && harness.aliases && harness.aliases[property], + prop = alias && alias.indexOf(",") < 0 ? alias : property, + pt = plugin._pt = new PropTween(plugin._pt, target, prop, 0, 0, _emptyFunc, 0, cache.set(target, prop, plugin)); + pt.u = _getUnit(cache.get(target, prop, forceUnit)) || 0; + pt.path = rawPath; + pt.pp = pathProperty; + + plugin._props.push(prop); +}, + _sliceModifier = function _sliceModifier(start, end) { + return function (rawPath) { + return start || end !== 1 ? sliceRawPath(rawPath, start, end) : rawPath; + }; +}; + +export var MotionPathPlugin = { + version: "3.12.7", + name: "motionPath", + register: function register(core, Plugin, propTween) { + gsap = core; + _getUnit = gsap.utils.getUnit; + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + + _reverting = gsap.core.reverting || function () {}; + + PropTween = propTween; + }, + init: function init(target, vars, tween) { + if (!gsap) { + console.warn("Please gsap.registerPlugin(MotionPathPlugin)"); + return false; + } + + if (!(typeof vars === "object" && !vars.style) || !vars.path) { + vars = { + path: vars + }; + } + + var rawPaths = [], + _vars = vars, + path = _vars.path, + autoRotate = _vars.autoRotate, + unitX = _vars.unitX, + unitY = _vars.unitY, + x = _vars.x, + y = _vars.y, + firstObj = path[0], + slicer = _sliceModifier(vars.start, "end" in vars ? vars.end : 1), + rawPath, + p; + + this.rawPaths = rawPaths; + this.target = target; + this.tween = tween; + this.styles = _getStyleSaver && _getStyleSaver(target, "transform"); + + if (this.rotate = autoRotate || autoRotate === 0) { + //get the rotational data FIRST so that the setTransform() method is called in the correct order in the render() loop - rotation gets set last. + this.rOffset = parseFloat(autoRotate) || 0; + this.radians = !!vars.useRadians; + this.rProp = vars.rotation || "rotation"; // rotation property + + this.rSet = target._gsap.set(target, this.rProp, this); // rotation setter + + this.ru = _getUnit(target._gsap.get(target, this.rProp)) || 0; // rotation units + } + + if (Array.isArray(path) && !("closed" in path) && typeof firstObj !== "number") { + for (p in firstObj) { + if (!x && ~_xProps.indexOf(p)) { + x = p; + } else if (!y && ~_yProps.indexOf(p)) { + y = p; + } + } + + if (x && y) { + //correlated values + rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray(_populateSegmentFromArray([], path, x, 0), path, y, 1), target, x, y, slicer, vars, unitX || _getUnit(path[0][x]), unitY || _getUnit(path[0][y]))); + } else { + x = y = 0; + } + + for (p in firstObj) { + p !== x && p !== y && rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray([], path, p, 2), target, p, 0, slicer, vars, _getUnit(path[0][p]))); + } + } else { + rawPath = slicer(_align(getRawPath(vars.path), target, vars)); + cacheRawPathMeasurements(rawPath, vars.resolution); + rawPaths.push(rawPath); + + _addDimensionalPropTween(this, target, vars.x || "x", rawPath, "x", vars.unitX || "px"); + + _addDimensionalPropTween(this, target, vars.y || "y", rawPath, "y", vars.unitY || "px"); + } + + tween.vars.immediateRender && this.render(tween.progress(), this); + }, + render: function render(ratio, data) { + var rawPaths = data.rawPaths, + i = rawPaths.length, + pt = data._pt; + + if (data.tween._time || !_reverting()) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + + while (i--) { + getPositionOnPath(rawPaths[i], ratio, !i && data.rotate, rawPaths[i]); + } + + while (pt) { + pt.set(pt.t, pt.p, pt.path[pt.pp] + pt.u, pt.d, ratio); + pt = pt._next; + } + + data.rotate && data.rSet(data.target, data.rProp, rawPaths[0].angle * (data.radians ? _DEG2RAD : 1) + data.rOffset + data.ru, data, ratio); + } else { + data.styles.revert(); + } + }, + getLength: function getLength(path) { + return cacheRawPathMeasurements(getRawPath(path)).totalLength; + }, + sliceRawPath: sliceRawPath, + getRawPath: getRawPath, + pointsToSegment: pointsToSegment, + stringToRawPath: stringToRawPath, + rawPathToString: rawPathToString, + transformRawPath: transformRawPath, + getGlobalMatrix: getGlobalMatrix, + getPositionOnPath: getPositionOnPath, + cacheRawPathMeasurements: cacheRawPathMeasurements, + convertToPath: function convertToPath(targets, swap) { + return _toArray(targets).map(function (target) { + return _convertToPath(target, swap !== false); + }); + }, + convertCoordinates: function convertCoordinates(fromElement, toElement, point) { + var m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }, + getAlignMatrix: _getAlignMatrix, + getRelativePosition: function getRelativePosition(fromElement, toElement, fromOrigin, toOrigin) { + var m = _getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin); + + return { + x: m.e, + y: m.f + }; + }, + arrayToRawPath: function arrayToRawPath(value, vars) { + vars = vars || {}; + + var segment = _populateSegmentFromArray(_populateSegmentFromArray([], value, vars.x || "x", 0), value, vars.y || "y", 1); + + vars.relative && _relativize(segment); + return [vars.type === "cubic" ? segment : pointsToSegment(segment, vars.curviness)]; + } +}; +_getGSAP() && gsap.registerPlugin(MotionPathPlugin); +export { MotionPathPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/Observer.js b/node_modules/gsap/Observer.js new file mode 100644 index 0000000000000000000000000000000000000000..3a1c9a1580983aad28b70e461a539a96a4ca48c8 --- /dev/null +++ b/node_modules/gsap/Observer.js @@ -0,0 +1,697 @@ +function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } + +function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } + +/*! + * Observer 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var gsap, + _coreInitted, + _clamp, + _win, + _doc, + _docEl, + _body, + _isTouch, + _pointerType, + ScrollTrigger, + _root, + _normalizer, + _eventTypes, + _context, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _startup = 1, + _observers = [], + _scrollers = [], + _proxies = [], + _getTime = Date.now, + _bridge = function _bridge(name, value) { + return value; +}, + _integrate = function _integrate() { + var core = ScrollTrigger.core, + data = core.bridge || {}, + scrollers = core._scrollers, + proxies = core._proxies; + scrollers.push.apply(scrollers, _scrollers); + proxies.push.apply(proxies, _proxies); + _scrollers = scrollers; + _proxies = proxies; + + _bridge = function _bridge(name, value) { + return data[name](value); + }; +}, + _getProxyProp = function _getProxyProp(element, property) { + return ~_proxies.indexOf(element) && _proxies[_proxies.indexOf(element) + 1][property]; +}, + _isViewport = function _isViewport(el) { + return !!~_root.indexOf(el); +}, + _addListener = function _addListener(element, type, func, passive, capture) { + return element.addEventListener(type, func, { + passive: passive !== false, + capture: !!capture + }); +}, + _removeListener = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); +}, + _scrollLeft = "scrollLeft", + _scrollTop = "scrollTop", + _onScroll = function _onScroll() { + return _normalizer && _normalizer.isPressed || _scrollers.cache++; +}, + _scrollCacheFunc = function _scrollCacheFunc(f, doNotCache) { + var cachingFunc = function cachingFunc(value) { + // since reading the scrollTop/scrollLeft/pageOffsetY/pageOffsetX can trigger a layout, this function allows us to cache the value so it only gets read fresh after a "scroll" event fires (or while we're refreshing because that can lengthen the page and alter the scroll position). when "soft" is true, that means don't actually set the scroll, but cache the new value instead (useful in ScrollSmoother) + if (value || value === 0) { + _startup && (_win.history.scrollRestoration = "manual"); // otherwise the new position will get overwritten by the browser onload. + + var isNormalizing = _normalizer && _normalizer.isPressed; + value = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); //TODO: iOS Bug: if you allow it to go to 0, Safari can start to report super strange (wildly inaccurate) touch positions! + + f(value); + cachingFunc.cacheID = _scrollers.cache; + isNormalizing && _bridge("ss", value); // set scroll (notify ScrollTrigger so it can dispatch a "scrollStart" event if necessary + } else if (doNotCache || _scrollers.cache !== cachingFunc.cacheID || _bridge("ref")) { + cachingFunc.cacheID = _scrollers.cache; + cachingFunc.v = f(); + } + + return cachingFunc.v + cachingFunc.offset; + }; + + cachingFunc.offset = 0; + return f && cachingFunc; +}, + _horizontal = { + s: _scrollLeft, + p: "left", + p2: "Left", + os: "right", + os2: "Right", + d: "width", + d2: "Width", + a: "x", + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win.scrollTo(value, _vertical.sc()) : _win.pageXOffset || _doc[_scrollLeft] || _docEl[_scrollLeft] || _body[_scrollLeft] || 0; + }) +}, + _vertical = { + s: _scrollTop, + p: "top", + p2: "Top", + os: "bottom", + os2: "Bottom", + d: "height", + d2: "Height", + a: "y", + op: _horizontal, + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win.scrollTo(_horizontal.sc(), value) : _win.pageYOffset || _doc[_scrollTop] || _docEl[_scrollTop] || _body[_scrollTop] || 0; + }) +}, + _getTarget = function _getTarget(t, self) { + return (self && self._ctx && self._ctx.selector || gsap.utils.toArray)(t)[0] || (typeof t === "string" && gsap.config().nullTargetWarn !== false ? console.warn("Element not found:", t) : null); +}, + _getScrollFunc = function _getScrollFunc(element, _ref) { + var s = _ref.s, + sc = _ref.sc; + // we store the scroller functions in an alternating sequenced Array like [element, verticalScrollFunc, horizontalScrollFunc, ...] so that we can minimize memory, maximize performance, and we also record the last position as a ".rec" property in order to revert to that after refreshing to ensure things don't shift around. + _isViewport(element) && (element = _doc.scrollingElement || _docEl); + + var i = _scrollers.indexOf(element), + offset = sc === _vertical.sc ? 1 : 2; + + !~i && (i = _scrollers.push(element) - 1); + _scrollers[i + offset] || _addListener(element, "scroll", _onScroll); // clear the cache when a scroll occurs + + var prev = _scrollers[i + offset], + func = prev || (_scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function (value) { + return arguments.length ? element[s] = value : element[s]; + }))); + func.target = element; + prev || (func.smooth = gsap.getProperty(element, "scrollBehavior") === "smooth"); // only set it the first time (don't reset every time a scrollFunc is requested because perhaps it happens during a refresh() when it's disabled in ScrollTrigger. + + return func; +}, + _getVelocityProp = function _getVelocityProp(value, minTimeRefresh, useDelta) { + var v1 = value, + v2 = value, + t1 = _getTime(), + t2 = t1, + min = minTimeRefresh || 50, + dropToZeroTime = Math.max(500, min * 3), + update = function update(value, force) { + var t = _getTime(); + + if (force || t - t1 > min) { + v2 = v1; + v1 = value; + t2 = t1; + t1 = t; + } else if (useDelta) { + v1 += value; + } else { + // not totally necessary, but makes it a bit more accurate by adjusting the v1 value according to the new slope. This way we're not just ignoring the incoming data. Removing for now because it doesn't seem to make much practical difference and it's probably not worth the kb. + v1 = v2 + (value - v2) / (t - t2) * (t1 - t2); + } + }, + reset = function reset() { + v2 = v1 = useDelta ? 0 : v1; + t2 = t1 = 0; + }, + getVelocity = function getVelocity(latestValue) { + var tOld = t2, + vOld = v2, + t = _getTime(); + + (latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue); + return t1 === t2 || t - t2 > dropToZeroTime ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000; + }; + + return { + update: update, + reset: reset, + getVelocity: getVelocity + }; +}, + _getEvent = function _getEvent(e, preventDefault) { + preventDefault && !e._gsapAllow && e.preventDefault(); + return e.changedTouches ? e.changedTouches[0] : e; +}, + _getAbsoluteMax = function _getAbsoluteMax(a) { + var max = Math.max.apply(Math, a), + min = Math.min.apply(Math, a); + return Math.abs(max) >= Math.abs(min) ? max : min; +}, + _setScrollTrigger = function _setScrollTrigger() { + ScrollTrigger = gsap.core.globals().ScrollTrigger; + ScrollTrigger && ScrollTrigger.core && _integrate(); +}, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (!_coreInitted && gsap && typeof document !== "undefined" && document.body) { + _win = window; + _doc = document; + _docEl = _doc.documentElement; + _body = _doc.body; + _root = [_win, _doc, _docEl, _body]; + _clamp = gsap.utils.clamp; + + _context = gsap.core.context || function () {}; + + _pointerType = "onpointerenter" in _body ? "pointer" : "mouse"; // isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer. + + _isTouch = Observer.isTouch = _win.matchMedia && _win.matchMedia("(hover: none), (pointer: coarse)").matches ? 1 : "ontouchstart" in _win || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0 ? 2 : 0; + _eventTypes = Observer.eventTypes = ("ontouchstart" in _docEl ? "touchstart,touchmove,touchcancel,touchend" : !("onpointerdown" in _docEl) ? "mousedown,mousemove,mouseup,mouseup" : "pointerdown,pointermove,pointercancel,pointerup").split(","); + setTimeout(function () { + return _startup = 0; + }, 500); + + _setScrollTrigger(); + + _coreInitted = 1; + } + + return _coreInitted; +}; + +_horizontal.op = _vertical; +_scrollers.cache = 0; +export var Observer = /*#__PURE__*/function () { + function Observer(vars) { + this.init(vars); + } + + var _proto = Observer.prototype; + + _proto.init = function init(vars) { + _coreInitted || _initCore(gsap) || console.warn("Please gsap.registerPlugin(Observer)"); + ScrollTrigger || _setScrollTrigger(); + var tolerance = vars.tolerance, + dragMinimum = vars.dragMinimum, + type = vars.type, + target = vars.target, + lineHeight = vars.lineHeight, + debounce = vars.debounce, + preventDefault = vars.preventDefault, + onStop = vars.onStop, + onStopDelay = vars.onStopDelay, + ignore = vars.ignore, + wheelSpeed = vars.wheelSpeed, + event = vars.event, + onDragStart = vars.onDragStart, + onDragEnd = vars.onDragEnd, + onDrag = vars.onDrag, + onPress = vars.onPress, + onRelease = vars.onRelease, + onRight = vars.onRight, + onLeft = vars.onLeft, + onUp = vars.onUp, + onDown = vars.onDown, + onChangeX = vars.onChangeX, + onChangeY = vars.onChangeY, + onChange = vars.onChange, + onToggleX = vars.onToggleX, + onToggleY = vars.onToggleY, + onHover = vars.onHover, + onHoverEnd = vars.onHoverEnd, + onMove = vars.onMove, + ignoreCheck = vars.ignoreCheck, + isNormalizer = vars.isNormalizer, + onGestureStart = vars.onGestureStart, + onGestureEnd = vars.onGestureEnd, + onWheel = vars.onWheel, + onEnable = vars.onEnable, + onDisable = vars.onDisable, + onClick = vars.onClick, + scrollSpeed = vars.scrollSpeed, + capture = vars.capture, + allowClicks = vars.allowClicks, + lockAxis = vars.lockAxis, + onLockAxis = vars.onLockAxis; + this.target = target = _getTarget(target) || _docEl; + this.vars = vars; + ignore && (ignore = gsap.utils.toArray(ignore)); + tolerance = tolerance || 1e-9; + dragMinimum = dragMinimum || 0; + wheelSpeed = wheelSpeed || 1; + scrollSpeed = scrollSpeed || 1; + type = type || "wheel,touch,pointer"; + debounce = debounce !== false; + lineHeight || (lineHeight = parseFloat(_win.getComputedStyle(_body).lineHeight) || 22); // note: browser may report "normal", so default to 22. + + var id, + onStopDelayedCall, + dragged, + moved, + wheeled, + locked, + axis, + self = this, + prevDeltaX = 0, + prevDeltaY = 0, + passive = vars.passive || !preventDefault && vars.passive !== false, + scrollFuncX = _getScrollFunc(target, _horizontal), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollX = scrollFuncX(), + scrollY = scrollFuncY(), + limitToTouch = ~type.indexOf("touch") && !~type.indexOf("pointer") && _eventTypes[0] === "pointerdown", + // for devices that accommodate mouse events and touch events, we need to distinguish. + isViewport = _isViewport(target), + ownerDoc = target.ownerDocument || _doc, + deltaX = [0, 0, 0], + // wheel, scroll, pointer/touch + deltaY = [0, 0, 0], + onClickTime = 0, + clickCapture = function clickCapture() { + return onClickTime = _getTime(); + }, + _ignoreCheck = function _ignoreCheck(e, isPointerOrTouch) { + return (self.event = e) && ignore && ~ignore.indexOf(e.target) || isPointerOrTouch && limitToTouch && e.pointerType !== "touch" || ignoreCheck && ignoreCheck(e, isPointerOrTouch); + }, + onStopFunc = function onStopFunc() { + self._vx.reset(); + + self._vy.reset(); + + onStopDelayedCall.pause(); + onStop && onStop(self); + }, + update = function update() { + var dx = self.deltaX = _getAbsoluteMax(deltaX), + dy = self.deltaY = _getAbsoluteMax(deltaY), + changedX = Math.abs(dx) >= tolerance, + changedY = Math.abs(dy) >= tolerance; + + onChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); // in ScrollTrigger.normalizeScroll(), we need to know if it was touch/pointer so we need access to the deltaX/deltaY Arrays before we clear them out. + + if (changedX) { + onRight && self.deltaX > 0 && onRight(self); + onLeft && self.deltaX < 0 && onLeft(self); + onChangeX && onChangeX(self); + onToggleX && self.deltaX < 0 !== prevDeltaX < 0 && onToggleX(self); + prevDeltaX = self.deltaX; + deltaX[0] = deltaX[1] = deltaX[2] = 0; + } + + if (changedY) { + onDown && self.deltaY > 0 && onDown(self); + onUp && self.deltaY < 0 && onUp(self); + onChangeY && onChangeY(self); + onToggleY && self.deltaY < 0 !== prevDeltaY < 0 && onToggleY(self); + prevDeltaY = self.deltaY; + deltaY[0] = deltaY[1] = deltaY[2] = 0; + } + + if (moved || dragged) { + onMove && onMove(self); + + if (dragged) { + onDragStart && dragged === 1 && onDragStart(self); + onDrag && onDrag(self); + dragged = 0; + } + + moved = false; + } + + locked && !(locked = false) && onLockAxis && onLockAxis(self); + + if (wheeled) { + onWheel(self); + wheeled = false; + } + + id = 0; + }, + onDelta = function onDelta(x, y, index) { + deltaX[index] += x; + deltaY[index] += y; + + self._vx.update(x); + + self._vy.update(y); + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + onTouchOrPointerDelta = function onTouchOrPointerDelta(x, y) { + if (lockAxis && !axis) { + self.axis = axis = Math.abs(x) > Math.abs(y) ? "x" : "y"; + locked = true; + } + + if (axis !== "y") { + deltaX[2] += x; + + self._vx.update(x, true); // update the velocity as frequently as possible instead of in the debounced function so that very quick touch-scrolls (flicks) feel natural. If it's the mouse/touch/pointer, force it so that we get snappy/accurate momentum scroll. + + } + + if (axis !== "x") { + deltaY[2] += y; + + self._vy.update(y, true); + } + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + _onDrag = function _onDrag(e) { + if (_ignoreCheck(e, 1)) { + return; + } + + e = _getEvent(e, preventDefault); + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y, + isDragging = self.isDragging; + self.x = x; + self.y = y; + + if (isDragging || (dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum)) { + dragged = isDragging ? 2 : 1; // dragged: 0 = not dragging, 1 = first drag, 2 = normal drag + + isDragging || (self.isDragging = true); + onTouchOrPointerDelta(dx, dy); + } + }, + _onPress = self.onPress = function (e) { + if (_ignoreCheck(e, 1) || e && e.button) { + return; + } + + self.axis = axis = null; + onStopDelayedCall.pause(); + self.isPressed = true; + e = _getEvent(e); // note: may need to preventDefault(?) Won't side-scroll on iOS Safari if we do, though. + + prevDeltaX = prevDeltaY = 0; + self.startX = self.x = e.clientX; + self.startY = self.y = e.clientY; + + self._vx.reset(); // otherwise the t2 may be stale if the user touches and flicks super fast and releases in less than 2 requestAnimationFrame ticks, causing velocity to be 0. + + + self._vy.reset(); + + _addListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true); + + self.deltaX = self.deltaY = 0; + onPress && onPress(self); + }, + _onRelease = self.onRelease = function (e) { + if (_ignoreCheck(e, 1)) { + return; + } + + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + + var isTrackingDrag = !isNaN(self.y - self.startY), + wasDragging = self.isDragging, + isDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), + // some touch devices need some wiggle room in terms of sensing clicks - the finger may move a few pixels. + eventData = _getEvent(e); + + if (!isDragNotClick && isTrackingDrag) { + self._vx.reset(); + + self._vy.reset(); //if (preventDefault && allowClicks && self.isPressed) { // check isPressed because in a rare edge case, the inputObserver in ScrollTrigger may stopPropagation() on the press/drag, so the onRelease may get fired without the onPress/onDrag ever getting called, thus it could trigger a click to occur on a link after scroll-dragging it. + + + if (preventDefault && allowClicks) { + gsap.delayedCall(0.08, function () { + // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular "click" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the "real"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the "real" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched. + if (_getTime() - onClickTime > 300 && !e.defaultPrevented) { + if (e.target.click) { + //some browsers (like mobile Safari) don't properly trigger the click event + e.target.click(); + } else if (ownerDoc.createEvent) { + var syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null); + e.target.dispatchEvent(syntheticEvent); + } + } + }); + } + } + + self.isDragging = self.isGesturing = self.isPressed = false; + onStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true); + dragged && update(); // in case debouncing, we don't want onDrag to fire AFTER onDragEnd(). + + onDragEnd && wasDragging && onDragEnd(self); + onRelease && onRelease(self, isDragNotClick); + }, + _onGestureStart = function _onGestureStart(e) { + return e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging); + }, + _onGestureEnd = function _onGestureEnd() { + return (self.isGesturing = false) || onGestureEnd(self); + }, + onScroll = function onScroll(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = scrollFuncX(), + y = scrollFuncY(); + onDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1); + scrollX = x; + scrollY = y; + onStop && onStopDelayedCall.restart(true); + }, + _onWheel = function _onWheel(e) { + if (_ignoreCheck(e)) { + return; + } + + e = _getEvent(e, preventDefault); + onWheel && (wheeled = true); + var multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win.innerHeight : 1) * wheelSpeed; + onDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0); + onStop && !isNormalizer && onStopDelayedCall.restart(true); + }, + _onMove = function _onMove(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y; + self.x = x; + self.y = y; + moved = true; + onStop && onStopDelayedCall.restart(true); + (dx || dy) && onTouchOrPointerDelta(dx, dy); + }, + _onHover = function _onHover(e) { + self.event = e; + onHover(self); + }, + _onHoverEnd = function _onHoverEnd(e) { + self.event = e; + onHoverEnd(self); + }, + _onClick = function _onClick(e) { + return _ignoreCheck(e) || _getEvent(e, preventDefault) && onClick(self); + }; + + onStopDelayedCall = self._dc = gsap.delayedCall(onStopDelay || 0.25, onStopFunc).pause(); + self.deltaX = self.deltaY = 0; + self._vx = _getVelocityProp(0, 50, true); + self._vy = _getVelocityProp(0, 50, true); + self.scrollX = scrollFuncX; + self.scrollY = scrollFuncY; + self.isDragging = self.isGesturing = self.isPressed = false; + + _context(this); + + self.enable = function (e) { + if (!self.isEnabled) { + _addListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + + type.indexOf("scroll") >= 0 && _addListener(isViewport ? ownerDoc : target, "scroll", onScroll, passive, capture); + type.indexOf("wheel") >= 0 && _addListener(target, "wheel", _onWheel, passive, capture); + + if (type.indexOf("touch") >= 0 && _isTouch || type.indexOf("pointer") >= 0) { + _addListener(target, _eventTypes[0], _onPress, passive, capture); + + _addListener(ownerDoc, _eventTypes[2], _onRelease); + + _addListener(ownerDoc, _eventTypes[3], _onRelease); + + allowClicks && _addListener(target, "click", clickCapture, true, true); + onClick && _addListener(target, "click", _onClick); + onGestureStart && _addListener(ownerDoc, "gesturestart", _onGestureStart); + onGestureEnd && _addListener(ownerDoc, "gestureend", _onGestureEnd); + onHover && _addListener(target, _pointerType + "enter", _onHover); + onHoverEnd && _addListener(target, _pointerType + "leave", _onHoverEnd); + onMove && _addListener(target, _pointerType + "move", _onMove); + } + + self.isEnabled = true; + self.isDragging = self.isGesturing = self.isPressed = moved = dragged = false; + + self._vx.reset(); + + self._vy.reset(); + + scrollX = scrollFuncX(); + scrollY = scrollFuncY(); + e && e.type && _onPress(e); + onEnable && onEnable(self); + } + + return self; + }; + + self.disable = function () { + if (self.isEnabled) { + // only remove the _onScroll listener if there aren't any others that rely on the functionality. + _observers.filter(function (o) { + return o !== self && _isViewport(o.target); + }).length || _removeListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + + if (self.isPressed) { + self._vx.reset(); + + self._vy.reset(); + + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + } + + _removeListener(isViewport ? ownerDoc : target, "scroll", onScroll, capture); + + _removeListener(target, "wheel", _onWheel, capture); + + _removeListener(target, _eventTypes[0], _onPress, capture); + + _removeListener(ownerDoc, _eventTypes[2], _onRelease); + + _removeListener(ownerDoc, _eventTypes[3], _onRelease); + + _removeListener(target, "click", clickCapture, true); + + _removeListener(target, "click", _onClick); + + _removeListener(ownerDoc, "gesturestart", _onGestureStart); + + _removeListener(ownerDoc, "gestureend", _onGestureEnd); + + _removeListener(target, _pointerType + "enter", _onHover); + + _removeListener(target, _pointerType + "leave", _onHoverEnd); + + _removeListener(target, _pointerType + "move", _onMove); + + self.isEnabled = self.isPressed = self.isDragging = false; + onDisable && onDisable(self); + } + }; + + self.kill = self.revert = function () { + self.disable(); + + var i = _observers.indexOf(self); + + i >= 0 && _observers.splice(i, 1); + _normalizer === self && (_normalizer = 0); + }; + + _observers.push(self); + + isNormalizer && _isViewport(target) && (_normalizer = self); + self.enable(event); + }; + + _createClass(Observer, [{ + key: "velocityX", + get: function get() { + return this._vx.getVelocity(); + } + }, { + key: "velocityY", + get: function get() { + return this._vy.getVelocity(); + } + }]); + + return Observer; +}(); +Observer.version = "3.12.7"; + +Observer.create = function (vars) { + return new Observer(vars); +}; + +Observer.register = _initCore; + +Observer.getAll = function () { + return _observers.slice(); +}; + +Observer.getById = function (id) { + return _observers.filter(function (o) { + return o.vars.id === id; + })[0]; +}; + +_getGSAP() && gsap.registerPlugin(Observer); +export { Observer as default, _isViewport, _scrollers, _getScrollFunc, _getProxyProp, _proxies, _getVelocityProp, _vertical, _horizontal, _getTarget }; \ No newline at end of file diff --git a/node_modules/gsap/PixiPlugin.js b/node_modules/gsap/PixiPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..2796b909027b797f2b5076978a56437f579335cf --- /dev/null +++ b/node_modules/gsap/PixiPlugin.js @@ -0,0 +1,471 @@ +/*! + * PixiPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var gsap, + _splitColor, + _coreInitted, + _PIXI, + PropTween, + _getSetter, + _isV4, + _isV8Plus, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _isFunction = function _isFunction(value) { + return typeof value === "function"; +}, + _warn = function _warn(message) { + return console.warn(message); +}, + _idMatrix = [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + _filterClass = function _filterClass(name) { + return _isFunction(_PIXI[name]) ? _PIXI[name] : _PIXI.filters[name]; +}, + // in PIXI 7.1, filters moved from PIXI.filters to just PIXI +_applyMatrix = function _applyMatrix(m, m2) { + var temp = [], + i = 0, + z = 0, + y, + x; + + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = x === 4 ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i + 1] * m2[x + 5] + m[i + 2] * m2[x + 10] + m[i + 3] * m2[x + 15] + z; + } + + i += 5; + } + + return temp; +}, + _setSaturation = function _setSaturation(m, n) { + var inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); +}, + _colorize = function _colorize(m, color, amount) { + var c = _splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); +}, + _setHue = function _setHue(m, n) { + n *= Math.PI / 180; + var c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([_lumR + c * (1 - _lumR) + s * -_lumR, _lumG + c * -_lumG + s * -_lumG, _lumB + c * -_lumB + s * (1 - _lumB), 0, 0, _lumR + c * -_lumR + s * 0.143, _lumG + c * (1 - _lumG) + s * 0.14, _lumB + c * -_lumB + s * -0.283, 0, 0, _lumR + c * -_lumR + s * -(1 - _lumR), _lumG + c * -_lumG + s * _lumG, _lumB + c * (1 - _lumB) + s * _lumB, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); +}, + _setContrast = function _setContrast(m, n) { + return _applyMatrix([n, 0, 0, 0, 0.5 * (1 - n), 0, n, 0, 0, 0.5 * (1 - n), 0, 0, n, 0, 0.5 * (1 - n), 0, 0, 0, 1, 0], m); +}, + _getFilter = function _getFilter(target, type) { + var filterClass = _filterClass(type), + filters = target.filters || [], + i = filters.length, + filter; + + filterClass || _warn(type + " not found. PixiPlugin.registerPIXI(PIXI)"); + + while (--i > -1) { + if (filters[i] instanceof filterClass) { + return filters[i]; + } + } + + filter = new filterClass(); + + if (type === "BlurFilter") { + filter.blur = 0; + } + + filters.push(filter); + target.filters = filters; + return filter; +}, + _addColorMatrixFilterCacheTween = function _addColorMatrixFilterCacheTween(p, plugin, cache, vars) { + //we cache the ColorMatrixFilter components in a _gsColorMatrixFilter object attached to the target object so that it's easy to grab the current value at any time. + plugin.add(cache, p, cache[p], vars[p]); + + plugin._props.push(p); +}, + _applyBrightnessToMatrix = function _applyBrightnessToMatrix(brightness, matrix) { + var filterClass = _filterClass("ColorMatrixFilter"), + temp = new filterClass(); + + temp.matrix = matrix; + temp.brightness(brightness, true); + return temp.matrix; +}, + _copy = function _copy(obj) { + var copy = {}, + p; + + for (p in obj) { + copy[p] = obj[p]; + } + + return copy; +}, + _CMFdefaults = { + contrast: 1, + saturation: 1, + colorizeAmount: 0, + colorize: "rgb(255,255,255)", + hue: 0, + brightness: 1 +}, + _parseColorMatrixFilter = function _parseColorMatrixFilter(target, v, pg) { + var filter = _getFilter(target, "ColorMatrixFilter"), + cache = target._gsColorMatrixFilter = target._gsColorMatrixFilter || _copy(_CMFdefaults), + combine = v.combineCMF && !("colorMatrixFilter" in v && !v.colorMatrixFilter), + i, + matrix, + startMatrix; + + startMatrix = filter.matrix; + + if (v.resolution) { + filter.resolution = v.resolution; + } + + if (v.matrix && v.matrix.length === startMatrix.length) { + matrix = v.matrix; + + if (cache.contrast !== 1) { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + + if (cache.hue) { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + + if (cache.brightness !== 1) { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + + if (cache.colorizeAmount) { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + + if (cache.saturation !== 1) { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } else { + matrix = _idMatrix.slice(); + + if (v.contrast != null) { + matrix = _setContrast(matrix, +v.contrast); + + _addColorMatrixFilterCacheTween("contrast", pg, cache, v); + } else if (cache.contrast !== 1) { + if (combine) { + matrix = _setContrast(matrix, cache.contrast); + } else { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + } + + if (v.hue != null) { + matrix = _setHue(matrix, +v.hue); + + _addColorMatrixFilterCacheTween("hue", pg, cache, v); + } else if (cache.hue) { + if (combine) { + matrix = _setHue(matrix, cache.hue); + } else { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + } + + if (v.brightness != null) { + matrix = _applyBrightnessToMatrix(+v.brightness, matrix); + + _addColorMatrixFilterCacheTween("brightness", pg, cache, v); + } else if (cache.brightness !== 1) { + if (combine) { + matrix = _applyBrightnessToMatrix(cache.brightness, matrix); + } else { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + } + + if (v.colorize != null) { + v.colorizeAmount = "colorizeAmount" in v ? +v.colorizeAmount : 1; + matrix = _colorize(matrix, v.colorize, v.colorizeAmount); + + _addColorMatrixFilterCacheTween("colorize", pg, cache, v); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, v); + } else if (cache.colorizeAmount) { + if (combine) { + matrix = _colorize(matrix, cache.colorize, cache.colorizeAmount); + } else { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + } + + if (v.saturation != null) { + matrix = _setSaturation(matrix, +v.saturation); + + _addColorMatrixFilterCacheTween("saturation", pg, cache, v); + } else if (cache.saturation !== 1) { + if (combine) { + matrix = _setSaturation(matrix, cache.saturation); + } else { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } + } + + i = matrix.length; + + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg.add(startMatrix, i, startMatrix[i], matrix[i], "colorMatrixFilter"); + } + } + + pg._props.push("colorMatrixFilter"); +}, + _renderColor = function _renderColor(ratio, _ref) { + var t = _ref.t, + p = _ref.p, + color = _ref.color, + set = _ref.set; + set(t, p, color[0] << 16 | color[1] << 8 | color[2]); +}, + _renderDirtyCache = function _renderDirtyCache(ratio, _ref2) { + var g = _ref2.g; + + if (_isV8Plus) { + g.fill(); + g.stroke(); + } else if (g) { + // in order for PixiJS to actually redraw GraphicsData, we've gotta increment the "dirty" and "clearDirty" values. If we don't do this, the values will be tween properly, but not rendered. + g.dirty++; + g.clearDirty++; + } +}, + _renderAutoAlpha = function _renderAutoAlpha(ratio, data) { + data.t.visible = !!data.t.alpha; +}, + _addColorTween = function _addColorTween(target, p, value, plugin) { + var currentValue = target[p], + startColor = _splitColor(_isFunction(currentValue) ? target[p.indexOf("set") || !_isFunction(target["get" + p.substr(3)]) ? p : "get" + p.substr(3)]() : currentValue), + endColor = _splitColor(value); + + plugin._pt = new PropTween(plugin._pt, target, p, 0, 0, _renderColor, { + t: target, + p: p, + color: startColor, + set: _getSetter(target, p) + }); + plugin.add(startColor, 0, startColor[0], endColor[0]); + plugin.add(startColor, 1, startColor[1], endColor[1]); + plugin.add(startColor, 2, startColor[2], endColor[2]); +}, + _colorProps = { + tint: 1, + lineColor: 1, + fillColor: 1, + strokeColor: 1 +}, + _xyContexts = "position,scale,skew,pivot,anchor,tilePosition,tileScale".split(","), + _contexts = { + x: "position", + y: "position", + tileX: "tilePosition", + tileY: "tilePosition" +}, + _colorMatrixFilterProps = { + colorMatrixFilter: 1, + saturation: 1, + contrast: 1, + hue: 1, + colorize: 1, + colorizeAmount: 1, + brightness: 1, + combineCMF: 1 +}, + _DEG2RAD = Math.PI / 180, + _isString = function _isString(value) { + return typeof value === "string"; +}, + _degreesToRadians = function _degreesToRadians(value) { + return _isString(value) && value.charAt(1) === "=" ? value.substr(0, 2) + parseFloat(value.substr(2)) * _DEG2RAD : value * _DEG2RAD; +}, + _renderPropWithEnd = function _renderPropWithEnd(ratio, data) { + return data.set(data.t, data.p, ratio === 1 ? data.e : Math.round((data.s + data.c * ratio) * 100000) / 100000, data); +}, + _addRotationalPropTween = function _addRotationalPropTween(plugin, target, property, startNum, endValue, radians) { + var cap = 360 * (radians ? _DEG2RAD : 1), + isString = _isString(endValue), + relative = isString && endValue.charAt(1) === "=" ? +(endValue.charAt(0) + "1") : 0, + endNum = parseFloat(relative ? endValue.substr(2) : endValue) * (radians ? _DEG2RAD : 1), + change = relative ? endNum * relative : endNum - startNum, + finalValue = startNum + change, + direction, + pt; + + if (isString) { + direction = endValue.split("_")[1]; + + if (direction === "short") { + change %= cap; + + if (change !== change % (cap / 2)) { + change += change < 0 ? cap : -cap; + } + } + + if (direction === "cw" && change < 0) { + change = (change + cap * 1e10) % cap - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = (change - cap * 1e10) % cap - ~~(change / cap) * cap; + } + } + + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + return pt; +}, + _initCore = function _initCore() { + if (!_coreInitted) { + gsap = _getGSAP(); + _PIXI = _coreInitted = _PIXI || _windowExists() && window.PIXI; + var version = _PIXI && _PIXI.VERSION && parseFloat(_PIXI.VERSION.split(".")[0]) || 0; + _isV4 = version === 4; + _isV8Plus = version >= 8; + + _splitColor = function _splitColor(color) { + return gsap.utils.splitColor((color + "").substr(0, 2) === "0x" ? "#" + color.substr(2) : color); + }; // some colors in PIXI are reported as "0xFF4421" instead of "#FF4421". + + } +}, + i, + p; //context setup... + + +for (i = 0; i < _xyContexts.length; i++) { + p = _xyContexts[i]; + _contexts[p + "X"] = p; + _contexts[p + "Y"] = p; +} + +export var PixiPlugin = { + version: "3.12.7", + name: "pixi", + register: function register(core, Plugin, propTween) { + gsap = core; + PropTween = propTween; + _getSetter = Plugin.getSetter; + + _initCore(); + }, + headless: true, + // doesn't need window + registerPIXI: function registerPIXI(pixi) { + _PIXI = pixi; + }, + init: function init(target, values, tween, index, targets) { + _PIXI || _initCore(); + + if (!_PIXI) { + _warn("PIXI was not found. PixiPlugin.registerPIXI(PIXI);"); + + return false; + } + + var context, axis, value, colorMatrix, filter, p, padding, i, data, subProp; + + for (p in values) { + context = _contexts[p]; + value = values[p]; + + if (context) { + axis = ~p.charAt(p.length - 1).toLowerCase().indexOf("x") ? "x" : "y"; + this.add(target[context], axis, target[context][axis], context === "skew" ? _degreesToRadians(value) : value, 0, 0, 0, 0, 0, 1); + } else if (p === "scale" || p === "anchor" || p === "pivot" || p === "tileScale") { + this.add(target[p], "x", target[p].x, value); + this.add(target[p], "y", target[p].y, value); + } else if (p === "rotation" || p === "angle") { + //PIXI expects rotation in radians, but as a convenience we let folks define it in degrees and we do the conversion. + _addRotationalPropTween(this, target, p, target[p], value, p === "rotation"); + } else if (_colorMatrixFilterProps[p]) { + if (!colorMatrix) { + _parseColorMatrixFilter(target, values.colorMatrixFilter || values, this); + + colorMatrix = true; + } + } else if (p === "blur" || p === "blurX" || p === "blurY" || p === "blurPadding") { + filter = _getFilter(target, "BlurFilter"); + this.add(filter, p, filter[p], value); + + if (values.blurPadding !== 0) { + padding = values.blurPadding || Math.max(filter[p], value) * 2; + i = target.filters.length; + + while (--i > -1) { + target.filters[i].padding = Math.max(target.filters[i].padding, padding); //if we don't expand the padding on all the filters, it can look clipped. + } + } + } else if (_colorProps[p]) { + if ((p === "lineColor" || p === "fillColor" || p === "strokeColor") && target instanceof _PIXI.Graphics) { + data = "fillStyle" in target ? [target] : (target.geometry || target).graphicsData; //"geometry" was introduced in PIXI version 5 + + subProp = p.substr(0, p.length - 5); + _isV8Plus && subProp === "line" && (subProp = "stroke"); // in v8, lineColor became strokeColor. + + this._pt = new PropTween(this._pt, target, p, 0, 0, _renderDirtyCache, { + g: target.geometry || target + }); + i = data.length; + + while (--i > -1) { + _addColorTween(_isV4 ? data[i] : data[i][subProp + "Style"], _isV4 ? p : "color", value, this); + } + } else { + _addColorTween(target, p, value, this); + } + } else if (p === "autoAlpha") { + this._pt = new PropTween(this._pt, target, "visible", 0, 0, _renderAutoAlpha); + this.add(target, "alpha", target.alpha, value); + + this._props.push("alpha", "visible"); + } else if (p !== "resolution") { + this.add(target, p, "get", value); + } + + this._props.push(p); + } + } +}; +_getGSAP() && gsap.registerPlugin(PixiPlugin); +export { PixiPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/README.md b/node_modules/gsap/README.md new file mode 100644 index 0000000000000000000000000000000000000000..3a02dea3b92bd9171c190c2c2636a51d8d4e3aeb --- /dev/null +++ b/node_modules/gsap/README.md @@ -0,0 +1,104 @@ +# GSAP (GreenSock Animation Platform) + +[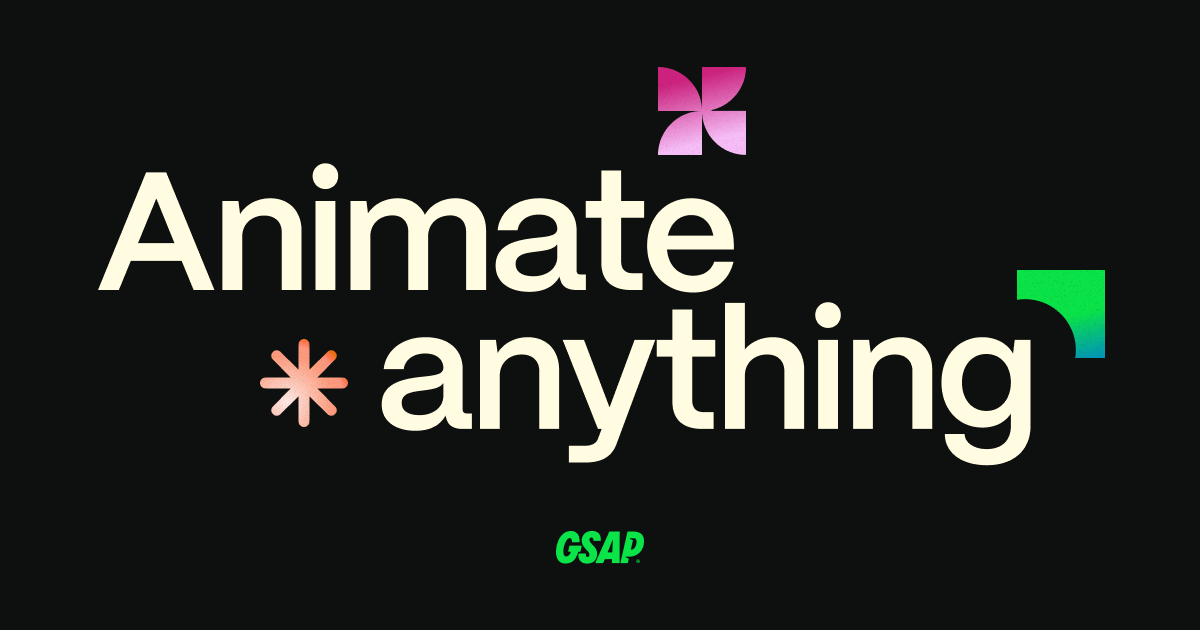](http://gsap.com) + +GSAP is a **framework-agnostic** JavaScript animation library that turns developers into animation superheroes. Build high-performance animations that work in **every** major browser. Animate CSS, SVG, canvas, React, Vue, WebGL, colors, strings, motion paths, generic objects...anything JavaScript can touch! GSAP's <a href="https://gsap.com/docs/v3/Plugins/ScrollTrigger/">ScrollTrigger</a> plugin delivers jaw-dropping scroll-based animations with minimal code. <a href="https://gsap.com/docs/v3/GSAP/gsap.matchMedia()">gsap.matchMedia()</a> makes building responsive, accessibility-friendly animations a breeze. + +No other library delivers such advanced sequencing, reliability, and tight control while solving real-world problems on over 12 million sites. GSAP works around countless browser inconsistencies; your animations ***just work***. At its core, GSAP is a high-speed property manipulator, updating values over time with extreme accuracy. It's up to 20x faster than jQuery! + +GSAP is completely flexible; sprinkle it wherever you want. **Zero dependencies.** + +There are many optional <a href="https://gsap.com/docs/v3/Plugins">plugins</a> and <a href="https://gsap.com/docs/v3/Eases">easing</a> functions for achieving advanced effects easily like <a href="https://gsap.com/docs/v3/Plugins/ScrollTrigger/">scrolling</a>, <a href="https://gsap.com/docs/v3/Plugins/MorphSVGPlugin">morphing</a>, animating along a <a href="https://gsap.com/docs/v3/Plugins/MotionPathPlugin">motion path</a> or <a href="https://gsap.com/docs/v3/Plugins/Flip/">FLIP</a> animations. There's even a handy <a href="https://gsap.com/docs/v3/Plugins/Observer/">Observer</a> for normalizing event detection across browsers/devices. + + +### Get Started + +[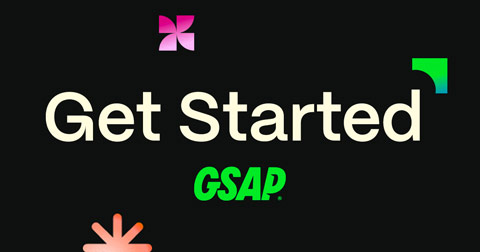](http://gsap.com/get-started) + + +## Docs & Installation + +View the <a href="https://gsap.com/docs">full documentation here</a>, including an <a href="https://gsap.com/install">installation guide</a>. + +### CDN + +```html +<script src="https://cdn.jsdelivr.net/npm/gsap@3.12/dist/gsap.min.js"></script> +``` + +See <a href="https://www.jsdelivr.com/gsap">JSDelivr's dedicated GSAP page</a> for quick CDN links to the core files/plugins. There are more <a href="https://gsap.com/install">installation instructions</a> at gsap.com. + +**Every major ad network excludes GSAP from file size calculations** and most have it on their own CDNs, so contact them for the appropriate URL(s). + +### NPM +See the <a href="https://gsap.com/install">guide to using GSAP via NPM here</a>. + +```javascript +npm install gsap +``` + +GSAP's core can animate almost anything including CSS and attributes, plus it includes all of the <a href="https://gsap.com/docs/v3/GSAP/UtilityMethods">utility methods</a> like <a href="https://gsap.com/docs/v3/GSAP/UtilityMethods/interpolate()">interpolate()</a>, <a href="https://gsap.com/docs/v3/GSAP/UtilityMethods/mapRange()">mapRange()</a>, most of the <a href="https://gsap.com/docs/v3/Eases">eases</a>, and it can do snapping and modifiers. + +```javascript +// typical import +import gsap from "gsap"; + +// get other plugins: +import ScrollTrigger from "gsap/ScrollTrigger"; +import Flip from "gsap/Flip"; +import Draggable from "gsap/Draggable"; + +// or all tools are exported from the "all" file (excluding members-only plugins): +import { gsap, ScrollTrigger, Draggable, MotionPathPlugin } from "gsap/all"; + +// don't forget to register plugins +gsap.registerPlugin(ScrollTrigger, Draggable, Flip, MotionPathPlugin); +``` + +The NPM files are ES modules, but there's also a /dist/ directory with <a href="https://www.davidbcalhoun.com/2014/what-is-amd-commonjs-and-umd/">UMD</a> files for extra compatibility. + +Download <a href="https://gsap.com/pricing/">Club GSAP</a> members-only plugins from your gsap.com account and then include them in your own JS payload. There's even a <a href="https://www.youtube.com/watch?v=znVi89_gazE">tarball file you can install with NPM/Yarn</a>. GSAP has a <a href="https://gsap.com/docs/v3/Installation#private">private NPM registry</a> for members too. Post questions in our <a href="https://gsap.com/community/">forums</a> and we'd be happy to help. + +### ScrollTrigger & ScrollSmoother + +If you're looking for scroll-driven animations, GSAP's <a href="https://gsap.com/docs/v3/Plugins/ScrollTrigger/">ScrollTrigger</a> plugin is the new standard. There's a companion <a href="https://gsap.com/docs/v3/Plugins/ScrollSmoother/">ScrollSmoother</a> as well. + +[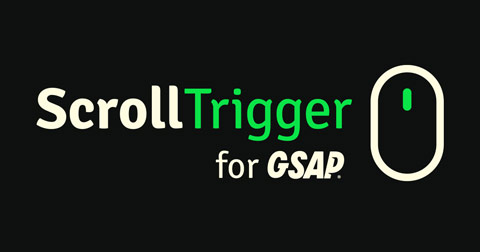](https://gsap.com/docs/v3/Plugins/ScrollTrigger) + +### Using React? + +There's a <a href="https://www.npmjs.com/package/@gsap/react">@gsap/react</a> package that exposes a `useGSAP()` hook which is a drop-in replacement for `useEffect()`/`useLayoutEffect()`, automating cleanup tasks. Please read the <a href="https://gsap.com/react">React guide</a> for details. + +### Resources + +* <a href="https://gsap.com/">gsap.com</a> +* <a href="https://gsap.com/get-started/">Getting started guide</a> +* <a href="https://gsap.com/docs/">Docs</a> +* <a href="https://gsap.com/demos">Demos & starter templates</a> +* <a href="https://gsap.com/community/">Community forums</a> +* <a href="https://gsap.com/docs/v3/Eases">Ease Visualizer</a> +* <a href="https://gsap.com/showcase">Showcase</a> +* <a href="https://www.youtube.com/@GreenSockLearning">YouTube Channel</a> +* <a href="https://gsap.com/cheatsheet">Cheat sheet</a> +* <a href="https://gsap.com/trial">Try bonus plugins for free</a> +* <a href="https://gsap.com/pricing/">Club GSAP</a> (get access to unrestricted bonus plugins that are not in this repository) + +### What is Club GSAP? + +There are 3 main reasons anyone signs up for <a href="https://gsap.com/pricing">Club GSAP</a>: +* To get access to snazzy <a href="https://gsap.com/pricing">members-only plugins</a> like MorphSVG, SplitText, ScrollSmoother, etc. +* To get the special <a href="https://gsap.com/licensing/">commercial license</a>. +* To support ongoing development efforts and **cheer us on**. + +<a href="https://gsap.com/pricing/">Learn more</a>. + +### Try all bonus plugins for free! +<a href="https://gsap.com/trial">https://gsap.com/trial</a> + +### Need help? +Ask in the friendly <a href="https://gsap.com/community/">GSAP forums</a>. Or share your knowledge and help someone else - it's a great way to sharpen your skills! Report any bugs there too (or <a href="https://github.com/greensock/GSAP/issues">file an issue here</a> if you prefer). + +### License +GreenSock's standard "no charge" license can be viewed at <a href="https://gsap.com/standard-license">https://gsap.com/standard-license</a>. <a href="https://gsap.com/pricing/">Club GSAP</a> members are granted additional rights. See <a href="https://gsap.com/licensing/">https://gsap.com/licensing/</a> for details. Why doesn't GSAP use an MIT (or similar) open source license, and why is that a **good** thing? This article explains it all: <a href="https://gsap.com/why-license/" target="_blank">https://gsap.com/why-license/</a> + +Copyright (c) 2008-2025, GreenSock Inc. All rights reserved. \ No newline at end of file diff --git a/node_modules/gsap/SECURITY.md b/node_modules/gsap/SECURITY.md new file mode 100644 index 0000000000000000000000000000000000000000..147c41b4ab788a07d5ec71506d1ac489a65e8776 --- /dev/null +++ b/node_modules/gsap/SECURITY.md @@ -0,0 +1,6 @@ +# Security Policy + +Please report (suspected) security vulnerabilities to +**[info@greensock.com](mailto:info@greensock.com)**. You will receive a response from +us within 72 hours. If the issue is confirmed, we will release a patch as soon +as possible depending on complexity. \ No newline at end of file diff --git a/node_modules/gsap/ScrollToPlugin.js b/node_modules/gsap/ScrollToPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..3e21ae660714bb2020db280dc442358fc44b92f3 --- /dev/null +++ b/node_modules/gsap/ScrollToPlugin.js @@ -0,0 +1,282 @@ +/*! + * ScrollToPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var gsap, + _coreInitted, + _window, + _docEl, + _body, + _toArray, + _config, + ScrollTrigger, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _isString = function _isString(value) { + return typeof value === "string"; +}, + _isFunction = function _isFunction(value) { + return typeof value === "function"; +}, + _max = function _max(element, axis) { + var dim = axis === "x" ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return element === _window || element === _docEl || element === _body ? Math.max(_docEl[scroll], _body[scroll]) - (_window["inner" + dim] || _docEl[client] || _body[client]) : element[scroll] - element["offset" + dim]; +}, + _buildGetter = function _buildGetter(e, axis) { + //pass in an element and an axis ("x" or "y") and it'll return a getter function for the scroll position of that element (like scrollTop or scrollLeft, although if the element is the window, it'll use the pageXOffset/pageYOffset or the documentElement's scrollTop/scrollLeft or document.body's. Basically this streamlines things and makes a very fast getter across browsers. + var p = "scroll" + (axis === "x" ? "Left" : "Top"); + + if (e === _window) { + if (e.pageXOffset != null) { + p = "page" + axis.toUpperCase() + "Offset"; + } else { + e = _docEl[p] != null ? _docEl : _body; + } + } + + return function () { + return e[p]; + }; +}, + _clean = function _clean(value, index, target, targets) { + _isFunction(value) && (value = value(index, target, targets)); + + if (typeof value !== "object") { + return _isString(value) && value !== "max" && value.charAt(1) !== "=" ? { + x: value, + y: value + } : { + y: value + }; //if we don't receive an object as the parameter, assume the user intends "y". + } else if (value.nodeType) { + return { + y: value, + x: value + }; + } else { + var result = {}, + p; + + for (p in value) { + result[p] = p !== "onAutoKill" && _isFunction(value[p]) ? value[p](index, target, targets) : value[p]; + } + + return result; + } +}, + _getOffset = function _getOffset(element, container) { + element = _toArray(element)[0]; + + if (!element || !element.getBoundingClientRect) { + return console.warn("scrollTo target doesn't exist. Using 0") || { + x: 0, + y: 0 + }; + } + + var rect = element.getBoundingClientRect(), + isRoot = !container || container === _window || container === _body, + cRect = isRoot ? { + top: _docEl.clientTop - (_window.pageYOffset || _docEl.scrollTop || _body.scrollTop || 0), + left: _docEl.clientLeft - (_window.pageXOffset || _docEl.scrollLeft || _body.scrollLeft || 0) + } : container.getBoundingClientRect(), + offsets = { + x: rect.left - cRect.left, + y: rect.top - cRect.top + }; + + if (!isRoot && container) { + //only add the current scroll position if it's not the window/body. + offsets.x += _buildGetter(container, "x")(); + offsets.y += _buildGetter(container, "y")(); + } + + return offsets; +}, + _parseVal = function _parseVal(value, target, axis, currentVal, offset) { + return !isNaN(value) && typeof value !== "object" ? parseFloat(value) - offset : _isString(value) && value.charAt(1) === "=" ? parseFloat(value.substr(2)) * (value.charAt(0) === "-" ? -1 : 1) + currentVal - offset : value === "max" ? _max(target, axis) - offset : Math.min(_max(target, axis), _getOffset(value, target)[axis] - offset); +}, + _initCore = function _initCore() { + gsap = _getGSAP(); + + if (_windowExists() && gsap && typeof document !== "undefined" && document.body) { + _window = window; + _body = document.body; + _docEl = document.documentElement; + _toArray = gsap.utils.toArray; + gsap.config({ + autoKillThreshold: 7 + }); + _config = gsap.config(); + _coreInitted = 1; + } +}; + +export var ScrollToPlugin = { + version: "3.12.7", + name: "scrollTo", + rawVars: 1, + register: function register(core) { + gsap = core; + + _initCore(); + }, + init: function init(target, value, tween, index, targets) { + _coreInitted || _initCore(); + var data = this, + snapType = gsap.getProperty(target, "scrollSnapType"); + data.isWin = target === _window; + data.target = target; + data.tween = tween; + value = _clean(value, index, target, targets); + data.vars = value; + data.autoKill = !!("autoKill" in value ? value : _config).autoKill; + data.getX = _buildGetter(target, "x"); + data.getY = _buildGetter(target, "y"); + data.x = data.xPrev = data.getX(); + data.y = data.yPrev = data.getY(); + ScrollTrigger || (ScrollTrigger = gsap.core.globals().ScrollTrigger); + gsap.getProperty(target, "scrollBehavior") === "smooth" && gsap.set(target, { + scrollBehavior: "auto" + }); + + if (snapType && snapType !== "none") { + // disable scroll snapping to avoid strange behavior + data.snap = 1; + data.snapInline = target.style.scrollSnapType; + target.style.scrollSnapType = "none"; + } + + if (value.x != null) { + data.add(data, "x", data.x, _parseVal(value.x, target, "x", data.x, value.offsetX || 0), index, targets); + + data._props.push("scrollTo_x"); + } else { + data.skipX = 1; + } + + if (value.y != null) { + data.add(data, "y", data.y, _parseVal(value.y, target, "y", data.y, value.offsetY || 0), index, targets); + + data._props.push("scrollTo_y"); + } else { + data.skipY = 1; + } + }, + render: function render(ratio, data) { + var pt = data._pt, + target = data.target, + tween = data.tween, + autoKill = data.autoKill, + xPrev = data.xPrev, + yPrev = data.yPrev, + isWin = data.isWin, + snap = data.snap, + snapInline = data.snapInline, + x, + y, + yDif, + xDif, + threshold; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + x = isWin || !data.skipX ? data.getX() : xPrev; + y = isWin || !data.skipY ? data.getY() : yPrev; + yDif = y - yPrev; + xDif = x - xPrev; + threshold = _config.autoKillThreshold; + + if (data.x < 0) { + //can't scroll to a position less than 0! Might happen if someone uses a Back.easeOut or Elastic.easeOut when scrolling back to the top of the page (for example) + data.x = 0; + } + + if (data.y < 0) { + data.y = 0; + } + + if (autoKill) { + //note: iOS has a bug that throws off the scroll by several pixels, so we need to check if it's within 7 pixels of the previous one that we set instead of just looking for an exact match. + if (!data.skipX && (xDif > threshold || xDif < -threshold) && x < _max(target, "x")) { + data.skipX = 1; //if the user scrolls separately, we should stop tweening! + } + + if (!data.skipY && (yDif > threshold || yDif < -threshold) && y < _max(target, "y")) { + data.skipY = 1; //if the user scrolls separately, we should stop tweening! + } + + if (data.skipX && data.skipY) { + tween.kill(); + data.vars.onAutoKill && data.vars.onAutoKill.apply(tween, data.vars.onAutoKillParams || []); + } + } + + if (isWin) { + _window.scrollTo(!data.skipX ? data.x : x, !data.skipY ? data.y : y); + } else { + data.skipY || (target.scrollTop = data.y); + data.skipX || (target.scrollLeft = data.x); + } + + if (snap && (ratio === 1 || ratio === 0)) { + y = target.scrollTop; + x = target.scrollLeft; + snapInline ? target.style.scrollSnapType = snapInline : target.style.removeProperty("scroll-snap-type"); + target.scrollTop = y + 1; // bug in Safari causes the element to totally reset its scroll position when scroll-snap-type changes, so we need to set it to a slightly different value and then back again to work around this bug. + + target.scrollLeft = x + 1; + target.scrollTop = y; + target.scrollLeft = x; + } + + data.xPrev = data.x; + data.yPrev = data.y; + ScrollTrigger && ScrollTrigger.update(); + }, + kill: function kill(property) { + var both = property === "scrollTo", + i = this._props.indexOf(property); + + if (both || property === "scrollTo_x") { + this.skipX = 1; + } + + if (both || property === "scrollTo_y") { + this.skipY = 1; + } + + i > -1 && this._props.splice(i, 1); + return !this._props.length; + } +}; +ScrollToPlugin.max = _max; +ScrollToPlugin.getOffset = _getOffset; +ScrollToPlugin.buildGetter = _buildGetter; + +ScrollToPlugin.config = function (vars) { + _config || _initCore() || (_config = gsap.config()); // in case the window hasn't been defined yet. + + for (var p in vars) { + _config[p] = vars[p]; + } +}; + +_getGSAP() && gsap.registerPlugin(ScrollToPlugin); +export { ScrollToPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/ScrollTrigger.js b/node_modules/gsap/ScrollTrigger.js new file mode 100644 index 0000000000000000000000000000000000000000..8f54c660ff511a3e6b0b09a98654aa7e895e0ea7 --- /dev/null +++ b/node_modules/gsap/ScrollTrigger.js @@ -0,0 +1,2684 @@ +/*! + * ScrollTrigger 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +import { Observer, _getTarget, _vertical, _horizontal, _scrollers, _proxies, _getScrollFunc, _getProxyProp, _getVelocityProp } from "./Observer.js"; + +var gsap, + _coreInitted, + _win, + _doc, + _docEl, + _body, + _root, + _resizeDelay, + _toArray, + _clamp, + _time2, + _syncInterval, + _refreshing, + _pointerIsDown, + _transformProp, + _i, + _prevWidth, + _prevHeight, + _autoRefresh, + _sort, + _suppressOverwrites, + _ignoreResize, + _normalizer, + _ignoreMobileResize, + _baseScreenHeight, + _baseScreenWidth, + _fixIOSBug, + _context, + _scrollRestoration, + _div100vh, + _100vh, + _isReverted, + _clampingMax, + _limitCallbacks, + // if true, we'll only trigger callbacks if the active state toggles, so if you scroll immediately past both the start and end positions of a ScrollTrigger (thus inactive to inactive), neither its onEnter nor onLeave will be called. This is useful during startup. +_startup = 1, + _getTime = Date.now, + _time1 = _getTime(), + _lastScrollTime = 0, + _enabled = 0, + _parseClamp = function _parseClamp(value, type, self) { + var clamp = _isString(value) && (value.substr(0, 6) === "clamp(" || value.indexOf("max") > -1); + self["_" + type + "Clamp"] = clamp; + return clamp ? value.substr(6, value.length - 7) : value; +}, + _keepClamp = function _keepClamp(value, clamp) { + return clamp && (!_isString(value) || value.substr(0, 6) !== "clamp(") ? "clamp(" + value + ")" : value; +}, + _rafBugFix = function _rafBugFix() { + return _enabled && requestAnimationFrame(_rafBugFix); +}, + // in some browsers (like Firefox), screen repaints weren't consistent unless we had SOMETHING queued up in requestAnimationFrame()! So this just creates a super simple loop to keep it alive and smooth out repaints. +_pointerDownHandler = function _pointerDownHandler() { + return _pointerIsDown = 1; +}, + _pointerUpHandler = function _pointerUpHandler() { + return _pointerIsDown = 0; +}, + _passThrough = function _passThrough(v) { + return v; +}, + _round = function _round(value) { + return Math.round(value * 100000) / 100000 || 0; +}, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}, + _isViewport = function _isViewport(e) { + return !!~_root.indexOf(e); +}, + _getViewportDimension = function _getViewportDimension(dimensionProperty) { + return (dimensionProperty === "Height" ? _100vh : _win["inner" + dimensionProperty]) || _docEl["client" + dimensionProperty] || _body["client" + dimensionProperty]; +}, + _getBoundsFunc = function _getBoundsFunc(element) { + return _getProxyProp(element, "getBoundingClientRect") || (_isViewport(element) ? function () { + _winOffsets.width = _win.innerWidth; + _winOffsets.height = _100vh; + return _winOffsets; + } : function () { + return _getBounds(element); + }); +}, + _getSizeFunc = function _getSizeFunc(scroller, isViewport, _ref) { + var d = _ref.d, + d2 = _ref.d2, + a = _ref.a; + return (a = _getProxyProp(scroller, "getBoundingClientRect")) ? function () { + return a()[d]; + } : function () { + return (isViewport ? _getViewportDimension(d2) : scroller["client" + d2]) || 0; + }; +}, + _getOffsetsFunc = function _getOffsetsFunc(element, isViewport) { + return !isViewport || ~_proxies.indexOf(element) ? _getBoundsFunc(element) : function () { + return _winOffsets; + }; +}, + _maxScroll = function _maxScroll(element, _ref2) { + var s = _ref2.s, + d2 = _ref2.d2, + d = _ref2.d, + a = _ref2.a; + return Math.max(0, (s = "scroll" + d2) && (a = _getProxyProp(element, s)) ? a() - _getBoundsFunc(element)()[d] : _isViewport(element) ? (_docEl[s] || _body[s]) - _getViewportDimension(d2) : element[s] - element["offset" + d2]); +}, + _iterateAutoRefresh = function _iterateAutoRefresh(func, events) { + for (var i = 0; i < _autoRefresh.length; i += 3) { + (!events || ~events.indexOf(_autoRefresh[i + 1])) && func(_autoRefresh[i], _autoRefresh[i + 1], _autoRefresh[i + 2]); + } +}, + _isString = function _isString(value) { + return typeof value === "string"; +}, + _isFunction = function _isFunction(value) { + return typeof value === "function"; +}, + _isNumber = function _isNumber(value) { + return typeof value === "number"; +}, + _isObject = function _isObject(value) { + return typeof value === "object"; +}, + _endAnimation = function _endAnimation(animation, reversed, pause) { + return animation && animation.progress(reversed ? 0 : 1) && pause && animation.pause(); +}, + _callback = function _callback(self, func) { + if (self.enabled) { + var result = self._ctx ? self._ctx.add(function () { + return func(self); + }) : func(self); + result && result.totalTime && (self.callbackAnimation = result); + } +}, + _abs = Math.abs, + _left = "left", + _top = "top", + _right = "right", + _bottom = "bottom", + _width = "width", + _height = "height", + _Right = "Right", + _Left = "Left", + _Top = "Top", + _Bottom = "Bottom", + _padding = "padding", + _margin = "margin", + _Width = "Width", + _Height = "Height", + _px = "px", + _getComputedStyle = function _getComputedStyle(element) { + return _win.getComputedStyle(element); +}, + _makePositionable = function _makePositionable(element) { + // if the element already has position: absolute or fixed, leave that, otherwise make it position: relative + var position = _getComputedStyle(element).position; + + element.style.position = position === "absolute" || position === "fixed" ? position : "relative"; +}, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + p in obj || (obj[p] = defaults[p]); + } + + return obj; +}, + _getBounds = function _getBounds(element, withoutTransforms) { + var tween = withoutTransforms && _getComputedStyle(element)[_transformProp] !== "matrix(1, 0, 0, 1, 0, 0)" && gsap.to(element, { + x: 0, + y: 0, + xPercent: 0, + yPercent: 0, + rotation: 0, + rotationX: 0, + rotationY: 0, + scale: 1, + skewX: 0, + skewY: 0 + }).progress(1), + bounds = element.getBoundingClientRect(); + tween && tween.progress(0).kill(); + return bounds; +}, + _getSize = function _getSize(element, _ref3) { + var d2 = _ref3.d2; + return element["offset" + d2] || element["client" + d2] || 0; +}, + _getLabelRatioArray = function _getLabelRatioArray(timeline) { + var a = [], + labels = timeline.labels, + duration = timeline.duration(), + p; + + for (p in labels) { + a.push(labels[p] / duration); + } + + return a; +}, + _getClosestLabel = function _getClosestLabel(animation) { + return function (value) { + return gsap.utils.snap(_getLabelRatioArray(animation), value); + }; +}, + _snapDirectional = function _snapDirectional(snapIncrementOrArray) { + var snap = gsap.utils.snap(snapIncrementOrArray), + a = Array.isArray(snapIncrementOrArray) && snapIncrementOrArray.slice(0).sort(function (a, b) { + return a - b; + }); + return a ? function (value, direction, threshold) { + if (threshold === void 0) { + threshold = 1e-3; + } + + var i; + + if (!direction) { + return snap(value); + } + + if (direction > 0) { + value -= threshold; // to avoid rounding errors. If we're too strict, it might snap forward, then immediately again, and again. + + for (i = 0; i < a.length; i++) { + if (a[i] >= value) { + return a[i]; + } + } + + return a[i - 1]; + } else { + i = a.length; + value += threshold; + + while (i--) { + if (a[i] <= value) { + return a[i]; + } + } + } + + return a[0]; + } : function (value, direction, threshold) { + if (threshold === void 0) { + threshold = 1e-3; + } + + var snapped = snap(value); + return !direction || Math.abs(snapped - value) < threshold || snapped - value < 0 === direction < 0 ? snapped : snap(direction < 0 ? value - snapIncrementOrArray : value + snapIncrementOrArray); + }; +}, + _getLabelAtDirection = function _getLabelAtDirection(timeline) { + return function (value, st) { + return _snapDirectional(_getLabelRatioArray(timeline))(value, st.direction); + }; +}, + _multiListener = function _multiListener(func, element, types, callback) { + return types.split(",").forEach(function (type) { + return func(element, type, callback); + }); +}, + _addListener = function _addListener(element, type, func, nonPassive, capture) { + return element.addEventListener(type, func, { + passive: !nonPassive, + capture: !!capture + }); +}, + _removeListener = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); +}, + _wheelListener = function _wheelListener(func, el, scrollFunc) { + scrollFunc = scrollFunc && scrollFunc.wheelHandler; + + if (scrollFunc) { + func(el, "wheel", scrollFunc); + func(el, "touchmove", scrollFunc); + } +}, + _markerDefaults = { + startColor: "green", + endColor: "red", + indent: 0, + fontSize: "16px", + fontWeight: "normal" +}, + _defaults = { + toggleActions: "play", + anticipatePin: 0 +}, + _keywords = { + top: 0, + left: 0, + center: 0.5, + bottom: 1, + right: 1 +}, + _offsetToPx = function _offsetToPx(value, size) { + if (_isString(value)) { + var eqIndex = value.indexOf("="), + relative = ~eqIndex ? +(value.charAt(eqIndex - 1) + 1) * parseFloat(value.substr(eqIndex + 1)) : 0; + + if (~eqIndex) { + value.indexOf("%") > eqIndex && (relative *= size / 100); + value = value.substr(0, eqIndex - 1); + } + + value = relative + (value in _keywords ? _keywords[value] * size : ~value.indexOf("%") ? parseFloat(value) * size / 100 : parseFloat(value) || 0); + } + + return value; +}, + _createMarker = function _createMarker(type, name, container, direction, _ref4, offset, matchWidthEl, containerAnimation) { + var startColor = _ref4.startColor, + endColor = _ref4.endColor, + fontSize = _ref4.fontSize, + indent = _ref4.indent, + fontWeight = _ref4.fontWeight; + + var e = _doc.createElement("div"), + useFixedPosition = _isViewport(container) || _getProxyProp(container, "pinType") === "fixed", + isScroller = type.indexOf("scroller") !== -1, + parent = useFixedPosition ? _body : container, + isStart = type.indexOf("start") !== -1, + color = isStart ? startColor : endColor, + css = "border-color:" + color + ";font-size:" + fontSize + ";color:" + color + ";font-weight:" + fontWeight + ";pointer-events:none;white-space:nowrap;font-family:sans-serif,Arial;z-index:1000;padding:4px 8px;border-width:0;border-style:solid;"; + + css += "position:" + ((isScroller || containerAnimation) && useFixedPosition ? "fixed;" : "absolute;"); + (isScroller || containerAnimation || !useFixedPosition) && (css += (direction === _vertical ? _right : _bottom) + ":" + (offset + parseFloat(indent)) + "px;"); + matchWidthEl && (css += "box-sizing:border-box;text-align:left;width:" + matchWidthEl.offsetWidth + "px;"); + e._isStart = isStart; + e.setAttribute("class", "gsap-marker-" + type + (name ? " marker-" + name : "")); + e.style.cssText = css; + e.innerText = name || name === 0 ? type + "-" + name : type; + parent.children[0] ? parent.insertBefore(e, parent.children[0]) : parent.appendChild(e); + e._offset = e["offset" + direction.op.d2]; + + _positionMarker(e, 0, direction, isStart); + + return e; +}, + _positionMarker = function _positionMarker(marker, start, direction, flipped) { + var vars = { + display: "block" + }, + side = direction[flipped ? "os2" : "p2"], + oppositeSide = direction[flipped ? "p2" : "os2"]; + marker._isFlipped = flipped; + vars[direction.a + "Percent"] = flipped ? -100 : 0; + vars[direction.a] = flipped ? "1px" : 0; + vars["border" + side + _Width] = 1; + vars["border" + oppositeSide + _Width] = 0; + vars[direction.p] = start + "px"; + gsap.set(marker, vars); +}, + _triggers = [], + _ids = {}, + _rafID, + _sync = function _sync() { + return _getTime() - _lastScrollTime > 34 && (_rafID || (_rafID = requestAnimationFrame(_updateAll))); +}, + _onScroll = function _onScroll() { + // previously, we tried to optimize performance by batching/deferring to the next requestAnimationFrame(), but discovered that Safari has a few bugs that make this unworkable (especially on iOS). See https://codepen.io/GreenSock/pen/16c435b12ef09c38125204818e7b45fc?editors=0010 and https://codepen.io/GreenSock/pen/JjOxYpQ/3dd65ccec5a60f1d862c355d84d14562?editors=0010 and https://codepen.io/GreenSock/pen/ExbrPNa/087cef197dc35445a0951e8935c41503?editors=0010 + if (!_normalizer || !_normalizer.isPressed || _normalizer.startX > _body.clientWidth) { + // if the user is dragging the scrollbar, allow it. + _scrollers.cache++; + + if (_normalizer) { + _rafID || (_rafID = requestAnimationFrame(_updateAll)); + } else { + _updateAll(); // Safari in particular (on desktop) NEEDS the immediate update rather than waiting for a requestAnimationFrame() whereas iOS seems to benefit from waiting for the requestAnimationFrame() tick, at least when normalizing. See https://codepen.io/GreenSock/pen/qBYozqO?editors=0110 + + } + + _lastScrollTime || _dispatch("scrollStart"); + _lastScrollTime = _getTime(); + } +}, + _setBaseDimensions = function _setBaseDimensions() { + _baseScreenWidth = _win.innerWidth; + _baseScreenHeight = _win.innerHeight; +}, + _onResize = function _onResize(force) { + _scrollers.cache++; + (force === true || !_refreshing && !_ignoreResize && !_doc.fullscreenElement && !_doc.webkitFullscreenElement && (!_ignoreMobileResize || _baseScreenWidth !== _win.innerWidth || Math.abs(_win.innerHeight - _baseScreenHeight) > _win.innerHeight * 0.25)) && _resizeDelay.restart(true); +}, + // ignore resizes triggered by refresh() +_listeners = {}, + _emptyArray = [], + _softRefresh = function _softRefresh() { + return _removeListener(ScrollTrigger, "scrollEnd", _softRefresh) || _refreshAll(true); +}, + _dispatch = function _dispatch(type) { + return _listeners[type] && _listeners[type].map(function (f) { + return f(); + }) || _emptyArray; +}, + _savedStyles = [], + // when ScrollTrigger.saveStyles() is called, the inline styles are recorded in this Array in a sequential format like [element, cssText, gsCache, media]. This keeps it very memory-efficient and fast to iterate through. +_revertRecorded = function _revertRecorded(media) { + for (var i = 0; i < _savedStyles.length; i += 5) { + if (!media || _savedStyles[i + 4] && _savedStyles[i + 4].query === media) { + _savedStyles[i].style.cssText = _savedStyles[i + 1]; + _savedStyles[i].getBBox && _savedStyles[i].setAttribute("transform", _savedStyles[i + 2] || ""); + _savedStyles[i + 3].uncache = 1; + } + } +}, + _revertAll = function _revertAll(kill, media) { + var trigger; + + for (_i = 0; _i < _triggers.length; _i++) { + trigger = _triggers[_i]; + + if (trigger && (!media || trigger._ctx === media)) { + if (kill) { + trigger.kill(1); + } else { + trigger.revert(true, true); + } + } + } + + _isReverted = true; + media && _revertRecorded(media); + media || _dispatch("revert"); +}, + _clearScrollMemory = function _clearScrollMemory(scrollRestoration, force) { + // zero-out all the recorded scroll positions. Don't use _triggers because if, for example, .matchMedia() is used to create some ScrollTriggers and then the user resizes and it removes ALL ScrollTriggers, and then go back to a size where there are ScrollTriggers, it would have kept the position(s) saved from the initial state. + _scrollers.cache++; + (force || !_refreshingAll) && _scrollers.forEach(function (obj) { + return _isFunction(obj) && obj.cacheID++ && (obj.rec = 0); + }); + _isString(scrollRestoration) && (_win.history.scrollRestoration = _scrollRestoration = scrollRestoration); +}, + _refreshingAll, + _refreshID = 0, + _queueRefreshID, + _queueRefreshAll = function _queueRefreshAll() { + // we don't want to call _refreshAll() every time we create a new ScrollTrigger (for performance reasons) - it's better to batch them. Some frameworks dynamically load content and we can't rely on the window's "load" or "DOMContentLoaded" events to trigger it. + if (_queueRefreshID !== _refreshID) { + var id = _queueRefreshID = _refreshID; + requestAnimationFrame(function () { + return id === _refreshID && _refreshAll(true); + }); + } +}, + _refresh100vh = function _refresh100vh() { + _body.appendChild(_div100vh); + + _100vh = !_normalizer && _div100vh.offsetHeight || _win.innerHeight; + + _body.removeChild(_div100vh); +}, + _hideAllMarkers = function _hideAllMarkers(hide) { + return _toArray(".gsap-marker-start, .gsap-marker-end, .gsap-marker-scroller-start, .gsap-marker-scroller-end").forEach(function (el) { + return el.style.display = hide ? "none" : "block"; + }); +}, + _refreshAll = function _refreshAll(force, skipRevert) { + _docEl = _doc.documentElement; // some frameworks like Astro may cache the <body> and replace it during routing, so we'll just re-record the _docEl and _body for safety (otherwise, the markers may not get added properly). + + _body = _doc.body; + _root = [_win, _doc, _docEl, _body]; + + if (_lastScrollTime && !force && !_isReverted) { + _addListener(ScrollTrigger, "scrollEnd", _softRefresh); + + return; + } + + _refresh100vh(); + + _refreshingAll = ScrollTrigger.isRefreshing = true; + + _scrollers.forEach(function (obj) { + return _isFunction(obj) && ++obj.cacheID && (obj.rec = obj()); + }); // force the clearing of the cache because some browsers take a little while to dispatch the "scroll" event and the user may have changed the scroll position and then called ScrollTrigger.refresh() right away + + + var refreshInits = _dispatch("refreshInit"); + + _sort && ScrollTrigger.sort(); + skipRevert || _revertAll(); + + _scrollers.forEach(function (obj) { + if (_isFunction(obj)) { + obj.smooth && (obj.target.style.scrollBehavior = "auto"); // smooth scrolling interferes + + obj(0); + } + }); + + _triggers.slice(0).forEach(function (t) { + return t.refresh(); + }); // don't loop with _i because during a refresh() someone could call ScrollTrigger.update() which would iterate through _i resulting in a skip. + + + _isReverted = false; + + _triggers.forEach(function (t) { + // nested pins (pinnedContainer) with pinSpacing may expand the container, so we must accommodate that here. + if (t._subPinOffset && t.pin) { + var prop = t.vars.horizontal ? "offsetWidth" : "offsetHeight", + original = t.pin[prop]; + t.revert(true, 1); + t.adjustPinSpacing(t.pin[prop] - original); + t.refresh(); + } + }); + + _clampingMax = 1; // pinSpacing might be propping a page open, thus when we .setPositions() to clamp a ScrollTrigger's end we should leave the pinSpacing alone. That's what this flag is for. + + _hideAllMarkers(true); + + _triggers.forEach(function (t) { + // the scroller's max scroll position may change after all the ScrollTriggers refreshed (like pinning could push it down), so we need to loop back and correct any with end: "max". Same for anything with a clamped end + var max = _maxScroll(t.scroller, t._dir), + endClamp = t.vars.end === "max" || t._endClamp && t.end > max, + startClamp = t._startClamp && t.start >= max; + + (endClamp || startClamp) && t.setPositions(startClamp ? max - 1 : t.start, endClamp ? Math.max(startClamp ? max : t.start + 1, max) : t.end, true); + }); + + _hideAllMarkers(false); + + _clampingMax = 0; + refreshInits.forEach(function (result) { + return result && result.render && result.render(-1); + }); // if the onRefreshInit() returns an animation (typically a gsap.set()), revert it. This makes it easy to put things in a certain spot before refreshing for measurement purposes, and then put things back. + + _scrollers.forEach(function (obj) { + if (_isFunction(obj)) { + obj.smooth && requestAnimationFrame(function () { + return obj.target.style.scrollBehavior = "smooth"; + }); + obj.rec && obj(obj.rec); + } + }); + + _clearScrollMemory(_scrollRestoration, 1); + + _resizeDelay.pause(); + + _refreshID++; + _refreshingAll = 2; + + _updateAll(2); + + _triggers.forEach(function (t) { + return _isFunction(t.vars.onRefresh) && t.vars.onRefresh(t); + }); + + _refreshingAll = ScrollTrigger.isRefreshing = false; + + _dispatch("refresh"); +}, + _lastScroll = 0, + _direction = 1, + _primary, + _updateAll = function _updateAll(force) { + if (force === 2 || !_refreshingAll && !_isReverted) { + // _isReverted could be true if, for example, a matchMedia() is in the process of executing. We don't want to update during the time everything is reverted. + ScrollTrigger.isUpdating = true; + _primary && _primary.update(0); // ScrollSmoother uses refreshPriority -9999 to become the primary that gets updated before all others because it affects the scroll position. + + var l = _triggers.length, + time = _getTime(), + recordVelocity = time - _time1 >= 50, + scroll = l && _triggers[0].scroll(); + + _direction = _lastScroll > scroll ? -1 : 1; + _refreshingAll || (_lastScroll = scroll); + + if (recordVelocity) { + if (_lastScrollTime && !_pointerIsDown && time - _lastScrollTime > 200) { + _lastScrollTime = 0; + + _dispatch("scrollEnd"); + } + + _time2 = _time1; + _time1 = time; + } + + if (_direction < 0) { + _i = l; + + while (_i-- > 0) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + + _direction = 1; + } else { + for (_i = 0; _i < l; _i++) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + } + + ScrollTrigger.isUpdating = false; + } + + _rafID = 0; +}, + _propNamesToCopy = [_left, _top, _bottom, _right, _margin + _Bottom, _margin + _Right, _margin + _Top, _margin + _Left, "display", "flexShrink", "float", "zIndex", "gridColumnStart", "gridColumnEnd", "gridRowStart", "gridRowEnd", "gridArea", "justifySelf", "alignSelf", "placeSelf", "order"], + _stateProps = _propNamesToCopy.concat([_width, _height, "boxSizing", "max" + _Width, "max" + _Height, "position", _margin, _padding, _padding + _Top, _padding + _Right, _padding + _Bottom, _padding + _Left]), + _swapPinOut = function _swapPinOut(pin, spacer, state) { + _setState(state); + + var cache = pin._gsap; + + if (cache.spacerIsNative) { + _setState(cache.spacerState); + } else if (pin._gsap.swappedIn) { + var parent = spacer.parentNode; + + if (parent) { + parent.insertBefore(pin, spacer); + parent.removeChild(spacer); + } + } + + pin._gsap.swappedIn = false; +}, + _swapPinIn = function _swapPinIn(pin, spacer, cs, spacerState) { + if (!pin._gsap.swappedIn) { + var i = _propNamesToCopy.length, + spacerStyle = spacer.style, + pinStyle = pin.style, + p; + + while (i--) { + p = _propNamesToCopy[i]; + spacerStyle[p] = cs[p]; + } + + spacerStyle.position = cs.position === "absolute" ? "absolute" : "relative"; + cs.display === "inline" && (spacerStyle.display = "inline-block"); + pinStyle[_bottom] = pinStyle[_right] = "auto"; + spacerStyle.flexBasis = cs.flexBasis || "auto"; + spacerStyle.overflow = "visible"; + spacerStyle.boxSizing = "border-box"; + spacerStyle[_width] = _getSize(pin, _horizontal) + _px; + spacerStyle[_height] = _getSize(pin, _vertical) + _px; + spacerStyle[_padding] = pinStyle[_margin] = pinStyle[_top] = pinStyle[_left] = "0"; + + _setState(spacerState); + + pinStyle[_width] = pinStyle["max" + _Width] = cs[_width]; + pinStyle[_height] = pinStyle["max" + _Height] = cs[_height]; + pinStyle[_padding] = cs[_padding]; + + if (pin.parentNode !== spacer) { + pin.parentNode.insertBefore(spacer, pin); + spacer.appendChild(pin); + } + + pin._gsap.swappedIn = true; + } +}, + _capsExp = /([A-Z])/g, + _setState = function _setState(state) { + if (state) { + var style = state.t.style, + l = state.length, + i = 0, + p, + value; + (state.t._gsap || gsap.core.getCache(state.t)).uncache = 1; // otherwise transforms may be off + + for (; i < l; i += 2) { + value = state[i + 1]; + p = state[i]; + + if (value) { + style[p] = value; + } else if (style[p]) { + style.removeProperty(p.replace(_capsExp, "-$1").toLowerCase()); + } + } + } +}, + _getState = function _getState(element) { + // returns an Array with alternating values like [property, value, property, value] and a "t" property pointing to the target (element). Makes it fast and cheap. + var l = _stateProps.length, + style = element.style, + state = [], + i = 0; + + for (; i < l; i++) { + state.push(_stateProps[i], style[_stateProps[i]]); + } + + state.t = element; + return state; +}, + _copyState = function _copyState(state, override, omitOffsets) { + var result = [], + l = state.length, + i = omitOffsets ? 8 : 0, + // skip top, left, right, bottom if omitOffsets is true + p; + + for (; i < l; i += 2) { + p = state[i]; + result.push(p, p in override ? override[p] : state[i + 1]); + } + + result.t = state.t; + return result; +}, + _winOffsets = { + left: 0, + top: 0 +}, + // // potential future feature (?) Allow users to calculate where a trigger hits (scroll position) like getScrollPosition("#id", "top bottom") +// _getScrollPosition = (trigger, position, {scroller, containerAnimation, horizontal}) => { +// scroller = _getTarget(scroller || _win); +// let direction = horizontal ? _horizontal : _vertical, +// isViewport = _isViewport(scroller); +// _getSizeFunc(scroller, isViewport, direction); +// return _parsePosition(position, _getTarget(trigger), _getSizeFunc(scroller, isViewport, direction)(), direction, _getScrollFunc(scroller, direction)(), 0, 0, 0, _getOffsetsFunc(scroller, isViewport)(), isViewport ? 0 : parseFloat(_getComputedStyle(scroller)["border" + direction.p2 + _Width]) || 0, 0, containerAnimation ? containerAnimation.duration() : _maxScroll(scroller), containerAnimation); +// }, +_parsePosition = function _parsePosition(value, trigger, scrollerSize, direction, scroll, marker, markerScroller, self, scrollerBounds, borderWidth, useFixedPosition, scrollerMax, containerAnimation, clampZeroProp) { + _isFunction(value) && (value = value(self)); + + if (_isString(value) && value.substr(0, 3) === "max") { + value = scrollerMax + (value.charAt(4) === "=" ? _offsetToPx("0" + value.substr(3), scrollerSize) : 0); + } + + var time = containerAnimation ? containerAnimation.time() : 0, + p1, + p2, + element; + containerAnimation && containerAnimation.seek(0); + isNaN(value) || (value = +value); // convert a string number like "45" to an actual number + + if (!_isNumber(value)) { + _isFunction(trigger) && (trigger = trigger(self)); + var offsets = (value || "0").split(" "), + bounds, + localOffset, + globalOffset, + display; + element = _getTarget(trigger, self) || _body; + bounds = _getBounds(element) || {}; + + if ((!bounds || !bounds.left && !bounds.top) && _getComputedStyle(element).display === "none") { + // if display is "none", it won't report getBoundingClientRect() properly + display = element.style.display; + element.style.display = "block"; + bounds = _getBounds(element); + display ? element.style.display = display : element.style.removeProperty("display"); + } + + localOffset = _offsetToPx(offsets[0], bounds[direction.d]); + globalOffset = _offsetToPx(offsets[1] || "0", scrollerSize); + value = bounds[direction.p] - scrollerBounds[direction.p] - borderWidth + localOffset + scroll - globalOffset; + markerScroller && _positionMarker(markerScroller, globalOffset, direction, scrollerSize - globalOffset < 20 || markerScroller._isStart && globalOffset > 20); + scrollerSize -= scrollerSize - globalOffset; // adjust for the marker + } else { + containerAnimation && (value = gsap.utils.mapRange(containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, 0, scrollerMax, value)); + markerScroller && _positionMarker(markerScroller, scrollerSize, direction, true); + } + + if (clampZeroProp) { + self[clampZeroProp] = value || -0.001; + value < 0 && (value = 0); + } + + if (marker) { + var position = value + scrollerSize, + isStart = marker._isStart; + p1 = "scroll" + direction.d2; + + _positionMarker(marker, position, direction, isStart && position > 20 || !isStart && (useFixedPosition ? Math.max(_body[p1], _docEl[p1]) : marker.parentNode[p1]) <= position + 1); + + if (useFixedPosition) { + scrollerBounds = _getBounds(markerScroller); + useFixedPosition && (marker.style[direction.op.p] = scrollerBounds[direction.op.p] - direction.op.m - marker._offset + _px); + } + } + + if (containerAnimation && element) { + p1 = _getBounds(element); + containerAnimation.seek(scrollerMax); + p2 = _getBounds(element); + containerAnimation._caScrollDist = p1[direction.p] - p2[direction.p]; + value = value / containerAnimation._caScrollDist * scrollerMax; + } + + containerAnimation && containerAnimation.seek(time); + return containerAnimation ? value : Math.round(value); +}, + _prefixExp = /(webkit|moz|length|cssText|inset)/i, + _reparent = function _reparent(element, parent, top, left) { + if (element.parentNode !== parent) { + var style = element.style, + p, + cs; + + if (parent === _body) { + element._stOrig = style.cssText; // record original inline styles so we can revert them later + + cs = _getComputedStyle(element); + + for (p in cs) { + // must copy all relevant styles to ensure that nothing changes visually when we reparent to the <body>. Skip the vendor prefixed ones. + if (!+p && !_prefixExp.test(p) && cs[p] && typeof style[p] === "string" && p !== "0") { + style[p] = cs[p]; + } + } + + style.top = top; + style.left = left; + } else { + style.cssText = element._stOrig; + } + + gsap.core.getCache(element).uncache = 1; + parent.appendChild(element); + } +}, + _interruptionTracker = function _interruptionTracker(getValueFunc, initialValue, onInterrupt) { + var last1 = initialValue, + last2 = last1; + return function (value) { + var current = Math.round(getValueFunc()); // round because in some [very uncommon] Windows environments, scroll can get reported with decimals even though it was set without. + + if (current !== last1 && current !== last2 && Math.abs(current - last1) > 3 && Math.abs(current - last2) > 3) { + // if the user scrolls, kill the tween. iOS Safari intermittently misreports the scroll position, it may be the most recently-set one or the one before that! When Safari is zoomed (CMD-+), it often misreports as 1 pixel off too! So if we set the scroll position to 125, for example, it'll actually report it as 124. + value = current; + onInterrupt && onInterrupt(); + } + + last2 = last1; + last1 = Math.round(value); + return last1; + }; +}, + _shiftMarker = function _shiftMarker(marker, direction, value) { + var vars = {}; + vars[direction.p] = "+=" + value; + gsap.set(marker, vars); +}, + // _mergeAnimations = animations => { +// let tl = gsap.timeline({smoothChildTiming: true}).startTime(Math.min(...animations.map(a => a.globalTime(0)))); +// animations.forEach(a => {let time = a.totalTime(); tl.add(a); a.totalTime(time); }); +// tl.smoothChildTiming = false; +// return tl; +// }, +// returns a function that can be used to tween the scroll position in the direction provided, and when doing so it'll add a .tween property to the FUNCTION itself, and remove it when the tween completes or gets killed. This gives us a way to have multiple ScrollTriggers use a central function for any given scroller and see if there's a scroll tween running (which would affect if/how things get updated) +_getTweenCreator = function _getTweenCreator(scroller, direction) { + var getScroll = _getScrollFunc(scroller, direction), + prop = "_scroll" + direction.p2, + // add a tweenable property to the scroller that's a getter/setter function, like _scrollTop or _scrollLeft. This way, if someone does gsap.killTweensOf(scroller) it'll kill the scroll tween. + getTween = function getTween(scrollTo, vars, initialValue, change1, change2) { + var tween = getTween.tween, + onComplete = vars.onComplete, + modifiers = {}; + initialValue = initialValue || getScroll(); + + var checkForInterruption = _interruptionTracker(getScroll, initialValue, function () { + tween.kill(); + getTween.tween = 0; + }); + + change2 = change1 && change2 || 0; // if change1 is 0, we set that to the difference and ignore change2. Otherwise, there would be a compound effect. + + change1 = change1 || scrollTo - initialValue; + tween && tween.kill(); + vars[prop] = scrollTo; + vars.inherit = false; + vars.modifiers = modifiers; + + modifiers[prop] = function () { + return checkForInterruption(initialValue + change1 * tween.ratio + change2 * tween.ratio * tween.ratio); + }; + + vars.onUpdate = function () { + _scrollers.cache++; + getTween.tween && _updateAll(); // if it was interrupted/killed, like in a context.revert(), don't force an updateAll() + }; + + vars.onComplete = function () { + getTween.tween = 0; + onComplete && onComplete.call(tween); + }; + + tween = getTween.tween = gsap.to(scroller, vars); + return tween; + }; + + scroller[prop] = getScroll; + + getScroll.wheelHandler = function () { + return getTween.tween && getTween.tween.kill() && (getTween.tween = 0); + }; + + _addListener(scroller, "wheel", getScroll.wheelHandler); // Windows machines handle mousewheel scrolling in chunks (like "3 lines per scroll") meaning the typical strategy for cancelling the scroll isn't as sensitive. It's much more likely to match one of the previous 2 scroll event positions. So we kill any snapping as soon as there's a wheel event. + + + ScrollTrigger.isTouch && _addListener(scroller, "touchmove", getScroll.wheelHandler); + return getTween; +}; + +export var ScrollTrigger = /*#__PURE__*/function () { + function ScrollTrigger(vars, animation) { + _coreInitted || ScrollTrigger.register(gsap) || console.warn("Please gsap.registerPlugin(ScrollTrigger)"); + + _context(this); + + this.init(vars, animation); + } + + var _proto = ScrollTrigger.prototype; + + _proto.init = function init(vars, animation) { + this.progress = this.start = 0; + this.vars && this.kill(true, true); // in case it's being initted again + + if (!_enabled) { + this.update = this.refresh = this.kill = _passThrough; + return; + } + + vars = _setDefaults(_isString(vars) || _isNumber(vars) || vars.nodeType ? { + trigger: vars + } : vars, _defaults); + + var _vars = vars, + onUpdate = _vars.onUpdate, + toggleClass = _vars.toggleClass, + id = _vars.id, + onToggle = _vars.onToggle, + onRefresh = _vars.onRefresh, + scrub = _vars.scrub, + trigger = _vars.trigger, + pin = _vars.pin, + pinSpacing = _vars.pinSpacing, + invalidateOnRefresh = _vars.invalidateOnRefresh, + anticipatePin = _vars.anticipatePin, + onScrubComplete = _vars.onScrubComplete, + onSnapComplete = _vars.onSnapComplete, + once = _vars.once, + snap = _vars.snap, + pinReparent = _vars.pinReparent, + pinSpacer = _vars.pinSpacer, + containerAnimation = _vars.containerAnimation, + fastScrollEnd = _vars.fastScrollEnd, + preventOverlaps = _vars.preventOverlaps, + direction = vars.horizontal || vars.containerAnimation && vars.horizontal !== false ? _horizontal : _vertical, + isToggle = !scrub && scrub !== 0, + scroller = _getTarget(vars.scroller || _win), + scrollerCache = gsap.core.getCache(scroller), + isViewport = _isViewport(scroller), + useFixedPosition = ("pinType" in vars ? vars.pinType : _getProxyProp(scroller, "pinType") || isViewport && "fixed") === "fixed", + callbacks = [vars.onEnter, vars.onLeave, vars.onEnterBack, vars.onLeaveBack], + toggleActions = isToggle && vars.toggleActions.split(" "), + markers = "markers" in vars ? vars.markers : _defaults.markers, + borderWidth = isViewport ? 0 : parseFloat(_getComputedStyle(scroller)["border" + direction.p2 + _Width]) || 0, + self = this, + onRefreshInit = vars.onRefreshInit && function () { + return vars.onRefreshInit(self); + }, + getScrollerSize = _getSizeFunc(scroller, isViewport, direction), + getScrollerOffsets = _getOffsetsFunc(scroller, isViewport), + lastSnap = 0, + lastRefresh = 0, + prevProgress = 0, + scrollFunc = _getScrollFunc(scroller, direction), + tweenTo, + pinCache, + snapFunc, + scroll1, + scroll2, + start, + end, + markerStart, + markerEnd, + markerStartTrigger, + markerEndTrigger, + markerVars, + executingOnRefresh, + change, + pinOriginalState, + pinActiveState, + pinState, + spacer, + offset, + pinGetter, + pinSetter, + pinStart, + pinChange, + spacingStart, + spacerState, + markerStartSetter, + pinMoves, + markerEndSetter, + cs, + snap1, + snap2, + scrubTween, + scrubSmooth, + snapDurClamp, + snapDelayedCall, + prevScroll, + prevAnimProgress, + caMarkerSetter, + customRevertReturn; // for the sake of efficiency, _startClamp/_endClamp serve like a truthy value indicating that clamping was enabled on the start/end, and ALSO store the actual pre-clamped numeric value. We tap into that in ScrollSmoother for speed effects. So for example, if start="clamp(top bottom)" results in a start of -100 naturally, it would get clamped to 0 but -100 would be stored in _startClamp. + + + self._startClamp = self._endClamp = false; + self._dir = direction; + anticipatePin *= 45; + self.scroller = scroller; + self.scroll = containerAnimation ? containerAnimation.time.bind(containerAnimation) : scrollFunc; + scroll1 = scrollFunc(); + self.vars = vars; + animation = animation || vars.animation; + + if ("refreshPriority" in vars) { + _sort = 1; + vars.refreshPriority === -9999 && (_primary = self); // used by ScrollSmoother + } + + scrollerCache.tweenScroll = scrollerCache.tweenScroll || { + top: _getTweenCreator(scroller, _vertical), + left: _getTweenCreator(scroller, _horizontal) + }; + self.tweenTo = tweenTo = scrollerCache.tweenScroll[direction.p]; + + self.scrubDuration = function (value) { + scrubSmooth = _isNumber(value) && value; + + if (!scrubSmooth) { + scrubTween && scrubTween.progress(1).kill(); + scrubTween = 0; + } else { + scrubTween ? scrubTween.duration(value) : scrubTween = gsap.to(animation, { + ease: "expo", + totalProgress: "+=0", + inherit: false, + duration: scrubSmooth, + paused: true, + onComplete: function onComplete() { + return onScrubComplete && onScrubComplete(self); + } + }); + } + }; + + if (animation) { + animation.vars.lazy = false; + animation._initted && !self.isReverted || animation.vars.immediateRender !== false && vars.immediateRender !== false && animation.duration() && animation.render(0, true, true); // special case: if this ScrollTrigger gets re-initted, a from() tween with a stagger could get initted initially and then reverted on the re-init which means it'll need to get rendered again here to properly display things. Otherwise, See https://gsap.com/forums/topic/36777-scrollsmoother-splittext-nextjs/ and https://codepen.io/GreenSock/pen/eYPyPpd?editors=0010 + + self.animation = animation.pause(); + animation.scrollTrigger = self; + self.scrubDuration(scrub); + snap1 = 0; + id || (id = animation.vars.id); + } + + if (snap) { + // TODO: potential idea: use legitimate CSS scroll snapping by pushing invisible elements into the DOM that serve as snap positions, and toggle the document.scrollingElement.style.scrollSnapType onToggle. See https://codepen.io/GreenSock/pen/JjLrgWM for a quick proof of concept. + if (!_isObject(snap) || snap.push) { + snap = { + snapTo: snap + }; + } + + "scrollBehavior" in _body.style && gsap.set(isViewport ? [_body, _docEl] : scroller, { + scrollBehavior: "auto" + }); // smooth scrolling doesn't work with snap. + + _scrollers.forEach(function (o) { + return _isFunction(o) && o.target === (isViewport ? _doc.scrollingElement || _docEl : scroller) && (o.smooth = false); + }); // note: set smooth to false on both the vertical and horizontal scroll getters/setters + + + snapFunc = _isFunction(snap.snapTo) ? snap.snapTo : snap.snapTo === "labels" ? _getClosestLabel(animation) : snap.snapTo === "labelsDirectional" ? _getLabelAtDirection(animation) : snap.directional !== false ? function (value, st) { + return _snapDirectional(snap.snapTo)(value, _getTime() - lastRefresh < 500 ? 0 : st.direction); + } : gsap.utils.snap(snap.snapTo); + snapDurClamp = snap.duration || { + min: 0.1, + max: 2 + }; + snapDurClamp = _isObject(snapDurClamp) ? _clamp(snapDurClamp.min, snapDurClamp.max) : _clamp(snapDurClamp, snapDurClamp); + snapDelayedCall = gsap.delayedCall(snap.delay || scrubSmooth / 2 || 0.1, function () { + var scroll = scrollFunc(), + refreshedRecently = _getTime() - lastRefresh < 500, + tween = tweenTo.tween; + + if ((refreshedRecently || Math.abs(self.getVelocity()) < 10) && !tween && !_pointerIsDown && lastSnap !== scroll) { + var progress = (scroll - start) / change, + totalProgress = animation && !isToggle ? animation.totalProgress() : progress, + velocity = refreshedRecently ? 0 : (totalProgress - snap2) / (_getTime() - _time2) * 1000 || 0, + change1 = gsap.utils.clamp(-progress, 1 - progress, _abs(velocity / 2) * velocity / 0.185), + naturalEnd = progress + (snap.inertia === false ? 0 : change1), + endValue, + endScroll, + _snap = snap, + onStart = _snap.onStart, + _onInterrupt = _snap.onInterrupt, + _onComplete = _snap.onComplete; + endValue = snapFunc(naturalEnd, self); + _isNumber(endValue) || (endValue = naturalEnd); // in case the function didn't return a number, fall back to using the naturalEnd + + endScroll = Math.max(0, Math.round(start + endValue * change)); + + if (scroll <= end && scroll >= start && endScroll !== scroll) { + if (tween && !tween._initted && tween.data <= _abs(endScroll - scroll)) { + // there's an overlapping snap! So we must figure out which one is closer and let that tween live. + return; + } + + if (snap.inertia === false) { + change1 = endValue - progress; + } + + tweenTo(endScroll, { + duration: snapDurClamp(_abs(Math.max(_abs(naturalEnd - totalProgress), _abs(endValue - totalProgress)) * 0.185 / velocity / 0.05 || 0)), + ease: snap.ease || "power3", + data: _abs(endScroll - scroll), + // record the distance so that if another snap tween occurs (conflict) we can prioritize the closest snap. + onInterrupt: function onInterrupt() { + return snapDelayedCall.restart(true) && _onInterrupt && _onInterrupt(self); + }, + onComplete: function onComplete() { + self.update(); + lastSnap = scrollFunc(); + + if (animation && !isToggle) { + // the resolution of the scrollbar is limited, so we should correct the scrubbed animation's playhead at the end to match EXACTLY where it was supposed to snap + scrubTween ? scrubTween.resetTo("totalProgress", endValue, animation._tTime / animation._tDur) : animation.progress(endValue); + } + + snap1 = snap2 = animation && !isToggle ? animation.totalProgress() : self.progress; + onSnapComplete && onSnapComplete(self); + _onComplete && _onComplete(self); + } + }, scroll, change1 * change, endScroll - scroll - change1 * change); + onStart && onStart(self, tweenTo.tween); + } + } else if (self.isActive && lastSnap !== scroll) { + snapDelayedCall.restart(true); + } + }).pause(); + } + + id && (_ids[id] = self); + trigger = self.trigger = _getTarget(trigger || pin !== true && pin); // if a trigger has some kind of scroll-related effect applied that could contaminate the "y" or "x" position (like a ScrollSmoother effect), we needed a way to temporarily revert it, so we use the stRevert property of the gsCache. It can return another function that we'll call at the end so it can return to its normal state. + + customRevertReturn = trigger && trigger._gsap && trigger._gsap.stRevert; + customRevertReturn && (customRevertReturn = customRevertReturn(self)); + pin = pin === true ? trigger : _getTarget(pin); + _isString(toggleClass) && (toggleClass = { + targets: trigger, + className: toggleClass + }); + + if (pin) { + pinSpacing === false || pinSpacing === _margin || (pinSpacing = !pinSpacing && pin.parentNode && pin.parentNode.style && _getComputedStyle(pin.parentNode).display === "flex" ? false : _padding); // if the parent is display: flex, don't apply pinSpacing by default. We should check that pin.parentNode is an element (not shadow dom window) + + self.pin = pin; + pinCache = gsap.core.getCache(pin); + + if (!pinCache.spacer) { + // record the spacer and pinOriginalState on the cache in case someone tries pinning the same element with MULTIPLE ScrollTriggers - we don't want to have multiple spacers or record the "original" pin state after it has already been affected by another ScrollTrigger. + if (pinSpacer) { + pinSpacer = _getTarget(pinSpacer); + pinSpacer && !pinSpacer.nodeType && (pinSpacer = pinSpacer.current || pinSpacer.nativeElement); // for React & Angular + + pinCache.spacerIsNative = !!pinSpacer; + pinSpacer && (pinCache.spacerState = _getState(pinSpacer)); + } + + pinCache.spacer = spacer = pinSpacer || _doc.createElement("div"); + spacer.classList.add("pin-spacer"); + id && spacer.classList.add("pin-spacer-" + id); + pinCache.pinState = pinOriginalState = _getState(pin); + } else { + pinOriginalState = pinCache.pinState; + } + + vars.force3D !== false && gsap.set(pin, { + force3D: true + }); + self.spacer = spacer = pinCache.spacer; + cs = _getComputedStyle(pin); + spacingStart = cs[pinSpacing + direction.os2]; + pinGetter = gsap.getProperty(pin); + pinSetter = gsap.quickSetter(pin, direction.a, _px); // pin.firstChild && !_maxScroll(pin, direction) && (pin.style.overflow = "hidden"); // protects from collapsing margins, but can have unintended consequences as demonstrated here: https://codepen.io/GreenSock/pen/1e42c7a73bfa409d2cf1e184e7a4248d so it was removed in favor of just telling people to set up their CSS to avoid the collapsing margins (overflow: hidden | auto is just one option. Another is border-top: 1px solid transparent). + + _swapPinIn(pin, spacer, cs); + + pinState = _getState(pin); + } + + if (markers) { + markerVars = _isObject(markers) ? _setDefaults(markers, _markerDefaults) : _markerDefaults; + markerStartTrigger = _createMarker("scroller-start", id, scroller, direction, markerVars, 0); + markerEndTrigger = _createMarker("scroller-end", id, scroller, direction, markerVars, 0, markerStartTrigger); + offset = markerStartTrigger["offset" + direction.op.d2]; + + var content = _getTarget(_getProxyProp(scroller, "content") || scroller); + + markerStart = this.markerStart = _createMarker("start", id, content, direction, markerVars, offset, 0, containerAnimation); + markerEnd = this.markerEnd = _createMarker("end", id, content, direction, markerVars, offset, 0, containerAnimation); + containerAnimation && (caMarkerSetter = gsap.quickSetter([markerStart, markerEnd], direction.a, _px)); + + if (!useFixedPosition && !(_proxies.length && _getProxyProp(scroller, "fixedMarkers") === true)) { + _makePositionable(isViewport ? _body : scroller); + + gsap.set([markerStartTrigger, markerEndTrigger], { + force3D: true + }); + markerStartSetter = gsap.quickSetter(markerStartTrigger, direction.a, _px); + markerEndSetter = gsap.quickSetter(markerEndTrigger, direction.a, _px); + } + } + + if (containerAnimation) { + var oldOnUpdate = containerAnimation.vars.onUpdate, + oldParams = containerAnimation.vars.onUpdateParams; + containerAnimation.eventCallback("onUpdate", function () { + self.update(0, 0, 1); + oldOnUpdate && oldOnUpdate.apply(containerAnimation, oldParams || []); + }); + } + + self.previous = function () { + return _triggers[_triggers.indexOf(self) - 1]; + }; + + self.next = function () { + return _triggers[_triggers.indexOf(self) + 1]; + }; + + self.revert = function (revert, temp) { + if (!temp) { + return self.kill(true); + } // for compatibility with gsap.context() and gsap.matchMedia() which call revert() + + + var r = revert !== false || !self.enabled, + prevRefreshing = _refreshing; + + if (r !== self.isReverted) { + if (r) { + prevScroll = Math.max(scrollFunc(), self.scroll.rec || 0); // record the scroll so we can revert later (repositioning/pinning things can affect scroll position). In the static refresh() method, we first record all the scroll positions as a reference. + + prevProgress = self.progress; + prevAnimProgress = animation && animation.progress(); + } + + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(function (m) { + return m.style.display = r ? "none" : "block"; + }); + + if (r) { + _refreshing = self; + self.update(r); // make sure the pin is back in its original position so that all the measurements are correct. do this BEFORE swapping the pin out + } + + if (pin && (!pinReparent || !self.isActive)) { + if (r) { + _swapPinOut(pin, spacer, pinOriginalState); + } else { + _swapPinIn(pin, spacer, _getComputedStyle(pin), spacerState); + } + } + + r || self.update(r); // when we're restoring, the update should run AFTER swapping the pin into its pin-spacer. + + _refreshing = prevRefreshing; // restore. We set it to true during the update() so that things fire properly in there. + + self.isReverted = r; + } + }; + + self.refresh = function (soft, force, position, pinOffset) { + // position is typically only defined if it's coming from setPositions() - it's a way to skip the normal parsing. pinOffset is also only from setPositions() and is mostly related to fancy stuff we need to do in ScrollSmoother with effects + if ((_refreshing || !self.enabled) && !force) { + return; + } + + if (pin && soft && _lastScrollTime) { + _addListener(ScrollTrigger, "scrollEnd", _softRefresh); + + return; + } + + !_refreshingAll && onRefreshInit && onRefreshInit(self); + _refreshing = self; + + if (tweenTo.tween && !position) { + // we skip this if a position is passed in because typically that's from .setPositions() and it's best to allow in-progress snapping to continue. + tweenTo.tween.kill(); + tweenTo.tween = 0; + } + + scrubTween && scrubTween.pause(); + invalidateOnRefresh && animation && animation.revert({ + kill: false + }).invalidate(); + self.isReverted || self.revert(true, true); + self._subPinOffset = false; // we'll set this to true in the sub-pins if we find any + + var size = getScrollerSize(), + scrollerBounds = getScrollerOffsets(), + max = containerAnimation ? containerAnimation.duration() : _maxScroll(scroller, direction), + isFirstRefresh = change <= 0.01, + offset = 0, + otherPinOffset = pinOffset || 0, + parsedEnd = _isObject(position) ? position.end : vars.end, + parsedEndTrigger = vars.endTrigger || trigger, + parsedStart = _isObject(position) ? position.start : vars.start || (vars.start === 0 || !trigger ? 0 : pin ? "0 0" : "0 100%"), + pinnedContainer = self.pinnedContainer = vars.pinnedContainer && _getTarget(vars.pinnedContainer, self), + triggerIndex = trigger && Math.max(0, _triggers.indexOf(self)) || 0, + i = triggerIndex, + cs, + bounds, + scroll, + isVertical, + override, + curTrigger, + curPin, + oppositeScroll, + initted, + revertedPins, + forcedOverflow, + markerStartOffset, + markerEndOffset; + + if (markers && _isObject(position)) { + // if we alter the start/end positions with .setPositions(), it generally feeds in absolute NUMBERS which don't convey information about where to line up the markers, so to keep it intuitive, we record how far the trigger positions shift after applying the new numbers and then offset by that much in the opposite direction. We do the same to the associated trigger markers too of course. + markerStartOffset = gsap.getProperty(markerStartTrigger, direction.p); + markerEndOffset = gsap.getProperty(markerEndTrigger, direction.p); + } + + while (i-- > 0) { + // user might try to pin the same element more than once, so we must find any prior triggers with the same pin, revert them, and determine how long they're pinning so that we can offset things appropriately. Make sure we revert from last to first so that things "rewind" properly. + curTrigger = _triggers[i]; + curTrigger.end || curTrigger.refresh(0, 1) || (_refreshing = self); // if it's a timeline-based trigger that hasn't been fully initialized yet because it's waiting for 1 tick, just force the refresh() here, otherwise if it contains a pin that's supposed to affect other ScrollTriggers further down the page, they won't be adjusted properly. + + curPin = curTrigger.pin; + + if (curPin && (curPin === trigger || curPin === pin || curPin === pinnedContainer) && !curTrigger.isReverted) { + revertedPins || (revertedPins = []); + revertedPins.unshift(curTrigger); // we'll revert from first to last to make sure things reach their end state properly + + curTrigger.revert(true, true); + } + + if (curTrigger !== _triggers[i]) { + // in case it got removed. + triggerIndex--; + i--; + } + } + + _isFunction(parsedStart) && (parsedStart = parsedStart(self)); + parsedStart = _parseClamp(parsedStart, "start", self); + start = _parsePosition(parsedStart, trigger, size, direction, scrollFunc(), markerStart, markerStartTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._startClamp && "_startClamp") || (pin ? -0.001 : 0); + _isFunction(parsedEnd) && (parsedEnd = parsedEnd(self)); + + if (_isString(parsedEnd) && !parsedEnd.indexOf("+=")) { + if (~parsedEnd.indexOf(" ")) { + parsedEnd = (_isString(parsedStart) ? parsedStart.split(" ")[0] : "") + parsedEnd; + } else { + offset = _offsetToPx(parsedEnd.substr(2), size); + parsedEnd = _isString(parsedStart) ? parsedStart : (containerAnimation ? gsap.utils.mapRange(0, containerAnimation.duration(), containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, start) : start) + offset; // _parsePosition won't factor in the offset if the start is a number, so do it here. + + parsedEndTrigger = trigger; + } + } + + parsedEnd = _parseClamp(parsedEnd, "end", self); + end = Math.max(start, _parsePosition(parsedEnd || (parsedEndTrigger ? "100% 0" : max), parsedEndTrigger, size, direction, scrollFunc() + offset, markerEnd, markerEndTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._endClamp && "_endClamp")) || -0.001; + offset = 0; + i = triggerIndex; + + while (i--) { + curTrigger = _triggers[i]; + curPin = curTrigger.pin; + + if (curPin && curTrigger.start - curTrigger._pinPush <= start && !containerAnimation && curTrigger.end > 0) { + cs = curTrigger.end - (self._startClamp ? Math.max(0, curTrigger.start) : curTrigger.start); + + if ((curPin === trigger && curTrigger.start - curTrigger._pinPush < start || curPin === pinnedContainer) && isNaN(parsedStart)) { + // numeric start values shouldn't be offset at all - treat them as absolute + offset += cs * (1 - curTrigger.progress); + } + + curPin === pin && (otherPinOffset += cs); + } + } + + start += offset; + end += offset; + self._startClamp && (self._startClamp += offset); + + if (self._endClamp && !_refreshingAll) { + self._endClamp = end || -0.001; + end = Math.min(end, _maxScroll(scroller, direction)); + } + + change = end - start || (start -= 0.01) && 0.001; + + if (isFirstRefresh) { + // on the very first refresh(), the prevProgress couldn't have been accurate yet because the start/end were never calculated, so we set it here. Before 3.11.5, it could lead to an inaccurate scroll position restoration with snapping. + prevProgress = gsap.utils.clamp(0, 1, gsap.utils.normalize(start, end, prevScroll)); + } + + self._pinPush = otherPinOffset; + + if (markerStart && offset) { + // offset the markers if necessary + cs = {}; + cs[direction.a] = "+=" + offset; + pinnedContainer && (cs[direction.p] = "-=" + scrollFunc()); + gsap.set([markerStart, markerEnd], cs); + } + + if (pin && !(_clampingMax && self.end >= _maxScroll(scroller, direction))) { + cs = _getComputedStyle(pin); + isVertical = direction === _vertical; + scroll = scrollFunc(); // recalculate because the triggers can affect the scroll + + pinStart = parseFloat(pinGetter(direction.a)) + otherPinOffset; + + if (!max && end > 1) { + // makes sure the scroller has a scrollbar, otherwise if something has width: 100%, for example, it would be too big (exclude the scrollbar). See https://gsap.com/forums/topic/25182-scrolltrigger-width-of-page-increase-where-markers-are-set-to-false/ + forcedOverflow = (isViewport ? _doc.scrollingElement || _docEl : scroller).style; + forcedOverflow = { + style: forcedOverflow, + value: forcedOverflow["overflow" + direction.a.toUpperCase()] + }; + + if (isViewport && _getComputedStyle(_body)["overflow" + direction.a.toUpperCase()] !== "scroll") { + // avoid an extra scrollbar if BOTH <html> and <body> have overflow set to "scroll" + forcedOverflow.style["overflow" + direction.a.toUpperCase()] = "scroll"; + } + } + + _swapPinIn(pin, spacer, cs); + + pinState = _getState(pin); // transforms will interfere with the top/left/right/bottom placement, so remove them temporarily. getBoundingClientRect() factors in transforms. + + bounds = _getBounds(pin, true); + oppositeScroll = useFixedPosition && _getScrollFunc(scroller, isVertical ? _horizontal : _vertical)(); + + if (pinSpacing) { + spacerState = [pinSpacing + direction.os2, change + otherPinOffset + _px]; + spacerState.t = spacer; + i = pinSpacing === _padding ? _getSize(pin, direction) + change + otherPinOffset : 0; + + if (i) { + spacerState.push(direction.d, i + _px); // for box-sizing: border-box (must include padding). + + spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + + _setState(spacerState); + + if (pinnedContainer) { + // in ScrollTrigger.refresh(), we need to re-evaluate the pinContainer's size because this pinSpacing may stretch it out, but we can't just add the exact distance because depending on layout, it may not push things down or it may only do so partially. + _triggers.forEach(function (t) { + if (t.pin === pinnedContainer && t.vars.pinSpacing !== false) { + t._subPinOffset = true; + } + }); + } + + useFixedPosition && scrollFunc(prevScroll); + } else { + i = _getSize(pin, direction); + i && spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + + if (useFixedPosition) { + override = { + top: bounds.top + (isVertical ? scroll - start : oppositeScroll) + _px, + left: bounds.left + (isVertical ? oppositeScroll : scroll - start) + _px, + boxSizing: "border-box", + position: "fixed" + }; + override[_width] = override["max" + _Width] = Math.ceil(bounds.width) + _px; + override[_height] = override["max" + _Height] = Math.ceil(bounds.height) + _px; + override[_margin] = override[_margin + _Top] = override[_margin + _Right] = override[_margin + _Bottom] = override[_margin + _Left] = "0"; + override[_padding] = cs[_padding]; + override[_padding + _Top] = cs[_padding + _Top]; + override[_padding + _Right] = cs[_padding + _Right]; + override[_padding + _Bottom] = cs[_padding + _Bottom]; + override[_padding + _Left] = cs[_padding + _Left]; + pinActiveState = _copyState(pinOriginalState, override, pinReparent); + _refreshingAll && scrollFunc(0); + } + + if (animation) { + // the animation might be affecting the transform, so we must jump to the end, check the value, and compensate accordingly. Otherwise, when it becomes unpinned, the pinSetter() will get set to a value that doesn't include whatever the animation did. + initted = animation._initted; // if not, we must invalidate() after this step, otherwise it could lock in starting values prematurely. + + _suppressOverwrites(1); + + animation.render(animation.duration(), true, true); + pinChange = pinGetter(direction.a) - pinStart + change + otherPinOffset; + pinMoves = Math.abs(change - pinChange) > 1; + useFixedPosition && pinMoves && pinActiveState.splice(pinActiveState.length - 2, 2); // transform is the last property/value set in the state Array. Since the animation is controlling that, we should omit it. + + animation.render(0, true, true); + initted || animation.invalidate(true); + animation.parent || animation.totalTime(animation.totalTime()); // if, for example, a toggleAction called play() and then refresh() happens and when we render(1) above, it would cause the animation to complete and get removed from its parent, so this makes sure it gets put back in. + + _suppressOverwrites(0); + } else { + pinChange = change; + } + + forcedOverflow && (forcedOverflow.value ? forcedOverflow.style["overflow" + direction.a.toUpperCase()] = forcedOverflow.value : forcedOverflow.style.removeProperty("overflow-" + direction.a)); + } else if (trigger && scrollFunc() && !containerAnimation) { + // it may be INSIDE a pinned element, so walk up the tree and look for any elements with _pinOffset to compensate because anything with pinSpacing that's already scrolled would throw off the measurements in getBoundingClientRect() + bounds = trigger.parentNode; + + while (bounds && bounds !== _body) { + if (bounds._pinOffset) { + start -= bounds._pinOffset; + end -= bounds._pinOffset; + } + + bounds = bounds.parentNode; + } + } + + revertedPins && revertedPins.forEach(function (t) { + return t.revert(false, true); + }); + self.start = start; + self.end = end; + scroll1 = scroll2 = _refreshingAll ? prevScroll : scrollFunc(); // reset velocity + + if (!containerAnimation && !_refreshingAll) { + scroll1 < prevScroll && scrollFunc(prevScroll); + self.scroll.rec = 0; + } + + self.revert(false, true); + lastRefresh = _getTime(); + + if (snapDelayedCall) { + lastSnap = -1; // just so snapping gets re-enabled, clear out any recorded last value + // self.isActive && scrollFunc(start + change * prevProgress); // previously this line was here to ensure that when snapping kicks in, it's from the previous progress but in some cases that's not desirable, like an all-page ScrollTrigger when new content gets added to the page, that'd totally change the progress. + + snapDelayedCall.restart(true); + } + + _refreshing = 0; + animation && isToggle && (animation._initted || prevAnimProgress) && animation.progress() !== prevAnimProgress && animation.progress(prevAnimProgress || 0, true).render(animation.time(), true, true); // must force a re-render because if saveStyles() was used on the target(s), the styles could have been wiped out during the refresh(). + + if (isFirstRefresh || prevProgress !== self.progress || containerAnimation || invalidateOnRefresh || animation && !animation._initted) { + // ensures that the direction is set properly (when refreshing, progress is set back to 0 initially, then back again to wherever it needs to be) and that callbacks are triggered. + animation && !isToggle && animation.totalProgress(containerAnimation && start < -0.001 && !prevProgress ? gsap.utils.normalize(start, end, 0) : prevProgress, true); // to avoid issues where animation callbacks like onStart aren't triggered. + + self.progress = isFirstRefresh || (scroll1 - start) / change === prevProgress ? 0 : prevProgress; + } + + pin && pinSpacing && (spacer._pinOffset = Math.round(self.progress * pinChange)); + scrubTween && scrubTween.invalidate(); + + if (!isNaN(markerStartOffset)) { + // numbers were passed in for the position which are absolute, so instead of just putting the markers at the very bottom of the viewport, we figure out how far they shifted down (it's safe to assume they were originally positioned in closer relation to the trigger element with values like "top", "center", a percentage or whatever, so we offset that much in the opposite direction to basically revert them to the relative position thy were at previously. + markerStartOffset -= gsap.getProperty(markerStartTrigger, direction.p); + markerEndOffset -= gsap.getProperty(markerEndTrigger, direction.p); + + _shiftMarker(markerStartTrigger, direction, markerStartOffset); + + _shiftMarker(markerStart, direction, markerStartOffset - (pinOffset || 0)); + + _shiftMarker(markerEndTrigger, direction, markerEndOffset); + + _shiftMarker(markerEnd, direction, markerEndOffset - (pinOffset || 0)); + } + + isFirstRefresh && !_refreshingAll && self.update(); // edge case - when you reload a page when it's already scrolled down, some browsers fire a "scroll" event before DOMContentLoaded, triggering an updateAll(). If we don't update the self.progress as part of refresh(), then when it happens next, it may record prevProgress as 0 when it really shouldn't, potentially causing a callback in an animation to fire again. + + if (onRefresh && !_refreshingAll && !executingOnRefresh) { + // when refreshing all, we do extra work to correct pinnedContainer sizes and ensure things don't exceed the maxScroll, so we should do all the refreshes at the end after all that work so that the start/end values are corrected. + executingOnRefresh = true; + onRefresh(self); + executingOnRefresh = false; + } + }; + + self.getVelocity = function () { + return (scrollFunc() - scroll2) / (_getTime() - _time2) * 1000 || 0; + }; + + self.endAnimation = function () { + _endAnimation(self.callbackAnimation); + + if (animation) { + scrubTween ? scrubTween.progress(1) : !animation.paused() ? _endAnimation(animation, animation.reversed()) : isToggle || _endAnimation(animation, self.direction < 0, 1); + } + }; + + self.labelToScroll = function (label) { + return animation && animation.labels && (start || self.refresh() || start) + animation.labels[label] / animation.duration() * change || 0; + }; + + self.getTrailing = function (name) { + var i = _triggers.indexOf(self), + a = self.direction > 0 ? _triggers.slice(0, i).reverse() : _triggers.slice(i + 1); + + return (_isString(name) ? a.filter(function (t) { + return t.vars.preventOverlaps === name; + }) : a).filter(function (t) { + return self.direction > 0 ? t.end <= start : t.start >= end; + }); + }; + + self.update = function (reset, recordVelocity, forceFake) { + if (containerAnimation && !forceFake && !reset) { + return; + } + + var scroll = _refreshingAll === true ? prevScroll : self.scroll(), + p = reset ? 0 : (scroll - start) / change, + clipped = p < 0 ? 0 : p > 1 ? 1 : p || 0, + prevProgress = self.progress, + isActive, + wasActive, + toggleState, + action, + stateChanged, + toggled, + isAtMax, + isTakingAction; + + if (recordVelocity) { + scroll2 = scroll1; + scroll1 = containerAnimation ? scrollFunc() : scroll; + + if (snap) { + snap2 = snap1; + snap1 = animation && !isToggle ? animation.totalProgress() : clipped; + } + } // anticipate the pinning a few ticks ahead of time based on velocity to avoid a visual glitch due to the fact that most browsers do scrolling on a separate thread (not synced with requestAnimationFrame). + + + if (anticipatePin && pin && !_refreshing && !_startup && _lastScrollTime) { + if (!clipped && start < scroll + (scroll - scroll2) / (_getTime() - _time2) * anticipatePin) { + clipped = 0.0001; + } else if (clipped === 1 && end > scroll + (scroll - scroll2) / (_getTime() - _time2) * anticipatePin) { + clipped = 0.9999; + } + } + + if (clipped !== prevProgress && self.enabled) { + isActive = self.isActive = !!clipped && clipped < 1; + wasActive = !!prevProgress && prevProgress < 1; + toggled = isActive !== wasActive; + stateChanged = toggled || !!clipped !== !!prevProgress; // could go from start all the way to end, thus it didn't toggle but it did change state in a sense (may need to fire a callback) + + self.direction = clipped > prevProgress ? 1 : -1; + self.progress = clipped; + + if (stateChanged && !_refreshing) { + toggleState = clipped && !prevProgress ? 0 : clipped === 1 ? 1 : prevProgress === 1 ? 2 : 3; // 0 = enter, 1 = leave, 2 = enterBack, 3 = leaveBack (we prioritize the FIRST encounter, thus if you scroll really fast past the onEnter and onLeave in one tick, it'd prioritize onEnter. + + if (isToggle) { + action = !toggled && toggleActions[toggleState + 1] !== "none" && toggleActions[toggleState + 1] || toggleActions[toggleState]; // if it didn't toggle, that means it shot right past and since we prioritize the "enter" action, we should switch to the "leave" in this case (but only if one is defined) + + isTakingAction = animation && (action === "complete" || action === "reset" || action in animation); + } + } + + preventOverlaps && (toggled || isTakingAction) && (isTakingAction || scrub || !animation) && (_isFunction(preventOverlaps) ? preventOverlaps(self) : self.getTrailing(preventOverlaps).forEach(function (t) { + return t.endAnimation(); + })); + + if (!isToggle) { + if (scrubTween && !_refreshing && !_startup) { + scrubTween._dp._time - scrubTween._start !== scrubTween._time && scrubTween.render(scrubTween._dp._time - scrubTween._start); // if there's a scrub on both the container animation and this one (or a ScrollSmoother), the update order would cause this one not to have rendered yet, so it wouldn't make any progress before we .restart() it heading toward the new progress so it'd appear stuck thus we force a render here. + + if (scrubTween.resetTo) { + scrubTween.resetTo("totalProgress", clipped, animation._tTime / animation._tDur); + } else { + // legacy support (courtesy), before 3.10.0 + scrubTween.vars.totalProgress = clipped; + scrubTween.invalidate().restart(); + } + } else if (animation) { + animation.totalProgress(clipped, !!(_refreshing && (lastRefresh || reset))); + } + } + + if (pin) { + reset && pinSpacing && (spacer.style[pinSpacing + direction.os2] = spacingStart); + + if (!useFixedPosition) { + pinSetter(_round(pinStart + pinChange * clipped)); + } else if (stateChanged) { + isAtMax = !reset && clipped > prevProgress && end + 1 > scroll && scroll + 1 >= _maxScroll(scroller, direction); // if it's at the VERY end of the page, don't switch away from position: fixed because it's pointless and it could cause a brief flash when the user scrolls back up (when it gets pinned again) + + if (pinReparent) { + if (!reset && (isActive || isAtMax)) { + var bounds = _getBounds(pin, true), + _offset = scroll - start; + + _reparent(pin, _body, bounds.top + (direction === _vertical ? _offset : 0) + _px, bounds.left + (direction === _vertical ? 0 : _offset) + _px); + } else { + _reparent(pin, spacer); + } + } + + _setState(isActive || isAtMax ? pinActiveState : pinState); + + pinMoves && clipped < 1 && isActive || pinSetter(pinStart + (clipped === 1 && !isAtMax ? pinChange : 0)); + } + } + + snap && !tweenTo.tween && !_refreshing && !_startup && snapDelayedCall.restart(true); + toggleClass && (toggled || once && clipped && (clipped < 1 || !_limitCallbacks)) && _toArray(toggleClass.targets).forEach(function (el) { + return el.classList[isActive || once ? "add" : "remove"](toggleClass.className); + }); // classes could affect positioning, so do it even if reset or refreshing is true. + + onUpdate && !isToggle && !reset && onUpdate(self); + + if (stateChanged && !_refreshing) { + if (isToggle) { + if (isTakingAction) { + if (action === "complete") { + animation.pause().totalProgress(1); + } else if (action === "reset") { + animation.restart(true).pause(); + } else if (action === "restart") { + animation.restart(true); + } else { + animation[action](); + } + } + + onUpdate && onUpdate(self); + } + + if (toggled || !_limitCallbacks) { + // on startup, the page could be scrolled and we don't want to fire callbacks that didn't toggle. For example onEnter shouldn't fire if the ScrollTrigger isn't actually entered. + onToggle && toggled && _callback(self, onToggle); + callbacks[toggleState] && _callback(self, callbacks[toggleState]); + once && (clipped === 1 ? self.kill(false, 1) : callbacks[toggleState] = 0); // a callback shouldn't be called again if once is true. + + if (!toggled) { + // it's possible to go completely past, like from before the start to after the end (or vice-versa) in which case BOTH callbacks should be fired in that order + toggleState = clipped === 1 ? 1 : 3; + callbacks[toggleState] && _callback(self, callbacks[toggleState]); + } + } + + if (fastScrollEnd && !isActive && Math.abs(self.getVelocity()) > (_isNumber(fastScrollEnd) ? fastScrollEnd : 2500)) { + _endAnimation(self.callbackAnimation); + + scrubTween ? scrubTween.progress(1) : _endAnimation(animation, action === "reverse" ? 1 : !clipped, 1); + } + } else if (isToggle && onUpdate && !_refreshing) { + onUpdate(self); + } + } // update absolutely-positioned markers (only if the scroller isn't the viewport) + + + if (markerEndSetter) { + var n = containerAnimation ? scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0) : scroll; + markerStartSetter(n + (markerStartTrigger._isFlipped ? 1 : 0)); + markerEndSetter(n); + } + + caMarkerSetter && caMarkerSetter(-scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0)); + }; + + self.enable = function (reset, refresh) { + if (!self.enabled) { + self.enabled = true; + + _addListener(scroller, "resize", _onResize); + + isViewport || _addListener(scroller, "scroll", _onScroll); + onRefreshInit && _addListener(ScrollTrigger, "refreshInit", onRefreshInit); + + if (reset !== false) { + self.progress = prevProgress = 0; + scroll1 = scroll2 = lastSnap = scrollFunc(); + } + + refresh !== false && self.refresh(); + } + }; + + self.getTween = function (snap) { + return snap && tweenTo ? tweenTo.tween : scrubTween; + }; + + self.setPositions = function (newStart, newEnd, keepClamp, pinOffset) { + // doesn't persist after refresh()! Intended to be a way to override values that were set during refresh(), like you could set it in onRefresh() + if (containerAnimation) { + // convert ratios into scroll positions. Remember, start/end values on ScrollTriggers that have a containerAnimation refer to the time (in seconds), NOT scroll positions. + var st = containerAnimation.scrollTrigger, + duration = containerAnimation.duration(), + _change = st.end - st.start; + + newStart = st.start + _change * newStart / duration; + newEnd = st.start + _change * newEnd / duration; + } + + self.refresh(false, false, { + start: _keepClamp(newStart, keepClamp && !!self._startClamp), + end: _keepClamp(newEnd, keepClamp && !!self._endClamp) + }, pinOffset); + self.update(); + }; + + self.adjustPinSpacing = function (amount) { + if (spacerState && amount) { + var i = spacerState.indexOf(direction.d) + 1; + spacerState[i] = parseFloat(spacerState[i]) + amount + _px; + spacerState[1] = parseFloat(spacerState[1]) + amount + _px; + + _setState(spacerState); + } + }; + + self.disable = function (reset, allowAnimation) { + if (self.enabled) { + reset !== false && self.revert(true, true); + self.enabled = self.isActive = false; + allowAnimation || scrubTween && scrubTween.pause(); + prevScroll = 0; + pinCache && (pinCache.uncache = 1); + onRefreshInit && _removeListener(ScrollTrigger, "refreshInit", onRefreshInit); + + if (snapDelayedCall) { + snapDelayedCall.pause(); + tweenTo.tween && tweenTo.tween.kill() && (tweenTo.tween = 0); + } + + if (!isViewport) { + var i = _triggers.length; + + while (i--) { + if (_triggers[i].scroller === scroller && _triggers[i] !== self) { + return; //don't remove the listeners if there are still other triggers referencing it. + } + } + + _removeListener(scroller, "resize", _onResize); + + isViewport || _removeListener(scroller, "scroll", _onScroll); + } + } + }; + + self.kill = function (revert, allowAnimation) { + self.disable(revert, allowAnimation); + scrubTween && !allowAnimation && scrubTween.kill(); + id && delete _ids[id]; + + var i = _triggers.indexOf(self); + + i >= 0 && _triggers.splice(i, 1); + i === _i && _direction > 0 && _i--; // if we're in the middle of a refresh() or update(), splicing would cause skips in the index, so adjust... + // if no other ScrollTrigger instances of the same scroller are found, wipe out any recorded scroll position. Otherwise, in a single page application, for example, it could maintain scroll position when it really shouldn't. + + i = 0; + + _triggers.forEach(function (t) { + return t.scroller === self.scroller && (i = 1); + }); + + i || _refreshingAll || (self.scroll.rec = 0); + + if (animation) { + animation.scrollTrigger = null; + revert && animation.revert({ + kill: false + }); + allowAnimation || animation.kill(); + } + + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(function (m) { + return m.parentNode && m.parentNode.removeChild(m); + }); + _primary === self && (_primary = 0); + + if (pin) { + pinCache && (pinCache.uncache = 1); + i = 0; + + _triggers.forEach(function (t) { + return t.pin === pin && i++; + }); + + i || (pinCache.spacer = 0); // if there aren't any more ScrollTriggers with the same pin, remove the spacer, otherwise it could be contaminated with old/stale values if the user re-creates a ScrollTrigger for the same element. + } + + vars.onKill && vars.onKill(self); + }; + + _triggers.push(self); + + self.enable(false, false); + customRevertReturn && customRevertReturn(self); + + if (animation && animation.add && !change) { + // if the animation is a timeline, it may not have been populated yet, so it wouldn't render at the proper place on the first refresh(), thus we should schedule one for the next tick. If "change" is defined, we know it must be re-enabling, thus we can refresh() right away. + var updateFunc = self.update; // some browsers may fire a scroll event BEFORE a tick elapses and/or the DOMContentLoaded fires. So there's a chance update() will be called BEFORE a refresh() has happened on a Timeline-attached ScrollTrigger which means the start/end won't be calculated yet. We don't want to add conditional logic inside the update() method (like check to see if end is defined and if not, force a refresh()) because that's a function that gets hit a LOT (performance). So we swap out the real update() method for this one that'll re-attach it the first time it gets called and of course forces a refresh(). + + self.update = function () { + self.update = updateFunc; + _scrollers.cache++; // otherwise a cached scroll position may get used in the refresh() in a very rare scenario, like if ScrollTriggers are created inside a DOMContentLoaded event and the queued requestAnimationFrame() fires beforehand. See https://gsap.com/community/forums/topic/41267-scrolltrigger-breaks-on-refresh-when-using-domcontentloaded/ + + start || end || self.refresh(); + }; + + gsap.delayedCall(0.01, self.update); + change = 0.01; + start = end = 0; + } else { + self.refresh(); + } + + pin && _queueRefreshAll(); // pinning could affect the positions of other things, so make sure we queue a full refresh() + }; + + ScrollTrigger.register = function register(core) { + if (!_coreInitted) { + gsap = core || _getGSAP(); + _windowExists() && window.document && ScrollTrigger.enable(); + _coreInitted = _enabled; + } + + return _coreInitted; + }; + + ScrollTrigger.defaults = function defaults(config) { + if (config) { + for (var p in config) { + _defaults[p] = config[p]; + } + } + + return _defaults; + }; + + ScrollTrigger.disable = function disable(reset, kill) { + _enabled = 0; + + _triggers.forEach(function (trigger) { + return trigger[kill ? "kill" : "disable"](reset); + }); + + _removeListener(_win, "wheel", _onScroll); + + _removeListener(_doc, "scroll", _onScroll); + + clearInterval(_syncInterval); + + _removeListener(_doc, "touchcancel", _passThrough); + + _removeListener(_body, "touchstart", _passThrough); + + _multiListener(_removeListener, _doc, "pointerdown,touchstart,mousedown", _pointerDownHandler); + + _multiListener(_removeListener, _doc, "pointerup,touchend,mouseup", _pointerUpHandler); + + _resizeDelay.kill(); + + _iterateAutoRefresh(_removeListener); + + for (var i = 0; i < _scrollers.length; i += 3) { + _wheelListener(_removeListener, _scrollers[i], _scrollers[i + 1]); + + _wheelListener(_removeListener, _scrollers[i], _scrollers[i + 2]); + } + }; + + ScrollTrigger.enable = function enable() { + _win = window; + _doc = document; + _docEl = _doc.documentElement; + _body = _doc.body; + + if (gsap) { + _toArray = gsap.utils.toArray; + _clamp = gsap.utils.clamp; + _context = gsap.core.context || _passThrough; + _suppressOverwrites = gsap.core.suppressOverwrites || _passThrough; + _scrollRestoration = _win.history.scrollRestoration || "auto"; + _lastScroll = _win.pageYOffset || 0; + gsap.core.globals("ScrollTrigger", ScrollTrigger); // must register the global manually because in Internet Explorer, functions (classes) don't have a "name" property. + + if (_body) { + _enabled = 1; + _div100vh = document.createElement("div"); // to solve mobile browser address bar show/hide resizing, we shouldn't rely on window.innerHeight. Instead, use a <div> with its height set to 100vh and measure that since that's what the scrolling is based on anyway and it's not affected by address bar showing/hiding. + + _div100vh.style.height = "100vh"; + _div100vh.style.position = "absolute"; + + _refresh100vh(); + + _rafBugFix(); + + Observer.register(gsap); // isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer. + + ScrollTrigger.isTouch = Observer.isTouch; + _fixIOSBug = Observer.isTouch && /(iPad|iPhone|iPod|Mac)/g.test(navigator.userAgent); // since 2017, iOS has had a bug that causes event.clientX/Y to be inaccurate when a scroll occurs, thus we must alternate ignoring every other touchmove event to work around it. See https://bugs.webkit.org/show_bug.cgi?id=181954 and https://codepen.io/GreenSock/pen/ExbrPNa/087cef197dc35445a0951e8935c41503 + + _ignoreMobileResize = Observer.isTouch === 1; + + _addListener(_win, "wheel", _onScroll); // mostly for 3rd party smooth scrolling libraries. + + + _root = [_win, _doc, _docEl, _body]; + + if (gsap.matchMedia) { + ScrollTrigger.matchMedia = function (vars) { + var mm = gsap.matchMedia(), + p; + + for (p in vars) { + mm.add(p, vars[p]); + } + + return mm; + }; + + gsap.addEventListener("matchMediaInit", function () { + return _revertAll(); + }); + gsap.addEventListener("matchMediaRevert", function () { + return _revertRecorded(); + }); + gsap.addEventListener("matchMedia", function () { + _refreshAll(0, 1); + + _dispatch("matchMedia"); + }); + gsap.matchMedia().add("(orientation: portrait)", function () { + // when orientation changes, we should take new base measurements for the ignoreMobileResize feature. + _setBaseDimensions(); + + return _setBaseDimensions; + }); + } else { + console.warn("Requires GSAP 3.11.0 or later"); + } + + _setBaseDimensions(); + + _addListener(_doc, "scroll", _onScroll); // some browsers (like Chrome), the window stops dispatching scroll events on the window if you scroll really fast, but it's consistent on the document! + + + var bodyHasStyle = _body.hasAttribute("style"), + bodyStyle = _body.style, + border = bodyStyle.borderTopStyle, + AnimationProto = gsap.core.Animation.prototype, + bounds, + i; + + AnimationProto.revert || Object.defineProperty(AnimationProto, "revert", { + value: function value() { + return this.time(-0.01, true); + } + }); // only for backwards compatibility (Animation.revert() was added after 3.10.4) + + bodyStyle.borderTopStyle = "solid"; // works around an issue where a margin of a child element could throw off the bounds of the _body, making it seem like there's a margin when there actually isn't. The border ensures that the bounds are accurate. + + bounds = _getBounds(_body); + _vertical.m = Math.round(bounds.top + _vertical.sc()) || 0; // accommodate the offset of the <body> caused by margins and/or padding + + _horizontal.m = Math.round(bounds.left + _horizontal.sc()) || 0; + border ? bodyStyle.borderTopStyle = border : bodyStyle.removeProperty("border-top-style"); + + if (!bodyHasStyle) { + // SSR frameworks like Next.js complain if this attribute gets added. + _body.setAttribute("style", ""); // it's not enough to just removeAttribute() - we must first set it to empty, otherwise Next.js complains. + + + _body.removeAttribute("style"); + } // TODO: (?) maybe move to leveraging the velocity mechanism in Observer and skip intervals. + + + _syncInterval = setInterval(_sync, 250); + gsap.delayedCall(0.5, function () { + return _startup = 0; + }); + + _addListener(_doc, "touchcancel", _passThrough); // some older Android devices intermittently stop dispatching "touchmove" events if we don't listen for "touchcancel" on the document. + + + _addListener(_body, "touchstart", _passThrough); //works around Safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/ + + + _multiListener(_addListener, _doc, "pointerdown,touchstart,mousedown", _pointerDownHandler); + + _multiListener(_addListener, _doc, "pointerup,touchend,mouseup", _pointerUpHandler); + + _transformProp = gsap.utils.checkPrefix("transform"); + + _stateProps.push(_transformProp); + + _coreInitted = _getTime(); + _resizeDelay = gsap.delayedCall(0.2, _refreshAll).pause(); + _autoRefresh = [_doc, "visibilitychange", function () { + var w = _win.innerWidth, + h = _win.innerHeight; + + if (_doc.hidden) { + _prevWidth = w; + _prevHeight = h; + } else if (_prevWidth !== w || _prevHeight !== h) { + _onResize(); + } + }, _doc, "DOMContentLoaded", _refreshAll, _win, "load", _refreshAll, _win, "resize", _onResize]; + + _iterateAutoRefresh(_addListener); + + _triggers.forEach(function (trigger) { + return trigger.enable(0, 1); + }); + + for (i = 0; i < _scrollers.length; i += 3) { + _wheelListener(_removeListener, _scrollers[i], _scrollers[i + 1]); + + _wheelListener(_removeListener, _scrollers[i], _scrollers[i + 2]); + } + } + } + }; + + ScrollTrigger.config = function config(vars) { + "limitCallbacks" in vars && (_limitCallbacks = !!vars.limitCallbacks); + var ms = vars.syncInterval; + ms && clearInterval(_syncInterval) || (_syncInterval = ms) && setInterval(_sync, ms); + "ignoreMobileResize" in vars && (_ignoreMobileResize = ScrollTrigger.isTouch === 1 && vars.ignoreMobileResize); + + if ("autoRefreshEvents" in vars) { + _iterateAutoRefresh(_removeListener) || _iterateAutoRefresh(_addListener, vars.autoRefreshEvents || "none"); + _ignoreResize = (vars.autoRefreshEvents + "").indexOf("resize") === -1; + } + }; + + ScrollTrigger.scrollerProxy = function scrollerProxy(target, vars) { + var t = _getTarget(target), + i = _scrollers.indexOf(t), + isViewport = _isViewport(t); + + if (~i) { + _scrollers.splice(i, isViewport ? 6 : 2); + } + + if (vars) { + isViewport ? _proxies.unshift(_win, vars, _body, vars, _docEl, vars) : _proxies.unshift(t, vars); + } + }; + + ScrollTrigger.clearMatchMedia = function clearMatchMedia(query) { + _triggers.forEach(function (t) { + return t._ctx && t._ctx.query === query && t._ctx.kill(true, true); + }); + }; + + ScrollTrigger.isInViewport = function isInViewport(element, ratio, horizontal) { + var bounds = (_isString(element) ? _getTarget(element) : element).getBoundingClientRect(), + offset = bounds[horizontal ? _width : _height] * ratio || 0; + return horizontal ? bounds.right - offset > 0 && bounds.left + offset < _win.innerWidth : bounds.bottom - offset > 0 && bounds.top + offset < _win.innerHeight; + }; + + ScrollTrigger.positionInViewport = function positionInViewport(element, referencePoint, horizontal) { + _isString(element) && (element = _getTarget(element)); + var bounds = element.getBoundingClientRect(), + size = bounds[horizontal ? _width : _height], + offset = referencePoint == null ? size / 2 : referencePoint in _keywords ? _keywords[referencePoint] * size : ~referencePoint.indexOf("%") ? parseFloat(referencePoint) * size / 100 : parseFloat(referencePoint) || 0; + return horizontal ? (bounds.left + offset) / _win.innerWidth : (bounds.top + offset) / _win.innerHeight; + }; + + ScrollTrigger.killAll = function killAll(allowListeners) { + _triggers.slice(0).forEach(function (t) { + return t.vars.id !== "ScrollSmoother" && t.kill(); + }); + + if (allowListeners !== true) { + var listeners = _listeners.killAll || []; + _listeners = {}; + listeners.forEach(function (f) { + return f(); + }); + } + }; + + return ScrollTrigger; +}(); +ScrollTrigger.version = "3.12.7"; + +ScrollTrigger.saveStyles = function (targets) { + return targets ? _toArray(targets).forEach(function (target) { + // saved styles are recorded in a consecutive alternating Array, like [element, cssText, transform attribute, cache, matchMedia, ...] + if (target && target.style) { + var i = _savedStyles.indexOf(target); + + i >= 0 && _savedStyles.splice(i, 5); + + _savedStyles.push(target, target.style.cssText, target.getBBox && target.getAttribute("transform"), gsap.core.getCache(target), _context()); + } + }) : _savedStyles; +}; + +ScrollTrigger.revert = function (soft, media) { + return _revertAll(!soft, media); +}; + +ScrollTrigger.create = function (vars, animation) { + return new ScrollTrigger(vars, animation); +}; + +ScrollTrigger.refresh = function (safe) { + return safe ? _onResize(true) : (_coreInitted || ScrollTrigger.register()) && _refreshAll(true); +}; + +ScrollTrigger.update = function (force) { + return ++_scrollers.cache && _updateAll(force === true ? 2 : 0); +}; + +ScrollTrigger.clearScrollMemory = _clearScrollMemory; + +ScrollTrigger.maxScroll = function (element, horizontal) { + return _maxScroll(element, horizontal ? _horizontal : _vertical); +}; + +ScrollTrigger.getScrollFunc = function (element, horizontal) { + return _getScrollFunc(_getTarget(element), horizontal ? _horizontal : _vertical); +}; + +ScrollTrigger.getById = function (id) { + return _ids[id]; +}; + +ScrollTrigger.getAll = function () { + return _triggers.filter(function (t) { + return t.vars.id !== "ScrollSmoother"; + }); +}; // it's common for people to ScrollTrigger.getAll(t => t.kill()) on page routes, for example, and we don't want it to ruin smooth scrolling by killing the main ScrollSmoother one. + + +ScrollTrigger.isScrolling = function () { + return !!_lastScrollTime; +}; + +ScrollTrigger.snapDirectional = _snapDirectional; + +ScrollTrigger.addEventListener = function (type, callback) { + var a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); +}; + +ScrollTrigger.removeEventListener = function (type, callback) { + var a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); +}; + +ScrollTrigger.batch = function (targets, vars) { + var result = [], + varsCopy = {}, + interval = vars.interval || 0.016, + batchMax = vars.batchMax || 1e9, + proxyCallback = function proxyCallback(type, callback) { + var elements = [], + triggers = [], + delay = gsap.delayedCall(interval, function () { + callback(elements, triggers); + elements = []; + triggers = []; + }).pause(); + return function (self) { + elements.length || delay.restart(true); + elements.push(self.trigger); + triggers.push(self); + batchMax <= elements.length && delay.progress(1); + }; + }, + p; + + for (p in vars) { + varsCopy[p] = p.substr(0, 2) === "on" && _isFunction(vars[p]) && p !== "onRefreshInit" ? proxyCallback(p, vars[p]) : vars[p]; + } + + if (_isFunction(batchMax)) { + batchMax = batchMax(); + + _addListener(ScrollTrigger, "refresh", function () { + return batchMax = vars.batchMax(); + }); + } + + _toArray(targets).forEach(function (target) { + var config = {}; + + for (p in varsCopy) { + config[p] = varsCopy[p]; + } + + config.trigger = target; + result.push(ScrollTrigger.create(config)); + }); + + return result; +}; // to reduce file size. clamps the scroll and also returns a duration multiplier so that if the scroll gets chopped shorter, the duration gets curtailed as well (otherwise if you're very close to the top of the page, for example, and swipe up really fast, it'll suddenly slow down and take a long time to reach the top). + + +var _clampScrollAndGetDurationMultiplier = function _clampScrollAndGetDurationMultiplier(scrollFunc, current, end, max) { + current > max ? scrollFunc(max) : current < 0 && scrollFunc(0); + return end > max ? (max - current) / (end - current) : end < 0 ? current / (current - end) : 1; +}, + _allowNativePanning = function _allowNativePanning(target, direction) { + if (direction === true) { + target.style.removeProperty("touch-action"); + } else { + target.style.touchAction = direction === true ? "auto" : direction ? "pan-" + direction + (Observer.isTouch ? " pinch-zoom" : "") : "none"; // note: Firefox doesn't support it pinch-zoom properly, at least in addition to a pan-x or pan-y. + } + + target === _docEl && _allowNativePanning(_body, direction); +}, + _overflow = { + auto: 1, + scroll: 1 +}, + _nestedScroll = function _nestedScroll(_ref5) { + var event = _ref5.event, + target = _ref5.target, + axis = _ref5.axis; + + var node = (event.changedTouches ? event.changedTouches[0] : event).target, + cache = node._gsap || gsap.core.getCache(node), + time = _getTime(), + cs; + + if (!cache._isScrollT || time - cache._isScrollT > 2000) { + // cache for 2 seconds to improve performance. + while (node && node !== _body && (node.scrollHeight <= node.clientHeight && node.scrollWidth <= node.clientWidth || !(_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]))) { + node = node.parentNode; + } + + cache._isScroll = node && node !== target && !_isViewport(node) && (_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]); + cache._isScrollT = time; + } + + if (cache._isScroll || axis === "x") { + event.stopPropagation(); + event._gsapAllow = true; + } +}, + // capture events on scrollable elements INSIDE the <body> and allow those by calling stopPropagation() when we find a scrollable ancestor +_inputObserver = function _inputObserver(target, type, inputs, nested) { + return Observer.create({ + target: target, + capture: true, + debounce: false, + lockAxis: true, + type: type, + onWheel: nested = nested && _nestedScroll, + onPress: nested, + onDrag: nested, + onScroll: nested, + onEnable: function onEnable() { + return inputs && _addListener(_doc, Observer.eventTypes[0], _captureInputs, false, true); + }, + onDisable: function onDisable() { + return _removeListener(_doc, Observer.eventTypes[0], _captureInputs, true); + } + }); +}, + _inputExp = /(input|label|select|textarea)/i, + _inputIsFocused, + _captureInputs = function _captureInputs(e) { + var isInput = _inputExp.test(e.target.tagName); + + if (isInput || _inputIsFocused) { + e._gsapAllow = true; + _inputIsFocused = isInput; + } +}, + _getScrollNormalizer = function _getScrollNormalizer(vars) { + _isObject(vars) || (vars = {}); + vars.preventDefault = vars.isNormalizer = vars.allowClicks = true; + vars.type || (vars.type = "wheel,touch"); + vars.debounce = !!vars.debounce; + vars.id = vars.id || "normalizer"; + + var _vars2 = vars, + normalizeScrollX = _vars2.normalizeScrollX, + momentum = _vars2.momentum, + allowNestedScroll = _vars2.allowNestedScroll, + onRelease = _vars2.onRelease, + self, + maxY, + target = _getTarget(vars.target) || _docEl, + smoother = gsap.core.globals().ScrollSmoother, + smootherInstance = smoother && smoother.get(), + content = _fixIOSBug && (vars.content && _getTarget(vars.content) || smootherInstance && vars.content !== false && !smootherInstance.smooth() && smootherInstance.content()), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollFuncX = _getScrollFunc(target, _horizontal), + scale = 1, + initialScale = (Observer.isTouch && _win.visualViewport ? _win.visualViewport.scale * _win.visualViewport.width : _win.outerWidth) / _win.innerWidth, + wheelRefresh = 0, + resolveMomentumDuration = _isFunction(momentum) ? function () { + return momentum(self); + } : function () { + return momentum || 2.8; + }, + lastRefreshID, + skipTouchMove, + inputObserver = _inputObserver(target, vars.type, true, allowNestedScroll), + resumeTouchMove = function resumeTouchMove() { + return skipTouchMove = false; + }, + scrollClampX = _passThrough, + scrollClampY = _passThrough, + updateClamps = function updateClamps() { + maxY = _maxScroll(target, _vertical); + scrollClampY = _clamp(_fixIOSBug ? 1 : 0, maxY); + normalizeScrollX && (scrollClampX = _clamp(0, _maxScroll(target, _horizontal))); + lastRefreshID = _refreshID; + }, + removeContentOffset = function removeContentOffset() { + content._gsap.y = _round(parseFloat(content._gsap.y) + scrollFuncY.offset) + "px"; + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + parseFloat(content._gsap.y) + ", 0, 1)"; + scrollFuncY.offset = scrollFuncY.cacheID = 0; + }, + ignoreDrag = function ignoreDrag() { + if (skipTouchMove) { + requestAnimationFrame(resumeTouchMove); + + var offset = _round(self.deltaY / 2), + scroll = scrollClampY(scrollFuncY.v - offset); + + if (content && scroll !== scrollFuncY.v + scrollFuncY.offset) { + scrollFuncY.offset = scroll - scrollFuncY.v; + + var y = _round((parseFloat(content && content._gsap.y) || 0) - scrollFuncY.offset); + + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + y + ", 0, 1)"; + content._gsap.y = y + "px"; + scrollFuncY.cacheID = _scrollers.cache; + + _updateAll(); + } + + return true; + } + + scrollFuncY.offset && removeContentOffset(); + skipTouchMove = true; + }, + tween, + startScrollX, + startScrollY, + onStopDelayedCall, + onResize = function onResize() { + // if the window resizes, like on an iPhone which Apple FORCES the address bar to show/hide even if we event.preventDefault(), it may be scrolling too far now that the address bar is showing, so we must dynamically adjust the momentum tween. + updateClamps(); + + if (tween.isActive() && tween.vars.scrollY > maxY) { + scrollFuncY() > maxY ? tween.progress(1) && scrollFuncY(maxY) : tween.resetTo("scrollY", maxY); + } + }; + + content && gsap.set(content, { + y: "+=0" + }); // to ensure there's a cache (element._gsap) + + vars.ignoreCheck = function (e) { + return _fixIOSBug && e.type === "touchmove" && ignoreDrag(e) || scale > 1.05 && e.type !== "touchstart" || self.isGesturing || e.touches && e.touches.length > 1; + }; + + vars.onPress = function () { + skipTouchMove = false; + var prevScale = scale; + scale = _round((_win.visualViewport && _win.visualViewport.scale || 1) / initialScale); + tween.pause(); + prevScale !== scale && _allowNativePanning(target, scale > 1.01 ? true : normalizeScrollX ? false : "x"); + startScrollX = scrollFuncX(); + startScrollY = scrollFuncY(); + updateClamps(); + lastRefreshID = _refreshID; + }; + + vars.onRelease = vars.onGestureStart = function (self, wasDragging) { + scrollFuncY.offset && removeContentOffset(); + + if (!wasDragging) { + onStopDelayedCall.restart(true); + } else { + _scrollers.cache++; // make sure we're pulling the non-cached value + // alternate algorithm: durX = Math.min(6, Math.abs(self.velocityX / 800)), dur = Math.max(durX, Math.min(6, Math.abs(self.velocityY / 800))); dur = dur * (0.4 + (1 - _power4In(dur / 6)) * 0.6)) * (momentumSpeed || 1) + + var dur = resolveMomentumDuration(), + currentScroll, + endScroll; + + if (normalizeScrollX) { + currentScroll = scrollFuncX(); + endScroll = currentScroll + dur * 0.05 * -self.velocityX / 0.227; // the constant .227 is from power4(0.05). velocity is inverted because scrolling goes in the opposite direction. + + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncX, currentScroll, endScroll, _maxScroll(target, _horizontal)); + tween.vars.scrollX = scrollClampX(endScroll); + } + + currentScroll = scrollFuncY(); + endScroll = currentScroll + dur * 0.05 * -self.velocityY / 0.227; // the constant .227 is from power4(0.05) + + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncY, currentScroll, endScroll, _maxScroll(target, _vertical)); + tween.vars.scrollY = scrollClampY(endScroll); + tween.invalidate().duration(dur).play(0.01); + + if (_fixIOSBug && tween.vars.scrollY >= maxY || currentScroll >= maxY - 1) { + // iOS bug: it'll show the address bar but NOT fire the window "resize" event until the animation is done but we must protect against overshoot so we leverage an onUpdate to do so. + gsap.to({}, { + onUpdate: onResize, + duration: dur + }); + } + } + + onRelease && onRelease(self); + }; + + vars.onWheel = function () { + tween._ts && tween.pause(); + + if (_getTime() - wheelRefresh > 1000) { + // after 1 second, refresh the clamps otherwise that'll only happen when ScrollTrigger.refresh() is called or for touch-scrolling. + lastRefreshID = 0; + wheelRefresh = _getTime(); + } + }; + + vars.onChange = function (self, dx, dy, xArray, yArray) { + _refreshID !== lastRefreshID && updateClamps(); + dx && normalizeScrollX && scrollFuncX(scrollClampX(xArray[2] === dx ? startScrollX + (self.startX - self.x) : scrollFuncX() + dx - xArray[1])); // for more precision, we track pointer/touch movement from the start, otherwise it'll drift. + + if (dy) { + scrollFuncY.offset && removeContentOffset(); + var isTouch = yArray[2] === dy, + y = isTouch ? startScrollY + self.startY - self.y : scrollFuncY() + dy - yArray[1], + yClamped = scrollClampY(y); + isTouch && y !== yClamped && (startScrollY += yClamped - y); + scrollFuncY(yClamped); + } + + (dy || dx) && _updateAll(); + }; + + vars.onEnable = function () { + _allowNativePanning(target, normalizeScrollX ? false : "x"); + + ScrollTrigger.addEventListener("refresh", onResize); + + _addListener(_win, "resize", onResize); + + if (scrollFuncY.smooth) { + scrollFuncY.target.style.scrollBehavior = "auto"; + scrollFuncY.smooth = scrollFuncX.smooth = false; + } + + inputObserver.enable(); + }; + + vars.onDisable = function () { + _allowNativePanning(target, true); + + _removeListener(_win, "resize", onResize); + + ScrollTrigger.removeEventListener("refresh", onResize); + inputObserver.kill(); + }; + + vars.lockAxis = vars.lockAxis !== false; + self = new Observer(vars); + self.iOS = _fixIOSBug; // used in the Observer getCachedScroll() function to work around an iOS bug that wreaks havoc with TouchEvent.clientY if we allow scroll to go all the way back to 0. + + _fixIOSBug && !scrollFuncY() && scrollFuncY(1); // iOS bug causes event.clientY values to freak out (wildly inaccurate) if the scroll position is exactly 0. + + _fixIOSBug && gsap.ticker.add(_passThrough); // prevent the ticker from sleeping + + onStopDelayedCall = self._dc; + tween = gsap.to(self, { + ease: "power4", + paused: true, + inherit: false, + scrollX: normalizeScrollX ? "+=0.1" : "+=0", + scrollY: "+=0.1", + modifiers: { + scrollY: _interruptionTracker(scrollFuncY, scrollFuncY(), function () { + return tween.pause(); + }) + }, + onUpdate: _updateAll, + onComplete: onStopDelayedCall.vars.onComplete + }); // we need the modifier to sense if the scroll position is altered outside of the momentum tween (like with a scrollTo tween) so we can pause() it to prevent conflicts. + + return self; +}; + +ScrollTrigger.sort = function (func) { + if (_isFunction(func)) { + return _triggers.sort(func); + } + + var scroll = _win.pageYOffset || 0; + ScrollTrigger.getAll().forEach(function (t) { + return t._sortY = t.trigger ? scroll + t.trigger.getBoundingClientRect().top : t.start + _win.innerHeight; + }); + return _triggers.sort(func || function (a, b) { + return (a.vars.refreshPriority || 0) * -1e6 + (a.vars.containerAnimation ? 1e6 : a._sortY) - ((b.vars.containerAnimation ? 1e6 : b._sortY) + (b.vars.refreshPriority || 0) * -1e6); + }); // anything with a containerAnimation should refresh last. +}; + +ScrollTrigger.observe = function (vars) { + return new Observer(vars); +}; + +ScrollTrigger.normalizeScroll = function (vars) { + if (typeof vars === "undefined") { + return _normalizer; + } + + if (vars === true && _normalizer) { + return _normalizer.enable(); + } + + if (vars === false) { + _normalizer && _normalizer.kill(); + _normalizer = vars; + return; + } + + var normalizer = vars instanceof Observer ? vars : _getScrollNormalizer(vars); + _normalizer && _normalizer.target === normalizer.target && _normalizer.kill(); + _isViewport(normalizer.target) && (_normalizer = normalizer); + return normalizer; +}; + +ScrollTrigger.core = { + // smaller file size way to leverage in ScrollSmoother and Observer + _getVelocityProp: _getVelocityProp, + _inputObserver: _inputObserver, + _scrollers: _scrollers, + _proxies: _proxies, + bridge: { + // when normalizeScroll sets the scroll position (ss = setScroll) + ss: function ss() { + _lastScrollTime || _dispatch("scrollStart"); + _lastScrollTime = _getTime(); + }, + // a way to get the _refreshing value in Observer + ref: function ref() { + return _refreshing; + } + } +}; +_getGSAP() && gsap.registerPlugin(ScrollTrigger); +export { ScrollTrigger as default }; \ No newline at end of file diff --git a/node_modules/gsap/TextPlugin.js b/node_modules/gsap/TextPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..fa4204242bd50f872574ff5b4c2833b3ed198a5a --- /dev/null +++ b/node_modules/gsap/TextPlugin.js @@ -0,0 +1,166 @@ +/*! + * TextPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +import { emojiSafeSplit, getText, splitInnerHTML } from "./utils/strings.js"; + +var gsap, + _tempDiv, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; +}; + +export var TextPlugin = { + version: "3.12.7", + name: "text", + init: function init(target, value, tween) { + typeof value !== "object" && (value = { + value: value + }); + + var i = target.nodeName.toUpperCase(), + data = this, + _value = value, + newClass = _value.newClass, + oldClass = _value.oldClass, + preserveSpaces = _value.preserveSpaces, + rtl = _value.rtl, + delimiter = data.delimiter = value.delimiter || "", + fillChar = data.fillChar = value.fillChar || (value.padSpace ? " " : ""), + _short, + text, + original, + j, + condensedText, + condensedOriginal, + aggregate, + s; + + data.svg = target.getBBox && (i === "TEXT" || i === "TSPAN"); + + if (!("innerHTML" in target) && !data.svg) { + return false; + } + + data.target = target; + + if (!("value" in value)) { + data.text = data.original = [""]; + return; + } + + original = splitInnerHTML(target, delimiter, false, preserveSpaces, data.svg); + _tempDiv || (_tempDiv = document.createElement("div")); + _tempDiv.innerHTML = value.value; + text = splitInnerHTML(_tempDiv, delimiter, false, preserveSpaces, data.svg); + data.from = tween._from; + + if ((data.from || rtl) && !(rtl && data.from)) { + // right-to-left or "from()" tweens should invert things (but if it's BOTH .from() and rtl, inverting twice equals not inverting at all :) + i = original; + original = text; + text = i; + } + + data.hasClass = !!(newClass || oldClass); + data.newClass = rtl ? oldClass : newClass; + data.oldClass = rtl ? newClass : oldClass; + i = original.length - text.length; + _short = i < 0 ? original : text; + + if (i < 0) { + i = -i; + } + + while (--i > -1) { + _short.push(fillChar); + } + + if (value.type === "diff") { + j = 0; + condensedText = []; + condensedOriginal = []; + aggregate = ""; + + for (i = 0; i < text.length; i++) { + s = text[i]; + + if (s === original[i]) { + aggregate += s; + } else { + condensedText[j] = aggregate + s; + condensedOriginal[j++] = aggregate + original[i]; + aggregate = ""; + } + } + + text = condensedText; + original = condensedOriginal; + + if (aggregate) { + text.push(aggregate); + original.push(aggregate); + } + } + + value.speed && tween.duration(Math.min(0.05 / value.speed * _short.length, value.maxDuration || 9999)); + data.rtl = rtl; + data.original = original; + data.text = text; + + data._props.push("text"); + }, + render: function render(ratio, data) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + + if (data.from) { + ratio = 1 - ratio; + } + + var text = data.text, + hasClass = data.hasClass, + newClass = data.newClass, + oldClass = data.oldClass, + delimiter = data.delimiter, + target = data.target, + fillChar = data.fillChar, + original = data.original, + rtl = data.rtl, + l = text.length, + i = (rtl ? 1 - ratio : ratio) * l + 0.5 | 0, + applyNew, + applyOld, + str; + + if (hasClass && ratio) { + applyNew = newClass && i; + applyOld = oldClass && i !== l; + str = (applyNew ? "<span class='" + newClass + "'>" : "") + text.slice(0, i).join(delimiter) + (applyNew ? "</span>" : "") + (applyOld ? "<span class='" + oldClass + "'>" : "") + delimiter + original.slice(i).join(delimiter) + (applyOld ? "</span>" : ""); + } else { + str = text.slice(0, i).join(delimiter) + delimiter + original.slice(i).join(delimiter); + } + + if (data.svg) { + //SVG text elements don't have an "innerHTML" in Microsoft browsers. + target.textContent = str; + } else { + target.innerHTML = fillChar === " " && ~str.indexOf(" ") ? str.split(" ").join(" ") : str; + } + } +}; +TextPlugin.splitInnerHTML = splitInnerHTML; +TextPlugin.emojiSafeSplit = emojiSafeSplit; +TextPlugin.getText = getText; +_getGSAP() && gsap.registerPlugin(TextPlugin); +export { TextPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/all.js b/node_modules/gsap/all.js new file mode 100644 index 0000000000000000000000000000000000000000..137ac7762245c630dc78eee7caa2a48b07b2d9c5 --- /dev/null +++ b/node_modules/gsap/all.js @@ -0,0 +1,31 @@ +import gsap from "./gsap-core.js"; +import CSSPlugin from "./CSSPlugin.js"; +var gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, + // to protect from tree shaking +TweenMaxWithCSS = gsapWithCSS.core.Tween; +export { gsapWithCSS as gsap, gsapWithCSS as default, TweenMaxWithCSS as TweenMax, CSSPlugin }; +export { TweenLite, TimelineMax, TimelineLite, Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ, wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, mapRange, pipe, unitize, interpolate, shuffle, selector } from "./gsap-core.js"; +export * from "./CustomEase.js"; +export * from "./Draggable.js"; +export * from "./CSSRulePlugin.js"; +export * from "./EaselPlugin.js"; +export * from "./EasePack.js"; +export * from "./Flip.js"; +export * from "./MotionPathPlugin.js"; +export * from "./Observer.js"; +export * from "./PixiPlugin.js"; +export * from "./ScrollToPlugin.js"; +export * from "./ScrollTrigger.js"; +export * from "./TextPlugin.js"; //BONUS EXPORTS +// export * from "./DrawSVGPlugin.js"; +// export * from "./Physics2DPlugin.js"; +// export * from "./PhysicsPropsPlugin.js"; +// export * from "./ScrambleTextPlugin.js"; +// export * from "./CustomBounce.js"; +// export * from "./CustomWiggle.js"; +// export * from "./GSDevTools.js"; +// export * from "./InertiaPlugin.js"; +// export * from "./MorphSVGPlugin.js"; +// export * from "./MotionPathHelper.js"; +// export * from "./ScrollSmoother.js"; +// export * from "./SplitText.js"; \ No newline at end of file diff --git a/node_modules/gsap/dist/CSSRulePlugin.js b/node_modules/gsap/dist/CSSRulePlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..69df1e23dd6787f3c18f623d12df3ada42a64d6c --- /dev/null +++ b/node_modules/gsap/dist/CSSRulePlugin.js @@ -0,0 +1,140 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + /*! + * CSSRulePlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _coreInitted, + _doc, + CSSPlugin, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _checkRegister = function _checkRegister() { + if (!_coreInitted) { + _initCore(); + + if (!CSSPlugin) { + console.warn("Please gsap.registerPlugin(CSSPlugin, CSSRulePlugin)"); + } + } + + return _coreInitted; + }, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (_windowExists()) { + _doc = document; + } + + if (gsap) { + CSSPlugin = gsap.plugins.css; + + if (CSSPlugin) { + _coreInitted = 1; + } + } + }; + + var CSSRulePlugin = { + version: "3.12.7", + name: "cssRule", + init: function init(target, value, tween, index, targets) { + if (!_checkRegister() || typeof target.cssText === "undefined") { + return false; + } + + var div = target._gsProxy = target._gsProxy || _doc.createElement("div"); + + this.ss = target; + this.style = div.style; + div.style.cssText = target.cssText; + CSSPlugin.prototype.init.call(this, div, value, tween, index, targets); + }, + render: function render(ratio, data) { + var pt = data._pt, + style = data.style, + ss = data.ss, + i; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + i = style.length; + + while (--i > -1) { + ss[style[i]] = style[style[i]]; + } + }, + getRule: function getRule(selector) { + _checkRegister(); + + var ruleProp = _doc.all ? "rules" : "cssRules", + styleSheets = _doc.styleSheets, + i = styleSheets.length, + pseudo = selector.charAt(0) === ":", + j, + curSS, + cs, + a; + selector = (pseudo ? "" : ",") + selector.split("::").join(":").toLowerCase() + ","; + + if (pseudo) { + a = []; + } + + while (i--) { + try { + curSS = styleSheets[i][ruleProp]; + + if (!curSS) { + continue; + } + + j = curSS.length; + } catch (e) { + console.warn(e); + continue; + } + + while (--j > -1) { + cs = curSS[j]; + + if (cs.selectorText && ("," + cs.selectorText.split("::").join(":").toLowerCase() + ",").indexOf(selector) !== -1) { + if (pseudo) { + a.push(cs.style); + } else { + return cs.style; + } + } + } + } + + return a; + }, + register: _initCore + }; + _getGSAP() && gsap.registerPlugin(CSSRulePlugin); + + exports.CSSRulePlugin = CSSRulePlugin; + exports.default = CSSRulePlugin; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/CSSRulePlugin.min.js b/node_modules/gsap/dist/CSSRulePlugin.min.js new file mode 100644 index 0000000000000000000000000000000000000000..287eccdd5572bb96964f18ea907eda3098cdc91a --- /dev/null +++ b/node_modules/gsap/dist/CSSRulePlugin.min.js @@ -0,0 +1,11 @@ +/*! + * CSSRulePlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(e){"use strict";function h(){return"undefined"!=typeof window}function i(){return t||h()&&(t=window.gsap)&&t.registerPlugin&&t}function j(){return n||(s(),o||console.warn("Please gsap.registerPlugin(CSSPlugin, CSSRulePlugin)")),n}var t,n,c,o,s=function _initCore(e){t=e||i(),h()&&(c=document),t&&(o=t.plugins.css)&&(n=1)},r={version:"3.12.7",name:"cssRule",init:function init(e,t,n,i,s){if(!j()||void 0===e.cssText)return!1;var r=e._gsProxy=e._gsProxy||c.createElement("div");this.ss=e,this.style=r.style,r.style.cssText=e.cssText,o.prototype.init.call(this,r,t,n,i,s)},render:function render(e,t){for(var n,i=t._pt,s=t.style,r=t.ss;i;)i.r(e,i.d),i=i._next;for(n=s.length;-1<--n;)r[s[n]]=s[s[n]]},getRule:function getRule(e){j();var t,n,i,s,r=c.all?"rules":"cssRules",o=c.styleSheets,l=o.length,u=":"===e.charAt(0);for(e=(u?"":",")+e.split("::").join(":").toLowerCase()+",",u&&(s=[]);l--;){try{if(!(n=o[l][r]))continue;t=n.length}catch(e){console.warn(e);continue}for(;-1<--t;)if((i=n[t]).selectorText&&-1!==(","+i.selectorText.split("::").join(":").toLowerCase()+",").indexOf(e)){if(!u)return i.style;s.push(i.style)}}return s},register:s};i()&&t.registerPlugin(r),e.CSSRulePlugin=r,e.default=r;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/CSSRulePlugin.min.js.map b/node_modules/gsap/dist/CSSRulePlugin.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..d0193c9dbbed7500c6d4595d4c9c851c4d4a5868 --- /dev/null +++ b/node_modules/gsap/dist/CSSRulePlugin.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"CSSRulePlugin.min.js","sources":["../src/CSSRulePlugin.js"],"sourcesContent":["/*!\n * CSSRulePlugin 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _coreInitted, _win, _doc, CSSPlugin,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_checkRegister = () => {\n\t\tif (!_coreInitted) {\n\t\t\t_initCore();\n\t\t\tif (!CSSPlugin) {\n\t\t\t\tconsole.warn(\"Please gsap.registerPlugin(CSSPlugin, CSSRulePlugin)\");\n\t\t\t}\n\t\t}\n\t\treturn _coreInitted;\n\t},\n\t_initCore = core => {\n\t\tgsap = core || _getGSAP();\n\t\tif (_windowExists()) {\n\t\t\t_win = window;\n\t\t\t_doc = document;\n\t\t}\n\t\tif (gsap) {\n\t\t\tCSSPlugin = gsap.plugins.css;\n\t\t\tif (CSSPlugin) {\n\t\t\t\t_coreInitted = 1;\n\t\t\t}\n\t\t}\n\t};\n\n\nexport const CSSRulePlugin = {\n\tversion: \"3.12.7\",\n\tname: \"cssRule\",\n\tinit(target, value, tween, index, targets) {\n\t\tif (!_checkRegister() || typeof(target.cssText) === \"undefined\") {\n\t\t\treturn false;\n\t\t}\n\t\tlet div = target._gsProxy = target._gsProxy || _doc.createElement(\"div\");\n\t\tthis.ss = target;\n\t\tthis.style = div.style;\n\t\tdiv.style.cssText = target.cssText;\n\t\tCSSPlugin.prototype.init.call(this, div, value, tween, index, targets); //we just offload all the work to the regular CSSPlugin and then copy the cssText back over to the rule in the render() method. This allows us to have all of the updates to CSSPlugin automatically flow through to CSSRulePlugin instead of having to maintain both\n\t},\n\trender(ratio, data) {\n\t\tlet pt = data._pt,\n\t\t\tstyle = data.style,\n\t\t\tss = data.ss,\n\t\t\ti;\n\t\twhile (pt) {\n\t\t\tpt.r(ratio, pt.d);\n\t\t\tpt = pt._next;\n\t\t}\n\t\ti = style.length;\n\t\twhile (--i > -1) {\n\t\t\tss[style[i]] = style[style[i]];\n\t\t}\n\t},\n\tgetRule(selector) {\n\t\t_checkRegister();\n\t\tlet ruleProp = _doc.all ? \"rules\" : \"cssRules\",\n\t\t\tstyleSheets = _doc.styleSheets,\n\t\t\ti = styleSheets.length,\n\t\t\tpseudo = (selector.charAt(0) === \":\"),\n\t\t\tj, curSS, cs, a;\n\t\tselector = (pseudo ? \"\" : \",\") + selector.split(\"::\").join(\":\").toLowerCase() + \",\"; //note: old versions of IE report tag name selectors as upper case, so we just change everything to lowercase.\n\t\tif (pseudo) {\n\t\t\ta = [];\n\t\t}\n\t\twhile (i--) {\n\t\t\t//Firefox may throw insecure operation errors when css is loaded from other domains, so try/catch.\n\t\t\ttry {\n\t\t\t\tcurSS = styleSheets[i][ruleProp];\n\t\t\t\tif (!curSS) {\n\t\t\t\t\tcontinue;\n\t\t\t\t}\n\t\t\t\tj = curSS.length;\n\t\t\t} catch (e) {\n\t\t\t\tconsole.warn(e);\n\t\t\t\tcontinue;\n\t\t\t}\n\t\t\twhile (--j > -1) {\n\t\t\t\tcs = curSS[j];\n\t\t\t\tif (cs.selectorText && (\",\" + cs.selectorText.split(\"::\").join(\":\").toLowerCase() + \",\").indexOf(selector) !== -1) { //note: IE adds an extra \":\" to pseudo selectors, so .myClass:after becomes .myClass::after, so we need to strip the extra one out.\n\t\t\t\t\tif (pseudo) {\n\t\t\t\t\t\ta.push(cs.style);\n\t\t\t\t\t} else {\n\t\t\t\t\t\treturn cs.style;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\treturn a;\n\t},\n\tregister: _initCore\n};\n\n_getGSAP() && gsap.registerPlugin(CSSRulePlugin);\n\nexport { CSSRulePlugin as default };"],"names":["_windowExists","window","_getGSAP","gsap","registerPlugin","_checkRegister","_coreInitted","_initCore","CSSPlugin","console","warn","_doc","core","document","plugins","css","CSSRulePlugin","version","name","init","target","value","tween","index","targets","cssText","div","_gsProxy","createElement","ss","style","prototype","call","this","render","ratio","data","i","pt","_pt","r","d","_next","length","getRule","selector","j","curSS","cs","a","ruleProp","all","styleSheets","pseudo","charAt","split","join","toLowerCase","e","selectorText","indexOf","push","register"],"mappings":";;;;;;;;;6MAYiB,SAAhBA,UAAyC,oBAAZC,OAClB,SAAXC,WAAiBC,GAASH,MAAoBG,EAAOF,OAAOE,OAASA,EAAKC,gBAAkBD,EAC3E,SAAjBE,WACMC,IACJC,IACKC,GACJC,QAAQC,KAAK,yDAGRJ,MAVLH,EAAMG,EAAoBK,EAAMH,EAYnCD,EAAY,SAAZA,UAAYK,GACXT,EAAOS,GAAQV,IACXF,MAEHW,EAAOE,UAEJV,IACHK,EAAYL,EAAKW,QAAQC,OAExBT,EAAe,IAMNU,EAAgB,CAC5BC,QAAS,SACTC,KAAM,UACNC,mBAAKC,EAAQC,EAAOC,EAAOC,EAAOC,OAC5BnB,UAA+C,IAApBe,EAAOK,eAC/B,MAEJC,EAAMN,EAAOO,SAAWP,EAAOO,UAAYhB,EAAKiB,cAAc,YAC7DC,GAAKT,OACLU,MAAQJ,EAAII,MACjBJ,EAAII,MAAML,QAAUL,EAAOK,QAC3BjB,EAAUuB,UAAUZ,KAAKa,KAAKC,KAAMP,EAAKL,EAAOC,EAAOC,EAAOC,IAE/DU,uBAAOC,EAAOC,WAIZC,EAHGC,EAAKF,EAAKG,IACbT,EAAQM,EAAKN,MACbD,EAAKO,EAAKP,GAEJS,GACNA,EAAGE,EAAEL,EAAOG,EAAGG,GACfH,EAAKA,EAAGI,UAETL,EAAIP,EAAMa,QACI,IAALN,GACRR,EAAGC,EAAMO,IAAMP,EAAMA,EAAMO,KAG7BO,yBAAQC,GACPxC,QAKCyC,EAAGC,EAAOC,EAAIC,EAJXC,EAAWvC,EAAKwC,IAAM,QAAU,WACnCC,EAAczC,EAAKyC,YACnBf,EAAIe,EAAYT,OAChBU,EAAiC,MAAvBR,EAASS,OAAO,OAE3BT,GAAYQ,EAAS,GAAK,KAAOR,EAASU,MAAM,MAAMC,KAAK,KAAKC,cAAgB,IAC5EJ,IACHJ,EAAI,IAEEZ,KAAK,UAGVU,EAAQK,EAAYf,GAAGa,aAIvBJ,EAAIC,EAAMJ,OACT,MAAOe,GACRjD,QAAQC,KAAKgD,kBAGA,IAALZ,OACRE,EAAKD,EAAMD,IACJa,eAAyG,KAAxF,IAAMX,EAAGW,aAAaJ,MAAM,MAAMC,KAAK,KAAKC,cAAgB,KAAKG,QAAQf,GAAkB,KAC9GQ,SAGIL,EAAGlB,MAFVmB,EAAEY,KAAKb,EAAGlB,eAOPmB,GAERa,SAAUvD,GAGXL,KAAcC,EAAKC,eAAeY"} \ No newline at end of file diff --git a/node_modules/gsap/dist/CustomEase.js b/node_modules/gsap/dist/CustomEase.js new file mode 100644 index 0000000000000000000000000000000000000000..1a4d78d891a83625f1f0e08628f1230db3dcf06e --- /dev/null +++ b/node_modules/gsap/dist/CustomEase.js @@ -0,0 +1,717 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + var _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + _DEG2RAD = Math.PI / 180, + _sin = Math.sin, + _cos = Math.cos, + _abs = Math.abs, + _sqrt = Math.sqrt, + _isNumber = function _isNumber(value) { + return typeof value === "number"; + }, + _roundingNum = 1e5, + _round = function _round(value) { + return Math.round(value * _roundingNum) / _roundingNum || 0; + }; + function transformRawPath(rawPath, a, b, c, d, tx, ty) { + var j = rawPath.length, + segment, + l, + i, + x, + y; + + while (--j > -1) { + segment = rawPath[j]; + l = segment.length; + + for (i = 0; i < l; i += 2) { + x = segment[i]; + y = segment[i + 1]; + segment[i] = x * a + y * c + tx; + segment[i + 1] = x * b + y * d + ty; + } + } + + rawPath._dirty = 1; + return rawPath; + } + + function arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) { + if (lastX === x && lastY === y) { + return; + } + + rx = _abs(rx); + ry = _abs(ry); + + var angleRad = angle % 360 * _DEG2RAD, + cosAngle = _cos(angleRad), + sinAngle = _sin(angleRad), + PI = Math.PI, + TWOPI = PI * 2, + dx2 = (lastX - x) / 2, + dy2 = (lastY - y) / 2, + x1 = cosAngle * dx2 + sinAngle * dy2, + y1 = -sinAngle * dx2 + cosAngle * dy2, + x1_sq = x1 * x1, + y1_sq = y1 * y1, + radiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry); + + if (radiiCheck > 1) { + rx = _sqrt(radiiCheck) * rx; + ry = _sqrt(radiiCheck) * ry; + } + + var rx_sq = rx * rx, + ry_sq = ry * ry, + sq = (rx_sq * ry_sq - rx_sq * y1_sq - ry_sq * x1_sq) / (rx_sq * y1_sq + ry_sq * x1_sq); + + if (sq < 0) { + sq = 0; + } + + var coef = (largeArcFlag === sweepFlag ? -1 : 1) * _sqrt(sq), + cx1 = coef * (rx * y1 / ry), + cy1 = coef * -(ry * x1 / rx), + sx2 = (lastX + x) / 2, + sy2 = (lastY + y) / 2, + cx = sx2 + (cosAngle * cx1 - sinAngle * cy1), + cy = sy2 + (sinAngle * cx1 + cosAngle * cy1), + ux = (x1 - cx1) / rx, + uy = (y1 - cy1) / ry, + vx = (-x1 - cx1) / rx, + vy = (-y1 - cy1) / ry, + temp = ux * ux + uy * uy, + angleStart = (uy < 0 ? -1 : 1) * Math.acos(ux / _sqrt(temp)), + angleExtent = (ux * vy - uy * vx < 0 ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt(temp * (vx * vx + vy * vy))); + + isNaN(angleExtent) && (angleExtent = PI); + + if (!sweepFlag && angleExtent > 0) { + angleExtent -= TWOPI; + } else if (sweepFlag && angleExtent < 0) { + angleExtent += TWOPI; + } + + angleStart %= TWOPI; + angleExtent %= TWOPI; + + var segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)), + rawPath = [], + angleIncrement = angleExtent / segments, + controlLength = 4 / 3 * _sin(angleIncrement / 2) / (1 + _cos(angleIncrement / 2)), + ma = cosAngle * rx, + mb = sinAngle * rx, + mc = sinAngle * -ry, + md = cosAngle * ry, + i; + + for (i = 0; i < segments; i++) { + angle = angleStart + i * angleIncrement; + x1 = _cos(angle); + y1 = _sin(angle); + ux = _cos(angle += angleIncrement); + uy = _sin(angle); + rawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy); + } + + for (i = 0; i < rawPath.length; i += 2) { + x1 = rawPath[i]; + y1 = rawPath[i + 1]; + rawPath[i] = x1 * ma + y1 * mc + cx; + rawPath[i + 1] = x1 * mb + y1 * md + cy; + } + + rawPath[i - 2] = x; + rawPath[i - 1] = y; + return rawPath; + } + + function stringToRawPath(d) { + var a = (d + "").replace(_scientific, function (m) { + var n = +m; + return n < 0.0001 && n > -0.0001 ? 0 : n; + }).match(_svgPathExp) || [], + path = [], + relativeX = 0, + relativeY = 0, + twoThirds = 2 / 3, + elements = a.length, + points = 0, + errorMessage = "ERROR: malformed path: " + d, + i, + j, + x, + y, + command, + isRelative, + segment, + startX, + startY, + difX, + difY, + beziers, + prevCommand, + flag1, + flag2, + line = function line(sx, sy, ex, ey) { + difX = (ex - sx) / 3; + difY = (ey - sy) / 3; + segment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey); + }; + + if (!d || !isNaN(a[0]) || isNaN(a[1])) { + console.log(errorMessage); + return path; + } + + for (i = 0; i < elements; i++) { + prevCommand = command; + + if (isNaN(a[i])) { + command = a[i].toUpperCase(); + isRelative = command !== a[i]; + } else { + i--; + } + + x = +a[i + 1]; + y = +a[i + 2]; + + if (isRelative) { + x += relativeX; + y += relativeY; + } + + if (!i) { + startX = x; + startY = y; + } + + if (command === "M") { + if (segment) { + if (segment.length < 8) { + path.length -= 1; + } else { + points += segment.length; + } + } + + relativeX = startX = x; + relativeY = startY = y; + segment = [x, y]; + path.push(segment); + i += 2; + command = "L"; + } else if (command === "C") { + if (!segment) { + segment = [0, 0]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(x, y, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, relativeX += a[i + 5] * 1, relativeY += a[i + 6] * 1); + i += 6; + } else if (command === "S") { + difX = relativeX; + difY = relativeY; + + if (prevCommand === "C" || prevCommand === "S") { + difX += relativeX - segment[segment.length - 4]; + difY += relativeY - segment[segment.length - 3]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(difX, difY, x, y, relativeX += a[i + 3] * 1, relativeY += a[i + 4] * 1); + i += 4; + } else if (command === "Q") { + difX = relativeX + (x - relativeX) * twoThirds; + difY = relativeY + (y - relativeY) * twoThirds; + + if (!isRelative) { + relativeX = relativeY = 0; + } + + relativeX += a[i + 3] * 1; + relativeY += a[i + 4] * 1; + segment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY); + i += 4; + } else if (command === "T") { + difX = relativeX - segment[segment.length - 4]; + difY = relativeY - segment[segment.length - 3]; + segment.push(relativeX + difX, relativeY + difY, x + (relativeX + difX * 1.5 - x) * twoThirds, y + (relativeY + difY * 1.5 - y) * twoThirds, relativeX = x, relativeY = y); + i += 2; + } else if (command === "H") { + line(relativeX, relativeY, relativeX = x, relativeY); + i += 1; + } else if (command === "V") { + line(relativeX, relativeY, relativeX, relativeY = x + (isRelative ? relativeY - relativeX : 0)); + i += 1; + } else if (command === "L" || command === "Z") { + if (command === "Z") { + x = startX; + y = startY; + segment.closed = true; + } + + if (command === "L" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) { + line(relativeX, relativeY, x, y); + + if (command === "L") { + i += 2; + } + } + + relativeX = x; + relativeY = y; + } else if (command === "A") { + flag1 = a[i + 4]; + flag2 = a[i + 5]; + difX = a[i + 6]; + difY = a[i + 7]; + j = 7; + + if (flag1.length > 1) { + if (flag1.length < 3) { + difY = difX; + difX = flag2; + j--; + } else { + difY = flag2; + difX = flag1.substr(2); + j -= 2; + } + + flag2 = flag1.charAt(1); + flag1 = flag1.charAt(0); + } + + beziers = arcToSegment(relativeX, relativeY, +a[i + 1], +a[i + 2], +a[i + 3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX * 1, (isRelative ? relativeY : 0) + difY * 1); + i += j; + + if (beziers) { + for (j = 0; j < beziers.length; j++) { + segment.push(beziers[j]); + } + } + + relativeX = segment[segment.length - 2]; + relativeY = segment[segment.length - 1]; + } else { + console.log(errorMessage); + } + } + + i = segment.length; + + if (i < 6) { + path.pop(); + i = 0; + } else if (segment[0] === segment[i - 2] && segment[1] === segment[i - 1]) { + segment.closed = true; + } + + path.totalPoints = points + i; + return path; + } + function rawPathToString(rawPath) { + if (_isNumber(rawPath[0])) { + rawPath = [rawPath]; + } + + var result = "", + l = rawPath.length, + sl, + s, + i, + segment; + + for (s = 0; s < l; s++) { + segment = rawPath[s]; + result += "M" + _round(segment[0]) + "," + _round(segment[1]) + " C"; + sl = segment.length; + + for (i = 2; i < sl; i++) { + result += _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i]) + " "; + } + + if (segment.closed) { + result += "z"; + } + } + + return result; + } + + /*! + * CustomEase 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var gsap, + _coreInitted, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _initCore = function _initCore() { + gsap = _getGSAP(); + + if (gsap) { + gsap.registerEase("_CE", CustomEase.create); + _coreInitted = 1; + } else { + console.warn("Please gsap.registerPlugin(CustomEase)"); + } + }, + _bigNum = 1e20, + _round$1 = function _round(value) { + return ~~(value * 1000 + (value < 0 ? -.5 : .5)) / 1000; + }, + _numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/gi, + _needsParsingExp = /[cLlsSaAhHvVtTqQ]/g, + _findMinimum = function _findMinimum(values) { + var l = values.length, + min = _bigNum, + i; + + for (i = 1; i < l; i += 6) { + +values[i] < min && (min = +values[i]); + } + + return min; + }, + _normalize = function _normalize(values, height, originY) { + if (!originY && originY !== 0) { + originY = Math.max(+values[values.length - 1], +values[1]); + } + + var tx = +values[0] * -1, + ty = -originY, + l = values.length, + sx = 1 / (+values[l - 2] + tx), + sy = -height || (Math.abs(+values[l - 1] - +values[1]) < 0.01 * (+values[l - 2] - +values[0]) ? _findMinimum(values) + ty : +values[l - 1] + ty), + i; + + if (sy) { + sy = 1 / sy; + } else { + sy = -sx; + } + + for (i = 0; i < l; i += 2) { + values[i] = (+values[i] + tx) * sx; + values[i + 1] = (+values[i + 1] + ty) * sy; + } + }, + _bezierToPoints = function _bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) { + var x12 = (x1 + x2) / 2, + y12 = (y1 + y2) / 2, + x23 = (x2 + x3) / 2, + y23 = (y2 + y3) / 2, + x34 = (x3 + x4) / 2, + y34 = (y3 + y4) / 2, + x123 = (x12 + x23) / 2, + y123 = (y12 + y23) / 2, + x234 = (x23 + x34) / 2, + y234 = (y23 + y34) / 2, + x1234 = (x123 + x234) / 2, + y1234 = (y123 + y234) / 2, + dx = x4 - x1, + dy = y4 - y1, + d2 = Math.abs((x2 - x4) * dy - (y2 - y4) * dx), + d3 = Math.abs((x3 - x4) * dy - (y3 - y4) * dx), + length; + + if (!points) { + points = [{ + x: x1, + y: y1 + }, { + x: x4, + y: y4 + }]; + index = 1; + } + + points.splice(index || points.length - 1, 0, { + x: x1234, + y: y1234 + }); + + if ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) { + length = points.length; + + _bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index); + + _bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 1 + (points.length - length)); + } + + return points; + }; + + var CustomEase = function () { + function CustomEase(id, data, config) { + _coreInitted || _initCore(); + this.id = id; + this.setData(data, config); + } + + var _proto = CustomEase.prototype; + + _proto.setData = function setData(data, config) { + config = config || {}; + data = data || "0,0,1,1"; + var values = data.match(_numExp), + closest = 1, + points = [], + lookup = [], + precision = config.precision || 1, + fast = precision <= 1, + l, + a1, + a2, + i, + inc, + j, + point, + prevPoint, + p; + this.data = data; + + if (_needsParsingExp.test(data) || ~data.indexOf("M") && data.indexOf("C") < 0) { + values = stringToRawPath(data)[0]; + } + + l = values.length; + + if (l === 4) { + values.unshift(0, 0); + values.push(1, 1); + l = 8; + } else if ((l - 2) % 6) { + throw "Invalid CustomEase"; + } + + if (+values[0] !== 0 || +values[l - 2] !== 1) { + _normalize(values, config.height, config.originY); + } + + this.segment = values; + + for (i = 2; i < l; i += 6) { + a1 = { + x: +values[i - 2], + y: +values[i - 1] + }; + a2 = { + x: +values[i + 4], + y: +values[i + 5] + }; + points.push(a1, a2); + + _bezierToPoints(a1.x, a1.y, +values[i], +values[i + 1], +values[i + 2], +values[i + 3], a2.x, a2.y, 1 / (precision * 200000), points, points.length - 1); + } + + l = points.length; + + for (i = 0; i < l; i++) { + point = points[i]; + prevPoint = points[i - 1] || point; + + if ((point.x > prevPoint.x || prevPoint.y !== point.y && prevPoint.x === point.x || point === prevPoint) && point.x <= 1) { + prevPoint.cx = point.x - prevPoint.x; + prevPoint.cy = point.y - prevPoint.y; + prevPoint.n = point; + prevPoint.nx = point.x; + + if (fast && i > 1 && Math.abs(prevPoint.cy / prevPoint.cx - points[i - 2].cy / points[i - 2].cx) > 2) { + fast = 0; + } + + if (prevPoint.cx < closest) { + if (!prevPoint.cx) { + prevPoint.cx = 0.001; + + if (i === l - 1) { + prevPoint.x -= 0.001; + closest = Math.min(closest, 0.001); + fast = 0; + } + } else { + closest = prevPoint.cx; + } + } + } else { + points.splice(i--, 1); + l--; + } + } + + l = 1 / closest + 1 | 0; + inc = 1 / l; + j = 0; + point = points[0]; + + if (fast) { + for (i = 0; i < l; i++) { + p = i * inc; + + if (point.nx < p) { + point = points[++j]; + } + + a1 = point.y + (p - point.x) / point.cx * point.cy; + lookup[i] = { + x: p, + cx: inc, + y: a1, + cy: 0, + nx: 9 + }; + + if (i) { + lookup[i - 1].cy = a1 - lookup[i - 1].y; + } + } + + j = points[points.length - 1]; + lookup[l - 1].cy = j.y - a1; + lookup[l - 1].cx = j.x - lookup[lookup.length - 1].x; + } else { + for (i = 0; i < l; i++) { + if (point.nx < i * inc) { + point = points[++j]; + } + + lookup[i] = point; + } + + if (j < points.length - 1) { + lookup[i - 1] = points[points.length - 2]; + } + } + + this.ease = function (p) { + var point = lookup[p * l | 0] || lookup[l - 1]; + + if (point.nx < p) { + point = point.n; + } + + return point.y + (p - point.x) / point.cx * point.cy; + }; + + this.ease.custom = this; + this.id && gsap && gsap.registerEase(this.id, this.ease); + return this; + }; + + _proto.getSVGData = function getSVGData(config) { + return CustomEase.getSVGData(this, config); + }; + + CustomEase.create = function create(id, data, config) { + return new CustomEase(id, data, config).ease; + }; + + CustomEase.register = function register(core) { + gsap = core; + + _initCore(); + }; + + CustomEase.get = function get(id) { + return gsap.parseEase(id); + }; + + CustomEase.getSVGData = function getSVGData(ease, config) { + config = config || {}; + var width = config.width || 100, + height = config.height || 100, + x = config.x || 0, + y = (config.y || 0) + height, + e = gsap.utils.toArray(config.path)[0], + a, + slope, + i, + inc, + tx, + ty, + precision, + threshold, + prevX, + prevY; + + if (config.invert) { + height = -height; + y = 0; + } + + if (typeof ease === "string") { + ease = gsap.parseEase(ease); + } + + if (ease.custom) { + ease = ease.custom; + } + + if (ease instanceof CustomEase) { + a = rawPathToString(transformRawPath([ease.segment], width, 0, 0, -height, x, y)); + } else { + a = [x, y]; + precision = Math.max(5, (config.precision || 1) * 200); + inc = 1 / precision; + precision += 2; + threshold = 5 / precision; + prevX = _round$1(x + inc * width); + prevY = _round$1(y + ease(inc) * -height); + slope = (prevY - y) / (prevX - x); + + for (i = 2; i < precision; i++) { + tx = _round$1(x + i * inc * width); + ty = _round$1(y + ease(i * inc) * -height); + + if (Math.abs((ty - prevY) / (tx - prevX) - slope) > threshold || i === precision - 1) { + a.push(prevX, prevY); + slope = (ty - prevY) / (tx - prevX); + } + + prevX = tx; + prevY = ty; + } + + a = "M" + a.join(","); + } + + e && e.setAttribute("d", a); + return a; + }; + + return CustomEase; + }(); + CustomEase.version = "3.12.7"; + CustomEase.headless = true; + _getGSAP() && gsap.registerPlugin(CustomEase); + + exports.CustomEase = CustomEase; + exports.default = CustomEase; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/CustomEase.min.js b/node_modules/gsap/dist/CustomEase.min.js new file mode 100644 index 0000000000000000000000000000000000000000..2a35d01824ee25d99b281a3bcfb8de5949d7f4cf --- /dev/null +++ b/node_modules/gsap/dist/CustomEase.min.js @@ -0,0 +1,11 @@ +/*! + * CustomEase 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(e){"use strict";function m(e){return Math.round(1e5*e)/1e5||0}var b=/[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/gi,w=/[\+\-]?\d*\.?\d+e[\+\-]?\d+/gi,Y=Math.PI/180,k=Math.sin,B=Math.cos,F=Math.abs,J=Math.sqrt;function arcToSegment(e,t,n,s,a,r,i,o,h){if(e!==o||t!==h){n=F(n),s=F(s);var u=a%360*Y,c=B(u),f=k(u),l=Math.PI,g=2*l,x=(e-o)/2,d=(t-h)/2,m=c*x+f*d,p=-f*x+c*d,y=m*m,M=p*p,v=y/(n*n)+M/(s*s);1<v&&(n=J(v)*n,s=J(v)*s);var C=n*n,E=s*s,b=(C*E-C*M-E*y)/(C*M+E*y);b<0&&(b=0);var w=(r===i?-1:1)*J(b),P=n*p/s*w,S=-s*m/n*w,N=c*P-f*S+(e+o)/2,D=f*P+c*S+(t+h)/2,T=(m-P)/n,V=(p-S)/s,_=(-m-P)/n,q=(-p-S)/s,A=T*T+V*V,R=(V<0?-1:1)*Math.acos(T/J(A)),G=(T*q-V*_<0?-1:1)*Math.acos((T*_+V*q)/J(A*(_*_+q*q)));isNaN(G)&&(G=l),!i&&0<G?G-=g:i&&G<0&&(G+=g),R%=g,G%=g;var L,O=Math.ceil(F(G)/(g/4)),j=[],z=G/O,I=4/3*k(z/2)/(1+B(z/2)),H=c*n,Q=f*n,Z=f*-s,U=c*s;for(L=0;L<O;L++)m=B(a=R+L*z),p=k(a),T=B(a+=z),V=k(a),j.push(m-I*p,p+I*m,T+I*V,V-I*T,T,V);for(L=0;L<j.length;L+=2)m=j[L],p=j[L+1],j[L]=m*H+p*Z+N,j[L+1]=m*Q+p*U+D;return j[L-2]=o,j[L-1]=h,j}}function stringToRawPath(e){function db(e,t,n,s){c=(n-e)/3,f=(s-t)/3,o.push(e+c,t+f,n-c,s-f,n,s)}var t,n,s,a,r,i,o,h,u,c,f,l,g,x,d,m=(e+"").replace(w,function(e){var t=+e;return t<1e-4&&-1e-4<t?0:t}).match(b)||[],p=[],y=0,M=0,v=m.length,C=0,E="ERROR: malformed path: "+e;if(!e||!isNaN(m[0])||isNaN(m[1]))return console.log(E),p;for(t=0;t<v;t++)if(g=r,isNaN(m[t])?i=(r=m[t].toUpperCase())!==m[t]:t--,s=+m[t+1],a=+m[t+2],i&&(s+=y,a+=M),t||(h=s,u=a),"M"===r)o&&(o.length<8?--p.length:C+=o.length),y=h=s,M=u=a,o=[s,a],p.push(o),t+=2,r="L";else if("C"===r)i||(y=M=0),(o=o||[0,0]).push(s,a,y+1*m[t+3],M+1*m[t+4],y+=1*m[t+5],M+=1*m[t+6]),t+=6;else if("S"===r)c=y,f=M,"C"!==g&&"S"!==g||(c+=y-o[o.length-4],f+=M-o[o.length-3]),i||(y=M=0),o.push(c,f,s,a,y+=1*m[t+3],M+=1*m[t+4]),t+=4;else if("Q"===r)c=y+2/3*(s-y),f=M+2/3*(a-M),i||(y=M=0),y+=1*m[t+3],M+=1*m[t+4],o.push(c,f,y+2/3*(s-y),M+2/3*(a-M),y,M),t+=4;else if("T"===r)c=y-o[o.length-4],f=M-o[o.length-3],o.push(y+c,M+f,s+2/3*(y+1.5*c-s),a+2/3*(M+1.5*f-a),y=s,M=a),t+=2;else if("H"===r)db(y,M,y=s,M),t+=1;else if("V"===r)db(y,M,y,M=s+(i?M-y:0)),t+=1;else if("L"===r||"Z"===r)"Z"===r&&(s=h,a=u,o.closed=!0),("L"===r||.5<F(y-s)||.5<F(M-a))&&(db(y,M,s,a),"L"===r&&(t+=2)),y=s,M=a;else if("A"===r){if(x=m[t+4],d=m[t+5],c=m[t+6],f=m[t+7],n=7,1<x.length&&(x.length<3?(f=c,c=d,n--):(f=d,c=x.substr(2),n-=2),d=x.charAt(1),x=x.charAt(0)),l=arcToSegment(y,M,+m[t+1],+m[t+2],+m[t+3],+x,+d,(i?y:0)+1*c,(i?M:0)+1*f),t+=n,l)for(n=0;n<l.length;n++)o.push(l[n]);y=o[o.length-2],M=o[o.length-1]}else console.log(E);return(t=o.length)<6?(p.pop(),t=0):o[0]===o[t-2]&&o[1]===o[t-1]&&(o.closed=!0),p.totalPoints=C+t,p}function p(){return M||"undefined"!=typeof window&&(M=window.gsap)&&M.registerPlugin&&M}function q(){(M=p())?(M.registerEase("_CE",n.create),a=1):console.warn("Please gsap.registerPlugin(CustomEase)")}function s(e){return~~(1e3*e+(e<0?-.5:.5))/1e3}function x(e,t,n,s,a,r,i,o,h,u,c){var f,l=(e+n)/2,g=(t+s)/2,d=(n+a)/2,m=(s+r)/2,p=(a+i)/2,y=(r+o)/2,M=(l+d)/2,v=(g+m)/2,C=(d+p)/2,E=(m+y)/2,b=(M+C)/2,w=(v+E)/2,P=i-e,S=o-t,N=Math.abs((n-i)*S-(s-o)*P),D=Math.abs((a-i)*S-(r-o)*P);return u||(u=[{x:e,y:t},{x:i,y:o}],c=1),u.splice(c||u.length-1,0,{x:b,y:w}),h*(P*P+S*S)<(N+D)*(N+D)&&(f=u.length,x(e,t,l,g,M,v,b,w,h,u,c),x(b,w,C,E,p,y,i,o,h,u,c+1+(u.length-f))),u}var M,a,t,y=/[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/gi,v=/[cLlsSaAhHvVtTqQ]/g,n=((t=CustomEase.prototype).setData=function setData(e,t){t=t||{};var n,s,a,r,i,o,h,u,c,f=(e=e||"0,0,1,1").match(y),l=1,g=[],d=[],m=t.precision||1,p=m<=1;if(this.data=e,(v.test(e)||~e.indexOf("M")&&e.indexOf("C")<0)&&(f=stringToRawPath(e)[0]),4===(n=f.length))f.unshift(0,0),f.push(1,1),n=8;else if((n-2)%6)throw"Invalid CustomEase";for(0==+f[0]&&1==+f[n-2]||function _normalize(e,t,n){n||0===n||(n=Math.max(+e[e.length-1],+e[1]));var s,a=-1*e[0],r=-n,i=e.length,o=1/(+e[i-2]+a),h=-t||(Math.abs(e[i-1]-e[1])<.01*(e[i-2]-e[0])?function _findMinimum(e){var t,n=e.length,s=1e20;for(t=1;t<n;t+=6)+e[t]<s&&(s=+e[t]);return s}(e)+r:+e[i-1]+r);for(h=h?1/h:-o,s=0;s<i;s+=2)e[s]=(+e[s]+a)*o,e[s+1]=(+e[s+1]+r)*h}(f,t.height,t.originY),this.segment=f,r=2;r<n;r+=6)s={x:+f[r-2],y:+f[r-1]},a={x:+f[r+4],y:+f[r+5]},g.push(s,a),x(s.x,s.y,+f[r],+f[r+1],+f[r+2],+f[r+3],a.x,a.y,1/(2e5*m),g,g.length-1);for(n=g.length,r=0;r<n;r++)h=g[r],u=g[r-1]||h,(h.x>u.x||u.y!==h.y&&u.x===h.x||h===u)&&h.x<=1?(u.cx=h.x-u.x,u.cy=h.y-u.y,u.n=h,u.nx=h.x,p&&1<r&&2<Math.abs(u.cy/u.cx-g[r-2].cy/g[r-2].cx)&&(p=0),u.cx<l&&(u.cx?l=u.cx:(u.cx=.001,r===n-1&&(u.x-=.001,l=Math.min(l,.001),p=0)))):(g.splice(r--,1),n--);if(i=1/(n=1/l+1|0),h=g[o=0],p){for(r=0;r<n;r++)c=r*i,h.nx<c&&(h=g[++o]),s=h.y+(c-h.x)/h.cx*h.cy,d[r]={x:c,cx:i,y:s,cy:0,nx:9},r&&(d[r-1].cy=s-d[r-1].y);o=g[g.length-1],d[n-1].cy=o.y-s,d[n-1].cx=o.x-d[d.length-1].x}else{for(r=0;r<n;r++)h.nx<r*i&&(h=g[++o]),d[r]=h;o<g.length-1&&(d[r-1]=g[g.length-2])}return this.ease=function(e){var t=d[e*n|0]||d[n-1];return t.nx<e&&(t=t.n),t.y+(e-t.x)/t.cx*t.cy},(this.ease.custom=this).id&&M&&M.registerEase(this.id,this.ease),this},t.getSVGData=function getSVGData(e){return CustomEase.getSVGData(this,e)},CustomEase.create=function create(e,t,n){return new CustomEase(e,t,n).ease},CustomEase.register=function register(e){M=e,q()},CustomEase.get=function get(e){return M.parseEase(e)},CustomEase.getSVGData=function getSVGData(e,t){var n,a,r,i,o,h,u,c,f,l,g=(t=t||{}).width||100,x=t.height||100,d=t.x||0,p=(t.y||0)+x,y=M.utils.toArray(t.path)[0];if(t.invert&&(x=-x,p=0),"string"==typeof e&&(e=M.parseEase(e)),e.custom&&(e=e.custom),e instanceof CustomEase)n=function rawPathToString(e){!function _isNumber(e){return"number"==typeof e}(e[0])||(e=[e]);var t,n,s,a,r="",i=e.length;for(n=0;n<i;n++){for(a=e[n],r+="M"+m(a[0])+","+m(a[1])+" C",t=a.length,s=2;s<t;s++)r+=m(a[s++])+","+m(a[s++])+" "+m(a[s++])+","+m(a[s++])+" "+m(a[s++])+","+m(a[s])+" ";a.closed&&(r+="z")}return r}(function transformRawPath(e,t,n,s,a,r,i){for(var o,h,u,c,f,l=e.length;-1<--l;)for(h=(o=e[l]).length,u=0;u<h;u+=2)c=o[u],f=o[u+1],o[u]=c*t+f*s+r,o[u+1]=c*n+f*a+i;return e._dirty=1,e}([e.segment],g,0,0,-x,d,p));else{for(n=[d,p],i=1/(u=Math.max(5,200*(t.precision||1))),c=5/(u+=2),f=s(d+i*g),a=((l=s(p+e(i)*-x))-p)/(f-d),r=2;r<u;r++)o=s(d+r*i*g),h=s(p+e(r*i)*-x),(Math.abs((h-l)/(o-f)-a)>c||r===u-1)&&(n.push(f,l),a=(h-l)/(o-f)),f=o,l=h;n="M"+n.join(",")}return y&&y.setAttribute("d",n),n},CustomEase);function CustomEase(e,t,n){a||q(),this.id=e,this.setData(t,n)}n.version="3.12.7",n.headless=!0,p()&&M.registerPlugin(n),e.CustomEase=n,e.default=n;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/CustomEase.min.js.map b/node_modules/gsap/dist/CustomEase.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..e4bc833969ac9e68855384f6f2801041795fb68f --- /dev/null +++ b/node_modules/gsap/dist/CustomEase.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"CustomEase.min.js","sources":["../src/utils/paths.js","../src/CustomEase.js"],"sourcesContent":["/*!\n * paths 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _svgPathExp = /[achlmqstvz]|(-?\\d*\\.?\\d*(?:e[\\-+]?\\d+)?)[0-9]/ig,\n\t_numbersExp = /(?:(-)?\\d*\\.?\\d*(?:e[\\-+]?\\d+)?)[0-9]/ig,\n\t_scientific = /[\\+\\-]?\\d*\\.?\\d+e[\\+\\-]?\\d+/ig,\n\t_selectorExp = /(^[#\\.][a-z]|[a-y][a-z])/i,\n\t_DEG2RAD = Math.PI / 180,\n\t_RAD2DEG = 180 / Math.PI,\n\t_sin = Math.sin,\n\t_cos = Math.cos,\n\t_abs = Math.abs,\n\t_sqrt = Math.sqrt,\n\t_atan2 = Math.atan2,\n\t_largeNum = 1e8,\n\t_isString = value => typeof(value) === \"string\",\n\t_isNumber = value => typeof(value) === \"number\",\n\t_isUndefined = value => typeof(value) === \"undefined\",\n\t_temp = {},\n\t_temp2 = {},\n\t_roundingNum = 1e5,\n\t_wrapProgress = progress => (Math.round((progress + _largeNum) % 1 * _roundingNum) / _roundingNum) || ((progress < 0) ? 0 : 1), //if progress lands on 1, the % will make it 0 which is why we || 1, but not if it's negative because it makes more sense for motion to end at 0 in that case.\n\t_round = value => (Math.round(value * _roundingNum) / _roundingNum) || 0,\n\t_roundPrecise = value => (Math.round(value * 1e10) / 1e10) || 0,\n\t_splitSegment = (rawPath, segIndex, i, t) => {\n\t\tlet segment = rawPath[segIndex],\n\t\t\tshift = t === 1 ? 6 : subdivideSegment(segment, i, t);\n\t\tif ((shift || !t) && shift + i + 2 < segment.length) {\n\t\t\trawPath.splice(segIndex, 0, segment.slice(0, i + shift + 2));\n\t\t\tsegment.splice(0, i + shift);\n\t\t\treturn 1;\n\t\t}\n\t},\n\t_getSampleIndex = (samples, length, progress) => {\n\t\t// slightly slower way than doing this (when there's no lookup): segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0;\n\t\tlet l = samples.length,\n\t\t\ti = ~~(progress * l);\n\t\tif (samples[i] > length) {\n\t\t\twhile (--i && samples[i] > length) {}\n\t\t\ti < 0 && (i = 0);\n\t\t} else {\n\t\t\twhile (samples[++i] < length && i < l) {}\n\t\t}\n\t\treturn i < l ? i : l - 1;\n\t},\n\t_reverseRawPath = (rawPath, skipOuter) => {\n\t\tlet i = rawPath.length;\n\t\tskipOuter || rawPath.reverse();\n\t\twhile (i--) {\n\t\t\trawPath[i].reversed || reverseSegment(rawPath[i]);\n\t\t}\n\t},\n\t_copyMetaData = (source, copy) => {\n\t\tcopy.totalLength = source.totalLength;\n\t\tif (source.samples) { //segment\n\t\t\tcopy.samples = source.samples.slice(0);\n\t\t\tcopy.lookup = source.lookup.slice(0);\n\t\t\tcopy.minLength = source.minLength;\n\t\t\tcopy.resolution = source.resolution;\n\t\t} else if (source.totalPoints) { //rawPath\n\t\t\tcopy.totalPoints = source.totalPoints;\n\t\t}\n\t\treturn copy;\n\t},\n\t//pushes a new segment into a rawPath, but if its starting values match the ending values of the last segment, it'll merge it into that same segment (to reduce the number of segments)\n\t_appendOrMerge = (rawPath, segment) => {\n\t\tlet index = rawPath.length,\n\t\t\tprevSeg = rawPath[index - 1] || [],\n\t\t\tl = prevSeg.length;\n\t\tif (index && segment[0] === prevSeg[l-2] && segment[1] === prevSeg[l-1]) {\n\t\t\tsegment = prevSeg.concat(segment.slice(2));\n\t\t\tindex--;\n\t\t}\n\t\trawPath[index] = segment;\n\t},\n\t_bestDistance;\n\n/* TERMINOLOGY\n - RawPath - an array of arrays, one for each Segment. A single RawPath could have multiple \"M\" commands, defining Segments (paths aren't always connected).\n - Segment - an array containing a sequence of Cubic Bezier coordinates in alternating x, y, x, y format. Starting anchor, then control point 1, control point 2, and ending anchor, then the next control point 1, control point 2, anchor, etc. Uses less memory than an array with a bunch of {x, y} points.\n - Bezier - a single cubic Bezier with a starting anchor, two control points, and an ending anchor.\n - the variable \"t\" is typically the position along an individual Bezier path (time) and it's NOT linear, meaning it could accelerate/decelerate based on the control points whereas the \"p\" or \"progress\" value is linearly mapped to the whole path, so it shouldn't really accelerate/decelerate based on control points. So a progress of 0.2 would be almost exactly 20% along the path. \"t\" is ONLY in an individual Bezier piece.\n */\n\n//accepts basic selector text, a path instance, a RawPath instance, or a Segment and returns a RawPath (makes it easy to homogenize things). If an element or selector text is passed in, it'll also cache the value so that if it's queried again, it'll just take the path data from there instead of parsing it all over again (as long as the path data itself hasn't changed - it'll check).\nexport function getRawPath(value) {\n\tvalue = (_isString(value) && _selectorExp.test(value)) ? document.querySelector(value) || value : value;\n\tlet e = value.getAttribute ? value : 0,\n\t\trawPath;\n\tif (e && (value = value.getAttribute(\"d\"))) {\n\t\t//implements caching\n\t\tif (!e._gsPath) {\n\t\t\te._gsPath = {};\n\t\t}\n\t\trawPath = e._gsPath[value];\n\t\treturn (rawPath && !rawPath._dirty) ? rawPath : (e._gsPath[value] = stringToRawPath(value));\n\t}\n\treturn !value ? console.warn(\"Expecting a <path> element or an SVG path data string\") : _isString(value) ? stringToRawPath(value) : (_isNumber(value[0])) ? [value] : value;\n}\n\n//copies a RawPath WITHOUT the length meta data (for speed)\nexport function copyRawPath(rawPath) {\n\tlet a = [],\n\t\ti = 0;\n\tfor (; i < rawPath.length; i++) {\n\t\ta[i] = _copyMetaData(rawPath[i], rawPath[i].slice(0));\n\t}\n\treturn _copyMetaData(rawPath, a);\n}\n\nexport function reverseSegment(segment) {\n\tlet i = 0,\n\t\ty;\n\tsegment.reverse(); //this will invert the order y, x, y, x so we must flip it back.\n\tfor (; i < segment.length; i += 2) {\n\t\ty = segment[i];\n\t\tsegment[i] = segment[i+1];\n\t\tsegment[i+1] = y;\n\t}\n\tsegment.reversed = !segment.reversed;\n}\n\n\n\nlet _createPath = (e, ignore) => {\n\t\tlet path = document.createElementNS(\"http://www.w3.org/2000/svg\", \"path\"),\n\t\t\tattr = [].slice.call(e.attributes),\n\t\t\ti = attr.length,\n\t\t\tname;\n\t\tignore = \",\" + ignore + \",\";\n\t\twhile (--i > -1) {\n\t\t\tname = attr[i].nodeName.toLowerCase(); //in Microsoft Edge, if you don't set the attribute with a lowercase name, it doesn't render correctly! Super weird.\n\t\t\tif (ignore.indexOf(\",\" + name + \",\") < 0) {\n\t\t\t\tpath.setAttributeNS(null, name, attr[i].nodeValue);\n\t\t\t}\n\t\t}\n\t\treturn path;\n\t},\n\t_typeAttrs = {\n\t\trect:\"rx,ry,x,y,width,height\",\n\t\tcircle:\"r,cx,cy\",\n\t\tellipse:\"rx,ry,cx,cy\",\n\t\tline:\"x1,x2,y1,y2\"\n\t},\n\t_attrToObj = (e, attrs) => {\n\t\tlet props = attrs ? attrs.split(\",\") : [],\n\t\t\tobj = {},\n\t\t\ti = props.length;\n\t\twhile (--i > -1) {\n\t\t\tobj[props[i]] = +e.getAttribute(props[i]) || 0;\n\t\t}\n\t\treturn obj;\n\t};\n\n//converts an SVG shape like <circle>, <rect>, <polygon>, <polyline>, <ellipse>, etc. to a <path>, swapping it in and copying the attributes to match.\nexport function convertToPath(element, swap) {\n\tlet type = element.tagName.toLowerCase(),\n\t\tcirc = 0.552284749831,\n\t\tdata, x, y, r, ry, path, rcirc, rycirc, points, w, h, x2, x3, x4, x5, x6, y2, y3, y4, y5, y6, attr;\n\tif (type === \"path\" || !element.getBBox) {\n\t\treturn element;\n\t}\n\tpath = _createPath(element, \"x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points\");\n\tattr = _attrToObj(element, _typeAttrs[type]);\n\tif (type === \"rect\") {\n\t\tr = attr.rx;\n\t\try = attr.ry || r;\n\t\tx = attr.x;\n\t\ty = attr.y;\n\t\tw = attr.width - r * 2;\n\t\th = attr.height - ry * 2;\n\t\tif (r || ry) { //if there are rounded corners, render cubic beziers\n\t\t\tx2 = x + r * (1 - circ);\n\t\t\tx3 = x + r;\n\t\t\tx4 = x3 + w;\n\t\t\tx5 = x4 + r * circ;\n\t\t\tx6 = x4 + r;\n\t\t\ty2 = y + ry * (1 - circ);\n\t\t\ty3 = y + ry;\n\t\t\ty4 = y3 + h;\n\t\t\ty5 = y4 + ry * circ;\n\t\t\ty6 = y4 + ry;\n\t\t\tdata = \"M\" + x6 + \",\" + y3 + \" V\" + y4 + \" C\" + [x6, y5, x5, y6, x4, y6, x4 - (x4 - x3) / 3, y6, x3 + (x4 - x3) / 3, y6, x3, y6, x2, y6, x, y5, x, y4, x, y4 - (y4 - y3) / 3, x, y3 + (y4 - y3) / 3, x, y3, x, y2, x2, y, x3, y, x3 + (x4 - x3) / 3, y, x4 - (x4 - x3) / 3, y, x4, y, x5, y, x6, y2, x6, y3].join(\",\") + \"z\";\n\t\t} else {\n\t\t\tdata = \"M\" + (x + w) + \",\" + y + \" v\" + h + \" h\" + (-w) + \" v\" + (-h) + \" h\" + w + \"z\";\n\t\t}\n\n\t} else if (type === \"circle\" || type === \"ellipse\") {\n\t\tif (type === \"circle\") {\n\t\t\tr = ry = attr.r;\n\t\t\trycirc = r * circ;\n\t\t} else {\n\t\t\tr = attr.rx;\n\t\t\try = attr.ry;\n\t\t\trycirc = ry * circ;\n\t\t}\n\t\tx = attr.cx;\n\t\ty = attr.cy;\n\t\trcirc = r * circ;\n\t\tdata = \"M\" + (x+r) + \",\" + y + \" C\" + [x+r, y + rycirc, x + rcirc, y + ry, x, y + ry, x - rcirc, y + ry, x - r, y + rycirc, x - r, y, x - r, y - rycirc, x - rcirc, y - ry, x, y - ry, x + rcirc, y - ry, x + r, y - rycirc, x + r, y].join(\",\") + \"z\";\n\t} else if (type === \"line\") {\n\t\tdata = \"M\" + attr.x1 + \",\" + attr.y1 + \" L\" + attr.x2 + \",\" + attr.y2; //previously, we just converted to \"Mx,y Lx,y\" but Safari has bugs that cause that not to render properly when using a stroke-dasharray that's not fully visible! Using a cubic bezier fixes that issue.\n\t} else if (type === \"polyline\" || type === \"polygon\") {\n\t\tpoints = (element.getAttribute(\"points\") + \"\").match(_numbersExp) || [];\n\t\tx = points.shift();\n\t\ty = points.shift();\n\t\tdata = \"M\" + x + \",\" + y + \" L\" + points.join(\",\");\n\t\tif (type === \"polygon\") {\n\t\t\tdata += \",\" + x + \",\" + y + \"z\";\n\t\t}\n\t}\n\tpath.setAttribute(\"d\", rawPathToString(path._gsRawPath = stringToRawPath(data)));\n\tif (swap && element.parentNode) {\n\t\telement.parentNode.insertBefore(path, element);\n\t\telement.parentNode.removeChild(element);\n\t}\n\treturn path;\n}\n\n\n\n//returns the rotation (in degrees) at a particular progress on a rawPath (the slope of the tangent)\nexport function getRotationAtProgress(rawPath, progress) {\n\tlet d = getProgressData(rawPath, progress >= 1 ? 1 - 1e-9 : progress ? progress : 1e-9);\n\treturn getRotationAtBezierT(d.segment, d.i, d.t);\n}\n\nfunction getRotationAtBezierT(segment, i, t) {\n\tlet a = segment[i],\n\t\tb = segment[i+2],\n\t\tc = segment[i+4],\n\t\tx;\n\ta += (b - a) * t;\n\tb += (c - b) * t;\n\ta += (b - a) * t;\n\tx = b + ((c + (segment[i+6] - c) * t) - b) * t - a;\n\ta = segment[i+1];\n\tb = segment[i+3];\n\tc = segment[i+5];\n\ta += (b - a) * t;\n\tb += (c - b) * t;\n\ta += (b - a) * t;\n\treturn _round(_atan2(b + ((c + (segment[i+7] - c) * t) - b) * t - a, x) * _RAD2DEG);\n}\n\nexport function sliceRawPath(rawPath, start, end) {\n\tend = _isUndefined(end) ? 1 : _roundPrecise(end) || 0; // we must round to avoid issues like 4.15 / 8 = 0.8300000000000001 instead of 0.83 or 2.8 / 5 = 0.5599999999999999 instead of 0.56 and if someone is doing a loop like start: 2.8 / 0.5, end: 2.8 / 0.5 + 1.\n\tstart = _roundPrecise(start) || 0;\n\tlet loops = Math.max(0, ~~(_abs(end - start) - 1e-8)),\n\t\tpath = copyRawPath(rawPath);\n\tif (start > end) {\n\t\tstart = 1 - start;\n\t\tend = 1 - end;\n\t\t_reverseRawPath(path);\n\t\tpath.totalLength = 0;\n\t}\n\tif (start < 0 || end < 0) {\n\t\tlet offset = Math.abs(~~Math.min(start, end)) + 1;\n\t\tstart += offset;\n\t\tend += offset;\n\t}\n\tpath.totalLength || cacheRawPathMeasurements(path);\n\tlet wrap = (end > 1),\n\t\ts = getProgressData(path, start, _temp, true),\n\t\te = getProgressData(path, end, _temp2),\n\t\teSeg = e.segment,\n\t\tsSeg = s.segment,\n\t\teSegIndex = e.segIndex,\n\t\tsSegIndex = s.segIndex,\n\t\tei = e.i,\n\t\tsi = s.i,\n\t\tsameSegment = (sSegIndex === eSegIndex),\n\t\tsameBezier = (ei === si && sameSegment),\n\t\twrapsBehind, sShift, eShift, i, copy, totalSegments, l, j;\n\tif (wrap || loops) {\n\t\twrapsBehind = eSegIndex < sSegIndex || (sameSegment && ei < si) || (sameBezier && e.t < s.t);\n\t\tif (_splitSegment(path, sSegIndex, si, s.t)) {\n\t\t\tsSegIndex++;\n\t\t\tif (!wrapsBehind) {\n\t\t\t\teSegIndex++;\n\t\t\t\tif (sameBezier) {\n\t\t\t\t\te.t = (e.t - s.t) / (1 - s.t);\n\t\t\t\t\tei = 0;\n\t\t\t\t} else if (sameSegment) {\n\t\t\t\t\tei -= si;\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tif (Math.abs(1 - (end - start)) < 1e-5) {\n\t\t\teSegIndex = sSegIndex - 1;\n\t\t} else if (!e.t && eSegIndex) {\n\t\t\teSegIndex--;\n\t\t} else if (_splitSegment(path, eSegIndex, ei, e.t) && wrapsBehind) {\n\t\t\tsSegIndex++;\n\t\t}\n\t\tif (s.t === 1) {\n\t\t\tsSegIndex = (sSegIndex + 1) % path.length;\n\t\t}\n\t\tcopy = [];\n\t\ttotalSegments = path.length;\n\t\tl = 1 + totalSegments * loops;\n\t\tj = sSegIndex;\n\t\tl += ((totalSegments - sSegIndex) + eSegIndex) % totalSegments;\n\t\tfor (i = 0; i < l; i++) {\n\t\t\t_appendOrMerge(copy, path[j++ % totalSegments]);\n\t\t}\n\t\tpath = copy;\n\t} else {\n\t\teShift = e.t === 1 ? 6 : subdivideSegment(eSeg, ei, e.t);\n\t\tif (start !== end) {\n\t\t\tsShift = subdivideSegment(sSeg, si, sameBezier ? s.t / e.t : s.t);\n\t\t\tsameSegment && (eShift += sShift);\n\t\t\teSeg.splice(ei + eShift + 2);\n\t\t\t(sShift || si) && sSeg.splice(0, si + sShift);\n\t\t\ti = path.length;\n\t\t\twhile (i--) {\n\t\t\t\t//chop off any extra segments\n\t\t\t\t(i < sSegIndex || i > eSegIndex) &&\tpath.splice(i, 1);\n\t\t\t}\n\t\t} else {\n\t\t\teSeg.angle = getRotationAtBezierT(eSeg, ei + eShift, 0); //record the value before we chop because it'll be impossible to determine the angle after its length is 0!\n\t\t\tei += eShift;\n\t\t\ts = eSeg[ei];\n\t\t\te = eSeg[ei+1];\n\t\t\teSeg.length = eSeg.totalLength = 0;\n\t\t\teSeg.totalPoints = path.totalPoints = 8;\n\t\t\teSeg.push(s, e, s, e, s, e, s, e);\n\t\t}\n\t}\n\tpath.totalLength = 0;\n\treturn path;\n}\n\n//measures a Segment according to its resolution (so if segment.resolution is 6, for example, it'll take 6 samples equally across each Bezier) and create/populate a \"samples\" Array that has the length up to each of those sample points (always increasing from the start) as well as a \"lookup\" array that's broken up according to the smallest distance between 2 samples. This gives us a very fast way of looking up a progress position rather than looping through all the points/Beziers. You can optionally have it only measure a subset, starting at startIndex and going for a specific number of beziers (remember, there are 3 x/y pairs each, for a total of 6 elements for each Bezier). It will also populate a \"totalLength\" property, but that's not generally super accurate because by default it'll only take 6 samples per Bezier. But for performance reasons, it's perfectly adequate for measuring progress values along the path. If you need a more accurate totalLength, either increase the resolution or use the more advanced bezierToPoints() method which keeps adding points until they don't deviate by more than a certain precision value.\nfunction measureSegment(segment, startIndex, bezierQty) {\n\tstartIndex = startIndex || 0;\n\tif (!segment.samples) {\n\t\tsegment.samples = [];\n\t\tsegment.lookup = [];\n\t}\n\tlet resolution = ~~segment.resolution || 12,\n\t\tinc = 1 / resolution,\n\t\tendIndex = bezierQty ? startIndex + bezierQty * 6 + 1 : segment.length,\n\t\tx1 = segment[startIndex],\n\t\ty1 = segment[startIndex + 1],\n\t\tsamplesIndex = startIndex ? (startIndex / 6) * resolution : 0,\n\t\tsamples = segment.samples,\n\t\tlookup = segment.lookup,\n\t\tmin = (startIndex ? segment.minLength : _largeNum) || _largeNum,\n\t\tprevLength = samples[samplesIndex + bezierQty * resolution - 1],\n\t\tlength = startIndex ? samples[samplesIndex-1] : 0,\n\t\ti, j, x4, x3, x2, xd, xd1, y4, y3, y2, yd, yd1, inv, t, lengthIndex, l, segLength;\n\tsamples.length = lookup.length = 0;\n\tfor (j = startIndex + 2; j < endIndex; j += 6) {\n\t\tx4 = segment[j + 4] - x1;\n\t\tx3 = segment[j + 2] - x1;\n\t\tx2 = segment[j] - x1;\n\t\ty4 = segment[j + 5] - y1;\n\t\ty3 = segment[j + 3] - y1;\n\t\ty2 = segment[j + 1] - y1;\n\t\txd = xd1 = yd = yd1 = 0;\n\t\tif (_abs(x4) < .01 && _abs(y4) < .01 && _abs(x2) + _abs(y2) < .01) { //dump points that are sufficiently close (basically right on top of each other, making a bezier super tiny or 0 length)\n\t\t\tif (segment.length > 8) {\n\t\t\t\tsegment.splice(j, 6);\n\t\t\t\tj -= 6;\n\t\t\t\tendIndex -= 6;\n\t\t\t}\n\t\t} else {\n\t\t\tfor (i = 1; i <= resolution; i++) {\n\t\t\t\tt = inc * i;\n\t\t\t\tinv = 1 - t;\n\t\t\t\txd = xd1 - (xd1 = (t * t * x4 + 3 * inv * (t * x3 + inv * x2)) * t);\n\t\t\t\tyd = yd1 - (yd1 = (t * t * y4 + 3 * inv * (t * y3 + inv * y2)) * t);\n\t\t\t\tl = _sqrt(yd * yd + xd * xd);\n\t\t\t\tif (l < min) {\n\t\t\t\t\tmin = l;\n\t\t\t\t}\n\t\t\t\tlength += l;\n\t\t\t\tsamples[samplesIndex++] = length;\n\t\t\t}\n\t\t}\n\t\tx1 += x4;\n\t\ty1 += y4;\n\t}\n\tif (prevLength) {\n\t\tprevLength -= length;\n\t\tfor (; samplesIndex < samples.length; samplesIndex++) {\n\t\t\tsamples[samplesIndex] += prevLength;\n\t\t}\n\t}\n\tif (samples.length && min) {\n\t\tsegment.totalLength = segLength = samples[samples.length-1] || 0;\n\t\tsegment.minLength = min;\n\t\tif (segLength / min < 9999) { // if the lookup would require too many values (memory problem), we skip this and instead we use a loop to lookup values directly in the samples Array\n\t\t\tl = lengthIndex = 0;\n\t\t\tfor (i = 0; i < segLength; i += min) {\n\t\t\t\tlookup[l++] = (samples[lengthIndex] < i) ? ++lengthIndex : lengthIndex;\n\t\t\t}\n\t\t}\n\t} else {\n\t\tsegment.totalLength = samples[0] = 0;\n\t}\n\treturn startIndex ? length - samples[startIndex / 2 - 1] : length;\n}\n\nexport function cacheRawPathMeasurements(rawPath, resolution) {\n\tlet pathLength, points, i;\n\tfor (i = pathLength = points = 0; i < rawPath.length; i++) {\n\t\trawPath[i].resolution = ~~resolution || 12; //steps per Bezier curve (anchor, 2 control points, to anchor)\n\t\tpoints += rawPath[i].length;\n\t\tpathLength += measureSegment(rawPath[i]);\n\t}\n\trawPath.totalPoints = points;\n\trawPath.totalLength = pathLength;\n\treturn rawPath;\n}\n\n//divide segment[i] at position t (value between 0 and 1, progress along that particular cubic bezier segment that starts at segment[i]). Returns how many elements were spliced into the segment array (either 0 or 6)\nexport function subdivideSegment(segment, i, t) {\n\tif (t <= 0 || t >= 1) {\n\t\treturn 0;\n\t}\n\tlet ax = segment[i],\n\t\tay = segment[i+1],\n\t\tcp1x = segment[i+2],\n\t\tcp1y = segment[i+3],\n\t\tcp2x = segment[i+4],\n\t\tcp2y = segment[i+5],\n\t\tbx = segment[i+6],\n\t\tby = segment[i+7],\n\t\tx1a = ax + (cp1x - ax) * t,\n\t\tx2 = cp1x + (cp2x - cp1x) * t,\n\t\ty1a = ay + (cp1y - ay) * t,\n\t\ty2 = cp1y + (cp2y - cp1y) * t,\n\t\tx1 = x1a + (x2 - x1a) * t,\n\t\ty1 = y1a + (y2 - y1a) * t,\n\t\tx2a = cp2x + (bx - cp2x) * t,\n\t\ty2a = cp2y + (by - cp2y) * t;\n\tx2 += (x2a - x2) * t;\n\ty2 += (y2a - y2) * t;\n\tsegment.splice(i + 2, 4,\n\t\t_round(x1a), //first control point\n\t\t_round(y1a),\n\t\t_round(x1), //second control point\n\t\t_round(y1),\n\t\t_round(x1 + (x2 - x1) * t), //new fabricated anchor on line\n\t\t_round(y1 + (y2 - y1) * t),\n\t\t_round(x2), //third control point\n\t\t_round(y2),\n\t\t_round(x2a), //fourth control point\n\t\t_round(y2a)\n\t);\n\tsegment.samples && segment.samples.splice(((i / 6) * segment.resolution) | 0, 0, 0, 0, 0, 0, 0, 0);\n\treturn 6;\n}\n\n// returns an object {path, segment, segIndex, i, t}\nfunction getProgressData(rawPath, progress, decoratee, pushToNextIfAtEnd) {\n\tdecoratee = decoratee || {};\n\trawPath.totalLength || cacheRawPathMeasurements(rawPath);\n\tif (progress < 0 || progress > 1) {\n\t\tprogress = _wrapProgress(progress);\n\t}\n\tlet segIndex = 0,\n\t\tsegment = rawPath[0],\n\t\tsamples, resolution, length, min, max, i, t;\n\tif (!progress) {\n\t\tt = i = segIndex = 0;\n\t\tsegment = rawPath[0];\n\t} else if (progress === 1) {\n\t\tt = 1;\n\t\tsegIndex = rawPath.length - 1;\n\t\tsegment = rawPath[segIndex];\n\t\ti = segment.length - 8;\n\t} else {\n\t\tif (rawPath.length > 1) { //speed optimization: most of the time, there's only one segment so skip the recursion.\n\t\t\tlength = rawPath.totalLength * progress;\n\t\t\tmax = i = 0;\n\t\t\twhile ((max += rawPath[i++].totalLength) < length) {\n\t\t\t\tsegIndex = i;\n\t\t\t}\n\t\t\tsegment = rawPath[segIndex];\n\t\t\tmin = max - segment.totalLength;\n\t\t\tprogress = ((length - min) / (max - min)) || 0;\n\t\t}\n\t\tsamples = segment.samples;\n\t\tresolution = segment.resolution; //how many samples per cubic bezier chunk\n\t\tlength = segment.totalLength * progress;\n\t\ti = segment.lookup.length ? segment.lookup[~~(length / segment.minLength)] || 0 : _getSampleIndex(samples, length, progress);\n\t\tmin = i ? samples[i-1] : 0;\n\t\tmax = samples[i];\n\t\tif (max < length) {\n\t\t\tmin = max;\n\t\t\tmax = samples[++i];\n\t\t}\n\t\tt = (1 / resolution) * (((length - min) / (max - min)) + ((i % resolution)));\n\t\ti = ~~(i / resolution) * 6;\n\t\tif (pushToNextIfAtEnd && t === 1) {\n\t\t\tif (i + 6 < segment.length) {\n\t\t\t\ti += 6;\n\t\t\t\tt = 0;\n\t\t\t} else if (segIndex + 1 < rawPath.length) {\n\t\t\t\ti = t = 0;\n\t\t\t\tsegment = rawPath[++segIndex];\n\t\t\t}\n\t\t}\n\t}\n\tdecoratee.t = t;\n\tdecoratee.i = i;\n\tdecoratee.path = rawPath;\n\tdecoratee.segment = segment;\n\tdecoratee.segIndex = segIndex;\n\treturn decoratee;\n}\n\nexport function getPositionOnPath(rawPath, progress, includeAngle, point) {\n\tlet segment = rawPath[0],\n\t\tresult = point || {},\n\t\tsamples, resolution, length, min, max, i, t, a, inv;\n\tif (progress < 0 || progress > 1) {\n\t\tprogress = _wrapProgress(progress);\n\t}\n\tsegment.lookup || cacheRawPathMeasurements(rawPath);\n\tif (rawPath.length > 1) { //speed optimization: most of the time, there's only one segment so skip the recursion.\n\t\tlength = rawPath.totalLength * progress;\n\t\tmax = i = 0;\n\t\twhile ((max += rawPath[i++].totalLength) < length) {\n\t\t\tsegment = rawPath[i];\n\t\t}\n\t\tmin = max - segment.totalLength;\n\t\tprogress = ((length - min) / (max - min)) || 0;\n\t}\n\tsamples = segment.samples;\n\tresolution = segment.resolution;\n\tlength = segment.totalLength * progress;\n\ti = segment.lookup.length ? segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0 : _getSampleIndex(samples, length, progress);\n\tmin = i ? samples[i-1] : 0;\n\tmax = samples[i];\n\tif (max < length) {\n\t\tmin = max;\n\t\tmax = samples[++i];\n\t}\n\tt = ((1 / resolution) * (((length - min) / (max - min)) + ((i % resolution)))) || 0;\n\tinv = 1 - t;\n\ti = ~~(i / resolution) * 6;\n\ta = segment[i];\n\tresult.x = _round((t * t * (segment[i + 6] - a) + 3 * inv * (t * (segment[i + 4] - a) + inv * (segment[i + 2] - a))) * t + a);\n\tresult.y = _round((t * t * (segment[i + 7] - (a = segment[i+1])) + 3 * inv * (t * (segment[i + 5] - a) + inv * (segment[i + 3] - a))) * t + a);\n\tif (includeAngle) {\n\t\tresult.angle = segment.totalLength ? getRotationAtBezierT(segment, i, t >= 1 ? 1 - 1e-9 : t ? t : 1e-9) : segment.angle || 0;\n\t}\n\treturn result;\n}\n\n\n\n//applies a matrix transform to RawPath (or a segment in a RawPath) and returns whatever was passed in (it transforms the values in the array(s), not a copy).\nexport function transformRawPath(rawPath, a, b, c, d, tx, ty) {\n\tlet j = rawPath.length,\n\t\tsegment, l, i, x, y;\n\twhile (--j > -1) {\n\t\tsegment = rawPath[j];\n\t\tl = segment.length;\n\t\tfor (i = 0; i < l; i += 2) {\n\t\t\tx = segment[i];\n\t\t\ty = segment[i+1];\n\t\t\tsegment[i] = x * a + y * c + tx;\n\t\t\tsegment[i+1] = x * b + y * d + ty;\n\t\t}\n\t}\n\trawPath._dirty = 1;\n\treturn rawPath;\n}\n\n\n\n// translates SVG arc data into a segment (cubic beziers). Angle is in degrees.\nfunction arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) {\n\tif (lastX === x && lastY === y) {\n\t\treturn;\n\t}\n\trx = _abs(rx);\n\try = _abs(ry);\n\tlet angleRad = (angle % 360) * _DEG2RAD,\n\t\tcosAngle = _cos(angleRad),\n\t\tsinAngle = _sin(angleRad),\n\t\tPI = Math.PI,\n\t\tTWOPI = PI * 2,\n\t\tdx2 = (lastX - x) / 2,\n\t\tdy2 = (lastY - y) / 2,\n\t\tx1 = (cosAngle * dx2 + sinAngle * dy2),\n\t\ty1 = (-sinAngle * dx2 + cosAngle * dy2),\n\t\tx1_sq = x1 * x1,\n\t\ty1_sq = y1 * y1,\n\t\tradiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry);\n\tif (radiiCheck > 1) {\n\t\trx = _sqrt(radiiCheck) * rx;\n\t\try = _sqrt(radiiCheck) * ry;\n\t}\n\tlet rx_sq = rx * rx,\n\t\try_sq = ry * ry,\n\t\tsq = ((rx_sq * ry_sq) - (rx_sq * y1_sq) - (ry_sq * x1_sq)) / ((rx_sq * y1_sq) + (ry_sq * x1_sq));\n\tif (sq < 0) {\n\t\tsq = 0;\n\t}\n\tlet coef = ((largeArcFlag === sweepFlag) ? -1 : 1) * _sqrt(sq),\n\t\tcx1 = coef * ((rx * y1) / ry),\n\t\tcy1 = coef * -((ry * x1) / rx),\n\t\tsx2 = (lastX + x) / 2,\n\t\tsy2 = (lastY + y) / 2,\n\t\tcx = sx2 + (cosAngle * cx1 - sinAngle * cy1),\n\t\tcy = sy2 + (sinAngle * cx1 + cosAngle * cy1),\n\t\tux = (x1 - cx1) / rx,\n\t\tuy = (y1 - cy1) / ry,\n\t\tvx = (-x1 - cx1) / rx,\n\t\tvy = (-y1 - cy1) / ry,\n\t\ttemp = ux * ux + uy * uy,\n\t\tangleStart = ((uy < 0) ? -1 : 1) * Math.acos(ux / _sqrt(temp)),\n\t\tangleExtent = ((ux * vy - uy * vx < 0) ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt(temp * (vx * vx + vy * vy)));\n\tisNaN(angleExtent) && (angleExtent = PI); //rare edge case. Math.cos(-1) is NaN.\n\tif (!sweepFlag && angleExtent > 0) {\n\t\tangleExtent -= TWOPI;\n\t} else if (sweepFlag && angleExtent < 0) {\n\t\tangleExtent += TWOPI;\n\t}\n\tangleStart %= TWOPI;\n\tangleExtent %= TWOPI;\n\tlet segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)),\n\t\trawPath = [],\n\t\tangleIncrement = angleExtent / segments,\n\t\tcontrolLength = 4 / 3 * _sin(angleIncrement / 2) / (1 + _cos(angleIncrement / 2)),\n\t\tma = cosAngle * rx,\n\t\tmb = sinAngle * rx,\n\t\tmc = sinAngle * -ry,\n\t\tmd = cosAngle * ry,\n\t\ti;\n\tfor (i = 0; i < segments; i++) {\n\t\tangle = angleStart + i * angleIncrement;\n\t\tx1 = _cos(angle);\n\t\ty1 = _sin(angle);\n\t\tux = _cos(angle += angleIncrement);\n\t\tuy = _sin(angle);\n\t\trawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy);\n\t}\n\t//now transform according to the actual size of the ellipse/arc (the beziers were noramlized, between 0 and 1 on a circle).\n\tfor (i = 0; i < rawPath.length; i+=2) {\n\t\tx1 = rawPath[i];\n\t\ty1 = rawPath[i+1];\n\t\trawPath[i] = x1 * ma + y1 * mc + cx;\n\t\trawPath[i+1] = x1 * mb + y1 * md + cy;\n\t}\n\trawPath[i-2] = x; //always set the end to exactly where it's supposed to be\n\trawPath[i-1] = y;\n\treturn rawPath;\n}\n\n//Spits back a RawPath with absolute coordinates. Each segment starts with a \"moveTo\" command (x coordinate, then y) and then 2 control points (x, y, x, y), then anchor. The goal is to minimize memory and maximize speed.\nexport function stringToRawPath(d) {\n\tlet a = (d + \"\").replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp) || [], //some authoring programs spit out very small numbers in scientific notation like \"1e-5\", so make sure we round that down to 0 first.\n\t\tpath = [],\n\t\trelativeX = 0,\n\t\trelativeY = 0,\n\t\ttwoThirds = 2 / 3,\n\t\telements = a.length,\n\t\tpoints = 0,\n\t\terrorMessage = \"ERROR: malformed path: \" + d,\n\t\ti, j, x, y, command, isRelative, segment, startX, startY, difX, difY, beziers, prevCommand, flag1, flag2,\n\t\tline = function(sx, sy, ex, ey) {\n\t\t\tdifX = (ex - sx) / 3;\n\t\t\tdifY = (ey - sy) / 3;\n\t\t\tsegment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey);\n\t\t};\n\tif (!d || !isNaN(a[0]) || isNaN(a[1])) {\n\t\tconsole.log(errorMessage);\n\t\treturn path;\n\t}\n\tfor (i = 0; i < elements; i++) {\n\t\tprevCommand = command;\n\t\tif (isNaN(a[i])) {\n\t\t\tcommand = a[i].toUpperCase();\n\t\t\tisRelative = (command !== a[i]); //lower case means relative\n\t\t} else { //commands like \"C\" can be strung together without any new command characters between.\n\t\t\ti--;\n\t\t}\n\t\tx = +a[i + 1];\n\t\ty = +a[i + 2];\n\t\tif (isRelative) {\n\t\t\tx += relativeX;\n\t\t\ty += relativeY;\n\t\t}\n\t\tif (!i) {\n\t\t\tstartX = x;\n\t\t\tstartY = y;\n\t\t}\n\n\t\t// \"M\" (move)\n\t\tif (command === \"M\") {\n\t\t\tif (segment) {\n\t\t\t\tif (segment.length < 8) { //if the path data was funky and just had a M with no actual drawing anywhere, skip it.\n\t\t\t\t\tpath.length -= 1;\n\t\t\t\t} else {\n\t\t\t\t\tpoints += segment.length;\n\t\t\t\t}\n\t\t\t}\n\t\t\trelativeX = startX = x;\n\t\t\trelativeY = startY = y;\n\t\t\tsegment = [x, y];\n\t\t\tpath.push(segment);\n\t\t\ti += 2;\n\t\t\tcommand = \"L\"; //an \"M\" with more than 2 values gets interpreted as \"lineTo\" commands (\"L\").\n\n\t\t// \"C\" (cubic bezier)\n\t\t} else if (command === \"C\") {\n\t\t\tif (!segment) {\n\t\t\t\tsegment = [0, 0];\n\t\t\t}\n\t\t\tif (!isRelative) {\n\t\t\t\trelativeX = relativeY = 0;\n\t\t\t}\n\t\t\t//note: \"*1\" is just a fast/short way to cast the value as a Number. WAAAY faster in Chrome, slightly slower in Firefox.\n\t\t\tsegment.push(x,\ty, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, (relativeX += a[i + 5] * 1),\t(relativeY += a[i + 6] * 1));\n\t\t\ti += 6;\n\n\t\t// \"S\" (continuation of cubic bezier)\n\t\t} else if (command === \"S\") {\n\t\t\tdifX = relativeX;\n\t\t\tdifY = relativeY;\n\t\t\tif (prevCommand === \"C\" || prevCommand === \"S\") {\n\t\t\t\tdifX += relativeX - segment[segment.length - 4];\n\t\t\t\tdifY += relativeY - segment[segment.length - 3];\n\t\t\t}\n\t\t\tif (!isRelative) {\n\t\t\t\trelativeX = relativeY = 0;\n\t\t\t}\n\t\t\tsegment.push(difX, difY, x,\ty, (relativeX += a[i + 3] * 1), (relativeY += a[i + 4] * 1));\n\t\t\ti += 4;\n\n\t\t// \"Q\" (quadratic bezier)\n\t\t} else if (command === \"Q\") {\n\t\t\tdifX = relativeX + (x - relativeX) * twoThirds;\n\t\t\tdifY = relativeY + (y - relativeY) * twoThirds;\n\t\t\tif (!isRelative) {\n\t\t\t\trelativeX = relativeY = 0;\n\t\t\t}\n\t\t\trelativeX += a[i + 3] * 1;\n\t\t\trelativeY += a[i + 4] * 1;\n\t\t\tsegment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY);\n\t\t\ti += 4;\n\n\t\t// \"T\" (continuation of quadratic bezier)\n\t\t} else if (command === \"T\") {\n\t\t\tdifX = relativeX - segment[segment.length - 4];\n\t\t\tdifY = relativeY - segment[segment.length - 3];\n\t\t\tsegment.push(relativeX + difX, relativeY + difY, x + ((relativeX + difX * 1.5) - x) * twoThirds, y + ((relativeY + difY * 1.5) - y) * twoThirds, (relativeX = x), (relativeY = y));\n\t\t\ti += 2;\n\n\t\t// \"H\" (horizontal line)\n\t\t} else if (command === \"H\") {\n\t\t\tline(relativeX, relativeY, (relativeX = x), relativeY);\n\t\t\ti += 1;\n\n\t\t// \"V\" (vertical line)\n\t\t} else if (command === \"V\") {\n\t\t\t//adjust values because the first (and only one) isn't x in this case, it's y.\n\t\t\tline(relativeX, relativeY, relativeX, (relativeY = x + (isRelative ? relativeY - relativeX : 0)));\n\t\t\ti += 1;\n\n\t\t// \"L\" (line) or \"Z\" (close)\n\t\t} else if (command === \"L\" || command === \"Z\") {\n\t\t\tif (command === \"Z\") {\n\t\t\t\tx = startX;\n\t\t\t\ty = startY;\n\t\t\t\tsegment.closed = true;\n\t\t\t}\n\t\t\tif (command === \"L\" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) {\n\t\t\t\tline(relativeX, relativeY, x, y);\n\t\t\t\tif (command === \"L\") {\n\t\t\t\t\ti += 2;\n\t\t\t\t}\n\t\t\t}\n\t\t\trelativeX = x;\n\t\t\trelativeY = y;\n\n\t\t// \"A\" (arc)\n\t\t} else if (command === \"A\") {\n\t\t\tflag1 = a[i+4];\n\t\t\tflag2 = a[i+5];\n\t\t\tdifX = a[i+6];\n\t\t\tdifY = a[i+7];\n\t\t\tj = 7;\n\t\t\tif (flag1.length > 1) { // for cases when the flags are merged, like \"a8 8 0 018 8\" (the 0 and 1 flags are WITH the x value of 8, but it could also be \"a8 8 0 01-8 8\" so it may include x or not)\n\t\t\t\tif (flag1.length < 3) {\n\t\t\t\t\tdifY = difX;\n\t\t\t\t\tdifX = flag2;\n\t\t\t\t\tj--;\n\t\t\t\t} else {\n\t\t\t\t\tdifY = flag2;\n\t\t\t\t\tdifX = flag1.substr(2);\n\t\t\t\t\tj-=2;\n\t\t\t\t}\n\t\t\t\tflag2 = flag1.charAt(1);\n\t\t\t\tflag1 = flag1.charAt(0);\n\t\t\t}\n\t\t\tbeziers = arcToSegment(relativeX, relativeY, +a[i+1], +a[i+2], +a[i+3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX*1, (isRelative ? relativeY : 0) + difY*1);\n\t\t\ti += j;\n\t\t\tif (beziers) {\n\t\t\t\tfor (j = 0; j < beziers.length; j++) {\n\t\t\t\t\tsegment.push(beziers[j]);\n\t\t\t\t}\n\t\t\t}\n\t\t\trelativeX = segment[segment.length-2];\n\t\t\trelativeY = segment[segment.length-1];\n\n\t\t} else {\n\t\t\tconsole.log(errorMessage);\n\t\t}\n\t}\n\ti = segment.length;\n\tif (i < 6) { //in case there's odd SVG like a M0,0 command at the very end.\n\t\tpath.pop();\n\t\ti = 0;\n\t} else if (segment[0] === segment[i-2] && segment[1] === segment[i-1]) {\n\t\tsegment.closed = true;\n\t}\n\tpath.totalPoints = points + i;\n\treturn path;\n}\n\n//populates the points array in alternating x/y values (like [x, y, x, y...] instead of individual point objects [{x, y}, {x, y}...] to conserve memory and stay in line with how we're handling segment arrays\nexport function bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) {\n\tlet x12 = (x1 + x2) / 2,\n\t\ty12 = (y1 + y2) / 2,\n\t\tx23 = (x2 + x3) / 2,\n\t\ty23 = (y2 + y3) / 2,\n\t\tx34 = (x3 + x4) / 2,\n\t\ty34 = (y3 + y4) / 2,\n\t\tx123 = (x12 + x23) / 2,\n\t\ty123 = (y12 + y23) / 2,\n\t\tx234 = (x23 + x34) / 2,\n\t\ty234 = (y23 + y34) / 2,\n\t\tx1234 = (x123 + x234) / 2,\n\t\ty1234 = (y123 + y234) / 2,\n\t\tdx = x4 - x1,\n\t\tdy = y4 - y1,\n\t\td2 = _abs((x2 - x4) * dy - (y2 - y4) * dx),\n\t\td3 = _abs((x3 - x4) * dy - (y3 - y4) * dx),\n\t\tlength;\n\tif (!points) {\n\t\tpoints = [x1, y1, x4, y4];\n\t\tindex = 2;\n\t}\n\tpoints.splice(index || points.length - 2, 0, x1234, y1234);\n\tif ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) {\n\t\tlength = points.length;\n\t\tbezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index);\n\t\tbezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 2 + (points.length - length));\n\t}\n\treturn points;\n}\n\n/*\nfunction getAngleBetweenPoints(x0, y0, x1, y1, x2, y2) { //angle between 3 points in radians\n\tvar dx1 = x1 - x0,\n\t\tdy1 = y1 - y0,\n\t\tdx2 = x2 - x1,\n\t\tdy2 = y2 - y1,\n\t\tdx3 = x2 - x0,\n\t\tdy3 = y2 - y0,\n\t\ta = dx1 * dx1 + dy1 * dy1,\n\t\tb = dx2 * dx2 + dy2 * dy2,\n\t\tc = dx3 * dx3 + dy3 * dy3;\n\treturn Math.acos( (a + b - c) / _sqrt(4 * a * b) );\n},\n*/\n\n//pointsToSegment() doesn't handle flat coordinates (where y is always 0) the way we need (the resulting control points are always right on top of the anchors), so this function basically makes the control points go directly up and down, varying in length based on the curviness (more curvy, further control points)\nexport function flatPointsToSegment(points, curviness=1) {\n\tlet x = points[0],\n\t\ty = 0,\n\t\tsegment = [x, y],\n\t\ti = 2;\n\tfor (; i < points.length; i+=2) {\n\t\tsegment.push(\n\t\t\tx,\n\t\t\ty,\n\t\t\tpoints[i],\n\t\t\t(y = (points[i] - x) * curviness / 2),\n\t\t\t(x = points[i]),\n\t\t\t-y\n\t\t);\n\t}\n\treturn segment;\n}\n\n//points is an array of x/y points, like [x, y, x, y, x, y]\nexport function pointsToSegment(points, curviness) {\n\t//points = simplifyPoints(points, tolerance);\n\t_abs(points[0] - points[2]) < 1e-4 && _abs(points[1] - points[3]) < 1e-4 && (points = points.slice(2)); // if the first two points are super close, dump the first one.\n\tlet l = points.length-2,\n\t\tx = +points[0],\n\t\ty = +points[1],\n\t\tnextX = +points[2],\n\t\tnextY = +points[3],\n\t\tsegment = [x, y, x, y],\n\t\tdx2 = nextX - x,\n\t\tdy2 = nextY - y,\n\t\tclosed = Math.abs(points[l] - x) < 0.001 && Math.abs(points[l+1] - y) < 0.001,\n\t\tprevX, prevY, i, dx1, dy1, r1, r2, r3, tl, mx1, mx2, mxm, my1, my2, mym;\n\tif (closed) { // if the start and end points are basically on top of each other, close the segment by adding the 2nd point to the end, and the 2nd-to-last point to the beginning (we'll remove them at the end, but this allows the curvature to look perfect)\n\t\tpoints.push(nextX, nextY);\n\t\tnextX = x;\n\t\tnextY = y;\n\t\tx = points[l-2];\n\t\ty = points[l-1];\n\t\tpoints.unshift(x, y);\n\t\tl+=4;\n\t}\n\tcurviness = (curviness || curviness === 0) ? +curviness : 1;\n\tfor (i = 2; i < l; i+=2) {\n\t\tprevX = x;\n\t\tprevY = y;\n\t\tx = nextX;\n\t\ty = nextY;\n\t\tnextX = +points[i+2];\n\t\tnextY = +points[i+3];\n\t\tif (x === nextX && y === nextY) {\n\t\t\tcontinue;\n\t\t}\n\t\tdx1 = dx2;\n\t\tdy1 = dy2;\n\t\tdx2 = nextX - x;\n\t\tdy2 = nextY - y;\n\t\tr1 = _sqrt(dx1 * dx1 + dy1 * dy1); // r1, r2, and r3 correlate x and y (and z in the future). Basically 2D or 3D hypotenuse\n\t\tr2 = _sqrt(dx2 * dx2 + dy2 * dy2);\n\t\tr3 = _sqrt((dx2 / r2 + dx1 / r1) ** 2 + (dy2 / r2 + dy1 / r1) ** 2);\n\t\ttl = ((r1 + r2) * curviness * 0.25) / r3;\n\t\tmx1 = x - (x - prevX) * (r1 ? tl / r1 : 0);\n\t\tmx2 = x + (nextX - x) * (r2 ? tl / r2 : 0);\n\t\tmxm = x - (mx1 + (((mx2 - mx1) * ((r1 * 3 / (r1 + r2)) + 0.5) / 4) || 0));\n\t\tmy1 = y - (y - prevY) * (r1 ? tl / r1 : 0);\n\t\tmy2 = y + (nextY - y) * (r2 ? tl / r2 : 0);\n\t\tmym = y - (my1 + (((my2 - my1) * ((r1 * 3 / (r1 + r2)) + 0.5) / 4) || 0));\n\t\tif (x !== prevX || y !== prevY) {\n\t\t\tsegment.push(\n\t\t\t\t_round(mx1 + mxm), // first control point\n\t\t\t\t_round(my1 + mym),\n\t\t\t\t_round(x), // anchor\n\t\t\t\t_round(y),\n\t\t\t\t_round(mx2 + mxm), // second control point\n\t\t\t\t_round(my2 + mym)\n\t\t\t);\n\t\t}\n\t}\n\tx !== nextX || y !== nextY || segment.length < 4 ? segment.push(_round(nextX), _round(nextY), _round(nextX), _round(nextY)) : (segment.length -= 2);\n\tif (segment.length === 2) { // only one point!\n\t\tsegment.push(x, y, x, y, x, y);\n\t} else if (closed) {\n\t\tsegment.splice(0, 6);\n\t\tsegment.length = segment.length - 6;\n\t}\n\treturn segment;\n}\n\n//returns the squared distance between an x/y coordinate and a segment between x1/y1 and x2/y2\nfunction pointToSegDist(x, y, x1, y1, x2, y2) {\n\tlet dx = x2 - x1,\n\t\tdy = y2 - y1,\n\t\tt;\n\tif (dx || dy) {\n\t\tt = ((x - x1) * dx + (y - y1) * dy) / (dx * dx + dy * dy);\n\t\tif (t > 1) {\n\t\t\tx1 = x2;\n\t\t\ty1 = y2;\n\t\t} else if (t > 0) {\n\t\t\tx1 += dx * t;\n\t\t\ty1 += dy * t;\n\t\t}\n\t}\n\treturn (x - x1) ** 2 + (y - y1) ** 2;\n}\n\nfunction simplifyStep(points, first, last, tolerance, simplified) {\n\tlet maxSqDist = tolerance,\n\t\tfirstX = points[first],\n\t\tfirstY = points[first+1],\n\t\tlastX = points[last],\n\t\tlastY = points[last+1],\n\t\tindex, i, d;\n\tfor (i = first + 2; i < last; i += 2) {\n\t\td = pointToSegDist(points[i], points[i+1], firstX, firstY, lastX, lastY);\n\t\tif (d > maxSqDist) {\n\t\t\tindex = i;\n\t\t\tmaxSqDist = d;\n\t\t}\n\t}\n\tif (maxSqDist > tolerance) {\n\t\tindex - first > 2 && simplifyStep(points, first, index, tolerance, simplified);\n\t\tsimplified.push(points[index], points[index+1]);\n\t\tlast - index > 2 && simplifyStep(points, index, last, tolerance, simplified);\n\t}\n}\n\n//points is an array of x/y values like [x, y, x, y, x, y]\nexport function simplifyPoints(points, tolerance) {\n\tlet prevX = parseFloat(points[0]),\n\t\tprevY = parseFloat(points[1]),\n\t\ttemp = [prevX, prevY],\n\t\tl = points.length - 2,\n\t\ti, x, y, dx, dy, result, last;\n\ttolerance = (tolerance || 1) ** 2;\n\tfor (i = 2; i < l; i += 2) {\n\t\tx = parseFloat(points[i]);\n\t\ty = parseFloat(points[i+1]);\n\t\tdx = prevX - x;\n\t\tdy = prevY - y;\n\t\tif (dx * dx + dy * dy > tolerance) {\n\t\t\ttemp.push(x, y);\n\t\t\tprevX = x;\n\t\t\tprevY = y;\n\t\t}\n\t}\n\ttemp.push(parseFloat(points[l]), parseFloat(points[l+1]));\n\tlast = temp.length - 2;\n\tresult = [temp[0], temp[1]];\n\tsimplifyStep(temp, 0, last, tolerance, result);\n\tresult.push(temp[last], temp[last+1]);\n\treturn result;\n}\n\nfunction getClosestProgressOnBezier(iterations, px, py, start, end, slices, x0, y0, x1, y1, x2, y2, x3, y3) {\n\tlet inc = (end - start) / slices,\n\t\tbest = 0,\n\t\tt = start,\n\t\tx, y, d, dx, dy, inv;\n\t_bestDistance = _largeNum;\n\twhile (t <= end) {\n\t\tinv = 1 - t;\n\t\tx = inv * inv * inv * x0 + 3 * inv * inv * t * x1 + 3 * inv * t * t * x2 + t * t * t * x3;\n\t\ty = inv * inv * inv * y0 + 3 * inv * inv * t * y1 + 3 * inv * t * t * y2 + t * t * t * y3;\n\t\tdx = x - px;\n\t\tdy = y - py;\n\t\td = dx * dx + dy * dy;\n\t\tif (d < _bestDistance) {\n\t\t\t_bestDistance = d;\n\t\t\tbest = t;\n\t\t}\n\t\tt += inc;\n\t}\n\treturn (iterations > 1) ? getClosestProgressOnBezier(iterations - 1, px, py, Math.max(best - inc, 0), Math.min(best + inc, 1), slices, x0, y0, x1, y1, x2, y2, x3, y3) : best;\n}\n\nexport function getClosestData(rawPath, x, y, slices) { //returns an object with the closest j, i, and t (j is the segment index, i is the index of the point in that segment, and t is the time/progress along that bezier)\n\tlet closest = {j:0, i:0, t:0},\n\t\tbestDistance = _largeNum,\n\t\ti, j, t, segment;\n\tfor (j = 0; j < rawPath.length; j++) {\n\t\tsegment = rawPath[j];\n\t\tfor (i = 0; i < segment.length; i+=6) {\n\t\t\tt = getClosestProgressOnBezier(1, x, y, 0, 1, slices || 20, segment[i], segment[i+1], segment[i+2], segment[i+3], segment[i+4], segment[i+5], segment[i+6], segment[i+7]);\n\t\t\tif (bestDistance > _bestDistance) {\n\t\t\t\tbestDistance = _bestDistance;\n\t\t\t\tclosest.j = j;\n\t\t\t\tclosest.i = i;\n\t\t\t\tclosest.t = t;\n\t\t\t}\n\t\t}\n\t}\n\treturn closest;\n}\n\n//subdivide a Segment closest to a specific x,y coordinate\nexport function subdivideSegmentNear(x, y, segment, slices, iterations) {\n\tlet l = segment.length,\n\t\tbestDistance = _largeNum,\n\t\tbestT = 0,\n\t\tbestSegmentIndex = 0,\n\t\tt, i;\n\tslices = slices || 20;\n\titerations = iterations || 3;\n\tfor (i = 0; i < l; i += 6) {\n\t\tt = getClosestProgressOnBezier(1, x, y, 0, 1, slices, segment[i], segment[i+1], segment[i+2], segment[i+3], segment[i+4], segment[i+5], segment[i+6], segment[i+7]);\n\t\tif (bestDistance > _bestDistance) {\n\t\t\tbestDistance = _bestDistance;\n\t\t\tbestT = t;\n\t\t\tbestSegmentIndex = i;\n\t\t}\n\t}\n\tt = getClosestProgressOnBezier(iterations, x, y, bestT - 0.05, bestT + 0.05, slices, segment[bestSegmentIndex], segment[bestSegmentIndex+1], segment[bestSegmentIndex+2], segment[bestSegmentIndex+3], segment[bestSegmentIndex+4], segment[bestSegmentIndex+5], segment[bestSegmentIndex+6], segment[bestSegmentIndex+7]);\n\tsubdivideSegment(segment, bestSegmentIndex, t);\n\treturn bestSegmentIndex + 6;\n}\n\n/*\nTakes any of the following and converts it to an all Cubic Bezier SVG data string:\n- A <path> data string like \"M0,0 L2,4 v20,15 H100\"\n- A RawPath, like [[x, y, x, y, x, y, x, y][[x, y, x, y, x, y, x, y]]\n- A Segment, like [x, y, x, y, x, y, x, y]\n\nNote: all numbers are rounded down to the closest 0.001 to minimize memory, maximize speed, and avoid odd numbers like 1e-13\n*/\nexport function rawPathToString(rawPath) {\n\tif (_isNumber(rawPath[0])) { //in case a segment is passed in instead\n\t\trawPath = [rawPath];\n\t}\n\tlet result = \"\",\n\t\tl = rawPath.length,\n\t\tsl, s, i, segment;\n\tfor (s = 0; s < l; s++) {\n\t\tsegment = rawPath[s];\n\t\tresult += \"M\" + _round(segment[0]) + \",\" + _round(segment[1]) + \" C\";\n\t\tsl = segment.length;\n\t\tfor (i = 2; i < sl; i++) {\n\t\t\tresult += _round(segment[i++]) + \",\" + _round(segment[i++]) + \" \" + _round(segment[i++]) + \",\" + _round(segment[i++]) + \" \" + _round(segment[i++]) + \",\" + _round(segment[i]) + \" \";\n\t\t}\n\t\tif (segment.closed) {\n\t\t\tresult += \"z\";\n\t\t}\n\t}\n\treturn result;\n}\n\n/*\n// takes a segment with coordinates [x, y, x, y, ...] and converts the control points into angles and lengths [x, y, angle, length, angle, length, x, y, angle, length, ...] so that it animates more cleanly and avoids odd breaks/kinks. For example, if you animate from 1 o'clock to 6 o'clock, it'd just go directly/linearly rather than around. So the length would be very short in the middle of the tween.\nexport function cpCoordsToAngles(segment, copy) {\n\tvar result = copy ? segment.slice(0) : segment,\n\t\tx, y, i;\n\tfor (i = 0; i < segment.length; i+=6) {\n\t\tx = segment[i+2] - segment[i];\n\t\ty = segment[i+3] - segment[i+1];\n\t\tresult[i+2] = Math.atan2(y, x);\n\t\tresult[i+3] = Math.sqrt(x * x + y * y);\n\t\tx = segment[i+6] - segment[i+4];\n\t\ty = segment[i+7] - segment[i+5];\n\t\tresult[i+4] = Math.atan2(y, x);\n\t\tresult[i+5] = Math.sqrt(x * x + y * y);\n\t}\n\treturn result;\n}\n\n// takes a segment that was converted with cpCoordsToAngles() to have angles and lengths instead of coordinates for the control points, and converts it BACK into coordinates.\nexport function cpAnglesToCoords(segment, copy) {\n\tvar result = copy ? segment.slice(0) : segment,\n\t\tlength = segment.length,\n\t\trnd = 1000,\n\t\tangle, l, i, j;\n\tfor (i = 0; i < length; i+=6) {\n\t\tangle = segment[i+2];\n\t\tl = segment[i+3]; //length\n\t\tresult[i+2] = (((segment[i] + Math.cos(angle) * l) * rnd) | 0) / rnd;\n\t\tresult[i+3] = (((segment[i+1] + Math.sin(angle) * l) * rnd) | 0) / rnd;\n\t\tangle = segment[i+4];\n\t\tl = segment[i+5]; //length\n\t\tresult[i+4] = (((segment[i+6] - Math.cos(angle) * l) * rnd) | 0) / rnd;\n\t\tresult[i+5] = (((segment[i+7] - Math.sin(angle) * l) * rnd) | 0) / rnd;\n\t}\n\treturn result;\n}\n\n//adds an \"isSmooth\" array to each segment and populates it with a boolean value indicating whether or not it's smooth (the control points have basically the same slope). For any smooth control points, it converts the coordinates into angle (x, in radians) and length (y) and puts them into the same index value in a smoothData array.\nexport function populateSmoothData(rawPath) {\n\tlet j = rawPath.length,\n\t\tsmooth, segment, x, y, x2, y2, i, l, a, a2, isSmooth, smoothData;\n\twhile (--j > -1) {\n\t\tsegment = rawPath[j];\n\t\tisSmooth = segment.isSmooth = segment.isSmooth || [0, 0, 0, 0];\n\t\tsmoothData = segment.smoothData = segment.smoothData || [0, 0, 0, 0];\n\t\tisSmooth.length = 4;\n\t\tl = segment.length - 2;\n\t\tfor (i = 6; i < l; i += 6) {\n\t\t\tx = segment[i] - segment[i - 2];\n\t\t\ty = segment[i + 1] - segment[i - 1];\n\t\t\tx2 = segment[i + 2] - segment[i];\n\t\t\ty2 = segment[i + 3] - segment[i + 1];\n\t\t\ta = _atan2(y, x);\n\t\t\ta2 = _atan2(y2, x2);\n\t\t\tsmooth = (Math.abs(a - a2) < 0.09);\n\t\t\tif (smooth) {\n\t\t\t\tsmoothData[i - 2] = a;\n\t\t\t\tsmoothData[i + 2] = a2;\n\t\t\t\tsmoothData[i - 1] = _sqrt(x * x + y * y);\n\t\t\t\tsmoothData[i + 3] = _sqrt(x2 * x2 + y2 * y2);\n\t\t\t}\n\t\t\tisSmooth.push(smooth, smooth, 0, 0, smooth, smooth);\n\t\t}\n\t\t//if the first and last points are identical, check to see if there's a smooth transition. We must handle this a bit differently due to their positions in the array.\n\t\tif (segment[l] === segment[0] && segment[l+1] === segment[1]) {\n\t\t\tx = segment[0] - segment[l-2];\n\t\t\ty = segment[1] - segment[l-1];\n\t\t\tx2 = segment[2] - segment[0];\n\t\t\ty2 = segment[3] - segment[1];\n\t\t\ta = _atan2(y, x);\n\t\t\ta2 = _atan2(y2, x2);\n\t\t\tif (Math.abs(a - a2) < 0.09) {\n\t\t\t\tsmoothData[l-2] = a;\n\t\t\t\tsmoothData[2] = a2;\n\t\t\t\tsmoothData[l-1] = _sqrt(x * x + y * y);\n\t\t\t\tsmoothData[3] = _sqrt(x2 * x2 + y2 * y2);\n\t\t\t\tisSmooth[l-2] = isSmooth[l-1] = true; //don't change indexes 2 and 3 because we'll trigger everything from the END, and this will optimize file size a bit.\n\t\t\t}\n\t\t}\n\t}\n\treturn rawPath;\n}\nexport function pointToScreen(svgElement, point) {\n\tif (arguments.length < 2) { //by default, take the first set of coordinates in the path as the point\n\t\tlet rawPath = getRawPath(svgElement);\n\t\tpoint = svgElement.ownerSVGElement.createSVGPoint();\n\t\tpoint.x = rawPath[0][0];\n\t\tpoint.y = rawPath[0][1];\n\t}\n\treturn point.matrixTransform(svgElement.getScreenCTM());\n}\n// takes a <path> and normalizes all of its coordinates to values between 0 and 1\nexport function normalizePath(path) {\n path = gsap.utils.toArray(path);\n if (!path[0].hasAttribute(\"d\")) {\n path = gsap.utils.toArray(path[0].children);\n }\n if (path.length > 1) {\n path.forEach(normalizePath);\n return path;\n }\n let _svgPathExp = /[achlmqstvz]|(-?\\d*\\.?\\d*(?:e[\\-+]?\\d+)?)[0-9]/ig,\n _scientific = /[\\+\\-]?\\d*\\.?\\d+e[\\+\\-]?\\d+/ig,\n d = path[0].getAttribute(\"d\"),\n a = d.replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp),\n nums = a.filter(n => !isNaN(n)).map(n => +n),\n normalize = gsap.utils.normalize(Math.min(...nums), Math.max(...nums)),\n finals = a.map(val => isNaN(val) ? val : normalize(+val)),\n s = \"\",\n prevWasCommand;\n finals.forEach((value, i) => {\n let isCommand = isNaN(value)\n s += (isCommand && i ? \" \" : prevWasCommand || !i ? \"\" : \",\") + value;\n prevWasCommand = isCommand;\n });\n path[0].setAttribute(\"d\", s);\n}\n*/","/*!\n * CustomEase 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nimport { stringToRawPath, rawPathToString, transformRawPath } from \"./utils/paths.js\";\n\nlet gsap, _coreInitted,\n\t_getGSAP = () => gsap || (typeof(window) !== \"undefined\" && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_initCore = () => {\n\t\tgsap = _getGSAP();\n\t\tif (gsap) {\n\t\t\tgsap.registerEase(\"_CE\", CustomEase.create);\n\t\t\t_coreInitted = 1;\n\t\t} else {\n\t\t\tconsole.warn(\"Please gsap.registerPlugin(CustomEase)\");\n\t\t}\n\t},\n\t_bigNum = 1e20,\n\t_round = value => ~~(value * 1000 + (value < 0 ? -.5 : .5)) / 1000,\n\t_bonusValidated = 1, //<name>CustomEase</name>\n\t_numExp = /[-+=.]*\\d+[.e\\-+]*\\d*[e\\-+]*\\d*/gi, //finds any numbers, including ones that start with += or -=, negative numbers, and ones in scientific notation like 1e-8.\n\t_needsParsingExp = /[cLlsSaAhHvVtTqQ]/g,\n\t_findMinimum = values => {\n\t\tlet l = values.length,\n\t\t\tmin = _bigNum,\n\t\t\ti;\n\t\tfor (i = 1; i < l; i += 6) {\n\t\t\t+values[i] < min && (min = +values[i]);\n\t\t}\n\t\treturn min;\n\t},\n\t//takes all the points and translates/scales them so that the x starts at 0 and ends at 1.\n\t_normalize = (values, height, originY) => {\n\t\tif (!originY && originY !== 0) {\n\t\t\toriginY = Math.max(+values[values.length-1], +values[1]);\n\t\t}\n\t\tlet tx = +values[0] * -1,\n\t\t\tty = -originY,\n\t\t\tl = values.length,\n\t\t\tsx = 1 / (+values[l - 2] + tx),\n\t\t\tsy = -height || ((Math.abs(+values[l - 1] - +values[1]) < 0.01 * (+values[l - 2] - +values[0])) ? _findMinimum(values) + ty : +values[l - 1] + ty),\n\t\t\ti;\n\t\tif (sy) { //typically y ends at 1 (so that the end values are reached)\n\t\t\tsy = 1 / sy;\n\t\t} else { //in case the ease returns to its beginning value, scale everything proportionally\n\t\t\tsy = -sx;\n\t\t}\n\t\tfor (i = 0; i < l; i += 2) {\n\t\t\tvalues[i] = (+values[i] + tx) * sx;\n\t\t\tvalues[i + 1] = (+values[i + 1] + ty) * sy;\n\t\t}\n\t},\n\t//note that this function returns point objects like {x, y} rather than working with segments which are arrays with alternating x, y values as in the similar function in paths.js\n\t_bezierToPoints = function (x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) {\n\t\tlet x12 = (x1 + x2) / 2,\n\t\t\ty12 = (y1 + y2) / 2,\n\t\t\tx23 = (x2 + x3) / 2,\n\t\t\ty23 = (y2 + y3) / 2,\n\t\t\tx34 = (x3 + x4) / 2,\n\t\t\ty34 = (y3 + y4) / 2,\n\t\t\tx123 = (x12 + x23) / 2,\n\t\t\ty123 = (y12 + y23) / 2,\n\t\t\tx234 = (x23 + x34) / 2,\n\t\t\ty234 = (y23 + y34) / 2,\n\t\t\tx1234 = (x123 + x234) / 2,\n\t\t\ty1234 = (y123 + y234) / 2,\n\t\t\tdx = x4 - x1,\n\t\t\tdy = y4 - y1,\n\t\t\td2 = Math.abs((x2 - x4) * dy - (y2 - y4) * dx),\n\t\t\td3 = Math.abs((x3 - x4) * dy - (y3 - y4) * dx),\n\t\t\tlength;\n\t\tif (!points) {\n\t\t\tpoints = [{x: x1, y: y1}, {x: x4, y: y4}];\n\t\t\tindex = 1;\n\t\t}\n\t\tpoints.splice(index || points.length - 1, 0, {x: x1234, y: y1234});\n\t\tif ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) {\n\t\t\tlength = points.length;\n\t\t\t_bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index);\n\t\t\t_bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 1 + (points.length - length));\n\t\t}\n\t\treturn points;\n\t};\n\nexport class CustomEase {\n\n\tconstructor(id, data, config) {\n\t\t_coreInitted || _initCore();\n\t\tthis.id = id;\n\t\t_bonusValidated && this.setData(data, config);\n\t}\n\n\tsetData(data, config) {\n\t\tconfig = config || {};\n\t\tdata = data || \"0,0,1,1\";\n\t\tlet values = data.match(_numExp),\n\t\t\tclosest = 1,\n\t\t\tpoints = [],\n\t\t\tlookup = [],\n\t\t\tprecision = config.precision || 1,\n\t\t\tfast = (precision <= 1),\n\t\t\tl, a1, a2, i, inc, j, point, prevPoint, p;\n\t\tthis.data = data;\n\t\tif (_needsParsingExp.test(data) || (~data.indexOf(\"M\") && data.indexOf(\"C\") < 0)) {\n\t\t\tvalues = stringToRawPath(data)[0];\n\t\t}\n\t\tl = values.length;\n\t\tif (l === 4) {\n\t\t\tvalues.unshift(0, 0);\n\t\t\tvalues.push(1, 1);\n\t\t\tl = 8;\n\t\t} else if ((l - 2) % 6) {\n\t\t\tthrow \"Invalid CustomEase\";\n\t\t}\n\t\tif (+values[0] !== 0 || +values[l - 2] !== 1) {\n\t\t\t_normalize(values, config.height, config.originY);\n\t\t}\n\t\tthis.segment = values;\n\t\tfor (i = 2; i < l; i += 6) {\n\t\t\ta1 = {x: +values[i - 2], y: +values[i - 1]};\n\t\t\ta2 = {x: +values[i + 4], y: +values[i + 5]};\n\t\t\tpoints.push(a1, a2);\n\t\t\t_bezierToPoints(a1.x, a1.y, +values[i], +values[i + 1], +values[i + 2], +values[i + 3], a2.x, a2.y, 1 / (precision * 200000), points, points.length - 1);\n\t\t}\n\t\tl = points.length;\n\t\tfor (i = 0; i < l; i++) {\n\t\t\tpoint = points[i];\n\t\t\tprevPoint = points[i - 1] || point;\n\t\t\tif ((point.x > prevPoint.x || (prevPoint.y !== point.y && prevPoint.x === point.x) || point === prevPoint) && point.x <= 1) { //if a point goes BACKWARD in time or is a duplicate, just drop it. Also it shouldn't go past 1 on the x axis, as could happen in a string like \"M0,0 C0,0 0.12,0.68 0.18,0.788 0.195,0.845 0.308,1 0.32,1 0.403,1.005 0.398,1 0.5,1 0.602,1 0.816,1.005 0.9,1 0.91,1 0.948,0.69 0.962,0.615 1.003,0.376 1,0 1,0\".\n\t\t\t\tprevPoint.cx = point.x - prevPoint.x; //change in x between this point and the next point (performance optimization)\n\t\t\t\tprevPoint.cy = point.y - prevPoint.y;\n\t\t\t\tprevPoint.n = point;\n\t\t\t\tprevPoint.nx = point.x; //next point's x value (performance optimization, making lookups faster in getRatio()). Remember, the lookup will always land on a spot where it's either this point or the very next one (never beyond that)\n\t\t\t\tif (fast && i > 1 && Math.abs(prevPoint.cy / prevPoint.cx - points[i - 2].cy / points[i - 2].cx) > 2) { //if there's a sudden change in direction, prioritize accuracy over speed. Like a bounce ease - you don't want to risk the sampling chunks landing on each side of the bounce anchor and having it clipped off.\n\t\t\t\t\tfast = 0;\n\t\t\t\t}\n\t\t\t\tif (prevPoint.cx < closest) {\n\t\t\t\t\tif (!prevPoint.cx) {\n\t\t\t\t\t\tprevPoint.cx = 0.001; //avoids math problems in getRatio() (dividing by zero)\n\t\t\t\t\t\tif (i === l - 1) { //in case the final segment goes vertical RIGHT at the end, make sure we end at the end.\n\t\t\t\t\t\t\tprevPoint.x -= 0.001;\n\t\t\t\t\t\t\tclosest = Math.min(closest, 0.001);\n\t\t\t\t\t\t\tfast = 0;\n\t\t\t\t\t\t}\n\t\t\t\t\t} else {\n\t\t\t\t\t\tclosest = prevPoint.cx;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t} else {\n\t\t\t\tpoints.splice(i--, 1);\n\t\t\t\tl--;\n\t\t\t}\n\t\t}\n\t\tl = (1 / closest + 1) | 0;\n\t\tinc = 1 / l;\n\t\tj = 0;\n\t\tpoint = points[0];\n\t\tif (fast) {\n\t\t\tfor (i = 0; i < l; i++) { //for fastest lookups, we just sample along the path at equal x (time) distance. Uses more memory and is slightly less accurate for anchors that don't land on the sampling points, but for the vast majority of eases it's excellent (and fast).\n\t\t\t\tp = i * inc;\n\t\t\t\tif (point.nx < p) {\n\t\t\t\t\tpoint = points[++j];\n\t\t\t\t}\n\t\t\t\ta1 = point.y + ((p - point.x) / point.cx) * point.cy;\n\t\t\t\tlookup[i] = {x: p, cx: inc, y: a1, cy: 0, nx: 9};\n\t\t\t\tif (i) {\n\t\t\t\t\tlookup[i - 1].cy = a1 - lookup[i - 1].y;\n\t\t\t\t}\n\t\t\t}\n\t\t\tj = points[points.length - 1];\n\t\t\tlookup[l - 1].cy = j.y - a1;\n\t\t\tlookup[l - 1].cx = j.x - lookup[lookup.length - 1].x; //make sure it lands EXACTLY where it should. Otherwise, it might be something like 0.9999999999 instead of 1.\n\t\t} else { //this option is more accurate, ensuring that EVERY anchor is hit perfectly. Clipping across a bounce, for example, would never happen.\n\t\t\tfor (i = 0; i < l; i++) { //build a lookup table based on the smallest distance so that we can instantly find the appropriate point (well, it'll either be that point or the very next one). We'll look up based on the linear progress. So it's it's 0.5 and the lookup table has 100 elements, it'd be like lookup[Math.floor(0.5 * 100)]\n\t\t\t\tif (point.nx < i * inc) {\n\t\t\t\t\tpoint = points[++j];\n\t\t\t\t}\n\t\t\t\tlookup[i] = point;\n\t\t\t}\n\n\t\t\tif (j < points.length - 1) {\n\t\t\t\tlookup[i-1] = points[points.length-2];\n\t\t\t}\n\t\t}\n\t\t//this._calcEnd = (points[points.length-1].y !== 1 || points[0].y !== 0); //ensures that we don't run into floating point errors. As long as we're starting at 0 and ending at 1, tell GSAP to skip the final calculation and use 0/1 as the factor.\n\n\t\tthis.ease = p => {\n\t\t\tlet point = lookup[(p * l) | 0] || lookup[l - 1];\n\t\t\tif (point.nx < p) {\n\t\t\t\tpoint = point.n;\n\t\t\t}\n\t\t\treturn point.y + ((p - point.x) / point.cx) * point.cy;\n\t\t};\n\n\t\tthis.ease.custom = this;\n\n\t\tthis.id && gsap && gsap.registerEase(this.id, this.ease);\n\n\t\treturn this;\n\t}\n\n\tgetSVGData(config) {\n\t\treturn CustomEase.getSVGData(this, config);\n\t}\n\n\tstatic create(id, data, config) {\n\t\treturn (new CustomEase(id, data, config)).ease;\n\t}\n\n\tstatic register(core) {\n\t\tgsap = core;\n\t\t_initCore();\n\t}\n\n\tstatic get(id) {\n\t\treturn gsap.parseEase(id);\n\t}\n\n\tstatic getSVGData(ease, config) {\n\t\tconfig = config || {};\n\t\tlet width = config.width || 100,\n\t\t\theight = config.height || 100,\n\t\t\tx = config.x || 0,\n\t\t\ty = (config.y || 0) + height,\n\t\t\te = gsap.utils.toArray(config.path)[0],\n\t\t\ta, slope, i, inc, tx, ty, precision, threshold, prevX, prevY;\n\t\tif (config.invert) {\n\t\t\theight = -height;\n\t\t\ty = 0;\n\t\t}\n\t\tif (typeof(ease) === \"string\") {\n\t\t\tease = gsap.parseEase(ease);\n\t\t}\n\t\tif (ease.custom) {\n\t\t\tease = ease.custom;\n\t\t}\n\t\tif (ease instanceof CustomEase) {\n\t\t\ta = rawPathToString(transformRawPath([ease.segment], width, 0, 0, -height, x, y));\n\t\t} else {\n\t\t\ta = [x, y];\n\t\t\tprecision = Math.max(5, (config.precision || 1) * 200);\n\t\t\tinc = 1 / precision;\n\t\t\tprecision += 2;\n\t\t\tthreshold = 5 / precision;\n\t\t\tprevX = _round(x + inc * width);\n\t\t\tprevY = _round(y + ease(inc) * -height);\n\t\t\tslope = (prevY - y) / (prevX - x);\n\t\t\tfor (i = 2; i < precision; i++) {\n\t\t\t\ttx = _round(x + i * inc * width);\n\t\t\t\tty = _round(y + ease(i * inc) * -height);\n\t\t\t\tif (Math.abs((ty - prevY) / (tx - prevX) - slope) > threshold || i === precision - 1) { //only add points when the slope changes beyond the threshold\n\t\t\t\t\ta.push(prevX, prevY);\n\t\t\t\t\tslope = (ty - prevY) / (tx - prevX);\n\t\t\t\t}\n\t\t\t\tprevX = tx;\n\t\t\t\tprevY = ty;\n\t\t\t}\n\t\t\ta = \"M\" + a.join(\",\");\n\t\t}\n\t\te && e.setAttribute(\"d\", a);\n\t\treturn a;\n\t}\n\n}\n\nCustomEase.version = \"3.12.7\";\nCustomEase.headless = true;\n\n_getGSAP() && gsap.registerPlugin(CustomEase);\n\nexport { CustomEase as default };"],"names":["_round","value","Math","round","_svgPathExp","_scientific","_DEG2RAD","PI","_sin","sin","_cos","cos","_abs","abs","_sqrt","sqrt","arcToSegment","lastX","lastY","rx","ry","angle","largeArcFlag","sweepFlag","x","y","angleRad","cosAngle","sinAngle","TWOPI","dx2","dy2","x1","y1","x1_sq","y1_sq","radiiCheck","rx_sq","ry_sq","sq","coef","cx1","cy1","cx","cy","ux","uy","vx","vy","temp","angleStart","acos","angleExtent","isNaN","i","segments","ceil","rawPath","angleIncrement","controlLength","ma","mb","mc","md","push","length","stringToRawPath","d","line","sx","sy","ex","ey","difX","difY","segment","j","command","isRelative","startX","startY","beziers","prevCommand","flag1","flag2","a","replace","m","n","match","path","relativeX","relativeY","elements","points","errorMessage","console","log","toUpperCase","closed","substr","charAt","pop","totalPoints","_getGSAP","gsap","window","registerPlugin","_initCore","registerEase","CustomEase","create","_coreInitted","warn","_bezierToPoints","x2","y2","x3","y3","x4","y4","threshold","index","x12","y12","x23","y23","x34","y34","x123","y123","x234","y234","x1234","y1234","dx","dy","d2","d3","splice","_bonusValidated","_needsParsingExp","setData","data","config","l","a1","a2","inc","point","prevPoint","p","values","_numExp","closest","lookup","precision","fast","test","indexOf","unshift","_normalize","height","originY","max","tx","ty","_findMinimum","min","nx","ease","custom","this","id","getSVGData","register","core","get","parseEase","slope","prevX","prevY","width","e","utils","toArray","invert","rawPathToString","_isNumber","sl","s","result","transformRawPath","b","c","_dirty","join","setAttribute","version","headless"],"mappings":";;;;;;;;;6MA8BU,SAATA,EAASC,UAAUC,KAAKC,MAFT,IAEeF,GAFf,KAEwD,MAnBpEG,EAAc,mDAEjBC,EAAc,gCAEdC,EAAWJ,KAAKK,GAAK,IAErBC,EAAON,KAAKO,IACZC,EAAOR,KAAKS,IACZC,EAAOV,KAAKW,IACZC,EAAQZ,KAAKa,KAqjBd,SAASC,aAAaC,EAAOC,EAAOC,EAAIC,EAAIC,EAAOC,EAAcC,EAAWC,EAAGC,MAC1ER,IAAUO,GAAKN,IAAUO,GAG7BN,EAAKP,EAAKO,GACVC,EAAKR,EAAKQ,OACNM,EAAYL,EAAQ,IAAOf,EAC9BqB,EAAWjB,EAAKgB,GAChBE,EAAWpB,EAAKkB,GAChBnB,EAAKL,KAAKK,GACVsB,EAAa,EAALtB,EACRuB,GAAOb,EAAQO,GAAK,EACpBO,GAAOb,EAAQO,GAAK,EACpBO,EAAML,EAAWG,EAAMF,EAAWG,EAClCE,GAAOL,EAAWE,EAAMH,EAAWI,EACnCG,EAAQF,EAAKA,EACbG,EAAQF,EAAKA,EACbG,EAAaF,GAASf,EAAKA,GAAMgB,GAASf,EAAKA,GAC/B,EAAbgB,IACHjB,EAAKL,EAAMsB,GAAcjB,EACzBC,EAAKN,EAAMsB,GAAchB,OAEtBiB,EAAQlB,EAAKA,EAChBmB,EAAQlB,EAAKA,EACbmB,GAAOF,EAAQC,EAAUD,EAAQF,EAAUG,EAAQJ,IAAYG,EAAQF,EAAUG,EAAQJ,GACtFK,EAAK,IACRA,EAAK,OAEFC,GAASlB,IAAiBC,GAAc,EAAI,GAAKT,EAAMyB,GAC1DE,EAAetB,EAAKc,EAAMb,EAApBoB,EACNE,GAAgBtB,EAAKY,EAAMb,EAArBqB,EAGNG,EAAYhB,EAAWc,EAAMb,EAAWc,GAFjCzB,EAAQO,GAAK,EAGpBoB,EAAYhB,EAAWa,EAAMd,EAAWe,GAFjCxB,EAAQO,GAAK,EAGpBoB,GAAMb,EAAKS,GAAOtB,EAClB2B,GAAMb,EAAKS,GAAOtB,EAClB2B,IAAOf,EAAKS,GAAOtB,EACnB6B,IAAOf,EAAKS,GAAOtB,EACnB6B,EAAOJ,EAAKA,EAAKC,EAAKA,EACtBI,GAAeJ,EAAK,GAAM,EAAI,GAAK5C,KAAKiD,KAAKN,EAAK/B,EAAMmC,IACxDG,GAAgBP,EAAKG,EAAKF,EAAKC,EAAK,GAAM,EAAI,GAAK7C,KAAKiD,MAAMN,EAAKE,EAAKD,EAAKE,GAAMlC,EAAMmC,GAAQF,EAAKA,EAAKC,EAAKA,KACjHK,MAAMD,KAAiBA,EAAc7C,IAChCgB,GAA2B,EAAd6B,EACjBA,GAAevB,EACLN,GAAa6B,EAAc,IACrCA,GAAevB,GAEhBqB,GAAcrB,EACduB,GAAevB,MASdyB,EARGC,EAAWrD,KAAKsD,KAAK5C,EAAKwC,IAAgBvB,EAAQ,IACrD4B,EAAU,GACVC,EAAiBN,EAAcG,EAC/BI,EAAgB,EAAI,EAAInD,EAAKkD,EAAiB,IAAM,EAAIhD,EAAKgD,EAAiB,IAC9EE,EAAKjC,EAAWR,EAChB0C,EAAKjC,EAAWT,EAChB2C,EAAKlC,GAAYR,EACjB2C,EAAKpC,EAAWP,MAEZkC,EAAI,EAAGA,EAAIC,EAAUD,IAEzBtB,EAAKtB,EADLW,EAAQ6B,EAAaI,EAAII,GAEzBzB,EAAKzB,EAAKa,GACVwB,EAAKnC,EAAKW,GAASqC,GACnBZ,EAAKtC,EAAKa,GACVoC,EAAQO,KAAKhC,EAAK2B,EAAgB1B,EAAIA,EAAK0B,EAAgB3B,EAAIa,EAAKc,EAAgBb,EAAIA,EAAKa,EAAgBd,EAAIA,EAAIC,OAGjHQ,EAAI,EAAGA,EAAIG,EAAQQ,OAAQX,GAAG,EAClCtB,EAAKyB,EAAQH,GACbrB,EAAKwB,EAAQH,EAAE,GACfG,EAAQH,GAAKtB,EAAK4B,EAAK3B,EAAK6B,EAAKnB,EACjCc,EAAQH,EAAE,GAAKtB,EAAK6B,EAAK5B,EAAK8B,EAAKnB,SAEpCa,EAAQH,EAAE,GAAK9B,EACfiC,EAAQH,EAAE,GAAK7B,EACRgC,GAID,SAASS,gBAAgBC,GAUvB,SAAPC,GAAgBC,EAAIC,EAAIC,EAAIC,GAC3BC,GAAQF,EAAKF,GAAM,EACnBK,GAAQF,EAAKF,GAAM,EACnBK,EAAQX,KAAKK,EAAKI,EAAMH,EAAKI,EAAMH,EAAKE,EAAMD,EAAKE,EAAMH,EAAIC,OAJ9DlB,EAAGsB,EAAGpD,EAAGC,EAAGoD,EAASC,EAAYH,EAASI,EAAQC,EAAQP,EAAMC,EAAMO,EAASC,EAAaC,EAAOC,EARhGC,GAAKlB,EAAI,IAAImB,QAAQjF,EAAa,SAAAkF,OAAWC,GAAKD,SAAWC,EAAI,OAAe,KAALA,EAAe,EAAIA,IAAMC,MAAMrF,IAAgB,GAC7HsF,EAAO,GACPC,EAAY,EACZC,EAAY,EAEZC,EAAWR,EAAEpB,OACb6B,EAAS,EACTC,EAAe,0BAA4B5B,MAOvCA,IAAMd,MAAMgC,EAAE,KAAOhC,MAAMgC,EAAE,WACjCW,QAAQC,IAAIF,GACLL,MAEHpC,EAAI,EAAGA,EAAIuC,EAAUvC,OACzB4B,EAAcL,EACVxB,MAAMgC,EAAE/B,IAEXwB,GADAD,EAAUQ,EAAE/B,GAAG4C,iBACWb,EAAE/B,GAE5BA,IAED9B,GAAK6D,EAAE/B,EAAI,GACX7B,GAAK4D,EAAE/B,EAAI,GACPwB,IACHtD,GAAKmE,EACLlE,GAAKmE,GAEDtC,IACJyB,EAASvD,EACTwD,EAASvD,GAIM,MAAZoD,EACCF,IACCA,EAAQV,OAAS,IACpByB,EAAKzB,OAEL6B,GAAUnB,EAAQV,QAGpB0B,EAAYZ,EAASvD,EACrBoE,EAAYZ,EAASvD,EACrBkD,EAAU,CAACnD,EAAGC,GACdiE,EAAK1B,KAAKW,GACVrB,GAAK,EACLuB,EAAU,SAGJ,GAAgB,MAAZA,EAILC,IACJa,EAAYC,EAAY,IAHxBjB,EADIA,GACM,CAAC,EAAG,IAMPX,KAAKxC,EAAGC,EAAGkE,EAAuB,EAAXN,EAAE/B,EAAI,GAAQsC,EAAuB,EAAXP,EAAE/B,EAAI,GAASqC,GAAwB,EAAXN,EAAE/B,EAAI,GAAUsC,GAAwB,EAAXP,EAAE/B,EAAI,IACxHA,GAAK,OAGC,GAAgB,MAAZuB,EACVJ,EAAOkB,EACPjB,EAAOkB,EACa,MAAhBV,GAAuC,MAAhBA,IAC1BT,GAAQkB,EAAYhB,EAAQA,EAAQV,OAAS,GAC7CS,GAAQkB,EAAYjB,EAAQA,EAAQV,OAAS,IAEzCa,IACJa,EAAYC,EAAY,GAEzBjB,EAAQX,KAAKS,EAAMC,EAAMlD,EAAGC,EAAIkE,GAAwB,EAAXN,EAAE/B,EAAI,GAAUsC,GAAwB,EAAXP,EAAE/B,EAAI,IAChFA,GAAK,OAGC,GAAgB,MAAZuB,EACVJ,EAAOkB,EA7EI,EAAI,GA6EKnE,EAAImE,GACxBjB,EAAOkB,EA9EI,EAAI,GA8EKnE,EAAImE,GACnBd,IACJa,EAAYC,EAAY,GAEzBD,GAAwB,EAAXN,EAAE/B,EAAI,GACnBsC,GAAwB,EAAXP,EAAE/B,EAAI,GACnBqB,EAAQX,KAAKS,EAAMC,EAAMiB,EApFd,EAAI,GAoFuBnE,EAAImE,GAAwBC,EApFvD,EAAI,GAoFgEnE,EAAImE,GAAwBD,EAAWC,GACtHtC,GAAK,OAGC,GAAgB,MAAZuB,EACVJ,EAAOkB,EAAYhB,EAAQA,EAAQV,OAAS,GAC5CS,EAAOkB,EAAYjB,EAAQA,EAAQV,OAAS,GAC5CU,EAAQX,KAAK2B,EAAYlB,EAAMmB,EAAYlB,EAAMlD,EA3FtC,EAAI,GA2FwCmE,EAAmB,IAAPlB,EAAcjD,GAAgBC,EA3FtF,EAAI,GA2FwFmE,EAAmB,IAAPlB,EAAcjD,GAAiBkE,EAAYnE,EAAKoE,EAAYnE,GAC/K6B,GAAK,OAGC,GAAgB,MAAZuB,EACVT,GAAKuB,EAAWC,EAAYD,EAAYnE,EAAIoE,GAC5CtC,GAAK,OAGC,GAAgB,MAAZuB,EAEVT,GAAKuB,EAAWC,EAAWD,EAAYC,EAAYpE,GAAKsD,EAAac,EAAYD,EAAY,IAC7FrC,GAAK,OAGC,GAAgB,MAAZuB,GAA+B,MAAZA,EACb,MAAZA,IACHrD,EAAIuD,EACJtD,EAAIuD,EACJL,EAAQwB,QAAS,IAEF,MAAZtB,GAAyC,GAAtBjE,EAAK+E,EAAYnE,IAAkC,GAAtBZ,EAAKgF,EAAYnE,MACpE2C,GAAKuB,EAAWC,EAAWpE,EAAGC,GACd,MAAZoD,IACHvB,GAAK,IAGPqC,EAAYnE,EACZoE,EAAYnE,OAGN,GAAgB,MAAZoD,EAAiB,IAC3BM,EAAQE,EAAE/B,EAAE,GACZ8B,EAAQC,EAAE/B,EAAE,GACZmB,EAAOY,EAAE/B,EAAE,GACXoB,EAAOW,EAAE/B,EAAE,GACXsB,EAAI,EACe,EAAfO,EAAMlB,SACLkB,EAAMlB,OAAS,GAClBS,EAAOD,EACPA,EAAOW,EACPR,MAEAF,EAAOU,EACPX,EAAOU,EAAMiB,OAAO,GACpBxB,GAAG,GAEJQ,EAAQD,EAAMkB,OAAO,GACrBlB,EAAQA,EAAMkB,OAAO,IAEtBpB,EAAUjE,aAAa2E,EAAWC,GAAYP,EAAE/B,EAAE,IAAK+B,EAAE/B,EAAE,IAAK+B,EAAE/B,EAAE,IAAK6B,GAAQC,GAAQN,EAAaa,EAAY,GAAU,EAALlB,GAASK,EAAac,EAAY,GAAU,EAALlB,GAC9JpB,GAAKsB,EACDK,MACEL,EAAI,EAAGA,EAAIK,EAAQhB,OAAQW,IAC/BD,EAAQX,KAAKiB,EAAQL,IAGvBe,EAAYhB,EAAQA,EAAQV,OAAO,GACnC2B,EAAYjB,EAAQA,EAAQV,OAAO,QAGnC+B,QAAQC,IAAIF,UAGdzC,EAAIqB,EAAQV,QACJ,GACPyB,EAAKY,MACLhD,EAAI,GACMqB,EAAQ,KAAOA,EAAQrB,EAAE,IAAMqB,EAAQ,KAAOA,EAAQrB,EAAE,KAClEqB,EAAQwB,QAAS,GAElBT,EAAKa,YAAcT,EAASxC,EACrBoC,ECnzBI,SAAXc,WAAiBC,GAA4B,oBAAZC,SAA4BD,EAAOC,OAAOD,OAASA,EAAKE,gBAAkBF,EAC/F,SAAZG,KACCH,EAAOD,MAENC,EAAKI,aAAa,MAAOC,EAAWC,QACpCC,EAAe,GAEfhB,QAAQiB,KAAK,0CAIN,SAATjH,EAASC,YAAoB,IAARA,GAAgBA,EAAQ,GAAK,GAAK,KAAO,IAmC5C,SAAlBiH,EAA4BlF,EAAIC,EAAIkF,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAW3B,EAAQ4B,OAiB7EzD,EAhBG0D,GAAO3F,EAAKmF,GAAM,EACrBS,GAAO3F,EAAKmF,GAAM,EAClBS,GAAOV,EAAKE,GAAM,EAClBS,GAAOV,EAAKE,GAAM,EAClBS,GAAOV,EAAKE,GAAM,EAClBS,GAAOV,EAAKE,GAAM,EAClBS,GAAQN,EAAME,GAAO,EACrBK,GAAQN,EAAME,GAAO,EACrBK,GAAQN,EAAME,GAAO,EACrBK,GAAQN,EAAME,GAAO,EACrBK,GAASJ,EAAOE,GAAQ,EACxBG,GAASJ,EAAOE,GAAQ,EACxBG,EAAKhB,EAAKvF,EACVwG,EAAKhB,EAAKvF,EACVwG,EAAKvI,KAAKW,KAAKsG,EAAKI,GAAMiB,GAAMpB,EAAKI,GAAMe,GAC3CG,EAAKxI,KAAKW,KAAKwG,EAAKE,GAAMiB,GAAMlB,EAAKE,GAAMe,UAEvCzC,IACJA,EAAS,CAAC,CAACtE,EAAGQ,EAAIP,EAAGQ,GAAK,CAACT,EAAG+F,EAAI9F,EAAG+F,IACrCE,EAAQ,GAET5B,EAAO6C,OAAOjB,GAAS5B,EAAO7B,OAAS,EAAG,EAAG,CAACzC,EAAG6G,EAAO5G,EAAG6G,IAC/Bb,GAAac,EAAKA,EAAKC,EAAKA,IAAnDC,EAAKC,IAAOD,EAAKC,KACrBzE,EAAS6B,EAAO7B,OAChBiD,EAAgBlF,EAAIC,EAAI0F,EAAKC,EAAKK,EAAMC,EAAMG,EAAOC,EAAOb,EAAW3B,EAAQ4B,GAC/ER,EAAgBmB,EAAOC,EAAOH,EAAMC,EAAML,EAAKC,EAAKT,EAAIC,EAAIC,EAAW3B,EAAQ4B,EAAQ,GAAK5B,EAAO7B,OAASA,KAEtG6B,MA3ELW,EAAMO,IAaT4B,EACU,oCACVC,EAAmB,qBA+DP/B,4BAQZgC,QAAA,iBAAQC,EAAMC,GACbA,EAASA,GAAU,OAQlBC,EAAGC,EAAIC,EAAI7F,EAAG8F,EAAKxE,EAAGyE,EAAOC,EAAWC,EANrCC,GADJT,EAAOA,GAAQ,WACGtD,MAAMgE,GACvBC,EAAU,EACV5D,EAAS,GACT6D,EAAS,GACTC,EAAYZ,EAAOY,WAAa,EAChCC,EAAQD,GAAa,UAEjBb,KAAOA,GACRF,EAAiBiB,KAAKf,KAAWA,EAAKgB,QAAQ,MAAQhB,EAAKgB,QAAQ,KAAO,KAC7EP,EAAStF,gBAAgB6E,GAAM,IAGtB,KADVE,EAAIO,EAAOvF,QAEVuF,EAAOQ,QAAQ,EAAG,GAClBR,EAAOxF,KAAK,EAAG,GACfiF,EAAI,OACE,IAAKA,EAAI,GAAK,OACd,yBAEY,IAAdO,EAAO,IAA+B,IAAlBA,EAAOP,EAAI,IAlFxB,SAAbgB,WAAcT,EAAQU,EAAQC,GACxBA,GAAuB,IAAZA,IACfA,EAAUjK,KAAKkK,KAAKZ,EAAOA,EAAOvF,OAAO,IAAKuF,EAAO,SAOrDlG,EALG+G,GAAmB,EAAbb,EAAO,GAChBc,GAAMH,EACNlB,EAAIO,EAAOvF,OACXI,EAAK,IAAMmF,EAAOP,EAAI,GAAKoB,GAC3B/F,GAAM4F,IAAYhK,KAAKW,IAAK2I,EAAOP,EAAI,GAAMO,EAAO,IAAM,KAASA,EAAOP,EAAI,GAAMO,EAAO,IAlB9E,SAAfe,aAAef,OAGblG,EAFG2F,EAAIO,EAAOvF,OACduG,EAPQ,SASJlH,EAAI,EAAGA,EAAI2F,EAAG3F,GAAK,GACtBkG,EAAOlG,GAAKkH,IAAQA,GAAOhB,EAAOlG,WAE7BkH,EAW4FD,CAAaf,GAAUc,GAAMd,EAAOP,EAAI,GAAKqB,OAG/IhG,EADGA,EACE,EAAIA,GAEHD,EAEFf,EAAI,EAAGA,EAAI2F,EAAG3F,GAAK,EACvBkG,EAAOlG,KAAOkG,EAAOlG,GAAK+G,GAAMhG,EAChCmF,EAAOlG,EAAI,KAAOkG,EAAOlG,EAAI,GAAKgH,GAAMhG,EAkExC2F,CAAWT,EAAQR,EAAOkB,OAAQlB,EAAOmB,cAErCxF,QAAU6E,EACVlG,EAAI,EAAGA,EAAI2F,EAAG3F,GAAK,EACvB4F,EAAK,CAAC1H,GAAIgI,EAAOlG,EAAI,GAAI7B,GAAI+H,EAAOlG,EAAI,IACxC6F,EAAK,CAAC3H,GAAIgI,EAAOlG,EAAI,GAAI7B,GAAI+H,EAAOlG,EAAI,IACxCwC,EAAO9B,KAAKkF,EAAIC,GAChBjC,EAAgBgC,EAAG1H,EAAG0H,EAAGzH,GAAI+H,EAAOlG,IAAKkG,EAAOlG,EAAI,IAAKkG,EAAOlG,EAAI,IAAKkG,EAAOlG,EAAI,GAAI6F,EAAG3H,EAAG2H,EAAG1H,EAAG,GAAiB,IAAZmI,GAAqB9D,EAAQA,EAAO7B,OAAS,OAEvJgF,EAAInD,EAAO7B,OACNX,EAAI,EAAGA,EAAI2F,EAAG3F,IAClB+F,EAAQvD,EAAOxC,GACfgG,EAAYxD,EAAOxC,EAAI,IAAM+F,GACxBA,EAAM7H,EAAI8H,EAAU9H,GAAM8H,EAAU7H,IAAM4H,EAAM5H,GAAK6H,EAAU9H,IAAM6H,EAAM7H,GAAM6H,IAAUC,IAAcD,EAAM7H,GAAK,GACxH8H,EAAU3G,GAAK0G,EAAM7H,EAAI8H,EAAU9H,EACnC8H,EAAU1G,GAAKyG,EAAM5H,EAAI6H,EAAU7H,EACnC6H,EAAU9D,EAAI6D,EACdC,EAAUmB,GAAKpB,EAAM7H,EACjBqI,GAAY,EAAJvG,GAAuF,EAA9EpD,KAAKW,IAAIyI,EAAU1G,GAAK0G,EAAU3G,GAAKmD,EAAOxC,EAAI,GAAGV,GAAKkD,EAAOxC,EAAI,GAAGX,MAC5FkH,EAAO,GAEJP,EAAU3G,GAAK+G,IACbJ,EAAU3G,GAQd+G,EAAUJ,EAAU3G,IAPpB2G,EAAU3G,GAAK,KACXW,IAAM2F,EAAI,IACbK,EAAU9H,GAAK,KACfkI,EAAUxJ,KAAKsK,IAAId,EAAS,MAC5BG,EAAO,OAOV/D,EAAO6C,OAAOrF,IAAK,GACnB2F,QAIFG,EAAM,GADNH,EAAK,EAAIS,EAAU,EAAK,GAGxBL,EAAQvD,EADRlB,EAAI,GAEAiF,EAAM,KACJvG,EAAI,EAAGA,EAAI2F,EAAG3F,IAClBiG,EAAIjG,EAAI8F,EACJC,EAAMoB,GAAKlB,IACdF,EAAQvD,IAASlB,IAElBsE,EAAKG,EAAM5H,GAAM8H,EAAIF,EAAM7H,GAAK6H,EAAM1G,GAAM0G,EAAMzG,GAClD+G,EAAOrG,GAAK,CAAC9B,EAAG+H,EAAG5G,GAAIyG,EAAK3H,EAAGyH,EAAItG,GAAI,EAAG6H,GAAI,GAC1CnH,IACHqG,EAAOrG,EAAI,GAAGV,GAAKsG,EAAKS,EAAOrG,EAAI,GAAG7B,GAGxCmD,EAAIkB,EAAOA,EAAO7B,OAAS,GAC3B0F,EAAOV,EAAI,GAAGrG,GAAKgC,EAAEnD,EAAIyH,EACzBS,EAAOV,EAAI,GAAGtG,GAAKiC,EAAEpD,EAAImI,EAAOA,EAAO1F,OAAS,GAAGzC,MAC7C,KACD8B,EAAI,EAAGA,EAAI2F,EAAG3F,IACd+F,EAAMoB,GAAKnH,EAAI8F,IAClBC,EAAQvD,IAASlB,IAElB+E,EAAOrG,GAAK+F,EAGTzE,EAAIkB,EAAO7B,OAAS,IACvB0F,EAAOrG,EAAE,GAAKwC,EAAOA,EAAO7B,OAAO,gBAKhCyG,KAAO,SAAAnB,OACPF,EAAQM,EAAQJ,EAAIN,EAAK,IAAMU,EAAOV,EAAI,UAC1CI,EAAMoB,GAAKlB,IACdF,EAAQA,EAAM7D,GAER6D,EAAM5H,GAAM8H,EAAIF,EAAM7H,GAAK6H,EAAM1G,GAAM0G,EAAMzG,UAGhD8H,KAAKC,OAASC,MAEdC,IAAMpE,GAAQA,EAAKI,aAAa+D,KAAKC,GAAID,KAAKF,MAE5CE,QAGRE,WAAA,oBAAW9B,UACHlC,WAAWgE,WAAWF,KAAM5B,eAG7BjC,OAAP,gBAAc8D,EAAI9B,EAAMC,UACf,IAAIlC,WAAW+D,EAAI9B,EAAMC,GAAS0B,iBAGpCK,SAAP,kBAAgBC,GACfvE,EAAOuE,EACPpE,gBAGMqE,IAAP,aAAWJ,UACHpE,EAAKyE,UAAUL,eAGhBC,WAAP,oBAAkBJ,EAAM1B,OAOtB3D,EAAG8F,EAAO7H,EAAG8F,EAAKiB,EAAIC,EAAIV,EAAWnC,EAAW2D,EAAOC,EALpDC,GADJtC,EAASA,GAAU,IACAsC,OAAS,IAC3BpB,EAASlB,EAAOkB,QAAU,IAC1B1I,EAAIwH,EAAOxH,GAAK,EAChBC,GAAKuH,EAAOvH,GAAK,GAAKyI,EACtBqB,EAAI9E,EAAK+E,MAAMC,QAAQzC,EAAOtD,MAAM,MAEjCsD,EAAO0C,SACVxB,GAAUA,EACVzI,EAAI,GAEgB,iBAAViJ,IACVA,EAAOjE,EAAKyE,UAAUR,IAEnBA,EAAKC,SACRD,EAAOA,EAAKC,QAETD,aAAgB5D,WACnBzB,EDg2BI,SAASsG,gBAAgBlI,IA5jCnB,SAAZmI,UAAY3L,SAA2B,iBAAXA,EA6jCxB2L,CAAUnI,EAAQ,MACrBA,EAAU,CAACA,QAIXoI,EAAIC,EAAGxI,EAAGqB,EAFPoH,EAAS,GACZ9C,EAAIxF,EAAQQ,WAER6H,EAAI,EAAGA,EAAI7C,EAAG6C,IAAK,KACvBnH,EAAUlB,EAAQqI,GAClBC,GAAU,IAAM/L,EAAO2E,EAAQ,IAAM,IAAM3E,EAAO2E,EAAQ,IAAM,KAChEkH,EAAKlH,EAAQV,OACRX,EAAI,EAAGA,EAAIuI,EAAIvI,IACnByI,GAAU/L,EAAO2E,EAAQrB,MAAQ,IAAMtD,EAAO2E,EAAQrB,MAAQ,IAAMtD,EAAO2E,EAAQrB,MAAQ,IAAMtD,EAAO2E,EAAQrB,MAAQ,IAAMtD,EAAO2E,EAAQrB,MAAQ,IAAMtD,EAAO2E,EAAQrB,IAAM,IAE7KqB,EAAQwB,SACX4F,GAAU,YAGLA,ECl3BDJ,CDiUA,SAASK,iBAAiBvI,EAAS4B,EAAG4G,EAAGC,EAAG/H,EAAGkG,EAAIC,WAExD3F,EAASsE,EAAG3F,EAAG9B,EAAGC,EADfmD,EAAInB,EAAQQ,QAEF,IAALW,OAERqE,GADAtE,EAAUlB,EAAQmB,IACNX,OACPX,EAAI,EAAGA,EAAI2F,EAAG3F,GAAK,EACvB9B,EAAImD,EAAQrB,GACZ7B,EAAIkD,EAAQrB,EAAE,GACdqB,EAAQrB,GAAK9B,EAAI6D,EAAI5D,EAAIyK,EAAI7B,EAC7B1F,EAAQrB,EAAE,GAAK9B,EAAIyK,EAAIxK,EAAI0C,EAAImG,SAGjC7G,EAAQ0I,OAAS,EACV1I,EC/UeuI,CAAiB,CAACtB,EAAK/F,SAAU2G,EAAO,EAAG,GAAIpB,EAAQ1I,EAAGC,QACxE,KACN4D,EAAI,CAAC7D,EAAGC,GAER2H,EAAM,GADNQ,EAAY1J,KAAKkK,IAAI,EAA6B,KAAzBpB,EAAOY,WAAa,KAG7CnC,EAAY,GADZmC,GAAa,GAEbwB,EAAQpL,EAAOwB,EAAI4H,EAAMkC,GAEzBH,IADAE,EAAQrL,EAAOyB,EAAIiJ,EAAKtB,IAAQc,IACfzI,IAAM2J,EAAQ5J,GAC1B8B,EAAI,EAAGA,EAAIsG,EAAWtG,IAC1B+G,EAAKrK,EAAOwB,EAAI8B,EAAI8F,EAAMkC,GAC1BhB,EAAKtK,EAAOyB,EAAIiJ,EAAKpH,EAAI8F,IAAQc,IAC7BhK,KAAKW,KAAKyJ,EAAKe,IAAUhB,EAAKe,GAASD,GAAS1D,GAAanE,IAAMsG,EAAY,KAClFvE,EAAErB,KAAKoH,EAAOC,GACdF,GAASb,EAAKe,IAAUhB,EAAKe,IAE9BA,EAAQf,EACRgB,EAAQf,EAETjF,EAAI,IAAMA,EAAE+G,KAAK,YAElBb,GAAKA,EAAEc,aAAa,IAAKhH,GAClBA,mCA9KIwF,EAAI9B,EAAMC,GACrBhC,GAAgBJ,SACXiE,GAAKA,EACSD,KAAK9B,QAAQC,EAAMC,GAgLxClC,EAAWwF,QAAU,SACrBxF,EAAWyF,UAAW,EAEtB/F,KAAcC,EAAKE,eAAeG"} \ No newline at end of file diff --git a/node_modules/gsap/dist/Draggable.js b/node_modules/gsap/dist/Draggable.js new file mode 100644 index 0000000000000000000000000000000000000000..a67f7acc5c70962dde57fc6d408805dfd77b4dc1 --- /dev/null +++ b/node_modules/gsap/dist/Draggable.js @@ -0,0 +1,2966 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + function _inheritsLoose(subClass, superClass) { + subClass.prototype = Object.create(superClass.prototype); + subClass.prototype.constructor = subClass; + subClass.__proto__ = superClass; + } + + function _assertThisInitialized(self) { + if (self === void 0) { + throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); + } + + return self; + } + + var _doc, + _win, + _docElement, + _body, + _divContainer, + _svgContainer, + _identityMatrix, + _gEl, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _hasOffsetBug, + _setDoc = function _setDoc(element) { + var doc = element.ownerDocument || element; + + if (!(_transformProp in element.style) && "msTransform" in element.style) { + _transformProp = "msTransform"; + _transformOriginProp = _transformProp + "Origin"; + } + + while (doc.parentNode && (doc = doc.parentNode)) {} + + _win = window; + _identityMatrix = new Matrix2D(); + + if (doc) { + _doc = doc; + _docElement = doc.documentElement; + _body = doc.body; + _gEl = _doc.createElementNS("http://www.w3.org/2000/svg", "g"); + _gEl.style.transform = "none"; + var d1 = doc.createElement("div"), + d2 = doc.createElement("div"), + root = doc && (doc.body || doc.firstElementChild); + + if (root && root.appendChild) { + root.appendChild(d1); + d1.appendChild(d2); + d1.setAttribute("style", "position:static;transform:translate3d(0,0,1px)"); + _hasOffsetBug = d2.offsetParent !== d1; + root.removeChild(d1); + } + } + + return doc; + }, + _forceNonZeroScale = function _forceNonZeroScale(e) { + var a, cache; + + while (e && e !== _body) { + cache = e._gsap; + cache && cache.uncache && cache.get(e, "x"); + + if (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) { + cache.scaleX = cache.scaleY = 1e-4; + cache.renderTransform(1, cache); + a ? a.push(cache) : a = [cache]; + } + + e = e.parentNode; + } + + return a; + }, + _svgTemps = [], + _divTemps = [], + _getDocScrollTop = function _getDocScrollTop() { + return _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0; + }, + _getDocScrollLeft = function _getDocScrollLeft() { + return _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0; + }, + _svgOwner = function _svgOwner(element) { + return element.ownerSVGElement || ((element.tagName + "").toLowerCase() === "svg" ? element : null); + }, + _isFixed = function _isFixed(element) { + if (_win.getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + return _isFixed(element); + } + }, + _createSibling = function _createSibling(element, i) { + if (element.parentNode && (_doc || _setDoc(element))) { + var svg = _svgOwner(element), + ns = svg ? svg.getAttribute("xmlns") || "http://www.w3.org/2000/svg" : "http://www.w3.org/1999/xhtml", + type = svg ? i ? "rect" : "g" : "div", + x = i !== 2 ? 0 : 100, + y = i === 3 ? 100 : 0, + css = "position:absolute;display:block;pointer-events:none;margin:0;padding:0;", + e = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, "http"), type) : _doc.createElement(type); + + if (i) { + if (!svg) { + if (!_divContainer) { + _divContainer = _createSibling(element); + _divContainer.style.cssText = css; + } + + e.style.cssText = css + "width:0.1px;height:0.1px;top:" + y + "px;left:" + x + "px"; + + _divContainer.appendChild(e); + } else { + _svgContainer || (_svgContainer = _createSibling(element)); + e.setAttribute("width", 0.01); + e.setAttribute("height", 0.01); + e.setAttribute("transform", "translate(" + x + "," + y + ")"); + + _svgContainer.appendChild(e); + } + } + + return e; + } + + throw "Need document and parent."; + }, + _consolidate = function _consolidate(m) { + var c = new Matrix2D(), + i = 0; + + for (; i < m.numberOfItems; i++) { + c.multiply(m.getItem(i).matrix); + } + + return c; + }, + _getCTM = function _getCTM(svg) { + var m = svg.getCTM(), + transform; + + if (!m) { + transform = svg.style[_transformProp]; + svg.style[_transformProp] = "none"; + svg.appendChild(_gEl); + m = _gEl.getCTM(); + svg.removeChild(_gEl); + transform ? svg.style[_transformProp] = transform : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, "-$1").toLowerCase()); + } + + return m || _identityMatrix.clone(); + }, + _placeSiblings = function _placeSiblings(element, adjustGOffset) { + var svg = _svgOwner(element), + isRootSVG = element === svg, + siblings = svg ? _svgTemps : _divTemps, + parent = element.parentNode, + container, + m, + b, + x, + y, + cs; + + if (element === _win) { + return element; + } + + siblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3)); + container = svg ? _svgContainer : _divContainer; + + if (svg) { + if (isRootSVG) { + b = _getCTM(element); + x = -b.e / b.a; + y = -b.f / b.d; + m = _identityMatrix; + } else if (element.getBBox) { + b = element.getBBox(); + m = element.transform ? element.transform.baseVal : {}; + m = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; + x = m.a * b.x + m.c * b.y; + y = m.b * b.x + m.d * b.y; + } else { + m = new Matrix2D(); + x = y = 0; + } + + if (adjustGOffset && element.tagName.toLowerCase() === "g") { + x = y = 0; + } + + (isRootSVG ? svg : parent).appendChild(container); + container.setAttribute("transform", "matrix(" + m.a + "," + m.b + "," + m.c + "," + m.d + "," + (m.e + x) + "," + (m.f + y) + ")"); + } else { + x = y = 0; + + if (_hasOffsetBug) { + m = element.offsetParent; + b = element; + + while (b && (b = b.parentNode) && b !== m && b.parentNode) { + if ((_win.getComputedStyle(b)[_transformProp] + "").length > 4) { + x = b.offsetLeft; + y = b.offsetTop; + b = 0; + } + } + } + + cs = _win.getComputedStyle(element); + + if (cs.position !== "absolute" && cs.position !== "fixed") { + m = element.offsetParent; + + while (parent && parent !== m) { + x += parent.scrollLeft || 0; + y += parent.scrollTop || 0; + parent = parent.parentNode; + } + } + + b = container.style; + b.top = element.offsetTop - y + "px"; + b.left = element.offsetLeft - x + "px"; + b[_transformProp] = cs[_transformProp]; + b[_transformOriginProp] = cs[_transformOriginProp]; + b.position = cs.position === "fixed" ? "fixed" : "absolute"; + element.parentNode.appendChild(container); + } + + return container; + }, + _setMatrix = function _setMatrix(m, a, b, c, d, e, f) { + m.a = a; + m.b = b; + m.c = c; + m.d = d; + m.e = e; + m.f = f; + return m; + }; + + var Matrix2D = function () { + function Matrix2D(a, b, c, d, e, f) { + if (a === void 0) { + a = 1; + } + + if (b === void 0) { + b = 0; + } + + if (c === void 0) { + c = 0; + } + + if (d === void 0) { + d = 1; + } + + if (e === void 0) { + e = 0; + } + + if (f === void 0) { + f = 0; + } + + _setMatrix(this, a, b, c, d, e, f); + } + + var _proto = Matrix2D.prototype; + + _proto.inverse = function inverse() { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + determinant = a * d - b * c || 1e-10; + return _setMatrix(this, d / determinant, -b / determinant, -c / determinant, a / determinant, (c * f - d * e) / determinant, -(a * f - b * e) / determinant); + }; + + _proto.multiply = function multiply(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + a2 = matrix.a, + b2 = matrix.c, + c2 = matrix.b, + d2 = matrix.d, + e2 = matrix.e, + f2 = matrix.f; + return _setMatrix(this, a2 * a + c2 * c, a2 * b + c2 * d, b2 * a + d2 * c, b2 * b + d2 * d, e + e2 * a + f2 * c, f + e2 * b + f2 * d); + }; + + _proto.clone = function clone() { + return new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f); + }; + + _proto.equals = function equals(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + return a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f; + }; + + _proto.apply = function apply(point, decoratee) { + if (decoratee === void 0) { + decoratee = {}; + } + + var x = point.x, + y = point.y, + a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + decoratee.x = x * a + y * c + e || 0; + decoratee.y = x * b + y * d + f || 0; + return decoratee; + }; + + return Matrix2D; + }(); + function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { + if (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) { + return new Matrix2D(); + } + + var zeroScales = _forceNonZeroScale(element), + svg = _svgOwner(element), + temps = svg ? _svgTemps : _divTemps, + container = _placeSiblings(element, adjustGOffset), + b1 = temps[0].getBoundingClientRect(), + b2 = temps[1].getBoundingClientRect(), + b3 = temps[2].getBoundingClientRect(), + parent = container.parentNode, + isFixed = !includeScrollInFixed && _isFixed(element), + m = new Matrix2D((b2.left - b1.left) / 100, (b2.top - b1.top) / 100, (b3.left - b1.left) / 100, (b3.top - b1.top) / 100, b1.left + (isFixed ? 0 : _getDocScrollLeft()), b1.top + (isFixed ? 0 : _getDocScrollTop())); + + parent.removeChild(container); + + if (zeroScales) { + b1 = zeroScales.length; + + while (b1--) { + b2 = zeroScales[b1]; + b2.scaleX = b2.scaleY = 0; + b2.renderTransform(1, b2); + } + } + + return inverse ? m.inverse() : m; + } + + var gsap, + _win$1, + _doc$1, + _docElement$1, + _body$1, + _tempDiv, + _placeholderDiv, + _coreInitted, + _checkPrefix, + _toArray, + _supportsPassive, + _isTouchDevice, + _touchEventLookup, + _isMultiTouching, + _isAndroid, + InertiaPlugin, + _defaultCursor, + _supportsPointer, + _context, + _getStyleSaver, + _dragCount = 0, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _isFunction = function _isFunction(value) { + return typeof value === "function"; + }, + _isObject = function _isObject(value) { + return typeof value === "object"; + }, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; + }, + _emptyFunc = function _emptyFunc() { + return false; + }, + _transformProp$1 = "transform", + _transformOriginProp$1 = "transformOrigin", + _round = function _round(value) { + return Math.round(value * 10000) / 10000; + }, + _isArray = Array.isArray, + _createElement = function _createElement(type, ns) { + var e = _doc$1.createElementNS ? _doc$1.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc$1.createElement(type); + return e.style ? e : _doc$1.createElement(type); + }, + _RAD2DEG = 180 / Math.PI, + _bigNum = 1e20, + _identityMatrix$1 = new Matrix2D(), + _getTime = Date.now || function () { + return new Date().getTime(); + }, + _renderQueue = [], + _lookup = {}, + _lookupCount = 0, + _clickableTagExp = /^(?:a|input|textarea|button|select)$/i, + _lastDragTime = 0, + _temp1 = {}, + _windowProxy = {}, + _copy = function _copy(obj, factor) { + var copy = {}, + p; + + for (p in obj) { + copy[p] = factor ? obj[p] * factor : obj[p]; + } + + return copy; + }, + _extend = function _extend(obj, defaults) { + for (var p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + + return obj; + }, + _setTouchActionForAllDescendants = function _setTouchActionForAllDescendants(elements, value) { + var i = elements.length, + children; + + while (i--) { + value ? elements[i].style.touchAction = value : elements[i].style.removeProperty("touch-action"); + children = elements[i].children; + children && children.length && _setTouchActionForAllDescendants(children, value); + } + }, + _renderQueueTick = function _renderQueueTick() { + return _renderQueue.forEach(function (func) { + return func(); + }); + }, + _addToRenderQueue = function _addToRenderQueue(func) { + _renderQueue.push(func); + + if (_renderQueue.length === 1) { + gsap.ticker.add(_renderQueueTick); + } + }, + _renderQueueTimeout = function _renderQueueTimeout() { + return !_renderQueue.length && gsap.ticker.remove(_renderQueueTick); + }, + _removeFromRenderQueue = function _removeFromRenderQueue(func) { + var i = _renderQueue.length; + + while (i--) { + if (_renderQueue[i] === func) { + _renderQueue.splice(i, 1); + } + } + + gsap.to(_renderQueueTimeout, { + overwrite: true, + delay: 15, + duration: 0, + onComplete: _renderQueueTimeout, + data: "_draggable" + }); + }, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + + return obj; + }, + _addListener = function _addListener(element, type, func, capture) { + if (element.addEventListener) { + var touchType = _touchEventLookup[type]; + capture = capture || (_supportsPassive ? { + passive: false + } : null); + element.addEventListener(touchType || type, func, capture); + touchType && type !== touchType && element.addEventListener(type, func, capture); + } + }, + _removeListener = function _removeListener(element, type, func, capture) { + if (element.removeEventListener) { + var touchType = _touchEventLookup[type]; + element.removeEventListener(touchType || type, func, capture); + touchType && type !== touchType && element.removeEventListener(type, func, capture); + } + }, + _preventDefault = function _preventDefault(event) { + event.preventDefault && event.preventDefault(); + event.preventManipulation && event.preventManipulation(); + }, + _hasTouchID = function _hasTouchID(list, ID) { + var i = list.length; + + while (i--) { + if (list[i].identifier === ID) { + return true; + } + } + }, + _onMultiTouchDocumentEnd = function _onMultiTouchDocumentEnd(event) { + _isMultiTouching = event.touches && _dragCount < event.touches.length; + + _removeListener(event.target, "touchend", _onMultiTouchDocumentEnd); + }, + _onMultiTouchDocument = function _onMultiTouchDocument(event) { + _isMultiTouching = event.touches && _dragCount < event.touches.length; + + _addListener(event.target, "touchend", _onMultiTouchDocumentEnd); + }, + _getDocScrollTop$1 = function _getDocScrollTop(doc) { + return _win$1.pageYOffset || doc.scrollTop || doc.documentElement.scrollTop || doc.body.scrollTop || 0; + }, + _getDocScrollLeft$1 = function _getDocScrollLeft(doc) { + return _win$1.pageXOffset || doc.scrollLeft || doc.documentElement.scrollLeft || doc.body.scrollLeft || 0; + }, + _addScrollListener = function _addScrollListener(e, callback) { + _addListener(e, "scroll", callback); + + if (!_isRoot(e.parentNode)) { + _addScrollListener(e.parentNode, callback); + } + }, + _removeScrollListener = function _removeScrollListener(e, callback) { + _removeListener(e, "scroll", callback); + + if (!_isRoot(e.parentNode)) { + _removeScrollListener(e.parentNode, callback); + } + }, + _isRoot = function _isRoot(e) { + return !!(!e || e === _docElement$1 || e.nodeType === 9 || e === _doc$1.body || e === _win$1 || !e.nodeType || !e.parentNode); + }, + _getMaxScroll = function _getMaxScroll(element, axis) { + var dim = axis === "x" ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return Math.max(0, _isRoot(element) ? Math.max(_docElement$1[scroll], _body$1[scroll]) - (_win$1["inner" + dim] || _docElement$1[client] || _body$1[client]) : element[scroll] - element[client]); + }, + _recordMaxScrolls = function _recordMaxScrolls(e, skipCurrent) { + var x = _getMaxScroll(e, "x"), + y = _getMaxScroll(e, "y"); + + if (_isRoot(e)) { + e = _windowProxy; + } else { + _recordMaxScrolls(e.parentNode, skipCurrent); + } + + e._gsMaxScrollX = x; + e._gsMaxScrollY = y; + + if (!skipCurrent) { + e._gsScrollX = e.scrollLeft || 0; + e._gsScrollY = e.scrollTop || 0; + } + }, + _setStyle = function _setStyle(element, property, value) { + var style = element.style; + + if (!style) { + return; + } + + if (_isUndefined(style[property])) { + property = _checkPrefix(property, element) || property; + } + + if (value == null) { + style.removeProperty && style.removeProperty(property.replace(/([A-Z])/g, "-$1").toLowerCase()); + } else { + style[property] = value; + } + }, + _getComputedStyle = function _getComputedStyle(element) { + return _win$1.getComputedStyle(element instanceof Element ? element : element.host || (element.parentNode || {}).host || element); + }, + _tempRect = {}, + _parseRect = function _parseRect(e) { + if (e === _win$1) { + _tempRect.left = _tempRect.top = 0; + _tempRect.width = _tempRect.right = _docElement$1.clientWidth || e.innerWidth || _body$1.clientWidth || 0; + _tempRect.height = _tempRect.bottom = (e.innerHeight || 0) - 20 < _docElement$1.clientHeight ? _docElement$1.clientHeight : e.innerHeight || _body$1.clientHeight || 0; + return _tempRect; + } + + var doc = e.ownerDocument || _doc$1, + r = !_isUndefined(e.pageX) ? { + left: e.pageX - _getDocScrollLeft$1(doc), + top: e.pageY - _getDocScrollTop$1(doc), + right: e.pageX - _getDocScrollLeft$1(doc) + 1, + bottom: e.pageY - _getDocScrollTop$1(doc) + 1 + } : !e.nodeType && !_isUndefined(e.left) && !_isUndefined(e.top) ? e : _toArray(e)[0].getBoundingClientRect(); + + if (_isUndefined(r.right) && !_isUndefined(r.width)) { + r.right = r.left + r.width; + r.bottom = r.top + r.height; + } else if (_isUndefined(r.width)) { + r = { + width: r.right - r.left, + height: r.bottom - r.top, + right: r.right, + left: r.left, + bottom: r.bottom, + top: r.top + }; + } + + return r; + }, + _dispatchEvent = function _dispatchEvent(target, type, callbackName) { + var vars = target.vars, + callback = vars[callbackName], + listeners = target._listeners[type], + result; + + if (_isFunction(callback)) { + result = callback.apply(vars.callbackScope || target, vars[callbackName + "Params"] || [target.pointerEvent]); + } + + if (listeners && target.dispatchEvent(type) === false) { + result = false; + } + + return result; + }, + _getBounds = function _getBounds(target, context) { + var e = _toArray(target)[0], + top, + left, + offset; + + if (!e.nodeType && e !== _win$1) { + if (!_isUndefined(target.left)) { + offset = { + x: 0, + y: 0 + }; + return { + left: target.left - offset.x, + top: target.top - offset.y, + width: target.width, + height: target.height + }; + } + + left = target.min || target.minX || target.minRotation || 0; + top = target.min || target.minY || 0; + return { + left: left, + top: top, + width: (target.max || target.maxX || target.maxRotation || 0) - left, + height: (target.max || target.maxY || 0) - top + }; + } + + return _getElementBounds(e, context); + }, + _point1 = {}, + _getElementBounds = function _getElementBounds(element, context) { + context = _toArray(context)[0]; + var isSVG = element.getBBox && element.ownerSVGElement, + doc = element.ownerDocument || _doc$1, + left, + right, + top, + bottom, + matrix, + p1, + p2, + p3, + p4, + bbox, + width, + height, + cs; + + if (element === _win$1) { + top = _getDocScrollTop$1(doc); + left = _getDocScrollLeft$1(doc); + right = left + (doc.documentElement.clientWidth || element.innerWidth || doc.body.clientWidth || 0); + bottom = top + ((element.innerHeight || 0) - 20 < doc.documentElement.clientHeight ? doc.documentElement.clientHeight : element.innerHeight || doc.body.clientHeight || 0); + } else if (context === _win$1 || _isUndefined(context)) { + return element.getBoundingClientRect(); + } else { + left = top = 0; + + if (isSVG) { + bbox = element.getBBox(); + width = bbox.width; + height = bbox.height; + } else { + if (element.viewBox && (bbox = element.viewBox.baseVal)) { + left = bbox.x || 0; + top = bbox.y || 0; + width = bbox.width; + height = bbox.height; + } + + if (!width) { + cs = _getComputedStyle(element); + bbox = cs.boxSizing === "border-box"; + width = (parseFloat(cs.width) || element.clientWidth || 0) + (bbox ? 0 : parseFloat(cs.borderLeftWidth) + parseFloat(cs.borderRightWidth)); + height = (parseFloat(cs.height) || element.clientHeight || 0) + (bbox ? 0 : parseFloat(cs.borderTopWidth) + parseFloat(cs.borderBottomWidth)); + } + } + + right = width; + bottom = height; + } + + if (element === context) { + return { + left: left, + top: top, + width: right - left, + height: bottom - top + }; + } + + matrix = getGlobalMatrix(context, true).multiply(getGlobalMatrix(element)); + p1 = matrix.apply({ + x: left, + y: top + }); + p2 = matrix.apply({ + x: right, + y: top + }); + p3 = matrix.apply({ + x: right, + y: bottom + }); + p4 = matrix.apply({ + x: left, + y: bottom + }); + left = Math.min(p1.x, p2.x, p3.x, p4.x); + top = Math.min(p1.y, p2.y, p3.y, p4.y); + return { + left: left, + top: top, + width: Math.max(p1.x, p2.x, p3.x, p4.x) - left, + height: Math.max(p1.y, p2.y, p3.y, p4.y) - top + }; + }, + _parseInertia = function _parseInertia(draggable, snap, max, min, factor, forceZeroVelocity) { + var vars = {}, + a, + i, + l; + + if (snap) { + if (factor !== 1 && snap instanceof Array) { + vars.end = a = []; + l = snap.length; + + if (_isObject(snap[0])) { + for (i = 0; i < l; i++) { + a[i] = _copy(snap[i], factor); + } + } else { + for (i = 0; i < l; i++) { + a[i] = snap[i] * factor; + } + } + + max += 1.1; + min -= 1.1; + } else if (_isFunction(snap)) { + vars.end = function (value) { + var result = snap.call(draggable, value), + copy, + p; + + if (factor !== 1) { + if (_isObject(result)) { + copy = {}; + + for (p in result) { + copy[p] = result[p] * factor; + } + + result = copy; + } else { + result *= factor; + } + } + + return result; + }; + } else { + vars.end = snap; + } + } + + if (max || max === 0) { + vars.max = max; + } + + if (min || min === 0) { + vars.min = min; + } + + if (forceZeroVelocity) { + vars.velocity = 0; + } + + return vars; + }, + _isClickable = function _isClickable(element) { + var data; + return !element || !element.getAttribute || element === _body$1 ? false : (data = element.getAttribute("data-clickable")) === "true" || data !== "false" && (_clickableTagExp.test(element.nodeName + "") || element.getAttribute("contentEditable") === "true") ? true : _isClickable(element.parentNode); + }, + _setSelectable = function _setSelectable(elements, selectable) { + var i = elements.length, + e; + + while (i--) { + e = elements[i]; + e.ondragstart = e.onselectstart = selectable ? null : _emptyFunc; + gsap.set(e, { + lazy: true, + userSelect: selectable ? "text" : "none" + }); + } + }, + _isFixed$1 = function _isFixed(element) { + if (_getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + return _isFixed(element); + } + }, + _supports3D, + _addPaddingBR, + ScrollProxy = function ScrollProxy(element, vars) { + element = gsap.utils.toArray(element)[0]; + vars = vars || {}; + var content = document.createElement("div"), + style = content.style, + node = element.firstChild, + offsetTop = 0, + offsetLeft = 0, + prevTop = element.scrollTop, + prevLeft = element.scrollLeft, + scrollWidth = element.scrollWidth, + scrollHeight = element.scrollHeight, + extraPadRight = 0, + maxLeft = 0, + maxTop = 0, + elementWidth, + elementHeight, + contentHeight, + nextNode, + transformStart, + transformEnd; + + if (_supports3D && vars.force3D !== false) { + transformStart = "translate3d("; + transformEnd = "px,0px)"; + } else if (_transformProp$1) { + transformStart = "translate("; + transformEnd = "px)"; + } + + this.scrollTop = function (value, force) { + if (!arguments.length) { + return -this.top(); + } + + this.top(-value, force); + }; + + this.scrollLeft = function (value, force) { + if (!arguments.length) { + return -this.left(); + } + + this.left(-value, force); + }; + + this.left = function (value, force) { + if (!arguments.length) { + return -(element.scrollLeft + offsetLeft); + } + + var dif = element.scrollLeft - prevLeft, + oldOffset = offsetLeft; + + if ((dif > 2 || dif < -2) && !force) { + prevLeft = element.scrollLeft; + gsap.killTweensOf(this, { + left: 1, + scrollLeft: 1 + }); + this.left(-prevLeft); + + if (vars.onKill) { + vars.onKill(); + } + + return; + } + + value = -value; + + if (value < 0) { + offsetLeft = value - 0.5 | 0; + value = 0; + } else if (value > maxLeft) { + offsetLeft = value - maxLeft | 0; + value = maxLeft; + } else { + offsetLeft = 0; + } + + if (offsetLeft || oldOffset) { + if (!this._skip) { + style[_transformProp$1] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + + if (offsetLeft + extraPadRight >= 0) { + style.paddingRight = offsetLeft + extraPadRight + "px"; + } + } + + element.scrollLeft = value | 0; + prevLeft = element.scrollLeft; + }; + + this.top = function (value, force) { + if (!arguments.length) { + return -(element.scrollTop + offsetTop); + } + + var dif = element.scrollTop - prevTop, + oldOffset = offsetTop; + + if ((dif > 2 || dif < -2) && !force) { + prevTop = element.scrollTop; + gsap.killTweensOf(this, { + top: 1, + scrollTop: 1 + }); + this.top(-prevTop); + + if (vars.onKill) { + vars.onKill(); + } + + return; + } + + value = -value; + + if (value < 0) { + offsetTop = value - 0.5 | 0; + value = 0; + } else if (value > maxTop) { + offsetTop = value - maxTop | 0; + value = maxTop; + } else { + offsetTop = 0; + } + + if (offsetTop || oldOffset) { + if (!this._skip) { + style[_transformProp$1] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + } + + element.scrollTop = value | 0; + prevTop = element.scrollTop; + }; + + this.maxScrollTop = function () { + return maxTop; + }; + + this.maxScrollLeft = function () { + return maxLeft; + }; + + this.disable = function () { + node = content.firstChild; + + while (node) { + nextNode = node.nextSibling; + element.appendChild(node); + node = nextNode; + } + + if (element === content.parentNode) { + element.removeChild(content); + } + }; + + this.enable = function () { + node = element.firstChild; + + if (node === content) { + return; + } + + while (node) { + nextNode = node.nextSibling; + content.appendChild(node); + node = nextNode; + } + + element.appendChild(content); + this.calibrate(); + }; + + this.calibrate = function (force) { + var widthMatches = element.clientWidth === elementWidth, + cs, + x, + y; + prevTop = element.scrollTop; + prevLeft = element.scrollLeft; + + if (widthMatches && element.clientHeight === elementHeight && content.offsetHeight === contentHeight && scrollWidth === element.scrollWidth && scrollHeight === element.scrollHeight && !force) { + return; + } + + if (offsetTop || offsetLeft) { + x = this.left(); + y = this.top(); + this.left(-element.scrollLeft); + this.top(-element.scrollTop); + } + + cs = _getComputedStyle(element); + + if (!widthMatches || force) { + style.display = "block"; + style.width = "auto"; + style.paddingRight = "0px"; + extraPadRight = Math.max(0, element.scrollWidth - element.clientWidth); + + if (extraPadRight) { + extraPadRight += parseFloat(cs.paddingLeft) + (_addPaddingBR ? parseFloat(cs.paddingRight) : 0); + } + } + + style.display = "inline-block"; + style.position = "relative"; + style.overflow = "visible"; + style.verticalAlign = "top"; + style.boxSizing = "content-box"; + style.width = "100%"; + style.paddingRight = extraPadRight + "px"; + + if (_addPaddingBR) { + style.paddingBottom = cs.paddingBottom; + } + + elementWidth = element.clientWidth; + elementHeight = element.clientHeight; + scrollWidth = element.scrollWidth; + scrollHeight = element.scrollHeight; + maxLeft = element.scrollWidth - elementWidth; + maxTop = element.scrollHeight - elementHeight; + contentHeight = content.offsetHeight; + style.display = "block"; + + if (x || y) { + this.left(x); + this.top(y); + } + }; + + this.content = content; + this.element = element; + this._skip = false; + this.enable(); + }, + _initCore = function _initCore(required) { + if (_windowExists() && document.body) { + var nav = window && window.navigator; + _win$1 = window; + _doc$1 = document; + _docElement$1 = _doc$1.documentElement; + _body$1 = _doc$1.body; + _tempDiv = _createElement("div"); + _supportsPointer = !!window.PointerEvent; + _placeholderDiv = _createElement("div"); + _placeholderDiv.style.cssText = "visibility:hidden;height:1px;top:-1px;pointer-events:none;position:relative;clear:both;cursor:grab"; + _defaultCursor = _placeholderDiv.style.cursor === "grab" ? "grab" : "move"; + _isAndroid = nav && nav.userAgent.toLowerCase().indexOf("android") !== -1; + _isTouchDevice = "ontouchstart" in _docElement$1 && "orientation" in _win$1 || nav && (nav.MaxTouchPoints > 0 || nav.msMaxTouchPoints > 0); + + _addPaddingBR = function () { + var div = _createElement("div"), + child = _createElement("div"), + childStyle = child.style, + parent = _body$1, + val; + + childStyle.display = "inline-block"; + childStyle.position = "relative"; + div.style.cssText = "width:90px;height:40px;padding:10px;overflow:auto;visibility:hidden"; + div.appendChild(child); + parent.appendChild(div); + val = child.offsetHeight + 18 > div.scrollHeight; + parent.removeChild(div); + return val; + }(); + + _touchEventLookup = function (types) { + var standard = types.split(","), + converted = ("onpointerdown" in _tempDiv ? "pointerdown,pointermove,pointerup,pointercancel" : "onmspointerdown" in _tempDiv ? "MSPointerDown,MSPointerMove,MSPointerUp,MSPointerCancel" : types).split(","), + obj = {}, + i = 4; + + while (--i > -1) { + obj[standard[i]] = converted[i]; + obj[converted[i]] = standard[i]; + } + + try { + _docElement$1.addEventListener("test", null, Object.defineProperty({}, "passive", { + get: function get() { + _supportsPassive = 1; + } + })); + } catch (e) {} + + return obj; + }("touchstart,touchmove,touchend,touchcancel"); + + _addListener(_doc$1, "touchcancel", _emptyFunc); + + _addListener(_win$1, "touchmove", _emptyFunc); + + _body$1 && _body$1.addEventListener("touchstart", _emptyFunc); + + _addListener(_doc$1, "contextmenu", function () { + for (var p in _lookup) { + if (_lookup[p].isPressed) { + _lookup[p].endDrag(); + } + } + }); + + gsap = _coreInitted = _getGSAP(); + } + + if (gsap) { + InertiaPlugin = gsap.plugins.inertia; + + _context = gsap.core.context || function () {}; + + _checkPrefix = gsap.utils.checkPrefix; + _transformProp$1 = _checkPrefix(_transformProp$1); + _transformOriginProp$1 = _checkPrefix(_transformOriginProp$1); + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + _supports3D = !!_checkPrefix("perspective"); + } else if (required) { + console.warn("Please gsap.registerPlugin(Draggable)"); + } + }; + + var EventDispatcher = function () { + function EventDispatcher(target) { + this._listeners = {}; + this.target = target || this; + } + + var _proto = EventDispatcher.prototype; + + _proto.addEventListener = function addEventListener(type, callback) { + var list = this._listeners[type] || (this._listeners[type] = []); + + if (!~list.indexOf(callback)) { + list.push(callback); + } + }; + + _proto.removeEventListener = function removeEventListener(type, callback) { + var list = this._listeners[type], + i = list && list.indexOf(callback); + i >= 0 && list.splice(i, 1); + }; + + _proto.dispatchEvent = function dispatchEvent(type) { + var _this = this; + + var result; + (this._listeners[type] || []).forEach(function (callback) { + return callback.call(_this, { + type: type, + target: _this.target + }) === false && (result = false); + }); + return result; + }; + + return EventDispatcher; + }(); + + var Draggable = function (_EventDispatcher) { + _inheritsLoose(Draggable, _EventDispatcher); + + function Draggable(target, vars) { + var _this2; + + _this2 = _EventDispatcher.call(this) || this; + _coreInitted || _initCore(1); + target = _toArray(target)[0]; + _this2.styles = _getStyleSaver && _getStyleSaver(target, "transform,left,top"); + + if (!InertiaPlugin) { + InertiaPlugin = gsap.plugins.inertia; + } + + _this2.vars = vars = _copy(vars || {}); + _this2.target = target; + _this2.x = _this2.y = _this2.rotation = 0; + _this2.dragResistance = parseFloat(vars.dragResistance) || 0; + _this2.edgeResistance = isNaN(vars.edgeResistance) ? 1 : parseFloat(vars.edgeResistance) || 0; + _this2.lockAxis = vars.lockAxis; + _this2.autoScroll = vars.autoScroll || 0; + _this2.lockedAxis = null; + _this2.allowEventDefault = !!vars.allowEventDefault; + gsap.getProperty(target, "x"); + + var type = (vars.type || "x,y").toLowerCase(), + xyMode = ~type.indexOf("x") || ~type.indexOf("y"), + rotationMode = type.indexOf("rotation") !== -1, + xProp = rotationMode ? "rotation" : xyMode ? "x" : "left", + yProp = xyMode ? "y" : "top", + allowX = !!(~type.indexOf("x") || ~type.indexOf("left") || type === "scroll"), + allowY = !!(~type.indexOf("y") || ~type.indexOf("top") || type === "scroll"), + minimumMovement = vars.minimumMovement || 2, + self = _assertThisInitialized(_this2), + triggers = _toArray(vars.trigger || vars.handle || target), + killProps = {}, + dragEndTime = 0, + checkAutoScrollBounds = false, + autoScrollMarginTop = vars.autoScrollMarginTop || 40, + autoScrollMarginRight = vars.autoScrollMarginRight || 40, + autoScrollMarginBottom = vars.autoScrollMarginBottom || 40, + autoScrollMarginLeft = vars.autoScrollMarginLeft || 40, + isClickable = vars.clickableTest || _isClickable, + clickTime = 0, + gsCache = target._gsap || gsap.core.getCache(target), + isFixed = _isFixed$1(target), + getPropAsNum = function getPropAsNum(property, unit) { + return parseFloat(gsCache.get(target, property, unit)); + }, + ownerDoc = target.ownerDocument || _doc$1, + enabled, + scrollProxy, + startPointerX, + startPointerY, + startElementX, + startElementY, + hasBounds, + hasDragCallback, + hasMoveCallback, + maxX, + minX, + maxY, + minY, + touch, + touchID, + rotationOrigin, + dirty, + old, + snapX, + snapY, + snapXY, + isClicking, + touchEventTarget, + matrix, + interrupted, + allowNativeTouchScrolling, + touchDragAxis, + isDispatching, + clickDispatch, + trustedClickDispatch, + isPreventingDefault, + innerMatrix, + dragged, + onContextMenu = function onContextMenu(e) { + _preventDefault(e); + + e.stopImmediatePropagation && e.stopImmediatePropagation(); + return false; + }, + render = function render(suppressEvents) { + if (self.autoScroll && self.isDragging && (checkAutoScrollBounds || dirty)) { + var e = target, + autoScrollFactor = self.autoScroll * 15, + parent, + isRoot, + rect, + pointerX, + pointerY, + changeX, + changeY, + gap; + checkAutoScrollBounds = false; + _windowProxy.scrollTop = _win$1.pageYOffset != null ? _win$1.pageYOffset : ownerDoc.documentElement.scrollTop != null ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop; + _windowProxy.scrollLeft = _win$1.pageXOffset != null ? _win$1.pageXOffset : ownerDoc.documentElement.scrollLeft != null ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft; + pointerX = self.pointerX - _windowProxy.scrollLeft; + pointerY = self.pointerY - _windowProxy.scrollTop; + + while (e && !isRoot) { + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + rect = isRoot ? { + bottom: Math.max(_docElement$1.clientHeight, _win$1.innerHeight || 0), + right: Math.max(_docElement$1.clientWidth, _win$1.innerWidth || 0), + left: 0, + top: 0 + } : parent.getBoundingClientRect(); + changeX = changeY = 0; + + if (allowY) { + gap = parent._gsMaxScrollY - parent.scrollTop; + + if (gap < 0) { + changeY = gap; + } else if (pointerY > rect.bottom - autoScrollMarginBottom && gap) { + checkAutoScrollBounds = true; + changeY = Math.min(gap, autoScrollFactor * (1 - Math.max(0, rect.bottom - pointerY) / autoScrollMarginBottom) | 0); + } else if (pointerY < rect.top + autoScrollMarginTop && parent.scrollTop) { + checkAutoScrollBounds = true; + changeY = -Math.min(parent.scrollTop, autoScrollFactor * (1 - Math.max(0, pointerY - rect.top) / autoScrollMarginTop) | 0); + } + + if (changeY) { + parent.scrollTop += changeY; + } + } + + if (allowX) { + gap = parent._gsMaxScrollX - parent.scrollLeft; + + if (gap < 0) { + changeX = gap; + } else if (pointerX > rect.right - autoScrollMarginRight && gap) { + checkAutoScrollBounds = true; + changeX = Math.min(gap, autoScrollFactor * (1 - Math.max(0, rect.right - pointerX) / autoScrollMarginRight) | 0); + } else if (pointerX < rect.left + autoScrollMarginLeft && parent.scrollLeft) { + checkAutoScrollBounds = true; + changeX = -Math.min(parent.scrollLeft, autoScrollFactor * (1 - Math.max(0, pointerX - rect.left) / autoScrollMarginLeft) | 0); + } + + if (changeX) { + parent.scrollLeft += changeX; + } + } + + if (isRoot && (changeX || changeY)) { + _win$1.scrollTo(parent.scrollLeft, parent.scrollTop); + + setPointerPosition(self.pointerX + changeX, self.pointerY + changeY); + } + + e = parent; + } + } + + if (dirty) { + var x = self.x, + y = self.y; + + if (rotationMode) { + self.deltaX = x - parseFloat(gsCache.rotation); + self.rotation = x; + gsCache.rotation = x + "deg"; + gsCache.renderTransform(1, gsCache); + } else { + if (scrollProxy) { + if (allowY) { + self.deltaY = y - scrollProxy.top(); + scrollProxy.top(y); + } + + if (allowX) { + self.deltaX = x - scrollProxy.left(); + scrollProxy.left(x); + } + } else if (xyMode) { + if (allowY) { + self.deltaY = y - parseFloat(gsCache.y); + gsCache.y = y + "px"; + } + + if (allowX) { + self.deltaX = x - parseFloat(gsCache.x); + gsCache.x = x + "px"; + } + + gsCache.renderTransform(1, gsCache); + } else { + if (allowY) { + self.deltaY = y - parseFloat(target.style.top || 0); + target.style.top = y + "px"; + } + + if (allowX) { + self.deltaX = x - parseFloat(target.style.left || 0); + target.style.left = x + "px"; + } + } + } + + if (hasDragCallback && !suppressEvents && !isDispatching) { + isDispatching = true; + + if (_dispatchEvent(self, "drag", "onDrag") === false) { + if (allowX) { + self.x -= self.deltaX; + } + + if (allowY) { + self.y -= self.deltaY; + } + + render(true); + } + + isDispatching = false; + } + } + + dirty = false; + }, + syncXY = function syncXY(skipOnUpdate, skipSnap) { + var x = self.x, + y = self.y, + snappedValue, + cs; + + if (!target._gsap) { + gsCache = gsap.core.getCache(target); + } + + gsCache.uncache && gsap.getProperty(target, "x"); + + if (xyMode) { + self.x = parseFloat(gsCache.x); + self.y = parseFloat(gsCache.y); + } else if (rotationMode) { + self.x = self.rotation = parseFloat(gsCache.rotation); + } else if (scrollProxy) { + self.y = scrollProxy.top(); + self.x = scrollProxy.left(); + } else { + self.y = parseFloat(target.style.top || (cs = _getComputedStyle(target)) && cs.top) || 0; + self.x = parseFloat(target.style.left || (cs || {}).left) || 0; + } + + if ((snapX || snapY || snapXY) && !skipSnap && (self.isDragging || self.isThrowing)) { + if (snapXY) { + _temp1.x = self.x; + _temp1.y = self.y; + snappedValue = snapXY(_temp1); + + if (snappedValue.x !== self.x) { + self.x = snappedValue.x; + dirty = true; + } + + if (snappedValue.y !== self.y) { + self.y = snappedValue.y; + dirty = true; + } + } + + if (snapX) { + snappedValue = snapX(self.x); + + if (snappedValue !== self.x) { + self.x = snappedValue; + + if (rotationMode) { + self.rotation = snappedValue; + } + + dirty = true; + } + } + + if (snapY) { + snappedValue = snapY(self.y); + + if (snappedValue !== self.y) { + self.y = snappedValue; + } + + dirty = true; + } + } + + dirty && render(true); + + if (!skipOnUpdate) { + self.deltaX = self.x - x; + self.deltaY = self.y - y; + + _dispatchEvent(self, "throwupdate", "onThrowUpdate"); + } + }, + buildSnapFunc = function buildSnapFunc(snap, min, max, factor) { + if (min == null) { + min = -_bigNum; + } + + if (max == null) { + max = _bigNum; + } + + if (_isFunction(snap)) { + return function (n) { + var edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance; + return snap.call(self, (n > max ? max + (n - max) * edgeTolerance : n < min ? min + (n - min) * edgeTolerance : n) * factor) * factor; + }; + } + + if (_isArray(snap)) { + return function (n) { + var i = snap.length, + closest = 0, + absDif = _bigNum, + val, + dif; + + while (--i > -1) { + val = snap[i]; + dif = val - n; + + if (dif < 0) { + dif = -dif; + } + + if (dif < absDif && val >= min && val <= max) { + closest = i; + absDif = dif; + } + } + + return snap[closest]; + }; + } + + return isNaN(snap) ? function (n) { + return n; + } : function () { + return snap * factor; + }; + }, + buildPointSnapFunc = function buildPointSnapFunc(snap, minX, maxX, minY, maxY, radius, factor) { + radius = radius && radius < _bigNum ? radius * radius : _bigNum; + + if (_isFunction(snap)) { + return function (point) { + var edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance, + x = point.x, + y = point.y, + result, + dx, + dy; + point.x = x = x > maxX ? maxX + (x - maxX) * edgeTolerance : x < minX ? minX + (x - minX) * edgeTolerance : x; + point.y = y = y > maxY ? maxY + (y - maxY) * edgeTolerance : y < minY ? minY + (y - minY) * edgeTolerance : y; + result = snap.call(self, point); + + if (result !== point) { + point.x = result.x; + point.y = result.y; + } + + if (factor !== 1) { + point.x *= factor; + point.y *= factor; + } + + if (radius < _bigNum) { + dx = point.x - x; + dy = point.y - y; + + if (dx * dx + dy * dy > radius) { + point.x = x; + point.y = y; + } + } + + return point; + }; + } + + if (_isArray(snap)) { + return function (p) { + var i = snap.length, + closest = 0, + minDist = _bigNum, + x, + y, + point, + dist; + + while (--i > -1) { + point = snap[i]; + x = point.x - p.x; + y = point.y - p.y; + dist = x * x + y * y; + + if (dist < minDist) { + closest = i; + minDist = dist; + } + } + + return minDist <= radius ? snap[closest] : p; + }; + } + + return function (n) { + return n; + }; + }, + calculateBounds = function calculateBounds() { + var bounds, targetBounds, snap, snapIsRaw; + hasBounds = false; + + if (scrollProxy) { + scrollProxy.calibrate(); + self.minX = minX = -scrollProxy.maxScrollLeft(); + self.minY = minY = -scrollProxy.maxScrollTop(); + self.maxX = maxX = self.maxY = maxY = 0; + hasBounds = true; + } else if (!!vars.bounds) { + bounds = _getBounds(vars.bounds, target.parentNode); + + if (rotationMode) { + self.minX = minX = bounds.left; + self.maxX = maxX = bounds.left + bounds.width; + self.minY = minY = self.maxY = maxY = 0; + } else if (!_isUndefined(vars.bounds.maxX) || !_isUndefined(vars.bounds.maxY)) { + bounds = vars.bounds; + self.minX = minX = bounds.minX; + self.minY = minY = bounds.minY; + self.maxX = maxX = bounds.maxX; + self.maxY = maxY = bounds.maxY; + } else { + targetBounds = _getBounds(target, target.parentNode); + self.minX = minX = Math.round(getPropAsNum(xProp, "px") + bounds.left - targetBounds.left); + self.minY = minY = Math.round(getPropAsNum(yProp, "px") + bounds.top - targetBounds.top); + self.maxX = maxX = Math.round(minX + (bounds.width - targetBounds.width)); + self.maxY = maxY = Math.round(minY + (bounds.height - targetBounds.height)); + } + + if (minX > maxX) { + self.minX = maxX; + self.maxX = maxX = minX; + minX = self.minX; + } + + if (minY > maxY) { + self.minY = maxY; + self.maxY = maxY = minY; + minY = self.minY; + } + + if (rotationMode) { + self.minRotation = minX; + self.maxRotation = maxX; + } + + hasBounds = true; + } + + if (vars.liveSnap) { + snap = vars.liveSnap === true ? vars.snap || {} : vars.liveSnap; + snapIsRaw = _isArray(snap) || _isFunction(snap); + + if (rotationMode) { + snapX = buildSnapFunc(snapIsRaw ? snap : snap.rotation, minX, maxX, 1); + snapY = null; + } else { + if (snap.points) { + snapXY = buildPointSnapFunc(snapIsRaw ? snap : snap.points, minX, maxX, minY, maxY, snap.radius, scrollProxy ? -1 : 1); + } else { + if (allowX) { + snapX = buildSnapFunc(snapIsRaw ? snap : snap.x || snap.left || snap.scrollLeft, minX, maxX, scrollProxy ? -1 : 1); + } + + if (allowY) { + snapY = buildSnapFunc(snapIsRaw ? snap : snap.y || snap.top || snap.scrollTop, minY, maxY, scrollProxy ? -1 : 1); + } + } + } + } + }, + onThrowComplete = function onThrowComplete() { + self.isThrowing = false; + + _dispatchEvent(self, "throwcomplete", "onThrowComplete"); + }, + onThrowInterrupt = function onThrowInterrupt() { + self.isThrowing = false; + }, + animate = function animate(inertia, forceZeroVelocity) { + var snap, snapIsRaw, tween, overshootTolerance; + + if (inertia && InertiaPlugin) { + if (inertia === true) { + snap = vars.snap || vars.liveSnap || {}; + snapIsRaw = _isArray(snap) || _isFunction(snap); + inertia = { + resistance: (vars.throwResistance || vars.resistance || 1000) / (rotationMode ? 10 : 1) + }; + + if (rotationMode) { + inertia.rotation = _parseInertia(self, snapIsRaw ? snap : snap.rotation, maxX, minX, 1, forceZeroVelocity); + } else { + if (allowX) { + inertia[xProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.x || snap.left, maxX, minX, scrollProxy ? -1 : 1, forceZeroVelocity || self.lockedAxis === "x"); + } + + if (allowY) { + inertia[yProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.y || snap.top, maxY, minY, scrollProxy ? -1 : 1, forceZeroVelocity || self.lockedAxis === "y"); + } + + if (snap.points || _isArray(snap) && _isObject(snap[0])) { + inertia.linkedProps = xProp + "," + yProp; + inertia.radius = snap.radius; + } + } + } + + self.isThrowing = true; + overshootTolerance = !isNaN(vars.overshootTolerance) ? vars.overshootTolerance : vars.edgeResistance === 1 ? 0 : 1 - self.edgeResistance + 0.2; + + if (!inertia.duration) { + inertia.duration = { + max: Math.max(vars.minDuration || 0, "maxDuration" in vars ? vars.maxDuration : 2), + min: !isNaN(vars.minDuration) ? vars.minDuration : overshootTolerance === 0 || _isObject(inertia) && inertia.resistance > 1000 ? 0 : 0.5, + overshoot: overshootTolerance + }; + } + + self.tween = tween = gsap.to(scrollProxy || target, { + inertia: inertia, + data: "_draggable", + inherit: false, + onComplete: onThrowComplete, + onInterrupt: onThrowInterrupt, + onUpdate: vars.fastMode ? _dispatchEvent : syncXY, + onUpdateParams: vars.fastMode ? [self, "onthrowupdate", "onThrowUpdate"] : snap && snap.radius ? [false, true] : [] + }); + + if (!vars.fastMode) { + if (scrollProxy) { + scrollProxy._skip = true; + } + + tween.render(1e9, true, true); + syncXY(true, true); + self.endX = self.x; + self.endY = self.y; + + if (rotationMode) { + self.endRotation = self.x; + } + + tween.play(0); + syncXY(true, true); + + if (scrollProxy) { + scrollProxy._skip = false; + } + } + } else if (hasBounds) { + self.applyBounds(); + } + }, + updateMatrix = function updateMatrix(shiftStart) { + var start = matrix, + p; + matrix = getGlobalMatrix(target.parentNode, true); + + if (shiftStart && self.isPressed && !matrix.equals(start || new Matrix2D())) { + p = start.inverse().apply({ + x: startPointerX, + y: startPointerY + }); + matrix.apply(p, p); + startPointerX = p.x; + startPointerY = p.y; + } + + if (matrix.equals(_identityMatrix$1)) { + matrix = null; + } + }, + recordStartPositions = function recordStartPositions() { + var edgeTolerance = 1 - self.edgeResistance, + offsetX = isFixed ? _getDocScrollLeft$1(ownerDoc) : 0, + offsetY = isFixed ? _getDocScrollTop$1(ownerDoc) : 0, + parsedOrigin, + x, + y; + + if (xyMode) { + gsCache.x = getPropAsNum(xProp, "px") + "px"; + gsCache.y = getPropAsNum(yProp, "px") + "px"; + gsCache.renderTransform(); + } + + updateMatrix(false); + _point1.x = self.pointerX - offsetX; + _point1.y = self.pointerY - offsetY; + matrix && matrix.apply(_point1, _point1); + startPointerX = _point1.x; + startPointerY = _point1.y; + + if (dirty) { + setPointerPosition(self.pointerX, self.pointerY); + render(true); + } + + innerMatrix = getGlobalMatrix(target); + + if (scrollProxy) { + calculateBounds(); + startElementY = scrollProxy.top(); + startElementX = scrollProxy.left(); + } else { + if (isTweening()) { + syncXY(true, true); + calculateBounds(); + } else { + self.applyBounds(); + } + + if (rotationMode) { + parsedOrigin = target.ownerSVGElement ? [gsCache.xOrigin - target.getBBox().x, gsCache.yOrigin - target.getBBox().y] : (_getComputedStyle(target)[_transformOriginProp$1] || "0 0").split(" "); + rotationOrigin = self.rotationOrigin = getGlobalMatrix(target).apply({ + x: parseFloat(parsedOrigin[0]) || 0, + y: parseFloat(parsedOrigin[1]) || 0 + }); + syncXY(true, true); + x = self.pointerX - rotationOrigin.x - offsetX; + y = rotationOrigin.y - self.pointerY + offsetY; + startElementX = self.x; + startElementY = self.y = Math.atan2(y, x) * _RAD2DEG; + } else { + startElementY = getPropAsNum(yProp, "px"); + startElementX = getPropAsNum(xProp, "px"); + } + } + + if (hasBounds && edgeTolerance) { + if (startElementX > maxX) { + startElementX = maxX + (startElementX - maxX) / edgeTolerance; + } else if (startElementX < minX) { + startElementX = minX - (minX - startElementX) / edgeTolerance; + } + + if (!rotationMode) { + if (startElementY > maxY) { + startElementY = maxY + (startElementY - maxY) / edgeTolerance; + } else if (startElementY < minY) { + startElementY = minY - (minY - startElementY) / edgeTolerance; + } + } + } + + self.startX = startElementX = _round(startElementX); + self.startY = startElementY = _round(startElementY); + }, + isTweening = function isTweening() { + return self.tween && self.tween.isActive(); + }, + removePlaceholder = function removePlaceholder() { + if (_placeholderDiv.parentNode && !isTweening() && !self.isDragging) { + _placeholderDiv.parentNode.removeChild(_placeholderDiv); + } + }, + onPress = function onPress(e, force) { + var i; + + if (!enabled || self.isPressed || !e || (e.type === "mousedown" || e.type === "pointerdown") && !force && _getTime() - clickTime < 30 && _touchEventLookup[self.pointerEvent.type]) { + isPreventingDefault && e && enabled && _preventDefault(e); + return; + } + + interrupted = isTweening(); + dragged = false; + self.pointerEvent = e; + + if (_touchEventLookup[e.type]) { + touchEventTarget = ~e.type.indexOf("touch") ? e.currentTarget || e.target : ownerDoc; + + _addListener(touchEventTarget, "touchend", onRelease); + + _addListener(touchEventTarget, "touchmove", onMove); + + _addListener(touchEventTarget, "touchcancel", onRelease); + + _addListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + touchEventTarget = null; + + _addListener(ownerDoc, "mousemove", onMove); + } + + touchDragAxis = null; + + if (!_supportsPointer || !touchEventTarget) { + _addListener(ownerDoc, "mouseup", onRelease); + + e && e.target && _addListener(e.target, "mouseup", onRelease); + } + + isClicking = isClickable.call(self, e.target) && vars.dragClickables === false && !force; + + if (isClicking) { + _addListener(e.target, "change", onRelease); + + _dispatchEvent(self, "pressInit", "onPressInit"); + + _dispatchEvent(self, "press", "onPress"); + + _setSelectable(triggers, true); + + isPreventingDefault = false; + return; + } + + allowNativeTouchScrolling = !touchEventTarget || allowX === allowY || self.vars.allowNativeTouchScrolling === false || self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2) ? false : allowX ? "y" : "x"; + isPreventingDefault = !allowNativeTouchScrolling && !self.allowEventDefault; + + if (isPreventingDefault) { + _preventDefault(e); + + _addListener(_win$1, "touchforcechange", _preventDefault); + } + + if (e.changedTouches) { + e = touch = e.changedTouches[0]; + touchID = e.identifier; + } else if (e.pointerId) { + touchID = e.pointerId; + } else { + touch = touchID = null; + } + + _dragCount++; + + _addToRenderQueue(render); + + startPointerY = self.pointerY = e.pageY; + startPointerX = self.pointerX = e.pageX; + + _dispatchEvent(self, "pressInit", "onPressInit"); + + if (allowNativeTouchScrolling || self.autoScroll) { + _recordMaxScrolls(target.parentNode); + } + + if (target.parentNode && self.autoScroll && !scrollProxy && !rotationMode && target.parentNode._gsMaxScrollX && !_placeholderDiv.parentNode && !target.getBBox) { + _placeholderDiv.style.width = target.parentNode.scrollWidth + "px"; + target.parentNode.appendChild(_placeholderDiv); + } + + recordStartPositions(); + self.tween && self.tween.kill(); + self.isThrowing = false; + gsap.killTweensOf(scrollProxy || target, killProps, true); + scrollProxy && gsap.killTweensOf(target, { + scrollTo: 1 + }, true); + self.tween = self.lockedAxis = null; + + if (vars.zIndexBoost || !rotationMode && !scrollProxy && vars.zIndexBoost !== false) { + target.style.zIndex = Draggable.zIndex++; + } + + self.isPressed = true; + hasDragCallback = !!(vars.onDrag || self._listeners.drag); + hasMoveCallback = !!(vars.onMove || self._listeners.move); + + if (vars.cursor !== false || vars.activeCursor) { + i = triggers.length; + + while (--i > -1) { + gsap.set(triggers[i], { + cursor: vars.activeCursor || vars.cursor || (_defaultCursor === "grab" ? "grabbing" : _defaultCursor) + }); + } + } + + _dispatchEvent(self, "press", "onPress"); + }, + onMove = function onMove(e) { + var originalEvent = e, + touches, + pointerX, + pointerY, + i, + dx, + dy; + + if (!enabled || _isMultiTouching || !self.isPressed || !e) { + isPreventingDefault && e && enabled && _preventDefault(e); + return; + } + + self.pointerEvent = e; + touches = e.changedTouches; + + if (touches) { + e = touches[0]; + + if (e !== touch && e.identifier !== touchID) { + i = touches.length; + + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} + + if (i < 0) { + return; + } + } + } else if (e.pointerId && touchID && e.pointerId !== touchID) { + return; + } + + if (touchEventTarget && allowNativeTouchScrolling && !touchDragAxis) { + _point1.x = e.pageX - (isFixed ? _getDocScrollLeft$1(ownerDoc) : 0); + _point1.y = e.pageY - (isFixed ? _getDocScrollTop$1(ownerDoc) : 0); + matrix && matrix.apply(_point1, _point1); + pointerX = _point1.x; + pointerY = _point1.y; + dx = Math.abs(pointerX - startPointerX); + dy = Math.abs(pointerY - startPointerY); + + if (dx !== dy && (dx > minimumMovement || dy > minimumMovement) || _isAndroid && allowNativeTouchScrolling === touchDragAxis) { + touchDragAxis = dx > dy && allowX ? "x" : "y"; + + if (allowNativeTouchScrolling && touchDragAxis !== allowNativeTouchScrolling) { + _addListener(_win$1, "touchforcechange", _preventDefault); + } + + if (self.vars.lockAxisOnTouchScroll !== false && allowX && allowY) { + self.lockedAxis = touchDragAxis === "x" ? "y" : "x"; + _isFunction(self.vars.onLockAxis) && self.vars.onLockAxis.call(self, originalEvent); + } + + if (_isAndroid && allowNativeTouchScrolling === touchDragAxis) { + onRelease(originalEvent); + return; + } + } + } + + if (!self.allowEventDefault && (!allowNativeTouchScrolling || touchDragAxis && allowNativeTouchScrolling !== touchDragAxis) && originalEvent.cancelable !== false) { + _preventDefault(originalEvent); + + isPreventingDefault = true; + } else if (isPreventingDefault) { + isPreventingDefault = false; + } + + if (self.autoScroll) { + checkAutoScrollBounds = true; + } + + setPointerPosition(e.pageX, e.pageY, hasMoveCallback); + }, + setPointerPosition = function setPointerPosition(pointerX, pointerY, invokeOnMove) { + var dragTolerance = 1 - self.dragResistance, + edgeTolerance = 1 - self.edgeResistance, + prevPointerX = self.pointerX, + prevPointerY = self.pointerY, + prevStartElementY = startElementY, + prevX = self.x, + prevY = self.y, + prevEndX = self.endX, + prevEndY = self.endY, + prevEndRotation = self.endRotation, + prevDirty = dirty, + xChange, + yChange, + x, + y, + dif, + temp; + self.pointerX = pointerX; + self.pointerY = pointerY; + + if (isFixed) { + pointerX -= _getDocScrollLeft$1(ownerDoc); + pointerY -= _getDocScrollTop$1(ownerDoc); + } + + if (rotationMode) { + y = Math.atan2(rotationOrigin.y - pointerY, pointerX - rotationOrigin.x) * _RAD2DEG; + dif = self.y - y; + + if (dif > 180) { + startElementY -= 360; + self.y = y; + } else if (dif < -180) { + startElementY += 360; + self.y = y; + } + + if (self.x !== startElementX || Math.max(Math.abs(startPointerX - pointerX), Math.abs(startPointerY - pointerY)) > minimumMovement) { + self.y = y; + x = startElementX + (startElementY - y) * dragTolerance; + } else { + x = startElementX; + } + } else { + if (matrix) { + temp = pointerX * matrix.a + pointerY * matrix.c + matrix.e; + pointerY = pointerX * matrix.b + pointerY * matrix.d + matrix.f; + pointerX = temp; + } + + yChange = pointerY - startPointerY; + xChange = pointerX - startPointerX; + + if (yChange < minimumMovement && yChange > -minimumMovement) { + yChange = 0; + } + + if (xChange < minimumMovement && xChange > -minimumMovement) { + xChange = 0; + } + + if ((self.lockAxis || self.lockedAxis) && (xChange || yChange)) { + temp = self.lockedAxis; + + if (!temp) { + self.lockedAxis = temp = allowX && Math.abs(xChange) > Math.abs(yChange) ? "y" : allowY ? "x" : null; + + if (temp && _isFunction(self.vars.onLockAxis)) { + self.vars.onLockAxis.call(self, self.pointerEvent); + } + } + + if (temp === "y") { + yChange = 0; + } else if (temp === "x") { + xChange = 0; + } + } + + x = _round(startElementX + xChange * dragTolerance); + y = _round(startElementY + yChange * dragTolerance); + } + + if ((snapX || snapY || snapXY) && (self.x !== x || self.y !== y && !rotationMode)) { + if (snapXY) { + _temp1.x = x; + _temp1.y = y; + temp = snapXY(_temp1); + x = _round(temp.x); + y = _round(temp.y); + } + + if (snapX) { + x = _round(snapX(x)); + } + + if (snapY) { + y = _round(snapY(y)); + } + } + + if (hasBounds) { + if (x > maxX) { + x = maxX + Math.round((x - maxX) * edgeTolerance); + } else if (x < minX) { + x = minX + Math.round((x - minX) * edgeTolerance); + } + + if (!rotationMode) { + if (y > maxY) { + y = Math.round(maxY + (y - maxY) * edgeTolerance); + } else if (y < minY) { + y = Math.round(minY + (y - minY) * edgeTolerance); + } + } + } + + if (self.x !== x || self.y !== y && !rotationMode) { + if (rotationMode) { + self.endRotation = self.x = self.endX = x; + dirty = true; + } else { + if (allowY) { + self.y = self.endY = y; + dirty = true; + } + + if (allowX) { + self.x = self.endX = x; + dirty = true; + } + } + + if (!invokeOnMove || _dispatchEvent(self, "move", "onMove") !== false) { + if (!self.isDragging && self.isPressed) { + self.isDragging = dragged = true; + + _dispatchEvent(self, "dragstart", "onDragStart"); + } + } else { + self.pointerX = prevPointerX; + self.pointerY = prevPointerY; + startElementY = prevStartElementY; + self.x = prevX; + self.y = prevY; + self.endX = prevEndX; + self.endY = prevEndY; + self.endRotation = prevEndRotation; + dirty = prevDirty; + } + } + }, + onRelease = function onRelease(e, force) { + if (!enabled || !self.isPressed || e && touchID != null && !force && (e.pointerId && e.pointerId !== touchID && e.target !== target || e.changedTouches && !_hasTouchID(e.changedTouches, touchID))) { + isPreventingDefault && e && enabled && _preventDefault(e); + return; + } + + self.isPressed = false; + var originalEvent = e, + wasDragging = self.isDragging, + isContextMenuRelease = self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2), + placeholderDelayedCall = gsap.delayedCall(0.001, removePlaceholder), + touches, + i, + syntheticEvent, + eventTarget, + syntheticClick; + + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchend", onRelease); + + _removeListener(touchEventTarget, "touchmove", onMove); + + _removeListener(touchEventTarget, "touchcancel", onRelease); + + _removeListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + _removeListener(ownerDoc, "mousemove", onMove); + } + + _removeListener(_win$1, "touchforcechange", _preventDefault); + + if (!_supportsPointer || !touchEventTarget) { + _removeListener(ownerDoc, "mouseup", onRelease); + + e && e.target && _removeListener(e.target, "mouseup", onRelease); + } + + dirty = false; + + if (wasDragging) { + dragEndTime = _lastDragTime = _getTime(); + self.isDragging = false; + } + + _removeFromRenderQueue(render); + + if (isClicking && !isContextMenuRelease) { + if (e) { + _removeListener(e.target, "change", onRelease); + + self.pointerEvent = originalEvent; + } + + _setSelectable(triggers, false); + + _dispatchEvent(self, "release", "onRelease"); + + _dispatchEvent(self, "click", "onClick"); + + isClicking = false; + return; + } + + i = triggers.length; + + while (--i > -1) { + _setStyle(triggers[i], "cursor", vars.cursor || (vars.cursor !== false ? _defaultCursor : null)); + } + + _dragCount--; + + if (e) { + touches = e.changedTouches; + + if (touches) { + e = touches[0]; + + if (e !== touch && e.identifier !== touchID) { + i = touches.length; + + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} + + if (i < 0 && !force) { + return; + } + } + } + + self.pointerEvent = originalEvent; + self.pointerX = e.pageX; + self.pointerY = e.pageY; + } + + if (isContextMenuRelease && originalEvent) { + _preventDefault(originalEvent); + + isPreventingDefault = true; + + _dispatchEvent(self, "release", "onRelease"); + } else if (originalEvent && !wasDragging) { + isPreventingDefault = false; + + if (interrupted && (vars.snap || vars.bounds)) { + animate(vars.inertia || vars.throwProps); + } + + _dispatchEvent(self, "release", "onRelease"); + + if ((!_isAndroid || originalEvent.type !== "touchmove") && originalEvent.type.indexOf("cancel") === -1) { + _dispatchEvent(self, "click", "onClick"); + + if (_getTime() - clickTime < 300) { + _dispatchEvent(self, "doubleclick", "onDoubleClick"); + } + + eventTarget = originalEvent.target || target; + clickTime = _getTime(); + + syntheticClick = function syntheticClick() { + if (clickTime !== clickDispatch && self.enabled() && !self.isPressed && !originalEvent.defaultPrevented) { + if (eventTarget.click) { + eventTarget.click(); + } else if (ownerDoc.createEvent) { + syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win$1, 1, self.pointerEvent.screenX, self.pointerEvent.screenY, self.pointerX, self.pointerY, false, false, false, false, 0, null); + eventTarget.dispatchEvent(syntheticEvent); + } + } + }; + + if (!_isAndroid && !originalEvent.defaultPrevented) { + gsap.delayedCall(0.05, syntheticClick); + } + } + } else { + animate(vars.inertia || vars.throwProps); + + if (!self.allowEventDefault && originalEvent && (vars.dragClickables !== false || !isClickable.call(self, originalEvent.target)) && wasDragging && (!allowNativeTouchScrolling || touchDragAxis && allowNativeTouchScrolling === touchDragAxis) && originalEvent.cancelable !== false) { + isPreventingDefault = true; + + _preventDefault(originalEvent); + } else { + isPreventingDefault = false; + } + + _dispatchEvent(self, "release", "onRelease"); + } + + isTweening() && placeholderDelayedCall.duration(self.tween.duration()); + wasDragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return true; + }, + updateScroll = function updateScroll(e) { + if (e && self.isDragging && !scrollProxy) { + var parent = e.target || target.parentNode, + deltaX = parent.scrollLeft - parent._gsScrollX, + deltaY = parent.scrollTop - parent._gsScrollY; + + if (deltaX || deltaY) { + if (matrix) { + startPointerX -= deltaX * matrix.a + deltaY * matrix.c; + startPointerY -= deltaY * matrix.d + deltaX * matrix.b; + } else { + startPointerX -= deltaX; + startPointerY -= deltaY; + } + + parent._gsScrollX += deltaX; + parent._gsScrollY += deltaY; + setPointerPosition(self.pointerX, self.pointerY); + } + } + }, + onClick = function onClick(e) { + var time = _getTime(), + recentlyClicked = time - clickTime < 100, + recentlyDragged = time - dragEndTime < 50, + alreadyDispatched = recentlyClicked && clickDispatch === clickTime, + defaultPrevented = self.pointerEvent && self.pointerEvent.defaultPrevented, + alreadyDispatchedTrusted = recentlyClicked && trustedClickDispatch === clickTime, + trusted = e.isTrusted || e.isTrusted == null && recentlyClicked && alreadyDispatched; + + if ((alreadyDispatched || recentlyDragged && self.vars.suppressClickOnDrag !== false) && e.stopImmediatePropagation) { + e.stopImmediatePropagation(); + } + + if (recentlyClicked && !(self.pointerEvent && self.pointerEvent.defaultPrevented) && (!alreadyDispatched || trusted && !alreadyDispatchedTrusted)) { + if (trusted && alreadyDispatched) { + trustedClickDispatch = clickTime; + } + + clickDispatch = clickTime; + return; + } + + if (self.isPressed || recentlyDragged || recentlyClicked) { + if (!trusted || !e.detail || !recentlyClicked || defaultPrevented) { + _preventDefault(e); + } + } + + if (!recentlyClicked && !recentlyDragged && !dragged) { + e && e.target && (self.pointerEvent = e); + + _dispatchEvent(self, "click", "onClick"); + } + }, + localizePoint = function localizePoint(p) { + return matrix ? { + x: p.x * matrix.a + p.y * matrix.c + matrix.e, + y: p.x * matrix.b + p.y * matrix.d + matrix.f + } : { + x: p.x, + y: p.y + }; + }; + + old = Draggable.get(target); + old && old.kill(); + + _this2.startDrag = function (event, align) { + var r1, r2, p1, p2; + onPress(event || self.pointerEvent, true); + + if (align && !self.hitTest(event || self.pointerEvent)) { + r1 = _parseRect(event || self.pointerEvent); + r2 = _parseRect(target); + p1 = localizePoint({ + x: r1.left + r1.width / 2, + y: r1.top + r1.height / 2 + }); + p2 = localizePoint({ + x: r2.left + r2.width / 2, + y: r2.top + r2.height / 2 + }); + startPointerX -= p1.x - p2.x; + startPointerY -= p1.y - p2.y; + } + + if (!self.isDragging) { + self.isDragging = dragged = true; + + _dispatchEvent(self, "dragstart", "onDragStart"); + } + }; + + _this2.drag = onMove; + + _this2.endDrag = function (e) { + return onRelease(e || self.pointerEvent, true); + }; + + _this2.timeSinceDrag = function () { + return self.isDragging ? 0 : (_getTime() - dragEndTime) / 1000; + }; + + _this2.timeSinceClick = function () { + return (_getTime() - clickTime) / 1000; + }; + + _this2.hitTest = function (target, threshold) { + return Draggable.hitTest(self.target, target, threshold); + }; + + _this2.getDirection = function (from, diagonalThreshold) { + var mode = from === "velocity" && InertiaPlugin ? from : _isObject(from) && !rotationMode ? "element" : "start", + xChange, + yChange, + ratio, + direction, + r1, + r2; + + if (mode === "element") { + r1 = _parseRect(self.target); + r2 = _parseRect(from); + } + + xChange = mode === "start" ? self.x - startElementX : mode === "velocity" ? InertiaPlugin.getVelocity(target, xProp) : r1.left + r1.width / 2 - (r2.left + r2.width / 2); + + if (rotationMode) { + return xChange < 0 ? "counter-clockwise" : "clockwise"; + } else { + diagonalThreshold = diagonalThreshold || 2; + yChange = mode === "start" ? self.y - startElementY : mode === "velocity" ? InertiaPlugin.getVelocity(target, yProp) : r1.top + r1.height / 2 - (r2.top + r2.height / 2); + ratio = Math.abs(xChange / yChange); + direction = ratio < 1 / diagonalThreshold ? "" : xChange < 0 ? "left" : "right"; + + if (ratio < diagonalThreshold) { + if (direction !== "") { + direction += "-"; + } + + direction += yChange < 0 ? "up" : "down"; + } + } + + return direction; + }; + + _this2.applyBounds = function (newBounds, sticky) { + var x, y, forceZeroVelocity, e, parent, isRoot; + + if (newBounds && vars.bounds !== newBounds) { + vars.bounds = newBounds; + return self.update(true, sticky); + } + + syncXY(true); + calculateBounds(); + + if (hasBounds && !isTweening()) { + x = self.x; + y = self.y; + + if (x > maxX) { + x = maxX; + } else if (x < minX) { + x = minX; + } + + if (y > maxY) { + y = maxY; + } else if (y < minY) { + y = minY; + } + + if (self.x !== x || self.y !== y) { + forceZeroVelocity = true; + self.x = self.endX = x; + + if (rotationMode) { + self.endRotation = x; + } else { + self.y = self.endY = y; + } + + dirty = true; + render(true); + + if (self.autoScroll && !self.isDragging) { + _recordMaxScrolls(target.parentNode); + + e = target; + _windowProxy.scrollTop = _win$1.pageYOffset != null ? _win$1.pageYOffset : ownerDoc.documentElement.scrollTop != null ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop; + _windowProxy.scrollLeft = _win$1.pageXOffset != null ? _win$1.pageXOffset : ownerDoc.documentElement.scrollLeft != null ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft; + + while (e && !isRoot) { + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + + if (allowY && parent.scrollTop > parent._gsMaxScrollY) { + parent.scrollTop = parent._gsMaxScrollY; + } + + if (allowX && parent.scrollLeft > parent._gsMaxScrollX) { + parent.scrollLeft = parent._gsMaxScrollX; + } + + e = parent; + } + } + } + + if (self.isThrowing && (forceZeroVelocity || self.endX > maxX || self.endX < minX || self.endY > maxY || self.endY < minY)) { + animate(vars.inertia || vars.throwProps, forceZeroVelocity); + } + } + + return self; + }; + + _this2.update = function (applyBounds, sticky, ignoreExternalChanges) { + if (sticky && self.isPressed) { + var m = getGlobalMatrix(target), + p = innerMatrix.apply({ + x: self.x - startElementX, + y: self.y - startElementY + }), + m2 = getGlobalMatrix(target.parentNode, true); + m2.apply({ + x: m.e - p.x, + y: m.f - p.y + }, p); + self.x -= p.x - m2.e; + self.y -= p.y - m2.f; + render(true); + recordStartPositions(); + } + + var x = self.x, + y = self.y; + updateMatrix(!sticky); + + if (applyBounds) { + self.applyBounds(); + } else { + dirty && ignoreExternalChanges && render(true); + syncXY(true); + } + + if (sticky) { + setPointerPosition(self.pointerX, self.pointerY); + dirty && render(true); + } + + if (self.isPressed && !sticky && (allowX && Math.abs(x - self.x) > 0.01 || allowY && Math.abs(y - self.y) > 0.01 && !rotationMode)) { + recordStartPositions(); + } + + if (self.autoScroll) { + _recordMaxScrolls(target.parentNode, self.isDragging); + + checkAutoScrollBounds = self.isDragging; + render(true); + + _removeScrollListener(target, updateScroll); + + _addScrollListener(target, updateScroll); + } + + return self; + }; + + _this2.enable = function (type) { + var setVars = { + lazy: true + }, + id, + i, + trigger; + + if (vars.cursor !== false) { + setVars.cursor = vars.cursor || _defaultCursor; + } + + if (gsap.utils.checkPrefix("touchCallout")) { + setVars.touchCallout = "none"; + } + + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, allowX === allowY ? "none" : vars.allowNativeTouchScrolling && target.scrollHeight === target.clientHeight === (target.scrollWidth === target.clientHeight) || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x"); + + i = triggers.length; + + while (--i > -1) { + trigger = triggers[i]; + _supportsPointer || _addListener(trigger, "mousedown", onPress); + + _addListener(trigger, "touchstart", onPress); + + _addListener(trigger, "click", onClick, true); + + gsap.set(trigger, setVars); + + if (trigger.getBBox && trigger.ownerSVGElement && allowX !== allowY) { + gsap.set(trigger.ownerSVGElement, { + touchAction: vars.allowNativeTouchScrolling || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x" + }); + } + + vars.allowContextMenu || _addListener(trigger, "contextmenu", onContextMenu); + } + + _setSelectable(triggers, false); + } + + _addScrollListener(target, updateScroll); + + enabled = true; + + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.track(scrollProxy || target, xyMode ? "x,y" : rotationMode ? "rotation" : "top,left"); + } + + target._gsDragID = id = target._gsDragID || "d" + _lookupCount++; + _lookup[id] = self; + + if (scrollProxy) { + scrollProxy.enable(); + scrollProxy.element._gsDragID = id; + } + + (vars.bounds || rotationMode) && recordStartPositions(); + vars.bounds && self.applyBounds(); + return self; + }; + + _this2.disable = function (type) { + var dragging = self.isDragging, + i = triggers.length, + trigger; + + while (--i > -1) { + _setStyle(triggers[i], "cursor", null); + } + + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, null); + + i = triggers.length; + + while (--i > -1) { + trigger = triggers[i]; + + _setStyle(trigger, "touchCallout", null); + + _removeListener(trigger, "mousedown", onPress); + + _removeListener(trigger, "touchstart", onPress); + + _removeListener(trigger, "click", onClick, true); + + _removeListener(trigger, "contextmenu", onContextMenu); + } + + _setSelectable(triggers, true); + + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchcancel", onRelease); + + _removeListener(touchEventTarget, "touchend", onRelease); + + _removeListener(touchEventTarget, "touchmove", onMove); + } + + _removeListener(ownerDoc, "mouseup", onRelease); + + _removeListener(ownerDoc, "mousemove", onMove); + } + + _removeScrollListener(target, updateScroll); + + enabled = false; + + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.untrack(scrollProxy || target, xyMode ? "x,y" : rotationMode ? "rotation" : "top,left"); + self.tween && self.tween.kill(); + } + + scrollProxy && scrollProxy.disable(); + + _removeFromRenderQueue(render); + + self.isDragging = self.isPressed = isClicking = false; + dragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return self; + }; + + _this2.enabled = function (value, type) { + return arguments.length ? value ? self.enable(type) : self.disable(type) : enabled; + }; + + _this2.kill = function () { + self.isThrowing = false; + self.tween && self.tween.kill(); + self.disable(); + gsap.set(triggers, { + clearProps: "userSelect" + }); + delete _lookup[target._gsDragID]; + return self; + }; + + _this2.revert = function () { + this.kill(); + this.styles && this.styles.revert(); + }; + + if (~type.indexOf("scroll")) { + scrollProxy = _this2.scrollProxy = new ScrollProxy(target, _extend({ + onKill: function onKill() { + self.isPressed && onRelease(null); + } + }, vars)); + target.style.overflowY = allowY && !_isTouchDevice ? "auto" : "hidden"; + target.style.overflowX = allowX && !_isTouchDevice ? "auto" : "hidden"; + target = scrollProxy.content; + } + + if (rotationMode) { + killProps.rotation = 1; + } else { + if (allowX) { + killProps[xProp] = 1; + } + + if (allowY) { + killProps[yProp] = 1; + } + } + + gsCache.force3D = "force3D" in vars ? vars.force3D : true; + + _context(_assertThisInitialized(_this2)); + + _this2.enable(); + + return _this2; + } + + Draggable.register = function register(core) { + gsap = core; + + _initCore(); + }; + + Draggable.create = function create(targets, vars) { + _coreInitted || _initCore(true); + return _toArray(targets).map(function (target) { + return new Draggable(target, vars); + }); + }; + + Draggable.get = function get(target) { + return _lookup[(_toArray(target)[0] || {})._gsDragID]; + }; + + Draggable.timeSinceDrag = function timeSinceDrag() { + return (_getTime() - _lastDragTime) / 1000; + }; + + Draggable.hitTest = function hitTest(obj1, obj2, threshold) { + if (obj1 === obj2) { + return false; + } + + var r1 = _parseRect(obj1), + r2 = _parseRect(obj2), + top = r1.top, + left = r1.left, + right = r1.right, + bottom = r1.bottom, + width = r1.width, + height = r1.height, + isOutside = r2.left > right || r2.right < left || r2.top > bottom || r2.bottom < top, + overlap, + area, + isRatio; + + if (isOutside || !threshold) { + return !isOutside; + } + + isRatio = (threshold + "").indexOf("%") !== -1; + threshold = parseFloat(threshold) || 0; + overlap = { + left: Math.max(left, r2.left), + top: Math.max(top, r2.top) + }; + overlap.width = Math.min(right, r2.right) - overlap.left; + overlap.height = Math.min(bottom, r2.bottom) - overlap.top; + + if (overlap.width < 0 || overlap.height < 0) { + return false; + } + + if (isRatio) { + threshold *= 0.01; + area = overlap.width * overlap.height; + return area >= width * height * threshold || area >= r2.width * r2.height * threshold; + } + + return overlap.width > threshold && overlap.height > threshold; + }; + + return Draggable; + }(EventDispatcher); + + _setDefaults(Draggable.prototype, { + pointerX: 0, + pointerY: 0, + startX: 0, + startY: 0, + deltaX: 0, + deltaY: 0, + isDragging: false, + isPressed: false + }); + + Draggable.zIndex = 1000; + Draggable.version = "3.12.7"; + _getGSAP() && gsap.registerPlugin(Draggable); + + exports.Draggable = Draggable; + exports.default = Draggable; + + if (typeof(window) === 'undefined' || window !== exports) {Object.defineProperty(exports, '__esModule', { value: true });} else {delete window.default;} + +}))); diff --git a/node_modules/gsap/dist/Draggable.min.js b/node_modules/gsap/dist/Draggable.min.js new file mode 100644 index 0000000000000000000000000000000000000000..d4e66b9cda50169a2ca9e3be231c7d5bac350bfd --- /dev/null +++ b/node_modules/gsap/dist/Draggable.min.js @@ -0,0 +1,11 @@ +/*! + * Draggable 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(e){"use strict";function _assertThisInitialized(e){if(void 0===e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return e}function w(e,t){if(e.parentNode&&(h||T(e))){var n=P(e),o=n?n.getAttribute("xmlns")||"http://www.w3.org/2000/svg":"http://www.w3.org/1999/xhtml",r=n?t?"rect":"g":"div",i=2!==t?0:100,a=3===t?100:0,l="position:absolute;display:block;pointer-events:none;margin:0;padding:0;",s=h.createElementNS?h.createElementNS(o.replace(/^https/,"http"),r):h.createElement(r);return t&&(n?(g=g||w(e),s.setAttribute("width",.01),s.setAttribute("height",.01),s.setAttribute("transform","translate("+i+","+a+")"),g.appendChild(s)):(f||((f=w(e)).style.cssText=l),s.style.cssText=l+"width:0.1px;height:0.1px;top:"+a+"px;left:"+i+"px",f.appendChild(s))),s}throw"Need document and parent."}function A(e,t,n,o,r,i,a){return e.a=t,e.b=n,e.c=o,e.d=r,e.e=i,e.f=a,e}var h,u,i,a,f,g,x,m,y,t,v="transform",b=v+"Origin",T=function _setDoc(e){var t=e.ownerDocument||e;!(v in e.style)&&"msTransform"in e.style&&(b=(v="msTransform")+"Origin");for(;t.parentNode&&(t=t.parentNode););if(u=window,x=new ge,t){i=(h=t).documentElement,a=t.body,(m=h.createElementNS("http://www.w3.org/2000/svg","g")).style.transform="none";var n=t.createElement("div"),o=t.createElement("div"),r=t&&(t.body||t.firstElementChild);r&&r.appendChild&&(r.appendChild(n),n.appendChild(o),n.setAttribute("style","position:static;transform:translate3d(0,0,1px)"),y=o.offsetParent!==n,r.removeChild(n))}return t},D=function _forceNonZeroScale(e){for(var t,n;e&&e!==a;)(n=e._gsap)&&n.uncache&&n.get(e,"x"),n&&!n.scaleX&&!n.scaleY&&n.renderTransform&&(n.scaleX=n.scaleY=1e-4,n.renderTransform(1,n),t?t.push(n):t=[n]),e=e.parentNode;return t},M=[],E=[],L=function _getDocScrollTop(){return u.pageYOffset||h.scrollTop||i.scrollTop||a.scrollTop||0},S=function _getDocScrollLeft(){return u.pageXOffset||h.scrollLeft||i.scrollLeft||a.scrollLeft||0},P=function _svgOwner(e){return e.ownerSVGElement||("svg"===(e.tagName+"").toLowerCase()?e:null)},C=function _isFixed(e){return"fixed"===u.getComputedStyle(e).position||((e=e.parentNode)&&1===e.nodeType?_isFixed(e):void 0)},N=function _placeSiblings(e,t){var n,o,r,i,a,l,s=P(e),c=e===s,d=s?M:E,p=e.parentNode;if(e===u)return e;if(d.length||d.push(w(e,1),w(e,2),w(e,3)),n=s?g:f,s)c?(i=-(r=function _getCTM(e){var t,n=e.getCTM();return n||(t=e.style[v],e.style[v]="none",e.appendChild(m),n=m.getCTM(),e.removeChild(m),t?e.style[v]=t:e.style.removeProperty(v.replace(/([A-Z])/g,"-$1").toLowerCase())),n||x.clone()}(e)).e/r.a,a=-r.f/r.d,o=x):e.getBBox?(r=e.getBBox(),i=(o=(o=e.transform?e.transform.baseVal:{}).numberOfItems?1<o.numberOfItems?function _consolidate(e){for(var t=new ge,n=0;n<e.numberOfItems;n++)t.multiply(e.getItem(n).matrix);return t}(o):o.getItem(0).matrix:x).a*r.x+o.c*r.y,a=o.b*r.x+o.d*r.y):(o=new ge,i=a=0),t&&"g"===e.tagName.toLowerCase()&&(i=a=0),(c?s:p).appendChild(n),n.setAttribute("transform","matrix("+o.a+","+o.b+","+o.c+","+o.d+","+(o.e+i)+","+(o.f+a)+")");else{if(i=a=0,y)for(o=e.offsetParent,r=e;(r=r&&r.parentNode)&&r!==o&&r.parentNode;)4<(u.getComputedStyle(r)[v]+"").length&&(i=r.offsetLeft,a=r.offsetTop,r=0);if("absolute"!==(l=u.getComputedStyle(e)).position&&"fixed"!==l.position)for(o=e.offsetParent;p&&p!==o;)i+=p.scrollLeft||0,a+=p.scrollTop||0,p=p.parentNode;(r=n.style).top=e.offsetTop-a+"px",r.left=e.offsetLeft-i+"px",r[v]=l[v],r[b]=l[b],r.position="fixed"===l.position?"fixed":"absolute",e.parentNode.appendChild(n)}return n},ge=((t=Matrix2D.prototype).inverse=function inverse(){var e=this.a,t=this.b,n=this.c,o=this.d,r=this.e,i=this.f,a=e*o-t*n||1e-10;return A(this,o/a,-t/a,-n/a,e/a,(n*i-o*r)/a,-(e*i-t*r)/a)},t.multiply=function multiply(e){var t=this.a,n=this.b,o=this.c,r=this.d,i=this.e,a=this.f,l=e.a,s=e.c,c=e.b,d=e.d,p=e.e,u=e.f;return A(this,l*t+c*o,l*n+c*r,s*t+d*o,s*n+d*r,i+p*t+u*o,a+p*n+u*r)},t.clone=function clone(){return new Matrix2D(this.a,this.b,this.c,this.d,this.e,this.f)},t.equals=function equals(e){var t=this.a,n=this.b,o=this.c,r=this.d,i=this.e,a=this.f;return t===e.a&&n===e.b&&o===e.c&&r===e.d&&i===e.e&&a===e.f},t.apply=function apply(e,t){void 0===t&&(t={});var n=e.x,o=e.y,r=this.a,i=this.b,a=this.c,l=this.d,s=this.e,c=this.f;return t.x=n*r+o*a+s||0,t.y=n*i+o*l+c||0,t},Matrix2D);function Matrix2D(e,t,n,o,r,i){void 0===e&&(e=1),void 0===t&&(t=0),void 0===n&&(n=0),void 0===o&&(o=1),void 0===r&&(r=0),void 0===i&&(i=0),A(this,e,t,n,o,r,i)}function getGlobalMatrix(e,t,n,o){if(!e||!e.parentNode||(h||T(e)).documentElement===e)return new ge;var r=D(e),i=P(e)?M:E,a=N(e,n),l=i[0].getBoundingClientRect(),s=i[1].getBoundingClientRect(),c=i[2].getBoundingClientRect(),d=a.parentNode,p=!o&&C(e),u=new ge((s.left-l.left)/100,(s.top-l.top)/100,(c.left-l.left)/100,(c.top-l.top)/100,l.left+(p?0:S()),l.top+(p?0:L()));if(d.removeChild(a),r)for(l=r.length;l--;)(s=r[l]).scaleX=s.scaleY=0,s.renderTransform(1,s);return t?u.inverse():u}function X(){return"undefined"!=typeof window}function Y(){return xe||X()&&(xe=window.gsap)&&xe.registerPlugin&&xe}function Z(e){return"function"==typeof e}function $(e){return"object"==typeof e}function _(e){return void 0===e}function aa(){return!1}function da(e){return Math.round(1e4*e)/1e4}function fa(e,t){var n=ye.createElementNS?ye.createElementNS((t||"http://www.w3.org/1999/xhtml").replace(/^https/,"http"),e):ye.createElement(e);return n.style?n:ye.createElement(e)}function ra(e,t){var n,o={};for(n in e)o[n]=t?e[n]*t:e[n];return o}function ta(e,t){for(var n,o=e.length;o--;)t?e[o].style.touchAction=t:e[o].style.removeProperty("touch-action"),(n=e[o].children)&&n.length&&ta(n,t)}function ua(){return Oe.forEach(function(e){return e()})}function wa(){return!Oe.length&&xe.ticker.remove(ua)}function xa(e){for(var t=Oe.length;t--;)Oe[t]===e&&Oe.splice(t,1);xe.to(wa,{overwrite:!0,delay:15,duration:0,onComplete:wa,data:"_draggable"})}function za(e,t,n,o){if(e.addEventListener){var r=Me[t];o=o||(d?{passive:!1}:null),e.addEventListener(r||t,n,o),r&&t!==r&&e.addEventListener(t,n,o)}}function Aa(e,t,n,o){if(e.removeEventListener){var r=Me[t];e.removeEventListener(r||t,n,o),r&&t!==r&&e.removeEventListener(t,n,o)}}function Ba(e){e.preventDefault&&e.preventDefault(),e.preventManipulation&&e.preventManipulation()}function Da(e){Ee=e.touches&&Ae<e.touches.length,Aa(e.target,"touchend",Da)}function Ea(e){Ee=e.touches&&Ae<e.touches.length,za(e.target,"touchend",Da)}function Fa(e){return me.pageYOffset||e.scrollTop||e.documentElement.scrollTop||e.body.scrollTop||0}function Ga(e){return me.pageXOffset||e.scrollLeft||e.documentElement.scrollLeft||e.body.scrollLeft||0}function Ha(e,t){za(e,"scroll",t),Qe(e.parentNode)||Ha(e.parentNode,t)}function Ia(e,t){Aa(e,"scroll",t),Qe(e.parentNode)||Ia(e.parentNode,t)}function Ka(e,t){var n="x"===t?"Width":"Height",o="scroll"+n,r="client"+n;return Math.max(0,Qe(e)?Math.max(ve[o],l[o])-(me["inner"+n]||ve[r]||l[r]):e[o]-e[r])}function La(e,t){var n=Ka(e,"x"),o=Ka(e,"y");Qe(e)?e=We:La(e.parentNode,t),e._gsMaxScrollX=n,e._gsMaxScrollY=o,t||(e._gsScrollX=e.scrollLeft||0,e._gsScrollY=e.scrollTop||0)}function Ma(e,t,n){var o=e.style;o&&(_(o[t])&&(t=c(t,e)||t),null==n?o.removeProperty&&o.removeProperty(t.replace(/([A-Z])/g,"-$1").toLowerCase()):o[t]=n)}function Na(e){return me.getComputedStyle(e instanceof Element?e:e.host||(e.parentNode||{}).host||e)}function Pa(e){if(e===me)return p.left=p.top=0,p.width=p.right=ve.clientWidth||e.innerWidth||l.clientWidth||0,p.height=p.bottom=(e.innerHeight||0)-20<ve.clientHeight?ve.clientHeight:e.innerHeight||l.clientHeight||0,p;var t=e.ownerDocument||ye,n=_(e.pageX)?e.nodeType||_(e.left)||_(e.top)?Te(e)[0].getBoundingClientRect():e:{left:e.pageX-Ga(t),top:e.pageY-Fa(t),right:e.pageX-Ga(t)+1,bottom:e.pageY-Fa(t)+1};return _(n.right)&&!_(n.width)?(n.right=n.left+n.width,n.bottom=n.top+n.height):_(n.width)&&(n={width:n.right-n.left,height:n.bottom-n.top,right:n.right,left:n.left,bottom:n.bottom,top:n.top}),n}function Qa(e,t,n){var o,r=e.vars,i=r[n],a=e._listeners[t];return Z(i)&&(o=i.apply(r.callbackScope||e,r[n+"Params"]||[e.pointerEvent])),a&&!1===e.dispatchEvent(t)&&(o=!1),o}function Ra(e,t){var n,o,r,i=Te(e)[0];return i.nodeType||i===me?O(i,t):_(e.left)?{left:o=e.min||e.minX||e.minRotation||0,top:n=e.min||e.minY||0,width:(e.max||e.maxX||e.maxRotation||0)-o,height:(e.max||e.maxY||0)-n}:(r={x:0,y:0},{left:e.left-r.x,top:e.top-r.y,width:e.width,height:e.height})}function Ua(r,i,e,t,a,n){var o,l,s,c={};if(i)if(1!==a&&i instanceof Array){if(c.end=o=[],s=i.length,$(i[0]))for(l=0;l<s;l++)o[l]=ra(i[l],a);else for(l=0;l<s;l++)o[l]=i[l]*a;e+=1.1,t-=1.1}else Z(i)?c.end=function(e){var t,n,o=i.call(r,e);if(1!==a)if($(o)){for(n in t={},o)t[n]=o[n]*a;o=t}else o*=a;return o}:c.end=i;return!e&&0!==e||(c.max=e),!t&&0!==t||(c.min=t),n&&(c.velocity=0),c}function Va(e){var t;return!(!e||!e.getAttribute||e===l)&&(!("true"!==(t=e.getAttribute("data-clickable"))&&("false"===t||!o.test(e.nodeName+"")&&"true"!==e.getAttribute("contentEditable")))||Va(e.parentNode))}function Wa(e,t){for(var n,o=e.length;o--;)(n=e[o]).ondragstart=n.onselectstart=t?null:aa,xe.set(n,{lazy:!0,userSelect:t?"text":"none"})}function $a(i,r){i=xe.utils.toArray(i)[0],r=r||{};var a,l,s,e,c,d,p=document.createElement("div"),u=p.style,t=i.firstChild,h=0,f=0,g=i.scrollTop,x=i.scrollLeft,m=i.scrollWidth,y=i.scrollHeight,v=0,w=0,b=0;k&&!1!==r.force3D?(c="translate3d(",d="px,0px)"):B&&(c="translate(",d="px)"),this.scrollTop=function(e,t){if(!arguments.length)return-this.top();this.top(-e,t)},this.scrollLeft=function(e,t){if(!arguments.length)return-this.left();this.left(-e,t)},this.left=function(e,t){if(!arguments.length)return-(i.scrollLeft+f);var n=i.scrollLeft-x,o=f;if((2<n||n<-2)&&!t)return x=i.scrollLeft,xe.killTweensOf(this,{left:1,scrollLeft:1}),this.left(-x),void(r.onKill&&r.onKill());(e=-e)<0?(f=e-.5|0,e=0):w<e?(f=e-w|0,e=w):f=0,(f||o)&&(this._skip||(u[B]=c+-f+"px,"+-h+d),0<=f+v&&(u.paddingRight=f+v+"px")),i.scrollLeft=0|e,x=i.scrollLeft},this.top=function(e,t){if(!arguments.length)return-(i.scrollTop+h);var n=i.scrollTop-g,o=h;if((2<n||n<-2)&&!t)return g=i.scrollTop,xe.killTweensOf(this,{top:1,scrollTop:1}),this.top(-g),void(r.onKill&&r.onKill());(e=-e)<0?(h=e-.5|0,e=0):b<e?(h=e-b|0,e=b):h=0,(h||o)&&(this._skip||(u[B]=c+-f+"px,"+-h+d)),i.scrollTop=0|e,g=i.scrollTop},this.maxScrollTop=function(){return b},this.maxScrollLeft=function(){return w},this.disable=function(){for(t=p.firstChild;t;)e=t.nextSibling,i.appendChild(t),t=e;i===p.parentNode&&i.removeChild(p)},this.enable=function(){if((t=i.firstChild)!==p){for(;t;)e=t.nextSibling,p.appendChild(t),t=e;i.appendChild(p),this.calibrate()}},this.calibrate=function(e){var t,n,o,r=i.clientWidth===a;g=i.scrollTop,x=i.scrollLeft,r&&i.clientHeight===l&&p.offsetHeight===s&&m===i.scrollWidth&&y===i.scrollHeight&&!e||((h||f)&&(n=this.left(),o=this.top(),this.left(-i.scrollLeft),this.top(-i.scrollTop)),t=Na(i),r&&!e||(u.display="block",u.width="auto",u.paddingRight="0px",(v=Math.max(0,i.scrollWidth-i.clientWidth))&&(v+=parseFloat(t.paddingLeft)+(R?parseFloat(t.paddingRight):0))),u.display="inline-block",u.position="relative",u.overflow="visible",u.verticalAlign="top",u.boxSizing="content-box",u.width="100%",u.paddingRight=v+"px",R&&(u.paddingBottom=t.paddingBottom),a=i.clientWidth,l=i.clientHeight,m=i.scrollWidth,y=i.scrollHeight,w=i.scrollWidth-a,b=i.scrollHeight-l,s=p.offsetHeight,u.display="block",(n||o)&&(this.left(n),this.top(o)))},this.content=p,this.element=i,this._skip=!1,this.enable()}function _a(e){if(X()&&document.body){var t=window&&window.navigator;me=window,ye=document,ve=ye.documentElement,l=ye.body,s=fa("div"),Pe=!!window.PointerEvent,(we=fa("div")).style.cssText="visibility:hidden;height:1px;top:-1px;pointer-events:none;position:relative;clear:both;cursor:grab",Se="grab"===we.style.cursor?"grab":"move",_e=t&&-1!==t.userAgent.toLowerCase().indexOf("android"),De="ontouchstart"in ve&&"orientation"in me||t&&(0<t.MaxTouchPoints||0<t.msMaxTouchPoints),o=fa("div"),r=fa("div"),i=r.style,a=l,i.display="inline-block",i.position="relative",o.style.cssText="width:90px;height:40px;padding:10px;overflow:auto;visibility:hidden",o.appendChild(r),a.appendChild(o),n=r.offsetHeight+18>o.scrollHeight,a.removeChild(o),R=n,Me=function(e){for(var t=e.split(","),n=(("onpointerdown"in s?"pointerdown,pointermove,pointerup,pointercancel":"onmspointerdown"in s?"MSPointerDown,MSPointerMove,MSPointerUp,MSPointerCancel":e).split(",")),o={},r=4;-1<--r;)o[t[r]]=n[r],o[n[r]]=t[r];try{ve.addEventListener("test",null,Object.defineProperty({},"passive",{get:function get(){d=1}}))}catch(e){}return o}("touchstart,touchmove,touchend,touchcancel"),za(ye,"touchcancel",aa),za(me,"touchmove",aa),l&&l.addEventListener("touchstart",aa),za(ye,"contextmenu",function(){for(var e in ze)ze[e].isPressed&&ze[e].endDrag()}),xe=be=Y()}var n,o,r,i,a;xe?(Le=xe.plugins.inertia,Xe=xe.core.context||function(){},c=xe.utils.checkPrefix,B=c(B),Ce=c(Ce),Te=xe.utils.toArray,Ye=xe.core.getStyleSaver,k=!!c("perspective")):e&&console.warn("Please gsap.registerPlugin(Draggable)")}var xe,me,ye,ve,l,s,we,be,c,Te,d,De,Me,Ee,_e,Le,Se,Pe,Xe,Ye,k,R,n,Ae=0,B="transform",Ce="transformOrigin",Ne=Array.isArray,ke=180/Math.PI,Re=1e20,r=new ge,Be=Date.now||function(){return(new Date).getTime()},Oe=[],ze={},Ie=0,o=/^(?:a|input|textarea|button|select)$/i,Fe=0,He={},We={},Qe=function _isRoot(e){return!(e&&e!==ve&&9!==e.nodeType&&e!==ye.body&&e!==me&&e.nodeType&&e.parentNode)},p={},Ge={},O=function _getElementBounds(e,t){t=Te(t)[0];var n,o,r,i,a,l,s,c,d,p,u,h,f,g=e.getBBox&&e.ownerSVGElement,x=e.ownerDocument||ye;if(e===me)r=Fa(x),o=(n=Ga(x))+(x.documentElement.clientWidth||e.innerWidth||x.body.clientWidth||0),i=r+((e.innerHeight||0)-20<x.documentElement.clientHeight?x.documentElement.clientHeight:e.innerHeight||x.body.clientHeight||0);else{if(t===me||_(t))return e.getBoundingClientRect();n=r=0,g?(u=(p=e.getBBox()).width,h=p.height):(e.viewBox&&(p=e.viewBox.baseVal)&&(n=p.x||0,r=p.y||0,u=p.width,h=p.height),u||(p="border-box"===(f=Na(e)).boxSizing,u=(parseFloat(f.width)||e.clientWidth||0)+(p?0:parseFloat(f.borderLeftWidth)+parseFloat(f.borderRightWidth)),h=(parseFloat(f.height)||e.clientHeight||0)+(p?0:parseFloat(f.borderTopWidth)+parseFloat(f.borderBottomWidth)))),o=u,i=h}return e===t?{left:n,top:r,width:o-n,height:i-r}:(l=(a=getGlobalMatrix(t,!0).multiply(getGlobalMatrix(e))).apply({x:n,y:r}),s=a.apply({x:o,y:r}),c=a.apply({x:o,y:i}),d=a.apply({x:n,y:i}),{left:n=Math.min(l.x,s.x,c.x,d.x),top:r=Math.min(l.y,s.y,c.y,d.y),width:Math.max(l.x,s.x,c.x,d.x)-n,height:Math.max(l.y,s.y,c.y,d.y)-r})},z=((n=EventDispatcher.prototype).addEventListener=function addEventListener(e,t){var n=this._listeners[e]||(this._listeners[e]=[]);~n.indexOf(t)||n.push(t)},n.removeEventListener=function removeEventListener(e,t){var n=this._listeners[e],o=n&&n.indexOf(t);0<=o&&n.splice(o,1)},n.dispatchEvent=function dispatchEvent(t){var n,o=this;return(this._listeners[t]||[]).forEach(function(e){return!1===e.call(o,{type:t,target:o.target})&&(n=!1)}),n},EventDispatcher);function EventDispatcher(e){this._listeners={},this.target=e||this}var Ke,I=(function _inheritsLoose(e,t){e.prototype=Object.create(t.prototype),(e.prototype.constructor=e).__proto__=t}(Draggable,Ke=z),Draggable.register=function register(e){xe=e,_a()},Draggable.create=function create(e,t){return be||_a(!0),Te(e).map(function(e){return new Draggable(e,t)})},Draggable.get=function get(e){return ze[(Te(e)[0]||{})._gsDragID]},Draggable.timeSinceDrag=function timeSinceDrag(){return(Be()-Fe)/1e3},Draggable.hitTest=function hitTest(e,t,n){if(e===t)return!1;var o,r,i,a=Pa(e),l=Pa(t),s=a.top,c=a.left,d=a.right,p=a.bottom,u=a.width,h=a.height,f=l.left>d||l.right<c||l.top>p||l.bottom<s;return f||!n?!f:(i=-1!==(n+"").indexOf("%"),n=parseFloat(n)||0,(o={left:Math.max(c,l.left),top:Math.max(s,l.top)}).width=Math.min(d,l.right)-o.left,o.height=Math.min(p,l.bottom)-o.top,!(o.width<0||o.height<0)&&(i?u*h*(n*=.01)<=(r=o.width*o.height)||r>=l.width*l.height*n:o.width>n&&o.height>n))},Draggable);function Draggable(h,p){var e;e=Ke.call(this)||this,be||_a(1),h=Te(h)[0],e.styles=Ye&&Ye(h,"transform,left,top"),Le=Le||xe.plugins.inertia,e.vars=p=ra(p||{}),e.target=h,e.x=e.y=e.rotation=0,e.dragResistance=parseFloat(p.dragResistance)||0,e.edgeResistance=isNaN(p.edgeResistance)?1:parseFloat(p.edgeResistance)||0,e.lockAxis=p.lockAxis,e.autoScroll=p.autoScroll||0,e.lockedAxis=null,e.allowEventDefault=!!p.allowEventDefault,xe.getProperty(h,"x");function Rg(e,t){return parseFloat(se.get(h,e,t))}function yh(e){return Ba(e),e.stopImmediatePropagation&&e.stopImmediatePropagation(),!1}function zh(e){if(q.autoScroll&&q.isDragging&&(te||Y)){var t,n,o,r,i,a,l,s,c=h,d=15*q.autoScroll;for(te=!1,We.scrollTop=null!=me.pageYOffset?me.pageYOffset:null!=de.documentElement.scrollTop?de.documentElement.scrollTop:de.body.scrollTop,We.scrollLeft=null!=me.pageXOffset?me.pageXOffset:null!=de.documentElement.scrollLeft?de.documentElement.scrollLeft:de.body.scrollLeft,r=q.pointerX-We.scrollLeft,i=q.pointerY-We.scrollTop;c&&!n;)t=(n=Qe(c.parentNode))?We:c.parentNode,o=n?{bottom:Math.max(ve.clientHeight,me.innerHeight||0),right:Math.max(ve.clientWidth,me.innerWidth||0),left:0,top:0}:t.getBoundingClientRect(),a=l=0,U&&((s=t._gsMaxScrollY-t.scrollTop)<0?l=s:i>o.bottom-re&&s?(te=!0,l=Math.min(s,d*(1-Math.max(0,o.bottom-i)/re)|0)):i<o.top+ne&&t.scrollTop&&(te=!0,l=-Math.min(t.scrollTop,d*(1-Math.max(0,i-o.top)/ne)|0)),l&&(t.scrollTop+=l)),V&&((s=t._gsMaxScrollX-t.scrollLeft)<0?a=s:r>o.right-oe&&s?(te=!0,a=Math.min(s,d*(1-Math.max(0,o.right-r)/oe)|0)):r<o.left+ie&&t.scrollLeft&&(te=!0,a=-Math.min(t.scrollLeft,d*(1-Math.max(0,r-o.left)/ie)|0)),a&&(t.scrollLeft+=a)),n&&(a||l)&&(me.scrollTo(t.scrollLeft,t.scrollTop),he(q.pointerX+a,q.pointerY+l)),c=t}if(Y){var p=q.x,u=q.y;Q?(q.deltaX=p-parseFloat(se.rotation),q.rotation=p,se.rotation=p+"deg",se.renderTransform(1,se)):f?(U&&(q.deltaY=u-f.top(),f.top(u)),V&&(q.deltaX=p-f.left(),f.left(p))):W?(U&&(q.deltaY=u-parseFloat(se.y),se.y=u+"px"),V&&(q.deltaX=p-parseFloat(se.x),se.x=p+"px"),se.renderTransform(1,se)):(U&&(q.deltaY=u-parseFloat(h.style.top||0),h.style.top=u+"px"),V&&(q.deltaX=p-parseFloat(h.style.left||0),h.style.left=p+"px")),!g||e||z||(!(z=!0)===Qa(q,"drag","onDrag")&&(V&&(q.x-=q.deltaX),U&&(q.y-=q.deltaY),zh(!0)),z=!1)}Y=!1}function Ah(e,t){var n,o,r=q.x,i=q.y;h._gsap||(se=xe.core.getCache(h)),se.uncache&&xe.getProperty(h,"x"),W?(q.x=parseFloat(se.x),q.y=parseFloat(se.y)):Q?q.x=q.rotation=parseFloat(se.rotation):f?(q.y=f.top(),q.x=f.left()):(q.y=parseFloat(h.style.top||(o=Na(h))&&o.top)||0,q.x=parseFloat(h.style.left||(o||{}).left)||0),(A||C||N)&&!t&&(q.isDragging||q.isThrowing)&&(N&&(He.x=q.x,He.y=q.y,(n=N(He)).x!==q.x&&(q.x=n.x,Y=!0),n.y!==q.y&&(q.y=n.y,Y=!0)),A&&(n=A(q.x))!==q.x&&(q.x=n,Q&&(q.rotation=n),Y=!0),C&&((n=C(q.y))!==q.y&&(q.y=n),Y=!0)),Y&&zh(!0),e||(q.deltaX=q.x-r,q.deltaY=q.y-i,Qa(q,"throwupdate","onThrowUpdate"))}function Bh(a,l,s,n){return null==l&&(l=-Re),null==s&&(s=Re),Z(a)?function(e){var t=q.isPressed?1-q.edgeResistance:1;return a.call(q,(s<e?s+(e-s)*t:e<l?l+(e-l)*t:e)*n)*n}:Ne(a)?function(e){for(var t,n,o=a.length,r=0,i=Re;-1<--o;)(n=(t=a[o])-e)<0&&(n=-n),n<i&&l<=t&&t<=s&&(r=o,i=n);return a[r]}:isNaN(a)?function(e){return e}:function(){return a*n}}function Dh(){var e,t,n,o;M=!1,f?(f.calibrate(),q.minX=L=-f.maxScrollLeft(),q.minY=P=-f.maxScrollTop(),q.maxX=E=q.maxY=S=0,M=!0):p.bounds&&(e=Ra(p.bounds,h.parentNode),Q?(q.minX=L=e.left,q.maxX=E=e.left+e.width,q.minY=P=q.maxY=S=0):_(p.bounds.maxX)&&_(p.bounds.maxY)?(t=Ra(h,h.parentNode),q.minX=L=Math.round(Rg(G,"px")+e.left-t.left),q.minY=P=Math.round(Rg(K,"px")+e.top-t.top),q.maxX=E=Math.round(L+(e.width-t.width)),q.maxY=S=Math.round(P+(e.height-t.height))):(e=p.bounds,q.minX=L=e.minX,q.minY=P=e.minY,q.maxX=E=e.maxX,q.maxY=S=e.maxY),E<L&&(q.minX=E,q.maxX=E=L,L=q.minX),S<P&&(q.minY=S,q.maxY=S=P,P=q.minY),Q&&(q.minRotation=L,q.maxRotation=E),M=!0),p.liveSnap&&(n=!0===p.liveSnap?p.snap||{}:p.liveSnap,o=Ne(n)||Z(n),Q?(A=Bh(o?n:n.rotation,L,E,1),C=null):n.points?N=function buildPointSnapFunc(s,l,c,d,p,u,h){return u=u&&u<Re?u*u:Re,Z(s)?function(e){var t,n,o,r=q.isPressed?1-q.edgeResistance:1,i=e.x,a=e.y;return e.x=i=c<i?c+(i-c)*r:i<l?l+(i-l)*r:i,e.y=a=p<a?p+(a-p)*r:a<d?d+(a-d)*r:a,(t=s.call(q,e))!==e&&(e.x=t.x,e.y=t.y),1!==h&&(e.x*=h,e.y*=h),u<Re&&(n=e.x-i,o=e.y-a,u<n*n+o*o&&(e.x=i,e.y=a)),e}:Ne(s)?function(e){for(var t,n,o,r,i=s.length,a=0,l=Re;-1<--i;)(r=(t=(o=s[i]).x-e.x)*t+(n=o.y-e.y)*n)<l&&(a=i,l=r);return l<=u?s[a]:e}:function(e){return e}}(o?n:n.points,L,E,P,S,n.radius,f?-1:1):(V&&(A=Bh(o?n:n.x||n.left||n.scrollLeft,L,E,f?-1:1)),U&&(C=Bh(o?n:n.y||n.top||n.scrollTop,P,S,f?-1:1))))}function Eh(){q.isThrowing=!1,Qa(q,"throwcomplete","onThrowComplete")}function Fh(){q.isThrowing=!1}function Gh(e,t){var n,o,r,i;e&&Le?(!0===e&&(n=p.snap||p.liveSnap||{},o=Ne(n)||Z(n),e={resistance:(p.throwResistance||p.resistance||1e3)/(Q?10:1)},Q?e.rotation=Ua(q,o?n:n.rotation,E,L,1,t):(V&&(e[G]=Ua(q,o?n:n.points||n.x||n.left,E,L,f?-1:1,t||"x"===q.lockedAxis)),U&&(e[K]=Ua(q,o?n:n.points||n.y||n.top,S,P,f?-1:1,t||"y"===q.lockedAxis)),(n.points||Ne(n)&&$(n[0]))&&(e.linkedProps=G+","+K,e.radius=n.radius))),q.isThrowing=!0,i=isNaN(p.overshootTolerance)?1===p.edgeResistance?0:1-q.edgeResistance+.2:p.overshootTolerance,e.duration||(e.duration={max:Math.max(p.minDuration||0,"maxDuration"in p?p.maxDuration:2),min:isNaN(p.minDuration)?0===i||$(e)&&1e3<e.resistance?0:.5:p.minDuration,overshoot:i}),q.tween=r=xe.to(f||h,{inertia:e,data:"_draggable",inherit:!1,onComplete:Eh,onInterrupt:Fh,onUpdate:p.fastMode?Qa:Ah,onUpdateParams:p.fastMode?[q,"onthrowupdate","onThrowUpdate"]:n&&n.radius?[!1,!0]:[]}),p.fastMode||(f&&(f._skip=!0),r.render(1e9,!0,!0),Ah(!0,!0),q.endX=q.x,q.endY=q.y,Q&&(q.endRotation=q.x),r.play(0),Ah(!0,!0),f&&(f._skip=!1))):M&&q.applyBounds()}function Hh(e){var t,n=k;k=getGlobalMatrix(h.parentNode,!0),e&&q.isPressed&&!k.equals(n||new ge)&&(t=n.inverse().apply({x:w,y:b}),k.apply(t,t),w=t.x,b=t.y),k.equals(r)&&(k=null)}function Ih(){var e,t,n,o=1-q.edgeResistance,r=ce?Ga(de):0,i=ce?Fa(de):0;W&&(se.x=Rg(G,"px")+"px",se.y=Rg(K,"px")+"px",se.renderTransform()),Hh(!1),Ge.x=q.pointerX-r,Ge.y=q.pointerY-i,k&&k.apply(Ge,Ge),w=Ge.x,b=Ge.y,Y&&(he(q.pointerX,q.pointerY),zh(!0)),d=getGlobalMatrix(h),f?(Dh(),D=f.top(),T=f.left()):(pe()?(Ah(!0,!0),Dh()):q.applyBounds(),Q?(e=h.ownerSVGElement?[se.xOrigin-h.getBBox().x,se.yOrigin-h.getBBox().y]:(Na(h)[Ce]||"0 0").split(" "),X=q.rotationOrigin=getGlobalMatrix(h).apply({x:parseFloat(e[0])||0,y:parseFloat(e[1])||0}),Ah(!0,!0),t=q.pointerX-X.x-r,n=X.y-q.pointerY+i,T=q.x,D=q.y=Math.atan2(n,t)*ke):(D=Rg(K,"px"),T=Rg(G,"px"))),M&&o&&(E<T?T=E+(T-E)/o:T<L&&(T=L-(L-T)/o),Q||(S<D?D=S+(D-S)/o:D<P&&(D=P-(P-D)/o))),q.startX=T=da(T),q.startY=D=da(D)}function Kh(){!we.parentNode||pe()||q.isDragging||we.parentNode.removeChild(we)}function Lh(e,t){var n;if(!u||q.isPressed||!e||!("mousedown"!==e.type&&"pointerdown"!==e.type||t)&&Be()-le<30&&Me[q.pointerEvent.type])F&&e&&u&&Ba(e);else{if(R=pe(),H=!1,q.pointerEvent=e,Me[e.type]?(v=~e.type.indexOf("touch")?e.currentTarget||e.target:de,za(v,"touchend",fe),za(v,"touchmove",ue),za(v,"touchcancel",fe),za(de,"touchstart",Ea)):(v=null,za(de,"mousemove",ue)),O=null,Pe&&v||(za(de,"mouseup",fe),e&&e.target&&za(e.target,"mouseup",fe)),y=ae.call(q,e.target)&&!1===p.dragClickables&&!t)return za(e.target,"change",fe),Qa(q,"pressInit","onPressInit"),Qa(q,"press","onPress"),Wa(J,!0),void(F=!1);if(B=!(!v||V==U||!1===q.vars.allowNativeTouchScrolling||q.vars.allowContextMenu&&e&&(e.ctrlKey||2<e.which))&&(V?"y":"x"),(F=!B&&!q.allowEventDefault)&&(Ba(e),za(me,"touchforcechange",Ba)),e.changedTouches?(e=x=e.changedTouches[0],m=e.identifier):e.pointerId?m=e.pointerId:x=m=null,Ae++,function _addToRenderQueue(e){Oe.push(e),1===Oe.length&&xe.ticker.add(ua)}(zh),b=q.pointerY=e.pageY,w=q.pointerX=e.pageX,Qa(q,"pressInit","onPressInit"),(B||q.autoScroll)&&La(h.parentNode),!h.parentNode||!q.autoScroll||f||Q||!h.parentNode._gsMaxScrollX||we.parentNode||h.getBBox||(we.style.width=h.parentNode.scrollWidth+"px",h.parentNode.appendChild(we)),Ih(),q.tween&&q.tween.kill(),q.isThrowing=!1,xe.killTweensOf(f||h,o,!0),f&&xe.killTweensOf(h,{scrollTo:1},!0),q.tween=q.lockedAxis=null,!p.zIndexBoost&&(Q||f||!1===p.zIndexBoost)||(h.style.zIndex=Draggable.zIndex++),q.isPressed=!0,g=!(!p.onDrag&&!q._listeners.drag),s=!(!p.onMove&&!q._listeners.move),!1!==p.cursor||p.activeCursor)for(n=J.length;-1<--n;)xe.set(J[n],{cursor:p.activeCursor||p.cursor||("grab"===Se?"grabbing":Se)});Qa(q,"press","onPress")}}function Ph(e){if(e&&q.isDragging&&!f){var t=e.target||h.parentNode,n=t.scrollLeft-t._gsScrollX,o=t.scrollTop-t._gsScrollY;(n||o)&&(k?(w-=n*k.a+o*k.c,b-=o*k.d+n*k.b):(w-=n,b-=o),t._gsScrollX+=n,t._gsScrollY+=o,he(q.pointerX,q.pointerY))}}function Qh(e){var t=Be(),n=t-le<100,o=t-ee<50,r=n&&I===le,i=q.pointerEvent&&q.pointerEvent.defaultPrevented,a=n&&c===le,l=e.isTrusted||null==e.isTrusted&&n&&r;if((r||o&&!1!==q.vars.suppressClickOnDrag)&&e.stopImmediatePropagation&&e.stopImmediatePropagation(),n&&(!q.pointerEvent||!q.pointerEvent.defaultPrevented)&&(!r||l&&!a))return l&&r&&(c=le),void(I=le);(q.isPressed||o||n)&&(l&&e.detail&&n&&!i||Ba(e)),n||o||H||(e&&e.target&&(q.pointerEvent=e),Qa(q,"click","onClick"))}function Rh(e){return k?{x:e.x*k.a+e.y*k.c+k.e,y:e.x*k.b+e.y*k.d+k.f}:{x:e.x,y:e.y}}var u,f,w,b,T,D,M,g,s,E,L,S,P,x,m,X,Y,t,A,C,N,y,v,k,R,B,O,z,I,c,F,d,H,n=(p.type||"x,y").toLowerCase(),W=~n.indexOf("x")||~n.indexOf("y"),Q=-1!==n.indexOf("rotation"),G=Q?"rotation":W?"x":"left",K=W?"y":"top",V=!(!~n.indexOf("x")&&!~n.indexOf("left")&&"scroll"!==n),U=!(!~n.indexOf("y")&&!~n.indexOf("top")&&"scroll"!==n),j=p.minimumMovement||2,q=_assertThisInitialized(e),J=Te(p.trigger||p.handle||h),o={},ee=0,te=!1,ne=p.autoScrollMarginTop||40,oe=p.autoScrollMarginRight||40,re=p.autoScrollMarginBottom||40,ie=p.autoScrollMarginLeft||40,ae=p.clickableTest||Va,le=0,se=h._gsap||xe.core.getCache(h),ce=function _isFixed(e){return"fixed"===Na(e).position||((e=e.parentNode)&&1===e.nodeType?_isFixed(e):void 0)}(h),de=h.ownerDocument||ye,pe=function isTweening(){return q.tween&&q.tween.isActive()},ue=function onMove(e){var t,n,o,r,i,a,l=e;if(u&&!Ee&&q.isPressed&&e){if(t=(q.pointerEvent=e).changedTouches){if((e=t[0])!==x&&e.identifier!==m){for(r=t.length;-1<--r&&(e=t[r]).identifier!==m&&e.target!==h;);if(r<0)return}}else if(e.pointerId&&m&&e.pointerId!==m)return;v&&B&&!O&&(Ge.x=e.pageX-(ce?Ga(de):0),Ge.y=e.pageY-(ce?Fa(de):0),k&&k.apply(Ge,Ge),n=Ge.x,o=Ge.y,((i=Math.abs(n-w))!==(a=Math.abs(o-b))&&(j<i||j<a)||_e&&B===O)&&(O=a<i&&V?"x":"y",B&&O!==B&&za(me,"touchforcechange",Ba),!1!==q.vars.lockAxisOnTouchScroll&&V&&U&&(q.lockedAxis="x"===O?"y":"x",Z(q.vars.onLockAxis)&&q.vars.onLockAxis.call(q,l)),_e&&B===O))?fe(l):(F=q.allowEventDefault||B&&(!O||B===O)||!1===l.cancelable?F&&!1:(Ba(l),!0),q.autoScroll&&(te=!0),he(e.pageX,e.pageY,s))}else F&&e&&u&&Ba(e)},he=function setPointerPosition(e,t,n){var o,r,i,a,l,s,c=1-q.dragResistance,d=1-q.edgeResistance,p=q.pointerX,u=q.pointerY,h=D,f=q.x,g=q.y,x=q.endX,m=q.endY,y=q.endRotation,v=Y;q.pointerX=e,q.pointerY=t,ce&&(e-=Ga(de),t-=Fa(de)),Q?(a=Math.atan2(X.y-t,e-X.x)*ke,180<(l=q.y-a)?(D-=360,q.y=a):l<-180&&(D+=360,q.y=a),i=q.x!==T||Math.max(Math.abs(w-e),Math.abs(b-t))>j?(q.y=a,T+(D-a)*c):T):(k&&(s=e*k.a+t*k.c+k.e,t=e*k.b+t*k.d+k.f,e=s),(r=t-b)<j&&-j<r&&(r=0),(o=e-w)<j&&-j<o&&(o=0),(q.lockAxis||q.lockedAxis)&&(o||r)&&((s=q.lockedAxis)||(q.lockedAxis=s=V&&Math.abs(o)>Math.abs(r)?"y":U?"x":null,s&&Z(q.vars.onLockAxis)&&q.vars.onLockAxis.call(q,q.pointerEvent)),"y"===s?r=0:"x"===s&&(o=0)),i=da(T+o*c),a=da(D+r*c)),(A||C||N)&&(q.x!==i||q.y!==a&&!Q)&&(N&&(He.x=i,He.y=a,s=N(He),i=da(s.x),a=da(s.y)),A&&(i=da(A(i))),C&&(a=da(C(a)))),M&&(E<i?i=E+Math.round((i-E)*d):i<L&&(i=L+Math.round((i-L)*d)),Q||(S<a?a=Math.round(S+(a-S)*d):a<P&&(a=Math.round(P+(a-P)*d)))),q.x===i&&(q.y===a||Q)||(Q?(q.endRotation=q.x=q.endX=i,Y=!0):(U&&(q.y=q.endY=a,Y=!0),V&&(q.x=q.endX=i,Y=!0)),n&&!1===Qa(q,"move","onMove")?(q.pointerX=p,q.pointerY=u,D=h,q.x=f,q.y=g,q.endX=x,q.endY=m,q.endRotation=y,Y=v):!q.isDragging&&q.isPressed&&(q.isDragging=H=!0,Qa(q,"dragstart","onDragStart")))},fe=function onRelease(e,t){if(u&&q.isPressed&&(!e||null==m||t||!(e.pointerId&&e.pointerId!==m&&e.target!==h||e.changedTouches&&!function _hasTouchID(e,t){for(var n=e.length;n--;)if(e[n].identifier===t)return!0}(e.changedTouches,m)))){q.isPressed=!1;var n,o,r,i,a,l=e,s=q.isDragging,c=q.vars.allowContextMenu&&e&&(e.ctrlKey||2<e.which),d=xe.delayedCall(.001,Kh);if(v?(Aa(v,"touchend",onRelease),Aa(v,"touchmove",ue),Aa(v,"touchcancel",onRelease),Aa(de,"touchstart",Ea)):Aa(de,"mousemove",ue),Aa(me,"touchforcechange",Ba),Pe&&v||(Aa(de,"mouseup",onRelease),e&&e.target&&Aa(e.target,"mouseup",onRelease)),Y=!1,s&&(ee=Fe=Be(),q.isDragging=!1),xa(zh),y&&!c)return e&&(Aa(e.target,"change",onRelease),q.pointerEvent=l),Wa(J,!1),Qa(q,"release","onRelease"),Qa(q,"click","onClick"),void(y=!1);for(o=J.length;-1<--o;)Ma(J[o],"cursor",p.cursor||(!1!==p.cursor?Se:null));if(Ae--,e){if((n=e.changedTouches)&&(e=n[0])!==x&&e.identifier!==m){for(o=n.length;-1<--o&&(e=n[o]).identifier!==m&&e.target!==h;);if(o<0&&!t)return}q.pointerEvent=l,q.pointerX=e.pageX,q.pointerY=e.pageY}return c&&l?(Ba(l),F=!0,Qa(q,"release","onRelease")):l&&!s?(F=!1,R&&(p.snap||p.bounds)&&Gh(p.inertia||p.throwProps),Qa(q,"release","onRelease"),_e&&"touchmove"===l.type||-1!==l.type.indexOf("cancel")||(Qa(q,"click","onClick"),Be()-le<300&&Qa(q,"doubleclick","onDoubleClick"),i=l.target||h,le=Be(),a=function syntheticClick(){le===I||!q.enabled()||q.isPressed||l.defaultPrevented||(i.click?i.click():de.createEvent&&((r=de.createEvent("MouseEvents")).initMouseEvent("click",!0,!0,me,1,q.pointerEvent.screenX,q.pointerEvent.screenY,q.pointerX,q.pointerY,!1,!1,!1,!1,0,null),i.dispatchEvent(r)))},_e||l.defaultPrevented||xe.delayedCall(.05,a))):(Gh(p.inertia||p.throwProps),q.allowEventDefault||!l||!1===p.dragClickables&&ae.call(q,l.target)||!s||B&&(!O||B!==O)||!1===l.cancelable?F=!1:(F=!0,Ba(l)),Qa(q,"release","onRelease")),pe()&&d.duration(q.tween.duration()),s&&Qa(q,"dragend","onDragEnd"),!0}F&&e&&u&&Ba(e)};return(t=Draggable.get(h))&&t.kill(),e.startDrag=function(e,t){var n,o,r,i;Lh(e||q.pointerEvent,!0),t&&!q.hitTest(e||q.pointerEvent)&&(n=Pa(e||q.pointerEvent),o=Pa(h),r=Rh({x:n.left+n.width/2,y:n.top+n.height/2}),i=Rh({x:o.left+o.width/2,y:o.top+o.height/2}),w-=r.x-i.x,b-=r.y-i.y),q.isDragging||(q.isDragging=H=!0,Qa(q,"dragstart","onDragStart"))},e.drag=ue,e.endDrag=function(e){return fe(e||q.pointerEvent,!0)},e.timeSinceDrag=function(){return q.isDragging?0:(Be()-ee)/1e3},e.timeSinceClick=function(){return(Be()-le)/1e3},e.hitTest=function(e,t){return Draggable.hitTest(q.target,e,t)},e.getDirection=function(e,t){var n,o,r,i,a,l,s="velocity"===e&&Le?e:$(e)&&!Q?"element":"start";return"element"===s&&(a=Pa(q.target),l=Pa(e)),n="start"===s?q.x-T:"velocity"===s?Le.getVelocity(h,G):a.left+a.width/2-(l.left+l.width/2),Q?n<0?"counter-clockwise":"clockwise":(t=t||2,o="start"===s?q.y-D:"velocity"===s?Le.getVelocity(h,K):a.top+a.height/2-(l.top+l.height/2),i=(r=Math.abs(n/o))<1/t?"":n<0?"left":"right",r<t&&(""!==i&&(i+="-"),i+=o<0?"up":"down"),i)},e.applyBounds=function(e,t){var n,o,r,i,a,l;if(e&&p.bounds!==e)return p.bounds=e,q.update(!0,t);if(Ah(!0),Dh(),M&&!pe()){if(n=q.x,o=q.y,E<n?n=E:n<L&&(n=L),S<o?o=S:o<P&&(o=P),(q.x!==n||q.y!==o)&&(r=!0,q.x=q.endX=n,Q?q.endRotation=n:q.y=q.endY=o,zh(Y=!0),q.autoScroll&&!q.isDragging))for(La(h.parentNode),i=h,We.scrollTop=null!=me.pageYOffset?me.pageYOffset:null!=de.documentElement.scrollTop?de.documentElement.scrollTop:de.body.scrollTop,We.scrollLeft=null!=me.pageXOffset?me.pageXOffset:null!=de.documentElement.scrollLeft?de.documentElement.scrollLeft:de.body.scrollLeft;i&&!l;)a=(l=Qe(i.parentNode))?We:i.parentNode,U&&a.scrollTop>a._gsMaxScrollY&&(a.scrollTop=a._gsMaxScrollY),V&&a.scrollLeft>a._gsMaxScrollX&&(a.scrollLeft=a._gsMaxScrollX),i=a;q.isThrowing&&(r||q.endX>E||q.endX<L||q.endY>S||q.endY<P)&&Gh(p.inertia||p.throwProps,r)}return q},e.update=function(e,t,n){if(t&&q.isPressed){var o=getGlobalMatrix(h),r=d.apply({x:q.x-T,y:q.y-D}),i=getGlobalMatrix(h.parentNode,!0);i.apply({x:o.e-r.x,y:o.f-r.y},r),q.x-=r.x-i.e,q.y-=r.y-i.f,zh(!0),Ih()}var a=q.x,l=q.y;return Hh(!t),e?q.applyBounds():(Y&&n&&zh(!0),Ah(!0)),t&&(he(q.pointerX,q.pointerY),Y&&zh(!0)),q.isPressed&&!t&&(V&&.01<Math.abs(a-q.x)||U&&.01<Math.abs(l-q.y)&&!Q)&&Ih(),q.autoScroll&&(La(h.parentNode,q.isDragging),te=q.isDragging,zh(!0),Ia(h,Ph),Ha(h,Ph)),q},e.enable=function(e){var t,n,o,r={lazy:!0};if(!1!==p.cursor&&(r.cursor=p.cursor||Se),xe.utils.checkPrefix("touchCallout")&&(r.touchCallout="none"),"soft"!==e){for(ta(J,V==U?"none":p.allowNativeTouchScrolling&&h.scrollHeight===h.clientHeight==(h.scrollWidth===h.clientHeight)||p.allowEventDefault?"manipulation":V?"pan-y":"pan-x"),n=J.length;-1<--n;)o=J[n],Pe||za(o,"mousedown",Lh),za(o,"touchstart",Lh),za(o,"click",Qh,!0),xe.set(o,r),o.getBBox&&o.ownerSVGElement&&V!=U&&xe.set(o.ownerSVGElement,{touchAction:p.allowNativeTouchScrolling||p.allowEventDefault?"manipulation":V?"pan-y":"pan-x"}),p.allowContextMenu||za(o,"contextmenu",yh);Wa(J,!1)}return Ha(h,Ph),u=!0,Le&&"soft"!==e&&Le.track(f||h,W?"x,y":Q?"rotation":"top,left"),h._gsDragID=t=h._gsDragID||"d"+Ie++,ze[t]=q,f&&(f.enable(),f.element._gsDragID=t),(p.bounds||Q)&&Ih(),p.bounds&&q.applyBounds(),q},e.disable=function(e){for(var t,n=q.isDragging,o=J.length;-1<--o;)Ma(J[o],"cursor",null);if("soft"!==e){for(ta(J,null),o=J.length;-1<--o;)t=J[o],Ma(t,"touchCallout",null),Aa(t,"mousedown",Lh),Aa(t,"touchstart",Lh),Aa(t,"click",Qh,!0),Aa(t,"contextmenu",yh);Wa(J,!0),v&&(Aa(v,"touchcancel",fe),Aa(v,"touchend",fe),Aa(v,"touchmove",ue)),Aa(de,"mouseup",fe),Aa(de,"mousemove",ue)}return Ia(h,Ph),u=!1,Le&&"soft"!==e&&(Le.untrack(f||h,W?"x,y":Q?"rotation":"top,left"),q.tween&&q.tween.kill()),f&&f.disable(),xa(zh),q.isDragging=q.isPressed=y=!1,n&&Qa(q,"dragend","onDragEnd"),q},e.enabled=function(e,t){return arguments.length?e?q.enable(t):q.disable(t):u},e.kill=function(){return q.isThrowing=!1,q.tween&&q.tween.kill(),q.disable(),xe.set(J,{clearProps:"userSelect"}),delete ze[h._gsDragID],q},e.revert=function(){this.kill(),this.styles&&this.styles.revert()},~n.indexOf("scroll")&&(f=e.scrollProxy=new $a(h,function _extend(e,t){for(var n in t)n in e||(e[n]=t[n]);return e}({onKill:function onKill(){q.isPressed&&fe(null)}},p)),h.style.overflowY=U&&!De?"auto":"hidden",h.style.overflowX=V&&!De?"auto":"hidden",h=f.content),Q?o.rotation=1:(V&&(o[G]=1),U&&(o[K]=1)),se.force3D=!("force3D"in p)||p.force3D,Xe(_assertThisInitialized(e)),e.enable(),e}!function _setDefaults(e,t){for(var n in t)n in e||(e[n]=t[n])}(I.prototype,{pointerX:0,pointerY:0,startX:0,startY:0,deltaX:0,deltaY:0,isDragging:!1,isPressed:!1}),I.zIndex=1e3,I.version="3.12.7",Y()&&xe.registerPlugin(I),e.Draggable=I,e.default=I;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/Draggable.min.js.map b/node_modules/gsap/dist/Draggable.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..b0755897f0b0ae30e6c8ee9921df2dff65452795 --- /dev/null +++ b/node_modules/gsap/dist/Draggable.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"Draggable.min.js","sources":["../src/utils/matrix.js","../src/Draggable.js"],"sourcesContent":["/*!\n * matrix 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _doc, _win, _docElement, _body,\t_divContainer, _svgContainer, _identityMatrix, _gEl,\n\t_transformProp = \"transform\",\n\t_transformOriginProp = _transformProp + \"Origin\",\n\t_hasOffsetBug,\n\t_setDoc = element => {\n\t\tlet doc = element.ownerDocument || element;\n\t\tif (!(_transformProp in element.style) && \"msTransform\" in element.style) { //to improve compatibility with old Microsoft browsers\n\t\t\t_transformProp = \"msTransform\";\n\t\t\t_transformOriginProp = _transformProp + \"Origin\";\n\t\t}\n\t\twhile (doc.parentNode && (doc = doc.parentNode)) {\t}\n\t\t_win = window;\n\t\t_identityMatrix = new Matrix2D();\n\t\tif (doc) {\n\t\t\t_doc = doc;\n\t\t\t_docElement = doc.documentElement;\n\t\t\t_body = doc.body;\n\t\t\t_gEl = _doc.createElementNS(\"http://www.w3.org/2000/svg\", \"g\");\n\t\t\t// prevent any existing CSS from transforming it\n\t\t\t_gEl.style.transform = \"none\";\n\t\t\t// now test for the offset reporting bug. Use feature detection instead of browser sniffing to make things more bulletproof and future-proof. Hopefully Safari will fix their bug soon.\n\t\t\tlet d1 = doc.createElement(\"div\"),\n\t\t\t\td2 = doc.createElement(\"div\"),\n\t\t\t\troot = doc && (doc.body || doc.firstElementChild);\n\t\t\tif (root && root.appendChild) {\n\t\t\t\troot.appendChild(d1);\n\t\t\t\td1.appendChild(d2);\n\t\t\t\td1.setAttribute(\"style\", \"position:static;transform:translate3d(0,0,1px)\");\n\t\t\t\t_hasOffsetBug = (d2.offsetParent !== d1);\n\t\t\t\troot.removeChild(d1);\n\t\t\t}\n\t\t}\n\t\treturn doc;\n\t},\n\t_forceNonZeroScale = e => { // walks up the element's ancestors and finds any that had their scale set to 0 via GSAP, and changes them to 0.0001 to ensure that measurements work. Firefox has a bug that causes it to incorrectly report getBoundingClientRect() when scale is 0.\n\t\tlet a, cache;\n\t\twhile (e && e !== _body) {\n\t\t\tcache = e._gsap;\n\t\t\tcache && cache.uncache && cache.get(e, \"x\"); // force re-parsing of transforms if necessary\n\t\t\tif (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) {\n\t\t\t\tcache.scaleX = cache.scaleY = 1e-4;\n\t\t\t\tcache.renderTransform(1, cache);\n\t\t\t\ta ? a.push(cache) : (a = [cache]);\n\t\t\t}\n\t\t\te = e.parentNode;\n\t\t}\n\t\treturn a;\n\t},\n\t// possible future addition: pass an element to _forceDisplay() and it'll walk up all its ancestors and make sure anything with display: none is set to display: block, and if there's no parentNode, it'll add it to the body. It returns an Array that you can then feed to _revertDisplay() to have it revert all the changes it made.\n\t// _forceDisplay = e => {\n\t// \tlet a = [],\n\t// \t\tparent;\n\t// \twhile (e && e !== _body) {\n\t// \t\tparent = e.parentNode;\n\t// \t\t(_win.getComputedStyle(e).display === \"none\" || !parent) && a.push(e, e.style.display, parent) && (e.style.display = \"block\");\n\t// \t\tparent || _body.appendChild(e);\n\t// \t\te = parent;\n\t// \t}\n\t// \treturn a;\n\t// },\n\t// _revertDisplay = a => {\n\t// \tfor (let i = 0; i < a.length; i+=3) {\n\t// \t\ta[i+1] ? (a[i].style.display = a[i+1]) : a[i].style.removeProperty(\"display\");\n\t// \t\ta[i+2] || a[i].parentNode.removeChild(a[i]);\n\t// \t}\n\t// },\n\t_svgTemps = [], //we create 3 elements for SVG, and 3 for other DOM elements and cache them for performance reasons. They get nested in _divContainer and _svgContainer so that just one element is added to the DOM on each successive attempt. Again, performance is key.\n\t_divTemps = [],\n\t_getDocScrollTop = () => _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0,\n\t_getDocScrollLeft = () => _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0,\n\t_svgOwner = element => element.ownerSVGElement || ((element.tagName + \"\").toLowerCase() === \"svg\" ? element : null),\n\t_isFixed = element => {\n\t\tif (_win.getComputedStyle(element).position === \"fixed\") {\n\t\t\treturn true;\n\t\t}\n\t\telement = element.parentNode;\n\t\tif (element && element.nodeType === 1) { // avoid document fragments which will throw an error.\n\t\t\treturn _isFixed(element);\n\t\t}\n\t},\n\t_createSibling = (element, i) => {\n\t\tif (element.parentNode && (_doc || _setDoc(element))) {\n\t\t\tlet svg = _svgOwner(element),\n\t\t\t\tns = svg ? (svg.getAttribute(\"xmlns\") || \"http://www.w3.org/2000/svg\") : \"http://www.w3.org/1999/xhtml\",\n\t\t\t\ttype = svg ? (i ? \"rect\" : \"g\") : \"div\",\n\t\t\t\tx = i !== 2 ? 0 : 100,\n\t\t\t\ty = i === 3 ? 100 : 0,\n\t\t\t\tcss = \"position:absolute;display:block;pointer-events:none;margin:0;padding:0;\",\n\t\t\t\te = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, \"http\"), type) : _doc.createElement(type);\n\t\t\tif (i) {\n\t\t\t\tif (!svg) {\n\t\t\t\t\tif (!_divContainer) {\n\t\t\t\t\t\t_divContainer = _createSibling(element);\n\t\t\t\t\t\t_divContainer.style.cssText = css;\n\t\t\t\t\t}\n\t\t\t\t\te.style.cssText = css + \"width:0.1px;height:0.1px;top:\" + y + \"px;left:\" + x + \"px\";\n\t\t\t\t\t_divContainer.appendChild(e);\n\n\t\t\t\t} else {\n\t\t\t\t\t_svgContainer || (_svgContainer = _createSibling(element));\n\t\t\t\t\te.setAttribute(\"width\", 0.01);\n\t\t\t\t\te.setAttribute(\"height\", 0.01);\n\t\t\t\t\te.setAttribute(\"transform\", \"translate(\" + x + \",\" + y + \")\");\n\t\t\t\t\t_svgContainer.appendChild(e);\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn e;\n\t\t}\n\t\tthrow \"Need document and parent.\";\n\t},\n\t_consolidate = m => { // replaces SVGTransformList.consolidate() because a bug in Firefox causes it to break pointer events. See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800\n\t\tlet c = new Matrix2D(),\n\t\t\ti = 0;\n\t\tfor (; i < m.numberOfItems; i++) {\n\t\t\tc.multiply(m.getItem(i).matrix);\n\t\t}\n\t\treturn c;\n\t},\n\t_getCTM = svg => {\n\t\tlet m = svg.getCTM(),\n\t\t\ttransform;\n\t\tif (!m) { // Firefox returns null for getCTM() on root <svg> elements, so this is a workaround using a <g> that we temporarily append.\n\t\t\ttransform = svg.style[_transformProp];\n\t\t\tsvg.style[_transformProp] = \"none\"; // a bug in Firefox causes css transforms to contaminate the getCTM()\n\t\t\tsvg.appendChild(_gEl);\n\t\t\tm = _gEl.getCTM();\n\t\t\tsvg.removeChild(_gEl);\n\t\t\ttransform ? (svg.style[_transformProp] = transform) : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, \"-$1\").toLowerCase());\n\t\t}\n\t\treturn m || _identityMatrix.clone(); // Firefox will still return null if the <svg> has a width/height of 0 in the browser.\n\t},\n\t_placeSiblings = (element, adjustGOffset) => {\n\t\tlet svg = _svgOwner(element),\n\t\t\tisRootSVG = element === svg,\n\t\t\tsiblings = svg ? _svgTemps : _divTemps,\n\t\t\tparent = element.parentNode,\n\t\t\tcontainer, m, b, x, y, cs;\n\t\tif (element === _win) {\n\t\t\treturn element;\n\t\t}\n\t\tsiblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3));\n\t\tcontainer = svg ? _svgContainer : _divContainer;\n\t\tif (svg) {\n\t\t\tif (isRootSVG) {\n\t\t\t\tb = _getCTM(element);\n\t\t\t\tx = -b.e / b.a;\n\t\t\t\ty = -b.f / b.d;\n\t\t\t\tm = _identityMatrix;\n\t\t\t} else if (element.getBBox) {\n\t\t\t\tb = element.getBBox();\n\t\t\t\tm = element.transform ? element.transform.baseVal : {}; // IE11 doesn't follow the spec.\n\t\t\t\tm = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; // don't call m.consolidate().matrix because a bug in Firefox makes pointer events not work when consolidate() is called on the same tick as getBoundingClientRect()! See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800\n\t\t\t\tx = m.a * b.x + m.c * b.y;\n\t\t\t\ty = m.b * b.x + m.d * b.y;\n\t\t\t} else { // may be a <mask> which has no getBBox() so just use defaults instead of throwing errors.\n\t\t\t\tm = new Matrix2D();\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\tif (adjustGOffset && element.tagName.toLowerCase() === \"g\") {\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\t(isRootSVG ? svg : parent).appendChild(container);\n\t\t\tcontainer.setAttribute(\"transform\", \"matrix(\" + m.a + \",\" + m.b + \",\" + m.c + \",\" + m.d + \",\" + (m.e + x) + \",\" + (m.f + y) + \")\");\n\t\t} else {\n\t\t\tx = y = 0;\n\t\t\tif (_hasOffsetBug) { // some browsers (like Safari) have a bug that causes them to misreport offset values. When an ancestor element has a transform applied, it's supposed to treat it as if it's position: relative (new context). Safari botches this, so we need to find the closest ancestor (between the element and its offsetParent) that has a transform applied and if one is found, grab its offsetTop/Left and subtract them to compensate.\n\t\t\t\tm = element.offsetParent;\n\t\t\t\tb = element;\n\t\t\t\twhile (b && (b = b.parentNode) && b !== m && b.parentNode) {\n\t\t\t\t\tif ((_win.getComputedStyle(b)[_transformProp] + \"\").length > 4) {\n\t\t\t\t\t\tx = b.offsetLeft;\n\t\t\t\t\t\ty = b.offsetTop;\n\t\t\t\t\t\tb = 0;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\tcs = _win.getComputedStyle(element);\n\t\t\tif (cs.position !== \"absolute\" && cs.position !== \"fixed\") {\n\t\t\t\tm = element.offsetParent;\n\t\t\t\twhile (parent && parent !== m) { // if there's an ancestor element between the element and its offsetParent that's scrolled, we must factor that in.\n\t\t\t\t\tx += parent.scrollLeft || 0;\n\t\t\t\t\ty += parent.scrollTop || 0;\n\t\t\t\t\tparent = parent.parentNode;\n\t\t\t\t}\n\t\t\t}\n\t\t\tb = container.style;\n\t\t\tb.top = (element.offsetTop - y) + \"px\";\n\t\t\tb.left = (element.offsetLeft - x) + \"px\";\n\t\t\tb[_transformProp] = cs[_transformProp];\n\t\t\tb[_transformOriginProp] = cs[_transformOriginProp];\n\t\t\t// b.border = m.border;\n\t\t\t// b.borderLeftStyle = m.borderLeftStyle;\n\t\t\t// b.borderTopStyle = m.borderTopStyle;\n\t\t\t// b.borderLeftWidth = m.borderLeftWidth;\n\t\t\t// b.borderTopWidth = m.borderTopWidth;\n\t\t\tb.position = cs.position === \"fixed\" ? \"fixed\" : \"absolute\";\n\t\t\telement.parentNode.appendChild(container);\n\t\t}\n\t\treturn container;\n\t},\n\t_setMatrix = (m, a, b, c, d, e, f) => {\n\t\tm.a = a;\n\t\tm.b = b;\n\t\tm.c = c;\n\t\tm.d = d;\n\t\tm.e = e;\n\t\tm.f = f;\n\t\treturn m;\n\t};\n\nexport class Matrix2D {\n\tconstructor(a=1, b=0, c=0, d=1, e=0, f=0) {\n\t\t_setMatrix(this, a, b, c, d, e, f);\n\t}\n\n\tinverse() {\n\t\tlet {a, b, c, d, e, f} = this,\n\t\t\tdeterminant = (a * d - b * c) || 1e-10;\n\t\treturn _setMatrix(\n\t\t\tthis,\n\t\t\td / determinant,\n\t\t\t-b / determinant,\n\t\t\t-c / determinant,\n\t\t\ta / determinant,\n\t\t\t(c * f - d * e) / determinant,\n\t\t\t-(a * f - b * e) / determinant\n\t\t);\n\t}\n\n\tmultiply(matrix) {\n\t\tlet {a, b, c, d, e, f} = this,\n\t\t\ta2 = matrix.a,\n\t\t\tb2 = matrix.c,\n\t\t\tc2 = matrix.b,\n\t\t\td2 = matrix.d,\n\t\t\te2 = matrix.e,\n\t\t\tf2 = matrix.f;\n\t\treturn _setMatrix(this,\n\t\t\ta2 * a + c2 * c,\n\t\t\ta2 * b + c2 * d,\n\t\t\tb2 * a + d2 * c,\n\t\t\tb2 * b + d2 * d,\n\t\t\te + e2 * a + f2 * c,\n\t\t\tf + e2 * b + f2 * d);\n\t}\n\n\tclone() {\n\t\treturn new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f);\n\t}\n\n\tequals(matrix) {\n\t\tlet {a, b, c, d, e, f} = this;\n\t\treturn (a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f);\n\t}\n\n\tapply(point, decoratee={}) {\n\t\tlet {x, y} = point,\n\t\t\t{a, b, c, d, e, f} = this;\n\t\tdecoratee.x = (x * a + y * c + e) || 0;\n\t\tdecoratee.y = (x * b + y * d + f) || 0;\n\t\treturn decoratee;\n\t}\n\n}\n\n// Feed in an element and it'll return a 2D matrix (optionally inverted) so that you can translate between coordinate spaces.\n// Inverting lets you translate a global point into a local coordinate space. No inverting lets you go the other way.\n// We needed this to work around various browser bugs, like Firefox doesn't accurately report getScreenCTM() when there\n// are transforms applied to ancestor elements.\n// The matrix math to convert any x/y coordinate is as follows, which is wrapped in a convenient apply() method of Matrix2D above:\n// tx = m.a * x + m.c * y + m.e\n// ty = m.b * x + m.d * y + m.f\nexport function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { // adjustGOffset is typically used only when grabbing an element's PARENT's global matrix, and it ignores the x/y offset of any SVG <g> elements because they behave in a special way.\n\tif (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) {\n\t\treturn new Matrix2D();\n\t}\n\tlet zeroScales = _forceNonZeroScale(element),\n\t\tsvg = _svgOwner(element),\n\t\ttemps = svg ? _svgTemps : _divTemps,\n\t\tcontainer = _placeSiblings(element, adjustGOffset),\n\t\tb1 = temps[0].getBoundingClientRect(),\n\t\tb2 = temps[1].getBoundingClientRect(),\n\t\tb3 = temps[2].getBoundingClientRect(),\n\t\tparent = container.parentNode,\n\t\tisFixed = !includeScrollInFixed && _isFixed(element),\n\t\tm = new Matrix2D(\n\t\t\t(b2.left - b1.left) / 100,\n\t\t\t(b2.top - b1.top) / 100,\n\t\t\t(b3.left - b1.left) / 100,\n\t\t\t(b3.top - b1.top) / 100,\n\t\t\tb1.left + (isFixed ? 0 : _getDocScrollLeft()),\n\t\t\tb1.top + (isFixed ? 0 : _getDocScrollTop())\n\t\t);\n\tparent.removeChild(container);\n\tif (zeroScales) {\n\t\tb1 = zeroScales.length;\n\t\twhile (b1--) {\n\t\t\tb2 = zeroScales[b1];\n\t\t\tb2.scaleX = b2.scaleY = 0;\n\t\t\tb2.renderTransform(1, b2);\n\t\t}\n\t}\n\treturn inverse ? m.inverse() : m;\n}\n\nexport { _getDocScrollTop, _getDocScrollLeft, _setDoc, _isFixed, _getCTM };\n\n// export function getMatrix(element) {\n// \t_doc || _setDoc(element);\n// \tlet m = (_win.getComputedStyle(element)[_transformProp] + \"\").substr(7).match(/[-.]*\\d+[.e\\-+]*\\d*[e\\-\\+]*\\d*/g),\n// \t\tis2D = m && m.length === 6;\n// \treturn !m || m.length < 6 ? new Matrix2D() : new Matrix2D(+m[0], +m[1], +m[is2D ? 2 : 4], +m[is2D ? 3 : 5], +m[is2D ? 4 : 12], +m[is2D ? 5 : 13]);\n// }","/*!\n * Draggable 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n */\n/* eslint-disable */\n\nimport { getGlobalMatrix, Matrix2D } from \"./utils/matrix.js\";\n\nlet gsap, _win, _doc, _docElement, _body, _tempDiv, _placeholderDiv, _coreInitted, _checkPrefix, _toArray, _supportsPassive, _isTouchDevice, _touchEventLookup, _isMultiTouching, _isAndroid, InertiaPlugin, _defaultCursor, _supportsPointer, _context, _getStyleSaver,\n\t_dragCount = 0,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_isFunction = value => typeof(value) === \"function\",\n\t_isObject = value => typeof(value) === \"object\",\n\t_isUndefined = value => typeof(value) === \"undefined\",\n\t_emptyFunc = () => false,\n\t_transformProp = \"transform\",\n\t_transformOriginProp = \"transformOrigin\",\n\t_round = value => Math.round(value * 10000) / 10000,\n\t_isArray = Array.isArray,\n\t_createElement = (type, ns) => {\n\t\tlet e = _doc.createElementNS ? _doc.createElementNS((ns || \"http://www.w3.org/1999/xhtml\").replace(/^https/, \"http\"), type) : _doc.createElement(type); //some servers swap in https for http in the namespace which can break things, making \"style\" inaccessible.\n\t\treturn e.style ? e : _doc.createElement(type); //some environments won't allow access to the element's style when created with a namespace in which case we default to the standard createElement() to work around the issue. Also note that when GSAP is embedded directly inside an SVG file, createElement() won't allow access to the style object in Firefox (see https://gsap.com/forums/topic/20215-problem-using-tweenmax-in-standalone-self-containing-svg-file-err-cannot-set-property-csstext-of-undefined/).\n\t},\n\t_RAD2DEG = 180 / Math.PI,\n\t_bigNum = 1e20,\n\t_identityMatrix = new Matrix2D(),\n\t_getTime = Date.now || (() => new Date().getTime()),\n\t_renderQueue = [],\n\t_lookup = {}, //when a Draggable is created, the target gets a unique _gsDragID property that allows gets associated with the Draggable instance for quick lookups in Draggable.get(). This avoids circular references that could cause gc problems.\n\t_lookupCount = 0,\n\t_clickableTagExp = /^(?:a|input|textarea|button|select)$/i,\n\t_lastDragTime = 0,\n\t_temp1 = {}, // a simple object we reuse and populate (usually x/y properties) to conserve memory and improve performance.\n\t_windowProxy = {}, //memory/performance optimization - we reuse this object during autoScroll to store window-related bounds/offsets.\n\t_copy = (obj, factor) => {\n\t\tlet copy = {}, p;\n\t\tfor (p in obj) {\n\t\t\tcopy[p] = factor ? obj[p] * factor : obj[p];\n\t\t}\n\t\treturn copy;\n\t},\n\t_extend = (obj, defaults) => {\n\t\tfor (let p in defaults) {\n\t\t\tif (!(p in obj)) {\n\t\t\t\tobj[p] = defaults[p];\n\t\t\t}\n\t\t}\n\t\treturn obj;\n\t},\n\t_setTouchActionForAllDescendants = (elements, value) => {\n\t\tlet i = elements.length,\n\t\t\tchildren;\n\t\twhile (i--) {\n\t\t\tvalue ? (elements[i].style.touchAction = value) : elements[i].style.removeProperty(\"touch-action\");\n\t\t\tchildren = elements[i].children;\n\t\t\tchildren && children.length && _setTouchActionForAllDescendants(children, value);\n\t\t}\n\t},\n\t_renderQueueTick = () => _renderQueue.forEach(func => func()),\n\t_addToRenderQueue = func => {\n\t\t_renderQueue.push(func);\n\t\tif (_renderQueue.length === 1) {\n\t\t\tgsap.ticker.add(_renderQueueTick);\n\t\t}\n\t},\n\t_renderQueueTimeout = () => !_renderQueue.length && gsap.ticker.remove(_renderQueueTick),\n\t_removeFromRenderQueue = func => {\n\t\tlet i = _renderQueue.length;\n\t\twhile (i--) {\n\t\t\tif (_renderQueue[i] === func) {\n\t\t\t\t_renderQueue.splice(i, 1);\n\t\t\t}\n\t\t}\n\t\tgsap.to(_renderQueueTimeout, {overwrite:true, delay:15, duration:0, onComplete:_renderQueueTimeout, data:\"_draggable\"}); //remove the \"tick\" listener only after the render queue is empty for 15 seconds (to improve performance). Adding/removing it constantly for every click/touch wouldn't deliver optimal speed, and we also don't want the ticker to keep calling the render method when things are idle for long periods of time (we want to improve battery life on mobile devices).\n\t},\n\t_setDefaults = (obj, defaults) => {\n\t\tfor (let p in defaults) {\n\t\t\tif (!(p in obj)) {\n\t\t\t\tobj[p] = defaults[p];\n\t\t\t}\n\t\t}\n\t\treturn obj;\n\t},\n\t_addListener = (element, type, func, capture) => {\n\t\tif (element.addEventListener) {\n\t\t\tlet touchType = _touchEventLookup[type];\n\t\t\tcapture = capture || (_supportsPassive ? {passive: false} : null);\n\t\t\telement.addEventListener(touchType || type, func, capture);\n\t\t\t(touchType && type !== touchType) && element.addEventListener(type, func, capture);//some browsers actually support both, so must we. But pointer events cover all.\n\t\t}\n\t},\n\t_removeListener = (element, type, func, capture) => {\n\t\tif (element.removeEventListener) {\n\t\t\tlet touchType = _touchEventLookup[type];\n\t\t\telement.removeEventListener(touchType || type, func, capture);\n\t\t\t(touchType && type !== touchType) && element.removeEventListener(type, func, capture);\n\t\t}\n\t},\n\t_preventDefault = event => {\n\t\tevent.preventDefault && event.preventDefault();\n\t\tevent.preventManipulation && event.preventManipulation(); //for some Microsoft browsers\n\t},\n\t_hasTouchID = (list, ID) => {\n\t\tlet i = list.length;\n\t\twhile (i--) {\n\t\t\tif (list[i].identifier === ID) {\n\t\t\t\treturn true;\n\t\t\t}\n\t\t}\n\t},\n\t_onMultiTouchDocumentEnd = event => {\n\t\t_isMultiTouching = (event.touches && _dragCount < event.touches.length);\n\t\t_removeListener(event.target, \"touchend\", _onMultiTouchDocumentEnd);\n\t},\n\n\t_onMultiTouchDocument = event => {\n\t\t_isMultiTouching = (event.touches && _dragCount < event.touches.length);\n\t\t_addListener(event.target, \"touchend\", _onMultiTouchDocumentEnd);\n\t},\n\t_getDocScrollTop = doc => _win.pageYOffset || doc.scrollTop || doc.documentElement.scrollTop || doc.body.scrollTop || 0,\n\t_getDocScrollLeft = doc => _win.pageXOffset || doc.scrollLeft || doc.documentElement.scrollLeft || doc.body.scrollLeft || 0,\n\t_addScrollListener = (e, callback) => {\n\t\t_addListener(e, \"scroll\", callback);\n\t\tif (!_isRoot(e.parentNode)) {\n\t\t\t_addScrollListener(e.parentNode, callback);\n\t\t}\n\t},\n\t_removeScrollListener = (e, callback) => {\n\t\t_removeListener(e, \"scroll\", callback);\n\t\tif (!_isRoot(e.parentNode)) {\n\t\t\t_removeScrollListener(e.parentNode, callback);\n\t\t}\n\t},\n\t_isRoot = e => !!(!e || e === _docElement || e.nodeType === 9 || e === _doc.body || e === _win || !e.nodeType || !e.parentNode),\n\t_getMaxScroll = (element, axis) => {\n\t\tlet dim = (axis === \"x\") ? \"Width\" : \"Height\",\n\t\t\tscroll = \"scroll\" + dim,\n\t\t\tclient = \"client\" + dim;\n\t\treturn Math.max(0, _isRoot(element) ? Math.max(_docElement[scroll], _body[scroll]) - (_win[\"inner\" + dim] || _docElement[client] || _body[client]) : element[scroll] - element[client]);\n\t},\n\t_recordMaxScrolls = (e, skipCurrent) => { //records _gsMaxScrollX and _gsMaxScrollY properties for the element and all ancestors up the chain so that we can cap it, otherwise dragging beyond the edges with autoScroll on can endlessly scroll.\n\t\tlet x = _getMaxScroll(e, \"x\"),\n\t\t\ty = _getMaxScroll(e, \"y\");\n\t\tif (_isRoot(e)) {\n\t\t\te = _windowProxy;\n\t\t} else {\n\t\t\t_recordMaxScrolls(e.parentNode, skipCurrent);\n\t\t}\n\t\te._gsMaxScrollX = x;\n\t\te._gsMaxScrollY = y;\n\t\tif (!skipCurrent) {\n\t\t\te._gsScrollX = e.scrollLeft || 0;\n\t\t\te._gsScrollY = e.scrollTop || 0;\n\t\t}\n\t},\n\t_setStyle = (element, property, value) => {\n\t\tlet style = element.style;\n\t\tif (!style) {\n\t\t\treturn;\n\t\t}\n\t\tif (_isUndefined(style[property])) {\n\t\t\tproperty = _checkPrefix(property, element) || property;\n\t\t}\n\t\tif (value == null) {\n\t\t\tstyle.removeProperty && style.removeProperty(property.replace(/([A-Z])/g, \"-$1\").toLowerCase());\n\t\t} else {\n\t\t\tstyle[property] = value;\n\t\t}\n\t},\n\t_getComputedStyle = element => _win.getComputedStyle((element instanceof Element) ? element : element.host || (element.parentNode || {}).host || element), //the \"host\" stuff helps to accommodate ShadowDom objects.\n\n\t_tempRect = {}, //reuse to reduce garbage collection tasks\n\t_parseRect = e => { //accepts a DOM element, a mouse event, or a rectangle object and returns the corresponding rectangle with left, right, width, height, top, and bottom properties\n\t\tif (e === _win) {\n\t\t\t_tempRect.left = _tempRect.top = 0;\n\t\t\t_tempRect.width = _tempRect.right = _docElement.clientWidth || e.innerWidth || _body.clientWidth || 0;\n\t\t\t_tempRect.height = _tempRect.bottom = ((e.innerHeight || 0) - 20 < _docElement.clientHeight) ? _docElement.clientHeight : e.innerHeight || _body.clientHeight || 0;\n\t\t\treturn _tempRect;\n\t\t}\n\t\tlet doc = e.ownerDocument || _doc,\n\t\t\tr = !_isUndefined(e.pageX) ? {left: e.pageX - _getDocScrollLeft(doc), top: e.pageY - _getDocScrollTop(doc), right: e.pageX - _getDocScrollLeft(doc) + 1, bottom: e.pageY - _getDocScrollTop(doc) + 1} : (!e.nodeType && !_isUndefined(e.left) && !_isUndefined(e.top)) ? e : _toArray(e)[0].getBoundingClientRect();\n\t\tif (_isUndefined(r.right) && !_isUndefined(r.width)) {\n\t\t\tr.right = r.left + r.width;\n\t\t\tr.bottom = r.top + r.height;\n\t\t} else if (_isUndefined(r.width)) { //some browsers don't include width and height properties. We can't just set them directly on r because some browsers throw errors, so create a new generic object.\n\t\t\tr = {width: r.right - r.left, height: r.bottom - r.top, right: r.right, left: r.left, bottom: r.bottom, top: r.top};\n\t\t}\n\t\treturn r;\n\t},\n\n\t_dispatchEvent = (target, type, callbackName) => {\n\t\tlet vars = target.vars,\n\t\t\tcallback = vars[callbackName],\n\t\t\tlisteners = target._listeners[type],\n\t\t\tresult;\n\t\tif (_isFunction(callback)) {\n\t\t\tresult = callback.apply(vars.callbackScope || target, vars[callbackName + \"Params\"] || [target.pointerEvent]);\n\t\t}\n\t\tif (listeners && target.dispatchEvent(type) === false) {\n\t\t\tresult = false;\n\t\t}\n\t\treturn result;\n\t},\n\t_getBounds = (target, context) => { //accepts any of the following: a DOM element, jQuery object, selector text, or an object defining bounds as {top, left, width, height} or {minX, maxX, minY, maxY}. Returns an object with left, top, width, and height properties.\n\t\tlet e = _toArray(target)[0],\n\t\t\ttop, left, offset;\n\t\tif (!e.nodeType && e !== _win) {\n\t\t\tif (!_isUndefined(target.left)) {\n\t\t\t\toffset = {x:0, y:0}; //_getOffsetTransformOrigin(context); //the bounds should be relative to the origin\n\t\t\t\treturn {left: target.left - offset.x, top: target.top - offset.y, width: target.width, height: target.height};\n\t\t\t}\n\t\t\tleft = target.min || target.minX || target.minRotation || 0;\n\t\t\ttop = target.min || target.minY || 0;\n\t\t\treturn {left:left, top:top, width:(target.max || target.maxX || target.maxRotation || 0) - left, height:(target.max || target.maxY || 0) - top};\n\t\t}\n\t\treturn _getElementBounds(e, context);\n\t},\n\t_point1 = {}, //we reuse to minimize garbage collection tasks.\n\t_getElementBounds = (element, context) => {\n\t\tcontext = _toArray(context)[0];\n\t\tlet isSVG = (element.getBBox && element.ownerSVGElement),\n\t\t\tdoc = element.ownerDocument || _doc,\n\t\t\tleft, right, top, bottom, matrix, p1, p2, p3, p4, bbox, width, height, cs;\n\t\tif (element === _win) {\n\t\t\ttop = _getDocScrollTop(doc);\n\t\t\tleft = _getDocScrollLeft(doc);\n\t\t\tright = left + (doc.documentElement.clientWidth || element.innerWidth || doc.body.clientWidth || 0);\n\t\t\tbottom = top + (((element.innerHeight || 0) - 20 < doc.documentElement.clientHeight) ? doc.documentElement.clientHeight : element.innerHeight || doc.body.clientHeight || 0); //some browsers (like Firefox) ignore absolutely positioned elements, and collapse the height of the documentElement, so it could be 8px, for example, if you have just an absolutely positioned div. In that case, we use the innerHeight to resolve this.\n\t\t} else if (context === _win || _isUndefined(context)) {\n\t\t\treturn element.getBoundingClientRect();\n\t\t} else {\n\t\t\tleft = top = 0;\n\t\t\tif (isSVG) {\n\t\t\t\tbbox = element.getBBox();\n\t\t\t\twidth = bbox.width;\n\t\t\t\theight = bbox.height;\n\t\t\t} else {\n\t\t\t\tif (element.viewBox && (bbox = element.viewBox.baseVal)) {\n\t\t\t\t\tleft = bbox.x || 0;\n\t\t\t\t\ttop = bbox.y || 0;\n\t\t\t\t\twidth = bbox.width;\n\t\t\t\t\theight = bbox.height;\n\t\t\t\t}\n\t\t\t\tif (!width) {\n\t\t\t\t\tcs = _getComputedStyle(element);\n\t\t\t\t\tbbox = cs.boxSizing === \"border-box\";\n\t\t\t\t\twidth = (parseFloat(cs.width) || element.clientWidth || 0) + (bbox ? 0 : parseFloat(cs.borderLeftWidth) + parseFloat(cs.borderRightWidth));\n\t\t\t\t\theight = (parseFloat(cs.height) || element.clientHeight || 0) + (bbox ? 0 : parseFloat(cs.borderTopWidth) + parseFloat(cs.borderBottomWidth));\n\t\t\t\t}\n\t\t\t}\n\t\t\tright = width;\n\t\t\tbottom = height;\n\t\t}\n\t\tif (element === context) {\n\t\t\treturn {left:left, top:top, width: right - left, height: bottom - top};\n\t\t}\n\t\tmatrix = getGlobalMatrix(context, true).multiply(getGlobalMatrix(element));\n\t\tp1 = matrix.apply({x:left, y:top});\n\t\tp2 = matrix.apply({x:right, y:top});\n\t\tp3 = matrix.apply({x:right, y:bottom});\n\t\tp4 = matrix.apply({x:left, y:bottom});\n\t\tleft = Math.min(p1.x, p2.x, p3.x, p4.x);\n\t\ttop = Math.min(p1.y, p2.y, p3.y, p4.y);\n\t\treturn {left: left, top: top, width: Math.max(p1.x, p2.x, p3.x, p4.x) - left, height: Math.max(p1.y, p2.y, p3.y, p4.y) - top};\n\t},\n\t_parseInertia = (draggable, snap, max, min, factor, forceZeroVelocity) => {\n\t\tlet vars = {},\n\t\t\ta, i, l;\n\t\tif (snap) {\n\t\t\tif (factor !== 1 && snap instanceof Array) { //some data must be altered to make sense, like if the user passes in an array of rotational values in degrees, we must convert it to radians. Or for scrollLeft and scrollTop, we invert the values.\n\t\t\t\tvars.end = a = [];\n\t\t\t\tl = snap.length;\n\t\t\t\tif (_isObject(snap[0])) { //if the array is populated with objects, like points ({x:100, y:200}), make copies before multiplying by the factor, otherwise we'll mess up the originals and the user may reuse it elsewhere.\n\t\t\t\t\tfor (i = 0; i < l; i++) {\n\t\t\t\t\t\ta[i] = _copy(snap[i], factor);\n\t\t\t\t\t}\n\t\t\t\t} else {\n\t\t\t\t\tfor (i = 0; i < l; i++) {\n\t\t\t\t\t\ta[i] = snap[i] * factor;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tmax += 1.1; //allow 1.1 pixels of wiggle room when snapping in order to work around some browser inconsistencies in the way bounds are reported which can make them roughly a pixel off. For example, if \"snap:[-$('#menu').width(), 0]\" was defined and #menu had a wrapper that was used as the bounds, some browsers would be one pixel off, making the minimum -752 for example when snap was [-753,0], thus instead of snapping to -753, it would snap to 0 since -753 was below the minimum.\n\t\t\t\tmin -= 1.1;\n\t\t\t} else if (_isFunction(snap)) {\n\t\t\t\tvars.end = value => {\n\t\t\t\t\tlet result = snap.call(draggable, value),\n\t\t\t\t\t\tcopy, p;\n\t\t\t\t\tif (factor !== 1) {\n\t\t\t\t\t\tif (_isObject(result)) {\n\t\t\t\t\t\t\tcopy = {};\n\t\t\t\t\t\t\tfor (p in result) {\n\t\t\t\t\t\t\t\tcopy[p] = result[p] * factor;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tresult = copy;\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tresult *= factor;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\treturn result; //we need to ensure that we can scope the function call to the Draggable instance itself so that users can access important values like maxX, minX, maxY, minY, x, and y from within that function.\n\t\t\t\t};\n\t\t\t} else {\n\t\t\t\tvars.end = snap;\n\t\t\t}\n\t\t}\n\t\tif (max || max === 0) {\n\t\t\tvars.max = max;\n\t\t}\n\t\tif (min || min === 0) {\n\t\t\tvars.min = min;\n\t\t}\n\t\tif (forceZeroVelocity) {\n\t\t\tvars.velocity = 0;\n\t\t}\n\t\treturn vars;\n\t},\n\t_isClickable = element => { //sometimes it's convenient to mark an element as clickable by adding a data-clickable=\"true\" attribute (in which case we won't preventDefault() the mouse/touch event). This method checks if the element is an <a>, <input>, or <button> or has the data-clickable or contentEditable attribute set to true (or any of its parent elements).\n\t\tlet data;\n\t\treturn (!element || !element.getAttribute || element === _body) ? false : ((data = element.getAttribute(\"data-clickable\")) === \"true\" || (data !== \"false\" && (_clickableTagExp.test(element.nodeName + \"\") || element.getAttribute(\"contentEditable\") === \"true\"))) ? true : _isClickable(element.parentNode);\n\t},\n\t_setSelectable = (elements, selectable) => {\n\t\tlet i = elements.length,\n\t\t\te;\n\t\twhile (i--) {\n\t\t\te = elements[i];\n\t\t\te.ondragstart = e.onselectstart = selectable ? null : _emptyFunc;\n\t\t\tgsap.set(e, {lazy:true, userSelect: (selectable ? \"text\" : \"none\")});\n\t\t}\n\t},\n\t_isFixed = element => {\n\t\tif (_getComputedStyle(element).position === \"fixed\") {\n\t\t\treturn true;\n\t\t}\n\t\telement = element.parentNode;\n\t\tif (element && element.nodeType === 1) { // avoid document fragments which will throw an error.\n\t\t\treturn _isFixed(element);\n\t\t}\n\t},\n\t_supports3D, _addPaddingBR,\n\n\t//The ScrollProxy class wraps an element's contents into another div (we call it \"content\") that we either add padding when necessary or apply a translate3d() transform in order to overscroll (scroll past the boundaries). This allows us to simply set the scrollTop/scrollLeft (or top/left for easier reverse-axis orientation, which is what we do in Draggable) and it'll do all the work for us. For example, if we tried setting scrollTop to -100 on a normal DOM element, it wouldn't work - it'd look the same as setting it to 0, but if we set scrollTop of a ScrollProxy to -100, it'll give the correct appearance by either setting paddingTop of the wrapper to 100 or applying a 100-pixel translateY.\n\tScrollProxy = function(element, vars) {\n\t\telement = gsap.utils.toArray(element)[0];\n\t\tvars = vars || {};\n\t\tlet content = document.createElement(\"div\"),\n\t\t\tstyle = content.style,\n\t\t\tnode = element.firstChild,\n\t\t\toffsetTop = 0,\n\t\t\toffsetLeft = 0,\n\t\t\tprevTop = element.scrollTop,\n\t\t\tprevLeft = element.scrollLeft,\n\t\t\tscrollWidth = element.scrollWidth,\n\t\t\tscrollHeight = element.scrollHeight,\n\t\t\textraPadRight = 0,\n\t\t\tmaxLeft = 0,\n\t\t\tmaxTop = 0,\n\t\t\telementWidth, elementHeight, contentHeight, nextNode, transformStart, transformEnd;\n\t\tif (_supports3D && vars.force3D !== false) {\n\t\t\ttransformStart = \"translate3d(\";\n\t\t\ttransformEnd = \"px,0px)\";\n\t\t} else if (_transformProp) {\n\t\t\ttransformStart = \"translate(\";\n\t\t\ttransformEnd = \"px)\";\n\t\t}\n\t\tthis.scrollTop = function(value, force) {\n\t\t\tif (!arguments.length) {\n\t\t\t\treturn -this.top();\n\t\t\t}\n\t\t\tthis.top(-value, force);\n\t\t};\n\t\tthis.scrollLeft = function(value, force) {\n\t\t\tif (!arguments.length) {\n\t\t\t\treturn -this.left();\n\t\t\t}\n\t\t\tthis.left(-value, force);\n\t\t};\n\t\tthis.left = function(value, force) {\n\t\t\tif (!arguments.length) {\n\t\t\t\treturn -(element.scrollLeft + offsetLeft);\n\t\t\t}\n\t\t\tlet dif = element.scrollLeft - prevLeft,\n\t\t\t\toldOffset = offsetLeft;\n\t\t\tif ((dif > 2 || dif < -2) && !force) { //if the user interacts with the scrollbar (or something else scrolls it, like the mouse wheel), we should kill any tweens of the ScrollProxy.\n\t\t\t\tprevLeft = element.scrollLeft;\n\t\t\t\tgsap.killTweensOf(this, {left:1, scrollLeft:1});\n\t\t\t\tthis.left(-prevLeft);\n\t\t\t\tif (vars.onKill) {\n\t\t\t\t\tvars.onKill();\n\t\t\t\t}\n\t\t\t\treturn;\n\t\t\t}\n\t\t\tvalue = -value; //invert because scrolling works in the opposite direction\n\t\t\tif (value < 0) {\n\t\t\t\toffsetLeft = (value - 0.5) | 0;\n\t\t\t\tvalue = 0;\n\t\t\t} else if (value > maxLeft) {\n\t\t\t\toffsetLeft = (value - maxLeft) | 0;\n\t\t\t\tvalue = maxLeft;\n\t\t\t} else {\n\t\t\t\toffsetLeft = 0;\n\t\t\t}\n\t\t\tif (offsetLeft || oldOffset) {\n\t\t\t\tif (!this._skip) {\n\t\t\t\t\tstyle[_transformProp] = transformStart + -offsetLeft + \"px,\" + -offsetTop + transformEnd;\n\t\t\t\t}\n\t\t\t\tif (offsetLeft + extraPadRight >= 0) {\n\t\t\t\t\tstyle.paddingRight = offsetLeft + extraPadRight + \"px\";\n\t\t\t\t}\n\t\t\t}\n\t\t\telement.scrollLeft = value | 0;\n\t\t\tprevLeft = element.scrollLeft; //don't merge this with the line above because some browsers adjust the scrollLeft after it's set, so in order to be 100% accurate in tracking it, we need to ask the browser to report it.\n\t\t};\n\t\tthis.top = function(value, force) {\n\t\t\tif (!arguments.length) {\n\t\t\t\treturn -(element.scrollTop + offsetTop);\n\t\t\t}\n\t\t\tlet dif = element.scrollTop - prevTop,\n\t\t\t\toldOffset = offsetTop;\n\t\t\tif ((dif > 2 || dif < -2) && !force) { //if the user interacts with the scrollbar (or something else scrolls it, like the mouse wheel), we should kill any tweens of the ScrollProxy.\n\t\t\t\tprevTop = element.scrollTop;\n\t\t\t\tgsap.killTweensOf(this, {top:1, scrollTop:1});\n\t\t\t\tthis.top(-prevTop);\n\t\t\t\tif (vars.onKill) {\n\t\t\t\t\tvars.onKill();\n\t\t\t\t}\n\t\t\t\treturn;\n\t\t\t}\n\t\t\tvalue = -value; //invert because scrolling works in the opposite direction\n\t\t\tif (value < 0) {\n\t\t\t\toffsetTop = (value - 0.5) | 0;\n\t\t\t\tvalue = 0;\n\t\t\t} else if (value > maxTop) {\n\t\t\t\toffsetTop = (value - maxTop) | 0;\n\t\t\t\tvalue = maxTop;\n\t\t\t} else {\n\t\t\t\toffsetTop = 0;\n\t\t\t}\n\t\t\tif (offsetTop || oldOffset) {\n\t\t\t\tif (!this._skip) {\n\t\t\t\t\tstyle[_transformProp] = transformStart + -offsetLeft + \"px,\" + -offsetTop + transformEnd;\n\t\t\t\t}\n\t\t\t}\n\t\t\telement.scrollTop = value | 0;\n\t\t\tprevTop = element.scrollTop;\n\t\t};\n\n\t\tthis.maxScrollTop = () => maxTop;\n\t\tthis.maxScrollLeft = () => maxLeft;\n\n\t\tthis.disable = function() {\n\t\t\tnode = content.firstChild;\n\t\t\twhile (node) {\n\t\t\t\tnextNode = node.nextSibling;\n\t\t\t\telement.appendChild(node);\n\t\t\t\tnode = nextNode;\n\t\t\t}\n\t\t\tif (element === content.parentNode) { //in case disable() is called when it's already disabled.\n\t\t\t\telement.removeChild(content);\n\t\t\t}\n\t\t};\n\t\tthis.enable = function() {\n\t\t\tnode = element.firstChild;\n\t\t\tif (node === content) {\n\t\t\t\treturn;\n\t\t\t}\n\t\t\twhile (node) {\n\t\t\t\tnextNode = node.nextSibling;\n\t\t\t\tcontent.appendChild(node);\n\t\t\t\tnode = nextNode;\n\t\t\t}\n\t\t\telement.appendChild(content);\n\t\t\tthis.calibrate();\n\t\t};\n\t\tthis.calibrate = function(force) {\n\t\t\tlet widthMatches = (element.clientWidth === elementWidth),\n\t\t\t\tcs, x, y;\n\t\t\tprevTop = element.scrollTop;\n\t\t\tprevLeft = element.scrollLeft;\n\t\t\tif (widthMatches && element.clientHeight === elementHeight && content.offsetHeight === contentHeight && scrollWidth === element.scrollWidth && scrollHeight === element.scrollHeight && !force) {\n\t\t\t\treturn; //no need to recalculate things if the width and height haven't changed.\n\t\t\t}\n\t\t\tif (offsetTop || offsetLeft) {\n\t\t\t\tx = this.left();\n\t\t\t\ty = this.top();\n\t\t\t\tthis.left(-element.scrollLeft);\n\t\t\t\tthis.top(-element.scrollTop);\n\t\t\t}\n\t\t\tcs = _getComputedStyle(element);\n\t\t\t//first, we need to remove any width constraints to see how the content naturally flows so that we can see if it's wider than the containing element. If so, we've got to record the amount of overage so that we can apply that as padding in order for browsers to correctly handle things. Then we switch back to a width of 100% (without that, some browsers don't flow the content correctly)\n\t\t\tif (!widthMatches || force) {\n\t\t\t\tstyle.display = \"block\";\n\t\t\t\tstyle.width = \"auto\";\n\t\t\t\tstyle.paddingRight = \"0px\";\n\t\t\t\textraPadRight = Math.max(0, element.scrollWidth - element.clientWidth);\n\t\t\t\t//if the content is wider than the container, we need to add the paddingLeft and paddingRight in order for things to behave correctly.\n\t\t\t\tif (extraPadRight) {\n\t\t\t\t\textraPadRight += parseFloat(cs.paddingLeft) + (_addPaddingBR ? parseFloat(cs.paddingRight) : 0);\n\t\t\t\t}\n\t\t\t}\n\t\t\tstyle.display = \"inline-block\";\n\t\t\tstyle.position = \"relative\";\n\t\t\tstyle.overflow = \"visible\";\n\t\t\tstyle.verticalAlign = \"top\";\n\t\t\tstyle.boxSizing = \"content-box\";\n\t\t\tstyle.width = \"100%\";\n\t\t\tstyle.paddingRight = extraPadRight + \"px\";\n\t\t\t//some browsers neglect to factor in the bottom padding when calculating the scrollHeight, so we need to add that padding to the content when that happens. Allow a 2px margin for error\n\t\t\tif (_addPaddingBR) {\n\t\t\t\tstyle.paddingBottom = cs.paddingBottom;\n\t\t\t}\n\t\t\telementWidth = element.clientWidth;\n\t\t\telementHeight = element.clientHeight;\n\t\t\tscrollWidth = element.scrollWidth;\n\t\t\tscrollHeight = element.scrollHeight;\n\t\t\tmaxLeft = element.scrollWidth - elementWidth;\n\t\t\tmaxTop = element.scrollHeight - elementHeight;\n\t\t\tcontentHeight = content.offsetHeight;\n\t\t\tstyle.display = \"block\";\n\t\t\tif (x || y) {\n\t\t\t\tthis.left(x);\n\t\t\t\tthis.top(y);\n\t\t\t}\n\t\t};\n\t\tthis.content = content;\n\t\tthis.element = element;\n\t\tthis._skip = false;\n\t\tthis.enable();\n\t},\n\t_initCore = required => {\n\t\tif (_windowExists() && document.body) {\n\t\t\tlet nav = window && window.navigator;\n\t\t\t_win = window;\n\t\t\t_doc = document;\n\t\t\t_docElement = _doc.documentElement;\n\t\t\t_body = _doc.body;\n\t\t\t_tempDiv = _createElement(\"div\");\n\t\t\t_supportsPointer = !!window.PointerEvent;\n\t\t\t_placeholderDiv = _createElement(\"div\");\n\t\t\t_placeholderDiv.style.cssText = \"visibility:hidden;height:1px;top:-1px;pointer-events:none;position:relative;clear:both;cursor:grab\";\n\t\t\t_defaultCursor = _placeholderDiv.style.cursor === \"grab\" ? \"grab\" : \"move\";\n\t\t\t_isAndroid = (nav && nav.userAgent.toLowerCase().indexOf(\"android\") !== -1); //Android handles touch events in an odd way and it's virtually impossible to \"feature test\" so we resort to UA sniffing\n\t\t\t_isTouchDevice = ((\"ontouchstart\" in _docElement) && (\"orientation\" in _win)) || (nav && (nav.MaxTouchPoints > 0 || nav.msMaxTouchPoints > 0));\n\t\t\t_addPaddingBR = (function() { //this function is in charge of analyzing browser behavior related to padding. It sets the _addPaddingBR to true if the browser doesn't normally factor in the bottom or right padding on the element inside the scrolling area, and it sets _addPaddingLeft to true if it's a browser that requires the extra offset (offsetLeft) to be added to the paddingRight (like Opera).\n\t\t\t\tlet div = _createElement(\"div\"),\n\t\t\t\t\tchild = _createElement(\"div\"),\n\t\t\t\t\tchildStyle = child.style,\n\t\t\t\t\tparent = _body,\n\t\t\t\t\tval;\n\t\t\t\tchildStyle.display = \"inline-block\";\n\t\t\t\tchildStyle.position = \"relative\";\n\t\t\t\tdiv.style.cssText = \"width:90px;height:40px;padding:10px;overflow:auto;visibility:hidden\";\n\t\t\t\tdiv.appendChild(child);\n\t\t\t\tparent.appendChild(div);\n\t\t\t\tval = (child.offsetHeight + 18 > div.scrollHeight); //div.scrollHeight should be child.offsetHeight + 20 because of the 10px of padding on each side, but some browsers ignore one side. We allow a 2px margin of error.\n\t\t\t\tparent.removeChild(div);\n\t\t\t\treturn val;\n\t\t\t}());\n\t\t\t_touchEventLookup = (function(types) { //we create an object that makes it easy to translate touch event types into their \"pointer\" counterparts if we're in a browser that uses those instead. Like IE10 uses \"MSPointerDown\" instead of \"touchstart\", for example.\n\t\t\t\tlet standard = types.split(\",\"),\n\t\t\t\t\tconverted = (\"onpointerdown\" in _tempDiv ? \"pointerdown,pointermove,pointerup,pointercancel\" : \"onmspointerdown\" in _tempDiv ? \"MSPointerDown,MSPointerMove,MSPointerUp,MSPointerCancel\" : types).split(\",\"),\n\t\t\t\t\tobj = {},\n\t\t\t\t\ti = 4;\n\t\t\t\twhile (--i > -1) {\n\t\t\t\t\tobj[standard[i]] = converted[i];\n\t\t\t\t\tobj[converted[i]] = standard[i];\n\t\t\t\t}\n\t\t\t\t//to avoid problems in iOS 9, test to see if the browser supports the \"passive\" option on addEventListener().\n\t\t\t\ttry {\n\t\t\t\t\t_docElement.addEventListener(\"test\", null, Object.defineProperty({}, \"passive\", {\n\t\t\t\t\t\tget: function () {\n\t\t\t\t\t\t\t_supportsPassive = 1;\n\t\t\t\t\t\t}\n\t\t\t\t\t}));\n\t\t\t\t} catch (e) {}\n\t\t\t\treturn obj;\n\t\t\t}(\"touchstart,touchmove,touchend,touchcancel\"));\n\t\t\t_addListener(_doc, \"touchcancel\", _emptyFunc); //some older Android devices intermittently stop dispatching \"touchmove\" events if we don't listen for \"touchcancel\" on the document. Very strange indeed.\n\t\t\t_addListener(_win, \"touchmove\", _emptyFunc); //works around Safari bugs that still allow the page to scroll even when we preventDefault() on the touchmove event.\n\t\t\t_body && _body.addEventListener(\"touchstart\", _emptyFunc); //works around Safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/\n\t\t\t_addListener(_doc, \"contextmenu\", function() {\n\t\t\t\tfor (let p in _lookup) {\n\t\t\t\t\tif (_lookup[p].isPressed) {\n\t\t\t\t\t\t_lookup[p].endDrag();\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t});\n\t\t\tgsap = _coreInitted = _getGSAP();\n\t\t}\n\t\tif (gsap) {\n\t\t\tInertiaPlugin = gsap.plugins.inertia;\n\t\t\t_context = gsap.core.context || function() {};\n\t\t\t_checkPrefix = gsap.utils.checkPrefix;\n\t\t\t_transformProp = _checkPrefix(_transformProp);\n\t\t\t_transformOriginProp = _checkPrefix(_transformOriginProp);\n\t\t\t_toArray = gsap.utils.toArray;\n\t\t\t_getStyleSaver = gsap.core.getStyleSaver;\n\t\t\t_supports3D = !!_checkPrefix(\"perspective\");\n\t\t} else if (required) {\n\t\t\tconsole.warn(\"Please gsap.registerPlugin(Draggable)\");\n\t\t}\n\t};\n\n\n\n\n\n\nclass EventDispatcher {\n\n\tconstructor(target) {\n\t\tthis._listeners = {};\n\t\tthis.target = target || this;\n\t}\n\n\taddEventListener(type, callback) {\n\t\tlet list = this._listeners[type] || (this._listeners[type] = []);\n\t\tif (!~list.indexOf(callback)) {\n\t\t\tlist.push(callback);\n\t\t}\n\t}\n\n\tremoveEventListener(type, callback) {\n\t\tlet list = this._listeners[type],\n\t\t\ti = (list && list.indexOf(callback));\n\t\t(i >= 0) && list.splice(i, 1);\n\t}\n\n\tdispatchEvent(type) {\n\t\tlet result;\n\t\t(this._listeners[type] || []).forEach(callback => (callback.call(this, {type: type, target: this.target}) === false) && (result = false));\n\t\treturn result; //if any of the callbacks return false, pass that along.\n\t}\n}\n\n\n\n\n\n\n\n\n\nexport class Draggable extends EventDispatcher {\n\n\tconstructor(target, vars) {\n\t\tsuper();\n\t\t_coreInitted || _initCore(1);\n\t\ttarget = _toArray(target)[0]; //in case the target is a selector object or selector text\n\t\tthis.styles = _getStyleSaver && _getStyleSaver(target, \"transform,left,top\");\n\t\tif (!InertiaPlugin) {\n\t\t\tInertiaPlugin = gsap.plugins.inertia;\n\t\t}\n\t\tthis.vars = vars = _copy(vars || {});\n\t\tthis.target = target;\n\t\tthis.x = this.y = this.rotation = 0;\n\t\tthis.dragResistance = parseFloat(vars.dragResistance) || 0;\n\t\tthis.edgeResistance = isNaN(vars.edgeResistance) ? 1 : parseFloat(vars.edgeResistance) || 0;\n\t\tthis.lockAxis = vars.lockAxis;\n\t\tthis.autoScroll = vars.autoScroll || 0;\n\t\tthis.lockedAxis = null;\n\t\tthis.allowEventDefault = !!vars.allowEventDefault;\n\n\t\tgsap.getProperty(target, \"x\"); // to ensure that transforms are instantiated.\n\n\t\tlet type = (vars.type || \"x,y\").toLowerCase(),\n\t\t\txyMode = (~type.indexOf(\"x\") || ~type.indexOf(\"y\")),\n\t\t\trotationMode = (type.indexOf(\"rotation\") !== -1),\n\t\t\txProp = rotationMode ? \"rotation\" : xyMode ? \"x\" : \"left\",\n\t\t\tyProp = xyMode ? \"y\" : \"top\",\n\t\t\tallowX = !!(~type.indexOf(\"x\") || ~type.indexOf(\"left\") || type === \"scroll\"),\n\t\t\tallowY = !!(~type.indexOf(\"y\") || ~type.indexOf(\"top\") || type === \"scroll\"),\n\t\t\tminimumMovement = vars.minimumMovement || 2,\n\t\t\tself = this,\n\t\t\ttriggers = _toArray(vars.trigger || vars.handle || target),\n\t\t\tkillProps = {},\n\t\t\tdragEndTime = 0,\n\t\t\tcheckAutoScrollBounds = false,\n\t\t\tautoScrollMarginTop = vars.autoScrollMarginTop || 40,\n\t\t\tautoScrollMarginRight = vars.autoScrollMarginRight || 40,\n\t\t\tautoScrollMarginBottom = vars.autoScrollMarginBottom || 40,\n\t\t\tautoScrollMarginLeft = vars.autoScrollMarginLeft || 40,\n\t\t\tisClickable = vars.clickableTest || _isClickable,\n\t\t\tclickTime = 0,\n\t\t\tgsCache = target._gsap || gsap.core.getCache(target),\n\t\t\tisFixed = _isFixed(target),\n\t\t\tgetPropAsNum = (property, unit) => parseFloat(gsCache.get(target, property, unit)),\n\t\t\townerDoc = target.ownerDocument || _doc,\n\t\t\tenabled, scrollProxy, startPointerX, startPointerY, startElementX, startElementY, hasBounds, hasDragCallback, hasMoveCallback, maxX, minX, maxY, minY, touch, touchID, rotationOrigin, dirty, old, snapX, snapY, snapXY, isClicking, touchEventTarget, matrix, interrupted, allowNativeTouchScrolling, touchDragAxis, isDispatching, clickDispatch, trustedClickDispatch, isPreventingDefault, innerMatrix, dragged,\n\n\t\t\tonContextMenu = e => { //used to prevent long-touch from triggering a context menu.\n\t\t\t\t// (self.isPressed && e.which < 2) && self.endDrag() // previously ended drag when context menu was triggered, but instead we should just stop propagation and prevent the default event behavior.\n\t\t\t\t_preventDefault(e);\n\t\t\t\te.stopImmediatePropagation && e.stopImmediatePropagation();\n\t\t\t\treturn false;\n\t\t\t},\n\n\t\t\t//this method gets called on every tick of TweenLite.ticker which allows us to synchronize the renders to the core engine (which is typically synchronized with the display refresh via requestAnimationFrame). This is an optimization - it's better than applying the values inside the \"mousemove\" or \"touchmove\" event handler which may get called many times inbetween refreshes.\n\t\t\trender = suppressEvents => {\n\t\t\t\tif (self.autoScroll && self.isDragging && (checkAutoScrollBounds || dirty)) {\n\t\t\t\t\tlet e = target,\n\t\t\t\t\t\tautoScrollFactor = self.autoScroll * 15, //multiplying by 15 just gives us a better \"feel\" speed-wise.\n\t\t\t\t\t\tparent, isRoot, rect, pointerX, pointerY, changeX, changeY, gap;\n\t\t\t\t\tcheckAutoScrollBounds = false;\n\t\t\t\t\t_windowProxy.scrollTop = ((_win.pageYOffset != null) ? _win.pageYOffset : (ownerDoc.documentElement.scrollTop != null) ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop);\n\t\t\t\t\t_windowProxy.scrollLeft = ((_win.pageXOffset != null) ? _win.pageXOffset : (ownerDoc.documentElement.scrollLeft != null) ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft);\n\t\t\t\t\tpointerX = self.pointerX - _windowProxy.scrollLeft;\n\t\t\t\t\tpointerY = self.pointerY - _windowProxy.scrollTop;\n\t\t\t\t\twhile (e && !isRoot) { //walk up the chain and sense wherever the pointer is within 40px of an edge that's scrollable.\n\t\t\t\t\t\tisRoot = _isRoot(e.parentNode);\n\t\t\t\t\t\tparent = isRoot ? _windowProxy : e.parentNode;\n\t\t\t\t\t\trect = isRoot ? {bottom:Math.max(_docElement.clientHeight, _win.innerHeight || 0), right: Math.max(_docElement.clientWidth, _win.innerWidth || 0), left:0, top:0} : parent.getBoundingClientRect();\n\t\t\t\t\t\tchangeX = changeY = 0;\n\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\tgap = parent._gsMaxScrollY - parent.scrollTop;\n\t\t\t\t\t\t\tif (gap < 0) {\n\t\t\t\t\t\t\t\tchangeY = gap;\n\t\t\t\t\t\t\t} else if (pointerY > rect.bottom - autoScrollMarginBottom && gap) {\n\t\t\t\t\t\t\t\tcheckAutoScrollBounds = true;\n\t\t\t\t\t\t\t\tchangeY = Math.min(gap, (autoScrollFactor * (1 - Math.max(0, (rect.bottom - pointerY)) / autoScrollMarginBottom)) | 0);\n\t\t\t\t\t\t\t} else if (pointerY < rect.top + autoScrollMarginTop && parent.scrollTop) {\n\t\t\t\t\t\t\t\tcheckAutoScrollBounds = true;\n\t\t\t\t\t\t\t\tchangeY = -Math.min(parent.scrollTop, (autoScrollFactor * (1 - Math.max(0, (pointerY - rect.top)) / autoScrollMarginTop)) | 0);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (changeY) {\n\t\t\t\t\t\t\t\tparent.scrollTop += changeY;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\tgap = parent._gsMaxScrollX - parent.scrollLeft;\n\t\t\t\t\t\t\tif (gap < 0) {\n\t\t\t\t\t\t\t\tchangeX = gap;\n\t\t\t\t\t\t\t} else if (pointerX > rect.right - autoScrollMarginRight && gap) {\n\t\t\t\t\t\t\t\tcheckAutoScrollBounds = true;\n\t\t\t\t\t\t\t\tchangeX = Math.min(gap, (autoScrollFactor * (1 - Math.max(0, (rect.right - pointerX)) / autoScrollMarginRight)) | 0);\n\t\t\t\t\t\t\t} else if (pointerX < rect.left + autoScrollMarginLeft && parent.scrollLeft) {\n\t\t\t\t\t\t\t\tcheckAutoScrollBounds = true;\n\t\t\t\t\t\t\t\tchangeX = -Math.min(parent.scrollLeft, (autoScrollFactor * (1 - Math.max(0, (pointerX - rect.left)) / autoScrollMarginLeft)) | 0);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (changeX) {\n\t\t\t\t\t\t\t\tparent.scrollLeft += changeX;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\n\t\t\t\t\t\tif (isRoot && (changeX || changeY)) {\n\t\t\t\t\t\t\t_win.scrollTo(parent.scrollLeft, parent.scrollTop);\n\t\t\t\t\t\t\tsetPointerPosition(self.pointerX + changeX, self.pointerY + changeY);\n\t\t\t\t\t\t}\n\t\t\t\t\t\te = parent;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (dirty) {\n\t\t\t\t\tlet {x, y} = self;\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tself.deltaX = x - parseFloat(gsCache.rotation);\n\t\t\t\t\t\tself.rotation = x;\n\t\t\t\t\t\tgsCache.rotation = x + \"deg\";\n\t\t\t\t\t\tgsCache.renderTransform(1, gsCache);\n\t\t\t\t\t} else {\n\t\t\t\t\t\tif (scrollProxy) {\n\t\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\t\tself.deltaY = y - scrollProxy.top();\n\t\t\t\t\t\t\t\tscrollProxy.top(y);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\t\tself.deltaX = x - scrollProxy.left();\n\t\t\t\t\t\t\t\tscrollProxy.left(x);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t} else if (xyMode) {\n\t\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\t\tself.deltaY = y - parseFloat(gsCache.y);\n\t\t\t\t\t\t\t\tgsCache.y = y + \"px\";\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\t\tself.deltaX = x - parseFloat(gsCache.x);\n\t\t\t\t\t\t\t\tgsCache.x = x + \"px\";\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tgsCache.renderTransform(1, gsCache);\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\t\tself.deltaY = y - parseFloat(target.style.top || 0);\n\t\t\t\t\t\t\t\ttarget.style.top = y + \"px\";\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\t\tself.deltaX = x - parseFloat(target.style.left || 0);\n\t\t\t\t\t\t\t\ttarget.style.left = x + \"px\";\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (hasDragCallback && !suppressEvents && !isDispatching) {\n\t\t\t\t\t\tisDispatching = true; //in case onDrag has an update() call (avoid endless loop)\n\t\t\t\t\t\tif (_dispatchEvent(self, \"drag\", \"onDrag\") === false) {\n\t\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\t\tself.x -= self.deltaX;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\t\tself.y -= self.deltaY;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\trender(true);\n\t\t\t\t\t\t}\n\t\t\t\t\t\tisDispatching = false;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tdirty = false;\n\t\t\t},\n\n\t\t\t//copies the x/y from the element (whether that be transforms, top/left, or ScrollProxy's top/left) to the Draggable's x and y (and rotation if necessary) properties so that they reflect reality and it also (optionally) applies any snapping necessary. This is used by the InertiaPlugin tween in an onUpdate to ensure things are synced and snapped.\n\t\t\tsyncXY = (skipOnUpdate, skipSnap) => {\n\t\t\t\tlet { x, y } = self,\n\t\t\t\t\tsnappedValue, cs;\n\t\t\t\tif (!target._gsap) { //just in case the _gsap cache got wiped, like if the user called clearProps on the transform or something (very rare).\n\t\t\t\t\tgsCache = gsap.core.getCache(target);\n\t\t\t\t}\n\t\t\t\tgsCache.uncache && gsap.getProperty(target, \"x\"); // trigger a re-cache\n\t\t\t\tif (xyMode) {\n\t\t\t\t\tself.x = parseFloat(gsCache.x);\n\t\t\t\t\tself.y = parseFloat(gsCache.y);\n\t\t\t\t} else if (rotationMode) {\n\t\t\t\t\tself.x = self.rotation = parseFloat(gsCache.rotation);\n\t\t\t\t} else if (scrollProxy) {\n\t\t\t\t\tself.y = scrollProxy.top();\n\t\t\t\t\tself.x = scrollProxy.left();\n\t\t\t\t} else {\n\t\t\t\t\tself.y = parseFloat(target.style.top || ((cs = _getComputedStyle(target)) && cs.top)) || 0;\n\t\t\t\t\tself.x = parseFloat(target.style.left || (cs || {}).left) || 0;\n\t\t\t\t}\n\t\t\t\tif ((snapX || snapY || snapXY) && !skipSnap && (self.isDragging || self.isThrowing)) {\n\t\t\t\t\tif (snapXY) {\n\t\t\t\t\t\t_temp1.x = self.x;\n\t\t\t\t\t\t_temp1.y = self.y;\n\t\t\t\t\t\tsnappedValue = snapXY(_temp1);\n\t\t\t\t\t\tif (snappedValue.x !== self.x) {\n\t\t\t\t\t\t\tself.x = snappedValue.x;\n\t\t\t\t\t\t\tdirty = true;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (snappedValue.y !== self.y) {\n\t\t\t\t\t\t\tself.y = snappedValue.y;\n\t\t\t\t\t\t\tdirty = true;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (snapX) {\n\t\t\t\t\t\tsnappedValue = snapX(self.x);\n\t\t\t\t\t\tif (snappedValue !== self.x) {\n\t\t\t\t\t\t\tself.x = snappedValue;\n\t\t\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\t\t\tself.rotation = snappedValue;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tdirty = true;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (snapY) {\n\t\t\t\t\t\tsnappedValue = snapY(self.y);\n\t\t\t\t\t\tif (snappedValue !== self.y) {\n\t\t\t\t\t\t\tself.y = snappedValue;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tdirty = true;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tdirty && render(true);\n\t\t\t\tif (!skipOnUpdate) {\n\t\t\t\t\tself.deltaX = self.x - x;\n\t\t\t\t\tself.deltaY = self.y - y;\n\t\t\t\t\t_dispatchEvent(self, \"throwupdate\", \"onThrowUpdate\");\n\t\t\t\t}\n\t\t\t},\n\n\t\t\tbuildSnapFunc = (snap, min, max, factor) => {\n\t\t\t\tif (min == null) {\n\t\t\t\t\tmin = -_bigNum;\n\t\t\t\t}\n\t\t\t\tif (max == null) {\n\t\t\t\t\tmax = _bigNum;\n\t\t\t\t}\n\t\t\t\tif (_isFunction(snap)) {\n\t\t\t\t\treturn n => {\n\t\t\t\t\t\tlet edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance; //if we're tweening, disable the edgeTolerance because it's already factored into the tweening values (we don't want to apply it multiple times)\n\t\t\t\t\t\treturn snap.call(self, (n > max ? max + (n - max) * edgeTolerance : (n < min) ? min + (n - min) * edgeTolerance : n) * factor) * factor;\n\t\t\t\t\t};\n\t\t\t\t}\n\t\t\t\tif (_isArray(snap)) {\n\t\t\t\t\treturn n => {\n\t\t\t\t\t\tlet i = snap.length,\n\t\t\t\t\t\t\tclosest = 0,\n\t\t\t\t\t\t\tabsDif = _bigNum,\n\t\t\t\t\t\t\tval, dif;\n\t\t\t\t\t\twhile (--i > -1) {\n\t\t\t\t\t\t\tval = snap[i];\n\t\t\t\t\t\t\tdif = val - n;\n\t\t\t\t\t\t\tif (dif < 0) {\n\t\t\t\t\t\t\t\tdif = -dif;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (dif < absDif && val >= min && val <= max) {\n\t\t\t\t\t\t\t\tclosest = i;\n\t\t\t\t\t\t\t\tabsDif = dif;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\treturn snap[closest];\n\t\t\t\t\t};\n\t\t\t\t}\n\t\t\t\treturn isNaN(snap) ? n => n : () => snap * factor;\n\t\t\t},\n\n\t\t\tbuildPointSnapFunc = (snap, minX, maxX, minY, maxY, radius, factor) => {\n\t\t\t\tradius = (radius && radius < _bigNum) ? radius * radius : _bigNum; //so we don't have to Math.sqrt() in the functions. Performance optimization.\n\t\t\t\tif (_isFunction(snap)) {\n\t\t\t\t\treturn point => {\n\t\t\t\t\t\tlet edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance,\n\t\t\t\t\t\t\tx = point.x,\n\t\t\t\t\t\t\ty = point.y,\n\t\t\t\t\t\t\tresult, dx, dy; //if we're tweening, disable the edgeTolerance because it's already factored into the tweening values (we don't want to apply it multiple times)\n\t\t\t\t\t\tpoint.x = x = (x > maxX ? maxX + (x - maxX) * edgeTolerance : (x < minX) ? minX + (x - minX) * edgeTolerance : x);\n\t\t\t\t\t\tpoint.y = y = (y > maxY ? maxY + (y - maxY) * edgeTolerance : (y < minY) ? minY + (y - minY) * edgeTolerance : y);\n\t\t\t\t\t\tresult = snap.call(self, point);\n\t\t\t\t\t\tif (result !== point) {\n\t\t\t\t\t\t\tpoint.x = result.x;\n\t\t\t\t\t\t\tpoint.y = result.y;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (factor !== 1) {\n\t\t\t\t\t\t\tpoint.x *= factor;\n\t\t\t\t\t\t\tpoint.y *= factor;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (radius < _bigNum) {\n\t\t\t\t\t\t\tdx = point.x - x;\n\t\t\t\t\t\t\tdy = point.y - y;\n\t\t\t\t\t\t\tif (dx * dx + dy * dy > radius) {\n\t\t\t\t\t\t\t\tpoint.x = x;\n\t\t\t\t\t\t\t\tpoint.y = y;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\treturn point;\n\t\t\t\t\t};\n\t\t\t\t}\n\t\t\t\tif (_isArray(snap)) {\n\t\t\t\t\treturn p => {\n\t\t\t\t\t\tlet i = snap.length,\n\t\t\t\t\t\t\tclosest = 0,\n\t\t\t\t\t\t\tminDist = _bigNum,\n\t\t\t\t\t\t\tx, y, point, dist;\n\t\t\t\t\t\twhile (--i > -1) {\n\t\t\t\t\t\t\tpoint = snap[i];\n\t\t\t\t\t\t\tx = point.x - p.x;\n\t\t\t\t\t\t\ty = point.y - p.y;\n\t\t\t\t\t\t\tdist = x * x + y * y;\n\t\t\t\t\t\t\tif (dist < minDist) {\n\t\t\t\t\t\t\t\tclosest = i;\n\t\t\t\t\t\t\t\tminDist = dist;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\treturn (minDist <= radius) ? snap[closest] : p;\n\t\t\t\t\t};\n\t\t\t\t}\n\t\t\t\treturn n => n;\n\t\t\t},\n\n\t\t\tcalculateBounds = () => {\n\t\t\t\tlet bounds, targetBounds, snap, snapIsRaw;\n\t\t\t\thasBounds = false;\n\t\t\t\tif (scrollProxy) {\n\t\t\t\t\tscrollProxy.calibrate();\n\t\t\t\t\tself.minX = minX = -scrollProxy.maxScrollLeft();\n\t\t\t\t\tself.minY = minY = -scrollProxy.maxScrollTop();\n\t\t\t\t\tself.maxX = maxX = self.maxY = maxY = 0;\n\t\t\t\t\thasBounds = true;\n\t\t\t\t} else if (!!vars.bounds) {\n\t\t\t\t\tbounds = _getBounds(vars.bounds, target.parentNode); //could be a selector/jQuery object or a DOM element or a generic object like {top:0, left:100, width:1000, height:800} or {minX:100, maxX:1100, minY:0, maxY:800}\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tself.minX = minX = bounds.left;\n\t\t\t\t\t\tself.maxX = maxX = bounds.left + bounds.width;\n\t\t\t\t\t\tself.minY = minY = self.maxY = maxY = 0;\n\t\t\t\t\t} else if (!_isUndefined(vars.bounds.maxX) || !_isUndefined(vars.bounds.maxY)) {\n\t\t\t\t\t\tbounds = vars.bounds;\n\t\t\t\t\t\tself.minX = minX = bounds.minX;\n\t\t\t\t\t\tself.minY = minY = bounds.minY;\n\t\t\t\t\t\tself.maxX = maxX = bounds.maxX;\n\t\t\t\t\t\tself.maxY = maxY = bounds.maxY;\n\t\t\t\t\t} else {\n\t\t\t\t\t\ttargetBounds = _getBounds(target, target.parentNode);\n\t\t\t\t\t\tself.minX = minX = Math.round(getPropAsNum(xProp, \"px\") + bounds.left - targetBounds.left);\n\t\t\t\t\t\tself.minY = minY = Math.round(getPropAsNum(yProp, \"px\") + bounds.top - targetBounds.top);\n\t\t\t\t\t\tself.maxX = maxX = Math.round(minX + (bounds.width - targetBounds.width));\n\t\t\t\t\t\tself.maxY = maxY = Math.round(minY + (bounds.height - targetBounds.height));\n\t\t\t\t\t}\n\t\t\t\t\tif (minX > maxX) {\n\t\t\t\t\t\tself.minX = maxX;\n\t\t\t\t\t\tself.maxX = maxX = minX;\n\t\t\t\t\t\tminX = self.minX;\n\t\t\t\t\t}\n\t\t\t\t\tif (minY > maxY) {\n\t\t\t\t\t\tself.minY = maxY;\n\t\t\t\t\t\tself.maxY = maxY = minY;\n\t\t\t\t\t\tminY = self.minY;\n\t\t\t\t\t}\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tself.minRotation = minX;\n\t\t\t\t\t\tself.maxRotation = maxX;\n\t\t\t\t\t}\n\t\t\t\t\thasBounds = true;\n\t\t\t\t}\n\t\t\t\tif (vars.liveSnap) {\n\t\t\t\t\tsnap = (vars.liveSnap === true) ? (vars.snap || {}) : vars.liveSnap;\n\t\t\t\t\tsnapIsRaw = (_isArray(snap) || _isFunction(snap));\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tsnapX = buildSnapFunc((snapIsRaw ? snap : snap.rotation), minX, maxX, 1);\n\t\t\t\t\t\tsnapY = null;\n\t\t\t\t\t} else {\n\t\t\t\t\t\tif (snap.points) {\n\t\t\t\t\t\t\tsnapXY = buildPointSnapFunc((snapIsRaw ? snap : snap.points), minX, maxX, minY, maxY, snap.radius, scrollProxy ? -1 : 1);\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\t\tsnapX = buildSnapFunc((snapIsRaw ? snap : snap.x || snap.left || snap.scrollLeft), minX, maxX, scrollProxy ? -1 : 1);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\t\tsnapY = buildSnapFunc((snapIsRaw ? snap : snap.y || snap.top || snap.scrollTop), minY, maxY, scrollProxy ? -1 : 1);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t},\n\n\t\t\tonThrowComplete = () => {\n\t\t\t\tself.isThrowing = false;\n\t\t\t\t_dispatchEvent(self, \"throwcomplete\", \"onThrowComplete\");\n\t\t\t},\n\t\t\tonThrowInterrupt = () => {\n\t\t\t\tself.isThrowing = false;\n\t\t\t},\n\n\t\t\tanimate = (inertia, forceZeroVelocity) => {\n\t\t\t\tlet snap, snapIsRaw, tween, overshootTolerance;\n\t\t\t\tif (inertia && InertiaPlugin) {\n\t\t\t\t\tif (inertia === true) {\n\t\t\t\t\t\tsnap = vars.snap || vars.liveSnap || {};\n\t\t\t\t\t\tsnapIsRaw = (_isArray(snap) || _isFunction(snap));\n\t\t\t\t\t\tinertia = {resistance:(vars.throwResistance || vars.resistance || 1000) / (rotationMode ? 10 : 1)};\n\t\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\t\tinertia.rotation = _parseInertia(self, snapIsRaw ? snap : snap.rotation, maxX, minX, 1, forceZeroVelocity);\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\t\tinertia[xProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.x || snap.left, maxX, minX, scrollProxy ? -1 : 1, forceZeroVelocity || (self.lockedAxis === \"x\"));\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\t\tinertia[yProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.y || snap.top, maxY, minY, scrollProxy ? -1 : 1, forceZeroVelocity || (self.lockedAxis === \"y\"));\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (snap.points || (_isArray(snap) && _isObject(snap[0]))) {\n\t\t\t\t\t\t\t\tinertia.linkedProps = xProp + \",\" + yProp;\n\t\t\t\t\t\t\t\tinertia.radius = snap.radius; //note: we also disable liveSnapping while throwing if there's a \"radius\" defined, otherwise it looks weird to have the item thrown past a snapping point but live-snapping mid-tween. We do this by altering the onUpdateParams so that \"skipSnap\" parameter is true for syncXY.\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tself.isThrowing = true;\n\t\t\t\t\tovershootTolerance = (!isNaN(vars.overshootTolerance)) ? vars.overshootTolerance : (vars.edgeResistance === 1) ? 0 : (1 - self.edgeResistance) + 0.2;\n\t\t\t\t\tif (!inertia.duration) {\n\t\t\t\t\t\tinertia.duration = {max: Math.max(vars.minDuration || 0, (\"maxDuration\" in vars) ? vars.maxDuration : 2), min: (!isNaN(vars.minDuration) ? vars.minDuration : (overshootTolerance === 0 || (_isObject(inertia) && inertia.resistance > 1000)) ? 0 : 0.5), overshoot: overshootTolerance};\n\t\t\t\t\t}\n\t\t\t\t\tself.tween = tween = gsap.to(scrollProxy || target, {\n\t\t\t\t\t\tinertia: inertia,\n\t\t\t\t\t\tdata: \"_draggable\",\n\t\t\t\t\t\tinherit: false,\n\t\t\t\t\t\tonComplete: onThrowComplete,\n\t\t\t\t\t\tonInterrupt: onThrowInterrupt,\n\t\t\t\t\t\tonUpdate: (vars.fastMode ? _dispatchEvent : syncXY),\n\t\t\t\t\t\tonUpdateParams: (vars.fastMode ? [self, \"onthrowupdate\", \"onThrowUpdate\"] : (snap && snap.radius) ? [false, true] : [])\n\t\t\t\t\t});\n\t\t\t\t\tif (!vars.fastMode) {\n\t\t\t\t\t\tif (scrollProxy) {\n\t\t\t\t\t\t\tscrollProxy._skip = true; // Microsoft browsers have a bug that causes them to briefly render the position incorrectly (it flashes to the end state when we seek() the tween even though we jump right back to the current position, and this only seems to happen when we're affecting both top and left), so we set a _suspendTransforms flag to prevent it from actually applying the values in the ScrollProxy.\n\t\t\t\t\t\t}\n\t\t\t\t\t\ttween.render(1e9, true, true); // force to the end. Remember, the duration will likely change upon initting because that's when InertiaPlugin calculates it.\n\t\t\t\t\t\tsyncXY(true, true);\n\t\t\t\t\t\tself.endX = self.x;\n\t\t\t\t\t\tself.endY = self.y;\n\t\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\t\tself.endRotation = self.x;\n\t\t\t\t\t\t}\n\t\t\t\t\t\ttween.play(0);\n\t\t\t\t\t\tsyncXY(true, true);\n\t\t\t\t\t\tif (scrollProxy) {\n\t\t\t\t\t\t\tscrollProxy._skip = false; //Microsoft browsers have a bug that causes them to briefly render the position incorrectly (it flashes to the end state when we seek() the tween even though we jump right back to the current position, and this only seems to happen when we're affecting both top and left), so we set a _suspendTransforms flag to prevent it from actually applying the values in the ScrollProxy.\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t} else if (hasBounds) {\n\t\t\t\t\tself.applyBounds();\n\t\t\t\t}\n\t\t\t},\n\n\t\t\tupdateMatrix = shiftStart => {\n\t\t\t\tlet start = matrix,\n\t\t\t\t\tp;\n\t\t\t\tmatrix = getGlobalMatrix(target.parentNode, true);\n\t\t\t\tif (shiftStart && self.isPressed && !matrix.equals(start || new Matrix2D())) { //if the matrix changes WHILE the element is pressed, we must adjust the startPointerX and startPointerY accordingly, so we invert the original matrix and figure out where the pointerX and pointerY were in the global space, then apply the new matrix to get the updated coordinates.\n\t\t\t\t\tp = start.inverse().apply({x:startPointerX, y:startPointerY});\n\t\t\t\t\tmatrix.apply(p, p);\n\t\t\t\t\tstartPointerX = p.x;\n\t\t\t\t\tstartPointerY = p.y;\n\t\t\t\t}\n\t\t\t\tif (matrix.equals(_identityMatrix)) { //if there are no transforms, we can optimize performance by not factoring in the matrix\n\t\t\t\t\tmatrix = null;\n\t\t\t\t}\n\t\t\t},\n\n\t\t\trecordStartPositions = () => {\n\t\t\t\tlet edgeTolerance = 1 - self.edgeResistance,\n\t\t\t\t\toffsetX = isFixed ? _getDocScrollLeft(ownerDoc) : 0,\n\t\t\t\t\toffsetY = isFixed ? _getDocScrollTop(ownerDoc) : 0,\n\t\t\t\t\tparsedOrigin, x, y;\n\t\t\t\tif (xyMode) { // in case the user set it as a different unit, like animating the x to \"100%\". We must convert it back to px!\n\t\t\t\t\tgsCache.x = getPropAsNum(xProp, \"px\") + \"px\";\n\t\t\t\t\tgsCache.y = getPropAsNum(yProp, \"px\") + \"px\";\n\t\t\t\t\tgsCache.renderTransform();\n\t\t\t\t}\n\t\t\t\tupdateMatrix(false);\n\t\t\t\t_point1.x = self.pointerX - offsetX;\n\t\t\t\t_point1.y = self.pointerY - offsetY;\n\t\t\t\tmatrix && matrix.apply(_point1, _point1);\n\t\t\t\tstartPointerX = _point1.x; //translate to local coordinate system\n\t\t\t\tstartPointerY = _point1.y;\n\t\t\t\tif (dirty) {\n\t\t\t\t\tsetPointerPosition(self.pointerX, self.pointerY);\n\t\t\t\t\trender(true);\n\t\t\t\t}\n\t\t\t\tinnerMatrix = getGlobalMatrix(target);\n\t\t\t\tif (scrollProxy) {\n\t\t\t\t\tcalculateBounds();\n\t\t\t\t\tstartElementY = scrollProxy.top();\n\t\t\t\t\tstartElementX = scrollProxy.left();\n\t\t\t\t} else {\n\t\t\t\t\t//if the element is in the process of tweening, don't force snapping to occur because it could make it jump. Imagine the user throwing, then before it's done, clicking on the element in its inbetween state.\n\t\t\t\t\tif (isTweening()) {\n\t\t\t\t\t\tsyncXY(true, true);\n\t\t\t\t\t\tcalculateBounds();\n\t\t\t\t\t} else {\n\t\t\t\t\t\tself.applyBounds();\n\t\t\t\t\t}\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tparsedOrigin = target.ownerSVGElement ? [gsCache.xOrigin - target.getBBox().x, gsCache.yOrigin - target.getBBox().y] : (_getComputedStyle(target)[_transformOriginProp] || \"0 0\").split(\" \");\n\t\t\t\t\t\trotationOrigin = self.rotationOrigin = getGlobalMatrix(target).apply({x: parseFloat(parsedOrigin[0]) || 0, y: parseFloat(parsedOrigin[1]) || 0});\n\t\t\t\t\t\tsyncXY(true, true);\n\t\t\t\t\t\tx = self.pointerX - rotationOrigin.x - offsetX;\n\t\t\t\t\t\ty = rotationOrigin.y - self.pointerY + offsetY;\n\t\t\t\t\t\tstartElementX = self.x; //starting rotation (x always refers to rotation in type:\"rotation\", measured in degrees)\n\t\t\t\t\t\tstartElementY = self.y = Math.atan2(y, x) * _RAD2DEG;\n\t\t\t\t\t} else {\n\t\t\t\t\t\t//parent = !isFixed && target.parentNode;\n\t\t\t\t\t\t//startScrollTop = parent ? parent.scrollTop || 0 : 0;\n\t\t\t\t\t\t//startScrollLeft = parent ? parent.scrollLeft || 0 : 0;\n\t\t\t\t\t\tstartElementY = getPropAsNum(yProp, \"px\"); //record the starting top and left values so that we can just add the mouse's movement to them later.\n\t\t\t\t\t\tstartElementX = getPropAsNum(xProp, \"px\");\n\t\t\t\t\t}\n\t\t\t\t}\n\n\t\t\t\tif (hasBounds && edgeTolerance) {\n\t\t\t\t\tif (startElementX > maxX) {\n\t\t\t\t\t\tstartElementX = maxX + (startElementX - maxX) / edgeTolerance;\n\t\t\t\t\t} else if (startElementX < minX) {\n\t\t\t\t\t\tstartElementX = minX - (minX - startElementX) / edgeTolerance;\n\t\t\t\t\t}\n\t\t\t\t\tif (!rotationMode) {\n\t\t\t\t\t\tif (startElementY > maxY) {\n\t\t\t\t\t\t\tstartElementY = maxY + (startElementY - maxY) / edgeTolerance;\n\t\t\t\t\t\t} else if (startElementY < minY) {\n\t\t\t\t\t\t\tstartElementY = minY - (minY - startElementY) / edgeTolerance;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tself.startX = startElementX = _round(startElementX);\n\t\t\t\tself.startY = startElementY = _round(startElementY);\n\t\t\t},\n\n\t\t\tisTweening = () => self.tween && self.tween.isActive(),\n\n\t\t\tremovePlaceholder = () => {\n\t\t\t\tif (_placeholderDiv.parentNode && !isTweening() && !self.isDragging) { //_placeholderDiv just props open auto-scrolling containers so they don't collapse as the user drags left/up. We remove it after dragging (and throwing, if necessary) finishes.\n\t\t\t\t\t_placeholderDiv.parentNode.removeChild(_placeholderDiv);\n\t\t\t\t}\n\t\t\t},\n\n\t\t\t//called when the mouse is pressed (or touch starts)\n\t\t\tonPress = (e, force) => {\n\t\t\t\tlet i;\n\t\t\t\tif (!enabled || self.isPressed || !e || ((e.type === \"mousedown\" || e.type === \"pointerdown\") && !force && _getTime() - clickTime < 30 && _touchEventLookup[self.pointerEvent.type])) { //when we DON'T preventDefault() in order to accommodate touch-scrolling and the user just taps, many browsers also fire a mousedown/mouseup sequence AFTER the touchstart/touchend sequence, thus it'd result in two quick \"click\" events being dispatched. This line senses that condition and halts it on the subsequent mousedown.\n\t\t\t\t\tisPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchstart, pointerdown, mousedown. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary.\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\tinterrupted = isTweening();\n\t\t\t\tdragged = false; // we need to track whether or not it was dragged in this interaction so that if, for example, the user calls .endDrag() to FORCE it to stop and then they keep the mouse pressed down and eventually release, that would normally cause an onClick but we have to skip it in that case if there was dragging that occurred.\n\t\t\t\tself.pointerEvent = e;\n\t\t\t\tif (_touchEventLookup[e.type]) { //note: on iOS, BOTH touchmove and mousemove are dispatched, but the mousemove has pageY and pageX of 0 which would mess up the calculations and needlessly hurt performance.\n\t\t\t\t\ttouchEventTarget = ~e.type.indexOf(\"touch\") ? (e.currentTarget || e.target) : ownerDoc; //pointer-based touches (for Microsoft browsers) don't remain locked to the original target like other browsers, so we must use the document instead. The event type would be \"MSPointerDown\" or \"pointerdown\".\n\t\t\t\t\t_addListener(touchEventTarget, \"touchend\", onRelease);\n\t\t\t\t\t_addListener(touchEventTarget, \"touchmove\", onMove); // possible future change if PointerEvents are more standardized: https://developer.mozilla.org/en-US/docs/Web/API/Element/setPointerCapture\n\t\t\t\t\t_addListener(touchEventTarget, \"touchcancel\", onRelease);\n\t\t\t\t\t_addListener(ownerDoc, \"touchstart\", _onMultiTouchDocument);\n\t\t\t\t} else {\n\t\t\t\t\ttouchEventTarget = null;\n\t\t\t\t\t_addListener(ownerDoc, \"mousemove\", onMove); //attach these to the document instead of the box itself so that if the user's mouse moves too quickly (and off of the box), things still work.\n\t\t\t\t}\n\t\t\t\ttouchDragAxis = null;\n\t\t\t\tif (!_supportsPointer || !touchEventTarget) {\n\t\t\t\t\t_addListener(ownerDoc, \"mouseup\", onRelease);\n\t\t\t\t\te && e.target && _addListener(e.target, \"mouseup\", onRelease); //we also have to listen directly on the element because some browsers don't bubble up the event to the _doc on elements with contentEditable=\"true\"\n\t\t\t\t}\n\t\t\t\tisClicking = (isClickable.call(self, e.target) && vars.dragClickables === false && !force);\n\t\t\t\tif (isClicking) {\n\t\t\t\t\t_addListener(e.target, \"change\", onRelease); //in some browsers, when you mousedown on a <select> element, no mouseup gets dispatched! So we listen for a \"change\" event instead.\n\t\t\t\t\t_dispatchEvent(self, \"pressInit\", \"onPressInit\");\n\t\t\t\t\t_dispatchEvent(self, \"press\", \"onPress\");\n\t\t\t\t\t_setSelectable(triggers, true); //accommodates things like inputs and elements with contentEditable=\"true\" (otherwise user couldn't drag to select text)\n\t\t\t\t\tisPreventingDefault = false;\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\tallowNativeTouchScrolling = (!touchEventTarget || allowX === allowY || self.vars.allowNativeTouchScrolling === false || (self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2))) ? false : allowX ? \"y\" : \"x\"; //note: in Chrome, right-clicking (for a context menu) fires onPress and it doesn't have the event.which set properly, so we must look for event.ctrlKey. If the user wants to allow context menus we should of course sense it here and not allow native touch scrolling.\n\t\t\t\tisPreventingDefault = !allowNativeTouchScrolling && !self.allowEventDefault;\n\t\t\t\tif (isPreventingDefault) {\n\t\t\t\t\t_preventDefault(e);\n\t\t\t\t\t_addListener(_win, \"touchforcechange\", _preventDefault); //works around safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/\n\t\t\t\t}\n\t\t\t\tif (e.changedTouches) { //touch events store the data slightly differently\n\t\t\t\t\te = touch = e.changedTouches[0];\n\t\t\t\t\ttouchID = e.identifier;\n\t\t\t\t} else if (e.pointerId) {\n\t\t\t\t\ttouchID = e.pointerId; //for some Microsoft browsers\n\t\t\t\t} else {\n\t\t\t\t\ttouch = touchID = null;\n\t\t\t\t}\n\t\t\t\t_dragCount++;\n\t\t\t\t_addToRenderQueue(render); //causes the Draggable to render on each \"tick\" of gsap.ticker (performance optimization - updating values in a mousemove can cause them to happen too frequently, like multiple times between frame redraws which is wasteful, and it also prevents values from updating properly in IE8)\n\t\t\t\tstartPointerY = self.pointerY = e.pageY; //record the starting x and y so that we can calculate the movement from the original in _onMouseMove\n\t\t\t\tstartPointerX = self.pointerX = e.pageX;\n\t\t\t\t_dispatchEvent(self, \"pressInit\", \"onPressInit\");\n\t\t\t\tif (allowNativeTouchScrolling || self.autoScroll) {\n\t\t\t\t\t_recordMaxScrolls(target.parentNode);\n\t\t\t\t}\n\t\t\t\tif (target.parentNode && self.autoScroll && !scrollProxy && !rotationMode && target.parentNode._gsMaxScrollX && !_placeholderDiv.parentNode && !target.getBBox) { //add a placeholder div to prevent the parent container from collapsing when the user drags the element left.\n\t\t\t\t\t_placeholderDiv.style.width = target.parentNode.scrollWidth + \"px\";\n\t\t\t\t\ttarget.parentNode.appendChild(_placeholderDiv);\n\t\t\t\t}\n\t\t\t\trecordStartPositions();\n\t\t\t\tself.tween && self.tween.kill();\n\t\t\t\tself.isThrowing = false;\n\t\t\t\tgsap.killTweensOf(scrollProxy || target, killProps, true); //in case the user tries to drag it before the last tween is done.\n\t\t\t\tscrollProxy && gsap.killTweensOf(target, {scrollTo:1}, true); //just in case the original target's scroll position is being tweened somewhere else.\n\t\t\t\tself.tween = self.lockedAxis = null;\n\t\t\t\tif (vars.zIndexBoost || (!rotationMode && !scrollProxy && vars.zIndexBoost !== false)) {\n\t\t\t\t\ttarget.style.zIndex = Draggable.zIndex++;\n\t\t\t\t}\n\t\t\t\tself.isPressed = true;\n\t\t\t\thasDragCallback = !!(vars.onDrag || self._listeners.drag);\n\t\t\t\thasMoveCallback = !!(vars.onMove || self._listeners.move);\n\t\t\t\tif (vars.cursor !== false || vars.activeCursor) {\n\t\t\t\t\ti = triggers.length;\n\t\t\t\t\twhile (--i > -1) {\n\t\t\t\t\t\tgsap.set(triggers[i], {cursor: vars.activeCursor || vars.cursor || (_defaultCursor === \"grab\" ? \"grabbing\" : _defaultCursor)});\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\t_dispatchEvent(self, \"press\", \"onPress\");\n\t\t\t},\n\n\t\t\t//called every time the mouse/touch moves\n\t\t\tonMove = e => {\n\t\t\t\tlet originalEvent = e,\n\t\t\t\t\ttouches, pointerX, pointerY, i, dx, dy;\n\t\t\t\tif (!enabled || _isMultiTouching || !self.isPressed || !e) {\n\t\t\t\t\tisPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchmove, pointermove, mousemove. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary.\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\tself.pointerEvent = e;\n\t\t\t\ttouches = e.changedTouches;\n\t\t\t\tif (touches) { //touch events store the data slightly differently\n\t\t\t\t\te = touches[0];\n\t\t\t\t\tif (e !== touch && e.identifier !== touchID) { //Usually changedTouches[0] will be what we're looking for, but in case it's not, look through the rest of the array...(and Android browsers don't reuse the event like iOS)\n\t\t\t\t\t\ti = touches.length;\n\t\t\t\t\t\twhile (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} // Some Android devices dispatch a touchstart AND pointerdown initially, and then only pointermove thus the touchID may not match because it was grabbed from the touchstart event whereas the pointer event is the one that the browser dispatches for move, so if the event target matches this Draggable's target, let it through.\n\t\t\t\t\t\tif (i < 0) {\n\t\t\t\t\t\t\treturn;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t} else if (e.pointerId && touchID && e.pointerId !== touchID) { //for some Microsoft browsers, we must attach the listener to the doc rather than the trigger so that when the finger moves outside the bounds of the trigger, things still work. So if the event we're receiving has a pointerId that doesn't match the touchID, ignore it (for multi-touch)\n\t\t\t\t\treturn;\n\t\t\t\t}\n\n\t\t\t\tif (touchEventTarget && allowNativeTouchScrolling && !touchDragAxis) { //Android browsers force us to decide on the first \"touchmove\" event if we should allow the default (scrolling) behavior or preventDefault(). Otherwise, a \"touchcancel\" will be fired and then no \"touchmove\" or \"touchend\" will fire during the scrolling (no good).\n\t\t\t\t\t_point1.x = e.pageX - (isFixed ? _getDocScrollLeft(ownerDoc) : 0);\n\t\t\t\t\t_point1.y = e.pageY - (isFixed ? _getDocScrollTop(ownerDoc) : 0);\n\t\t\t\t\tmatrix && matrix.apply(_point1, _point1);\n\t\t\t\t\tpointerX = _point1.x;\n\t\t\t\t\tpointerY = _point1.y;\n\t\t\t\t\tdx = Math.abs(pointerX - startPointerX);\n\t\t\t\t\tdy = Math.abs(pointerY - startPointerY);\n\t\t\t\t\tif ((dx !== dy && (dx > minimumMovement || dy > minimumMovement)) || (_isAndroid && allowNativeTouchScrolling === touchDragAxis)) {\n\t\t\t\t\t\ttouchDragAxis = (dx > dy && allowX) ? \"x\" : \"y\";\n\t\t\t\t\t\tif (allowNativeTouchScrolling && touchDragAxis !== allowNativeTouchScrolling) {\n\t\t\t\t\t\t\t_addListener(_win, \"touchforcechange\", _preventDefault); // prevents native touch scrolling from taking over if the user started dragging in the other direction in iOS Safari\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (self.vars.lockAxisOnTouchScroll !== false && allowX && allowY) {\n\t\t\t\t\t\t\tself.lockedAxis = (touchDragAxis === \"x\") ? \"y\" : \"x\";\n\t\t\t\t\t\t\t_isFunction(self.vars.onLockAxis) && self.vars.onLockAxis.call(self, originalEvent);\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (_isAndroid && allowNativeTouchScrolling === touchDragAxis) {\n\t\t\t\t\t\t\tonRelease(originalEvent);\n\t\t\t\t\t\t\treturn;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (!self.allowEventDefault && (!allowNativeTouchScrolling || (touchDragAxis && allowNativeTouchScrolling !== touchDragAxis)) && originalEvent.cancelable !== false) {\n\t\t\t\t\t_preventDefault(originalEvent);\n\t\t\t\t\tisPreventingDefault = true;\n\t\t\t\t} else if (isPreventingDefault) {\n\t\t\t\t\tisPreventingDefault = false;\n\t\t\t\t}\n\n\t\t\t\tif (self.autoScroll) {\n\t\t\t\t\tcheckAutoScrollBounds = true;\n\t\t\t\t}\n\t\t\t\tsetPointerPosition(e.pageX, e.pageY, hasMoveCallback);\n\t\t\t},\n\n\t\t\tsetPointerPosition = (pointerX, pointerY, invokeOnMove) => {\n\t\t\t\tlet dragTolerance = 1 - self.dragResistance,\n\t\t\t\t\tedgeTolerance = 1 - self.edgeResistance,\n\t\t\t\t\tprevPointerX = self.pointerX,\n\t\t\t\t\tprevPointerY = self.pointerY,\n\t\t\t\t\tprevStartElementY = startElementY,\n\t\t\t\t\tprevX = self.x,\n\t\t\t\t\tprevY = self.y,\n\t\t\t\t\tprevEndX = self.endX,\n\t\t\t\t\tprevEndY = self.endY,\n\t\t\t\t\tprevEndRotation = self.endRotation,\n\t\t\t\t\tprevDirty = dirty,\n\t\t\t\t\txChange, yChange, x, y, dif, temp;\n\t\t\t\tself.pointerX = pointerX;\n\t\t\t\tself.pointerY = pointerY;\n\t\t\t\tif (isFixed) {\n\t\t\t\t\tpointerX -= _getDocScrollLeft(ownerDoc);\n\t\t\t\t\tpointerY -= _getDocScrollTop(ownerDoc);\n\t\t\t\t}\n\t\t\t\tif (rotationMode) {\n\t\t\t\t\ty = Math.atan2(rotationOrigin.y - pointerY, pointerX - rotationOrigin.x) * _RAD2DEG;\n\t\t\t\t\tdif = self.y - y;\n\t\t\t\t\tif (dif > 180) {\n\t\t\t\t\t\tstartElementY -= 360;\n\t\t\t\t\t\tself.y = y;\n\t\t\t\t\t} else if (dif < -180) {\n\t\t\t\t\t\tstartElementY += 360;\n\t\t\t\t\t\tself.y = y;\n\t\t\t\t\t}\n\t\t\t\t\tif (self.x !== startElementX || Math.max(Math.abs(startPointerX - pointerX), Math.abs(startPointerY - pointerY)) > minimumMovement) {\n\t\t\t\t\t\tself.y = y;\n\t\t\t\t\t\tx = startElementX + (startElementY - y) * dragTolerance;\n\t\t\t\t\t} else {\n\t\t\t\t\t\tx = startElementX;\n\t\t\t\t\t}\n\n\t\t\t\t} else {\n\t\t\t\t\tif (matrix) {\n\t\t\t\t\t\ttemp = pointerX * matrix.a + pointerY * matrix.c + matrix.e;\n\t\t\t\t\t\tpointerY = pointerX * matrix.b + pointerY * matrix.d + matrix.f;\n\t\t\t\t\t\tpointerX = temp;\n\t\t\t\t\t}\n\t\t\t\t\tyChange = (pointerY - startPointerY);\n\t\t\t\t\txChange = (pointerX - startPointerX);\n\t\t\t\t\tif (yChange < minimumMovement && yChange > -minimumMovement) {\n\t\t\t\t\t\tyChange = 0;\n\t\t\t\t\t}\n\t\t\t\t\tif (xChange < minimumMovement && xChange > -minimumMovement) {\n\t\t\t\t\t\txChange = 0;\n\t\t\t\t\t}\n\t\t\t\t\tif ((self.lockAxis || self.lockedAxis) && (xChange || yChange)) {\n\t\t\t\t\t\ttemp = self.lockedAxis;\n\t\t\t\t\t\tif (!temp) {\n\t\t\t\t\t\t\tself.lockedAxis = temp = (allowX && Math.abs(xChange) > Math.abs(yChange)) ? \"y\" : allowY ? \"x\" : null;\n\t\t\t\t\t\t\tif (temp && _isFunction(self.vars.onLockAxis)) {\n\t\t\t\t\t\t\t\tself.vars.onLockAxis.call(self, self.pointerEvent);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (temp === \"y\") {\n\t\t\t\t\t\t\tyChange = 0;\n\t\t\t\t\t\t} else if (temp === \"x\") {\n\t\t\t\t\t\t\txChange = 0;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tx = _round(startElementX + xChange * dragTolerance);\n\t\t\t\t\ty = _round(startElementY + yChange * dragTolerance);\n\t\t\t\t}\n\n\t\t\t\tif ((snapX || snapY || snapXY) && (self.x !== x || (self.y !== y && !rotationMode))) {\n\t\t\t\t\tif (snapXY) {\n\t\t\t\t\t\t_temp1.x = x;\n\t\t\t\t\t\t_temp1.y = y;\n\t\t\t\t\t\ttemp = snapXY(_temp1);\n\t\t\t\t\t\tx = _round(temp.x);\n\t\t\t\t\t\ty = _round(temp.y);\n\t\t\t\t\t}\n\t\t\t\t\tif (snapX) {\n\t\t\t\t\t\tx = _round(snapX(x));\n\t\t\t\t\t}\n\t\t\t\t\tif (snapY) {\n\t\t\t\t\t\ty = _round(snapY(y));\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (hasBounds) {\n\t\t\t\t\tif (x > maxX) {\n\t\t\t\t\t\tx = maxX + Math.round((x - maxX) * edgeTolerance);\n\t\t\t\t\t} else if (x < minX) {\n\t\t\t\t\t\tx = minX + Math.round((x - minX) * edgeTolerance);\n\t\t\t\t\t}\n\t\t\t\t\tif (!rotationMode) {\n\t\t\t\t\t\tif (y > maxY) {\n\t\t\t\t\t\t\ty = Math.round(maxY + (y - maxY) * edgeTolerance);\n\t\t\t\t\t\t} else if (y < minY) {\n\t\t\t\t\t\t\ty = Math.round(minY + (y - minY) * edgeTolerance);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (self.x !== x || (self.y !== y && !rotationMode)) {\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tself.endRotation = self.x = self.endX = x;\n\t\t\t\t\t\tdirty = true;\n\t\t\t\t\t} else {\n\t\t\t\t\t\tif (allowY) {\n\t\t\t\t\t\t\tself.y = self.endY = y;\n\t\t\t\t\t\t\tdirty = true; //a flag that indicates we need to render the target next time the TweenLite.ticker dispatches a \"tick\" event (typically on a requestAnimationFrame) - this is a performance optimization (we shouldn't render on every move because sometimes many move events can get dispatched between screen refreshes, and that'd be wasteful to render every time)\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (allowX) {\n\t\t\t\t\t\t\tself.x = self.endX = x;\n\t\t\t\t\t\t\tdirty = true;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (!invokeOnMove || _dispatchEvent(self, \"move\", \"onMove\") !== false) {\n\t\t\t\t\t\tif (!self.isDragging && self.isPressed) {\n\t\t\t\t\t\t\tself.isDragging = dragged = true;\n\t\t\t\t\t\t\t_dispatchEvent(self, \"dragstart\", \"onDragStart\");\n\t\t\t\t\t\t}\n\t\t\t\t\t} else { //revert because the onMove returned false!\n\t\t\t\t\t\tself.pointerX = prevPointerX;\n\t\t\t\t\t\tself.pointerY = prevPointerY;\n\t\t\t\t\t\tstartElementY = prevStartElementY;\n\t\t\t\t\t\tself.x = prevX;\n\t\t\t\t\t\tself.y = prevY;\n\t\t\t\t\t\tself.endX = prevEndX;\n\t\t\t\t\t\tself.endY = prevEndY;\n\t\t\t\t\t\tself.endRotation = prevEndRotation;\n\t\t\t\t\t\tdirty = prevDirty;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t},\n\n\t\t\t//called when the mouse/touch is released\n\t\t\tonRelease = (e, force) => {\n\t\t\t\tif (!enabled || !self.isPressed || (e && touchID != null && !force && ((e.pointerId && e.pointerId !== touchID && e.target !== target) || (e.changedTouches && !_hasTouchID(e.changedTouches, touchID))))) { //for some Microsoft browsers, we must attach the listener to the doc rather than the trigger so that when the finger moves outside the bounds of the trigger, things still work. So if the event we're receiving has a pointerId that doesn't match the touchID, ignore it (for multi-touch)\n\t\t\t\t\tisPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchend, pointerup, mouseup. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary.\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\tself.isPressed = false;\n\t\t\t\tlet originalEvent = e,\n\t\t\t\t\twasDragging = self.isDragging,\n\t\t\t\t\tisContextMenuRelease = (self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2)),\n\t\t\t\t\tplaceholderDelayedCall = gsap.delayedCall(0.001, removePlaceholder),\n\t\t\t\t\ttouches, i, syntheticEvent, eventTarget, syntheticClick;\n\t\t\t\tif (touchEventTarget) {\n\t\t\t\t\t_removeListener(touchEventTarget, \"touchend\", onRelease);\n\t\t\t\t\t_removeListener(touchEventTarget, \"touchmove\", onMove);\n\t\t\t\t\t_removeListener(touchEventTarget, \"touchcancel\", onRelease);\n\t\t\t\t\t_removeListener(ownerDoc, \"touchstart\", _onMultiTouchDocument);\n\t\t\t\t} else {\n\t\t\t\t\t_removeListener(ownerDoc, \"mousemove\", onMove);\n\t\t\t\t}\n\t\t\t\t_removeListener(_win, \"touchforcechange\", _preventDefault);\n\t\t\t\tif (!_supportsPointer || !touchEventTarget) {\n\t\t\t\t\t_removeListener(ownerDoc, \"mouseup\", onRelease);\n\t\t\t\t\te && e.target && _removeListener(e.target, \"mouseup\", onRelease);\n\t\t\t\t}\n\t\t\t\tdirty = false;\n\t\t\t\tif (wasDragging) {\n\t\t\t\t\tdragEndTime = _lastDragTime = _getTime();\n\t\t\t\t\tself.isDragging = false;\n\t\t\t\t}\n\t\t\t\t_removeFromRenderQueue(render);\n\t\t\t\tif (isClicking && !isContextMenuRelease) {\n\t\t\t\t\tif (e) {\n\t\t\t\t\t\t_removeListener(e.target, \"change\", onRelease);\n\t\t\t\t\t\tself.pointerEvent = originalEvent;\n\t\t\t\t\t}\n\t\t\t\t\t_setSelectable(triggers, false);\n\t\t\t\t\t_dispatchEvent(self, \"release\", \"onRelease\");\n\t\t\t\t\t_dispatchEvent(self, \"click\", \"onClick\");\n\t\t\t\t\tisClicking = false;\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\ti = triggers.length;\n\t\t\t\twhile (--i > -1) {\n\t\t\t\t\t_setStyle(triggers[i], \"cursor\", vars.cursor || (vars.cursor !== false ? _defaultCursor : null));\n\t\t\t\t}\n\t\t\t\t_dragCount--;\n\t\t\t\tif (e) {\n\t\t\t\t\ttouches = e.changedTouches;\n\t\t\t\t\tif (touches) { //touch events store the data slightly differently\n\t\t\t\t\t\te = touches[0];\n\t\t\t\t\t\tif (e !== touch && e.identifier !== touchID) { //Usually changedTouches[0] will be what we're looking for, but in case it's not, look through the rest of the array...(and Android browsers don't reuse the event like iOS)\n\t\t\t\t\t\t\ti = touches.length;\n\t\t\t\t\t\t\twhile (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {}\n\t\t\t\t\t\t\tif (i < 0 && !force) {\n\t\t\t\t\t\t\t\treturn;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tself.pointerEvent = originalEvent;\n\t\t\t\t\tself.pointerX = e.pageX;\n\t\t\t\t\tself.pointerY = e.pageY;\n\t\t\t\t}\n\t\t\t\tif (isContextMenuRelease && originalEvent) {\n\t\t\t\t\t_preventDefault(originalEvent);\n\t\t\t\t\tisPreventingDefault = true;\n\t\t\t\t\t_dispatchEvent(self, \"release\", \"onRelease\");\n\t\t\t\t} else if (originalEvent && !wasDragging) {\n\t\t\t\t\tisPreventingDefault = false;\n\t\t\t\t\tif (interrupted && (vars.snap || vars.bounds)) { //otherwise, if the user clicks on the object while it's animating to a snapped position, and then releases without moving 3 pixels, it will just stay there (it should animate/snap)\n\t\t\t\t\t\tanimate(vars.inertia || vars.throwProps);\n\t\t\t\t\t}\n\t\t\t\t\t_dispatchEvent(self, \"release\", \"onRelease\");\n\t\t\t\t\tif ((!_isAndroid || originalEvent.type !== \"touchmove\") && originalEvent.type.indexOf(\"cancel\") === -1) { //to accommodate native scrolling on Android devices, we have to immediately call onRelease() on the first touchmove event, but that shouldn't trigger a \"click\".\n\t\t\t\t\t\t_dispatchEvent(self, \"click\", \"onClick\");\n\t\t\t\t\t\tif (_getTime() - clickTime < 300) {\n\t\t\t\t\t\t\t_dispatchEvent(self, \"doubleclick\", \"onDoubleClick\");\n\t\t\t\t\t\t}\n\t\t\t\t\t\teventTarget = originalEvent.target || target; //old IE uses srcElement\n\t\t\t\t\t\tclickTime = _getTime();\n\t\t\t\t\t\tsyntheticClick = () => { // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular \"click\" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the \"real\"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the \"real\" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched.\n\t\t\t\t\t\t\tif (clickTime !== clickDispatch && self.enabled() && !self.isPressed && !originalEvent.defaultPrevented) {\n\t\t\t\t\t\t\t\tif (eventTarget.click) { //some browsers (like mobile Safari) don't properly trigger the click event\n\t\t\t\t\t\t\t\t\teventTarget.click();\n\t\t\t\t\t\t\t\t} else if (ownerDoc.createEvent) {\n\t\t\t\t\t\t\t\t\tsyntheticEvent = ownerDoc.createEvent(\"MouseEvents\");\n\t\t\t\t\t\t\t\t\tsyntheticEvent.initMouseEvent(\"click\", true, true, _win, 1, self.pointerEvent.screenX, self.pointerEvent.screenY, self.pointerX, self.pointerY, false, false, false, false, 0, null);\n\t\t\t\t\t\t\t\t\teventTarget.dispatchEvent(syntheticEvent);\n\t\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t};\n\t\t\t\t\t\tif (!_isAndroid && !originalEvent.defaultPrevented) { //iOS Safari requires the synthetic click to happen immediately or else it simply won't work, but Android doesn't play nice.\n\t\t\t\t\t\t\tgsap.delayedCall(0.05, syntheticClick); //in addition to the iOS bug workaround, there's a Firefox issue with clicking on things like a video to play, so we must fake a click event in a slightly delayed fashion. Previously, we listened for the \"click\" event with \"capture\" false which solved the video-click-to-play issue, but it would allow the \"click\" event to be dispatched twice like if you were using a jQuery.click() because that was handled in the capture phase, thus we had to switch to the capture phase to avoid the double-dispatching, but do the delayed synthetic click. Don't fire it too fast (like 0.00001) because we want to give the native event a chance to fire first as it's \"trusted\".\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t} else {\n\t\t\t\t\tanimate(vars.inertia || vars.throwProps); //will skip if inertia/throwProps isn't defined or InertiaPlugin isn't loaded.\n\t\t\t\t\tif (!self.allowEventDefault && originalEvent && (vars.dragClickables !== false || !isClickable.call(self, originalEvent.target)) && wasDragging && (!allowNativeTouchScrolling || (touchDragAxis && allowNativeTouchScrolling === touchDragAxis)) && originalEvent.cancelable !== false) {\n\t\t\t\t\t\tisPreventingDefault = true;\n\t\t\t\t\t\t_preventDefault(originalEvent);\n\t\t\t\t\t} else {\n\t\t\t\t\t\tisPreventingDefault = false;\n\t\t\t\t\t}\n\t\t\t\t\t_dispatchEvent(self, \"release\", \"onRelease\");\n\t\t\t\t}\n\t\t\t\tisTweening() && placeholderDelayedCall.duration( self.tween.duration() ); //sync the timing so that the placeholder DIV gets\n\t\t\t\twasDragging && _dispatchEvent(self, \"dragend\", \"onDragEnd\");\n\t\t\t\treturn true;\n\t\t\t},\n\n\t\t\tupdateScroll = e => {\n\t\t\t\tif (e && self.isDragging && !scrollProxy) {\n\t\t\t\t\tlet parent = e.target || target.parentNode,\n\t\t\t\t\t\tdeltaX = parent.scrollLeft - parent._gsScrollX,\n\t\t\t\t\t\tdeltaY = parent.scrollTop - parent._gsScrollY;\n\t\t\t\t\tif (deltaX || deltaY) {\n\t\t\t\t\t\tif (matrix) {\n\t\t\t\t\t\t\tstartPointerX -= deltaX * matrix.a + deltaY * matrix.c;\n\t\t\t\t\t\t\tstartPointerY -= deltaY * matrix.d + deltaX * matrix.b;\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tstartPointerX -= deltaX;\n\t\t\t\t\t\t\tstartPointerY -= deltaY;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tparent._gsScrollX += deltaX;\n\t\t\t\t\t\tparent._gsScrollY += deltaY;\n\t\t\t\t\t\tsetPointerPosition(self.pointerX, self.pointerY);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t},\n\n\t\t\tonClick = e => { //this was a huge pain in the neck to align all the various browsers and their behaviors. Chrome, Firefox, Safari, Opera, Android, and Microsoft Edge all handle events differently! Some will only trigger native behavior (like checkbox toggling) from trusted events. Others don't even support isTrusted, but require 2 events to flow through before triggering native behavior. Edge treats everything as trusted but also mandates that 2 flow through to trigger the correct native behavior.\n\t\t\t\tlet time = _getTime(),\n\t\t\t\t\trecentlyClicked = (time - clickTime < 100),\n\t\t\t\t\trecentlyDragged = (time - dragEndTime < 50),\n\t\t\t\t\talreadyDispatched = (recentlyClicked && clickDispatch === clickTime),\n\t\t\t\t\tdefaultPrevented = (self.pointerEvent && self.pointerEvent.defaultPrevented),\n\t\t\t\t\talreadyDispatchedTrusted = (recentlyClicked && trustedClickDispatch === clickTime),\n\t\t\t\t\ttrusted = e.isTrusted || (e.isTrusted == null && recentlyClicked && alreadyDispatched); //note: Safari doesn't support isTrusted, and it won't properly execute native behavior (like toggling checkboxes) on the first synthetic \"click\" event - we must wait for the 2nd and treat it as trusted (but stop propagation at that point). Confusing, I know. Don't you love cross-browser compatibility challenges?\n\t\t\t\tif ((alreadyDispatched || (recentlyDragged && self.vars.suppressClickOnDrag !== false) ) && e.stopImmediatePropagation) {\n\t\t\t\t\te.stopImmediatePropagation();\n\t\t\t\t}\n\t\t\t\tif (recentlyClicked && !(self.pointerEvent && self.pointerEvent.defaultPrevented) && (!alreadyDispatched || (trusted && !alreadyDispatchedTrusted))) { //let the first click pass through unhindered. Let the next one only if it's trusted, then no more (stop quick-succession ones)\n\t\t\t\t\tif (trusted && alreadyDispatched) {\n\t\t\t\t\t\ttrustedClickDispatch = clickTime;\n\t\t\t\t\t}\n\t\t\t\t\tclickDispatch = clickTime;\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\tif (self.isPressed || recentlyDragged || recentlyClicked) {\n\t\t\t\t\tif (!trusted || !e.detail || !recentlyClicked || defaultPrevented) {\n\t\t\t\t\t\t_preventDefault(e);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (!recentlyClicked && !recentlyDragged && !dragged) { // for script-triggered event dispatches, like element.click()\n\t\t\t\t\te && e.target && (self.pointerEvent = e);\n\t\t\t\t\t_dispatchEvent(self, \"click\", \"onClick\");\n\t\t\t\t}\n\t\t\t},\n\n\t\t\tlocalizePoint = p => matrix ? {x:p.x * matrix.a + p.y * matrix.c + matrix.e, y:p.x * matrix.b + p.y * matrix.d + matrix.f} : {x:p.x, y:p.y};\n\n\t\told = Draggable.get(target);\n\t\told && old.kill(); // avoids duplicates (an element can only be controlled by one Draggable)\n\n\t\t//give the user access to start/stop dragging...\n\t\tthis.startDrag = (event, align) => {\n\t\t\tlet r1, r2, p1, p2;\n\t\t\tonPress(event || self.pointerEvent, true);\n\t\t\t//if the pointer isn't on top of the element, adjust things accordingly\n\t\t\tif (align && !self.hitTest(event || self.pointerEvent)) {\n\t\t\t\tr1 = _parseRect(event || self.pointerEvent);\n\t\t\t\tr2 = _parseRect(target);\n\t\t\t\tp1 = localizePoint({x:r1.left + r1.width / 2, y:r1.top + r1.height / 2});\n\t\t\t\tp2 = localizePoint({x:r2.left + r2.width / 2, y:r2.top + r2.height / 2});\n\t\t\t\tstartPointerX -= p1.x - p2.x;\n\t\t\t\tstartPointerY -= p1.y - p2.y;\n\t\t\t}\n\t\t\tif (!self.isDragging) {\n\t\t\t\tself.isDragging = dragged = true;\n\t\t\t\t_dispatchEvent(self, \"dragstart\", \"onDragStart\");\n\t\t\t}\n\t\t};\n\t\tthis.drag = onMove;\n\t\tthis.endDrag = e =>\tonRelease(e || self.pointerEvent, true);\n\t\tthis.timeSinceDrag = () => self.isDragging ? 0 : (_getTime() - dragEndTime) / 1000;\n\t\tthis.timeSinceClick = () => (_getTime() - clickTime) / 1000;\n\t\tthis.hitTest = (target, threshold) => Draggable.hitTest(self.target, target, threshold);\n\n\t\tthis.getDirection = (from, diagonalThreshold) => { //from can be \"start\" (default), \"velocity\", or an element\n\t\t\tlet mode = (from === \"velocity\" && InertiaPlugin) ? from : (_isObject(from) && !rotationMode) ? \"element\" : \"start\",\n\t\t\t\txChange, yChange, ratio, direction, r1, r2;\n\t\t\tif (mode === \"element\") {\n\t\t\t\tr1 = _parseRect(self.target);\n\t\t\t\tr2 = _parseRect(from);\n\t\t\t}\n\t\t\txChange = (mode === \"start\") ? self.x - startElementX : (mode === \"velocity\") ? InertiaPlugin.getVelocity(target, xProp) : (r1.left + r1.width / 2) - (r2.left + r2.width / 2);\n\t\t\tif (rotationMode) {\n\t\t\t\treturn xChange < 0 ? \"counter-clockwise\" : \"clockwise\";\n\t\t\t} else {\n\t\t\t\tdiagonalThreshold = diagonalThreshold || 2;\n\t\t\t\tyChange = (mode === \"start\") ? self.y - startElementY : (mode === \"velocity\") ? InertiaPlugin.getVelocity(target, yProp) : (r1.top + r1.height / 2) - (r2.top + r2.height / 2);\n\t\t\t\tratio = Math.abs(xChange / yChange);\n\t\t\t\tdirection = (ratio < 1 / diagonalThreshold) ? \"\" : (xChange < 0) ? \"left\" : \"right\";\n\t\t\t\tif (ratio < diagonalThreshold) {\n\t\t\t\t\tif (direction !== \"\") {\n\t\t\t\t\t\tdirection += \"-\";\n\t\t\t\t\t}\n\t\t\t\t\tdirection += (yChange < 0) ? \"up\" : \"down\";\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn direction;\n\t\t};\n\n\t\tthis.applyBounds = (newBounds, sticky) => {\n\t\t\tlet x, y, forceZeroVelocity, e, parent, isRoot;\n\t\t\tif (newBounds && vars.bounds !== newBounds) {\n\t\t\t\tvars.bounds = newBounds;\n\t\t\t\treturn self.update(true, sticky);\n\t\t\t}\n\t\t\tsyncXY(true);\n\t\t\tcalculateBounds();\n\t\t\tif (hasBounds && !isTweening()) {\n\t\t\t\tx = self.x;\n\t\t\t\ty = self.y;\n\t\t\t\tif (x > maxX) {\n\t\t\t\t\tx = maxX;\n\t\t\t\t} else if (x < minX) {\n\t\t\t\t\tx = minX;\n\t\t\t\t}\n\t\t\t\tif (y > maxY) {\n\t\t\t\t\ty = maxY;\n\t\t\t\t} else if (y < minY) {\n\t\t\t\t\ty = minY;\n\t\t\t\t}\n\t\t\t\tif (self.x !== x || self.y !== y) {\n\t\t\t\t\tforceZeroVelocity = true;\n\t\t\t\t\tself.x = self.endX = x;\n\t\t\t\t\tif (rotationMode) {\n\t\t\t\t\t\tself.endRotation = x;\n\t\t\t\t\t} else {\n\t\t\t\t\t\tself.y = self.endY = y;\n\t\t\t\t\t}\n\t\t\t\t\tdirty = true;\n\t\t\t\t\trender(true);\n\t\t\t\t\tif (self.autoScroll && !self.isDragging) {\n\t\t\t\t\t\t_recordMaxScrolls(target.parentNode);\n\t\t\t\t\t\te = target;\n\t\t\t\t\t\t_windowProxy.scrollTop = ((_win.pageYOffset != null) ? _win.pageYOffset : (ownerDoc.documentElement.scrollTop != null) ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop);\n\t\t\t\t\t\t_windowProxy.scrollLeft = ((_win.pageXOffset != null) ? _win.pageXOffset : (ownerDoc.documentElement.scrollLeft != null) ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft);\n\t\t\t\t\t\twhile (e && !isRoot) { //walk up the chain and sense wherever the scrollTop/scrollLeft exceeds the maximum.\n\t\t\t\t\t\t\tisRoot = _isRoot(e.parentNode);\n\t\t\t\t\t\t\tparent = isRoot ? _windowProxy : e.parentNode;\n\t\t\t\t\t\t\tif (allowY && parent.scrollTop > parent._gsMaxScrollY) {\n\t\t\t\t\t\t\t\tparent.scrollTop = parent._gsMaxScrollY;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\tif (allowX && parent.scrollLeft > parent._gsMaxScrollX) {\n\t\t\t\t\t\t\t\tparent.scrollLeft = parent._gsMaxScrollX;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\te = parent;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (self.isThrowing && (forceZeroVelocity || self.endX > maxX || self.endX < minX || self.endY > maxY || self.endY < minY)) {\n\t\t\t\t\tanimate(vars.inertia || vars.throwProps, forceZeroVelocity);\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn self;\n\t\t};\n\n\t\tthis.update = (applyBounds, sticky, ignoreExternalChanges) => {\n\t\t\tif (sticky && self.isPressed) { // in case the element was repositioned in the document flow, thus its x/y may be identical but its position is actually quite different.\n\t\t\t\tlet m = getGlobalMatrix(target),\n\t\t\t\t\tp = innerMatrix.apply({x: self.x - startElementX, y: self.y - startElementY}),\n\t\t\t\t\tm2 = getGlobalMatrix(target.parentNode, true);\n\t\t\t\tm2.apply({x: m.e - p.x, y: m.f - p.y}, p);\n\t\t\t\tself.x -= p.x - m2.e;\n\t\t\t\tself.y -= p.y - m2.f;\n\t\t\t\trender(true);\n\t\t\t\trecordStartPositions();\n\t\t\t}\n\t\t\tlet { x, y } = self;\n\t\t\tupdateMatrix(!sticky);\n\t\t\tif (applyBounds) {\n\t\t\t\tself.applyBounds();\n\t\t\t} else {\n\t\t\t\tdirty && ignoreExternalChanges && render(true);\n\t\t\t\tsyncXY(true);\n\t\t\t}\n\t\t\tif (sticky) {\n\t\t\t\tsetPointerPosition(self.pointerX, self.pointerY);\n\t\t\t\tdirty && render(true);\n\t\t\t}\n\t\t\tif (self.isPressed && !sticky && ((allowX && Math.abs(x - self.x) > 0.01) || (allowY && (Math.abs(y - self.y) > 0.01 && !rotationMode)))) {\n\t\t\t\trecordStartPositions();\n\t\t\t}\n\t\t\tif (self.autoScroll) {\n\t\t\t\t_recordMaxScrolls(target.parentNode, self.isDragging);\n\t\t\t\tcheckAutoScrollBounds = self.isDragging;\n\t\t\t\trender(true);\n\t\t\t\t//in case reparenting occurred.\n\t\t\t\t_removeScrollListener(target, updateScroll);\n\t\t\t\t_addScrollListener(target, updateScroll);\n\t\t\t}\n\t\t\treturn self;\n\t\t};\n\n\t\tthis.enable = type => {\n\t\t\tlet setVars = {lazy: true},\n\t\t\t\tid, i, trigger;\n\t\t\tif (vars.cursor !== false) {\n\t\t\t\tsetVars.cursor = vars.cursor || _defaultCursor;\n\t\t\t}\n\t\t\tif (gsap.utils.checkPrefix(\"touchCallout\")) {\n\t\t\t\tsetVars.touchCallout = \"none\";\n\t\t\t}\n\t\t\tif (type !== \"soft\") {\n\t\t\t\t_setTouchActionForAllDescendants(triggers, (allowX === allowY) ? \"none\" : (vars.allowNativeTouchScrolling && (target.scrollHeight === target.clientHeight) === (target.scrollWidth === target.clientHeight)) || vars.allowEventDefault ? \"manipulation\" : allowX ? \"pan-y\" : \"pan-x\"); // Some browsers like Internet Explorer will fire a pointercancel event when the user attempts to drag when touchAction is \"manipulate\" because it's perceived as a pan. If the element has scrollable content in only one direction, we should use pan-x or pan-y accordingly so that the pointercancel doesn't prevent dragging.\n\t\t\t\ti = triggers.length;\n\t\t\t\twhile (--i > -1) {\n\t\t\t\t\ttrigger = triggers[i];\n\t\t\t\t\t_supportsPointer || _addListener(trigger, \"mousedown\", onPress);\n\t\t\t\t\t_addListener(trigger, \"touchstart\", onPress);\n\t\t\t\t\t_addListener(trigger, \"click\", onClick, true); // note: used to pass true for capture but it prevented click-to-play-video functionality in Firefox.\n\t\t\t\t\tgsap.set(trigger, setVars);\n\t\t\t\t\tif (trigger.getBBox && trigger.ownerSVGElement && allowX !== allowY) { // a bug in chrome doesn't respect touch-action on SVG elements - it only works if we set it on the parent SVG.\n\t\t\t\t\t\tgsap.set(trigger.ownerSVGElement, {touchAction: vars.allowNativeTouchScrolling || vars.allowEventDefault ? \"manipulation\" : allowX ? \"pan-y\" : \"pan-x\"});\n\t\t\t\t\t}\n\t\t\t\t\tvars.allowContextMenu || _addListener(trigger, \"contextmenu\", onContextMenu);\n\t\t\t\t}\n\t\t\t\t_setSelectable(triggers, false);\n\t\t\t}\n\t\t\t_addScrollListener(target, updateScroll);\n\t\t\tenabled = true;\n\t\t\tif (InertiaPlugin && type !== \"soft\") {\n\t\t\t\tInertiaPlugin.track(scrollProxy || target, (xyMode ? \"x,y\" : rotationMode ? \"rotation\" : \"top,left\"));\n\t\t\t}\n\t\t\ttarget._gsDragID = id = target._gsDragID || (\"d\" + (_lookupCount++));\n\t\t\t_lookup[id] = self;\n\t\t\tif (scrollProxy) {\n\t\t\t\tscrollProxy.enable();\n\t\t\t\tscrollProxy.element._gsDragID = id;\n\t\t\t}\n\t\t\t(vars.bounds || rotationMode) && recordStartPositions();\n\t\t\tvars.bounds && self.applyBounds();\n\t\t\treturn self;\n\t\t};\n\n\t\tthis.disable = type => {\n\t\t\tlet dragging = self.isDragging,\n\t\t\t\ti = triggers.length,\n\t\t\t\ttrigger;\n\t\t\twhile (--i > -1) {\n\t\t\t\t_setStyle(triggers[i], \"cursor\", null);\n\t\t\t}\n\t\t\tif (type !== \"soft\") {\n\t\t\t\t_setTouchActionForAllDescendants(triggers, null);\n\t\t\t\ti = triggers.length;\n\t\t\t\twhile (--i > -1) {\n\t\t\t\t\ttrigger = triggers[i];\n\t\t\t\t\t_setStyle(trigger, \"touchCallout\", null);\n\t\t\t\t\t_removeListener(trigger, \"mousedown\", onPress);\n\t\t\t\t\t_removeListener(trigger, \"touchstart\", onPress);\n\t\t\t\t\t_removeListener(trigger, \"click\", onClick, true);\n\t\t\t\t\t_removeListener(trigger, \"contextmenu\", onContextMenu);\n\t\t\t\t}\n\t\t\t\t_setSelectable(triggers, true);\n\t\t\t\tif (touchEventTarget) {\n\t\t\t\t\t_removeListener(touchEventTarget, \"touchcancel\", onRelease);\n\t\t\t\t\t_removeListener(touchEventTarget, \"touchend\", onRelease);\n\t\t\t\t\t_removeListener(touchEventTarget, \"touchmove\", onMove);\n\t\t\t\t}\n\t\t\t\t_removeListener(ownerDoc, \"mouseup\", onRelease);\n\t\t\t\t_removeListener(ownerDoc, \"mousemove\", onMove);\n\t\t\t}\n\t\t\t_removeScrollListener(target, updateScroll);\n\t\t\tenabled = false;\n\t\t\tif (InertiaPlugin && type !== \"soft\") {\n\t\t\t\tInertiaPlugin.untrack(scrollProxy || target, (xyMode ? \"x,y\" : rotationMode ? \"rotation\" : \"top,left\"));\n\t\t\t\tself.tween && self.tween.kill();\n\t\t\t}\n\t\t\tscrollProxy && scrollProxy.disable();\n\t\t\t_removeFromRenderQueue(render);\n\t\t\tself.isDragging = self.isPressed = isClicking = false;\n\t\t\tdragging && _dispatchEvent(self, \"dragend\", \"onDragEnd\");\n\t\t\treturn self;\n\t\t};\n\n\t\tthis.enabled = function(value, type) {\n\t\t\treturn arguments.length ? (value ? self.enable(type) : self.disable(type)) : enabled;\n\t\t};\n\n\t\tthis.kill = function() {\n\t\t\tself.isThrowing = false;\n\t\t\tself.tween && self.tween.kill();\n\t\t\tself.disable();\n\t\t\tgsap.set(triggers, {clearProps:\"userSelect\"});\n\t\t\tdelete _lookup[target._gsDragID];\n\t\t\treturn self;\n\t\t};\n\n\t\tthis.revert = function() {\n\t\t\tthis.kill();\n\t\t\tthis.styles && this.styles.revert();\n\t\t};\n\n\t\tif (~type.indexOf(\"scroll\")) {\n\t\t\tscrollProxy = this.scrollProxy = new ScrollProxy(target, _extend({onKill:function() { //ScrollProxy's onKill() gets called if/when the ScrollProxy senses that the user interacted with the scroll position manually (like using the scrollbar). IE9 doesn't fire the \"mouseup\" properly when users drag the scrollbar of an element, so this works around that issue.\n\t\t\t\t\tself.isPressed && onRelease(null);\n\t\t\t}}, vars));\n\t\t\t//a bug in many Android devices' stock browser causes scrollTop to get forced back to 0 after it is altered via JS, so we set overflow to \"hidden\" on mobile/touch devices (they hide the scroll bar anyway). That works around the bug. (This bug is discussed at https://code.google.com/p/android/issues/detail?id=19625)\n\t\t\ttarget.style.overflowY = (allowY && !_isTouchDevice) ? \"auto\" : \"hidden\";\n\t\t\ttarget.style.overflowX = (allowX && !_isTouchDevice) ? \"auto\" : \"hidden\";\n\t\t\ttarget = scrollProxy.content;\n\t\t}\n\n\t\tif (rotationMode) {\n\t\t\tkillProps.rotation = 1;\n\t\t} else {\n\t\t\tif (allowX) {\n\t\t\t\tkillProps[xProp] = 1;\n\t\t\t}\n\t\t\tif (allowY) {\n\t\t\t\tkillProps[yProp] = 1;\n\t\t\t}\n\t\t}\n\n\t\tgsCache.force3D = (\"force3D\" in vars) ? vars.force3D : true; //otherwise, normal dragging would be in 2D and then as soon as it's released and there's an inertia tween, it'd jump to 3D which can create an initial jump due to the work the browser must to do layerize it.\n\n\t\t_context(this);\n\t\tthis.enable();\n\t}\n\n\n\n\n\tstatic register(core) {\n\t\tgsap = core;\n\t\t_initCore();\n\t}\n\n\tstatic create(targets, vars) {\n\t\t_coreInitted || _initCore(true);\n\t\treturn _toArray(targets).map(target => new Draggable(target, vars));\n\t}\n\n\tstatic get(target) {\n\t\treturn _lookup[(_toArray(target)[0] || {})._gsDragID];\n\t}\n\n\tstatic timeSinceDrag() {\n\t\treturn (_getTime() - _lastDragTime) / 1000;\n\t}\n\n\tstatic hitTest(obj1, obj2, threshold) {\n\t\tif (obj1 === obj2) {\n\t\t\treturn false;\n\t\t}\n\t\tlet r1 = _parseRect(obj1),\n\t\t\tr2 = _parseRect(obj2),\n\t\t\t{ top, left, right, bottom, width, height } = r1,\n\t\t\tisOutside = (r2.left > right || r2.right < left || r2.top > bottom || r2.bottom < top),\n\t\t\toverlap, area, isRatio;\n\t\tif (isOutside || !threshold) {\n\t\t\treturn !isOutside;\n\t\t}\n\t\tisRatio = ((threshold + \"\").indexOf(\"%\") !== -1);\n\t\tthreshold = parseFloat(threshold) || 0;\n\t\toverlap = {left: Math.max(left, r2.left), top: Math.max(top, r2.top)};\n\t\toverlap.width = Math.min(right, r2.right) - overlap.left;\n\t\toverlap.height = Math.min(bottom, r2.bottom) - overlap.top;\n\t\tif (overlap.width < 0 || overlap.height < 0) {\n\t\t\treturn false;\n\t\t}\n\t\tif (isRatio) {\n\t\t\tthreshold *= 0.01;\n\t\t\tarea = overlap.width * overlap.height;\n\t\t\treturn (area >= width * height * threshold || area >= r2.width * r2.height * threshold);\n\t\t}\n\t\treturn (overlap.width > threshold && overlap.height > threshold);\n\t}\n\n}\n\n_setDefaults(Draggable.prototype, {pointerX:0, pointerY: 0, startX: 0, startY: 0, deltaX: 0, deltaY: 0, isDragging: false, isPressed: false});\n\nDraggable.zIndex = 1000;\nDraggable.version = \"3.12.7\";\n\n_getGSAP() && gsap.registerPlugin(Draggable);\n\nexport { Draggable as default };"],"names":["_createSibling","element","i","parentNode","_doc","_setDoc","svg","_svgOwner","ns","getAttribute","type","x","y","css","e","createElementNS","replace","createElement","_svgContainer","setAttribute","appendChild","_divContainer","style","cssText","_setMatrix","m","a","b","c","d","f","_win","_docElement","_body","_identityMatrix","_gEl","_hasOffsetBug","_transformProp","_transformOriginProp","doc","ownerDocument","window","Matrix2D","documentElement","body","transform","d1","d2","root","firstElementChild","offsetParent","removeChild","_forceNonZeroScale","cache","_gsap","uncache","get","scaleX","scaleY","renderTransform","push","_svgTemps","_divTemps","_getDocScrollTop","pageYOffset","scrollTop","_getDocScrollLeft","pageXOffset","scrollLeft","ownerSVGElement","tagName","toLowerCase","_isFixed","getComputedStyle","position","nodeType","_placeSiblings","adjustGOffset","container","cs","isRootSVG","siblings","parent","length","_getCTM","getCTM","removeProperty","clone","getBBox","baseVal","numberOfItems","_consolidate","multiply","getItem","matrix","offsetLeft","offsetTop","top","left","inverse","this","determinant","a2","b2","c2","e2","f2","equals","apply","point","decoratee","getGlobalMatrix","includeScrollInFixed","zeroScales","temps","b1","getBoundingClientRect","b3","isFixed","_windowExists","_getGSAP","gsap","registerPlugin","_isFunction","value","_isObject","_isUndefined","_emptyFunc","_round","Math","round","_createElement","_copy","obj","factor","p","copy","_setTouchActionForAllDescendants","elements","children","touchAction","_renderQueueTick","_renderQueue","forEach","func","_renderQueueTimeout","ticker","remove","_removeFromRenderQueue","splice","to","overwrite","delay","duration","onComplete","data","_addListener","capture","addEventListener","touchType","_touchEventLookup","_supportsPassive","passive","_removeListener","removeEventListener","_preventDefault","event","preventDefault","preventManipulation","_onMultiTouchDocumentEnd","_isMultiTouching","touches","_dragCount","target","_onMultiTouchDocument","_addScrollListener","callback","_isRoot","_removeScrollListener","_getMaxScroll","axis","dim","scroll","client","max","_recordMaxScrolls","skipCurrent","_windowProxy","_gsMaxScrollX","_gsMaxScrollY","_gsScrollX","_gsScrollY","_setStyle","property","_checkPrefix","_getComputedStyle","Element","host","_parseRect","_tempRect","width","right","clientWidth","innerWidth","height","bottom","innerHeight","clientHeight","r","pageX","_toArray","pageY","_dispatchEvent","callbackName","result","vars","listeners","_listeners","callbackScope","pointerEvent","dispatchEvent","_getBounds","context","offset","_getElementBounds","min","minX","minRotation","minY","maxX","maxRotation","maxY","_parseInertia","draggable","snap","forceZeroVelocity","l","Array","end","call","velocity","_isClickable","_clickableTagExp","test","nodeName","_setSelectable","selectable","ondragstart","onselectstart","set","lazy","userSelect","ScrollProxy","utils","toArray","elementWidth","elementHeight","contentHeight","nextNode","transformStart","transformEnd","content","document","node","firstChild","prevTop","prevLeft","scrollWidth","scrollHeight","extraPadRight","maxLeft","maxTop","_supports3D","force3D","force","arguments","dif","oldOffset","killTweensOf","onKill","_skip","paddingRight","maxScrollTop","maxScrollLeft","disable","nextSibling","enable","calibrate","widthMatches","offsetHeight","display","parseFloat","paddingLeft","_addPaddingBR","overflow","verticalAlign","boxSizing","paddingBottom","_initCore","required","nav","navigator","_tempDiv","_supportsPointer","PointerEvent","_placeholderDiv","_defaultCursor","cursor","_isAndroid","userAgent","indexOf","_isTouchDevice","MaxTouchPoints","msMaxTouchPoints","div","child","childStyle","val","types","standard","split","converted","Object","defineProperty","_lookup","isPressed","endDrag","_coreInitted","InertiaPlugin","plugins","inertia","_context","core","checkPrefix","_getStyleSaver","getStyleSaver","console","warn","_isArray","isArray","_RAD2DEG","PI","_bigNum","_getTime","Date","now","getTime","_lookupCount","_lastDragTime","_temp1","_point1","p1","p2","p3","p4","bbox","isSVG","viewBox","borderLeftWidth","borderRightWidth","borderTopWidth","borderBottomWidth","EventDispatcher","list","_this","Draggable","register","create","targets","map","_gsDragID","timeSinceDrag","hitTest","obj1","obj2","threshold","overlap","area","isRatio","r1","r2","isOutside","styles","_this2","rotation","dragResistance","edgeResistance","isNaN","lockAxis","autoScroll","lockedAxis","allowEventDefault","getProperty","getPropAsNum","unit","gsCache","onContextMenu","stopImmediatePropagation","render","suppressEvents","self","isDragging","checkAutoScrollBounds","dirty","isRoot","rect","pointerX","pointerY","changeX","changeY","gap","autoScrollFactor","ownerDoc","allowY","autoScrollMarginBottom","autoScrollMarginTop","allowX","autoScrollMarginRight","autoScrollMarginLeft","scrollTo","setPointerPosition","rotationMode","deltaX","scrollProxy","deltaY","xyMode","hasDragCallback","isDispatching","syncXY","skipOnUpdate","skipSnap","snappedValue","getCache","snapX","snapY","snapXY","isThrowing","buildSnapFunc","n","edgeTolerance","closest","absDif","calculateBounds","bounds","targetBounds","snapIsRaw","hasBounds","xProp","yProp","liveSnap","points","buildPointSnapFunc","radius","dx","dy","dist","minDist","onThrowComplete","onThrowInterrupt","animate","tween","overshootTolerance","resistance","throwResistance","linkedProps","minDuration","maxDuration","overshoot","inherit","onInterrupt","onUpdate","fastMode","onUpdateParams","endX","endY","endRotation","play","applyBounds","updateMatrix","shiftStart","start","startPointerX","startPointerY","recordStartPositions","parsedOrigin","offsetX","offsetY","innerMatrix","startElementY","startElementX","isTweening","xOrigin","yOrigin","rotationOrigin","atan2","startX","startY","removePlaceholder","onPress","enabled","clickTime","isPreventingDefault","interrupted","dragged","touchEventTarget","currentTarget","onRelease","onMove","touchDragAxis","isClicking","isClickable","dragClickables","triggers","allowNativeTouchScrolling","allowContextMenu","ctrlKey","which","changedTouches","touch","touchID","identifier","pointerId","_addToRenderQueue","add","kill","killProps","zIndexBoost","zIndex","onDrag","drag","hasMoveCallback","move","activeCursor","updateScroll","onClick","time","recentlyClicked","recentlyDragged","dragEndTime","alreadyDispatched","clickDispatch","defaultPrevented","alreadyDispatchedTrusted","trustedClickDispatch","trusted","isTrusted","suppressClickOnDrag","detail","localizePoint","old","minimumMovement","trigger","handle","clickableTest","isActive","originalEvent","abs","lockAxisOnTouchScroll","onLockAxis","cancelable","invokeOnMove","xChange","yChange","temp","dragTolerance","prevPointerX","prevPointerY","prevStartElementY","prevX","prevY","prevEndX","prevEndY","prevEndRotation","prevDirty","_hasTouchID","ID","syntheticEvent","eventTarget","syntheticClick","wasDragging","isContextMenuRelease","placeholderDelayedCall","delayedCall","throwProps","click","createEvent","initMouseEvent","screenX","screenY","startDrag","align","timeSinceClick","getDirection","from","diagonalThreshold","ratio","direction","mode","getVelocity","newBounds","sticky","update","ignoreExternalChanges","m2","id","setVars","touchCallout","track","dragging","untrack","clearProps","revert","_extend","defaults","overflowY","overflowX","_setDefaults","prototype","version"],"mappings":";;;;;;;;;6VA2FkB,SAAjBA,EAAkBC,EAASC,MACtBD,EAAQE,aAAeC,GAAQC,EAAQJ,IAAW,KACjDK,EAAMC,EAAUN,GACnBO,EAAKF,EAAOA,EAAIG,aAAa,UAAY,6BAAgC,+BACzEC,EAAOJ,EAAOJ,EAAI,OAAS,IAAO,MAClCS,EAAU,IAANT,EAAU,EAAI,IAClBU,EAAU,IAANV,EAAU,IAAM,EACpBW,EAAM,0EACNC,EAAIV,EAAKW,gBAAkBX,EAAKW,gBAAgBP,EAAGQ,QAAQ,SAAU,QAASN,GAAQN,EAAKa,cAAcP,UACtGR,IACEI,GAScY,EAAlBA,GAAkClB,EAAeC,GACjDa,EAAEK,aAAa,QAAS,KACxBL,EAAEK,aAAa,SAAU,KACzBL,EAAEK,aAAa,YAAa,aAAeR,EAAI,IAAMC,EAAI,KACzDM,EAAcE,YAAYN,KAZrBO,KACJA,EAAgBrB,EAAeC,IACjBqB,MAAMC,QAAUV,GAE/BC,EAAEQ,MAAMC,QAAUV,EAAM,gCAAkCD,EAAI,WAAaD,EAAI,KAC/EU,EAAcD,YAAYN,KAUrBA,OAEF,4BA4FM,SAAbU,EAAcC,EAAGC,EAAGC,EAAGC,EAAGC,EAAGf,EAAGgB,UAC/BL,EAAEC,EAAIA,EACND,EAAEE,EAAIA,EACNF,EAAEG,EAAIA,EACNH,EAAEI,EAAIA,EACNJ,EAAEX,EAAIA,EACNW,EAAEK,EAAIA,EACCL,EA/MT,IAAIrB,EAAM2B,EAAMC,EAAaC,EAAOZ,EAAeH,EAAegB,EAAiBC,EAGlFC,IAFAC,EAAiB,YACjBC,EAAuBD,EAAiB,SAExChC,EAAU,SAAVA,QAAUJ,OACLsC,EAAMtC,EAAQuC,eAAiBvC,IAC7BoC,KAAkBpC,EAAQqB,QAAU,gBAAiBrB,EAAQqB,QAElEgB,GADAD,EAAiB,eACuB,eAElCE,EAAIpC,aAAeoC,EAAMA,EAAIpC,iBACpC4B,EAAOU,OACPP,EAAkB,IAAIQ,GAClBH,EAAK,CAERP,GADA5B,EAAOmC,GACWI,gBAClBV,EAAQM,EAAIK,MACZT,EAAO/B,EAAKW,gBAAgB,6BAA8B,MAErDO,MAAMuB,UAAY,WAEnBC,EAAKP,EAAItB,cAAc,OAC1B8B,EAAKR,EAAItB,cAAc,OACvB+B,EAAOT,IAAQA,EAAIK,MAAQL,EAAIU,mBAC5BD,GAAQA,EAAK5B,cAChB4B,EAAK5B,YAAY0B,GACjBA,EAAG1B,YAAY2B,GACfD,EAAG3B,aAAa,QAAS,kDACzBiB,EAAiBW,EAAGG,eAAiBJ,EACrCE,EAAKG,YAAYL,WAGZP,GAERa,EAAqB,SAArBA,mBAAqBtC,WAChBY,EAAG2B,EACAvC,GAAKA,IAAMmB,IACjBoB,EAAQvC,EAAEwC,QACDD,EAAME,SAAWF,EAAMG,IAAI1C,EAAG,KACnCuC,IAAUA,EAAMI,SAAWJ,EAAMK,QAAUL,EAAMM,kBACpDN,EAAMI,OAASJ,EAAMK,OAAS,KAC9BL,EAAMM,gBAAgB,EAAGN,GACzB3B,EAAIA,EAAEkC,KAAKP,GAAU3B,EAAI,CAAC2B,IAE3BvC,EAAIA,EAAEX,kBAEAuB,GAoBRmC,EAAY,GACZC,EAAY,GACZC,EAAmB,SAAnBA,0BAAyBhC,EAAKiC,aAAgB5D,EAAK6D,WAAajC,EAAYiC,WAAahC,EAAMgC,WAAa,GAC5GC,EAAoB,SAApBA,2BAA0BnC,EAAKoC,aAAe/D,EAAKgE,YAAcpC,EAAYoC,YAAcnC,EAAMmC,YAAc,GAC/G7D,EAAY,SAAZA,UAAYN,UAAWA,EAAQoE,kBAA6D,SAAxCpE,EAAQqE,QAAU,IAAIC,cAA0BtE,EAAU,OAC9GuE,EAAW,SAAXA,SAAWvE,SACsC,UAA5C8B,EAAK0C,iBAAiBxE,GAASyE,YAGnCzE,EAAUA,EAAQE,aACkB,IAArBF,EAAQ0E,SACfH,SAASvE,YAsDlB2E,EAAiB,SAAjBA,eAAkB3E,EAAS4E,OAKzBC,EAAWrD,EAAGE,EAAGhB,EAAGC,EAAGmE,EAJpBzE,EAAMC,EAAUN,GACnB+E,EAAY/E,IAAYK,EACxB2E,EAAW3E,EAAMuD,EAAYC,EAC7BoB,EAASjF,EAAQE,cAEdF,IAAY8B,SACR9B,KAERgF,EAASE,QAAUF,EAASrB,KAAK5D,EAAeC,EAAS,GAAID,EAAeC,EAAS,GAAID,EAAeC,EAAS,IACjH6E,EAAYxE,EAAMY,EAAgBG,EAC9Bf,EACC0E,GAEHrE,IADAgB,EA1BO,SAAVyD,QAAU9E,OAERuC,EADGpB,EAAInB,EAAI+E,gBAEP5D,IACJoB,EAAYvC,EAAIgB,MAAMe,GACtB/B,EAAIgB,MAAMe,GAAkB,OAC5B/B,EAAIc,YAAYe,GAChBV,EAAIU,EAAKkD,SACT/E,EAAI6C,YAAYhB,GAChBU,EAAavC,EAAIgB,MAAMe,GAAkBQ,EAAavC,EAAIgB,MAAMgE,eAAejD,EAAerB,QAAQ,WAAY,OAAOuD,gBAEnH9C,GAAKS,EAAgBqD,QAetBH,CAAQnF,IACLa,EAAIa,EAAED,EACbd,GAAKe,EAAEG,EAAIH,EAAEE,EACbJ,EAAIS,GACMjC,EAAQuF,SAClB7D,EAAI1B,EAAQuF,UAGZ7E,GADAc,GADAA,EAAIxB,EAAQ4C,UAAY5C,EAAQ4C,UAAU4C,QAAU,IAC7CC,cAAoD,EAAlBjE,EAAEiE,cAzC/B,SAAfC,aAAelE,WACVG,EAAI,IAAIc,GACXxC,EAAI,EACEA,EAAIuB,EAAEiE,cAAexF,IAC3B0B,EAAEgE,SAASnE,EAAEoE,QAAQ3F,GAAG4F,eAElBlE,EAmC0D+D,CAAalE,GAAKA,EAAEoE,QAAQ,GAAGC,OAAvE5D,GACjBR,EAAIC,EAAEhB,EAAIc,EAAEG,EAAID,EAAEf,EACxBA,EAAIa,EAAEE,EAAIA,EAAEhB,EAAIc,EAAEI,EAAIF,EAAEf,IAExBa,EAAI,IAAIiB,GACR/B,EAAIC,EAAI,GAELiE,GAAmD,MAAlC5E,EAAQqE,QAAQC,gBACpC5D,EAAIC,EAAI,IAERoE,EAAY1E,EAAM4E,GAAQ9D,YAAY0D,GACvCA,EAAU3D,aAAa,YAAa,UAAYM,EAAEC,EAAI,IAAMD,EAAEE,EAAI,IAAMF,EAAEG,EAAI,IAAMH,EAAEI,EAAI,KAAOJ,EAAEX,EAAIH,GAAK,KAAOc,EAAEK,EAAIlB,GAAK,SACxH,IACND,EAAIC,EAAI,EACJwB,MACHX,EAAIxB,EAAQiD,aACZvB,EAAI1B,GACS0B,EAANA,GAAUA,EAAExB,aAAewB,IAAMF,GAAKE,EAAExB,YACe,GAAxD4B,EAAK0C,iBAAiB9C,GAAGU,GAAkB,IAAI8C,SACnDxE,EAAIgB,EAAEoE,WACNnF,EAAIe,EAAEqE,UACNrE,EAAI,MAKa,cADpBoD,EAAKhD,EAAK0C,iBAAiBxE,IACpByE,UAA2C,UAAhBK,EAAGL,aACpCjD,EAAIxB,EAAQiD,aACLgC,GAAUA,IAAWzD,GAC3Bd,GAAKuE,EAAOd,YAAc,EAC1BxD,GAAKsE,EAAOjB,WAAa,EACzBiB,EAASA,EAAO/E,YAGlBwB,EAAImD,EAAUxD,OACZ2E,IAAOhG,EAAQ+F,UAAYpF,EAAK,KAClCe,EAAEuE,KAAQjG,EAAQ8F,WAAapF,EAAK,KACpCgB,EAAEU,GAAkB0C,EAAG1C,GACvBV,EAAEW,GAAwByC,EAAGzC,GAM7BX,EAAE+C,SAA2B,UAAhBK,EAAGL,SAAuB,QAAU,WACjDzE,EAAQE,WAAWiB,YAAY0D,UAEzBA,GAYIpC,2BAKZyD,QAAA,uBACMzE,EAAoB0E,KAApB1E,EAAGC,EAAiByE,KAAjBzE,EAAGC,EAAcwE,KAAdxE,EAAGC,EAAWuE,KAAXvE,EAAGf,EAAQsF,KAARtF,EAAGgB,EAAKsE,KAALtE,EACnBuE,EAAe3E,EAAIG,EAAIF,EAAIC,GAAM,aAC3BJ,EACN4E,KACAvE,EAAIwE,GACH1E,EAAI0E,GACJzE,EAAIyE,EACL3E,EAAI2E,GACHzE,EAAIE,EAAID,EAAIf,GAAKuF,IAChB3E,EAAII,EAAIH,EAAIb,GAAKuF,MAIrBT,SAAA,kBAASE,OACHpE,EAAoB0E,KAApB1E,EAAGC,EAAiByE,KAAjBzE,EAAGC,EAAcwE,KAAdxE,EAAGC,EAAWuE,KAAXvE,EAAGf,EAAQsF,KAARtF,EAAGgB,EAAKsE,KAALtE,EACnBwE,EAAKR,EAAOpE,EACZ6E,EAAKT,EAAOlE,EACZ4E,EAAKV,EAAOnE,EACZoB,EAAK+C,EAAOjE,EACZ4E,EAAKX,EAAOhF,EACZ4F,EAAKZ,EAAOhE,SACNN,EAAW4E,KACjBE,EAAK5E,EAAI8E,EAAK5E,EACd0E,EAAK3E,EAAI6E,EAAK3E,EACd0E,EAAK7E,EAAIqB,EAAKnB,EACd2E,EAAK5E,EAAIoB,EAAKlB,EACdf,EAAI2F,EAAK/E,EAAIgF,EAAK9E,EAClBE,EAAI2E,EAAK9E,EAAI+E,EAAK7E,MAGpB0D,MAAA,wBACQ,IAAI7C,SAAS0D,KAAK1E,EAAG0E,KAAKzE,EAAGyE,KAAKxE,EAAGwE,KAAKvE,EAAGuE,KAAKtF,EAAGsF,KAAKtE,MAGlE6E,OAAA,gBAAOb,OACDpE,EAAoB0E,KAApB1E,EAAGC,EAAiByE,KAAjBzE,EAAGC,EAAcwE,KAAdxE,EAAGC,EAAWuE,KAAXvE,EAAGf,EAAQsF,KAARtF,EAAGgB,EAAKsE,KAALtE,SACZJ,IAAMoE,EAAOpE,GAAKC,IAAMmE,EAAOnE,GAAKC,IAAMkE,EAAOlE,GAAKC,IAAMiE,EAAOjE,GAAKf,IAAMgF,EAAOhF,GAAKgB,IAAMgE,EAAOhE,KAGhH8E,MAAA,eAAMC,EAAOC,YAAAA,IAAAA,EAAU,QACjBnG,EAAQkG,EAARlG,EAAGC,EAAKiG,EAALjG,EACNc,EAAoB0E,KAApB1E,EAAGC,EAAiByE,KAAjBzE,EAAGC,EAAcwE,KAAdxE,EAAGC,EAAWuE,KAAXvE,EAAGf,EAAQsF,KAARtF,EAAGgB,EAAKsE,KAALtE,SACjBgF,EAAUnG,EAAKA,EAAIe,EAAId,EAAIgB,EAAId,GAAM,EACrCgG,EAAUlG,EAAKD,EAAIgB,EAAIf,EAAIiB,EAAIC,GAAM,EAC9BgF,+BAjDIpF,EAAKC,EAAKC,EAAKC,EAAKf,EAAKgB,YAAzBJ,IAAAA,EAAE,YAAGC,IAAAA,EAAE,YAAGC,IAAAA,EAAE,YAAGC,IAAAA,EAAE,YAAGf,IAAAA,EAAE,YAAGgB,IAAAA,EAAE,GACtCN,EAAW4E,KAAM1E,EAAGC,EAAGC,EAAGC,EAAGf,EAAGgB,GA4D3B,SAASiF,gBAAgB9G,EAASkG,EAAStB,EAAemC,OAC3D/G,IAAYA,EAAQE,aAAeC,GAAQC,EAAQJ,IAAU0C,kBAAoB1C,SAC9E,IAAIyC,OAERuE,EAAa7D,EAAmBnD,GAEnCiH,EADM3G,EAAUN,GACF4D,EAAYC,EAC1BgB,EAAYF,EAAe3E,EAAS4E,GACpCsC,EAAKD,EAAM,GAAGE,wBACdb,EAAKW,EAAM,GAAGE,wBACdC,EAAKH,EAAM,GAAGE,wBACdlC,EAASJ,EAAU3E,WACnBmH,GAAWN,GAAwBxC,EAASvE,GAC5CwB,EAAI,IAAIiB,IACN6D,EAAGL,KAAOiB,EAAGjB,MAAQ,KACrBK,EAAGN,IAAMkB,EAAGlB,KAAO,KACnBoB,EAAGnB,KAAOiB,EAAGjB,MAAQ,KACrBmB,EAAGpB,IAAMkB,EAAGlB,KAAO,IACpBkB,EAAGjB,MAAQoB,EAAU,EAAIpD,KACzBiD,EAAGlB,KAAOqB,EAAU,EAAIvD,SAE1BmB,EAAO/B,YAAY2B,GACfmC,MACHE,EAAKF,EAAW9B,OACTgC,MACNZ,EAAKU,EAAWE,IACb1D,OAAS8C,EAAG7C,OAAS,EACxB6C,EAAG5C,gBAAgB,EAAG4C,UAGjBJ,EAAU1E,EAAE0E,UAAY1E,EC1Sf,SAAhB8F,UAAyC,oBAAZ9E,OAClB,SAAX+E,WAAiBC,IAASF,MAAoBE,GAAOhF,OAAOgF,OAASA,GAAKC,gBAAkBD,GAC9E,SAAdE,EAAcC,SAA2B,mBAAXA,EAClB,SAAZC,EAAYD,SAA2B,iBAAXA,EACb,SAAfE,EAAeF,eAA2B,IAAXA,EAClB,SAAbG,YAAmB,EAGV,SAATC,GAASJ,UAASK,KAAKC,MAAc,IAARN,GAAiB,IAE7B,SAAjBO,GAAkBzH,EAAMF,OACnBM,EAAIV,GAAKW,gBAAkBX,GAAKW,iBAAiBP,GAAM,gCAAgCQ,QAAQ,SAAU,QAASN,GAAQN,GAAKa,cAAcP,UAC1II,EAAEQ,MAAQR,EAAIV,GAAKa,cAAcP,GAajC,SAAR0H,GAASC,EAAKC,OACEC,EAAXC,EAAO,OACND,KAAKF,EACTG,EAAKD,GAAKD,EAASD,EAAIE,GAAKD,EAASD,EAAIE,UAEnCC,EAU2B,SAAnCC,GAAoCC,EAAUd,WAE5Ce,EADGzI,EAAIwI,EAASvD,OAEVjF,KACN0H,EAASc,EAASxI,GAAGoB,MAAMsH,YAAchB,EAASc,EAASxI,GAAGoB,MAAMgE,eAAe,iBACnFqD,EAAWD,EAASxI,GAAGyI,WACXA,EAASxD,QAAUsD,GAAiCE,EAAUf,GAGzD,SAAnBiB,YAAyBC,GAAaC,QAAQ,SAAAC,UAAQA,MAOhC,SAAtBC,YAA6BH,GAAa3D,QAAUsC,GAAKyB,OAAOC,OAAON,IAC9C,SAAzBO,GAAyBJ,WACpB9I,EAAI4I,GAAa3D,OACdjF,KACF4I,GAAa5I,KAAO8I,GACvBF,GAAaO,OAAOnJ,EAAG,GAGzBuH,GAAK6B,GAAGL,GAAqB,CAACM,WAAU,EAAMC,MAAM,GAAIC,SAAS,EAAGC,WAAWT,GAAqBU,KAAK,eAU3F,SAAfC,GAAgB3J,EAASS,EAAMsI,EAAMa,MAChC5J,EAAQ6J,iBAAkB,KACzBC,EAAYC,GAAkBtJ,GAClCmJ,EAAUA,IAAYI,EAAmB,CAACC,SAAS,GAAS,MAC5DjK,EAAQ6J,iBAAiBC,GAAarJ,EAAMsI,EAAMa,GACjDE,GAAarJ,IAASqJ,GAAc9J,EAAQ6J,iBAAiBpJ,EAAMsI,EAAMa,IAG1D,SAAlBM,GAAmBlK,EAASS,EAAMsI,EAAMa,MACnC5J,EAAQmK,oBAAqB,KAC5BL,EAAYC,GAAkBtJ,GAClCT,EAAQmK,oBAAoBL,GAAarJ,EAAMsI,EAAMa,GACpDE,GAAarJ,IAASqJ,GAAc9J,EAAQmK,oBAAoB1J,EAAMsI,EAAMa,IAG7D,SAAlBQ,GAAkBC,GACjBA,EAAMC,gBAAkBD,EAAMC,iBAC9BD,EAAME,qBAAuBF,EAAME,sBAUT,SAA3BC,GAA2BH,GAC1BI,GAAoBJ,EAAMK,SAAWC,GAAaN,EAAMK,QAAQxF,OAChEgF,GAAgBG,EAAMO,OAAQ,WAAYJ,IAGnB,SAAxBK,GAAwBR,GACvBI,GAAoBJ,EAAMK,SAAWC,GAAaN,EAAMK,QAAQxF,OAChEyE,GAAaU,EAAMO,OAAQ,WAAYJ,IAErB,SAAnB1G,GAAmBxB,UAAOR,GAAKiC,aAAgBzB,EAAI0B,WAAa1B,EAAII,gBAAgBsB,WAAa1B,EAAIK,KAAKqB,WAAa,EACnG,SAApBC,GAAoB3B,UAAOR,GAAKoC,aAAe5B,EAAI6B,YAAc7B,EAAII,gBAAgByB,YAAc7B,EAAIK,KAAKwB,YAAc,EACrG,SAArB2G,GAAsBjK,EAAGkK,GACxBpB,GAAa9I,EAAG,SAAUkK,GACrBC,GAAQnK,EAAEX,aACd4K,GAAmBjK,EAAEX,WAAY6K,GAGX,SAAxBE,GAAyBpK,EAAGkK,GAC3Bb,GAAgBrJ,EAAG,SAAUkK,GACxBC,GAAQnK,EAAEX,aACd+K,GAAsBpK,EAAEX,WAAY6K,GAItB,SAAhBG,GAAiBlL,EAASmL,OACrBC,EAAgB,MAATD,EAAgB,QAAU,SACpCE,EAAS,SAAWD,EACpBE,EAAS,SAAWF,SACdpD,KAAKuD,IAAI,EAAGP,GAAQhL,GAAWgI,KAAKuD,IAAIxJ,GAAYsJ,GAASrJ,EAAMqJ,KAAYvJ,GAAK,QAAUsJ,IAAQrJ,GAAYuJ,IAAWtJ,EAAMsJ,IAAWtL,EAAQqL,GAAUrL,EAAQsL,IAE5J,SAApBE,GAAqB3K,EAAG4K,OACnB/K,EAAIwK,GAAcrK,EAAG,KACxBF,EAAIuK,GAAcrK,EAAG,KAClBmK,GAAQnK,GACXA,EAAI6K,GAEJF,GAAkB3K,EAAEX,WAAYuL,GAEjC5K,EAAE8K,cAAgBjL,EAClBG,EAAE+K,cAAgBjL,EACb8K,IACJ5K,EAAEgL,WAAahL,EAAEsD,YAAc,EAC/BtD,EAAEiL,WAAajL,EAAEmD,WAAa,GAGpB,SAAZ+H,GAAa/L,EAASgM,EAAUrE,OAC3BtG,EAAQrB,EAAQqB,MACfA,IAGDwG,EAAaxG,EAAM2K,MACtBA,EAAWC,EAAaD,EAAUhM,IAAYgM,GAElC,MAATrE,EACHtG,EAAMgE,gBAAkBhE,EAAMgE,eAAe2G,EAASjL,QAAQ,WAAY,OAAOuD,eAEjFjD,EAAM2K,GAAYrE,GAGA,SAApBuE,GAAoBlM,UAAW8B,GAAK0C,iBAAkBxE,aAAmBmM,QAAWnM,EAAUA,EAAQoM,OAASpM,EAAQE,YAAc,IAAIkM,MAAQpM,GAGpI,SAAbqM,GAAaxL,MACRA,IAAMiB,UACTwK,EAAUrG,KAAOqG,EAAUtG,IAAM,EACjCsG,EAAUC,MAAQD,EAAUE,MAAQzK,GAAY0K,aAAe5L,EAAE6L,YAAc1K,EAAMyK,aAAe,EACpGH,EAAUK,OAASL,EAAUM,QAAW/L,EAAEgM,aAAe,GAAK,GAAK9K,GAAY+K,aAAgB/K,GAAY+K,aAAejM,EAAEgM,aAAe7K,EAAM8K,cAAgB,EAC1JR,MAEJhK,EAAMzB,EAAE0B,eAAiBpC,GAC5B4M,EAAKlF,EAAahH,EAAEmM,OAAsLnM,EAAE6D,UAAamD,EAAahH,EAAEoF,OAAU4B,EAAahH,EAAEmF,KAAYiH,GAASpM,GAAG,GAAGsG,wBAAnBtG,EAA5O,CAACoF,KAAMpF,EAAEmM,MAAQ/I,GAAkB3B,GAAM0D,IAAKnF,EAAEqM,MAAQpJ,GAAiBxB,GAAMkK,MAAO3L,EAAEmM,MAAQ/I,GAAkB3B,GAAO,EAAGsK,OAAQ/L,EAAEqM,MAAQpJ,GAAiBxB,GAAO,UAChMuF,EAAakF,EAAEP,SAAW3E,EAAakF,EAAER,QAC5CQ,EAAEP,MAAQO,EAAE9G,KAAO8G,EAAER,MACrBQ,EAAEH,OAASG,EAAE/G,IAAM+G,EAAEJ,QACX9E,EAAakF,EAAER,SACzBQ,EAAI,CAACR,MAAOQ,EAAEP,MAAQO,EAAE9G,KAAM0G,OAAQI,EAAEH,OAASG,EAAE/G,IAAKwG,MAAOO,EAAEP,MAAOvG,KAAM8G,EAAE9G,KAAM2G,OAAQG,EAAEH,OAAQ5G,IAAK+G,EAAE/G,MAEzG+G,EAGS,SAAjBI,GAAkBvC,EAAQnK,EAAM2M,OAI9BC,EAHGC,EAAO1C,EAAO0C,KACjBvC,EAAWuC,EAAKF,GAChBG,EAAY3C,EAAO4C,WAAW/M,UAE3BiH,EAAYqD,KACfsC,EAAStC,EAASpE,MAAM2G,EAAKG,eAAiB7C,EAAQ0C,EAAKF,EAAe,WAAa,CAACxC,EAAO8C,gBAE5FH,IAA4C,IAA/B3C,EAAO+C,cAAclN,KACrC4M,GAAS,GAEHA,EAEK,SAAbO,GAAchD,EAAQiD,OAEpB7H,EAAKC,EAAM6H,EADRjN,EAAIoM,GAASrC,GAAQ,UAEpB/J,EAAE6D,UAAY7D,IAAMiB,GASlBiM,EAAkBlN,EAAGgN,GARtBhG,EAAa+C,EAAO3E,MAMlB,CAACA,KAFRA,EAAO2E,EAAOoD,KAAOpD,EAAOqD,MAAQrD,EAAOsD,aAAe,EAEvClI,IADnBA,EAAM4E,EAAOoD,KAAOpD,EAAOuD,MAAQ,EACP5B,OAAO3B,EAAOW,KAAOX,EAAOwD,MAAQxD,EAAOyD,aAAe,GAAKpI,EAAM0G,QAAQ/B,EAAOW,KAAOX,EAAO0D,MAAQ,GAAKtI,IAL1I8H,EAAS,CAACpN,EAAE,EAAGC,EAAE,GACV,CAACsF,KAAM2E,EAAO3E,KAAO6H,EAAOpN,EAAGsF,IAAK4E,EAAO5E,IAAM8H,EAAOnN,EAAG4L,MAAO3B,EAAO2B,MAAOI,OAAQ/B,EAAO+B,SAwDzF,SAAhB4B,GAAiBC,EAAWC,EAAMlD,EAAKyC,EAAK3F,EAAQqG,OAElDjN,EAAGxB,EAAG0O,EADHrB,EAAO,MAEPmB,KACY,IAAXpG,GAAgBoG,aAAgBG,MAAO,IAC1CtB,EAAKuB,IAAMpN,EAAI,GACfkN,EAAIF,EAAKvJ,OACL0C,EAAU6G,EAAK,QACbxO,EAAI,EAAGA,EAAI0O,EAAG1O,IAClBwB,EAAExB,GAAKkI,GAAMsG,EAAKxO,GAAIoI,YAGlBpI,EAAI,EAAGA,EAAI0O,EAAG1O,IAClBwB,EAAExB,GAAKwO,EAAKxO,GAAKoI,EAGnBkD,GAAO,IACPyC,GAAO,SACGtG,EAAY+G,GACtBnB,EAAKuB,IAAM,SAAAlH,OAETY,EAAMD,EADH+E,EAASoB,EAAKK,KAAKN,EAAW7G,MAEnB,IAAXU,KACCT,EAAUyF,GAAS,KAEjB/E,KADLC,EAAO,GACG8E,EACT9E,EAAKD,GAAK+E,EAAO/E,GAAKD,EAEvBgF,EAAS9E,OAET8E,GAAUhF,SAGLgF,GAGRC,EAAKuB,IAAMJ,SAGTlD,GAAe,IAARA,IACV+B,EAAK/B,IAAMA,IAERyC,GAAe,IAARA,IACVV,EAAKU,IAAMA,GAERU,IACHpB,EAAKyB,SAAW,GAEVzB,EAEO,SAAf0B,GAAehP,OACV0J,WACK1J,IAAYA,EAAQQ,cAAgBR,IAAYgC,OAAsE,UAAnD0H,EAAO1J,EAAQQ,aAAa,qBAA2C,UAATkJ,IAAqBuF,EAAiBC,KAAKlP,EAAQmP,SAAW,KAAmD,SAA5CnP,EAAQQ,aAAa,sBAA0CwO,GAAahP,EAAQE,aAEnR,SAAjBkP,GAAkB3G,EAAU4G,WAE1BxO,EADGZ,EAAIwI,EAASvD,OAEVjF,MACNY,EAAI4H,EAASxI,IACXqP,YAAczO,EAAE0O,cAAgBF,EAAa,KAAOvH,GACtDN,GAAKgI,IAAI3O,EAAG,CAAC4O,MAAK,EAAMC,WAAaL,EAAa,OAAS,SAe/C,SAAdM,GAAuB3P,EAASsN,GAC/BtN,EAAUwH,GAAKoI,MAAMC,QAAQ7P,GAAS,GACtCsN,EAAOA,GAAQ,OAadwC,EAAcC,EAAeC,EAAeC,EAAUC,EAAgBC,EAZnEC,EAAUC,SAASrP,cAAc,OACpCK,EAAQ+O,EAAQ/O,MAChBiP,EAAOtQ,EAAQuQ,WACfxK,EAAY,EACZD,EAAa,EACb0K,EAAUxQ,EAAQgE,UAClByM,EAAWzQ,EAAQmE,WACnBuM,EAAc1Q,EAAQ0Q,YACtBC,EAAe3Q,EAAQ2Q,aACvBC,EAAgB,EAChBC,EAAU,EACVC,EAAS,EAENC,IAAgC,IAAjBzD,EAAK0D,SACvBd,EAAiB,eACjBC,EAAe,WACL/N,IACV8N,EAAiB,aACjBC,EAAe,YAEXnM,UAAY,SAAS2D,EAAOsJ,OAC3BC,UAAUhM,cACNiB,KAAKH,WAETA,KAAK2B,EAAOsJ,SAEb9M,WAAa,SAASwD,EAAOsJ,OAC5BC,UAAUhM,cACNiB,KAAKF,YAETA,MAAM0B,EAAOsJ,SAEdhL,KAAO,SAAS0B,EAAOsJ,OACtBC,UAAUhM,eACLlF,EAAQmE,WAAa2B,OAE3BqL,EAAMnR,EAAQmE,WAAasM,EAC9BW,EAAYtL,MACF,EAANqL,GAAWA,GAAO,KAAOF,SAC7BR,EAAWzQ,EAAQmE,WACnBqD,GAAK6J,aAAalL,KAAM,CAACF,KAAK,EAAG9B,WAAW,SACvC8B,MAAMwK,QACPnD,EAAKgE,QACRhE,EAAKgE,WAIP3J,GAASA,GACG,GACX7B,EAAc6B,EAAQ,GAAO,EAC7BA,EAAQ,GACUkJ,EAARlJ,GACV7B,EAAc6B,EAAQkJ,EAAW,EACjClJ,EAAQkJ,GAER/K,EAAa,GAEVA,GAAcsL,KACZjL,KAAKoL,QACTlQ,EAAMe,GAAkB8N,GAAkBpK,EAAa,OAASC,EAAYoK,GAE3C,GAA9BrK,EAAa8K,IAChBvP,EAAMmQ,aAAgB1L,EAAa8K,EAAgB,OAGrD5Q,EAAQmE,WAAqB,EAARwD,EACrB8I,EAAWzQ,EAAQmE,iBAEf6B,IAAM,SAAS2B,EAAOsJ,OACrBC,UAAUhM,eACLlF,EAAQgE,UAAY+B,OAE1BoL,EAAMnR,EAAQgE,UAAYwM,EAC7BY,EAAYrL,MACF,EAANoL,GAAWA,GAAO,KAAOF,SAC7BT,EAAUxQ,EAAQgE,UAClBwD,GAAK6J,aAAalL,KAAM,CAACH,IAAI,EAAGhC,UAAU,SACrCgC,KAAKwK,QACNlD,EAAKgE,QACRhE,EAAKgE,WAIP3J,GAASA,GACG,GACX5B,EAAa4B,EAAQ,GAAO,EAC5BA,EAAQ,GACUmJ,EAARnJ,GACV5B,EAAa4B,EAAQmJ,EAAU,EAC/BnJ,EAAQmJ,GAER/K,EAAY,GAETA,GAAaqL,KACXjL,KAAKoL,QACTlQ,EAAMe,GAAkB8N,GAAkBpK,EAAa,OAASC,EAAYoK,IAG9EnQ,EAAQgE,UAAoB,EAAR2D,EACpB6I,EAAUxQ,EAAQgE,gBAGdyN,aAAe,kBAAMX,QACrBY,cAAgB,kBAAMb,QAEtBc,QAAU,eACdrB,EAAOF,EAAQG,WACRD,GACNL,EAAWK,EAAKsB,YAChB5R,EAAQmB,YAAYmP,GACpBA,EAAOL,EAEJjQ,IAAYoQ,EAAQlQ,YACvBF,EAAQkD,YAAYkN,SAGjByB,OAAS,eACbvB,EAAOtQ,EAAQuQ,cACFH,QAGNE,GACNL,EAAWK,EAAKsB,YAChBxB,EAAQjP,YAAYmP,GACpBA,EAAOL,EAERjQ,EAAQmB,YAAYiP,QACf0B,mBAEDA,UAAY,SAASb,OAExBnM,EAAIpE,EAAGC,EADJoR,EAAgB/R,EAAQyM,cAAgBqD,EAE5CU,EAAUxQ,EAAQgE,UAClByM,EAAWzQ,EAAQmE,WACf4N,GAAgB/R,EAAQ8M,eAAiBiD,GAAiBK,EAAQ4B,eAAiBhC,GAAiBU,IAAgB1Q,EAAQ0Q,aAAeC,IAAiB3Q,EAAQ2Q,eAAiBM,KAGrLlL,GAAaD,KAChBpF,EAAIyF,KAAKF,OACTtF,EAAIwF,KAAKH,WACJC,MAAMjG,EAAQmE,iBACd6B,KAAKhG,EAAQgE,YAEnBc,EAAKoH,GAAkBlM,GAElB+R,IAAgBd,IACpB5P,EAAM4Q,QAAU,QAChB5Q,EAAMkL,MAAQ,OACdlL,EAAMmQ,aAAe,OACrBZ,EAAgB5I,KAAKuD,IAAI,EAAGvL,EAAQ0Q,YAAc1Q,EAAQyM,gBAGzDmE,GAAiBsB,WAAWpN,EAAGqN,cAAgBC,EAAgBF,WAAWpN,EAAG0M,cAAgB,KAG/FnQ,EAAM4Q,QAAU,eAChB5Q,EAAMoD,SAAW,WACjBpD,EAAMgR,SAAW,UACjBhR,EAAMiR,cAAgB,MACtBjR,EAAMkR,UAAY,cAClBlR,EAAMkL,MAAQ,OACdlL,EAAMmQ,aAAeZ,EAAgB,KAEjCwB,IACH/Q,EAAMmR,cAAgB1N,EAAG0N,eAE1B1C,EAAe9P,EAAQyM,YACvBsD,EAAgB/P,EAAQ8M,aACxB4D,EAAc1Q,EAAQ0Q,YACtBC,EAAe3Q,EAAQ2Q,aACvBE,EAAU7Q,EAAQ0Q,YAAcZ,EAChCgB,EAAS9Q,EAAQ2Q,aAAeZ,EAChCC,EAAgBI,EAAQ4B,aACxB3Q,EAAM4Q,QAAU,SACZvR,GAAKC,UACHsF,KAAKvF,QACLsF,IAAIrF,WAGNyP,QAAUA,OACVpQ,QAAUA,OACVuR,OAAQ,OACRM,SAEM,SAAZY,GAAYC,MACPpL,KAAmB+I,SAAS1N,KAAM,KACjCgQ,EAAMnQ,QAAUA,OAAOoQ,UAC3B9Q,GAAOU,OACPrC,GAAOkQ,SACPtO,GAAc5B,GAAKuC,gBACnBV,EAAQ7B,GAAKwC,KACbkQ,EAAW3K,GAAe,OAC1B4K,KAAqBtQ,OAAOuQ,cAC5BC,GAAkB9K,GAAe,QACjB7G,MAAMC,QAAU,qGAChC2R,GAAkD,SAAjCD,GAAgB3R,MAAM6R,OAAoB,OAAS,OACpEC,GAAcR,IAA2D,IAApDA,EAAIS,UAAU9O,cAAc+O,QAAQ,WACzDC,GAAmB,iBAAkBvR,IAAiB,gBAAiBD,IAAW6Q,IAA6B,EAArBA,EAAIY,gBAA6C,EAAvBZ,EAAIa,kBAEnHC,EAAMvL,GAAe,OACxBwL,EAAQxL,GAAe,OACvByL,EAAaD,EAAMrS,MACnB4D,EAASjD,EAEV2R,EAAW1B,QAAU,eACrB0B,EAAWlP,SAAW,WACtBgP,EAAIpS,MAAMC,QAAU,sEACpBmS,EAAItS,YAAYuS,GAChBzO,EAAO9D,YAAYsS,GACnBG,EAAOF,EAAM1B,aAAe,GAAKyB,EAAI9C,aACrC1L,EAAO/B,YAAYuQ,GAZpBrB,EAaQwB,EAER7J,GAAqB,SAAS8J,WACzBC,EAAWD,EAAME,MAAM,KAC1BC,IAAa,kBAAmBnB,EAAW,kDAAoD,oBAAqBA,EAAW,0DAA4DgB,GAAOE,MAAM,MACxM3L,EAAM,GACNnI,EAAI,GACS,IAALA,GACRmI,EAAI0L,EAAS7T,IAAM+T,EAAU/T,GAC7BmI,EAAI4L,EAAU/T,IAAM6T,EAAS7T,OAI7B8B,GAAY8H,iBAAiB,OAAQ,KAAMoK,OAAOC,eAAe,GAAI,UAAW,CAC/E3Q,IAAK,eACJyG,EAAmB,MAGpB,MAAOnJ,WACFuH,EAjBa,CAkBnB,6CACFuB,GAAaxJ,GAAM,cAAe2H,IAClC6B,GAAa7H,GAAM,YAAagG,IAChC9F,GAASA,EAAM6H,iBAAiB,aAAc/B,IAC9C6B,GAAaxJ,GAAM,cAAe,eAC5B,IAAImI,KAAK6L,GACTA,GAAQ7L,GAAG8L,WACdD,GAAQ7L,GAAG+L,YAId7M,GAAO8M,GAAe/M,IA5CL,IAKfqM,EAJGH,EACHC,EACAC,EACA1O,EA0CCuC,IACH+M,GAAgB/M,GAAKgN,QAAQC,QAC7BC,GAAWlN,GAAKmN,KAAK9G,SAAW,aAChC5B,EAAezE,GAAKoI,MAAMgF,YAC1BxS,EAAiB6J,EAAa7J,GAC9BC,GAAuB4J,EAAa5J,IACpC4K,GAAWzF,GAAKoI,MAAMC,QACtBgF,GAAiBrN,GAAKmN,KAAKG,cAC3B/D,IAAgB9E,EAAa,gBACnByG,GACVqC,QAAQC,KAAK,yCA9kBhB,IAAIxN,GAAM1F,GAAM3B,GAAM4B,GAAaC,EAAO6Q,EAAUG,GAAiBsB,GAAcrI,EAAcgB,GAAUjD,EAAkBsJ,GAAgBvJ,GAAmBU,GAAkB0I,GAAYoB,GAAetB,GAAgBH,GAAkB4B,GAAUG,GA0UxP9D,EAAaqB,IAzUbzH,GAAa,EAObvI,EAAiB,YACjBC,GAAuB,kBAEvB4S,GAAWrG,MAAMsG,QAKjBC,GAAW,IAAMnN,KAAKoN,GACtBC,GAAU,KACVpT,EAAkB,IAAIQ,GACtB6S,GAAWC,KAAKC,KAAQ,kBAAM,IAAID,MAAOE,WACzC5M,GAAe,GACfsL,GAAU,GACVuB,GAAe,EACfzG,EAAmB,wCACnB0G,GAAgB,EAChBC,GAAS,GACTlK,GAAe,GAoGfV,GAAU,SAAVA,QAAUnK,WAASA,GAAKA,IAAMkB,IAA8B,IAAflB,EAAE6D,UAAkB7D,IAAMV,GAAKwC,MAAQ9B,IAAMiB,IAASjB,EAAE6D,UAAa7D,EAAEX,aAsCpHoM,EAAY,GA8CZuJ,GAAU,GACV9H,EAAoB,SAApBA,kBAAqB/N,EAAS6N,GAC7BA,EAAUZ,GAASY,GAAS,OAG3B5H,EAAMuG,EAAOxG,EAAK4G,EAAQ/G,EAAQiQ,EAAIC,EAAIC,EAAIC,EAAIC,EAAM3J,EAAOI,EAAQ7H,EAFpEqR,EAASnW,EAAQuF,SAAWvF,EAAQoE,gBACvC9B,EAAMtC,EAAQuC,eAAiBpC,MAE5BH,IAAY8B,GACfkE,EAAMlC,GAAiBxB,GAEvBkK,GADAvG,EAAOhC,GAAkB3B,KACTA,EAAII,gBAAgB+J,aAAezM,EAAQ0M,YAAcpK,EAAIK,KAAK8J,aAAe,GACjGG,EAAS5G,IAAShG,EAAQ6M,aAAe,GAAK,GAAKvK,EAAII,gBAAgBoK,aAAgBxK,EAAII,gBAAgBoK,aAAe9M,EAAQ6M,aAAevK,EAAIK,KAAKmK,cAAgB,OACpK,CAAA,GAAIe,IAAY/L,IAAQ+F,EAAagG,UACpC7N,EAAQmH,wBAEflB,EAAOD,EAAO,EACVmQ,GAEH5J,GADA2J,EAAOlW,EAAQuF,WACFgH,MACbI,EAASuJ,EAAKvJ,SAEV3M,EAAQoW,UAAYF,EAAOlW,EAAQoW,QAAQ5Q,WAC9CS,EAAOiQ,EAAKxV,GAAK,EACjBsF,EAAMkQ,EAAKvV,GAAK,EAChB4L,EAAQ2J,EAAK3J,MACbI,EAASuJ,EAAKvJ,QAEVJ,IAEJ2J,EAAwB,gBADxBpR,EAAKoH,GAAkBlM,IACbuS,UACVhG,GAAS2F,WAAWpN,EAAGyH,QAAUvM,EAAQyM,aAAe,IAAMyJ,EAAO,EAAIhE,WAAWpN,EAAGuR,iBAAmBnE,WAAWpN,EAAGwR,mBACxH3J,GAAUuF,WAAWpN,EAAG6H,SAAW3M,EAAQ8M,cAAgB,IAAMoJ,EAAO,EAAIhE,WAAWpN,EAAGyR,gBAAkBrE,WAAWpN,EAAG0R,sBAG5HhK,EAAQD,EACRK,EAASD,SAEN3M,IAAY6N,EACR,CAAC5H,KAAKA,EAAMD,IAAIA,EAAKuG,MAAOC,EAAQvG,EAAM0G,OAAQC,EAAS5G,IAGnE8P,GADAjQ,EAASiB,gBAAgB+G,GAAS,GAAMlI,SAASmB,gBAAgB9G,KACrD2G,MAAM,CAACjG,EAAEuF,EAAMtF,EAAEqF,IAC7B+P,EAAKlQ,EAAOc,MAAM,CAACjG,EAAE8L,EAAO7L,EAAEqF,IAC9BgQ,EAAKnQ,EAAOc,MAAM,CAACjG,EAAE8L,EAAO7L,EAAEiM,IAC9BqJ,EAAKpQ,EAAOc,MAAM,CAACjG,EAAEuF,EAAMtF,EAAEiM,IAGtB,CAAC3G,KAFRA,EAAO+B,KAAKgG,IAAI8H,EAAGpV,EAAGqV,EAAGrV,EAAGsV,EAAGtV,EAAGuV,EAAGvV,GAEjBsF,IADpBA,EAAMgC,KAAKgG,IAAI8H,EAAGnV,EAAGoV,EAAGpV,EAAGqV,EAAGrV,EAAGsV,EAAGtV,GACN4L,MAAOvE,KAAKuD,IAAIuK,EAAGpV,EAAGqV,EAAGrV,EAAGsV,EAAGtV,EAAGuV,EAAGvV,GAAKuF,EAAM0G,OAAQ3E,KAAKuD,IAAIuK,EAAGnV,EAAGoV,EAAGpV,EAAGqV,EAAGrV,EAAGsV,EAAGtV,GAAKqF,KAuVrHyQ,iCAOL5M,iBAAA,0BAAiBpJ,EAAMsK,OAClB2L,EAAOvQ,KAAKqH,WAAW/M,KAAU0F,KAAKqH,WAAW/M,GAAQ,KACvDiW,EAAKrD,QAAQtI,IAClB2L,EAAK/S,KAAKoH,MAIZZ,oBAAA,6BAAoB1J,EAAMsK,OACrB2L,EAAOvQ,KAAKqH,WAAW/M,GAC1BR,EAAKyW,GAAQA,EAAKrD,QAAQtI,GACrB,GAAL9K,GAAWyW,EAAKtN,OAAOnJ,EAAG,MAG5B0N,cAAA,uBAAclN,OACT4M,gBACHlH,KAAKqH,WAAW/M,IAAS,IAAIqI,QAAQ,SAAAiC,UAAwE,IAA3DA,EAAS+D,KAAK6H,EAAM,CAAClW,KAAMA,EAAMmK,OAAQ+L,EAAK/L,WAAwByC,GAAS,KAC3HA,6CArBIzC,QACN4C,WAAa,QACb5C,OAASA,GAAUzE,YA+BbyQ,6HAAkBH,aAstCvBI,SAAP,kBAAgBlC,GACfnN,GAAOmN,EACPlC,gBAGMqE,OAAP,gBAAcC,EAASzJ,UACtBgH,IAAgB7B,IAAU,GACnBxF,GAAS8J,GAASC,IAAI,SAAApM,UAAU,IAAIgM,UAAUhM,EAAQ0C,gBAGvD/J,IAAP,aAAWqH,UACHuJ,IAASlH,GAASrC,GAAQ,IAAM,IAAIqM,sBAGrCC,cAAP,gCACS5B,KAAaK,IAAiB,eAGhCwB,QAAP,iBAAeC,EAAMC,EAAMC,MACtBF,IAASC,SACL,MAMPE,EAASC,EAAMC,EAJZC,EAAKrL,GAAW+K,GACnBO,EAAKtL,GAAWgL,GACdrR,EAA4C0R,EAA5C1R,IAAKC,EAAuCyR,EAAvCzR,KAAMuG,EAAiCkL,EAAjClL,MAAOI,EAA0B8K,EAA1B9K,OAAQL,EAAkBmL,EAAlBnL,MAAOI,EAAW+K,EAAX/K,OACnCiL,EAAaD,EAAG1R,KAAOuG,GAASmL,EAAGnL,MAAQvG,GAAQ0R,EAAG3R,IAAM4G,GAAU+K,EAAG/K,OAAS5G,SAE/E4R,IAAcN,GACTM,GAETH,GAA8C,KAAlCH,EAAY,IAAIjE,QAAQ,KACpCiE,EAAYpF,WAAWoF,IAAc,GACrCC,EAAU,CAACtR,KAAM+B,KAAKuD,IAAItF,EAAM0R,EAAG1R,MAAOD,IAAKgC,KAAKuD,IAAIvF,EAAK2R,EAAG3R,OACxDuG,MAAQvE,KAAKgG,IAAIxB,EAAOmL,EAAGnL,OAAS+K,EAAQtR,KACpDsR,EAAQ5K,OAAS3E,KAAKgG,IAAIpB,EAAQ+K,EAAG/K,QAAU2K,EAAQvR,MACnDuR,EAAQhL,MAAQ,GAAKgL,EAAQ5K,OAAS,KAGtC8K,EAGalL,EAAQI,GAFxB2K,GAAa,OACbE,EAAOD,EAAQhL,MAAQgL,EAAQ5K,SACe6K,GAAQG,EAAGpL,MAAQoL,EAAGhL,OAAS2K,EAEtEC,EAAQhL,MAAQ+K,GAAaC,EAAQ5K,OAAS2K,mCA/vC3C1M,EAAQ0C,+BAEnBgH,IAAgB7B,GAAU,GAC1B7H,EAASqC,GAASrC,GAAQ,KACrBiN,OAAShD,IAAkBA,GAAejK,EAAQ,sBAEtD2J,GADIA,IACY/M,GAAKgN,QAAQC,UAEzBnH,KAAOA,EAAOnF,GAAMmF,GAAQ,MAC5B1C,OAASA,IACTlK,EAAIoX,EAAKnX,EAAImX,EAAKC,SAAW,IAC7BC,eAAiB9F,WAAW5E,EAAK0K,iBAAmB,IACpDC,eAAiBC,MAAM5K,EAAK2K,gBAAkB,EAAI/F,WAAW5E,EAAK2K,iBAAmB,IACrFE,SAAW7K,EAAK6K,WAChBC,WAAa9K,EAAK8K,YAAc,IAChCC,WAAa,OACbC,oBAAsBhL,EAAKgL,kBAEhC9Q,GAAK+Q,YAAY3N,EAAQ,KAuBT,SAAf4N,GAAgBxM,EAAUyM,UAASvG,WAAWwG,GAAQnV,IAAIqH,EAAQoB,EAAUyM,IAI5D,SAAhBE,GAAgB9X,UAEfuJ,GAAgBvJ,GAChBA,EAAE+X,0BAA4B/X,EAAE+X,4BACzB,EAIC,SAATC,GAASC,MACJC,EAAKX,YAAcW,EAAKC,aAAeC,IAAyBC,GAAQ,KAG1EjU,EAAQkU,EAAQC,EAAMC,EAAUC,EAAUC,EAASC,EAASC,EAFzD5Y,EAAI+J,EACP8O,EAAqC,GAAlBX,EAAKX,eAEzBa,IAAwB,EACxBvN,GAAa1H,UAAkC,MAApBlC,GAAKiC,YAAuBjC,GAAKiC,YAAqD,MAAtC4V,GAASjX,gBAAgBsB,UAAqB2V,GAASjX,gBAAgBsB,UAAY2V,GAAShX,KAAKqB,UAC5K0H,GAAavH,WAAmC,MAApBrC,GAAKoC,YAAuBpC,GAAKoC,YAAsD,MAAvCyV,GAASjX,gBAAgByB,WAAsBwV,GAASjX,gBAAgByB,WAAawV,GAAShX,KAAKwB,WAC/KkV,EAAWN,EAAKM,SAAW3N,GAAavH,WACxCmV,EAAWP,EAAKO,SAAW5N,GAAa1H,UACjCnD,IAAMsY,GAEZlU,GADAkU,EAASnO,GAAQnK,EAAEX,aACDwL,GAAe7K,EAAEX,WACnCkZ,EAAOD,EAAS,CAACvM,OAAO5E,KAAKuD,IAAIxJ,GAAY+K,aAAchL,GAAK+K,aAAe,GAAIL,MAAOxE,KAAKuD,IAAIxJ,GAAY0K,YAAa3K,GAAK4K,YAAc,GAAIzG,KAAK,EAAGD,IAAI,GAAKf,EAAOkC,wBAC3KoS,EAAUC,EAAU,EAChBI,KACHH,EAAMxU,EAAO2G,cAAgB3G,EAAOjB,WAC1B,EACTwV,EAAUC,EACAH,EAAWF,EAAKxM,OAASiN,IAA0BJ,GAC7DR,IAAwB,EACxBO,EAAUxR,KAAKgG,IAAIyL,EAAMC,GAAoB,EAAI1R,KAAKuD,IAAI,EAAI6N,EAAKxM,OAAS0M,GAAaO,IAA2B,IAC1GP,EAAWF,EAAKpT,IAAM8T,IAAuB7U,EAAOjB,YAC9DiV,IAAwB,EACxBO,GAAWxR,KAAKgG,IAAI/I,EAAOjB,UAAY0V,GAAoB,EAAI1R,KAAKuD,IAAI,EAAI+N,EAAWF,EAAKpT,KAAQ8T,IAAwB,IAEzHN,IACHvU,EAAOjB,WAAawV,IAGlBO,KACHN,EAAMxU,EAAO0G,cAAgB1G,EAAOd,YAC1B,EACToV,EAAUE,EACAJ,EAAWD,EAAK5M,MAAQwN,IAAyBP,GAC3DR,IAAwB,EACxBM,EAAUvR,KAAKgG,IAAIyL,EAAMC,GAAoB,EAAI1R,KAAKuD,IAAI,EAAI6N,EAAK5M,MAAQ6M,GAAaW,IAA0B,IACxGX,EAAWD,EAAKnT,KAAOgU,IAAwBhV,EAAOd,aAChE8U,IAAwB,EACxBM,GAAWvR,KAAKgG,IAAI/I,EAAOd,WAAauV,GAAoB,EAAI1R,KAAKuD,IAAI,EAAI8N,EAAWD,EAAKnT,MAASgU,IAAyB,IAE5HV,IACHtU,EAAOd,YAAcoV,IAInBJ,IAAWI,GAAWC,KACzB1X,GAAKoY,SAASjV,EAAOd,WAAYc,EAAOjB,WACxCmW,GAAmBpB,EAAKM,SAAWE,EAASR,EAAKO,SAAWE,IAE7D3Y,EAAIoE,KAGFiU,EAAO,KACLxY,EAAQqY,EAARrY,EAAGC,EAAKoY,EAALpY,EACJyZ,GACHrB,EAAKsB,OAAS3Z,EAAIwR,WAAWwG,GAAQX,UACrCgB,EAAKhB,SAAWrX,EAChBgY,GAAQX,SAAWrX,EAAI,MACvBgY,GAAQhV,gBAAgB,EAAGgV,KAEvB4B,GACCV,IACHb,EAAKwB,OAAS5Z,EAAI2Z,EAAYtU,MAC9BsU,EAAYtU,IAAIrF,IAEboZ,IACHhB,EAAKsB,OAAS3Z,EAAI4Z,EAAYrU,OAC9BqU,EAAYrU,KAAKvF,KAER8Z,GACNZ,IACHb,EAAKwB,OAAS5Z,EAAIuR,WAAWwG,GAAQ/X,GACrC+X,GAAQ/X,EAAIA,EAAI,MAEboZ,IACHhB,EAAKsB,OAAS3Z,EAAIwR,WAAWwG,GAAQhY,GACrCgY,GAAQhY,EAAIA,EAAI,MAEjBgY,GAAQhV,gBAAgB,EAAGgV,MAEvBkB,IACHb,EAAKwB,OAAS5Z,EAAIuR,WAAWtH,EAAOvJ,MAAM2E,KAAO,GACjD4E,EAAOvJ,MAAM2E,IAAMrF,EAAI,MAEpBoZ,IACHhB,EAAKsB,OAAS3Z,EAAIwR,WAAWtH,EAAOvJ,MAAM4E,MAAQ,GAClD2E,EAAOvJ,MAAM4E,KAAOvF,EAAI,QAIvB+Z,GAAoB3B,GAAmB4B,MAC1CA,GAAgB,KACZvN,GAAe4L,EAAM,OAAQ,YAC5BgB,IACHhB,EAAKrY,GAAKqY,EAAKsB,QAEZT,IACHb,EAAKpY,GAAKoY,EAAKwB,QAEhB1B,IAAO,IAER6B,GAAgB,GAGlBxB,GAAQ,EAIA,SAATyB,GAAUC,EAAcC,OAEtBC,EAAchW,EADTpE,EAASqY,EAATrY,EAAGC,EAAMoY,EAANpY,EAEJiK,EAAOvH,QACXqV,GAAUlR,GAAKmN,KAAKoG,SAASnQ,IAE9B8N,GAAQpV,SAAWkE,GAAK+Q,YAAY3N,EAAQ,KACxC4P,GACHzB,EAAKrY,EAAIwR,WAAWwG,GAAQhY,GAC5BqY,EAAKpY,EAAIuR,WAAWwG,GAAQ/X,IAClByZ,EACVrB,EAAKrY,EAAIqY,EAAKhB,SAAW7F,WAAWwG,GAAQX,UAClCuC,GACVvB,EAAKpY,EAAI2Z,EAAYtU,MACrB+S,EAAKrY,EAAI4Z,EAAYrU,SAErB8S,EAAKpY,EAAIuR,WAAWtH,EAAOvJ,MAAM2E,MAASlB,EAAKoH,GAAkBtB,KAAY9F,EAAGkB,MAAS,EACzF+S,EAAKrY,EAAIwR,WAAWtH,EAAOvJ,MAAM4E,OAASnB,GAAM,IAAImB,OAAS,IAEzD+U,GAASC,GAASC,KAAYL,IAAa9B,EAAKC,YAAcD,EAAKoC,cACnED,IACHtF,GAAOlV,EAAIqY,EAAKrY,EAChBkV,GAAOjV,EAAIoY,EAAKpY,GAChBma,EAAeI,EAAOtF,KACLlV,IAAMqY,EAAKrY,IAC3BqY,EAAKrY,EAAIoa,EAAapa,EACtBwY,GAAQ,GAEL4B,EAAana,IAAMoY,EAAKpY,IAC3BoY,EAAKpY,EAAIma,EAAana,EACtBuY,GAAQ,IAGN8B,IACHF,EAAeE,EAAMjC,EAAKrY,MACLqY,EAAKrY,IACzBqY,EAAKrY,EAAIoa,EACLV,IACHrB,EAAKhB,SAAW+C,GAEjB5B,GAAQ,GAGN+B,KACHH,EAAeG,EAAMlC,EAAKpY,MACLoY,EAAKpY,IACzBoY,EAAKpY,EAAIma,GAEV5B,GAAQ,IAGVA,GAASL,IAAO,GACX+B,IACJ7B,EAAKsB,OAAStB,EAAKrY,EAAIA,EACvBqY,EAAKwB,OAASxB,EAAKpY,EAAIA,EACvBwM,GAAe4L,EAAM,cAAe,kBAItB,SAAhBqC,GAAiB3M,EAAMT,EAAKzC,EAAKlD,UACrB,MAAP2F,IACHA,GAAOqH,IAEG,MAAP9J,IACHA,EAAM8J,IAEH3N,EAAY+G,GACR,SAAA4M,OACFC,EAAiBvC,EAAK3E,UAAgB,EAAI2E,EAAKd,eAAb,SAC/BxJ,EAAKK,KAAKiK,GAAWxN,EAAJ8P,EAAU9P,GAAO8P,EAAI9P,GAAO+P,EAAiBD,EAAIrN,EAAOA,GAAOqN,EAAIrN,GAAOsN,EAAgBD,GAAKhT,GAAUA,GAG/H4M,GAASxG,GACL,SAAA4M,WAILzH,EAAKzC,EAHFlR,EAAIwO,EAAKvJ,OACZqW,EAAU,EACVC,EAASnG,IAEI,IAALpV,IAERkR,GADAyC,EAAMnF,EAAKxO,IACCob,GACF,IACTlK,GAAOA,GAEJA,EAAMqK,GAAiBxN,GAAP4F,GAAcA,GAAOrI,IACxCgQ,EAAUtb,EACVub,EAASrK,UAGJ1C,EAAK8M,IAGPrD,MAAMzJ,GAAQ,SAAA4M,UAAKA,GAAI,kBAAM5M,EAAOpG,GAuD1B,SAAlBoT,SACKC,EAAQC,EAAclN,EAAMmN,EAChCC,GAAY,EACRvB,GACHA,EAAYxI,YACZiH,EAAK9K,KAAOA,GAAQqM,EAAY5I,gBAChCqH,EAAK5K,KAAOA,GAAQmM,EAAY7I,eAChCsH,EAAK3K,KAAOA,EAAO2K,EAAKzK,KAAOA,EAAO,EACtCuN,GAAY,GACAvO,EAAKoO,SACjBA,EAAS9N,GAAWN,EAAKoO,OAAQ9Q,EAAO1K,YACpCka,GACHrB,EAAK9K,KAAOA,EAAOyN,EAAOzV,KAC1B8S,EAAK3K,KAAOA,EAAOsN,EAAOzV,KAAOyV,EAAOnP,MACxCwM,EAAK5K,KAAOA,EAAO4K,EAAKzK,KAAOA,EAAO,GAC3BzG,EAAayF,EAAKoO,OAAOtN,OAAUvG,EAAayF,EAAKoO,OAAOpN,OAOvEqN,EAAe/N,GAAWhD,EAAQA,EAAO1K,YACzC6Y,EAAK9K,KAAOA,EAAOjG,KAAKC,MAAMuQ,GAAasD,EAAO,MAAQJ,EAAOzV,KAAO0V,EAAa1V,MACrF8S,EAAK5K,KAAOA,EAAOnG,KAAKC,MAAMuQ,GAAauD,EAAO,MAAQL,EAAO1V,IAAM2V,EAAa3V,KACpF+S,EAAK3K,KAAOA,EAAOpG,KAAKC,MAAMgG,GAAQyN,EAAOnP,MAAQoP,EAAapP,QAClEwM,EAAKzK,KAAOA,EAAOtG,KAAKC,MAAMkG,GAAQuN,EAAO/O,OAASgP,EAAahP,WAVnE+O,EAASpO,EAAKoO,OACd3C,EAAK9K,KAAOA,EAAOyN,EAAOzN,KAC1B8K,EAAK5K,KAAOA,EAAOuN,EAAOvN,KAC1B4K,EAAK3K,KAAOA,EAAOsN,EAAOtN,KAC1B2K,EAAKzK,KAAOA,EAAOoN,EAAOpN,MAQhBF,EAAPH,IACH8K,EAAK9K,KAAOG,EACZ2K,EAAK3K,KAAOA,EAAOH,EACnBA,EAAO8K,EAAK9K,MAEFK,EAAPH,IACH4K,EAAK5K,KAAOG,EACZyK,EAAKzK,KAAOA,EAAOH,EACnBA,EAAO4K,EAAK5K,MAETiM,IACHrB,EAAK7K,YAAcD,EACnB8K,EAAK1K,YAAcD,GAEpByN,GAAY,GAETvO,EAAK0O,WACRvN,GAA0B,IAAlBnB,EAAK0O,SAAsB1O,EAAKmB,MAAQ,GAAMnB,EAAK0O,SAC3DJ,EAAa3G,GAASxG,IAAS/G,EAAY+G,GACvC2L,GACHY,EAAQI,GAAeQ,EAAYnN,EAAOA,EAAKsJ,SAAW9J,EAAMG,EAAM,GACtE6M,EAAQ,MAEJxM,EAAKwN,OACRf,EAxGiB,SAArBgB,mBAAsBzN,EAAMR,EAAMG,EAAMD,EAAMG,EAAM6N,EAAQ9T,UAC3D8T,EAAUA,GAAUA,EAAS9G,GAAW8G,EAASA,EAAS9G,GACtD3N,EAAY+G,GACR,SAAA7H,OAILyG,EAAQ+O,EAAIC,EAHTf,EAAiBvC,EAAK3E,UAAgB,EAAI2E,EAAKd,eAAb,EACrCvX,EAAIkG,EAAMlG,EACVC,EAAIiG,EAAMjG,SAEXiG,EAAMlG,EAAIA,EAAS0N,EAAJ1N,EAAW0N,GAAQ1N,EAAI0N,GAAQkN,EAAiB5a,EAAIuN,EAAQA,GAAQvN,EAAIuN,GAAQqN,EAAgB5a,EAC/GkG,EAAMjG,EAAIA,EAAS2N,EAAJ3N,EAAW2N,GAAQ3N,EAAI2N,GAAQgN,EAAiB3a,EAAIwN,EAAQA,GAAQxN,EAAIwN,GAAQmN,EAAgB3a,GAC/G0M,EAASoB,EAAKK,KAAKiK,EAAMnS,MACVA,IACdA,EAAMlG,EAAI2M,EAAO3M,EACjBkG,EAAMjG,EAAI0M,EAAO1M,GAEH,IAAX0H,IACHzB,EAAMlG,GAAK2H,EACXzB,EAAMjG,GAAK0H,GAER8T,EAAS9G,KACZ+G,EAAKxV,EAAMlG,EAAIA,EACf2b,EAAKzV,EAAMjG,EAAIA,EACSwb,EAApBC,EAAKA,EAAKC,EAAKA,IAClBzV,EAAMlG,EAAIA,EACVkG,EAAMjG,EAAIA,IAGLiG,GAGLqO,GAASxG,GACL,SAAAnG,WAIL5H,EAAGC,EAAGiG,EAAO0V,EAHVrc,EAAIwO,EAAKvJ,OACZqW,EAAU,EACVgB,EAAUlH,IAEG,IAALpV,IAIRqc,GAFA5b,GADAkG,EAAQ6H,EAAKxO,IACHS,EAAI4H,EAAE5H,GAELA,GADXC,EAAIiG,EAAMjG,EAAI2H,EAAE3H,GACGA,GACR4b,IACVhB,EAAUtb,EACVsc,EAAUD,UAGJC,GAAWJ,EAAU1N,EAAK8M,GAAWjT,GAGxC,SAAA+S,UAAKA,GAuDAa,CAAoBN,EAAYnN,EAAOA,EAAKwN,OAAShO,EAAMG,EAAMD,EAAMG,EAAMG,EAAK0N,OAAQ7B,GAAe,EAAI,IAElHP,IACHiB,EAAQI,GAAeQ,EAAYnN,EAAOA,EAAK/N,GAAK+N,EAAKxI,MAAQwI,EAAKtK,WAAa8J,EAAMG,EAAMkM,GAAe,EAAI,IAE/GV,IACHqB,EAAQG,GAAeQ,EAAYnN,EAAOA,EAAK9N,GAAK8N,EAAKzI,KAAOyI,EAAKzK,UAAYmK,EAAMG,EAAMgM,GAAe,EAAI,MAOnG,SAAlBkC,KACCzD,EAAKoC,YAAa,EAClBhO,GAAe4L,EAAM,gBAAiB,mBAEpB,SAAnB0D,KACC1D,EAAKoC,YAAa,EAGT,SAAVuB,GAAWjI,EAAS/F,OACfD,EAAMmN,EAAWe,EAAOC,EACxBnI,GAAWF,KACE,IAAZE,IACHhG,EAAOnB,EAAKmB,MAAQnB,EAAK0O,UAAY,GACrCJ,EAAa3G,GAASxG,IAAS/G,EAAY+G,GAC3CgG,EAAU,CAACoI,YAAYvP,EAAKwP,iBAAmBxP,EAAKuP,YAAc,MAASzC,EAAe,GAAK,IAC3FA,EACH3F,EAAQsD,SAAWxJ,GAAcwK,EAAM6C,EAAYnN,EAAOA,EAAKsJ,SAAU3J,EAAMH,EAAM,EAAGS,IAEpFqL,IACHtF,EAAQqH,GAASvN,GAAcwK,EAAM6C,EAAYnN,EAAOA,EAAKwN,QAAUxN,EAAK/N,GAAK+N,EAAKxI,KAAMmI,EAAMH,EAAMqM,GAAe,EAAI,EAAG5L,GAA0C,MAApBqK,EAAKV,aAEtJuB,IACHnF,EAAQsH,GAASxN,GAAcwK,EAAM6C,EAAYnN,EAAOA,EAAKwN,QAAUxN,EAAK9N,GAAK8N,EAAKzI,IAAKsI,EAAMH,EAAMmM,GAAe,EAAI,EAAG5L,GAA0C,MAApBqK,EAAKV,cAErJ5J,EAAKwN,QAAWhH,GAASxG,IAAS7G,EAAU6G,EAAK,OACpDgG,EAAQsI,YAAcjB,EAAQ,IAAMC,EACpCtH,EAAQ0H,OAAS1N,EAAK0N,UAIzBpD,EAAKoC,YAAa,EAClByB,EAAuB1E,MAAM5K,EAAKsP,oBAA0E,IAAxBtP,EAAK2K,eAAwB,EAAK,EAAIc,EAAKd,eAAkB,GAAxF3K,EAAKsP,mBACzDnI,EAAQjL,WACZiL,EAAQjL,SAAW,CAAC+B,IAAKvD,KAAKuD,IAAI+B,EAAK0P,aAAe,EAAI,gBAAiB1P,EAAQA,EAAK2P,YAAc,GAAIjP,IAAOkK,MAAM5K,EAAK0P,aAA0D,IAAvBJ,GAA6BhV,EAAU6M,IAAiC,IAArBA,EAAQoI,WAAsB,EAAI,GAAzGvP,EAAK0P,YAA0GE,UAAWN,IAEtQ7D,EAAK4D,MAAQA,EAAQnV,GAAK6B,GAAGiR,GAAe1P,EAAQ,CACnD6J,QAASA,EACT/K,KAAM,aACNyT,SAAS,EACT1T,WAAY+S,GACZY,YAAaX,GACbY,SAAW/P,EAAKgQ,SAAWnQ,GAAiBwN,GAC5C4C,eAAiBjQ,EAAKgQ,SAAW,CAACvE,EAAM,gBAAiB,iBAAoBtK,GAAQA,EAAK0N,OAAU,EAAC,GAAO,GAAQ,KAEhH7O,EAAKgQ,WACLhD,IACHA,EAAY/I,OAAQ,GAErBoL,EAAM9D,OAAO,KAAK,GAAM,GACxB8B,IAAO,GAAM,GACb5B,EAAKyE,KAAOzE,EAAKrY,EACjBqY,EAAK0E,KAAO1E,EAAKpY,EACbyZ,IACHrB,EAAK2E,YAAc3E,EAAKrY,GAEzBic,EAAMgB,KAAK,GACXhD,IAAO,GAAM,GACTL,IACHA,EAAY/I,OAAQ,KAGZsK,GACV9C,EAAK6E,cAIQ,SAAfC,GAAeC,OAEbxV,EADGyV,EAAQlY,EAEZA,EAASiB,gBAAgB8D,EAAO1K,YAAY,GACxC4d,GAAc/E,EAAK3E,YAAcvO,EAAOa,OAAOqX,GAAS,IAAItb,MAC/D6F,EAAIyV,EAAM7X,UAAUS,MAAM,CAACjG,EAAEsd,EAAerd,EAAEsd,IAC9CpY,EAAOc,MAAM2B,EAAGA,GAChB0V,EAAgB1V,EAAE5H,EAClBud,EAAgB3V,EAAE3H,GAEfkF,EAAOa,OAAOzE,KACjB4D,EAAS,MAIY,SAAvBqY,SAIEC,EAAczd,EAAGC,EAHd2a,EAAgB,EAAIvC,EAAKd,eAC5BmG,EAAU/W,GAAUpD,GAAkB0V,IAAY,EAClD0E,EAAUhX,GAAUvD,GAAiB6V,IAAY,EAE9Ca,IACH9B,GAAQhY,EAAI8X,GAAasD,EAAO,MAAQ,KACxCpD,GAAQ/X,EAAI6X,GAAauD,EAAO,MAAQ,KACxCrD,GAAQhV,mBAETma,IAAa,GACbhI,GAAQnV,EAAIqY,EAAKM,SAAW+E,EAC5BvI,GAAQlV,EAAIoY,EAAKO,SAAW+E,EAC5BxY,GAAUA,EAAOc,MAAMkP,GAASA,IAChCmI,EAAgBnI,GAAQnV,EACxBud,EAAgBpI,GAAQlV,EACpBuY,IACHiB,GAAmBpB,EAAKM,SAAUN,EAAKO,UACvCT,IAAO,IAERyF,EAAcxX,gBAAgB8D,GAC1B0P,GACHmB,KACA8C,EAAgBjE,EAAYtU,MAC5BwY,EAAgBlE,EAAYrU,SAGxBwY,MACH9D,IAAO,GAAM,GACbc,MAEA1C,EAAK6E,cAEFxD,GACH+D,EAAevT,EAAOxG,gBAAkB,CAACsU,GAAQgG,QAAU9T,EAAOrF,UAAU7E,EAAGgY,GAAQiG,QAAU/T,EAAOrF,UAAU5E,IAAMuL,GAAkBtB,GAAQvI,KAAyB,OAAO0R,MAAM,KACxL6K,EAAiB7F,EAAK6F,eAAiB9X,gBAAgB8D,GAAQjE,MAAM,CAACjG,EAAGwR,WAAWiM,EAAa,KAAO,EAAGxd,EAAGuR,WAAWiM,EAAa,KAAO,IAC7IxD,IAAO,GAAM,GACbja,EAAIqY,EAAKM,SAAWuF,EAAele,EAAI0d,EACvCzd,EAAIie,EAAeje,EAAIoY,EAAKO,SAAW+E,EACvCG,EAAgBzF,EAAKrY,EACrB6d,EAAgBxF,EAAKpY,EAAIqH,KAAK6W,MAAMle,EAAGD,GAAKyU,KAK5CoJ,EAAgB/F,GAAauD,EAAO,MACpCyC,EAAgBhG,GAAasD,EAAO,QAIlCD,GAAaP,IACIlN,EAAhBoQ,EACHA,EAAgBpQ,GAAQoQ,EAAgBpQ,GAAQkN,EACtCkD,EAAgBvQ,IAC1BuQ,EAAgBvQ,GAAQA,EAAOuQ,GAAiBlD,GAE5ClB,IACgB9L,EAAhBiQ,EACHA,EAAgBjQ,GAAQiQ,EAAgBjQ,GAAQgN,EACtCiD,EAAgBpQ,IAC1BoQ,EAAgBpQ,GAAQA,EAAOoQ,GAAiBjD,KAInDvC,EAAK+F,OAASN,EAAgBzW,GAAOyW,GACrCzF,EAAKgG,OAASR,EAAgBxW,GAAOwW,GAKlB,SAApBS,MACKhM,GAAgB9S,YAAeue,MAAiB1F,EAAKC,YACxDhG,GAAgB9S,WAAWgD,YAAY8P,IAK/B,SAAViM,GAAWpe,EAAGoQ,OACThR,MACCif,GAAWnG,EAAK3E,YAAcvT,KAAkB,cAAXA,EAAEJ,MAAmC,gBAAXI,EAAEJ,MAA4BwQ,IAASqE,KAAa6J,GAAY,IAAMpV,GAAkBgP,EAAKrL,aAAajN,MAC7K2e,GAAuBve,GAAKqe,GAAW9U,GAAgBvJ,WAGxDwe,EAAcZ,KACda,GAAU,EACVvG,EAAKrL,aAAe7M,EAChBkJ,GAAkBlJ,EAAEJ,OACvB8e,GAAoB1e,EAAEJ,KAAK4S,QAAQ,SAAYxS,EAAE2e,eAAiB3e,EAAE+J,OAAU+O,GAC9EhQ,GAAa4V,EAAkB,WAAYE,IAC3C9V,GAAa4V,EAAkB,YAAaG,IAC5C/V,GAAa4V,EAAkB,cAAeE,IAC9C9V,GAAagQ,GAAU,aAAc9O,MAErC0U,EAAmB,KACnB5V,GAAagQ,GAAU,YAAa+F,KAErCC,EAAgB,KACX7M,IAAsByM,IAC1B5V,GAAagQ,GAAU,UAAW8F,IAClC5e,GAAKA,EAAE+J,QAAUjB,GAAa9I,EAAE+J,OAAQ,UAAW6U,KAEpDG,EAAcC,GAAY/Q,KAAKiK,EAAMlY,EAAE+J,UAAmC,IAAxB0C,EAAKwS,iBAA6B7O,SAEnFtH,GAAa9I,EAAE+J,OAAQ,SAAU6U,IACjCtS,GAAe4L,EAAM,YAAa,eAClC5L,GAAe4L,EAAM,QAAS,WAC9B3J,GAAe2Q,GAAU,QACzBX,GAAsB,MAGvBY,KAA8BT,GAAoBxF,GAAWH,IAAkD,IAAxCb,EAAKzL,KAAK0S,2BAAwCjH,EAAKzL,KAAK2S,kBAAoBpf,IAAMA,EAAEqf,SAAqB,EAAVrf,EAAEsf,UAAuBpG,EAAS,IAAM,MAClNqF,GAAuBY,IAA8BjH,EAAKT,qBAEzDlO,GAAgBvJ,GAChB8I,GAAa7H,GAAM,mBAAoBsI,KAEpCvJ,EAAEuf,gBACLvf,EAAIwf,EAAQxf,EAAEuf,eAAe,GAC7BE,EAAUzf,EAAE0f,YACF1f,EAAE2f,UACZF,EAAUzf,EAAE2f,UAEZH,EAAQC,EAAU,KAEnB3V,KA3oCiB,SAApB8V,kBAAoB1X,GACnBF,GAAalF,KAAKoF,GACU,IAAxBF,GAAa3D,QAChBsC,GAAKyB,OAAOyX,IAAI9X,IAyoCf6X,CAAkB5H,IAClBoF,EAAgBlF,EAAKO,SAAWzY,EAAEqM,MAClC8Q,EAAgBjF,EAAKM,SAAWxY,EAAEmM,MAClCG,GAAe4L,EAAM,YAAa,gBAC9BiH,GAA6BjH,EAAKX,aACrC5M,GAAkBZ,EAAO1K,aAEtB0K,EAAO1K,aAAc6Y,EAAKX,YAAekC,GAAgBF,IAAgBxP,EAAO1K,WAAWyL,eAAkBqH,GAAgB9S,YAAe0K,EAAOrF,UACtJyN,GAAgB3R,MAAMkL,MAAQ3B,EAAO1K,WAAWwQ,YAAc,KAC9D9F,EAAO1K,WAAWiB,YAAY6R,KAE/BkL,KACAnF,EAAK4D,OAAS5D,EAAK4D,MAAMgE,OACzB5H,EAAKoC,YAAa,EAClB3T,GAAK6J,aAAaiJ,GAAe1P,EAAQgW,GAAW,GACpDtG,GAAe9S,GAAK6J,aAAazG,EAAQ,CAACsP,SAAS,IAAI,GACvDnB,EAAK4D,MAAQ5D,EAAKV,WAAa,MAC3B/K,EAAKuT,cAAiBzG,GAAiBE,IAAoC,IAArBhN,EAAKuT,eAC9DjW,EAAOvJ,MAAMyf,OAASlK,UAAUkK,UAEjC/H,EAAK3E,WAAY,EACjBqG,KAAqBnN,EAAKyT,SAAUhI,EAAKvL,WAAWwT,MACpDC,KAAqB3T,EAAKoS,SAAU3G,EAAKvL,WAAW0T,OAChC,IAAhB5T,EAAK4F,QAAoB5F,EAAK6T,iBACjClhB,EAAI8f,EAAS7a,QACC,IAALjF,GACRuH,GAAKgI,IAAIuQ,EAAS9f,GAAI,CAACiT,OAAQ5F,EAAK6T,cAAgB7T,EAAK4F,SAA8B,SAAnBD,GAA4B,WAAaA,MAG/G9F,GAAe4L,EAAM,QAAS,YA8ShB,SAAfqI,GAAevgB,MACVA,GAAKkY,EAAKC,aAAesB,EAAa,KACrCrV,EAASpE,EAAE+J,QAAUA,EAAO1K,WAC/Bma,EAASpV,EAAOd,WAAac,EAAO4G,WACpC0O,EAAStV,EAAOjB,UAAYiB,EAAO6G,YAChCuO,GAAUE,KACT1U,GACHmY,GAAiB3D,EAASxU,EAAOpE,EAAI8Y,EAAS1U,EAAOlE,EACrDsc,GAAiB1D,EAAS1U,EAAOjE,EAAIyY,EAASxU,EAAOnE,IAErDsc,GAAiB3D,EACjB4D,GAAiB1D,GAElBtV,EAAO4G,YAAcwO,EACrBpV,EAAO6G,YAAcyO,EACrBJ,GAAmBpB,EAAKM,SAAUN,EAAKO,YAKhC,SAAV+H,GAAUxgB,OACLygB,EAAOhM,KACViM,EAAmBD,EAAOnC,GAAY,IACtCqC,EAAmBF,EAAOG,GAAc,GACxCC,EAAqBH,GAAmBI,IAAkBxC,GAC1DyC,EAAoB7I,EAAKrL,cAAgBqL,EAAKrL,aAAakU,iBAC3DC,EAA4BN,GAAmBO,IAAyB3C,GACxE4C,EAAUlhB,EAAEmhB,WAA6B,MAAfnhB,EAAEmhB,WAAqBT,GAAmBG,MAChEA,GAAsBF,IAAqD,IAAlCzI,EAAKzL,KAAK2U,sBAAoCphB,EAAE+X,0BAC7F/X,EAAE+X,2BAEC2I,KAAqBxI,EAAKrL,eAAgBqL,EAAKrL,aAAakU,qBAAuBF,GAAsBK,IAAYF,UACpHE,GAAWL,IACdI,EAAuB3C,SAExBwC,EAAgBxC,KAGbpG,EAAK3E,WAAaoN,GAAmBD,KACnCQ,GAAYlhB,EAAEqhB,QAAWX,IAAmBK,GAChDxX,GAAgBvJ,IAGb0gB,GAAoBC,GAAoBlC,IAC5Cze,GAAKA,EAAE+J,SAAWmO,EAAKrL,aAAe7M,GACtCsM,GAAe4L,EAAM,QAAS,YAIhB,SAAhBoJ,GAAgB7Z,UAAKzC,EAAS,CAACnF,EAAE4H,EAAE5H,EAAImF,EAAOpE,EAAI6G,EAAE3H,EAAIkF,EAAOlE,EAAIkE,EAAOhF,EAAGF,EAAE2H,EAAE5H,EAAImF,EAAOnE,EAAI4G,EAAE3H,EAAIkF,EAAOjE,EAAIiE,EAAOhE,GAAK,CAACnB,EAAE4H,EAAE5H,EAAGC,EAAE2H,EAAE3H,OAr5BzIue,EAAS5E,EAAa0D,EAAeC,EAAeO,EAAeD,EAAe1C,EAAWpB,EAAiBwG,EAAiB7S,EAAMH,EAAMK,EAAMH,EAAMkS,EAAOC,EAAS1B,EAAgB1F,EAAOkJ,EAAKpH,EAAOC,EAAOC,EAAQ0E,EAAYL,EAAkB1Z,EAAQwZ,EAAaW,EAA2BL,EAAejF,EAAeiH,EAAeG,EAAsB1C,EAAqBd,EAAagB,EAvBzY7e,GAAQ6M,EAAK7M,MAAQ,OAAO6D,cAC/BkW,GAAW/Z,EAAK4S,QAAQ,OAAS5S,EAAK4S,QAAQ,KAC9C+G,GAA8C,IAA9B3Z,EAAK4S,QAAQ,YAC7ByI,EAAQ1B,EAAe,WAAaI,EAAS,IAAM,OACnDuB,EAAQvB,EAAS,IAAM,MACvBT,MAAatZ,EAAK4S,QAAQ,QAAS5S,EAAK4S,QAAQ,SAAoB,WAAT5S,GAC3DmZ,MAAanZ,EAAK4S,QAAQ,QAAS5S,EAAK4S,QAAQ,QAAmB,WAAT5S,GAC1D4hB,EAAkB/U,EAAK+U,iBAAmB,EAC1CtJ,4BACAgH,EAAW9S,GAASK,EAAKgV,SAAWhV,EAAKiV,QAAU3X,GACnDgW,EAAY,GACZa,GAAc,EACdxI,IAAwB,EACxBa,GAAsBxM,EAAKwM,qBAAuB,GAClDE,GAAwB1M,EAAK0M,uBAAyB,GACtDH,GAAyBvM,EAAKuM,wBAA0B,GACxDI,GAAuB3M,EAAK2M,sBAAwB,GACpD4F,GAAcvS,EAAKkV,eAAiBxT,GACpCmQ,GAAY,EACZzG,GAAU9N,EAAOvH,OAASmE,GAAKmN,KAAKoG,SAASnQ,GAC7CvD,GAnWS,SAAX9C,SAAWvE,SACkC,UAAxCkM,GAAkBlM,GAASyE,YAG/BzE,EAAUA,EAAQE,aACkB,IAArBF,EAAQ0E,SACfH,SAASvE,WA6VNuE,CAASqG,GAEnB+O,GAAW/O,EAAOrI,eAAiBpC,GAienCse,GAAa,SAAbA,oBAAmB1F,EAAK4D,OAAS5D,EAAK4D,MAAM8F,YA0F5C/C,GAAS,SAATA,OAAS7e,OAEP6J,EAAS2O,EAAUC,EAAUrZ,EAAGmc,EAAIC,EADjCqG,EAAgB7hB,KAEfqe,IAAWzU,IAAqBsO,EAAK3E,WAAcvT,MAKxD6J,GADAqO,EAAKrL,aAAe7M,GACRuf,oBAEXvf,EAAI6J,EAAQ,MACF2V,GAASxf,EAAE0f,aAAeD,EAAS,KAC5CrgB,EAAIyK,EAAQxF,QACE,IAALjF,IAAWY,EAAI6J,EAAQzK,IAAIsgB,aAAeD,GAAWzf,EAAE+J,SAAWA,OACvE3K,EAAI,eAIH,GAAIY,EAAE2f,WAAaF,GAAWzf,EAAE2f,YAAcF,SAIjDf,GAAoBS,IAA8BL,IACrD9J,GAAQnV,EAAIG,EAAEmM,OAAS3F,GAAUpD,GAAkB0V,IAAY,GAC/D9D,GAAQlV,EAAIE,EAAEqM,OAAS7F,GAAUvD,GAAiB6V,IAAY,GAC9D9T,GAAUA,EAAOc,MAAMkP,GAASA,IAChCwD,EAAWxD,GAAQnV,EACnB4Y,EAAWzD,GAAQlV,IACnByb,EAAKpU,KAAK2a,IAAItJ,EAAW2E,OACzB3B,EAAKrU,KAAK2a,IAAIrJ,EAAW2E,MACDoE,EAALjG,GAA6BiG,EAALhG,IAA2BlJ,IAAc6M,IAA8BL,KACjHA,EAAsBtD,EAALD,GAAWrC,EAAU,IAAM,IACxCiG,GAA6BL,IAAkBK,GAClDrW,GAAa7H,GAAM,mBAAoBsI,KAEA,IAApC2O,EAAKzL,KAAKsV,uBAAmC7I,GAAUH,IAC1Db,EAAKV,WAAgC,MAAlBsH,EAAyB,IAAM,IAClDjY,EAAYqR,EAAKzL,KAAKuV,aAAe9J,EAAKzL,KAAKuV,WAAW/T,KAAKiK,EAAM2J,IAElEvP,IAAc6M,IAA8BL,IAC/CF,GAAUiD,IASZtD,EAJIrG,EAAKT,mBAAuB0H,KAA8BL,GAAiBK,IAA8BL,KAAgD,IAA7B+C,EAAcI,WAGpI1D,IACY,GAHtBhV,GAAgBsY,IACM,GAKnB3J,EAAKX,aACRa,IAAwB,GAEzBkB,GAAmBtZ,EAAEmM,MAAOnM,EAAEqM,MAAO+T,SAnDpC7B,GAAuBve,GAAKqe,GAAW9U,GAAgBvJ,IAsDzDsZ,GAAqB,SAArBA,mBAAsBd,EAAUC,EAAUyJ,OAYxCC,EAASC,EAASviB,EAAGC,EAAGwQ,EAAK+R,EAX1BC,EAAgB,EAAIpK,EAAKf,eAC5BsD,EAAgB,EAAIvC,EAAKd,eACzBmL,EAAerK,EAAKM,SACpBgK,EAAetK,EAAKO,SACpBgK,EAAoB/E,EACpBgF,EAAQxK,EAAKrY,EACb8iB,EAAQzK,EAAKpY,EACb8iB,EAAW1K,EAAKyE,KAChBkG,EAAW3K,EAAK0E,KAChBkG,EAAkB5K,EAAK2E,YACvBkG,EAAY1K,EAEbH,EAAKM,SAAWA,EAChBN,EAAKO,SAAWA,EACZjS,KACHgS,GAAYpV,GAAkB0V,IAC9BL,GAAYxV,GAAiB6V,KAE1BS,GACHzZ,EAAIqH,KAAK6W,MAAMD,EAAeje,EAAI2Y,EAAUD,EAAWuF,EAAele,GAAKyU,GAEjE,KADVhE,EAAM4H,EAAKpY,EAAIA,IAEd4d,GAAiB,IACjBxF,EAAKpY,EAAIA,GACCwQ,GAAO,MACjBoN,GAAiB,IACjBxF,EAAKpY,EAAIA,GAITD,EAFGqY,EAAKrY,IAAM8d,GAAiBxW,KAAKuD,IAAIvD,KAAK2a,IAAI3E,EAAgB3E,GAAWrR,KAAK2a,IAAI1E,EAAgB3E,IAAa+I,GAClHtJ,EAAKpY,EAAIA,EACL6d,GAAiBD,EAAgB5d,GAAKwiB,GAEtC3E,IAID3Y,IACHqd,EAAO7J,EAAWxT,EAAOpE,EAAI6X,EAAWzT,EAAOlE,EAAIkE,EAAOhF,EAC1DyY,EAAWD,EAAWxT,EAAOnE,EAAI4X,EAAWzT,EAAOjE,EAAIiE,EAAOhE,EAC9DwX,EAAW6J,IAEZD,EAAW3J,EAAW2E,GAERoE,IAA8BA,EAAXY,IAChCA,EAAU,IAFXD,EAAW3J,EAAW2E,GAIRqE,IAA8BA,EAAXW,IAChCA,EAAU,IAENjK,EAAKZ,UAAYY,EAAKV,cAAgB2K,GAAWC,MACrDC,EAAOnK,EAAKV,cAEXU,EAAKV,WAAa6K,EAAQnJ,GAAU/R,KAAK2a,IAAIK,GAAWhb,KAAK2a,IAAIM,GAAY,IAAMrJ,EAAS,IAAM,KAC9FsJ,GAAQxb,EAAYqR,EAAKzL,KAAKuV,aACjC9J,EAAKzL,KAAKuV,WAAW/T,KAAKiK,EAAMA,EAAKrL,eAG1B,MAATwV,EACHD,EAAU,EACS,MAATC,IACVF,EAAU,IAGZtiB,EAAIqH,GAAOyW,EAAgBwE,EAAUG,GACrCxiB,EAAIoH,GAAOwW,EAAgB0E,EAAUE,KAGjCnI,GAASC,GAASC,KAAYnC,EAAKrY,IAAMA,GAAMqY,EAAKpY,IAAMA,IAAMyZ,KAChEc,IACHtF,GAAOlV,EAAIA,EACXkV,GAAOjV,EAAIA,EACXuiB,EAAOhI,EAAOtF,IACdlV,EAAIqH,GAAOmb,EAAKxiB,GAChBC,EAAIoH,GAAOmb,EAAKviB,IAEbqa,IACHta,EAAIqH,GAAOiT,EAAMta,KAEdua,IACHta,EAAIoH,GAAOkT,EAAMta,MAGfkb,IACKzN,EAAJ1N,EACHA,EAAI0N,EAAOpG,KAAKC,OAAOvH,EAAI0N,GAAQkN,GACzB5a,EAAIuN,IACdvN,EAAIuN,EAAOjG,KAAKC,OAAOvH,EAAIuN,GAAQqN,IAE/BlB,IACI9L,EAAJ3N,EACHA,EAAIqH,KAAKC,MAAMqG,GAAQ3N,EAAI2N,GAAQgN,GACzB3a,EAAIwN,IACdxN,EAAIqH,KAAKC,MAAMkG,GAAQxN,EAAIwN,GAAQmN,MAIlCvC,EAAKrY,IAAMA,IAAMqY,EAAKpY,IAAMA,GAAMyZ,KACjCA,GACHrB,EAAK2E,YAAc3E,EAAKrY,EAAIqY,EAAKyE,KAAO9c,EACxCwY,GAAQ,IAEJU,IACHb,EAAKpY,EAAIoY,EAAK0E,KAAO9c,EACrBuY,GAAQ,GAELa,IACHhB,EAAKrY,EAAIqY,EAAKyE,KAAO9c,EACrBwY,GAAQ,IAGL6J,IAA2D,IAA3C5V,GAAe4L,EAAM,OAAQ,WAMjDA,EAAKM,SAAW+J,EAChBrK,EAAKO,SAAW+J,EAChB9E,EAAgB+E,EAChBvK,EAAKrY,EAAI6iB,EACTxK,EAAKpY,EAAI6iB,EACTzK,EAAKyE,KAAOiG,EACZ1K,EAAK0E,KAAOiG,EACZ3K,EAAK2E,YAAciG,EACnBzK,EAAQ0K,IAbH7K,EAAKC,YAAcD,EAAK3E,YAC5B2E,EAAKC,WAAasG,GAAU,EAC5BnS,GAAe4L,EAAM,YAAa,kBAiBtC0G,GAAY,SAAZA,UAAa5e,EAAGoQ,MACViO,GAAYnG,EAAK3E,aAAcvT,GAAgB,MAAXyf,GAAoBrP,KAAWpQ,EAAE2f,WAAa3f,EAAE2f,YAAcF,GAAWzf,EAAE+J,SAAWA,GAAY/J,EAAEuf,iBAh0ClI,SAAdyD,YAAenN,EAAMoN,WAChB7jB,EAAIyW,EAAKxR,OACNjF,QACFyW,EAAKzW,GAAGsgB,aAAeuD,SACnB,EA4zCyJD,CAAYhjB,EAAEuf,eAAgBE,MAI9LvH,EAAK3E,WAAY,MAKhB1J,EAASzK,EAAG8jB,EAAgBC,EAAaC,EAJtCvB,EAAgB7hB,EACnBqjB,EAAcnL,EAAKC,WACnBmL,EAAwBpL,EAAKzL,KAAK2S,kBAAoBpf,IAAMA,EAAEqf,SAAqB,EAAVrf,EAAEsf,OAC3EiE,EAAyB5c,GAAK6c,YAAY,KAAOrF,OAE9CO,GACHrV,GAAgBqV,EAAkB,WAAYE,WAC9CvV,GAAgBqV,EAAkB,YAAaG,IAC/CxV,GAAgBqV,EAAkB,cAAeE,WACjDvV,GAAgByP,GAAU,aAAc9O,KAExCX,GAAgByP,GAAU,YAAa+F,IAExCxV,GAAgBpI,GAAM,mBAAoBsI,IACrC0I,IAAqByM,IACzBrV,GAAgByP,GAAU,UAAW8F,WACrC5e,GAAKA,EAAE+J,QAAUV,GAAgBrJ,EAAE+J,OAAQ,UAAW6U,YAEvDvG,GAAQ,EACJgL,IACHzC,GAAc9L,GAAgBL,KAC9ByD,EAAKC,YAAa,GAEnB7P,GAAuB0P,IACnB+G,IAAeuE,SACdtjB,IACHqJ,GAAgBrJ,EAAE+J,OAAQ,SAAU6U,WACpC1G,EAAKrL,aAAegV,GAErBtT,GAAe2Q,GAAU,GACzB5S,GAAe4L,EAAM,UAAW,aAChC5L,GAAe4L,EAAM,QAAS,gBAC9B6G,GAAa,OAGd3f,EAAI8f,EAAS7a,QACC,IAALjF,GACR8L,GAAUgU,EAAS9f,GAAI,SAAUqN,EAAK4F,UAA2B,IAAhB5F,EAAK4F,OAAmBD,GAAiB,UAE3FtI,KACI9J,EAAG,KACN6J,EAAU7J,EAAEuf,kBAEXvf,EAAI6J,EAAQ,MACF2V,GAASxf,EAAE0f,aAAeD,EAAS,KAC5CrgB,EAAIyK,EAAQxF,QACE,IAALjF,IAAWY,EAAI6J,EAAQzK,IAAIsgB,aAAeD,GAAWzf,EAAE+J,SAAWA,OACvE3K,EAAI,IAAMgR,SAKhB8H,EAAKrL,aAAegV,EACpB3J,EAAKM,SAAWxY,EAAEmM,MAClB+L,EAAKO,SAAWzY,EAAEqM,aAEfiX,GAAwBzB,GAC3BtY,GAAgBsY,GAChBtD,GAAsB,EACtBjS,GAAe4L,EAAM,UAAW,cACtB2J,IAAkBwB,GAC5B9E,GAAsB,EAClBC,IAAgB/R,EAAKmB,MAAQnB,EAAKoO,SACrCgB,GAAQpP,EAAKmH,SAAWnH,EAAKgX,YAE9BnX,GAAe4L,EAAM,UAAW,aAC1B5F,IAAqC,cAAvBuP,EAAcjiB,OAAmE,IAA1CiiB,EAAcjiB,KAAK4S,QAAQ,YACrFlG,GAAe4L,EAAM,QAAS,WAC1BzD,KAAa6J,GAAY,KAC5BhS,GAAe4L,EAAM,cAAe,iBAErCiL,EAActB,EAAc9X,QAAUA,EACtCuU,GAAY7J,KACZ2O,EAAiB,0BACZ9E,KAAcwC,IAAiB5I,EAAKmG,WAAcnG,EAAK3E,WAAcsO,EAAcd,mBAClFoC,EAAYO,MACfP,EAAYO,QACF5K,GAAS6K,eACnBT,EAAiBpK,GAAS6K,YAAY,gBACvBC,eAAe,SAAS,GAAM,EAAM3iB,GAAM,EAAGiX,EAAKrL,aAAagX,QAAS3L,EAAKrL,aAAaiX,QAAS5L,EAAKM,SAAUN,EAAKO,UAAU,GAAO,GAAO,GAAO,EAAO,EAAG,MAC/K0K,EAAYrW,cAAcoW,MAIxB5Q,IAAeuP,EAAcd,kBACjCpa,GAAK6c,YAAY,IAAMJ,MAIzBvH,GAAQpP,EAAKmH,SAAWnH,EAAKgX,YACxBvL,EAAKT,oBAAqBoK,IAA0C,IAAxBpV,EAAKwS,gBAA6BD,GAAY/Q,KAAKiK,EAAM2J,EAAc9X,UAAYsZ,GAAiBlE,KAA8BL,GAAiBK,IAA8BL,KAAgD,IAA7B+C,EAAcI,WAIlQ1D,GAAsB,GAHtBA,GAAsB,EACtBhV,GAAgBsY,IAIjBvV,GAAe4L,EAAM,UAAW,cAEjC0F,MAAgB2F,EAAuB5a,SAAUuP,EAAK4D,MAAMnT,YAC5D0a,GAAe/W,GAAe4L,EAAM,UAAW,cACxC,EAxGNqG,GAAuBve,GAAKqe,GAAW9U,GAAgBvJ,WA8J1DuhB,EAAMxL,UAAUrT,IAAIqH,KACbwX,EAAIzB,SAGNiE,UAAY,SAACva,EAAOwa,OACpBnN,EAAIC,EAAI7B,EAAIC,EAChBkJ,GAAQ5U,GAAS0O,EAAKrL,cAAc,GAEhCmX,IAAU9L,EAAK5B,QAAQ9M,GAAS0O,EAAKrL,gBACxCgK,EAAKrL,GAAWhC,GAAS0O,EAAKrL,cAC9BiK,EAAKtL,GAAWzB,GAChBkL,EAAKqM,GAAc,CAACzhB,EAAEgX,EAAGzR,KAAOyR,EAAGnL,MAAQ,EAAG5L,EAAE+W,EAAG1R,IAAM0R,EAAG/K,OAAS,IACrEoJ,EAAKoM,GAAc,CAACzhB,EAAEiX,EAAG1R,KAAO0R,EAAGpL,MAAQ,EAAG5L,EAAEgX,EAAG3R,IAAM2R,EAAGhL,OAAS,IACrEqR,GAAiBlI,EAAGpV,EAAIqV,EAAGrV,EAC3Bud,GAAiBnI,EAAGnV,EAAIoV,EAAGpV,GAEvBoY,EAAKC,aACTD,EAAKC,WAAasG,GAAU,EAC5BnS,GAAe4L,EAAM,YAAa,mBAG/BiI,KAAOtB,KACPrL,QAAU,SAAAxT,UAAK4e,GAAU5e,GAAKkY,EAAKrL,cAAc,MACjDwJ,cAAgB,kBAAM6B,EAAKC,WAAa,GAAK1D,KAAamM,IAAe,OACzEqD,eAAiB,kBAAOxP,KAAa6J,IAAa,OAClDhI,QAAU,SAACvM,EAAQ0M,UAAcV,UAAUO,QAAQ4B,EAAKnO,OAAQA,EAAQ0M,MAExEyN,aAAe,SAACC,EAAMC,OAEzBjC,EAASC,EAASiC,EAAOC,EAAWzN,EAAIC,EADrCyN,EAAiB,aAATJ,GAAuBzQ,GAAiByQ,EAAQpd,EAAUod,KAAU5K,EAAgB,UAAY,cAE/F,YAATgL,IACH1N,EAAKrL,GAAW0M,EAAKnO,QACrB+M,EAAKtL,GAAW2Y,IAEjBhC,EAAoB,UAAToC,EAAoBrM,EAAKrY,EAAI8d,EAA0B,aAAT4G,EAAuB7Q,GAAc8Q,YAAYza,EAAQkR,GAAUpE,EAAGzR,KAAOyR,EAAGnL,MAAQ,GAAMoL,EAAG1R,KAAO0R,EAAGpL,MAAQ,GACxK6N,EACI4I,EAAU,EAAI,oBAAsB,aAE3CiC,EAAoBA,GAAqB,EACzChC,EAAoB,UAATmC,EAAoBrM,EAAKpY,EAAI4d,EAA0B,aAAT6G,EAAuB7Q,GAAc8Q,YAAYza,EAAQmR,GAAUrE,EAAG1R,IAAM0R,EAAG/K,OAAS,GAAMgL,EAAG3R,IAAM2R,EAAGhL,OAAS,GAE5KwY,GADAD,EAAQld,KAAK2a,IAAIK,EAAUC,IACN,EAAIgC,EAAqB,GAAMjC,EAAU,EAAK,OAAS,QACxEkC,EAAQD,IACO,KAAdE,IACHA,GAAa,KAEdA,GAAclC,EAAU,EAAK,KAAO,QAG/BkC,MAGHvH,YAAc,SAAC0H,EAAWC,OAC1B7kB,EAAGC,EAAG+N,EAAmB7N,EAAGoE,EAAQkU,KACpCmM,GAAahY,EAAKoO,SAAW4J,SAChChY,EAAKoO,OAAS4J,EACPvM,EAAKyM,QAAO,EAAMD,MAE1B5K,IAAO,GACPc,KACII,IAAc4C,KAAc,IAC/B/d,EAAIqY,EAAKrY,EACTC,EAAIoY,EAAKpY,EACDyN,EAAJ1N,EACHA,EAAI0N,EACM1N,EAAIuN,IACdvN,EAAIuN,GAEGK,EAAJ3N,EACHA,EAAI2N,EACM3N,EAAIwN,IACdxN,EAAIwN,IAED4K,EAAKrY,IAAMA,GAAKqY,EAAKpY,IAAMA,KAC9B+N,GAAoB,EACpBqK,EAAKrY,EAAIqY,EAAKyE,KAAO9c,EACjB0Z,EACHrB,EAAK2E,YAAchd,EAEnBqY,EAAKpY,EAAIoY,EAAK0E,KAAO9c,EAGtBkY,GADAK,GAAQ,GAEJH,EAAKX,aAAeW,EAAKC,gBAC5BxN,GAAkBZ,EAAO1K,YACzBW,EAAI+J,EACJc,GAAa1H,UAAkC,MAApBlC,GAAKiC,YAAuBjC,GAAKiC,YAAqD,MAAtC4V,GAASjX,gBAAgBsB,UAAqB2V,GAASjX,gBAAgBsB,UAAY2V,GAAShX,KAAKqB,UAC5K0H,GAAavH,WAAmC,MAApBrC,GAAKoC,YAAuBpC,GAAKoC,YAAsD,MAAvCyV,GAASjX,gBAAgByB,WAAsBwV,GAASjX,gBAAgByB,WAAawV,GAAShX,KAAKwB,WACxKtD,IAAMsY,GAEZlU,GADAkU,EAASnO,GAAQnK,EAAEX,aACDwL,GAAe7K,EAAEX,WAC/B0Z,GAAU3U,EAAOjB,UAAYiB,EAAO2G,gBACvC3G,EAAOjB,UAAYiB,EAAO2G,eAEvBmO,GAAU9U,EAAOd,WAAac,EAAO0G,gBACxC1G,EAAOd,WAAac,EAAO0G,eAE5B9K,EAAIoE,EAIH8T,EAAKoC,aAAezM,GAAqBqK,EAAKyE,KAAOpP,GAAQ2K,EAAKyE,KAAOvP,GAAQ8K,EAAK0E,KAAOnP,GAAQyK,EAAK0E,KAAOtP,IACpHuO,GAAQpP,EAAKmH,SAAWnH,EAAKgX,WAAY5V,UAGpCqK,KAGHyM,OAAS,SAAC5H,EAAa2H,EAAQE,MAC/BF,GAAUxM,EAAK3E,UAAW,KACzB5S,EAAIsF,gBAAgB8D,GACvBtC,EAAIgW,EAAY3X,MAAM,CAACjG,EAAGqY,EAAKrY,EAAI8d,EAAe7d,EAAGoY,EAAKpY,EAAI4d,IAC9DmH,EAAK5e,gBAAgB8D,EAAO1K,YAAY,GACzCwlB,EAAG/e,MAAM,CAACjG,EAAGc,EAAEX,EAAIyH,EAAE5H,EAAGC,EAAGa,EAAEK,EAAIyG,EAAE3H,GAAI2H,GACvCyQ,EAAKrY,GAAK4H,EAAE5H,EAAIglB,EAAG7kB,EACnBkY,EAAKpY,GAAK2H,EAAE3H,EAAI+kB,EAAG7jB,EACnBgX,IAAO,GACPqF,SAEKxd,EAASqY,EAATrY,EAAGC,EAAMoY,EAANpY,SACTkd,IAAc0H,GACV3H,EACH7E,EAAK6E,eAEL1E,GAASuM,GAAyB5M,IAAO,GACzC8B,IAAO,IAEJ4K,IACHpL,GAAmBpB,EAAKM,SAAUN,EAAKO,UACvCJ,GAASL,IAAO,IAEbE,EAAK3E,YAAcmR,IAAYxL,GAAiC,IAAvB/R,KAAK2a,IAAIjiB,EAAIqY,EAAKrY,IAAekZ,GAAkC,IAAvB5R,KAAK2a,IAAIhiB,EAAIoY,EAAKpY,KAAcyZ,IACxH8D,KAEGnF,EAAKX,aACR5M,GAAkBZ,EAAO1K,WAAY6Y,EAAKC,YAC1CC,GAAwBF,EAAKC,WAC7BH,IAAO,GAEP5N,GAAsBL,EAAQwW,IAC9BtW,GAAmBF,EAAQwW,KAErBrI,KAGHlH,OAAS,SAAApR,OAEZklB,EAAI1lB,EAAGqiB,EADJsD,EAAU,CAACnW,MAAM,OAED,IAAhBnC,EAAK4F,SACR0S,EAAQ1S,OAAS5F,EAAK4F,QAAUD,IAE7BzL,GAAKoI,MAAMgF,YAAY,kBAC1BgR,EAAQC,aAAe,QAEX,SAATplB,EAAiB,KACpB+H,GAAiCuX,EAAWhG,GAAWH,EAAU,OAAUtM,EAAK0S,2BAA8BpV,EAAO+F,eAAiB/F,EAAOkC,eAAmBlC,EAAO8F,cAAgB9F,EAAOkC,eAAkBQ,EAAKgL,kBAAoB,eAAiByB,EAAS,QAAU,SAC7Q9Z,EAAI8f,EAAS7a,QACC,IAALjF,GACRqiB,EAAUvC,EAAS9f,GACnB6S,IAAoBnJ,GAAa2Y,EAAS,YAAarD,IACvDtV,GAAa2Y,EAAS,aAAcrD,IACpCtV,GAAa2Y,EAAS,QAASjB,IAAS,GACxC7Z,GAAKgI,IAAI8S,EAASsD,GACdtD,EAAQ/c,SAAW+c,EAAQle,iBAAmB2V,GAAWH,GAC5DpS,GAAKgI,IAAI8S,EAAQle,gBAAiB,CAACuE,YAAa2E,EAAK0S,2BAA6B1S,EAAKgL,kBAAoB,eAAiByB,EAAS,QAAU,UAEhJzM,EAAK2S,kBAAoBtW,GAAa2Y,EAAS,cAAe3J,IAE/DvJ,GAAe2Q,GAAU,UAE1BjV,GAAmBF,EAAQwW,IAC3BlC,GAAU,EACN3K,IAA0B,SAAT9T,GACpB8T,GAAcuR,MAAMxL,GAAe1P,EAAS4P,EAAS,MAAQJ,EAAe,WAAa,YAE1FxP,EAAOqM,UAAY0O,EAAK/a,EAAOqM,WAAc,IAAOvB,KACpDvB,GAAQwR,GAAM5M,EACVuB,IACHA,EAAYzI,SACZyI,EAAYta,QAAQiX,UAAY0O,IAEhCrY,EAAKoO,QAAUtB,IAAiB8D,KACjC5Q,EAAKoO,QAAU3C,EAAK6E,cACb7E,KAGHpH,QAAU,SAAAlR,WAGb6hB,EAFGyD,EAAWhN,EAAKC,WACnB/Y,EAAI8f,EAAS7a,QAEA,IAALjF,GACR8L,GAAUgU,EAAS9f,GAAI,SAAU,SAErB,SAATQ,EAAiB,KACpB+H,GAAiCuX,EAAU,MAC3C9f,EAAI8f,EAAS7a,QACC,IAALjF,GACRqiB,EAAUvC,EAAS9f,GACnB8L,GAAUuW,EAAS,eAAgB,MACnCpY,GAAgBoY,EAAS,YAAarD,IACtC/U,GAAgBoY,EAAS,aAAcrD,IACvC/U,GAAgBoY,EAAS,QAASjB,IAAS,GAC3CnX,GAAgBoY,EAAS,cAAe3J,IAEzCvJ,GAAe2Q,GAAU,GACrBR,IACHrV,GAAgBqV,EAAkB,cAAeE,IACjDvV,GAAgBqV,EAAkB,WAAYE,IAC9CvV,GAAgBqV,EAAkB,YAAaG,KAEhDxV,GAAgByP,GAAU,UAAW8F,IACrCvV,GAAgByP,GAAU,YAAa+F,WAExCzU,GAAsBL,EAAQwW,IAC9BlC,GAAU,EACN3K,IAA0B,SAAT9T,IACpB8T,GAAcyR,QAAQ1L,GAAe1P,EAAS4P,EAAS,MAAQJ,EAAe,WAAa,YAC3FrB,EAAK4D,OAAS5D,EAAK4D,MAAMgE,QAE1BrG,GAAeA,EAAY3I,UAC3BxI,GAAuB0P,IACvBE,EAAKC,WAAaD,EAAK3E,UAAYwL,GAAa,EAChDmG,GAAY5Y,GAAe4L,EAAM,UAAW,aACrCA,KAGHmG,QAAU,SAASvX,EAAOlH,UACvByQ,UAAUhM,OAAUyC,EAAQoR,EAAKlH,OAAOpR,GAAQsY,EAAKpH,QAAQlR,GAASye,KAGzEyB,KAAO,kBACX5H,EAAKoC,YAAa,EAClBpC,EAAK4D,OAAS5D,EAAK4D,MAAMgE,OACzB5H,EAAKpH,UACLnK,GAAKgI,IAAIuQ,EAAU,CAACkG,WAAW,sBACxB9R,GAAQvJ,EAAOqM,WACf8B,KAGHmN,OAAS,gBACRvF,YACA9I,QAAU1R,KAAK0R,OAAOqO,WAGvBzlB,EAAK4S,QAAQ,YACjBiH,EAAcxC,EAAKwC,YAAc,IAAI3K,GAAY/E,EAjxDzC,SAAVub,QAAW/d,EAAKge,OACV,IAAI9d,KAAK8d,EACP9d,KAAKF,IACVA,EAAIE,GAAK8d,EAAS9d,WAGbF,EA2wDmD+d,CAAQ,CAAC7U,OAAO,kBACvEyH,EAAK3E,WAAaqL,GAAU,QAC1BnS,IAEJ1C,EAAOvJ,MAAMglB,UAAazM,IAAWtG,GAAkB,OAAS,SAChE1I,EAAOvJ,MAAMilB,UAAavM,IAAWzG,GAAkB,OAAS,SAChE1I,EAAS0P,EAAYlK,SAGlBgK,EACHwG,EAAU7I,SAAW,GAEjBgC,IACH6G,EAAU9E,GAAS,GAEhBlC,IACHgH,EAAU7E,GAAS,IAIrBrD,GAAQ1H,UAAW,YAAa1D,IAAQA,EAAK0D,QAE7C0D,gCACK7C,YAtwDS,SAAf0U,aAAgBne,EAAKge,OACf,IAAI9d,KAAK8d,EACP9d,KAAKF,IACVA,EAAIE,GAAK8d,EAAS9d,IAyzDtBie,CAAa3P,EAAU4P,UAAW,CAACnN,SAAS,EAAGC,SAAU,EAAGwF,OAAQ,EAAGC,OAAQ,EAAG1E,OAAQ,EAAGE,OAAQ,EAAGvB,YAAY,EAAO5E,WAAW,IAEtIwC,EAAUkK,OAAS,IACnBlK,EAAU6P,QAAU,SAEpBlf,KAAcC,GAAKC,eAAemP"} \ No newline at end of file diff --git a/node_modules/gsap/dist/EasePack.js b/node_modules/gsap/dist/EasePack.js new file mode 100644 index 0000000000000000000000000000000000000000..21280cb62ecfa8baadb761f9bf0de455bdc0f41e --- /dev/null +++ b/node_modules/gsap/dist/EasePack.js @@ -0,0 +1,217 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + /*! + * EasePack 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _registerEase, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _boolean = function _boolean(value, defaultValue) { + return !!(typeof value === "undefined" ? defaultValue : value && !~(value + "").indexOf("false")); + }, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (gsap) { + _registerEase = gsap.registerEase; + + var eases = gsap.parseEase(), + createConfig = function createConfig(ease) { + return function (ratio) { + var y = 0.5 + ratio / 2; + + ease.config = function (p) { + return ease(2 * (1 - p) * p * y + p * p); + }; + }; + }, + p; + + for (p in eases) { + if (!eases[p].config) { + createConfig(eases[p]); + } + } + + _registerEase("slow", SlowMo); + + _registerEase("expoScale", ExpoScaleEase); + + _registerEase("rough", RoughEase); + + for (p in EasePack) { + p !== "version" && gsap.core.globals(p, EasePack[p]); + } + } + }, + _createSlowMo = function _createSlowMo(linearRatio, power, yoyoMode) { + linearRatio = Math.min(1, linearRatio || 0.7); + + var pow = linearRatio < 1 ? power || power === 0 ? power : 0.7 : 0, + p1 = (1 - linearRatio) / 2, + p3 = p1 + linearRatio, + calcEnd = _boolean(yoyoMode); + + return function (p) { + var r = p + (0.5 - p) * pow; + return p < p1 ? calcEnd ? 1 - (p = 1 - p / p1) * p : r - (p = 1 - p / p1) * p * p * p * r : p > p3 ? calcEnd ? p === 1 ? 0 : 1 - (p = (p - p3) / p1) * p : r + (p - r) * (p = (p - p3) / p1) * p * p * p : calcEnd ? 1 : r; + }; + }, + _createExpoScale = function _createExpoScale(start, end, ease) { + var p1 = Math.log(end / start), + p2 = end - start; + ease && (ease = gsap.parseEase(ease)); + return function (p) { + return (start * Math.exp(p1 * (ease ? ease(p) : p)) - start) / p2; + }; + }, + EasePoint = function EasePoint(time, value, next) { + this.t = time; + this.v = value; + + if (next) { + this.next = next; + next.prev = this; + this.c = next.v - value; + this.gap = next.t - time; + } + }, + _createRoughEase = function _createRoughEase(vars) { + if (typeof vars !== "object") { + vars = { + points: +vars || 20 + }; + } + + var taper = vars.taper || "none", + a = [], + cnt = 0, + points = (+vars.points || 20) | 0, + i = points, + randomize = _boolean(vars.randomize, true), + clamp = _boolean(vars.clamp), + template = gsap ? gsap.parseEase(vars.template) : 0, + strength = (+vars.strength || 1) * 0.4, + x, + y, + bump, + invX, + obj, + pnt, + recent; + + while (--i > -1) { + x = randomize ? Math.random() : 1 / points * i; + y = template ? template(x) : x; + + if (taper === "none") { + bump = strength; + } else if (taper === "out") { + invX = 1 - x; + bump = invX * invX * strength; + } else if (taper === "in") { + bump = x * x * strength; + } else if (x < 0.5) { + invX = x * 2; + bump = invX * invX * 0.5 * strength; + } else { + invX = (1 - x) * 2; + bump = invX * invX * 0.5 * strength; + } + + if (randomize) { + y += Math.random() * bump - bump * 0.5; + } else if (i % 2) { + y += bump * 0.5; + } else { + y -= bump * 0.5; + } + + if (clamp) { + if (y > 1) { + y = 1; + } else if (y < 0) { + y = 0; + } + } + + a[cnt++] = { + x: x, + y: y + }; + } + + a.sort(function (a, b) { + return a.x - b.x; + }); + pnt = new EasePoint(1, 1, null); + i = points; + + while (i--) { + obj = a[i]; + pnt = new EasePoint(obj.x, obj.y, pnt); + } + + recent = new EasePoint(0, 0, pnt.t ? pnt : pnt.next); + return function (p) { + var pnt = recent; + + if (p > pnt.t) { + while (pnt.next && p >= pnt.t) { + pnt = pnt.next; + } + + pnt = pnt.prev; + } else { + while (pnt.prev && p <= pnt.t) { + pnt = pnt.prev; + } + } + + recent = pnt; + return pnt.v + (p - pnt.t) / pnt.gap * pnt.c; + }; + }; + + var SlowMo = _createSlowMo(0.7); + SlowMo.ease = SlowMo; + SlowMo.config = _createSlowMo; + var ExpoScaleEase = _createExpoScale(1, 2); + ExpoScaleEase.config = _createExpoScale; + var RoughEase = _createRoughEase(); + RoughEase.ease = RoughEase; + RoughEase.config = _createRoughEase; + var EasePack = { + SlowMo: SlowMo, + RoughEase: RoughEase, + ExpoScaleEase: ExpoScaleEase + }; + + for (var p in EasePack) { + EasePack[p].register = _initCore; + EasePack[p].version = "3.12.7"; + } + + _getGSAP() && gsap.registerPlugin(SlowMo); + + exports.EasePack = EasePack; + exports.ExpoScaleEase = ExpoScaleEase; + exports.RoughEase = RoughEase; + exports.SlowMo = SlowMo; + exports.default = EasePack; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/EasePack.min.js b/node_modules/gsap/dist/EasePack.min.js new file mode 100644 index 0000000000000000000000000000000000000000..bd3ca2520d0162711cfdb59ae6520d4bda08224c --- /dev/null +++ b/node_modules/gsap/dist/EasePack.min.js @@ -0,0 +1,11 @@ +/*! + * EasePack 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,n){"object"==typeof exports&&"undefined"!=typeof module?n(exports):"function"==typeof define&&define.amd?define(["exports"],n):n((e=e||self).window=e.window||{})}(this,function(e){"use strict";function f(){return w||"undefined"!=typeof window&&(w=window.gsap)&&w.registerPlugin&&w}function g(e,n){return!!(void 0===e?n:e&&!~(e+"").indexOf("false"))}function h(e){if(w=e||f()){r=w.registerEase;var n,t=w.parseEase(),o=function createConfig(t){return function(e){var n=.5+e/2;t.config=function(e){return t(2*(1-e)*e*n+e*e)}}};for(n in t)t[n].config||o(t[n]);for(n in r("slow",a),r("expoScale",s),r("rough",u),c)"version"!==n&&w.core.globals(n,c[n])}}function i(e,n,t){var o=(e=Math.min(1,e||.7))<1?n||0===n?n:.7:0,r=(1-e)/2,i=r+e,a=g(t);return function(e){var n=e+(.5-e)*o;return e<r?a?1-(e=1-e/r)*e:n-(e=1-e/r)*e*e*e*n:i<e?a?1===e?0:1-(e=(e-i)/r)*e:n+(e-n)*(e=(e-i)/r)*e*e*e:a?1:n}}function j(n,e,t){var o=Math.log(e/n),r=e-n;return t=t&&w.parseEase(t),function(e){return(n*Math.exp(o*(t?t(e):e))-n)/r}}function k(e,n,t){this.t=e,this.v=n,t&&(((this.next=t).prev=this).c=t.v-n,this.gap=t.t-e)}function l(e){"object"!=typeof e&&(e={points:+e||20});for(var n,t,o,r,i,a,f,s=e.taper||"none",u=[],c=0,p=0|(+e.points||20),l=p,v=g(e.randomize,!0),d=g(e.clamp),h=w?w.parseEase(e.template):0,x=.4*(+e.strength||1);-1<--l;)n=v?Math.random():1/p*l,t=h?h(n):n,o="none"===s?x:"out"===s?(r=1-n)*r*x:"in"===s?n*n*x:n<.5?(r=2*n)*r*.5*x:(r=2*(1-n))*r*.5*x,v?t+=Math.random()*o-.5*o:l%2?t+=.5*o:t-=.5*o,d&&(1<t?t=1:t<0&&(t=0)),u[c++]={x:n,y:t};for(u.sort(function(e,n){return e.x-n.x}),a=new k(1,1,null),l=p;l--;)i=u[l],a=new k(i.x,i.y,a);return f=new k(0,0,a.t?a:a.next),function(e){var n=f;if(e>n.t){for(;n.next&&e>=n.t;)n=n.next;n=n.prev}else for(;n.prev&&e<=n.t;)n=n.prev;return(f=n).v+(e-n.t)/n.gap*n.c}}var w,r,a=i(.7);(a.ease=a).config=i;var s=j(1,2);s.config=j;var u=l();(u.ease=u).config=l;var c={SlowMo:a,RoughEase:u,ExpoScaleEase:s};for(var n in c)c[n].register=h,c[n].version="3.12.7";f()&&w.registerPlugin(a),e.EasePack=c,e.ExpoScaleEase=s,e.RoughEase=u,e.SlowMo=a,e.default=c;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/EasePack.min.js.map b/node_modules/gsap/dist/EasePack.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..7eb2dd1049efdcb3d7884df0bbba34ca8726cbb3 --- /dev/null +++ b/node_modules/gsap/dist/EasePack.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"EasePack.min.js","sources":["../src/EasePack.js"],"sourcesContent":["/*!\n * EasePack 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _coreInitted, _registerEase,\n\t_getGSAP = () => gsap || (typeof(window) !== \"undefined\" && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_boolean = (value, defaultValue) => !!(typeof(value) === \"undefined\" ? defaultValue : value && !~((value + \"\").indexOf(\"false\"))),\n\t_initCore = core => {\n\t\tgsap = core || _getGSAP();\n\t\tif (gsap) {\n\t\t\t_registerEase = gsap.registerEase;\n\t\t\t//add weighted ease capabilities to standard eases so users can do \"power2.inOut(0.8)\" for example to push everything toward the \"out\", or (-0.8) to push it toward the \"in\" (0 is neutral)\n\t\t\tlet eases = gsap.parseEase(),\n\t\t\t\tcreateConfig = ease => ratio => {\n\t\t\t\t\tlet y = 0.5 + ratio / 2;\n\t\t\t\t\tease.config = p => ease(2 * (1 - p) * p * y + p * p);\n\t\t\t\t},\n\t\t\t\tp;\n\t\t\tfor (p in eases) {\n\t\t\t\tif (!eases[p].config) {\n\t\t\t\t\tcreateConfig(eases[p]);\n\t\t\t\t}\n\t\t\t}\n\t\t\t_registerEase(\"slow\", SlowMo);\n\t\t\t_registerEase(\"expoScale\", ExpoScaleEase);\n\t\t\t_registerEase(\"rough\", RoughEase);\n\t\t\tfor (p in EasePack) {\n\t\t\t\tp !== \"version\" && gsap.core.globals(p, EasePack[p]);\n\t\t\t}\n\t\t\t_coreInitted = 1;\n\t\t}\n\t},\n\t_createSlowMo = (linearRatio, power, yoyoMode) => {\n\t\tlinearRatio = Math.min(1, linearRatio || 0.7);\n\t\tlet pow = linearRatio < 1 ? ((power || power === 0) ? power : 0.7) : 0,\n\t\t\tp1 = (1 - linearRatio) / 2,\n\t\t\tp3 = p1 + linearRatio,\n\t\t\tcalcEnd = _boolean(yoyoMode);\n\t\treturn p => {\n\t\t\tlet r = p + (0.5 - p) * pow;\n\t\t\treturn (p < p1) ? (calcEnd ? 1 - ((p = 1 - (p / p1)) * p) : r - ((p = 1 - (p / p1)) * p * p * p * r)) : (p > p3) ? (calcEnd ? (p === 1 ? 0 : 1 - (p = (p - p3) / p1) * p) : r + ((p - r) * (p = (p - p3) / p1) * p * p * p)) : (calcEnd ? 1 : r);\n\t\t}\n\t},\n\t_createExpoScale = (start, end, ease) => {\n\t\tlet p1 = Math.log(end / start),\n\t\t\tp2 = end - start;\n\t\tease && (ease = gsap.parseEase(ease));\n\t\treturn p => (start * Math.exp(p1 * (ease ? ease(p) : p)) - start) / p2;\n\t},\n\tEasePoint = function(time, value, next) {\n\t\tthis.t = time;\n\t\tthis.v = value;\n\t\tif (next) {\n\t\t\tthis.next = next;\n\t\t\tnext.prev = this;\n\t\t\tthis.c = next.v - value;\n\t\t\tthis.gap = next.t - time;\n\t\t}\n\t},\n\t_createRoughEase = vars => {\n\t\tif (typeof(vars) !== \"object\") { //users may pass in via a string, like \"rough(30)\"\n\t\t\tvars = {points: +vars || 20};\n\t\t}\n\t\tlet taper = vars.taper || \"none\",\n\t\t\ta = [],\n\t\t\tcnt = 0,\n\t\t\tpoints = (+vars.points || 20) | 0,\n\t\t\ti = points,\n\t\t\trandomize = _boolean(vars.randomize, true),\n\t\t\tclamp = _boolean(vars.clamp),\n\t\t\ttemplate = gsap ? gsap.parseEase(vars.template) : 0,\n\t\t\tstrength = (+vars.strength || 1) * 0.4,\n\t\t\tx, y, bump, invX, obj, pnt, recent;\n\t\twhile (--i > -1) {\n\t\t\tx = randomize ? Math.random() : (1 / points) * i;\n\t\t\ty = template ? template(x) : x;\n\t\t\tif (taper === \"none\") {\n\t\t\t\tbump = strength;\n\t\t\t} else if (taper === \"out\") {\n\t\t\t\tinvX = 1 - x;\n\t\t\t\tbump = invX * invX * strength;\n\t\t\t} else if (taper === \"in\") {\n\t\t\t\tbump = x * x * strength;\n\t\t\t} else if (x < 0.5) { //\"both\" (start)\n\t\t\t\tinvX = x * 2;\n\t\t\t\tbump = invX * invX * 0.5 * strength;\n\t\t\t} else {\t\t\t\t//\"both\" (end)\n\t\t\t\tinvX = (1 - x) * 2;\n\t\t\t\tbump = invX * invX * 0.5 * strength;\n\t\t\t}\n\t\t\tif (randomize) {\n\t\t\t\ty += (Math.random() * bump) - (bump * 0.5);\n\t\t\t} else if (i % 2) {\n\t\t\t\ty += bump * 0.5;\n\t\t\t} else {\n\t\t\t\ty -= bump * 0.5;\n\t\t\t}\n\t\t\tif (clamp) {\n\t\t\t\tif (y > 1) {\n\t\t\t\t\ty = 1;\n\t\t\t\t} else if (y < 0) {\n\t\t\t\t\ty = 0;\n\t\t\t\t}\n\t\t\t}\n\t\t\ta[cnt++] = {x:x, y:y};\n\t\t}\n\t\ta.sort((a, b) => a.x - b.x);\n\t\tpnt = new EasePoint(1, 1, null);\n\t\ti = points;\n\t\twhile (i--) {\n\t\t\tobj = a[i];\n\t\t\tpnt = new EasePoint(obj.x, obj.y, pnt);\n\t\t}\n\t\trecent = new EasePoint(0, 0, pnt.t ? pnt : pnt.next);\n\t\treturn p => {\n\t\t\tlet pnt = recent;\n\t\t\tif (p > pnt.t) {\n\t\t\t\twhile (pnt.next && p >= pnt.t) {\n\t\t\t\t\tpnt = pnt.next;\n\t\t\t\t}\n\t\t\t\tpnt = pnt.prev;\n\t\t\t} else {\n\t\t\t\twhile (pnt.prev && p <= pnt.t) {\n\t\t\t\t\tpnt = pnt.prev;\n\t\t\t\t}\n\t\t\t}\n\t\t\trecent = pnt;\n\t\t\treturn pnt.v + ((p - pnt.t) / pnt.gap) * pnt.c;\n\t\t};\n\t};\n\nexport const SlowMo = _createSlowMo(0.7);\nSlowMo.ease = SlowMo; //for backward compatibility\nSlowMo.config = _createSlowMo;\n\nexport const ExpoScaleEase = _createExpoScale(1, 2);\nExpoScaleEase.config = _createExpoScale;\n\nexport const RoughEase = _createRoughEase();\nRoughEase.ease = RoughEase; //for backward compatibility\nRoughEase.config = _createRoughEase;\n\nexport const EasePack = {\n\tSlowMo: SlowMo,\n\tRoughEase: RoughEase,\n\tExpoScaleEase: ExpoScaleEase\n};\n\nfor (let p in EasePack) {\n\tEasePack[p].register = _initCore;\n\tEasePack[p].version = \"3.12.7\";\n}\n\n_getGSAP() && gsap.registerPlugin(SlowMo);\n\nexport { EasePack as default };"],"names":["_getGSAP","gsap","window","registerPlugin","_boolean","value","defaultValue","indexOf","_initCore","core","_registerEase","registerEase","p","eases","parseEase","createConfig","ease","ratio","y","config","SlowMo","ExpoScaleEase","RoughEase","EasePack","globals","_createSlowMo","linearRatio","power","yoyoMode","pow","Math","min","p1","p3","calcEnd","r","_createExpoScale","start","end","log","p2","exp","EasePoint","time","next","t","v","prev","this","c","gap","_createRoughEase","vars","points","x","bump","invX","obj","pnt","recent","taper","a","cnt","i","randomize","clamp","template","strength","random","sort","b","register","version"],"mappings":";;;;;;;;;6MAYY,SAAXA,WAAiBC,GAA4B,oBAAZC,SAA4BD,EAAOC,OAAOD,OAASA,EAAKE,gBAAkBF,EAChG,SAAXG,EAAYC,EAAOC,iBAAsC,IAAXD,EAAyBC,EAAeD,MAAaA,EAAQ,IAAIE,QAAQ,UAC3G,SAAZC,EAAYC,MACXR,EAAOQ,GAAQT,IACL,CACTU,EAAgBT,EAAKU,iBAOpBC,EALGC,EAAQZ,EAAKa,YAChBC,EAAe,SAAfA,aAAeC,UAAQ,SAAAC,OAClBC,EAAI,GAAMD,EAAQ,EACtBD,EAAKG,OAAS,SAAAP,UAAKI,EAAK,GAAK,EAAIJ,GAAKA,EAAIM,EAAIN,EAAIA,UAG/CA,KAAKC,EACJA,EAAMD,GAAGO,QACbJ,EAAaF,EAAMD,QAMhBA,KAHLF,EAAc,OAAQU,GACtBV,EAAc,YAAaW,GAC3BX,EAAc,QAASY,GACbC,EACH,YAANX,GAAmBX,EAAKQ,KAAKe,QAAQZ,EAAGW,EAASX,KAKpC,SAAhBa,EAAiBC,EAAaC,EAAOC,OAEhCC,GADJH,EAAcI,KAAKC,IAAI,EAAGL,GAAe,KACjB,EAAMC,GAAmB,IAAVA,EAAeA,EAAQ,GAAO,EACpEK,GAAM,EAAIN,GAAe,EACzBO,EAAKD,EAAKN,EACVQ,EAAU9B,EAASwB,UACb,SAAAhB,OACFuB,EAAIvB,GAAK,GAAMA,GAAKiB,SAChBjB,EAAIoB,EAAOE,EAAU,GAAMtB,EAAI,EAAKA,EAAIoB,GAAOpB,EAAKuB,GAAMvB,EAAI,EAAKA,EAAIoB,GAAOpB,EAAIA,EAAIA,EAAIuB,EAAWF,EAAJrB,EAAWsB,EAAiB,IAANtB,EAAU,EAAI,GAAKA,GAAKA,EAAIqB,GAAMD,GAAMpB,EAAKuB,GAAMvB,EAAIuB,IAAMvB,GAAKA,EAAIqB,GAAMD,GAAMpB,EAAIA,EAAIA,EAAOsB,EAAU,EAAIC,GAG7N,SAAnBC,EAAoBC,EAAOC,EAAKtB,OAC3BgB,EAAKF,KAAKS,IAAID,EAAMD,GACvBG,EAAKF,EAAMD,SACHrB,EAATA,GAAgBf,EAAKa,UAAUE,GACxB,SAAAJ,UAAMyB,EAAQP,KAAKW,IAAIT,GAAMhB,EAAOA,EAAKJ,GAAKA,IAAMyB,GAASG,GAEzD,SAAZE,EAAqBC,EAAMtC,EAAOuC,QAC5BC,EAAIF,OACJG,EAAIzC,EACLuC,WACEA,KAAOA,GACPG,KAAOC,MACPC,EAAIL,EAAKE,EAAIzC,OACb6C,IAAMN,EAAKC,EAAIF,GAGH,SAAnBQ,EAAmBC,GACG,iBAAVA,IACVA,EAAO,CAACC,QAASD,GAAQ,aAWzBE,EAAGpC,EAAGqC,EAAMC,EAAMC,EAAKC,EAAKC,EATzBC,EAAQR,EAAKQ,OAAS,OACzBC,EAAI,GACJC,EAAM,EACNT,EAAgC,IAArBD,EAAKC,QAAU,IAC1BU,EAAIV,EACJW,EAAY5D,EAASgD,EAAKY,WAAW,GACrCC,EAAQ7D,EAASgD,EAAKa,OACtBC,EAAWjE,EAAOA,EAAKa,UAAUsC,EAAKc,UAAY,EAClDC,EAAmC,KAAtBf,EAAKe,UAAY,IAEjB,IAALJ,GACRT,EAAIU,EAAYlC,KAAKsC,SAAY,EAAIf,EAAUU,EAC/C7C,EAAIgD,EAAWA,EAASZ,GAAKA,EAE5BC,EADa,SAAVK,EACIO,EACa,QAAVP,GACVJ,EAAO,EAAIF,GACGE,EAAOW,EACD,OAAVP,EACHN,EAAIA,EAAIa,EACLb,EAAI,IACdE,EAAW,EAAJF,GACOE,EAAO,GAAMW,GAE3BX,EAAiB,GAAT,EAAIF,IACEE,EAAO,GAAMW,EAExBH,EACH9C,GAAMY,KAAKsC,SAAWb,EAAgB,GAAPA,EACrBQ,EAAI,EACd7C,GAAY,GAAPqC,EAELrC,GAAY,GAAPqC,EAEFU,IACK,EAAJ/C,EACHA,EAAI,EACMA,EAAI,IACdA,EAAI,IAGN2C,EAAEC,KAAS,CAACR,EAAEA,EAAGpC,EAAEA,OAEpB2C,EAAEQ,KAAK,SAACR,EAAGS,UAAMT,EAAEP,EAAIgB,EAAEhB,IACzBI,EAAM,IAAIhB,EAAU,EAAG,EAAG,MAC1BqB,EAAIV,EACGU,KACNN,EAAMI,EAAEE,GACRL,EAAM,IAAIhB,EAAUe,EAAIH,EAAGG,EAAIvC,EAAGwC,UAEnCC,EAAS,IAAIjB,EAAU,EAAG,EAAGgB,EAAIb,EAAIa,EAAMA,EAAId,MACxC,SAAAhC,OACF8C,EAAMC,KACN/C,EAAI8C,EAAIb,EAAG,MACPa,EAAId,MAAQhC,GAAK8C,EAAIb,GAC3Ba,EAAMA,EAAId,KAEXc,EAAMA,EAAIX,eAEHW,EAAIX,MAAQnC,GAAK8C,EAAIb,GAC3Ba,EAAMA,EAAIX,YAGZY,EAASD,GACEZ,GAAMlC,EAAI8C,EAAIb,GAAKa,EAAIR,IAAOQ,EAAIT,OA3H5ChD,EAAoBS,EA+HXU,EAASK,EAAc,KACpCL,EAAOJ,KAAOI,GACPD,OAASM,MAEHJ,EAAgBe,EAAiB,EAAG,GACjDf,EAAcF,OAASiB,MAEVd,EAAY6B,KACzB7B,EAAUN,KAAOM,GACPH,OAASgC,MAEN5B,EAAW,CACvBH,OAAQA,EACRE,UAAWA,EACXD,cAAeA,GAGhB,IAAK,IAAIT,KAAKW,EACbA,EAASX,GAAG2D,SAAW/D,EACvBe,EAASX,GAAG4D,QAAU,SAGvBxE,KAAcC,EAAKE,eAAeiB"} \ No newline at end of file diff --git a/node_modules/gsap/dist/EaselPlugin.js b/node_modules/gsap/dist/EaselPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..fff0b761136524ea32d47ef3527d1f462251589e --- /dev/null +++ b/node_modules/gsap/dist/EaselPlugin.js @@ -0,0 +1,351 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + /*! + * EaselPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _coreInitted, + _win, + _createJS, + _ColorFilter, + _ColorMatrixFilter, + _colorProps = "redMultiplier,greenMultiplier,blueMultiplier,alphaMultiplier,redOffset,greenOffset,blueOffset,alphaOffset".split(","), + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _getCreateJS = function _getCreateJS() { + return _createJS || _win && _win.createjs || _win || {}; + }, + _warn = function _warn(message) { + return console.warn(message); + }, + _cache = function _cache(target) { + var b = target.getBounds && target.getBounds(); + + if (!b) { + b = target.nominalBounds || { + x: 0, + y: 0, + width: 100, + height: 100 + }; + target.setBounds && target.setBounds(b.x, b.y, b.width, b.height); + } + + target.cache && target.cache(b.x, b.y, b.width, b.height); + + _warn("EaselPlugin: for filters to display in EaselJS, you must call the object's cache() method first. GSAP attempted to use the target's getBounds() for the cache but that may not be completely accurate. " + target); + }, + _parseColorFilter = function _parseColorFilter(target, v, plugin) { + if (!_ColorFilter) { + _ColorFilter = _getCreateJS().ColorFilter; + + if (!_ColorFilter) { + _warn("EaselPlugin error: The EaselJS ColorFilter JavaScript file wasn't loaded."); + } + } + + var filters = target.filters || [], + i = filters.length, + c, + s, + e, + a, + p, + pt; + + while (i--) { + if (filters[i] instanceof _ColorFilter) { + s = filters[i]; + break; + } + } + + if (!s) { + s = new _ColorFilter(); + filters.push(s); + target.filters = filters; + } + + e = s.clone(); + + if (v.tint != null) { + c = gsap.utils.splitColor(v.tint); + a = v.tintAmount != null ? +v.tintAmount : 1; + e.redOffset = +c[0] * a; + e.greenOffset = +c[1] * a; + e.blueOffset = +c[2] * a; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - a; + } else { + for (p in v) { + if (p !== "exposure") if (p !== "brightness") { + e[p] = +v[p]; + } + } + } + + if (v.exposure != null) { + e.redOffset = e.greenOffset = e.blueOffset = 255 * (+v.exposure - 1); + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1; + } else if (v.brightness != null) { + a = +v.brightness - 1; + e.redOffset = e.greenOffset = e.blueOffset = a > 0 ? a * 255 : 0; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - Math.abs(a); + } + + i = 8; + + while (i--) { + p = _colorProps[i]; + + if (s[p] !== e[p]) { + pt = plugin.add(s, p, s[p], e[p], 0, 0, 0, 0, 0, 1); + + if (pt) { + pt.op = "easel_colorFilter"; + } + } + } + + plugin._props.push("easel_colorFilter"); + + if (!target.cacheID) { + _cache(target); + } + }, + _idMatrix = [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + _applyMatrix = function _applyMatrix(m, m2) { + if (!(m instanceof Array) || !(m2 instanceof Array)) { + return m2; + } + + var temp = [], + i = 0, + z = 0, + y, + x; + + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = x === 4 ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i + 1] * m2[x + 5] + m[i + 2] * m2[x + 10] + m[i + 3] * m2[x + 15] + z; + } + + i += 5; + } + + return temp; + }, + _setSaturation = function _setSaturation(m, n) { + if (isNaN(n)) { + return m; + } + + var inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); + }, + _colorize = function _colorize(m, color, amount) { + if (isNaN(amount)) { + amount = 1; + } + + var c = gsap.utils.splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); + }, + _setHue = function _setHue(m, n) { + if (isNaN(n)) { + return m; + } + + n *= Math.PI / 180; + var c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([_lumR + c * (1 - _lumR) + s * -_lumR, _lumG + c * -_lumG + s * -_lumG, _lumB + c * -_lumB + s * (1 - _lumB), 0, 0, _lumR + c * -_lumR + s * 0.143, _lumG + c * (1 - _lumG) + s * 0.14, _lumB + c * -_lumB + s * -0.283, 0, 0, _lumR + c * -_lumR + s * -(1 - _lumR), _lumG + c * -_lumG + s * _lumG, _lumB + c * (1 - _lumB) + s * _lumB, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); + }, + _setContrast = function _setContrast(m, n) { + if (isNaN(n)) { + return m; + } + + n += 0.01; + return _applyMatrix([n, 0, 0, 0, 128 * (1 - n), 0, n, 0, 0, 128 * (1 - n), 0, 0, n, 0, 128 * (1 - n), 0, 0, 0, 1, 0], m); + }, + _parseColorMatrixFilter = function _parseColorMatrixFilter(target, v, plugin) { + if (!_ColorMatrixFilter) { + _ColorMatrixFilter = _getCreateJS().ColorMatrixFilter; + + if (!_ColorMatrixFilter) { + _warn("EaselPlugin: The EaselJS ColorMatrixFilter JavaScript file wasn't loaded."); + } + } + + var filters = target.filters || [], + i = filters.length, + matrix, + startMatrix, + s, + pg; + + while (--i > -1) { + if (filters[i] instanceof _ColorMatrixFilter) { + s = filters[i]; + break; + } + } + + if (!s) { + s = new _ColorMatrixFilter(_idMatrix.slice()); + filters.push(s); + target.filters = filters; + } + + startMatrix = s.matrix; + matrix = _idMatrix.slice(); + + if (v.colorize != null) { + matrix = _colorize(matrix, v.colorize, Number(v.colorizeAmount)); + } + + if (v.contrast != null) { + matrix = _setContrast(matrix, Number(v.contrast)); + } + + if (v.hue != null) { + matrix = _setHue(matrix, Number(v.hue)); + } + + if (v.saturation != null) { + matrix = _setSaturation(matrix, Number(v.saturation)); + } + + i = matrix.length; + + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg = plugin.add(startMatrix, i, startMatrix[i], matrix[i], 0, 0, 0, 0, 0, 1); + + if (pg) { + pg.op = "easel_colorMatrixFilter"; + } + } + } + + plugin._props.push("easel_colorMatrixFilter"); + + if (!target.cacheID) { + _cache(); + } + + plugin._matrix = startMatrix; + }, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (_windowExists()) { + _win = window; + } + + if (gsap) { + _coreInitted = 1; + } + }; + + var EaselPlugin = { + version: "3.12.7", + name: "easel", + init: function init(target, value, tween, index, targets) { + if (!_coreInitted) { + _initCore(); + + if (!gsap) { + _warn("Please gsap.registerPlugin(EaselPlugin)"); + } + } + + this.target = target; + var p, pt, tint, colorMatrix, end, labels, i; + + for (p in value) { + end = value[p]; + + if (p === "colorFilter" || p === "tint" || p === "tintAmount" || p === "exposure" || p === "brightness") { + if (!tint) { + _parseColorFilter(target, value.colorFilter || value, this); + + tint = true; + } + } else if (p === "saturation" || p === "contrast" || p === "hue" || p === "colorize" || p === "colorizeAmount") { + if (!colorMatrix) { + _parseColorMatrixFilter(target, value.colorMatrixFilter || value, this); + + colorMatrix = true; + } + } else if (p === "frame") { + if (typeof end === "string" && end.charAt(1) !== "=" && (labels = target.labels)) { + for (i = 0; i < labels.length; i++) { + if (labels[i].label === end) { + end = labels[i].position; + } + } + } + + pt = this.add(target, "gotoAndStop", target.currentFrame, end, index, targets, Math.round, 0, 0, 1); + + if (pt) { + pt.op = p; + } + } else if (target[p] != null) { + this.add(target, p, "get", end); + } + } + }, + render: function render(ratio, data) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + if (data.target.cacheID) { + data.target.updateCache(); + } + }, + register: _initCore + }; + + EaselPlugin.registerCreateJS = function (createjs) { + _createJS = createjs; + }; + + _getGSAP() && gsap.registerPlugin(EaselPlugin); + + exports.EaselPlugin = EaselPlugin; + exports.default = EaselPlugin; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/EaselPlugin.min.js b/node_modules/gsap/dist/EaselPlugin.min.js new file mode 100644 index 0000000000000000000000000000000000000000..540ec13b5f4a3fcee857e738caa65288dc5a6a6b --- /dev/null +++ b/node_modules/gsap/dist/EaselPlugin.min.js @@ -0,0 +1,11 @@ +/*! + * EaselPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(e){"use strict";function k(){return"undefined"!=typeof window}function l(){return h||k()&&(h=window.gsap)&&h.registerPlugin&&h}function m(){return r||t&&t.createjs||t||{}}function n(e){return console.warn(e)}function o(e){var t=e.getBounds&&e.getBounds();t||(t=e.nominalBounds||{x:0,y:0,width:100,height:100},e.setBounds&&e.setBounds(t.x,t.y,t.width,t.height)),e.cache&&e.cache(t.x,t.y,t.width,t.height),n("EaselPlugin: for filters to display in EaselJS, you must call the object's cache() method first. GSAP attempted to use the target's getBounds() for the cache but that may not be completely accurate. "+e)}function p(e,t,r){(b=b||m().ColorFilter)||n("EaselPlugin error: The EaselJS ColorFilter JavaScript file wasn't loaded.");for(var i,l,s,u,a,f,c=e.filters||[],d=c.length;d--;)if(c[d]instanceof b){l=c[d];break}if(l||(l=new b,c.push(l),e.filters=c),s=l.clone(),null!=t.tint)i=h.utils.splitColor(t.tint),u=null!=t.tintAmount?+t.tintAmount:1,s.redOffset=i[0]*u,s.greenOffset=i[1]*u,s.blueOffset=i[2]*u,s.redMultiplier=s.greenMultiplier=s.blueMultiplier=1-u;else for(a in t)"exposure"!==a&&"brightness"!==a&&(s[a]=+t[a]);for(null!=t.exposure?(s.redOffset=s.greenOffset=s.blueOffset=255*(t.exposure-1),s.redMultiplier=s.greenMultiplier=s.blueMultiplier=1):null!=t.brightness&&(u=t.brightness-1,s.redOffset=s.greenOffset=s.blueOffset=0<u?255*u:0,s.redMultiplier=s.greenMultiplier=s.blueMultiplier=1-Math.abs(u)),d=8;d--;)l[a=M[d]]!==s[a]&&(f=r.add(l,a,l[a],s[a],0,0,0,0,0,1))&&(f.op="easel_colorFilter");r._props.push("easel_colorFilter"),e.cacheID||o(e)}function u(e,t){if(!(e instanceof Array&&t instanceof Array))return t;var r,i,n=[],l=0,o=0;for(r=0;r<4;r++){for(i=0;i<5;i++)o=4===i?e[l+4]:0,n[l+i]=e[l]*t[i]+e[l+1]*t[i+5]+e[l+2]*t[i+10]+e[l+3]*t[i+15]+o;l+=5}return n}function z(e,t,r){(d=d||m().ColorMatrixFilter)||n("EaselPlugin: The EaselJS ColorMatrixFilter JavaScript file wasn't loaded.");for(var i,l,s,a,f=e.filters||[],c=f.length;-1<--c;)if(f[c]instanceof d){s=f[c];break}for(s||(s=new d(w.slice()),f.push(s),e.filters=f),l=s.matrix,i=w.slice(),null!=t.colorize&&(i=function _colorize(e,t,r){isNaN(r)&&(r=1);var i=h.utils.splitColor(t),n=i[0]/255,l=i[1]/255,o=i[2]/255,s=1-r;return u([s+r*n*x,r*n*y,r*n*_,0,0,r*l*x,s+r*l*y,r*l*_,0,0,r*o*x,r*o*y,s+r*o*_,0,0,0,0,0,1,0],e)}(i,t.colorize,Number(t.colorizeAmount))),null!=t.contrast&&(i=function _setContrast(e,t){return isNaN(t)?e:u([t+=.01,0,0,0,128*(1-t),0,t,0,0,128*(1-t),0,0,t,0,128*(1-t),0,0,0,1,0],e)}(i,Number(t.contrast))),null!=t.hue&&(i=function _setHue(e,t){if(isNaN(t))return e;t*=Math.PI/180;var r=Math.cos(t),i=Math.sin(t);return u([x+r*(1-x)+i*-x,y+r*-y+i*-y,_+r*-_+i*(1-_),0,0,x+r*-x+.143*i,y+r*(1-y)+.14*i,_+r*-_+-.283*i,0,0,x+r*-x+i*-(1-x),y+r*-y+i*y,_+r*(1-_)+i*_,0,0,0,0,0,1,0,0,0,0,0,1],e)}(i,Number(t.hue))),null!=t.saturation&&(i=function _setSaturation(e,t){if(isNaN(t))return e;var r=1-t,i=r*x,n=r*y,l=r*_;return u([i+t,n,l,0,0,i,n+t,l,0,0,i,n,l+t,0,0,0,0,0,1,0],e)}(i,Number(t.saturation))),c=i.length;-1<--c;)i[c]!==l[c]&&(a=r.add(l,c,l[c],i[c],0,0,0,0,0,1))&&(a.op="easel_colorMatrixFilter");r._props.push("easel_colorMatrixFilter"),e.cacheID||o(),r._matrix=l}function A(e){h=e||l(),k()&&(t=window),h&&(g=1)}var h,g,t,r,b,d,M="redMultiplier,greenMultiplier,blueMultiplier,alphaMultiplier,redOffset,greenOffset,blueOffset,alphaOffset".split(","),w=[1,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,0,1,0],x=.212671,y=.71516,_=.072169,i={version:"3.12.7",name:"easel",init:function init(e,t,r,i,l){var o,s,u,a,f,c,d;for(o in g||(A(),h||n("Please gsap.registerPlugin(EaselPlugin)")),this.target=e,t)if(f=t[o],"colorFilter"===o||"tint"===o||"tintAmount"===o||"exposure"===o||"brightness"===o)u||(p(e,t.colorFilter||t,this),u=!0);else if("saturation"===o||"contrast"===o||"hue"===o||"colorize"===o||"colorizeAmount"===o)a||(z(e,t.colorMatrixFilter||t,this),a=!0);else if("frame"===o){if("string"==typeof f&&"="!==f.charAt(1)&&(c=e.labels))for(d=0;d<c.length;d++)c[d].label===f&&(f=c[d].position);(s=this.add(e,"gotoAndStop",e.currentFrame,f,i,l,Math.round,0,0,1))&&(s.op=o)}else null!=e[o]&&this.add(e,o,"get",f)},render:function render(e,t){for(var r=t._pt;r;)r.r(e,r.d),r=r._next;t.target.cacheID&&t.target.updateCache()},register:A,registerCreateJS:function(e){r=e}};l()&&h.registerPlugin(i),e.EaselPlugin=i,e.default=i;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/EaselPlugin.min.js.map b/node_modules/gsap/dist/EaselPlugin.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..133701575476ac592181df07a1df2e3b37747168 --- /dev/null +++ b/node_modules/gsap/dist/EaselPlugin.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"EaselPlugin.min.js","sources":["../src/EaselPlugin.js"],"sourcesContent":["/*!\n * EaselPlugin 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _coreInitted, _win, _createJS, _ColorFilter, _ColorMatrixFilter,\n\t_colorProps = \"redMultiplier,greenMultiplier,blueMultiplier,alphaMultiplier,redOffset,greenOffset,blueOffset,alphaOffset\".split(\",\"),\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_getCreateJS = () => _createJS || (_win && _win.createjs) || _win || {},\n\t_warn = message => console.warn(message),\n\t_cache = target => {\n\t\tlet b = target.getBounds && target.getBounds();\n\t\tif (!b) {\n\t\t\tb = target.nominalBounds || {x:0, y:0, width: 100, height: 100};\n\t\t\ttarget.setBounds && target.setBounds(b.x, b.y, b.width, b.height);\n\t\t}\n\t\ttarget.cache && target.cache(b.x, b.y, b.width, b.height);\n\t\t_warn(\"EaselPlugin: for filters to display in EaselJS, you must call the object's cache() method first. GSAP attempted to use the target's getBounds() for the cache but that may not be completely accurate. \" + target);\n\t},\n\t_parseColorFilter = (target, v, plugin) => {\n\t\tif (!_ColorFilter) {\n\t\t\t_ColorFilter = _getCreateJS().ColorFilter;\n\t\t\tif (!_ColorFilter) {\n\t\t\t\t_warn(\"EaselPlugin error: The EaselJS ColorFilter JavaScript file wasn't loaded.\");\n\t\t\t}\n\t\t}\n\t\tlet filters = target.filters || [],\n\t\t\ti = filters.length,\n\t\t\tc, s, e, a, p, pt;\n\t\twhile (i--) {\n\t\t\tif (filters[i] instanceof _ColorFilter) {\n\t\t\t\ts = filters[i];\n\t\t\t\tbreak;\n\t\t\t}\n\t\t}\n\t\tif (!s) {\n\t\t\ts = new _ColorFilter();\n\t\t\tfilters.push(s);\n\t\t\ttarget.filters = filters;\n\t\t}\n\t\te = s.clone();\n\t\tif (v.tint != null) {\n\t\t\tc = gsap.utils.splitColor(v.tint);\n\t\t\ta = (v.tintAmount != null) ? +v.tintAmount : 1;\n\t\t\te.redOffset = +c[0] * a;\n\t\t\te.greenOffset = +c[1] * a;\n\t\t\te.blueOffset = +c[2] * a;\n\t\t\te.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - a;\n\t\t} else {\n\t\t\tfor (p in v) {\n\t\t\t\tif (p !== \"exposure\") if (p !== \"brightness\") {\n\t\t\t\t\te[p] = +v[p];\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tif (v.exposure != null) {\n\t\t\te.redOffset = e.greenOffset = e.blueOffset = 255 * (+v.exposure - 1);\n\t\t\te.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1;\n\t\t} else if (v.brightness != null) {\n\t\t\ta = +v.brightness - 1;\n\t\t\te.redOffset = e.greenOffset = e.blueOffset = (a > 0) ? a * 255 : 0;\n\t\t\te.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - Math.abs(a);\n\t\t}\n\t\ti = 8;\n\t\twhile (i--) {\n\t\t\tp = _colorProps[i];\n\t\t\tif (s[p] !== e[p]) {\n\t\t\t\tpt = plugin.add(s, p, s[p], e[p], 0, 0, 0, 0, 0, 1);\n\t\t\t\tif (pt) {\n\t\t\t\t\tpt.op = \"easel_colorFilter\";\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tplugin._props.push(\"easel_colorFilter\");\n\t\tif (!target.cacheID) {\n\t\t\t_cache(target);\n\t\t}\n\t},\n\n\t_idMatrix = [1,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,0,1,0],\n\t_lumR = 0.212671,\n\t_lumG = 0.715160,\n\t_lumB = 0.072169,\n\n\t_applyMatrix = (m, m2) => {\n\t\tif (!(m instanceof Array) || !(m2 instanceof Array)) {\n\t\t\treturn m2;\n\t\t}\n\t\tlet temp = [],\n\t\t\ti = 0,\n\t\t\tz = 0,\n\t\t\ty, x;\n\t\tfor (y = 0; y < 4; y++) {\n\t\t\tfor (x = 0; x < 5; x++) {\n\t\t\t\tz = (x === 4) ? m[i + 4] : 0;\n\t\t\t\ttemp[i + x] = m[i] * m2[x] + m[i+1] * m2[x + 5] +\tm[i+2] * m2[x + 10] + m[i+3] * m2[x + 15] +\tz;\n\t\t\t}\n\t\t\ti += 5;\n\t\t}\n\t\treturn temp;\n\t},\n\n\t_setSaturation = (m, n) => {\n\t\tif (isNaN(n)) {\n\t\t\treturn m;\n\t\t}\n\t\tlet inv = 1 - n,\n\t\t\tr = inv * _lumR,\n\t\t\tg = inv * _lumG,\n\t\t\tb = inv * _lumB;\n\t\treturn _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m);\n\t},\n\n\t_colorize = (m, color, amount) => {\n\t\tif (isNaN(amount)) {\n\t\t\tamount = 1;\n\t\t}\n\t\tlet c = gsap.utils.splitColor(color),\n\t\t\tr = c[0] / 255,\n\t\t\tg = c[1] / 255,\n\t\t\tb = c[2] / 255,\n\t\t\tinv = 1 - amount;\n\t\treturn _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m);\n\t},\n\n\t_setHue = (m, n) => {\n\t\tif (isNaN(n)) {\n\t\t\treturn m;\n\t\t}\n\t\tn *= Math.PI / 180;\n\t\tlet c = Math.cos(n),\n\t\t\ts = Math.sin(n);\n\t\treturn _applyMatrix([(_lumR + (c * (1 - _lumR))) + (s * (-_lumR)), (_lumG + (c * (-_lumG))) + (s * (-_lumG)), (_lumB + (c * (-_lumB))) + (s * (1 - _lumB)), 0, 0, (_lumR + (c * (-_lumR))) + (s * 0.143), (_lumG + (c * (1 - _lumG))) + (s * 0.14), (_lumB + (c * (-_lumB))) + (s * -0.283), 0, 0, (_lumR + (c * (-_lumR))) + (s * (-(1 - _lumR))), (_lumG + (c * (-_lumG))) + (s * _lumG), (_lumB + (c * (1 - _lumB))) + (s * _lumB), 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m);\n\t},\n\n\t_setContrast = (m, n) => {\n\t\tif (isNaN(n)) {\n\t\t\treturn m;\n\t\t}\n\t\tn += 0.01;\n\t\treturn _applyMatrix([n,0,0,0,128 * (1 - n), 0,n,0,0,128 * (1 - n), 0,0,n,0,128 * (1 - n), 0,0,0,1,0], m);\n\t},\n\n\t_parseColorMatrixFilter = (target, v, plugin) => {\n\t\tif (!_ColorMatrixFilter) {\n\t\t\t_ColorMatrixFilter = _getCreateJS().ColorMatrixFilter;\n\t\t\tif (!_ColorMatrixFilter) {\n\t\t\t\t_warn(\"EaselPlugin: The EaselJS ColorMatrixFilter JavaScript file wasn't loaded.\");\n\t\t\t}\n\t\t}\n\t\tlet filters = target.filters || [],\n\t\t\ti = filters.length,\n\t\t\tmatrix, startMatrix, s, pg;\n\t\twhile (--i > -1) {\n\t\t\tif (filters[i] instanceof _ColorMatrixFilter) {\n\t\t\t\ts = filters[i];\n\t\t\t\tbreak;\n\t\t\t}\n\t\t}\n\t\tif (!s) {\n\t\t\ts = new _ColorMatrixFilter(_idMatrix.slice());\n\t\t\tfilters.push(s);\n\t\t\ttarget.filters = filters;\n\t\t}\n\t\tstartMatrix = s.matrix;\n\t\tmatrix = _idMatrix.slice();\n\t\tif (v.colorize != null) {\n\t\t\tmatrix = _colorize(matrix, v.colorize, Number(v.colorizeAmount));\n\t\t}\n\t\tif (v.contrast != null) {\n\t\t\tmatrix = _setContrast(matrix, Number(v.contrast));\n\t\t}\n\t\tif (v.hue != null) {\n\t\t\tmatrix = _setHue(matrix, Number(v.hue));\n\t\t}\n\t\tif (v.saturation != null) {\n\t\t\tmatrix = _setSaturation(matrix, Number(v.saturation));\n\t\t}\n\n\t\ti = matrix.length;\n\t\twhile (--i > -1) {\n\t\t\tif (matrix[i] !== startMatrix[i]) {\n\t\t\t\tpg = plugin.add(startMatrix, i, startMatrix[i], matrix[i], 0, 0, 0, 0, 0, 1);\n\t\t\t\tif (pg) {\n\t\t\t\t\tpg.op = \"easel_colorMatrixFilter\";\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\n\t\tplugin._props.push(\"easel_colorMatrixFilter\");\n\t\tif (!target.cacheID) {\n\t\t\t_cache();\n\t\t}\n\n\t\tplugin._matrix = startMatrix;\n\t},\n\n\t_initCore = core => {\n\t\tgsap = core || _getGSAP();\n\t\tif (_windowExists()) {\n\t\t\t_win = window;\n\t\t}\n\t\tif (gsap) {\n\n\t\t\t_coreInitted = 1;\n\t\t}\n\t};\n\n\nexport const EaselPlugin = {\n\tversion: \"3.12.7\",\n\tname: \"easel\",\n\tinit(target, value, tween, index, targets) {\n\t\tif (!_coreInitted) {\n\t\t\t_initCore();\n\t\t\tif (!gsap) {\n\t\t\t\t_warn(\"Please gsap.registerPlugin(EaselPlugin)\");\n\t\t\t}\n\t\t}\n\t\tthis.target = target;\n\t\tlet p, pt, tint, colorMatrix, end, labels, i;\n\t\tfor (p in value) {\n\n\t\t\tend = value[p];\n\t\t\tif (p === \"colorFilter\" || p === \"tint\" || p === \"tintAmount\" || p === \"exposure\" || p === \"brightness\") {\n\t\t\t\tif (!tint) {\n\t\t\t\t\t_parseColorFilter(target, value.colorFilter || value, this);\n\t\t\t\t\ttint = true;\n\t\t\t\t}\n\n\t\t\t} else if (p === \"saturation\" || p === \"contrast\" || p === \"hue\" || p === \"colorize\" || p === \"colorizeAmount\") {\n\t\t\t\tif (!colorMatrix) {\n\t\t\t\t\t_parseColorMatrixFilter(target, value.colorMatrixFilter || value, this);\n\t\t\t\t\tcolorMatrix = true;\n\t\t\t\t}\n\n\t\t\t} else if (p === \"frame\") {\n\t\t\t\tif (typeof(end) === \"string\" && end.charAt(1) !== \"=\" && (labels = target.labels)) {\n\t\t\t\t\tfor (i = 0; i < labels.length; i++) {\n\t\t\t\t\t\tif (labels[i].label === end) {\n\t\t\t\t\t\t\tend = labels[i].position;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tpt = this.add(target, \"gotoAndStop\", target.currentFrame, end, index, targets, Math.round, 0, 0, 1);\n\t\t\t\tif (pt) {\n\t\t\t\t\tpt.op = p;\n\t\t\t\t}\n\n\t\t\t} else if (target[p] != null) {\n\t\t\t\tthis.add(target, p, \"get\", end);\n\t\t\t}\n\n\t\t}\n\t},\n\trender(ratio, data) {\n\t\tlet pt = data._pt;\n\t\twhile (pt) {\n\t\t\tpt.r(ratio, pt.d);\n\t\t\tpt = pt._next;\n\t\t}\n\t\tif (data.target.cacheID) {\n\t\t\tdata.target.updateCache();\n\t\t}\n\t},\n\tregister: _initCore\n};\n\nEaselPlugin.registerCreateJS = createjs => {\n\t_createJS = createjs;\n};\n\n_getGSAP() && gsap.registerPlugin(EaselPlugin);\n\nexport { EaselPlugin as default };"],"names":["_windowExists","window","_getGSAP","gsap","registerPlugin","_getCreateJS","_createJS","_win","createjs","_warn","message","console","warn","_cache","target","b","getBounds","nominalBounds","x","y","width","height","setBounds","cache","_parseColorFilter","v","plugin","_ColorFilter","ColorFilter","c","s","e","a","p","pt","filters","i","length","push","clone","tint","utils","splitColor","tintAmount","redOffset","greenOffset","blueOffset","redMultiplier","greenMultiplier","blueMultiplier","exposure","brightness","Math","abs","_colorProps","add","op","_props","cacheID","_applyMatrix","m","m2","Array","temp","z","_parseColorMatrixFilter","_ColorMatrixFilter","ColorMatrixFilter","matrix","startMatrix","pg","_idMatrix","slice","colorize","_colorize","color","amount","isNaN","r","g","inv","_lumR","_lumG","_lumB","Number","colorizeAmount","contrast","_setContrast","n","hue","_setHue","PI","cos","sin","saturation","_setSaturation","_matrix","_initCore","core","_coreInitted","split","EaselPlugin","version","name","init","value","tween","index","targets","colorMatrix","end","labels","colorFilter","this","colorMatrixFilter","charAt","label","position","currentFrame","round","render","ratio","data","_pt","d","_next","updateCache","register"],"mappings":";;;;;;;;;6MAaiB,SAAhBA,UAAyC,oBAAZC,OAClB,SAAXC,WAAiBC,GAASH,MAAoBG,EAAOF,OAAOE,OAASA,EAAKC,gBAAkBD,EAC7E,SAAfE,WAAqBC,GAAcC,GAAQA,EAAKC,UAAaD,GAAQ,GAC7D,SAARE,EAAQC,UAAWC,QAAQC,KAAKF,GACvB,SAATG,EAASC,OACJC,EAAID,EAAOE,WAAaF,EAAOE,YAC9BD,IACJA,EAAID,EAAOG,eAAiB,CAACC,EAAE,EAAGC,EAAE,EAAGC,MAAO,IAAKC,OAAQ,KAC3DP,EAAOQ,WAAaR,EAAOQ,UAAUP,EAAEG,EAAGH,EAAEI,EAAGJ,EAAEK,MAAOL,EAAEM,SAE3DP,EAAOS,OAAST,EAAOS,MAAMR,EAAEG,EAAGH,EAAEI,EAAGJ,EAAEK,MAAOL,EAAEM,QAClDZ,EAAM,0MAA4MK,GAE/L,SAApBU,EAAqBV,EAAQW,EAAGC,IAE9BC,EADIA,GACWtB,IAAeuB,cAE7BnB,EAAM,qFAKPoB,EAAGC,EAAGC,EAAGC,EAAGC,EAAGC,EAFZC,EAAUrB,EAAOqB,SAAW,GAC/BC,EAAID,EAAQE,OAEND,QACFD,EAAQC,aAAcT,EAAc,CACvCG,EAAIK,EAAQC,YAITN,IACJA,EAAI,IAAIH,EACRQ,EAAQG,KAAKR,GACbhB,EAAOqB,QAAUA,GAElBJ,EAAID,EAAES,QACQ,MAAVd,EAAEe,KACLX,EAAI1B,EAAKsC,MAAMC,WAAWjB,EAAEe,MAC5BR,EAAqB,MAAhBP,EAAEkB,YAAuBlB,EAAEkB,WAAa,EAC7CZ,EAAEa,UAAaf,EAAE,GAAKG,EACtBD,EAAEc,YAAehB,EAAE,GAAKG,EACxBD,EAAEe,WAAcjB,EAAE,GAAKG,EACvBD,EAAEgB,cAAgBhB,EAAEiB,gBAAkBjB,EAAEkB,eAAiB,EAAIjB,WAExDC,KAAKR,EACC,aAANQ,GAA4B,eAANA,IACzBF,EAAEE,IAAMR,EAAEQ,QAIK,MAAdR,EAAEyB,UACLnB,EAAEa,UAAYb,EAAEc,YAAcd,EAAEe,WAAa,KAAQrB,EAAEyB,SAAW,GAClEnB,EAAEgB,cAAgBhB,EAAEiB,gBAAkBjB,EAAEkB,eAAiB,GAC/B,MAAhBxB,EAAE0B,aACZnB,EAAKP,EAAE0B,WAAa,EACpBpB,EAAEa,UAAYb,EAAEc,YAAcd,EAAEe,WAAkB,EAAJd,EAAa,IAAJA,EAAU,EACjED,EAAEgB,cAAgBhB,EAAEiB,gBAAkBjB,EAAEkB,eAAiB,EAAIG,KAAKC,IAAIrB,IAEvEI,EAAI,EACGA,KAEFN,EADJG,EAAIqB,EAAYlB,MACHL,EAAEE,KACdC,EAAKR,EAAO6B,IAAIzB,EAAGG,EAAGH,EAAEG,GAAIF,EAAEE,GAAI,EAAG,EAAG,EAAG,EAAG,EAAG,MAEhDC,EAAGsB,GAAK,qBAIX9B,EAAO+B,OAAOnB,KAAK,qBACdxB,EAAO4C,SACX7C,EAAOC,GASM,SAAf6C,EAAgBC,EAAGC,QACZD,aAAaE,OAAYD,aAAcC,cACrCD,MAKP1C,EAAGD,EAHA6C,EAAO,GACV3B,EAAI,EACJ4B,EAAI,MAEA7C,EAAI,EAAGA,EAAI,EAAGA,IAAK,KAClBD,EAAI,EAAGA,EAAI,EAAGA,IAClB8C,EAAW,IAAN9C,EAAW0C,EAAExB,EAAI,GAAK,EAC3B2B,EAAK3B,EAAIlB,GAAK0C,EAAExB,GAAOyB,EAAG3C,GAAK0C,EAAExB,EAAE,GAAKyB,EAAG3C,EAAI,GAAK0C,EAAExB,EAAE,GAAKyB,EAAG3C,EAAI,IAAM0C,EAAExB,EAAE,GAAKyB,EAAG3C,EAAI,IAAM8C,EAEjG5B,GAAK,SAEC2B,EA4CkB,SAA1BE,EAA2BnD,EAAQW,EAAGC,IAEpCwC,EADIA,GACiB7D,IAAe8D,oBAEnC1D,EAAM,qFAKP2D,EAAQC,EAAavC,EAAGwC,EAFrBnC,EAAUrB,EAAOqB,SAAW,GAC/BC,EAAID,EAAQE,QAEC,IAALD,MACJD,EAAQC,aAAc8B,EAAoB,CAC7CpC,EAAIK,EAAQC,aAITN,IACJA,EAAI,IAAIoC,EAAmBK,EAAUC,SACrCrC,EAAQG,KAAKR,GACbhB,EAAOqB,QAAUA,GAElBkC,EAAcvC,EAAEsC,OAChBA,EAASG,EAAUC,QACD,MAAd/C,EAAEgD,WACLL,EAtDU,SAAZM,UAAad,EAAGe,EAAOC,GAClBC,MAAMD,KACTA,EAAS,OAEN/C,EAAI1B,EAAKsC,MAAMC,WAAWiC,GAC7BG,EAAIjD,EAAE,GAAK,IACXkD,EAAIlD,EAAE,GAAK,IACXd,EAAIc,EAAE,GAAK,IACXmD,EAAM,EAAIJ,SACJjB,EAAa,CAACqB,EAAMJ,EAASE,EAAIG,EAAOL,EAASE,EAAII,EAAON,EAASE,EAAIK,EAAO,EAAG,EAAGP,EAASG,EAAIE,EAAOD,EAAMJ,EAASG,EAAIG,EAAON,EAASG,EAAII,EAAO,EAAG,EAAGP,EAAS7D,EAAIkE,EAAOL,EAAS7D,EAAImE,EAAOF,EAAMJ,EAAS7D,EAAIoE,EAAO,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GAAIvB,GA6CnPc,CAAUN,EAAQ3C,EAAEgD,SAAUW,OAAO3D,EAAE4D,kBAE/B,MAAd5D,EAAE6D,WACLlB,EAnCa,SAAfmB,aAAgB3B,EAAG4B,UACdX,MAAMW,GACF5B,EAGDD,EAAa,CADpB6B,GAAK,IACkB,EAAE,EAAE,EAAE,KAAO,EAAIA,GAAI,EAAEA,EAAE,EAAE,EAAE,KAAO,EAAIA,GAAI,EAAE,EAAEA,EAAE,EAAE,KAAO,EAAIA,GAAI,EAAE,EAAE,EAAE,EAAE,GAAI5B,GA8B5F2B,CAAanB,EAAQgB,OAAO3D,EAAE6D,YAE3B,MAAT7D,EAAEgE,MACLrB,EAhDQ,SAAVsB,QAAW9B,EAAG4B,MACTX,MAAMW,UACF5B,EAER4B,GAAKpC,KAAKuC,GAAK,QACX9D,EAAIuB,KAAKwC,IAAIJ,GAChB1D,EAAIsB,KAAKyC,IAAIL,UACP7B,EAAa,CAAEsB,EAASpD,GAAK,EAAIoD,GAAYnD,GAAMmD,EAAUC,EAASrD,GAAMqD,EAAYpD,GAAMoD,EAAUC,EAAStD,GAAMsD,EAAYrD,GAAK,EAAIqD,GAAS,EAAG,EAAIF,EAASpD,GAAMoD,EAAgB,KAAJnD,EAAaoD,EAASrD,GAAK,EAAIqD,GAAgB,IAAJpD,EAAYqD,EAAStD,GAAMsD,GAAiB,KAALrD,EAAa,EAAG,EAAImD,EAASpD,GAAMoD,EAAYnD,IAAO,EAAImD,GAAWC,EAASrD,GAAMqD,EAAYpD,EAAIoD,EAASC,EAAStD,GAAK,EAAIsD,GAAYrD,EAAIqD,EAAQ,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GAAIvB,GAyClc8B,CAAQtB,EAAQgB,OAAO3D,EAAEgE,OAEf,MAAhBhE,EAAEqE,aACL1B,EA1Ee,SAAjB2B,eAAkBnC,EAAG4B,MAChBX,MAAMW,UACF5B,MAEJoB,EAAM,EAAIQ,EACbV,EAAIE,EAAMC,EACVF,EAAIC,EAAME,EACVnE,EAAIiE,EAAMG,SACJxB,EAAa,CAACmB,EAAIU,EAAGT,EAAGhE,EAAG,EAAG,EAAG+D,EAAGC,EAAIS,EAAGzE,EAAG,EAAG,EAAG+D,EAAGC,EAAGhE,EAAIyE,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GAAI5B,GAkEpFmC,CAAe3B,EAAQgB,OAAO3D,EAAEqE,cAG1C1D,EAAIgC,EAAO/B,QACG,IAALD,GACJgC,EAAOhC,KAAOiC,EAAYjC,KAC7BkC,EAAK5C,EAAO6B,IAAIc,EAAajC,EAAGiC,EAAYjC,GAAIgC,EAAOhC,GAAI,EAAG,EAAG,EAAG,EAAG,EAAG,MAEzEkC,EAAGd,GAAK,2BAKX9B,EAAO+B,OAAOnB,KAAK,2BACdxB,EAAO4C,SACX7C,IAGDa,EAAOsE,QAAU3B,EAGN,SAAZ4B,EAAYC,GACX/F,EAAO+F,GAAQhG,IACXF,MACHO,EAAON,QAEJE,IAEHgG,EAAe,GAxMlB,IAAIhG,EAAMgG,EAAc5F,EAAMD,EAAWqB,EAAcuC,EACtDZ,EAAc,4GAA4G8C,MAAM,KA0EhI7B,EAAY,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,GACnDU,EAAQ,QACRC,EAAQ,OACRC,EAAQ,QA+HIkB,EAAc,CAC1BC,QAAS,SACTC,KAAM,QACNC,mBAAK1F,EAAQ2F,EAAOC,EAAOC,EAAOC,OAQ7B3E,EAAGC,EAAIM,EAAMqE,EAAaC,EAAKC,EAAQ3E,MACtCH,KARAkE,IACJF,IACK9F,GACJM,EAAM,iDAGHK,OAASA,EAEJ2F,KAETK,EAAML,EAAMxE,GACF,gBAANA,GAA6B,SAANA,GAAsB,eAANA,GAA4B,aAANA,GAA0B,eAANA,EAC/EO,IACJhB,EAAkBV,EAAQ2F,EAAMO,aAAeP,EAAOQ,MACtDzE,GAAO,QAGF,GAAU,eAANP,GAA4B,aAANA,GAA0B,QAANA,GAAqB,aAANA,GAA0B,mBAANA,EAClF4E,IACJ5C,EAAwBnD,EAAQ2F,EAAMS,mBAAqBT,EAAOQ,MAClEJ,GAAc,QAGT,GAAU,UAAN5E,EAAe,IACL,iBAAT6E,GAAuC,MAAlBA,EAAIK,OAAO,KAAeJ,EAASjG,EAAOiG,YACpE3E,EAAI,EAAGA,EAAI2E,EAAO1E,OAAQD,IAC1B2E,EAAO3E,GAAGgF,QAAUN,IACvBA,EAAMC,EAAO3E,GAAGiF,WAInBnF,EAAK+E,KAAK1D,IAAIzC,EAAQ,cAAeA,EAAOwG,aAAcR,EAAKH,EAAOC,EAASxD,KAAKmE,MAAO,EAAG,EAAG,MAEhGrF,EAAGsB,GAAKvB,QAGc,MAAbnB,EAAOmB,SACZsB,IAAIzC,EAAQmB,EAAG,MAAO6E,IAK9BU,uBAAOC,EAAOC,WACTxF,EAAKwF,EAAKC,IACPzF,GACNA,EAAG4C,EAAE2C,EAAOvF,EAAG0F,GACf1F,EAAKA,EAAG2F,MAELH,EAAK5G,OAAO4C,SACfgE,EAAK5G,OAAOgH,eAGdC,SAAU9B,EAGXI,iBAA+B,SAAA7F,GAC9BF,EAAYE,IAGbN,KAAcC,EAAKC,eAAeiG"} \ No newline at end of file diff --git a/node_modules/gsap/dist/Flip.js b/node_modules/gsap/dist/Flip.js new file mode 100644 index 0000000000000000000000000000000000000000..d3a25a6f9864cd6d71f3a74e783e2166c17dd0a4 --- /dev/null +++ b/node_modules/gsap/dist/Flip.js @@ -0,0 +1,1824 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + var _doc, + _win, + _docElement, + _body, + _divContainer, + _svgContainer, + _identityMatrix, + _gEl, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _hasOffsetBug, + _setDoc = function _setDoc(element) { + var doc = element.ownerDocument || element; + + if (!(_transformProp in element.style) && "msTransform" in element.style) { + _transformProp = "msTransform"; + _transformOriginProp = _transformProp + "Origin"; + } + + while (doc.parentNode && (doc = doc.parentNode)) {} + + _win = window; + _identityMatrix = new Matrix2D(); + + if (doc) { + _doc = doc; + _docElement = doc.documentElement; + _body = doc.body; + _gEl = _doc.createElementNS("http://www.w3.org/2000/svg", "g"); + _gEl.style.transform = "none"; + var d1 = doc.createElement("div"), + d2 = doc.createElement("div"), + root = doc && (doc.body || doc.firstElementChild); + + if (root && root.appendChild) { + root.appendChild(d1); + d1.appendChild(d2); + d1.setAttribute("style", "position:static;transform:translate3d(0,0,1px)"); + _hasOffsetBug = d2.offsetParent !== d1; + root.removeChild(d1); + } + } + + return doc; + }, + _forceNonZeroScale = function _forceNonZeroScale(e) { + var a, cache; + + while (e && e !== _body) { + cache = e._gsap; + cache && cache.uncache && cache.get(e, "x"); + + if (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) { + cache.scaleX = cache.scaleY = 1e-4; + cache.renderTransform(1, cache); + a ? a.push(cache) : a = [cache]; + } + + e = e.parentNode; + } + + return a; + }, + _svgTemps = [], + _divTemps = [], + _getDocScrollTop = function _getDocScrollTop() { + return _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0; + }, + _getDocScrollLeft = function _getDocScrollLeft() { + return _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0; + }, + _svgOwner = function _svgOwner(element) { + return element.ownerSVGElement || ((element.tagName + "").toLowerCase() === "svg" ? element : null); + }, + _isFixed = function _isFixed(element) { + if (_win.getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + return _isFixed(element); + } + }, + _createSibling = function _createSibling(element, i) { + if (element.parentNode && (_doc || _setDoc(element))) { + var svg = _svgOwner(element), + ns = svg ? svg.getAttribute("xmlns") || "http://www.w3.org/2000/svg" : "http://www.w3.org/1999/xhtml", + type = svg ? i ? "rect" : "g" : "div", + x = i !== 2 ? 0 : 100, + y = i === 3 ? 100 : 0, + css = "position:absolute;display:block;pointer-events:none;margin:0;padding:0;", + e = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, "http"), type) : _doc.createElement(type); + + if (i) { + if (!svg) { + if (!_divContainer) { + _divContainer = _createSibling(element); + _divContainer.style.cssText = css; + } + + e.style.cssText = css + "width:0.1px;height:0.1px;top:" + y + "px;left:" + x + "px"; + + _divContainer.appendChild(e); + } else { + _svgContainer || (_svgContainer = _createSibling(element)); + e.setAttribute("width", 0.01); + e.setAttribute("height", 0.01); + e.setAttribute("transform", "translate(" + x + "," + y + ")"); + + _svgContainer.appendChild(e); + } + } + + return e; + } + + throw "Need document and parent."; + }, + _consolidate = function _consolidate(m) { + var c = new Matrix2D(), + i = 0; + + for (; i < m.numberOfItems; i++) { + c.multiply(m.getItem(i).matrix); + } + + return c; + }, + _getCTM = function _getCTM(svg) { + var m = svg.getCTM(), + transform; + + if (!m) { + transform = svg.style[_transformProp]; + svg.style[_transformProp] = "none"; + svg.appendChild(_gEl); + m = _gEl.getCTM(); + svg.removeChild(_gEl); + transform ? svg.style[_transformProp] = transform : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, "-$1").toLowerCase()); + } + + return m || _identityMatrix.clone(); + }, + _placeSiblings = function _placeSiblings(element, adjustGOffset) { + var svg = _svgOwner(element), + isRootSVG = element === svg, + siblings = svg ? _svgTemps : _divTemps, + parent = element.parentNode, + container, + m, + b, + x, + y, + cs; + + if (element === _win) { + return element; + } + + siblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3)); + container = svg ? _svgContainer : _divContainer; + + if (svg) { + if (isRootSVG) { + b = _getCTM(element); + x = -b.e / b.a; + y = -b.f / b.d; + m = _identityMatrix; + } else if (element.getBBox) { + b = element.getBBox(); + m = element.transform ? element.transform.baseVal : {}; + m = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; + x = m.a * b.x + m.c * b.y; + y = m.b * b.x + m.d * b.y; + } else { + m = new Matrix2D(); + x = y = 0; + } + + if (adjustGOffset && element.tagName.toLowerCase() === "g") { + x = y = 0; + } + + (isRootSVG ? svg : parent).appendChild(container); + container.setAttribute("transform", "matrix(" + m.a + "," + m.b + "," + m.c + "," + m.d + "," + (m.e + x) + "," + (m.f + y) + ")"); + } else { + x = y = 0; + + if (_hasOffsetBug) { + m = element.offsetParent; + b = element; + + while (b && (b = b.parentNode) && b !== m && b.parentNode) { + if ((_win.getComputedStyle(b)[_transformProp] + "").length > 4) { + x = b.offsetLeft; + y = b.offsetTop; + b = 0; + } + } + } + + cs = _win.getComputedStyle(element); + + if (cs.position !== "absolute" && cs.position !== "fixed") { + m = element.offsetParent; + + while (parent && parent !== m) { + x += parent.scrollLeft || 0; + y += parent.scrollTop || 0; + parent = parent.parentNode; + } + } + + b = container.style; + b.top = element.offsetTop - y + "px"; + b.left = element.offsetLeft - x + "px"; + b[_transformProp] = cs[_transformProp]; + b[_transformOriginProp] = cs[_transformOriginProp]; + b.position = cs.position === "fixed" ? "fixed" : "absolute"; + element.parentNode.appendChild(container); + } + + return container; + }, + _setMatrix = function _setMatrix(m, a, b, c, d, e, f) { + m.a = a; + m.b = b; + m.c = c; + m.d = d; + m.e = e; + m.f = f; + return m; + }; + + var Matrix2D = function () { + function Matrix2D(a, b, c, d, e, f) { + if (a === void 0) { + a = 1; + } + + if (b === void 0) { + b = 0; + } + + if (c === void 0) { + c = 0; + } + + if (d === void 0) { + d = 1; + } + + if (e === void 0) { + e = 0; + } + + if (f === void 0) { + f = 0; + } + + _setMatrix(this, a, b, c, d, e, f); + } + + var _proto = Matrix2D.prototype; + + _proto.inverse = function inverse() { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + determinant = a * d - b * c || 1e-10; + return _setMatrix(this, d / determinant, -b / determinant, -c / determinant, a / determinant, (c * f - d * e) / determinant, -(a * f - b * e) / determinant); + }; + + _proto.multiply = function multiply(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + a2 = matrix.a, + b2 = matrix.c, + c2 = matrix.b, + d2 = matrix.d, + e2 = matrix.e, + f2 = matrix.f; + return _setMatrix(this, a2 * a + c2 * c, a2 * b + c2 * d, b2 * a + d2 * c, b2 * b + d2 * d, e + e2 * a + f2 * c, f + e2 * b + f2 * d); + }; + + _proto.clone = function clone() { + return new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f); + }; + + _proto.equals = function equals(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + return a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f; + }; + + _proto.apply = function apply(point, decoratee) { + if (decoratee === void 0) { + decoratee = {}; + } + + var x = point.x, + y = point.y, + a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + decoratee.x = x * a + y * c + e || 0; + decoratee.y = x * b + y * d + f || 0; + return decoratee; + }; + + return Matrix2D; + }(); + function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { + if (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) { + return new Matrix2D(); + } + + var zeroScales = _forceNonZeroScale(element), + svg = _svgOwner(element), + temps = svg ? _svgTemps : _divTemps, + container = _placeSiblings(element, adjustGOffset), + b1 = temps[0].getBoundingClientRect(), + b2 = temps[1].getBoundingClientRect(), + b3 = temps[2].getBoundingClientRect(), + parent = container.parentNode, + isFixed = !includeScrollInFixed && _isFixed(element), + m = new Matrix2D((b2.left - b1.left) / 100, (b2.top - b1.top) / 100, (b3.left - b1.left) / 100, (b3.top - b1.top) / 100, b1.left + (isFixed ? 0 : _getDocScrollLeft()), b1.top + (isFixed ? 0 : _getDocScrollTop())); + + parent.removeChild(container); + + if (zeroScales) { + b1 = zeroScales.length; + + while (b1--) { + b2 = zeroScales[b1]; + b2.scaleX = b2.scaleY = 0; + b2.renderTransform(1, b2); + } + } + + return inverse ? m.inverse() : m; + } + + /*! + * Flip 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var _id = 1, + _toArray, + gsap, + _batch, + _batchAction, + _body$1, + _closestTenth, + _getStyleSaver, + _forEachBatch = function _forEachBatch(batch, name) { + return batch.actions.forEach(function (a) { + return a.vars[name] && a.vars[name](a); + }); + }, + _batchLookup = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _emptyObj = {}, + _dashedNameLookup = {}, + _memoizedRemoveProps = {}, + _listToArray = function _listToArray(list) { + return typeof list === "string" ? list.split(" ").join("").split(",") : list; + }, + _callbacks = _listToArray("onStart,onUpdate,onComplete,onReverseComplete,onInterrupt"), + _removeProps = _listToArray("transform,transformOrigin,width,height,position,top,left,opacity,zIndex,maxWidth,maxHeight,minWidth,minHeight"), + _getEl = function _getEl(target) { + return _toArray(target)[0] || console.warn("Element not found:", target); + }, + _round = function _round(value) { + return Math.round(value * 10000) / 10000 || 0; + }, + _toggleClass = function _toggleClass(targets, className, action) { + return targets.forEach(function (el) { + return el.classList[action](className); + }); + }, + _reserved = { + zIndex: 1, + kill: 1, + simple: 1, + spin: 1, + clearProps: 1, + targets: 1, + toggleClass: 1, + onComplete: 1, + onUpdate: 1, + onInterrupt: 1, + onStart: 1, + delay: 1, + repeat: 1, + repeatDelay: 1, + yoyo: 1, + scale: 1, + fade: 1, + absolute: 1, + props: 1, + onEnter: 1, + onLeave: 1, + custom: 1, + paused: 1, + nested: 1, + prune: 1, + absoluteOnLeave: 1 + }, + _fitReserved = { + zIndex: 1, + simple: 1, + clearProps: 1, + scale: 1, + absolute: 1, + fitChild: 1, + getVars: 1, + props: 1 + }, + _camelToDashed = function _camelToDashed(p) { + return p.replace(/([A-Z])/g, "-$1").toLowerCase(); + }, + _copy = function _copy(obj, exclude) { + var result = {}, + p; + + for (p in obj) { + exclude[p] || (result[p] = obj[p]); + } + + return result; + }, + _memoizedProps = {}, + _memoizeProps = function _memoizeProps(props) { + var p = _memoizedProps[props] = _listToArray(props); + + _memoizedRemoveProps[props] = p.concat(_removeProps); + return p; + }, + _getInverseGlobalMatrix = function _getInverseGlobalMatrix(el) { + var cache = el._gsap || gsap.core.getCache(el); + + if (cache.gmCache === gsap.ticker.frame) { + return cache.gMatrix; + } + + cache.gmCache = gsap.ticker.frame; + return cache.gMatrix = getGlobalMatrix(el, true, false, true); + }, + _getDOMDepth = function _getDOMDepth(el, invert, level) { + if (level === void 0) { + level = 0; + } + + var parent = el.parentNode, + inc = 1000 * Math.pow(10, level) * (invert ? -1 : 1), + l = invert ? -inc * 900 : 0; + + while (el) { + l += inc; + el = el.previousSibling; + } + + return parent ? l + _getDOMDepth(parent, invert, level + 1) : l; + }, + _orderByDOMDepth = function _orderByDOMDepth(comps, invert, isElStates) { + comps.forEach(function (comp) { + return comp.d = _getDOMDepth(isElStates ? comp.element : comp.t, invert); + }); + comps.sort(function (c1, c2) { + return c1.d - c2.d; + }); + return comps; + }, + _recordInlineStyles = function _recordInlineStyles(elState, props) { + var style = elState.element.style, + a = elState.css = elState.css || [], + i = props.length, + p, + v; + + while (i--) { + p = props[i]; + v = style[p] || style.getPropertyValue(p); + a.push(v ? p : _dashedNameLookup[p] || (_dashedNameLookup[p] = _camelToDashed(p)), v); + } + + return style; + }, + _applyInlineStyles = function _applyInlineStyles(state) { + var css = state.css, + style = state.element.style, + i = 0; + state.cache.uncache = 1; + + for (; i < css.length; i += 2) { + css[i + 1] ? style[css[i]] = css[i + 1] : style.removeProperty(css[i]); + } + + if (!css[css.indexOf("transform") + 1] && style.translate) { + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } + }, + _setFinalStates = function _setFinalStates(comps, onlyTransforms) { + comps.forEach(function (c) { + return c.a.cache.uncache = 1; + }); + onlyTransforms || comps.finalStates.forEach(_applyInlineStyles); + }, + _absoluteProps = "paddingTop,paddingRight,paddingBottom,paddingLeft,gridArea,transition".split(","), + _makeAbsolute = function _makeAbsolute(elState, fallbackNode, ignoreBatch) { + var element = elState.element, + width = elState.width, + height = elState.height, + uncache = elState.uncache, + getProp = elState.getProp, + style = element.style, + i = 4, + result, + displayIsNone, + cs; + typeof fallbackNode !== "object" && (fallbackNode = elState); + + if (_batch && ignoreBatch !== 1) { + _batch._abs.push({ + t: element, + b: elState, + a: elState, + sd: 0 + }); + + _batch._final.push(function () { + return (elState.cache.uncache = 1) && _applyInlineStyles(elState); + }); + + return element; + } + + displayIsNone = getProp("display") === "none"; + + if (!elState.isVisible || displayIsNone) { + displayIsNone && (_recordInlineStyles(elState, ["display"]).display = fallbackNode.display); + elState.matrix = fallbackNode.matrix; + elState.width = width = elState.width || fallbackNode.width; + elState.height = height = elState.height || fallbackNode.height; + } + + _recordInlineStyles(elState, _absoluteProps); + + cs = window.getComputedStyle(element); + + while (i--) { + style[_absoluteProps[i]] = cs[_absoluteProps[i]]; + } + + style.gridArea = "1 / 1 / 1 / 1"; + style.transition = "none"; + style.position = "absolute"; + style.width = width + "px"; + style.height = height + "px"; + style.top || (style.top = "0px"); + style.left || (style.left = "0px"); + + if (uncache) { + result = new ElementState(element); + } else { + result = _copy(elState, _emptyObj); + result.position = "absolute"; + + if (elState.simple) { + var bounds = element.getBoundingClientRect(); + result.matrix = new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()); + } else { + result.matrix = getGlobalMatrix(element, false, false, true); + } + } + + result = _fit(result, elState, true); + elState.x = _closestTenth(result.x, 0.01); + elState.y = _closestTenth(result.y, 0.01); + return element; + }, + _filterComps = function _filterComps(comps, targets) { + if (targets !== true) { + targets = _toArray(targets); + comps = comps.filter(function (c) { + if (targets.indexOf((c.sd < 0 ? c.b : c.a).element) !== -1) { + return true; + } else { + c.t._gsap.renderTransform(1); + + if (c.b.isVisible) { + c.t.style.width = c.b.width + "px"; + c.t.style.height = c.b.height + "px"; + } + } + }); + } + + return comps; + }, + _makeCompsAbsolute = function _makeCompsAbsolute(comps) { + return _orderByDOMDepth(comps, true).forEach(function (c) { + return (c.a.isVisible || c.b.isVisible) && _makeAbsolute(c.sd < 0 ? c.b : c.a, c.b, 1); + }); + }, + _findElStateInState = function _findElStateInState(state, other) { + return other && state.idLookup[_parseElementState(other).id] || state.elementStates[0]; + }, + _parseElementState = function _parseElementState(elOrNode, props, simple, other) { + return elOrNode instanceof ElementState ? elOrNode : elOrNode instanceof FlipState ? _findElStateInState(elOrNode, other) : new ElementState(typeof elOrNode === "string" ? _getEl(elOrNode) || console.warn(elOrNode + " not found") : elOrNode, props, simple); + }, + _recordProps = function _recordProps(elState, props) { + var getProp = gsap.getProperty(elState.element, null, "native"), + obj = elState.props = {}, + i = props.length; + + while (i--) { + obj[props[i]] = (getProp(props[i]) + "").trim(); + } + + obj.zIndex && (obj.zIndex = parseFloat(obj.zIndex) || 0); + return elState; + }, + _applyProps = function _applyProps(element, props) { + var style = element.style || element, + p; + + for (p in props) { + style[p] = props[p]; + } + }, + _getID = function _getID(el) { + var id = el.getAttribute("data-flip-id"); + id || el.setAttribute("data-flip-id", id = "auto-" + _id++); + return id; + }, + _elementsFromElementStates = function _elementsFromElementStates(elStates) { + return elStates.map(function (elState) { + return elState.element; + }); + }, + _handleCallback = function _handleCallback(callback, elStates, tl) { + return callback && elStates.length && tl.add(callback(_elementsFromElementStates(elStates), tl, new FlipState(elStates, 0, true)), 0); + }, + _fit = function _fit(fromState, toState, scale, applyProps, fitChild, vars) { + var element = fromState.element, + cache = fromState.cache, + parent = fromState.parent, + x = fromState.x, + y = fromState.y, + width = toState.width, + height = toState.height, + scaleX = toState.scaleX, + scaleY = toState.scaleY, + rotation = toState.rotation, + bounds = toState.bounds, + styles = vars && _getStyleSaver && _getStyleSaver(element, "transform,width,height"), + dimensionState = fromState, + _toState$matrix = toState.matrix, + e = _toState$matrix.e, + f = _toState$matrix.f, + deep = fromState.bounds.width !== bounds.width || fromState.bounds.height !== bounds.height || fromState.scaleX !== scaleX || fromState.scaleY !== scaleY || fromState.rotation !== rotation, + simple = !deep && fromState.simple && toState.simple && !fitChild, + skewX, + fromPoint, + toPoint, + getProp, + parentMatrix, + matrix, + bbox; + + if (simple || !parent) { + scaleX = scaleY = 1; + rotation = skewX = 0; + } else { + parentMatrix = _getInverseGlobalMatrix(parent); + matrix = parentMatrix.clone().multiply(toState.ctm ? toState.matrix.clone().multiply(toState.ctm) : toState.matrix); + rotation = _round(Math.atan2(matrix.b, matrix.a) * _RAD2DEG); + skewX = _round(Math.atan2(matrix.c, matrix.d) * _RAD2DEG + rotation) % 360; + scaleX = Math.sqrt(Math.pow(matrix.a, 2) + Math.pow(matrix.b, 2)); + scaleY = Math.sqrt(Math.pow(matrix.c, 2) + Math.pow(matrix.d, 2)) * Math.cos(skewX * _DEG2RAD); + + if (fitChild) { + fitChild = _toArray(fitChild)[0]; + getProp = gsap.getProperty(fitChild); + bbox = fitChild.getBBox && typeof fitChild.getBBox === "function" && fitChild.getBBox(); + dimensionState = { + scaleX: getProp("scaleX"), + scaleY: getProp("scaleY"), + width: bbox ? bbox.width : Math.ceil(parseFloat(getProp("width", "px"))), + height: bbox ? bbox.height : parseFloat(getProp("height", "px")) + }; + } + + cache.rotation = rotation + "deg"; + cache.skewX = skewX + "deg"; + } + + if (scale) { + scaleX *= width === dimensionState.width || !dimensionState.width ? 1 : width / dimensionState.width; + scaleY *= height === dimensionState.height || !dimensionState.height ? 1 : height / dimensionState.height; + cache.scaleX = scaleX; + cache.scaleY = scaleY; + } else { + width = _closestTenth(width * scaleX / dimensionState.scaleX, 0); + height = _closestTenth(height * scaleY / dimensionState.scaleY, 0); + element.style.width = width + "px"; + element.style.height = height + "px"; + } + + applyProps && _applyProps(element, toState.props); + + if (simple || !parent) { + x += e - fromState.matrix.e; + y += f - fromState.matrix.f; + } else if (deep || parent !== toState.parent) { + cache.renderTransform(1, cache); + matrix = getGlobalMatrix(fitChild || element, false, false, true); + fromPoint = parentMatrix.apply({ + x: matrix.e, + y: matrix.f + }); + toPoint = parentMatrix.apply({ + x: e, + y: f + }); + x += toPoint.x - fromPoint.x; + y += toPoint.y - fromPoint.y; + } else { + parentMatrix.e = parentMatrix.f = 0; + toPoint = parentMatrix.apply({ + x: e - fromState.matrix.e, + y: f - fromState.matrix.f + }); + x += toPoint.x; + y += toPoint.y; + } + + x = _closestTenth(x, 0.02); + y = _closestTenth(y, 0.02); + + if (vars && !(vars instanceof ElementState)) { + styles && styles.revert(); + } else { + cache.x = x + "px"; + cache.y = y + "px"; + cache.renderTransform(1, cache); + } + + if (vars) { + vars.x = x; + vars.y = y; + vars.rotation = rotation; + vars.skewX = skewX; + + if (scale) { + vars.scaleX = scaleX; + vars.scaleY = scaleY; + } else { + vars.width = width; + vars.height = height; + } + } + + return vars || cache; + }, + _parseState = function _parseState(targetsOrState, vars) { + return targetsOrState instanceof FlipState ? targetsOrState : new FlipState(targetsOrState, vars); + }, + _getChangingElState = function _getChangingElState(toState, fromState, id) { + var to1 = toState.idLookup[id], + to2 = toState.alt[id]; + return to2.isVisible && (!(fromState.getElementState(to2.element) || to2).isVisible || !to1.isVisible) ? to2 : to1; + }, + _bodyMetrics = [], + _bodyProps = "width,height,overflowX,overflowY".split(","), + _bodyLocked, + _lockBodyScroll = function _lockBodyScroll(lock) { + if (lock !== _bodyLocked) { + var s = _body$1.style, + w = _body$1.clientWidth === window.outerWidth, + h = _body$1.clientHeight === window.outerHeight, + i = 4; + + if (lock && (w || h)) { + while (i--) { + _bodyMetrics[i] = s[_bodyProps[i]]; + } + + if (w) { + s.width = _body$1.clientWidth + "px"; + s.overflowY = "hidden"; + } + + if (h) { + s.height = _body$1.clientHeight + "px"; + s.overflowX = "hidden"; + } + + _bodyLocked = lock; + } else if (_bodyLocked) { + while (i--) { + _bodyMetrics[i] ? s[_bodyProps[i]] = _bodyMetrics[i] : s.removeProperty(_camelToDashed(_bodyProps[i])); + } + + _bodyLocked = lock; + } + } + }, + _fromTo = function _fromTo(fromState, toState, vars, relative) { + fromState instanceof FlipState && toState instanceof FlipState || console.warn("Not a valid state object."); + vars = vars || {}; + + var _vars = vars, + clearProps = _vars.clearProps, + onEnter = _vars.onEnter, + onLeave = _vars.onLeave, + absolute = _vars.absolute, + absoluteOnLeave = _vars.absoluteOnLeave, + custom = _vars.custom, + delay = _vars.delay, + paused = _vars.paused, + repeat = _vars.repeat, + repeatDelay = _vars.repeatDelay, + yoyo = _vars.yoyo, + toggleClass = _vars.toggleClass, + nested = _vars.nested, + _zIndex = _vars.zIndex, + scale = _vars.scale, + fade = _vars.fade, + stagger = _vars.stagger, + spin = _vars.spin, + prune = _vars.prune, + props = ("props" in vars ? vars : fromState).props, + tweenVars = _copy(vars, _reserved), + animation = gsap.timeline({ + delay: delay, + paused: paused, + repeat: repeat, + repeatDelay: repeatDelay, + yoyo: yoyo, + data: "isFlip" + }), + remainingProps = tweenVars, + entering = [], + leaving = [], + comps = [], + swapOutTargets = [], + spinNum = spin === true ? 1 : spin || 0, + spinFunc = typeof spin === "function" ? spin : function () { + return spinNum; + }, + interrupted = fromState.interrupted || toState.interrupted, + addFunc = animation[relative !== 1 ? "to" : "from"], + v, + p, + endTime, + i, + el, + comp, + state, + targets, + finalStates, + fromNode, + toNode, + run, + a, + b; + + for (p in toState.idLookup) { + toNode = !toState.alt[p] ? toState.idLookup[p] : _getChangingElState(toState, fromState, p); + el = toNode.element; + fromNode = fromState.idLookup[p]; + fromState.alt[p] && el === fromNode.element && (fromState.alt[p].isVisible || !toNode.isVisible) && (fromNode = fromState.alt[p]); + + if (fromNode) { + comp = { + t: el, + b: fromNode, + a: toNode, + sd: fromNode.element === el ? 0 : toNode.isVisible ? 1 : -1 + }; + comps.push(comp); + + if (comp.sd) { + if (comp.sd < 0) { + comp.b = toNode; + comp.a = fromNode; + } + + interrupted && _recordInlineStyles(comp.b, props ? _memoizedRemoveProps[props] : _removeProps); + fade && comps.push(comp.swap = { + t: fromNode.element, + b: comp.b, + a: comp.a, + sd: -comp.sd, + swap: comp + }); + } + + el._flip = fromNode.element._flip = _batch ? _batch.timeline : animation; + } else if (toNode.isVisible) { + comps.push({ + t: el, + b: _copy(toNode, { + isVisible: 1 + }), + a: toNode, + sd: 0, + entering: 1 + }); + el._flip = _batch ? _batch.timeline : animation; + } + } + + props && (_memoizedProps[props] || _memoizeProps(props)).forEach(function (p) { + return tweenVars[p] = function (i) { + return comps[i].a.props[p]; + }; + }); + comps.finalStates = finalStates = []; + + run = function run() { + _orderByDOMDepth(comps); + + _lockBodyScroll(true); + + for (i = 0; i < comps.length; i++) { + comp = comps[i]; + a = comp.a; + b = comp.b; + + if (prune && !a.isDifferent(b) && !comp.entering) { + comps.splice(i--, 1); + } else { + el = comp.t; + nested && !(comp.sd < 0) && i && (a.matrix = getGlobalMatrix(el, false, false, true)); + + if (b.isVisible && a.isVisible) { + if (comp.sd < 0) { + state = new ElementState(el, props, fromState.simple); + + _fit(state, a, scale, 0, 0, state); + + state.matrix = getGlobalMatrix(el, false, false, true); + state.css = comp.b.css; + comp.a = a = state; + fade && (el.style.opacity = interrupted ? b.opacity : a.opacity); + stagger && swapOutTargets.push(el); + } else if (comp.sd > 0 && fade) { + el.style.opacity = interrupted ? a.opacity - b.opacity : "0"; + } + + _fit(a, b, scale, props); + } else if (b.isVisible !== a.isVisible) { + if (!b.isVisible) { + a.isVisible && entering.push(a); + comps.splice(i--, 1); + } else if (!a.isVisible) { + b.css = a.css; + leaving.push(b); + comps.splice(i--, 1); + absolute && nested && _fit(a, b, scale, props); + } + } + + if (!scale) { + el.style.maxWidth = Math.max(a.width, b.width) + "px"; + el.style.maxHeight = Math.max(a.height, b.height) + "px"; + el.style.minWidth = Math.min(a.width, b.width) + "px"; + el.style.minHeight = Math.min(a.height, b.height) + "px"; + } + + nested && toggleClass && el.classList.add(toggleClass); + } + + finalStates.push(a); + } + + var classTargets; + + if (toggleClass) { + classTargets = finalStates.map(function (s) { + return s.element; + }); + nested && classTargets.forEach(function (e) { + return e.classList.remove(toggleClass); + }); + } + + _lockBodyScroll(false); + + if (scale) { + tweenVars.scaleX = function (i) { + return comps[i].a.scaleX; + }; + + tweenVars.scaleY = function (i) { + return comps[i].a.scaleY; + }; + } else { + tweenVars.width = function (i) { + return comps[i].a.width + "px"; + }; + + tweenVars.height = function (i) { + return comps[i].a.height + "px"; + }; + + tweenVars.autoRound = vars.autoRound || false; + } + + tweenVars.x = function (i) { + return comps[i].a.x + "px"; + }; + + tweenVars.y = function (i) { + return comps[i].a.y + "px"; + }; + + tweenVars.rotation = function (i) { + return comps[i].a.rotation + (spin ? spinFunc(i, targets[i], targets) * 360 : 0); + }; + + tweenVars.skewX = function (i) { + return comps[i].a.skewX; + }; + + targets = comps.map(function (c) { + return c.t; + }); + + if (_zIndex || _zIndex === 0) { + tweenVars.modifiers = { + zIndex: function zIndex() { + return _zIndex; + } + }; + tweenVars.zIndex = _zIndex; + tweenVars.immediateRender = vars.immediateRender !== false; + } + + fade && (tweenVars.opacity = function (i) { + return comps[i].sd < 0 ? 0 : comps[i].sd > 0 ? comps[i].a.opacity : "+=0"; + }); + + if (swapOutTargets.length) { + stagger = gsap.utils.distribute(stagger); + var dummyArray = targets.slice(swapOutTargets.length); + + tweenVars.stagger = function (i, el) { + return stagger(~swapOutTargets.indexOf(el) ? targets.indexOf(comps[i].swap.t) : i, el, dummyArray); + }; + } + + _callbacks.forEach(function (name) { + return vars[name] && animation.eventCallback(name, vars[name], vars[name + "Params"]); + }); + + if (custom && targets.length) { + remainingProps = _copy(tweenVars, _reserved); + + if ("scale" in custom) { + custom.scaleX = custom.scaleY = custom.scale; + delete custom.scale; + } + + for (p in custom) { + v = _copy(custom[p], _fitReserved); + v[p] = tweenVars[p]; + !("duration" in v) && "duration" in tweenVars && (v.duration = tweenVars.duration); + v.stagger = tweenVars.stagger; + addFunc.call(animation, targets, v, 0); + delete remainingProps[p]; + } + } + + if (targets.length || leaving.length || entering.length) { + toggleClass && animation.add(function () { + return _toggleClass(classTargets, toggleClass, animation._zTime < 0 ? "remove" : "add"); + }, 0) && !paused && _toggleClass(classTargets, toggleClass, "add"); + targets.length && addFunc.call(animation, targets, remainingProps, 0); + } + + _handleCallback(onEnter, entering, animation); + + _handleCallback(onLeave, leaving, animation); + + var batchTl = _batch && _batch.timeline; + + if (batchTl) { + batchTl.add(animation, 0); + + _batch._final.push(function () { + return _setFinalStates(comps, !clearProps); + }); + } + + endTime = animation.duration(); + animation.call(function () { + var forward = animation.time() >= endTime; + forward && !batchTl && _setFinalStates(comps, !clearProps); + toggleClass && _toggleClass(classTargets, toggleClass, forward ? "remove" : "add"); + }); + }; + + absoluteOnLeave && (absolute = comps.filter(function (comp) { + return !comp.sd && !comp.a.isVisible && comp.b.isVisible; + }).map(function (comp) { + return comp.a.element; + })); + + if (_batch) { + var _batch$_abs; + + absolute && (_batch$_abs = _batch._abs).push.apply(_batch$_abs, _filterComps(comps, absolute)); + + _batch._run.push(run); + } else { + absolute && _makeCompsAbsolute(_filterComps(comps, absolute)); + run(); + } + + var anim = _batch ? _batch.timeline : animation; + + anim.revert = function () { + return _killFlip(anim, 1, 1); + }; + + return anim; + }, + _interrupt = function _interrupt(tl) { + tl.vars.onInterrupt && tl.vars.onInterrupt.apply(tl, tl.vars.onInterruptParams || []); + tl.getChildren(true, false, true).forEach(_interrupt); + }, + _killFlip = function _killFlip(tl, action, force) { + if (tl && tl.progress() < 1 && (!tl.paused() || force)) { + if (action) { + _interrupt(tl); + + action < 2 && tl.progress(1); + tl.kill(); + } + + return true; + } + }, + _createLookup = function _createLookup(state) { + var lookup = state.idLookup = {}, + alt = state.alt = {}, + elStates = state.elementStates, + i = elStates.length, + elState; + + while (i--) { + elState = elStates[i]; + lookup[elState.id] ? alt[elState.id] = elState : lookup[elState.id] = elState; + } + }; + + var FlipState = function () { + function FlipState(targets, vars, targetsAreElementStates) { + this.props = vars && vars.props; + this.simple = !!(vars && vars.simple); + + if (targetsAreElementStates) { + this.targets = _elementsFromElementStates(targets); + this.elementStates = targets; + + _createLookup(this); + } else { + this.targets = _toArray(targets); + var soft = vars && (vars.kill === false || vars.batch && !vars.kill); + _batch && !soft && _batch._kill.push(this); + this.update(soft || !!_batch); + } + } + + var _proto = FlipState.prototype; + + _proto.update = function update(soft) { + var _this = this; + + this.elementStates = this.targets.map(function (el) { + return new ElementState(el, _this.props, _this.simple); + }); + + _createLookup(this); + + this.interrupt(soft); + this.recordInlineStyles(); + return this; + }; + + _proto.clear = function clear() { + this.targets.length = this.elementStates.length = 0; + + _createLookup(this); + + return this; + }; + + _proto.fit = function fit(state, scale, nested) { + var elStatesInOrder = _orderByDOMDepth(this.elementStates.slice(0), false, true), + toElStates = (state || this).idLookup, + i = 0, + fromNode, + toNode; + + for (; i < elStatesInOrder.length; i++) { + fromNode = elStatesInOrder[i]; + nested && (fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true)); + toNode = toElStates[fromNode.id]; + toNode && _fit(fromNode, toNode, scale, true, 0, fromNode); + fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true); + } + + return this; + }; + + _proto.getProperty = function getProperty(element, property) { + var es = this.getElementState(element) || _emptyObj; + + return (property in es ? es : es.props || _emptyObj)[property]; + }; + + _proto.add = function add(state) { + var i = state.targets.length, + lookup = this.idLookup, + alt = this.alt, + index, + es, + es2; + + while (i--) { + es = state.elementStates[i]; + es2 = lookup[es.id]; + + if (es2 && (es.element === es2.element || alt[es.id] && alt[es.id].element === es.element)) { + index = this.elementStates.indexOf(es.element === es2.element ? es2 : alt[es.id]); + this.targets.splice(index, 1, state.targets[i]); + this.elementStates.splice(index, 1, es); + } else { + this.targets.push(state.targets[i]); + this.elementStates.push(es); + } + } + + state.interrupted && (this.interrupted = true); + state.simple || (this.simple = false); + + _createLookup(this); + + return this; + }; + + _proto.compare = function compare(state) { + var l1 = state.idLookup, + l2 = this.idLookup, + unchanged = [], + changed = [], + enter = [], + leave = [], + targets = [], + a1 = state.alt, + a2 = this.alt, + place = function place(s1, s2, el) { + return (s1.isVisible !== s2.isVisible ? s1.isVisible ? enter : leave : s1.isVisible ? changed : unchanged).push(el) && targets.push(el); + }, + placeIfDoesNotExist = function placeIfDoesNotExist(s1, s2, el) { + return targets.indexOf(el) < 0 && place(s1, s2, el); + }, + s1, + s2, + p, + el, + s1Alt, + s2Alt, + c1, + c2; + + for (p in l1) { + s1Alt = a1[p]; + s2Alt = a2[p]; + s1 = !s1Alt ? l1[p] : _getChangingElState(state, this, p); + el = s1.element; + s2 = l2[p]; + + if (s2Alt) { + c2 = s2.isVisible || !s2Alt.isVisible && el === s2.element ? s2 : s2Alt; + c1 = s1Alt && !s1.isVisible && !s1Alt.isVisible && c2.element === s1Alt.element ? s1Alt : s1; + + if (c1.isVisible && c2.isVisible && c1.element !== c2.element) { + (c1.isDifferent(c2) ? changed : unchanged).push(c1.element, c2.element); + targets.push(c1.element, c2.element); + } else { + place(c1, c2, c1.element); + } + + s1Alt && c1.element === s1Alt.element && (s1Alt = l1[p]); + placeIfDoesNotExist(c1.element !== s2.element && s1Alt ? s1Alt : c1, s2, s2.element); + placeIfDoesNotExist(s1Alt && s1Alt.element === s2Alt.element ? s1Alt : c1, s2Alt, s2Alt.element); + s1Alt && placeIfDoesNotExist(s1Alt, s2Alt.element === s1Alt.element ? s2Alt : s2, s1Alt.element); + } else { + !s2 ? enter.push(el) : !s2.isDifferent(s1) ? unchanged.push(el) : place(s1, s2, el); + s1Alt && placeIfDoesNotExist(s1Alt, s2, s1Alt.element); + } + } + + for (p in l2) { + if (!l1[p]) { + leave.push(l2[p].element); + a2[p] && leave.push(a2[p].element); + } + } + + return { + changed: changed, + unchanged: unchanged, + enter: enter, + leave: leave + }; + }; + + _proto.recordInlineStyles = function recordInlineStyles() { + var props = _memoizedRemoveProps[this.props] || _removeProps, + i = this.elementStates.length; + + while (i--) { + _recordInlineStyles(this.elementStates[i], props); + } + }; + + _proto.interrupt = function interrupt(soft) { + var _this2 = this; + + var timelines = []; + this.targets.forEach(function (t) { + var tl = t._flip, + foundInProgress = _killFlip(tl, soft ? 0 : 1); + + soft && foundInProgress && timelines.indexOf(tl) < 0 && tl.add(function () { + return _this2.updateVisibility(); + }); + foundInProgress && timelines.push(tl); + }); + !soft && timelines.length && this.updateVisibility(); + this.interrupted || (this.interrupted = !!timelines.length); + }; + + _proto.updateVisibility = function updateVisibility() { + this.elementStates.forEach(function (es) { + var b = es.element.getBoundingClientRect(); + es.isVisible = !!(b.width || b.height || b.top || b.left); + es.uncache = 1; + }); + }; + + _proto.getElementState = function getElementState(element) { + return this.elementStates[this.targets.indexOf(_getEl(element))]; + }; + + _proto.makeAbsolute = function makeAbsolute() { + return _orderByDOMDepth(this.elementStates.slice(0), true, true).map(_makeAbsolute); + }; + + return FlipState; + }(); + + var ElementState = function () { + function ElementState(element, props, simple) { + this.element = element; + this.update(props, simple); + } + + var _proto2 = ElementState.prototype; + + _proto2.isDifferent = function isDifferent(state) { + var b1 = this.bounds, + b2 = state.bounds; + return b1.top !== b2.top || b1.left !== b2.left || b1.width !== b2.width || b1.height !== b2.height || !this.matrix.equals(state.matrix) || this.opacity !== state.opacity || this.props && state.props && JSON.stringify(this.props) !== JSON.stringify(state.props); + }; + + _proto2.update = function update(props, simple) { + var self = this, + element = self.element, + getProp = gsap.getProperty(element), + cache = gsap.core.getCache(element), + bounds = element.getBoundingClientRect(), + bbox = element.getBBox && typeof element.getBBox === "function" && element.nodeName.toLowerCase() !== "svg" && element.getBBox(), + m = simple ? new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()) : getGlobalMatrix(element, false, false, true); + self.getProp = getProp; + self.element = element; + self.id = _getID(element); + self.matrix = m; + self.cache = cache; + self.bounds = bounds; + self.isVisible = !!(bounds.width || bounds.height || bounds.left || bounds.top); + self.display = getProp("display"); + self.position = getProp("position"); + self.parent = element.parentNode; + self.x = getProp("x"); + self.y = getProp("y"); + self.scaleX = cache.scaleX; + self.scaleY = cache.scaleY; + self.rotation = getProp("rotation"); + self.skewX = getProp("skewX"); + self.opacity = getProp("opacity"); + self.width = bbox ? bbox.width : _closestTenth(getProp("width", "px"), 0.04); + self.height = bbox ? bbox.height : _closestTenth(getProp("height", "px"), 0.04); + props && _recordProps(self, _memoizedProps[props] || _memoizeProps(props)); + self.ctm = element.getCTM && element.nodeName.toLowerCase() === "svg" && _getCTM(element).inverse(); + self.simple = simple || _round(m.a) === 1 && !_round(m.b) && !_round(m.c) && _round(m.d) === 1; + self.uncache = 0; + }; + + return ElementState; + }(); + + var FlipAction = function () { + function FlipAction(vars, batch) { + this.vars = vars; + this.batch = batch; + this.states = []; + this.timeline = batch.timeline; + } + + var _proto3 = FlipAction.prototype; + + _proto3.getStateById = function getStateById(id) { + var i = this.states.length; + + while (i--) { + if (this.states[i].idLookup[id]) { + return this.states[i]; + } + } + }; + + _proto3.kill = function kill() { + this.batch.remove(this); + }; + + return FlipAction; + }(); + + var FlipBatch = function () { + function FlipBatch(id) { + this.id = id; + this.actions = []; + this._kill = []; + this._final = []; + this._abs = []; + this._run = []; + this.data = {}; + this.state = new FlipState(); + this.timeline = gsap.timeline(); + } + + var _proto4 = FlipBatch.prototype; + + _proto4.add = function add(config) { + var result = this.actions.filter(function (action) { + return action.vars === config; + }); + + if (result.length) { + return result[0]; + } + + result = new FlipAction(typeof config === "function" ? { + animate: config + } : config, this); + this.actions.push(result); + return result; + }; + + _proto4.remove = function remove(action) { + var i = this.actions.indexOf(action); + i >= 0 && this.actions.splice(i, 1); + return this; + }; + + _proto4.getState = function getState(merge) { + var _this3 = this; + + var prevBatch = _batch, + prevAction = _batchAction; + _batch = this; + this.state.clear(); + this._kill.length = 0; + this.actions.forEach(function (action) { + if (action.vars.getState) { + action.states.length = 0; + _batchAction = action; + action.state = action.vars.getState(action); + } + + merge && action.states.forEach(function (s) { + return _this3.state.add(s); + }); + }); + _batchAction = prevAction; + _batch = prevBatch; + this.killConflicts(); + return this; + }; + + _proto4.animate = function animate() { + var _this4 = this; + + var prevBatch = _batch, + tl = this.timeline, + i = this.actions.length, + finalStates, + endTime; + _batch = this; + tl.clear(); + this._abs.length = this._final.length = this._run.length = 0; + this.actions.forEach(function (a) { + a.vars.animate && a.vars.animate(a); + var onEnter = a.vars.onEnter, + onLeave = a.vars.onLeave, + targets = a.targets, + s, + result; + + if (targets && targets.length && (onEnter || onLeave)) { + s = new FlipState(); + a.states.forEach(function (state) { + return s.add(state); + }); + result = s.compare(Flip.getState(targets)); + result.enter.length && onEnter && onEnter(result.enter); + result.leave.length && onLeave && onLeave(result.leave); + } + }); + + _makeCompsAbsolute(this._abs); + + this._run.forEach(function (f) { + return f(); + }); + + endTime = tl.duration(); + finalStates = this._final.slice(0); + tl.add(function () { + if (endTime <= tl.time()) { + finalStates.forEach(function (f) { + return f(); + }); + + _forEachBatch(_this4, "onComplete"); + } + }); + _batch = prevBatch; + + while (i--) { + this.actions[i].vars.once && this.actions[i].kill(); + } + + _forEachBatch(this, "onStart"); + + tl.restart(); + return this; + }; + + _proto4.loadState = function loadState(done) { + done || (done = function done() { + return 0; + }); + var queue = []; + this.actions.forEach(function (c) { + if (c.vars.loadState) { + var i, + f = function f(targets) { + targets && (c.targets = targets); + i = queue.indexOf(f); + + if (~i) { + queue.splice(i, 1); + queue.length || done(); + } + }; + + queue.push(f); + c.vars.loadState(f); + } + }); + queue.length || done(); + return this; + }; + + _proto4.setState = function setState() { + this.actions.forEach(function (c) { + return c.targets = c.vars.setState && c.vars.setState(c); + }); + return this; + }; + + _proto4.killConflicts = function killConflicts(soft) { + this.state.interrupt(soft); + + this._kill.forEach(function (state) { + return state.interrupt(soft); + }); + + return this; + }; + + _proto4.run = function run(skipGetState, merge) { + var _this5 = this; + + if (this !== _batch) { + skipGetState || this.getState(merge); + this.loadState(function () { + if (!_this5._killed) { + _this5.setState(); + + _this5.animate(); + } + }); + } + + return this; + }; + + _proto4.clear = function clear(stateOnly) { + this.state.clear(); + stateOnly || (this.actions.length = 0); + }; + + _proto4.getStateById = function getStateById(id) { + var i = this.actions.length, + s; + + while (i--) { + s = this.actions[i].getStateById(id); + + if (s) { + return s; + } + } + + return this.state.idLookup[id] && this.state; + }; + + _proto4.kill = function kill() { + this._killed = 1; + this.clear(); + delete _batchLookup[this.id]; + }; + + return FlipBatch; + }(); + + var Flip = function () { + function Flip() {} + + Flip.getState = function getState(targets, vars) { + var state = _parseState(targets, vars); + + _batchAction && _batchAction.states.push(state); + vars && vars.batch && Flip.batch(vars.batch).state.add(state); + return state; + }; + + Flip.from = function from(state, vars) { + vars = vars || {}; + "clearProps" in vars || (vars.clearProps = true); + return _fromTo(state, _parseState(vars.targets || state.targets, { + props: vars.props || state.props, + simple: vars.simple, + kill: !!vars.kill + }), vars, -1); + }; + + Flip.to = function to(state, vars) { + return _fromTo(state, _parseState(vars.targets || state.targets, { + props: vars.props || state.props, + simple: vars.simple, + kill: !!vars.kill + }), vars, 1); + }; + + Flip.fromTo = function fromTo(fromState, toState, vars) { + return _fromTo(fromState, toState, vars); + }; + + Flip.fit = function fit(fromEl, toEl, vars) { + var v = vars ? _copy(vars, _fitReserved) : {}, + _ref = vars || v, + absolute = _ref.absolute, + scale = _ref.scale, + getVars = _ref.getVars, + props = _ref.props, + runBackwards = _ref.runBackwards, + onComplete = _ref.onComplete, + simple = _ref.simple, + fitChild = vars && vars.fitChild && _getEl(vars.fitChild), + before = _parseElementState(toEl, props, simple, fromEl), + after = _parseElementState(fromEl, 0, simple, before), + inlineProps = props ? _memoizedRemoveProps[props] : _removeProps, + ctx = gsap.context(); + + props && _applyProps(v, before.props); + + _recordInlineStyles(after, inlineProps); + + if (runBackwards) { + "immediateRender" in v || (v.immediateRender = true); + + v.onComplete = function () { + _applyInlineStyles(after); + + onComplete && onComplete.apply(this, arguments); + }; + } + + absolute && _makeAbsolute(after, before); + v = _fit(after, before, scale || fitChild, props, fitChild, v.duration || getVars ? v : 0); + typeof vars === "object" && "zIndex" in vars && (v.zIndex = vars.zIndex); + ctx && !getVars && ctx.add(function () { + return function () { + return _applyInlineStyles(after); + }; + }); + return getVars ? v : v.duration ? gsap.to(after.element, v) : null; + }; + + Flip.makeAbsolute = function makeAbsolute(targetsOrStates, vars) { + return (targetsOrStates instanceof FlipState ? targetsOrStates : new FlipState(targetsOrStates, vars)).makeAbsolute(); + }; + + Flip.batch = function batch(id) { + id || (id = "default"); + return _batchLookup[id] || (_batchLookup[id] = new FlipBatch(id)); + }; + + Flip.killFlipsOf = function killFlipsOf(targets, complete) { + (targets instanceof FlipState ? targets.targets : _toArray(targets)).forEach(function (t) { + return t && _killFlip(t._flip, complete !== false ? 1 : 2); + }); + }; + + Flip.isFlipping = function isFlipping(target) { + var f = Flip.getByTarget(target); + return !!f && f.isActive(); + }; + + Flip.getByTarget = function getByTarget(target) { + return (_getEl(target) || _emptyObj)._flip; + }; + + Flip.getElementState = function getElementState(target, props) { + return new ElementState(_getEl(target), props); + }; + + Flip.convertCoordinates = function convertCoordinates(fromElement, toElement, point) { + var m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }; + + Flip.register = function register(core) { + _body$1 = typeof document !== "undefined" && document.body; + + if (_body$1) { + gsap = core; + + _setDoc(_body$1); + + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + var snap = gsap.utils.snap(0.1); + + _closestTenth = function _closestTenth(value, add) { + return snap(parseFloat(value) + add); + }; + } + }; + + return Flip; + }(); + Flip.version = "3.12.7"; + typeof window !== "undefined" && window.gsap && window.gsap.registerPlugin(Flip); + + exports.Flip = Flip; + exports.default = Flip; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/Flip.min.js b/node_modules/gsap/dist/Flip.min.js new file mode 100644 index 0000000000000000000000000000000000000000..5b81fb73b6cb526f251d7550b179742726f3f73f --- /dev/null +++ b/node_modules/gsap/dist/Flip.min.js @@ -0,0 +1,11 @@ +/*! + * Flip 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?e(exports):"function"==typeof define&&define.amd?define(["exports"],e):e((t=t||self).window=t.window||{})}(this,function(e){"use strict";function p(t){var e=t.ownerDocument||t;!(w in t.style)&&"msTransform"in t.style&&(k=(w="msTransform")+"Origin");for(;e.parentNode&&(e=e.parentNode););if(y=window,d=new M,e){a=(g=e).documentElement,b=e.body,(s=g.createElementNS("http://www.w3.org/2000/svg","g")).style.transform="none";var i=e.createElement("div"),n=e.createElement("div"),r=e&&(e.body||e.firstElementChild);r&&r.appendChild&&(r.appendChild(i),i.appendChild(n),i.setAttribute("style","position:static;transform:translate3d(0,0,1px)"),m=n.offsetParent!==i,r.removeChild(i))}return e}function t(){return y.pageYOffset||g.scrollTop||a.scrollTop||b.scrollTop||0}function u(){return y.pageXOffset||g.scrollLeft||a.scrollLeft||b.scrollLeft||0}function v(t){return t.ownerSVGElement||("svg"===(t.tagName+"").toLowerCase()?t:null)}function x(t,e){if(t.parentNode&&(g||p(t))){var i=v(t),n=i?i.getAttribute("xmlns")||"http://www.w3.org/2000/svg":"http://www.w3.org/1999/xhtml",r=i?e?"rect":"g":"div",a=2!==e?0:100,s=3===e?100:0,o="position:absolute;display:block;pointer-events:none;margin:0;padding:0;",l=g.createElementNS?g.createElementNS(n.replace(/^https/,"http"),r):g.createElement(r);return e&&(i?(f=f||x(t),l.setAttribute("width",.01),l.setAttribute("height",.01),l.setAttribute("transform","translate("+a+","+s+")"),f.appendChild(l)):(c||((c=x(t)).style.cssText=o),l.style.cssText=o+"width:0.1px;height:0.1px;top:"+s+"px;left:"+a+"px",c.appendChild(l))),l}throw"Need document and parent."}function z(t){var e,i=t.getCTM();return i||(e=t.style[w],t.style[w]="none",t.appendChild(s),i=s.getCTM(),t.removeChild(s),e?t.style[w]=e:t.style.removeProperty(w.replace(/([A-Z])/g,"-$1").toLowerCase())),i||d.clone()}function A(t,e){var i,n,r,a,s,o,l=v(t),u=t===l,p=l?C:E,h=t.parentNode;if(t===y)return t;if(p.length||p.push(x(t,1),x(t,2),x(t,3)),i=l?f:c,l)u?(a=-(r=z(t)).e/r.a,s=-r.f/r.d,n=d):t.getBBox?(r=t.getBBox(),a=(n=(n=t.transform?t.transform.baseVal:{}).numberOfItems?1<n.numberOfItems?function _consolidate(t){for(var e=new M,i=0;i<t.numberOfItems;i++)e.multiply(t.getItem(i).matrix);return e}(n):n.getItem(0).matrix:d).a*r.x+n.c*r.y,s=n.b*r.x+n.d*r.y):(n=new M,a=s=0),e&&"g"===t.tagName.toLowerCase()&&(a=s=0),(u?l:h).appendChild(i),i.setAttribute("transform","matrix("+n.a+","+n.b+","+n.c+","+n.d+","+(n.e+a)+","+(n.f+s)+")");else{if(a=s=0,m)for(n=t.offsetParent,r=t;(r=r&&r.parentNode)&&r!==n&&r.parentNode;)4<(y.getComputedStyle(r)[w]+"").length&&(a=r.offsetLeft,s=r.offsetTop,r=0);if("absolute"!==(o=y.getComputedStyle(t)).position&&"fixed"!==o.position)for(n=t.offsetParent;h&&h!==n;)a+=h.scrollLeft||0,s+=h.scrollTop||0,h=h.parentNode;(r=i.style).top=t.offsetTop-s+"px",r.left=t.offsetLeft-a+"px",r[w]=o[w],r[k]=o[k],r.position="fixed"===o.position?"fixed":"absolute",t.parentNode.appendChild(i)}return i}function B(t,e,i,n,r,a,s){return t.a=e,t.b=i,t.c=n,t.d=r,t.e=a,t.f=s,t}var g,y,a,b,c,f,d,s,m,i,w="transform",k=w+"Origin",C=[],E=[],M=((i=Matrix2D.prototype).inverse=function inverse(){var t=this.a,e=this.b,i=this.c,n=this.d,r=this.e,a=this.f,s=t*n-e*i||1e-10;return B(this,n/s,-e/s,-i/s,t/s,(i*a-n*r)/s,-(t*a-e*r)/s)},i.multiply=function multiply(t){var e=this.a,i=this.b,n=this.c,r=this.d,a=this.e,s=this.f,o=t.a,l=t.c,u=t.b,p=t.d,h=t.e,c=t.f;return B(this,o*e+u*n,o*i+u*r,l*e+p*n,l*i+p*r,a+h*e+c*n,s+h*i+c*r)},i.clone=function clone(){return new Matrix2D(this.a,this.b,this.c,this.d,this.e,this.f)},i.equals=function equals(t){var e=this.a,i=this.b,n=this.c,r=this.d,a=this.e,s=this.f;return e===t.a&&i===t.b&&n===t.c&&r===t.d&&a===t.e&&s===t.f},i.apply=function apply(t,e){void 0===e&&(e={});var i=t.x,n=t.y,r=this.a,a=this.b,s=this.c,o=this.d,l=this.e,u=this.f;return e.x=i*r+n*s+l||0,e.y=i*a+n*o+u||0,e},Matrix2D);function Matrix2D(t,e,i,n,r,a){void 0===t&&(t=1),void 0===e&&(e=0),void 0===i&&(i=0),void 0===n&&(n=1),void 0===r&&(r=0),void 0===a&&(a=0),B(this,t,e,i,n,r,a)}function getGlobalMatrix(e,i,n,r){if(!e||!e.parentNode||(g||p(e)).documentElement===e)return new M;var a=function _forceNonZeroScale(t){for(var e,i;t&&t!==b;)(i=t._gsap)&&i.uncache&&i.get(t,"x"),i&&!i.scaleX&&!i.scaleY&&i.renderTransform&&(i.scaleX=i.scaleY=1e-4,i.renderTransform(1,i),e?e.push(i):e=[i]),t=t.parentNode;return e}(e),s=v(e)?C:E,o=A(e,n),l=s[0].getBoundingClientRect(),h=s[1].getBoundingClientRect(),c=s[2].getBoundingClientRect(),f=o.parentNode,d=!r&&function _isFixed(t){return"fixed"===y.getComputedStyle(t).position||((t=t.parentNode)&&1===t.nodeType?_isFixed(t):void 0)}(e),m=new M((h.left-l.left)/100,(h.top-l.top)/100,(c.left-l.left)/100,(c.top-l.top)/100,l.left+(d?0:u()),l.top+(d?0:t()));if(f.removeChild(o),a)for(l=a.length;l--;)(h=a[l]).scaleX=h.scaleY=0,h.renderTransform(1,h);return i?m.inverse():m}function L(t,e){return t.actions.forEach(function(t){return t.vars[e]&&t.vars[e](t)})}function S(t){return"string"==typeof t?t.split(" ").join("").split(","):t}function V(t){return I(t)[0]||console.warn("Element not found:",t)}function W(t){return Math.round(1e4*t)/1e4||0}function X(t,e,i){return t.forEach(function(t){return t.classList[i](e)})}function $(t){return t.replace(/([A-Z])/g,"-$1").toLowerCase()}function _(t,e){var i,n={};for(i in t)e[i]||(n[i]=t[i]);return n}function ba(t){var e=st[t]=S(t);return et[t]=e.concat(nt),e}function ea(t,e,i){return t.forEach(function(t){return t.d=function _getDOMDepth(t,e,i){void 0===i&&(i=0);for(var n=t.parentNode,r=1e3*Math.pow(10,i)*(e?-1:1),a=e?900*-r:0;t;)a+=r,t=t.previousSibling;return n?a+_getDOMDepth(n,e,i+1):a}(i?t.element:t.t,e)}),t.sort(function(t,e){return t.d-e.d}),t}function fa(t,e){for(var i,n,r=t.element.style,a=t.css=t.css||[],s=e.length;s--;)n=r[i=e[s]]||r.getPropertyValue(i),a.push(n?i:Y[i]||(Y[i]=$(i)),n);return r}function ga(t){var e=t.css,i=t.element.style,n=0;for(t.cache.uncache=1;n<e.length;n+=2)e[n+1]?i[e[n]]=e[n+1]:i.removeProperty(e[n]);!e[e.indexOf("transform")+1]&&i.translate&&(i.removeProperty("translate"),i.removeProperty("scale"),i.removeProperty("rotate"))}function ha(t,e){t.forEach(function(t){return t.a.cache.uncache=1}),e||t.finalStates.forEach(ga)}function ja(e,i,n){var r,a,s,o=e.element,l=e.width,p=e.height,h=e.uncache,c=e.getProp,f=o.style,d=4;if("object"!=typeof i&&(i=e),tt&&1!==n)return tt._abs.push({t:o,b:e,a:e,sd:0}),tt._final.push(function(){return(e.cache.uncache=1)&&ga(e)}),o;for(a="none"===c("display"),e.isVisible&&!a||(a&&(fa(e,["display"]).display=i.display),e.matrix=i.matrix,e.width=l=e.width||i.width,e.height=p=e.height||i.height),fa(e,R),s=window.getComputedStyle(o);d--;)f[R[d]]=s[R[d]];if(f.gridArea="1 / 1 / 1 / 1",f.transition="none",f.position="absolute",f.width=l+"px",f.height=p+"px",f.top||(f.top="0px"),f.left||(f.left="0px"),h)r=new pt(o);else if((r=_(e,D)).position="absolute",e.simple){var m=o.getBoundingClientRect();r.matrix=new M(1,0,0,1,m.left+u(),m.top+t())}else r.matrix=getGlobalMatrix(o,!1,!1,!0);return r=ot(r,e,!0),e.x=P(r.x,.01),e.y=P(r.y,.01),o}function ka(t,e){return!0!==e&&(e=I(e),t=t.filter(function(t){if(-1!==e.indexOf((t.sd<0?t.b:t.a).element))return!0;t.t._gsap.renderTransform(1),t.b.isVisible&&(t.t.style.width=t.b.width+"px",t.t.style.height=t.b.height+"px")})),t}function la(t){return ea(t,!0).forEach(function(t){return(t.a.isVisible||t.b.isVisible)&&ja(t.sd<0?t.b:t.a,t.b,1)})}function pa(t,e){var i,n=t.style||t;for(i in e)n[i]=e[i]}function ra(t){return t.map(function(t){return t.element})}function sa(t,e,i){return t&&e.length&&i.add(t(ra(e),i,new ut(e,0,!0)),0)}function ua(t,e){return t instanceof ut?t:new ut(t,e)}function va(t,e,i){var n=t.idLookup[i],r=t.alt[i];return!r.isVisible||(e.getElementState(r.element)||r).isVisible&&n.isVisible?n:r}function za(t){if(t!==l){var e=o.style,i=o.clientWidth===window.outerWidth,n=o.clientHeight===window.outerHeight,r=4;if(t&&(i||n)){for(;r--;)j[r]=e[H[r]];i&&(e.width=o.clientWidth+"px",e.overflowY="hidden"),n&&(e.height=o.clientHeight+"px",e.overflowX="hidden"),l=t}else if(l){for(;r--;)j[r]?e[H[r]]=j[r]:e.removeProperty($(H[r]));l=t}}}function Aa(t,e,r,i){t instanceof ut&&e instanceof ut||console.warn("Not a valid state object.");var a,s,o,l,u,p,h,c,f,n,d,m,g,v,y,x=(r=r||{}).clearProps,b=r.onEnter,w=r.onLeave,S=r.absolute,k=r.absoluteOnLeave,C=r.custom,V=r.delay,E=r.paused,M=r.repeat,B=r.repeatDelay,F=r.yoyo,I=r.toggleClass,L=r.nested,P=r.zIndex,A=r.scale,T=r.fade,O=r.stagger,N=r.spin,D=r.prune,z=("props"in r?r:t).props,Y=_(r,rt),R=Q.timeline({delay:V,paused:E,repeat:M,repeatDelay:B,yoyo:F,data:"isFlip"}),W=Y,G=[],j=[],H=[],q=[],$=!0===N?1:N||0,Z="function"==typeof N?N:function(){return $},J=t.interrupted||e.interrupted,U=R[1!==i?"to":"from"];for(s in e.idLookup)d=e.alt[s]?va(e,t,s):e.idLookup[s],u=d.element,n=t.idLookup[s],!t.alt[s]||u!==n.element||!t.alt[s].isVisible&&d.isVisible||(n=t.alt[s]),n?(p={t:u,b:n,a:d,sd:n.element===u?0:d.isVisible?1:-1},H.push(p),p.sd&&(p.sd<0&&(p.b=d,p.a=n),J&&fa(p.b,z?et[z]:nt),T&&H.push(p.swap={t:n.element,b:p.b,a:p.a,sd:-p.sd,swap:p})),u._flip=n.element._flip=tt?tt.timeline:R):d.isVisible&&(H.push({t:u,b:_(d,{isVisible:1}),a:d,sd:0,entering:1}),u._flip=tt?tt.timeline:R);z&&(st[z]||ba(z)).forEach(function(e){return Y[e]=function(t){return H[t].a.props[e]}}),H.finalStates=f=[],m=function run(){for(ea(H),za(!0),l=0;l<H.length;l++)p=H[l],g=p.a,v=p.b,!D||g.isDifferent(v)||p.entering?(u=p.t,!L||p.sd<0||!l||(g.matrix=getGlobalMatrix(u,!1,!1,!0)),v.isVisible&&g.isVisible?(p.sd<0?(h=new pt(u,z,t.simple),ot(h,g,A,0,0,h),h.matrix=getGlobalMatrix(u,!1,!1,!0),h.css=p.b.css,p.a=g=h,T&&(u.style.opacity=J?v.opacity:g.opacity),O&&q.push(u)):0<p.sd&&T&&(u.style.opacity=J?g.opacity-v.opacity:"0"),ot(g,v,A,z)):v.isVisible!==g.isVisible&&(v.isVisible?g.isVisible||(v.css=g.css,j.push(v),H.splice(l--,1),S&&L&&ot(g,v,A,z)):(g.isVisible&&G.push(g),H.splice(l--,1))),A||(u.style.maxWidth=Math.max(g.width,v.width)+"px",u.style.maxHeight=Math.max(g.height,v.height)+"px",u.style.minWidth=Math.min(g.width,v.width)+"px",u.style.minHeight=Math.min(g.height,v.height)+"px"),L&&I&&u.classList.add(I)):H.splice(l--,1),f.push(g);var e;if(I&&(e=f.map(function(t){return t.element}),L&&e.forEach(function(t){return t.classList.remove(I)})),za(!1),A?(Y.scaleX=function(t){return H[t].a.scaleX},Y.scaleY=function(t){return H[t].a.scaleY}):(Y.width=function(t){return H[t].a.width+"px"},Y.height=function(t){return H[t].a.height+"px"},Y.autoRound=r.autoRound||!1),Y.x=function(t){return H[t].a.x+"px"},Y.y=function(t){return H[t].a.y+"px"},Y.rotation=function(t){return H[t].a.rotation+(N?360*Z(t,c[t],c):0)},Y.skewX=function(t){return H[t].a.skewX},c=H.map(function(t){return t.t}),!P&&0!==P||(Y.modifiers={zIndex:function zIndex(){return P}},Y.zIndex=P,Y.immediateRender=!1!==r.immediateRender),T&&(Y.opacity=function(t){return H[t].sd<0?0:0<H[t].sd?H[t].a.opacity:"+=0"}),q.length){O=Q.utils.distribute(O);var i=c.slice(q.length);Y.stagger=function(t,e){return O(~q.indexOf(e)?c.indexOf(H[t].swap.t):t,e,i)}}if(it.forEach(function(t){return r[t]&&R.eventCallback(t,r[t],r[t+"Params"])}),C&&c.length)for(s in W=_(Y,rt),"scale"in C&&(C.scaleX=C.scaleY=C.scale,delete C.scale),C)(a=_(C[s],at))[s]=Y[s],!("duration"in a)&&"duration"in Y&&(a.duration=Y.duration),a.stagger=Y.stagger,U.call(R,c,a,0),delete W[s];(c.length||j.length||G.length)&&(I&&R.add(function(){return X(e,I,R._zTime<0?"remove":"add")},0)&&!E&&X(e,I,"add"),c.length&&U.call(R,c,W,0)),sa(b,G,R),sa(w,j,R);var n=tt&&tt.timeline;n&&(n.add(R,0),tt._final.push(function(){return ha(H,!x)})),o=R.duration(),R.call(function(){var t=R.time()>=o;t&&!n&&ha(H,!x),I&&X(e,I,t?"remove":"add")})},k&&(S=H.filter(function(t){return!t.sd&&!t.a.isVisible&&t.b.isVisible}).map(function(t){return t.a.element})),tt?(S&&(y=tt._abs).push.apply(y,ka(H,S)),tt._run.push(m)):(S&&la(ka(H,S)),m());var K=tt?tt.timeline:R;return K.revert=function(){return lt(K,1,1)},K}function Da(t){for(var e,i=t.idLookup={},n=t.alt={},r=t.elementStates,a=r.length;a--;)i[(e=r[a]).id]?n[e.id]=e:i[e.id]=e}var I,Q,tt,r,o,P,T,l,n,h=1,F={},O=180/Math.PI,N=Math.PI/180,D={},Y={},et={},it=S("onStart,onUpdate,onComplete,onReverseComplete,onInterrupt"),nt=S("transform,transformOrigin,width,height,position,top,left,opacity,zIndex,maxWidth,maxHeight,minWidth,minHeight"),rt={zIndex:1,kill:1,simple:1,spin:1,clearProps:1,targets:1,toggleClass:1,onComplete:1,onUpdate:1,onInterrupt:1,onStart:1,delay:1,repeat:1,repeatDelay:1,yoyo:1,scale:1,fade:1,absolute:1,props:1,onEnter:1,onLeave:1,custom:1,paused:1,nested:1,prune:1,absoluteOnLeave:1},at={zIndex:1,simple:1,clearProps:1,scale:1,absolute:1,fitChild:1,getVars:1,props:1},st={},R="paddingTop,paddingRight,paddingBottom,paddingLeft,gridArea,transition".split(","),G=function _parseElementState(t,e,i,n){return t instanceof pt?t:t instanceof ut?function _findElStateInState(t,e){return e&&t.idLookup[G(e).id]||t.elementStates[0]}(t,n):new pt("string"==typeof t?V(t)||console.warn(t+" not found"):t,e,i)},ot=function _fit(t,e,i,n,r,a){var s,o,l,u,p,h,c,f=t.element,d=t.cache,m=t.parent,g=t.x,v=t.y,y=e.width,x=e.height,b=e.scaleX,w=e.scaleY,S=e.rotation,k=e.bounds,_=a&&T&&T(f,"transform,width,height"),C=t,V=e.matrix,E=V.e,M=V.f,B=t.bounds.width!==k.width||t.bounds.height!==k.height||t.scaleX!==b||t.scaleY!==w||t.rotation!==S,F=!B&&t.simple&&e.simple&&!r;return F||!m?(b=w=1,S=s=0):(h=(p=function _getInverseGlobalMatrix(t){var e=t._gsap||Q.core.getCache(t);return e.gmCache===Q.ticker.frame?e.gMatrix:(e.gmCache=Q.ticker.frame,e.gMatrix=getGlobalMatrix(t,!0,!1,!0))}(m)).clone().multiply(e.ctm?e.matrix.clone().multiply(e.ctm):e.matrix),S=W(Math.atan2(h.b,h.a)*O),s=W(Math.atan2(h.c,h.d)*O+S)%360,b=Math.sqrt(Math.pow(h.a,2)+Math.pow(h.b,2)),w=Math.sqrt(Math.pow(h.c,2)+Math.pow(h.d,2))*Math.cos(s*N),r&&(r=I(r)[0],u=Q.getProperty(r),c=r.getBBox&&"function"==typeof r.getBBox&&r.getBBox(),C={scaleX:u("scaleX"),scaleY:u("scaleY"),width:c?c.width:Math.ceil(parseFloat(u("width","px"))),height:c?c.height:parseFloat(u("height","px"))}),d.rotation=S+"deg",d.skewX=s+"deg"),i?(b*=y!==C.width&&C.width?y/C.width:1,w*=x!==C.height&&C.height?x/C.height:1,d.scaleX=b,d.scaleY=w):(y=P(y*b/C.scaleX,0),x=P(x*w/C.scaleY,0),f.style.width=y+"px",f.style.height=x+"px"),n&&pa(f,e.props),F||!m?(g+=E-t.matrix.e,v+=M-t.matrix.f):B||m!==e.parent?(d.renderTransform(1,d),h=getGlobalMatrix(r||f,!1,!1,!0),o=p.apply({x:h.e,y:h.f}),g+=(l=p.apply({x:E,y:M})).x-o.x,v+=l.y-o.y):(p.e=p.f=0,g+=(l=p.apply({x:E-t.matrix.e,y:M-t.matrix.f})).x,v+=l.y),g=P(g,.02),v=P(v,.02),!a||a instanceof pt?(d.x=g+"px",d.y=v+"px",d.renderTransform(1,d)):_&&_.revert(),a&&(a.x=g,a.y=v,a.rotation=S,a.skewX=s,i?(a.scaleX=b,a.scaleY=w):(a.width=y,a.height=x)),a||d},j=[],H="width,height,overflowX,overflowY".split(","),lt=function _killFlip(t,e,i){if(t&&t.progress()<1&&(!t.paused()||i))return e&&(function _interrupt(t){t.vars.onInterrupt&&t.vars.onInterrupt.apply(t,t.vars.onInterruptParams||[]),t.getChildren(!0,!1,!0).forEach(_interrupt)}(t),e<2&&t.progress(1),t.kill()),!0},ut=((n=FlipState.prototype).update=function update(t){var e=this;return this.elementStates=this.targets.map(function(t){return new pt(t,e.props,e.simple)}),Da(this),this.interrupt(t),this.recordInlineStyles(),this},n.clear=function clear(){return this.targets.length=this.elementStates.length=0,Da(this),this},n.fit=function fit(t,e,i){for(var n,r,a=ea(this.elementStates.slice(0),!1,!0),s=(t||this).idLookup,o=0;o<a.length;o++)n=a[o],i&&(n.matrix=getGlobalMatrix(n.element,!1,!1,!0)),(r=s[n.id])&&ot(n,r,e,!0,0,n),n.matrix=getGlobalMatrix(n.element,!1,!1,!0);return this},n.getProperty=function getProperty(t,e){var i=this.getElementState(t)||D;return(e in i?i:i.props||D)[e]},n.add=function add(t){for(var e,i,n,r=t.targets.length,a=this.idLookup,s=this.alt;r--;)(n=a[(i=t.elementStates[r]).id])&&(i.element===n.element||s[i.id]&&s[i.id].element===i.element)?(e=this.elementStates.indexOf(i.element===n.element?n:s[i.id]),this.targets.splice(e,1,t.targets[r]),this.elementStates.splice(e,1,i)):(this.targets.push(t.targets[r]),this.elementStates.push(i));return t.interrupted&&(this.interrupted=!0),t.simple||(this.simple=!1),Da(this),this},n.compare=function compare(t){function kh(t,e,i){return(t.isVisible!==e.isVisible?t.isVisible?f:d:t.isVisible?c:h).push(i)&&m.push(i)}function lh(t,e,i){return m.indexOf(i)<0&&kh(t,e,i)}var e,i,n,r,a,s,o,l,u=t.idLookup,p=this.idLookup,h=[],c=[],f=[],d=[],m=[],g=t.alt,v=this.alt;for(n in u)a=g[n],s=v[n],r=(e=a?va(t,this,n):u[n]).element,i=p[n],s?(l=i.isVisible||!s.isVisible&&r===i.element?i:s,(o=!a||e.isVisible||a.isVisible||l.element!==a.element?e:a).isVisible&&l.isVisible&&o.element!==l.element?((o.isDifferent(l)?c:h).push(o.element,l.element),m.push(o.element,l.element)):kh(o,l,o.element),a&&o.element===a.element&&(a=u[n]),lh(o.element!==i.element&&a?a:o,i,i.element),lh(a&&a.element===s.element?a:o,s,s.element),a&&lh(a,s.element===a.element?s:i,a.element)):(i?i.isDifferent(e)?kh(e,i,r):h.push(r):f.push(r),a&&lh(a,i,a.element));for(n in p)u[n]||(d.push(p[n].element),v[n]&&d.push(v[n].element));return{changed:c,unchanged:h,enter:f,leave:d}},n.recordInlineStyles=function recordInlineStyles(){for(var t=et[this.props]||nt,e=this.elementStates.length;e--;)fa(this.elementStates[e],t)},n.interrupt=function interrupt(n){var r=this,a=[];this.targets.forEach(function(t){var e=t._flip,i=lt(e,n?0:1);n&&i&&a.indexOf(e)<0&&e.add(function(){return r.updateVisibility()}),i&&a.push(e)}),!n&&a.length&&this.updateVisibility(),this.interrupted||(this.interrupted=!!a.length)},n.updateVisibility=function updateVisibility(){this.elementStates.forEach(function(t){var e=t.element.getBoundingClientRect();t.isVisible=!!(e.width||e.height||e.top||e.left),t.uncache=1})},n.getElementState=function getElementState(t){return this.elementStates[this.targets.indexOf(V(t))]},n.makeAbsolute=function makeAbsolute(){return ea(this.elementStates.slice(0),!0,!0).map(ja)},FlipState);function FlipState(t,e,i){if(this.props=e&&e.props,this.simple=!(!e||!e.simple),i)this.targets=ra(t),this.elementStates=t,Da(this);else{this.targets=I(t);var n=e&&(!1===e.kill||e.batch&&!e.kill);tt&&!n&&tt._kill.push(this),this.update(n||!!tt)}}var q,pt=((q=ElementState.prototype).isDifferent=function isDifferent(t){var e=this.bounds,i=t.bounds;return e.top!==i.top||e.left!==i.left||e.width!==i.width||e.height!==i.height||!this.matrix.equals(t.matrix)||this.opacity!==t.opacity||this.props&&t.props&&JSON.stringify(this.props)!==JSON.stringify(t.props)},q.update=function update(e,i){var n=this,r=n.element,a=Q.getProperty(r),s=Q.core.getCache(r),o=r.getBoundingClientRect(),l=r.getBBox&&"function"==typeof r.getBBox&&"svg"!==r.nodeName.toLowerCase()&&r.getBBox(),p=i?new M(1,0,0,1,o.left+u(),o.top+t()):getGlobalMatrix(r,!1,!1,!0);n.getProp=a,n.element=r,n.id=function _getID(t){var e=t.getAttribute("data-flip-id");return e||t.setAttribute("data-flip-id",e="auto-"+h++),e}(r),n.matrix=p,n.cache=s,n.bounds=o,n.isVisible=!!(o.width||o.height||o.left||o.top),n.display=a("display"),n.position=a("position"),n.parent=r.parentNode,n.x=a("x"),n.y=a("y"),n.scaleX=s.scaleX,n.scaleY=s.scaleY,n.rotation=a("rotation"),n.skewX=a("skewX"),n.opacity=a("opacity"),n.width=l?l.width:P(a("width","px"),.04),n.height=l?l.height:P(a("height","px"),.04),e&&function _recordProps(t,e){for(var i=Q.getProperty(t.element,null,"native"),n=t.props={},r=e.length;r--;)n[e[r]]=(i(e[r])+"").trim();n.zIndex&&(n.zIndex=parseFloat(n.zIndex)||0)}(n,st[e]||ba(e)),n.ctm=r.getCTM&&"svg"===r.nodeName.toLowerCase()&&z(r).inverse(),n.simple=i||1===W(p.a)&&!W(p.b)&&!W(p.c)&&1===W(p.d),n.uncache=0},ElementState);function ElementState(t,e,i){this.element=t,this.update(e,i)}var Z,J=((Z=FlipAction.prototype).getStateById=function getStateById(t){for(var e=this.states.length;e--;)if(this.states[e].idLookup[t])return this.states[e]},Z.kill=function kill(){this.batch.remove(this)},FlipAction);function FlipAction(t,e){this.vars=t,this.batch=e,this.states=[],this.timeline=e.timeline}var U,K=((U=FlipBatch.prototype).add=function add(e){var t=this.actions.filter(function(t){return t.vars===e});return t.length?t[0]:(t=new J("function"==typeof e?{animate:e}:e,this),this.actions.push(t),t)},U.remove=function remove(t){var e=this.actions.indexOf(t);return 0<=e&&this.actions.splice(e,1),this},U.getState=function getState(e){var i=this,t=tt,n=r;return(tt=this).state.clear(),this._kill.length=0,this.actions.forEach(function(t){t.vars.getState&&(t.states.length=0,(r=t).state=t.vars.getState(t)),e&&t.states.forEach(function(t){return i.state.add(t)})}),r=n,tt=t,this.killConflicts(),this},U.animate=function animate(){var t,e,i=this,n=tt,r=this.timeline,a=this.actions.length;for(tt=this,r.clear(),this._abs.length=this._final.length=this._run.length=0,this.actions.forEach(function(t){t.vars.animate&&t.vars.animate(t);var e,i,n=t.vars.onEnter,r=t.vars.onLeave,a=t.targets;a&&a.length&&(n||r)&&(e=new ut,t.states.forEach(function(t){return e.add(t)}),(i=e.compare(ht.getState(a))).enter.length&&n&&n(i.enter),i.leave.length&&r&&r(i.leave))}),la(this._abs),this._run.forEach(function(t){return t()}),e=r.duration(),t=this._final.slice(0),r.add(function(){e<=r.time()&&(t.forEach(function(t){return t()}),L(i,"onComplete"))}),tt=n;a--;)this.actions[a].vars.once&&this.actions[a].kill();return L(this,"onStart"),r.restart(),this},U.loadState=function loadState(n){n=n||function done(){return 0};var r=[];return this.actions.forEach(function(e){if(e.vars.loadState){var i,t=function f(t){t&&(e.targets=t),~(i=r.indexOf(f))&&(r.splice(i,1),r.length||n())};r.push(t),e.vars.loadState(t)}}),r.length||n(),this},U.setState=function setState(){return this.actions.forEach(function(t){return t.targets=t.vars.setState&&t.vars.setState(t)}),this},U.killConflicts=function killConflicts(e){return this.state.interrupt(e),this._kill.forEach(function(t){return t.interrupt(e)}),this},U.run=function run(t,e){var i=this;return this!==tt&&(t||this.getState(e),this.loadState(function(){i._killed||(i.setState(),i.animate())})),this},U.clear=function clear(t){this.state.clear(),t||(this.actions.length=0)},U.getStateById=function getStateById(t){for(var e,i=this.actions.length;i--;)if(e=this.actions[i].getStateById(t))return e;return this.state.idLookup[t]&&this.state},U.kill=function kill(){this._killed=1,this.clear(),delete F[this.id]},FlipBatch);function FlipBatch(t){this.id=t,this.actions=[],this._kill=[],this._final=[],this._abs=[],this._run=[],this.data={},this.state=new ut,this.timeline=Q.timeline()}var ht=(Flip.getState=function getState(t,e){var i=ua(t,e);return r&&r.states.push(i),e&&e.batch&&Flip.batch(e.batch).state.add(i),i},Flip.from=function from(t,e){return"clearProps"in(e=e||{})||(e.clearProps=!0),Aa(t,ua(e.targets||t.targets,{props:e.props||t.props,simple:e.simple,kill:!!e.kill}),e,-1)},Flip.to=function to(t,e){return Aa(t,ua(e.targets||t.targets,{props:e.props||t.props,simple:e.simple,kill:!!e.kill}),e,1)},Flip.fromTo=function fromTo(t,e,i){return Aa(t,e,i)},Flip.fit=function fit(t,e,i){var n=i?_(i,at):{},r=i||n,a=r.absolute,s=r.scale,o=r.getVars,l=r.props,u=r.runBackwards,p=r.onComplete,h=r.simple,c=i&&i.fitChild&&V(i.fitChild),f=G(e,l,h,t),d=G(t,0,h,f),m=l?et[l]:nt,g=Q.context();return l&&pa(n,f.props),fa(d,m),u&&("immediateRender"in n||(n.immediateRender=!0),n.onComplete=function(){ga(d),p&&p.apply(this,arguments)}),a&&ja(d,f),n=ot(d,f,s||c,l,c,n.duration||o?n:0),"object"==typeof i&&"zIndex"in i&&(n.zIndex=i.zIndex),g&&!o&&g.add(function(){return function(){return ga(d)}}),o?n:n.duration?Q.to(d.element,n):null},Flip.makeAbsolute=function makeAbsolute(t,e){return(t instanceof ut?t:new ut(t,e)).makeAbsolute()},Flip.batch=function batch(t){return F[t=t||"default"]||(F[t]=new K(t))},Flip.killFlipsOf=function killFlipsOf(t,e){(t instanceof ut?t.targets:I(t)).forEach(function(t){return t&<(t._flip,!1!==e?1:2)})},Flip.isFlipping=function isFlipping(t){var e=Flip.getByTarget(t);return!!e&&e.isActive()},Flip.getByTarget=function getByTarget(t){return(V(t)||D)._flip},Flip.getElementState=function getElementState(t,e){return new pt(V(t),e)},Flip.convertCoordinates=function convertCoordinates(t,e,i){var n=getGlobalMatrix(e,!0,!0).multiply(getGlobalMatrix(t));return i?n.apply(i):n},Flip.register=function register(t){if(o="undefined"!=typeof document&&document.body){Q=t,p(o),I=Q.utils.toArray,T=Q.core.getStyleSaver;var i=Q.utils.snap(.1);P=function _closestTenth(t,e){return i(parseFloat(t)+e)}}},Flip);function Flip(){}ht.version="3.12.7","undefined"!=typeof window&&window.gsap&&window.gsap.registerPlugin(ht),e.Flip=ht,e.default=ht;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/Flip.min.js.map b/node_modules/gsap/dist/Flip.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..ad76f3da6b523c67f20a7db2191a1bd279993cb9 --- /dev/null +++ b/node_modules/gsap/dist/Flip.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"Flip.min.js","sources":["../src/utils/matrix.js","../src/Flip.js"],"sourcesContent":["/*!\n * matrix 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _doc, _win, _docElement, _body,\t_divContainer, _svgContainer, _identityMatrix, _gEl,\n\t_transformProp = \"transform\",\n\t_transformOriginProp = _transformProp + \"Origin\",\n\t_hasOffsetBug,\n\t_setDoc = element => {\n\t\tlet doc = element.ownerDocument || element;\n\t\tif (!(_transformProp in element.style) && \"msTransform\" in element.style) { //to improve compatibility with old Microsoft browsers\n\t\t\t_transformProp = \"msTransform\";\n\t\t\t_transformOriginProp = _transformProp + \"Origin\";\n\t\t}\n\t\twhile (doc.parentNode && (doc = doc.parentNode)) {\t}\n\t\t_win = window;\n\t\t_identityMatrix = new Matrix2D();\n\t\tif (doc) {\n\t\t\t_doc = doc;\n\t\t\t_docElement = doc.documentElement;\n\t\t\t_body = doc.body;\n\t\t\t_gEl = _doc.createElementNS(\"http://www.w3.org/2000/svg\", \"g\");\n\t\t\t// prevent any existing CSS from transforming it\n\t\t\t_gEl.style.transform = \"none\";\n\t\t\t// now test for the offset reporting bug. Use feature detection instead of browser sniffing to make things more bulletproof and future-proof. Hopefully Safari will fix their bug soon.\n\t\t\tlet d1 = doc.createElement(\"div\"),\n\t\t\t\td2 = doc.createElement(\"div\"),\n\t\t\t\troot = doc && (doc.body || doc.firstElementChild);\n\t\t\tif (root && root.appendChild) {\n\t\t\t\troot.appendChild(d1);\n\t\t\t\td1.appendChild(d2);\n\t\t\t\td1.setAttribute(\"style\", \"position:static;transform:translate3d(0,0,1px)\");\n\t\t\t\t_hasOffsetBug = (d2.offsetParent !== d1);\n\t\t\t\troot.removeChild(d1);\n\t\t\t}\n\t\t}\n\t\treturn doc;\n\t},\n\t_forceNonZeroScale = e => { // walks up the element's ancestors and finds any that had their scale set to 0 via GSAP, and changes them to 0.0001 to ensure that measurements work. Firefox has a bug that causes it to incorrectly report getBoundingClientRect() when scale is 0.\n\t\tlet a, cache;\n\t\twhile (e && e !== _body) {\n\t\t\tcache = e._gsap;\n\t\t\tcache && cache.uncache && cache.get(e, \"x\"); // force re-parsing of transforms if necessary\n\t\t\tif (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) {\n\t\t\t\tcache.scaleX = cache.scaleY = 1e-4;\n\t\t\t\tcache.renderTransform(1, cache);\n\t\t\t\ta ? a.push(cache) : (a = [cache]);\n\t\t\t}\n\t\t\te = e.parentNode;\n\t\t}\n\t\treturn a;\n\t},\n\t// possible future addition: pass an element to _forceDisplay() and it'll walk up all its ancestors and make sure anything with display: none is set to display: block, and if there's no parentNode, it'll add it to the body. It returns an Array that you can then feed to _revertDisplay() to have it revert all the changes it made.\n\t// _forceDisplay = e => {\n\t// \tlet a = [],\n\t// \t\tparent;\n\t// \twhile (e && e !== _body) {\n\t// \t\tparent = e.parentNode;\n\t// \t\t(_win.getComputedStyle(e).display === \"none\" || !parent) && a.push(e, e.style.display, parent) && (e.style.display = \"block\");\n\t// \t\tparent || _body.appendChild(e);\n\t// \t\te = parent;\n\t// \t}\n\t// \treturn a;\n\t// },\n\t// _revertDisplay = a => {\n\t// \tfor (let i = 0; i < a.length; i+=3) {\n\t// \t\ta[i+1] ? (a[i].style.display = a[i+1]) : a[i].style.removeProperty(\"display\");\n\t// \t\ta[i+2] || a[i].parentNode.removeChild(a[i]);\n\t// \t}\n\t// },\n\t_svgTemps = [], //we create 3 elements for SVG, and 3 for other DOM elements and cache them for performance reasons. They get nested in _divContainer and _svgContainer so that just one element is added to the DOM on each successive attempt. Again, performance is key.\n\t_divTemps = [],\n\t_getDocScrollTop = () => _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0,\n\t_getDocScrollLeft = () => _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0,\n\t_svgOwner = element => element.ownerSVGElement || ((element.tagName + \"\").toLowerCase() === \"svg\" ? element : null),\n\t_isFixed = element => {\n\t\tif (_win.getComputedStyle(element).position === \"fixed\") {\n\t\t\treturn true;\n\t\t}\n\t\telement = element.parentNode;\n\t\tif (element && element.nodeType === 1) { // avoid document fragments which will throw an error.\n\t\t\treturn _isFixed(element);\n\t\t}\n\t},\n\t_createSibling = (element, i) => {\n\t\tif (element.parentNode && (_doc || _setDoc(element))) {\n\t\t\tlet svg = _svgOwner(element),\n\t\t\t\tns = svg ? (svg.getAttribute(\"xmlns\") || \"http://www.w3.org/2000/svg\") : \"http://www.w3.org/1999/xhtml\",\n\t\t\t\ttype = svg ? (i ? \"rect\" : \"g\") : \"div\",\n\t\t\t\tx = i !== 2 ? 0 : 100,\n\t\t\t\ty = i === 3 ? 100 : 0,\n\t\t\t\tcss = \"position:absolute;display:block;pointer-events:none;margin:0;padding:0;\",\n\t\t\t\te = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, \"http\"), type) : _doc.createElement(type);\n\t\t\tif (i) {\n\t\t\t\tif (!svg) {\n\t\t\t\t\tif (!_divContainer) {\n\t\t\t\t\t\t_divContainer = _createSibling(element);\n\t\t\t\t\t\t_divContainer.style.cssText = css;\n\t\t\t\t\t}\n\t\t\t\t\te.style.cssText = css + \"width:0.1px;height:0.1px;top:\" + y + \"px;left:\" + x + \"px\";\n\t\t\t\t\t_divContainer.appendChild(e);\n\n\t\t\t\t} else {\n\t\t\t\t\t_svgContainer || (_svgContainer = _createSibling(element));\n\t\t\t\t\te.setAttribute(\"width\", 0.01);\n\t\t\t\t\te.setAttribute(\"height\", 0.01);\n\t\t\t\t\te.setAttribute(\"transform\", \"translate(\" + x + \",\" + y + \")\");\n\t\t\t\t\t_svgContainer.appendChild(e);\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn e;\n\t\t}\n\t\tthrow \"Need document and parent.\";\n\t},\n\t_consolidate = m => { // replaces SVGTransformList.consolidate() because a bug in Firefox causes it to break pointer events. See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800\n\t\tlet c = new Matrix2D(),\n\t\t\ti = 0;\n\t\tfor (; i < m.numberOfItems; i++) {\n\t\t\tc.multiply(m.getItem(i).matrix);\n\t\t}\n\t\treturn c;\n\t},\n\t_getCTM = svg => {\n\t\tlet m = svg.getCTM(),\n\t\t\ttransform;\n\t\tif (!m) { // Firefox returns null for getCTM() on root <svg> elements, so this is a workaround using a <g> that we temporarily append.\n\t\t\ttransform = svg.style[_transformProp];\n\t\t\tsvg.style[_transformProp] = \"none\"; // a bug in Firefox causes css transforms to contaminate the getCTM()\n\t\t\tsvg.appendChild(_gEl);\n\t\t\tm = _gEl.getCTM();\n\t\t\tsvg.removeChild(_gEl);\n\t\t\ttransform ? (svg.style[_transformProp] = transform) : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, \"-$1\").toLowerCase());\n\t\t}\n\t\treturn m || _identityMatrix.clone(); // Firefox will still return null if the <svg> has a width/height of 0 in the browser.\n\t},\n\t_placeSiblings = (element, adjustGOffset) => {\n\t\tlet svg = _svgOwner(element),\n\t\t\tisRootSVG = element === svg,\n\t\t\tsiblings = svg ? _svgTemps : _divTemps,\n\t\t\tparent = element.parentNode,\n\t\t\tcontainer, m, b, x, y, cs;\n\t\tif (element === _win) {\n\t\t\treturn element;\n\t\t}\n\t\tsiblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3));\n\t\tcontainer = svg ? _svgContainer : _divContainer;\n\t\tif (svg) {\n\t\t\tif (isRootSVG) {\n\t\t\t\tb = _getCTM(element);\n\t\t\t\tx = -b.e / b.a;\n\t\t\t\ty = -b.f / b.d;\n\t\t\t\tm = _identityMatrix;\n\t\t\t} else if (element.getBBox) {\n\t\t\t\tb = element.getBBox();\n\t\t\t\tm = element.transform ? element.transform.baseVal : {}; // IE11 doesn't follow the spec.\n\t\t\t\tm = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; // don't call m.consolidate().matrix because a bug in Firefox makes pointer events not work when consolidate() is called on the same tick as getBoundingClientRect()! See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800\n\t\t\t\tx = m.a * b.x + m.c * b.y;\n\t\t\t\ty = m.b * b.x + m.d * b.y;\n\t\t\t} else { // may be a <mask> which has no getBBox() so just use defaults instead of throwing errors.\n\t\t\t\tm = new Matrix2D();\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\tif (adjustGOffset && element.tagName.toLowerCase() === \"g\") {\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\t(isRootSVG ? svg : parent).appendChild(container);\n\t\t\tcontainer.setAttribute(\"transform\", \"matrix(\" + m.a + \",\" + m.b + \",\" + m.c + \",\" + m.d + \",\" + (m.e + x) + \",\" + (m.f + y) + \")\");\n\t\t} else {\n\t\t\tx = y = 0;\n\t\t\tif (_hasOffsetBug) { // some browsers (like Safari) have a bug that causes them to misreport offset values. When an ancestor element has a transform applied, it's supposed to treat it as if it's position: relative (new context). Safari botches this, so we need to find the closest ancestor (between the element and its offsetParent) that has a transform applied and if one is found, grab its offsetTop/Left and subtract them to compensate.\n\t\t\t\tm = element.offsetParent;\n\t\t\t\tb = element;\n\t\t\t\twhile (b && (b = b.parentNode) && b !== m && b.parentNode) {\n\t\t\t\t\tif ((_win.getComputedStyle(b)[_transformProp] + \"\").length > 4) {\n\t\t\t\t\t\tx = b.offsetLeft;\n\t\t\t\t\t\ty = b.offsetTop;\n\t\t\t\t\t\tb = 0;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\tcs = _win.getComputedStyle(element);\n\t\t\tif (cs.position !== \"absolute\" && cs.position !== \"fixed\") {\n\t\t\t\tm = element.offsetParent;\n\t\t\t\twhile (parent && parent !== m) { // if there's an ancestor element between the element and its offsetParent that's scrolled, we must factor that in.\n\t\t\t\t\tx += parent.scrollLeft || 0;\n\t\t\t\t\ty += parent.scrollTop || 0;\n\t\t\t\t\tparent = parent.parentNode;\n\t\t\t\t}\n\t\t\t}\n\t\t\tb = container.style;\n\t\t\tb.top = (element.offsetTop - y) + \"px\";\n\t\t\tb.left = (element.offsetLeft - x) + \"px\";\n\t\t\tb[_transformProp] = cs[_transformProp];\n\t\t\tb[_transformOriginProp] = cs[_transformOriginProp];\n\t\t\t// b.border = m.border;\n\t\t\t// b.borderLeftStyle = m.borderLeftStyle;\n\t\t\t// b.borderTopStyle = m.borderTopStyle;\n\t\t\t// b.borderLeftWidth = m.borderLeftWidth;\n\t\t\t// b.borderTopWidth = m.borderTopWidth;\n\t\t\tb.position = cs.position === \"fixed\" ? \"fixed\" : \"absolute\";\n\t\t\telement.parentNode.appendChild(container);\n\t\t}\n\t\treturn container;\n\t},\n\t_setMatrix = (m, a, b, c, d, e, f) => {\n\t\tm.a = a;\n\t\tm.b = b;\n\t\tm.c = c;\n\t\tm.d = d;\n\t\tm.e = e;\n\t\tm.f = f;\n\t\treturn m;\n\t};\n\nexport class Matrix2D {\n\tconstructor(a=1, b=0, c=0, d=1, e=0, f=0) {\n\t\t_setMatrix(this, a, b, c, d, e, f);\n\t}\n\n\tinverse() {\n\t\tlet {a, b, c, d, e, f} = this,\n\t\t\tdeterminant = (a * d - b * c) || 1e-10;\n\t\treturn _setMatrix(\n\t\t\tthis,\n\t\t\td / determinant,\n\t\t\t-b / determinant,\n\t\t\t-c / determinant,\n\t\t\ta / determinant,\n\t\t\t(c * f - d * e) / determinant,\n\t\t\t-(a * f - b * e) / determinant\n\t\t);\n\t}\n\n\tmultiply(matrix) {\n\t\tlet {a, b, c, d, e, f} = this,\n\t\t\ta2 = matrix.a,\n\t\t\tb2 = matrix.c,\n\t\t\tc2 = matrix.b,\n\t\t\td2 = matrix.d,\n\t\t\te2 = matrix.e,\n\t\t\tf2 = matrix.f;\n\t\treturn _setMatrix(this,\n\t\t\ta2 * a + c2 * c,\n\t\t\ta2 * b + c2 * d,\n\t\t\tb2 * a + d2 * c,\n\t\t\tb2 * b + d2 * d,\n\t\t\te + e2 * a + f2 * c,\n\t\t\tf + e2 * b + f2 * d);\n\t}\n\n\tclone() {\n\t\treturn new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f);\n\t}\n\n\tequals(matrix) {\n\t\tlet {a, b, c, d, e, f} = this;\n\t\treturn (a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f);\n\t}\n\n\tapply(point, decoratee={}) {\n\t\tlet {x, y} = point,\n\t\t\t{a, b, c, d, e, f} = this;\n\t\tdecoratee.x = (x * a + y * c + e) || 0;\n\t\tdecoratee.y = (x * b + y * d + f) || 0;\n\t\treturn decoratee;\n\t}\n\n}\n\n// Feed in an element and it'll return a 2D matrix (optionally inverted) so that you can translate between coordinate spaces.\n// Inverting lets you translate a global point into a local coordinate space. No inverting lets you go the other way.\n// We needed this to work around various browser bugs, like Firefox doesn't accurately report getScreenCTM() when there\n// are transforms applied to ancestor elements.\n// The matrix math to convert any x/y coordinate is as follows, which is wrapped in a convenient apply() method of Matrix2D above:\n// tx = m.a * x + m.c * y + m.e\n// ty = m.b * x + m.d * y + m.f\nexport function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { // adjustGOffset is typically used only when grabbing an element's PARENT's global matrix, and it ignores the x/y offset of any SVG <g> elements because they behave in a special way.\n\tif (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) {\n\t\treturn new Matrix2D();\n\t}\n\tlet zeroScales = _forceNonZeroScale(element),\n\t\tsvg = _svgOwner(element),\n\t\ttemps = svg ? _svgTemps : _divTemps,\n\t\tcontainer = _placeSiblings(element, adjustGOffset),\n\t\tb1 = temps[0].getBoundingClientRect(),\n\t\tb2 = temps[1].getBoundingClientRect(),\n\t\tb3 = temps[2].getBoundingClientRect(),\n\t\tparent = container.parentNode,\n\t\tisFixed = !includeScrollInFixed && _isFixed(element),\n\t\tm = new Matrix2D(\n\t\t\t(b2.left - b1.left) / 100,\n\t\t\t(b2.top - b1.top) / 100,\n\t\t\t(b3.left - b1.left) / 100,\n\t\t\t(b3.top - b1.top) / 100,\n\t\t\tb1.left + (isFixed ? 0 : _getDocScrollLeft()),\n\t\t\tb1.top + (isFixed ? 0 : _getDocScrollTop())\n\t\t);\n\tparent.removeChild(container);\n\tif (zeroScales) {\n\t\tb1 = zeroScales.length;\n\t\twhile (b1--) {\n\t\t\tb2 = zeroScales[b1];\n\t\t\tb2.scaleX = b2.scaleY = 0;\n\t\t\tb2.renderTransform(1, b2);\n\t\t}\n\t}\n\treturn inverse ? m.inverse() : m;\n}\n\nexport { _getDocScrollTop, _getDocScrollLeft, _setDoc, _isFixed, _getCTM };\n\n// export function getMatrix(element) {\n// \t_doc || _setDoc(element);\n// \tlet m = (_win.getComputedStyle(element)[_transformProp] + \"\").substr(7).match(/[-.]*\\d+[.e\\-+]*\\d*[e\\-\\+]*\\d*/g),\n// \t\tis2D = m && m.length === 6;\n// \treturn !m || m.length < 6 ? new Matrix2D() : new Matrix2D(+m[0], +m[1], +m[is2D ? 2 : 4], +m[is2D ? 3 : 5], +m[is2D ? 4 : 12], +m[is2D ? 5 : 13]);\n// }","/*!\n * Flip 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nimport { getGlobalMatrix, _getDocScrollTop, _getDocScrollLeft, Matrix2D, _setDoc, _getCTM } from \"./utils/matrix.js\";\n\nlet _id = 1,\n\t_toArray, gsap, _batch, _batchAction, _body, _closestTenth, _getStyleSaver,\n\t_forEachBatch = (batch, name) => batch.actions.forEach(a => a.vars[name] && a.vars[name](a)),\n\t_batchLookup = {},\n\t_RAD2DEG = 180 / Math.PI,\n\t_DEG2RAD = Math.PI / 180,\n\t_emptyObj = {},\n\t_dashedNameLookup = {},\n\t_memoizedRemoveProps = {},\n\t_listToArray = list => typeof(list) === \"string\" ? list.split(\" \").join(\"\").split(\",\") : list, // removes extra spaces contaminating the names, returns an Array.\n\t_callbacks = _listToArray(\"onStart,onUpdate,onComplete,onReverseComplete,onInterrupt\"),\n\t_removeProps = _listToArray(\"transform,transformOrigin,width,height,position,top,left,opacity,zIndex,maxWidth,maxHeight,minWidth,minHeight\"),\n\t_getEl = target => _toArray(target)[0] || console.warn(\"Element not found:\", target),\n\t_round = value => Math.round(value * 10000) / 10000 || 0,\n\t_toggleClass = (targets, className, action) => targets.forEach(el => el.classList[action](className)),\n\t_reserved = {zIndex:1, kill:1, simple:1, spin:1, clearProps:1, targets:1, toggleClass:1, onComplete:1, onUpdate:1, onInterrupt:1, onStart:1, delay:1, repeat:1, repeatDelay:1, yoyo:1, scale:1, fade:1, absolute:1, props:1, onEnter:1, onLeave:1, custom:1, paused:1, nested:1, prune:1, absoluteOnLeave: 1},\n\t_fitReserved = {zIndex:1, simple:1, clearProps:1, scale:1, absolute:1, fitChild:1, getVars:1, props:1},\n\t_camelToDashed = p => p.replace(/([A-Z])/g, \"-$1\").toLowerCase(),\n\t_copy = (obj, exclude) => {\n\t\tlet result = {}, p;\n\t\tfor (p in obj) {\n\t\t\texclude[p] || (result[p] = obj[p]);\n\t\t}\n\t\treturn result;\n\t},\n\t_memoizedProps = {},\n\t_memoizeProps = props => {\n\t\tlet p = _memoizedProps[props] = _listToArray(props);\n\t\t_memoizedRemoveProps[props] = p.concat(_removeProps);\n\t\treturn p;\n\t},\n\t_getInverseGlobalMatrix = el => { // integrates caching for improved performance\n\t\tlet cache = el._gsap || gsap.core.getCache(el);\n\t\tif (cache.gmCache === gsap.ticker.frame) {\n\t\t\treturn cache.gMatrix;\n\t\t}\n\t\tcache.gmCache = gsap.ticker.frame;\n\t\treturn (cache.gMatrix = getGlobalMatrix(el, true, false, true));\n\t},\n\t_getDOMDepth = (el, invert, level = 0) => { // In invert is true, the sibling depth is increments of 1, and parent/nesting depth is increments of 1000. This lets us order elements in an Array to reflect document flow.\n\t\tlet parent = el.parentNode,\n\t\t\tinc = 1000 * (10 ** level) * (invert ? -1 : 1),\n\t\t\tl = invert ? -inc * 900 : 0;\n\t\twhile (el) {\n\t\t\tl += inc;\n\t\t\tel = el.previousSibling;\n\t\t}\n\t\treturn parent ? l + _getDOMDepth(parent, invert, level + 1) : l;\n\t},\n\t_orderByDOMDepth = (comps, invert, isElStates) => {\n\t\tcomps.forEach(comp => comp.d = _getDOMDepth(isElStates ? comp.element : comp.t, invert));\n\t\tcomps.sort((c1, c2) => c1.d - c2.d);\n\t\treturn comps;\n\t},\n\t_recordInlineStyles = (elState, props) => { // records the current inline CSS properties into an Array in alternating name/value pairs that's stored in a \"css\" property on the state object so that we can revert later.\n\t\tlet style = elState.element.style,\n\t\t\ta = elState.css = elState.css || [],\n\t\t\ti = props.length,\n\t\t\tp, v;\n\t\twhile (i--) {\n\t\t\tp = props[i];\n\t\t\tv = style[p] || style.getPropertyValue(p);\n\t\t\ta.push(v ? p : _dashedNameLookup[p] || (_dashedNameLookup[p] = _camelToDashed(p)), v);\n\t\t}\n\t\treturn style;\n\t},\n\t_applyInlineStyles = state => {\n\t\tlet css = state.css,\n\t\t\tstyle = state.element.style,\n\t\t\ti = 0;\n\t\tstate.cache.uncache = 1;\n\t\tfor (; i < css.length; i+=2) {\n\t\t\tcss[i+1] ? (style[css[i]] = css[i+1]) : style.removeProperty(css[i]);\n\t\t}\n\t\tif (!css[css.indexOf(\"transform\")+1] && style.translate) { // CSSPlugin adds scale, translate, and rotate inline CSS as \"none\" in order to keep CSS rules from contaminating transforms.\n\t\t\tstyle.removeProperty(\"translate\");\n\t\t\tstyle.removeProperty(\"scale\");\n\t\t\tstyle.removeProperty(\"rotate\");\n\t\t}\n\t},\n\t_setFinalStates = (comps, onlyTransforms) => {\n\t\tcomps.forEach(c => c.a.cache.uncache = 1);\n\t\tonlyTransforms || comps.finalStates.forEach(_applyInlineStyles);\n\t},\n\t_absoluteProps = \"paddingTop,paddingRight,paddingBottom,paddingLeft,gridArea,transition\".split(\",\"), // properties that we must record just\n\t_makeAbsolute = (elState, fallbackNode, ignoreBatch) => {\n\t\tlet { element, width, height, uncache, getProp } = elState,\n\t\t\tstyle = element.style,\n\t\t\ti = 4,\n\t\t\tresult, displayIsNone, cs;\n\t\t(typeof(fallbackNode) !== \"object\") && (fallbackNode = elState);\n\t\tif (_batch && ignoreBatch !== 1) {\n\t\t\t_batch._abs.push({t: element, b: elState, a: elState, sd: 0});\n\t\t\t_batch._final.push(() => (elState.cache.uncache = 1) && _applyInlineStyles(elState));\n\t\t\treturn element;\n\t\t}\n\t\tdisplayIsNone = getProp(\"display\") === \"none\";\n\n\t\tif (!elState.isVisible || displayIsNone) {\n\t\t\tdisplayIsNone && (_recordInlineStyles(elState, [\"display\"]).display = fallbackNode.display);\n\t\t\telState.matrix = fallbackNode.matrix;\n\t\t\telState.width = width = elState.width || fallbackNode.width;\n\t\t\telState.height = height = elState.height || fallbackNode.height;\n\t\t}\n\n\t\t_recordInlineStyles(elState, _absoluteProps);\n\t\tcs = window.getComputedStyle(element);\n\t\twhile (i--) {\n\t\t\tstyle[_absoluteProps[i]] = cs[_absoluteProps[i]]; // record paddings as px-based because if removed from grid, percentage-based ones could be altered.\n\t\t}\n\t\tstyle.gridArea = \"1 / 1 / 1 / 1\";\n\t\tstyle.transition = \"none\";\n\n\t\tstyle.position = \"absolute\";\n\t\tstyle.width = width + \"px\";\n\t\tstyle.height = height + \"px\";\n\t\tstyle.top || (style.top = \"0px\");\n\t\tstyle.left || (style.left = \"0px\");\n\t\tif (uncache) {\n\t\t\tresult = new ElementState(element);\n\t\t} else { // better performance\n\t\t\tresult = _copy(elState, _emptyObj);\n\t\t\tresult.position = \"absolute\";\n\t\t\tif (elState.simple) {\n\t\t\t\tlet bounds = element.getBoundingClientRect();\n\t\t\t\tresult.matrix = new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop());\n\t\t\t} else {\n\t\t\t\tresult.matrix = getGlobalMatrix(element, false, false, true);\n\t\t\t}\n\t\t}\n\t\tresult = _fit(result, elState, true);\n\t\telState.x = _closestTenth(result.x, 0.01);\n\t\telState.y = _closestTenth(result.y, 0.01);\n\t\treturn element;\n\t},\n\t_filterComps = (comps, targets) => {\n\t\tif (targets !== true) {\n\t\t\ttargets = _toArray(targets);\n\t\t\tcomps = comps.filter(c => {\n\t\t\t\tif (targets.indexOf((c.sd < 0 ? c.b : c.a).element) !== -1) {\n\t\t\t\t return true;\n\t\t\t\t} else {\n\t\t\t\t\tc.t._gsap.renderTransform(1); // we must force transforms to render on anything that isn't being made position: absolute, otherwise the absolute position happens and then when animation begins it applies transforms which can create a new stacking context, throwing off positioning!\n\t\t\t\t\tif (c.b.isVisible) {\n\t\t\t\t\t\tc.t.style.width = c.b.width + \"px\"; // otherwise things can collapse when contents are made position: absolute.\n\t\t\t\t\t\tc.t.style.height = c.b.height + \"px\";\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t});\n\t\t}\n\t\treturn comps;\n\t},\n\t_makeCompsAbsolute = comps => _orderByDOMDepth(comps, true).forEach(c => (c.a.isVisible || c.b.isVisible) && _makeAbsolute(c.sd < 0 ? c.b : c.a, c.b, 1)),\n\t_findElStateInState = (state, other) => (other && state.idLookup[_parseElementState(other).id]) || state.elementStates[0],\n\t_parseElementState = (elOrNode, props, simple, other) => elOrNode instanceof ElementState ? elOrNode : elOrNode instanceof FlipState ? _findElStateInState(elOrNode, other) : new ElementState(typeof(elOrNode) === \"string\" ? _getEl(elOrNode) || console.warn(elOrNode + \" not found\") : elOrNode, props, simple),\n\t_recordProps = (elState, props) => {\n\t\tlet getProp = gsap.getProperty(elState.element, null, \"native\"),\n\t\t\tobj = elState.props = {},\n\t\t\ti = props.length;\n\t\twhile (i--) {\n\t\t\tobj[props[i]] = (getProp(props[i]) + \"\").trim();\n\t\t}\n\t\tobj.zIndex && (obj.zIndex = parseFloat(obj.zIndex) || 0);\n\t\treturn elState;\n\t},\n\t_applyProps = (element, props) => {\n\t\tlet style = element.style || element, // could pass in a vars object.\n\t\t\tp;\n\t\tfor (p in props) {\n\t\t\tstyle[p] = props[p];\n\t\t}\n\t},\n\t_getID = el => {\n\t\tlet id = el.getAttribute(\"data-flip-id\");\n\t\tid || el.setAttribute(\"data-flip-id\", (id = \"auto-\" + _id++));\n\t\treturn id;\n\t},\n\t_elementsFromElementStates = elStates => elStates.map(elState => elState.element),\n\t_handleCallback = (callback, elStates, tl) => callback && elStates.length && tl.add(callback(_elementsFromElementStates(elStates), tl, new FlipState(elStates, 0, true)), 0),\n\n\t_fit = (fromState, toState, scale, applyProps, fitChild, vars) => {\n\t\tlet { element, cache, parent, x, y } = fromState,\n\t\t\t{ width, height, scaleX, scaleY, rotation, bounds } = toState,\n\t\t\tstyles = vars && _getStyleSaver && _getStyleSaver(element, \"transform,width,height\"), // requires at least 3.11.5\n\t\t\tdimensionState = fromState,\n\t\t\t{e, f} = toState.matrix,\n\t\t\tdeep = fromState.bounds.width !== bounds.width || fromState.bounds.height !== bounds.height || fromState.scaleX !== scaleX || fromState.scaleY !== scaleY || fromState.rotation !== rotation,\n\t\t\tsimple = !deep && fromState.simple && toState.simple && !fitChild,\n\t\t\tskewX, fromPoint, toPoint, getProp, parentMatrix, matrix, bbox;\n\t\tif (simple || !parent) {\n\t\t\tscaleX = scaleY = 1;\n\t\t\trotation = skewX = 0;\n\t\t} else {\n\t\t\tparentMatrix = _getInverseGlobalMatrix(parent);\n\t\t\tmatrix = parentMatrix.clone().multiply(toState.ctm ? toState.matrix.clone().multiply(toState.ctm) : toState.matrix); // root SVG elements have a ctm that we must factor out (for example, viewBox:\"0 0 94 94\" with a width of 200px would scale the internals by 2.127 but when we're matching the size of the root <svg> element itself, that scaling shouldn't factor in!)\n\t\t\trotation = _round(Math.atan2(matrix.b, matrix.a) * _RAD2DEG);\n\t\t\tskewX = _round(Math.atan2(matrix.c, matrix.d) * _RAD2DEG + rotation) % 360; // in very rare cases, minor rounding might end up with 360 which should be 0.\n\t\t\tscaleX = Math.sqrt(matrix.a ** 2 + matrix.b ** 2);\n\t\t\tscaleY = Math.sqrt(matrix.c ** 2 + matrix.d ** 2) * Math.cos(skewX * _DEG2RAD);\n\t\t\tif (fitChild) {\n\t\t\t\tfitChild = _toArray(fitChild)[0];\n\t\t\t\tgetProp = gsap.getProperty(fitChild);\n\t\t\t\tbbox = fitChild.getBBox && typeof(fitChild.getBBox) === \"function\" && fitChild.getBBox();\n\t\t\t\tdimensionState = {scaleX: getProp(\"scaleX\"), scaleY: getProp(\"scaleY\"), width: bbox ? bbox.width : Math.ceil(parseFloat(getProp(\"width\", \"px\"))), height: bbox ? bbox.height : parseFloat(getProp(\"height\", \"px\")) };\n\t\t\t}\n\t\t\tcache.rotation = rotation + \"deg\";\n\t\t\tcache.skewX = skewX + \"deg\";\n\t\t}\n\t\tif (scale) {\n\t\t\tscaleX *= width === dimensionState.width || !dimensionState.width ? 1 : width / dimensionState.width; // note if widths are both 0, we should make scaleX 1 - some elements have box-sizing that incorporates padding, etc. and we don't want it to collapse in that case.\n\t\t\tscaleY *= height === dimensionState.height || !dimensionState.height ? 1 : height / dimensionState.height;\n\t\t\tcache.scaleX = scaleX;\n\t\t\tcache.scaleY = scaleY;\n\t\t} else {\n\t\t\twidth = _closestTenth(width * scaleX / dimensionState.scaleX, 0);\n\t\t\theight = _closestTenth(height * scaleY / dimensionState.scaleY, 0);\n\t\t\telement.style.width = width + \"px\";\n\t\t\telement.style.height = height + \"px\";\n\t\t}\n\t\t// if (fromState.isFixed) { // commented out because it's now taken care of in getGlobalMatrix() with a flag at the end.\n\t\t// \te -= _getDocScrollLeft();\n\t\t// \tf -= _getDocScrollTop();\n\t\t// }\n\t\tapplyProps && _applyProps(element, toState.props);\n\t\tif (simple || !parent) {\n\t\t\tx += e - fromState.matrix.e;\n\t\t\ty += f - fromState.matrix.f;\n\t\t} else if (deep || parent !== toState.parent) {\n\t\t\tcache.renderTransform(1, cache);\n\t\t\tmatrix = getGlobalMatrix(fitChild || element, false, false, true);\n\t\t\tfromPoint = parentMatrix.apply({x: matrix.e, y: matrix.f});\n\t\t\ttoPoint = parentMatrix.apply({x: e, y: f});\n\t\t\tx += toPoint.x - fromPoint.x;\n\t\t\ty += toPoint.y - fromPoint.y;\n\t\t} else { // use a faster/cheaper algorithm if we're just moving x/y\n\t\t\tparentMatrix.e = parentMatrix.f = 0;\n\t\t\ttoPoint = parentMatrix.apply({x: e - fromState.matrix.e, y: f - fromState.matrix.f});\n\t\t\tx += toPoint.x;\n\t\t\ty += toPoint.y;\n\t\t}\n\t\tx = _closestTenth(x, 0.02);\n\t\ty = _closestTenth(y, 0.02);\n\t\tif (vars && !(vars instanceof ElementState)) { // revert\n\t\t\tstyles && styles.revert();\n\t\t} else { // or apply the transform immediately\n\t\t\tcache.x = x + \"px\";\n\t\t\tcache.y = y + \"px\";\n\t\t\tcache.renderTransform(1, cache);\n\t\t}\n\t\tif (vars) {\n\t\t\tvars.x = x;\n\t\t\tvars.y = y;\n\t\t\tvars.rotation = rotation;\n\t\t\tvars.skewX = skewX;\n\t\t\tif (scale) {\n\t\t\t\tvars.scaleX = scaleX;\n\t\t\t\tvars.scaleY = scaleY;\n\t\t\t} else {\n\t\t\t\tvars.width = width;\n\t\t\t\tvars.height = height;\n\t\t\t}\n\t\t}\n\t\treturn vars || cache;\n\t},\n\n\t_parseState = (targetsOrState, vars) => targetsOrState instanceof FlipState ? targetsOrState : new FlipState(targetsOrState, vars),\n\t_getChangingElState = (toState, fromState, id) => {\n\t\tlet to1 = toState.idLookup[id],\n\t\t\tto2 = toState.alt[id];\n\t\treturn to2.isVisible && (!(fromState.getElementState(to2.element) || to2).isVisible || !to1.isVisible) ? to2 : to1;\n\t},\n\t_bodyMetrics = [], _bodyProps = \"width,height,overflowX,overflowY\".split(\",\"), _bodyLocked,\n\t_lockBodyScroll = lock => { // if there's no scrollbar, we should lock that so that measurements don't get affected by temporary repositioning, like if something is centered in the window.\n\t\tif (lock !== _bodyLocked) {\n\t\t\tlet s = _body.style,\n\t\t\t\tw = _body.clientWidth === window.outerWidth,\n\t\t\t\th = _body.clientHeight === window.outerHeight,\n\t\t\t\ti = 4;\n\t\t\tif (lock && (w || h)) {\n\t\t\t\twhile (i--) {\n\t\t\t\t\t_bodyMetrics[i] = s[_bodyProps[i]];\n\t\t\t\t}\n\t\t\t\tif (w) {\n\t\t\t\t\ts.width = _body.clientWidth + \"px\";\n\t\t\t\t\ts.overflowY = \"hidden\";\n\t\t\t\t}\n\t\t\t\tif (h) {\n\t\t\t\t\ts.height = _body.clientHeight + \"px\";\n\t\t\t\t\ts.overflowX = \"hidden\";\n\t\t\t\t}\n\t\t\t\t_bodyLocked= lock;\n\t\t\t} else if (_bodyLocked) {\n\t\t\t\twhile (i--) {\n\t\t\t\t\t_bodyMetrics[i] ? (s[_bodyProps[i]] = _bodyMetrics[i]) : s.removeProperty(_camelToDashed(_bodyProps[i]));\n\t\t\t\t}\n\t\t\t\t_bodyLocked = lock;\n\t\t\t}\n\t\t}\n\t},\n\n\t_fromTo = (fromState, toState, vars, relative) => { // relative is -1 if \"from()\", and 1 if \"to()\"\n\t\t(fromState instanceof FlipState && toState instanceof FlipState) || console.warn(\"Not a valid state object.\");\n\t\tvars = vars || {};\n\t\tlet { clearProps, onEnter, onLeave, absolute, absoluteOnLeave, custom, delay, paused, repeat, repeatDelay, yoyo, toggleClass, nested, zIndex, scale, fade, stagger, spin, prune } = vars,\n\t\t\tprops = (\"props\" in vars ? vars : fromState).props,\n\t\t\ttweenVars = _copy(vars, _reserved),\n\t\t\tanimation = gsap.timeline({ delay, paused, repeat, repeatDelay, yoyo, data: \"isFlip\" }),\n\t\t\tremainingProps = tweenVars,\n\t\t\tentering = [],\n\t\t\tleaving = [],\n\t\t\tcomps = [],\n\t\t\tswapOutTargets = [],\n\t\t\tspinNum = spin === true ? 1 : spin || 0,\n\t\t\tspinFunc = typeof(spin) === \"function\" ? spin : () => spinNum,\n\t\t\tinterrupted = fromState.interrupted || toState.interrupted,\n\t\t\taddFunc = animation[relative !== 1 ? \"to\" : \"from\"],\n\t\t\tv, p, endTime, i, el, comp, state, targets, finalStates, fromNode, toNode, run, a, b;\n\t\t//relative || (toState = (new FlipState(toState.targets, {props: props})).fit(toState, scale));\n\t\tfor (p in toState.idLookup) {\n\t\t\ttoNode = !toState.alt[p] ? toState.idLookup[p] : _getChangingElState(toState, fromState, p);\n\t\t\tel = toNode.element;\n\t\t\tfromNode = fromState.idLookup[p];\n\t\t\tfromState.alt[p] && el === fromNode.element && (fromState.alt[p].isVisible || !toNode.isVisible) && (fromNode = fromState.alt[p]);\n\t\t\tif (fromNode) {\n\t\t\t\tcomp = {t: el, b: fromNode, a: toNode, sd: fromNode.element === el ? 0 : toNode.isVisible ? 1 : -1};\n\t\t\t\tcomps.push(comp);\n\t\t\t\tif (comp.sd) {\n\t\t\t\t\tif (comp.sd < 0) {\n\t\t\t\t\t\tcomp.b = toNode;\n\t\t\t\t\t\tcomp.a = fromNode;\n\t\t\t\t\t}\n\t\t\t\t\t// for swapping elements that got interrupted, we must re-record the inline styles to ensure they're not tainted. Remember, .batch() permits getState() not to force in-progress flips to their end state.\n\t\t\t\t\tinterrupted && _recordInlineStyles(comp.b, props ? _memoizedRemoveProps[props] : _removeProps);\n\t\t\t\t\tfade && comps.push(comp.swap = {t: fromNode.element, b: comp.b, a: comp.a, sd: -comp.sd, swap: comp});\n\t\t\t\t}\n\t\t\t\tel._flip = fromNode.element._flip = _batch ? _batch.timeline : animation;\n\t\t\t} else if (toNode.isVisible) {\n\t\t\t\tcomps.push({t: el, b: _copy(toNode, {isVisible:1}), a: toNode, sd: 0, entering: 1}); // to include it in the \"entering\" Array and do absolute positioning if necessary\n\t\t\t\tel._flip = _batch ? _batch.timeline : animation;\n\t\t\t}\n\t\t}\n\n\t\tprops && (_memoizedProps[props] || _memoizeProps(props)).forEach(p => tweenVars[p] = i => comps[i].a.props[p]);\n\t\tcomps.finalStates = finalStates = [];\n\n\t\trun = () => {\n\t\t\t_orderByDOMDepth(comps);\n\t\t\t_lockBodyScroll(true); // otherwise, measurements may get thrown off when things get fit.\n\t\t\t// TODO: cache the matrix, especially for parent because it'll probably get reused quite a bit, but lock it to a particular cycle(?).\n\t\t\tfor (i = 0; i < comps.length; i++) {\n\t\t\t\tcomp = comps[i];\n\t\t\t\ta = comp.a;\n\t\t\t\tb = comp.b;\n\t\t\t\tif (prune && !a.isDifferent(b) && !comp.entering) { // only flip if things changed! Don't omit it from comps initially because that'd prevent the element from being positioned absolutely (if necessary)\n\t\t\t\t\tcomps.splice(i--, 1);\n\t\t\t\t} else {\n\t\t\t\t\tel = comp.t;\n\t\t\t\t\tnested && !(comp.sd < 0) && i && (a.matrix = getGlobalMatrix(el, false, false, true)); // moving a parent affects the position of children\n\t\t\t\t\tif (b.isVisible && a.isVisible) {\n\t\t\t\t\t\tif (comp.sd < 0) { // swapping OUT (swap direction of -1 is out)\n\t\t\t\t\t\t\tstate = new ElementState(el, props, fromState.simple);\n\t\t\t\t\t\t\t_fit(state, a, scale, 0, 0, state);\n\t\t\t\t\t\t\tstate.matrix = getGlobalMatrix(el, false, false, true);\n\t\t\t\t\t\t\tstate.css = comp.b.css;\n\t\t\t\t\t\t\tcomp.a = a = state;\n\t\t\t\t\t\t\tfade && (el.style.opacity = interrupted ? b.opacity : a.opacity);\n\t\t\t\t\t\t\tstagger && swapOutTargets.push(el);\n\t\t\t\t\t\t} else if (comp.sd > 0 && fade) { // swapping IN (swap direction of 1 is in)\n\t\t\t\t\t\t\tel.style.opacity = interrupted ? a.opacity - b.opacity : \"0\";\n\t\t\t\t\t\t}\n\t\t\t\t\t\t_fit(a, b, scale, props);\n\n\t\t\t\t\t} else if (b.isVisible !== a.isVisible) { // either entering or leaving (one side is invisible)\n\t\t\t\t\t\tif (!b.isVisible) { // entering\n\t\t\t\t\t\t\ta.isVisible && entering.push(a);\n\t\t\t\t\t\t\tcomps.splice(i--, 1);\n\t\t\t\t\t\t} else if (!a.isVisible) { // leaving\n\t\t\t\t\t\t\tb.css = a.css;\n\t\t\t\t\t\t\tleaving.push(b);\n\t\t\t\t\t\t\tcomps.splice(i--, 1);\n\t\t\t\t\t\t\tabsolute && nested && _fit(a, b, scale, props);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (!scale) {\n\t\t\t\t\t\tel.style.maxWidth = Math.max(a.width, b.width) + \"px\";\n\t\t\t\t\t\tel.style.maxHeight = Math.max(a.height, b.height) + \"px\";\n\t\t\t\t\t\tel.style.minWidth = Math.min(a.width, b.width) + \"px\";\n\t\t\t\t\t\tel.style.minHeight = Math.min(a.height, b.height) + \"px\";\n\t\t\t\t\t}\n\t\t\t\t\tnested && toggleClass && el.classList.add(toggleClass);\n\t\t\t\t}\n\t\t\t\tfinalStates.push(a);\n\t\t\t}\n\t\t\tlet classTargets;\n\t\t\tif (toggleClass) {\n\t\t\t\tclassTargets = finalStates.map(s => s.element);\n\t\t\t\tnested && classTargets.forEach(e => e.classList.remove(toggleClass)); // there could be a delay, so don't leave the classes applied (we'll do it in a timeline callback)\n\t\t\t}\n\n\t\t\t_lockBodyScroll(false);\n\n\t\t\tif (scale) {\n\t\t\t\ttweenVars.scaleX = i => comps[i].a.scaleX;\n\t\t\t\ttweenVars.scaleY = i => comps[i].a.scaleY;\n\t\t\t} else {\n\t\t\t\ttweenVars.width = i => comps[i].a.width + \"px\";\n\t\t\t\ttweenVars.height = i => comps[i].a.height + \"px\";\n\t\t\t\ttweenVars.autoRound = vars.autoRound || false;\n\t\t\t}\n\t\t\ttweenVars.x = i => comps[i].a.x + \"px\";\n\t\t\ttweenVars.y = i => comps[i].a.y + \"px\";\n\t\t\ttweenVars.rotation = i => comps[i].a.rotation + (spin ? spinFunc(i, targets[i], targets) * 360 : 0);\n\t\t\ttweenVars.skewX = i => comps[i].a.skewX;\n\n\t\t\ttargets = comps.map(c => c.t);\n\n\t\t\tif (zIndex || zIndex === 0) {\n\t\t\t\ttweenVars.modifiers = {zIndex: () => zIndex};\n\t\t\t\ttweenVars.zIndex = zIndex;\n\t\t\t\ttweenVars.immediateRender = vars.immediateRender !== false;\n\t\t\t}\n\n\t\t\tfade && (tweenVars.opacity = i => comps[i].sd < 0 ? 0 : comps[i].sd > 0 ? comps[i].a.opacity : \"+=0\");\n\n\t\t\tif (swapOutTargets.length) {\n\t\t\t\tstagger = gsap.utils.distribute(stagger);\n\t\t\t\tlet dummyArray = targets.slice(swapOutTargets.length);\n\t\t\t\ttweenVars.stagger = (i, el) => stagger(~swapOutTargets.indexOf(el) ? targets.indexOf(comps[i].swap.t) : i, el, dummyArray);\n\t\t\t}\n\n\t\t\t// // for testing...\n\t\t\t// gsap.delayedCall(vars.data ? 50 : 1, function() {\n\t\t\t// \tanimation.eventCallback(\"onComplete\", () => _setFinalStates(comps, !clearProps));\n\t\t\t// \taddFunc.call(animation, targets, tweenVars, 0).play();\n\t\t\t// });\n\t\t\t// return;\n\n\t\t\t_callbacks.forEach(name => vars[name] && animation.eventCallback(name, vars[name], vars[name + \"Params\"])); // apply callbacks to the timeline, not tweens (because \"custom\" timing can make multiple tweens)\n\n\t\t\tif (custom && targets.length) { // bust out the custom properties as their own tweens so they can use different eases, durations, etc.\n\t\t\t\tremainingProps = _copy(tweenVars, _reserved);\n\t\t\t\tif (\"scale\" in custom) {\n\t\t\t\t\tcustom.scaleX = custom.scaleY = custom.scale;\n\t\t\t\t\tdelete custom.scale;\n\t\t\t\t}\n\t\t\t\tfor (p in custom) {\n\t\t\t\t\tv = _copy(custom[p], _fitReserved);\n\t\t\t\t\tv[p] = tweenVars[p];\n\t\t\t\t\t!(\"duration\" in v) && (\"duration\" in tweenVars) && (v.duration = tweenVars.duration);\n\t\t\t\t\tv.stagger = tweenVars.stagger;\n\t\t\t\t\taddFunc.call(animation, targets, v, 0);\n\t\t\t\t\tdelete remainingProps[p];\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (targets.length || leaving.length || entering.length) {\n\t\t\t\ttoggleClass && animation.add(() => _toggleClass(classTargets, toggleClass, animation._zTime < 0 ? \"remove\" : \"add\"), 0) && !paused && _toggleClass(classTargets, toggleClass, \"add\");\n\t\t\t\ttargets.length && addFunc.call(animation, targets, remainingProps, 0);\n\t\t\t}\n\n\t\t\t_handleCallback(onEnter, entering, animation);\n\t\t\t_handleCallback(onLeave, leaving, animation);\n\n\t\t\tlet batchTl = _batch && _batch.timeline;\n\n\t\t\tif (batchTl) {\n\t\t\t\tbatchTl.add(animation, 0);\n\t\t\t\t_batch._final.push(() => _setFinalStates(comps, !clearProps));\n\t\t\t}\n\n\t\t\tendTime = animation.duration();\n\t\t\tanimation.call(() => {\n\t\t\t\tlet forward = animation.time() >= endTime;\n\t\t\t\tforward && !batchTl && _setFinalStates(comps, !clearProps);\n\t\t\t\ttoggleClass && _toggleClass(classTargets, toggleClass, forward ? \"remove\" : \"add\");\n\t\t\t});\n\t\t};\n\n\t\tabsoluteOnLeave && (absolute = comps.filter(comp => !comp.sd && !comp.a.isVisible && comp.b.isVisible).map(comp => comp.a.element));\n\t\tif (_batch) {\n\t\t\tabsolute && _batch._abs.push(..._filterComps(comps, absolute));\n\t\t\t_batch._run.push(run);\n\t\t} else {\n\t\t\tabsolute && _makeCompsAbsolute(_filterComps(comps, absolute)); // when making absolute, we must go in a very particular order so that document flow changes don't affect things. Don't make it visible if both the before and after states are invisible! There's no point, and it could make things appear visible during the flip that shouldn't be.\n\t\t\trun();\n\t\t}\n\n\t\tlet anim = _batch ? _batch.timeline : animation;\n\t\tanim.revert = () => _killFlip(anim, 1, 1); // a Flip timeline should behave very different when reverting - it should actually jump to the end so that styles get cleared out.\n\n\t\treturn anim;\n\t},\n\t_interrupt = tl => {\n\t\ttl.vars.onInterrupt && tl.vars.onInterrupt.apply(tl, tl.vars.onInterruptParams || []);\n\t\ttl.getChildren(true, false, true).forEach(_interrupt);\n\t},\n\t_killFlip = (tl, action, force) => { // action: 0 = nothing, 1 = complete, 2 = only kill (don't complete)\n\t\tif (tl && tl.progress() < 1 && (!tl.paused() || force)) {\n\t\t\tif (action) {\n\t\t\t\t_interrupt(tl);\n\t\t\t\taction < 2 && tl.progress(1); // we should also kill it in case it was added to a parent timeline.\n\t\t\t\ttl.kill();\n\t\t\t}\n\t\t\treturn true;\n\t\t}\n\t},\n\t_createLookup = state => {\n\t\tlet lookup = state.idLookup = {},\n\t\t\talt = state.alt = {},\n\t\t\telStates = state.elementStates,\n\t\t\ti = elStates.length,\n\t\t\telState;\n\t\twhile (i--) {\n\t\t\telState = elStates[i];\n\t\t\tlookup[elState.id] ? (alt[elState.id] = elState) : (lookup[elState.id] = elState);\n\t\t}\n\t};\n\n\n\n\n\n\nclass FlipState {\n\n\tconstructor(targets, vars, targetsAreElementStates) {\n\t\tthis.props = vars && vars.props;\n\t\tthis.simple = !!(vars && vars.simple);\n\t\tif (targetsAreElementStates) {\n\t\t\tthis.targets = _elementsFromElementStates(targets);\n\t\t\tthis.elementStates = targets;\n\t\t\t_createLookup(this);\n\t\t} else {\n\t\t\tthis.targets = _toArray(targets);\n\t\t\tlet soft = vars && (vars.kill === false || (vars.batch && !vars.kill));\n\t\t\t_batch && !soft && _batch._kill.push(this);\n\t\t\tthis.update(soft || !!_batch); // when batching, don't force in-progress flips to their end; we need to do that AFTER all getStates() are called.\n\t\t}\n\t}\n\n\tupdate(soft) {\n\t\tthis.elementStates = this.targets.map(el => new ElementState(el, this.props, this.simple));\n\t\t_createLookup(this);\n\t\tthis.interrupt(soft);\n\t\tthis.recordInlineStyles();\n\t\treturn this;\n\t}\n\n\tclear() {\n\t\tthis.targets.length = this.elementStates.length = 0;\n\t\t_createLookup(this);\n\t\treturn this;\n\t}\n\n\tfit(state, scale, nested) {\n\t\tlet elStatesInOrder = _orderByDOMDepth(this.elementStates.slice(0), false, true),\n\t\t\ttoElStates = (state || this).idLookup,\n\t\t\ti = 0,\n\t\t\tfromNode, toNode;\n\t\tfor (; i < elStatesInOrder.length; i++) {\n\t\t\tfromNode = elStatesInOrder[i];\n\t\t\tnested && (fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true)); // moving a parent affects the position of children\n\t\t\ttoNode = toElStates[fromNode.id];\n\t\t\ttoNode && _fit(fromNode, toNode, scale, true, 0, fromNode);\n\t\t\tfromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true);\n\t\t}\n\t\treturn this;\n\t}\n\n\tgetProperty(element, property) {\n\t\tlet es = this.getElementState(element) || _emptyObj;\n\t\treturn (property in es ? es : es.props || _emptyObj)[property];\n\t}\n\n\tadd(state) {\n\t\tlet i = state.targets.length,\n\t\t\tlookup = this.idLookup,\n\t\t\talt = this.alt,\n\t\t\tindex, es, es2;\n\t\twhile (i--) {\n\t\t\tes = state.elementStates[i];\n\t\t\tes2 = lookup[es.id];\n\t\t\tif (es2 && (es.element === es2.element || (alt[es.id] && alt[es.id].element === es.element))) { // if the flip id is already in this FlipState, replace it!\n\t\t\t\tindex = this.elementStates.indexOf(es.element === es2.element ? es2 : alt[es.id]);\n\t\t\t\tthis.targets.splice(index, 1, state.targets[i]);\n\t\t\t\tthis.elementStates.splice(index, 1, es);\n\t\t\t} else {\n\t\t\t\tthis.targets.push(state.targets[i]);\n\t\t\t\tthis.elementStates.push(es);\n\t\t\t}\n\t\t}\n\t\tstate.interrupted && (this.interrupted = true);\n\t\tstate.simple || (this.simple = false);\n\t\t_createLookup(this);\n\t\treturn this;\n\t}\n\n\tcompare(state) {\n\t\tlet l1 = state.idLookup,\n\t\t\tl2 = this.idLookup,\n\t\t\tunchanged = [],\n\t\t\tchanged = [],\n\t\t\tenter = [],\n\t\t\tleave = [],\n\t\t\ttargets = [],\n\t\t\ta1 = state.alt,\n\t\t\ta2 = this.alt,\n\t\t\tplace = (s1, s2, el) => (s1.isVisible !== s2.isVisible ? (s1.isVisible ? enter : leave) : s1.isVisible ? changed : unchanged).push(el) && targets.push(el),\n\t\t\tplaceIfDoesNotExist = (s1, s2, el) => targets.indexOf(el) < 0 && place(s1, s2, el),\n\t\t\ts1, s2, p, el, s1Alt, s2Alt, c1, c2;\n\t\tfor (p in l1) {\n\t\t\ts1Alt = a1[p];\n\t\t\ts2Alt = a2[p];\n\t\t\ts1 = !s1Alt ? l1[p] : _getChangingElState(state, this, p);\n\t\t\tel = s1.element;\n\t\t\ts2 = l2[p];\n\t\t\tif (s2Alt) {\n\t\t\t\tc2 = s2.isVisible || (!s2Alt.isVisible && el === s2.element) ? s2 : s2Alt;\n\t\t\t\tc1 = s1Alt && !s1.isVisible && !s1Alt.isVisible && c2.element === s1Alt.element ? s1Alt : s1;\n\t\t\t\t//c1.element !== c2.element && c1.element === s2.element && (c2 = s2);\n\t\t\t\tif (c1.isVisible && c2.isVisible && c1.element !== c2.element) { // swapping, so force into \"changed\" array\n\t\t\t\t\t(c1.isDifferent(c2) ? changed : unchanged).push(c1.element, c2.element);\n\t\t\t\t\ttargets.push(c1.element, c2.element);\n\t\t\t\t} else {\n\t\t\t\t\tplace(c1, c2, c1.element);\n\t\t\t\t}\n\t\t\t\ts1Alt && c1.element === s1Alt.element && (s1Alt = l1[p]);\n\t\t\t\tplaceIfDoesNotExist(c1.element !== s2.element && s1Alt ? s1Alt : c1, s2, s2.element);\n\t\t\t\tplaceIfDoesNotExist(s1Alt && s1Alt.element === s2Alt.element ? s1Alt : c1, s2Alt, s2Alt.element);\n\t\t\t\ts1Alt && placeIfDoesNotExist(s1Alt, s2Alt.element === s1Alt.element ? s2Alt : s2, s1Alt.element);\n\t\t\t} else {\n\t\t\t\t!s2 ? enter.push(el) : !s2.isDifferent(s1) ? unchanged.push(el) : place(s1, s2, el);\n\t\t\t\ts1Alt && placeIfDoesNotExist(s1Alt, s2, s1Alt.element);\n\t\t\t}\n\t\t}\n\t\tfor (p in l2) {\n\t\t\tif (!l1[p]) {\n\t\t\t\tleave.push(l2[p].element);\n\t\t\t\ta2[p] && leave.push(a2[p].element);\n\t\t\t}\n\t\t}\n\t\treturn {changed, unchanged, enter, leave};\n\t}\n\n\trecordInlineStyles() {\n\t\tlet props = _memoizedRemoveProps[this.props] || _removeProps,\n\t\t\ti = this.elementStates.length;\n\t\twhile (i--) {\n\t\t\t_recordInlineStyles(this.elementStates[i], props);\n\t\t}\n\t}\n\n\tinterrupt(soft) { // soft = DON'T force in-progress flip animations to completion (like when running a batch, we can't immediately kill flips when getting states because it could contaminate positioning and other .getState() calls that will run in the batch (we kill AFTER all the .getState() calls complete).\n\t\tlet timelines = [];\n\t\tthis.targets.forEach(t => {\n\t\t\tlet tl = t._flip,\n\t\t\t\tfoundInProgress = _killFlip(tl, soft ? 0 : 1);\n\t\t\tsoft && foundInProgress && timelines.indexOf(tl) < 0 && tl.add(() => this.updateVisibility());\n\t\t\tfoundInProgress && timelines.push(tl);\n\t\t});\n\t\t!soft && timelines.length && this.updateVisibility(); // if we found an in-progress Flip animation, we must record all the values in their current state at that point BUT we should update the isVisible value AFTER pushing that flip to completion so that elements that are entering or leaving will populate those Arrays properly.\n\t\tthis.interrupted || (this.interrupted = !!timelines.length);\n\t}\n\n\tupdateVisibility() {\n\t\tthis.elementStates.forEach(es => {\n\t\t\tlet b = es.element.getBoundingClientRect();\n\t\t\tes.isVisible = !!(b.width || b.height || b.top || b.left);\n\t\t\tes.uncache = 1;\n\t\t});\n\t}\n\n\tgetElementState(element) {\n\t\treturn this.elementStates[this.targets.indexOf(_getEl(element))];\n\t}\n\n\tmakeAbsolute() {\n\t\treturn _orderByDOMDepth(this.elementStates.slice(0), true, true).map(_makeAbsolute);\n\t}\n\n}\n\n\n\nclass ElementState {\n\n\tconstructor(element, props, simple) {\n\t\tthis.element = element;\n\t\tthis.update(props, simple);\n\t}\n\n\tisDifferent(state) {\n\t\tlet b1 = this.bounds,\n\t\t\tb2 = state.bounds;\n\t\treturn b1.top !== b2.top || b1.left !== b2.left || b1.width !== b2.width || b1.height !== b2.height || !this.matrix.equals(state.matrix) || this.opacity !== state.opacity || (this.props && state.props && JSON.stringify(this.props) !== JSON.stringify(state.props));\n\t}\n\n\tupdate(props, simple) {\n\t\tlet self = this,\n\t\t\telement = self.element,\n\t\t\tgetProp = gsap.getProperty(element),\n\t\t\tcache = gsap.core.getCache(element),\n\t\t\tbounds = element.getBoundingClientRect(),\n\t\t\tbbox = element.getBBox && typeof(element.getBBox) === \"function\" && element.nodeName.toLowerCase() !== \"svg\" && element.getBBox(),\n\t\t\tm = simple ? new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()) : getGlobalMatrix(element, false, false, true);\n\t\tself.getProp = getProp;\n\t\tself.element = element;\n\t\tself.id = _getID(element);\n\t\tself.matrix = m;\n\t\tself.cache = cache;\n\t\tself.bounds = bounds;\n\t\tself.isVisible = !!(bounds.width || bounds.height || bounds.left || bounds.top);\n\t\tself.display = getProp(\"display\");\n\t\tself.position = getProp(\"position\");\n\t\tself.parent = element.parentNode;\n\t\tself.x = getProp(\"x\");\n\t\tself.y = getProp(\"y\");\n\t\tself.scaleX = cache.scaleX;\n\t\tself.scaleY = cache.scaleY;\n\t\tself.rotation = getProp(\"rotation\");\n\t\tself.skewX = getProp(\"skewX\");\n\t\tself.opacity = getProp(\"opacity\");\n\t\tself.width = bbox ? bbox.width : _closestTenth(getProp(\"width\", \"px\"), 0.04); // round up to the closest 0.1 so that text doesn't wrap.\n\t\tself.height = bbox ? bbox.height : _closestTenth(getProp(\"height\", \"px\"), 0.04);\n\t\tprops && _recordProps(self, _memoizedProps[props] || _memoizeProps(props));\n\t\tself.ctm = element.getCTM && element.nodeName.toLowerCase() === \"svg\" && _getCTM(element).inverse();\n\t\tself.simple = simple || (_round(m.a) === 1 && !_round(m.b) && !_round(m.c) && _round(m.d) === 1); // allows us to speed through some other tasks if it's not scale/rotated\n\t\tself.uncache = 0;\n\t}\n\n}\n\nclass FlipAction {\n\tconstructor(vars, batch) {\n\t\tthis.vars = vars;\n\t\tthis.batch = batch;\n\t\tthis.states = [];\n\t\tthis.timeline = batch.timeline;\n\t}\n\n\tgetStateById(id) {\n\t\tlet i = this.states.length;\n\t\twhile (i--) {\n\t\t\tif (this.states[i].idLookup[id]) {\n\t\t\t\treturn this.states[i];\n\t\t\t}\n\t\t}\n\t}\n\n\tkill() {\n\t\tthis.batch.remove(this);\n\t}\n}\n\nclass FlipBatch {\n\tconstructor(id) {\n\t\tthis.id = id;\n\t\tthis.actions = [];\n\t\tthis._kill = [];\n\t\tthis._final = [];\n\t\tthis._abs = [];\n\t\tthis._run = [];\n\t\tthis.data = {};\n\t\tthis.state = new FlipState();\n\t\tthis.timeline = gsap.timeline();\n\t}\n\n\tadd(config) {\n\t\tlet result = this.actions.filter(action => action.vars === config);\n\t\tif (result.length) {\n\t\t\treturn result[0];\n\t\t}\n\t\tresult = new FlipAction(typeof(config) === \"function\" ? {animate: config} : config, this);\n\t\tthis.actions.push(result);\n\t\treturn result;\n\t}\n\n\tremove(action) {\n\t\tlet i = this.actions.indexOf(action);\n\t\ti >= 0 && this.actions.splice(i, 1);\n\t\treturn this;\n\t}\n\n\tgetState(merge) {\n\t\tlet prevBatch = _batch,\n\t\t\tprevAction = _batchAction;\n\t\t_batch = this;\n\t\tthis.state.clear();\n\t\tthis._kill.length = 0;\n\t\tthis.actions.forEach(action => {\n\t\t\tif (action.vars.getState) {\n\t\t\t\taction.states.length = 0;\n\t\t\t\t_batchAction = action;\n\t\t\t\taction.state = action.vars.getState(action);\n\t\t\t}\n\t\t\tmerge && action.states.forEach(s => this.state.add(s));\n\t\t});\n\t\t_batchAction = prevAction;\n\t\t_batch = prevBatch;\n\t\tthis.killConflicts();\n\t\treturn this;\n\t}\n\n\tanimate() {\n\t\tlet prevBatch = _batch,\n\t\t\ttl = this.timeline,\n\t\t\ti = this.actions.length,\n\t\t\tfinalStates, endTime;\n\t\t_batch = this;\n\t\ttl.clear();\n\t\tthis._abs.length = this._final.length = this._run.length = 0;\n\t\tthis.actions.forEach(a => {\n\t\t\ta.vars.animate && a.vars.animate(a);\n\t\t\tlet onEnter = a.vars.onEnter,\n\t\t\t\tonLeave = a.vars.onLeave,\n\t\t\t\ttargets = a.targets, s, result;\n\t\t\tif (targets && targets.length && (onEnter || onLeave)) {\n\t\t\t\ts = new FlipState();\n\t\t\t\ta.states.forEach(state => s.add(state));\n\t\t\t\tresult = s.compare(Flip.getState(targets));\n\t\t\t\tresult.enter.length && onEnter && onEnter(result.enter);\n\t\t\t\tresult.leave.length && onLeave && onLeave(result.leave);\n\t\t\t}\n\t\t});\n\t\t_makeCompsAbsolute(this._abs);\n\t\tthis._run.forEach(f => f());\n\t\tendTime = tl.duration();\n\t\tfinalStates = this._final.slice(0);\n\t\ttl.add(() => {\n\t\t\tif (endTime <= tl.time()) { // only call if moving forward in the timeline (in case it's nested in a timeline that gets reversed)\n\t\t\t\tfinalStates.forEach(f => f());\n\t\t\t\t_forEachBatch(this, \"onComplete\");\n\t\t\t}\n\t\t});\n\t\t_batch = prevBatch;\n\t\twhile (i--) {\n\t\t\tthis.actions[i].vars.once && this.actions[i].kill();\n\t\t}\n\t\t_forEachBatch(this, \"onStart\");\n\t\ttl.restart();\n\t\treturn this;\n\t}\n\n\tloadState(done) {\n\t\tdone || (done = () => 0);\n\t\tlet queue = [];\n\t\tthis.actions.forEach(c => {\n\t\t\tif (c.vars.loadState) {\n\t\t\t\tlet i, f = targets => {\n\t\t\t\t\ttargets && (c.targets = targets);\n\t\t\t\t\ti = queue.indexOf(f);\n\t\t\t\t\tif (~i) {\n\t\t\t\t\t\tqueue.splice(i, 1);\n\t\t\t\t\t\tqueue.length || done();\n\t\t\t\t\t}\n\t\t\t\t};\n\t\t\t\tqueue.push(f);\n\t\t\t\tc.vars.loadState(f);\n\t\t\t}\n\t\t});\n\t\tqueue.length || done();\n\t\treturn this;\n\t}\n\n\tsetState() {\n\t\tthis.actions.forEach(c => c.targets = c.vars.setState && c.vars.setState(c));\n\t\treturn this;\n\t}\n\n\tkillConflicts(soft) {\n\t\tthis.state.interrupt(soft);\n\t\tthis._kill.forEach(state => state.interrupt(soft));\n\t\treturn this;\n\t}\n\n\trun(skipGetState, merge) {\n\t\tif (this !== _batch) {\n\t\t\tskipGetState || this.getState(merge);\n\t\t\tthis.loadState(() => {\n\t\t\t\tif (!this._killed) {\n\t\t\t\t\tthis.setState();\n\t\t\t\t\tthis.animate();\n\t\t\t\t}\n\t\t\t});\n\t\t}\n\t\treturn this;\n\t}\n\n\tclear(stateOnly) {\n\t\tthis.state.clear();\n\t\tstateOnly || (this.actions.length = 0);\n\t}\n\n\tgetStateById(id) {\n\t\tlet i = this.actions.length,\n\t\t\ts;\n\t\twhile (i--) {\n\t\t\ts = this.actions[i].getStateById(id);\n\t\t\tif (s) {\n\t\t\t\treturn s;\n\t\t\t}\n\t\t}\n\t\treturn this.state.idLookup[id] && this.state;\n\t}\n\n\tkill() {\n\t\tthis._killed = 1;\n\t\tthis.clear();\n\t\tdelete _batchLookup[this.id];\n\t}\n}\n\n\nexport class Flip {\n\n\tstatic getState(targets, vars) {\n\t\tlet state = _parseState(targets, vars);\n\t\t_batchAction && _batchAction.states.push(state);\n\t\tvars && vars.batch && Flip.batch(vars.batch).state.add(state);\n\t\treturn state;\n\t}\n\n\tstatic from(state, vars) {\n\t\tvars = vars || {};\n\t\t(\"clearProps\" in vars) || (vars.clearProps = true);\n\t\treturn _fromTo(state, _parseState(vars.targets || state.targets, {props: vars.props || state.props, simple: vars.simple, kill: !!vars.kill}), vars, -1);\n\t}\n\n\tstatic to(state, vars) {\n\t\treturn _fromTo(state, _parseState(vars.targets || state.targets, {props: vars.props || state.props, simple: vars.simple, kill: !!vars.kill}), vars, 1);\n\t}\n\n\tstatic fromTo(fromState, toState, vars) {\n\t\treturn _fromTo(fromState, toState, vars);\n\t}\n\n\tstatic fit(fromEl, toEl, vars) {\n\t\tlet v = vars ? _copy(vars, _fitReserved) : {},\n\t\t\t{absolute, scale, getVars, props, runBackwards, onComplete, simple} = vars || v,\n\t\t\tfitChild = vars && vars.fitChild && _getEl(vars.fitChild),\n\t\t\tbefore = _parseElementState(toEl, props, simple, fromEl),\n\t\t\tafter = _parseElementState(fromEl, 0, simple, before),\n\t\t\tinlineProps = props ? _memoizedRemoveProps[props] : _removeProps,\n\t\t\tctx = gsap.context();\n\t\tprops && _applyProps(v, before.props);\n\t\t_recordInlineStyles(after, inlineProps);\n\t\tif (runBackwards) {\n\t\t\t(\"immediateRender\" in v) || (v.immediateRender = true);\n\t\t\tv.onComplete = function() {\n\t\t\t\t_applyInlineStyles(after);\n\t\t\t\tonComplete && onComplete.apply(this, arguments);\n\t\t\t};\n\t\t}\n\t\tabsolute && _makeAbsolute(after, before);\n\t\tv = _fit(after, before, scale || fitChild, props, fitChild, v.duration || getVars ? v : 0);\n\t\ttypeof(vars) === \"object\" && \"zIndex\" in vars && (v.zIndex = vars.zIndex);\n\t\tctx && !getVars && ctx.add(() => () => _applyInlineStyles(after));\n\t\treturn getVars ? v : v.duration ? gsap.to(after.element, v) : null;\n\t}\n\n\tstatic makeAbsolute(targetsOrStates, vars) {\n\t\treturn (targetsOrStates instanceof FlipState ? targetsOrStates : new FlipState(targetsOrStates, vars)).makeAbsolute();\n\t}\n\n\tstatic batch(id) {\n\t\tid || (id = \"default\");\n\t\treturn _batchLookup[id] || (_batchLookup[id] = new FlipBatch(id));\n\t}\n\n\tstatic killFlipsOf(targets, complete) {\n\t\t(targets instanceof FlipState ? targets.targets : _toArray(targets)).forEach(t => t && _killFlip(t._flip, complete !== false ? 1 : 2));\n\t}\n\n\tstatic isFlipping(target) {\n\t\tlet f = Flip.getByTarget(target);\n\t\treturn !!f && f.isActive();\n\t}\n\n\tstatic getByTarget(target) {\n\t\treturn (_getEl(target) || _emptyObj)._flip;\n\t}\n\n\tstatic getElementState(target, props) {\n\t\treturn new ElementState(_getEl(target), props);\n\t}\n\n\tstatic convertCoordinates(fromElement, toElement, point) {\n\t\tlet m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement));\n\t\treturn point ? m.apply(point) : m;\n\t}\n\n\n\tstatic register(core) {\n\t\t_body = typeof(document) !== \"undefined\" && document.body;\n\t\tif (_body) {\n\t\t\tgsap = core;\n\t\t\t_setDoc(_body);\n\t\t\t_toArray = gsap.utils.toArray;\n\t\t\t_getStyleSaver = gsap.core.getStyleSaver;\n\t\t\tlet snap = gsap.utils.snap(0.1);\n\t\t\t_closestTenth = (value, add) => snap(parseFloat(value) + add);\n\t\t}\n\t}\n}\n\nFlip.version = \"3.12.7\";\n\n// function whenImagesLoad(el, func) {\n// \tlet pending = [],\n// \t\tonLoad = e => {\n// \t\t\tpending.splice(pending.indexOf(e.target), 1);\n// \t\t\te.target.removeEventListener(\"load\", onLoad);\n// \t\t\tpending.length || func();\n// \t\t};\n// \tgsap.utils.toArray(el.tagName.toLowerCase() === \"img\" ? el : el.querySelectorAll(\"img\")).forEach(img => img.complete || img.addEventListener(\"load\", onLoad) || pending.push(img));\n// \tpending.length || func();\n// }\n\ntypeof(window) !== \"undefined\" && window.gsap && window.gsap.registerPlugin(Flip);\n\nexport { Flip as default };"],"names":["_setDoc","element","doc","ownerDocument","_transformProp","style","_transformOriginProp","parentNode","_win","window","_identityMatrix","Matrix2D","_docElement","_doc","documentElement","_body","body","_gEl","createElementNS","transform","d1","createElement","d2","root","firstElementChild","appendChild","setAttribute","_hasOffsetBug","offsetParent","removeChild","_getDocScrollTop","pageYOffset","scrollTop","_getDocScrollLeft","pageXOffset","scrollLeft","_svgOwner","ownerSVGElement","tagName","toLowerCase","_createSibling","i","svg","ns","getAttribute","type","x","y","css","e","replace","_svgContainer","_divContainer","cssText","_getCTM","m","getCTM","removeProperty","clone","_placeSiblings","adjustGOffset","container","b","cs","isRootSVG","siblings","_svgTemps","_divTemps","parent","length","push","a","f","d","getBBox","baseVal","numberOfItems","_consolidate","c","multiply","getItem","matrix","getComputedStyle","offsetLeft","offsetTop","position","top","left","_setMatrix","inverse","this","determinant","a2","b2","c2","e2","f2","equals","apply","point","decoratee","getGlobalMatrix","includeScrollInFixed","zeroScales","_forceNonZeroScale","cache","_gsap","uncache","get","scaleX","scaleY","renderTransform","temps","b1","getBoundingClientRect","b3","isFixed","_isFixed","nodeType","_forEachBatch","batch","name","actions","forEach","vars","_listToArray","list","split","join","_getEl","target","_toArray","console","warn","_round","value","Math","round","_toggleClass","targets","className","action","el","classList","_camelToDashed","p","_copy","obj","exclude","result","_memoizeProps","props","_memoizedProps","_memoizedRemoveProps","concat","_removeProps","_orderByDOMDepth","comps","invert","isElStates","comp","_getDOMDepth","level","inc","l","previousSibling","t","sort","c1","_recordInlineStyles","elState","v","getPropertyValue","_dashedNameLookup","_applyInlineStyles","state","indexOf","translate","_setFinalStates","onlyTransforms","finalStates","_makeAbsolute","fallbackNode","ignoreBatch","displayIsNone","width","height","getProp","_batch","_abs","sd","_final","isVisible","display","_absoluteProps","gridArea","transition","ElementState","_emptyObj","simple","bounds","_fit","_closestTenth","_filterComps","filter","_makeCompsAbsolute","_applyProps","_elementsFromElementStates","elStates","map","_handleCallback","callback","tl","add","FlipState","_parseState","targetsOrState","_getChangingElState","toState","fromState","id","to1","idLookup","to2","alt","getElementState","_lockBodyScroll","lock","_bodyLocked","s","w","clientWidth","outerWidth","h","clientHeight","outerHeight","_bodyMetrics","_bodyProps","overflowY","overflowX","_fromTo","relative","endTime","fromNode","toNode","run","clearProps","onEnter","onLeave","absolute","absoluteOnLeave","custom","delay","paused","repeat","repeatDelay","yoyo","toggleClass","nested","zIndex","scale","fade","stagger","spin","prune","tweenVars","_reserved","animation","gsap","timeline","data","remainingProps","entering","leaving","swapOutTargets","spinNum","spinFunc","interrupted","addFunc","swap","_flip","isDifferent","opacity","splice","maxWidth","max","maxHeight","minWidth","min","minHeight","classTargets","remove","autoRound","rotation","skewX","modifiers","immediateRender","utils","distribute","dummyArray","slice","_callbacks","eventCallback","_fitReserved","duration","call","_zTime","batchTl","forward","time","_run","anim","revert","_killFlip","_createLookup","lookup","elementStates","_batchAction","_getStyleSaver","_id","_batchLookup","_RAD2DEG","PI","_DEG2RAD","kill","onComplete","onUpdate","onInterrupt","onStart","fitChild","getVars","_parseElementState","elOrNode","other","_findElStateInState","applyProps","fromPoint","toPoint","parentMatrix","bbox","styles","dimensionState","deep","_getInverseGlobalMatrix","core","getCache","gmCache","ticker","frame","gMatrix","ctm","atan2","sqrt","cos","getProperty","ceil","parseFloat","force","progress","_interrupt","onInterruptParams","getChildren","update","soft","_this","interrupt","recordInlineStyles","clear","fit","elStatesInOrder","toElStates","property","es","index","es2","compare","place","s1","s2","enter","leave","changed","unchanged","placeIfDoesNotExist","s1Alt","s2Alt","l1","l2","a1","timelines","foundInProgress","_this2","updateVisibility","makeAbsolute","targetsAreElementStates","_kill","JSON","stringify","self","nodeName","_getID","_recordProps","trim","FlipAction","getStateById","states","FlipBatch","config","animate","getState","merge","prevBatch","prevAction","_this3","killConflicts","Flip","_this4","once","restart","loadState","done","queue","setState","skipGetState","_this5","_killed","stateOnly","from","to","fromTo","fromEl","toEl","runBackwards","before","after","inlineProps","ctx","context","arguments","targetsOrStates","killFlipsOf","complete","isFlipping","getByTarget","isActive","convertCoordinates","fromElement","toElement","register","document","toArray","getStyleSaver","snap","version","registerPlugin"],"mappings":";;;;;;;;;6MAeW,SAAVA,EAAUC,OACLC,EAAMD,EAAQE,eAAiBF,IAC7BG,KAAkBH,EAAQI,QAAU,gBAAiBJ,EAAQI,QAElEC,GADAF,EAAiB,eACuB,eAElCF,EAAIK,aAAeL,EAAMA,EAAIK,iBACpCC,EAAOC,OACPC,EAAkB,IAAIC,EAClBT,EAAK,CAERU,GADAC,EAAOX,GACWY,gBAClBC,EAAQb,EAAIc,MACZC,EAAOJ,EAAKK,gBAAgB,6BAA8B,MAErDb,MAAMc,UAAY,WAEnBC,EAAKlB,EAAImB,cAAc,OAC1BC,EAAKpB,EAAImB,cAAc,OACvBE,EAAOrB,IAAQA,EAAIc,MAAQd,EAAIsB,mBAC5BD,GAAQA,EAAKE,cAChBF,EAAKE,YAAYL,GACjBA,EAAGK,YAAYH,GACfF,EAAGM,aAAa,QAAS,kDACzBC,EAAiBL,EAAGM,eAAiBR,EACrCG,EAAKM,YAAYT,WAGZlB,EAoCW,SAAnB4B,WAAyBtB,EAAKuB,aAAgBlB,EAAKmB,WAAapB,EAAYoB,WAAajB,EAAMiB,WAAa,EACxF,SAApBC,WAA0BzB,EAAK0B,aAAerB,EAAKsB,YAAcvB,EAAYuB,YAAcpB,EAAMoB,YAAc,EACnG,SAAZC,EAAYnC,UAAWA,EAAQoC,kBAA6D,SAAxCpC,EAAQqC,QAAU,IAAIC,cAA0BtC,EAAU,MAU7F,SAAjBuC,EAAkBvC,EAASwC,MACtBxC,EAAQM,aAAeM,GAAQb,EAAQC,IAAW,KACjDyC,EAAMN,EAAUnC,GACnB0C,EAAKD,EAAOA,EAAIE,aAAa,UAAY,6BAAgC,+BACzEC,EAAOH,EAAOD,EAAI,OAAS,IAAO,MAClCK,EAAU,IAANL,EAAU,EAAI,IAClBM,EAAU,IAANN,EAAU,IAAM,EACpBO,EAAM,0EACNC,EAAIpC,EAAKK,gBAAkBL,EAAKK,gBAAgByB,EAAGO,QAAQ,SAAU,QAASL,GAAQhC,EAAKQ,cAAcwB,UACtGJ,IACEC,GAScS,EAAlBA,GAAkCX,EAAevC,GACjDgD,EAAEvB,aAAa,QAAS,KACxBuB,EAAEvB,aAAa,SAAU,KACzBuB,EAAEvB,aAAa,YAAa,aAAeoB,EAAI,IAAMC,EAAI,KACzDI,EAAc1B,YAAYwB,KAZrBG,KACJA,EAAgBZ,EAAevC,IACjBI,MAAMgD,QAAUL,GAE/BC,EAAE5C,MAAMgD,QAAUL,EAAM,gCAAkCD,EAAI,WAAaD,EAAI,KAC/EM,EAAc3B,YAAYwB,KAUrBA,OAEF,4BAUG,SAAVK,EAAUZ,OAERvB,EADGoC,EAAIb,EAAIc,gBAEPD,IACJpC,EAAYuB,EAAIrC,MAAMD,GACtBsC,EAAIrC,MAAMD,GAAkB,OAC5BsC,EAAIjB,YAAYR,GAChBsC,EAAItC,EAAKuC,SACTd,EAAIb,YAAYZ,GAChBE,EAAauB,EAAIrC,MAAMD,GAAkBe,EAAauB,EAAIrC,MAAMoD,eAAerD,EAAe8C,QAAQ,WAAY,OAAOX,gBAEnHgB,GAAK7C,EAAgBgD,QAEZ,SAAjBC,EAAkB1D,EAAS2D,OAKzBC,EAAWN,EAAGO,EAAGhB,EAAGC,EAAGgB,EAJpBrB,EAAMN,EAAUnC,GACnB+D,EAAY/D,IAAYyC,EACxBuB,EAAWvB,EAAMwB,EAAYC,EAC7BC,EAASnE,EAAQM,cAEdN,IAAYO,SACRP,KAERgE,EAASI,QAAUJ,EAASK,KAAK9B,EAAevC,EAAS,GAAIuC,EAAevC,EAAS,GAAIuC,EAAevC,EAAS,IACjH4D,EAAYnB,EAAMS,EAAgBC,EAC9BV,EACCsB,GAEHlB,IADAgB,EAAIR,EAAQrD,IACLgD,EAAIa,EAAES,EACbxB,GAAKe,EAAEU,EAAIV,EAAEW,EACblB,EAAI7C,GACMT,EAAQyE,SAClBZ,EAAI7D,EAAQyE,UAGZ5B,GADAS,GADAA,EAAItD,EAAQkB,UAAYlB,EAAQkB,UAAUwD,QAAU,IAC7CC,cAAoD,EAAlBrB,EAAEqB,cAzC/B,SAAfC,aAAetB,WACVuB,EAAI,IAAInE,EACX8B,EAAI,EACEA,EAAIc,EAAEqB,cAAenC,IAC3BqC,EAAEC,SAASxB,EAAEyB,QAAQvC,GAAGwC,eAElBH,EAmC0DD,CAAatB,GAAKA,EAAEyB,QAAQ,GAAGC,OAAvEvE,GACjB6D,EAAIT,EAAEhB,EAAIS,EAAEuB,EAAIhB,EAAEf,EACxBA,EAAIQ,EAAEO,EAAIA,EAAEhB,EAAIS,EAAEkB,EAAIX,EAAEf,IAExBQ,EAAI,IAAI5C,EACRmC,EAAIC,EAAI,GAELa,GAAmD,MAAlC3D,EAAQqC,QAAQC,gBACpCO,EAAIC,EAAI,IAERiB,EAAYtB,EAAM0B,GAAQ3C,YAAYoC,GACvCA,EAAUnC,aAAa,YAAa,UAAY6B,EAAEgB,EAAI,IAAMhB,EAAEO,EAAI,IAAMP,EAAEuB,EAAI,IAAMvB,EAAEkB,EAAI,KAAOlB,EAAEN,EAAIH,GAAK,KAAOS,EAAEiB,EAAIzB,GAAK,SACxH,IACND,EAAIC,EAAI,EACJpB,MACH4B,EAAItD,EAAQ2B,aACZkC,EAAI7D,GACS6D,EAANA,GAAUA,EAAEvD,aAAeuD,IAAMP,GAAKO,EAAEvD,YACe,GAAxDC,EAAK0E,iBAAiBpB,GAAG1D,GAAkB,IAAIiE,SACnDvB,EAAIgB,EAAEqB,WACNpC,EAAIe,EAAEsB,UACNtB,EAAI,MAKa,cADpBC,EAAKvD,EAAK0E,iBAAiBjF,IACpBoF,UAA2C,UAAhBtB,EAAGsB,aACpC9B,EAAItD,EAAQ2B,aACLwC,GAAUA,IAAWb,GAC3BT,GAAKsB,EAAOjC,YAAc,EAC1BY,GAAKqB,EAAOpC,WAAa,EACzBoC,EAASA,EAAO7D,YAGlBuD,EAAID,EAAUxD,OACZiF,IAAOrF,EAAQmF,UAAYrC,EAAK,KAClCe,EAAEyB,KAAQtF,EAAQkF,WAAarC,EAAK,KACpCgB,EAAE1D,GAAkB2D,EAAG3D,GACvB0D,EAAExD,GAAwByD,EAAGzD,GAM7BwD,EAAEuB,SAA2B,UAAhBtB,EAAGsB,SAAuB,QAAU,WACjDpF,EAAQM,WAAWkB,YAAYoC,UAEzBA,EAEK,SAAb2B,EAAcjC,EAAGgB,EAAGT,EAAGgB,EAAGL,EAAGxB,EAAGuB,UAC/BjB,EAAEgB,EAAIA,EACNhB,EAAEO,EAAIA,EACNP,EAAEuB,EAAIA,EACNvB,EAAEkB,EAAIA,EACNlB,EAAEN,EAAIA,EACNM,EAAEiB,EAAIA,EACCjB,EA/MT,IAAI1C,EAAML,EAAMI,EAAaG,EAAOqC,EAAeD,EAAezC,EAAiBO,EAGlFU,IAFAvB,EAAiB,YACjBE,EAAuBF,EAAiB,SAgExC8D,EAAY,GACZC,EAAY,GA+IAxD,0BAKZ8E,QAAA,uBACMlB,EAAoBmB,KAApBnB,EAAGT,EAAiB4B,KAAjB5B,EAAGgB,EAAcY,KAAdZ,EAAGL,EAAWiB,KAAXjB,EAAGxB,EAAQyC,KAARzC,EAAGuB,EAAKkB,KAALlB,EACnBmB,EAAepB,EAAIE,EAAIX,EAAIgB,GAAM,aAC3BU,EACNE,KACAjB,EAAIkB,GACH7B,EAAI6B,GACJb,EAAIa,EACLpB,EAAIoB,GACHb,EAAIN,EAAIC,EAAIxB,GAAK0C,IAChBpB,EAAIC,EAAIV,EAAIb,GAAK0C,MAIrBZ,SAAA,kBAASE,OACHV,EAAoBmB,KAApBnB,EAAGT,EAAiB4B,KAAjB5B,EAAGgB,EAAcY,KAAdZ,EAAGL,EAAWiB,KAAXjB,EAAGxB,EAAQyC,KAARzC,EAAGuB,EAAKkB,KAALlB,EACnBoB,EAAKX,EAAOV,EACZsB,EAAKZ,EAAOH,EACZgB,EAAKb,EAAOnB,EACZxC,EAAK2D,EAAOR,EACZsB,EAAKd,EAAOhC,EACZ+C,EAAKf,EAAOT,SACNgB,EAAWE,KACjBE,EAAKrB,EAAIuB,EAAKhB,EACdc,EAAK9B,EAAIgC,EAAKrB,EACdoB,EAAKtB,EAAIjD,EAAKwD,EACde,EAAK/B,EAAIxC,EAAKmD,EACdxB,EAAI8C,EAAKxB,EAAIyB,EAAKlB,EAClBN,EAAIuB,EAAKjC,EAAIkC,EAAKvB,MAGpBf,MAAA,wBACQ,IAAI/C,SAAS+E,KAAKnB,EAAGmB,KAAK5B,EAAG4B,KAAKZ,EAAGY,KAAKjB,EAAGiB,KAAKzC,EAAGyC,KAAKlB,MAGlEyB,OAAA,gBAAOhB,OACDV,EAAoBmB,KAApBnB,EAAGT,EAAiB4B,KAAjB5B,EAAGgB,EAAcY,KAAdZ,EAAGL,EAAWiB,KAAXjB,EAAGxB,EAAQyC,KAARzC,EAAGuB,EAAKkB,KAALlB,SACZD,IAAMU,EAAOV,GAAKT,IAAMmB,EAAOnB,GAAKgB,IAAMG,EAAOH,GAAKL,IAAMQ,EAAOR,GAAKxB,IAAMgC,EAAOhC,GAAKuB,IAAMS,EAAOT,KAGhH0B,MAAA,eAAMC,EAAOC,YAAAA,IAAAA,EAAU,QACjBtD,EAAQqD,EAARrD,EAAGC,EAAKoD,EAALpD,EACNwB,EAAoBmB,KAApBnB,EAAGT,EAAiB4B,KAAjB5B,EAAGgB,EAAcY,KAAdZ,EAAGL,EAAWiB,KAAXjB,EAAGxB,EAAQyC,KAARzC,EAAGuB,EAAKkB,KAALlB,SACjB4B,EAAUtD,EAAKA,EAAIyB,EAAIxB,EAAI+B,EAAI7B,GAAM,EACrCmD,EAAUrD,EAAKD,EAAIgB,EAAIf,EAAI0B,EAAID,GAAM,EAC9B4B,+BAjDI7B,EAAKT,EAAKgB,EAAKL,EAAKxB,EAAKuB,YAAzBD,IAAAA,EAAE,YAAGT,IAAAA,EAAE,YAAGgB,IAAAA,EAAE,YAAGL,IAAAA,EAAE,YAAGxB,IAAAA,EAAE,YAAGuB,IAAAA,EAAE,GACtCgB,EAAWE,KAAMnB,EAAGT,EAAGgB,EAAGL,EAAGxB,EAAGuB,GA4D3B,SAAS6B,gBAAgBpG,EAASwF,EAAS7B,EAAe0C,OAC3DrG,IAAYA,EAAQM,aAAeM,GAAQb,EAAQC,IAAUa,kBAAoBb,SAC9E,IAAIU,MAER4F,EAlPiB,SAArBC,mBAAqBvD,WAChBsB,EAAGkC,EACAxD,GAAKA,IAAMlC,IACjB0F,EAAQxD,EAAEyD,QACDD,EAAME,SAAWF,EAAMG,IAAI3D,EAAG,KACnCwD,IAAUA,EAAMI,SAAWJ,EAAMK,QAAUL,EAAMM,kBACpDN,EAAMI,OAASJ,EAAMK,OAAS,KAC9BL,EAAMM,gBAAgB,EAAGN,GACzBlC,EAAIA,EAAED,KAAKmC,GAAUlC,EAAI,CAACkC,IAE3BxD,EAAIA,EAAE1C,kBAEAgE,EAsOSiC,CAAmBvG,GAEnC+G,EADM5E,EAAUnC,GACFiE,EAAYC,EAC1BN,EAAYF,EAAe1D,EAAS2D,GACpCqD,EAAKD,EAAM,GAAGE,wBACdrB,EAAKmB,EAAM,GAAGE,wBACdC,EAAKH,EAAM,GAAGE,wBACd9C,EAASP,EAAUtD,WACnB6G,GAAWd,GArND,SAAXe,SAAWpH,SACsC,UAA5CO,EAAK0E,iBAAiBjF,GAASoF,YAGnCpF,EAAUA,EAAQM,aACkB,IAArBN,EAAQqH,SACfD,SAASpH,WA+MkBoH,CAASpH,GAC5CsD,EAAI,IAAI5C,GACNkF,EAAGN,KAAO0B,EAAG1B,MAAQ,KACrBM,EAAGP,IAAM2B,EAAG3B,KAAO,KACnB6B,EAAG5B,KAAO0B,EAAG1B,MAAQ,KACrB4B,EAAG7B,IAAM2B,EAAG3B,KAAO,IACpB2B,EAAG1B,MAAQ6B,EAAU,EAAInF,KACzBgF,EAAG3B,KAAO8B,EAAU,EAAItF,SAE1BsC,EAAOvC,YAAYgC,GACf0C,MACHU,EAAKV,EAAWlC,OACT4C,MACNpB,EAAKU,EAAWU,IACbJ,OAAShB,EAAGiB,OAAS,EACxBjB,EAAGkB,gBAAgB,EAAGlB,UAGjBJ,EAAUlC,EAAEkC,UAAYlC,EC1Sf,SAAhBgE,EAAiBC,EAAOC,UAASD,EAAME,QAAQC,QAAQ,SAAApD,UAAKA,EAAEqD,KAAKH,IAASlD,EAAEqD,KAAKH,GAAMlD,KAO1E,SAAfsD,EAAeC,SAAyB,iBAAVA,EAAqBA,EAAKC,MAAM,KAAKC,KAAK,IAAID,MAAM,KAAOD,EAGhF,SAATG,EAASC,UAAUC,EAASD,GAAQ,IAAME,QAAQC,KAAK,qBAAsBH,GACpE,SAATI,EAASC,UAASC,KAAKC,MAAc,IAARF,GAAiB,KAAS,EACxC,SAAfG,EAAgBC,EAASC,EAAWC,UAAWF,EAAQhB,QAAQ,SAAAmB,UAAMA,EAAGC,UAAUF,GAAQD,KAGzE,SAAjBI,EAAiBC,UAAKA,EAAE/F,QAAQ,WAAY,OAAOX,cAC3C,SAAR2G,EAASC,EAAKC,OACIH,EAAbI,EAAS,OACRJ,KAAKE,EACTC,EAAQH,KAAOI,EAAOJ,GAAKE,EAAIF,WAEzBI,EAGQ,SAAhBC,GAAgBC,OACXN,EAAIO,GAAeD,GAAS1B,EAAa0B,UAC7CE,GAAqBF,GAASN,EAAES,OAAOC,IAChCV,EAoBW,SAAnBW,GAAoBC,EAAOC,EAAQC,UAClCF,EAAMlC,QAAQ,SAAAqC,UAAQA,EAAKvF,EAXb,SAAfwF,aAAgBnB,EAAIgB,EAAQI,YAAAA,IAAAA,EAAQ,WAC/B9F,EAAS0E,EAAGvI,WACf4J,EAAM,aAAQ,GAAMD,IAAUJ,GAAU,EAAI,GAC5CM,EAAIN,EAAgB,KAANK,EAAY,EACpBrB,GACNsB,GAAKD,EACLrB,EAAKA,EAAGuB,uBAEFjG,EAASgG,EAAIH,aAAa7F,EAAQ0F,EAAQI,EAAQ,GAAKE,EAG/BH,CAAaF,EAAaC,EAAK/J,QAAU+J,EAAKM,EAAGR,KAChFD,EAAMU,KAAK,SAACC,EAAI1E,UAAO0E,EAAG/F,EAAIqB,EAAGrB,IAC1BoF,EAEc,SAAtBY,GAAuBC,EAASnB,WAI9BN,EAAG0B,EAHAtK,EAAQqK,EAAQzK,QAAQI,MAC3BkE,EAAImG,EAAQ1H,IAAM0H,EAAQ1H,KAAO,GACjCP,EAAI8G,EAAMlF,OAEJ5B,KAENkI,EAAItK,EADJ4I,EAAIM,EAAM9G,KACMpC,EAAMuK,iBAAiB3B,GACvC1E,EAAED,KAAKqG,EAAI1B,EAAI4B,EAAkB5B,KAAO4B,EAAkB5B,GAAKD,EAAeC,IAAK0B,UAE7EtK,EAEa,SAArByK,GAAqBC,OAChB/H,EAAM+H,EAAM/H,IACf3C,EAAQ0K,EAAM9K,QAAQI,MACtBoC,EAAI,MACLsI,EAAMtE,MAAME,QAAU,EACflE,EAAIO,EAAIqB,OAAQ5B,GAAG,EACzBO,EAAIP,EAAE,GAAMpC,EAAM2C,EAAIP,IAAMO,EAAIP,EAAE,GAAMpC,EAAMoD,eAAeT,EAAIP,KAE7DO,EAAIA,EAAIgI,QAAQ,aAAa,IAAM3K,EAAM4K,YAC7C5K,EAAMoD,eAAe,aACrBpD,EAAMoD,eAAe,SACrBpD,EAAMoD,eAAe,WAGL,SAAlByH,GAAmBrB,EAAOsB,GACzBtB,EAAMlC,QAAQ,SAAA7C,UAAKA,EAAEP,EAAEkC,MAAME,QAAU,IACvCwE,GAAkBtB,EAAMuB,YAAYzD,QAAQmD,IAG7B,SAAhBO,GAAiBX,EAASY,EAAcC,OAItClC,EAAQmC,EAAezH,EAHlB9D,EAA6CyK,EAA7CzK,QAASwL,EAAoCf,EAApCe,MAAOC,EAA6BhB,EAA7BgB,OAAQ/E,EAAqB+D,EAArB/D,QAASgF,EAAYjB,EAAZiB,QACtCtL,EAAQJ,EAAQI,MAChBoC,EAAI,KAEqB,iBAAlB6I,IAAgCA,EAAeZ,GACnDkB,IAA0B,IAAhBL,SACbK,GAAOC,KAAKvH,KAAK,CAACgG,EAAGrK,EAAS6D,EAAG4G,EAASnG,EAAGmG,EAASoB,GAAI,IAC1DF,GAAOG,OAAOzH,KAAK,kBAAOoG,EAAQjE,MAAME,QAAU,IAAMmE,GAAmBJ,KACpEzK,MAERuL,EAAuC,SAAvBG,EAAQ,WAEnBjB,EAAQsB,YAAaR,IACzBA,IAAkBf,GAAoBC,EAAS,CAAC,YAAYuB,QAAUX,EAAaW,SACnFvB,EAAQzF,OAASqG,EAAarG,OAC9ByF,EAAQe,MAAQA,EAAQf,EAAQe,OAASH,EAAaG,MACtDf,EAAQgB,OAASA,EAAShB,EAAQgB,QAAUJ,EAAaI,QAG1DjB,GAAoBC,EAASwB,GAC7BnI,EAAKtD,OAAOyE,iBAAiBjF,GACtBwC,KACNpC,EAAM6L,EAAezJ,IAAMsB,EAAGmI,EAAezJ,OAE9CpC,EAAM8L,SAAW,gBACjB9L,EAAM+L,WAAa,OAEnB/L,EAAMgF,SAAW,WACjBhF,EAAMoL,MAAQA,EAAQ,KACtBpL,EAAMqL,OAASA,EAAS,KACxBrL,EAAMiF,MAAQjF,EAAMiF,IAAM,OAC1BjF,EAAMkF,OAASlF,EAAMkF,KAAO,OACxBoB,EACH0C,EAAS,IAAIgD,GAAapM,YAE1BoJ,EAASH,EAAMwB,EAAS4B,IACjBjH,SAAW,WACdqF,EAAQ6B,OAAQ,KACfC,EAASvM,EAAQiH,wBACrBmC,EAAOpE,OAAS,IAAItE,EAAS,EAAG,EAAG,EAAG,EAAG6L,EAAOjH,KAAOtD,IAAqBuK,EAAOlH,IAAMxD,UAEzFuH,EAAOpE,OAASoB,gBAAgBpG,GAAS,GAAO,GAAO,UAGzDoJ,EAASoD,GAAKpD,EAAQqB,GAAS,GAC/BA,EAAQ5H,EAAI4J,EAAcrD,EAAOvG,EAAG,KACpC4H,EAAQ3H,EAAI2J,EAAcrD,EAAOtG,EAAG,KAC7B9C,EAEO,SAAf0M,GAAgB9C,EAAOlB,UACN,IAAZA,IACHA,EAAUR,EAASQ,GACnBkB,EAAQA,EAAM+C,OAAO,SAAA9H,OACqC,IAArD6D,EAAQqC,SAASlG,EAAEgH,GAAK,EAAIhH,EAAEhB,EAAIgB,EAAEP,GAAGtE,gBAChC,EAEV6E,EAAEwF,EAAE5D,MAAMK,gBAAgB,GACtBjC,EAAEhB,EAAEkI,YACPlH,EAAEwF,EAAEjK,MAAMoL,MAAQ3G,EAAEhB,EAAE2H,MAAQ,KAC9B3G,EAAEwF,EAAEjK,MAAMqL,OAAS5G,EAAEhB,EAAE4H,OAAS,SAK7B7B,EAEa,SAArBgD,GAAqBhD,UAASD,GAAiBC,GAAO,GAAMlC,QAAQ,SAAA7C,UAAMA,EAAEP,EAAEyH,WAAalH,EAAEhB,EAAEkI,YAAcX,GAAcvG,EAAEgH,GAAK,EAAIhH,EAAEhB,EAAIgB,EAAEP,EAAGO,EAAEhB,EAAG,KAaxI,SAAdgJ,GAAe7M,EAASsJ,OAEtBN,EADG5I,EAAQJ,EAAQI,OAASJ,MAExBgJ,KAAKM,EACTlJ,EAAM4I,GAAKM,EAAMN,GAQU,SAA7B8D,GAA6BC,UAAYA,EAASC,IAAI,SAAAvC,UAAWA,EAAQzK,UACvD,SAAlBiN,GAAmBC,EAAUH,EAAUI,UAAOD,GAAYH,EAAS3I,QAAU+I,EAAGC,IAAIF,EAASJ,GAA2BC,GAAWI,EAAI,IAAIE,GAAUN,EAAU,GAAG,IAAQ,GAuF5J,SAAdO,GAAeC,EAAgB5F,UAAS4F,aAA0BF,GAAYE,EAAiB,IAAIF,GAAUE,EAAgB5F,GACvG,SAAtB6F,GAAuBC,EAASC,EAAWC,OACtCC,EAAMH,EAAQI,SAASF,GAC1BG,EAAML,EAAQM,IAAIJ,UACZG,EAAI/B,YAAgB2B,EAAUM,gBAAgBF,EAAI9N,UAAY8N,GAAK/B,WAAc6B,EAAI7B,UAAmB6B,EAANE,EAGxF,SAAlBG,GAAkBC,MACbA,IAASC,EAAa,KACrBC,EAAItN,EAAMV,MACbiO,EAAIvN,EAAMwN,cAAgB9N,OAAO+N,WACjCC,EAAI1N,EAAM2N,eAAiBjO,OAAOkO,YAClClM,EAAI,KACD0L,IAASG,GAAKG,GAAI,MACdhM,KACNmM,EAAanM,GAAK4L,EAAEQ,EAAWpM,IAE5B6L,IACHD,EAAE5C,MAAQ1K,EAAMwN,YAAc,KAC9BF,EAAES,UAAY,UAEXL,IACHJ,EAAE3C,OAAS3K,EAAM2N,aAAe,KAChCL,EAAEU,UAAY,UAEfX,EAAaD,OACP,GAAIC,EAAa,MAChB3L,KACNmM,EAAanM,GAAM4L,EAAEQ,EAAWpM,IAAMmM,EAAanM,GAAM4L,EAAE5K,eAAeuF,EAAe6F,EAAWpM,KAErG2L,EAAcD,IAKP,SAAVa,GAAWrB,EAAWD,EAAS9F,EAAMqH,GACnCtB,aAAqBL,IAAaI,aAAmBJ,IAAclF,QAAQC,KAAK,iCAehFsC,EAAG1B,EAAGiG,EAASzM,EAAGqG,EAAIkB,EAAMe,EAAOpC,EAASyC,EAAa+D,EAAUC,EAAQC,EAAK9K,EAAGT,IAb9EwL,GADN1H,EAAOA,GAAQ,IACT0H,WAAYC,EAAkK3H,EAAlK2H,QAASC,EAAyJ5H,EAAzJ4H,QAASC,EAAgJ7H,EAAhJ6H,SAAUC,EAAsI9H,EAAtI8H,gBAAiBC,EAAqH/H,EAArH+H,OAAQC,EAA6GhI,EAA7GgI,MAAOC,EAAsGjI,EAAtGiI,OAAQC,EAA8FlI,EAA9FkI,OAAQC,EAAsFnI,EAAtFmI,YAAaC,EAAyEpI,EAAzEoI,KAAMC,EAAmErI,EAAnEqI,YAAaC,EAAsDtI,EAAtDsI,OAAQC,EAA8CvI,EAA9CuI,OAAQC,EAAsCxI,EAAtCwI,MAAOC,EAA+BzI,EAA/ByI,KAAMC,EAAyB1I,EAAzB0I,QAASC,EAAgB3I,EAAhB2I,KAAMC,EAAU5I,EAAV4I,MACzKjH,GAAS,UAAW3B,EAAOA,EAAO+F,GAAWpE,MAC7CkH,EAAYvH,EAAMtB,EAAM8I,IACxBC,EAAYC,EAAKC,SAAS,CAAEjB,MAAAA,EAAOC,OAAAA,EAAQC,OAAAA,EAAQC,YAAAA,EAAaC,KAAAA,EAAMc,KAAM,WAC5EC,EAAiBN,EACjBO,EAAW,GACXC,EAAU,GACVpH,EAAQ,GACRqH,EAAiB,GACjBC,GAAmB,IAATZ,EAAgB,EAAIA,GAAQ,EACtCa,EAA4B,mBAAVb,EAAuBA,EAAO,kBAAMY,GACtDE,EAAc1D,EAAU0D,aAAe3D,EAAQ2D,YAC/CC,EAAUX,EAAuB,IAAb1B,EAAiB,KAAO,YAGxChG,KAAKyE,EAAQI,SACjBsB,EAAU1B,EAAQM,IAAI/E,GAA2BwE,GAAoBC,EAASC,EAAW1E,GAA9DyE,EAAQI,SAAS7E,GAC5CH,EAAKsG,EAAOnP,QACZkP,EAAWxB,EAAUG,SAAS7E,IAC9B0E,EAAUK,IAAI/E,IAAMH,IAAOqG,EAASlP,UAAY0N,EAAUK,IAAI/E,GAAG+C,WAAcoD,EAAOpD,YAAemD,EAAWxB,EAAUK,IAAI/E,IAC1HkG,GACHnF,EAAO,CAACM,EAAGxB,EAAIhF,EAAGqL,EAAU5K,EAAG6K,EAAQtD,GAAIqD,EAASlP,UAAY6I,EAAK,EAAIsG,EAAOpD,UAAY,GAAK,GACjGnC,EAAMvF,KAAK0F,GACPA,EAAK8B,KACJ9B,EAAK8B,GAAK,IACb9B,EAAKlG,EAAIsL,EACTpF,EAAKzF,EAAI4K,GAGVkC,GAAe5G,GAAoBT,EAAKlG,EAAGyF,EAAQE,GAAqBF,GAASI,IACjF0G,GAAQxG,EAAMvF,KAAK0F,EAAKuH,KAAO,CAACjH,EAAG6E,EAASlP,QAAS6D,EAAGkG,EAAKlG,EAAGS,EAAGyF,EAAKzF,EAAGuH,IAAK9B,EAAK8B,GAAIyF,KAAMvH,KAEhGlB,EAAG0I,MAAQrC,EAASlP,QAAQuR,MAAQ5F,GAASA,GAAOiF,SAAWF,GACrDvB,EAAOpD,YACjBnC,EAAMvF,KAAK,CAACgG,EAAGxB,EAAIhF,EAAGoF,EAAMkG,EAAQ,CAACpD,UAAU,IAAKzH,EAAG6K,EAAQtD,GAAI,EAAGkF,SAAU,IAChFlI,EAAG0I,MAAQ5F,GAASA,GAAOiF,SAAWF,GAIxCpH,IAAUC,GAAeD,IAAUD,GAAcC,IAAQ5B,QAAQ,SAAAsB,UAAKwH,EAAUxH,GAAK,SAAAxG,UAAKoH,EAAMpH,GAAG8B,EAAEgF,MAAMN,MAC3GY,EAAMuB,YAAcA,EAAc,GAElCiE,EAAM,mBACLzF,GAAiBC,GACjBqE,IAAgB,GAEXzL,EAAI,EAAGA,EAAIoH,EAAMxF,OAAQ5B,IAC7BuH,EAAOH,EAAMpH,GACb8B,EAAIyF,EAAKzF,EACTT,EAAIkG,EAAKlG,GACL0M,GAAUjM,EAAEkN,YAAY3N,IAAOkG,EAAKgH,UAGvClI,EAAKkB,EAAKM,GACV4F,GAAYlG,EAAK8B,GAAK,IAAMrJ,IAAM8B,EAAEU,OAASoB,gBAAgByC,GAAI,GAAO,GAAO,IAC3EhF,EAAEkI,WAAazH,EAAEyH,WAChBhC,EAAK8B,GAAK,GACbf,EAAQ,IAAIsB,GAAavD,EAAIS,EAAOoE,EAAUpB,QAC9CE,GAAK1B,EAAOxG,EAAG6L,EAAO,EAAG,EAAGrF,GAC5BA,EAAM9F,OAASoB,gBAAgByC,GAAI,GAAO,GAAO,GACjDiC,EAAM/H,IAAMgH,EAAKlG,EAAEd,IACnBgH,EAAKzF,EAAIA,EAAIwG,EACbsF,IAASvH,EAAGzI,MAAMqR,QAAUL,EAAcvN,EAAE4N,QAAUnN,EAAEmN,SACxDpB,GAAWY,EAAe5M,KAAKwE,IACX,EAAVkB,EAAK8B,IAAUuE,IACzBvH,EAAGzI,MAAMqR,QAAUL,EAAc9M,EAAEmN,QAAU5N,EAAE4N,QAAU,KAE1DjF,GAAKlI,EAAGT,EAAGsM,EAAO7G,IAERzF,EAAEkI,YAAczH,EAAEyH,YACvBlI,EAAEkI,UAGKzH,EAAEyH,YACblI,EAAEd,IAAMuB,EAAEvB,IACViO,EAAQ3M,KAAKR,GACb+F,EAAM8H,OAAOlP,IAAK,GAClBgN,GAAYS,GAAUzD,GAAKlI,EAAGT,EAAGsM,EAAO7G,KANxChF,EAAEyH,WAAagF,EAAS1M,KAAKC,GAC7BsF,EAAM8H,OAAOlP,IAAK,KAQf2N,IACJtH,EAAGzI,MAAMuR,SAAWpJ,KAAKqJ,IAAItN,EAAEkH,MAAO3H,EAAE2H,OAAS,KACjD3C,EAAGzI,MAAMyR,UAAYtJ,KAAKqJ,IAAItN,EAAEmH,OAAQ5H,EAAE4H,QAAU,KACpD5C,EAAGzI,MAAM0R,SAAWvJ,KAAKwJ,IAAIzN,EAAEkH,MAAO3H,EAAE2H,OAAS,KACjD3C,EAAGzI,MAAM4R,UAAYzJ,KAAKwJ,IAAIzN,EAAEmH,OAAQ5H,EAAE4H,QAAU,MAErDwE,GAAUD,GAAenH,EAAGC,UAAUsE,IAAI4C,IAnC1CpG,EAAM8H,OAAOlP,IAAK,GAqCnB2I,EAAY9G,KAAKC,OAEd2N,KACAjC,IACHiC,EAAe9G,EAAY6B,IAAI,SAAAoB,UAAKA,EAAEpO,UACtCiQ,GAAUgC,EAAavK,QAAQ,SAAA1E,UAAKA,EAAE8F,UAAUoJ,OAAOlC,MAGxD/B,IAAgB,GAEZkC,GACHK,EAAU5J,OAAS,SAAApE,UAAKoH,EAAMpH,GAAG8B,EAAEsC,QACnC4J,EAAU3J,OAAS,SAAArE,UAAKoH,EAAMpH,GAAG8B,EAAEuC,UAEnC2J,EAAUhF,MAAQ,SAAAhJ,UAAKoH,EAAMpH,GAAG8B,EAAEkH,MAAQ,MAC1CgF,EAAU/E,OAAS,SAAAjJ,UAAKoH,EAAMpH,GAAG8B,EAAEmH,OAAS,MAC5C+E,EAAU2B,UAAYxK,EAAKwK,YAAa,GAEzC3B,EAAU3N,EAAI,SAAAL,UAAKoH,EAAMpH,GAAG8B,EAAEzB,EAAI,MAClC2N,EAAU1N,EAAI,SAAAN,UAAKoH,EAAMpH,GAAG8B,EAAExB,EAAI,MAClC0N,EAAU4B,SAAW,SAAA5P,UAAKoH,EAAMpH,GAAG8B,EAAE8N,UAAY9B,EAA0C,IAAnCa,EAAS3O,EAAGkG,EAAQlG,GAAIkG,GAAiB,IACjG8H,EAAU6B,MAAQ,SAAA7P,UAAKoH,EAAMpH,GAAG8B,EAAE+N,OAElC3J,EAAUkB,EAAMoD,IAAI,SAAAnI,UAAKA,EAAEwF,KAEvB6F,GAAqB,IAAXA,IACbM,EAAU8B,UAAY,CAACpC,OAAQ,yBAAMA,IACrCM,EAAUN,OAASA,EACnBM,EAAU+B,iBAA2C,IAAzB5K,EAAK4K,iBAGlCnC,IAASI,EAAUiB,QAAU,SAAAjP,UAAKoH,EAAMpH,GAAGqJ,GAAK,EAAI,EAAkB,EAAdjC,EAAMpH,GAAGqJ,GAASjC,EAAMpH,GAAG8B,EAAEmN,QAAU,QAE3FR,EAAe7M,OAAQ,CAC1BiM,EAAUM,EAAK6B,MAAMC,WAAWpC,OAC5BqC,EAAahK,EAAQiK,MAAM1B,EAAe7M,QAC9CoM,EAAUH,QAAU,SAAC7N,EAAGqG,UAAOwH,GAASY,EAAelG,QAAQlC,GAAMH,EAAQqC,QAAQnB,EAAMpH,GAAG8O,KAAKjH,GAAK7H,EAAGqG,EAAI6J,OAUhHE,GAAWlL,QAAQ,SAAAF,UAAQG,EAAKH,IAASkJ,EAAUmC,cAAcrL,EAAMG,EAAKH,GAAOG,EAAKH,EAAO,aAE3FkI,GAAUhH,EAAQtE,WAMhB4E,KALL8H,EAAiB7H,EAAMuH,EAAWC,IAC9B,UAAWf,IACdA,EAAO9I,OAAS8I,EAAO7I,OAAS6I,EAAOS,aAChCT,EAAOS,OAELT,GACThF,EAAIzB,EAAMyG,EAAO1G,GAAI8J,KACnB9J,GAAKwH,EAAUxH,KACf,aAAc0B,IAAO,aAAc8F,IAAe9F,EAAEqI,SAAWvC,EAAUuC,UAC3ErI,EAAE2F,QAAUG,EAAUH,QACtBgB,EAAQ2B,KAAKtC,EAAWhI,EAASgC,EAAG,UAC7BoG,EAAe9H,IAGpBN,EAAQtE,QAAU4M,EAAQ5M,QAAU2M,EAAS3M,UAChD4L,GAAeU,EAAUtD,IAAI,kBAAM3E,EAAawJ,EAAcjC,EAAaU,EAAUuC,OAAS,EAAI,SAAW,QAAQ,KAAOrD,GAAUnH,EAAawJ,EAAcjC,EAAa,OAC9KtH,EAAQtE,QAAUiN,EAAQ2B,KAAKtC,EAAWhI,EAASoI,EAAgB,IAGpE7D,GAAgBqC,EAASyB,EAAUL,GACnCzD,GAAgBsC,EAASyB,EAASN,OAE9BwC,EAAUvH,IAAUA,GAAOiF,SAE3BsC,IACHA,EAAQ9F,IAAIsD,EAAW,GACvB/E,GAAOG,OAAOzH,KAAK,kBAAM4G,GAAgBrB,GAAQyF,MAGlDJ,EAAUyB,EAAUqC,WACpBrC,EAAUsC,KAAK,eACVG,EAAUzC,EAAU0C,QAAUnE,EAClCkE,IAAYD,GAAWjI,GAAgBrB,GAAQyF,GAC/CW,GAAevH,EAAawJ,EAAcjC,EAAamD,EAAU,SAAW,UAI9E1D,IAAoBD,EAAW5F,EAAM+C,OAAO,SAAA5C,UAASA,EAAK8B,KAAO9B,EAAKzF,EAAEyH,WAAahC,EAAKlG,EAAEkI,YAAWiB,IAAI,SAAAjD,UAAQA,EAAKzF,EAAEtE,WACtH2L,IACH6D,MAAY7D,GAAOC,MAAKvH,aAAQqI,GAAa9C,EAAO4F,IACpD7D,GAAO0H,KAAKhP,KAAK+K,KAEjBI,GAAY5C,GAAmBF,GAAa9C,EAAO4F,IACnDJ,SAGGkE,EAAO3H,GAASA,GAAOiF,SAAWF,SACtC4C,EAAKC,OAAS,kBAAMC,GAAUF,EAAM,EAAG,IAEhCA,EAgBQ,SAAhBG,GAAgB3I,WAKdL,EAJGiJ,EAAS5I,EAAM+C,SAAW,GAC7BE,EAAMjD,EAAMiD,IAAM,GAClBhB,EAAWjC,EAAM6I,cACjBnR,EAAIuK,EAAS3I,OAEP5B,KAENkR,GADAjJ,EAAUsC,EAASvK,IACJmL,IAAOI,EAAItD,EAAQkD,IAAMlD,EAAYiJ,EAAOjJ,EAAQkD,IAAMlD,EAjgB5E,IACCvC,EAAUyI,EAAMhF,GAAQiI,EAAc9S,EAAO2L,EAAeoH,EA8QmB1F,IA/Q5E2F,EAAM,EAGTC,EAAe,GACfC,EAAW,IAAMzL,KAAK0L,GACtBC,EAAW3L,KAAK0L,GAAK,IACrB5H,EAAY,GACZzB,EAAoB,GACpBpB,GAAuB,GAEvBoJ,GAAahL,EAAa,6DAC1B8B,GAAe9B,EAAa,iHAI5B6I,GAAY,CAACP,OAAO,EAAGiE,KAAK,EAAG7H,OAAO,EAAGgE,KAAK,EAAGjB,WAAW,EAAG3G,QAAQ,EAAGsH,YAAY,EAAGoE,WAAW,EAAGC,SAAS,EAAGC,YAAY,EAAGC,QAAQ,EAAG5E,MAAM,EAAGE,OAAO,EAAGC,YAAY,EAAGC,KAAK,EAAGI,MAAM,EAAGC,KAAK,EAAGZ,SAAS,EAAGlG,MAAM,EAAGgG,QAAQ,EAAGC,QAAQ,EAAGG,OAAO,EAAGE,OAAO,EAAGK,OAAO,EAAGM,MAAM,EAAGd,gBAAiB,GAC3SqD,GAAe,CAAC5C,OAAO,EAAG5D,OAAO,EAAG+C,WAAW,EAAGc,MAAM,EAAGX,SAAS,EAAGgF,SAAS,EAAGC,QAAQ,EAAGnL,MAAM,GASpGC,GAAiB,GA2DjB0C,EAAiB,wEAAwEnE,MAAM,KAsE/F4M,EAAqB,SAArBA,mBAAsBC,EAAUrL,EAAOgD,EAAQsI,UAAUD,aAAoBvI,GAAeuI,EAAWA,aAAoBtH,GADrG,SAAtBwH,oBAAuB/J,EAAO8J,UAAWA,GAAS9J,EAAM+C,SAAS6G,EAAmBE,GAAOjH,KAAQ7C,EAAM6I,cAAc,GACgBkB,CAAoBF,EAAUC,GAAS,IAAIxI,GAAkC,iBAAduI,EAAyB3M,EAAO2M,IAAaxM,QAAQC,KAAKuM,EAAW,cAAgBA,EAAUrL,EAAOgD,IA0B5SE,GAAO,SAAPA,KAAQkB,EAAWD,EAAS0C,EAAO2E,EAAYN,EAAU7M,OAQvD0K,EAAO0C,EAAWC,EAAStJ,EAASuJ,EAAcjQ,EAAQkQ,EAPrDlV,EAAiC0N,EAAjC1N,QAASwG,EAAwBkH,EAAxBlH,MAAOrC,EAAiBuJ,EAAjBvJ,OAAQtB,EAAS6K,EAAT7K,EAAGC,EAAM4K,EAAN5K,EAC9B0I,EAAoDiC,EAApDjC,MAAOC,EAA6CgC,EAA7ChC,OAAQ7E,EAAqC6G,EAArC7G,OAAQC,EAA6B4G,EAA7B5G,OAAQuL,EAAqB3E,EAArB2E,SAAU7F,EAAWkB,EAAXlB,OAC3C4I,EAASxN,GAAQkM,GAAkBA,EAAe7T,EAAS,0BAC3DoV,EAAiB1H,IACRD,EAAQzI,OAAhBhC,IAAAA,EAAGuB,IAAAA,EACJ8Q,EAAO3H,EAAUnB,OAAOf,QAAUe,EAAOf,OAASkC,EAAUnB,OAAOd,SAAWc,EAAOd,QAAUiC,EAAU9G,SAAWA,GAAU8G,EAAU7G,SAAWA,GAAU6G,EAAU0E,WAAaA,EACpL9F,GAAU+I,GAAQ3H,EAAUpB,QAAUmB,EAAQnB,SAAWkI,SAEtDlI,IAAWnI,GACdyC,EAASC,EAAS,EAClBuL,EAAWC,EAAQ,IAGnBrN,GADAiQ,EAlKwB,SAA1BK,wBAA0BzM,OACrBrC,EAAQqC,EAAGpC,OAASkK,EAAK4E,KAAKC,SAAS3M,UACvCrC,EAAMiP,UAAY9E,EAAK+E,OAAOC,MAC1BnP,EAAMoP,SAEdpP,EAAMiP,QAAU9E,EAAK+E,OAAOC,MACpBnP,EAAMoP,QAAUxP,gBAAgByC,GAAI,GAAM,GAAO,IA4JzCyM,CAAwBnR,IACjBV,QAAQqB,SAAS2I,EAAQoI,IAAMpI,EAAQzI,OAAOvB,QAAQqB,SAAS2I,EAAQoI,KAAOpI,EAAQzI,QAC5GoN,EAAW/J,EAAOE,KAAKuN,MAAM9Q,EAAOnB,EAAGmB,EAAOV,GAAK0P,GACnD3B,EAAQhK,EAAOE,KAAKuN,MAAM9Q,EAAOH,EAAGG,EAAOR,GAAKwP,EAAW5B,GAAY,IACvExL,EAAS2B,KAAKwN,KAAKxN,SAAAvD,EAAOV,EAAK,YAAIU,EAAOnB,EAAK,IAC/CgD,EAAS0B,KAAKwN,KAAKxN,SAAAvD,EAAOH,EAAK,YAAIG,EAAOR,EAAK,IAAK+D,KAAKyN,IAAI3D,EAAQ6B,GACjEM,IACHA,EAAWtM,EAASsM,GAAU,GAC9B9I,EAAUiF,EAAKsF,YAAYzB,GAC3BU,EAAOV,EAAS/P,SAAwC,mBAAtB+P,EAAS/P,SAA2B+P,EAAS/P,UAC/E2Q,EAAiB,CAACxO,OAAQ8E,EAAQ,UAAW7E,OAAQ6E,EAAQ,UAAWF,MAAO0J,EAAOA,EAAK1J,MAAQjD,KAAK2N,KAAKC,WAAWzK,EAAQ,QAAS,QAASD,OAAQyJ,EAAOA,EAAKzJ,OAAS0K,WAAWzK,EAAQ,SAAU,SAE7MlF,EAAM4L,SAAWA,EAAW,MAC5B5L,EAAM6L,MAAQA,EAAQ,OAEnBlC,GACHvJ,GAAU4E,IAAU4J,EAAe5J,OAAU4J,EAAe5J,MAAYA,EAAQ4J,EAAe5J,MAA3B,EACpE3E,GAAU4E,IAAW2J,EAAe3J,QAAW2J,EAAe3J,OAAaA,EAAS2J,EAAe3J,OAA5B,EACvEjF,EAAMI,OAASA,EACfJ,EAAMK,OAASA,IAEf2E,EAAQiB,EAAcjB,EAAQ5E,EAASwO,EAAexO,OAAQ,GAC9D6E,EAASgB,EAAchB,EAAS5E,EAASuO,EAAevO,OAAQ,GAChE7G,EAAQI,MAAMoL,MAAQA,EAAQ,KAC9BxL,EAAQI,MAAMqL,OAASA,EAAS,MAMjCqJ,GAAcjI,GAAY7M,EAASyN,EAAQnE,OACvCgD,IAAWnI,GACdtB,GAAKG,EAAI0K,EAAU1I,OAAOhC,EAC1BF,GAAKyB,EAAImJ,EAAU1I,OAAOT,GAChB8Q,GAAQlR,IAAWsJ,EAAQtJ,QACrCqC,EAAMM,gBAAgB,EAAGN,GACzBxB,EAASoB,gBAAgBoO,GAAYxU,GAAS,GAAO,GAAO,GAC5D+U,EAAYE,EAAahP,MAAM,CAACpD,EAAGmC,EAAOhC,EAAGF,EAAGkC,EAAOT,IAEvD1B,IADAmS,EAAUC,EAAahP,MAAM,CAACpD,EAAGG,EAAGF,EAAGyB,KAC1B1B,EAAIkS,EAAUlS,EAC3BC,GAAKkS,EAAQlS,EAAIiS,EAAUjS,IAE3BmS,EAAajS,EAAIiS,EAAa1Q,EAAI,EAElC1B,IADAmS,EAAUC,EAAahP,MAAM,CAACpD,EAAGG,EAAI0K,EAAU1I,OAAOhC,EAAGF,EAAGyB,EAAImJ,EAAU1I,OAAOT,KACpE1B,EACbC,GAAKkS,EAAQlS,GAEdD,EAAI4J,EAAc5J,EAAG,KACrBC,EAAI2J,EAAc3J,EAAG,MACjB6E,GAAUA,aAAgByE,IAG7B5F,EAAM3D,EAAIA,EAAI,KACd2D,EAAM1D,EAAIA,EAAI,KACd0D,EAAMM,gBAAgB,EAAGN,IAJzB2O,GAAUA,EAAO5B,SAMd5L,IACHA,EAAK9E,EAAIA,EACT8E,EAAK7E,EAAIA,EACT6E,EAAKyK,SAAWA,EAChBzK,EAAK0K,MAAQA,EACTlC,GACHxI,EAAKf,OAASA,EACde,EAAKd,OAASA,IAEdc,EAAK6D,MAAQA,EACb7D,EAAK8D,OAASA,IAGT9D,GAAQnB,GAShBmI,EAAe,GAAIC,EAAa,mCAAmC9G,MAAM,KAgOzE0L,GAAY,SAAZA,UAAarG,EAAIvE,EAAQwN,MACpBjJ,GAAMA,EAAGkJ,WAAa,KAAOlJ,EAAGyC,UAAYwG,UAC3CxN,IANO,SAAb0N,WAAanJ,GACZA,EAAGxF,KAAK2M,aAAenH,EAAGxF,KAAK2M,YAAYrO,MAAMkH,EAAIA,EAAGxF,KAAK4O,mBAAqB,IAClFpJ,EAAGqJ,aAAY,GAAM,GAAO,GAAM9O,QAAQ4O,YAKxCA,CAAWnJ,GACXvE,EAAS,GAAKuE,EAAGkJ,SAAS,GAC1BlJ,EAAGgH,SAEG,GAoBJ9G,4BAiBLoJ,OAAA,gBAAOC,0BACD/C,cAAgBlO,KAAKiD,QAAQsE,IAAI,SAAAnE,UAAM,IAAIuD,GAAavD,EAAI8N,EAAKrN,MAAOqN,EAAKrK,UAClFmH,GAAchO,WACTmR,UAAUF,QACVG,qBACEpR,QAGRqR,MAAA,6BACMpO,QAAQtE,OAASqB,KAAKkO,cAAcvP,OAAS,EAClDqP,GAAchO,MACPA,QAGRsR,IAAA,aAAIjM,EAAOqF,EAAOF,WAIhBf,EAAUC,EAHP6H,EAAkBrN,GAAiBlE,KAAKkO,cAAchB,MAAM,IAAI,GAAO,GAC1EsE,GAAcnM,GAASrF,MAAMoI,SAC7BrL,EAAI,EAEEA,EAAIwU,EAAgB5S,OAAQ5B,IAClC0M,EAAW8H,EAAgBxU,GAC3ByN,IAAWf,EAASlK,OAASoB,gBAAgB8I,EAASlP,SAAS,GAAO,GAAO,KAC7EmP,EAAS8H,EAAW/H,EAASvB,MACnBnB,GAAK0C,EAAUC,EAAQgB,GAAO,EAAM,EAAGjB,GACjDA,EAASlK,OAASoB,gBAAgB8I,EAASlP,SAAS,GAAO,GAAO,UAE5DyF,QAGRwQ,YAAA,qBAAYjW,EAASkX,OAChBC,EAAK1R,KAAKuI,gBAAgBhO,IAAYqM,SAClC6K,KAAYC,EAAKA,EAAKA,EAAG7N,OAAS+C,GAAW6K,MAGtD9J,IAAA,aAAItC,WAIFsM,EAAOD,EAAIE,EAHR7U,EAAIsI,EAAMpC,QAAQtE,OACrBsP,EAASjO,KAAKoI,SACdE,EAAMtI,KAAKsI,IAELvL,MAEN6U,EAAM3D,GADNyD,EAAKrM,EAAM6I,cAAcnR,IACTmL,OACJwJ,EAAGnX,UAAYqX,EAAIrX,SAAY+N,EAAIoJ,EAAGxJ,KAAOI,EAAIoJ,EAAGxJ,IAAI3N,UAAYmX,EAAGnX,UAClFoX,EAAQ3R,KAAKkO,cAAc5I,QAAQoM,EAAGnX,UAAYqX,EAAIrX,QAAUqX,EAAMtJ,EAAIoJ,EAAGxJ,UACxEjF,QAAQgJ,OAAO0F,EAAO,EAAGtM,EAAMpC,QAAQlG,SACvCmR,cAAcjC,OAAO0F,EAAO,EAAGD,UAE/BzO,QAAQrE,KAAKyG,EAAMpC,QAAQlG,SAC3BmR,cAActP,KAAK8S,WAG1BrM,EAAMsG,cAAgB3L,KAAK2L,aAAc,GACzCtG,EAAMwB,SAAW7G,KAAK6G,QAAS,GAC/BmH,GAAchO,MACPA,QAGR6R,QAAA,iBAAQxM,GAUE,SAARyM,GAASC,EAAIC,EAAI5O,UAAQ2O,EAAGzL,YAAc0L,EAAG1L,UAAayL,EAAGzL,UAAY2L,EAAQC,EAASH,EAAGzL,UAAY6L,EAAUC,GAAWxT,KAAKwE,IAAOH,EAAQrE,KAAKwE,GACjI,SAAtBiP,GAAuBN,EAAIC,EAAI5O,UAAOH,EAAQqC,QAAQlC,GAAM,GAAK0O,GAAMC,EAAIC,EAAI5O,OAC/E2O,EAAIC,EAAIzO,EAAGH,EAAIkP,EAAOC,EAAOzN,EAAI1E,EAX9BoS,EAAKnN,EAAM+C,SACdqK,EAAKzS,KAAKoI,SACVgK,EAAY,GACZD,EAAU,GACVF,EAAQ,GACRC,EAAQ,GACRjP,EAAU,GACVyP,EAAKrN,EAAMiD,IACXpI,EAAKF,KAAKsI,QAIN/E,KAAKiP,EACTF,EAAQI,EAAGnP,GACXgP,EAAQrS,EAAGqD,GAEXH,GADA2O,EAAMO,EAAgBvK,GAAoB1C,EAAOrF,KAAMuD,GAAzCiP,EAAGjP,IACThJ,QACRyX,EAAKS,EAAGlP,GACJgP,GACHnS,EAAK4R,EAAG1L,YAAeiM,EAAMjM,WAAalD,IAAO4O,EAAGzX,QAAWyX,EAAKO,GACpEzN,GAAKwN,GAAUP,EAAGzL,WAAcgM,EAAMhM,WAAalG,EAAG7F,UAAY+X,EAAM/X,QAAkBwX,EAARO,GAE3EhM,WAAalG,EAAGkG,WAAaxB,EAAGvK,UAAY6F,EAAG7F,UACpDuK,EAAGiH,YAAY3L,GAAM+R,EAAUC,GAAWxT,KAAKkG,EAAGvK,QAAS6F,EAAG7F,SAC/D0I,EAAQrE,KAAKkG,EAAGvK,QAAS6F,EAAG7F,UAE5BuX,GAAMhN,EAAI1E,EAAI0E,EAAGvK,SAElB+X,GAASxN,EAAGvK,UAAY+X,EAAM/X,UAAY+X,EAAQE,EAAGjP,IACrD8O,GAAoBvN,EAAGvK,UAAYyX,EAAGzX,SAAW+X,EAAQA,EAAQxN,EAAIkN,EAAIA,EAAGzX,SAC5E8X,GAAoBC,GAASA,EAAM/X,UAAYgY,EAAMhY,QAAU+X,EAAQxN,EAAIyN,EAAOA,EAAMhY,SACxF+X,GAASD,GAAoBC,EAAOC,EAAMhY,UAAY+X,EAAM/X,QAAUgY,EAAQP,EAAIM,EAAM/X,WAEvFyX,EAAuBA,EAAGjG,YAAYgG,GAA2BD,GAAMC,EAAIC,EAAI5O,GAAnCgP,EAAUxT,KAAKwE,GAAtD6O,EAAMrT,KAAKwE,GACjBkP,GAASD,GAAoBC,EAAON,EAAIM,EAAM/X,cAG3CgJ,KAAKkP,EACJD,EAAGjP,KACP2O,EAAMtT,KAAK6T,EAAGlP,GAAGhJ,SACjB2F,EAAGqD,IAAM2O,EAAMtT,KAAKsB,EAAGqD,GAAGhJ,gBAGrB,CAAC4X,QAAAA,EAASC,UAAAA,EAAWH,MAAAA,EAAOC,MAAAA,MAGpCd,mBAAA,sCACKvN,EAAQE,GAAqB/D,KAAK6D,QAAUI,GAC/ClH,EAAIiD,KAAKkO,cAAcvP,OACjB5B,KACNgI,GAAoB/E,KAAKkO,cAAcnR,GAAI8G,MAI7CsN,UAAA,mBAAUF,cACL0B,EAAY,QACX1P,QAAQhB,QAAQ,SAAA2C,OAChB8C,EAAK9C,EAAEkH,MACV8G,EAAkB7E,GAAUrG,EAAIuJ,EAAO,EAAI,GAC5CA,GAAQ2B,GAAmBD,EAAUrN,QAAQoC,GAAM,GAAKA,EAAGC,IAAI,kBAAMkL,EAAKC,qBAC1EF,GAAmBD,EAAU/T,KAAK8I,MAElCuJ,GAAQ0B,EAAUhU,QAAUqB,KAAK8S,wBAC7BnH,cAAgB3L,KAAK2L,cAAgBgH,EAAUhU,WAGrDmU,iBAAA,iCACM5E,cAAcjM,QAAQ,SAAAyP,OACtBtT,EAAIsT,EAAGnX,QAAQiH,wBACnBkQ,EAAGpL,aAAelI,EAAE2H,OAAS3H,EAAE4H,QAAU5H,EAAEwB,KAAOxB,EAAEyB,MACpD6R,EAAGzQ,QAAU,OAIfsH,gBAAA,yBAAgBhO,UACRyF,KAAKkO,cAAclO,KAAKiD,QAAQqC,QAAQ/C,EAAOhI,QAGvDwY,aAAA,+BACQ7O,GAAiBlE,KAAKkO,cAAchB,MAAM,IAAI,GAAM,GAAM3F,IAAI5B,mCAxJ1D1C,EAASf,EAAM8Q,WACrBnP,MAAQ3B,GAAQA,EAAK2B,WACrBgD,UAAY3E,IAAQA,EAAK2E,QAC1BmM,OACE/P,QAAUoE,GAA2BpE,QACrCiL,cAAgBjL,EACrB+K,GAAchO,UACR,MACDiD,QAAUR,EAASQ,OACpBgO,EAAO/O,KAAuB,IAAdA,EAAKwM,MAAmBxM,EAAKJ,QAAUI,EAAKwM,MAChExI,KAAW+K,GAAQ/K,GAAO+M,MAAMrU,KAAKoB,WAChCgR,OAAOC,KAAU/K,WAoJnBS,+BAOLoF,YAAA,qBAAY1G,OACP9D,EAAKvB,KAAK8G,OACb3G,EAAKkF,EAAMyB,cACLvF,EAAG3B,MAAQO,EAAGP,KAAO2B,EAAG1B,OAASM,EAAGN,MAAQ0B,EAAGwE,QAAU5F,EAAG4F,OAASxE,EAAGyE,SAAW7F,EAAG6F,SAAWhG,KAAKT,OAAOgB,OAAO8E,EAAM9F,SAAWS,KAAKgM,UAAY3G,EAAM2G,SAAYhM,KAAK6D,OAASwB,EAAMxB,OAASqP,KAAKC,UAAUnT,KAAK6D,SAAWqP,KAAKC,UAAU9N,EAAMxB,UAGjQmN,OAAA,gBAAOnN,EAAOgD,OACTuM,EAAOpT,KACVzF,EAAU6Y,EAAK7Y,QACf0L,EAAUiF,EAAKsF,YAAYjW,GAC3BwG,EAAQmK,EAAK4E,KAAKC,SAASxV,GAC3BuM,EAASvM,EAAQiH,wBACjBiO,EAAOlV,EAAQyE,SAAuC,mBAArBzE,EAAQyE,SAA8D,QAAnCzE,EAAQ8Y,SAASxW,eAA2BtC,EAAQyE,UACxHnB,EAAIgJ,EAAS,IAAI5L,EAAS,EAAG,EAAG,EAAG,EAAG6L,EAAOjH,KAAOtD,IAAqBuK,EAAOlH,IAAMxD,KAAsBuE,gBAAgBpG,GAAS,GAAO,GAAO,GACpJ6Y,EAAKnN,QAAUA,EACfmN,EAAK7Y,QAAUA,EACf6Y,EAAKlL,GAthBG,SAAToL,OAASlQ,OACJ8E,EAAK9E,EAAGlG,aAAa,uBACzBgL,GAAM9E,EAAGpH,aAAa,eAAiBkM,EAAK,QAAUmG,KAC/CnG,EAmhBGoL,CAAO/Y,GACjB6Y,EAAK7T,OAAS1B,EACduV,EAAKrS,MAAQA,EACbqS,EAAKtM,OAASA,EACdsM,EAAK9M,aAAeQ,EAAOf,OAASe,EAAOd,QAAUc,EAAOjH,MAAQiH,EAAOlH,KAC3EwT,EAAK7M,QAAUN,EAAQ,WACvBmN,EAAKzT,SAAWsG,EAAQ,YACxBmN,EAAK1U,OAASnE,EAAQM,WACtBuY,EAAKhW,EAAI6I,EAAQ,KACjBmN,EAAK/V,EAAI4I,EAAQ,KACjBmN,EAAKjS,OAASJ,EAAMI,OACpBiS,EAAKhS,OAASL,EAAMK,OACpBgS,EAAKzG,SAAW1G,EAAQ,YACxBmN,EAAKxG,MAAQ3G,EAAQ,SACrBmN,EAAKpH,QAAU/F,EAAQ,WACvBmN,EAAKrN,MAAS0J,EAAOA,EAAK1J,MAAQiB,EAAcf,EAAQ,QAAS,MAAO,KACxEmN,EAAKpN,OAASyJ,EAAOA,EAAKzJ,OAASgB,EAAcf,EAAQ,SAAU,MAAO,KAC1EpC,GAxjBc,SAAf0P,aAAgBvO,EAASnB,WACpBoC,EAAUiF,EAAKsF,YAAYxL,EAAQzK,QAAS,KAAM,UACrDkJ,EAAMuB,EAAQnB,MAAQ,GACtB9G,EAAI8G,EAAMlF,OACJ5B,KACN0G,EAAII,EAAM9G,KAAOkJ,EAAQpC,EAAM9G,IAAM,IAAIyW,OAE1C/P,EAAIgH,SAAWhH,EAAIgH,OAASiG,WAAWjN,EAAIgH,SAAW,GAijB7C8I,CAAaH,EAAMtP,GAAeD,IAAUD,GAAcC,IACnEuP,EAAKhD,IAAM7V,EAAQuD,QAA6C,QAAnCvD,EAAQ8Y,SAASxW,eAA2Be,EAAQrD,GAASwF,UAC1FqT,EAAKvM,OAASA,GAA2B,IAAhBjE,EAAO/E,EAAEgB,KAAa+D,EAAO/E,EAAEO,KAAOwE,EAAO/E,EAAEuB,IAAsB,IAAhBwD,EAAO/E,EAAEkB,GACvFqU,EAAKnS,QAAU,uCAzCJ1G,EAASsJ,EAAOgD,QACtBtM,QAAUA,OACVyW,OAAOnN,EAAOgD,SA4Cf4M,4BAQLC,aAAA,sBAAaxL,WACRnL,EAAIiD,KAAK2T,OAAOhV,OACb5B,QACFiD,KAAK2T,OAAO5W,GAAGqL,SAASF,UACpBlI,KAAK2T,OAAO5W,MAKtB2R,KAAA,qBACM5M,MAAM2K,OAAOzM,uCAjBPkC,EAAMJ,QACZI,KAAOA,OACPJ,MAAQA,OACR6R,OAAS,QACTxI,SAAWrJ,EAAMqJ,eAiBlByI,2BAaLjM,IAAA,aAAIkM,OACClQ,EAAS3D,KAAKgC,QAAQkF,OAAO,SAAA/D,UAAUA,EAAOjB,OAAS2R,WACvDlQ,EAAOhF,OACHgF,EAAO,IAEfA,EAAS,IAAI8P,EAA8B,mBAAZI,EAAyB,CAACC,QAASD,GAAUA,EAAQ7T,WAC/EgC,QAAQpD,KAAK+E,GACXA,MAGR8I,OAAA,gBAAOtJ,OACFpG,EAAIiD,KAAKgC,QAAQsD,QAAQnC,UACxB,GAALpG,GAAUiD,KAAKgC,QAAQiK,OAAOlP,EAAG,GAC1BiD,QAGR+T,SAAA,kBAASC,cACJC,EAAY/N,GACfgO,EAAa/F,SACdjI,GAASlG,MACJqF,MAAMgM,aACN4B,MAAMtU,OAAS,OACfqD,QAAQC,QAAQ,SAAAkB,GAChBA,EAAOjB,KAAK6R,WACf5Q,EAAOwQ,OAAOhV,OAAS,GACvBwP,EAAehL,GACRkC,MAAQlC,EAAOjB,KAAK6R,SAAS5Q,IAErC6Q,GAAS7Q,EAAOwQ,OAAO1R,QAAQ,SAAA0G,UAAKwL,EAAK9O,MAAMsC,IAAIgB,OAEpDwF,EAAe+F,EACfhO,GAAS+N,OACJG,gBACEpU,QAGR8T,QAAA,uBAIEpO,EAAa8D,SAHVyK,EAAY/N,GACfwB,EAAK1H,KAAKmL,SACVpO,EAAIiD,KAAKgC,QAAQrD,WAElBuH,GAASlG,KACT0H,EAAG2J,aACElL,KAAKxH,OAASqB,KAAKqG,OAAO1H,OAASqB,KAAK4N,KAAKjP,OAAS,OACtDqD,QAAQC,QAAQ,SAAApD,GACpBA,EAAEqD,KAAK4R,SAAWjV,EAAEqD,KAAK4R,QAAQjV,OAGX8J,EAAGhF,EAFrBkG,EAAUhL,EAAEqD,KAAK2H,QACpBC,EAAUjL,EAAEqD,KAAK4H,QACjB7G,EAAUpE,EAAEoE,QACTA,GAAWA,EAAQtE,SAAWkL,GAAWC,KAC5CnB,EAAI,IAAIf,GACR/I,EAAE8U,OAAO1R,QAAQ,SAAAoD,UAASsD,EAAEhB,IAAItC,MAChC1B,EAASgF,EAAEkJ,QAAQwC,GAAKN,SAAS9Q,KAC1BgP,MAAMtT,QAAUkL,GAAWA,EAAQlG,EAAOsO,OACjDtO,EAAOuO,MAAMvT,QAAUmL,GAAWA,EAAQnG,EAAOuO,UAGnD/K,GAAmBnH,KAAKmG,WACnByH,KAAK3L,QAAQ,SAAAnD,UAAKA,MACvB0K,EAAU9B,EAAG4F,WACb5H,EAAc1F,KAAKqG,OAAO6G,MAAM,GAChCxF,EAAGC,IAAI,WACF6B,GAAW9B,EAAGiG,SACjBjI,EAAYzD,QAAQ,SAAAnD,UAAKA,MACzB+C,EAAcyS,EAAM,iBAGtBpO,GAAS+N,EACFlX,UACDiF,QAAQjF,GAAGmF,KAAKqS,MAAQvU,KAAKgC,QAAQjF,GAAG2R,cAE9C7M,EAAc7B,KAAM,WACpB0H,EAAG8M,UACIxU,QAGRyU,UAAA,mBAAUC,GACAA,EAATA,GAAgB,uBAAM,OAClBC,EAAQ,eACP3S,QAAQC,QAAQ,SAAA7C,MAChBA,EAAE8C,KAAKuS,UAAW,KACjB1X,EAAG+B,EAAI,SAAJA,EAAImE,GACVA,IAAY7D,EAAE6D,QAAUA,KACxBlG,EAAI4X,EAAMrP,QAAQxG,MAEjB6V,EAAM1I,OAAOlP,EAAG,GAChB4X,EAAMhW,QAAU+V,MAGlBC,EAAM/V,KAAKE,GACXM,EAAE8C,KAAKuS,UAAU3V,MAGnB6V,EAAMhW,QAAU+V,IACT1U,QAGR4U,SAAA,gCACM5S,QAAQC,QAAQ,SAAA7C,UAAKA,EAAE6D,QAAU7D,EAAE8C,KAAK0S,UAAYxV,EAAE8C,KAAK0S,SAASxV,KAClEY,QAGRoU,cAAA,uBAAcnD,eACR5L,MAAM8L,UAAUF,QAChBgC,MAAMhR,QAAQ,SAAAoD,UAASA,EAAM8L,UAAUF,KACrCjR,QAGR2J,IAAA,aAAIkL,EAAcb,qBACbhU,OAASkG,KACZ2O,GAAgB7U,KAAK+T,SAASC,QACzBS,UAAU,WACTK,EAAKC,UACTD,EAAKF,WACLE,EAAKhB,cAID9T,QAGRqR,MAAA,eAAM2D,QACA3P,MAAMgM,QACX2D,IAAchV,KAAKgC,QAAQrD,OAAS,MAGrC+U,aAAA,sBAAaxL,WAEXS,EADG5L,EAAIiD,KAAKgC,QAAQrD,OAEd5B,QACN4L,EAAI3I,KAAKgC,QAAQjF,GAAG2W,aAAaxL,UAEzBS,SAGF3I,KAAKqF,MAAM+C,SAASF,IAAOlI,KAAKqF,SAGxCqJ,KAAA,qBACMqG,QAAU,OACV1D,eACE/C,EAAatO,KAAKkI,mCAzJdA,QACNA,GAAKA,OACLlG,QAAU,QACViR,MAAQ,QACR5M,OAAS,QACTF,KAAO,QACPyH,KAAO,QACPxC,KAAO,QACP/F,MAAQ,IAAIuC,QACZuD,SAAWD,EAAKC,eAqJVkJ,SAELN,SAAP,kBAAgB9Q,EAASf,OACpBmD,EAAQwC,GAAY5E,EAASf,UACjCiM,GAAgBA,EAAawF,OAAO/U,KAAKyG,GACzCnD,GAAQA,EAAKJ,OAASuS,KAAKvS,MAAMI,EAAKJ,OAAOuD,MAAMsC,IAAItC,GAChDA,QAGD4P,KAAP,cAAY5P,EAAOnD,wBAClBA,EAAOA,GAAQ,MACYA,EAAK0H,YAAa,GACtCN,GAAQjE,EAAOwC,GAAY3F,EAAKe,SAAWoC,EAAMpC,QAAS,CAACY,MAAO3B,EAAK2B,OAASwB,EAAMxB,MAAOgD,OAAQ3E,EAAK2E,OAAQ6H,OAAQxM,EAAKwM,OAAQxM,GAAO,SAG/IgT,GAAP,YAAU7P,EAAOnD,UACToH,GAAQjE,EAAOwC,GAAY3F,EAAKe,SAAWoC,EAAMpC,QAAS,CAACY,MAAO3B,EAAK2B,OAASwB,EAAMxB,MAAOgD,OAAQ3E,EAAK2E,OAAQ6H,OAAQxM,EAAKwM,OAAQxM,EAAM,SAG9IiT,OAAP,gBAAclN,EAAWD,EAAS9F,UAC1BoH,GAAQrB,EAAWD,EAAS9F,SAG7BoP,IAAP,aAAW8D,EAAQC,EAAMnT,OACpB+C,EAAI/C,EAAOsB,EAAMtB,EAAMmL,IAAgB,KAC4BnL,GAAQ+C,EAA7E8E,IAAAA,SAAUW,IAAAA,MAAOsE,IAAAA,QAASnL,IAAAA,MAAOyR,IAAAA,aAAc3G,IAAAA,WAAY9H,IAAAA,OAC5DkI,EAAW7M,GAAQA,EAAK6M,UAAYxM,EAAOL,EAAK6M,UAChDwG,EAAStG,EAAmBoG,EAAMxR,EAAOgD,EAAQuO,GACjDI,EAAQvG,EAAmBmG,EAAQ,EAAGvO,EAAQ0O,GAC9CE,EAAc5R,EAAQE,GAAqBF,GAASI,GACpDyR,EAAMxK,EAAKyK,iBACZ9R,GAASuD,GAAYnC,EAAGsQ,EAAO1R,OAC/BkB,GAAoByQ,EAAOC,GACvBH,wBACmBrQ,IAAOA,EAAE6H,iBAAkB,GACjD7H,EAAE0J,WAAa,WACdvJ,GAAmBoQ,GACnB7G,GAAcA,EAAWnO,MAAMR,KAAM4V,aAGvC7L,GAAYpE,GAAc6P,EAAOD,GACjCtQ,EAAI8B,GAAKyO,EAAOD,EAAQ7K,GAASqE,EAAUlL,EAAOkL,EAAU9J,EAAEqI,UAAY0B,EAAU/J,EAAI,GACvE,iBAAV/C,GAAsB,WAAYA,IAAS+C,EAAEwF,OAASvI,EAAKuI,QAClEiL,IAAQ1G,GAAW0G,EAAI/N,IAAI,kBAAM,kBAAMvC,GAAmBoQ,MACnDxG,EAAU/J,EAAIA,EAAEqI,SAAWpC,EAAKgK,GAAGM,EAAMjb,QAAS0K,GAAK,WAGxD8N,aAAP,sBAAoB8C,EAAiB3T,UAC5B2T,aAA2BjO,GAAYiO,EAAkB,IAAIjO,GAAUiO,EAAiB3T,IAAO6Q,qBAGjGjR,MAAP,eAAaoG,UAELoG,EADApG,EAAPA,GAAY,aACgBoG,EAAapG,GAAM,IAAI0L,EAAU1L,UAGvD4N,YAAP,qBAAmB7S,EAAS8S,IAC1B9S,aAAmB2E,GAAY3E,EAAQA,QAAUR,EAASQ,IAAUhB,QAAQ,SAAA2C,UAAKA,GAAKmJ,GAAUnJ,EAAEkH,OAAoB,IAAbiK,EAAqB,EAAI,WAG7HC,WAAP,oBAAkBxT,OACb1D,EAAIuV,KAAK4B,YAAYzT,WAChB1D,GAAKA,EAAEoX,iBAGVD,YAAP,qBAAmBzT,UACVD,EAAOC,IAAWoE,GAAWkF,YAG/BvD,gBAAP,yBAAuB/F,EAAQqB,UACvB,IAAI8C,GAAapE,EAAOC,GAASqB,SAGlCsS,mBAAP,4BAA0BC,EAAaC,EAAW5V,OAC7C5C,EAAI8C,gBAAgB0V,GAAW,GAAM,GAAMhX,SAASsB,gBAAgByV,WACjE3V,EAAQ5C,EAAE2C,MAAMC,GAAS5C,QAI1ByY,SAAP,kBAAgBxG,MACfzU,EAA6B,oBAAdkb,UAA6BA,SAASjb,KAC1C,CACV4P,EAAO4E,EACPxV,EAAQe,GACRoH,EAAWyI,EAAK6B,MAAMyJ,QACtBpI,EAAiBlD,EAAK4E,KAAK2G,kBACvBC,EAAOxL,EAAK6B,MAAM2J,KAAK,IAC3B1P,EAAgB,uBAACnE,EAAO8E,UAAQ+O,EAAKhG,WAAW7N,GAAS8E,6BAK5D0M,GAAKsC,QAAU,SAaI,oBAAZ5b,QAA2BA,OAAOmQ,MAAQnQ,OAAOmQ,KAAK0L,eAAevC"} \ No newline at end of file diff --git a/node_modules/gsap/dist/MotionPathPlugin.js b/node_modules/gsap/dist/MotionPathPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..a3c1c3537206f28762760f394e0adfc9302c2a08 --- /dev/null +++ b/node_modules/gsap/dist/MotionPathPlugin.js @@ -0,0 +1,1809 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + var _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _numbersExp = /(?:(-)?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + _selectorExp = /(^[#\.][a-z]|[a-y][a-z])/i, + _DEG2RAD = Math.PI / 180, + _RAD2DEG = 180 / Math.PI, + _sin = Math.sin, + _cos = Math.cos, + _abs = Math.abs, + _sqrt = Math.sqrt, + _atan2 = Math.atan2, + _largeNum = 1e8, + _isString = function _isString(value) { + return typeof value === "string"; + }, + _isNumber = function _isNumber(value) { + return typeof value === "number"; + }, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; + }, + _temp = {}, + _temp2 = {}, + _roundingNum = 1e5, + _wrapProgress = function _wrapProgress(progress) { + return Math.round((progress + _largeNum) % 1 * _roundingNum) / _roundingNum || (progress < 0 ? 0 : 1); + }, + _round = function _round(value) { + return Math.round(value * _roundingNum) / _roundingNum || 0; + }, + _roundPrecise = function _roundPrecise(value) { + return Math.round(value * 1e10) / 1e10 || 0; + }, + _splitSegment = function _splitSegment(rawPath, segIndex, i, t) { + var segment = rawPath[segIndex], + shift = t === 1 ? 6 : subdivideSegment(segment, i, t); + + if ((shift || !t) && shift + i + 2 < segment.length) { + rawPath.splice(segIndex, 0, segment.slice(0, i + shift + 2)); + segment.splice(0, i + shift); + return 1; + } + }, + _getSampleIndex = function _getSampleIndex(samples, length, progress) { + var l = samples.length, + i = ~~(progress * l); + + if (samples[i] > length) { + while (--i && samples[i] > length) {} + + i < 0 && (i = 0); + } else { + while (samples[++i] < length && i < l) {} + } + + return i < l ? i : l - 1; + }, + _reverseRawPath = function _reverseRawPath(rawPath, skipOuter) { + var i = rawPath.length; + skipOuter || rawPath.reverse(); + + while (i--) { + rawPath[i].reversed || reverseSegment(rawPath[i]); + } + }, + _copyMetaData = function _copyMetaData(source, copy) { + copy.totalLength = source.totalLength; + + if (source.samples) { + copy.samples = source.samples.slice(0); + copy.lookup = source.lookup.slice(0); + copy.minLength = source.minLength; + copy.resolution = source.resolution; + } else if (source.totalPoints) { + copy.totalPoints = source.totalPoints; + } + + return copy; + }, + _appendOrMerge = function _appendOrMerge(rawPath, segment) { + var index = rawPath.length, + prevSeg = rawPath[index - 1] || [], + l = prevSeg.length; + + if (index && segment[0] === prevSeg[l - 2] && segment[1] === prevSeg[l - 1]) { + segment = prevSeg.concat(segment.slice(2)); + index--; + } + + rawPath[index] = segment; + }; + + function getRawPath(value) { + value = _isString(value) && _selectorExp.test(value) ? document.querySelector(value) || value : value; + var e = value.getAttribute ? value : 0, + rawPath; + + if (e && (value = value.getAttribute("d"))) { + if (!e._gsPath) { + e._gsPath = {}; + } + + rawPath = e._gsPath[value]; + return rawPath && !rawPath._dirty ? rawPath : e._gsPath[value] = stringToRawPath(value); + } + + return !value ? console.warn("Expecting a <path> element or an SVG path data string") : _isString(value) ? stringToRawPath(value) : _isNumber(value[0]) ? [value] : value; + } + function copyRawPath(rawPath) { + var a = [], + i = 0; + + for (; i < rawPath.length; i++) { + a[i] = _copyMetaData(rawPath[i], rawPath[i].slice(0)); + } + + return _copyMetaData(rawPath, a); + } + function reverseSegment(segment) { + var i = 0, + y; + segment.reverse(); + + for (; i < segment.length; i += 2) { + y = segment[i]; + segment[i] = segment[i + 1]; + segment[i + 1] = y; + } + + segment.reversed = !segment.reversed; + } + + var _createPath = function _createPath(e, ignore) { + var path = document.createElementNS("http://www.w3.org/2000/svg", "path"), + attr = [].slice.call(e.attributes), + i = attr.length, + name; + ignore = "," + ignore + ","; + + while (--i > -1) { + name = attr[i].nodeName.toLowerCase(); + + if (ignore.indexOf("," + name + ",") < 0) { + path.setAttributeNS(null, name, attr[i].nodeValue); + } + } + + return path; + }, + _typeAttrs = { + rect: "rx,ry,x,y,width,height", + circle: "r,cx,cy", + ellipse: "rx,ry,cx,cy", + line: "x1,x2,y1,y2" + }, + _attrToObj = function _attrToObj(e, attrs) { + var props = attrs ? attrs.split(",") : [], + obj = {}, + i = props.length; + + while (--i > -1) { + obj[props[i]] = +e.getAttribute(props[i]) || 0; + } + + return obj; + }; + + function convertToPath(element, swap) { + var type = element.tagName.toLowerCase(), + circ = 0.552284749831, + data, + x, + y, + r, + ry, + path, + rcirc, + rycirc, + points, + w, + h, + x2, + x3, + x4, + x5, + x6, + y2, + y3, + y4, + y5, + y6, + attr; + + if (type === "path" || !element.getBBox) { + return element; + } + + path = _createPath(element, "x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points"); + attr = _attrToObj(element, _typeAttrs[type]); + + if (type === "rect") { + r = attr.rx; + ry = attr.ry || r; + x = attr.x; + y = attr.y; + w = attr.width - r * 2; + h = attr.height - ry * 2; + + if (r || ry) { + x2 = x + r * (1 - circ); + x3 = x + r; + x4 = x3 + w; + x5 = x4 + r * circ; + x6 = x4 + r; + y2 = y + ry * (1 - circ); + y3 = y + ry; + y4 = y3 + h; + y5 = y4 + ry * circ; + y6 = y4 + ry; + data = "M" + x6 + "," + y3 + " V" + y4 + " C" + [x6, y5, x5, y6, x4, y6, x4 - (x4 - x3) / 3, y6, x3 + (x4 - x3) / 3, y6, x3, y6, x2, y6, x, y5, x, y4, x, y4 - (y4 - y3) / 3, x, y3 + (y4 - y3) / 3, x, y3, x, y2, x2, y, x3, y, x3 + (x4 - x3) / 3, y, x4 - (x4 - x3) / 3, y, x4, y, x5, y, x6, y2, x6, y3].join(",") + "z"; + } else { + data = "M" + (x + w) + "," + y + " v" + h + " h" + -w + " v" + -h + " h" + w + "z"; + } + } else if (type === "circle" || type === "ellipse") { + if (type === "circle") { + r = ry = attr.r; + rycirc = r * circ; + } else { + r = attr.rx; + ry = attr.ry; + rycirc = ry * circ; + } + + x = attr.cx; + y = attr.cy; + rcirc = r * circ; + data = "M" + (x + r) + "," + y + " C" + [x + r, y + rycirc, x + rcirc, y + ry, x, y + ry, x - rcirc, y + ry, x - r, y + rycirc, x - r, y, x - r, y - rycirc, x - rcirc, y - ry, x, y - ry, x + rcirc, y - ry, x + r, y - rycirc, x + r, y].join(",") + "z"; + } else if (type === "line") { + data = "M" + attr.x1 + "," + attr.y1 + " L" + attr.x2 + "," + attr.y2; + } else if (type === "polyline" || type === "polygon") { + points = (element.getAttribute("points") + "").match(_numbersExp) || []; + x = points.shift(); + y = points.shift(); + data = "M" + x + "," + y + " L" + points.join(","); + + if (type === "polygon") { + data += "," + x + "," + y + "z"; + } + } + + path.setAttribute("d", rawPathToString(path._gsRawPath = stringToRawPath(data))); + + if (swap && element.parentNode) { + element.parentNode.insertBefore(path, element); + element.parentNode.removeChild(element); + } + + return path; + } + + function getRotationAtBezierT(segment, i, t) { + var a = segment[i], + b = segment[i + 2], + c = segment[i + 4], + x; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + x = b + (c + (segment[i + 6] - c) * t - b) * t - a; + a = segment[i + 1]; + b = segment[i + 3]; + c = segment[i + 5]; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + return _round(_atan2(b + (c + (segment[i + 7] - c) * t - b) * t - a, x) * _RAD2DEG); + } + + function sliceRawPath(rawPath, start, end) { + end = _isUndefined(end) ? 1 : _roundPrecise(end) || 0; + start = _roundPrecise(start) || 0; + var loops = Math.max(0, ~~(_abs(end - start) - 1e-8)), + path = copyRawPath(rawPath); + + if (start > end) { + start = 1 - start; + end = 1 - end; + + _reverseRawPath(path); + + path.totalLength = 0; + } + + if (start < 0 || end < 0) { + var offset = Math.abs(~~Math.min(start, end)) + 1; + start += offset; + end += offset; + } + + path.totalLength || cacheRawPathMeasurements(path); + var wrap = end > 1, + s = getProgressData(path, start, _temp, true), + e = getProgressData(path, end, _temp2), + eSeg = e.segment, + sSeg = s.segment, + eSegIndex = e.segIndex, + sSegIndex = s.segIndex, + ei = e.i, + si = s.i, + sameSegment = sSegIndex === eSegIndex, + sameBezier = ei === si && sameSegment, + wrapsBehind, + sShift, + eShift, + i, + copy, + totalSegments, + l, + j; + + if (wrap || loops) { + wrapsBehind = eSegIndex < sSegIndex || sameSegment && ei < si || sameBezier && e.t < s.t; + + if (_splitSegment(path, sSegIndex, si, s.t)) { + sSegIndex++; + + if (!wrapsBehind) { + eSegIndex++; + + if (sameBezier) { + e.t = (e.t - s.t) / (1 - s.t); + ei = 0; + } else if (sameSegment) { + ei -= si; + } + } + } + + if (Math.abs(1 - (end - start)) < 1e-5) { + eSegIndex = sSegIndex - 1; + } else if (!e.t && eSegIndex) { + eSegIndex--; + } else if (_splitSegment(path, eSegIndex, ei, e.t) && wrapsBehind) { + sSegIndex++; + } + + if (s.t === 1) { + sSegIndex = (sSegIndex + 1) % path.length; + } + + copy = []; + totalSegments = path.length; + l = 1 + totalSegments * loops; + j = sSegIndex; + l += (totalSegments - sSegIndex + eSegIndex) % totalSegments; + + for (i = 0; i < l; i++) { + _appendOrMerge(copy, path[j++ % totalSegments]); + } + + path = copy; + } else { + eShift = e.t === 1 ? 6 : subdivideSegment(eSeg, ei, e.t); + + if (start !== end) { + sShift = subdivideSegment(sSeg, si, sameBezier ? s.t / e.t : s.t); + sameSegment && (eShift += sShift); + eSeg.splice(ei + eShift + 2); + (sShift || si) && sSeg.splice(0, si + sShift); + i = path.length; + + while (i--) { + (i < sSegIndex || i > eSegIndex) && path.splice(i, 1); + } + } else { + eSeg.angle = getRotationAtBezierT(eSeg, ei + eShift, 0); + ei += eShift; + s = eSeg[ei]; + e = eSeg[ei + 1]; + eSeg.length = eSeg.totalLength = 0; + eSeg.totalPoints = path.totalPoints = 8; + eSeg.push(s, e, s, e, s, e, s, e); + } + } + + path.totalLength = 0; + return path; + } + + function measureSegment(segment, startIndex, bezierQty) { + startIndex = startIndex || 0; + + if (!segment.samples) { + segment.samples = []; + segment.lookup = []; + } + + var resolution = ~~segment.resolution || 12, + inc = 1 / resolution, + endIndex = bezierQty ? startIndex + bezierQty * 6 + 1 : segment.length, + x1 = segment[startIndex], + y1 = segment[startIndex + 1], + samplesIndex = startIndex ? startIndex / 6 * resolution : 0, + samples = segment.samples, + lookup = segment.lookup, + min = (startIndex ? segment.minLength : _largeNum) || _largeNum, + prevLength = samples[samplesIndex + bezierQty * resolution - 1], + length = startIndex ? samples[samplesIndex - 1] : 0, + i, + j, + x4, + x3, + x2, + xd, + xd1, + y4, + y3, + y2, + yd, + yd1, + inv, + t, + lengthIndex, + l, + segLength; + samples.length = lookup.length = 0; + + for (j = startIndex + 2; j < endIndex; j += 6) { + x4 = segment[j + 4] - x1; + x3 = segment[j + 2] - x1; + x2 = segment[j] - x1; + y4 = segment[j + 5] - y1; + y3 = segment[j + 3] - y1; + y2 = segment[j + 1] - y1; + xd = xd1 = yd = yd1 = 0; + + if (_abs(x4) < .01 && _abs(y4) < .01 && _abs(x2) + _abs(y2) < .01) { + if (segment.length > 8) { + segment.splice(j, 6); + j -= 6; + endIndex -= 6; + } + } else { + for (i = 1; i <= resolution; i++) { + t = inc * i; + inv = 1 - t; + xd = xd1 - (xd1 = (t * t * x4 + 3 * inv * (t * x3 + inv * x2)) * t); + yd = yd1 - (yd1 = (t * t * y4 + 3 * inv * (t * y3 + inv * y2)) * t); + l = _sqrt(yd * yd + xd * xd); + + if (l < min) { + min = l; + } + + length += l; + samples[samplesIndex++] = length; + } + } + + x1 += x4; + y1 += y4; + } + + if (prevLength) { + prevLength -= length; + + for (; samplesIndex < samples.length; samplesIndex++) { + samples[samplesIndex] += prevLength; + } + } + + if (samples.length && min) { + segment.totalLength = segLength = samples[samples.length - 1] || 0; + segment.minLength = min; + + if (segLength / min < 9999) { + l = lengthIndex = 0; + + for (i = 0; i < segLength; i += min) { + lookup[l++] = samples[lengthIndex] < i ? ++lengthIndex : lengthIndex; + } + } + } else { + segment.totalLength = samples[0] = 0; + } + + return startIndex ? length - samples[startIndex / 2 - 1] : length; + } + + function cacheRawPathMeasurements(rawPath, resolution) { + var pathLength, points, i; + + for (i = pathLength = points = 0; i < rawPath.length; i++) { + rawPath[i].resolution = ~~resolution || 12; + points += rawPath[i].length; + pathLength += measureSegment(rawPath[i]); + } + + rawPath.totalPoints = points; + rawPath.totalLength = pathLength; + return rawPath; + } + function subdivideSegment(segment, i, t) { + if (t <= 0 || t >= 1) { + return 0; + } + + var ax = segment[i], + ay = segment[i + 1], + cp1x = segment[i + 2], + cp1y = segment[i + 3], + cp2x = segment[i + 4], + cp2y = segment[i + 5], + bx = segment[i + 6], + by = segment[i + 7], + x1a = ax + (cp1x - ax) * t, + x2 = cp1x + (cp2x - cp1x) * t, + y1a = ay + (cp1y - ay) * t, + y2 = cp1y + (cp2y - cp1y) * t, + x1 = x1a + (x2 - x1a) * t, + y1 = y1a + (y2 - y1a) * t, + x2a = cp2x + (bx - cp2x) * t, + y2a = cp2y + (by - cp2y) * t; + x2 += (x2a - x2) * t; + y2 += (y2a - y2) * t; + segment.splice(i + 2, 4, _round(x1a), _round(y1a), _round(x1), _round(y1), _round(x1 + (x2 - x1) * t), _round(y1 + (y2 - y1) * t), _round(x2), _round(y2), _round(x2a), _round(y2a)); + segment.samples && segment.samples.splice(i / 6 * segment.resolution | 0, 0, 0, 0, 0, 0, 0, 0); + return 6; + } + + function getProgressData(rawPath, progress, decoratee, pushToNextIfAtEnd) { + decoratee = decoratee || {}; + rawPath.totalLength || cacheRawPathMeasurements(rawPath); + + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + + var segIndex = 0, + segment = rawPath[0], + samples, + resolution, + length, + min, + max, + i, + t; + + if (!progress) { + t = i = segIndex = 0; + segment = rawPath[0]; + } else if (progress === 1) { + t = 1; + segIndex = rawPath.length - 1; + segment = rawPath[segIndex]; + i = segment.length - 8; + } else { + if (rawPath.length > 1) { + length = rawPath.totalLength * progress; + max = i = 0; + + while ((max += rawPath[i++].totalLength) < length) { + segIndex = i; + } + + segment = rawPath[segIndex]; + min = max - segment.totalLength; + progress = (length - min) / (max - min) || 0; + } + + samples = segment.samples; + resolution = segment.resolution; + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[~~(length / segment.minLength)] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i - 1] : 0; + max = samples[i]; + + if (max < length) { + min = max; + max = samples[++i]; + } + + t = 1 / resolution * ((length - min) / (max - min) + i % resolution); + i = ~~(i / resolution) * 6; + + if (pushToNextIfAtEnd && t === 1) { + if (i + 6 < segment.length) { + i += 6; + t = 0; + } else if (segIndex + 1 < rawPath.length) { + i = t = 0; + segment = rawPath[++segIndex]; + } + } + } + + decoratee.t = t; + decoratee.i = i; + decoratee.path = rawPath; + decoratee.segment = segment; + decoratee.segIndex = segIndex; + return decoratee; + } + + function getPositionOnPath(rawPath, progress, includeAngle, point) { + var segment = rawPath[0], + result = point || {}, + samples, + resolution, + length, + min, + max, + i, + t, + a, + inv; + + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + + segment.lookup || cacheRawPathMeasurements(rawPath); + + if (rawPath.length > 1) { + length = rawPath.totalLength * progress; + max = i = 0; + + while ((max += rawPath[i++].totalLength) < length) { + segment = rawPath[i]; + } + + min = max - segment.totalLength; + progress = (length - min) / (max - min) || 0; + } + + samples = segment.samples; + resolution = segment.resolution; + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i - 1] : 0; + max = samples[i]; + + if (max < length) { + min = max; + max = samples[++i]; + } + + t = 1 / resolution * ((length - min) / (max - min) + i % resolution) || 0; + inv = 1 - t; + i = ~~(i / resolution) * 6; + a = segment[i]; + result.x = _round((t * t * (segment[i + 6] - a) + 3 * inv * (t * (segment[i + 4] - a) + inv * (segment[i + 2] - a))) * t + a); + result.y = _round((t * t * (segment[i + 7] - (a = segment[i + 1])) + 3 * inv * (t * (segment[i + 5] - a) + inv * (segment[i + 3] - a))) * t + a); + + if (includeAngle) { + result.angle = segment.totalLength ? getRotationAtBezierT(segment, i, t >= 1 ? 1 - 1e-9 : t ? t : 1e-9) : segment.angle || 0; + } + + return result; + } + function transformRawPath(rawPath, a, b, c, d, tx, ty) { + var j = rawPath.length, + segment, + l, + i, + x, + y; + + while (--j > -1) { + segment = rawPath[j]; + l = segment.length; + + for (i = 0; i < l; i += 2) { + x = segment[i]; + y = segment[i + 1]; + segment[i] = x * a + y * c + tx; + segment[i + 1] = x * b + y * d + ty; + } + } + + rawPath._dirty = 1; + return rawPath; + } + + function arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) { + if (lastX === x && lastY === y) { + return; + } + + rx = _abs(rx); + ry = _abs(ry); + + var angleRad = angle % 360 * _DEG2RAD, + cosAngle = _cos(angleRad), + sinAngle = _sin(angleRad), + PI = Math.PI, + TWOPI = PI * 2, + dx2 = (lastX - x) / 2, + dy2 = (lastY - y) / 2, + x1 = cosAngle * dx2 + sinAngle * dy2, + y1 = -sinAngle * dx2 + cosAngle * dy2, + x1_sq = x1 * x1, + y1_sq = y1 * y1, + radiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry); + + if (radiiCheck > 1) { + rx = _sqrt(radiiCheck) * rx; + ry = _sqrt(radiiCheck) * ry; + } + + var rx_sq = rx * rx, + ry_sq = ry * ry, + sq = (rx_sq * ry_sq - rx_sq * y1_sq - ry_sq * x1_sq) / (rx_sq * y1_sq + ry_sq * x1_sq); + + if (sq < 0) { + sq = 0; + } + + var coef = (largeArcFlag === sweepFlag ? -1 : 1) * _sqrt(sq), + cx1 = coef * (rx * y1 / ry), + cy1 = coef * -(ry * x1 / rx), + sx2 = (lastX + x) / 2, + sy2 = (lastY + y) / 2, + cx = sx2 + (cosAngle * cx1 - sinAngle * cy1), + cy = sy2 + (sinAngle * cx1 + cosAngle * cy1), + ux = (x1 - cx1) / rx, + uy = (y1 - cy1) / ry, + vx = (-x1 - cx1) / rx, + vy = (-y1 - cy1) / ry, + temp = ux * ux + uy * uy, + angleStart = (uy < 0 ? -1 : 1) * Math.acos(ux / _sqrt(temp)), + angleExtent = (ux * vy - uy * vx < 0 ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt(temp * (vx * vx + vy * vy))); + + isNaN(angleExtent) && (angleExtent = PI); + + if (!sweepFlag && angleExtent > 0) { + angleExtent -= TWOPI; + } else if (sweepFlag && angleExtent < 0) { + angleExtent += TWOPI; + } + + angleStart %= TWOPI; + angleExtent %= TWOPI; + + var segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)), + rawPath = [], + angleIncrement = angleExtent / segments, + controlLength = 4 / 3 * _sin(angleIncrement / 2) / (1 + _cos(angleIncrement / 2)), + ma = cosAngle * rx, + mb = sinAngle * rx, + mc = sinAngle * -ry, + md = cosAngle * ry, + i; + + for (i = 0; i < segments; i++) { + angle = angleStart + i * angleIncrement; + x1 = _cos(angle); + y1 = _sin(angle); + ux = _cos(angle += angleIncrement); + uy = _sin(angle); + rawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy); + } + + for (i = 0; i < rawPath.length; i += 2) { + x1 = rawPath[i]; + y1 = rawPath[i + 1]; + rawPath[i] = x1 * ma + y1 * mc + cx; + rawPath[i + 1] = x1 * mb + y1 * md + cy; + } + + rawPath[i - 2] = x; + rawPath[i - 1] = y; + return rawPath; + } + + function stringToRawPath(d) { + var a = (d + "").replace(_scientific, function (m) { + var n = +m; + return n < 0.0001 && n > -0.0001 ? 0 : n; + }).match(_svgPathExp) || [], + path = [], + relativeX = 0, + relativeY = 0, + twoThirds = 2 / 3, + elements = a.length, + points = 0, + errorMessage = "ERROR: malformed path: " + d, + i, + j, + x, + y, + command, + isRelative, + segment, + startX, + startY, + difX, + difY, + beziers, + prevCommand, + flag1, + flag2, + line = function line(sx, sy, ex, ey) { + difX = (ex - sx) / 3; + difY = (ey - sy) / 3; + segment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey); + }; + + if (!d || !isNaN(a[0]) || isNaN(a[1])) { + console.log(errorMessage); + return path; + } + + for (i = 0; i < elements; i++) { + prevCommand = command; + + if (isNaN(a[i])) { + command = a[i].toUpperCase(); + isRelative = command !== a[i]; + } else { + i--; + } + + x = +a[i + 1]; + y = +a[i + 2]; + + if (isRelative) { + x += relativeX; + y += relativeY; + } + + if (!i) { + startX = x; + startY = y; + } + + if (command === "M") { + if (segment) { + if (segment.length < 8) { + path.length -= 1; + } else { + points += segment.length; + } + } + + relativeX = startX = x; + relativeY = startY = y; + segment = [x, y]; + path.push(segment); + i += 2; + command = "L"; + } else if (command === "C") { + if (!segment) { + segment = [0, 0]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(x, y, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, relativeX += a[i + 5] * 1, relativeY += a[i + 6] * 1); + i += 6; + } else if (command === "S") { + difX = relativeX; + difY = relativeY; + + if (prevCommand === "C" || prevCommand === "S") { + difX += relativeX - segment[segment.length - 4]; + difY += relativeY - segment[segment.length - 3]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(difX, difY, x, y, relativeX += a[i + 3] * 1, relativeY += a[i + 4] * 1); + i += 4; + } else if (command === "Q") { + difX = relativeX + (x - relativeX) * twoThirds; + difY = relativeY + (y - relativeY) * twoThirds; + + if (!isRelative) { + relativeX = relativeY = 0; + } + + relativeX += a[i + 3] * 1; + relativeY += a[i + 4] * 1; + segment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY); + i += 4; + } else if (command === "T") { + difX = relativeX - segment[segment.length - 4]; + difY = relativeY - segment[segment.length - 3]; + segment.push(relativeX + difX, relativeY + difY, x + (relativeX + difX * 1.5 - x) * twoThirds, y + (relativeY + difY * 1.5 - y) * twoThirds, relativeX = x, relativeY = y); + i += 2; + } else if (command === "H") { + line(relativeX, relativeY, relativeX = x, relativeY); + i += 1; + } else if (command === "V") { + line(relativeX, relativeY, relativeX, relativeY = x + (isRelative ? relativeY - relativeX : 0)); + i += 1; + } else if (command === "L" || command === "Z") { + if (command === "Z") { + x = startX; + y = startY; + segment.closed = true; + } + + if (command === "L" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) { + line(relativeX, relativeY, x, y); + + if (command === "L") { + i += 2; + } + } + + relativeX = x; + relativeY = y; + } else if (command === "A") { + flag1 = a[i + 4]; + flag2 = a[i + 5]; + difX = a[i + 6]; + difY = a[i + 7]; + j = 7; + + if (flag1.length > 1) { + if (flag1.length < 3) { + difY = difX; + difX = flag2; + j--; + } else { + difY = flag2; + difX = flag1.substr(2); + j -= 2; + } + + flag2 = flag1.charAt(1); + flag1 = flag1.charAt(0); + } + + beziers = arcToSegment(relativeX, relativeY, +a[i + 1], +a[i + 2], +a[i + 3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX * 1, (isRelative ? relativeY : 0) + difY * 1); + i += j; + + if (beziers) { + for (j = 0; j < beziers.length; j++) { + segment.push(beziers[j]); + } + } + + relativeX = segment[segment.length - 2]; + relativeY = segment[segment.length - 1]; + } else { + console.log(errorMessage); + } + } + + i = segment.length; + + if (i < 6) { + path.pop(); + i = 0; + } else if (segment[0] === segment[i - 2] && segment[1] === segment[i - 1]) { + segment.closed = true; + } + + path.totalPoints = points + i; + return path; + } + function flatPointsToSegment(points, curviness) { + if (curviness === void 0) { + curviness = 1; + } + + var x = points[0], + y = 0, + segment = [x, y], + i = 2; + + for (; i < points.length; i += 2) { + segment.push(x, y, points[i], y = (points[i] - x) * curviness / 2, x = points[i], -y); + } + + return segment; + } + function pointsToSegment(points, curviness) { + _abs(points[0] - points[2]) < 1e-4 && _abs(points[1] - points[3]) < 1e-4 && (points = points.slice(2)); + var l = points.length - 2, + x = +points[0], + y = +points[1], + nextX = +points[2], + nextY = +points[3], + segment = [x, y, x, y], + dx2 = nextX - x, + dy2 = nextY - y, + closed = Math.abs(points[l] - x) < 0.001 && Math.abs(points[l + 1] - y) < 0.001, + prevX, + prevY, + i, + dx1, + dy1, + r1, + r2, + r3, + tl, + mx1, + mx2, + mxm, + my1, + my2, + mym; + + if (closed) { + points.push(nextX, nextY); + nextX = x; + nextY = y; + x = points[l - 2]; + y = points[l - 1]; + points.unshift(x, y); + l += 4; + } + + curviness = curviness || curviness === 0 ? +curviness : 1; + + for (i = 2; i < l; i += 2) { + prevX = x; + prevY = y; + x = nextX; + y = nextY; + nextX = +points[i + 2]; + nextY = +points[i + 3]; + + if (x === nextX && y === nextY) { + continue; + } + + dx1 = dx2; + dy1 = dy2; + dx2 = nextX - x; + dy2 = nextY - y; + r1 = _sqrt(dx1 * dx1 + dy1 * dy1); + r2 = _sqrt(dx2 * dx2 + dy2 * dy2); + r3 = _sqrt(Math.pow(dx2 / r2 + dx1 / r1, 2) + Math.pow(dy2 / r2 + dy1 / r1, 2)); + tl = (r1 + r2) * curviness * 0.25 / r3; + mx1 = x - (x - prevX) * (r1 ? tl / r1 : 0); + mx2 = x + (nextX - x) * (r2 ? tl / r2 : 0); + mxm = x - (mx1 + ((mx2 - mx1) * (r1 * 3 / (r1 + r2) + 0.5) / 4 || 0)); + my1 = y - (y - prevY) * (r1 ? tl / r1 : 0); + my2 = y + (nextY - y) * (r2 ? tl / r2 : 0); + mym = y - (my1 + ((my2 - my1) * (r1 * 3 / (r1 + r2) + 0.5) / 4 || 0)); + + if (x !== prevX || y !== prevY) { + segment.push(_round(mx1 + mxm), _round(my1 + mym), _round(x), _round(y), _round(mx2 + mxm), _round(my2 + mym)); + } + } + + x !== nextX || y !== nextY || segment.length < 4 ? segment.push(_round(nextX), _round(nextY), _round(nextX), _round(nextY)) : segment.length -= 2; + + if (segment.length === 2) { + segment.push(x, y, x, y, x, y); + } else if (closed) { + segment.splice(0, 6); + segment.length = segment.length - 6; + } + + return segment; + } + function rawPathToString(rawPath) { + if (_isNumber(rawPath[0])) { + rawPath = [rawPath]; + } + + var result = "", + l = rawPath.length, + sl, + s, + i, + segment; + + for (s = 0; s < l; s++) { + segment = rawPath[s]; + result += "M" + _round(segment[0]) + "," + _round(segment[1]) + " C"; + sl = segment.length; + + for (i = 2; i < sl; i++) { + result += _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i]) + " "; + } + + if (segment.closed) { + result += "z"; + } + } + + return result; + } + + var _doc, + _win, + _docElement, + _body, + _divContainer, + _svgContainer, + _identityMatrix, + _gEl, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _hasOffsetBug, + _setDoc = function _setDoc(element) { + var doc = element.ownerDocument || element; + + if (!(_transformProp in element.style) && "msTransform" in element.style) { + _transformProp = "msTransform"; + _transformOriginProp = _transformProp + "Origin"; + } + + while (doc.parentNode && (doc = doc.parentNode)) {} + + _win = window; + _identityMatrix = new Matrix2D(); + + if (doc) { + _doc = doc; + _docElement = doc.documentElement; + _body = doc.body; + _gEl = _doc.createElementNS("http://www.w3.org/2000/svg", "g"); + _gEl.style.transform = "none"; + var d1 = doc.createElement("div"), + d2 = doc.createElement("div"), + root = doc && (doc.body || doc.firstElementChild); + + if (root && root.appendChild) { + root.appendChild(d1); + d1.appendChild(d2); + d1.setAttribute("style", "position:static;transform:translate3d(0,0,1px)"); + _hasOffsetBug = d2.offsetParent !== d1; + root.removeChild(d1); + } + } + + return doc; + }, + _forceNonZeroScale = function _forceNonZeroScale(e) { + var a, cache; + + while (e && e !== _body) { + cache = e._gsap; + cache && cache.uncache && cache.get(e, "x"); + + if (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) { + cache.scaleX = cache.scaleY = 1e-4; + cache.renderTransform(1, cache); + a ? a.push(cache) : a = [cache]; + } + + e = e.parentNode; + } + + return a; + }, + _svgTemps = [], + _divTemps = [], + _getDocScrollTop = function _getDocScrollTop() { + return _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0; + }, + _getDocScrollLeft = function _getDocScrollLeft() { + return _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0; + }, + _svgOwner = function _svgOwner(element) { + return element.ownerSVGElement || ((element.tagName + "").toLowerCase() === "svg" ? element : null); + }, + _isFixed = function _isFixed(element) { + if (_win.getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + return _isFixed(element); + } + }, + _createSibling = function _createSibling(element, i) { + if (element.parentNode && (_doc || _setDoc(element))) { + var svg = _svgOwner(element), + ns = svg ? svg.getAttribute("xmlns") || "http://www.w3.org/2000/svg" : "http://www.w3.org/1999/xhtml", + type = svg ? i ? "rect" : "g" : "div", + x = i !== 2 ? 0 : 100, + y = i === 3 ? 100 : 0, + css = "position:absolute;display:block;pointer-events:none;margin:0;padding:0;", + e = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, "http"), type) : _doc.createElement(type); + + if (i) { + if (!svg) { + if (!_divContainer) { + _divContainer = _createSibling(element); + _divContainer.style.cssText = css; + } + + e.style.cssText = css + "width:0.1px;height:0.1px;top:" + y + "px;left:" + x + "px"; + + _divContainer.appendChild(e); + } else { + _svgContainer || (_svgContainer = _createSibling(element)); + e.setAttribute("width", 0.01); + e.setAttribute("height", 0.01); + e.setAttribute("transform", "translate(" + x + "," + y + ")"); + + _svgContainer.appendChild(e); + } + } + + return e; + } + + throw "Need document and parent."; + }, + _consolidate = function _consolidate(m) { + var c = new Matrix2D(), + i = 0; + + for (; i < m.numberOfItems; i++) { + c.multiply(m.getItem(i).matrix); + } + + return c; + }, + _getCTM = function _getCTM(svg) { + var m = svg.getCTM(), + transform; + + if (!m) { + transform = svg.style[_transformProp]; + svg.style[_transformProp] = "none"; + svg.appendChild(_gEl); + m = _gEl.getCTM(); + svg.removeChild(_gEl); + transform ? svg.style[_transformProp] = transform : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, "-$1").toLowerCase()); + } + + return m || _identityMatrix.clone(); + }, + _placeSiblings = function _placeSiblings(element, adjustGOffset) { + var svg = _svgOwner(element), + isRootSVG = element === svg, + siblings = svg ? _svgTemps : _divTemps, + parent = element.parentNode, + container, + m, + b, + x, + y, + cs; + + if (element === _win) { + return element; + } + + siblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3)); + container = svg ? _svgContainer : _divContainer; + + if (svg) { + if (isRootSVG) { + b = _getCTM(element); + x = -b.e / b.a; + y = -b.f / b.d; + m = _identityMatrix; + } else if (element.getBBox) { + b = element.getBBox(); + m = element.transform ? element.transform.baseVal : {}; + m = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; + x = m.a * b.x + m.c * b.y; + y = m.b * b.x + m.d * b.y; + } else { + m = new Matrix2D(); + x = y = 0; + } + + if (adjustGOffset && element.tagName.toLowerCase() === "g") { + x = y = 0; + } + + (isRootSVG ? svg : parent).appendChild(container); + container.setAttribute("transform", "matrix(" + m.a + "," + m.b + "," + m.c + "," + m.d + "," + (m.e + x) + "," + (m.f + y) + ")"); + } else { + x = y = 0; + + if (_hasOffsetBug) { + m = element.offsetParent; + b = element; + + while (b && (b = b.parentNode) && b !== m && b.parentNode) { + if ((_win.getComputedStyle(b)[_transformProp] + "").length > 4) { + x = b.offsetLeft; + y = b.offsetTop; + b = 0; + } + } + } + + cs = _win.getComputedStyle(element); + + if (cs.position !== "absolute" && cs.position !== "fixed") { + m = element.offsetParent; + + while (parent && parent !== m) { + x += parent.scrollLeft || 0; + y += parent.scrollTop || 0; + parent = parent.parentNode; + } + } + + b = container.style; + b.top = element.offsetTop - y + "px"; + b.left = element.offsetLeft - x + "px"; + b[_transformProp] = cs[_transformProp]; + b[_transformOriginProp] = cs[_transformOriginProp]; + b.position = cs.position === "fixed" ? "fixed" : "absolute"; + element.parentNode.appendChild(container); + } + + return container; + }, + _setMatrix = function _setMatrix(m, a, b, c, d, e, f) { + m.a = a; + m.b = b; + m.c = c; + m.d = d; + m.e = e; + m.f = f; + return m; + }; + + var Matrix2D = function () { + function Matrix2D(a, b, c, d, e, f) { + if (a === void 0) { + a = 1; + } + + if (b === void 0) { + b = 0; + } + + if (c === void 0) { + c = 0; + } + + if (d === void 0) { + d = 1; + } + + if (e === void 0) { + e = 0; + } + + if (f === void 0) { + f = 0; + } + + _setMatrix(this, a, b, c, d, e, f); + } + + var _proto = Matrix2D.prototype; + + _proto.inverse = function inverse() { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + determinant = a * d - b * c || 1e-10; + return _setMatrix(this, d / determinant, -b / determinant, -c / determinant, a / determinant, (c * f - d * e) / determinant, -(a * f - b * e) / determinant); + }; + + _proto.multiply = function multiply(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + a2 = matrix.a, + b2 = matrix.c, + c2 = matrix.b, + d2 = matrix.d, + e2 = matrix.e, + f2 = matrix.f; + return _setMatrix(this, a2 * a + c2 * c, a2 * b + c2 * d, b2 * a + d2 * c, b2 * b + d2 * d, e + e2 * a + f2 * c, f + e2 * b + f2 * d); + }; + + _proto.clone = function clone() { + return new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f); + }; + + _proto.equals = function equals(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + return a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f; + }; + + _proto.apply = function apply(point, decoratee) { + if (decoratee === void 0) { + decoratee = {}; + } + + var x = point.x, + y = point.y, + a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + decoratee.x = x * a + y * c + e || 0; + decoratee.y = x * b + y * d + f || 0; + return decoratee; + }; + + return Matrix2D; + }(); + function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { + if (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) { + return new Matrix2D(); + } + + var zeroScales = _forceNonZeroScale(element), + svg = _svgOwner(element), + temps = svg ? _svgTemps : _divTemps, + container = _placeSiblings(element, adjustGOffset), + b1 = temps[0].getBoundingClientRect(), + b2 = temps[1].getBoundingClientRect(), + b3 = temps[2].getBoundingClientRect(), + parent = container.parentNode, + isFixed = !includeScrollInFixed && _isFixed(element), + m = new Matrix2D((b2.left - b1.left) / 100, (b2.top - b1.top) / 100, (b3.left - b1.left) / 100, (b3.top - b1.top) / 100, b1.left + (isFixed ? 0 : _getDocScrollLeft()), b1.top + (isFixed ? 0 : _getDocScrollTop())); + + parent.removeChild(container); + + if (zeroScales) { + b1 = zeroScales.length; + + while (b1--) { + b2 = zeroScales[b1]; + b2.scaleX = b2.scaleY = 0; + b2.renderTransform(1, b2); + } + } + + return inverse ? m.inverse() : m; + } + + /*! + * MotionPathPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var _xProps = "x,translateX,left,marginLeft,xPercent".split(","), + _yProps = "y,translateY,top,marginTop,yPercent".split(","), + _DEG2RAD$1 = Math.PI / 180, + gsap, + PropTween, + _getUnit, + _toArray, + _getStyleSaver, + _reverting, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _populateSegmentFromArray = function _populateSegmentFromArray(segment, values, property, mode) { + var l = values.length, + si = mode === 2 ? 0 : mode, + i = 0, + v; + + for (; i < l; i++) { + segment[si] = v = parseFloat(values[i][property]); + mode === 2 && (segment[si + 1] = 0); + si += 2; + } + + return segment; + }, + _getPropNum = function _getPropNum(target, prop, unit) { + return parseFloat(target._gsap.get(target, prop, unit || "px")) || 0; + }, + _relativize = function _relativize(segment) { + var x = segment[0], + y = segment[1], + i; + + for (i = 2; i < segment.length; i += 2) { + x = segment[i] += x; + y = segment[i + 1] += y; + } + }, + _segmentToRawPath = function _segmentToRawPath(plugin, segment, target, x, y, slicer, vars, unitX, unitY) { + if (vars.type === "cubic") { + segment = [segment]; + } else { + vars.fromCurrent !== false && segment.unshift(_getPropNum(target, x, unitX), y ? _getPropNum(target, y, unitY) : 0); + vars.relative && _relativize(segment); + var pointFunc = y ? pointsToSegment : flatPointsToSegment; + segment = [pointFunc(segment, vars.curviness)]; + } + + segment = slicer(_align(segment, target, vars)); + + _addDimensionalPropTween(plugin, target, x, segment, "x", unitX); + + y && _addDimensionalPropTween(plugin, target, y, segment, "y", unitY); + return cacheRawPathMeasurements(segment, vars.resolution || (vars.curviness === 0 ? 20 : 12)); + }, + _emptyFunc = function _emptyFunc(v) { + return v; + }, + _numExp = /[-+\.]*\d+\.?(?:e-|e\+)?\d*/g, + _originToPoint = function _originToPoint(element, origin, parentMatrix) { + var m = getGlobalMatrix(element), + x = 0, + y = 0, + svg; + + if ((element.tagName + "").toLowerCase() === "svg") { + svg = element.viewBox.baseVal; + svg.width || (svg = { + width: +element.getAttribute("width"), + height: +element.getAttribute("height") + }); + } else { + svg = origin && element.getBBox && element.getBBox(); + } + + if (origin && origin !== "auto") { + x = origin.push ? origin[0] * (svg ? svg.width : element.offsetWidth || 0) : origin.x; + y = origin.push ? origin[1] * (svg ? svg.height : element.offsetHeight || 0) : origin.y; + } + + return parentMatrix.apply(x || y ? m.apply({ + x: x, + y: y + }) : { + x: m.e, + y: m.f + }); + }, + _getAlignMatrix = function _getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin) { + var parentMatrix = getGlobalMatrix(fromElement.parentNode, true, true), + m = parentMatrix.clone().multiply(getGlobalMatrix(toElement)), + fromPoint = _originToPoint(fromElement, fromOrigin, parentMatrix), + _originToPoint2 = _originToPoint(toElement, toOrigin, parentMatrix), + x = _originToPoint2.x, + y = _originToPoint2.y, + p; + + m.e = m.f = 0; + + if (toOrigin === "auto" && toElement.getTotalLength && toElement.tagName.toLowerCase() === "path") { + p = toElement.getAttribute("d").match(_numExp) || []; + p = m.apply({ + x: +p[0], + y: +p[1] + }); + x += p.x; + y += p.y; + } + + if (p) { + p = m.apply(toElement.getBBox()); + x -= p.x; + y -= p.y; + } + + m.e = x - fromPoint.x; + m.f = y - fromPoint.y; + return m; + }, + _align = function _align(rawPath, target, _ref) { + var align = _ref.align, + matrix = _ref.matrix, + offsetX = _ref.offsetX, + offsetY = _ref.offsetY, + alignOrigin = _ref.alignOrigin; + + var x = rawPath[0][0], + y = rawPath[0][1], + curX = _getPropNum(target, "x"), + curY = _getPropNum(target, "y"), + alignTarget, + m, + p; + + if (!rawPath || !rawPath.length) { + return getRawPath("M0,0L0,0"); + } + + if (align) { + if (align === "self" || (alignTarget = _toArray(align)[0] || target) === target) { + transformRawPath(rawPath, 1, 0, 0, 1, curX - x, curY - y); + } else { + if (alignOrigin && alignOrigin[2] !== false) { + gsap.set(target, { + transformOrigin: alignOrigin[0] * 100 + "% " + alignOrigin[1] * 100 + "%" + }); + } else { + alignOrigin = [_getPropNum(target, "xPercent") / -100, _getPropNum(target, "yPercent") / -100]; + } + + m = _getAlignMatrix(target, alignTarget, alignOrigin, "auto"); + p = m.apply({ + x: x, + y: y + }); + transformRawPath(rawPath, m.a, m.b, m.c, m.d, curX + m.e - (p.x - m.e), curY + m.f - (p.y - m.f)); + } + } + + if (matrix) { + transformRawPath(rawPath, matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f); + } else if (offsetX || offsetY) { + transformRawPath(rawPath, 1, 0, 0, 1, offsetX || 0, offsetY || 0); + } + + return rawPath; + }, + _addDimensionalPropTween = function _addDimensionalPropTween(plugin, target, property, rawPath, pathProperty, forceUnit) { + var cache = target._gsap, + harness = cache.harness, + alias = harness && harness.aliases && harness.aliases[property], + prop = alias && alias.indexOf(",") < 0 ? alias : property, + pt = plugin._pt = new PropTween(plugin._pt, target, prop, 0, 0, _emptyFunc, 0, cache.set(target, prop, plugin)); + pt.u = _getUnit(cache.get(target, prop, forceUnit)) || 0; + pt.path = rawPath; + pt.pp = pathProperty; + + plugin._props.push(prop); + }, + _sliceModifier = function _sliceModifier(start, end) { + return function (rawPath) { + return start || end !== 1 ? sliceRawPath(rawPath, start, end) : rawPath; + }; + }; + + var MotionPathPlugin = { + version: "3.12.7", + name: "motionPath", + register: function register(core, Plugin, propTween) { + gsap = core; + _getUnit = gsap.utils.getUnit; + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + + _reverting = gsap.core.reverting || function () {}; + + PropTween = propTween; + }, + init: function init(target, vars, tween) { + if (!gsap) { + console.warn("Please gsap.registerPlugin(MotionPathPlugin)"); + return false; + } + + if (!(typeof vars === "object" && !vars.style) || !vars.path) { + vars = { + path: vars + }; + } + + var rawPaths = [], + _vars = vars, + path = _vars.path, + autoRotate = _vars.autoRotate, + unitX = _vars.unitX, + unitY = _vars.unitY, + x = _vars.x, + y = _vars.y, + firstObj = path[0], + slicer = _sliceModifier(vars.start, "end" in vars ? vars.end : 1), + rawPath, + p; + + this.rawPaths = rawPaths; + this.target = target; + this.tween = tween; + this.styles = _getStyleSaver && _getStyleSaver(target, "transform"); + + if (this.rotate = autoRotate || autoRotate === 0) { + this.rOffset = parseFloat(autoRotate) || 0; + this.radians = !!vars.useRadians; + this.rProp = vars.rotation || "rotation"; + this.rSet = target._gsap.set(target, this.rProp, this); + this.ru = _getUnit(target._gsap.get(target, this.rProp)) || 0; + } + + if (Array.isArray(path) && !("closed" in path) && typeof firstObj !== "number") { + for (p in firstObj) { + if (!x && ~_xProps.indexOf(p)) { + x = p; + } else if (!y && ~_yProps.indexOf(p)) { + y = p; + } + } + + if (x && y) { + rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray(_populateSegmentFromArray([], path, x, 0), path, y, 1), target, x, y, slicer, vars, unitX || _getUnit(path[0][x]), unitY || _getUnit(path[0][y]))); + } else { + x = y = 0; + } + + for (p in firstObj) { + p !== x && p !== y && rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray([], path, p, 2), target, p, 0, slicer, vars, _getUnit(path[0][p]))); + } + } else { + rawPath = slicer(_align(getRawPath(vars.path), target, vars)); + cacheRawPathMeasurements(rawPath, vars.resolution); + rawPaths.push(rawPath); + + _addDimensionalPropTween(this, target, vars.x || "x", rawPath, "x", vars.unitX || "px"); + + _addDimensionalPropTween(this, target, vars.y || "y", rawPath, "y", vars.unitY || "px"); + } + + tween.vars.immediateRender && this.render(tween.progress(), this); + }, + render: function render(ratio, data) { + var rawPaths = data.rawPaths, + i = rawPaths.length, + pt = data._pt; + + if (data.tween._time || !_reverting()) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + + while (i--) { + getPositionOnPath(rawPaths[i], ratio, !i && data.rotate, rawPaths[i]); + } + + while (pt) { + pt.set(pt.t, pt.p, pt.path[pt.pp] + pt.u, pt.d, ratio); + pt = pt._next; + } + + data.rotate && data.rSet(data.target, data.rProp, rawPaths[0].angle * (data.radians ? _DEG2RAD$1 : 1) + data.rOffset + data.ru, data, ratio); + } else { + data.styles.revert(); + } + }, + getLength: function getLength(path) { + return cacheRawPathMeasurements(getRawPath(path)).totalLength; + }, + sliceRawPath: sliceRawPath, + getRawPath: getRawPath, + pointsToSegment: pointsToSegment, + stringToRawPath: stringToRawPath, + rawPathToString: rawPathToString, + transformRawPath: transformRawPath, + getGlobalMatrix: getGlobalMatrix, + getPositionOnPath: getPositionOnPath, + cacheRawPathMeasurements: cacheRawPathMeasurements, + convertToPath: function convertToPath$1(targets, swap) { + return _toArray(targets).map(function (target) { + return convertToPath(target, swap !== false); + }); + }, + convertCoordinates: function convertCoordinates(fromElement, toElement, point) { + var m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }, + getAlignMatrix: _getAlignMatrix, + getRelativePosition: function getRelativePosition(fromElement, toElement, fromOrigin, toOrigin) { + var m = _getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin); + + return { + x: m.e, + y: m.f + }; + }, + arrayToRawPath: function arrayToRawPath(value, vars) { + vars = vars || {}; + + var segment = _populateSegmentFromArray(_populateSegmentFromArray([], value, vars.x || "x", 0), value, vars.y || "y", 1); + + vars.relative && _relativize(segment); + return [vars.type === "cubic" ? segment : pointsToSegment(segment, vars.curviness)]; + } + }; + _getGSAP() && gsap.registerPlugin(MotionPathPlugin); + + exports.MotionPathPlugin = MotionPathPlugin; + exports.default = MotionPathPlugin; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/MotionPathPlugin.min.js b/node_modules/gsap/dist/MotionPathPlugin.min.js new file mode 100644 index 0000000000000000000000000000000000000000..79fcc8ed23e575a4d638d0a9e3de8212a10a9d84 --- /dev/null +++ b/node_modules/gsap/dist/MotionPathPlugin.min.js @@ -0,0 +1,11 @@ +/*! + * MotionPathPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?e(exports):"function"==typeof define&&define.amd?define(["exports"],e):e((t=t||self).window=t.window||{})}(this,function(t){"use strict";function p(t){return"string"==typeof t}function x(t){return Math.round(1e10*t)/1e10||0}function y(t,e,n,r){var a=t[e],o=1===r?6:subdivideSegment(a,n,r);if((o||!r)&&o+n+2<a.length)return t.splice(e,0,a.slice(0,n+o+2)),a.splice(0,n+o),1}function C(t,e){var n=t.length,r=t[n-1]||[],a=r.length;n&&e[0]===r[a-2]&&e[1]===r[a-1]&&(e=r.concat(e.slice(2)),n--),t[n]=e}var M=/[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/gi,T=/(?:(-)?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/gi,L=/[\+\-]?\d*\.?\d+e[\+\-]?\d+/gi,r=/(^[#\.][a-z]|[a-y][a-z])/i,V=Math.PI/180,s=180/Math.PI,F=Math.sin,U=Math.cos,H=Math.abs,$=Math.sqrt,l=Math.atan2,A=1e8,h=function _isNumber(t){return"number"==typeof t},S={},_={},e=1e5,d=function _wrapProgress(t){return Math.round((t+A)%1*e)/e||(t<0?0:1)},N=function _round(t){return Math.round(t*e)/e||0},m=function _getSampleIndex(t,e,n){var r=t.length,a=~~(n*r);if(t[a]>e){for(;--a&&t[a]>e;);a<0&&(a=0)}else for(;t[++a]<e&&a<r;);return a<r?a:r-1},O=function _copyMetaData(t,e){return e.totalLength=t.totalLength,t.samples?(e.samples=t.samples.slice(0),e.lookup=t.lookup.slice(0),e.minLength=t.minLength,e.resolution=t.resolution):t.totalPoints&&(e.totalPoints=t.totalPoints),e};function getRawPath(t){var e,n=(t=p(t)&&r.test(t)&&document.querySelector(t)||t).getAttribute?t:0;return n&&(t=t.getAttribute("d"))?(n._gsPath||(n._gsPath={}),(e=n._gsPath[t])&&!e._dirty?e:n._gsPath[t]=stringToRawPath(t)):t?p(t)?stringToRawPath(t):h(t[0])?[t]:t:console.warn("Expecting a <path> element or an SVG path data string")}function reverseSegment(t){var e,n=0;for(t.reverse();n<t.length;n+=2)e=t[n],t[n]=t[n+1],t[n+1]=e;t.reversed=!t.reversed}var B={rect:"rx,ry,x,y,width,height",circle:"r,cx,cy",ellipse:"rx,ry,cx,cy",line:"x1,x2,y1,y2"};function convertToPath(t,e){var n,r,a,o,i,s,l,h,u,g,f,p,c,d,m,v,y,x,w,P,b,M,R=t.tagName.toLowerCase(),L=.552284749831;return"path"!==R&&t.getBBox?(s=function _createPath(t,e){var n,r=document.createElementNS("http://www.w3.org/2000/svg","path"),a=[].slice.call(t.attributes),o=a.length;for(e=","+e+",";-1<--o;)n=a[o].nodeName.toLowerCase(),e.indexOf(","+n+",")<0&&r.setAttributeNS(null,n,a[o].nodeValue);return r}(t,"x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points"),M=function _attrToObj(t,e){for(var n=e?e.split(","):[],r={},a=n.length;-1<--a;)r[n[a]]=+t.getAttribute(n[a])||0;return r}(t,B[R]),"rect"===R?(o=M.rx,i=M.ry||o,r=M.x,a=M.y,g=M.width-2*o,f=M.height-2*i,n=o||i?"M"+(v=(d=(c=r+o)+g)+o)+","+(x=a+i)+" V"+(w=x+f)+" C"+[v,P=w+i*L,m=d+o*L,b=w+i,d,b,d-(d-c)/3,b,c+(d-c)/3,b,c,b,p=r+o*(1-L),b,r,P,r,w,r,w-(w-x)/3,r,x+(w-x)/3,r,x,r,y=a+i*(1-L),p,a,c,a,c+(d-c)/3,a,d-(d-c)/3,a,d,a,m,a,v,y,v,x].join(",")+"z":"M"+(r+g)+","+a+" v"+f+" h"+-g+" v"+-f+" h"+g+"z"):"circle"===R||"ellipse"===R?(h="circle"===R?(o=i=M.r)*L:(o=M.rx,(i=M.ry)*L),n="M"+((r=M.cx)+o)+","+(a=M.cy)+" C"+[r+o,a+h,r+(l=o*L),a+i,r,a+i,r-l,a+i,r-o,a+h,r-o,a,r-o,a-h,r-l,a-i,r,a-i,r+l,a-i,r+o,a-h,r+o,a].join(",")+"z"):"line"===R?n="M"+M.x1+","+M.y1+" L"+M.x2+","+M.y2:"polyline"!==R&&"polygon"!==R||(n="M"+(r=(u=(t.getAttribute("points")+"").match(T)||[]).shift())+","+(a=u.shift())+" L"+u.join(","),"polygon"===R&&(n+=","+r+","+a+"z")),s.setAttribute("d",rawPathToString(s._gsRawPath=stringToRawPath(n))),e&&t.parentNode&&(t.parentNode.insertBefore(s,t),t.parentNode.removeChild(t)),s):t}function getRotationAtBezierT(t,e,n){var r,a=t[e],o=t[e+2],i=t[e+4];return a+=(o-a)*n,a+=((o+=(i-o)*n)-a)*n,r=o+(i+(t[e+6]-i)*n-o)*n-a,a=t[e+1],a+=((o=t[e+3])-a)*n,a+=((o+=((i=t[e+5])-o)*n)-a)*n,N(l(o+(i+(t[e+7]-i)*n-o)*n-a,r)*s)}function sliceRawPath(t,e,n){n=function _isUndefined(t){return void 0===t}(n)?1:x(n)||0,e=x(e)||0;var r=Math.max(0,~~(H(n-e)-1e-8)),a=function copyRawPath(t){for(var e=[],n=0;n<t.length;n++)e[n]=O(t[n],t[n].slice(0));return O(t,e)}(t);if(n<e&&(e=1-e,n=1-n,function _reverseRawPath(t,e){var n=t.length;for(e||t.reverse();n--;)t[n].reversed||reverseSegment(t[n])}(a),a.totalLength=0),e<0||n<0){var o=Math.abs(~~Math.min(e,n))+1;e+=o,n+=o}a.totalLength||cacheRawPathMeasurements(a);var i,s,l,h,u,g,f,p,c=1<n,d=getProgressData(a,e,S,!0),m=getProgressData(a,n,_),v=m.segment,w=d.segment,P=m.segIndex,b=d.segIndex,M=m.i,R=d.i,L=b===P,T=M===R&&L;if(c||r){for(i=P<b||L&&M<R||T&&m.t<d.t,y(a,b,R,d.t)&&(b++,i||(P++,T?(m.t=(m.t-d.t)/(1-d.t),M=0):L&&(M-=R))),Math.abs(1-(n-e))<1e-5?P=b-1:!m.t&&P?P--:y(a,P,M,m.t)&&i&&b++,1===d.t&&(b=(b+1)%a.length),u=[],f=1+(g=a.length)*r,f+=(g-(p=b)+P)%g,h=0;h<f;h++)C(u,a[p++%g]);a=u}else if(l=1===m.t?6:subdivideSegment(v,M,m.t),e!==n)for(s=subdivideSegment(w,R,T?d.t/m.t:d.t),L&&(l+=s),v.splice(M+l+2),(s||R)&&w.splice(0,R+s),h=a.length;h--;)(h<b||P<h)&&a.splice(h,1);else v.angle=getRotationAtBezierT(v,M+l,0),d=v[M+=l],m=v[M+1],v.length=v.totalLength=0,v.totalPoints=a.totalPoints=8,v.push(d,m,d,m,d,m,d,m);return a.totalLength=0,a}function measureSegment(t,e,n){e=e||0,t.samples||(t.samples=[],t.lookup=[]);var r,a,o,i,s,l,h,u,g,f,p,c,d,m,v,y,x,w=~~t.resolution||12,P=1/w,b=n?e+6*n+1:t.length,M=t[e],R=t[e+1],L=e?e/6*w:0,T=t.samples,C=t.lookup,S=(e?t.minLength:A)||A,_=T[L+n*w-1],N=e?T[L-1]:0;for(T.length=C.length=0,a=e+2;a<b;a+=6){if(o=t[a+4]-M,i=t[a+2]-M,s=t[a]-M,u=t[a+5]-R,g=t[a+3]-R,f=t[a+1]-R,l=h=p=c=0,H(o)<.01&&H(u)<.01&&H(s)+H(f)<.01)8<t.length&&(t.splice(a,6),a-=6,b-=6);else for(r=1;r<=w;r++)l=h-(h=((m=P*r)*m*o+3*(d=1-m)*(m*i+d*s))*m),p=c-(c=(m*m*u+3*d*(m*g+d*f))*m),(y=$(p*p+l*l))<S&&(S=y),N+=y,T[L++]=N;M+=o,R+=u}if(_)for(_-=N;L<T.length;L++)T[L]+=_;if(T.length&&S){if(t.totalLength=x=T[T.length-1]||0,x/(t.minLength=S)<9999)for(y=v=0,r=0;r<x;r+=S)C[y++]=T[v]<r?++v:v}else t.totalLength=T[0]=0;return e?N-T[e/2-1]:N}function cacheRawPathMeasurements(t,e){var n,r,a;for(a=n=r=0;a<t.length;a++)t[a].resolution=~~e||12,r+=t[a].length,n+=measureSegment(t[a]);return t.totalPoints=r,t.totalLength=n,t}function subdivideSegment(t,e,n){if(n<=0||1<=n)return 0;var r=t[e],a=t[e+1],o=t[e+2],i=t[e+3],s=t[e+4],l=t[e+5],h=r+(o-r)*n,u=o+(s-o)*n,g=a+(i-a)*n,f=i+(l-i)*n,p=h+(u-h)*n,c=g+(f-g)*n,d=s+(t[e+6]-s)*n,m=l+(t[e+7]-l)*n;return u+=(d-u)*n,f+=(m-f)*n,t.splice(e+2,4,N(h),N(g),N(p),N(c),N(p+(u-p)*n),N(c+(f-c)*n),N(u),N(f),N(d),N(m)),t.samples&&t.samples.splice(e/6*t.resolution|0,0,0,0,0,0,0,0),6}function getProgressData(t,e,n,r){n=n||{},t.totalLength||cacheRawPathMeasurements(t),(e<0||1<e)&&(e=d(e));var a,o,i,s,l,h,u,g=0,f=t[0];if(e)if(1===e)u=1,h=(f=t[g=t.length-1]).length-8;else{if(1<t.length){for(i=t.totalLength*e,l=h=0;(l+=t[h++].totalLength)<i;)g=h;e=(i-(s=l-(f=t[g]).totalLength))/(l-s)||0}a=f.samples,o=f.resolution,i=f.totalLength*e,s=(h=f.lookup.length?f.lookup[~~(i/f.minLength)]||0:m(a,i,e))?a[h-1]:0,(l=a[h])<i&&(s=l,l=a[++h]),u=1/o*((i-s)/(l-s)+h%o),h=6*~~(h/o),r&&1===u&&(h+6<f.length?(h+=6,u=0):g+1<t.length&&(h=u=0,f=t[++g]))}else u=h=g=0,f=t[0];return n.t=u,n.i=h,n.path=t,n.segment=f,n.segIndex=g,n}function getPositionOnPath(t,e,n,r){var a,o,i,s,l,h,u,g,f,p=t[0],c=r||{};if((e<0||1<e)&&(e=d(e)),p.lookup||cacheRawPathMeasurements(t),1<t.length){for(i=t.totalLength*e,l=h=0;(l+=t[h++].totalLength)<i;)p=t[h];e=(i-(s=l-p.totalLength))/(l-s)||0}return a=p.samples,o=p.resolution,i=p.totalLength*e,s=(h=p.lookup.length?p.lookup[e<1?~~(i/p.minLength):p.lookup.length-1]||0:m(a,i,e))?a[h-1]:0,(l=a[h])<i&&(s=l,l=a[++h]),f=1-(u=1/o*((i-s)/(l-s)+h%o)||0),g=p[h=6*~~(h/o)],c.x=N((u*u*(p[h+6]-g)+3*f*(u*(p[h+4]-g)+f*(p[h+2]-g)))*u+g),c.y=N((u*u*(p[h+7]-(g=p[h+1]))+3*f*(u*(p[h+5]-g)+f*(p[h+3]-g)))*u+g),n&&(c.angle=p.totalLength?getRotationAtBezierT(p,h,1<=u?1-1e-9:u||1e-9):p.angle||0),c}function transformRawPath(t,e,n,r,a,o,i){for(var s,l,h,u,g,f=t.length;-1<--f;)for(l=(s=t[f]).length,h=0;h<l;h+=2)u=s[h],g=s[h+1],s[h]=u*e+g*r+o,s[h+1]=u*n+g*a+i;return t._dirty=1,t}function arcToSegment(t,e,n,r,a,o,i,s,l){if(t!==s||e!==l){n=H(n),r=H(r);var h=a%360*V,u=U(h),g=F(h),f=Math.PI,p=2*f,c=(t-s)/2,d=(e-l)/2,m=u*c+g*d,v=-g*c+u*d,y=m*m,x=v*v,w=y/(n*n)+x/(r*r);1<w&&(n=$(w)*n,r=$(w)*r);var P=n*n,b=r*r,M=(P*b-P*x-b*y)/(P*x+b*y);M<0&&(M=0);var R=(o===i?-1:1)*$(M),L=n*v/r*R,T=-r*m/n*R,C=u*L-g*T+(t+s)/2,S=g*L+u*T+(e+l)/2,_=(m-L)/n,N=(v-T)/r,A=(-m-L)/n,O=(-v-T)/r,B=_*_+N*N,E=(N<0?-1:1)*Math.acos(_/$(B)),I=(_*O-N*A<0?-1:1)*Math.acos((_*A+N*O)/$(B*(A*A+O*O)));isNaN(I)&&(I=f),!i&&0<I?I-=p:i&&I<0&&(I+=p),E%=p,I%=p;var D,X=Math.ceil(H(I)/(p/4)),k=[],z=I/X,G=4/3*F(z/2)/(1+U(z/2)),Z=u*n,q=g*n,Y=g*-r,j=u*r;for(D=0;D<X;D++)m=U(a=E+D*z),v=F(a),_=U(a+=z),N=F(a),k.push(m-G*v,v+G*m,_+G*N,N-G*_,_,N);for(D=0;D<k.length;D+=2)m=k[D],v=k[D+1],k[D]=m*Z+v*Y+C,k[D+1]=m*q+v*j+S;return k[D-2]=s,k[D-1]=l,k}}function stringToRawPath(t){function Cf(t,e,n,r){u=(n-t)/3,g=(r-e)/3,s.push(t+u,e+g,n-u,r-g,n,r)}var e,n,r,a,o,i,s,l,h,u,g,f,p,c,d,m=(t+"").replace(L,function(t){var e=+t;return e<1e-4&&-1e-4<e?0:e}).match(M)||[],v=[],y=0,x=0,w=m.length,P=0,b="ERROR: malformed path: "+t;if(!t||!isNaN(m[0])||isNaN(m[1]))return console.log(b),v;for(e=0;e<w;e++)if(p=o,isNaN(m[e])?i=(o=m[e].toUpperCase())!==m[e]:e--,r=+m[e+1],a=+m[e+2],i&&(r+=y,a+=x),e||(l=r,h=a),"M"===o)s&&(s.length<8?--v.length:P+=s.length),y=l=r,x=h=a,s=[r,a],v.push(s),e+=2,o="L";else if("C"===o)i||(y=x=0),(s=s||[0,0]).push(r,a,y+1*m[e+3],x+1*m[e+4],y+=1*m[e+5],x+=1*m[e+6]),e+=6;else if("S"===o)u=y,g=x,"C"!==p&&"S"!==p||(u+=y-s[s.length-4],g+=x-s[s.length-3]),i||(y=x=0),s.push(u,g,r,a,y+=1*m[e+3],x+=1*m[e+4]),e+=4;else if("Q"===o)u=y+2/3*(r-y),g=x+2/3*(a-x),i||(y=x=0),y+=1*m[e+3],x+=1*m[e+4],s.push(u,g,y+2/3*(r-y),x+2/3*(a-x),y,x),e+=4;else if("T"===o)u=y-s[s.length-4],g=x-s[s.length-3],s.push(y+u,x+g,r+2/3*(y+1.5*u-r),a+2/3*(x+1.5*g-a),y=r,x=a),e+=2;else if("H"===o)Cf(y,x,y=r,x),e+=1;else if("V"===o)Cf(y,x,y,x=r+(i?x-y:0)),e+=1;else if("L"===o||"Z"===o)"Z"===o&&(r=l,a=h,s.closed=!0),("L"===o||.5<H(y-r)||.5<H(x-a))&&(Cf(y,x,r,a),"L"===o&&(e+=2)),y=r,x=a;else if("A"===o){if(c=m[e+4],d=m[e+5],u=m[e+6],g=m[e+7],n=7,1<c.length&&(c.length<3?(g=u,u=d,n--):(g=d,u=c.substr(2),n-=2),d=c.charAt(1),c=c.charAt(0)),f=arcToSegment(y,x,+m[e+1],+m[e+2],+m[e+3],+c,+d,(i?y:0)+1*u,(i?x:0)+1*g),e+=n,f)for(n=0;n<f.length;n++)s.push(f[n]);y=s[s.length-2],x=s[s.length-1]}else console.log(b);return(e=s.length)<6?(v.pop(),e=0):s[0]===s[e-2]&&s[1]===s[e-1]&&(s.closed=!0),v.totalPoints=P+e,v}function flatPointsToSegment(t,e){void 0===e&&(e=1);for(var n=t[0],r=0,a=[n,r],o=2;o<t.length;o+=2)a.push(n,r,t[o],r=(t[o]-n)*e/2,n=t[o],-r);return a}function pointsToSegment(t,e){H(t[0]-t[2])<1e-4&&H(t[1]-t[3])<1e-4&&(t=t.slice(2));var n,r,a,o,i,s,l,h,u,g,f,p,c,d,m=t.length-2,v=+t[0],y=+t[1],x=+t[2],w=+t[3],P=[v,y,v,y],b=x-v,M=w-y,R=Math.abs(t[m]-v)<.001&&Math.abs(t[m+1]-y)<.001;for(R&&(t.push(x,w),x=v,w=y,v=t[m-2],y=t[m-1],t.unshift(v,y),m+=4),e=e||0===e?+e:1,a=2;a<m;a+=2)n=v,r=y,v=x,y=w,x=+t[a+2],w=+t[a+3],v===x&&y===w||(o=b,i=M,b=x-v,M=w-y,h=((s=$(o*o+i*i))+(l=$(b*b+M*M)))*e*.25/$(Math.pow(b/l+o/s,2)+Math.pow(M/l+i/s,2)),f=v-((u=v-(v-n)*(s?h/s:0))+(((g=v+(x-v)*(l?h/l:0))-u)*(3*s/(s+l)+.5)/4||0)),d=y-((p=y-(y-r)*(s?h/s:0))+(((c=y+(w-y)*(l?h/l:0))-p)*(3*s/(s+l)+.5)/4||0)),v===n&&y===r||P.push(N(u+f),N(p+d),N(v),N(y),N(g+f),N(c+d)));return v!==x||y!==w||P.length<4?P.push(N(x),N(w),N(x),N(w)):P.length-=2,2===P.length?P.push(v,y,v,y,v,y):R&&(P.splice(0,6),P.length=P.length-6),P}function rawPathToString(t){h(t[0])&&(t=[t]);var e,n,r,a,o="",i=t.length;for(n=0;n<i;n++){for(a=t[n],o+="M"+N(a[0])+","+N(a[1])+" C",e=a.length,r=2;r<e;r++)o+=N(a[r++])+","+N(a[r++])+" "+N(a[r++])+","+N(a[r++])+" "+N(a[r++])+","+N(a[r])+" ";a.closed&&(o+="z")}return o}function R(t){var e=t.ownerDocument||t;!(k in t.style)&&"msTransform"in t.style&&(z=(k="msTransform")+"Origin");for(;e.parentNode&&(e=e.parentNode););if(v=window,E=new Y,e){w=(c=e).documentElement,P=e.body,(I=c.createElementNS("http://www.w3.org/2000/svg","g")).style.transform="none";var n=e.createElement("div"),r=e.createElement("div"),a=e&&(e.body||e.firstElementChild);a&&a.appendChild&&(a.appendChild(n),n.appendChild(r),n.setAttribute("style","position:static;transform:translate3d(0,0,1px)"),D=r.offsetParent!==n,a.removeChild(n))}return e}function X(t){return t.ownerSVGElement||("svg"===(t.tagName+"").toLowerCase()?t:null)}function Z(t,e){if(t.parentNode&&(c||R(t))){var n=X(t),r=n?n.getAttribute("xmlns")||"http://www.w3.org/2000/svg":"http://www.w3.org/1999/xhtml",a=n?e?"rect":"g":"div",o=2!==e?0:100,i=3===e?100:0,s="position:absolute;display:block;pointer-events:none;margin:0;padding:0;",l=c.createElementNS?c.createElementNS(r.replace(/^https/,"http"),a):c.createElement(a);return e&&(n?(b=b||Z(t),l.setAttribute("width",.01),l.setAttribute("height",.01),l.setAttribute("transform","translate("+o+","+i+")"),b.appendChild(l)):(f||((f=Z(t)).style.cssText=s),l.style.cssText=s+"width:0.1px;height:0.1px;top:"+i+"px;left:"+o+"px",f.appendChild(l))),l}throw"Need document and parent."}function aa(t,e){var n,r,a,o,i,s,l=X(t),h=t===l,u=l?G:q,g=t.parentNode;if(t===v)return t;if(u.length||u.push(Z(t,1),Z(t,2),Z(t,3)),n=l?b:f,l)h?(o=-(a=function _getCTM(t){var e,n=t.getCTM();return n||(e=t.style[k],t.style[k]="none",t.appendChild(I),n=I.getCTM(),t.removeChild(I),e?t.style[k]=e:t.style.removeProperty(k.replace(/([A-Z])/g,"-$1").toLowerCase())),n||E.clone()}(t)).e/a.a,i=-a.f/a.d,r=E):t.getBBox?(a=t.getBBox(),o=(r=(r=t.transform?t.transform.baseVal:{}).numberOfItems?1<r.numberOfItems?function _consolidate(t){for(var e=new Y,n=0;n<t.numberOfItems;n++)e.multiply(t.getItem(n).matrix);return e}(r):r.getItem(0).matrix:E).a*a.x+r.c*a.y,i=r.b*a.x+r.d*a.y):(r=new Y,o=i=0),e&&"g"===t.tagName.toLowerCase()&&(o=i=0),(h?l:g).appendChild(n),n.setAttribute("transform","matrix("+r.a+","+r.b+","+r.c+","+r.d+","+(r.e+o)+","+(r.f+i)+")");else{if(o=i=0,D)for(r=t.offsetParent,a=t;(a=a&&a.parentNode)&&a!==r&&a.parentNode;)4<(v.getComputedStyle(a)[k]+"").length&&(o=a.offsetLeft,i=a.offsetTop,a=0);if("absolute"!==(s=v.getComputedStyle(t)).position&&"fixed"!==s.position)for(r=t.offsetParent;g&&g!==r;)o+=g.scrollLeft||0,i+=g.scrollTop||0,g=g.parentNode;(a=n.style).top=t.offsetTop-i+"px",a.left=t.offsetLeft-o+"px",a[k]=s[k],a[z]=s[z],a.position="fixed"===s.position?"fixed":"absolute",t.parentNode.appendChild(n)}return n}function ba(t,e,n,r,a,o,i){return t.a=e,t.b=n,t.c=r,t.d=a,t.e=o,t.f=i,t}var c,v,w,P,f,b,E,I,D,n,k="transform",z=k+"Origin",G=[],q=[],Y=((n=Matrix2D.prototype).inverse=function inverse(){var t=this.a,e=this.b,n=this.c,r=this.d,a=this.e,o=this.f,i=t*r-e*n||1e-10;return ba(this,r/i,-e/i,-n/i,t/i,(n*o-r*a)/i,-(t*o-e*a)/i)},n.multiply=function multiply(t){var e=this.a,n=this.b,r=this.c,a=this.d,o=this.e,i=this.f,s=t.a,l=t.c,h=t.b,u=t.d,g=t.e,f=t.f;return ba(this,s*e+h*r,s*n+h*a,l*e+u*r,l*n+u*a,o+g*e+f*r,i+g*n+f*a)},n.clone=function clone(){return new Matrix2D(this.a,this.b,this.c,this.d,this.e,this.f)},n.equals=function equals(t){var e=this.a,n=this.b,r=this.c,a=this.d,o=this.e,i=this.f;return e===t.a&&n===t.b&&r===t.c&&a===t.d&&o===t.e&&i===t.f},n.apply=function apply(t,e){void 0===e&&(e={});var n=t.x,r=t.y,a=this.a,o=this.b,i=this.c,s=this.d,l=this.e,h=this.f;return e.x=n*a+r*i+l||0,e.y=n*o+r*s+h||0,e},Matrix2D);function Matrix2D(t,e,n,r,a,o){void 0===t&&(t=1),void 0===e&&(e=0),void 0===n&&(n=0),void 0===r&&(r=1),void 0===a&&(a=0),void 0===o&&(o=0),ba(this,t,e,n,r,a,o)}function getGlobalMatrix(t,e,n,r){if(!t||!t.parentNode||(c||R(t)).documentElement===t)return new Y;var a=function _forceNonZeroScale(t){for(var e,n;t&&t!==P;)(n=t._gsap)&&n.uncache&&n.get(t,"x"),n&&!n.scaleX&&!n.scaleY&&n.renderTransform&&(n.scaleX=n.scaleY=1e-4,n.renderTransform(1,n),e?e.push(n):e=[n]),t=t.parentNode;return e}(t),o=X(t)?G:q,i=aa(t,n),s=o[0].getBoundingClientRect(),l=o[1].getBoundingClientRect(),h=o[2].getBoundingClientRect(),u=i.parentNode,g=!r&&function _isFixed(t){return"fixed"===v.getComputedStyle(t).position||((t=t.parentNode)&&1===t.nodeType?_isFixed(t):void 0)}(t),f=new Y((l.left-s.left)/100,(l.top-s.top)/100,(h.left-s.left)/100,(h.top-s.top)/100,s.left+(g?0:function _getDocScrollLeft(){return v.pageXOffset||c.scrollLeft||w.scrollLeft||P.scrollLeft||0}()),s.top+(g?0:function _getDocScrollTop(){return v.pageYOffset||c.scrollTop||w.scrollTop||P.scrollTop||0}()));if(u.removeChild(i),a)for(s=a.length;s--;)(l=a[s]).scaleX=l.scaleY=0,l.renderTransform(1,l);return e?f.inverse():f}function na(t,e,n,r){for(var a=e.length,o=2===r?0:r,i=0;i<a;i++)t[o]=parseFloat(e[i][n]),2===r&&(t[o+1]=0),o+=2;return t}function oa(t,e,n){return parseFloat(t._gsap.get(t,e,n||"px"))||0}function pa(t){var e,n=t[0],r=t[1];for(e=2;e<t.length;e+=2)n=t[e]+=n,r=t[e+1]+=r}function qa(t,e,n,r,a,o,i,s,l){return e="cubic"===i.type?[e]:(!1!==i.fromCurrent&&e.unshift(oa(n,r,s),a?oa(n,a,l):0),i.relative&&pa(e),[(a?pointsToSegment:flatPointsToSegment)(e,i.curviness)]),e=o(nt(e,n,i)),rt(t,n,r,e,"x",s),a&&rt(t,n,a,e,"y",l),cacheRawPathMeasurements(e,i.resolution||(0===i.curviness?20:12))}function ra(t){return t}function ta(t,e,n){var r,a=getGlobalMatrix(t),o=0,i=0;return"svg"===(t.tagName+"").toLowerCase()?(r=t.viewBox.baseVal).width||(r={width:+t.getAttribute("width"),height:+t.getAttribute("height")}):r=e&&t.getBBox&&t.getBBox(),e&&"auto"!==e&&(o=e.push?e[0]*(r?r.width:t.offsetWidth||0):e.x,i=e.push?e[1]*(r?r.height:t.offsetHeight||0):e.y),n.apply(o||i?a.apply({x:o,y:i}):{x:a.e,y:a.f})}function ua(t,e,n,r){var a,o=getGlobalMatrix(t.parentNode,!0,!0),i=o.clone().multiply(getGlobalMatrix(e)),s=ta(t,n,o),l=ta(e,r,o),h=l.x,u=l.y;return i.e=i.f=0,"auto"===r&&e.getTotalLength&&"path"===e.tagName.toLowerCase()&&(a=e.getAttribute("d").match(et)||[],h+=(a=i.apply({x:+a[0],y:+a[1]})).x,u+=a.y),a&&(h-=(a=i.apply(e.getBBox())).x,u-=a.y),i.e=h-s.x,i.f=u-s.y,i}var j,g,Q,W,J,o,K="x,translateX,left,marginLeft,xPercent".split(","),tt="y,translateY,top,marginTop,yPercent".split(","),i=Math.PI/180,et=/[-+\.]*\d+\.?(?:e-|e\+)?\d*/g,nt=function _align(t,e,n){var r,a,o,i=n.align,s=n.matrix,l=n.offsetX,h=n.offsetY,u=n.alignOrigin,g=t[0][0],f=t[0][1],p=oa(e,"x"),c=oa(e,"y");return t&&t.length?(i&&("self"===i||(r=W(i)[0]||e)===e?transformRawPath(t,1,0,0,1,p-g,c-f):(u&&!1!==u[2]?j.set(e,{transformOrigin:100*u[0]+"% "+100*u[1]+"%"}):u=[oa(e,"xPercent")/-100,oa(e,"yPercent")/-100],o=(a=ua(e,r,u,"auto")).apply({x:g,y:f}),transformRawPath(t,a.a,a.b,a.c,a.d,p+a.e-(o.x-a.e),c+a.f-(o.y-a.f)))),s?transformRawPath(t,s.a,s.b,s.c,s.d,s.e,s.f):(l||h)&&transformRawPath(t,1,0,0,1,l||0,h||0),t):getRawPath("M0,0L0,0")},rt=function _addDimensionalPropTween(t,e,n,r,a,o){var i=e._gsap,s=i.harness,l=s&&s.aliases&&s.aliases[n],h=l&&l.indexOf(",")<0?l:n,u=t._pt=new g(t._pt,e,h,0,0,ra,0,i.set(e,h,t));u.u=Q(i.get(e,h,o))||0,u.path=r,u.pp=a,t._props.push(h)},a={version:"3.12.7",name:"motionPath",register:function register(t,e,n){Q=(j=t).utils.getUnit,W=j.utils.toArray,J=j.core.getStyleSaver,o=j.core.reverting||function(){},g=n},init:function init(t,e,n){if(!j)return console.warn("Please gsap.registerPlugin(MotionPathPlugin)"),!1;"object"==typeof e&&!e.style&&e.path||(e={path:e});var r,a,o=[],i=e.path,s=e.autoRotate,l=e.unitX,h=e.unitY,u=e.x,g=e.y,f=i[0],p=function _sliceModifier(e,n){return function(t){return e||1!==n?sliceRawPath(t,e,n):t}}(e.start,"end"in e?e.end:1);if(this.rawPaths=o,this.target=t,this.tween=n,this.styles=J&&J(t,"transform"),(this.rotate=s||0===s)&&(this.rOffset=parseFloat(s)||0,this.radians=!!e.useRadians,this.rProp=e.rotation||"rotation",this.rSet=t._gsap.set(t,this.rProp,this),this.ru=Q(t._gsap.get(t,this.rProp))||0),!Array.isArray(i)||"closed"in i||"number"==typeof f)cacheRawPathMeasurements(r=p(nt(getRawPath(e.path),t,e)),e.resolution),o.push(r),rt(this,t,e.x||"x",r,"x",e.unitX||"px"),rt(this,t,e.y||"y",r,"y",e.unitY||"px");else{for(a in f)!u&&~K.indexOf(a)?u=a:!g&&~tt.indexOf(a)&&(g=a);for(a in u&&g?o.push(qa(this,na(na([],i,u,0),i,g,1),t,u,g,p,e,l||Q(i[0][u]),h||Q(i[0][g]))):u=g=0,f)a!==u&&a!==g&&o.push(qa(this,na([],i,a,2),t,a,0,p,e,Q(i[0][a])))}n.vars.immediateRender&&this.render(n.progress(),this)},render:function render(t,e){var n=e.rawPaths,r=n.length,a=e._pt;if(e.tween._time||!o()){for(1<t?t=1:t<0&&(t=0);r--;)getPositionOnPath(n[r],t,!r&&e.rotate,n[r]);for(;a;)a.set(a.t,a.p,a.path[a.pp]+a.u,a.d,t),a=a._next;e.rotate&&e.rSet(e.target,e.rProp,n[0].angle*(e.radians?i:1)+e.rOffset+e.ru,e,t)}else e.styles.revert()},getLength:function getLength(t){return cacheRawPathMeasurements(getRawPath(t)).totalLength},sliceRawPath:sliceRawPath,getRawPath:getRawPath,pointsToSegment:pointsToSegment,stringToRawPath:stringToRawPath,rawPathToString:rawPathToString,transformRawPath:transformRawPath,getGlobalMatrix:getGlobalMatrix,getPositionOnPath:getPositionOnPath,cacheRawPathMeasurements:cacheRawPathMeasurements,convertToPath:function convertToPath$1(t,e){return W(t).map(function(t){return convertToPath(t,!1!==e)})},convertCoordinates:function convertCoordinates(t,e,n){var r=getGlobalMatrix(e,!0,!0).multiply(getGlobalMatrix(t));return n?r.apply(n):r},getAlignMatrix:ua,getRelativePosition:function getRelativePosition(t,e,n,r){var a=ua(t,e,n,r);return{x:a.e,y:a.f}},arrayToRawPath:function arrayToRawPath(t,e){var n=na(na([],t,(e=e||{}).x||"x",0),t,e.y||"y",1);return e.relative&&pa(n),["cubic"===e.type?n:pointsToSegment(n,e.curviness)]}};!function _getGSAP(){return j||"undefined"!=typeof window&&(j=window.gsap)&&j.registerPlugin&&j}()||j.registerPlugin(a),t.MotionPathPlugin=a,t.default=a;if (typeof(window)==="undefined"||window!==t){Object.defineProperty(t,"__esModule",{value:!0})} else {delete t.default}}); + diff --git a/node_modules/gsap/dist/MotionPathPlugin.min.js.map b/node_modules/gsap/dist/MotionPathPlugin.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..b610bc4222159ab8e1b50e3b2c064f8cfd454d52 --- /dev/null +++ b/node_modules/gsap/dist/MotionPathPlugin.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"MotionPathPlugin.min.js","sources":["../src/utils/paths.js","../src/utils/matrix.js","../src/MotionPathPlugin.js"],"sourcesContent":["/*!\n * paths 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _svgPathExp = /[achlmqstvz]|(-?\\d*\\.?\\d*(?:e[\\-+]?\\d+)?)[0-9]/ig,\n\t_numbersExp = /(?:(-)?\\d*\\.?\\d*(?:e[\\-+]?\\d+)?)[0-9]/ig,\n\t_scientific = /[\\+\\-]?\\d*\\.?\\d+e[\\+\\-]?\\d+/ig,\n\t_selectorExp = /(^[#\\.][a-z]|[a-y][a-z])/i,\n\t_DEG2RAD = Math.PI / 180,\n\t_RAD2DEG = 180 / Math.PI,\n\t_sin = Math.sin,\n\t_cos = Math.cos,\n\t_abs = Math.abs,\n\t_sqrt = Math.sqrt,\n\t_atan2 = Math.atan2,\n\t_largeNum = 1e8,\n\t_isString = value => typeof(value) === \"string\",\n\t_isNumber = value => typeof(value) === \"number\",\n\t_isUndefined = value => typeof(value) === \"undefined\",\n\t_temp = {},\n\t_temp2 = {},\n\t_roundingNum = 1e5,\n\t_wrapProgress = progress => (Math.round((progress + _largeNum) % 1 * _roundingNum) / _roundingNum) || ((progress < 0) ? 0 : 1), //if progress lands on 1, the % will make it 0 which is why we || 1, but not if it's negative because it makes more sense for motion to end at 0 in that case.\n\t_round = value => (Math.round(value * _roundingNum) / _roundingNum) || 0,\n\t_roundPrecise = value => (Math.round(value * 1e10) / 1e10) || 0,\n\t_splitSegment = (rawPath, segIndex, i, t) => {\n\t\tlet segment = rawPath[segIndex],\n\t\t\tshift = t === 1 ? 6 : subdivideSegment(segment, i, t);\n\t\tif ((shift || !t) && shift + i + 2 < segment.length) {\n\t\t\trawPath.splice(segIndex, 0, segment.slice(0, i + shift + 2));\n\t\t\tsegment.splice(0, i + shift);\n\t\t\treturn 1;\n\t\t}\n\t},\n\t_getSampleIndex = (samples, length, progress) => {\n\t\t// slightly slower way than doing this (when there's no lookup): segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0;\n\t\tlet l = samples.length,\n\t\t\ti = ~~(progress * l);\n\t\tif (samples[i] > length) {\n\t\t\twhile (--i && samples[i] > length) {}\n\t\t\ti < 0 && (i = 0);\n\t\t} else {\n\t\t\twhile (samples[++i] < length && i < l) {}\n\t\t}\n\t\treturn i < l ? i : l - 1;\n\t},\n\t_reverseRawPath = (rawPath, skipOuter) => {\n\t\tlet i = rawPath.length;\n\t\tskipOuter || rawPath.reverse();\n\t\twhile (i--) {\n\t\t\trawPath[i].reversed || reverseSegment(rawPath[i]);\n\t\t}\n\t},\n\t_copyMetaData = (source, copy) => {\n\t\tcopy.totalLength = source.totalLength;\n\t\tif (source.samples) { //segment\n\t\t\tcopy.samples = source.samples.slice(0);\n\t\t\tcopy.lookup = source.lookup.slice(0);\n\t\t\tcopy.minLength = source.minLength;\n\t\t\tcopy.resolution = source.resolution;\n\t\t} else if (source.totalPoints) { //rawPath\n\t\t\tcopy.totalPoints = source.totalPoints;\n\t\t}\n\t\treturn copy;\n\t},\n\t//pushes a new segment into a rawPath, but if its starting values match the ending values of the last segment, it'll merge it into that same segment (to reduce the number of segments)\n\t_appendOrMerge = (rawPath, segment) => {\n\t\tlet index = rawPath.length,\n\t\t\tprevSeg = rawPath[index - 1] || [],\n\t\t\tl = prevSeg.length;\n\t\tif (index && segment[0] === prevSeg[l-2] && segment[1] === prevSeg[l-1]) {\n\t\t\tsegment = prevSeg.concat(segment.slice(2));\n\t\t\tindex--;\n\t\t}\n\t\trawPath[index] = segment;\n\t},\n\t_bestDistance;\n\n/* TERMINOLOGY\n - RawPath - an array of arrays, one for each Segment. A single RawPath could have multiple \"M\" commands, defining Segments (paths aren't always connected).\n - Segment - an array containing a sequence of Cubic Bezier coordinates in alternating x, y, x, y format. Starting anchor, then control point 1, control point 2, and ending anchor, then the next control point 1, control point 2, anchor, etc. Uses less memory than an array with a bunch of {x, y} points.\n - Bezier - a single cubic Bezier with a starting anchor, two control points, and an ending anchor.\n - the variable \"t\" is typically the position along an individual Bezier path (time) and it's NOT linear, meaning it could accelerate/decelerate based on the control points whereas the \"p\" or \"progress\" value is linearly mapped to the whole path, so it shouldn't really accelerate/decelerate based on control points. So a progress of 0.2 would be almost exactly 20% along the path. \"t\" is ONLY in an individual Bezier piece.\n */\n\n//accepts basic selector text, a path instance, a RawPath instance, or a Segment and returns a RawPath (makes it easy to homogenize things). If an element or selector text is passed in, it'll also cache the value so that if it's queried again, it'll just take the path data from there instead of parsing it all over again (as long as the path data itself hasn't changed - it'll check).\nexport function getRawPath(value) {\n\tvalue = (_isString(value) && _selectorExp.test(value)) ? document.querySelector(value) || value : value;\n\tlet e = value.getAttribute ? value : 0,\n\t\trawPath;\n\tif (e && (value = value.getAttribute(\"d\"))) {\n\t\t//implements caching\n\t\tif (!e._gsPath) {\n\t\t\te._gsPath = {};\n\t\t}\n\t\trawPath = e._gsPath[value];\n\t\treturn (rawPath && !rawPath._dirty) ? rawPath : (e._gsPath[value] = stringToRawPath(value));\n\t}\n\treturn !value ? console.warn(\"Expecting a <path> element or an SVG path data string\") : _isString(value) ? stringToRawPath(value) : (_isNumber(value[0])) ? [value] : value;\n}\n\n//copies a RawPath WITHOUT the length meta data (for speed)\nexport function copyRawPath(rawPath) {\n\tlet a = [],\n\t\ti = 0;\n\tfor (; i < rawPath.length; i++) {\n\t\ta[i] = _copyMetaData(rawPath[i], rawPath[i].slice(0));\n\t}\n\treturn _copyMetaData(rawPath, a);\n}\n\nexport function reverseSegment(segment) {\n\tlet i = 0,\n\t\ty;\n\tsegment.reverse(); //this will invert the order y, x, y, x so we must flip it back.\n\tfor (; i < segment.length; i += 2) {\n\t\ty = segment[i];\n\t\tsegment[i] = segment[i+1];\n\t\tsegment[i+1] = y;\n\t}\n\tsegment.reversed = !segment.reversed;\n}\n\n\n\nlet _createPath = (e, ignore) => {\n\t\tlet path = document.createElementNS(\"http://www.w3.org/2000/svg\", \"path\"),\n\t\t\tattr = [].slice.call(e.attributes),\n\t\t\ti = attr.length,\n\t\t\tname;\n\t\tignore = \",\" + ignore + \",\";\n\t\twhile (--i > -1) {\n\t\t\tname = attr[i].nodeName.toLowerCase(); //in Microsoft Edge, if you don't set the attribute with a lowercase name, it doesn't render correctly! Super weird.\n\t\t\tif (ignore.indexOf(\",\" + name + \",\") < 0) {\n\t\t\t\tpath.setAttributeNS(null, name, attr[i].nodeValue);\n\t\t\t}\n\t\t}\n\t\treturn path;\n\t},\n\t_typeAttrs = {\n\t\trect:\"rx,ry,x,y,width,height\",\n\t\tcircle:\"r,cx,cy\",\n\t\tellipse:\"rx,ry,cx,cy\",\n\t\tline:\"x1,x2,y1,y2\"\n\t},\n\t_attrToObj = (e, attrs) => {\n\t\tlet props = attrs ? attrs.split(\",\") : [],\n\t\t\tobj = {},\n\t\t\ti = props.length;\n\t\twhile (--i > -1) {\n\t\t\tobj[props[i]] = +e.getAttribute(props[i]) || 0;\n\t\t}\n\t\treturn obj;\n\t};\n\n//converts an SVG shape like <circle>, <rect>, <polygon>, <polyline>, <ellipse>, etc. to a <path>, swapping it in and copying the attributes to match.\nexport function convertToPath(element, swap) {\n\tlet type = element.tagName.toLowerCase(),\n\t\tcirc = 0.552284749831,\n\t\tdata, x, y, r, ry, path, rcirc, rycirc, points, w, h, x2, x3, x4, x5, x6, y2, y3, y4, y5, y6, attr;\n\tif (type === \"path\" || !element.getBBox) {\n\t\treturn element;\n\t}\n\tpath = _createPath(element, \"x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points\");\n\tattr = _attrToObj(element, _typeAttrs[type]);\n\tif (type === \"rect\") {\n\t\tr = attr.rx;\n\t\try = attr.ry || r;\n\t\tx = attr.x;\n\t\ty = attr.y;\n\t\tw = attr.width - r * 2;\n\t\th = attr.height - ry * 2;\n\t\tif (r || ry) { //if there are rounded corners, render cubic beziers\n\t\t\tx2 = x + r * (1 - circ);\n\t\t\tx3 = x + r;\n\t\t\tx4 = x3 + w;\n\t\t\tx5 = x4 + r * circ;\n\t\t\tx6 = x4 + r;\n\t\t\ty2 = y + ry * (1 - circ);\n\t\t\ty3 = y + ry;\n\t\t\ty4 = y3 + h;\n\t\t\ty5 = y4 + ry * circ;\n\t\t\ty6 = y4 + ry;\n\t\t\tdata = \"M\" + x6 + \",\" + y3 + \" V\" + y4 + \" C\" + [x6, y5, x5, y6, x4, y6, x4 - (x4 - x3) / 3, y6, x3 + (x4 - x3) / 3, y6, x3, y6, x2, y6, x, y5, x, y4, x, y4 - (y4 - y3) / 3, x, y3 + (y4 - y3) / 3, x, y3, x, y2, x2, y, x3, y, x3 + (x4 - x3) / 3, y, x4 - (x4 - x3) / 3, y, x4, y, x5, y, x6, y2, x6, y3].join(\",\") + \"z\";\n\t\t} else {\n\t\t\tdata = \"M\" + (x + w) + \",\" + y + \" v\" + h + \" h\" + (-w) + \" v\" + (-h) + \" h\" + w + \"z\";\n\t\t}\n\n\t} else if (type === \"circle\" || type === \"ellipse\") {\n\t\tif (type === \"circle\") {\n\t\t\tr = ry = attr.r;\n\t\t\trycirc = r * circ;\n\t\t} else {\n\t\t\tr = attr.rx;\n\t\t\try = attr.ry;\n\t\t\trycirc = ry * circ;\n\t\t}\n\t\tx = attr.cx;\n\t\ty = attr.cy;\n\t\trcirc = r * circ;\n\t\tdata = \"M\" + (x+r) + \",\" + y + \" C\" + [x+r, y + rycirc, x + rcirc, y + ry, x, y + ry, x - rcirc, y + ry, x - r, y + rycirc, x - r, y, x - r, y - rycirc, x - rcirc, y - ry, x, y - ry, x + rcirc, y - ry, x + r, y - rycirc, x + r, y].join(\",\") + \"z\";\n\t} else if (type === \"line\") {\n\t\tdata = \"M\" + attr.x1 + \",\" + attr.y1 + \" L\" + attr.x2 + \",\" + attr.y2; //previously, we just converted to \"Mx,y Lx,y\" but Safari has bugs that cause that not to render properly when using a stroke-dasharray that's not fully visible! Using a cubic bezier fixes that issue.\n\t} else if (type === \"polyline\" || type === \"polygon\") {\n\t\tpoints = (element.getAttribute(\"points\") + \"\").match(_numbersExp) || [];\n\t\tx = points.shift();\n\t\ty = points.shift();\n\t\tdata = \"M\" + x + \",\" + y + \" L\" + points.join(\",\");\n\t\tif (type === \"polygon\") {\n\t\t\tdata += \",\" + x + \",\" + y + \"z\";\n\t\t}\n\t}\n\tpath.setAttribute(\"d\", rawPathToString(path._gsRawPath = stringToRawPath(data)));\n\tif (swap && element.parentNode) {\n\t\telement.parentNode.insertBefore(path, element);\n\t\telement.parentNode.removeChild(element);\n\t}\n\treturn path;\n}\n\n\n\n//returns the rotation (in degrees) at a particular progress on a rawPath (the slope of the tangent)\nexport function getRotationAtProgress(rawPath, progress) {\n\tlet d = getProgressData(rawPath, progress >= 1 ? 1 - 1e-9 : progress ? progress : 1e-9);\n\treturn getRotationAtBezierT(d.segment, d.i, d.t);\n}\n\nfunction getRotationAtBezierT(segment, i, t) {\n\tlet a = segment[i],\n\t\tb = segment[i+2],\n\t\tc = segment[i+4],\n\t\tx;\n\ta += (b - a) * t;\n\tb += (c - b) * t;\n\ta += (b - a) * t;\n\tx = b + ((c + (segment[i+6] - c) * t) - b) * t - a;\n\ta = segment[i+1];\n\tb = segment[i+3];\n\tc = segment[i+5];\n\ta += (b - a) * t;\n\tb += (c - b) * t;\n\ta += (b - a) * t;\n\treturn _round(_atan2(b + ((c + (segment[i+7] - c) * t) - b) * t - a, x) * _RAD2DEG);\n}\n\nexport function sliceRawPath(rawPath, start, end) {\n\tend = _isUndefined(end) ? 1 : _roundPrecise(end) || 0; // we must round to avoid issues like 4.15 / 8 = 0.8300000000000001 instead of 0.83 or 2.8 / 5 = 0.5599999999999999 instead of 0.56 and if someone is doing a loop like start: 2.8 / 0.5, end: 2.8 / 0.5 + 1.\n\tstart = _roundPrecise(start) || 0;\n\tlet loops = Math.max(0, ~~(_abs(end - start) - 1e-8)),\n\t\tpath = copyRawPath(rawPath);\n\tif (start > end) {\n\t\tstart = 1 - start;\n\t\tend = 1 - end;\n\t\t_reverseRawPath(path);\n\t\tpath.totalLength = 0;\n\t}\n\tif (start < 0 || end < 0) {\n\t\tlet offset = Math.abs(~~Math.min(start, end)) + 1;\n\t\tstart += offset;\n\t\tend += offset;\n\t}\n\tpath.totalLength || cacheRawPathMeasurements(path);\n\tlet wrap = (end > 1),\n\t\ts = getProgressData(path, start, _temp, true),\n\t\te = getProgressData(path, end, _temp2),\n\t\teSeg = e.segment,\n\t\tsSeg = s.segment,\n\t\teSegIndex = e.segIndex,\n\t\tsSegIndex = s.segIndex,\n\t\tei = e.i,\n\t\tsi = s.i,\n\t\tsameSegment = (sSegIndex === eSegIndex),\n\t\tsameBezier = (ei === si && sameSegment),\n\t\twrapsBehind, sShift, eShift, i, copy, totalSegments, l, j;\n\tif (wrap || loops) {\n\t\twrapsBehind = eSegIndex < sSegIndex || (sameSegment && ei < si) || (sameBezier && e.t < s.t);\n\t\tif (_splitSegment(path, sSegIndex, si, s.t)) {\n\t\t\tsSegIndex++;\n\t\t\tif (!wrapsBehind) {\n\t\t\t\teSegIndex++;\n\t\t\t\tif (sameBezier) {\n\t\t\t\t\te.t = (e.t - s.t) / (1 - s.t);\n\t\t\t\t\tei = 0;\n\t\t\t\t} else if (sameSegment) {\n\t\t\t\t\tei -= si;\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tif (Math.abs(1 - (end - start)) < 1e-5) {\n\t\t\teSegIndex = sSegIndex - 1;\n\t\t} else if (!e.t && eSegIndex) {\n\t\t\teSegIndex--;\n\t\t} else if (_splitSegment(path, eSegIndex, ei, e.t) && wrapsBehind) {\n\t\t\tsSegIndex++;\n\t\t}\n\t\tif (s.t === 1) {\n\t\t\tsSegIndex = (sSegIndex + 1) % path.length;\n\t\t}\n\t\tcopy = [];\n\t\ttotalSegments = path.length;\n\t\tl = 1 + totalSegments * loops;\n\t\tj = sSegIndex;\n\t\tl += ((totalSegments - sSegIndex) + eSegIndex) % totalSegments;\n\t\tfor (i = 0; i < l; i++) {\n\t\t\t_appendOrMerge(copy, path[j++ % totalSegments]);\n\t\t}\n\t\tpath = copy;\n\t} else {\n\t\teShift = e.t === 1 ? 6 : subdivideSegment(eSeg, ei, e.t);\n\t\tif (start !== end) {\n\t\t\tsShift = subdivideSegment(sSeg, si, sameBezier ? s.t / e.t : s.t);\n\t\t\tsameSegment && (eShift += sShift);\n\t\t\teSeg.splice(ei + eShift + 2);\n\t\t\t(sShift || si) && sSeg.splice(0, si + sShift);\n\t\t\ti = path.length;\n\t\t\twhile (i--) {\n\t\t\t\t//chop off any extra segments\n\t\t\t\t(i < sSegIndex || i > eSegIndex) &&\tpath.splice(i, 1);\n\t\t\t}\n\t\t} else {\n\t\t\teSeg.angle = getRotationAtBezierT(eSeg, ei + eShift, 0); //record the value before we chop because it'll be impossible to determine the angle after its length is 0!\n\t\t\tei += eShift;\n\t\t\ts = eSeg[ei];\n\t\t\te = eSeg[ei+1];\n\t\t\teSeg.length = eSeg.totalLength = 0;\n\t\t\teSeg.totalPoints = path.totalPoints = 8;\n\t\t\teSeg.push(s, e, s, e, s, e, s, e);\n\t\t}\n\t}\n\tpath.totalLength = 0;\n\treturn path;\n}\n\n//measures a Segment according to its resolution (so if segment.resolution is 6, for example, it'll take 6 samples equally across each Bezier) and create/populate a \"samples\" Array that has the length up to each of those sample points (always increasing from the start) as well as a \"lookup\" array that's broken up according to the smallest distance between 2 samples. This gives us a very fast way of looking up a progress position rather than looping through all the points/Beziers. You can optionally have it only measure a subset, starting at startIndex and going for a specific number of beziers (remember, there are 3 x/y pairs each, for a total of 6 elements for each Bezier). It will also populate a \"totalLength\" property, but that's not generally super accurate because by default it'll only take 6 samples per Bezier. But for performance reasons, it's perfectly adequate for measuring progress values along the path. If you need a more accurate totalLength, either increase the resolution or use the more advanced bezierToPoints() method which keeps adding points until they don't deviate by more than a certain precision value.\nfunction measureSegment(segment, startIndex, bezierQty) {\n\tstartIndex = startIndex || 0;\n\tif (!segment.samples) {\n\t\tsegment.samples = [];\n\t\tsegment.lookup = [];\n\t}\n\tlet resolution = ~~segment.resolution || 12,\n\t\tinc = 1 / resolution,\n\t\tendIndex = bezierQty ? startIndex + bezierQty * 6 + 1 : segment.length,\n\t\tx1 = segment[startIndex],\n\t\ty1 = segment[startIndex + 1],\n\t\tsamplesIndex = startIndex ? (startIndex / 6) * resolution : 0,\n\t\tsamples = segment.samples,\n\t\tlookup = segment.lookup,\n\t\tmin = (startIndex ? segment.minLength : _largeNum) || _largeNum,\n\t\tprevLength = samples[samplesIndex + bezierQty * resolution - 1],\n\t\tlength = startIndex ? samples[samplesIndex-1] : 0,\n\t\ti, j, x4, x3, x2, xd, xd1, y4, y3, y2, yd, yd1, inv, t, lengthIndex, l, segLength;\n\tsamples.length = lookup.length = 0;\n\tfor (j = startIndex + 2; j < endIndex; j += 6) {\n\t\tx4 = segment[j + 4] - x1;\n\t\tx3 = segment[j + 2] - x1;\n\t\tx2 = segment[j] - x1;\n\t\ty4 = segment[j + 5] - y1;\n\t\ty3 = segment[j + 3] - y1;\n\t\ty2 = segment[j + 1] - y1;\n\t\txd = xd1 = yd = yd1 = 0;\n\t\tif (_abs(x4) < .01 && _abs(y4) < .01 && _abs(x2) + _abs(y2) < .01) { //dump points that are sufficiently close (basically right on top of each other, making a bezier super tiny or 0 length)\n\t\t\tif (segment.length > 8) {\n\t\t\t\tsegment.splice(j, 6);\n\t\t\t\tj -= 6;\n\t\t\t\tendIndex -= 6;\n\t\t\t}\n\t\t} else {\n\t\t\tfor (i = 1; i <= resolution; i++) {\n\t\t\t\tt = inc * i;\n\t\t\t\tinv = 1 - t;\n\t\t\t\txd = xd1 - (xd1 = (t * t * x4 + 3 * inv * (t * x3 + inv * x2)) * t);\n\t\t\t\tyd = yd1 - (yd1 = (t * t * y4 + 3 * inv * (t * y3 + inv * y2)) * t);\n\t\t\t\tl = _sqrt(yd * yd + xd * xd);\n\t\t\t\tif (l < min) {\n\t\t\t\t\tmin = l;\n\t\t\t\t}\n\t\t\t\tlength += l;\n\t\t\t\tsamples[samplesIndex++] = length;\n\t\t\t}\n\t\t}\n\t\tx1 += x4;\n\t\ty1 += y4;\n\t}\n\tif (prevLength) {\n\t\tprevLength -= length;\n\t\tfor (; samplesIndex < samples.length; samplesIndex++) {\n\t\t\tsamples[samplesIndex] += prevLength;\n\t\t}\n\t}\n\tif (samples.length && min) {\n\t\tsegment.totalLength = segLength = samples[samples.length-1] || 0;\n\t\tsegment.minLength = min;\n\t\tif (segLength / min < 9999) { // if the lookup would require too many values (memory problem), we skip this and instead we use a loop to lookup values directly in the samples Array\n\t\t\tl = lengthIndex = 0;\n\t\t\tfor (i = 0; i < segLength; i += min) {\n\t\t\t\tlookup[l++] = (samples[lengthIndex] < i) ? ++lengthIndex : lengthIndex;\n\t\t\t}\n\t\t}\n\t} else {\n\t\tsegment.totalLength = samples[0] = 0;\n\t}\n\treturn startIndex ? length - samples[startIndex / 2 - 1] : length;\n}\n\nexport function cacheRawPathMeasurements(rawPath, resolution) {\n\tlet pathLength, points, i;\n\tfor (i = pathLength = points = 0; i < rawPath.length; i++) {\n\t\trawPath[i].resolution = ~~resolution || 12; //steps per Bezier curve (anchor, 2 control points, to anchor)\n\t\tpoints += rawPath[i].length;\n\t\tpathLength += measureSegment(rawPath[i]);\n\t}\n\trawPath.totalPoints = points;\n\trawPath.totalLength = pathLength;\n\treturn rawPath;\n}\n\n//divide segment[i] at position t (value between 0 and 1, progress along that particular cubic bezier segment that starts at segment[i]). Returns how many elements were spliced into the segment array (either 0 or 6)\nexport function subdivideSegment(segment, i, t) {\n\tif (t <= 0 || t >= 1) {\n\t\treturn 0;\n\t}\n\tlet ax = segment[i],\n\t\tay = segment[i+1],\n\t\tcp1x = segment[i+2],\n\t\tcp1y = segment[i+3],\n\t\tcp2x = segment[i+4],\n\t\tcp2y = segment[i+5],\n\t\tbx = segment[i+6],\n\t\tby = segment[i+7],\n\t\tx1a = ax + (cp1x - ax) * t,\n\t\tx2 = cp1x + (cp2x - cp1x) * t,\n\t\ty1a = ay + (cp1y - ay) * t,\n\t\ty2 = cp1y + (cp2y - cp1y) * t,\n\t\tx1 = x1a + (x2 - x1a) * t,\n\t\ty1 = y1a + (y2 - y1a) * t,\n\t\tx2a = cp2x + (bx - cp2x) * t,\n\t\ty2a = cp2y + (by - cp2y) * t;\n\tx2 += (x2a - x2) * t;\n\ty2 += (y2a - y2) * t;\n\tsegment.splice(i + 2, 4,\n\t\t_round(x1a), //first control point\n\t\t_round(y1a),\n\t\t_round(x1), //second control point\n\t\t_round(y1),\n\t\t_round(x1 + (x2 - x1) * t), //new fabricated anchor on line\n\t\t_round(y1 + (y2 - y1) * t),\n\t\t_round(x2), //third control point\n\t\t_round(y2),\n\t\t_round(x2a), //fourth control point\n\t\t_round(y2a)\n\t);\n\tsegment.samples && segment.samples.splice(((i / 6) * segment.resolution) | 0, 0, 0, 0, 0, 0, 0, 0);\n\treturn 6;\n}\n\n// returns an object {path, segment, segIndex, i, t}\nfunction getProgressData(rawPath, progress, decoratee, pushToNextIfAtEnd) {\n\tdecoratee = decoratee || {};\n\trawPath.totalLength || cacheRawPathMeasurements(rawPath);\n\tif (progress < 0 || progress > 1) {\n\t\tprogress = _wrapProgress(progress);\n\t}\n\tlet segIndex = 0,\n\t\tsegment = rawPath[0],\n\t\tsamples, resolution, length, min, max, i, t;\n\tif (!progress) {\n\t\tt = i = segIndex = 0;\n\t\tsegment = rawPath[0];\n\t} else if (progress === 1) {\n\t\tt = 1;\n\t\tsegIndex = rawPath.length - 1;\n\t\tsegment = rawPath[segIndex];\n\t\ti = segment.length - 8;\n\t} else {\n\t\tif (rawPath.length > 1) { //speed optimization: most of the time, there's only one segment so skip the recursion.\n\t\t\tlength = rawPath.totalLength * progress;\n\t\t\tmax = i = 0;\n\t\t\twhile ((max += rawPath[i++].totalLength) < length) {\n\t\t\t\tsegIndex = i;\n\t\t\t}\n\t\t\tsegment = rawPath[segIndex];\n\t\t\tmin = max - segment.totalLength;\n\t\t\tprogress = ((length - min) / (max - min)) || 0;\n\t\t}\n\t\tsamples = segment.samples;\n\t\tresolution = segment.resolution; //how many samples per cubic bezier chunk\n\t\tlength = segment.totalLength * progress;\n\t\ti = segment.lookup.length ? segment.lookup[~~(length / segment.minLength)] || 0 : _getSampleIndex(samples, length, progress);\n\t\tmin = i ? samples[i-1] : 0;\n\t\tmax = samples[i];\n\t\tif (max < length) {\n\t\t\tmin = max;\n\t\t\tmax = samples[++i];\n\t\t}\n\t\tt = (1 / resolution) * (((length - min) / (max - min)) + ((i % resolution)));\n\t\ti = ~~(i / resolution) * 6;\n\t\tif (pushToNextIfAtEnd && t === 1) {\n\t\t\tif (i + 6 < segment.length) {\n\t\t\t\ti += 6;\n\t\t\t\tt = 0;\n\t\t\t} else if (segIndex + 1 < rawPath.length) {\n\t\t\t\ti = t = 0;\n\t\t\t\tsegment = rawPath[++segIndex];\n\t\t\t}\n\t\t}\n\t}\n\tdecoratee.t = t;\n\tdecoratee.i = i;\n\tdecoratee.path = rawPath;\n\tdecoratee.segment = segment;\n\tdecoratee.segIndex = segIndex;\n\treturn decoratee;\n}\n\nexport function getPositionOnPath(rawPath, progress, includeAngle, point) {\n\tlet segment = rawPath[0],\n\t\tresult = point || {},\n\t\tsamples, resolution, length, min, max, i, t, a, inv;\n\tif (progress < 0 || progress > 1) {\n\t\tprogress = _wrapProgress(progress);\n\t}\n\tsegment.lookup || cacheRawPathMeasurements(rawPath);\n\tif (rawPath.length > 1) { //speed optimization: most of the time, there's only one segment so skip the recursion.\n\t\tlength = rawPath.totalLength * progress;\n\t\tmax = i = 0;\n\t\twhile ((max += rawPath[i++].totalLength) < length) {\n\t\t\tsegment = rawPath[i];\n\t\t}\n\t\tmin = max - segment.totalLength;\n\t\tprogress = ((length - min) / (max - min)) || 0;\n\t}\n\tsamples = segment.samples;\n\tresolution = segment.resolution;\n\tlength = segment.totalLength * progress;\n\ti = segment.lookup.length ? segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0 : _getSampleIndex(samples, length, progress);\n\tmin = i ? samples[i-1] : 0;\n\tmax = samples[i];\n\tif (max < length) {\n\t\tmin = max;\n\t\tmax = samples[++i];\n\t}\n\tt = ((1 / resolution) * (((length - min) / (max - min)) + ((i % resolution)))) || 0;\n\tinv = 1 - t;\n\ti = ~~(i / resolution) * 6;\n\ta = segment[i];\n\tresult.x = _round((t * t * (segment[i + 6] - a) + 3 * inv * (t * (segment[i + 4] - a) + inv * (segment[i + 2] - a))) * t + a);\n\tresult.y = _round((t * t * (segment[i + 7] - (a = segment[i+1])) + 3 * inv * (t * (segment[i + 5] - a) + inv * (segment[i + 3] - a))) * t + a);\n\tif (includeAngle) {\n\t\tresult.angle = segment.totalLength ? getRotationAtBezierT(segment, i, t >= 1 ? 1 - 1e-9 : t ? t : 1e-9) : segment.angle || 0;\n\t}\n\treturn result;\n}\n\n\n\n//applies a matrix transform to RawPath (or a segment in a RawPath) and returns whatever was passed in (it transforms the values in the array(s), not a copy).\nexport function transformRawPath(rawPath, a, b, c, d, tx, ty) {\n\tlet j = rawPath.length,\n\t\tsegment, l, i, x, y;\n\twhile (--j > -1) {\n\t\tsegment = rawPath[j];\n\t\tl = segment.length;\n\t\tfor (i = 0; i < l; i += 2) {\n\t\t\tx = segment[i];\n\t\t\ty = segment[i+1];\n\t\t\tsegment[i] = x * a + y * c + tx;\n\t\t\tsegment[i+1] = x * b + y * d + ty;\n\t\t}\n\t}\n\trawPath._dirty = 1;\n\treturn rawPath;\n}\n\n\n\n// translates SVG arc data into a segment (cubic beziers). Angle is in degrees.\nfunction arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) {\n\tif (lastX === x && lastY === y) {\n\t\treturn;\n\t}\n\trx = _abs(rx);\n\try = _abs(ry);\n\tlet angleRad = (angle % 360) * _DEG2RAD,\n\t\tcosAngle = _cos(angleRad),\n\t\tsinAngle = _sin(angleRad),\n\t\tPI = Math.PI,\n\t\tTWOPI = PI * 2,\n\t\tdx2 = (lastX - x) / 2,\n\t\tdy2 = (lastY - y) / 2,\n\t\tx1 = (cosAngle * dx2 + sinAngle * dy2),\n\t\ty1 = (-sinAngle * dx2 + cosAngle * dy2),\n\t\tx1_sq = x1 * x1,\n\t\ty1_sq = y1 * y1,\n\t\tradiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry);\n\tif (radiiCheck > 1) {\n\t\trx = _sqrt(radiiCheck) * rx;\n\t\try = _sqrt(radiiCheck) * ry;\n\t}\n\tlet rx_sq = rx * rx,\n\t\try_sq = ry * ry,\n\t\tsq = ((rx_sq * ry_sq) - (rx_sq * y1_sq) - (ry_sq * x1_sq)) / ((rx_sq * y1_sq) + (ry_sq * x1_sq));\n\tif (sq < 0) {\n\t\tsq = 0;\n\t}\n\tlet coef = ((largeArcFlag === sweepFlag) ? -1 : 1) * _sqrt(sq),\n\t\tcx1 = coef * ((rx * y1) / ry),\n\t\tcy1 = coef * -((ry * x1) / rx),\n\t\tsx2 = (lastX + x) / 2,\n\t\tsy2 = (lastY + y) / 2,\n\t\tcx = sx2 + (cosAngle * cx1 - sinAngle * cy1),\n\t\tcy = sy2 + (sinAngle * cx1 + cosAngle * cy1),\n\t\tux = (x1 - cx1) / rx,\n\t\tuy = (y1 - cy1) / ry,\n\t\tvx = (-x1 - cx1) / rx,\n\t\tvy = (-y1 - cy1) / ry,\n\t\ttemp = ux * ux + uy * uy,\n\t\tangleStart = ((uy < 0) ? -1 : 1) * Math.acos(ux / _sqrt(temp)),\n\t\tangleExtent = ((ux * vy - uy * vx < 0) ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt(temp * (vx * vx + vy * vy)));\n\tisNaN(angleExtent) && (angleExtent = PI); //rare edge case. Math.cos(-1) is NaN.\n\tif (!sweepFlag && angleExtent > 0) {\n\t\tangleExtent -= TWOPI;\n\t} else if (sweepFlag && angleExtent < 0) {\n\t\tangleExtent += TWOPI;\n\t}\n\tangleStart %= TWOPI;\n\tangleExtent %= TWOPI;\n\tlet segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)),\n\t\trawPath = [],\n\t\tangleIncrement = angleExtent / segments,\n\t\tcontrolLength = 4 / 3 * _sin(angleIncrement / 2) / (1 + _cos(angleIncrement / 2)),\n\t\tma = cosAngle * rx,\n\t\tmb = sinAngle * rx,\n\t\tmc = sinAngle * -ry,\n\t\tmd = cosAngle * ry,\n\t\ti;\n\tfor (i = 0; i < segments; i++) {\n\t\tangle = angleStart + i * angleIncrement;\n\t\tx1 = _cos(angle);\n\t\ty1 = _sin(angle);\n\t\tux = _cos(angle += angleIncrement);\n\t\tuy = _sin(angle);\n\t\trawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy);\n\t}\n\t//now transform according to the actual size of the ellipse/arc (the beziers were noramlized, between 0 and 1 on a circle).\n\tfor (i = 0; i < rawPath.length; i+=2) {\n\t\tx1 = rawPath[i];\n\t\ty1 = rawPath[i+1];\n\t\trawPath[i] = x1 * ma + y1 * mc + cx;\n\t\trawPath[i+1] = x1 * mb + y1 * md + cy;\n\t}\n\trawPath[i-2] = x; //always set the end to exactly where it's supposed to be\n\trawPath[i-1] = y;\n\treturn rawPath;\n}\n\n//Spits back a RawPath with absolute coordinates. Each segment starts with a \"moveTo\" command (x coordinate, then y) and then 2 control points (x, y, x, y), then anchor. The goal is to minimize memory and maximize speed.\nexport function stringToRawPath(d) {\n\tlet a = (d + \"\").replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp) || [], //some authoring programs spit out very small numbers in scientific notation like \"1e-5\", so make sure we round that down to 0 first.\n\t\tpath = [],\n\t\trelativeX = 0,\n\t\trelativeY = 0,\n\t\ttwoThirds = 2 / 3,\n\t\telements = a.length,\n\t\tpoints = 0,\n\t\terrorMessage = \"ERROR: malformed path: \" + d,\n\t\ti, j, x, y, command, isRelative, segment, startX, startY, difX, difY, beziers, prevCommand, flag1, flag2,\n\t\tline = function(sx, sy, ex, ey) {\n\t\t\tdifX = (ex - sx) / 3;\n\t\t\tdifY = (ey - sy) / 3;\n\t\t\tsegment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey);\n\t\t};\n\tif (!d || !isNaN(a[0]) || isNaN(a[1])) {\n\t\tconsole.log(errorMessage);\n\t\treturn path;\n\t}\n\tfor (i = 0; i < elements; i++) {\n\t\tprevCommand = command;\n\t\tif (isNaN(a[i])) {\n\t\t\tcommand = a[i].toUpperCase();\n\t\t\tisRelative = (command !== a[i]); //lower case means relative\n\t\t} else { //commands like \"C\" can be strung together without any new command characters between.\n\t\t\ti--;\n\t\t}\n\t\tx = +a[i + 1];\n\t\ty = +a[i + 2];\n\t\tif (isRelative) {\n\t\t\tx += relativeX;\n\t\t\ty += relativeY;\n\t\t}\n\t\tif (!i) {\n\t\t\tstartX = x;\n\t\t\tstartY = y;\n\t\t}\n\n\t\t// \"M\" (move)\n\t\tif (command === \"M\") {\n\t\t\tif (segment) {\n\t\t\t\tif (segment.length < 8) { //if the path data was funky and just had a M with no actual drawing anywhere, skip it.\n\t\t\t\t\tpath.length -= 1;\n\t\t\t\t} else {\n\t\t\t\t\tpoints += segment.length;\n\t\t\t\t}\n\t\t\t}\n\t\t\trelativeX = startX = x;\n\t\t\trelativeY = startY = y;\n\t\t\tsegment = [x, y];\n\t\t\tpath.push(segment);\n\t\t\ti += 2;\n\t\t\tcommand = \"L\"; //an \"M\" with more than 2 values gets interpreted as \"lineTo\" commands (\"L\").\n\n\t\t// \"C\" (cubic bezier)\n\t\t} else if (command === \"C\") {\n\t\t\tif (!segment) {\n\t\t\t\tsegment = [0, 0];\n\t\t\t}\n\t\t\tif (!isRelative) {\n\t\t\t\trelativeX = relativeY = 0;\n\t\t\t}\n\t\t\t//note: \"*1\" is just a fast/short way to cast the value as a Number. WAAAY faster in Chrome, slightly slower in Firefox.\n\t\t\tsegment.push(x,\ty, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, (relativeX += a[i + 5] * 1),\t(relativeY += a[i + 6] * 1));\n\t\t\ti += 6;\n\n\t\t// \"S\" (continuation of cubic bezier)\n\t\t} else if (command === \"S\") {\n\t\t\tdifX = relativeX;\n\t\t\tdifY = relativeY;\n\t\t\tif (prevCommand === \"C\" || prevCommand === \"S\") {\n\t\t\t\tdifX += relativeX - segment[segment.length - 4];\n\t\t\t\tdifY += relativeY - segment[segment.length - 3];\n\t\t\t}\n\t\t\tif (!isRelative) {\n\t\t\t\trelativeX = relativeY = 0;\n\t\t\t}\n\t\t\tsegment.push(difX, difY, x,\ty, (relativeX += a[i + 3] * 1), (relativeY += a[i + 4] * 1));\n\t\t\ti += 4;\n\n\t\t// \"Q\" (quadratic bezier)\n\t\t} else if (command === \"Q\") {\n\t\t\tdifX = relativeX + (x - relativeX) * twoThirds;\n\t\t\tdifY = relativeY + (y - relativeY) * twoThirds;\n\t\t\tif (!isRelative) {\n\t\t\t\trelativeX = relativeY = 0;\n\t\t\t}\n\t\t\trelativeX += a[i + 3] * 1;\n\t\t\trelativeY += a[i + 4] * 1;\n\t\t\tsegment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY);\n\t\t\ti += 4;\n\n\t\t// \"T\" (continuation of quadratic bezier)\n\t\t} else if (command === \"T\") {\n\t\t\tdifX = relativeX - segment[segment.length - 4];\n\t\t\tdifY = relativeY - segment[segment.length - 3];\n\t\t\tsegment.push(relativeX + difX, relativeY + difY, x + ((relativeX + difX * 1.5) - x) * twoThirds, y + ((relativeY + difY * 1.5) - y) * twoThirds, (relativeX = x), (relativeY = y));\n\t\t\ti += 2;\n\n\t\t// \"H\" (horizontal line)\n\t\t} else if (command === \"H\") {\n\t\t\tline(relativeX, relativeY, (relativeX = x), relativeY);\n\t\t\ti += 1;\n\n\t\t// \"V\" (vertical line)\n\t\t} else if (command === \"V\") {\n\t\t\t//adjust values because the first (and only one) isn't x in this case, it's y.\n\t\t\tline(relativeX, relativeY, relativeX, (relativeY = x + (isRelative ? relativeY - relativeX : 0)));\n\t\t\ti += 1;\n\n\t\t// \"L\" (line) or \"Z\" (close)\n\t\t} else if (command === \"L\" || command === \"Z\") {\n\t\t\tif (command === \"Z\") {\n\t\t\t\tx = startX;\n\t\t\t\ty = startY;\n\t\t\t\tsegment.closed = true;\n\t\t\t}\n\t\t\tif (command === \"L\" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) {\n\t\t\t\tline(relativeX, relativeY, x, y);\n\t\t\t\tif (command === \"L\") {\n\t\t\t\t\ti += 2;\n\t\t\t\t}\n\t\t\t}\n\t\t\trelativeX = x;\n\t\t\trelativeY = y;\n\n\t\t// \"A\" (arc)\n\t\t} else if (command === \"A\") {\n\t\t\tflag1 = a[i+4];\n\t\t\tflag2 = a[i+5];\n\t\t\tdifX = a[i+6];\n\t\t\tdifY = a[i+7];\n\t\t\tj = 7;\n\t\t\tif (flag1.length > 1) { // for cases when the flags are merged, like \"a8 8 0 018 8\" (the 0 and 1 flags are WITH the x value of 8, but it could also be \"a8 8 0 01-8 8\" so it may include x or not)\n\t\t\t\tif (flag1.length < 3) {\n\t\t\t\t\tdifY = difX;\n\t\t\t\t\tdifX = flag2;\n\t\t\t\t\tj--;\n\t\t\t\t} else {\n\t\t\t\t\tdifY = flag2;\n\t\t\t\t\tdifX = flag1.substr(2);\n\t\t\t\t\tj-=2;\n\t\t\t\t}\n\t\t\t\tflag2 = flag1.charAt(1);\n\t\t\t\tflag1 = flag1.charAt(0);\n\t\t\t}\n\t\t\tbeziers = arcToSegment(relativeX, relativeY, +a[i+1], +a[i+2], +a[i+3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX*1, (isRelative ? relativeY : 0) + difY*1);\n\t\t\ti += j;\n\t\t\tif (beziers) {\n\t\t\t\tfor (j = 0; j < beziers.length; j++) {\n\t\t\t\t\tsegment.push(beziers[j]);\n\t\t\t\t}\n\t\t\t}\n\t\t\trelativeX = segment[segment.length-2];\n\t\t\trelativeY = segment[segment.length-1];\n\n\t\t} else {\n\t\t\tconsole.log(errorMessage);\n\t\t}\n\t}\n\ti = segment.length;\n\tif (i < 6) { //in case there's odd SVG like a M0,0 command at the very end.\n\t\tpath.pop();\n\t\ti = 0;\n\t} else if (segment[0] === segment[i-2] && segment[1] === segment[i-1]) {\n\t\tsegment.closed = true;\n\t}\n\tpath.totalPoints = points + i;\n\treturn path;\n}\n\n//populates the points array in alternating x/y values (like [x, y, x, y...] instead of individual point objects [{x, y}, {x, y}...] to conserve memory and stay in line with how we're handling segment arrays\nexport function bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) {\n\tlet x12 = (x1 + x2) / 2,\n\t\ty12 = (y1 + y2) / 2,\n\t\tx23 = (x2 + x3) / 2,\n\t\ty23 = (y2 + y3) / 2,\n\t\tx34 = (x3 + x4) / 2,\n\t\ty34 = (y3 + y4) / 2,\n\t\tx123 = (x12 + x23) / 2,\n\t\ty123 = (y12 + y23) / 2,\n\t\tx234 = (x23 + x34) / 2,\n\t\ty234 = (y23 + y34) / 2,\n\t\tx1234 = (x123 + x234) / 2,\n\t\ty1234 = (y123 + y234) / 2,\n\t\tdx = x4 - x1,\n\t\tdy = y4 - y1,\n\t\td2 = _abs((x2 - x4) * dy - (y2 - y4) * dx),\n\t\td3 = _abs((x3 - x4) * dy - (y3 - y4) * dx),\n\t\tlength;\n\tif (!points) {\n\t\tpoints = [x1, y1, x4, y4];\n\t\tindex = 2;\n\t}\n\tpoints.splice(index || points.length - 2, 0, x1234, y1234);\n\tif ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) {\n\t\tlength = points.length;\n\t\tbezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index);\n\t\tbezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 2 + (points.length - length));\n\t}\n\treturn points;\n}\n\n/*\nfunction getAngleBetweenPoints(x0, y0, x1, y1, x2, y2) { //angle between 3 points in radians\n\tvar dx1 = x1 - x0,\n\t\tdy1 = y1 - y0,\n\t\tdx2 = x2 - x1,\n\t\tdy2 = y2 - y1,\n\t\tdx3 = x2 - x0,\n\t\tdy3 = y2 - y0,\n\t\ta = dx1 * dx1 + dy1 * dy1,\n\t\tb = dx2 * dx2 + dy2 * dy2,\n\t\tc = dx3 * dx3 + dy3 * dy3;\n\treturn Math.acos( (a + b - c) / _sqrt(4 * a * b) );\n},\n*/\n\n//pointsToSegment() doesn't handle flat coordinates (where y is always 0) the way we need (the resulting control points are always right on top of the anchors), so this function basically makes the control points go directly up and down, varying in length based on the curviness (more curvy, further control points)\nexport function flatPointsToSegment(points, curviness=1) {\n\tlet x = points[0],\n\t\ty = 0,\n\t\tsegment = [x, y],\n\t\ti = 2;\n\tfor (; i < points.length; i+=2) {\n\t\tsegment.push(\n\t\t\tx,\n\t\t\ty,\n\t\t\tpoints[i],\n\t\t\t(y = (points[i] - x) * curviness / 2),\n\t\t\t(x = points[i]),\n\t\t\t-y\n\t\t);\n\t}\n\treturn segment;\n}\n\n//points is an array of x/y points, like [x, y, x, y, x, y]\nexport function pointsToSegment(points, curviness) {\n\t//points = simplifyPoints(points, tolerance);\n\t_abs(points[0] - points[2]) < 1e-4 && _abs(points[1] - points[3]) < 1e-4 && (points = points.slice(2)); // if the first two points are super close, dump the first one.\n\tlet l = points.length-2,\n\t\tx = +points[0],\n\t\ty = +points[1],\n\t\tnextX = +points[2],\n\t\tnextY = +points[3],\n\t\tsegment = [x, y, x, y],\n\t\tdx2 = nextX - x,\n\t\tdy2 = nextY - y,\n\t\tclosed = Math.abs(points[l] - x) < 0.001 && Math.abs(points[l+1] - y) < 0.001,\n\t\tprevX, prevY, i, dx1, dy1, r1, r2, r3, tl, mx1, mx2, mxm, my1, my2, mym;\n\tif (closed) { // if the start and end points are basically on top of each other, close the segment by adding the 2nd point to the end, and the 2nd-to-last point to the beginning (we'll remove them at the end, but this allows the curvature to look perfect)\n\t\tpoints.push(nextX, nextY);\n\t\tnextX = x;\n\t\tnextY = y;\n\t\tx = points[l-2];\n\t\ty = points[l-1];\n\t\tpoints.unshift(x, y);\n\t\tl+=4;\n\t}\n\tcurviness = (curviness || curviness === 0) ? +curviness : 1;\n\tfor (i = 2; i < l; i+=2) {\n\t\tprevX = x;\n\t\tprevY = y;\n\t\tx = nextX;\n\t\ty = nextY;\n\t\tnextX = +points[i+2];\n\t\tnextY = +points[i+3];\n\t\tif (x === nextX && y === nextY) {\n\t\t\tcontinue;\n\t\t}\n\t\tdx1 = dx2;\n\t\tdy1 = dy2;\n\t\tdx2 = nextX - x;\n\t\tdy2 = nextY - y;\n\t\tr1 = _sqrt(dx1 * dx1 + dy1 * dy1); // r1, r2, and r3 correlate x and y (and z in the future). Basically 2D or 3D hypotenuse\n\t\tr2 = _sqrt(dx2 * dx2 + dy2 * dy2);\n\t\tr3 = _sqrt((dx2 / r2 + dx1 / r1) ** 2 + (dy2 / r2 + dy1 / r1) ** 2);\n\t\ttl = ((r1 + r2) * curviness * 0.25) / r3;\n\t\tmx1 = x - (x - prevX) * (r1 ? tl / r1 : 0);\n\t\tmx2 = x + (nextX - x) * (r2 ? tl / r2 : 0);\n\t\tmxm = x - (mx1 + (((mx2 - mx1) * ((r1 * 3 / (r1 + r2)) + 0.5) / 4) || 0));\n\t\tmy1 = y - (y - prevY) * (r1 ? tl / r1 : 0);\n\t\tmy2 = y + (nextY - y) * (r2 ? tl / r2 : 0);\n\t\tmym = y - (my1 + (((my2 - my1) * ((r1 * 3 / (r1 + r2)) + 0.5) / 4) || 0));\n\t\tif (x !== prevX || y !== prevY) {\n\t\t\tsegment.push(\n\t\t\t\t_round(mx1 + mxm), // first control point\n\t\t\t\t_round(my1 + mym),\n\t\t\t\t_round(x), // anchor\n\t\t\t\t_round(y),\n\t\t\t\t_round(mx2 + mxm), // second control point\n\t\t\t\t_round(my2 + mym)\n\t\t\t);\n\t\t}\n\t}\n\tx !== nextX || y !== nextY || segment.length < 4 ? segment.push(_round(nextX), _round(nextY), _round(nextX), _round(nextY)) : (segment.length -= 2);\n\tif (segment.length === 2) { // only one point!\n\t\tsegment.push(x, y, x, y, x, y);\n\t} else if (closed) {\n\t\tsegment.splice(0, 6);\n\t\tsegment.length = segment.length - 6;\n\t}\n\treturn segment;\n}\n\n//returns the squared distance between an x/y coordinate and a segment between x1/y1 and x2/y2\nfunction pointToSegDist(x, y, x1, y1, x2, y2) {\n\tlet dx = x2 - x1,\n\t\tdy = y2 - y1,\n\t\tt;\n\tif (dx || dy) {\n\t\tt = ((x - x1) * dx + (y - y1) * dy) / (dx * dx + dy * dy);\n\t\tif (t > 1) {\n\t\t\tx1 = x2;\n\t\t\ty1 = y2;\n\t\t} else if (t > 0) {\n\t\t\tx1 += dx * t;\n\t\t\ty1 += dy * t;\n\t\t}\n\t}\n\treturn (x - x1) ** 2 + (y - y1) ** 2;\n}\n\nfunction simplifyStep(points, first, last, tolerance, simplified) {\n\tlet maxSqDist = tolerance,\n\t\tfirstX = points[first],\n\t\tfirstY = points[first+1],\n\t\tlastX = points[last],\n\t\tlastY = points[last+1],\n\t\tindex, i, d;\n\tfor (i = first + 2; i < last; i += 2) {\n\t\td = pointToSegDist(points[i], points[i+1], firstX, firstY, lastX, lastY);\n\t\tif (d > maxSqDist) {\n\t\t\tindex = i;\n\t\t\tmaxSqDist = d;\n\t\t}\n\t}\n\tif (maxSqDist > tolerance) {\n\t\tindex - first > 2 && simplifyStep(points, first, index, tolerance, simplified);\n\t\tsimplified.push(points[index], points[index+1]);\n\t\tlast - index > 2 && simplifyStep(points, index, last, tolerance, simplified);\n\t}\n}\n\n//points is an array of x/y values like [x, y, x, y, x, y]\nexport function simplifyPoints(points, tolerance) {\n\tlet prevX = parseFloat(points[0]),\n\t\tprevY = parseFloat(points[1]),\n\t\ttemp = [prevX, prevY],\n\t\tl = points.length - 2,\n\t\ti, x, y, dx, dy, result, last;\n\ttolerance = (tolerance || 1) ** 2;\n\tfor (i = 2; i < l; i += 2) {\n\t\tx = parseFloat(points[i]);\n\t\ty = parseFloat(points[i+1]);\n\t\tdx = prevX - x;\n\t\tdy = prevY - y;\n\t\tif (dx * dx + dy * dy > tolerance) {\n\t\t\ttemp.push(x, y);\n\t\t\tprevX = x;\n\t\t\tprevY = y;\n\t\t}\n\t}\n\ttemp.push(parseFloat(points[l]), parseFloat(points[l+1]));\n\tlast = temp.length - 2;\n\tresult = [temp[0], temp[1]];\n\tsimplifyStep(temp, 0, last, tolerance, result);\n\tresult.push(temp[last], temp[last+1]);\n\treturn result;\n}\n\nfunction getClosestProgressOnBezier(iterations, px, py, start, end, slices, x0, y0, x1, y1, x2, y2, x3, y3) {\n\tlet inc = (end - start) / slices,\n\t\tbest = 0,\n\t\tt = start,\n\t\tx, y, d, dx, dy, inv;\n\t_bestDistance = _largeNum;\n\twhile (t <= end) {\n\t\tinv = 1 - t;\n\t\tx = inv * inv * inv * x0 + 3 * inv * inv * t * x1 + 3 * inv * t * t * x2 + t * t * t * x3;\n\t\ty = inv * inv * inv * y0 + 3 * inv * inv * t * y1 + 3 * inv * t * t * y2 + t * t * t * y3;\n\t\tdx = x - px;\n\t\tdy = y - py;\n\t\td = dx * dx + dy * dy;\n\t\tif (d < _bestDistance) {\n\t\t\t_bestDistance = d;\n\t\t\tbest = t;\n\t\t}\n\t\tt += inc;\n\t}\n\treturn (iterations > 1) ? getClosestProgressOnBezier(iterations - 1, px, py, Math.max(best - inc, 0), Math.min(best + inc, 1), slices, x0, y0, x1, y1, x2, y2, x3, y3) : best;\n}\n\nexport function getClosestData(rawPath, x, y, slices) { //returns an object with the closest j, i, and t (j is the segment index, i is the index of the point in that segment, and t is the time/progress along that bezier)\n\tlet closest = {j:0, i:0, t:0},\n\t\tbestDistance = _largeNum,\n\t\ti, j, t, segment;\n\tfor (j = 0; j < rawPath.length; j++) {\n\t\tsegment = rawPath[j];\n\t\tfor (i = 0; i < segment.length; i+=6) {\n\t\t\tt = getClosestProgressOnBezier(1, x, y, 0, 1, slices || 20, segment[i], segment[i+1], segment[i+2], segment[i+3], segment[i+4], segment[i+5], segment[i+6], segment[i+7]);\n\t\t\tif (bestDistance > _bestDistance) {\n\t\t\t\tbestDistance = _bestDistance;\n\t\t\t\tclosest.j = j;\n\t\t\t\tclosest.i = i;\n\t\t\t\tclosest.t = t;\n\t\t\t}\n\t\t}\n\t}\n\treturn closest;\n}\n\n//subdivide a Segment closest to a specific x,y coordinate\nexport function subdivideSegmentNear(x, y, segment, slices, iterations) {\n\tlet l = segment.length,\n\t\tbestDistance = _largeNum,\n\t\tbestT = 0,\n\t\tbestSegmentIndex = 0,\n\t\tt, i;\n\tslices = slices || 20;\n\titerations = iterations || 3;\n\tfor (i = 0; i < l; i += 6) {\n\t\tt = getClosestProgressOnBezier(1, x, y, 0, 1, slices, segment[i], segment[i+1], segment[i+2], segment[i+3], segment[i+4], segment[i+5], segment[i+6], segment[i+7]);\n\t\tif (bestDistance > _bestDistance) {\n\t\t\tbestDistance = _bestDistance;\n\t\t\tbestT = t;\n\t\t\tbestSegmentIndex = i;\n\t\t}\n\t}\n\tt = getClosestProgressOnBezier(iterations, x, y, bestT - 0.05, bestT + 0.05, slices, segment[bestSegmentIndex], segment[bestSegmentIndex+1], segment[bestSegmentIndex+2], segment[bestSegmentIndex+3], segment[bestSegmentIndex+4], segment[bestSegmentIndex+5], segment[bestSegmentIndex+6], segment[bestSegmentIndex+7]);\n\tsubdivideSegment(segment, bestSegmentIndex, t);\n\treturn bestSegmentIndex + 6;\n}\n\n/*\nTakes any of the following and converts it to an all Cubic Bezier SVG data string:\n- A <path> data string like \"M0,0 L2,4 v20,15 H100\"\n- A RawPath, like [[x, y, x, y, x, y, x, y][[x, y, x, y, x, y, x, y]]\n- A Segment, like [x, y, x, y, x, y, x, y]\n\nNote: all numbers are rounded down to the closest 0.001 to minimize memory, maximize speed, and avoid odd numbers like 1e-13\n*/\nexport function rawPathToString(rawPath) {\n\tif (_isNumber(rawPath[0])) { //in case a segment is passed in instead\n\t\trawPath = [rawPath];\n\t}\n\tlet result = \"\",\n\t\tl = rawPath.length,\n\t\tsl, s, i, segment;\n\tfor (s = 0; s < l; s++) {\n\t\tsegment = rawPath[s];\n\t\tresult += \"M\" + _round(segment[0]) + \",\" + _round(segment[1]) + \" C\";\n\t\tsl = segment.length;\n\t\tfor (i = 2; i < sl; i++) {\n\t\t\tresult += _round(segment[i++]) + \",\" + _round(segment[i++]) + \" \" + _round(segment[i++]) + \",\" + _round(segment[i++]) + \" \" + _round(segment[i++]) + \",\" + _round(segment[i]) + \" \";\n\t\t}\n\t\tif (segment.closed) {\n\t\t\tresult += \"z\";\n\t\t}\n\t}\n\treturn result;\n}\n\n/*\n// takes a segment with coordinates [x, y, x, y, ...] and converts the control points into angles and lengths [x, y, angle, length, angle, length, x, y, angle, length, ...] so that it animates more cleanly and avoids odd breaks/kinks. For example, if you animate from 1 o'clock to 6 o'clock, it'd just go directly/linearly rather than around. So the length would be very short in the middle of the tween.\nexport function cpCoordsToAngles(segment, copy) {\n\tvar result = copy ? segment.slice(0) : segment,\n\t\tx, y, i;\n\tfor (i = 0; i < segment.length; i+=6) {\n\t\tx = segment[i+2] - segment[i];\n\t\ty = segment[i+3] - segment[i+1];\n\t\tresult[i+2] = Math.atan2(y, x);\n\t\tresult[i+3] = Math.sqrt(x * x + y * y);\n\t\tx = segment[i+6] - segment[i+4];\n\t\ty = segment[i+7] - segment[i+5];\n\t\tresult[i+4] = Math.atan2(y, x);\n\t\tresult[i+5] = Math.sqrt(x * x + y * y);\n\t}\n\treturn result;\n}\n\n// takes a segment that was converted with cpCoordsToAngles() to have angles and lengths instead of coordinates for the control points, and converts it BACK into coordinates.\nexport function cpAnglesToCoords(segment, copy) {\n\tvar result = copy ? segment.slice(0) : segment,\n\t\tlength = segment.length,\n\t\trnd = 1000,\n\t\tangle, l, i, j;\n\tfor (i = 0; i < length; i+=6) {\n\t\tangle = segment[i+2];\n\t\tl = segment[i+3]; //length\n\t\tresult[i+2] = (((segment[i] + Math.cos(angle) * l) * rnd) | 0) / rnd;\n\t\tresult[i+3] = (((segment[i+1] + Math.sin(angle) * l) * rnd) | 0) / rnd;\n\t\tangle = segment[i+4];\n\t\tl = segment[i+5]; //length\n\t\tresult[i+4] = (((segment[i+6] - Math.cos(angle) * l) * rnd) | 0) / rnd;\n\t\tresult[i+5] = (((segment[i+7] - Math.sin(angle) * l) * rnd) | 0) / rnd;\n\t}\n\treturn result;\n}\n\n//adds an \"isSmooth\" array to each segment and populates it with a boolean value indicating whether or not it's smooth (the control points have basically the same slope). For any smooth control points, it converts the coordinates into angle (x, in radians) and length (y) and puts them into the same index value in a smoothData array.\nexport function populateSmoothData(rawPath) {\n\tlet j = rawPath.length,\n\t\tsmooth, segment, x, y, x2, y2, i, l, a, a2, isSmooth, smoothData;\n\twhile (--j > -1) {\n\t\tsegment = rawPath[j];\n\t\tisSmooth = segment.isSmooth = segment.isSmooth || [0, 0, 0, 0];\n\t\tsmoothData = segment.smoothData = segment.smoothData || [0, 0, 0, 0];\n\t\tisSmooth.length = 4;\n\t\tl = segment.length - 2;\n\t\tfor (i = 6; i < l; i += 6) {\n\t\t\tx = segment[i] - segment[i - 2];\n\t\t\ty = segment[i + 1] - segment[i - 1];\n\t\t\tx2 = segment[i + 2] - segment[i];\n\t\t\ty2 = segment[i + 3] - segment[i + 1];\n\t\t\ta = _atan2(y, x);\n\t\t\ta2 = _atan2(y2, x2);\n\t\t\tsmooth = (Math.abs(a - a2) < 0.09);\n\t\t\tif (smooth) {\n\t\t\t\tsmoothData[i - 2] = a;\n\t\t\t\tsmoothData[i + 2] = a2;\n\t\t\t\tsmoothData[i - 1] = _sqrt(x * x + y * y);\n\t\t\t\tsmoothData[i + 3] = _sqrt(x2 * x2 + y2 * y2);\n\t\t\t}\n\t\t\tisSmooth.push(smooth, smooth, 0, 0, smooth, smooth);\n\t\t}\n\t\t//if the first and last points are identical, check to see if there's a smooth transition. We must handle this a bit differently due to their positions in the array.\n\t\tif (segment[l] === segment[0] && segment[l+1] === segment[1]) {\n\t\t\tx = segment[0] - segment[l-2];\n\t\t\ty = segment[1] - segment[l-1];\n\t\t\tx2 = segment[2] - segment[0];\n\t\t\ty2 = segment[3] - segment[1];\n\t\t\ta = _atan2(y, x);\n\t\t\ta2 = _atan2(y2, x2);\n\t\t\tif (Math.abs(a - a2) < 0.09) {\n\t\t\t\tsmoothData[l-2] = a;\n\t\t\t\tsmoothData[2] = a2;\n\t\t\t\tsmoothData[l-1] = _sqrt(x * x + y * y);\n\t\t\t\tsmoothData[3] = _sqrt(x2 * x2 + y2 * y2);\n\t\t\t\tisSmooth[l-2] = isSmooth[l-1] = true; //don't change indexes 2 and 3 because we'll trigger everything from the END, and this will optimize file size a bit.\n\t\t\t}\n\t\t}\n\t}\n\treturn rawPath;\n}\nexport function pointToScreen(svgElement, point) {\n\tif (arguments.length < 2) { //by default, take the first set of coordinates in the path as the point\n\t\tlet rawPath = getRawPath(svgElement);\n\t\tpoint = svgElement.ownerSVGElement.createSVGPoint();\n\t\tpoint.x = rawPath[0][0];\n\t\tpoint.y = rawPath[0][1];\n\t}\n\treturn point.matrixTransform(svgElement.getScreenCTM());\n}\n// takes a <path> and normalizes all of its coordinates to values between 0 and 1\nexport function normalizePath(path) {\n path = gsap.utils.toArray(path);\n if (!path[0].hasAttribute(\"d\")) {\n path = gsap.utils.toArray(path[0].children);\n }\n if (path.length > 1) {\n path.forEach(normalizePath);\n return path;\n }\n let _svgPathExp = /[achlmqstvz]|(-?\\d*\\.?\\d*(?:e[\\-+]?\\d+)?)[0-9]/ig,\n _scientific = /[\\+\\-]?\\d*\\.?\\d+e[\\+\\-]?\\d+/ig,\n d = path[0].getAttribute(\"d\"),\n a = d.replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp),\n nums = a.filter(n => !isNaN(n)).map(n => +n),\n normalize = gsap.utils.normalize(Math.min(...nums), Math.max(...nums)),\n finals = a.map(val => isNaN(val) ? val : normalize(+val)),\n s = \"\",\n prevWasCommand;\n finals.forEach((value, i) => {\n let isCommand = isNaN(value)\n s += (isCommand && i ? \" \" : prevWasCommand || !i ? \"\" : \",\") + value;\n prevWasCommand = isCommand;\n });\n path[0].setAttribute(\"d\", s);\n}\n*/","/*!\n * matrix 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _doc, _win, _docElement, _body,\t_divContainer, _svgContainer, _identityMatrix, _gEl,\n\t_transformProp = \"transform\",\n\t_transformOriginProp = _transformProp + \"Origin\",\n\t_hasOffsetBug,\n\t_setDoc = element => {\n\t\tlet doc = element.ownerDocument || element;\n\t\tif (!(_transformProp in element.style) && \"msTransform\" in element.style) { //to improve compatibility with old Microsoft browsers\n\t\t\t_transformProp = \"msTransform\";\n\t\t\t_transformOriginProp = _transformProp + \"Origin\";\n\t\t}\n\t\twhile (doc.parentNode && (doc = doc.parentNode)) {\t}\n\t\t_win = window;\n\t\t_identityMatrix = new Matrix2D();\n\t\tif (doc) {\n\t\t\t_doc = doc;\n\t\t\t_docElement = doc.documentElement;\n\t\t\t_body = doc.body;\n\t\t\t_gEl = _doc.createElementNS(\"http://www.w3.org/2000/svg\", \"g\");\n\t\t\t// prevent any existing CSS from transforming it\n\t\t\t_gEl.style.transform = \"none\";\n\t\t\t// now test for the offset reporting bug. Use feature detection instead of browser sniffing to make things more bulletproof and future-proof. Hopefully Safari will fix their bug soon.\n\t\t\tlet d1 = doc.createElement(\"div\"),\n\t\t\t\td2 = doc.createElement(\"div\"),\n\t\t\t\troot = doc && (doc.body || doc.firstElementChild);\n\t\t\tif (root && root.appendChild) {\n\t\t\t\troot.appendChild(d1);\n\t\t\t\td1.appendChild(d2);\n\t\t\t\td1.setAttribute(\"style\", \"position:static;transform:translate3d(0,0,1px)\");\n\t\t\t\t_hasOffsetBug = (d2.offsetParent !== d1);\n\t\t\t\troot.removeChild(d1);\n\t\t\t}\n\t\t}\n\t\treturn doc;\n\t},\n\t_forceNonZeroScale = e => { // walks up the element's ancestors and finds any that had their scale set to 0 via GSAP, and changes them to 0.0001 to ensure that measurements work. Firefox has a bug that causes it to incorrectly report getBoundingClientRect() when scale is 0.\n\t\tlet a, cache;\n\t\twhile (e && e !== _body) {\n\t\t\tcache = e._gsap;\n\t\t\tcache && cache.uncache && cache.get(e, \"x\"); // force re-parsing of transforms if necessary\n\t\t\tif (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) {\n\t\t\t\tcache.scaleX = cache.scaleY = 1e-4;\n\t\t\t\tcache.renderTransform(1, cache);\n\t\t\t\ta ? a.push(cache) : (a = [cache]);\n\t\t\t}\n\t\t\te = e.parentNode;\n\t\t}\n\t\treturn a;\n\t},\n\t// possible future addition: pass an element to _forceDisplay() and it'll walk up all its ancestors and make sure anything with display: none is set to display: block, and if there's no parentNode, it'll add it to the body. It returns an Array that you can then feed to _revertDisplay() to have it revert all the changes it made.\n\t// _forceDisplay = e => {\n\t// \tlet a = [],\n\t// \t\tparent;\n\t// \twhile (e && e !== _body) {\n\t// \t\tparent = e.parentNode;\n\t// \t\t(_win.getComputedStyle(e).display === \"none\" || !parent) && a.push(e, e.style.display, parent) && (e.style.display = \"block\");\n\t// \t\tparent || _body.appendChild(e);\n\t// \t\te = parent;\n\t// \t}\n\t// \treturn a;\n\t// },\n\t// _revertDisplay = a => {\n\t// \tfor (let i = 0; i < a.length; i+=3) {\n\t// \t\ta[i+1] ? (a[i].style.display = a[i+1]) : a[i].style.removeProperty(\"display\");\n\t// \t\ta[i+2] || a[i].parentNode.removeChild(a[i]);\n\t// \t}\n\t// },\n\t_svgTemps = [], //we create 3 elements for SVG, and 3 for other DOM elements and cache them for performance reasons. They get nested in _divContainer and _svgContainer so that just one element is added to the DOM on each successive attempt. Again, performance is key.\n\t_divTemps = [],\n\t_getDocScrollTop = () => _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0,\n\t_getDocScrollLeft = () => _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0,\n\t_svgOwner = element => element.ownerSVGElement || ((element.tagName + \"\").toLowerCase() === \"svg\" ? element : null),\n\t_isFixed = element => {\n\t\tif (_win.getComputedStyle(element).position === \"fixed\") {\n\t\t\treturn true;\n\t\t}\n\t\telement = element.parentNode;\n\t\tif (element && element.nodeType === 1) { // avoid document fragments which will throw an error.\n\t\t\treturn _isFixed(element);\n\t\t}\n\t},\n\t_createSibling = (element, i) => {\n\t\tif (element.parentNode && (_doc || _setDoc(element))) {\n\t\t\tlet svg = _svgOwner(element),\n\t\t\t\tns = svg ? (svg.getAttribute(\"xmlns\") || \"http://www.w3.org/2000/svg\") : \"http://www.w3.org/1999/xhtml\",\n\t\t\t\ttype = svg ? (i ? \"rect\" : \"g\") : \"div\",\n\t\t\t\tx = i !== 2 ? 0 : 100,\n\t\t\t\ty = i === 3 ? 100 : 0,\n\t\t\t\tcss = \"position:absolute;display:block;pointer-events:none;margin:0;padding:0;\",\n\t\t\t\te = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, \"http\"), type) : _doc.createElement(type);\n\t\t\tif (i) {\n\t\t\t\tif (!svg) {\n\t\t\t\t\tif (!_divContainer) {\n\t\t\t\t\t\t_divContainer = _createSibling(element);\n\t\t\t\t\t\t_divContainer.style.cssText = css;\n\t\t\t\t\t}\n\t\t\t\t\te.style.cssText = css + \"width:0.1px;height:0.1px;top:\" + y + \"px;left:\" + x + \"px\";\n\t\t\t\t\t_divContainer.appendChild(e);\n\n\t\t\t\t} else {\n\t\t\t\t\t_svgContainer || (_svgContainer = _createSibling(element));\n\t\t\t\t\te.setAttribute(\"width\", 0.01);\n\t\t\t\t\te.setAttribute(\"height\", 0.01);\n\t\t\t\t\te.setAttribute(\"transform\", \"translate(\" + x + \",\" + y + \")\");\n\t\t\t\t\t_svgContainer.appendChild(e);\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn e;\n\t\t}\n\t\tthrow \"Need document and parent.\";\n\t},\n\t_consolidate = m => { // replaces SVGTransformList.consolidate() because a bug in Firefox causes it to break pointer events. See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800\n\t\tlet c = new Matrix2D(),\n\t\t\ti = 0;\n\t\tfor (; i < m.numberOfItems; i++) {\n\t\t\tc.multiply(m.getItem(i).matrix);\n\t\t}\n\t\treturn c;\n\t},\n\t_getCTM = svg => {\n\t\tlet m = svg.getCTM(),\n\t\t\ttransform;\n\t\tif (!m) { // Firefox returns null for getCTM() on root <svg> elements, so this is a workaround using a <g> that we temporarily append.\n\t\t\ttransform = svg.style[_transformProp];\n\t\t\tsvg.style[_transformProp] = \"none\"; // a bug in Firefox causes css transforms to contaminate the getCTM()\n\t\t\tsvg.appendChild(_gEl);\n\t\t\tm = _gEl.getCTM();\n\t\t\tsvg.removeChild(_gEl);\n\t\t\ttransform ? (svg.style[_transformProp] = transform) : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, \"-$1\").toLowerCase());\n\t\t}\n\t\treturn m || _identityMatrix.clone(); // Firefox will still return null if the <svg> has a width/height of 0 in the browser.\n\t},\n\t_placeSiblings = (element, adjustGOffset) => {\n\t\tlet svg = _svgOwner(element),\n\t\t\tisRootSVG = element === svg,\n\t\t\tsiblings = svg ? _svgTemps : _divTemps,\n\t\t\tparent = element.parentNode,\n\t\t\tcontainer, m, b, x, y, cs;\n\t\tif (element === _win) {\n\t\t\treturn element;\n\t\t}\n\t\tsiblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3));\n\t\tcontainer = svg ? _svgContainer : _divContainer;\n\t\tif (svg) {\n\t\t\tif (isRootSVG) {\n\t\t\t\tb = _getCTM(element);\n\t\t\t\tx = -b.e / b.a;\n\t\t\t\ty = -b.f / b.d;\n\t\t\t\tm = _identityMatrix;\n\t\t\t} else if (element.getBBox) {\n\t\t\t\tb = element.getBBox();\n\t\t\t\tm = element.transform ? element.transform.baseVal : {}; // IE11 doesn't follow the spec.\n\t\t\t\tm = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; // don't call m.consolidate().matrix because a bug in Firefox makes pointer events not work when consolidate() is called on the same tick as getBoundingClientRect()! See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800\n\t\t\t\tx = m.a * b.x + m.c * b.y;\n\t\t\t\ty = m.b * b.x + m.d * b.y;\n\t\t\t} else { // may be a <mask> which has no getBBox() so just use defaults instead of throwing errors.\n\t\t\t\tm = new Matrix2D();\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\tif (adjustGOffset && element.tagName.toLowerCase() === \"g\") {\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\t(isRootSVG ? svg : parent).appendChild(container);\n\t\t\tcontainer.setAttribute(\"transform\", \"matrix(\" + m.a + \",\" + m.b + \",\" + m.c + \",\" + m.d + \",\" + (m.e + x) + \",\" + (m.f + y) + \")\");\n\t\t} else {\n\t\t\tx = y = 0;\n\t\t\tif (_hasOffsetBug) { // some browsers (like Safari) have a bug that causes them to misreport offset values. When an ancestor element has a transform applied, it's supposed to treat it as if it's position: relative (new context). Safari botches this, so we need to find the closest ancestor (between the element and its offsetParent) that has a transform applied and if one is found, grab its offsetTop/Left and subtract them to compensate.\n\t\t\t\tm = element.offsetParent;\n\t\t\t\tb = element;\n\t\t\t\twhile (b && (b = b.parentNode) && b !== m && b.parentNode) {\n\t\t\t\t\tif ((_win.getComputedStyle(b)[_transformProp] + \"\").length > 4) {\n\t\t\t\t\t\tx = b.offsetLeft;\n\t\t\t\t\t\ty = b.offsetTop;\n\t\t\t\t\t\tb = 0;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\tcs = _win.getComputedStyle(element);\n\t\t\tif (cs.position !== \"absolute\" && cs.position !== \"fixed\") {\n\t\t\t\tm = element.offsetParent;\n\t\t\t\twhile (parent && parent !== m) { // if there's an ancestor element between the element and its offsetParent that's scrolled, we must factor that in.\n\t\t\t\t\tx += parent.scrollLeft || 0;\n\t\t\t\t\ty += parent.scrollTop || 0;\n\t\t\t\t\tparent = parent.parentNode;\n\t\t\t\t}\n\t\t\t}\n\t\t\tb = container.style;\n\t\t\tb.top = (element.offsetTop - y) + \"px\";\n\t\t\tb.left = (element.offsetLeft - x) + \"px\";\n\t\t\tb[_transformProp] = cs[_transformProp];\n\t\t\tb[_transformOriginProp] = cs[_transformOriginProp];\n\t\t\t// b.border = m.border;\n\t\t\t// b.borderLeftStyle = m.borderLeftStyle;\n\t\t\t// b.borderTopStyle = m.borderTopStyle;\n\t\t\t// b.borderLeftWidth = m.borderLeftWidth;\n\t\t\t// b.borderTopWidth = m.borderTopWidth;\n\t\t\tb.position = cs.position === \"fixed\" ? \"fixed\" : \"absolute\";\n\t\t\telement.parentNode.appendChild(container);\n\t\t}\n\t\treturn container;\n\t},\n\t_setMatrix = (m, a, b, c, d, e, f) => {\n\t\tm.a = a;\n\t\tm.b = b;\n\t\tm.c = c;\n\t\tm.d = d;\n\t\tm.e = e;\n\t\tm.f = f;\n\t\treturn m;\n\t};\n\nexport class Matrix2D {\n\tconstructor(a=1, b=0, c=0, d=1, e=0, f=0) {\n\t\t_setMatrix(this, a, b, c, d, e, f);\n\t}\n\n\tinverse() {\n\t\tlet {a, b, c, d, e, f} = this,\n\t\t\tdeterminant = (a * d - b * c) || 1e-10;\n\t\treturn _setMatrix(\n\t\t\tthis,\n\t\t\td / determinant,\n\t\t\t-b / determinant,\n\t\t\t-c / determinant,\n\t\t\ta / determinant,\n\t\t\t(c * f - d * e) / determinant,\n\t\t\t-(a * f - b * e) / determinant\n\t\t);\n\t}\n\n\tmultiply(matrix) {\n\t\tlet {a, b, c, d, e, f} = this,\n\t\t\ta2 = matrix.a,\n\t\t\tb2 = matrix.c,\n\t\t\tc2 = matrix.b,\n\t\t\td2 = matrix.d,\n\t\t\te2 = matrix.e,\n\t\t\tf2 = matrix.f;\n\t\treturn _setMatrix(this,\n\t\t\ta2 * a + c2 * c,\n\t\t\ta2 * b + c2 * d,\n\t\t\tb2 * a + d2 * c,\n\t\t\tb2 * b + d2 * d,\n\t\t\te + e2 * a + f2 * c,\n\t\t\tf + e2 * b + f2 * d);\n\t}\n\n\tclone() {\n\t\treturn new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f);\n\t}\n\n\tequals(matrix) {\n\t\tlet {a, b, c, d, e, f} = this;\n\t\treturn (a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f);\n\t}\n\n\tapply(point, decoratee={}) {\n\t\tlet {x, y} = point,\n\t\t\t{a, b, c, d, e, f} = this;\n\t\tdecoratee.x = (x * a + y * c + e) || 0;\n\t\tdecoratee.y = (x * b + y * d + f) || 0;\n\t\treturn decoratee;\n\t}\n\n}\n\n// Feed in an element and it'll return a 2D matrix (optionally inverted) so that you can translate between coordinate spaces.\n// Inverting lets you translate a global point into a local coordinate space. No inverting lets you go the other way.\n// We needed this to work around various browser bugs, like Firefox doesn't accurately report getScreenCTM() when there\n// are transforms applied to ancestor elements.\n// The matrix math to convert any x/y coordinate is as follows, which is wrapped in a convenient apply() method of Matrix2D above:\n// tx = m.a * x + m.c * y + m.e\n// ty = m.b * x + m.d * y + m.f\nexport function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { // adjustGOffset is typically used only when grabbing an element's PARENT's global matrix, and it ignores the x/y offset of any SVG <g> elements because they behave in a special way.\n\tif (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) {\n\t\treturn new Matrix2D();\n\t}\n\tlet zeroScales = _forceNonZeroScale(element),\n\t\tsvg = _svgOwner(element),\n\t\ttemps = svg ? _svgTemps : _divTemps,\n\t\tcontainer = _placeSiblings(element, adjustGOffset),\n\t\tb1 = temps[0].getBoundingClientRect(),\n\t\tb2 = temps[1].getBoundingClientRect(),\n\t\tb3 = temps[2].getBoundingClientRect(),\n\t\tparent = container.parentNode,\n\t\tisFixed = !includeScrollInFixed && _isFixed(element),\n\t\tm = new Matrix2D(\n\t\t\t(b2.left - b1.left) / 100,\n\t\t\t(b2.top - b1.top) / 100,\n\t\t\t(b3.left - b1.left) / 100,\n\t\t\t(b3.top - b1.top) / 100,\n\t\t\tb1.left + (isFixed ? 0 : _getDocScrollLeft()),\n\t\t\tb1.top + (isFixed ? 0 : _getDocScrollTop())\n\t\t);\n\tparent.removeChild(container);\n\tif (zeroScales) {\n\t\tb1 = zeroScales.length;\n\t\twhile (b1--) {\n\t\t\tb2 = zeroScales[b1];\n\t\t\tb2.scaleX = b2.scaleY = 0;\n\t\t\tb2.renderTransform(1, b2);\n\t\t}\n\t}\n\treturn inverse ? m.inverse() : m;\n}\n\nexport { _getDocScrollTop, _getDocScrollLeft, _setDoc, _isFixed, _getCTM };\n\n// export function getMatrix(element) {\n// \t_doc || _setDoc(element);\n// \tlet m = (_win.getComputedStyle(element)[_transformProp] + \"\").substr(7).match(/[-.]*\\d+[.e\\-+]*\\d*[e\\-\\+]*\\d*/g),\n// \t\tis2D = m && m.length === 6;\n// \treturn !m || m.length < 6 ? new Matrix2D() : new Matrix2D(+m[0], +m[1], +m[is2D ? 2 : 4], +m[is2D ? 3 : 5], +m[is2D ? 4 : 12], +m[is2D ? 5 : 13]);\n// }","/*!\n * MotionPathPlugin 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nimport { getRawPath, cacheRawPathMeasurements, getPositionOnPath, pointsToSegment, flatPointsToSegment, sliceRawPath, stringToRawPath, rawPathToString, transformRawPath, convertToPath } from \"./utils/paths.js\";\nimport { getGlobalMatrix } from \"./utils/matrix.js\";\n\nlet _xProps = \"x,translateX,left,marginLeft,xPercent\".split(\",\"),\n\t_yProps = \"y,translateY,top,marginTop,yPercent\".split(\",\"),\n\t_DEG2RAD = Math.PI / 180,\n\tgsap, PropTween, _getUnit, _toArray, _getStyleSaver, _reverting,\n\t_getGSAP = () => gsap || (typeof(window) !== \"undefined\" && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_populateSegmentFromArray = (segment, values, property, mode) => { //mode: 0 = x but don't fill y yet, 1 = y, 2 = x and fill y with 0.\n\t\tlet l = values.length,\n\t\t\tsi = mode === 2 ? 0 : mode,\n\t\t\ti = 0,\n\t\t\tv;\n\t\tfor (; i < l; i++) {\n\t\t\tsegment[si] = v = parseFloat(values[i][property]);\n\t\t\tmode === 2 && (segment[si+1] = 0);\n\t\t\tsi += 2;\n\t\t}\n\t\treturn segment;\n\t},\n\t_getPropNum = (target, prop, unit) => parseFloat(target._gsap.get(target, prop, unit || \"px\")) || 0,\n\t_relativize = segment => {\n\t\tlet x = segment[0],\n\t\t\ty = segment[1],\n\t\t\ti;\n\t\tfor (i = 2; i < segment.length; i+=2) {\n\t\t\tx = (segment[i] += x);\n\t\t\ty = (segment[i+1] += y);\n\t\t}\n\t},\n\t// feed in an array of quadratic bezier points like [{x: 0, y: 0}, ...] and it'll convert it to cubic bezier\n\t// _quadToCubic = points => {\n\t// \tlet cubic = [],\n\t// \t\tl = points.length - 1,\n\t// \t\ti = 1,\n\t// \t\ta, b, c;\n\t// \tfor (; i < l; i+=2) {\n\t// \t\ta = points[i-1];\n\t// \t\tb = points[i];\n\t// \t\tc = points[i+1];\n\t// \t\tcubic.push(a, {x: (2 * b.x + a.x) / 3, y: (2 * b.y + a.y) / 3}, {x: (2 * b.x + c.x) / 3, y: (2 * b.y + c.y) / 3});\n\t// \t}\n\t// \tcubic.push(points[l]);\n\t// \treturn cubic;\n\t// },\n\t_segmentToRawPath = (plugin, segment, target, x, y, slicer, vars, unitX, unitY) => {\n\t\tif (vars.type === \"cubic\") {\n\t\t\tsegment = [segment];\n\t\t} else {\n\t\t\tvars.fromCurrent !== false && segment.unshift(_getPropNum(target, x, unitX), y ? _getPropNum(target, y, unitY) : 0);\n\t\t\tvars.relative && _relativize(segment);\n\t\t\tlet pointFunc = y ? pointsToSegment : flatPointsToSegment;\n\t\t\tsegment = [pointFunc(segment, vars.curviness)];\n\t\t}\n\t\tsegment = slicer(_align(segment, target, vars));\n\t\t_addDimensionalPropTween(plugin, target, x, segment, \"x\", unitX);\n\t\ty && _addDimensionalPropTween(plugin, target, y, segment, \"y\", unitY);\n\t\treturn cacheRawPathMeasurements(segment, vars.resolution || (vars.curviness === 0 ? 20 : 12)); //when curviness is 0, it creates control points right on top of the anchors which makes it more sensitive to resolution, thus we change the default accordingly.\n\t},\n\t_emptyFunc = v => v,\n\t_numExp = /[-+\\.]*\\d+\\.?(?:e-|e\\+)?\\d*/g,\n\t_originToPoint = (element, origin, parentMatrix) => { // origin is an array of normalized values (0-1) in relation to the width/height, so [0.5, 0.5] would be the center. It can also be \"auto\" in which case it will be the top left unless it's a <path>, when it will start at the beginning of the path itself.\n\t\tlet m = getGlobalMatrix(element),\n\t\t\tx = 0,\n\t\t\ty = 0,\n\t\t\tsvg;\n\t\tif ((element.tagName + \"\").toLowerCase() === \"svg\") {\n\t\t\tsvg = element.viewBox.baseVal;\n\t\t\tsvg.width || (svg = {width: +element.getAttribute(\"width\"), height: +element.getAttribute(\"height\")});\n\t\t} else {\n\t\t\tsvg = origin && element.getBBox && element.getBBox();\n\t\t}\n\t\tif (origin && origin !== \"auto\") {\n\t\t\tx = origin.push ? origin[0] * (svg ? svg.width : element.offsetWidth || 0) : origin.x;\n\t\t\ty = origin.push ? origin[1] * (svg ? svg.height : element.offsetHeight || 0) : origin.y;\n\t\t}\n\t\treturn parentMatrix.apply( x || y ? m.apply({x: x, y: y}) : {x: m.e, y: m.f} );\n\t},\n\t_getAlignMatrix = (fromElement, toElement, fromOrigin, toOrigin) => {\n\t\tlet parentMatrix = getGlobalMatrix(fromElement.parentNode, true, true),\n\t\t\tm = parentMatrix.clone().multiply(getGlobalMatrix(toElement)),\n\t\t\tfromPoint = _originToPoint(fromElement, fromOrigin, parentMatrix),\n\t\t\t{x, y} = _originToPoint(toElement, toOrigin, parentMatrix),\n\t\t\tp;\n\t\tm.e = m.f = 0;\n\t\tif (toOrigin === \"auto\" && toElement.getTotalLength && toElement.tagName.toLowerCase() === \"path\") {\n\t\t\tp = toElement.getAttribute(\"d\").match(_numExp) || [];\n\t\t\tp = m.apply({x:+p[0], y:+p[1]});\n\t\t\tx += p.x;\n\t\t\ty += p.y;\n\t\t}\n\t\t//if (p || (toElement.getBBox && fromElement.getBBox && toElement.ownerSVGElement === fromElement.ownerSVGElement)) {\n\t\tif (p) {\n\t\t\tp = m.apply(toElement.getBBox());\n\t\t\tx -= p.x;\n\t\t\ty -= p.y;\n\t\t}\n\t\tm.e = x - fromPoint.x;\n\t\tm.f = y - fromPoint.y;\n\t\treturn m;\n\t},\n\t_align = (rawPath, target, {align, matrix, offsetX, offsetY, alignOrigin}) => {\n\t\tlet x = rawPath[0][0],\n\t\t\ty = rawPath[0][1],\n\t\t\tcurX = _getPropNum(target, \"x\"),\n\t\t\tcurY = _getPropNum(target, \"y\"),\n\t\t\talignTarget, m, p;\n\t\tif (!rawPath || !rawPath.length) {\n\t\t\treturn getRawPath(\"M0,0L0,0\");\n\t\t}\n\t\tif (align) {\n\t\t\tif (align === \"self\" || ((alignTarget = _toArray(align)[0] || target) === target)) {\n\t\t\t\ttransformRawPath(rawPath, 1, 0, 0, 1, curX - x, curY - y);\n\t\t\t} else {\n\t\t\t\tif (alignOrigin && alignOrigin[2] !== false) {\n\t\t\t\t\tgsap.set(target, {transformOrigin:(alignOrigin[0] * 100) + \"% \" + (alignOrigin[1] * 100) + \"%\"});\n\t\t\t\t} else {\n\t\t\t\t\talignOrigin = [_getPropNum(target, \"xPercent\") / -100, _getPropNum(target, \"yPercent\") / -100];\n\t\t\t\t}\n\t\t\t\tm = _getAlignMatrix(target, alignTarget, alignOrigin, \"auto\");\n\t\t\t\tp = m.apply({x: x, y: y});\n\t\t\t\ttransformRawPath(rawPath, m.a, m.b, m.c, m.d, curX + m.e - (p.x - m.e), curY + m.f - (p.y - m.f));\n\t\t\t}\n\t\t}\n\t\tif (matrix) {\n\t\t\ttransformRawPath(rawPath, matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f);\n\t\t} else if (offsetX || offsetY) {\n\t\t\ttransformRawPath(rawPath, 1, 0, 0, 1, offsetX || 0, offsetY || 0);\n\t\t}\n\t\treturn rawPath;\n\t},\n\t_addDimensionalPropTween = (plugin, target, property, rawPath, pathProperty, forceUnit) => {\n\t\tlet cache = target._gsap,\n\t\t\tharness = cache.harness,\n\t\t\talias = (harness && harness.aliases && harness.aliases[property]),\n\t\t\tprop = alias && alias.indexOf(\",\") < 0 ? alias : property,\n\t\t\tpt = plugin._pt = new PropTween(plugin._pt, target, prop, 0, 0, _emptyFunc, 0, cache.set(target, prop, plugin));\n\t\tpt.u = _getUnit(cache.get(target, prop, forceUnit)) || 0;\n\t\tpt.path = rawPath;\n\t\tpt.pp = pathProperty;\n\t\tplugin._props.push(prop);\n\t},\n\t_sliceModifier = (start, end) => rawPath => (start || end !== 1) ? sliceRawPath(rawPath, start, end) : rawPath;\n\n\nexport const MotionPathPlugin = {\n\tversion: \"3.12.7\",\n\tname: \"motionPath\",\n\tregister(core, Plugin, propTween) {\n\t\tgsap = core;\n\t\t_getUnit = gsap.utils.getUnit;\n\t\t_toArray = gsap.utils.toArray;\n\t\t_getStyleSaver = gsap.core.getStyleSaver;\n\t\t_reverting = gsap.core.reverting || function() {};\n\t\tPropTween = propTween;\n\t},\n\tinit(target, vars, tween) {\n\t\tif (!gsap) {\n\t\t\tconsole.warn(\"Please gsap.registerPlugin(MotionPathPlugin)\");\n\t\t\treturn false;\n\t\t}\n\t\tif (!(typeof(vars) === \"object\" && !vars.style) || !vars.path) {\n\t\t\tvars = {path:vars};\n\t\t}\n\t\tlet rawPaths = [],\n\t\t\t{path, autoRotate, unitX, unitY, x, y} = vars,\n\t\t\tfirstObj = path[0],\n\t\t\tslicer = _sliceModifier(vars.start, (\"end\" in vars) ? vars.end : 1),\n\t\t\trawPath, p;\n\t\tthis.rawPaths = rawPaths;\n\t\tthis.target = target;\n\t\tthis.tween = tween;\n\t\tthis.styles = _getStyleSaver && _getStyleSaver(target, \"transform\");\n\t\tif ((this.rotate = (autoRotate || autoRotate === 0))) { //get the rotational data FIRST so that the setTransform() method is called in the correct order in the render() loop - rotation gets set last.\n\t\t\tthis.rOffset = parseFloat(autoRotate) || 0;\n\t\t\tthis.radians = !!vars.useRadians;\n\t\t\tthis.rProp = vars.rotation || \"rotation\"; // rotation property\n\t\t\tthis.rSet = target._gsap.set(target, this.rProp, this); // rotation setter\n\t\t\tthis.ru = _getUnit(target._gsap.get(target, this.rProp)) || 0; // rotation units\n\t\t}\n\t\tif (Array.isArray(path) && !(\"closed\" in path) && typeof(firstObj) !== \"number\") {\n\t\t\tfor (p in firstObj) {\n\t\t\t\tif (!x && ~_xProps.indexOf(p)) {\n\t\t\t\t\tx = p;\n\t\t\t\t} else if (!y && ~_yProps.indexOf(p)) {\n\t\t\t\t\ty = p;\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (x && y) { //correlated values\n\t\t\t\trawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray(_populateSegmentFromArray([], path, x, 0), path, y, 1), target, x, y, slicer, vars, unitX || _getUnit(path[0][x]), unitY || _getUnit(path[0][y])));\n\t\t\t} else {\n\t\t\t\tx = y = 0;\n\t\t\t}\n\t\t\tfor (p in firstObj) {\n\t\t\t\tp !== x && p !== y && rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray([], path, p, 2), target, p, 0, slicer, vars, _getUnit(path[0][p])));\n\t\t\t}\n\t\t} else {\n\t\t\trawPath = slicer(_align(getRawPath(vars.path), target, vars));\n\t\t\tcacheRawPathMeasurements(rawPath, vars.resolution);\n\t\t\trawPaths.push(rawPath);\n\t\t\t_addDimensionalPropTween(this, target, vars.x || \"x\", rawPath, \"x\", vars.unitX || \"px\");\n\t\t\t_addDimensionalPropTween(this, target, vars.y || \"y\", rawPath, \"y\", vars.unitY || \"px\");\n\t\t}\n\t\ttween.vars.immediateRender && this.render(tween.progress(), this);\n\t},\n\trender(ratio, data) {\n\t\tlet rawPaths = data.rawPaths,\n\t\t\ti = rawPaths.length,\n\t\t\tpt = data._pt;\n\t\tif (data.tween._time || !_reverting()) {\n\t\t\tif (ratio > 1) {\n\t\t\t\tratio = 1;\n\t\t\t} else if (ratio < 0) {\n\t\t\t\tratio = 0;\n\t\t\t}\n\t\t\twhile (i--) {\n\t\t\t\tgetPositionOnPath(rawPaths[i], ratio, !i && data.rotate, rawPaths[i]);\n\t\t\t}\n\t\t\twhile (pt) {\n\t\t\t\tpt.set(pt.t, pt.p, pt.path[pt.pp] + pt.u, pt.d, ratio);\n\t\t\t\tpt = pt._next;\n\t\t\t}\n\t\t\tdata.rotate && data.rSet(data.target, data.rProp, rawPaths[0].angle * (data.radians ? _DEG2RAD : 1) + data.rOffset + data.ru, data, ratio);\n\t\t} else {\n\t\t\tdata.styles.revert();\n\t\t}\n\t},\n\tgetLength(path) {\n\t\treturn cacheRawPathMeasurements(getRawPath(path)).totalLength;\n\t},\n\tsliceRawPath,\n\tgetRawPath,\n\tpointsToSegment,\n\tstringToRawPath,\n\trawPathToString,\n\ttransformRawPath,\n\tgetGlobalMatrix,\n\tgetPositionOnPath,\n\tcacheRawPathMeasurements,\n\tconvertToPath: (targets, swap) => _toArray(targets).map(target => convertToPath(target, swap !== false)),\n\tconvertCoordinates(fromElement, toElement, point) {\n\t\tlet m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement));\n\t\treturn point ? m.apply(point) : m;\n\t},\n\tgetAlignMatrix: _getAlignMatrix,\n\tgetRelativePosition(fromElement, toElement, fromOrigin, toOrigin) {\n\t\tlet m =_getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin);\n\t\treturn {x: m.e, y: m.f};\n\t},\n\tarrayToRawPath(value, vars) {\n\t\tvars = vars || {};\n\t\tlet segment = _populateSegmentFromArray(_populateSegmentFromArray([], value, vars.x || \"x\", 0), value, vars.y || \"y\", 1);\n\t\tvars.relative && _relativize(segment);\n\t\treturn [(vars.type === \"cubic\") ? segment : pointsToSegment(segment, vars.curviness)];\n\t}\n};\n\n_getGSAP() && gsap.registerPlugin(MotionPathPlugin);\n\nexport { MotionPathPlugin as default };"],"names":["_isString","value","_roundPrecise","Math","round","_splitSegment","rawPath","segIndex","i","t","segment","shift","subdivideSegment","length","splice","slice","_appendOrMerge","index","prevSeg","l","concat","_svgPathExp","_numbersExp","_scientific","_selectorExp","_DEG2RAD","PI","_RAD2DEG","_sin","sin","_cos","cos","_abs","abs","_sqrt","sqrt","_atan2","atan2","_largeNum","_isNumber","_temp","_temp2","_roundingNum","_wrapProgress","progress","_round","_getSampleIndex","samples","_copyMetaData","source","copy","totalLength","lookup","minLength","resolution","totalPoints","getRawPath","e","test","document","querySelector","getAttribute","_gsPath","_dirty","stringToRawPath","console","warn","reverseSegment","y","reverse","reversed","_typeAttrs","rect","circle","ellipse","line","convertToPath","element","swap","data","x","r","ry","path","rcirc","rycirc","points","w","h","x2","x3","x4","x5","x6","y2","y3","y4","y5","y6","attr","type","tagName","toLowerCase","circ","getBBox","_createPath","ignore","name","createElementNS","call","attributes","nodeName","indexOf","setAttributeNS","nodeValue","_attrToObj","attrs","props","split","obj","rx","width","height","join","cx","cy","x1","y1","match","setAttribute","rawPathToString","_gsRawPath","parentNode","insertBefore","removeChild","getRotationAtBezierT","a","b","c","sliceRawPath","start","end","_isUndefined","loops","max","copyRawPath","_reverseRawPath","skipOuter","offset","min","cacheRawPathMeasurements","wrapsBehind","sShift","eShift","totalSegments","j","wrap","s","getProgressData","eSeg","sSeg","eSegIndex","sSegIndex","ei","si","sameSegment","sameBezier","angle","push","measureSegment","startIndex","bezierQty","xd","xd1","yd","yd1","inv","lengthIndex","segLength","inc","endIndex","samplesIndex","prevLength","pathLength","ax","ay","cp1x","cp1y","cp2x","cp2y","x1a","y1a","x2a","y2a","decoratee","pushToNextIfAtEnd","getPositionOnPath","includeAngle","point","result","transformRawPath","d","tx","ty","arcToSegment","lastX","lastY","largeArcFlag","sweepFlag","angleRad","cosAngle","sinAngle","TWOPI","dx2","dy2","x1_sq","y1_sq","radiiCheck","rx_sq","ry_sq","sq","coef","cx1","cy1","ux","uy","vx","vy","temp","angleStart","acos","angleExtent","isNaN","segments","ceil","angleIncrement","controlLength","ma","mb","mc","md","sx","sy","ex","ey","difX","difY","command","isRelative","startX","startY","beziers","prevCommand","flag1","flag2","replace","m","n","relativeX","relativeY","elements","errorMessage","log","toUpperCase","closed","substr","charAt","pop","flatPointsToSegment","curviness","pointsToSegment","prevX","prevY","dx1","dy1","r1","r2","tl","mx1","mx2","mxm","my1","my2","mym","nextX","nextY","unshift","sl","_setDoc","doc","ownerDocument","_transformProp","style","_transformOriginProp","_win","window","_identityMatrix","Matrix2D","_docElement","_doc","documentElement","_body","body","_gEl","transform","d1","createElement","d2","root","firstElementChild","appendChild","_hasOffsetBug","offsetParent","_svgOwner","ownerSVGElement","_createSibling","svg","ns","css","_svgContainer","_divContainer","cssText","_placeSiblings","adjustGOffset","container","cs","isRootSVG","siblings","_svgTemps","_divTemps","parent","_getCTM","getCTM","removeProperty","clone","f","baseVal","numberOfItems","_consolidate","multiply","getItem","matrix","getComputedStyle","offsetLeft","offsetTop","position","scrollLeft","scrollTop","top","left","_setMatrix","inverse","this","determinant","a2","b2","c2","e2","f2","equals","apply","getGlobalMatrix","includeScrollInFixed","zeroScales","_forceNonZeroScale","cache","_gsap","uncache","get","scaleX","scaleY","renderTransform","temps","b1","getBoundingClientRect","b3","isFixed","_isFixed","nodeType","_getDocScrollLeft","pageXOffset","_getDocScrollTop","pageYOffset","_populateSegmentFromArray","values","property","mode","parseFloat","_getPropNum","target","prop","unit","_relativize","_segmentToRawPath","plugin","slicer","vars","unitX","unitY","fromCurrent","relative","_align","_addDimensionalPropTween","_emptyFunc","v","_originToPoint","origin","parentMatrix","viewBox","offsetWidth","offsetHeight","_getAlignMatrix","fromElement","toElement","fromOrigin","toOrigin","p","fromPoint","getTotalLength","_numExp","gsap","PropTween","_getUnit","_toArray","_getStyleSaver","_reverting","_xProps","_yProps","alignTarget","align","offsetX","offsetY","alignOrigin","curX","curY","set","transformOrigin","pathProperty","forceUnit","harness","alias","aliases","pt","_pt","u","pp","_props","MotionPathPlugin","version","register","core","Plugin","propTween","utils","getUnit","toArray","getStyleSaver","reverting","init","tween","rawPaths","autoRotate","firstObj","_sliceModifier","styles","rotate","rOffset","radians","useRadians","rProp","rotation","rSet","ru","Array","isArray","immediateRender","render","ratio","_time","_next","revert","getLength","targets","map","convertCoordinates","getAlignMatrix","getRelativePosition","arrayToRawPath","_getGSAP","registerPlugin"],"mappings":";;;;;;;;;6MAuBa,SAAZA,EAAYC,SAA2B,iBAAXA,EAQZ,SAAhBC,EAAgBD,UAAUE,KAAKC,MAAc,KAARH,GAAgB,MAAS,EAC9C,SAAhBI,EAAiBC,EAASC,EAAUC,EAAGC,OAClCC,EAAUJ,EAAQC,GACrBI,EAAc,IAANF,EAAU,EAAIG,iBAAiBF,EAASF,EAAGC,OAC/CE,IAAUF,IAAME,EAAQH,EAAI,EAAIE,EAAQG,cAC5CP,EAAQQ,OAAOP,EAAU,EAAGG,EAAQK,MAAM,EAAGP,EAAIG,EAAQ,IACzDD,EAAQI,OAAO,EAAGN,EAAIG,GACf,EAmCQ,SAAjBK,EAAkBV,EAASI,OACtBO,EAAQX,EAAQO,OACnBK,EAAUZ,EAAQW,EAAQ,IAAM,GAChCE,EAAID,EAAQL,OACTI,GAASP,EAAQ,KAAOQ,EAAQC,EAAE,IAAMT,EAAQ,KAAOQ,EAAQC,EAAE,KACpET,EAAUQ,EAAQE,OAAOV,EAAQK,MAAM,IACvCE,KAEDX,EAAQW,GAASP,MAtEfW,EAAc,mDACjBC,EAAc,0CACdC,EAAc,gCACdC,EAAe,4BACfC,EAAWtB,KAAKuB,GAAK,IACrBC,EAAW,IAAMxB,KAAKuB,GACtBE,EAAOzB,KAAK0B,IACZC,EAAO3B,KAAK4B,IACZC,EAAO7B,KAAK8B,IACZC,EAAQ/B,KAAKgC,KACbC,EAASjC,KAAKkC,MACdC,EAAY,IAEZC,EAAY,SAAZA,UAAYtC,SAA2B,iBAAXA,GAE5BuC,EAAQ,GACRC,EAAS,GACTC,EAAe,IACfC,EAAgB,SAAhBA,cAAgBC,UAAazC,KAAKC,OAAOwC,EAAWN,GAAa,EAAII,GAAgBA,IAAmBE,EAAW,EAAK,EAAI,IAC5HC,EAAS,SAATA,OAAS5C,UAAUE,KAAKC,MAAMH,EAAQyC,GAAgBA,GAAiB,GAWvEI,EAAkB,SAAlBA,gBAAmBC,EAASlC,EAAQ+B,OAE/BzB,EAAI4B,EAAQlC,OACfL,KAAOoC,EAAWzB,MACf4B,EAAQvC,GAAKK,EAAQ,QACfL,GAAKuC,EAAQvC,GAAKK,IAC3BL,EAAI,IAAMA,EAAI,aAEPuC,IAAUvC,GAAKK,GAAUL,EAAIW,WAE9BX,EAAIW,EAAIX,EAAIW,EAAI,GASxB6B,EAAgB,SAAhBA,cAAiBC,EAAQC,UACxBA,EAAKC,YAAcF,EAAOE,YACtBF,EAAOF,SACVG,EAAKH,QAAUE,EAAOF,QAAQhC,MAAM,GACpCmC,EAAKE,OAASH,EAAOG,OAAOrC,MAAM,GAClCmC,EAAKG,UAAYJ,EAAOI,UACxBH,EAAKI,WAAaL,EAAOK,YACfL,EAAOM,cACjBL,EAAKK,YAAcN,EAAOM,aAEpBL,GAuBF,SAASM,WAAWvD,OAGzBK,EADGmD,GADJxD,EAASD,EAAUC,IAAUuB,EAAakC,KAAKzD,IAAU0D,SAASC,cAAc3D,IAAkBA,GACpF4D,aAAe5D,EAAQ,SAEjCwD,IAAMxD,EAAQA,EAAM4D,aAAa,OAE/BJ,EAAEK,UACNL,EAAEK,QAAU,KAEbxD,EAAUmD,EAAEK,QAAQ7D,MACAK,EAAQyD,OAAUzD,EAAWmD,EAAEK,QAAQ7D,GAAS+D,gBAAgB/D,IAE7EA,EAAgFD,EAAUC,GAAS+D,gBAAgB/D,GAAUsC,EAAUtC,EAAM,IAAO,CAACA,GAASA,EAAtJgE,QAAQC,KAAK,yDAavB,SAASC,eAAezD,OAE7B0D,EADG5D,EAAI,MAERE,EAAQ2D,UACD7D,EAAIE,EAAQG,OAAQL,GAAK,EAC/B4D,EAAI1D,EAAQF,GACZE,EAAQF,GAAKE,EAAQF,EAAE,GACvBE,EAAQF,EAAE,GAAK4D,EAEhB1D,EAAQ4D,UAAY5D,EAAQ4D,SAK7B,IAcCC,EAAa,CACZC,KAAK,yBACLC,OAAO,UACPC,QAAQ,cACRC,KAAK,eAaA,SAASC,cAAcC,EAASC,OAGrCC,EAAMC,EAAGZ,EAAGa,EAAGC,EAAIC,EAAMC,EAAOC,EAAQC,EAAQC,EAAGC,EAAGC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAAIC,EAF3FC,EAAOvB,EAAQwB,QAAQC,cAC1BC,EAAO,oBAEK,SAATH,GAAoBvB,EAAQ2B,SAGhCrB,EAtCiB,SAAdsB,YAAehD,EAAGiD,OAInBC,EAHGxB,EAAOxB,SAASiD,gBAAgB,6BAA8B,QACjET,EAAO,GAAGpF,MAAM8F,KAAKpD,EAAEqD,YACvBtG,EAAI2F,EAAKtF,WAEV6F,EAAS,IAAMA,EAAS,KACV,IAALlG,GACRmG,EAAOR,EAAK3F,GAAGuG,SAAST,cACpBI,EAAOM,QAAQ,IAAML,EAAO,KAAO,GACtCxB,EAAK8B,eAAe,KAAMN,EAAMR,EAAK3F,GAAG0G,kBAGnC/B,EA0BDsB,CAAY5B,EAAS,qDAC5BsB,EAnBa,SAAbgB,WAAc1D,EAAG2D,WACZC,EAAQD,EAAQA,EAAME,MAAM,KAAO,GACtCC,EAAM,GACN/G,EAAI6G,EAAMxG,QACG,IAALL,GACR+G,EAAIF,EAAM7G,KAAOiD,EAAEI,aAAawD,EAAM7G,KAAO,SAEvC+G,EAYDJ,CAAWtC,EAASN,EAAW6B,IACzB,SAATA,GACHnB,EAAIkB,EAAKqB,GACTtC,EAAKiB,EAAKjB,IAAMD,EAChBD,EAAImB,EAAKnB,EACTZ,EAAI+B,EAAK/B,EACTmB,EAAIY,EAAKsB,MAAY,EAAJxC,EACjBO,EAAIW,EAAKuB,OAAc,EAALxC,EAYjBH,EAXGE,GAAKC,EAWD,KANPW,GAFAF,GADAD,EAAKV,EAAIC,GACCM,GAEAN,GAMQ,KAJlBc,EAAK3B,EAAIc,GAIoB,MAH7Bc,EAAKD,EAAKP,GAG+B,KAAO,CAACK,EAFjDI,EAAKD,EAAKd,EAAKqB,EALfX,EAAKD,EAAKV,EAAIsB,EAMdL,EAAKF,EAAKd,EACuDS,EAAIO,EAAIP,GAAMA,EAAKD,GAAM,EAAGQ,EAAIR,GAAMC,EAAKD,GAAM,EAAGQ,EAAIR,EAAIQ,EAV7HT,EAAKT,EAAIC,GAAK,EAAIsB,GAUmHL,EAAIlB,EAAGiB,EAAIjB,EAAGgB,EAAIhB,EAAGgB,GAAMA,EAAKD,GAAM,EAAGf,EAAGe,GAAMC,EAAKD,GAAM,EAAGf,EAAGe,EAAIf,EAL5Mc,EAAK1B,EAAIc,GAAM,EAAIqB,GAKgMd,EAAIrB,EAAGsB,EAAItB,EAAGsB,GAAMC,EAAKD,GAAM,EAAGtB,EAAGuB,GAAMA,EAAKD,GAAM,EAAGtB,EAAGuB,EAAIvB,EAAGwB,EAAIxB,EAAGyB,EAAIC,EAAID,EAAIE,GAAI4B,KAAK,KAAO,IAElT,KAAO3C,EAAIO,GAAK,IAAMnB,EAAI,KAAOoB,EAAI,MAASD,EAAK,MAASC,EAAK,KAAOD,EAAI,KAGjE,WAATa,GAA8B,YAATA,GAG9Bf,EAFY,WAATe,GACHnB,EAAIC,EAAKiB,EAAKlB,GACDsB,GAEbtB,EAAIkB,EAAKqB,IACTtC,EAAKiB,EAAKjB,IACIqB,GAKfxB,EAAO,MAHPC,EAAImB,EAAKyB,IAGO3C,GAAK,KAFrBb,EAAI+B,EAAK0B,IAEsB,KAAO,CAAC7C,EAAEC,EAAGb,EAAIiB,EAAQL,GADxDI,EAAQH,EAAIsB,GACuDnC,EAAIc,EAAIF,EAAGZ,EAAIc,EAAIF,EAAII,EAAOhB,EAAIc,EAAIF,EAAIC,EAAGb,EAAIiB,EAAQL,EAAIC,EAAGb,EAAGY,EAAIC,EAAGb,EAAIiB,EAAQL,EAAII,EAAOhB,EAAIc,EAAIF,EAAGZ,EAAIc,EAAIF,EAAII,EAAOhB,EAAIc,EAAIF,EAAIC,EAAGb,EAAIiB,EAAQL,EAAIC,EAAGb,GAAGuD,KAAK,KAAO,KAChO,SAATvB,EACVrB,EAAO,IAAMoB,EAAK2B,GAAK,IAAM3B,EAAK4B,GAAK,KAAO5B,EAAKV,GAAK,IAAMU,EAAKL,GAChD,aAATM,GAAgC,YAATA,IAIjCrB,EAAO,KAFPC,GADAM,GAAUT,EAAQhB,aAAa,UAAY,IAAImE,MAAM1G,IAAgB,IAC1DX,SAEM,KADjByD,EAAIkB,EAAO3E,SACgB,KAAO2E,EAAOqC,KAAK,KACjC,YAATvB,IACHrB,GAAQ,IAAMC,EAAI,IAAMZ,EAAI,MAG9Be,EAAK8C,aAAa,IAAKC,gBAAgB/C,EAAKgD,WAAanE,gBAAgBe,KACrED,GAAQD,EAAQuD,aACnBvD,EAAQuD,WAAWC,aAAalD,EAAMN,GACtCA,EAAQuD,WAAWE,YAAYzD,IAEzBM,GAxDCN,EAmET,SAAS0D,qBAAqB7H,EAASF,EAAGC,OAIxCuE,EAHGwD,EAAI9H,EAAQF,GACfiI,EAAI/H,EAAQF,EAAE,GACdkI,EAAIhI,EAAQF,EAAE,UAEfgI,IAAMC,EAAID,GAAK/H,EAEf+H,KADAC,IAAMC,EAAID,GAAKhI,GACL+H,GAAK/H,EACfuE,EAAIyD,GAAMC,GAAKhI,EAAQF,EAAE,GAAKkI,GAAKjI,EAAKgI,GAAKhI,EAAI+H,EACjDA,EAAI9H,EAAQF,EAAE,GAGdgI,KAFAC,EAAI/H,EAAQF,EAAE,IAEJgI,GAAK/H,EAEf+H,KADAC,KAFAC,EAAIhI,EAAQF,EAAE,IAEJiI,GAAKhI,GACL+H,GAAK/H,EACRoC,EAAOT,EAAOqG,GAAMC,GAAKhI,EAAQF,EAAE,GAAKkI,GAAKjI,EAAKgI,GAAKhI,EAAI+H,EAAGxD,GAAKrD,GAGpE,SAASgH,aAAarI,EAASsI,EAAOC,GAC5CA,EArOe,SAAfC,aAAe7I,eAA2B,IAAXA,EAqOzB6I,CAAaD,GAAO,EAAI3I,EAAc2I,IAAQ,EACpDD,EAAQ1I,EAAc0I,IAAU,MAC5BG,EAAQ5I,KAAK6I,IAAI,KAAMhH,EAAK6G,EAAMD,GAAS,OAC9CzD,EApJK,SAAS8D,YAAY3I,WACvBkI,EAAI,GACPhI,EAAI,EACEA,EAAIF,EAAQO,OAAQL,IAC1BgI,EAAEhI,GAAKwC,EAAc1C,EAAQE,GAAIF,EAAQE,GAAGO,MAAM,WAE5CiC,EAAc1C,EAASkI,GA8ItBS,CAAY3I,MACRuI,EAARD,IACHA,EAAQ,EAAIA,EACZC,EAAM,EAAIA,EA/MO,SAAlBK,gBAAmB5I,EAAS6I,OACvB3I,EAAIF,EAAQO,WAChBsI,GAAa7I,EAAQ+D,UACd7D,KACNF,EAAQE,GAAG8D,UAAYH,eAAe7D,EAAQE,IA4M/C0I,CAAgB/D,GAChBA,EAAKhC,YAAc,GAEhByF,EAAQ,GAAKC,EAAM,EAAG,KACrBO,EAASjJ,KAAK8B,MAAM9B,KAAKkJ,IAAIT,EAAOC,IAAQ,EAChDD,GAASQ,EACTP,GAAOO,EAERjE,EAAKhC,aAAemG,yBAAyBnE,OAY5CoE,EAAaC,EAAQC,EAAQjJ,EAAG0C,EAAMwG,EAAevI,EAAGwI,EAXrDC,EAAc,EAANf,EACXgB,EAAIC,gBAAgB3E,EAAMyD,EAAOpG,GAAO,GACxCiB,EAAIqG,gBAAgB3E,EAAM0D,EAAKpG,GAC/BsH,EAAOtG,EAAE/C,QACTsJ,EAAOH,EAAEnJ,QACTuJ,EAAYxG,EAAElD,SACd2J,EAAYL,EAAEtJ,SACd4J,EAAK1G,EAAEjD,EACP4J,EAAKP,EAAErJ,EACP6J,EAAeH,IAAcD,EAC7BK,EAAcH,IAAOC,GAAMC,KAExBT,GAAQb,EAAO,KAClBQ,EAAcU,EAAYC,GAAcG,GAAeF,EAAKC,GAAQE,GAAc7G,EAAEhD,EAAIoJ,EAAEpJ,EACtFJ,EAAc8E,EAAM+E,EAAWE,EAAIP,EAAEpJ,KACxCyJ,IACKX,IACJU,IACIK,GACH7G,EAAEhD,GAAKgD,EAAEhD,EAAIoJ,EAAEpJ,IAAM,EAAIoJ,EAAEpJ,GAC3B0J,EAAK,GACKE,IACVF,GAAMC,KAILjK,KAAK8B,IAAI,GAAK4G,EAAMD,IAAU,KACjCqB,EAAYC,EAAY,GACbzG,EAAEhD,GAAKwJ,EAClBA,IACU5J,EAAc8E,EAAM8E,EAAWE,EAAI1G,EAAEhD,IAAM8I,GACrDW,IAEW,IAARL,EAAEpJ,IACLyJ,GAAaA,EAAY,GAAK/E,EAAKtE,QAEpCqC,EAAO,GAEP/B,EAAI,GADJuI,EAAgBvE,EAAKtE,QACGkI,EAExB5H,IAAOuI,GADPC,EAAIO,GACgCD,GAAaP,EAC5ClJ,EAAI,EAAGA,EAAIW,EAAGX,IAClBQ,EAAekC,EAAMiC,EAAKwE,IAAMD,IAEjCvE,EAAOjC,UAEPuG,EAAiB,IAARhG,EAAEhD,EAAU,EAAIG,iBAAiBmJ,EAAMI,EAAI1G,EAAEhD,GAClDmI,IAAUC,MACbW,EAAS5I,iBAAiBoJ,EAAMI,EAAIE,EAAaT,EAAEpJ,EAAIgD,EAAEhD,EAAIoJ,EAAEpJ,GAC/D4J,IAAgBZ,GAAUD,GAC1BO,EAAKjJ,OAAOqJ,EAAKV,EAAS,IACzBD,GAAUY,IAAOJ,EAAKlJ,OAAO,EAAGsJ,EAAKZ,GACtChJ,EAAI2E,EAAKtE,OACFL,MAELA,EAAI0J,GAAiBD,EAAJzJ,IAAkB2E,EAAKrE,OAAON,EAAG,QAGpDuJ,EAAKQ,MAAQhC,qBAAqBwB,EAAMI,EAAKV,EAAQ,GAErDI,EAAIE,EADJI,GAAMV,GAENhG,EAAIsG,EAAKI,EAAG,GACZJ,EAAKlJ,OAASkJ,EAAK5G,YAAc,EACjC4G,EAAKxG,YAAc4B,EAAK5B,YAAc,EACtCwG,EAAKS,KAAKX,EAAGpG,EAAGoG,EAAGpG,EAAGoG,EAAGpG,EAAGoG,EAAGpG,UAGjC0B,EAAKhC,YAAc,EACZgC,EAIR,SAASsF,eAAe/J,EAASgK,EAAYC,GAC5CD,EAAaA,GAAc,EACtBhK,EAAQqC,UACZrC,EAAQqC,QAAU,GAClBrC,EAAQ0C,OAAS,QAajB5C,EAAGmJ,EAAGhE,EAAID,EAAID,EAAImF,EAAIC,EAAK7E,EAAID,EAAID,EAAIgF,EAAIC,EAAKC,EAAKvK,EAAGwK,EAAa9J,EAAG+J,EAXrE5H,IAAe5C,EAAQ4C,YAAc,GACxC6H,EAAM,EAAI7H,EACV8H,EAAWT,EAAYD,EAAyB,EAAZC,EAAgB,EAAIjK,EAAQG,OAChEiH,EAAKpH,EAAQgK,GACb3C,EAAKrH,EAAQgK,EAAa,GAC1BW,EAAeX,EAAcA,EAAa,EAAKpH,EAAa,EAC5DP,EAAUrC,EAAQqC,QAClBK,EAAS1C,EAAQ0C,OACjBiG,GAAOqB,EAAahK,EAAQ2C,UAAYf,IAAcA,EACtDgJ,EAAavI,EAAQsI,EAAeV,EAAYrH,EAAa,GAC7DzC,EAAS6J,EAAa3H,EAAQsI,EAAa,GAAK,MAEjDtI,EAAQlC,OAASuC,EAAOvC,OAAS,EAC5B8I,EAAIe,EAAa,EAAGf,EAAIyB,EAAUzB,GAAK,EAAG,IAC9ChE,EAAKjF,EAAQiJ,EAAI,GAAK7B,EACtBpC,EAAKhF,EAAQiJ,EAAI,GAAK7B,EACtBrC,EAAK/E,EAAQiJ,GAAK7B,EAClB9B,EAAKtF,EAAQiJ,EAAI,GAAK5B,EACtBhC,EAAKrF,EAAQiJ,EAAI,GAAK5B,EACtBjC,EAAKpF,EAAQiJ,EAAI,GAAK5B,EACtB6C,EAAKC,EAAMC,EAAKC,EAAM,EAClB/I,EAAK2D,GAAM,KAAO3D,EAAKgE,GAAM,KAAOhE,EAAKyD,GAAMzD,EAAK8D,GAAM,IACxC,EAAjBpF,EAAQG,SACXH,EAAQI,OAAO6I,EAAG,GAClBA,GAAK,EACLyB,GAAY,YAGR5K,EAAI,EAAGA,GAAK8C,EAAY9C,IAG5BoK,EAAKC,GAAOA,IAFZpK,EAAI0K,EAAM3K,GAEaC,EAAIkF,EAAK,GADhCqF,EAAM,EAAIvK,IACiCA,EAAIiF,EAAKsF,EAAMvF,IAAOhF,GACjEqK,EAAKC,GAAOA,GAAOtK,EAAIA,EAAIuF,EAAK,EAAIgF,GAAOvK,EAAIsF,EAAKiF,EAAMlF,IAAOrF,IACjEU,EAAIe,EAAM4I,EAAKA,EAAKF,EAAKA,IACjBvB,IACPA,EAAMlI,GAEPN,GAAUM,EACV4B,EAAQsI,KAAkBxK,EAG5BiH,GAAMnC,EACNoC,GAAM/B,KAEHsF,MACHA,GAAczK,EACPwK,EAAetI,EAAQlC,OAAQwK,IACrCtI,EAAQsI,IAAiBC,KAGvBvI,EAAQlC,QAAUwI,MACrB3I,EAAQyC,YAAc+H,EAAYnI,EAAQA,EAAQlC,OAAO,IAAM,EAE3DqK,GADJxK,EAAQ2C,UAAYgG,GACE,SACrBlI,EAAI8J,EAAc,EACbzK,EAAI,EAAGA,EAAI0K,EAAW1K,GAAK6I,EAC/BjG,EAAOjC,KAAQ4B,EAAQkI,GAAezK,IAAOyK,EAAcA,OAI7DvK,EAAQyC,YAAcJ,EAAQ,GAAK,SAE7B2H,EAAa7J,EAASkC,EAAQ2H,EAAa,EAAI,GAAK7J,EAGrD,SAASyI,yBAAyBhJ,EAASgD,OAC7CiI,EAAYjG,EAAQ9E,MACnBA,EAAI+K,EAAajG,EAAS,EAAG9E,EAAIF,EAAQO,OAAQL,IACrDF,EAAQE,GAAG8C,aAAeA,GAAc,GACxCgC,GAAUhF,EAAQE,GAAGK,OACrB0K,GAAcd,eAAenK,EAAQE,WAEtCF,EAAQiD,YAAc+B,EACtBhF,EAAQ6C,YAAcoI,EACfjL,EAID,SAASM,iBAAiBF,EAASF,EAAGC,MACxCA,GAAK,GAAU,GAALA,SACN,MAEJ+K,EAAK9K,EAAQF,GAChBiL,EAAK/K,EAAQF,EAAE,GACfkL,EAAOhL,EAAQF,EAAE,GACjBmL,EAAOjL,EAAQF,EAAE,GACjBoL,EAAOlL,EAAQF,EAAE,GACjBqL,EAAOnL,EAAQF,EAAE,GAGjBsL,EAAMN,GAAME,EAAOF,GAAM/K,EACzBgF,EAAKiG,GAAQE,EAAOF,GAAQjL,EAC5BsL,EAAMN,GAAME,EAAOF,GAAMhL,EACzBqF,EAAK6F,GAAQE,EAAOF,GAAQlL,EAC5BqH,EAAKgE,GAAOrG,EAAKqG,GAAOrL,EACxBsH,EAAKgE,GAAOjG,EAAKiG,GAAOtL,EACxBuL,EAAMJ,GARDlL,EAAQF,EAAE,GAQIoL,GAAQnL,EAC3BwL,EAAMJ,GARDnL,EAAQF,EAAE,GAQIqL,GAAQpL,SAC5BgF,IAAOuG,EAAMvG,GAAMhF,EACnBqF,IAAOmG,EAAMnG,GAAMrF,EACnBC,EAAQI,OAAON,EAAI,EAAG,EACrBqC,EAAOiJ,GACPjJ,EAAOkJ,GACPlJ,EAAOiF,GACPjF,EAAOkF,GACPlF,EAAOiF,GAAMrC,EAAKqC,GAAMrH,GACxBoC,EAAOkF,GAAMjC,EAAKiC,GAAMtH,GACxBoC,EAAO4C,GACP5C,EAAOiD,GACPjD,EAAOmJ,GACPnJ,EAAOoJ,IAERvL,EAAQqC,SAAWrC,EAAQqC,QAAQjC,OAASN,EAAI,EAAKE,EAAQ4C,WAAc,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GACzF,EAIR,SAASwG,gBAAgBxJ,EAASsC,EAAUsJ,EAAWC,GACtDD,EAAYA,GAAa,GACzB5L,EAAQ6C,aAAemG,yBAAyBhJ,IAC5CsC,EAAW,GAAgB,EAAXA,KACnBA,EAAWD,EAAcC,QAIzBG,EAASO,EAAYzC,EAAQwI,EAAKL,EAAKxI,EAAGC,EAFvCF,EAAW,EACdG,EAAUJ,EAAQ,MAEdsC,EAGE,GAAiB,IAAbA,EACVnC,EAAI,EAGJD,GADAE,EAAUJ,EADVC,EAAWD,EAAQO,OAAS,IAEhBA,OAAS,MACf,IACe,EAAjBP,EAAQO,OAAY,KACvBA,EAASP,EAAQ6C,YAAcP,EAC/BoG,EAAMxI,EAAI,GACFwI,GAAO1I,EAAQE,KAAK2C,aAAetC,GAC1CN,EAAWC,EAIZoC,GAAa/B,GADbwI,EAAML,GADNtI,EAAUJ,EAAQC,IACE4C,eACU6F,EAAMK,IAAS,EAE9CtG,EAAUrC,EAAQqC,QAClBO,EAAa5C,EAAQ4C,WACrBzC,EAASH,EAAQyC,YAAcP,EAE/ByG,GADA7I,EAAIE,EAAQ0C,OAAOvC,OAASH,EAAQ0C,UAAUvC,EAASH,EAAQ2C,aAAe,EAAIP,EAAgBC,EAASlC,EAAQ+B,IACzGG,EAAQvC,EAAE,GAAK,GACzBwI,EAAMjG,EAAQvC,IACJK,IACTwI,EAAML,EACNA,EAAMjG,IAAUvC,IAEjBC,EAAK,EAAI6C,IAAiBzC,EAASwI,IAAQL,EAAMK,GAAU7I,EAAI8C,GAC/D9C,EAAyB,KAAlBA,EAAI8C,GACP6I,GAA2B,IAAN1L,IACpBD,EAAI,EAAIE,EAAQG,QACnBL,GAAK,EACLC,EAAI,GACMF,EAAW,EAAID,EAAQO,SACjCL,EAAIC,EAAI,EACRC,EAAUJ,IAAUC,UApCtBE,EAAID,EAAID,EAAW,EACnBG,EAAUJ,EAAQ,UAuCnB4L,EAAUzL,EAAIA,EACdyL,EAAU1L,EAAIA,EACd0L,EAAU/G,KAAO7E,EACjB4L,EAAUxL,QAAUA,EACpBwL,EAAU3L,SAAWA,EACd2L,EAGD,SAASE,kBAAkB9L,EAASsC,EAAUyJ,EAAcC,OAGjEvJ,EAASO,EAAYzC,EAAQwI,EAAKL,EAAKxI,EAAGC,EAAG+H,EAAGwC,EAF7CtK,EAAUJ,EAAQ,GACrBiM,EAASD,GAAS,OAEf1J,EAAW,GAAgB,EAAXA,KACnBA,EAAWD,EAAcC,IAE1BlC,EAAQ0C,QAAUkG,yBAAyBhJ,GACtB,EAAjBA,EAAQO,OAAY,KACvBA,EAASP,EAAQ6C,YAAcP,EAC/BoG,EAAMxI,EAAI,GACFwI,GAAO1I,EAAQE,KAAK2C,aAAetC,GAC1CH,EAAUJ,EAAQE,GAGnBoC,GAAa/B,GADbwI,EAAML,EAAMtI,EAAQyC,eACU6F,EAAMK,IAAS,SAE9CtG,EAAUrC,EAAQqC,QAClBO,EAAa5C,EAAQ4C,WACrBzC,EAASH,EAAQyC,YAAcP,EAE/ByG,GADA7I,EAAIE,EAAQ0C,OAAOvC,OAASH,EAAQ0C,OAAOR,EAAW,KAAO/B,EAASH,EAAQ2C,WAAa3C,EAAQ0C,OAAOvC,OAAS,IAAM,EAAIiC,EAAgBC,EAASlC,EAAQ+B,IACpJG,EAAQvC,EAAE,GAAK,GACzBwI,EAAMjG,EAAQvC,IACJK,IACTwI,EAAML,EACNA,EAAMjG,IAAUvC,IAGjBwK,EAAM,GADNvK,EAAM,EAAI6C,IAAiBzC,EAASwI,IAAQL,EAAMK,GAAU7I,EAAI8C,IAAkB,GAGlFkF,EAAI9H,EADJF,EAAyB,KAAlBA,EAAI8C,IAEXiJ,EAAOvH,EAAInC,GAAQpC,EAAIA,GAAKC,EAAQF,EAAI,GAAKgI,GAAK,EAAIwC,GAAOvK,GAAKC,EAAQF,EAAI,GAAKgI,GAAKwC,GAAOtK,EAAQF,EAAI,GAAKgI,KAAO/H,EAAI+H,GAC3H+D,EAAOnI,EAAIvB,GAAQpC,EAAIA,GAAKC,EAAQF,EAAI,IAAMgI,EAAI9H,EAAQF,EAAE,KAAO,EAAIwK,GAAOvK,GAAKC,EAAQF,EAAI,GAAKgI,GAAKwC,GAAOtK,EAAQF,EAAI,GAAKgI,KAAO/H,EAAI+H,GACxI6D,IACHE,EAAOhC,MAAQ7J,EAAQyC,YAAcoF,qBAAqB7H,EAASF,EAAQ,GAALC,EAAS,EAAI,KAAOA,GAAQ,MAAQC,EAAQ6J,OAAS,GAErHgC,EAMD,SAASC,iBAAiBlM,EAASkI,EAAGC,EAAGC,EAAG+D,EAAGC,EAAIC,WAExDjM,EAASS,EAAGX,EAAGwE,EAAGZ,EADfuF,EAAIrJ,EAAQO,QAEF,IAAL8I,OAERxI,GADAT,EAAUJ,EAAQqJ,IACN9I,OACPL,EAAI,EAAGA,EAAIW,EAAGX,GAAK,EACvBwE,EAAItE,EAAQF,GACZ4D,EAAI1D,EAAQF,EAAE,GACdE,EAAQF,GAAKwE,EAAIwD,EAAIpE,EAAIsE,EAAIgE,EAC7BhM,EAAQF,EAAE,GAAKwE,EAAIyD,EAAIrE,EAAIqI,EAAIE,SAGjCrM,EAAQyD,OAAS,EACVzD,EAMR,SAASsM,aAAaC,EAAOC,EAAOtF,EAAItC,EAAIqF,EAAOwC,EAAcC,EAAWhI,EAAGZ,MAC1EyI,IAAU7H,GAAK8H,IAAU1I,GAG7BoD,EAAKxF,EAAKwF,GACVtC,EAAKlD,EAAKkD,OACN+H,EAAY1C,EAAQ,IAAO9I,EAC9ByL,EAAWpL,EAAKmL,GAChBE,EAAWvL,EAAKqL,GAChBvL,EAAKvB,KAAKuB,GACV0L,EAAa,EAAL1L,EACR2L,GAAOR,EAAQ7H,GAAK,EACpBsI,GAAOR,EAAQ1I,GAAK,EACpB0D,EAAMoF,EAAWG,EAAMF,EAAWG,EAClCvF,GAAOoF,EAAWE,EAAMH,EAAWI,EACnCC,EAAQzF,EAAKA,EACb0F,EAAQzF,EAAKA,EACb0F,EAAaF,GAAS/F,EAAKA,GAAMgG,GAAStI,EAAKA,GAC/B,EAAbuI,IACHjG,EAAKtF,EAAMuL,GAAcjG,EACzBtC,EAAKhD,EAAMuL,GAAcvI,OAEtBwI,EAAQlG,EAAKA,EAChBmG,EAAQzI,EAAKA,EACb0I,GAAOF,EAAQC,EAAUD,EAAQF,EAAUG,EAAQJ,IAAYG,EAAQF,EAAUG,EAAQJ,GACtFK,EAAK,IACRA,EAAK,OAEFC,GAASd,IAAiBC,GAAc,EAAI,GAAK9K,EAAM0L,GAC1DE,EAAetG,EAAKO,EAAM7C,EAApB2I,EACNE,GAAgB7I,EAAK4C,EAAMN,EAArBqG,EAGNjG,EAAYsF,EAAWY,EAAMX,EAAWY,GAFjClB,EAAQ7H,GAAK,EAGpB6C,EAAYsF,EAAWW,EAAMZ,EAAWa,GAFjCjB,EAAQ1I,GAAK,EAGpB4J,GAAMlG,EAAKgG,GAAOtG,EAClByG,GAAMlG,EAAKgG,GAAO7I,EAClBgJ,IAAOpG,EAAKgG,GAAOtG,EACnB2G,IAAOpG,EAAKgG,GAAO7I,EACnBkJ,EAAOJ,EAAKA,EAAKC,EAAKA,EACtBI,GAAeJ,EAAK,GAAM,EAAI,GAAK9N,KAAKmO,KAAKN,EAAK9L,EAAMkM,IACxDG,GAAgBP,EAAKG,EAAKF,EAAKC,EAAK,GAAM,EAAI,GAAK/N,KAAKmO,MAAMN,EAAKE,EAAKD,EAAKE,GAAMjM,EAAMkM,GAAQF,EAAKA,EAAKC,EAAKA,KACjHK,MAAMD,KAAiBA,EAAc7M,IAChCsL,GAA2B,EAAduB,EACjBA,GAAenB,EACLJ,GAAauB,EAAc,IACrCA,GAAenB,GAEhBiB,GAAcjB,EACdmB,GAAenB,MASd5M,EARGiO,EAAWtO,KAAKuO,KAAK1M,EAAKuM,IAAgBnB,EAAQ,IACrD9M,EAAU,GACVqO,EAAiBJ,EAAcE,EAC/BG,EAAgB,EAAI,EAAIhN,EAAK+M,EAAiB,IAAM,EAAI7M,EAAK6M,EAAiB,IAC9EE,EAAK3B,EAAW1F,EAChBsH,EAAK3B,EAAW3F,EAChBuH,EAAK5B,GAAYjI,EACjB8J,EAAK9B,EAAWhI,MAEZ1E,EAAI,EAAGA,EAAIiO,EAAUjO,IAEzBsH,EAAKhG,EADLyI,EAAQ8D,EAAa7N,EAAImO,GAEzB5G,EAAKnG,EAAK2I,GACVyD,EAAKlM,EAAKyI,GAASoE,GACnBV,EAAKrM,EAAK2I,GACVjK,EAAQkK,KAAK1C,EAAK8G,EAAgB7G,EAAIA,EAAK6G,EAAgB9G,EAAIkG,EAAKY,EAAgBX,EAAIA,EAAKW,EAAgBZ,EAAIA,EAAIC,OAGjHzN,EAAI,EAAGA,EAAIF,EAAQO,OAAQL,GAAG,EAClCsH,EAAKxH,EAAQE,GACbuH,EAAKzH,EAAQE,EAAE,GACfF,EAAQE,GAAKsH,EAAK+G,EAAK9G,EAAKgH,EAAKnH,EACjCtH,EAAQE,EAAE,GAAKsH,EAAKgH,EAAK/G,EAAKiH,EAAKnH,SAEpCvH,EAAQE,EAAE,GAAKwE,EACf1E,EAAQE,EAAE,GAAK4D,EACR9D,GAID,SAAS0D,gBAAgByI,GAUvB,SAAP9H,GAAgBsK,EAAIC,EAAIC,EAAIC,GAC3BC,GAAQF,EAAKF,GAAM,EACnBK,GAAQF,EAAKF,GAAM,EACnBxO,EAAQ8J,KAAKyE,EAAKI,EAAMH,EAAKI,EAAMH,EAAKE,EAAMD,EAAKE,EAAMH,EAAIC,OAJ9D5O,EAAGmJ,EAAG3E,EAAGZ,EAAGmL,EAASC,EAAY9O,EAAS+O,EAAQC,EAAQL,EAAMC,EAAMK,EAASC,EAAaC,EAAOC,EARhGtH,GAAKiE,EAAI,IAAIsD,QAAQxO,EAAa,SAAAyO,OAAWC,GAAKD,SAAWC,EAAI,OAAe,KAALA,EAAe,EAAIA,IAAMjI,MAAM3G,IAAgB,GAC7H8D,EAAO,GACP+K,EAAY,EACZC,EAAY,EAEZC,EAAW5H,EAAE3H,OACbyE,EAAS,EACT+K,EAAe,0BAA4B5D,MAOvCA,IAAM+B,MAAMhG,EAAE,KAAOgG,MAAMhG,EAAE,WACjCvE,QAAQqM,IAAID,GACLlL,MAEH3E,EAAI,EAAGA,EAAI4P,EAAU5P,OACzBoP,EAAcL,EACVf,MAAMhG,EAAEhI,IAEXgP,GADAD,EAAU/G,EAAEhI,GAAG+P,iBACW/H,EAAEhI,GAE5BA,IAEDwE,GAAKwD,EAAEhI,EAAI,GACX4D,GAAKoE,EAAEhI,EAAI,GACPgP,IACHxK,GAAKkL,EACL9L,GAAK+L,GAED3P,IACJiP,EAASzK,EACT0K,EAAStL,GAIM,MAAZmL,EACC7O,IACCA,EAAQG,OAAS,IACpBsE,EAAKtE,OAELyE,GAAU5E,EAAQG,QAGpBqP,EAAYT,EAASzK,EACrBmL,EAAYT,EAAStL,EACrB1D,EAAU,CAACsE,EAAGZ,GACde,EAAKqF,KAAK9J,GACVF,GAAK,EACL+O,EAAU,SAGJ,GAAgB,MAAZA,EAILC,IACJU,EAAYC,EAAY,IAHxBzP,EADIA,GACM,CAAC,EAAG,IAMP8J,KAAKxF,EAAGZ,EAAG8L,EAAuB,EAAX1H,EAAEhI,EAAI,GAAQ2P,EAAuB,EAAX3H,EAAEhI,EAAI,GAAS0P,GAAwB,EAAX1H,EAAEhI,EAAI,GAAU2P,GAAwB,EAAX3H,EAAEhI,EAAI,IACxHA,GAAK,OAGC,GAAgB,MAAZ+O,EACVF,EAAOa,EACPZ,EAAOa,EACa,MAAhBP,GAAuC,MAAhBA,IAC1BP,GAAQa,EAAYxP,EAAQA,EAAQG,OAAS,GAC7CyO,GAAQa,EAAYzP,EAAQA,EAAQG,OAAS,IAEzC2O,IACJU,EAAYC,EAAY,GAEzBzP,EAAQ8J,KAAK6E,EAAMC,EAAMtK,EAAGZ,EAAI8L,GAAwB,EAAX1H,EAAEhI,EAAI,GAAU2P,GAAwB,EAAX3H,EAAEhI,EAAI,IAChFA,GAAK,OAGC,GAAgB,MAAZ+O,EACVF,EAAOa,EA7EI,EAAI,GA6EKlL,EAAIkL,GACxBZ,EAAOa,EA9EI,EAAI,GA8EK/L,EAAI+L,GACnBX,IACJU,EAAYC,EAAY,GAEzBD,GAAwB,EAAX1H,EAAEhI,EAAI,GACnB2P,GAAwB,EAAX3H,EAAEhI,EAAI,GACnBE,EAAQ8J,KAAK6E,EAAMC,EAAMY,EApFd,EAAI,GAoFuBlL,EAAIkL,GAAwBC,EApFvD,EAAI,GAoFgE/L,EAAI+L,GAAwBD,EAAWC,GACtH3P,GAAK,OAGC,GAAgB,MAAZ+O,EACVF,EAAOa,EAAYxP,EAAQA,EAAQG,OAAS,GAC5CyO,EAAOa,EAAYzP,EAAQA,EAAQG,OAAS,GAC5CH,EAAQ8J,KAAK0F,EAAYb,EAAMc,EAAYb,EAAMtK,EA3FtC,EAAI,GA2FwCkL,EAAmB,IAAPb,EAAcrK,GAAgBZ,EA3FtF,EAAI,GA2FwF+L,EAAmB,IAAPb,EAAclL,GAAiB8L,EAAYlL,EAAKmL,EAAY/L,GAC/K5D,GAAK,OAGC,GAAgB,MAAZ+O,EACV5K,GAAKuL,EAAWC,EAAYD,EAAYlL,EAAImL,GAC5C3P,GAAK,OAGC,GAAgB,MAAZ+O,EAEV5K,GAAKuL,EAAWC,EAAWD,EAAYC,EAAYnL,GAAKwK,EAAaW,EAAYD,EAAY,IAC7F1P,GAAK,OAGC,GAAgB,MAAZ+O,GAA+B,MAAZA,EACb,MAAZA,IACHvK,EAAIyK,EACJrL,EAAIsL,EACJhP,EAAQ8P,QAAS,IAEF,MAAZjB,GAAyC,GAAtBvN,EAAKkO,EAAYlL,IAAkC,GAAtBhD,EAAKmO,EAAY/L,MACpEO,GAAKuL,EAAWC,EAAWnL,EAAGZ,GACd,MAAZmL,IACH/O,GAAK,IAGP0P,EAAYlL,EACZmL,EAAY/L,OAGN,GAAgB,MAAZmL,EAAiB,IAC3BM,EAAQrH,EAAEhI,EAAE,GACZsP,EAAQtH,EAAEhI,EAAE,GACZ6O,EAAO7G,EAAEhI,EAAE,GACX8O,EAAO9G,EAAEhI,EAAE,GACXmJ,EAAI,EACe,EAAfkG,EAAMhP,SACLgP,EAAMhP,OAAS,GAClByO,EAAOD,EACPA,EAAOS,EACPnG,MAEA2F,EAAOQ,EACPT,EAAOQ,EAAMY,OAAO,GACpB9G,GAAG,GAEJmG,EAAQD,EAAMa,OAAO,GACrBb,EAAQA,EAAMa,OAAO,IAEtBf,EAAU/C,aAAasD,EAAWC,GAAY3H,EAAEhI,EAAE,IAAKgI,EAAEhI,EAAE,IAAKgI,EAAEhI,EAAE,IAAKqP,GAAQC,GAAQN,EAAaU,EAAY,GAAU,EAALb,GAASG,EAAaW,EAAY,GAAU,EAALb,GAC9J9O,GAAKmJ,EACDgG,MACEhG,EAAI,EAAGA,EAAIgG,EAAQ9O,OAAQ8I,IAC/BjJ,EAAQ8J,KAAKmF,EAAQhG,IAGvBuG,EAAYxP,EAAQA,EAAQG,OAAO,GACnCsP,EAAYzP,EAAQA,EAAQG,OAAO,QAGnCoD,QAAQqM,IAAID,UAGd7P,EAAIE,EAAQG,QACJ,GACPsE,EAAKwL,MACLnQ,EAAI,GACME,EAAQ,KAAOA,EAAQF,EAAE,IAAME,EAAQ,KAAOA,EAAQF,EAAE,KAClEE,EAAQ8P,QAAS,GAElBrL,EAAK5B,YAAc+B,EAAS9E,EACrB2E,EAmDD,SAASyL,oBAAoBtL,EAAQuL,YAAAA,IAAAA,EAAU,WACjD7L,EAAIM,EAAO,GACdlB,EAAI,EACJ1D,EAAU,CAACsE,EAAGZ,GACd5D,EAAI,EACEA,EAAI8E,EAAOzE,OAAQL,GAAG,EAC5BE,EAAQ8J,KACPxF,EACAZ,EACAkB,EAAO9E,GACN4D,GAAKkB,EAAO9E,GAAKwE,GAAK6L,EAAY,EAClC7L,EAAIM,EAAO9E,IACX4D,UAGI1D,EAID,SAASoQ,gBAAgBxL,EAAQuL,GAEvC7O,EAAKsD,EAAO,GAAKA,EAAO,IAAM,MAAQtD,EAAKsD,EAAO,GAAKA,EAAO,IAAM,OAASA,EAASA,EAAOvE,MAAM,QAUlGgQ,EAAOC,EAAOxQ,EAAGyQ,EAAKC,EAAKC,EAAIC,EAAQC,EAAIC,EAAKC,EAAKC,EAAKC,EAAKC,EAAKC,EATjExQ,EAAImE,EAAOzE,OAAO,EACrBmE,GAAKM,EAAO,GACZlB,GAAKkB,EAAO,GACZsM,GAAStM,EAAO,GAChBuM,GAASvM,EAAO,GAChB5E,EAAU,CAACsE,EAAGZ,EAAGY,EAAGZ,GACpBiJ,EAAMuE,EAAQ5M,EACdsI,EAAMuE,EAAQzN,EACdoM,EAASrQ,KAAK8B,IAAIqD,EAAOnE,GAAK6D,GAAK,MAAS7E,KAAK8B,IAAIqD,EAAOnE,EAAE,GAAKiD,GAAK,SAErEoM,IACHlL,EAAOkF,KAAKoH,EAAOC,GACnBD,EAAQ5M,EACR6M,EAAQzN,EACRY,EAAIM,EAAOnE,EAAE,GACbiD,EAAIkB,EAAOnE,EAAE,GACbmE,EAAOwM,QAAQ9M,EAAGZ,GAClBjD,GAAG,GAEJ0P,EAAaA,GAA2B,IAAdA,GAAoBA,EAAY,EACrDrQ,EAAI,EAAGA,EAAIW,EAAGX,GAAG,EACrBuQ,EAAQ/L,EACRgM,EAAQ5M,EACRY,EAAI4M,EACJxN,EAAIyN,EACJD,GAAStM,EAAO9E,EAAE,GAClBqR,GAASvM,EAAO9E,EAAE,GACdwE,IAAM4M,GAASxN,IAAMyN,IAGzBZ,EAAM5D,EACN6D,EAAM5D,EACND,EAAMuE,EAAQ5M,EACdsI,EAAMuE,EAAQzN,EAIdiN,IAHAF,EAAKjP,EAAM+O,EAAMA,EAAMC,EAAMA,KAC7BE,EAAKlP,EAAMmL,EAAMA,EAAMC,EAAMA,KAEXuD,EAAY,IADxB3O,EAAM/B,SAACkN,EAAM+D,EAAKH,EAAME,EAAO,YAAK7D,EAAM8D,EAAKF,EAAMC,EAAO,IAIlEK,EAAMxM,IAFNsM,EAAMtM,GAAKA,EAAI+L,IAAUI,EAAKE,EAAKF,EAAK,OACxCI,EAAMvM,GAAK4M,EAAQ5M,IAAMoM,EAAKC,EAAKD,EAAK,IACdE,IAAc,EAALH,GAAUA,EAAKC,GAAO,IAAO,GAAM,IAGtEO,EAAMvN,IAFNqN,EAAMrN,GAAKA,EAAI4M,IAAUG,EAAKE,EAAKF,EAAK,OACxCO,EAAMtN,GAAKyN,EAAQzN,IAAMgN,EAAKC,EAAKD,EAAK,IACdK,IAAc,EAALN,GAAUA,EAAKC,GAAO,IAAO,GAAM,IAClEpM,IAAM+L,GAAS3M,IAAM4M,GACxBtQ,EAAQ8J,KACP3H,EAAOyO,EAAME,GACb3O,EAAO4O,EAAME,GACb9O,EAAOmC,GACPnC,EAAOuB,GACPvB,EAAO0O,EAAMC,GACb3O,EAAO6O,EAAMC,YAIhB3M,IAAM4M,GAASxN,IAAMyN,GAASnR,EAAQG,OAAS,EAAIH,EAAQ8J,KAAK3H,EAAO+O,GAAQ/O,EAAOgP,GAAQhP,EAAO+O,GAAQ/O,EAAOgP,IAAWnR,EAAQG,QAAU,EAC1H,IAAnBH,EAAQG,OACXH,EAAQ8J,KAAKxF,EAAGZ,EAAGY,EAAGZ,EAAGY,EAAGZ,GAClBoM,IACV9P,EAAQI,OAAO,EAAG,GAClBJ,EAAQG,OAASH,EAAQG,OAAS,GAE5BH,EA4ID,SAASwH,gBAAgB5H,GAC3BiC,EAAUjC,EAAQ,MACrBA,EAAU,CAACA,QAIXyR,EAAIlI,EAAGrJ,EAAGE,EAFP6L,EAAS,GACZpL,EAAIb,EAAQO,WAERgJ,EAAI,EAAGA,EAAI1I,EAAG0I,IAAK,KACvBnJ,EAAUJ,EAAQuJ,GAClB0C,GAAU,IAAM1J,EAAOnC,EAAQ,IAAM,IAAMmC,EAAOnC,EAAQ,IAAM,KAChEqR,EAAKrR,EAAQG,OACRL,EAAI,EAAGA,EAAIuR,EAAIvR,IACnB+L,GAAU1J,EAAOnC,EAAQF,MAAQ,IAAMqC,EAAOnC,EAAQF,MAAQ,IAAMqC,EAAOnC,EAAQF,MAAQ,IAAMqC,EAAOnC,EAAQF,MAAQ,IAAMqC,EAAOnC,EAAQF,MAAQ,IAAMqC,EAAOnC,EAAQF,IAAM,IAE7KE,EAAQ8P,SACXjE,GAAU,YAGLA,ECvlCG,SAAVyF,EAAUnN,OACLoN,EAAMpN,EAAQqN,eAAiBrN,IAC7BsN,KAAkBtN,EAAQuN,QAAU,gBAAiBvN,EAAQuN,QAElEC,GADAF,EAAiB,eACuB,eAElCF,EAAI7J,aAAe6J,EAAMA,EAAI7J,iBACpCkK,EAAOC,OACPC,EAAkB,IAAIC,EAClBR,EAAK,CAERS,GADAC,EAAOV,GACWW,gBAClBC,EAAQZ,EAAIa,MACZC,EAAOJ,EAAK/L,gBAAgB,6BAA8B,MAErDwL,MAAMY,UAAY,WAEnBC,EAAKhB,EAAIiB,cAAc,OAC1BC,EAAKlB,EAAIiB,cAAc,OACvBE,EAAOnB,IAAQA,EAAIa,MAAQb,EAAIoB,mBAC5BD,GAAQA,EAAKE,cAChBF,EAAKE,YAAYL,GACjBA,EAAGK,YAAYH,GACfF,EAAGhL,aAAa,QAAS,kDACzBsL,EAAiBJ,EAAGK,eAAiBP,EACrCG,EAAK9K,YAAY2K,WAGZhB,EAsCI,SAAZwB,EAAY5O,UAAWA,EAAQ6O,kBAA6D,SAAxC7O,EAAQwB,QAAU,IAAIC,cAA0BzB,EAAU,MAU7F,SAAjB8O,EAAkB9O,EAASrE,MACtBqE,EAAQuD,aAAeuK,GAAQX,EAAQnN,IAAW,KACjD+O,EAAMH,EAAU5O,GACnBgP,EAAKD,EAAOA,EAAI/P,aAAa,UAAY,6BAAgC,+BACzEuC,EAAOwN,EAAOpT,EAAI,OAAS,IAAO,MAClCwE,EAAU,IAANxE,EAAU,EAAI,IAClB4D,EAAU,IAAN5D,EAAU,IAAM,EACpBsT,EAAM,0EACNrQ,EAAIkP,EAAK/L,gBAAkB+L,EAAK/L,gBAAgBiN,EAAG9D,QAAQ,SAAU,QAAS3J,GAAQuM,EAAKO,cAAc9M,UACtG5F,IACEoT,GAScG,EAAlBA,GAAkCJ,EAAe9O,GACjDpB,EAAEwE,aAAa,QAAS,KACxBxE,EAAEwE,aAAa,SAAU,KACzBxE,EAAEwE,aAAa,YAAa,aAAejD,EAAI,IAAMZ,EAAI,KACzD2P,EAAcT,YAAY7P,KAZrBuQ,KACJA,EAAgBL,EAAe9O,IACjBuN,MAAM6B,QAAUH,GAE/BrQ,EAAE2O,MAAM6B,QAAUH,EAAM,gCAAkC1P,EAAI,WAAaY,EAAI,KAC/EgP,EAAcV,YAAY7P,KAUrBA,OAEF,4BAuBU,SAAjByQ,GAAkBrP,EAASsP,OAKzBC,EAAWpE,EAAGvH,EAAGzD,EAAGZ,EAAGiQ,EAJpBT,EAAMH,EAAU5O,GACnByP,EAAYzP,IAAY+O,EACxBW,EAAWX,EAAMY,EAAYC,EAC7BC,EAAS7P,EAAQuD,cAEdvD,IAAYyN,SACRzN,KAER0P,EAAS1T,QAAU0T,EAAS/J,KAAKmJ,EAAe9O,EAAS,GAAI8O,EAAe9O,EAAS,GAAI8O,EAAe9O,EAAS,IACjHuP,EAAYR,EAAMG,EAAgBC,EAC9BJ,EACCU,GAEHtP,IADAyD,EA1BO,SAAVkM,QAAUf,OAERZ,EADGhD,EAAI4D,EAAIgB,gBAEP5E,IACJgD,EAAYY,EAAIxB,MAAMD,GACtByB,EAAIxB,MAAMD,GAAkB,OAC5ByB,EAAIN,YAAYP,GAChB/C,EAAI+C,EAAK6B,SACThB,EAAItL,YAAYyK,GAChBC,EAAaY,EAAIxB,MAAMD,GAAkBa,EAAaY,EAAIxB,MAAMyC,eAAe1C,EAAepC,QAAQ,WAAY,OAAOzJ,gBAEnH0J,GAAKwC,EAAgBsC,QAetBH,CAAQ9P,IACLpB,EAAIgF,EAAED,EACbpE,GAAKqE,EAAEsM,EAAItM,EAAEgE,EACbuD,EAAIwC,GACM3N,EAAQ2B,SAClBiC,EAAI5D,EAAQ2B,UAGZxB,GADAgL,GADAA,EAAInL,EAAQmO,UAAYnO,EAAQmO,UAAUgC,QAAU,IAC7CC,cAAoD,EAAlBjF,EAAEiF,cAzC/B,SAAfC,aAAelF,WACVtH,EAAI,IAAI+J,EACXjS,EAAI,EACEA,EAAIwP,EAAEiF,cAAezU,IAC3BkI,EAAEyM,SAASnF,EAAEoF,QAAQ5U,GAAG6U,eAElB3M,EAmC0DwM,CAAalF,GAAKA,EAAEoF,QAAQ,GAAGC,OAAvE7C,GACjBhK,EAAIC,EAAEzD,EAAIgL,EAAEtH,EAAID,EAAErE,EACxBA,EAAI4L,EAAEvH,EAAIA,EAAEzD,EAAIgL,EAAEvD,EAAIhE,EAAErE,IAExB4L,EAAI,IAAIyC,EACRzN,EAAIZ,EAAI,GAEL+P,GAAmD,MAAlCtP,EAAQwB,QAAQC,gBACpCtB,EAAIZ,EAAI,IAERkQ,EAAYV,EAAMc,GAAQpB,YAAYc,GACvCA,EAAUnM,aAAa,YAAa,UAAY+H,EAAExH,EAAI,IAAMwH,EAAEvH,EAAI,IAAMuH,EAAEtH,EAAI,IAAMsH,EAAEvD,EAAI,KAAOuD,EAAEvM,EAAIuB,GAAK,KAAOgL,EAAE+E,EAAI3Q,GAAK,SACxH,IACNY,EAAIZ,EAAI,EACJmP,MACHvD,EAAInL,EAAQ2O,aACZ/K,EAAI5D,GACS4D,EAANA,GAAUA,EAAEL,aAAeK,IAAMuH,GAAKvH,EAAEL,YACe,GAAxDkK,EAAKgD,iBAAiB7M,GAAG0J,GAAkB,IAAItR,SACnDmE,EAAIyD,EAAE8M,WACNnR,EAAIqE,EAAE+M,UACN/M,EAAI,MAKa,cADpB4L,EAAK/B,EAAKgD,iBAAiBzQ,IACpB4Q,UAA2C,UAAhBpB,EAAGoB,aACpCzF,EAAInL,EAAQ2O,aACLkB,GAAUA,IAAW1E,GAC3BhL,GAAK0P,EAAOgB,YAAc,EAC1BtR,GAAKsQ,EAAOiB,WAAa,EACzBjB,EAASA,EAAOtM,YAGlBK,EAAI2L,EAAUhC,OACZwD,IAAO/Q,EAAQ2Q,UAAYpR,EAAK,KAClCqE,EAAEoN,KAAQhR,EAAQ0Q,WAAavQ,EAAK,KACpCyD,EAAE0J,GAAkBkC,EAAGlC,GACvB1J,EAAE4J,GAAwBgC,EAAGhC,GAM7B5J,EAAEgN,SAA2B,UAAhBpB,EAAGoB,SAAuB,QAAU,WACjD5Q,EAAQuD,WAAWkL,YAAYc,UAEzBA,EAEK,SAAb0B,GAAc9F,EAAGxH,EAAGC,EAAGC,EAAG+D,EAAGhJ,EAAGsR,UAC/B/E,EAAExH,EAAIA,EACNwH,EAAEvH,EAAIA,EACNuH,EAAEtH,EAAIA,EACNsH,EAAEvD,EAAIA,EACNuD,EAAEvM,EAAIA,EACNuM,EAAE+E,EAAIA,EACC/E,EA/MT,IAAI2C,EAAML,EAAMI,EAAaG,EAAOmB,EAAeD,EAAevB,EAAiBO,EAGlFQ,IAFApB,EAAiB,YACjBE,EAAuBF,EAAiB,SAgExCqC,EAAY,GACZC,EAAY,GA+IAhC,0BAKZsD,QAAA,uBACMvN,EAAoBwN,KAApBxN,EAAGC,EAAiBuN,KAAjBvN,EAAGC,EAAcsN,KAAdtN,EAAG+D,EAAWuJ,KAAXvJ,EAAGhJ,EAAQuS,KAARvS,EAAGsR,EAAKiB,KAALjB,EACnBkB,EAAezN,EAAIiE,EAAIhE,EAAIC,GAAM,aAC3BoN,GACNE,KACAvJ,EAAIwJ,GACHxN,EAAIwN,GACJvN,EAAIuN,EACLzN,EAAIyN,GACHvN,EAAIqM,EAAItI,EAAIhJ,GAAKwS,IAChBzN,EAAIuM,EAAItM,EAAIhF,GAAKwS,MAIrBd,SAAA,kBAASE,OACH7M,EAAoBwN,KAApBxN,EAAGC,EAAiBuN,KAAjBvN,EAAGC,EAAcsN,KAAdtN,EAAG+D,EAAWuJ,KAAXvJ,EAAGhJ,EAAQuS,KAARvS,EAAGsR,EAAKiB,KAALjB,EACnBmB,EAAKb,EAAO7M,EACZ2N,EAAKd,EAAO3M,EACZ0N,EAAKf,EAAO5M,EACZ0K,EAAKkC,EAAO5I,EACZ4J,EAAKhB,EAAO5R,EACZ6S,EAAKjB,EAAON,SACNe,GAAWE,KACjBE,EAAK1N,EAAI4N,EAAK1N,EACdwN,EAAKzN,EAAI2N,EAAK3J,EACd0J,EAAK3N,EAAI2K,EAAKzK,EACdyN,EAAK1N,EAAI0K,EAAK1G,EACdhJ,EAAI4S,EAAK7N,EAAI8N,EAAK5N,EAClBqM,EAAIsB,EAAK5N,EAAI6N,EAAK7J,MAGpBqI,MAAA,wBACQ,IAAIrC,SAASuD,KAAKxN,EAAGwN,KAAKvN,EAAGuN,KAAKtN,EAAGsN,KAAKvJ,EAAGuJ,KAAKvS,EAAGuS,KAAKjB,MAGlEwB,OAAA,gBAAOlB,OACD7M,EAAoBwN,KAApBxN,EAAGC,EAAiBuN,KAAjBvN,EAAGC,EAAcsN,KAAdtN,EAAG+D,EAAWuJ,KAAXvJ,EAAGhJ,EAAQuS,KAARvS,EAAGsR,EAAKiB,KAALjB,SACZvM,IAAM6M,EAAO7M,GAAKC,IAAM4M,EAAO5M,GAAKC,IAAM2M,EAAO3M,GAAK+D,IAAM4I,EAAO5I,GAAKhJ,IAAM4R,EAAO5R,GAAKsR,IAAMM,EAAON,KAGhHyB,MAAA,eAAMlK,EAAOJ,YAAAA,IAAAA,EAAU,QACjBlH,EAAQsH,EAARtH,EAAGZ,EAAKkI,EAALlI,EACNoE,EAAoBwN,KAApBxN,EAAGC,EAAiBuN,KAAjBvN,EAAGC,EAAcsN,KAAdtN,EAAG+D,EAAWuJ,KAAXvJ,EAAGhJ,EAAQuS,KAARvS,EAAGsR,EAAKiB,KAALjB,SACjB7I,EAAUlH,EAAKA,EAAIwD,EAAIpE,EAAIsE,EAAIjF,GAAM,EACrCyI,EAAU9H,EAAKY,EAAIyD,EAAIrE,EAAIqI,EAAIsI,GAAM,EAC9B7I,+BAjDI1D,EAAKC,EAAKC,EAAK+D,EAAKhJ,EAAKsR,YAAzBvM,IAAAA,EAAE,YAAGC,IAAAA,EAAE,YAAGC,IAAAA,EAAE,YAAG+D,IAAAA,EAAE,YAAGhJ,IAAAA,EAAE,YAAGsR,IAAAA,EAAE,GACtCe,GAAWE,KAAMxN,EAAGC,EAAGC,EAAG+D,EAAGhJ,EAAGsR,GA4D3B,SAAS0B,gBAAgB5R,EAASkR,EAAS5B,EAAeuC,OAC3D7R,IAAYA,EAAQuD,aAAeuK,GAAQX,EAAQnN,IAAU+N,kBAAoB/N,SAC9E,IAAI4N,MAERkE,EAlPiB,SAArBC,mBAAqBnT,WAChB+E,EAAGqO,EACApT,GAAKA,IAAMoP,IACjBgE,EAAQpT,EAAEqT,QACDD,EAAME,SAAWF,EAAMG,IAAIvT,EAAG,KACnCoT,IAAUA,EAAMI,SAAWJ,EAAMK,QAAUL,EAAMM,kBACpDN,EAAMI,OAASJ,EAAMK,OAAS,KAC9BL,EAAMM,gBAAgB,EAAGN,GACzBrO,EAAIA,EAAEgC,KAAKqM,GAAUrO,EAAI,CAACqO,IAE3BpT,EAAIA,EAAE2E,kBAEAI,EAsOSoO,CAAmB/R,GAEnCuS,EADM3D,EAAU5O,GACF2P,EAAYC,EAC1BL,EAAYF,GAAerP,EAASsP,GACpCkD,EAAKD,EAAM,GAAGE,wBACdnB,EAAKiB,EAAM,GAAGE,wBACdC,EAAKH,EAAM,GAAGE,wBACd5C,EAASN,EAAUhM,WACnBoP,GAAWd,GArND,SAAXe,SAAW5S,SACsC,UAA5CyN,EAAKgD,iBAAiBzQ,GAAS4Q,YAGnC5Q,EAAUA,EAAQuD,aACkB,IAArBvD,EAAQ6S,SACfD,SAAS5S,WA+MkB4S,CAAS5S,GAC5CmL,EAAI,IAAIyC,GACN0D,EAAGN,KAAOwB,EAAGxB,MAAQ,KACrBM,EAAGP,IAAMyB,EAAGzB,KAAO,KACnB2B,EAAG1B,KAAOwB,EAAGxB,MAAQ,KACrB0B,EAAG3B,IAAMyB,EAAGzB,KAAO,IACpByB,EAAGxB,MAAQ2B,EAAU,EA7NH,SAApBG,2BAA0BrF,EAAKsF,aAAejF,EAAK+C,YAAchD,EAAYgD,YAAc7C,EAAM6C,YAAc,EA6NpFiC,IACzBN,EAAGzB,KAAO4B,EAAU,EA/NH,SAAnBK,0BAAyBvF,EAAKwF,aAAgBnF,EAAKgD,WAAajD,EAAYiD,WAAa9C,EAAM8C,WAAa,EA+NlFkC,QAE1BnD,EAAOpM,YAAY8L,GACfuC,MACHU,EAAKV,EAAW9V,OACTwW,MACNlB,EAAKQ,EAAWU,IACbJ,OAASd,EAAGe,OAAS,EACxBf,EAAGgB,gBAAgB,EAAGhB,UAGjBJ,EAAU/F,EAAE+F,UAAY/F,ECtSH,SAA5B+H,GAA6BrX,EAASsX,EAAQC,EAAUC,WACnD/W,EAAI6W,EAAOnX,OACduJ,EAAc,IAAT8N,EAAa,EAAIA,EACtB1X,EAAI,EAEEA,EAAIW,EAAGX,IACbE,EAAQ0J,GAAU+N,WAAWH,EAAOxX,GAAGyX,IAC9B,IAATC,IAAexX,EAAQ0J,EAAG,GAAK,GAC/BA,GAAM,SAEA1J,EAEM,SAAd0X,GAAeC,EAAQC,EAAMC,UAASJ,WAAWE,EAAOvB,MAAME,IAAIqB,EAAQC,EAAMC,GAAQ,QAAU,EACpF,SAAdC,GAAc9X,OAGZF,EAFGwE,EAAItE,EAAQ,GACf0D,EAAI1D,EAAQ,OAERF,EAAI,EAAGA,EAAIE,EAAQG,OAAQL,GAAG,EAClCwE,EAAKtE,EAAQF,IAAMwE,EACnBZ,EAAK1D,EAAQF,EAAE,IAAM4D,EAkBH,SAApBqU,GAAqBC,EAAQhY,EAAS2X,EAAQrT,EAAGZ,EAAGuU,EAAQC,EAAMC,EAAOC,UAEvEpY,EADiB,UAAdkY,EAAKxS,KACE,CAAC1F,KAEU,IAArBkY,EAAKG,aAAyBrY,EAAQoR,QAAQsG,GAAYC,EAAQrT,EAAG6T,GAAQzU,EAAIgU,GAAYC,EAAQjU,EAAG0U,GAAS,GACjHF,EAAKI,UAAYR,GAAY9X,GAEnB,EADM0D,EAAI0M,gBAAkBF,qBACjBlQ,EAASkY,EAAK/H,aAEpCnQ,EAAUiY,EAAOM,GAAOvY,EAAS2X,EAAQO,IACzCM,GAAyBR,EAAQL,EAAQrT,EAAGtE,EAAS,IAAKmY,GAC1DzU,GAAK8U,GAAyBR,EAAQL,EAAQjU,EAAG1D,EAAS,IAAKoY,GACxDxP,yBAAyB5I,EAASkY,EAAKtV,aAAkC,IAAnBsV,EAAK/H,UAAkB,GAAK,KAE7E,SAAbsI,GAAaC,UAAKA,EAED,SAAjBC,GAAkBxU,EAASyU,EAAQC,OAIjC3F,EAHG5D,EAAIyG,gBAAgB5R,GACvBG,EAAI,EACJZ,EAAI,QAEwC,SAAxCS,EAAQwB,QAAU,IAAIC,eAC1BsN,EAAM/O,EAAQ2U,QAAQxE,SAClBvN,QAAUmM,EAAM,CAACnM,OAAQ5C,EAAQhB,aAAa,SAAU6D,QAAS7C,EAAQhB,aAAa,YAE1F+P,EAAM0F,GAAUzU,EAAQ2B,SAAW3B,EAAQ2B,UAExC8S,GAAqB,SAAXA,IACbtU,EAAIsU,EAAO9O,KAAO8O,EAAO,IAAM1F,EAAMA,EAAInM,MAAQ5C,EAAQ4U,aAAe,GAAKH,EAAOtU,EACpFZ,EAAIkV,EAAO9O,KAAO8O,EAAO,IAAM1F,EAAMA,EAAIlM,OAAS7C,EAAQ6U,cAAgB,GAAKJ,EAAOlV,GAEhFmV,EAAa/C,MAAOxR,GAAKZ,EAAI4L,EAAEwG,MAAM,CAACxR,EAAGA,EAAGZ,EAAGA,IAAM,CAACY,EAAGgL,EAAEvM,EAAGW,EAAG4L,EAAE+E,IAEzD,SAAlB4E,GAAmBC,EAAaC,EAAWC,EAAYC,OAKrDC,EAJGT,EAAe9C,gBAAgBmD,EAAYxR,YAAY,GAAM,GAChE4H,EAAIuJ,EAAazE,QAAQK,SAASsB,gBAAgBoD,IAClDI,EAAYZ,GAAeO,EAAaE,EAAYP,KAC3CF,GAAeQ,EAAWE,EAAUR,GAA5CvU,IAAAA,EAAGZ,IAAAA,SAEL4L,EAAEvM,EAAIuM,EAAE+E,EAAI,EACK,SAAbgF,GAAuBF,EAAUK,gBAAsD,SAApCL,EAAUxT,QAAQC,gBACxE0T,EAAIH,EAAUhW,aAAa,KAAKmE,MAAMmS,KAAY,GAElDnV,IADAgV,EAAIhK,EAAEwG,MAAM,CAACxR,GAAGgV,EAAE,GAAI5V,GAAG4V,EAAE,MACpBhV,EACPZ,GAAK4V,EAAE5V,GAGJ4V,IAEHhV,IADAgV,EAAIhK,EAAEwG,MAAMqD,EAAUrT,YACfxB,EACPZ,GAAK4V,EAAE5V,GAER4L,EAAEvM,EAAIuB,EAAIiV,EAAUjV,EACpBgL,EAAE+E,EAAI3Q,EAAI6V,EAAU7V,EACb4L,EAhGT,IAGCoK,EAAMC,EAAWC,EAAUC,EAAUC,EAAgBC,EAHlDC,EAAU,wCAAwCpT,MAAM,KAC3DqT,GAAU,sCAAsCrT,MAAM,KACtD7F,EAAWtB,KAAKuB,GAAK,IAuDrByY,GAAU,+BAyCVlB,GAAS,SAATA,OAAU3Y,EAAS+X,SAKjBuC,EAAa5K,EAAGgK,EALUa,IAAAA,MAAOxF,IAAAA,OAAQyF,IAAAA,QAASC,IAAAA,QAASC,IAAAA,YACxDhW,EAAI1E,EAAQ,GAAG,GAClB8D,EAAI9D,EAAQ,GAAG,GACf2a,EAAO7C,GAAYC,EAAQ,KAC3B6C,EAAO9C,GAAYC,EAAQ,YAEvB/X,GAAYA,EAAQO,QAGrBga,IACW,SAAVA,IAAsBD,EAAcL,EAASM,GAAO,IAAMxC,KAAYA,EACzE7L,iBAAiBlM,EAAS,EAAG,EAAG,EAAG,EAAG2a,EAAOjW,EAAGkW,EAAO9W,IAEnD4W,IAAkC,IAAnBA,EAAY,GAC9BZ,EAAKe,IAAI9C,EAAQ,CAAC+C,gBAAkC,IAAjBJ,EAAY,GAAY,KAAyB,IAAjBA,EAAY,GAAY,MAE3FA,EAAc,CAAC5C,GAAYC,EAAQ,aAAe,IAAKD,GAAYC,EAAQ,aAAe,KAG3F2B,GADAhK,EAAI2J,GAAgBtB,EAAQuC,EAAaI,EAAa,SAChDxE,MAAM,CAACxR,EAAGA,EAAGZ,EAAGA,IACtBoI,iBAAiBlM,EAAS0P,EAAExH,EAAGwH,EAAEvH,EAAGuH,EAAEtH,EAAGsH,EAAEvD,EAAGwO,EAAOjL,EAAEvM,GAAKuW,EAAEhV,EAAIgL,EAAEvM,GAAIyX,EAAOlL,EAAE+E,GAAKiF,EAAE5V,EAAI4L,EAAE+E,MAG5FM,EACH7I,iBAAiBlM,EAAS+U,EAAO7M,EAAG6M,EAAO5M,EAAG4M,EAAO3M,EAAG2M,EAAO5I,EAAG4I,EAAO5R,EAAG4R,EAAON,IACzE+F,GAAWC,IACrBvO,iBAAiBlM,EAAS,EAAG,EAAG,EAAG,EAAGwa,GAAW,EAAGC,GAAW,GAEzDza,GArBCkD,WAAW,aAuBpB0V,GAA2B,SAA3BA,yBAA4BR,EAAQL,EAAQJ,EAAU3X,EAAS+a,EAAcC,OACxEzE,EAAQwB,EAAOvB,MAClByE,EAAU1E,EAAM0E,QAChBC,EAASD,GAAWA,EAAQE,SAAWF,EAAQE,QAAQxD,GACvDK,EAAOkD,GAASA,EAAMxU,QAAQ,KAAO,EAAIwU,EAAQvD,EACjDyD,EAAKhD,EAAOiD,IAAM,IAAItB,EAAU3B,EAAOiD,IAAKtD,EAAQC,EAAM,EAAG,EAAGa,GAAY,EAAGtC,EAAMsE,IAAI9C,EAAQC,EAAMI,IACxGgD,EAAGE,EAAItB,EAASzD,EAAMG,IAAIqB,EAAQC,EAAMgD,KAAe,EACvDI,EAAGvW,KAAO7E,EACVob,EAAGG,GAAKR,EACR3C,EAAOoD,OAAOtR,KAAK8N,IAKRyD,EAAmB,CAC/BC,QAAS,SACTrV,KAAM,aACNsV,2BAASC,EAAMC,EAAQC,GAEtB9B,GADAF,EAAO8B,GACSG,MAAMC,QACtB/B,EAAWH,EAAKiC,MAAME,QACtB/B,EAAiBJ,EAAK8B,KAAKM,cAC3B/B,EAAaL,EAAK8B,KAAKO,WAAa,aACpCpC,EAAY+B,GAEbM,mBAAKrE,EAAQO,EAAM+D,OACbvC,SACJnW,QAAQC,KAAK,iDACN,EAEe,iBAAV0U,IAAuBA,EAAKxG,OAAWwG,EAAKzT,OACxDyT,EAAO,CAACzT,KAAKyT,QAMbtY,EAAS0Z,EAJN4C,EAAW,GACbzX,EAAwCyT,EAAxCzT,KAAM0X,EAAkCjE,EAAlCiE,WAAYhE,EAAsBD,EAAtBC,MAAOC,EAAeF,EAAfE,MAAO9T,EAAQ4T,EAAR5T,EAAGZ,EAAKwU,EAALxU,EACpC0Y,EAAW3X,EAAK,GAChBwT,EAzBe,SAAjBoE,eAAkBnU,EAAOC,UAAQ,SAAAvI,UAAYsI,GAAiB,IAARC,EAAaF,aAAarI,EAASsI,EAAOC,GAAOvI,GAyB5Fyc,CAAenE,EAAKhQ,MAAQ,QAASgQ,EAAQA,EAAK/P,IAAM,WAE7D+T,SAAWA,OACXvE,OAASA,OACTsE,MAAQA,OACRK,OAASxC,GAAkBA,EAAenC,EAAQ,cAClDrC,KAAKiH,OAAUJ,GAA6B,IAAfA,UAC5BK,QAAU/E,WAAW0E,IAAe,OACpCM,UAAYvE,EAAKwE,gBACjBC,MAAQzE,EAAK0E,UAAY,gBACzBC,KAAOlF,EAAOvB,MAAMqE,IAAI9C,EAAQrC,KAAKqH,MAAOrH,WAC5CwH,GAAKlD,EAASjC,EAAOvB,MAAME,IAAIqB,EAAQrC,KAAKqH,SAAW,IAEzDI,MAAMC,QAAQvY,IAAW,WAAYA,GAA8B,iBAAd2X,EAkBxDxT,yBADAhJ,EAAUqY,EAAOM,GAAOzV,WAAWoV,EAAKzT,MAAOkT,EAAQO,IACrBA,EAAKtV,YACvCsZ,EAASpS,KAAKlK,GACd4Y,GAAyBlD,KAAMqC,EAAQO,EAAK5T,GAAK,IAAK1E,EAAS,IAAKsY,EAAKC,OAAS,MAClFK,GAAyBlD,KAAMqC,EAAQO,EAAKxU,GAAK,IAAK9D,EAAS,IAAKsY,EAAKE,OAAS,UArBF,KAC3EkB,KAAK8C,GACJ9X,IAAM0V,EAAQ1T,QAAQgT,GAC1BhV,EAAIgV,GACO5V,IAAMuW,GAAQ3T,QAAQgT,KACjC5V,EAAI4V,OAQDA,KALDhV,GAAKZ,EACRwY,EAASpS,KAAKiO,GAAkBzC,KAAM+B,GAA0BA,GAA0B,GAAI5S,EAAMH,EAAG,GAAIG,EAAMf,EAAG,GAAIiU,EAAQrT,EAAGZ,EAAGuU,EAAQC,EAAMC,GAASyB,EAASnV,EAAK,GAAGH,IAAK8T,GAASwB,EAASnV,EAAK,GAAGf,MAE7MY,EAAIZ,EAAI,EAEC0Y,EACT9C,IAAMhV,GAAKgV,IAAM5V,GAAKwY,EAASpS,KAAKiO,GAAkBzC,KAAM+B,GAA0B,GAAI5S,EAAM6U,EAAG,GAAI3B,EAAQ2B,EAAG,EAAGrB,EAAQC,EAAM0B,EAASnV,EAAK,GAAG6U,MAStJ2C,EAAM/D,KAAK+E,iBAAmB3H,KAAK4H,OAAOjB,EAAM/Z,WAAYoT,OAE7D4H,uBAAOC,EAAO9Y,OACT6X,EAAW7X,EAAK6X,SACnBpc,EAAIoc,EAAS/b,OACb6a,EAAK3W,EAAK4W,OACP5W,EAAK4X,MAAMmB,QAAUrD,IAAc,KAC1B,EAARoD,EACHA,EAAQ,EACEA,EAAQ,IAClBA,EAAQ,GAEFrd,KACN4L,kBAAkBwQ,EAASpc,GAAIqd,GAAQrd,GAAKuE,EAAKkY,OAAQL,EAASpc,SAE5Dkb,GACNA,EAAGP,IAAIO,EAAGjb,EAAGib,EAAG1B,EAAG0B,EAAGvW,KAAKuW,EAAGG,IAAMH,EAAGE,EAAGF,EAAGjP,EAAGoR,GAChDnC,EAAKA,EAAGqC,MAEThZ,EAAKkY,QAAUlY,EAAKwY,KAAKxY,EAAKsT,OAAQtT,EAAKsY,MAAOT,EAAS,GAAGrS,OAASxF,EAAKoY,QAAU1b,EAAW,GAAKsD,EAAKmY,QAAUnY,EAAKyY,GAAIzY,EAAM8Y,QAEpI9Y,EAAKiY,OAAOgB,UAGdC,6BAAU9Y,UACFmE,yBAAyB9F,WAAW2B,IAAOhC,aAEnDwF,aAAAA,aACAnF,WAAAA,WACAsN,gBAAAA,gBACA9M,gBAAAA,gBACAkE,gBAAAA,gBACAsE,iBAAAA,iBACAiK,gBAAAA,gBACArK,kBAAAA,kBACA9C,yBAAAA,yBACA1E,cAAe,yBAACsZ,EAASpZ,UAASyV,EAAS2D,GAASC,IAAI,SAAA9F,UAAUzT,cAAcyT,GAAiB,IAATvT,MACxFsZ,+CAAmBxE,EAAaC,EAAWvN,OACtC0D,EAAIyG,gBAAgBoD,GAAW,GAAM,GAAM1E,SAASsB,gBAAgBmD,WACjEtN,EAAQ0D,EAAEwG,MAAMlK,GAAS0D,GAEjCqO,eAAgB1E,GAChB2E,iDAAoB1E,EAAaC,EAAWC,EAAYC,OACnD/J,EAAG2J,GAAgBC,EAAaC,EAAWC,EAAYC,SACpD,CAAC/U,EAAGgL,EAAEvM,EAAGW,EAAG4L,EAAE+E,IAEtBwJ,uCAAete,EAAO2Y,OAEjBlY,EAAUqX,GAA0BA,GAA0B,GAAI9X,GADtE2Y,EAAOA,GAAQ,IACmE5T,GAAK,IAAK,GAAI/E,EAAO2Y,EAAKxU,GAAK,IAAK,UACtHwU,EAAKI,UAAYR,GAAY9X,GACtB,CAAgB,UAAdkY,EAAKxS,KAAoB1F,EAAUoQ,gBAAgBpQ,EAASkY,EAAK/H,eAtPhE,SAAX2N,kBAAiBpE,GAA4B,oBAAZ7H,SAA4B6H,EAAO7H,OAAO6H,OAASA,EAAKqE,gBAAkBrE,EA0P5GoE,IAAcpE,EAAKqE,eAAe1C"} \ No newline at end of file diff --git a/node_modules/gsap/dist/Observer.js b/node_modules/gsap/dist/Observer.js new file mode 100644 index 0000000000000000000000000000000000000000..a7267ba2b6a7a29e2cec9e057ab5192dd7b3f66f --- /dev/null +++ b/node_modules/gsap/dist/Observer.js @@ -0,0 +1,706 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + function _defineProperties(target, props) { + for (var i = 0; i < props.length; i++) { + var descriptor = props[i]; + descriptor.enumerable = descriptor.enumerable || false; + descriptor.configurable = true; + if ("value" in descriptor) descriptor.writable = true; + Object.defineProperty(target, descriptor.key, descriptor); + } + } + + function _createClass(Constructor, protoProps, staticProps) { + if (protoProps) _defineProperties(Constructor.prototype, protoProps); + if (staticProps) _defineProperties(Constructor, staticProps); + return Constructor; + } + + /*! + * Observer 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _coreInitted, + _clamp, + _win, + _doc, + _docEl, + _body, + _isTouch, + _pointerType, + ScrollTrigger, + _root, + _normalizer, + _eventTypes, + _context, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _startup = 1, + _observers = []; + exports._scrollers = []; + exports._proxies = []; + var _getTime = Date.now, + _bridge = function _bridge(name, value) { + return value; + }, + _integrate = function _integrate() { + var core = ScrollTrigger.core, + data = core.bridge || {}, + scrollers = core._scrollers, + proxies = core._proxies; + scrollers.push.apply(scrollers, exports._scrollers); + proxies.push.apply(proxies, exports._proxies); + exports._scrollers = scrollers; + exports._proxies = proxies; + + _bridge = function _bridge(name, value) { + return data[name](value); + }; + }, + _getProxyProp = function _getProxyProp(element, property) { + return ~exports._proxies.indexOf(element) && exports._proxies[exports._proxies.indexOf(element) + 1][property]; + }, + _isViewport = function _isViewport(el) { + return !!~_root.indexOf(el); + }, + _addListener = function _addListener(element, type, func, passive, capture) { + return element.addEventListener(type, func, { + passive: passive !== false, + capture: !!capture + }); + }, + _removeListener = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); + }, + _scrollLeft = "scrollLeft", + _scrollTop = "scrollTop", + _onScroll = function _onScroll() { + return _normalizer && _normalizer.isPressed || exports._scrollers.cache++; + }, + _scrollCacheFunc = function _scrollCacheFunc(f, doNotCache) { + var cachingFunc = function cachingFunc(value) { + if (value || value === 0) { + _startup && (_win.history.scrollRestoration = "manual"); + var isNormalizing = _normalizer && _normalizer.isPressed; + value = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); + f(value); + cachingFunc.cacheID = exports._scrollers.cache; + isNormalizing && _bridge("ss", value); + } else if (doNotCache || exports._scrollers.cache !== cachingFunc.cacheID || _bridge("ref")) { + cachingFunc.cacheID = exports._scrollers.cache; + cachingFunc.v = f(); + } + + return cachingFunc.v + cachingFunc.offset; + }; + + cachingFunc.offset = 0; + return f && cachingFunc; + }, + _horizontal = { + s: _scrollLeft, + p: "left", + p2: "Left", + os: "right", + os2: "Right", + d: "width", + d2: "Width", + a: "x", + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win.scrollTo(value, _vertical.sc()) : _win.pageXOffset || _doc[_scrollLeft] || _docEl[_scrollLeft] || _body[_scrollLeft] || 0; + }) + }, + _vertical = { + s: _scrollTop, + p: "top", + p2: "Top", + os: "bottom", + os2: "Bottom", + d: "height", + d2: "Height", + a: "y", + op: _horizontal, + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win.scrollTo(_horizontal.sc(), value) : _win.pageYOffset || _doc[_scrollTop] || _docEl[_scrollTop] || _body[_scrollTop] || 0; + }) + }, + _getTarget = function _getTarget(t, self) { + return (self && self._ctx && self._ctx.selector || gsap.utils.toArray)(t)[0] || (typeof t === "string" && gsap.config().nullTargetWarn !== false ? console.warn("Element not found:", t) : null); + }, + _getScrollFunc = function _getScrollFunc(element, _ref) { + var s = _ref.s, + sc = _ref.sc; + _isViewport(element) && (element = _doc.scrollingElement || _docEl); + + var i = exports._scrollers.indexOf(element), + offset = sc === _vertical.sc ? 1 : 2; + + !~i && (i = exports._scrollers.push(element) - 1); + exports._scrollers[i + offset] || _addListener(element, "scroll", _onScroll); + var prev = exports._scrollers[i + offset], + func = prev || (exports._scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function (value) { + return arguments.length ? element[s] = value : element[s]; + }))); + func.target = element; + prev || (func.smooth = gsap.getProperty(element, "scrollBehavior") === "smooth"); + return func; + }, + _getVelocityProp = function _getVelocityProp(value, minTimeRefresh, useDelta) { + var v1 = value, + v2 = value, + t1 = _getTime(), + t2 = t1, + min = minTimeRefresh || 50, + dropToZeroTime = Math.max(500, min * 3), + update = function update(value, force) { + var t = _getTime(); + + if (force || t - t1 > min) { + v2 = v1; + v1 = value; + t2 = t1; + t1 = t; + } else if (useDelta) { + v1 += value; + } else { + v1 = v2 + (value - v2) / (t - t2) * (t1 - t2); + } + }, + reset = function reset() { + v2 = v1 = useDelta ? 0 : v1; + t2 = t1 = 0; + }, + getVelocity = function getVelocity(latestValue) { + var tOld = t2, + vOld = v2, + t = _getTime(); + + (latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue); + return t1 === t2 || t - t2 > dropToZeroTime ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000; + }; + + return { + update: update, + reset: reset, + getVelocity: getVelocity + }; + }, + _getEvent = function _getEvent(e, preventDefault) { + preventDefault && !e._gsapAllow && e.preventDefault(); + return e.changedTouches ? e.changedTouches[0] : e; + }, + _getAbsoluteMax = function _getAbsoluteMax(a) { + var max = Math.max.apply(Math, a), + min = Math.min.apply(Math, a); + return Math.abs(max) >= Math.abs(min) ? max : min; + }, + _setScrollTrigger = function _setScrollTrigger() { + ScrollTrigger = gsap.core.globals().ScrollTrigger; + ScrollTrigger && ScrollTrigger.core && _integrate(); + }, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (!_coreInitted && gsap && typeof document !== "undefined" && document.body) { + _win = window; + _doc = document; + _docEl = _doc.documentElement; + _body = _doc.body; + _root = [_win, _doc, _docEl, _body]; + _clamp = gsap.utils.clamp; + + _context = gsap.core.context || function () {}; + + _pointerType = "onpointerenter" in _body ? "pointer" : "mouse"; + _isTouch = Observer.isTouch = _win.matchMedia && _win.matchMedia("(hover: none), (pointer: coarse)").matches ? 1 : "ontouchstart" in _win || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0 ? 2 : 0; + _eventTypes = Observer.eventTypes = ("ontouchstart" in _docEl ? "touchstart,touchmove,touchcancel,touchend" : !("onpointerdown" in _docEl) ? "mousedown,mousemove,mouseup,mouseup" : "pointerdown,pointermove,pointercancel,pointerup").split(","); + setTimeout(function () { + return _startup = 0; + }, 500); + + _setScrollTrigger(); + + _coreInitted = 1; + } + + return _coreInitted; + }; + + _horizontal.op = _vertical; + exports._scrollers.cache = 0; + var Observer = function () { + function Observer(vars) { + this.init(vars); + } + + var _proto = Observer.prototype; + + _proto.init = function init(vars) { + _coreInitted || _initCore(gsap) || console.warn("Please gsap.registerPlugin(Observer)"); + ScrollTrigger || _setScrollTrigger(); + var tolerance = vars.tolerance, + dragMinimum = vars.dragMinimum, + type = vars.type, + target = vars.target, + lineHeight = vars.lineHeight, + debounce = vars.debounce, + preventDefault = vars.preventDefault, + onStop = vars.onStop, + onStopDelay = vars.onStopDelay, + ignore = vars.ignore, + wheelSpeed = vars.wheelSpeed, + event = vars.event, + onDragStart = vars.onDragStart, + onDragEnd = vars.onDragEnd, + onDrag = vars.onDrag, + onPress = vars.onPress, + onRelease = vars.onRelease, + onRight = vars.onRight, + onLeft = vars.onLeft, + onUp = vars.onUp, + onDown = vars.onDown, + onChangeX = vars.onChangeX, + onChangeY = vars.onChangeY, + onChange = vars.onChange, + onToggleX = vars.onToggleX, + onToggleY = vars.onToggleY, + onHover = vars.onHover, + onHoverEnd = vars.onHoverEnd, + onMove = vars.onMove, + ignoreCheck = vars.ignoreCheck, + isNormalizer = vars.isNormalizer, + onGestureStart = vars.onGestureStart, + onGestureEnd = vars.onGestureEnd, + onWheel = vars.onWheel, + onEnable = vars.onEnable, + onDisable = vars.onDisable, + onClick = vars.onClick, + scrollSpeed = vars.scrollSpeed, + capture = vars.capture, + allowClicks = vars.allowClicks, + lockAxis = vars.lockAxis, + onLockAxis = vars.onLockAxis; + this.target = target = _getTarget(target) || _docEl; + this.vars = vars; + ignore && (ignore = gsap.utils.toArray(ignore)); + tolerance = tolerance || 1e-9; + dragMinimum = dragMinimum || 0; + wheelSpeed = wheelSpeed || 1; + scrollSpeed = scrollSpeed || 1; + type = type || "wheel,touch,pointer"; + debounce = debounce !== false; + lineHeight || (lineHeight = parseFloat(_win.getComputedStyle(_body).lineHeight) || 22); + + var id, + onStopDelayedCall, + dragged, + moved, + wheeled, + locked, + axis, + self = this, + prevDeltaX = 0, + prevDeltaY = 0, + passive = vars.passive || !preventDefault && vars.passive !== false, + scrollFuncX = _getScrollFunc(target, _horizontal), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollX = scrollFuncX(), + scrollY = scrollFuncY(), + limitToTouch = ~type.indexOf("touch") && !~type.indexOf("pointer") && _eventTypes[0] === "pointerdown", + isViewport = _isViewport(target), + ownerDoc = target.ownerDocument || _doc, + deltaX = [0, 0, 0], + deltaY = [0, 0, 0], + onClickTime = 0, + clickCapture = function clickCapture() { + return onClickTime = _getTime(); + }, + _ignoreCheck = function _ignoreCheck(e, isPointerOrTouch) { + return (self.event = e) && ignore && ~ignore.indexOf(e.target) || isPointerOrTouch && limitToTouch && e.pointerType !== "touch" || ignoreCheck && ignoreCheck(e, isPointerOrTouch); + }, + onStopFunc = function onStopFunc() { + self._vx.reset(); + + self._vy.reset(); + + onStopDelayedCall.pause(); + onStop && onStop(self); + }, + update = function update() { + var dx = self.deltaX = _getAbsoluteMax(deltaX), + dy = self.deltaY = _getAbsoluteMax(deltaY), + changedX = Math.abs(dx) >= tolerance, + changedY = Math.abs(dy) >= tolerance; + + onChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); + + if (changedX) { + onRight && self.deltaX > 0 && onRight(self); + onLeft && self.deltaX < 0 && onLeft(self); + onChangeX && onChangeX(self); + onToggleX && self.deltaX < 0 !== prevDeltaX < 0 && onToggleX(self); + prevDeltaX = self.deltaX; + deltaX[0] = deltaX[1] = deltaX[2] = 0; + } + + if (changedY) { + onDown && self.deltaY > 0 && onDown(self); + onUp && self.deltaY < 0 && onUp(self); + onChangeY && onChangeY(self); + onToggleY && self.deltaY < 0 !== prevDeltaY < 0 && onToggleY(self); + prevDeltaY = self.deltaY; + deltaY[0] = deltaY[1] = deltaY[2] = 0; + } + + if (moved || dragged) { + onMove && onMove(self); + + if (dragged) { + onDragStart && dragged === 1 && onDragStart(self); + onDrag && onDrag(self); + dragged = 0; + } + + moved = false; + } + + locked && !(locked = false) && onLockAxis && onLockAxis(self); + + if (wheeled) { + onWheel(self); + wheeled = false; + } + + id = 0; + }, + onDelta = function onDelta(x, y, index) { + deltaX[index] += x; + deltaY[index] += y; + + self._vx.update(x); + + self._vy.update(y); + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + onTouchOrPointerDelta = function onTouchOrPointerDelta(x, y) { + if (lockAxis && !axis) { + self.axis = axis = Math.abs(x) > Math.abs(y) ? "x" : "y"; + locked = true; + } + + if (axis !== "y") { + deltaX[2] += x; + + self._vx.update(x, true); + } + + if (axis !== "x") { + deltaY[2] += y; + + self._vy.update(y, true); + } + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + _onDrag = function _onDrag(e) { + if (_ignoreCheck(e, 1)) { + return; + } + + e = _getEvent(e, preventDefault); + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y, + isDragging = self.isDragging; + self.x = x; + self.y = y; + + if (isDragging || (dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum)) { + dragged = isDragging ? 2 : 1; + isDragging || (self.isDragging = true); + onTouchOrPointerDelta(dx, dy); + } + }, + _onPress = self.onPress = function (e) { + if (_ignoreCheck(e, 1) || e && e.button) { + return; + } + + self.axis = axis = null; + onStopDelayedCall.pause(); + self.isPressed = true; + e = _getEvent(e); + prevDeltaX = prevDeltaY = 0; + self.startX = self.x = e.clientX; + self.startY = self.y = e.clientY; + + self._vx.reset(); + + self._vy.reset(); + + _addListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true); + + self.deltaX = self.deltaY = 0; + onPress && onPress(self); + }, + _onRelease = self.onRelease = function (e) { + if (_ignoreCheck(e, 1)) { + return; + } + + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + + var isTrackingDrag = !isNaN(self.y - self.startY), + wasDragging = self.isDragging, + isDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), + eventData = _getEvent(e); + + if (!isDragNotClick && isTrackingDrag) { + self._vx.reset(); + + self._vy.reset(); + + if (preventDefault && allowClicks) { + gsap.delayedCall(0.08, function () { + if (_getTime() - onClickTime > 300 && !e.defaultPrevented) { + if (e.target.click) { + e.target.click(); + } else if (ownerDoc.createEvent) { + var syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null); + e.target.dispatchEvent(syntheticEvent); + } + } + }); + } + } + + self.isDragging = self.isGesturing = self.isPressed = false; + onStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true); + dragged && update(); + onDragEnd && wasDragging && onDragEnd(self); + onRelease && onRelease(self, isDragNotClick); + }, + _onGestureStart = function _onGestureStart(e) { + return e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging); + }, + _onGestureEnd = function _onGestureEnd() { + return (self.isGesturing = false) || onGestureEnd(self); + }, + onScroll = function onScroll(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = scrollFuncX(), + y = scrollFuncY(); + onDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1); + scrollX = x; + scrollY = y; + onStop && onStopDelayedCall.restart(true); + }, + _onWheel = function _onWheel(e) { + if (_ignoreCheck(e)) { + return; + } + + e = _getEvent(e, preventDefault); + onWheel && (wheeled = true); + var multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win.innerHeight : 1) * wheelSpeed; + onDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0); + onStop && !isNormalizer && onStopDelayedCall.restart(true); + }, + _onMove = function _onMove(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y; + self.x = x; + self.y = y; + moved = true; + onStop && onStopDelayedCall.restart(true); + (dx || dy) && onTouchOrPointerDelta(dx, dy); + }, + _onHover = function _onHover(e) { + self.event = e; + onHover(self); + }, + _onHoverEnd = function _onHoverEnd(e) { + self.event = e; + onHoverEnd(self); + }, + _onClick = function _onClick(e) { + return _ignoreCheck(e) || _getEvent(e, preventDefault) && onClick(self); + }; + + onStopDelayedCall = self._dc = gsap.delayedCall(onStopDelay || 0.25, onStopFunc).pause(); + self.deltaX = self.deltaY = 0; + self._vx = _getVelocityProp(0, 50, true); + self._vy = _getVelocityProp(0, 50, true); + self.scrollX = scrollFuncX; + self.scrollY = scrollFuncY; + self.isDragging = self.isGesturing = self.isPressed = false; + + _context(this); + + self.enable = function (e) { + if (!self.isEnabled) { + _addListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + + type.indexOf("scroll") >= 0 && _addListener(isViewport ? ownerDoc : target, "scroll", onScroll, passive, capture); + type.indexOf("wheel") >= 0 && _addListener(target, "wheel", _onWheel, passive, capture); + + if (type.indexOf("touch") >= 0 && _isTouch || type.indexOf("pointer") >= 0) { + _addListener(target, _eventTypes[0], _onPress, passive, capture); + + _addListener(ownerDoc, _eventTypes[2], _onRelease); + + _addListener(ownerDoc, _eventTypes[3], _onRelease); + + allowClicks && _addListener(target, "click", clickCapture, true, true); + onClick && _addListener(target, "click", _onClick); + onGestureStart && _addListener(ownerDoc, "gesturestart", _onGestureStart); + onGestureEnd && _addListener(ownerDoc, "gestureend", _onGestureEnd); + onHover && _addListener(target, _pointerType + "enter", _onHover); + onHoverEnd && _addListener(target, _pointerType + "leave", _onHoverEnd); + onMove && _addListener(target, _pointerType + "move", _onMove); + } + + self.isEnabled = true; + self.isDragging = self.isGesturing = self.isPressed = moved = dragged = false; + + self._vx.reset(); + + self._vy.reset(); + + scrollX = scrollFuncX(); + scrollY = scrollFuncY(); + e && e.type && _onPress(e); + onEnable && onEnable(self); + } + + return self; + }; + + self.disable = function () { + if (self.isEnabled) { + _observers.filter(function (o) { + return o !== self && _isViewport(o.target); + }).length || _removeListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + + if (self.isPressed) { + self._vx.reset(); + + self._vy.reset(); + + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + } + + _removeListener(isViewport ? ownerDoc : target, "scroll", onScroll, capture); + + _removeListener(target, "wheel", _onWheel, capture); + + _removeListener(target, _eventTypes[0], _onPress, capture); + + _removeListener(ownerDoc, _eventTypes[2], _onRelease); + + _removeListener(ownerDoc, _eventTypes[3], _onRelease); + + _removeListener(target, "click", clickCapture, true); + + _removeListener(target, "click", _onClick); + + _removeListener(ownerDoc, "gesturestart", _onGestureStart); + + _removeListener(ownerDoc, "gestureend", _onGestureEnd); + + _removeListener(target, _pointerType + "enter", _onHover); + + _removeListener(target, _pointerType + "leave", _onHoverEnd); + + _removeListener(target, _pointerType + "move", _onMove); + + self.isEnabled = self.isPressed = self.isDragging = false; + onDisable && onDisable(self); + } + }; + + self.kill = self.revert = function () { + self.disable(); + + var i = _observers.indexOf(self); + + i >= 0 && _observers.splice(i, 1); + _normalizer === self && (_normalizer = 0); + }; + + _observers.push(self); + + isNormalizer && _isViewport(target) && (_normalizer = self); + self.enable(event); + }; + + _createClass(Observer, [{ + key: "velocityX", + get: function get() { + return this._vx.getVelocity(); + } + }, { + key: "velocityY", + get: function get() { + return this._vy.getVelocity(); + } + }]); + + return Observer; + }(); + Observer.version = "3.12.7"; + + Observer.create = function (vars) { + return new Observer(vars); + }; + + Observer.register = _initCore; + + Observer.getAll = function () { + return _observers.slice(); + }; + + Observer.getById = function (id) { + return _observers.filter(function (o) { + return o.vars.id === id; + })[0]; + }; + + _getGSAP() && gsap.registerPlugin(Observer); + + exports.Observer = Observer; + exports._getProxyProp = _getProxyProp; + exports._getScrollFunc = _getScrollFunc; + exports._getTarget = _getTarget; + exports._getVelocityProp = _getVelocityProp; + exports._horizontal = _horizontal; + exports._isViewport = _isViewport; + exports._vertical = _vertical; + exports.default = Observer; + + if (typeof(window) === 'undefined' || window !== exports) {Object.defineProperty(exports, '__esModule', { value: true });} else {delete window.default;} + +}))); diff --git a/node_modules/gsap/dist/Observer.min.js b/node_modules/gsap/dist/Observer.min.js new file mode 100644 index 0000000000000000000000000000000000000000..dd7309d26ff5887a736b7ca373301b86a67ee2ea --- /dev/null +++ b/node_modules/gsap/dist/Observer.min.js @@ -0,0 +1,11 @@ +/*! + * Observer 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(a){"use strict";function _defineProperties(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}function r(){return Me||"undefined"!=typeof window&&(Me=window.gsap)&&Me.registerPlugin&&Me}var Me,Pe,De,Ae,Ee,Oe,Ye,ze,Xe,t,qe,Te,ke,o=1,Ce=[];a._scrollers=[],a._proxies=[];function x(e,t){return~a._proxies.indexOf(e)&&a._proxies[a._proxies.indexOf(e)+1][t]}function y(e){return!!~t.indexOf(e)}function z(e,t,n,r,o){return e.addEventListener(t,n,{passive:!1!==r,capture:!!o})}function A(e,t,n,r){return e.removeEventListener(t,n,!!r)}function D(){return qe&&qe.isPressed||a._scrollers.cache++}function E(n,r){function qa(e){if(e||0===e){o&&(De.history.scrollRestoration="manual");var t=qe&&qe.isPressed;e=qa.v=Math.round(e)||(qe&&qe.iOS?1:0),n(e),qa.cacheID=a._scrollers.cache,t&&i("ss",e)}else(r||a._scrollers.cache!==qa.cacheID||i("ref"))&&(qa.cacheID=a._scrollers.cache,qa.v=n());return qa.v+qa.offset}return qa.offset=0,n&&qa}function H(e,t){return(t&&t._ctx&&t._ctx.selector||Me.utils.toArray)(e)[0]||("string"==typeof e&&!1!==Me.config().nullTargetWarn?console.warn("Element not found:",e):null)}function I(t,e){var n=e.s,r=e.sc;y(t)&&(t=Ae.scrollingElement||Ee);var o=a._scrollers.indexOf(t),i=r===Le.sc?1:2;~o||(o=a._scrollers.push(t)-1),a._scrollers[o+i]||z(t,"scroll",D);var s=a._scrollers[o+i],c=s||(a._scrollers[o+i]=E(x(t,n),!0)||(y(t)?r:E(function(e){return arguments.length?t[n]=e:t[n]})));return c.target=t,s||(c.smooth="smooth"===Me.getProperty(t,"scrollBehavior")),c}function J(e,t,o){function Pa(e,t){var n=Se();t||r<n-c?(s=i,i=e,a=c,c=n):o?i+=e:i=s+(e-s)/(n-a)*(c-a)}var i=e,s=e,c=Se(),a=c,r=t||50,l=Math.max(500,3*r);return{update:Pa,reset:function reset(){s=i=o?0:i,a=c=0},getVelocity:function getVelocity(e){var t=a,n=s,r=Se();return!e&&0!==e||e===i||Pa(e),c===a||l<r-a?0:(i+(o?n:-n))/((o?r:c)-t)*1e3}}}function K(e,t){return t&&!e._gsapAllow&&e.preventDefault(),e.changedTouches?e.changedTouches[0]:e}function L(e){var t=Math.max.apply(Math,e),n=Math.min.apply(Math,e);return Math.abs(t)>=Math.abs(n)?t:n}function M(){(Xe=Me.core.globals().ScrollTrigger)&&Xe.core&&function _integrate(){var e=Xe.core,n=e.bridge||{},t=e._scrollers,r=e._proxies;t.push.apply(t,a._scrollers),r.push.apply(r,a._proxies),a._scrollers=t,a._proxies=r,i=function _bridge(e,t){return n[e](t)}}()}function N(e){return Me=e||r(),!Pe&&Me&&"undefined"!=typeof document&&document.body&&(De=window,Ee=(Ae=document).documentElement,Oe=Ae.body,t=[De,Ae,Ee,Oe],Me.utils.clamp,ke=Me.core.context||function(){},ze="onpointerenter"in Oe?"pointer":"mouse",Ye=c.isTouch=De.matchMedia&&De.matchMedia("(hover: none), (pointer: coarse)").matches?1:"ontouchstart"in De||0<navigator.maxTouchPoints||0<navigator.msMaxTouchPoints?2:0,Te=c.eventTypes=("ontouchstart"in Ee?"touchstart,touchmove,touchcancel,touchend":"onpointerdown"in Ee?"pointerdown,pointermove,pointercancel,pointerup":"mousedown,mousemove,mouseup,mouseup").split(","),setTimeout(function(){return o=0},500),M(),Pe=1),Pe}var Se=Date.now,i=function _bridge(e,t){return t},n="scrollLeft",s="scrollTop",He={s:n,p:"left",p2:"Left",os:"right",os2:"Right",d:"width",d2:"Width",a:"x",sc:E(function(e){return arguments.length?De.scrollTo(e,Le.sc()):De.pageXOffset||Ae[n]||Ee[n]||Oe[n]||0})},Le={s:s,p:"top",p2:"Top",os:"bottom",os2:"Bottom",d:"height",d2:"Height",a:"y",op:He,sc:E(function(e){return arguments.length?De.scrollTo(He.sc(),e):De.pageYOffset||Ae[s]||Ee[s]||Oe[s]||0})};He.op=Le,a._scrollers.cache=0;var c=(Observer.prototype.init=function init(e){Pe||N(Me)||console.warn("Please gsap.registerPlugin(Observer)"),Xe||M();var o=e.tolerance,s=e.dragMinimum,t=e.type,i=e.target,n=e.lineHeight,r=e.debounce,c=e.preventDefault,a=e.onStop,l=e.onStopDelay,u=e.ignore,f=e.wheelSpeed,d=e.event,g=e.onDragStart,p=e.onDragEnd,v=e.onDrag,h=e.onPress,_=e.onRelease,x=e.onRight,m=e.onLeft,b=e.onUp,w=e.onDown,P=e.onChangeX,E=e.onChangeY,O=e.onChange,Y=e.onToggleX,X=e.onToggleY,q=e.onHover,T=e.onHoverEnd,k=e.onMove,C=e.ignoreCheck,S=e.isNormalizer,F=e.onGestureStart,G=e.onGestureEnd,B=e.onWheel,V=e.onEnable,R=e.onDisable,j=e.onClick,W=e.scrollSpeed,U=e.capture,Q=e.allowClicks,Z=e.lockAxis,$=e.onLockAxis;function pc(){return me=Se()}function qc(e,t){return(ce.event=e)&&u&&~u.indexOf(e.target)||t&&ve&&"touch"!==e.pointerType||C&&C(e,t)}function sc(){var e=ce.deltaX=L(_e),t=ce.deltaY=L(xe),n=Math.abs(e)>=o,r=Math.abs(t)>=o;O&&(n||r)&&O(ce,e,t,_e,xe),n&&(x&&0<ce.deltaX&&x(ce),m&&ce.deltaX<0&&m(ce),P&&P(ce),Y&&ce.deltaX<0!=ae<0&&Y(ce),ae=ce.deltaX,_e[0]=_e[1]=_e[2]=0),r&&(w&&0<ce.deltaY&&w(ce),b&&ce.deltaY<0&&b(ce),E&&E(ce),X&&ce.deltaY<0!=le<0&&X(ce),le=ce.deltaY,xe[0]=xe[1]=xe[2]=0),(re||ne)&&(k&&k(ce),ne&&(g&&1===ne&&g(ce),v&&v(ce),ne=0),re=!1),ie&&!(ie=!1)&&$&&$(ce),oe&&(B(ce),oe=!1),ee=0}function tc(e,t,n){_e[n]+=e,xe[n]+=t,ce._vx.update(e),ce._vy.update(t),r?ee=ee||requestAnimationFrame(sc):sc()}function uc(e,t){Z&&!se&&(ce.axis=se=Math.abs(e)>Math.abs(t)?"x":"y",ie=!0),"y"!==se&&(_e[2]+=e,ce._vx.update(e,!0)),"x"!==se&&(xe[2]+=t,ce._vy.update(t,!0)),r?ee=ee||requestAnimationFrame(sc):sc()}function vc(e){if(!qc(e,1)){var t=(e=K(e,c)).clientX,n=e.clientY,r=t-ce.x,o=n-ce.y,i=ce.isDragging;ce.x=t,ce.y=n,(i||(r||o)&&(Math.abs(ce.startX-t)>=s||Math.abs(ce.startY-n)>=s))&&(ne=i?2:1,i||(ce.isDragging=!0),uc(r,o))}}function yc(e){return e.touches&&1<e.touches.length&&(ce.isGesturing=!0)&&F(e,ce.isDragging)}function zc(){return(ce.isGesturing=!1)||G(ce)}function Ac(e){if(!qc(e)){var t=fe(),n=de();tc((t-ge)*W,(n-pe)*W,1),ge=t,pe=n,a&&te.restart(!0)}}function Bc(e){if(!qc(e)){e=K(e,c),B&&(oe=!0);var t=(1===e.deltaMode?n:2===e.deltaMode?De.innerHeight:1)*f;tc(e.deltaX*t,e.deltaY*t,0),a&&!S&&te.restart(!0)}}function Cc(e){if(!qc(e)){var t=e.clientX,n=e.clientY,r=t-ce.x,o=n-ce.y;ce.x=t,ce.y=n,re=!0,a&&te.restart(!0),(r||o)&&uc(r,o)}}function Dc(e){ce.event=e,q(ce)}function Ec(e){ce.event=e,T(ce)}function Fc(e){return qc(e)||K(e,c)&&j(ce)}this.target=i=H(i)||Ee,this.vars=e,u=u&&Me.utils.toArray(u),o=o||1e-9,s=s||0,f=f||1,W=W||1,t=t||"wheel,touch,pointer",r=!1!==r,n=n||parseFloat(De.getComputedStyle(Oe).lineHeight)||22;var ee,te,ne,re,oe,ie,se,ce=this,ae=0,le=0,ue=e.passive||!c&&!1!==e.passive,fe=I(i,He),de=I(i,Le),ge=fe(),pe=de(),ve=~t.indexOf("touch")&&!~t.indexOf("pointer")&&"pointerdown"===Te[0],he=y(i),ye=i.ownerDocument||Ae,_e=[0,0,0],xe=[0,0,0],me=0,be=ce.onPress=function(e){qc(e,1)||e&&e.button||(ce.axis=se=null,te.pause(),ce.isPressed=!0,e=K(e),ae=le=0,ce.startX=ce.x=e.clientX,ce.startY=ce.y=e.clientY,ce._vx.reset(),ce._vy.reset(),z(S?i:ye,Te[1],vc,ue,!0),ce.deltaX=ce.deltaY=0,h&&h(ce))},we=ce.onRelease=function(t){if(!qc(t,1)){A(S?i:ye,Te[1],vc,!0);var e=!isNaN(ce.y-ce.startY),n=ce.isDragging,r=n&&(3<Math.abs(ce.x-ce.startX)||3<Math.abs(ce.y-ce.startY)),o=K(t);!r&&e&&(ce._vx.reset(),ce._vy.reset(),c&&Q&&Me.delayedCall(.08,function(){if(300<Se()-me&&!t.defaultPrevented)if(t.target.click)t.target.click();else if(ye.createEvent){var e=ye.createEvent("MouseEvents");e.initMouseEvent("click",!0,!0,De,1,o.screenX,o.screenY,o.clientX,o.clientY,!1,!1,!1,!1,0,null),t.target.dispatchEvent(e)}})),ce.isDragging=ce.isGesturing=ce.isPressed=!1,a&&n&&!S&&te.restart(!0),ne&&sc(),p&&n&&p(ce),_&&_(ce,r)}};te=ce._dc=Me.delayedCall(l||.25,function onStopFunc(){ce._vx.reset(),ce._vy.reset(),te.pause(),a&&a(ce)}).pause(),ce.deltaX=ce.deltaY=0,ce._vx=J(0,50,!0),ce._vy=J(0,50,!0),ce.scrollX=fe,ce.scrollY=de,ce.isDragging=ce.isGesturing=ce.isPressed=!1,ke(this),ce.enable=function(e){return ce.isEnabled||(z(he?ye:i,"scroll",D),0<=t.indexOf("scroll")&&z(he?ye:i,"scroll",Ac,ue,U),0<=t.indexOf("wheel")&&z(i,"wheel",Bc,ue,U),(0<=t.indexOf("touch")&&Ye||0<=t.indexOf("pointer"))&&(z(i,Te[0],be,ue,U),z(ye,Te[2],we),z(ye,Te[3],we),Q&&z(i,"click",pc,!0,!0),j&&z(i,"click",Fc),F&&z(ye,"gesturestart",yc),G&&z(ye,"gestureend",zc),q&&z(i,ze+"enter",Dc),T&&z(i,ze+"leave",Ec),k&&z(i,ze+"move",Cc)),ce.isEnabled=!0,ce.isDragging=ce.isGesturing=ce.isPressed=re=ne=!1,ce._vx.reset(),ce._vy.reset(),ge=fe(),pe=de(),e&&e.type&&be(e),V&&V(ce)),ce},ce.disable=function(){ce.isEnabled&&(Ce.filter(function(e){return e!==ce&&y(e.target)}).length||A(he?ye:i,"scroll",D),ce.isPressed&&(ce._vx.reset(),ce._vy.reset(),A(S?i:ye,Te[1],vc,!0)),A(he?ye:i,"scroll",Ac,U),A(i,"wheel",Bc,U),A(i,Te[0],be,U),A(ye,Te[2],we),A(ye,Te[3],we),A(i,"click",pc,!0),A(i,"click",Fc),A(ye,"gesturestart",yc),A(ye,"gestureend",zc),A(i,ze+"enter",Dc),A(i,ze+"leave",Ec),A(i,ze+"move",Cc),ce.isEnabled=ce.isPressed=ce.isDragging=!1,R&&R(ce))},ce.kill=ce.revert=function(){ce.disable();var e=Ce.indexOf(ce);0<=e&&Ce.splice(e,1),qe===ce&&(qe=0)},Ce.push(ce),S&&y(i)&&(qe=ce),ce.enable(d)},function _createClass(e,t,n){return t&&_defineProperties(e.prototype,t),n&&_defineProperties(e,n),e}(Observer,[{key:"velocityX",get:function get(){return this._vx.getVelocity()}},{key:"velocityY",get:function get(){return this._vy.getVelocity()}}]),Observer);function Observer(e){this.init(e)}c.version="3.12.7",c.create=function(e){return new c(e)},c.register=N,c.getAll=function(){return Ce.slice()},c.getById=function(t){return Ce.filter(function(e){return e.vars.id===t})[0]},r()&&Me.registerPlugin(c),a.Observer=c,a._getProxyProp=x,a._getScrollFunc=I,a._getTarget=H,a._getVelocityProp=J,a._horizontal=He,a._isViewport=y,a._vertical=Le,a.default=c;if (typeof(window)==="undefined"||window!==a){Object.defineProperty(a,"__esModule",{value:!0})} else {delete a.default}}); + diff --git a/node_modules/gsap/dist/Observer.min.js.map b/node_modules/gsap/dist/Observer.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..c15f53f1b77c2bdd29e0af57a661332d7c5bcc9e --- /dev/null +++ b/node_modules/gsap/dist/Observer.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"Observer.min.js","sources":["../src/Observer.js"],"sourcesContent":["/*!\n * Observer 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _coreInitted, _clamp, _win, _doc, _docEl, _body, _isTouch, _pointerType, ScrollTrigger, _root, _normalizer, _eventTypes, _context,\n\t_getGSAP = () => gsap || (typeof(window) !== \"undefined\" && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_startup = 1,\n\t_observers = [],\n\t_scrollers = [],\n\t_proxies = [],\n\t_getTime = Date.now,\n\t_bridge = (name, value) => value,\n\t_integrate = () => {\n\t\tlet core = ScrollTrigger.core,\n\t\t\tdata = core.bridge || {},\n\t\t\tscrollers = core._scrollers,\n\t\t\tproxies = core._proxies;\n\t\tscrollers.push(..._scrollers);\n\t\tproxies.push(..._proxies);\n\t\t_scrollers = scrollers;\n\t\t_proxies = proxies;\n\t\t_bridge = (name, value) => data[name](value);\n\t},\n\t_getProxyProp = (element, property) => ~_proxies.indexOf(element) && _proxies[_proxies.indexOf(element) + 1][property],\n\t_isViewport = el => !!~_root.indexOf(el),\n\t_addListener = (element, type, func, passive, capture) => element.addEventListener(type, func, {passive: passive !== false, capture: !!capture}),\n\t_removeListener = (element, type, func, capture) => element.removeEventListener(type, func, !!capture),\n\t_scrollLeft = \"scrollLeft\",\n\t_scrollTop = \"scrollTop\",\n\t_onScroll = () => (_normalizer && _normalizer.isPressed) || _scrollers.cache++,\n\t_scrollCacheFunc = (f, doNotCache) => {\n\t\tlet cachingFunc = value => { // since reading the scrollTop/scrollLeft/pageOffsetY/pageOffsetX can trigger a layout, this function allows us to cache the value so it only gets read fresh after a \"scroll\" event fires (or while we're refreshing because that can lengthen the page and alter the scroll position). when \"soft\" is true, that means don't actually set the scroll, but cache the new value instead (useful in ScrollSmoother)\n\t\t\tif (value || value === 0) {\n\t\t\t\t_startup && (_win.history.scrollRestoration = \"manual\"); // otherwise the new position will get overwritten by the browser onload.\n\t\t\t\tlet isNormalizing = _normalizer && _normalizer.isPressed;\n\t\t\t\tvalue = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); //TODO: iOS Bug: if you allow it to go to 0, Safari can start to report super strange (wildly inaccurate) touch positions!\n\t\t\t\tf(value);\n\t\t\t\tcachingFunc.cacheID = _scrollers.cache;\n\t\t\t\tisNormalizing && _bridge(\"ss\", value); // set scroll (notify ScrollTrigger so it can dispatch a \"scrollStart\" event if necessary\n\t\t\t} else if (doNotCache || _scrollers.cache !== cachingFunc.cacheID || _bridge(\"ref\")) {\n\t\t\t\tcachingFunc.cacheID = _scrollers.cache;\n\t\t\t\tcachingFunc.v = f();\n\t\t\t}\n\t\t\treturn cachingFunc.v + cachingFunc.offset;\n\t\t};\n\t\tcachingFunc.offset = 0;\n\t\treturn f && cachingFunc;\n\t},\n\t_horizontal = {s: _scrollLeft, p: \"left\", p2: \"Left\", os: \"right\", os2: \"Right\", d: \"width\", d2: \"Width\", a: \"x\", sc: _scrollCacheFunc(function(value) { return arguments.length ? _win.scrollTo(value, _vertical.sc()) : _win.pageXOffset || _doc[_scrollLeft] || _docEl[_scrollLeft] || _body[_scrollLeft] || 0})},\n\t_vertical = {s: _scrollTop, p: \"top\", p2: \"Top\", os: \"bottom\", os2: \"Bottom\", d: \"height\", d2: \"Height\", a: \"y\", op: _horizontal, sc: _scrollCacheFunc(function(value) { return arguments.length ? _win.scrollTo(_horizontal.sc(), value) : _win.pageYOffset || _doc[_scrollTop] || _docEl[_scrollTop] || _body[_scrollTop] || 0})},\n\t_getTarget = (t, self) => ((self && self._ctx && self._ctx.selector) || gsap.utils.toArray)(t)[0] || (typeof(t) === \"string\" && gsap.config().nullTargetWarn !== false ? console.warn(\"Element not found:\", t) : null),\n\n\t_getScrollFunc = (element, {s, sc}) => { // we store the scroller functions in an alternating sequenced Array like [element, verticalScrollFunc, horizontalScrollFunc, ...] so that we can minimize memory, maximize performance, and we also record the last position as a \".rec\" property in order to revert to that after refreshing to ensure things don't shift around.\n\t\t_isViewport(element) && (element = _doc.scrollingElement || _docEl);\n\t\tlet i = _scrollers.indexOf(element),\n\t\t\toffset = sc === _vertical.sc ? 1 : 2;\n\t\t!~i && (i = _scrollers.push(element) - 1);\n\t\t_scrollers[i + offset] || _addListener(element, \"scroll\", _onScroll); // clear the cache when a scroll occurs\n\t\tlet prev = _scrollers[i + offset],\n\t\t\tfunc = prev || (_scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function(value) { return arguments.length ? (element[s] = value) : element[s]; })));\n\t\tfunc.target = element;\n\t\tprev || (func.smooth = gsap.getProperty(element, \"scrollBehavior\") === \"smooth\"); // only set it the first time (don't reset every time a scrollFunc is requested because perhaps it happens during a refresh() when it's disabled in ScrollTrigger.\n\t\treturn func;\n\t},\n\t_getVelocityProp = (value, minTimeRefresh, useDelta) => {\n\t\tlet v1 = value,\n\t\t\tv2 = value,\n\t\t\tt1 = _getTime(),\n\t\t\tt2 = t1,\n\t\t\tmin = minTimeRefresh || 50,\n\t\t\tdropToZeroTime = Math.max(500, min * 3),\n\t\t\tupdate = (value, force) => {\n\t\t\t\tlet t = _getTime();\n\t\t\t\tif (force || t - t1 > min) {\n\t\t\t\t\tv2 = v1;\n\t\t\t\t\tv1 = value;\n\t\t\t\t\tt2 = t1;\n\t\t\t\t\tt1 = t;\n\t\t\t\t} else if (useDelta) {\n\t\t\t\t\tv1 += value;\n\t\t\t\t} else { // not totally necessary, but makes it a bit more accurate by adjusting the v1 value according to the new slope. This way we're not just ignoring the incoming data. Removing for now because it doesn't seem to make much practical difference and it's probably not worth the kb.\n\t\t\t\t\tv1 = v2 + (value - v2) / (t - t2) * (t1 - t2);\n\t\t\t\t}\n\t\t\t},\n\t\t\treset = () => { v2 = v1 = useDelta ? 0 : v1; t2 = t1 = 0; },\n\t\t\tgetVelocity = latestValue => {\n\t\t\t\tlet tOld = t2,\n\t\t\t\t\tvOld = v2,\n\t\t\t\t\tt = _getTime();\n\t\t\t\t(latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue);\n\t\t\t\treturn (t1 === t2 || t - t2 > dropToZeroTime) ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000;\n\t\t\t};\n\t\treturn {update, reset, getVelocity};\n\t},\n\t_getEvent = (e, preventDefault) => {\n\t\tpreventDefault && !e._gsapAllow && e.preventDefault();\n\t\treturn e.changedTouches ? e.changedTouches[0] : e;\n\t},\n\t_getAbsoluteMax = a => {\n\t\tlet max = Math.max(...a),\n\t\t\tmin = Math.min(...a);\n\t\treturn Math.abs(max) >= Math.abs(min) ? max : min;\n\t},\n\t_setScrollTrigger = () => {\n\t\tScrollTrigger = gsap.core.globals().ScrollTrigger;\n\t\tScrollTrigger && ScrollTrigger.core && _integrate();\n\t},\n\t_initCore = core => {\n\t\tgsap = core || _getGSAP();\n\t\tif (!_coreInitted && gsap && typeof(document) !== \"undefined\" && document.body) {\n\t\t\t_win = window;\n\t\t\t_doc = document;\n\t\t\t_docEl = _doc.documentElement;\n\t\t\t_body = _doc.body;\n\t\t\t_root = [_win, _doc, _docEl, _body];\n\t\t\t_clamp = gsap.utils.clamp;\n\t\t\t_context = gsap.core.context || function() {};\n\t\t\t_pointerType = \"onpointerenter\" in _body ? \"pointer\" : \"mouse\";\n\t\t\t// isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer.\n\t\t\t_isTouch = Observer.isTouch = _win.matchMedia && _win.matchMedia(\"(hover: none), (pointer: coarse)\").matches ? 1 : (\"ontouchstart\" in _win || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0) ? 2 : 0;\n\t\t\t_eventTypes = Observer.eventTypes = (\"ontouchstart\" in _docEl ? \"touchstart,touchmove,touchcancel,touchend\" : !(\"onpointerdown\" in _docEl) ? \"mousedown,mousemove,mouseup,mouseup\" : \"pointerdown,pointermove,pointercancel,pointerup\").split(\",\");\n\t\t\tsetTimeout(() => _startup = 0, 500);\n\t\t\t_setScrollTrigger();\n\t\t\t_coreInitted = 1;\n\t\t}\n\t\treturn _coreInitted;\n\t};\n\n_horizontal.op = _vertical;\n_scrollers.cache = 0;\n\nexport class Observer {\n\tconstructor(vars) {\n\t\tthis.init(vars);\n\t}\n\n\tinit(vars) {\n\t\t_coreInitted || _initCore(gsap) || console.warn(\"Please gsap.registerPlugin(Observer)\");\n\t\tScrollTrigger || _setScrollTrigger();\n\t\tlet {tolerance, dragMinimum, type, target, lineHeight, debounce, preventDefault, onStop, onStopDelay, ignore, wheelSpeed, event, onDragStart, onDragEnd, onDrag, onPress, onRelease, onRight, onLeft, onUp, onDown, onChangeX, onChangeY, onChange, onToggleX, onToggleY, onHover, onHoverEnd, onMove, ignoreCheck, isNormalizer, onGestureStart, onGestureEnd, onWheel, onEnable, onDisable, onClick, scrollSpeed, capture, allowClicks, lockAxis, onLockAxis} = vars;\n\t\tthis.target = target = _getTarget(target) || _docEl;\n\t\tthis.vars = vars;\n\t\tignore && (ignore = gsap.utils.toArray(ignore));\n\t\ttolerance = tolerance || 1e-9;\n\t\tdragMinimum = dragMinimum || 0;\n\t\twheelSpeed = wheelSpeed || 1;\n\t\tscrollSpeed = scrollSpeed || 1;\n\t\ttype = type || \"wheel,touch,pointer\";\n\t\tdebounce = debounce !== false;\n\t\tlineHeight || (lineHeight = parseFloat(_win.getComputedStyle(_body).lineHeight) || 22); // note: browser may report \"normal\", so default to 22.\n\t\tlet id, onStopDelayedCall, dragged, moved, wheeled, locked, axis,\n\t\t\tself = this,\n\t\t\tprevDeltaX = 0,\n\t\t\tprevDeltaY = 0,\n\t\t\tpassive = vars.passive || (!preventDefault && vars.passive !== false),\n\t\t\tscrollFuncX = _getScrollFunc(target, _horizontal),\n\t\t\tscrollFuncY = _getScrollFunc(target, _vertical),\n\t\t\tscrollX = scrollFuncX(),\n\t\t\tscrollY = scrollFuncY(),\n\t\t\tlimitToTouch = ~type.indexOf(\"touch\") && !~type.indexOf(\"pointer\") && _eventTypes[0] === \"pointerdown\", // for devices that accommodate mouse events and touch events, we need to distinguish.\n\t\t\tisViewport = _isViewport(target),\n\t\t\townerDoc = target.ownerDocument || _doc,\n\t\t\tdeltaX = [0, 0, 0], // wheel, scroll, pointer/touch\n\t\t\tdeltaY = [0, 0, 0],\n\t\t\tonClickTime = 0,\n\t\t\tclickCapture = () => onClickTime = _getTime(),\n\t\t\t_ignoreCheck = (e, isPointerOrTouch) => (self.event = e) && (ignore && ~ignore.indexOf(e.target)) || (isPointerOrTouch && limitToTouch && e.pointerType !== \"touch\") || (ignoreCheck && ignoreCheck(e, isPointerOrTouch)),\n\t\t\tonStopFunc = () => {\n\t\t\t\tself._vx.reset();\n\t\t\t\tself._vy.reset();\n\t\t\t\tonStopDelayedCall.pause();\n\t\t\t\tonStop && onStop(self);\n\t\t\t},\n\t\t\tupdate = () => {\n\t\t\t\tlet dx = self.deltaX = _getAbsoluteMax(deltaX),\n\t\t\t\t\tdy = self.deltaY = _getAbsoluteMax(deltaY),\n\t\t\t\t\tchangedX = Math.abs(dx) >= tolerance,\n\t\t\t\t\tchangedY = Math.abs(dy) >= tolerance;\n\t\t\t\tonChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); // in ScrollTrigger.normalizeScroll(), we need to know if it was touch/pointer so we need access to the deltaX/deltaY Arrays before we clear them out.\n\t\t\t\tif (changedX) {\n\t\t\t\t\tonRight && self.deltaX > 0 && onRight(self);\n\t\t\t\t\tonLeft && self.deltaX < 0 && onLeft(self);\n\t\t\t\t\tonChangeX && onChangeX(self);\n\t\t\t\t\tonToggleX && ((self.deltaX < 0) !== (prevDeltaX < 0)) && onToggleX(self);\n\t\t\t\t\tprevDeltaX = self.deltaX;\n\t\t\t\t\tdeltaX[0] = deltaX[1] = deltaX[2] = 0\n\t\t\t\t}\n\t\t\t\tif (changedY) {\n\t\t\t\t\tonDown && self.deltaY > 0 && onDown(self);\n\t\t\t\t\tonUp && self.deltaY < 0 && onUp(self);\n\t\t\t\t\tonChangeY && onChangeY(self);\n\t\t\t\t\tonToggleY && ((self.deltaY < 0) !== (prevDeltaY < 0)) && onToggleY(self);\n\t\t\t\t\tprevDeltaY = self.deltaY;\n\t\t\t\t\tdeltaY[0] = deltaY[1] = deltaY[2] = 0\n\t\t\t\t}\n\t\t\t\tif (moved || dragged) {\n\t\t\t\t\tonMove && onMove(self);\n\t\t\t\t\tif (dragged) {\n\t\t\t\t\t\tonDragStart && dragged === 1 && onDragStart(self);\n\t\t\t\t\t\tonDrag && onDrag(self);\n\t\t\t\t\t\tdragged = 0;\n\t\t\t\t\t}\n\t\t\t\t\tmoved = false;\n\t\t\t\t}\n\t\t\t\tlocked && !(locked = false) && onLockAxis && onLockAxis(self);\n\t\t\t\tif (wheeled) {\n\t\t\t\t\tonWheel(self);\n\t\t\t\t\twheeled = false;\n\t\t\t\t}\n\t\t\t\tid = 0;\n\t\t\t},\n\t\t\tonDelta = (x, y, index) => {\n\t\t\t\tdeltaX[index] += x;\n\t\t\t\tdeltaY[index] += y;\n\t\t\t\tself._vx.update(x);\n\t\t\t\tself._vy.update(y);\n\t\t\t\tdebounce ? id || (id = requestAnimationFrame(update)) : update();\n\t\t\t},\n\t\t\tonTouchOrPointerDelta = (x, y) => {\n\t\t\t\tif (lockAxis && !axis) {\n\t\t\t\t\tself.axis = axis = Math.abs(x) > Math.abs(y) ? \"x\" : \"y\";\n\t\t\t\t\tlocked = true;\n\t\t\t\t}\n\t\t\t\tif (axis !== \"y\") {\n\t\t\t\t\tdeltaX[2] += x;\n\t\t\t\t\tself._vx.update(x, true); // update the velocity as frequently as possible instead of in the debounced function so that very quick touch-scrolls (flicks) feel natural. If it's the mouse/touch/pointer, force it so that we get snappy/accurate momentum scroll.\n\t\t\t\t}\n\t\t\t\tif (axis !== \"x\") {\n\t\t\t\t\tdeltaY[2] += y;\n\t\t\t\t\tself._vy.update(y, true);\n\t\t\t\t}\n\t\t\t\tdebounce ? id || (id = requestAnimationFrame(update)) : update();\n\t\t\t},\n\t\t\t_onDrag = e => {\n\t\t\t\tif (_ignoreCheck(e, 1)) {return;}\n\t\t\t\te = _getEvent(e, preventDefault);\n\t\t\t\tlet x = e.clientX,\n\t\t\t\t\ty = e.clientY,\n\t\t\t\t\tdx = x - self.x,\n\t\t\t\t\tdy = y - self.y,\n\t\t\t\t\tisDragging = self.isDragging;\n\t\t\t\tself.x = x;\n\t\t\t\tself.y = y;\n\t\t\t\tif (isDragging || ((dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum))) {\n\t\t\t\t\tdragged = isDragging ? 2 : 1; // dragged: 0 = not dragging, 1 = first drag, 2 = normal drag\n\t\t\t\t\tisDragging || (self.isDragging = true);\n\t\t\t\t\tonTouchOrPointerDelta(dx, dy);\n\t\t\t\t}\n\t\t\t},\n\t\t\t_onPress = self.onPress = e => {\n\t\t\t\tif (_ignoreCheck(e, 1) || (e && e.button)) {return;}\n\t\t\t\tself.axis = axis = null;\n\t\t\t\tonStopDelayedCall.pause();\n\t\t\t\tself.isPressed = true;\n\t\t\t\te = _getEvent(e); // note: may need to preventDefault(?) Won't side-scroll on iOS Safari if we do, though.\n\t\t\t\tprevDeltaX = prevDeltaY = 0;\n\t\t\t\tself.startX = self.x = e.clientX;\n\t\t\t\tself.startY = self.y = e.clientY;\n\t\t\t\tself._vx.reset(); // otherwise the t2 may be stale if the user touches and flicks super fast and releases in less than 2 requestAnimationFrame ticks, causing velocity to be 0.\n\t\t\t\tself._vy.reset();\n\t\t\t\t_addListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true);\n\t\t\t\tself.deltaX = self.deltaY = 0;\n\t\t\t\tonPress && onPress(self);\n\t\t\t},\n\t\t\t_onRelease = self.onRelease = e => {\n\t\t\t\tif (_ignoreCheck(e, 1)) {return;}\n\t\t\t\t_removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true);\n\t\t\t\tlet isTrackingDrag = !isNaN(self.y - self.startY),\n\t\t\t\t\twasDragging = self.isDragging,\n\t\t\t\t\tisDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), // some touch devices need some wiggle room in terms of sensing clicks - the finger may move a few pixels.\n\t\t\t\t\teventData = _getEvent(e);\n\t\t\t\tif (!isDragNotClick && isTrackingDrag) {\n\t\t\t\t\tself._vx.reset();\n\t\t\t\t\tself._vy.reset();\n\t\t\t\t\t//if (preventDefault && allowClicks && self.isPressed) { // check isPressed because in a rare edge case, the inputObserver in ScrollTrigger may stopPropagation() on the press/drag, so the onRelease may get fired without the onPress/onDrag ever getting called, thus it could trigger a click to occur on a link after scroll-dragging it.\n\t\t\t\t\tif (preventDefault && allowClicks) {\n\t\t\t\t\t\tgsap.delayedCall(0.08, () => { // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular \"click\" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the \"real\"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the \"real\" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched.\n\t\t\t\t\t\t\tif (_getTime() - onClickTime > 300 && !e.defaultPrevented) {\n\t\t\t\t\t\t\t\tif (e.target.click) { //some browsers (like mobile Safari) don't properly trigger the click event\n\t\t\t\t\t\t\t\t\te.target.click();\n\t\t\t\t\t\t\t\t} else if (ownerDoc.createEvent) {\n\t\t\t\t\t\t\t\t\tlet syntheticEvent = ownerDoc.createEvent(\"MouseEvents\");\n\t\t\t\t\t\t\t\t\tsyntheticEvent.initMouseEvent(\"click\", true, true, _win, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null);\n\t\t\t\t\t\t\t\t\te.target.dispatchEvent(syntheticEvent);\n\t\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t});\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tself.isDragging = self.isGesturing = self.isPressed = false;\n\t\t\t\tonStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true);\n\t\t\t\tdragged && update(); // in case debouncing, we don't want onDrag to fire AFTER onDragEnd().\n\t\t\t\tonDragEnd && wasDragging && onDragEnd(self);\n\t\t\t\tonRelease && onRelease(self, isDragNotClick);\n\t\t\t},\n\t\t\t_onGestureStart = e => e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging),\n\t\t\t_onGestureEnd = () => (self.isGesturing = false) || onGestureEnd(self),\n\t\t\tonScroll = e => {\n\t\t\t\tif (_ignoreCheck(e)) {return;}\n\t\t\t\tlet x = scrollFuncX(),\n\t\t\t\t\ty = scrollFuncY();\n\t\t\t\tonDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1);\n\t\t\t\tscrollX = x;\n\t\t\t\tscrollY = y;\n\t\t\t\tonStop && onStopDelayedCall.restart(true);\n\t\t\t},\n\t\t\t_onWheel = e => {\n\t\t\t\tif (_ignoreCheck(e)) {return;}\n\t\t\t\te = _getEvent(e, preventDefault);\n\t\t\t\tonWheel && (wheeled = true);\n\t\t\t\tlet multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win.innerHeight : 1) * wheelSpeed;\n\t\t\t\tonDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0);\n\t\t\t\tonStop && !isNormalizer && onStopDelayedCall.restart(true);\n\t\t\t},\n\t\t\t_onMove = e => {\n\t\t\t\tif (_ignoreCheck(e)) {return;}\n\t\t\t\tlet x = e.clientX,\n\t\t\t\t\ty = e.clientY,\n\t\t\t\t\tdx = x - self.x,\n\t\t\t\t\tdy = y - self.y;\n\t\t\t\tself.x = x;\n\t\t\t\tself.y = y;\n\t\t\t\tmoved = true;\n\t\t\t\tonStop && onStopDelayedCall.restart(true);\n\t\t\t\t(dx || dy) && onTouchOrPointerDelta(dx, dy);\n\t\t\t},\n\t\t\t_onHover = e => {self.event = e; onHover(self);},\n\t\t\t_onHoverEnd = e => {self.event = e; onHoverEnd(self);},\n\t\t\t_onClick = e => _ignoreCheck(e) || (_getEvent(e, preventDefault) && onClick(self));\n\n\t\tonStopDelayedCall = self._dc = gsap.delayedCall(onStopDelay || 0.25, onStopFunc).pause();\n\n\t\tself.deltaX = self.deltaY = 0;\n\t\tself._vx = _getVelocityProp(0, 50, true);\n\t\tself._vy = _getVelocityProp(0, 50, true);\n\t\tself.scrollX = scrollFuncX;\n\t\tself.scrollY = scrollFuncY;\n\t\tself.isDragging = self.isGesturing = self.isPressed = false;\n\t\t_context(this);\n\t\tself.enable = e => {\n\t\t\tif (!self.isEnabled) {\n\t\t\t\t_addListener(isViewport ? ownerDoc : target, \"scroll\", _onScroll);\n\t\t\t\ttype.indexOf(\"scroll\") >= 0 && _addListener(isViewport ? ownerDoc : target, \"scroll\", onScroll, passive, capture);\n\t\t\t\ttype.indexOf(\"wheel\") >= 0 && _addListener(target, \"wheel\", _onWheel, passive, capture);\n\t\t\t\tif ((type.indexOf(\"touch\") >= 0 && _isTouch) || type.indexOf(\"pointer\") >= 0) {\n\t\t\t\t\t_addListener(target, _eventTypes[0], _onPress, passive, capture);\n\t\t\t\t\t_addListener(ownerDoc, _eventTypes[2], _onRelease);\n\t\t\t\t\t_addListener(ownerDoc, _eventTypes[3], _onRelease);\n\t\t\t\t\tallowClicks && _addListener(target, \"click\", clickCapture, true, true);\n\t\t\t\t\tonClick && _addListener(target, \"click\", _onClick);\n\t\t\t\t\tonGestureStart && _addListener(ownerDoc, \"gesturestart\", _onGestureStart);\n\t\t\t\t\tonGestureEnd && _addListener(ownerDoc, \"gestureend\", _onGestureEnd);\n\t\t\t\t\tonHover && _addListener(target, _pointerType + \"enter\", _onHover);\n\t\t\t\t\tonHoverEnd && _addListener(target, _pointerType + \"leave\", _onHoverEnd);\n\t\t\t\t\tonMove && _addListener(target, _pointerType + \"move\", _onMove);\n\t\t\t\t}\n\t\t\t\tself.isEnabled = true;\n\t\t\t\tself.isDragging = self.isGesturing = self.isPressed = moved = dragged = false;\n\t\t\t\tself._vx.reset();\n\t\t\t\tself._vy.reset();\n\t\t\t\tscrollX = scrollFuncX();\n\t\t\t\tscrollY = scrollFuncY();\n\t\t\t\te && e.type && _onPress(e);\n\t\t\t\tonEnable && onEnable(self);\n\t\t\t}\n\t\t\treturn self;\n\t\t};\n\t\tself.disable = () => {\n\t\t\tif (self.isEnabled) {\n\t\t\t\t// only remove the _onScroll listener if there aren't any others that rely on the functionality.\n\t\t\t\t_observers.filter(o => o !== self && _isViewport(o.target)).length || _removeListener(isViewport ? ownerDoc : target, \"scroll\", _onScroll);\n\t\t\t\tif (self.isPressed) {\n\t\t\t\t\tself._vx.reset();\n\t\t\t\t\tself._vy.reset();\n\t\t\t\t\t_removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true);\n\t\t\t\t}\n\t\t\t\t_removeListener(isViewport ? ownerDoc : target, \"scroll\", onScroll, capture);\n\t\t\t\t_removeListener(target, \"wheel\", _onWheel, capture);\n\t\t\t\t_removeListener(target, _eventTypes[0], _onPress, capture);\n\t\t\t\t_removeListener(ownerDoc, _eventTypes[2], _onRelease);\n\t\t\t\t_removeListener(ownerDoc, _eventTypes[3], _onRelease);\n\t\t\t\t_removeListener(target, \"click\", clickCapture, true);\n\t\t\t\t_removeListener(target, \"click\", _onClick);\n\t\t\t\t_removeListener(ownerDoc, \"gesturestart\", _onGestureStart);\n\t\t\t\t_removeListener(ownerDoc, \"gestureend\", _onGestureEnd);\n\t\t\t\t_removeListener(target, _pointerType + \"enter\", _onHover);\n\t\t\t\t_removeListener(target, _pointerType + \"leave\", _onHoverEnd);\n\t\t\t\t_removeListener(target, _pointerType + \"move\", _onMove);\n\t\t\t\tself.isEnabled = self.isPressed = self.isDragging = false;\n\t\t\t\tonDisable && onDisable(self);\n\t\t\t}\n\t\t};\n\n\t\tself.kill = self.revert = () => {\n\t\t\tself.disable();\n\t\t\tlet i = _observers.indexOf(self);\n\t\t\ti >= 0 && _observers.splice(i, 1);\n\t\t\t_normalizer === self && (_normalizer = 0);\n\t\t}\n\n\t\t_observers.push(self);\n\t\tisNormalizer && _isViewport(target) && (_normalizer = self);\n\n\t\tself.enable(event);\n\t}\n\n\tget velocityX() {\n\t\treturn this._vx.getVelocity();\n\t}\n\tget velocityY() {\n\t\treturn this._vy.getVelocity();\n\t}\n\n}\n\nObserver.version = \"3.12.7\";\nObserver.create = vars => new Observer(vars);\nObserver.register = _initCore;\nObserver.getAll = () => _observers.slice();\nObserver.getById = id => _observers.filter(o => o.vars.id === id)[0];\n\n_getGSAP() && gsap.registerPlugin(Observer);\n\nexport { Observer as default, _isViewport, _scrollers, _getScrollFunc, _getProxyProp, _proxies, _getVelocityProp, _vertical, _horizontal, _getTarget };"],"names":["_getGSAP","gsap","window","registerPlugin","_coreInitted","_win","_doc","_docEl","_body","_isTouch","_pointerType","ScrollTrigger","_root","_normalizer","_eventTypes","_context","_startup","_observers","_scrollers","_proxies","_getProxyProp","element","property","indexOf","_isViewport","el","_addListener","type","func","passive","capture","addEventListener","_removeListener","removeEventListener","_onScroll","isPressed","cache","_scrollCacheFunc","f","doNotCache","cachingFunc","value","history","scrollRestoration","isNormalizing","v","Math","round","iOS","cacheID","_bridge","offset","_getTarget","t","self","_ctx","selector","utils","toArray","config","nullTargetWarn","console","warn","_getScrollFunc","s","sc","scrollingElement","i","_vertical","push","prev","arguments","length","target","smooth","getProperty","_getVelocityProp","minTimeRefresh","useDelta","update","force","_getTime","min","t1","v2","v1","t2","dropToZeroTime","max","reset","getVelocity","latestValue","tOld","vOld","_getEvent","e","preventDefault","_gsapAllow","changedTouches","_getAbsoluteMax","a","abs","_setScrollTrigger","core","globals","_integrate","data","bridge","scrollers","proxies","name","_initCore","document","body","documentElement","clamp","context","Observer","isTouch","matchMedia","matches","navigator","maxTouchPoints","msMaxTouchPoints","eventTypes","split","setTimeout","Date","now","_scrollLeft","_scrollTop","_horizontal","p","p2","os","os2","d","d2","scrollTo","pageXOffset","op","pageYOffset","init","vars","tolerance","dragMinimum","lineHeight","debounce","onStop","onStopDelay","ignore","wheelSpeed","event","onDragStart","onDragEnd","onDrag","onPress","onRelease","onRight","onLeft","onUp","onDown","onChangeX","onChangeY","onChange","onToggleX","onToggleY","onHover","onHoverEnd","onMove","ignoreCheck","isNormalizer","onGestureStart","onGestureEnd","onWheel","onEnable","onDisable","onClick","scrollSpeed","allowClicks","lockAxis","onLockAxis","clickCapture","onClickTime","_ignoreCheck","isPointerOrTouch","limitToTouch","pointerType","dx","deltaX","dy","deltaY","changedX","changedY","prevDeltaX","prevDeltaY","moved","dragged","locked","wheeled","id","onDelta","x","y","index","_vx","_vy","requestAnimationFrame","onTouchOrPointerDelta","axis","_onDrag","clientX","clientY","isDragging","startX","startY","_onGestureStart","touches","isGesturing","_onGestureEnd","onScroll","scrollFuncX","scrollFuncY","scrollX","scrollY","onStopDelayedCall","restart","_onWheel","multiplier","deltaMode","innerHeight","_onMove","_onHover","_onHoverEnd","_onClick","parseFloat","getComputedStyle","this","isViewport","ownerDoc","ownerDocument","_onPress","button","pause","_onRelease","isTrackingDrag","isNaN","wasDragging","isDragNotClick","eventData","delayedCall","defaultPrevented","click","createEvent","syntheticEvent","initMouseEvent","screenX","screenY","dispatchEvent","_dc","onStopFunc","enable","isEnabled","disable","filter","o","kill","revert","splice","version","create","register","getAll","slice","getById"],"mappings":";;;;;;;;;mYAYY,SAAXA,WAAiBC,IAA4B,oBAAZC,SAA4BD,GAAOC,OAAOD,OAASA,GAAKE,gBAAkBF,OADxGA,GAAMG,GAAsBC,GAAMC,GAAMC,GAAQC,GAAOC,GAAUC,GAAcC,GAAeC,EAAOC,GAAaC,GAAaC,GAElIC,EAAW,EACXC,GAAa,GACbC,aAAa,GACbC,WAAW,GAcK,SAAhBC,EAAiBC,EAASC,UAAcH,WAASI,QAAQF,IAAYF,WAASA,WAASI,QAAQF,GAAW,GAAGC,GAC/F,SAAdE,EAAcC,YAASb,EAAMW,QAAQE,GACtB,SAAfC,EAAgBL,EAASM,EAAMC,EAAMC,EAASC,UAAYT,EAAQU,iBAAiBJ,EAAMC,EAAM,CAACC,SAAqB,IAAZA,EAAmBC,UAAWA,IACrH,SAAlBE,EAAmBX,EAASM,EAAMC,EAAME,UAAYT,EAAQY,oBAAoBN,EAAMC,IAAQE,GAGlF,SAAZI,WAAmBrB,IAAeA,GAAYsB,WAAcjB,aAAWkB,QACpD,SAAnBC,EAAoBC,EAAGC,GACJ,SAAdC,GAAcC,MACbA,GAAmB,IAAVA,EAAa,CACzBzB,IAAaX,GAAKqC,QAAQC,kBAAoB,cAC1CC,EAAgB/B,IAAeA,GAAYsB,UAC/CM,EAAQD,GAAYK,EAAIC,KAAKC,MAAMN,KAAW5B,IAAeA,GAAYmC,IAAM,EAAI,GACnFV,EAAEG,GACFD,GAAYS,QAAU/B,aAAWkB,MACjCQ,GAAiBM,EAAQ,KAAMT,QACrBF,GAAcrB,aAAWkB,QAAUI,GAAYS,SAAWC,EAAQ,UAC5EV,GAAYS,QAAU/B,aAAWkB,MACjCI,GAAYK,EAAIP,YAEVE,GAAYK,EAAIL,GAAYW,cAEpCX,GAAYW,OAAS,EACdb,GAAKE,GAIA,SAAbY,EAAcC,EAAGC,UAAWA,GAAQA,EAAKC,MAAQD,EAAKC,KAAKC,UAAavD,GAAKwD,MAAMC,SAASL,GAAG,KAAqB,iBAAPA,IAAoD,IAAjCpD,GAAK0D,SAASC,eAA2BC,QAAQC,KAAK,qBAAsBT,GAAK,MAEhM,SAAjBU,EAAkB1C,SAAU2C,IAAAA,EAAGC,IAAAA,GAC9BzC,EAAYH,KAAaA,EAAUf,GAAK4D,kBAAoB3D,QACxD4D,EAAIjD,aAAWK,QAAQF,GAC1B8B,EAASc,IAAOG,GAAUH,GAAK,EAAI,GAClCE,IAAMA,EAAIjD,aAAWmD,KAAKhD,GAAW,GACvCH,aAAWiD,EAAIhB,IAAWzB,EAAaL,EAAS,SAAUa,OACtDoC,EAAOpD,aAAWiD,EAAIhB,GACzBvB,EAAO0C,IAASpD,aAAWiD,EAAIhB,GAAUd,EAAiBjB,EAAcC,EAAS2C,IAAI,KAAUxC,EAAYH,GAAW4C,EAAK5B,EAAiB,SAASI,UAAgB8B,UAAUC,OAAUnD,EAAQ2C,GAAKvB,EAASpB,EAAQ2C,cACxNpC,EAAK6C,OAASpD,EACdiD,IAAS1C,EAAK8C,OAAyD,WAAhDzE,GAAK0E,YAAYtD,EAAS,mBAC1CO,EAEW,SAAnBgD,EAAoBnC,EAAOoC,EAAgBC,GAOhC,SAATC,GAAUtC,EAAOuC,OACZ3B,EAAI4B,KACJD,GAAkBE,EAAT7B,EAAI8B,GAChBC,EAAKC,EACLA,EAAK5C,EACL6C,EAAKH,EACLA,EAAK9B,GACKyB,EACVO,GAAM5C,EAEN4C,EAAKD,GAAM3C,EAAQ2C,IAAO/B,EAAIiC,IAAOH,EAAKG,OAhBzCD,EAAK5C,EACR2C,EAAK3C,EACL0C,EAAKF,KACLK,EAAKH,EACLD,EAAML,GAAkB,GACxBU,EAAiBzC,KAAK0C,IAAI,IAAW,EAANN,SAsBzB,CAACH,OAAAA,GAAQU,MARP,SAARA,QAAgBL,EAAKC,EAAKP,EAAW,EAAIO,EAAIC,EAAKH,EAAK,GAQjCO,YAPR,SAAdA,YAAcC,OACTC,EAAON,EACVO,EAAOT,EACP/B,EAAI4B,YACJU,GAA+B,IAAhBA,GAAsBA,IAAgBN,GAAMN,GAAOY,GAC3DR,IAAOG,GAAeC,EAATlC,EAAIiC,EAAuB,GAAKD,GAAMP,EAAWe,GAAQA,MAAWf,EAAWzB,EAAI8B,GAAMS,GAAQ,MAI7G,SAAZE,EAAaC,EAAGC,UACfA,IAAmBD,EAAEE,YAAcF,EAAEC,iBAC9BD,EAAEG,eAAiBH,EAAEG,eAAe,GAAKH,EAE/B,SAAlBI,EAAkBC,OACbZ,EAAM1C,KAAK0C,UAAL1C,KAAYsD,GACrBlB,EAAMpC,KAAKoC,UAALpC,KAAYsD,UACZtD,KAAKuD,IAAIb,IAAQ1C,KAAKuD,IAAInB,GAAOM,EAAMN,EAE3B,SAApBoB,KACC3F,GAAgBV,GAAKsG,KAAKC,UAAU7F,gBACnBA,GAAc4F,MA7FnB,SAAbE,iBACKF,EAAO5F,GAAc4F,KACxBG,EAAOH,EAAKI,QAAU,GACtBC,EAAYL,EAAKrF,WACjB2F,EAAUN,EAAKpF,SAChByF,EAAUvC,WAAVuC,EAAkB1F,cAClB2F,EAAQxC,WAARwC,EAAgB1F,YAChBD,aAAa0F,EACbzF,WAAW0F,EACX3D,EAAU,iBAAC4D,EAAMrE,UAAUiE,EAAKI,GAAMrE,IAoFCgE,GAE5B,SAAZM,EAAYR,UACXtG,GAAOsG,GAAQvG,KACVI,IAAgBH,IAA6B,oBAAd+G,UAA6BA,SAASC,OACzE5G,GAAOH,OAEPK,IADAD,GAAO0G,UACOE,gBACd1G,GAAQF,GAAK2G,KACbrG,EAAQ,CAACP,GAAMC,GAAMC,GAAQC,IACpBP,GAAKwD,MAAM0D,MACpBpG,GAAWd,GAAKsG,KAAKa,SAAW,aAChC1G,GAAe,mBAAoBF,GAAQ,UAAY,QAEvDC,GAAW4G,EAASC,QAAUjH,GAAKkH,YAAclH,GAAKkH,WAAW,oCAAoCC,QAAU,EAAK,iBAAkBnH,IAAmC,EAA3BoH,UAAUC,gBAAmD,EAA7BD,UAAUE,iBAAwB,EAAI,EACpN7G,GAAcuG,EAASO,YAAc,iBAAkBrH,GAAS,4CAAgD,kBAAmBA,GAAkD,kDAAxC,uCAA2FsH,MAAM,KAC9OC,WAAW,kBAAM9G,EAAW,GAAG,KAC/BsF,IACAlG,GAAe,GAETA,OAnHR6E,GAAW8C,KAAKC,IAChB9E,EAAU,iBAAC4D,EAAMrE,UAAUA,GAgB3BwF,EAAc,aACdC,EAAa,YAoBbC,GAAc,CAACnE,EAAGiE,EAAaG,EAAG,OAAQC,GAAI,OAAQC,GAAI,QAASC,IAAK,QAASC,EAAG,QAASC,GAAI,QAASrC,EAAG,IAAKnC,GAAI5B,EAAiB,SAASI,UAAgB8B,UAAUC,OAASnE,GAAKqI,SAASjG,EAAO2B,GAAUH,MAAQ5D,GAAKsI,aAAerI,GAAK2H,IAAgB1H,GAAO0H,IAAgBzH,GAAMyH,IAAgB,KAChT7D,GAAY,CAACJ,EAAGkE,EAAYE,EAAG,MAAOC,GAAI,MAAOC,GAAI,SAAUC,IAAK,SAAUC,EAAG,SAAUC,GAAI,SAAUrC,EAAG,IAAKwC,GAAIT,GAAalE,GAAI5B,EAAiB,SAASI,UAAgB8B,UAAUC,OAASnE,GAAKqI,SAASP,GAAYlE,KAAMxB,GAASpC,GAAKwI,aAAevI,GAAK4H,IAAe3H,GAAO2H,IAAe1H,GAAM0H,IAAe,KA+EhUC,GAAYS,GAAKxE,gBACNhC,MAAQ,MAENiF,sBAKZyB,KAAA,cAAKC,GACJ3I,IAAgB2G,EAAU9G,KAAS4D,QAAQC,KAAK,wCAChDnD,IAAiB2F,QACZ0C,EAA6bD,EAA7bC,UAAWC,EAAkbF,EAAlbE,YAAatH,EAAqaoH,EAArapH,KAAM8C,EAA+ZsE,EAA/ZtE,OAAQyE,EAAuZH,EAAvZG,WAAYC,EAA2YJ,EAA3YI,SAAUnD,EAAiY+C,EAAjY/C,eAAgBoD,EAAiXL,EAAjXK,OAAQC,EAAyWN,EAAzWM,YAAaC,EAA4VP,EAA5VO,OAAQC,EAAoVR,EAApVQ,WAAYC,EAAwUT,EAAxUS,MAAOC,EAAiUV,EAAjUU,YAAaC,EAAoTX,EAApTW,UAAWC,EAAySZ,EAAzSY,OAAQC,EAAiSb,EAAjSa,QAASC,EAAwRd,EAAxRc,UAAWC,EAA6Qf,EAA7Qe,QAASC,EAAoQhB,EAApQgB,OAAQC,EAA4PjB,EAA5PiB,KAAMC,EAAsPlB,EAAtPkB,OAAQC,EAA8OnB,EAA9OmB,UAAWC,EAAmOpB,EAAnOoB,UAAWC,EAAwNrB,EAAxNqB,SAAUC,EAA8MtB,EAA9MsB,UAAWC,EAAmMvB,EAAnMuB,UAAWC,EAAwLxB,EAAxLwB,QAASC,EAA+KzB,EAA/KyB,WAAYC,EAAmK1B,EAAnK0B,OAAQC,EAA2J3B,EAA3J2B,YAAaC,EAA8I5B,EAA9I4B,aAAcC,EAAgI7B,EAAhI6B,eAAgBC,EAAgH9B,EAAhH8B,aAAcC,EAAkG/B,EAAlG+B,QAASC,EAAyFhC,EAAzFgC,SAAUC,EAA+EjC,EAA/EiC,UAAWC,EAAoElC,EAApEkC,QAASC,EAA2DnC,EAA3DmC,YAAapJ,EAA8CiH,EAA9CjH,QAASqJ,EAAqCpC,EAArCoC,YAAaC,EAAwBrC,EAAxBqC,SAAUC,EAActC,EAAdsC,WA0Bpa,SAAfC,YAAqBC,GAActG,KACpB,SAAfuG,GAAgBzF,EAAG0F,UAAsBnI,GAAKkG,MAAQzD,IAAOuD,IAAWA,EAAO/H,QAAQwE,EAAEtB,SAAagH,GAAoBC,IAAkC,UAAlB3F,EAAE4F,aAA6BjB,GAAeA,EAAY3E,EAAG0F,GAO9L,SAAT1G,SACK6G,EAAKtI,GAAKuI,OAAS1F,EAAgB0F,IACtCC,EAAKxI,GAAKyI,OAAS5F,EAAgB4F,IACnCC,EAAWlJ,KAAKuD,IAAIuF,IAAO5C,EAC3BiD,EAAWnJ,KAAKuD,IAAIyF,IAAO9C,EAC5BoB,IAAa4B,GAAYC,IAAa7B,EAAS9G,GAAMsI,EAAIE,EAAID,GAAQE,IACjEC,IACHlC,GAAyB,EAAdxG,GAAKuI,QAAc/B,EAAQxG,IACtCyG,GAAUzG,GAAKuI,OAAS,GAAK9B,EAAOzG,IACpC4G,GAAaA,EAAU5G,IACvB+G,GAAe/G,GAAKuI,OAAS,GAAQK,GAAa,GAAO7B,EAAU/G,IACnE4I,GAAa5I,GAAKuI,OAClBA,GAAO,GAAKA,GAAO,GAAKA,GAAO,GAAK,GAEjCI,IACHhC,GAAwB,EAAd3G,GAAKyI,QAAc9B,EAAO3G,IACpC0G,GAAQ1G,GAAKyI,OAAS,GAAK/B,EAAK1G,IAChC6G,GAAaA,EAAU7G,IACvBgH,GAAehH,GAAKyI,OAAS,GAAQI,GAAa,GAAO7B,EAAUhH,IACnE6I,GAAa7I,GAAKyI,OAClBA,GAAO,GAAKA,GAAO,GAAKA,GAAO,GAAK,IAEjCK,IAASC,MACZ5B,GAAUA,EAAOnH,IACb+I,KACH5C,GAA2B,IAAZ4C,IAAiB5C,EAAYnG,IAC5CqG,GAAUA,EAAOrG,IACjB+I,GAAU,GAEXD,IAAQ,GAETE,MAAYA,IAAS,IAAUjB,GAAcA,EAAW/H,IACpDiJ,KACHzB,EAAQxH,IACRiJ,IAAU,GAEXC,GAAK,EAEI,SAAVC,GAAWC,EAAGC,EAAGC,GAChBf,GAAOe,IAAUF,EACjBX,GAAOa,IAAUD,EACjBrJ,GAAKuJ,IAAI9H,OAAO2H,GAChBpJ,GAAKwJ,IAAI/H,OAAO4H,GAChBxD,EAAkBqD,GAAPA,IAAYO,sBAAsBhI,IAAWA,KAEjC,SAAxBiI,GAAyBN,EAAGC,GACvBvB,IAAa6B,KAChB3J,GAAK2J,KAAOA,GAAOnK,KAAKuD,IAAIqG,GAAK5J,KAAKuD,IAAIsG,GAAK,IAAM,IACrDL,IAAS,GAEG,MAATW,KACHpB,GAAO,IAAMa,EACbpJ,GAAKuJ,IAAI9H,OAAO2H,GAAG,IAEP,MAATO,KACHlB,GAAO,IAAMY,EACbrJ,GAAKwJ,IAAI/H,OAAO4H,GAAG,IAEpBxD,EAAkBqD,GAAPA,IAAYO,sBAAsBhI,IAAWA,KAE/C,SAAVmI,GAAUnH,OACLyF,GAAazF,EAAG,QAEhB2G,GADJ3G,EAAID,EAAUC,EAAGC,IACPmH,QACTR,EAAI5G,EAAEqH,QACNxB,EAAKc,EAAIpJ,GAAKoJ,EACdZ,EAAKa,EAAIrJ,GAAKqJ,EACdU,EAAa/J,GAAK+J,WACnB/J,GAAKoJ,EAAIA,EACTpJ,GAAKqJ,EAAIA,GACLU,IAAgBzB,GAAME,KAAQhJ,KAAKuD,IAAI/C,GAAKgK,OAASZ,IAAMzD,GAAenG,KAAKuD,IAAI/C,GAAKiK,OAASZ,IAAM1D,MAC1GoD,GAAUgB,EAAa,EAAI,EAC3BA,IAAe/J,GAAK+J,YAAa,GACjCL,GAAsBpB,EAAIE,KAiDV,SAAlB0B,GAAkBzH,UAAKA,EAAE0H,SAA8B,EAAnB1H,EAAE0H,QAAQjJ,SAAelB,GAAKoK,aAAc,IAAS9C,EAAe7E,EAAGzC,GAAK+J,YAChG,SAAhBM,YAAuBrK,GAAKoK,aAAc,IAAU7C,EAAavH,IACtD,SAAXsK,GAAW7H,OACNyF,GAAazF,QACb2G,EAAImB,KACPlB,EAAImB,KACLrB,IAASC,EAAIqB,IAAW7C,GAAcyB,EAAIqB,IAAW9C,EAAa,GAClE6C,GAAUrB,EACVsB,GAAUrB,EACVvD,GAAU6E,GAAkBC,SAAQ,IAE1B,SAAXC,GAAWpI,OACNyF,GAAazF,IACjBA,EAAID,EAAUC,EAAGC,GACjB8E,IAAYyB,IAAU,OAClB6B,GAA8B,IAAhBrI,EAAEsI,UAAkBnF,EAA6B,IAAhBnD,EAAEsI,UAAkBhO,GAAKiO,YAAc,GAAK/E,EAC/FkD,GAAQ1G,EAAE8F,OAASuC,EAAYrI,EAAEgG,OAASqC,EAAY,GACtDhF,IAAWuB,GAAgBsD,GAAkBC,SAAQ,IAE5C,SAAVK,GAAUxI,OACLyF,GAAazF,QACb2G,EAAI3G,EAAEoH,QACTR,EAAI5G,EAAEqH,QACNxB,EAAKc,EAAIpJ,GAAKoJ,EACdZ,EAAKa,EAAIrJ,GAAKqJ,EACfrJ,GAAKoJ,EAAIA,EACTpJ,GAAKqJ,EAAIA,EACTP,IAAQ,EACRhD,GAAU6E,GAAkBC,SAAQ,IACnCtC,GAAME,IAAOkB,GAAsBpB,EAAIE,IAE9B,SAAX0C,GAAWzI,GAAMzC,GAAKkG,MAAQzD,EAAGwE,EAAQjH,IAC3B,SAAdmL,GAAc1I,GAAMzC,GAAKkG,MAAQzD,EAAGyE,EAAWlH,IACpC,SAAXoL,GAAW3I,UAAKyF,GAAazF,IAAOD,EAAUC,EAAGC,IAAmBiF,EAAQ3H,SA5LxEmB,OAASA,EAASrB,EAAWqB,IAAWlE,QACxCwI,KAAOA,EACDO,EAAXA,GAAoBrJ,GAAKwD,MAAMC,QAAQ4F,GACvCN,EAAYA,GAAa,KACzBC,EAAcA,GAAe,EAC7BM,EAAaA,GAAc,EAC3B2B,EAAcA,GAAe,EAC7BvJ,EAAOA,GAAQ,sBACfwH,GAAwB,IAAbA,EACID,EAAfA,GAA4ByF,WAAWtO,GAAKuO,iBAAiBpO,IAAO0I,aAAe,OAC/EsD,GAAIyB,GAAmB5B,GAASD,GAAOG,GAASD,GAAQW,GAC3D3J,GAAOuL,KACP3C,GAAa,EACbC,GAAa,EACbtK,GAAUkH,EAAKlH,UAAamE,IAAmC,IAAjB+C,EAAKlH,QACnDgM,GAAc9J,EAAeU,EAAQ0D,IACrC2F,GAAc/J,EAAeU,EAAQL,IACrC2J,GAAUF,KACVG,GAAUF,KACVpC,IAAgB/J,EAAKJ,QAAQ,YAAcI,EAAKJ,QAAQ,YAAiC,gBAAnBT,GAAY,GAClFgO,GAAatN,EAAYiD,GACzBsK,GAAWtK,EAAOuK,eAAiB1O,GACnCuL,GAAS,CAAC,EAAG,EAAG,GAChBE,GAAS,CAAC,EAAG,EAAG,GAChBR,GAAc,EAqFd0D,GAAW3L,GAAKsG,QAAU,SAAA7D,GACrByF,GAAazF,EAAG,IAAOA,GAAKA,EAAEmJ,SAClC5L,GAAK2J,KAAOA,GAAO,KACnBgB,GAAkBkB,QAClB7L,GAAKnB,WAAY,EACjB4D,EAAID,EAAUC,GACdmG,GAAaC,GAAa,EAC1B7I,GAAKgK,OAAShK,GAAKoJ,EAAI3G,EAAEoH,QACzB7J,GAAKiK,OAASjK,GAAKqJ,EAAI5G,EAAEqH,QACzB9J,GAAKuJ,IAAIpH,QACTnC,GAAKwJ,IAAIrH,QACT/D,EAAaiJ,EAAelG,EAASsK,GAAUjO,GAAY,GAAIoM,GAASrL,IAAS,GACjFyB,GAAKuI,OAASvI,GAAKyI,OAAS,EAC5BnC,GAAWA,EAAQtG,MAEpB8L,GAAa9L,GAAKuG,UAAY,SAAA9D,OACzByF,GAAazF,EAAG,IACpB/D,EAAgB2I,EAAelG,EAASsK,GAAUjO,GAAY,GAAIoM,IAAS,OACvEmC,GAAkBC,MAAMhM,GAAKqJ,EAAIrJ,GAAKiK,QACzCgC,EAAcjM,GAAK+J,WACnBmC,EAAiBD,IAAiD,EAAjCzM,KAAKuD,IAAI/C,GAAKoJ,EAAIpJ,GAAKgK,SAAgD,EAAjCxK,KAAKuD,IAAI/C,GAAKqJ,EAAIrJ,GAAKiK,SAC9FkC,EAAY3J,EAAUC,IAClByJ,GAAkBH,IACtB/L,GAAKuJ,IAAIpH,QACTnC,GAAKwJ,IAAIrH,QAELO,GAAkBmF,GACrBlL,GAAKyP,YAAY,IAAM,cACS,IAA3BzK,KAAasG,KAAsBxF,EAAE4J,oBACpC5J,EAAEtB,OAAOmL,MACZ7J,EAAEtB,OAAOmL,aACH,GAAIb,GAASc,YAAa,KAC5BC,EAAiBf,GAASc,YAAY,eAC1CC,EAAeC,eAAe,SAAS,GAAM,EAAM1P,GAAM,EAAGoP,EAAUO,QAASP,EAAUQ,QAASR,EAAUtC,QAASsC,EAAUrC,SAAS,GAAO,GAAO,GAAO,EAAO,EAAG,MACvKrH,EAAEtB,OAAOyL,cAAcJ,OAM5BxM,GAAK+J,WAAa/J,GAAKoK,YAAcpK,GAAKnB,WAAY,EACtDiH,GAAUmG,IAAgB5E,GAAgBsD,GAAkBC,SAAQ,GACpE7B,IAAWtH,KACX2E,GAAa6F,GAAe7F,EAAUpG,IACtCuG,GAAaA,EAAUvG,GAAMkM,KAqC/BvB,GAAoB3K,GAAK6M,IAAMlQ,GAAKyP,YAAYrG,GAAe,IAnKjD,SAAb+G,aACC9M,GAAKuJ,IAAIpH,QACTnC,GAAKwJ,IAAIrH,QACTwI,GAAkBkB,QAClB/F,GAAUA,EAAO9F,MA+J8D6L,QAEjF7L,GAAKuI,OAASvI,GAAKyI,OAAS,EAC5BzI,GAAKuJ,IAAMjI,EAAiB,EAAG,IAAI,GACnCtB,GAAKwJ,IAAMlI,EAAiB,EAAG,IAAI,GACnCtB,GAAKyK,QAAUF,GACfvK,GAAK0K,QAAUF,GACfxK,GAAK+J,WAAa/J,GAAKoK,YAAcpK,GAAKnB,WAAY,EACtDpB,GAAS8N,MACTvL,GAAK+M,OAAS,SAAAtK,UACRzC,GAAKgN,YACT5O,EAAaoN,GAAaC,GAAWtK,EAAQ,SAAUvC,GAC7B,GAA1BP,EAAKJ,QAAQ,WAAkBG,EAAaoN,GAAaC,GAAWtK,EAAQ,SAAUmJ,GAAU/L,GAASC,GAChF,GAAzBH,EAAKJ,QAAQ,UAAiBG,EAAa+C,EAAQ,QAAS0J,GAAUtM,GAASC,IACjD,GAAzBH,EAAKJ,QAAQ,UAAiBd,IAAwC,GAA3BkB,EAAKJ,QAAQ,cAC5DG,EAAa+C,EAAQ3D,GAAY,GAAImO,GAAUpN,GAASC,GACxDJ,EAAaqN,GAAUjO,GAAY,GAAIsO,IACvC1N,EAAaqN,GAAUjO,GAAY,GAAIsO,IACvCjE,GAAezJ,EAAa+C,EAAQ,QAAS6G,IAAc,GAAM,GACjEL,GAAWvJ,EAAa+C,EAAQ,QAASiK,IACzC9D,GAAkBlJ,EAAaqN,GAAU,eAAgBvB,IACzD3C,GAAgBnJ,EAAaqN,GAAU,aAAcpB,IACrDpD,GAAW7I,EAAa+C,EAAQ/D,GAAe,QAAS8N,IACxDhE,GAAc9I,EAAa+C,EAAQ/D,GAAe,QAAS+N,IAC3DhE,GAAU/I,EAAa+C,EAAQ/D,GAAe,OAAQ6N,KAEvDjL,GAAKgN,WAAY,EACjBhN,GAAK+J,WAAa/J,GAAKoK,YAAcpK,GAAKnB,UAAYiK,GAAQC,IAAU,EACxE/I,GAAKuJ,IAAIpH,QACTnC,GAAKwJ,IAAIrH,QACTsI,GAAUF,KACVG,GAAUF,KACV/H,GAAKA,EAAEpE,MAAQsN,GAASlJ,GACxBgF,GAAYA,EAASzH,KAEfA,IAERA,GAAKiN,QAAU,WACVjN,GAAKgN,YAERrP,GAAWuP,OAAO,SAAAC,UAAKA,IAAMnN,IAAQ9B,EAAYiP,EAAEhM,UAASD,QAAUxC,EAAgB8M,GAAaC,GAAWtK,EAAQ,SAAUvC,GAC5HoB,GAAKnB,YACRmB,GAAKuJ,IAAIpH,QACTnC,GAAKwJ,IAAIrH,QACTzD,EAAgB2I,EAAelG,EAASsK,GAAUjO,GAAY,GAAIoM,IAAS,IAE5ElL,EAAgB8M,GAAaC,GAAWtK,EAAQ,SAAUmJ,GAAU9L,GACpEE,EAAgByC,EAAQ,QAAS0J,GAAUrM,GAC3CE,EAAgByC,EAAQ3D,GAAY,GAAImO,GAAUnN,GAClDE,EAAgB+M,GAAUjO,GAAY,GAAIsO,IAC1CpN,EAAgB+M,GAAUjO,GAAY,GAAIsO,IAC1CpN,EAAgByC,EAAQ,QAAS6G,IAAc,GAC/CtJ,EAAgByC,EAAQ,QAASiK,IACjC1M,EAAgB+M,GAAU,eAAgBvB,IAC1CxL,EAAgB+M,GAAU,aAAcpB,IACxC3L,EAAgByC,EAAQ/D,GAAe,QAAS8N,IAChDxM,EAAgByC,EAAQ/D,GAAe,QAAS+N,IAChDzM,EAAgByC,EAAQ/D,GAAe,OAAQ6N,IAC/CjL,GAAKgN,UAAYhN,GAAKnB,UAAYmB,GAAK+J,YAAa,EACpDrC,GAAaA,EAAU1H,MAIzBA,GAAKoN,KAAOpN,GAAKqN,OAAS,WACzBrN,GAAKiN,cACDpM,EAAIlD,GAAWM,QAAQ+B,IACtB,GAALa,GAAUlD,GAAW2P,OAAOzM,EAAG,GAC/BtD,KAAgByC,KAASzC,GAAc,IAGxCI,GAAWoD,KAAKf,IAChBqH,GAAgBnJ,EAAYiD,KAAY5D,GAAcyC,IAEtDA,GAAK+M,OAAO7G,8JAILqF,KAAKhC,IAAInH,2DAGTmJ,KAAK/B,IAAIpH,8CAtRLqD,QACND,KAAKC,GA0RZ1B,EAASwJ,QAAU,SACnBxJ,EAASyJ,OAAS,SAAA/H,UAAQ,IAAI1B,EAAS0B,IACvC1B,EAAS0J,SAAWhK,EACpBM,EAAS2J,OAAS,kBAAM/P,GAAWgQ,SACnC5J,EAAS6J,QAAU,SAAA1E,UAAMvL,GAAWuP,OAAO,SAAAC,UAAKA,EAAE1H,KAAKyD,KAAOA,IAAI,IAElExM,KAAcC,GAAKE,eAAekH"} \ No newline at end of file diff --git a/node_modules/gsap/dist/PixiPlugin.js b/node_modules/gsap/dist/PixiPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..777c290309eace17b7ac9415f54b112ef3d23b2d --- /dev/null +++ b/node_modules/gsap/dist/PixiPlugin.js @@ -0,0 +1,472 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + /*! + * PixiPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _splitColor, + _coreInitted, + _PIXI, + PropTween, + _getSetter, + _isV4, + _isV8Plus, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _isFunction = function _isFunction(value) { + return typeof value === "function"; + }, + _warn = function _warn(message) { + return console.warn(message); + }, + _idMatrix = [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + _filterClass = function _filterClass(name) { + return _isFunction(_PIXI[name]) ? _PIXI[name] : _PIXI.filters[name]; + }, + _applyMatrix = function _applyMatrix(m, m2) { + var temp = [], + i = 0, + z = 0, + y, + x; + + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = x === 4 ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i + 1] * m2[x + 5] + m[i + 2] * m2[x + 10] + m[i + 3] * m2[x + 15] + z; + } + + i += 5; + } + + return temp; + }, + _setSaturation = function _setSaturation(m, n) { + var inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); + }, + _colorize = function _colorize(m, color, amount) { + var c = _splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); + }, + _setHue = function _setHue(m, n) { + n *= Math.PI / 180; + var c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([_lumR + c * (1 - _lumR) + s * -_lumR, _lumG + c * -_lumG + s * -_lumG, _lumB + c * -_lumB + s * (1 - _lumB), 0, 0, _lumR + c * -_lumR + s * 0.143, _lumG + c * (1 - _lumG) + s * 0.14, _lumB + c * -_lumB + s * -0.283, 0, 0, _lumR + c * -_lumR + s * -(1 - _lumR), _lumG + c * -_lumG + s * _lumG, _lumB + c * (1 - _lumB) + s * _lumB, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); + }, + _setContrast = function _setContrast(m, n) { + return _applyMatrix([n, 0, 0, 0, 0.5 * (1 - n), 0, n, 0, 0, 0.5 * (1 - n), 0, 0, n, 0, 0.5 * (1 - n), 0, 0, 0, 1, 0], m); + }, + _getFilter = function _getFilter(target, type) { + var filterClass = _filterClass(type), + filters = target.filters || [], + i = filters.length, + filter; + + filterClass || _warn(type + " not found. PixiPlugin.registerPIXI(PIXI)"); + + while (--i > -1) { + if (filters[i] instanceof filterClass) { + return filters[i]; + } + } + + filter = new filterClass(); + + if (type === "BlurFilter") { + filter.blur = 0; + } + + filters.push(filter); + target.filters = filters; + return filter; + }, + _addColorMatrixFilterCacheTween = function _addColorMatrixFilterCacheTween(p, plugin, cache, vars) { + plugin.add(cache, p, cache[p], vars[p]); + + plugin._props.push(p); + }, + _applyBrightnessToMatrix = function _applyBrightnessToMatrix(brightness, matrix) { + var filterClass = _filterClass("ColorMatrixFilter"), + temp = new filterClass(); + + temp.matrix = matrix; + temp.brightness(brightness, true); + return temp.matrix; + }, + _copy = function _copy(obj) { + var copy = {}, + p; + + for (p in obj) { + copy[p] = obj[p]; + } + + return copy; + }, + _CMFdefaults = { + contrast: 1, + saturation: 1, + colorizeAmount: 0, + colorize: "rgb(255,255,255)", + hue: 0, + brightness: 1 + }, + _parseColorMatrixFilter = function _parseColorMatrixFilter(target, v, pg) { + var filter = _getFilter(target, "ColorMatrixFilter"), + cache = target._gsColorMatrixFilter = target._gsColorMatrixFilter || _copy(_CMFdefaults), + combine = v.combineCMF && !("colorMatrixFilter" in v && !v.colorMatrixFilter), + i, + matrix, + startMatrix; + + startMatrix = filter.matrix; + + if (v.resolution) { + filter.resolution = v.resolution; + } + + if (v.matrix && v.matrix.length === startMatrix.length) { + matrix = v.matrix; + + if (cache.contrast !== 1) { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + + if (cache.hue) { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + + if (cache.brightness !== 1) { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + + if (cache.colorizeAmount) { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + + if (cache.saturation !== 1) { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } else { + matrix = _idMatrix.slice(); + + if (v.contrast != null) { + matrix = _setContrast(matrix, +v.contrast); + + _addColorMatrixFilterCacheTween("contrast", pg, cache, v); + } else if (cache.contrast !== 1) { + if (combine) { + matrix = _setContrast(matrix, cache.contrast); + } else { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + } + + if (v.hue != null) { + matrix = _setHue(matrix, +v.hue); + + _addColorMatrixFilterCacheTween("hue", pg, cache, v); + } else if (cache.hue) { + if (combine) { + matrix = _setHue(matrix, cache.hue); + } else { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + } + + if (v.brightness != null) { + matrix = _applyBrightnessToMatrix(+v.brightness, matrix); + + _addColorMatrixFilterCacheTween("brightness", pg, cache, v); + } else if (cache.brightness !== 1) { + if (combine) { + matrix = _applyBrightnessToMatrix(cache.brightness, matrix); + } else { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + } + + if (v.colorize != null) { + v.colorizeAmount = "colorizeAmount" in v ? +v.colorizeAmount : 1; + matrix = _colorize(matrix, v.colorize, v.colorizeAmount); + + _addColorMatrixFilterCacheTween("colorize", pg, cache, v); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, v); + } else if (cache.colorizeAmount) { + if (combine) { + matrix = _colorize(matrix, cache.colorize, cache.colorizeAmount); + } else { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + } + + if (v.saturation != null) { + matrix = _setSaturation(matrix, +v.saturation); + + _addColorMatrixFilterCacheTween("saturation", pg, cache, v); + } else if (cache.saturation !== 1) { + if (combine) { + matrix = _setSaturation(matrix, cache.saturation); + } else { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } + } + + i = matrix.length; + + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg.add(startMatrix, i, startMatrix[i], matrix[i], "colorMatrixFilter"); + } + } + + pg._props.push("colorMatrixFilter"); + }, + _renderColor = function _renderColor(ratio, _ref) { + var t = _ref.t, + p = _ref.p, + color = _ref.color, + set = _ref.set; + set(t, p, color[0] << 16 | color[1] << 8 | color[2]); + }, + _renderDirtyCache = function _renderDirtyCache(ratio, _ref2) { + var g = _ref2.g; + + if (_isV8Plus) { + g.fill(); + g.stroke(); + } else if (g) { + g.dirty++; + g.clearDirty++; + } + }, + _renderAutoAlpha = function _renderAutoAlpha(ratio, data) { + data.t.visible = !!data.t.alpha; + }, + _addColorTween = function _addColorTween(target, p, value, plugin) { + var currentValue = target[p], + startColor = _splitColor(_isFunction(currentValue) ? target[p.indexOf("set") || !_isFunction(target["get" + p.substr(3)]) ? p : "get" + p.substr(3)]() : currentValue), + endColor = _splitColor(value); + + plugin._pt = new PropTween(plugin._pt, target, p, 0, 0, _renderColor, { + t: target, + p: p, + color: startColor, + set: _getSetter(target, p) + }); + plugin.add(startColor, 0, startColor[0], endColor[0]); + plugin.add(startColor, 1, startColor[1], endColor[1]); + plugin.add(startColor, 2, startColor[2], endColor[2]); + }, + _colorProps = { + tint: 1, + lineColor: 1, + fillColor: 1, + strokeColor: 1 + }, + _xyContexts = "position,scale,skew,pivot,anchor,tilePosition,tileScale".split(","), + _contexts = { + x: "position", + y: "position", + tileX: "tilePosition", + tileY: "tilePosition" + }, + _colorMatrixFilterProps = { + colorMatrixFilter: 1, + saturation: 1, + contrast: 1, + hue: 1, + colorize: 1, + colorizeAmount: 1, + brightness: 1, + combineCMF: 1 + }, + _DEG2RAD = Math.PI / 180, + _isString = function _isString(value) { + return typeof value === "string"; + }, + _degreesToRadians = function _degreesToRadians(value) { + return _isString(value) && value.charAt(1) === "=" ? value.substr(0, 2) + parseFloat(value.substr(2)) * _DEG2RAD : value * _DEG2RAD; + }, + _renderPropWithEnd = function _renderPropWithEnd(ratio, data) { + return data.set(data.t, data.p, ratio === 1 ? data.e : Math.round((data.s + data.c * ratio) * 100000) / 100000, data); + }, + _addRotationalPropTween = function _addRotationalPropTween(plugin, target, property, startNum, endValue, radians) { + var cap = 360 * (radians ? _DEG2RAD : 1), + isString = _isString(endValue), + relative = isString && endValue.charAt(1) === "=" ? +(endValue.charAt(0) + "1") : 0, + endNum = parseFloat(relative ? endValue.substr(2) : endValue) * (radians ? _DEG2RAD : 1), + change = relative ? endNum * relative : endNum - startNum, + finalValue = startNum + change, + direction, + pt; + + if (isString) { + direction = endValue.split("_")[1]; + + if (direction === "short") { + change %= cap; + + if (change !== change % (cap / 2)) { + change += change < 0 ? cap : -cap; + } + } + + if (direction === "cw" && change < 0) { + change = (change + cap * 1e10) % cap - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = (change - cap * 1e10) % cap - ~~(change / cap) * cap; + } + } + + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + return pt; + }, + _initCore = function _initCore() { + if (!_coreInitted) { + gsap = _getGSAP(); + _PIXI = _coreInitted = _PIXI || _windowExists() && window.PIXI; + var version = _PIXI && _PIXI.VERSION && parseFloat(_PIXI.VERSION.split(".")[0]) || 0; + _isV4 = version === 4; + _isV8Plus = version >= 8; + + _splitColor = function _splitColor(color) { + return gsap.utils.splitColor((color + "").substr(0, 2) === "0x" ? "#" + color.substr(2) : color); + }; + } + }, + i, + p; + + for (i = 0; i < _xyContexts.length; i++) { + p = _xyContexts[i]; + _contexts[p + "X"] = p; + _contexts[p + "Y"] = p; + } + + var PixiPlugin = { + version: "3.12.7", + name: "pixi", + register: function register(core, Plugin, propTween) { + gsap = core; + PropTween = propTween; + _getSetter = Plugin.getSetter; + + _initCore(); + }, + headless: true, + registerPIXI: function registerPIXI(pixi) { + _PIXI = pixi; + }, + init: function init(target, values, tween, index, targets) { + _PIXI || _initCore(); + + if (!_PIXI) { + _warn("PIXI was not found. PixiPlugin.registerPIXI(PIXI);"); + + return false; + } + + var context, axis, value, colorMatrix, filter, p, padding, i, data, subProp; + + for (p in values) { + context = _contexts[p]; + value = values[p]; + + if (context) { + axis = ~p.charAt(p.length - 1).toLowerCase().indexOf("x") ? "x" : "y"; + this.add(target[context], axis, target[context][axis], context === "skew" ? _degreesToRadians(value) : value, 0, 0, 0, 0, 0, 1); + } else if (p === "scale" || p === "anchor" || p === "pivot" || p === "tileScale") { + this.add(target[p], "x", target[p].x, value); + this.add(target[p], "y", target[p].y, value); + } else if (p === "rotation" || p === "angle") { + _addRotationalPropTween(this, target, p, target[p], value, p === "rotation"); + } else if (_colorMatrixFilterProps[p]) { + if (!colorMatrix) { + _parseColorMatrixFilter(target, values.colorMatrixFilter || values, this); + + colorMatrix = true; + } + } else if (p === "blur" || p === "blurX" || p === "blurY" || p === "blurPadding") { + filter = _getFilter(target, "BlurFilter"); + this.add(filter, p, filter[p], value); + + if (values.blurPadding !== 0) { + padding = values.blurPadding || Math.max(filter[p], value) * 2; + i = target.filters.length; + + while (--i > -1) { + target.filters[i].padding = Math.max(target.filters[i].padding, padding); + } + } + } else if (_colorProps[p]) { + if ((p === "lineColor" || p === "fillColor" || p === "strokeColor") && target instanceof _PIXI.Graphics) { + data = "fillStyle" in target ? [target] : (target.geometry || target).graphicsData; + subProp = p.substr(0, p.length - 5); + _isV8Plus && subProp === "line" && (subProp = "stroke"); + this._pt = new PropTween(this._pt, target, p, 0, 0, _renderDirtyCache, { + g: target.geometry || target + }); + i = data.length; + + while (--i > -1) { + _addColorTween(_isV4 ? data[i] : data[i][subProp + "Style"], _isV4 ? p : "color", value, this); + } + } else { + _addColorTween(target, p, value, this); + } + } else if (p === "autoAlpha") { + this._pt = new PropTween(this._pt, target, "visible", 0, 0, _renderAutoAlpha); + this.add(target, "alpha", target.alpha, value); + + this._props.push("alpha", "visible"); + } else if (p !== "resolution") { + this.add(target, p, "get", value); + } + + this._props.push(p); + } + } + }; + _getGSAP() && gsap.registerPlugin(PixiPlugin); + + exports.PixiPlugin = PixiPlugin; + exports.default = PixiPlugin; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/PixiPlugin.min.js b/node_modules/gsap/dist/PixiPlugin.min.js new file mode 100644 index 0000000000000000000000000000000000000000..d946d9c650e027ee6aad9b633af9c3bd867c486f --- /dev/null +++ b/node_modules/gsap/dist/PixiPlugin.min.js @@ -0,0 +1,11 @@ +/*! + * PixiPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(t,r){"object"==typeof exports&&"undefined"!=typeof module?r(exports):"function"==typeof define&&define.amd?define(["exports"],r):r((t=t||self).window=t.window||{})}(this,function(r){"use strict";function l(){return"undefined"!=typeof window}function m(){return e||l()&&(e=window.gsap)&&e.registerPlugin&&e}function n(t){return"function"==typeof t}function o(t){return console.warn(t)}function t(t){return n(p[t])?p[t]:p.filters[t]}function u(t,r){var i,o,e=[],n=0,s=0;for(i=0;i<4;i++){for(o=0;o<5;o++)s=4===o?t[n+4]:0,e[n+o]=t[n]*r[o]+t[n+1]*r[o+5]+t[n+2]*r[o+10]+t[n+3]*r[o+15]+s;n+=5}return e}function v(t,r){var i=1-r,o=i*M,e=i*_,n=i*C;return u([o+r,e,n,0,0,o,e+r,n,0,0,o,e,n+r,0,0,0,0,0,1,0],t)}function w(t,r,i){var o=a(r),e=o[0]/255,n=o[1]/255,s=o[2]/255,l=1-i;return u([l+i*e*M,i*e*_,i*e*C,0,0,i*n*M,l+i*n*_,i*n*C,0,0,i*s*M,i*s*_,l+i*s*C,0,0,0,0,0,1,0],t)}function x(t,r){r*=Math.PI/180;var i=Math.cos(r),o=Math.sin(r);return u([M+i*(1-M)+o*-M,_+i*-_+o*-_,C+i*-C+o*(1-C),0,0,M+i*-M+.143*o,_+i*(1-_)+.14*o,C+i*-C+-.283*o,0,0,M+i*-M+o*-(1-M),_+i*-_+o*_,C+i*(1-C)+o*C,0,0,0,0,0,1,0,0,0,0,0,1],t)}function y(t,r){return u([r,0,0,0,.5*(1-r),0,r,0,0,.5*(1-r),0,0,r,0,.5*(1-r),0,0,0,1,0],t)}function z(r,i){var e,n=t(i),s=r.filters||[],l=s.length;for(n||o(i+" not found. PixiPlugin.registerPIXI(PIXI)");-1<--l;)if(s[l]instanceof n)return s[l];return e=new n,"BlurFilter"===i&&(e.blur=0),s.push(e),r.filters=s,e}function A(t,r,i,o){r.add(i,t,i[t],o[t]),r._props.push(t)}function B(r,i){var o=new(t("ColorMatrixFilter"));return o.matrix=i,o.brightness(r,!0),o.matrix}function E(t,r,i){var o,e,n,s=z(t,"ColorMatrixFilter"),l=t._gsColorMatrixFilter=t._gsColorMatrixFilter||function _copy(t){var r,i={};for(r in t)i[r]=t[r];return i}(X),u=r.combineCMF&&!("colorMatrixFilter"in r&&!r.colorMatrixFilter);n=s.matrix,r.resolution&&(s.resolution=r.resolution),r.matrix&&r.matrix.length===n.length?(e=r.matrix,1!==l.contrast&&A("contrast",i,l,X),l.hue&&A("hue",i,l,X),1!==l.brightness&&A("brightness",i,l,X),l.colorizeAmount&&(A("colorize",i,l,X),A("colorizeAmount",i,l,X)),1!==l.saturation&&A("saturation",i,l,X)):(e=h.slice(),null!=r.contrast?(e=y(e,+r.contrast),A("contrast",i,l,r)):1!==l.contrast&&(u?e=y(e,l.contrast):A("contrast",i,l,X)),null!=r.hue?(e=x(e,+r.hue),A("hue",i,l,r)):l.hue&&(u?e=x(e,l.hue):A("hue",i,l,X)),null!=r.brightness?(e=B(+r.brightness,e),A("brightness",i,l,r)):1!==l.brightness&&(u?e=B(l.brightness,e):A("brightness",i,l,X)),null!=r.colorize?(r.colorizeAmount="colorizeAmount"in r?+r.colorizeAmount:1,e=w(e,r.colorize,r.colorizeAmount),A("colorize",i,l,r),A("colorizeAmount",i,l,r)):l.colorizeAmount&&(u?e=w(e,l.colorize,l.colorizeAmount):(A("colorize",i,l,X),A("colorizeAmount",i,l,X))),null!=r.saturation?(e=v(e,+r.saturation),A("saturation",i,l,r)):1!==l.saturation&&(u?e=v(e,l.saturation):A("saturation",i,l,X))),o=e.length;for(;-1<--o;)e[o]!==n[o]&&i.add(n,o,n[o],e[o],"colorMatrixFilter");i._props.push("colorMatrixFilter")}function F(t,r){var i=r.t,o=r.p,e=r.color;(0,r.set)(i,o,e[0]<<16|e[1]<<8|e[2])}function G(t,r){var i=r.g;b?(i.fill(),i.stroke()):i&&(i.dirty++,i.clearDirty++)}function H(t,r){r.t.visible=!!r.t.alpha}function I(t,r,i,o){var e=t[r],s=a(n(e)?t[r.indexOf("set")||!n(t["get"+r.substr(3)])?r:"get"+r.substr(3)]():e),l=a(i);o._pt=new d(o._pt,t,r,0,0,F,{t:t,p:r,color:s,set:c(t,r)}),o.add(s,0,s[0],l[0]),o.add(s,1,s[1],l[1]),o.add(s,2,s[2],l[2])}function O(t){return"string"==typeof t}function P(t){return O(t)&&"="===t.charAt(1)?t.substr(0,2)+parseFloat(t.substr(2))*N:t*N}function Q(t,r){return r.set(r.t,r.p,1===t?r.e:Math.round(1e5*(r.s+r.c*t))/1e5,r)}function R(t,r,i,o,e,n){var s,l,u=360*(n?N:1),a=O(e),c=a&&"="===e.charAt(1)?+(e.charAt(0)+"1"):0,f=parseFloat(c?e.substr(2):e)*(n?N:1),h=c?f*c:f-o,p=o+h;return a&&("short"===(s=e.split("_")[1])&&(h%=u)!==h%(u/2)&&(h+=h<0?u:-u),"cw"===s&&h<0?h=(h+1e10*u)%u-~~(h/u)*u:"ccw"===s&&0<h&&(h=(h-1e10*u)%u-~~(h/u)*u)),t._pt=l=new d(t._pt,r,i,o,h,Q),l.e=p,l}function S(){if(!i){e=m();var t=(p=i=p||l()&&window.PIXI)&&p.VERSION&&parseFloat(p.VERSION.split(".")[0])||0;g=4===t,b=8<=t,a=function _splitColor(t){return e.utils.splitColor("0x"===(t+"").substr(0,2)?"#"+t.substr(2):t)}}}var e,a,i,p,d,c,g,b,s,f,h=[1,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,0,1,0],M=.212671,_=.71516,C=.072169,X={contrast:1,saturation:1,colorizeAmount:0,colorize:"rgb(255,255,255)",hue:0,brightness:1},k={tint:1,lineColor:1,fillColor:1,strokeColor:1},Y="position,scale,skew,pivot,anchor,tilePosition,tileScale".split(","),j={x:"position",y:"position",tileX:"tilePosition",tileY:"tilePosition"},D={colorMatrixFilter:1,saturation:1,contrast:1,hue:1,colorize:1,colorizeAmount:1,brightness:1,combineCMF:1},N=Math.PI/180;for(s=0;s<Y.length;s++)f=Y[s],j[f+"X"]=f,j[f+"Y"]=f;var V={version:"3.12.7",name:"pixi",register:function register(t,r,i){e=t,d=i,c=r.getSetter,S()},headless:!0,registerPIXI:function registerPIXI(t){p=t},init:function init(t,r){if(p||S(),!p)return o("PIXI was not found. PixiPlugin.registerPIXI(PIXI);"),!1;var i,e,n,s,l,u,a,c,f,h;for(u in r){if(i=j[u],n=r[u],i)e=~u.charAt(u.length-1).toLowerCase().indexOf("x")?"x":"y",this.add(t[i],e,t[i][e],"skew"===i?P(n):n,0,0,0,0,0,1);else if("scale"===u||"anchor"===u||"pivot"===u||"tileScale"===u)this.add(t[u],"x",t[u].x,n),this.add(t[u],"y",t[u].y,n);else if("rotation"===u||"angle"===u)R(this,t,u,t[u],n,"rotation"===u);else if(D[u])s||(E(t,r.colorMatrixFilter||r,this),s=!0);else if("blur"===u||"blurX"===u||"blurY"===u||"blurPadding"===u){if(l=z(t,"BlurFilter"),this.add(l,u,l[u],n),0!==r.blurPadding)for(a=r.blurPadding||2*Math.max(l[u],n),c=t.filters.length;-1<--c;)t.filters[c].padding=Math.max(t.filters[c].padding,a)}else if(k[u])if(("lineColor"===u||"fillColor"===u||"strokeColor"===u)&&t instanceof p.Graphics){f="fillStyle"in t?[t]:(t.geometry||t).graphicsData,h=u.substr(0,u.length-5),b&&"line"===h&&(h="stroke"),this._pt=new d(this._pt,t,u,0,0,G,{g:t.geometry||t}),c=f.length;for(;-1<--c;)I(g?f[c]:f[c][h+"Style"],g?u:"color",n,this)}else I(t,u,n,this);else"autoAlpha"===u?(this._pt=new d(this._pt,t,"visible",0,0,H),this.add(t,"alpha",t.alpha,n),this._props.push("alpha","visible")):"resolution"!==u&&this.add(t,u,"get",n);this._props.push(u)}}};m()&&e.registerPlugin(V),r.PixiPlugin=V,r.default=V;if (typeof(window)==="undefined"||window!==r){Object.defineProperty(r,"__esModule",{value:!0})} else {delete r.default}}); + diff --git a/node_modules/gsap/dist/PixiPlugin.min.js.map b/node_modules/gsap/dist/PixiPlugin.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..a48f74d1c17690c801417d39912fe7ff345c9259 --- /dev/null +++ b/node_modules/gsap/dist/PixiPlugin.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"PixiPlugin.min.js","sources":["../src/PixiPlugin.js"],"sourcesContent":["/*!\n * PixiPlugin 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _splitColor, _coreInitted, _PIXI, PropTween, _getSetter, _isV4, _isV8Plus,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_isFunction = value => typeof(value) === \"function\",\n\t_warn = message => console.warn(message),\n\t_idMatrix = [1,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,0,1,0],\n\t_lumR = 0.212671,\n\t_lumG = 0.715160,\n\t_lumB = 0.072169,\n\t_filterClass = name => _isFunction(_PIXI[name]) ? _PIXI[name] : _PIXI.filters[name], // in PIXI 7.1, filters moved from PIXI.filters to just PIXI\n\t_applyMatrix = (m, m2) => {\n\t\tlet temp = [],\n\t\t\ti = 0,\n\t\t\tz = 0,\n\t\t\ty, x;\n\t\tfor (y = 0; y < 4; y++) {\n\t\t\tfor (x = 0; x < 5; x++) {\n\t\t\t\tz = (x === 4) ? m[i + 4] : 0;\n\t\t\t\ttemp[i + x] = m[i] * m2[x] + m[i+1] * m2[x + 5] +\tm[i+2] * m2[x + 10] + m[i+3] * m2[x + 15] +\tz;\n\t\t\t}\n\t\t\ti += 5;\n\t\t}\n\t\treturn temp;\n\t},\n\t_setSaturation = (m, n) => {\n\t\tlet inv = 1 - n,\n\t\t\tr = inv * _lumR,\n\t\t\tg = inv * _lumG,\n\t\t\tb = inv * _lumB;\n\t\treturn _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m);\n\t},\n\t_colorize = (m, color, amount) => {\n\t\tlet c = _splitColor(color),\n\t\t\tr = c[0] / 255,\n\t\t\tg = c[1] / 255,\n\t\t\tb = c[2] / 255,\n\t\t\tinv = 1 - amount;\n\t\treturn _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m);\n\t},\n\t_setHue = (m, n) => {\n\t\tn *= Math.PI / 180;\n\t\tlet c = Math.cos(n),\n\t\t\ts = Math.sin(n);\n\t\treturn _applyMatrix([(_lumR + (c * (1 - _lumR))) + (s * (-_lumR)), (_lumG + (c * (-_lumG))) + (s * (-_lumG)), (_lumB + (c * (-_lumB))) + (s * (1 - _lumB)), 0, 0, (_lumR + (c * (-_lumR))) + (s * 0.143), (_lumG + (c * (1 - _lumG))) + (s * 0.14), (_lumB + (c * (-_lumB))) + (s * -0.283), 0, 0, (_lumR + (c * (-_lumR))) + (s * (-(1 - _lumR))), (_lumG + (c * (-_lumG))) + (s * _lumG), (_lumB + (c * (1 - _lumB))) + (s * _lumB), 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m);\n\t},\n\t_setContrast = (m, n) => _applyMatrix([n,0,0,0,0.5 * (1 - n), 0,n,0,0,0.5 * (1 - n), 0,0,n,0,0.5 * (1 - n), 0,0,0,1,0], m),\n\t_getFilter = (target, type) => {\n\t\tlet filterClass = _filterClass(type),\n\t\t\tfilters = target.filters || [],\n\t\t\ti = filters.length,\n\t\t\tfilter;\n\t\tfilterClass || _warn(type + \" not found. PixiPlugin.registerPIXI(PIXI)\");\n\t\twhile (--i > -1) {\n\t\t\tif (filters[i] instanceof filterClass) {\n\t\t\t\treturn filters[i];\n\t\t\t}\n\t\t}\n\t\tfilter = new filterClass();\n\t\tif (type === \"BlurFilter\") {\n\t\t\tfilter.blur = 0;\n\t\t}\n\t\tfilters.push(filter);\n\t\ttarget.filters = filters;\n\t\treturn filter;\n\t},\n\t_addColorMatrixFilterCacheTween = (p, plugin, cache, vars) => { //we cache the ColorMatrixFilter components in a _gsColorMatrixFilter object attached to the target object so that it's easy to grab the current value at any time.\n\t\tplugin.add(cache, p, cache[p], vars[p]);\n\t\tplugin._props.push(p);\n\t},\n\t_applyBrightnessToMatrix = (brightness, matrix) => {\n\t\tlet filterClass = _filterClass(\"ColorMatrixFilter\"),\n\t\t\ttemp = new filterClass();\n\t\ttemp.matrix = matrix;\n\t\ttemp.brightness(brightness, true);\n\t\treturn temp.matrix;\n\t},\n\t_copy = obj => {\n\t\tlet copy = {},\n\t\t\tp;\n\t\tfor (p in obj) {\n\t\t\tcopy[p] = obj[p];\n\t\t}\n\t\treturn copy;\n\t},\n\t_CMFdefaults = {contrast:1, saturation:1, colorizeAmount:0, colorize:\"rgb(255,255,255)\", hue:0, brightness:1},\n\t_parseColorMatrixFilter = (target, v, pg) => {\n\t\tlet filter = _getFilter(target, \"ColorMatrixFilter\"),\n\t\t\tcache = target._gsColorMatrixFilter = target._gsColorMatrixFilter || _copy(_CMFdefaults),\n\t\t\tcombine = v.combineCMF && !(\"colorMatrixFilter\" in v && !v.colorMatrixFilter),\n\t\t\ti, matrix, startMatrix;\n\t\tstartMatrix = filter.matrix;\n\t\tif (v.resolution) {\n\t\t\tfilter.resolution = v.resolution;\n\t\t}\n\t\tif (v.matrix && v.matrix.length === startMatrix.length) {\n\t\t\tmatrix = v.matrix;\n\t\t\tif (cache.contrast !== 1) {\n\t\t\t\t_addColorMatrixFilterCacheTween(\"contrast\", pg, cache, _CMFdefaults);\n\t\t\t}\n\t\t\tif (cache.hue) {\n\t\t\t\t_addColorMatrixFilterCacheTween(\"hue\", pg, cache, _CMFdefaults);\n\t\t\t}\n\t\t\tif (cache.brightness !== 1) {\n\t\t\t\t_addColorMatrixFilterCacheTween(\"brightness\", pg, cache, _CMFdefaults);\n\t\t\t}\n\t\t\tif (cache.colorizeAmount) {\n\t\t\t\t_addColorMatrixFilterCacheTween(\"colorize\", pg, cache, _CMFdefaults);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"colorizeAmount\", pg, cache, _CMFdefaults);\n\t\t\t}\n\t\t\tif (cache.saturation !== 1) {\n\t\t\t\t_addColorMatrixFilterCacheTween(\"saturation\", pg, cache, _CMFdefaults);\n\t\t\t}\n\n\t\t} else {\n\t\t\tmatrix = _idMatrix.slice();\n\t\t\tif (v.contrast != null) {\n\t\t\t\tmatrix = _setContrast(matrix, +v.contrast);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"contrast\", pg, cache, v);\n\t\t\t} else if (cache.contrast !== 1) {\n\t\t\t\tif (combine) {\n\t\t\t\t\tmatrix = _setContrast(matrix, cache.contrast);\n\t\t\t\t} else {\n\t\t\t\t\t_addColorMatrixFilterCacheTween(\"contrast\", pg, cache, _CMFdefaults);\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (v.hue != null) {\n\t\t\t\tmatrix = _setHue(matrix, +v.hue);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"hue\", pg, cache, v);\n\t\t\t} else if (cache.hue) {\n\t\t\t\tif (combine) {\n\t\t\t\t\tmatrix = _setHue(matrix, cache.hue);\n\t\t\t\t} else {\n\t\t\t\t\t_addColorMatrixFilterCacheTween(\"hue\", pg, cache, _CMFdefaults);\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (v.brightness != null) {\n\t\t\t\tmatrix = _applyBrightnessToMatrix(+v.brightness, matrix);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"brightness\", pg, cache, v);\n\t\t\t} else if (cache.brightness !== 1) {\n\t\t\t\tif (combine) {\n\t\t\t\t\tmatrix = _applyBrightnessToMatrix(cache.brightness, matrix);\n\t\t\t\t} else {\n\t\t\t\t\t_addColorMatrixFilterCacheTween(\"brightness\", pg, cache, _CMFdefaults);\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (v.colorize != null) {\n\t\t\t\tv.colorizeAmount = (\"colorizeAmount\" in v) ? +v.colorizeAmount : 1;\n\t\t\t\tmatrix = _colorize(matrix, v.colorize, v.colorizeAmount);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"colorize\", pg, cache, v);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"colorizeAmount\", pg, cache, v);\n\t\t\t} else if (cache.colorizeAmount) {\n\t\t\t\tif (combine) {\n\t\t\t\t\tmatrix = _colorize(matrix, cache.colorize, cache.colorizeAmount);\n\t\t\t\t} else {\n\t\t\t\t\t_addColorMatrixFilterCacheTween(\"colorize\", pg, cache, _CMFdefaults);\n\t\t\t\t\t_addColorMatrixFilterCacheTween(\"colorizeAmount\", pg, cache, _CMFdefaults);\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (v.saturation != null) {\n\t\t\t\tmatrix = _setSaturation(matrix, +v.saturation);\n\t\t\t\t_addColorMatrixFilterCacheTween(\"saturation\", pg, cache, v);\n\t\t\t} else if (cache.saturation !== 1) {\n\t\t\t\tif (combine) {\n\t\t\t\t\tmatrix = _setSaturation(matrix, cache.saturation);\n\t\t\t\t} else {\n\t\t\t\t\t_addColorMatrixFilterCacheTween(\"saturation\", pg, cache, _CMFdefaults);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\ti = matrix.length;\n\t\twhile (--i > -1) {\n\t\t\tif (matrix[i] !== startMatrix[i]) {\n\t\t\t\tpg.add(startMatrix, i, startMatrix[i], matrix[i], \"colorMatrixFilter\");\n\t\t\t}\n\t\t}\n\t\tpg._props.push(\"colorMatrixFilter\");\n\t},\n\t_renderColor = (ratio, {t, p, color, set}) => {\n\t\tset(t, p, color[0] << 16 | color[1] << 8 | color[2]);\n\t},\n\t_renderDirtyCache = (ratio, {g}) => {\n\t\tif (_isV8Plus) {\n\t\t\tg.fill();\n\t\t\tg.stroke();\n\t\t} else if (g) { // in order for PixiJS to actually redraw GraphicsData, we've gotta increment the \"dirty\" and \"clearDirty\" values. If we don't do this, the values will be tween properly, but not rendered.\n\t\t\tg.dirty++;\n\t\t\tg.clearDirty++;\n\t\t}\n\t},\n\t_renderAutoAlpha = (ratio, data) => {\n\t\tdata.t.visible = !!data.t.alpha;\n\t},\n\t_addColorTween = (target, p, value, plugin) => {\n\t\tlet currentValue = target[p],\n\t\t\tstartColor = _splitColor(_isFunction(currentValue) ? target[ ((p.indexOf(\"set\") || !_isFunction(target[\"get\" + p.substr(3)])) ? p : \"get\" + p.substr(3)) ]() : currentValue),\n\t\t\tendColor = _splitColor(value);\n\t\tplugin._pt = new PropTween(plugin._pt, target, p, 0, 0, _renderColor, {t:target, p:p, color:startColor, set:_getSetter(target, p)});\n\t\tplugin.add(startColor, 0, startColor[0], endColor[0]);\n\t\tplugin.add(startColor, 1, startColor[1], endColor[1]);\n\t\tplugin.add(startColor, 2, startColor[2], endColor[2]);\n\t},\n\n\t_colorProps = {tint:1, lineColor:1, fillColor:1, strokeColor:1},\n\t_xyContexts = \"position,scale,skew,pivot,anchor,tilePosition,tileScale\".split(\",\"),\n\t_contexts = {x:\"position\", y:\"position\", tileX:\"tilePosition\", tileY:\"tilePosition\"},\n\t_colorMatrixFilterProps = {colorMatrixFilter:1, saturation:1, contrast:1, hue:1, colorize:1, colorizeAmount:1, brightness:1, combineCMF:1},\n\t_DEG2RAD = Math.PI / 180,\n\t_isString = value => typeof(value) === \"string\",\n\t_degreesToRadians = value => (_isString(value) && value.charAt(1) === \"=\") ? value.substr(0, 2) + (parseFloat(value.substr(2)) * _DEG2RAD) : value * _DEG2RAD,\n\t_renderPropWithEnd = (ratio, data) => data.set(data.t, data.p, ratio === 1 ? data.e : (Math.round((data.s + data.c * ratio) * 100000) / 100000), data),\n\t_addRotationalPropTween = (plugin, target, property, startNum, endValue, radians) => {\n\t\tlet cap = 360 * (radians ? _DEG2RAD : 1),\n\t\t\tisString = _isString(endValue),\n\t\t\trelative = (isString && endValue.charAt(1) === \"=\") ? +(endValue.charAt(0) + \"1\") : 0,\n\t\t\tendNum = parseFloat(relative ? endValue.substr(2) : endValue) * (radians ? _DEG2RAD : 1),\n\t\t\tchange = relative ? endNum * relative : endNum - startNum,\n\t\t\tfinalValue = startNum + change,\n\t\t\tdirection, pt;\n\t\tif (isString) {\n\t\t\tdirection = endValue.split(\"_\")[1];\n\t\t\tif (direction === \"short\") {\n\t\t\t\tchange %= cap;\n\t\t\t\tif (change !== change % (cap / 2)) {\n\t\t\t\t\tchange += (change < 0) ? cap : -cap;\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (direction === \"cw\" && change < 0) {\n\t\t\t\tchange = ((change + cap * 1e10) % cap) - ~~(change / cap) * cap;\n\t\t\t} else if (direction === \"ccw\" && change > 0) {\n\t\t\t\tchange = ((change - cap * 1e10) % cap) - ~~(change / cap) * cap;\n\t\t\t}\n\t\t}\n\t\tplugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd);\n\t\tpt.e = finalValue;\n\t\treturn pt;\n\t},\n\t_initCore = () => {\n\t\tif (!_coreInitted) {\n\t\t\tgsap = _getGSAP();\n\t\t\t_PIXI = _coreInitted = _PIXI || (_windowExists() && window.PIXI);\n\t\t\tlet version = (_PIXI && _PIXI.VERSION && parseFloat(_PIXI.VERSION.split(\".\")[0])) || 0;\n\t\t\t_isV4 = version === 4;\n\t\t\t_isV8Plus = version >= 8;\n\t\t\t_splitColor = color => gsap.utils.splitColor((color + \"\").substr(0,2) === \"0x\" ? \"#\" + color.substr(2) : color); // some colors in PIXI are reported as \"0xFF4421\" instead of \"#FF4421\".\n\t\t}\n\t}, i, p;\n\n//context setup...\nfor (i = 0; i < _xyContexts.length; i++) {\n\tp = _xyContexts[i];\n\t_contexts[p + \"X\"] = p;\n\t_contexts[p + \"Y\"] = p;\n}\n\n\nexport const PixiPlugin = {\n\tversion: \"3.12.7\",\n\tname: \"pixi\",\n\tregister(core, Plugin, propTween) {\n\t\tgsap = core;\n\t\tPropTween = propTween;\n\t\t_getSetter = Plugin.getSetter;\n\t\t_initCore();\n\t},\n\theadless: true, // doesn't need window\n\tregisterPIXI(pixi) {\n\t\t_PIXI = pixi;\n\t},\n\tinit(target, values, tween, index, targets) {\n\t\t_PIXI || _initCore();\n\t\tif (!_PIXI) {\n\t\t\t_warn(\"PIXI was not found. PixiPlugin.registerPIXI(PIXI);\");\n\t\t\treturn false;\n\t\t}\n\t\tlet context, axis, value, colorMatrix, filter, p, padding, i, data, subProp;\n\t\tfor (p in values) {\n\t\t\tcontext = _contexts[p];\n\t\t\tvalue = values[p];\n\t\t\tif (context) {\n\t\t\t\taxis = ~p.charAt(p.length-1).toLowerCase().indexOf(\"x\") ? \"x\" : \"y\";\n\t\t\t\tthis.add(target[context], axis, target[context][axis], (context === \"skew\") ? _degreesToRadians(value) : value, 0, 0, 0, 0, 0, 1);\n\t\t\t} else if (p === \"scale\" || p === \"anchor\" || p === \"pivot\" || p === \"tileScale\") {\n\t\t\t\tthis.add(target[p], \"x\", target[p].x, value);\n\t\t\t\tthis.add(target[p], \"y\", target[p].y, value);\n\t\t\t} else if (p === \"rotation\" || p === \"angle\") { //PIXI expects rotation in radians, but as a convenience we let folks define it in degrees and we do the conversion.\n\t\t\t\t_addRotationalPropTween(this, target, p, target[p], value, p === \"rotation\");\n\t\t\t} else if (_colorMatrixFilterProps[p]) {\n\t\t\t\tif (!colorMatrix) {\n\t\t\t\t\t_parseColorMatrixFilter(target, values.colorMatrixFilter || values, this);\n\t\t\t\t\tcolorMatrix = true;\n\t\t\t\t}\n\t\t\t} else if (p === \"blur\" || p === \"blurX\" || p === \"blurY\" || p === \"blurPadding\") {\n\t\t\t\tfilter = _getFilter(target, \"BlurFilter\");\n\t\t\t\tthis.add(filter, p, filter[p], value);\n\t\t\t\tif (values.blurPadding !== 0) {\n\t\t\t\t\tpadding = values.blurPadding || Math.max(filter[p], value) * 2;\n\t\t\t\t\ti = target.filters.length;\n\t\t\t\t\twhile (--i > -1) {\n\t\t\t\t\t\ttarget.filters[i].padding = Math.max(target.filters[i].padding, padding); //if we don't expand the padding on all the filters, it can look clipped.\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t} else if (_colorProps[p]) {\n\t\t\t\tif ((p === \"lineColor\" || p === \"fillColor\" || p === \"strokeColor\") && target instanceof _PIXI.Graphics) {\n\t\t\t\t\tdata = \"fillStyle\" in target ? [target] : (target.geometry || target).graphicsData; //\"geometry\" was introduced in PIXI version 5\n\t\t\t\t\tsubProp = p.substr(0, p.length - 5);\n\t\t\t\t\t_isV8Plus && subProp === \"line\" && (subProp = \"stroke\"); // in v8, lineColor became strokeColor.\n\t\t\t\t\tthis._pt = new PropTween(this._pt, target, p, 0, 0, _renderDirtyCache, {g: target.geometry || target});\n\t\t\t\t\ti = data.length;\n\t\t\t\t\twhile (--i > -1) {\n\t\t\t\t\t\t_addColorTween(_isV4 ? data[i] : data[i][subProp + \"Style\"], _isV4 ? p : \"color\", value, this);\n\t\t\t\t\t}\n\t\t\t\t} else {\n\t\t\t\t\t_addColorTween(target, p, value, this);\n\t\t\t\t}\n\t\t\t} else if (p === \"autoAlpha\") {\n\t\t\t\tthis._pt = new PropTween(this._pt, target, \"visible\", 0, 0, _renderAutoAlpha);\n\t\t\t\tthis.add(target, \"alpha\", target.alpha, value);\n\t\t\t\tthis._props.push(\"alpha\", \"visible\");\n\t\t\t} else if (p !== \"resolution\") {\n\t\t\t\tthis.add(target, p, \"get\", value);\n\t\t\t}\n\t\t\tthis._props.push(p);\n\t\t}\n\t}\n};\n\n_getGSAP() && gsap.registerPlugin(PixiPlugin);\n\nexport { PixiPlugin as default };"],"names":["_windowExists","window","_getGSAP","gsap","registerPlugin","_isFunction","value","_warn","message","console","warn","_filterClass","name","_PIXI","filters","_applyMatrix","m","m2","y","x","temp","i","z","_setSaturation","n","inv","r","_lumR","g","_lumG","b","_lumB","_colorize","color","amount","c","_splitColor","_setHue","Math","PI","cos","s","sin","_setContrast","_getFilter","target","type","filter","filterClass","length","blur","push","_addColorMatrixFilterCacheTween","p","plugin","cache","vars","add","_props","_applyBrightnessToMatrix","brightness","matrix","_parseColorMatrixFilter","v","pg","startMatrix","_gsColorMatrixFilter","_copy","obj","copy","_CMFdefaults","combine","combineCMF","colorMatrixFilter","resolution","contrast","hue","colorizeAmount","saturation","_idMatrix","slice","colorize","_renderColor","ratio","t","set","_renderDirtyCache","_isV8Plus","fill","stroke","dirty","clearDirty","_renderAutoAlpha","data","visible","alpha","_addColorTween","currentValue","startColor","indexOf","substr","endColor","_pt","PropTween","_getSetter","_isString","_degreesToRadians","charAt","parseFloat","_DEG2RAD","_renderPropWithEnd","e","round","_addRotationalPropTween","property","startNum","endValue","radians","direction","pt","cap","isString","relative","endNum","change","finalValue","split","_initCore","_coreInitted","version","PIXI","VERSION","_isV4","utils","splitColor","_colorProps","tint","lineColor","fillColor","strokeColor","_xyContexts","_contexts","tileX","tileY","_colorMatrixFilterProps","PixiPlugin","register","core","Plugin","propTween","getSetter","headless","registerPIXI","pixi","init","values","context","axis","colorMatrix","padding","subProp","toLowerCase","this","blurPadding","max","Graphics","geometry","graphicsData"],"mappings":";;;;;;;;;6MAYiB,SAAhBA,UAAyC,oBAAZC,OAClB,SAAXC,WAAiBC,GAASH,MAAoBG,EAAOF,OAAOE,OAASA,EAAKC,gBAAkBD,EAC9E,SAAdE,EAAcC,SAA2B,mBAAXA,EACtB,SAARC,EAAQC,UAAWC,QAAQC,KAAKF,GAKjB,SAAfG,EAAeC,UAAQP,EAAYQ,EAAMD,IAASC,EAAMD,GAAQC,EAAMC,QAAQF,GAC/D,SAAfG,EAAgBC,EAAGC,OAIjBC,EAAGC,EAHAC,EAAO,GACVC,EAAI,EACJC,EAAI,MAEAJ,EAAI,EAAGA,EAAI,EAAGA,IAAK,KAClBC,EAAI,EAAGA,EAAI,EAAGA,IAClBG,EAAW,IAANH,EAAWH,EAAEK,EAAI,GAAK,EAC3BD,EAAKC,EAAIF,GAAKH,EAAEK,GAAOJ,EAAGE,GAAKH,EAAEK,EAAE,GAAKJ,EAAGE,EAAI,GAAKH,EAAEK,EAAE,GAAKJ,EAAGE,EAAI,IAAMH,EAAEK,EAAE,GAAKJ,EAAGE,EAAI,IAAMG,EAEjGD,GAAK,SAECD,EAES,SAAjBG,EAAkBP,EAAGQ,OAChBC,EAAM,EAAID,EACbE,EAAID,EAAME,EACVC,EAAIH,EAAMI,EACVC,EAAIL,EAAMM,SACJhB,EAAa,CAACW,EAAIF,EAAGI,EAAGE,EAAG,EAAG,EAAGJ,EAAGE,EAAIJ,EAAGM,EAAG,EAAG,EAAGJ,EAAGE,EAAGE,EAAIN,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GAAIR,GAEnF,SAAZgB,EAAahB,EAAGiB,EAAOC,OAClBC,EAAIC,EAAYH,GACnBP,EAAIS,EAAE,GAAK,IACXP,EAAIO,EAAE,GAAK,IACXL,EAAIK,EAAE,GAAK,IACXV,EAAM,EAAIS,SACJnB,EAAa,CAACU,EAAMS,EAASR,EAAIC,EAAOO,EAASR,EAAIG,EAAOK,EAASR,EAAIK,EAAO,EAAG,EAAGG,EAASN,EAAID,EAAOF,EAAMS,EAASN,EAAIC,EAAOK,EAASN,EAAIG,EAAO,EAAG,EAAGG,EAASJ,EAAIH,EAAOO,EAASJ,EAAID,EAAOJ,EAAMS,EAASJ,EAAIC,EAAO,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GAAIf,GAEpP,SAAVqB,EAAWrB,EAAGQ,GACbA,GAAKc,KAAKC,GAAK,QACXJ,EAAIG,KAAKE,IAAIhB,GAChBiB,EAAIH,KAAKI,IAAIlB,UACPT,EAAa,CAAEY,EAASQ,GAAK,EAAIR,GAAYc,GAAMd,EAAUE,EAASM,GAAMN,EAAYY,GAAMZ,EAAUE,EAASI,GAAMJ,EAAYU,GAAK,EAAIV,GAAS,EAAG,EAAIJ,EAASQ,GAAMR,EAAgB,KAAJc,EAAaZ,EAASM,GAAK,EAAIN,GAAgB,IAAJY,EAAYV,EAASI,GAAMJ,GAAiB,KAALU,EAAa,EAAG,EAAId,EAASQ,GAAMR,EAAYc,IAAO,EAAId,GAAWE,EAASM,GAAMN,EAAYY,EAAIZ,EAASE,EAASI,GAAK,EAAIJ,GAAYU,EAAIV,EAAQ,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,EAAG,GAAIf,GAE9b,SAAf2B,EAAgB3B,EAAGQ,UAAMT,EAAa,CAACS,EAAE,EAAE,EAAE,EAAE,IAAO,EAAIA,GAAI,EAAEA,EAAE,EAAE,EAAE,IAAO,EAAIA,GAAI,EAAE,EAAEA,EAAE,EAAE,IAAO,EAAIA,GAAI,EAAE,EAAE,EAAE,EAAE,GAAIR,GAC3G,SAAb4B,EAAcC,EAAQC,OAIpBC,EAHGC,EAAcrC,EAAamC,GAC9BhC,EAAU+B,EAAO/B,SAAW,GAC5BO,EAAIP,EAAQmC,WAEbD,GAAezC,EAAMuC,EAAO,8CACd,IAALzB,MACJP,EAAQO,aAAc2B,SAClBlC,EAAQO,UAGjB0B,EAAS,IAAIC,EACA,eAATF,IACHC,EAAOG,KAAO,GAEfpC,EAAQqC,KAAKJ,GACbF,EAAO/B,QAAUA,EACViC,EAE0B,SAAlCK,EAAmCC,EAAGC,EAAQC,EAAOC,GACpDF,EAAOG,IAAIF,EAAOF,EAAGE,EAAMF,GAAIG,EAAKH,IACpCC,EAAOI,OAAOP,KAAKE,GAEO,SAA3BM,EAA4BC,EAAYC,OAEtCzC,EAAO,IADUT,EAAa,6BAE/BS,EAAKyC,OAASA,EACdzC,EAAKwC,WAAWA,GAAY,GACrBxC,EAAKyC,OAWa,SAA1BC,EAA2BjB,EAAQkB,EAAGC,OAIpC3C,EAAGwC,EAAQI,EAHRlB,EAASH,EAAWC,EAAQ,qBAC/BU,EAAQV,EAAOqB,qBAAuBrB,EAAOqB,sBAXvC,SAARC,MAAQC,OAENf,EADGgB,EAAO,OAENhB,KAAKe,EACTC,EAAKhB,GAAKe,EAAIf,UAERgB,EAK+DF,CAAMG,GAC3EC,EAAUR,EAAES,cAAgB,sBAAuBT,IAAMA,EAAEU,mBAE5DR,EAAclB,EAAOc,OACjBE,EAAEW,aACL3B,EAAO2B,WAAaX,EAAEW,YAEnBX,EAAEF,QAAUE,EAAEF,OAAOZ,SAAWgB,EAAYhB,QAC/CY,EAASE,EAAEF,OACY,IAAnBN,EAAMoB,UACTvB,EAAgC,WAAYY,EAAIT,EAAOe,GAEpDf,EAAMqB,KACTxB,EAAgC,MAAOY,EAAIT,EAAOe,GAE1B,IAArBf,EAAMK,YACTR,EAAgC,aAAcY,EAAIT,EAAOe,GAEtDf,EAAMsB,iBACTzB,EAAgC,WAAYY,EAAIT,EAAOe,GACvDlB,EAAgC,iBAAkBY,EAAIT,EAAOe,IAErC,IAArBf,EAAMuB,YACT1B,EAAgC,aAAcY,EAAIT,EAAOe,KAI1DT,EAASkB,EAAUC,QACD,MAAdjB,EAAEY,UACLd,EAASlB,EAAakB,GAASE,EAAEY,UACjCvB,EAAgC,WAAYY,EAAIT,EAAOQ,IAC1B,IAAnBR,EAAMoB,WACZJ,EACHV,EAASlB,EAAakB,EAAQN,EAAMoB,UAEpCvB,EAAgC,WAAYY,EAAIT,EAAOe,IAG5C,MAATP,EAAEa,KACLf,EAASxB,EAAQwB,GAASE,EAAEa,KAC5BxB,EAAgC,MAAOY,EAAIT,EAAOQ,IACxCR,EAAMqB,MACZL,EACHV,EAASxB,EAAQwB,EAAQN,EAAMqB,KAE/BxB,EAAgC,MAAOY,EAAIT,EAAOe,IAGhC,MAAhBP,EAAEH,YACLC,EAASF,GAA0BI,EAAEH,WAAYC,GACjDT,EAAgC,aAAcY,EAAIT,EAAOQ,IAC1B,IAArBR,EAAMK,aACZW,EACHV,EAASF,EAAyBJ,EAAMK,WAAYC,GAEpDT,EAAgC,aAAcY,EAAIT,EAAOe,IAGzC,MAAdP,EAAEkB,UACLlB,EAAEc,eAAkB,mBAAoBd,GAAMA,EAAEc,eAAiB,EACjEhB,EAAS7B,EAAU6B,EAAQE,EAAEkB,SAAUlB,EAAEc,gBACzCzB,EAAgC,WAAYY,EAAIT,EAAOQ,GACvDX,EAAgC,iBAAkBY,EAAIT,EAAOQ,IACnDR,EAAMsB,iBACZN,EACHV,EAAS7B,EAAU6B,EAAQN,EAAM0B,SAAU1B,EAAMsB,iBAEjDzB,EAAgC,WAAYY,EAAIT,EAAOe,GACvDlB,EAAgC,iBAAkBY,EAAIT,EAAOe,KAG3C,MAAhBP,EAAEe,YACLjB,EAAStC,EAAesC,GAASE,EAAEe,YACnC1B,EAAgC,aAAcY,EAAIT,EAAOQ,IAC1B,IAArBR,EAAMuB,aACZP,EACHV,EAAStC,EAAesC,EAAQN,EAAMuB,YAEtC1B,EAAgC,aAAcY,EAAIT,EAAOe,KAI5DjD,EAAIwC,EAAOZ,aACG,IAAL5B,GACJwC,EAAOxC,KAAO4C,EAAY5C,IAC7B2C,EAAGP,IAAIQ,EAAa5C,EAAG4C,EAAY5C,GAAIwC,EAAOxC,GAAI,qBAGpD2C,EAAGN,OAAOP,KAAK,qBAED,SAAf+B,EAAgBC,SAAQC,IAAAA,EAAG/B,IAAAA,EAAGpB,IAAAA,OAC7BoD,IADoCA,KAChCD,EAAG/B,EAAGpB,EAAM,IAAM,GAAKA,EAAM,IAAM,EAAIA,EAAM,IAE9B,SAApBqD,EAAqBH,SAAQvD,IAAAA,EACxB2D,GACH3D,EAAE4D,OACF5D,EAAE6D,UACQ7D,IACVA,EAAE8D,QACF9D,EAAE+D,cAGe,SAAnBC,EAAoBT,EAAOU,GAC1BA,EAAKT,EAAEU,UAAYD,EAAKT,EAAEW,MAEV,SAAjBC,EAAkBnD,EAAQQ,EAAG/C,EAAOgD,OAC/B2C,EAAepD,EAAOQ,GACzB6C,EAAa9D,EAAY/B,EAAY4F,GAAgBpD,EAAUQ,EAAE8C,QAAQ,SAAW9F,EAAYwC,EAAO,MAAQQ,EAAE+C,OAAO,KAAQ/C,EAAI,MAAQA,EAAE+C,OAAO,MAAUH,GAC/JI,EAAWjE,EAAY9B,GACxBgD,EAAOgD,IAAM,IAAIC,EAAUjD,EAAOgD,IAAKzD,EAAQQ,EAAG,EAAG,EAAG6B,EAAc,CAACE,EAAEvC,EAAQQ,EAAEA,EAAGpB,MAAMiE,EAAYb,IAAImB,EAAW3D,EAAQQ,KAC/HC,EAAOG,IAAIyC,EAAY,EAAGA,EAAW,GAAIG,EAAS,IAClD/C,EAAOG,IAAIyC,EAAY,EAAGA,EAAW,GAAIG,EAAS,IAClD/C,EAAOG,IAAIyC,EAAY,EAAGA,EAAW,GAAIG,EAAS,IAQvC,SAAZI,EAAYnG,SAA2B,iBAAXA,EACR,SAApBoG,EAAoBpG,UAAUmG,EAAUnG,IAA8B,MAApBA,EAAMqG,OAAO,GAAcrG,EAAM8F,OAAO,EAAG,GAAMQ,WAAWtG,EAAM8F,OAAO,IAAMS,EAAYvG,EAAQuG,EAChI,SAArBC,EAAsB3B,EAAOU,UAASA,EAAKR,IAAIQ,EAAKT,EAAGS,EAAKxC,EAAa,IAAV8B,EAAcU,EAAKkB,EAAKzE,KAAK0E,MAAkC,KAA3BnB,EAAKpD,EAAIoD,EAAK1D,EAAIgD,IAAmB,IAASU,GACvH,SAA1BoB,EAA2B3D,EAAQT,EAAQqE,EAAUC,EAAUC,EAAUC,OAOvEC,EAAWC,EANRC,EAAM,KAAOH,EAAUR,EAAW,GACrCY,EAAWhB,EAAUW,GACrBM,EAAYD,GAAmC,MAAvBL,EAAST,OAAO,KAAgBS,EAAST,OAAO,GAAK,KAAO,EACpFgB,EAASf,WAAWc,EAAWN,EAAShB,OAAO,GAAKgB,IAAaC,EAAUR,EAAW,GACtFe,EAASF,EAAWC,EAASD,EAAWC,EAASR,EACjDU,EAAaV,EAAWS,SAErBH,IAEe,WADlBH,EAAYF,EAASU,MAAM,KAAK,MAE/BF,GAAUJ,KACKI,GAAUJ,EAAM,KAC9BI,GAAWA,EAAS,EAAKJ,GAAOA,GAGhB,OAAdF,GAAsBM,EAAS,EAClCA,GAAWA,EAAe,KAANJ,GAAcA,KAAUI,EAASJ,GAAOA,EACpC,QAAdF,GAAgC,EAATM,IACjCA,GAAWA,EAAe,KAANJ,GAAcA,KAAUI,EAASJ,GAAOA,IAG9DlE,EAAOgD,IAAMiB,EAAK,IAAIhB,EAAUjD,EAAOgD,IAAKzD,EAAQqE,EAAUC,EAAUS,EAAQd,GAChFS,EAAGR,EAAIc,EACAN,EAEI,SAAZQ,QACMC,EAAc,CAClB7H,EAAOD,QAEH+H,GADJpH,EAAQmH,EAAenH,GAAUb,KAAmBC,OAAOiI,OACnCrH,EAAMsH,SAAWvB,WAAW/F,EAAMsH,QAAQL,MAAM,KAAK,KAAQ,EACrFM,EAAoB,IAAZH,EACR1C,EAAuB,GAAX0C,EACZ7F,EAAc,qBAAAH,UAAS9B,EAAKkI,MAAMC,WAAwC,QAA5BrG,EAAQ,IAAImE,OAAO,EAAE,GAAc,IAAMnE,EAAMmE,OAAO,GAAKnE,KAnP5G,IAAI9B,EAAMiC,EAAa4F,EAAcnH,EAAO0F,EAAWC,EAAY4B,EAAO7C,EAqPtElE,EAAGgC,EAhPN0B,EAAY,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,GACnDpD,EAAQ,QACRE,EAAQ,OACRE,EAAQ,QA4ERuC,EAAe,CAACK,SAAS,EAAGG,WAAW,EAAGD,eAAe,EAAGI,SAAS,mBAAoBL,IAAI,EAAGhB,WAAW,GAsH3G2E,EAAc,CAACC,KAAK,EAAGC,UAAU,EAAGC,UAAU,EAAGC,YAAY,GAC7DC,EAAc,0DAA0Dd,MAAM,KAC9Ee,EAAY,CAAC1H,EAAE,WAAYD,EAAE,WAAY4H,MAAM,eAAgBC,MAAM,gBACrEC,EAA0B,CAACvE,kBAAkB,EAAGK,WAAW,EAAGH,SAAS,EAAGC,IAAI,EAAGK,SAAS,EAAGJ,eAAe,EAAGjB,WAAW,EAAGY,WAAW,GACxIqC,EAAWvE,KAAKC,GAAK,IA0CtB,IAAKlB,EAAI,EAAGA,EAAIuH,EAAY3F,OAAQ5B,IACnCgC,EAAIuF,EAAYvH,GAChBwH,EAAUxF,EAAI,KAAOA,EACrBwF,EAAUxF,EAAI,KAAOA,MAIT4F,EAAa,CACzBhB,QAAS,SACTrH,KAAM,OACNsI,2BAASC,EAAMC,EAAQC,GACtBlJ,EAAOgJ,EACP5C,EAAY8C,EACZ7C,EAAa4C,EAAOE,UACpBvB,KAEDwB,UAAU,EACVC,mCAAaC,GACZ5I,EAAQ4I,GAETC,mBAAK7G,EAAQ8G,MACZ9I,GAASkH,KACJlH,SACJN,EAAM,uDACC,MAEJqJ,EAASC,EAAMvJ,EAAOwJ,EAAa/G,EAAQM,EAAG0G,EAAS1I,EAAGwE,EAAMmE,MAC/D3G,KAAKsG,EAAQ,IACjBC,EAAUf,EAAUxF,GACpB/C,EAAQqJ,EAAOtG,GACXuG,EACHC,GAAQxG,EAAEsD,OAAOtD,EAAEJ,OAAO,GAAGgH,cAAc9D,QAAQ,KAAO,IAAM,SAC3D1C,IAAIZ,EAAO+G,GAAUC,EAAMhH,EAAO+G,GAASC,GAAoB,SAAZD,EAAsBlD,EAAkBpG,GAASA,EAAO,EAAG,EAAG,EAAG,EAAG,EAAG,QACzH,GAAU,UAAN+C,GAAuB,WAANA,GAAwB,UAANA,GAAuB,cAANA,OACzDI,IAAIZ,EAAOQ,GAAI,IAAKR,EAAOQ,GAAGlC,EAAGb,QACjCmD,IAAIZ,EAAOQ,GAAI,IAAKR,EAAOQ,GAAGnC,EAAGZ,QAChC,GAAU,aAAN+C,GAA0B,UAANA,EAC9B4D,EAAwBiD,KAAMrH,EAAQQ,EAAGR,EAAOQ,GAAI/C,EAAa,aAAN+C,QACrD,GAAI2F,EAAwB3F,GAC7ByG,IACJhG,EAAwBjB,EAAQ8G,EAAOlF,mBAAqBkF,EAAQO,MACpEJ,GAAc,QAET,GAAU,SAANzG,GAAsB,UAANA,GAAuB,UAANA,GAAuB,gBAANA,MAC5DN,EAASH,EAAWC,EAAQ,mBACvBY,IAAIV,EAAQM,EAAGN,EAAOM,GAAI/C,GACJ,IAAvBqJ,EAAOQ,gBACVJ,EAAUJ,EAAOQ,aAA4C,EAA7B7H,KAAK8H,IAAIrH,EAAOM,GAAI/C,GACpDe,EAAIwB,EAAO/B,QAAQmC,QACL,IAAL5B,GACRwB,EAAO/B,QAAQO,GAAG0I,QAAUzH,KAAK8H,IAAIvH,EAAO/B,QAAQO,GAAG0I,QAASA,QAG5D,GAAIxB,EAAYlF,OACX,cAANA,GAA2B,cAANA,GAA2B,gBAANA,IAAwBR,aAAkBhC,EAAMwJ,SAAU,CACxGxE,EAAO,cAAehD,EAAS,CAACA,IAAWA,EAAOyH,UAAYzH,GAAQ0H,aACtEP,EAAU3G,EAAE+C,OAAO,EAAG/C,EAAEJ,OAAS,GACjCsC,GAAyB,SAAZyE,IAAuBA,EAAU,eACzC1D,IAAM,IAAIC,EAAU2D,KAAK5D,IAAKzD,EAAQQ,EAAG,EAAG,EAAGiC,EAAmB,CAAC1D,EAAGiB,EAAOyH,UAAYzH,IAC9FxB,EAAIwE,EAAK5C,aACK,IAAL5B,GACR2E,EAAeoC,EAAQvC,EAAKxE,GAAKwE,EAAKxE,GAAG2I,EAAU,SAAU5B,EAAQ/E,EAAI,QAAS/C,EAAO4J,WAG1FlE,EAAenD,EAAQQ,EAAG/C,EAAO4J,UAElB,cAAN7G,QACLiD,IAAM,IAAIC,EAAU2D,KAAK5D,IAAKzD,EAAQ,UAAW,EAAG,EAAG+C,QACvDnC,IAAIZ,EAAQ,QAASA,EAAOkD,MAAOzF,QACnCoD,OAAOP,KAAK,QAAS,YACV,eAANE,QACLI,IAAIZ,EAAQQ,EAAG,MAAO/C,QAEvBoD,OAAOP,KAAKE,MAKpBnD,KAAcC,EAAKC,eAAe6I"} \ No newline at end of file diff --git a/node_modules/gsap/dist/ScrollToPlugin.js b/node_modules/gsap/dist/ScrollToPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..eabe2cc025f8cddf6dcb505985a0e42094223876 --- /dev/null +++ b/node_modules/gsap/dist/ScrollToPlugin.js @@ -0,0 +1,286 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + /*! + * ScrollToPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _coreInitted, + _window, + _docEl, + _body, + _toArray, + _config, + ScrollTrigger, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP = function _getGSAP() { + return gsap || _windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _isString = function _isString(value) { + return typeof value === "string"; + }, + _isFunction = function _isFunction(value) { + return typeof value === "function"; + }, + _max = function _max(element, axis) { + var dim = axis === "x" ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return element === _window || element === _docEl || element === _body ? Math.max(_docEl[scroll], _body[scroll]) - (_window["inner" + dim] || _docEl[client] || _body[client]) : element[scroll] - element["offset" + dim]; + }, + _buildGetter = function _buildGetter(e, axis) { + var p = "scroll" + (axis === "x" ? "Left" : "Top"); + + if (e === _window) { + if (e.pageXOffset != null) { + p = "page" + axis.toUpperCase() + "Offset"; + } else { + e = _docEl[p] != null ? _docEl : _body; + } + } + + return function () { + return e[p]; + }; + }, + _clean = function _clean(value, index, target, targets) { + _isFunction(value) && (value = value(index, target, targets)); + + if (typeof value !== "object") { + return _isString(value) && value !== "max" && value.charAt(1) !== "=" ? { + x: value, + y: value + } : { + y: value + }; + } else if (value.nodeType) { + return { + y: value, + x: value + }; + } else { + var result = {}, + p; + + for (p in value) { + result[p] = p !== "onAutoKill" && _isFunction(value[p]) ? value[p](index, target, targets) : value[p]; + } + + return result; + } + }, + _getOffset = function _getOffset(element, container) { + element = _toArray(element)[0]; + + if (!element || !element.getBoundingClientRect) { + return console.warn("scrollTo target doesn't exist. Using 0") || { + x: 0, + y: 0 + }; + } + + var rect = element.getBoundingClientRect(), + isRoot = !container || container === _window || container === _body, + cRect = isRoot ? { + top: _docEl.clientTop - (_window.pageYOffset || _docEl.scrollTop || _body.scrollTop || 0), + left: _docEl.clientLeft - (_window.pageXOffset || _docEl.scrollLeft || _body.scrollLeft || 0) + } : container.getBoundingClientRect(), + offsets = { + x: rect.left - cRect.left, + y: rect.top - cRect.top + }; + + if (!isRoot && container) { + offsets.x += _buildGetter(container, "x")(); + offsets.y += _buildGetter(container, "y")(); + } + + return offsets; + }, + _parseVal = function _parseVal(value, target, axis, currentVal, offset) { + return !isNaN(value) && typeof value !== "object" ? parseFloat(value) - offset : _isString(value) && value.charAt(1) === "=" ? parseFloat(value.substr(2)) * (value.charAt(0) === "-" ? -1 : 1) + currentVal - offset : value === "max" ? _max(target, axis) - offset : Math.min(_max(target, axis), _getOffset(value, target)[axis] - offset); + }, + _initCore = function _initCore() { + gsap = _getGSAP(); + + if (_windowExists() && gsap && typeof document !== "undefined" && document.body) { + _window = window; + _body = document.body; + _docEl = document.documentElement; + _toArray = gsap.utils.toArray; + gsap.config({ + autoKillThreshold: 7 + }); + _config = gsap.config(); + _coreInitted = 1; + } + }; + + var ScrollToPlugin = { + version: "3.12.7", + name: "scrollTo", + rawVars: 1, + register: function register(core) { + gsap = core; + + _initCore(); + }, + init: function init(target, value, tween, index, targets) { + _coreInitted || _initCore(); + var data = this, + snapType = gsap.getProperty(target, "scrollSnapType"); + data.isWin = target === _window; + data.target = target; + data.tween = tween; + value = _clean(value, index, target, targets); + data.vars = value; + data.autoKill = !!("autoKill" in value ? value : _config).autoKill; + data.getX = _buildGetter(target, "x"); + data.getY = _buildGetter(target, "y"); + data.x = data.xPrev = data.getX(); + data.y = data.yPrev = data.getY(); + ScrollTrigger || (ScrollTrigger = gsap.core.globals().ScrollTrigger); + gsap.getProperty(target, "scrollBehavior") === "smooth" && gsap.set(target, { + scrollBehavior: "auto" + }); + + if (snapType && snapType !== "none") { + data.snap = 1; + data.snapInline = target.style.scrollSnapType; + target.style.scrollSnapType = "none"; + } + + if (value.x != null) { + data.add(data, "x", data.x, _parseVal(value.x, target, "x", data.x, value.offsetX || 0), index, targets); + + data._props.push("scrollTo_x"); + } else { + data.skipX = 1; + } + + if (value.y != null) { + data.add(data, "y", data.y, _parseVal(value.y, target, "y", data.y, value.offsetY || 0), index, targets); + + data._props.push("scrollTo_y"); + } else { + data.skipY = 1; + } + }, + render: function render(ratio, data) { + var pt = data._pt, + target = data.target, + tween = data.tween, + autoKill = data.autoKill, + xPrev = data.xPrev, + yPrev = data.yPrev, + isWin = data.isWin, + snap = data.snap, + snapInline = data.snapInline, + x, + y, + yDif, + xDif, + threshold; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + x = isWin || !data.skipX ? data.getX() : xPrev; + y = isWin || !data.skipY ? data.getY() : yPrev; + yDif = y - yPrev; + xDif = x - xPrev; + threshold = _config.autoKillThreshold; + + if (data.x < 0) { + data.x = 0; + } + + if (data.y < 0) { + data.y = 0; + } + + if (autoKill) { + if (!data.skipX && (xDif > threshold || xDif < -threshold) && x < _max(target, "x")) { + data.skipX = 1; + } + + if (!data.skipY && (yDif > threshold || yDif < -threshold) && y < _max(target, "y")) { + data.skipY = 1; + } + + if (data.skipX && data.skipY) { + tween.kill(); + data.vars.onAutoKill && data.vars.onAutoKill.apply(tween, data.vars.onAutoKillParams || []); + } + } + + if (isWin) { + _window.scrollTo(!data.skipX ? data.x : x, !data.skipY ? data.y : y); + } else { + data.skipY || (target.scrollTop = data.y); + data.skipX || (target.scrollLeft = data.x); + } + + if (snap && (ratio === 1 || ratio === 0)) { + y = target.scrollTop; + x = target.scrollLeft; + snapInline ? target.style.scrollSnapType = snapInline : target.style.removeProperty("scroll-snap-type"); + target.scrollTop = y + 1; + target.scrollLeft = x + 1; + target.scrollTop = y; + target.scrollLeft = x; + } + + data.xPrev = data.x; + data.yPrev = data.y; + ScrollTrigger && ScrollTrigger.update(); + }, + kill: function kill(property) { + var both = property === "scrollTo", + i = this._props.indexOf(property); + + if (both || property === "scrollTo_x") { + this.skipX = 1; + } + + if (both || property === "scrollTo_y") { + this.skipY = 1; + } + + i > -1 && this._props.splice(i, 1); + return !this._props.length; + } + }; + ScrollToPlugin.max = _max; + ScrollToPlugin.getOffset = _getOffset; + ScrollToPlugin.buildGetter = _buildGetter; + + ScrollToPlugin.config = function (vars) { + _config || _initCore() || (_config = gsap.config()); + + for (var p in vars) { + _config[p] = vars[p]; + } + }; + + _getGSAP() && gsap.registerPlugin(ScrollToPlugin); + + exports.ScrollToPlugin = ScrollToPlugin; + exports.default = ScrollToPlugin; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/ScrollToPlugin.min.js b/node_modules/gsap/dist/ScrollToPlugin.min.js new file mode 100644 index 0000000000000000000000000000000000000000..16cf8e03a61ea9e91b0c72f3a4f5ce10a6096439 --- /dev/null +++ b/node_modules/gsap/dist/ScrollToPlugin.min.js @@ -0,0 +1,11 @@ +/*! + * ScrollToPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(e){"use strict";function l(){return"undefined"!=typeof window}function m(){return f||l()&&(f=window.gsap)&&f.registerPlugin&&f}function n(e){return"string"==typeof e}function o(e){return"function"==typeof e}function p(e,t){var o="x"===t?"Width":"Height",n="scroll"+o,r="client"+o;return e===T||e===i||e===c?Math.max(i[n],c[n])-(T["inner"+o]||i[r]||c[r]):e[n]-e["offset"+o]}function q(e,t){var o="scroll"+("x"===t?"Left":"Top");return e===T&&(null!=e.pageXOffset?o="page"+t.toUpperCase()+"Offset":e=null!=i[o]?i:c),function(){return e[o]}}function s(e,t){if(!(e=y(e)[0])||!e.getBoundingClientRect)return console.warn("scrollTo target doesn't exist. Using 0")||{x:0,y:0};var o=e.getBoundingClientRect(),n=!t||t===T||t===c,r=n?{top:i.clientTop-(T.pageYOffset||i.scrollTop||c.scrollTop||0),left:i.clientLeft-(T.pageXOffset||i.scrollLeft||c.scrollLeft||0)}:t.getBoundingClientRect(),l={x:o.left-r.left,y:o.top-r.top};return!n&&t&&(l.x+=q(t,"x")(),l.y+=q(t,"y")()),l}function t(e,t,o,r,l){return isNaN(e)||"object"==typeof e?n(e)&&"="===e.charAt(1)?parseFloat(e.substr(2))*("-"===e.charAt(0)?-1:1)+r-l:"max"===e?p(t,o)-l:Math.min(p(t,o),s(e,t)[o]-l):parseFloat(e)-l}function u(){f=m(),l()&&f&&"undefined"!=typeof document&&document.body&&(T=window,c=document.body,i=document.documentElement,y=f.utils.toArray,f.config({autoKillThreshold:7}),h=f.config(),a=1)}var f,a,T,i,c,y,h,v,r={version:"3.12.7",name:"scrollTo",rawVars:1,register:function register(e){f=e,u()},init:function init(e,r,l,i,s){a||u();var p=this,c=f.getProperty(e,"scrollSnapType");p.isWin=e===T,p.target=e,p.tween=l,r=function _clean(e,t,r,l){if(o(e)&&(e=e(t,r,l)),"object"!=typeof e)return n(e)&&"max"!==e&&"="!==e.charAt(1)?{x:e,y:e}:{y:e};if(e.nodeType)return{y:e,x:e};var i,s={};for(i in e)s[i]="onAutoKill"!==i&&o(e[i])?e[i](t,r,l):e[i];return s}(r,i,e,s),p.vars=r,p.autoKill=!!("autoKill"in r?r:h).autoKill,p.getX=q(e,"x"),p.getY=q(e,"y"),p.x=p.xPrev=p.getX(),p.y=p.yPrev=p.getY(),v=v||f.core.globals().ScrollTrigger,"smooth"===f.getProperty(e,"scrollBehavior")&&f.set(e,{scrollBehavior:"auto"}),c&&"none"!==c&&(p.snap=1,p.snapInline=e.style.scrollSnapType,e.style.scrollSnapType="none"),null!=r.x?(p.add(p,"x",p.x,t(r.x,e,"x",p.x,r.offsetX||0),i,s),p._props.push("scrollTo_x")):p.skipX=1,null!=r.y?(p.add(p,"y",p.y,t(r.y,e,"y",p.y,r.offsetY||0),i,s),p._props.push("scrollTo_y")):p.skipY=1},render:function render(e,t){for(var o,n,r,l,i,s=t._pt,c=t.target,u=t.tween,f=t.autoKill,a=t.xPrev,y=t.yPrev,d=t.isWin,g=t.snap,x=t.snapInline;s;)s.r(e,s.d),s=s._next;o=d||!t.skipX?t.getX():a,r=(n=d||!t.skipY?t.getY():y)-y,l=o-a,i=h.autoKillThreshold,t.x<0&&(t.x=0),t.y<0&&(t.y=0),f&&(!t.skipX&&(i<l||l<-i)&&o<p(c,"x")&&(t.skipX=1),!t.skipY&&(i<r||r<-i)&&n<p(c,"y")&&(t.skipY=1),t.skipX&&t.skipY&&(u.kill(),t.vars.onAutoKill&&t.vars.onAutoKill.apply(u,t.vars.onAutoKillParams||[]))),d?T.scrollTo(t.skipX?o:t.x,t.skipY?n:t.y):(t.skipY||(c.scrollTop=t.y),t.skipX||(c.scrollLeft=t.x)),!g||1!==e&&0!==e||(n=c.scrollTop,o=c.scrollLeft,x?c.style.scrollSnapType=x:c.style.removeProperty("scroll-snap-type"),c.scrollTop=n+1,c.scrollLeft=o+1,c.scrollTop=n,c.scrollLeft=o),t.xPrev=t.x,t.yPrev=t.y,v&&v.update()},kill:function kill(e){var t="scrollTo"===e,o=this._props.indexOf(e);return!t&&"scrollTo_x"!==e||(this.skipX=1),!t&&"scrollTo_y"!==e||(this.skipY=1),-1<o&&this._props.splice(o,1),!this._props.length}};r.max=p,r.getOffset=s,r.buildGetter=q,r.config=function(e){for(var t in h||u()||(h=f.config()),e)h[t]=e[t]},m()&&f.registerPlugin(r),e.ScrollToPlugin=r,e.default=r;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/ScrollToPlugin.min.js.map b/node_modules/gsap/dist/ScrollToPlugin.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..90e469f7d98cf205428e5ab77ac482fa75b0a65b --- /dev/null +++ b/node_modules/gsap/dist/ScrollToPlugin.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"ScrollToPlugin.min.js","sources":["../src/ScrollToPlugin.js"],"sourcesContent":["/*!\n * ScrollToPlugin 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _coreInitted, _window, _docEl, _body, _toArray, _config, ScrollTrigger,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_isString = value => typeof(value) === \"string\",\n\t_isFunction = value => typeof(value) === \"function\",\n\t_max = (element, axis) => {\n\t\tlet dim = (axis === \"x\") ? \"Width\" : \"Height\",\n\t\t\tscroll = \"scroll\" + dim,\n\t\t\tclient = \"client\" + dim;\n\t\treturn (element === _window || element === _docEl || element === _body) ? Math.max(_docEl[scroll], _body[scroll]) - (_window[\"inner\" + dim] || _docEl[client] || _body[client]) : element[scroll] - element[\"offset\" + dim];\n\t},\n\t_buildGetter = (e, axis) => { //pass in an element and an axis (\"x\" or \"y\") and it'll return a getter function for the scroll position of that element (like scrollTop or scrollLeft, although if the element is the window, it'll use the pageXOffset/pageYOffset or the documentElement's scrollTop/scrollLeft or document.body's. Basically this streamlines things and makes a very fast getter across browsers.\n\t\tlet p = \"scroll\" + ((axis === \"x\") ? \"Left\" : \"Top\");\n\t\tif (e === _window) {\n\t\t\tif (e.pageXOffset != null) {\n\t\t\t\tp = \"page\" + axis.toUpperCase() + \"Offset\";\n\t\t\t} else {\n\t\t\t\te = _docEl[p] != null ? _docEl : _body;\n\t\t\t}\n\t\t}\n\t\treturn () => e[p];\n\t},\n\t_clean = (value, index, target, targets) => {\n\t\t_isFunction(value) && (value = value(index, target, targets));\n\t\tif (typeof(value) !== \"object\") {\n\t\t\treturn _isString(value) && value !== \"max\" && value.charAt(1) !== \"=\" ? {x: value, y: value} : {y: value}; //if we don't receive an object as the parameter, assume the user intends \"y\".\n\t\t} else if (value.nodeType) {\n\t\t\treturn {y: value, x: value};\n\t\t} else {\n\t\t\tlet result = {}, p;\n\t\t\tfor (p in value) {\n\t\t\t\tresult[p] = p !== \"onAutoKill\" && _isFunction(value[p]) ? value[p](index, target, targets) : value[p];\n\t\t\t}\n\t\t\treturn result;\n\t\t}\n\t},\n\t_getOffset = (element, container) => {\n\t\telement = _toArray(element)[0];\n\t\tif (!element || !element.getBoundingClientRect) {\n\t\t\treturn console.warn(\"scrollTo target doesn't exist. Using 0\") || {x:0, y:0};\n\t\t}\n\t\tlet rect = element.getBoundingClientRect(),\n\t\t\tisRoot = (!container || container === _window || container === _body),\n\t\t\tcRect = isRoot ? {top:_docEl.clientTop - (_window.pageYOffset || _docEl.scrollTop || _body.scrollTop || 0), left:_docEl.clientLeft - (_window.pageXOffset || _docEl.scrollLeft || _body.scrollLeft || 0)} : container.getBoundingClientRect(),\n\t\t\toffsets = {x: rect.left - cRect.left, y: rect.top - cRect.top};\n\t\tif (!isRoot && container) { //only add the current scroll position if it's not the window/body.\n\t\t\toffsets.x += _buildGetter(container, \"x\")();\n\t\t\toffsets.y += _buildGetter(container, \"y\")();\n\t\t}\n\t\treturn offsets;\n\t},\n\t_parseVal = (value, target, axis, currentVal, offset) => !isNaN(value) && typeof(value) !== \"object\" ? parseFloat(value) - offset : (_isString(value) && value.charAt(1) === \"=\") ? parseFloat(value.substr(2)) * (value.charAt(0) === \"-\" ? -1 : 1) + currentVal - offset : (value === \"max\") ? _max(target, axis) - offset : Math.min(_max(target, axis), _getOffset(value, target)[axis] - offset),\n\t_initCore = () => {\n\t\tgsap = _getGSAP();\n\t\tif (_windowExists() && gsap && typeof(document) !== \"undefined\" && document.body) {\n\t\t\t_window = window;\n\t\t\t_body = document.body;\n\t\t\t_docEl = document.documentElement;\n\t\t\t_toArray = gsap.utils.toArray;\n\t\t\tgsap.config({autoKillThreshold:7});\n\t\t\t_config = gsap.config();\n\t\t\t_coreInitted = 1;\n\t\t}\n\t};\n\n\nexport const ScrollToPlugin = {\n\tversion: \"3.12.7\",\n\tname: \"scrollTo\",\n\trawVars: 1,\n\tregister(core) {\n\t\tgsap = core;\n\t\t_initCore();\n\t},\n\tinit(target, value, tween, index, targets) {\n\t\t_coreInitted || _initCore();\n\t\tlet data = this,\n\t\t\tsnapType = gsap.getProperty(target, \"scrollSnapType\");\n\t\tdata.isWin = (target === _window);\n\t\tdata.target = target;\n\t\tdata.tween = tween;\n\t\tvalue = _clean(value, index, target, targets);\n\t\tdata.vars = value;\n\t\tdata.autoKill = !!(\"autoKill\" in value ? value : _config).autoKill;\n\t\tdata.getX = _buildGetter(target, \"x\");\n\t\tdata.getY = _buildGetter(target, \"y\");\n\t\tdata.x = data.xPrev = data.getX();\n\t\tdata.y = data.yPrev = data.getY();\n\t\tScrollTrigger || (ScrollTrigger = gsap.core.globals().ScrollTrigger);\n\t\tgsap.getProperty(target, \"scrollBehavior\") === \"smooth\" && gsap.set(target, {scrollBehavior: \"auto\"});\n\t\tif (snapType && snapType !== \"none\") { // disable scroll snapping to avoid strange behavior\n\t\t\tdata.snap = 1;\n\t\t\tdata.snapInline = target.style.scrollSnapType;\n\t\t\ttarget.style.scrollSnapType = \"none\";\n\t\t}\n\t\tif (value.x != null) {\n\t\t\tdata.add(data, \"x\", data.x, _parseVal(value.x, target, \"x\", data.x, value.offsetX || 0), index, targets);\n\t\t\tdata._props.push(\"scrollTo_x\");\n\t\t} else {\n\t\t\tdata.skipX = 1;\n\t\t}\n\t\tif (value.y != null) {\n\t\t\tdata.add(data, \"y\", data.y, _parseVal(value.y, target, \"y\", data.y, value.offsetY || 0), index, targets);\n\t\t\tdata._props.push(\"scrollTo_y\");\n\t\t} else {\n\t\t\tdata.skipY = 1;\n\t\t}\n\t},\n\trender(ratio, data) {\n\t\tlet pt = data._pt,\n\t\t\t{ target, tween, autoKill, xPrev, yPrev, isWin, snap, snapInline } = data,\n\t\t\tx, y, yDif, xDif, threshold;\n\t\twhile (pt) {\n\t\t\tpt.r(ratio, pt.d);\n\t\t\tpt = pt._next;\n\t\t}\n\t\tx = (isWin || !data.skipX) ? data.getX() : xPrev;\n\t\ty = (isWin || !data.skipY) ? data.getY() : yPrev;\n\t\tyDif = y - yPrev;\n\t\txDif = x - xPrev;\n\t\tthreshold = _config.autoKillThreshold;\n\t\tif (data.x < 0) { //can't scroll to a position less than 0! Might happen if someone uses a Back.easeOut or Elastic.easeOut when scrolling back to the top of the page (for example)\n\t\t\tdata.x = 0;\n\t\t}\n\t\tif (data.y < 0) {\n\t\t\tdata.y = 0;\n\t\t}\n\t\tif (autoKill) {\n\t\t\t//note: iOS has a bug that throws off the scroll by several pixels, so we need to check if it's within 7 pixels of the previous one that we set instead of just looking for an exact match.\n\t\t\tif (!data.skipX && (xDif > threshold || xDif < -threshold) && x < _max(target, \"x\")) {\n\t\t\t\tdata.skipX = 1; //if the user scrolls separately, we should stop tweening!\n\t\t\t}\n\t\t\tif (!data.skipY && (yDif > threshold || yDif < -threshold) && y < _max(target, \"y\")) {\n\t\t\t\tdata.skipY = 1; //if the user scrolls separately, we should stop tweening!\n\t\t\t}\n\t\t\tif (data.skipX && data.skipY) {\n\t\t\t\ttween.kill();\n\t\t\t\tdata.vars.onAutoKill && data.vars.onAutoKill.apply(tween, data.vars.onAutoKillParams || []);\n\t\t\t}\n\t\t}\n\t\tif (isWin) {\n\t\t\t_window.scrollTo((!data.skipX) ? data.x : x, (!data.skipY) ? data.y : y);\n\t\t} else {\n\t\t\tdata.skipY || (target.scrollTop = data.y);\n\t\t\tdata.skipX || (target.scrollLeft = data.x);\n\t\t}\n\t\tif (snap && (ratio === 1 || ratio === 0)) {\n\t\t\ty = target.scrollTop;\n\t\t\tx = target.scrollLeft;\n\t\t\tsnapInline ? (target.style.scrollSnapType = snapInline) : target.style.removeProperty(\"scroll-snap-type\");\n\t\t\ttarget.scrollTop = y + 1; // bug in Safari causes the element to totally reset its scroll position when scroll-snap-type changes, so we need to set it to a slightly different value and then back again to work around this bug.\n\t\t\ttarget.scrollLeft = x + 1;\n\t\t\ttarget.scrollTop = y;\n\t\t\ttarget.scrollLeft = x;\n\t\t}\n\t\tdata.xPrev = data.x;\n\t\tdata.yPrev = data.y;\n\t\tScrollTrigger && ScrollTrigger.update();\n\t},\n\tkill(property) {\n\t\tlet both = (property === \"scrollTo\"),\n\t\t\ti = this._props.indexOf(property);\n\t\tif (both || property === \"scrollTo_x\") {\n\t\t\tthis.skipX = 1;\n\t\t}\n\t\tif (both || property === \"scrollTo_y\") {\n\t\t\tthis.skipY = 1;\n\t\t}\n\t\ti > -1 && this._props.splice(i, 1);\n\t\treturn !this._props.length;\n\t}\n};\n\nScrollToPlugin.max = _max;\nScrollToPlugin.getOffset = _getOffset;\nScrollToPlugin.buildGetter = _buildGetter;\nScrollToPlugin.config = vars => {\n\t_config || _initCore() || (_config = gsap.config()); // in case the window hasn't been defined yet.\n\tfor (let p in vars) {\n\t\t_config[p] = vars[p];\n\t}\n}\n\n_getGSAP() && gsap.registerPlugin(ScrollToPlugin);\n\nexport { ScrollToPlugin as default };"],"names":["_windowExists","window","_getGSAP","gsap","registerPlugin","_isString","value","_isFunction","_max","element","axis","dim","scroll","client","_window","_docEl","_body","Math","max","_buildGetter","e","p","pageXOffset","toUpperCase","_getOffset","container","_toArray","getBoundingClientRect","console","warn","x","y","rect","isRoot","cRect","top","clientTop","pageYOffset","scrollTop","left","clientLeft","scrollLeft","offsets","_parseVal","target","currentVal","offset","isNaN","charAt","parseFloat","substr","min","_initCore","document","body","documentElement","utils","toArray","config","autoKillThreshold","_config","_coreInitted","ScrollTrigger","ScrollToPlugin","version","name","rawVars","register","core","init","tween","index","targets","data","this","snapType","getProperty","isWin","_clean","nodeType","result","vars","autoKill","getX","getY","xPrev","yPrev","globals","set","scrollBehavior","snap","snapInline","style","scrollSnapType","add","offsetX","_props","push","skipX","offsetY","skipY","render","ratio","yDif","xDif","threshold","pt","_pt","r","d","_next","kill","onAutoKill","apply","onAutoKillParams","scrollTo","removeProperty","update","property","both","i","indexOf","splice","length","getOffset","buildGetter"],"mappings":";;;;;;;;;6MAYiB,SAAhBA,UAAyC,oBAAZC,OAClB,SAAXC,WAAiBC,GAASH,MAAoBG,EAAOF,OAAOE,OAASA,EAAKC,gBAAkBD,EAChF,SAAZE,EAAYC,SAA2B,iBAAXA,EACd,SAAdC,EAAcD,SAA2B,mBAAXA,EACvB,SAAPE,EAAQC,EAASC,OACZC,EAAgB,MAATD,EAAgB,QAAU,SACpCE,EAAS,SAAWD,EACpBE,EAAS,SAAWF,SACbF,IAAYK,GAAWL,IAAYM,GAAUN,IAAYO,EAASC,KAAKC,IAAIH,EAAOH,GAASI,EAAMJ,KAAYE,EAAQ,QAAUH,IAAQI,EAAOF,IAAWG,EAAMH,IAAWJ,EAAQG,GAAUH,EAAQ,SAAWE,GAEzM,SAAfQ,EAAgBC,EAAGV,OACdW,EAAI,UAAsB,MAATX,EAAgB,OAAS,cAC1CU,IAAMN,IACY,MAAjBM,EAAEE,YACLD,EAAI,OAASX,EAAKa,cAAgB,SAElCH,EAAiB,MAAbL,EAAOM,GAAaN,EAASC,GAG5B,kBAAMI,EAAEC,IAgBH,SAAbG,EAAcf,EAASgB,QACtBhB,EAAUiB,EAASjB,GAAS,MACXA,EAAQkB,6BACjBC,QAAQC,KAAK,2CAA6C,CAACC,EAAE,EAAGC,EAAE,OAEtEC,EAAOvB,EAAQkB,wBAClBM,GAAWR,GAAaA,IAAcX,GAAWW,IAAcT,EAC/DkB,EAAQD,EAAS,CAACE,IAAIpB,EAAOqB,WAAatB,EAAQuB,aAAetB,EAAOuB,WAAatB,EAAMsB,WAAa,GAAIC,KAAKxB,EAAOyB,YAAc1B,EAAQQ,aAAeP,EAAO0B,YAAczB,EAAMyB,YAAc,IAAMhB,EAAUE,wBACtNe,EAAU,CAACZ,EAAGE,EAAKO,KAAOL,EAAMK,KAAMR,EAAGC,EAAKG,IAAMD,EAAMC,YACtDF,GAAUR,IACdiB,EAAQZ,GAAKX,EAAaM,EAAW,IAAxBN,GACbuB,EAAQX,GAAKZ,EAAaM,EAAW,IAAxBN,IAEPuB,EAEI,SAAZC,EAAarC,EAAOsC,EAAQlC,EAAMmC,EAAYC,UAAYC,MAAMzC,IAA4B,iBAAXA,EAAoDD,EAAUC,IAA8B,MAApBA,EAAM0C,OAAO,GAAcC,WAAW3C,EAAM4C,OAAO,KAA2B,MAApB5C,EAAM0C,OAAO,IAAc,EAAI,GAAKH,EAAaC,EAAoB,QAAVxC,EAAmBE,EAAKoC,EAAQlC,GAAQoC,EAAS7B,KAAKkC,IAAI3C,EAAKoC,EAAQlC,GAAOc,EAAWlB,EAAOsC,GAAQlC,GAAQoC,GAAvRG,WAAW3C,GAASwC,EAC/G,SAAZM,IACCjD,EAAOD,IACHF,KAAmBG,GAA6B,oBAAdkD,UAA6BA,SAASC,OAC3ExC,EAAUb,OACVe,EAAQqC,SAASC,KACjBvC,EAASsC,SAASE,gBAClB7B,EAAWvB,EAAKqD,MAAMC,QACtBtD,EAAKuD,OAAO,CAACC,kBAAkB,IAC/BC,EAAUzD,EAAKuD,SACfG,EAAe,GA7DlB,IAAI1D,EAAM0D,EAAc/C,EAASC,EAAQC,EAAOU,EAAUkC,EAASE,EAkEtDC,EAAiB,CAC7BC,QAAS,SACTC,KAAM,WACNC,QAAS,EACTC,2BAASC,GACRjE,EAAOiE,EACPhB,KAEDiB,mBAAKzB,EAAQtC,EAAOgE,EAAOC,EAAOC,GACjCX,GAAgBT,QACZqB,EAAOC,KACVC,EAAWxE,EAAKyE,YAAYhC,EAAQ,kBACrC6B,EAAKI,MAASjC,IAAW9B,EACzB2D,EAAK7B,OAASA,EACd6B,EAAKH,MAAQA,EACbhE,EA3DQ,SAATwE,OAAUxE,EAAOiE,EAAO3B,EAAQ4B,MAC/BjE,EAAYD,KAAWA,EAAQA,EAAMiE,EAAO3B,EAAQ4B,IAC9B,iBAAXlE,SACHD,EAAUC,IAAoB,QAAVA,GAAuC,MAApBA,EAAM0C,OAAO,GAAa,CAAClB,EAAGxB,EAAOyB,EAAGzB,GAAS,CAACyB,EAAGzB,GAC7F,GAAIA,EAAMyE,eACT,CAAChD,EAAGzB,EAAOwB,EAAGxB,OAEJe,EAAb2D,EAAS,OACR3D,KAAKf,EACT0E,EAAO3D,GAAW,eAANA,GAAsBd,EAAYD,EAAMe,IAAMf,EAAMe,GAAGkD,EAAO3B,EAAQ4B,GAAWlE,EAAMe,UAE7F2D,EAgDAF,CAAOxE,EAAOiE,EAAO3B,EAAQ4B,GACrCC,EAAKQ,KAAO3E,EACZmE,EAAKS,YAAc,aAAc5E,EAAQA,EAAQsD,GAASsB,SAC1DT,EAAKU,KAAOhE,EAAayB,EAAQ,KACjC6B,EAAKW,KAAOjE,EAAayB,EAAQ,KACjC6B,EAAK3C,EAAI2C,EAAKY,MAAQZ,EAAKU,OAC3BV,EAAK1C,EAAI0C,EAAKa,MAAQb,EAAKW,OACTtB,EAAlBA,GAAkC3D,EAAKiE,KAAKmB,UAAUzB,cACP,WAA/C3D,EAAKyE,YAAYhC,EAAQ,mBAAkCzC,EAAKqF,IAAI5C,EAAQ,CAAC6C,eAAgB,SACzFd,GAAyB,SAAbA,IACfF,EAAKiB,KAAO,EACZjB,EAAKkB,WAAa/C,EAAOgD,MAAMC,eAC/BjD,EAAOgD,MAAMC,eAAiB,QAEhB,MAAXvF,EAAMwB,GACT2C,EAAKqB,IAAIrB,EAAM,IAAKA,EAAK3C,EAAGa,EAAUrC,EAAMwB,EAAGc,EAAQ,IAAK6B,EAAK3C,EAAGxB,EAAMyF,SAAW,GAAIxB,EAAOC,GAChGC,EAAKuB,OAAOC,KAAK,eAEjBxB,EAAKyB,MAAQ,EAEC,MAAX5F,EAAMyB,GACT0C,EAAKqB,IAAIrB,EAAM,IAAKA,EAAK1C,EAAGY,EAAUrC,EAAMyB,EAAGa,EAAQ,IAAK6B,EAAK1C,EAAGzB,EAAM6F,SAAW,GAAI5B,EAAOC,GAChGC,EAAKuB,OAAOC,KAAK,eAEjBxB,EAAK2B,MAAQ,GAGfC,uBAAOC,EAAO7B,WAGZ3C,EAAGC,EAAGwE,EAAMC,EAAMC,EAFfC,EAAKjC,EAAKkC,IACX/D,EAAmE6B,EAAnE7B,OAAQ0B,EAA2DG,EAA3DH,MAAOY,EAAoDT,EAApDS,SAAUG,EAA0CZ,EAA1CY,MAAOC,EAAmCb,EAAnCa,MAAOT,EAA4BJ,EAA5BI,MAAOa,EAAqBjB,EAArBiB,KAAMC,EAAelB,EAAfkB,WAEhDe,GACNA,EAAGE,EAAEN,EAAOI,EAAGG,GACfH,EAAKA,EAAGI,MAEThF,EAAK+C,IAAUJ,EAAKyB,MAASzB,EAAKU,OAASE,EAE3CkB,GADAxE,EAAK8C,IAAUJ,EAAK2B,MAAS3B,EAAKW,OAASE,GAChCA,EACXkB,EAAO1E,EAAIuD,EACXoB,EAAY7C,EAAQD,kBAChBc,EAAK3C,EAAI,IACZ2C,EAAK3C,EAAI,GAEN2C,EAAK1C,EAAI,IACZ0C,EAAK1C,EAAI,GAENmD,KAEET,EAAKyB,QAAiBO,EAAPD,GAAoBA,GAAQC,IAAc3E,EAAItB,EAAKoC,EAAQ,OAC9E6B,EAAKyB,MAAQ,IAETzB,EAAK2B,QAAiBK,EAAPF,GAAoBA,GAAQE,IAAc1E,EAAIvB,EAAKoC,EAAQ,OAC9E6B,EAAK2B,MAAQ,GAEV3B,EAAKyB,OAASzB,EAAK2B,QACtB9B,EAAMyC,OACNtC,EAAKQ,KAAK+B,YAAcvC,EAAKQ,KAAK+B,WAAWC,MAAM3C,EAAOG,EAAKQ,KAAKiC,kBAAoB,MAGtFrC,EACH/D,EAAQqG,SAAW1C,EAAKyB,MAAkBpE,EAAT2C,EAAK3C,EAAS2C,EAAK2B,MAAkBrE,EAAT0C,EAAK1C,IAElE0C,EAAK2B,QAAUxD,EAAON,UAAYmC,EAAK1C,GACvC0C,EAAKyB,QAAUtD,EAAOH,WAAagC,EAAK3C,KAErC4D,GAAmB,IAAVY,GAAyB,IAAVA,IAC3BvE,EAAIa,EAAON,UACXR,EAAIc,EAAOH,WACXkD,EAAc/C,EAAOgD,MAAMC,eAAiBF,EAAc/C,EAAOgD,MAAMwB,eAAe,oBACtFxE,EAAON,UAAYP,EAAI,EACvBa,EAAOH,WAAaX,EAAI,EACxBc,EAAON,UAAYP,EACnBa,EAAOH,WAAaX,GAErB2C,EAAKY,MAAQZ,EAAK3C,EAClB2C,EAAKa,MAAQb,EAAK1C,EAClB+B,GAAiBA,EAAcuD,UAEhCN,mBAAKO,OACAC,EAAqB,aAAbD,EACXE,EAAI9C,KAAKsB,OAAOyB,QAAQH,UACrBC,GAAqB,eAAbD,SACNpB,MAAQ,IAEVqB,GAAqB,eAAbD,SACNlB,MAAQ,IAET,EAALoB,GAAU9C,KAAKsB,OAAO0B,OAAOF,EAAG,IACxB9C,KAAKsB,OAAO2B,SAItB5D,EAAe7C,IAAMV,EACrBuD,EAAe6D,UAAYpG,EAC3BuC,EAAe8D,YAAc1G,EAC7B4C,EAAeL,OAAS,SAAAuB,OAElB,IAAI5D,KADTuC,GAAWR,MAAgBQ,EAAUzD,EAAKuD,UAC5BuB,EACbrB,EAAQvC,GAAK4D,EAAK5D,IAIpBnB,KAAcC,EAAKC,eAAe2D"} \ No newline at end of file diff --git a/node_modules/gsap/dist/ScrollTrigger.js b/node_modules/gsap/dist/ScrollTrigger.js new file mode 100644 index 0000000000000000000000000000000000000000..a1bb53416d505eaf75f30ff39f4e26e149ae1ea0 --- /dev/null +++ b/node_modules/gsap/dist/ScrollTrigger.js @@ -0,0 +1,3250 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + function _defineProperties(target, props) { + for (var i = 0; i < props.length; i++) { + var descriptor = props[i]; + descriptor.enumerable = descriptor.enumerable || false; + descriptor.configurable = true; + if ("value" in descriptor) descriptor.writable = true; + Object.defineProperty(target, descriptor.key, descriptor); + } + } + + function _createClass(Constructor, protoProps, staticProps) { + if (protoProps) _defineProperties(Constructor.prototype, protoProps); + if (staticProps) _defineProperties(Constructor, staticProps); + return Constructor; + } + + /*! + * Observer 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap, + _coreInitted, + _clamp, + _win, + _doc, + _docEl, + _body, + _isTouch, + _pointerType, + ScrollTrigger, + _root, + _normalizer, + _eventTypes, + _context, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }, + _startup = 1, + _observers = [], + _scrollers = [], + _proxies = [], + _getTime = Date.now, + _bridge = function _bridge(name, value) { + return value; + }, + _integrate = function _integrate() { + var core = ScrollTrigger.core, + data = core.bridge || {}, + scrollers = core._scrollers, + proxies = core._proxies; + scrollers.push.apply(scrollers, _scrollers); + proxies.push.apply(proxies, _proxies); + _scrollers = scrollers; + _proxies = proxies; + + _bridge = function _bridge(name, value) { + return data[name](value); + }; + }, + _getProxyProp = function _getProxyProp(element, property) { + return ~_proxies.indexOf(element) && _proxies[_proxies.indexOf(element) + 1][property]; + }, + _isViewport = function _isViewport(el) { + return !!~_root.indexOf(el); + }, + _addListener = function _addListener(element, type, func, passive, capture) { + return element.addEventListener(type, func, { + passive: passive !== false, + capture: !!capture + }); + }, + _removeListener = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); + }, + _scrollLeft = "scrollLeft", + _scrollTop = "scrollTop", + _onScroll = function _onScroll() { + return _normalizer && _normalizer.isPressed || _scrollers.cache++; + }, + _scrollCacheFunc = function _scrollCacheFunc(f, doNotCache) { + var cachingFunc = function cachingFunc(value) { + if (value || value === 0) { + _startup && (_win.history.scrollRestoration = "manual"); + var isNormalizing = _normalizer && _normalizer.isPressed; + value = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); + f(value); + cachingFunc.cacheID = _scrollers.cache; + isNormalizing && _bridge("ss", value); + } else if (doNotCache || _scrollers.cache !== cachingFunc.cacheID || _bridge("ref")) { + cachingFunc.cacheID = _scrollers.cache; + cachingFunc.v = f(); + } + + return cachingFunc.v + cachingFunc.offset; + }; + + cachingFunc.offset = 0; + return f && cachingFunc; + }, + _horizontal = { + s: _scrollLeft, + p: "left", + p2: "Left", + os: "right", + os2: "Right", + d: "width", + d2: "Width", + a: "x", + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win.scrollTo(value, _vertical.sc()) : _win.pageXOffset || _doc[_scrollLeft] || _docEl[_scrollLeft] || _body[_scrollLeft] || 0; + }) + }, + _vertical = { + s: _scrollTop, + p: "top", + p2: "Top", + os: "bottom", + os2: "Bottom", + d: "height", + d2: "Height", + a: "y", + op: _horizontal, + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win.scrollTo(_horizontal.sc(), value) : _win.pageYOffset || _doc[_scrollTop] || _docEl[_scrollTop] || _body[_scrollTop] || 0; + }) + }, + _getTarget = function _getTarget(t, self) { + return (self && self._ctx && self._ctx.selector || gsap.utils.toArray)(t)[0] || (typeof t === "string" && gsap.config().nullTargetWarn !== false ? console.warn("Element not found:", t) : null); + }, + _getScrollFunc = function _getScrollFunc(element, _ref) { + var s = _ref.s, + sc = _ref.sc; + _isViewport(element) && (element = _doc.scrollingElement || _docEl); + + var i = _scrollers.indexOf(element), + offset = sc === _vertical.sc ? 1 : 2; + + !~i && (i = _scrollers.push(element) - 1); + _scrollers[i + offset] || _addListener(element, "scroll", _onScroll); + var prev = _scrollers[i + offset], + func = prev || (_scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function (value) { + return arguments.length ? element[s] = value : element[s]; + }))); + func.target = element; + prev || (func.smooth = gsap.getProperty(element, "scrollBehavior") === "smooth"); + return func; + }, + _getVelocityProp = function _getVelocityProp(value, minTimeRefresh, useDelta) { + var v1 = value, + v2 = value, + t1 = _getTime(), + t2 = t1, + min = minTimeRefresh || 50, + dropToZeroTime = Math.max(500, min * 3), + update = function update(value, force) { + var t = _getTime(); + + if (force || t - t1 > min) { + v2 = v1; + v1 = value; + t2 = t1; + t1 = t; + } else if (useDelta) { + v1 += value; + } else { + v1 = v2 + (value - v2) / (t - t2) * (t1 - t2); + } + }, + reset = function reset() { + v2 = v1 = useDelta ? 0 : v1; + t2 = t1 = 0; + }, + getVelocity = function getVelocity(latestValue) { + var tOld = t2, + vOld = v2, + t = _getTime(); + + (latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue); + return t1 === t2 || t - t2 > dropToZeroTime ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000; + }; + + return { + update: update, + reset: reset, + getVelocity: getVelocity + }; + }, + _getEvent = function _getEvent(e, preventDefault) { + preventDefault && !e._gsapAllow && e.preventDefault(); + return e.changedTouches ? e.changedTouches[0] : e; + }, + _getAbsoluteMax = function _getAbsoluteMax(a) { + var max = Math.max.apply(Math, a), + min = Math.min.apply(Math, a); + return Math.abs(max) >= Math.abs(min) ? max : min; + }, + _setScrollTrigger = function _setScrollTrigger() { + ScrollTrigger = gsap.core.globals().ScrollTrigger; + ScrollTrigger && ScrollTrigger.core && _integrate(); + }, + _initCore = function _initCore(core) { + gsap = core || _getGSAP(); + + if (!_coreInitted && gsap && typeof document !== "undefined" && document.body) { + _win = window; + _doc = document; + _docEl = _doc.documentElement; + _body = _doc.body; + _root = [_win, _doc, _docEl, _body]; + _clamp = gsap.utils.clamp; + + _context = gsap.core.context || function () {}; + + _pointerType = "onpointerenter" in _body ? "pointer" : "mouse"; + _isTouch = Observer.isTouch = _win.matchMedia && _win.matchMedia("(hover: none), (pointer: coarse)").matches ? 1 : "ontouchstart" in _win || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0 ? 2 : 0; + _eventTypes = Observer.eventTypes = ("ontouchstart" in _docEl ? "touchstart,touchmove,touchcancel,touchend" : !("onpointerdown" in _docEl) ? "mousedown,mousemove,mouseup,mouseup" : "pointerdown,pointermove,pointercancel,pointerup").split(","); + setTimeout(function () { + return _startup = 0; + }, 500); + + _setScrollTrigger(); + + _coreInitted = 1; + } + + return _coreInitted; + }; + + _horizontal.op = _vertical; + _scrollers.cache = 0; + var Observer = function () { + function Observer(vars) { + this.init(vars); + } + + var _proto = Observer.prototype; + + _proto.init = function init(vars) { + _coreInitted || _initCore(gsap) || console.warn("Please gsap.registerPlugin(Observer)"); + ScrollTrigger || _setScrollTrigger(); + var tolerance = vars.tolerance, + dragMinimum = vars.dragMinimum, + type = vars.type, + target = vars.target, + lineHeight = vars.lineHeight, + debounce = vars.debounce, + preventDefault = vars.preventDefault, + onStop = vars.onStop, + onStopDelay = vars.onStopDelay, + ignore = vars.ignore, + wheelSpeed = vars.wheelSpeed, + event = vars.event, + onDragStart = vars.onDragStart, + onDragEnd = vars.onDragEnd, + onDrag = vars.onDrag, + onPress = vars.onPress, + onRelease = vars.onRelease, + onRight = vars.onRight, + onLeft = vars.onLeft, + onUp = vars.onUp, + onDown = vars.onDown, + onChangeX = vars.onChangeX, + onChangeY = vars.onChangeY, + onChange = vars.onChange, + onToggleX = vars.onToggleX, + onToggleY = vars.onToggleY, + onHover = vars.onHover, + onHoverEnd = vars.onHoverEnd, + onMove = vars.onMove, + ignoreCheck = vars.ignoreCheck, + isNormalizer = vars.isNormalizer, + onGestureStart = vars.onGestureStart, + onGestureEnd = vars.onGestureEnd, + onWheel = vars.onWheel, + onEnable = vars.onEnable, + onDisable = vars.onDisable, + onClick = vars.onClick, + scrollSpeed = vars.scrollSpeed, + capture = vars.capture, + allowClicks = vars.allowClicks, + lockAxis = vars.lockAxis, + onLockAxis = vars.onLockAxis; + this.target = target = _getTarget(target) || _docEl; + this.vars = vars; + ignore && (ignore = gsap.utils.toArray(ignore)); + tolerance = tolerance || 1e-9; + dragMinimum = dragMinimum || 0; + wheelSpeed = wheelSpeed || 1; + scrollSpeed = scrollSpeed || 1; + type = type || "wheel,touch,pointer"; + debounce = debounce !== false; + lineHeight || (lineHeight = parseFloat(_win.getComputedStyle(_body).lineHeight) || 22); + + var id, + onStopDelayedCall, + dragged, + moved, + wheeled, + locked, + axis, + self = this, + prevDeltaX = 0, + prevDeltaY = 0, + passive = vars.passive || !preventDefault && vars.passive !== false, + scrollFuncX = _getScrollFunc(target, _horizontal), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollX = scrollFuncX(), + scrollY = scrollFuncY(), + limitToTouch = ~type.indexOf("touch") && !~type.indexOf("pointer") && _eventTypes[0] === "pointerdown", + isViewport = _isViewport(target), + ownerDoc = target.ownerDocument || _doc, + deltaX = [0, 0, 0], + deltaY = [0, 0, 0], + onClickTime = 0, + clickCapture = function clickCapture() { + return onClickTime = _getTime(); + }, + _ignoreCheck = function _ignoreCheck(e, isPointerOrTouch) { + return (self.event = e) && ignore && ~ignore.indexOf(e.target) || isPointerOrTouch && limitToTouch && e.pointerType !== "touch" || ignoreCheck && ignoreCheck(e, isPointerOrTouch); + }, + onStopFunc = function onStopFunc() { + self._vx.reset(); + + self._vy.reset(); + + onStopDelayedCall.pause(); + onStop && onStop(self); + }, + update = function update() { + var dx = self.deltaX = _getAbsoluteMax(deltaX), + dy = self.deltaY = _getAbsoluteMax(deltaY), + changedX = Math.abs(dx) >= tolerance, + changedY = Math.abs(dy) >= tolerance; + + onChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); + + if (changedX) { + onRight && self.deltaX > 0 && onRight(self); + onLeft && self.deltaX < 0 && onLeft(self); + onChangeX && onChangeX(self); + onToggleX && self.deltaX < 0 !== prevDeltaX < 0 && onToggleX(self); + prevDeltaX = self.deltaX; + deltaX[0] = deltaX[1] = deltaX[2] = 0; + } + + if (changedY) { + onDown && self.deltaY > 0 && onDown(self); + onUp && self.deltaY < 0 && onUp(self); + onChangeY && onChangeY(self); + onToggleY && self.deltaY < 0 !== prevDeltaY < 0 && onToggleY(self); + prevDeltaY = self.deltaY; + deltaY[0] = deltaY[1] = deltaY[2] = 0; + } + + if (moved || dragged) { + onMove && onMove(self); + + if (dragged) { + onDragStart && dragged === 1 && onDragStart(self); + onDrag && onDrag(self); + dragged = 0; + } + + moved = false; + } + + locked && !(locked = false) && onLockAxis && onLockAxis(self); + + if (wheeled) { + onWheel(self); + wheeled = false; + } + + id = 0; + }, + onDelta = function onDelta(x, y, index) { + deltaX[index] += x; + deltaY[index] += y; + + self._vx.update(x); + + self._vy.update(y); + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + onTouchOrPointerDelta = function onTouchOrPointerDelta(x, y) { + if (lockAxis && !axis) { + self.axis = axis = Math.abs(x) > Math.abs(y) ? "x" : "y"; + locked = true; + } + + if (axis !== "y") { + deltaX[2] += x; + + self._vx.update(x, true); + } + + if (axis !== "x") { + deltaY[2] += y; + + self._vy.update(y, true); + } + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + _onDrag = function _onDrag(e) { + if (_ignoreCheck(e, 1)) { + return; + } + + e = _getEvent(e, preventDefault); + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y, + isDragging = self.isDragging; + self.x = x; + self.y = y; + + if (isDragging || (dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum)) { + dragged = isDragging ? 2 : 1; + isDragging || (self.isDragging = true); + onTouchOrPointerDelta(dx, dy); + } + }, + _onPress = self.onPress = function (e) { + if (_ignoreCheck(e, 1) || e && e.button) { + return; + } + + self.axis = axis = null; + onStopDelayedCall.pause(); + self.isPressed = true; + e = _getEvent(e); + prevDeltaX = prevDeltaY = 0; + self.startX = self.x = e.clientX; + self.startY = self.y = e.clientY; + + self._vx.reset(); + + self._vy.reset(); + + _addListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true); + + self.deltaX = self.deltaY = 0; + onPress && onPress(self); + }, + _onRelease = self.onRelease = function (e) { + if (_ignoreCheck(e, 1)) { + return; + } + + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + + var isTrackingDrag = !isNaN(self.y - self.startY), + wasDragging = self.isDragging, + isDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), + eventData = _getEvent(e); + + if (!isDragNotClick && isTrackingDrag) { + self._vx.reset(); + + self._vy.reset(); + + if (preventDefault && allowClicks) { + gsap.delayedCall(0.08, function () { + if (_getTime() - onClickTime > 300 && !e.defaultPrevented) { + if (e.target.click) { + e.target.click(); + } else if (ownerDoc.createEvent) { + var syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null); + e.target.dispatchEvent(syntheticEvent); + } + } + }); + } + } + + self.isDragging = self.isGesturing = self.isPressed = false; + onStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true); + dragged && update(); + onDragEnd && wasDragging && onDragEnd(self); + onRelease && onRelease(self, isDragNotClick); + }, + _onGestureStart = function _onGestureStart(e) { + return e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging); + }, + _onGestureEnd = function _onGestureEnd() { + return (self.isGesturing = false) || onGestureEnd(self); + }, + onScroll = function onScroll(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = scrollFuncX(), + y = scrollFuncY(); + onDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1); + scrollX = x; + scrollY = y; + onStop && onStopDelayedCall.restart(true); + }, + _onWheel = function _onWheel(e) { + if (_ignoreCheck(e)) { + return; + } + + e = _getEvent(e, preventDefault); + onWheel && (wheeled = true); + var multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win.innerHeight : 1) * wheelSpeed; + onDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0); + onStop && !isNormalizer && onStopDelayedCall.restart(true); + }, + _onMove = function _onMove(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y; + self.x = x; + self.y = y; + moved = true; + onStop && onStopDelayedCall.restart(true); + (dx || dy) && onTouchOrPointerDelta(dx, dy); + }, + _onHover = function _onHover(e) { + self.event = e; + onHover(self); + }, + _onHoverEnd = function _onHoverEnd(e) { + self.event = e; + onHoverEnd(self); + }, + _onClick = function _onClick(e) { + return _ignoreCheck(e) || _getEvent(e, preventDefault) && onClick(self); + }; + + onStopDelayedCall = self._dc = gsap.delayedCall(onStopDelay || 0.25, onStopFunc).pause(); + self.deltaX = self.deltaY = 0; + self._vx = _getVelocityProp(0, 50, true); + self._vy = _getVelocityProp(0, 50, true); + self.scrollX = scrollFuncX; + self.scrollY = scrollFuncY; + self.isDragging = self.isGesturing = self.isPressed = false; + + _context(this); + + self.enable = function (e) { + if (!self.isEnabled) { + _addListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + + type.indexOf("scroll") >= 0 && _addListener(isViewport ? ownerDoc : target, "scroll", onScroll, passive, capture); + type.indexOf("wheel") >= 0 && _addListener(target, "wheel", _onWheel, passive, capture); + + if (type.indexOf("touch") >= 0 && _isTouch || type.indexOf("pointer") >= 0) { + _addListener(target, _eventTypes[0], _onPress, passive, capture); + + _addListener(ownerDoc, _eventTypes[2], _onRelease); + + _addListener(ownerDoc, _eventTypes[3], _onRelease); + + allowClicks && _addListener(target, "click", clickCapture, true, true); + onClick && _addListener(target, "click", _onClick); + onGestureStart && _addListener(ownerDoc, "gesturestart", _onGestureStart); + onGestureEnd && _addListener(ownerDoc, "gestureend", _onGestureEnd); + onHover && _addListener(target, _pointerType + "enter", _onHover); + onHoverEnd && _addListener(target, _pointerType + "leave", _onHoverEnd); + onMove && _addListener(target, _pointerType + "move", _onMove); + } + + self.isEnabled = true; + self.isDragging = self.isGesturing = self.isPressed = moved = dragged = false; + + self._vx.reset(); + + self._vy.reset(); + + scrollX = scrollFuncX(); + scrollY = scrollFuncY(); + e && e.type && _onPress(e); + onEnable && onEnable(self); + } + + return self; + }; + + self.disable = function () { + if (self.isEnabled) { + _observers.filter(function (o) { + return o !== self && _isViewport(o.target); + }).length || _removeListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + + if (self.isPressed) { + self._vx.reset(); + + self._vy.reset(); + + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + } + + _removeListener(isViewport ? ownerDoc : target, "scroll", onScroll, capture); + + _removeListener(target, "wheel", _onWheel, capture); + + _removeListener(target, _eventTypes[0], _onPress, capture); + + _removeListener(ownerDoc, _eventTypes[2], _onRelease); + + _removeListener(ownerDoc, _eventTypes[3], _onRelease); + + _removeListener(target, "click", clickCapture, true); + + _removeListener(target, "click", _onClick); + + _removeListener(ownerDoc, "gesturestart", _onGestureStart); + + _removeListener(ownerDoc, "gestureend", _onGestureEnd); + + _removeListener(target, _pointerType + "enter", _onHover); + + _removeListener(target, _pointerType + "leave", _onHoverEnd); + + _removeListener(target, _pointerType + "move", _onMove); + + self.isEnabled = self.isPressed = self.isDragging = false; + onDisable && onDisable(self); + } + }; + + self.kill = self.revert = function () { + self.disable(); + + var i = _observers.indexOf(self); + + i >= 0 && _observers.splice(i, 1); + _normalizer === self && (_normalizer = 0); + }; + + _observers.push(self); + + isNormalizer && _isViewport(target) && (_normalizer = self); + self.enable(event); + }; + + _createClass(Observer, [{ + key: "velocityX", + get: function get() { + return this._vx.getVelocity(); + } + }, { + key: "velocityY", + get: function get() { + return this._vy.getVelocity(); + } + }]); + + return Observer; + }(); + Observer.version = "3.12.7"; + + Observer.create = function (vars) { + return new Observer(vars); + }; + + Observer.register = _initCore; + + Observer.getAll = function () { + return _observers.slice(); + }; + + Observer.getById = function (id) { + return _observers.filter(function (o) { + return o.vars.id === id; + })[0]; + }; + + _getGSAP() && gsap.registerPlugin(Observer); + + /*! + * ScrollTrigger 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var gsap$1, + _coreInitted$1, + _win$1, + _doc$1, + _docEl$1, + _body$1, + _root$1, + _resizeDelay, + _toArray, + _clamp$1, + _time2, + _syncInterval, + _refreshing, + _pointerIsDown, + _transformProp, + _i, + _prevWidth, + _prevHeight, + _autoRefresh, + _sort, + _suppressOverwrites, + _ignoreResize, + _normalizer$1, + _ignoreMobileResize, + _baseScreenHeight, + _baseScreenWidth, + _fixIOSBug, + _context$1, + _scrollRestoration, + _div100vh, + _100vh, + _isReverted, + _clampingMax, + _limitCallbacks, + _startup$1 = 1, + _getTime$1 = Date.now, + _time1 = _getTime$1(), + _lastScrollTime = 0, + _enabled = 0, + _parseClamp = function _parseClamp(value, type, self) { + var clamp = _isString(value) && (value.substr(0, 6) === "clamp(" || value.indexOf("max") > -1); + self["_" + type + "Clamp"] = clamp; + return clamp ? value.substr(6, value.length - 7) : value; + }, + _keepClamp = function _keepClamp(value, clamp) { + return clamp && (!_isString(value) || value.substr(0, 6) !== "clamp(") ? "clamp(" + value + ")" : value; + }, + _rafBugFix = function _rafBugFix() { + return _enabled && requestAnimationFrame(_rafBugFix); + }, + _pointerDownHandler = function _pointerDownHandler() { + return _pointerIsDown = 1; + }, + _pointerUpHandler = function _pointerUpHandler() { + return _pointerIsDown = 0; + }, + _passThrough = function _passThrough(v) { + return v; + }, + _round = function _round(value) { + return Math.round(value * 100000) / 100000 || 0; + }, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$1 = function _getGSAP() { + return gsap$1 || _windowExists() && (gsap$1 = window.gsap) && gsap$1.registerPlugin && gsap$1; + }, + _isViewport$1 = function _isViewport(e) { + return !!~_root$1.indexOf(e); + }, + _getViewportDimension = function _getViewportDimension(dimensionProperty) { + return (dimensionProperty === "Height" ? _100vh : _win$1["inner" + dimensionProperty]) || _docEl$1["client" + dimensionProperty] || _body$1["client" + dimensionProperty]; + }, + _getBoundsFunc = function _getBoundsFunc(element) { + return _getProxyProp(element, "getBoundingClientRect") || (_isViewport$1(element) ? function () { + _winOffsets.width = _win$1.innerWidth; + _winOffsets.height = _100vh; + return _winOffsets; + } : function () { + return _getBounds(element); + }); + }, + _getSizeFunc = function _getSizeFunc(scroller, isViewport, _ref) { + var d = _ref.d, + d2 = _ref.d2, + a = _ref.a; + return (a = _getProxyProp(scroller, "getBoundingClientRect")) ? function () { + return a()[d]; + } : function () { + return (isViewport ? _getViewportDimension(d2) : scroller["client" + d2]) || 0; + }; + }, + _getOffsetsFunc = function _getOffsetsFunc(element, isViewport) { + return !isViewport || ~_proxies.indexOf(element) ? _getBoundsFunc(element) : function () { + return _winOffsets; + }; + }, + _maxScroll = function _maxScroll(element, _ref2) { + var s = _ref2.s, + d2 = _ref2.d2, + d = _ref2.d, + a = _ref2.a; + return Math.max(0, (s = "scroll" + d2) && (a = _getProxyProp(element, s)) ? a() - _getBoundsFunc(element)()[d] : _isViewport$1(element) ? (_docEl$1[s] || _body$1[s]) - _getViewportDimension(d2) : element[s] - element["offset" + d2]); + }, + _iterateAutoRefresh = function _iterateAutoRefresh(func, events) { + for (var i = 0; i < _autoRefresh.length; i += 3) { + (!events || ~events.indexOf(_autoRefresh[i + 1])) && func(_autoRefresh[i], _autoRefresh[i + 1], _autoRefresh[i + 2]); + } + }, + _isString = function _isString(value) { + return typeof value === "string"; + }, + _isFunction = function _isFunction(value) { + return typeof value === "function"; + }, + _isNumber = function _isNumber(value) { + return typeof value === "number"; + }, + _isObject = function _isObject(value) { + return typeof value === "object"; + }, + _endAnimation = function _endAnimation(animation, reversed, pause) { + return animation && animation.progress(reversed ? 0 : 1) && pause && animation.pause(); + }, + _callback = function _callback(self, func) { + if (self.enabled) { + var result = self._ctx ? self._ctx.add(function () { + return func(self); + }) : func(self); + result && result.totalTime && (self.callbackAnimation = result); + } + }, + _abs = Math.abs, + _left = "left", + _top = "top", + _right = "right", + _bottom = "bottom", + _width = "width", + _height = "height", + _Right = "Right", + _Left = "Left", + _Top = "Top", + _Bottom = "Bottom", + _padding = "padding", + _margin = "margin", + _Width = "Width", + _Height = "Height", + _px = "px", + _getComputedStyle = function _getComputedStyle(element) { + return _win$1.getComputedStyle(element); + }, + _makePositionable = function _makePositionable(element) { + var position = _getComputedStyle(element).position; + + element.style.position = position === "absolute" || position === "fixed" ? position : "relative"; + }, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + p in obj || (obj[p] = defaults[p]); + } + + return obj; + }, + _getBounds = function _getBounds(element, withoutTransforms) { + var tween = withoutTransforms && _getComputedStyle(element)[_transformProp] !== "matrix(1, 0, 0, 1, 0, 0)" && gsap$1.to(element, { + x: 0, + y: 0, + xPercent: 0, + yPercent: 0, + rotation: 0, + rotationX: 0, + rotationY: 0, + scale: 1, + skewX: 0, + skewY: 0 + }).progress(1), + bounds = element.getBoundingClientRect(); + tween && tween.progress(0).kill(); + return bounds; + }, + _getSize = function _getSize(element, _ref3) { + var d2 = _ref3.d2; + return element["offset" + d2] || element["client" + d2] || 0; + }, + _getLabelRatioArray = function _getLabelRatioArray(timeline) { + var a = [], + labels = timeline.labels, + duration = timeline.duration(), + p; + + for (p in labels) { + a.push(labels[p] / duration); + } + + return a; + }, + _getClosestLabel = function _getClosestLabel(animation) { + return function (value) { + return gsap$1.utils.snap(_getLabelRatioArray(animation), value); + }; + }, + _snapDirectional = function _snapDirectional(snapIncrementOrArray) { + var snap = gsap$1.utils.snap(snapIncrementOrArray), + a = Array.isArray(snapIncrementOrArray) && snapIncrementOrArray.slice(0).sort(function (a, b) { + return a - b; + }); + return a ? function (value, direction, threshold) { + if (threshold === void 0) { + threshold = 1e-3; + } + + var i; + + if (!direction) { + return snap(value); + } + + if (direction > 0) { + value -= threshold; + + for (i = 0; i < a.length; i++) { + if (a[i] >= value) { + return a[i]; + } + } + + return a[i - 1]; + } else { + i = a.length; + value += threshold; + + while (i--) { + if (a[i] <= value) { + return a[i]; + } + } + } + + return a[0]; + } : function (value, direction, threshold) { + if (threshold === void 0) { + threshold = 1e-3; + } + + var snapped = snap(value); + return !direction || Math.abs(snapped - value) < threshold || snapped - value < 0 === direction < 0 ? snapped : snap(direction < 0 ? value - snapIncrementOrArray : value + snapIncrementOrArray); + }; + }, + _getLabelAtDirection = function _getLabelAtDirection(timeline) { + return function (value, st) { + return _snapDirectional(_getLabelRatioArray(timeline))(value, st.direction); + }; + }, + _multiListener = function _multiListener(func, element, types, callback) { + return types.split(",").forEach(function (type) { + return func(element, type, callback); + }); + }, + _addListener$1 = function _addListener(element, type, func, nonPassive, capture) { + return element.addEventListener(type, func, { + passive: !nonPassive, + capture: !!capture + }); + }, + _removeListener$1 = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); + }, + _wheelListener = function _wheelListener(func, el, scrollFunc) { + scrollFunc = scrollFunc && scrollFunc.wheelHandler; + + if (scrollFunc) { + func(el, "wheel", scrollFunc); + func(el, "touchmove", scrollFunc); + } + }, + _markerDefaults = { + startColor: "green", + endColor: "red", + indent: 0, + fontSize: "16px", + fontWeight: "normal" + }, + _defaults = { + toggleActions: "play", + anticipatePin: 0 + }, + _keywords = { + top: 0, + left: 0, + center: 0.5, + bottom: 1, + right: 1 + }, + _offsetToPx = function _offsetToPx(value, size) { + if (_isString(value)) { + var eqIndex = value.indexOf("="), + relative = ~eqIndex ? +(value.charAt(eqIndex - 1) + 1) * parseFloat(value.substr(eqIndex + 1)) : 0; + + if (~eqIndex) { + value.indexOf("%") > eqIndex && (relative *= size / 100); + value = value.substr(0, eqIndex - 1); + } + + value = relative + (value in _keywords ? _keywords[value] * size : ~value.indexOf("%") ? parseFloat(value) * size / 100 : parseFloat(value) || 0); + } + + return value; + }, + _createMarker = function _createMarker(type, name, container, direction, _ref4, offset, matchWidthEl, containerAnimation) { + var startColor = _ref4.startColor, + endColor = _ref4.endColor, + fontSize = _ref4.fontSize, + indent = _ref4.indent, + fontWeight = _ref4.fontWeight; + + var e = _doc$1.createElement("div"), + useFixedPosition = _isViewport$1(container) || _getProxyProp(container, "pinType") === "fixed", + isScroller = type.indexOf("scroller") !== -1, + parent = useFixedPosition ? _body$1 : container, + isStart = type.indexOf("start") !== -1, + color = isStart ? startColor : endColor, + css = "border-color:" + color + ";font-size:" + fontSize + ";color:" + color + ";font-weight:" + fontWeight + ";pointer-events:none;white-space:nowrap;font-family:sans-serif,Arial;z-index:1000;padding:4px 8px;border-width:0;border-style:solid;"; + + css += "position:" + ((isScroller || containerAnimation) && useFixedPosition ? "fixed;" : "absolute;"); + (isScroller || containerAnimation || !useFixedPosition) && (css += (direction === _vertical ? _right : _bottom) + ":" + (offset + parseFloat(indent)) + "px;"); + matchWidthEl && (css += "box-sizing:border-box;text-align:left;width:" + matchWidthEl.offsetWidth + "px;"); + e._isStart = isStart; + e.setAttribute("class", "gsap-marker-" + type + (name ? " marker-" + name : "")); + e.style.cssText = css; + e.innerText = name || name === 0 ? type + "-" + name : type; + parent.children[0] ? parent.insertBefore(e, parent.children[0]) : parent.appendChild(e); + e._offset = e["offset" + direction.op.d2]; + + _positionMarker(e, 0, direction, isStart); + + return e; + }, + _positionMarker = function _positionMarker(marker, start, direction, flipped) { + var vars = { + display: "block" + }, + side = direction[flipped ? "os2" : "p2"], + oppositeSide = direction[flipped ? "p2" : "os2"]; + marker._isFlipped = flipped; + vars[direction.a + "Percent"] = flipped ? -100 : 0; + vars[direction.a] = flipped ? "1px" : 0; + vars["border" + side + _Width] = 1; + vars["border" + oppositeSide + _Width] = 0; + vars[direction.p] = start + "px"; + gsap$1.set(marker, vars); + }, + _triggers = [], + _ids = {}, + _rafID, + _sync = function _sync() { + return _getTime$1() - _lastScrollTime > 34 && (_rafID || (_rafID = requestAnimationFrame(_updateAll))); + }, + _onScroll$1 = function _onScroll() { + if (!_normalizer$1 || !_normalizer$1.isPressed || _normalizer$1.startX > _body$1.clientWidth) { + _scrollers.cache++; + + if (_normalizer$1) { + _rafID || (_rafID = requestAnimationFrame(_updateAll)); + } else { + _updateAll(); + } + + _lastScrollTime || _dispatch("scrollStart"); + _lastScrollTime = _getTime$1(); + } + }, + _setBaseDimensions = function _setBaseDimensions() { + _baseScreenWidth = _win$1.innerWidth; + _baseScreenHeight = _win$1.innerHeight; + }, + _onResize = function _onResize(force) { + _scrollers.cache++; + (force === true || !_refreshing && !_ignoreResize && !_doc$1.fullscreenElement && !_doc$1.webkitFullscreenElement && (!_ignoreMobileResize || _baseScreenWidth !== _win$1.innerWidth || Math.abs(_win$1.innerHeight - _baseScreenHeight) > _win$1.innerHeight * 0.25)) && _resizeDelay.restart(true); + }, + _listeners = {}, + _emptyArray = [], + _softRefresh = function _softRefresh() { + return _removeListener$1(ScrollTrigger$1, "scrollEnd", _softRefresh) || _refreshAll(true); + }, + _dispatch = function _dispatch(type) { + return _listeners[type] && _listeners[type].map(function (f) { + return f(); + }) || _emptyArray; + }, + _savedStyles = [], + _revertRecorded = function _revertRecorded(media) { + for (var i = 0; i < _savedStyles.length; i += 5) { + if (!media || _savedStyles[i + 4] && _savedStyles[i + 4].query === media) { + _savedStyles[i].style.cssText = _savedStyles[i + 1]; + _savedStyles[i].getBBox && _savedStyles[i].setAttribute("transform", _savedStyles[i + 2] || ""); + _savedStyles[i + 3].uncache = 1; + } + } + }, + _revertAll = function _revertAll(kill, media) { + var trigger; + + for (_i = 0; _i < _triggers.length; _i++) { + trigger = _triggers[_i]; + + if (trigger && (!media || trigger._ctx === media)) { + if (kill) { + trigger.kill(1); + } else { + trigger.revert(true, true); + } + } + } + + _isReverted = true; + media && _revertRecorded(media); + media || _dispatch("revert"); + }, + _clearScrollMemory = function _clearScrollMemory(scrollRestoration, force) { + _scrollers.cache++; + (force || !_refreshingAll) && _scrollers.forEach(function (obj) { + return _isFunction(obj) && obj.cacheID++ && (obj.rec = 0); + }); + _isString(scrollRestoration) && (_win$1.history.scrollRestoration = _scrollRestoration = scrollRestoration); + }, + _refreshingAll, + _refreshID = 0, + _queueRefreshID, + _queueRefreshAll = function _queueRefreshAll() { + if (_queueRefreshID !== _refreshID) { + var id = _queueRefreshID = _refreshID; + requestAnimationFrame(function () { + return id === _refreshID && _refreshAll(true); + }); + } + }, + _refresh100vh = function _refresh100vh() { + _body$1.appendChild(_div100vh); + + _100vh = !_normalizer$1 && _div100vh.offsetHeight || _win$1.innerHeight; + + _body$1.removeChild(_div100vh); + }, + _hideAllMarkers = function _hideAllMarkers(hide) { + return _toArray(".gsap-marker-start, .gsap-marker-end, .gsap-marker-scroller-start, .gsap-marker-scroller-end").forEach(function (el) { + return el.style.display = hide ? "none" : "block"; + }); + }, + _refreshAll = function _refreshAll(force, skipRevert) { + _docEl$1 = _doc$1.documentElement; + _body$1 = _doc$1.body; + _root$1 = [_win$1, _doc$1, _docEl$1, _body$1]; + + if (_lastScrollTime && !force && !_isReverted) { + _addListener$1(ScrollTrigger$1, "scrollEnd", _softRefresh); + + return; + } + + _refresh100vh(); + + _refreshingAll = ScrollTrigger$1.isRefreshing = true; + + _scrollers.forEach(function (obj) { + return _isFunction(obj) && ++obj.cacheID && (obj.rec = obj()); + }); + + var refreshInits = _dispatch("refreshInit"); + + _sort && ScrollTrigger$1.sort(); + skipRevert || _revertAll(); + + _scrollers.forEach(function (obj) { + if (_isFunction(obj)) { + obj.smooth && (obj.target.style.scrollBehavior = "auto"); + obj(0); + } + }); + + _triggers.slice(0).forEach(function (t) { + return t.refresh(); + }); + + _isReverted = false; + + _triggers.forEach(function (t) { + if (t._subPinOffset && t.pin) { + var prop = t.vars.horizontal ? "offsetWidth" : "offsetHeight", + original = t.pin[prop]; + t.revert(true, 1); + t.adjustPinSpacing(t.pin[prop] - original); + t.refresh(); + } + }); + + _clampingMax = 1; + + _hideAllMarkers(true); + + _triggers.forEach(function (t) { + var max = _maxScroll(t.scroller, t._dir), + endClamp = t.vars.end === "max" || t._endClamp && t.end > max, + startClamp = t._startClamp && t.start >= max; + + (endClamp || startClamp) && t.setPositions(startClamp ? max - 1 : t.start, endClamp ? Math.max(startClamp ? max : t.start + 1, max) : t.end, true); + }); + + _hideAllMarkers(false); + + _clampingMax = 0; + refreshInits.forEach(function (result) { + return result && result.render && result.render(-1); + }); + + _scrollers.forEach(function (obj) { + if (_isFunction(obj)) { + obj.smooth && requestAnimationFrame(function () { + return obj.target.style.scrollBehavior = "smooth"; + }); + obj.rec && obj(obj.rec); + } + }); + + _clearScrollMemory(_scrollRestoration, 1); + + _resizeDelay.pause(); + + _refreshID++; + _refreshingAll = 2; + + _updateAll(2); + + _triggers.forEach(function (t) { + return _isFunction(t.vars.onRefresh) && t.vars.onRefresh(t); + }); + + _refreshingAll = ScrollTrigger$1.isRefreshing = false; + + _dispatch("refresh"); + }, + _lastScroll = 0, + _direction = 1, + _primary, + _updateAll = function _updateAll(force) { + if (force === 2 || !_refreshingAll && !_isReverted) { + ScrollTrigger$1.isUpdating = true; + _primary && _primary.update(0); + + var l = _triggers.length, + time = _getTime$1(), + recordVelocity = time - _time1 >= 50, + scroll = l && _triggers[0].scroll(); + + _direction = _lastScroll > scroll ? -1 : 1; + _refreshingAll || (_lastScroll = scroll); + + if (recordVelocity) { + if (_lastScrollTime && !_pointerIsDown && time - _lastScrollTime > 200) { + _lastScrollTime = 0; + + _dispatch("scrollEnd"); + } + + _time2 = _time1; + _time1 = time; + } + + if (_direction < 0) { + _i = l; + + while (_i-- > 0) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + + _direction = 1; + } else { + for (_i = 0; _i < l; _i++) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + } + + ScrollTrigger$1.isUpdating = false; + } + + _rafID = 0; + }, + _propNamesToCopy = [_left, _top, _bottom, _right, _margin + _Bottom, _margin + _Right, _margin + _Top, _margin + _Left, "display", "flexShrink", "float", "zIndex", "gridColumnStart", "gridColumnEnd", "gridRowStart", "gridRowEnd", "gridArea", "justifySelf", "alignSelf", "placeSelf", "order"], + _stateProps = _propNamesToCopy.concat([_width, _height, "boxSizing", "max" + _Width, "max" + _Height, "position", _margin, _padding, _padding + _Top, _padding + _Right, _padding + _Bottom, _padding + _Left]), + _swapPinOut = function _swapPinOut(pin, spacer, state) { + _setState(state); + + var cache = pin._gsap; + + if (cache.spacerIsNative) { + _setState(cache.spacerState); + } else if (pin._gsap.swappedIn) { + var parent = spacer.parentNode; + + if (parent) { + parent.insertBefore(pin, spacer); + parent.removeChild(spacer); + } + } + + pin._gsap.swappedIn = false; + }, + _swapPinIn = function _swapPinIn(pin, spacer, cs, spacerState) { + if (!pin._gsap.swappedIn) { + var i = _propNamesToCopy.length, + spacerStyle = spacer.style, + pinStyle = pin.style, + p; + + while (i--) { + p = _propNamesToCopy[i]; + spacerStyle[p] = cs[p]; + } + + spacerStyle.position = cs.position === "absolute" ? "absolute" : "relative"; + cs.display === "inline" && (spacerStyle.display = "inline-block"); + pinStyle[_bottom] = pinStyle[_right] = "auto"; + spacerStyle.flexBasis = cs.flexBasis || "auto"; + spacerStyle.overflow = "visible"; + spacerStyle.boxSizing = "border-box"; + spacerStyle[_width] = _getSize(pin, _horizontal) + _px; + spacerStyle[_height] = _getSize(pin, _vertical) + _px; + spacerStyle[_padding] = pinStyle[_margin] = pinStyle[_top] = pinStyle[_left] = "0"; + + _setState(spacerState); + + pinStyle[_width] = pinStyle["max" + _Width] = cs[_width]; + pinStyle[_height] = pinStyle["max" + _Height] = cs[_height]; + pinStyle[_padding] = cs[_padding]; + + if (pin.parentNode !== spacer) { + pin.parentNode.insertBefore(spacer, pin); + spacer.appendChild(pin); + } + + pin._gsap.swappedIn = true; + } + }, + _capsExp = /([A-Z])/g, + _setState = function _setState(state) { + if (state) { + var style = state.t.style, + l = state.length, + i = 0, + p, + value; + (state.t._gsap || gsap$1.core.getCache(state.t)).uncache = 1; + + for (; i < l; i += 2) { + value = state[i + 1]; + p = state[i]; + + if (value) { + style[p] = value; + } else if (style[p]) { + style.removeProperty(p.replace(_capsExp, "-$1").toLowerCase()); + } + } + } + }, + _getState = function _getState(element) { + var l = _stateProps.length, + style = element.style, + state = [], + i = 0; + + for (; i < l; i++) { + state.push(_stateProps[i], style[_stateProps[i]]); + } + + state.t = element; + return state; + }, + _copyState = function _copyState(state, override, omitOffsets) { + var result = [], + l = state.length, + i = omitOffsets ? 8 : 0, + p; + + for (; i < l; i += 2) { + p = state[i]; + result.push(p, p in override ? override[p] : state[i + 1]); + } + + result.t = state.t; + return result; + }, + _winOffsets = { + left: 0, + top: 0 + }, + _parsePosition = function _parsePosition(value, trigger, scrollerSize, direction, scroll, marker, markerScroller, self, scrollerBounds, borderWidth, useFixedPosition, scrollerMax, containerAnimation, clampZeroProp) { + _isFunction(value) && (value = value(self)); + + if (_isString(value) && value.substr(0, 3) === "max") { + value = scrollerMax + (value.charAt(4) === "=" ? _offsetToPx("0" + value.substr(3), scrollerSize) : 0); + } + + var time = containerAnimation ? containerAnimation.time() : 0, + p1, + p2, + element; + containerAnimation && containerAnimation.seek(0); + isNaN(value) || (value = +value); + + if (!_isNumber(value)) { + _isFunction(trigger) && (trigger = trigger(self)); + var offsets = (value || "0").split(" "), + bounds, + localOffset, + globalOffset, + display; + element = _getTarget(trigger, self) || _body$1; + bounds = _getBounds(element) || {}; + + if ((!bounds || !bounds.left && !bounds.top) && _getComputedStyle(element).display === "none") { + display = element.style.display; + element.style.display = "block"; + bounds = _getBounds(element); + display ? element.style.display = display : element.style.removeProperty("display"); + } + + localOffset = _offsetToPx(offsets[0], bounds[direction.d]); + globalOffset = _offsetToPx(offsets[1] || "0", scrollerSize); + value = bounds[direction.p] - scrollerBounds[direction.p] - borderWidth + localOffset + scroll - globalOffset; + markerScroller && _positionMarker(markerScroller, globalOffset, direction, scrollerSize - globalOffset < 20 || markerScroller._isStart && globalOffset > 20); + scrollerSize -= scrollerSize - globalOffset; + } else { + containerAnimation && (value = gsap$1.utils.mapRange(containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, 0, scrollerMax, value)); + markerScroller && _positionMarker(markerScroller, scrollerSize, direction, true); + } + + if (clampZeroProp) { + self[clampZeroProp] = value || -0.001; + value < 0 && (value = 0); + } + + if (marker) { + var position = value + scrollerSize, + isStart = marker._isStart; + p1 = "scroll" + direction.d2; + + _positionMarker(marker, position, direction, isStart && position > 20 || !isStart && (useFixedPosition ? Math.max(_body$1[p1], _docEl$1[p1]) : marker.parentNode[p1]) <= position + 1); + + if (useFixedPosition) { + scrollerBounds = _getBounds(markerScroller); + useFixedPosition && (marker.style[direction.op.p] = scrollerBounds[direction.op.p] - direction.op.m - marker._offset + _px); + } + } + + if (containerAnimation && element) { + p1 = _getBounds(element); + containerAnimation.seek(scrollerMax); + p2 = _getBounds(element); + containerAnimation._caScrollDist = p1[direction.p] - p2[direction.p]; + value = value / containerAnimation._caScrollDist * scrollerMax; + } + + containerAnimation && containerAnimation.seek(time); + return containerAnimation ? value : Math.round(value); + }, + _prefixExp = /(webkit|moz|length|cssText|inset)/i, + _reparent = function _reparent(element, parent, top, left) { + if (element.parentNode !== parent) { + var style = element.style, + p, + cs; + + if (parent === _body$1) { + element._stOrig = style.cssText; + cs = _getComputedStyle(element); + + for (p in cs) { + if (!+p && !_prefixExp.test(p) && cs[p] && typeof style[p] === "string" && p !== "0") { + style[p] = cs[p]; + } + } + + style.top = top; + style.left = left; + } else { + style.cssText = element._stOrig; + } + + gsap$1.core.getCache(element).uncache = 1; + parent.appendChild(element); + } + }, + _interruptionTracker = function _interruptionTracker(getValueFunc, initialValue, onInterrupt) { + var last1 = initialValue, + last2 = last1; + return function (value) { + var current = Math.round(getValueFunc()); + + if (current !== last1 && current !== last2 && Math.abs(current - last1) > 3 && Math.abs(current - last2) > 3) { + value = current; + onInterrupt && onInterrupt(); + } + + last2 = last1; + last1 = Math.round(value); + return last1; + }; + }, + _shiftMarker = function _shiftMarker(marker, direction, value) { + var vars = {}; + vars[direction.p] = "+=" + value; + gsap$1.set(marker, vars); + }, + _getTweenCreator = function _getTweenCreator(scroller, direction) { + var getScroll = _getScrollFunc(scroller, direction), + prop = "_scroll" + direction.p2, + getTween = function getTween(scrollTo, vars, initialValue, change1, change2) { + var tween = getTween.tween, + onComplete = vars.onComplete, + modifiers = {}; + initialValue = initialValue || getScroll(); + + var checkForInterruption = _interruptionTracker(getScroll, initialValue, function () { + tween.kill(); + getTween.tween = 0; + }); + + change2 = change1 && change2 || 0; + change1 = change1 || scrollTo - initialValue; + tween && tween.kill(); + vars[prop] = scrollTo; + vars.inherit = false; + vars.modifiers = modifiers; + + modifiers[prop] = function () { + return checkForInterruption(initialValue + change1 * tween.ratio + change2 * tween.ratio * tween.ratio); + }; + + vars.onUpdate = function () { + _scrollers.cache++; + getTween.tween && _updateAll(); + }; + + vars.onComplete = function () { + getTween.tween = 0; + onComplete && onComplete.call(tween); + }; + + tween = getTween.tween = gsap$1.to(scroller, vars); + return tween; + }; + + scroller[prop] = getScroll; + + getScroll.wheelHandler = function () { + return getTween.tween && getTween.tween.kill() && (getTween.tween = 0); + }; + + _addListener$1(scroller, "wheel", getScroll.wheelHandler); + + ScrollTrigger$1.isTouch && _addListener$1(scroller, "touchmove", getScroll.wheelHandler); + return getTween; + }; + + var ScrollTrigger$1 = function () { + function ScrollTrigger(vars, animation) { + _coreInitted$1 || ScrollTrigger.register(gsap$1) || console.warn("Please gsap.registerPlugin(ScrollTrigger)"); + + _context$1(this); + + this.init(vars, animation); + } + + var _proto = ScrollTrigger.prototype; + + _proto.init = function init(vars, animation) { + this.progress = this.start = 0; + this.vars && this.kill(true, true); + + if (!_enabled) { + this.update = this.refresh = this.kill = _passThrough; + return; + } + + vars = _setDefaults(_isString(vars) || _isNumber(vars) || vars.nodeType ? { + trigger: vars + } : vars, _defaults); + + var _vars = vars, + onUpdate = _vars.onUpdate, + toggleClass = _vars.toggleClass, + id = _vars.id, + onToggle = _vars.onToggle, + onRefresh = _vars.onRefresh, + scrub = _vars.scrub, + trigger = _vars.trigger, + pin = _vars.pin, + pinSpacing = _vars.pinSpacing, + invalidateOnRefresh = _vars.invalidateOnRefresh, + anticipatePin = _vars.anticipatePin, + onScrubComplete = _vars.onScrubComplete, + onSnapComplete = _vars.onSnapComplete, + once = _vars.once, + snap = _vars.snap, + pinReparent = _vars.pinReparent, + pinSpacer = _vars.pinSpacer, + containerAnimation = _vars.containerAnimation, + fastScrollEnd = _vars.fastScrollEnd, + preventOverlaps = _vars.preventOverlaps, + direction = vars.horizontal || vars.containerAnimation && vars.horizontal !== false ? _horizontal : _vertical, + isToggle = !scrub && scrub !== 0, + scroller = _getTarget(vars.scroller || _win$1), + scrollerCache = gsap$1.core.getCache(scroller), + isViewport = _isViewport$1(scroller), + useFixedPosition = ("pinType" in vars ? vars.pinType : _getProxyProp(scroller, "pinType") || isViewport && "fixed") === "fixed", + callbacks = [vars.onEnter, vars.onLeave, vars.onEnterBack, vars.onLeaveBack], + toggleActions = isToggle && vars.toggleActions.split(" "), + markers = "markers" in vars ? vars.markers : _defaults.markers, + borderWidth = isViewport ? 0 : parseFloat(_getComputedStyle(scroller)["border" + direction.p2 + _Width]) || 0, + self = this, + onRefreshInit = vars.onRefreshInit && function () { + return vars.onRefreshInit(self); + }, + getScrollerSize = _getSizeFunc(scroller, isViewport, direction), + getScrollerOffsets = _getOffsetsFunc(scroller, isViewport), + lastSnap = 0, + lastRefresh = 0, + prevProgress = 0, + scrollFunc = _getScrollFunc(scroller, direction), + tweenTo, + pinCache, + snapFunc, + scroll1, + scroll2, + start, + end, + markerStart, + markerEnd, + markerStartTrigger, + markerEndTrigger, + markerVars, + executingOnRefresh, + change, + pinOriginalState, + pinActiveState, + pinState, + spacer, + offset, + pinGetter, + pinSetter, + pinStart, + pinChange, + spacingStart, + spacerState, + markerStartSetter, + pinMoves, + markerEndSetter, + cs, + snap1, + snap2, + scrubTween, + scrubSmooth, + snapDurClamp, + snapDelayedCall, + prevScroll, + prevAnimProgress, + caMarkerSetter, + customRevertReturn; + + self._startClamp = self._endClamp = false; + self._dir = direction; + anticipatePin *= 45; + self.scroller = scroller; + self.scroll = containerAnimation ? containerAnimation.time.bind(containerAnimation) : scrollFunc; + scroll1 = scrollFunc(); + self.vars = vars; + animation = animation || vars.animation; + + if ("refreshPriority" in vars) { + _sort = 1; + vars.refreshPriority === -9999 && (_primary = self); + } + + scrollerCache.tweenScroll = scrollerCache.tweenScroll || { + top: _getTweenCreator(scroller, _vertical), + left: _getTweenCreator(scroller, _horizontal) + }; + self.tweenTo = tweenTo = scrollerCache.tweenScroll[direction.p]; + + self.scrubDuration = function (value) { + scrubSmooth = _isNumber(value) && value; + + if (!scrubSmooth) { + scrubTween && scrubTween.progress(1).kill(); + scrubTween = 0; + } else { + scrubTween ? scrubTween.duration(value) : scrubTween = gsap$1.to(animation, { + ease: "expo", + totalProgress: "+=0", + inherit: false, + duration: scrubSmooth, + paused: true, + onComplete: function onComplete() { + return onScrubComplete && onScrubComplete(self); + } + }); + } + }; + + if (animation) { + animation.vars.lazy = false; + animation._initted && !self.isReverted || animation.vars.immediateRender !== false && vars.immediateRender !== false && animation.duration() && animation.render(0, true, true); + self.animation = animation.pause(); + animation.scrollTrigger = self; + self.scrubDuration(scrub); + snap1 = 0; + id || (id = animation.vars.id); + } + + if (snap) { + if (!_isObject(snap) || snap.push) { + snap = { + snapTo: snap + }; + } + + "scrollBehavior" in _body$1.style && gsap$1.set(isViewport ? [_body$1, _docEl$1] : scroller, { + scrollBehavior: "auto" + }); + + _scrollers.forEach(function (o) { + return _isFunction(o) && o.target === (isViewport ? _doc$1.scrollingElement || _docEl$1 : scroller) && (o.smooth = false); + }); + + snapFunc = _isFunction(snap.snapTo) ? snap.snapTo : snap.snapTo === "labels" ? _getClosestLabel(animation) : snap.snapTo === "labelsDirectional" ? _getLabelAtDirection(animation) : snap.directional !== false ? function (value, st) { + return _snapDirectional(snap.snapTo)(value, _getTime$1() - lastRefresh < 500 ? 0 : st.direction); + } : gsap$1.utils.snap(snap.snapTo); + snapDurClamp = snap.duration || { + min: 0.1, + max: 2 + }; + snapDurClamp = _isObject(snapDurClamp) ? _clamp$1(snapDurClamp.min, snapDurClamp.max) : _clamp$1(snapDurClamp, snapDurClamp); + snapDelayedCall = gsap$1.delayedCall(snap.delay || scrubSmooth / 2 || 0.1, function () { + var scroll = scrollFunc(), + refreshedRecently = _getTime$1() - lastRefresh < 500, + tween = tweenTo.tween; + + if ((refreshedRecently || Math.abs(self.getVelocity()) < 10) && !tween && !_pointerIsDown && lastSnap !== scroll) { + var progress = (scroll - start) / change, + totalProgress = animation && !isToggle ? animation.totalProgress() : progress, + velocity = refreshedRecently ? 0 : (totalProgress - snap2) / (_getTime$1() - _time2) * 1000 || 0, + change1 = gsap$1.utils.clamp(-progress, 1 - progress, _abs(velocity / 2) * velocity / 0.185), + naturalEnd = progress + (snap.inertia === false ? 0 : change1), + endValue, + endScroll, + _snap = snap, + onStart = _snap.onStart, + _onInterrupt = _snap.onInterrupt, + _onComplete = _snap.onComplete; + endValue = snapFunc(naturalEnd, self); + _isNumber(endValue) || (endValue = naturalEnd); + endScroll = Math.max(0, Math.round(start + endValue * change)); + + if (scroll <= end && scroll >= start && endScroll !== scroll) { + if (tween && !tween._initted && tween.data <= _abs(endScroll - scroll)) { + return; + } + + if (snap.inertia === false) { + change1 = endValue - progress; + } + + tweenTo(endScroll, { + duration: snapDurClamp(_abs(Math.max(_abs(naturalEnd - totalProgress), _abs(endValue - totalProgress)) * 0.185 / velocity / 0.05 || 0)), + ease: snap.ease || "power3", + data: _abs(endScroll - scroll), + onInterrupt: function onInterrupt() { + return snapDelayedCall.restart(true) && _onInterrupt && _onInterrupt(self); + }, + onComplete: function onComplete() { + self.update(); + lastSnap = scrollFunc(); + + if (animation && !isToggle) { + scrubTween ? scrubTween.resetTo("totalProgress", endValue, animation._tTime / animation._tDur) : animation.progress(endValue); + } + + snap1 = snap2 = animation && !isToggle ? animation.totalProgress() : self.progress; + onSnapComplete && onSnapComplete(self); + _onComplete && _onComplete(self); + } + }, scroll, change1 * change, endScroll - scroll - change1 * change); + onStart && onStart(self, tweenTo.tween); + } + } else if (self.isActive && lastSnap !== scroll) { + snapDelayedCall.restart(true); + } + }).pause(); + } + + id && (_ids[id] = self); + trigger = self.trigger = _getTarget(trigger || pin !== true && pin); + customRevertReturn = trigger && trigger._gsap && trigger._gsap.stRevert; + customRevertReturn && (customRevertReturn = customRevertReturn(self)); + pin = pin === true ? trigger : _getTarget(pin); + _isString(toggleClass) && (toggleClass = { + targets: trigger, + className: toggleClass + }); + + if (pin) { + pinSpacing === false || pinSpacing === _margin || (pinSpacing = !pinSpacing && pin.parentNode && pin.parentNode.style && _getComputedStyle(pin.parentNode).display === "flex" ? false : _padding); + self.pin = pin; + pinCache = gsap$1.core.getCache(pin); + + if (!pinCache.spacer) { + if (pinSpacer) { + pinSpacer = _getTarget(pinSpacer); + pinSpacer && !pinSpacer.nodeType && (pinSpacer = pinSpacer.current || pinSpacer.nativeElement); + pinCache.spacerIsNative = !!pinSpacer; + pinSpacer && (pinCache.spacerState = _getState(pinSpacer)); + } + + pinCache.spacer = spacer = pinSpacer || _doc$1.createElement("div"); + spacer.classList.add("pin-spacer"); + id && spacer.classList.add("pin-spacer-" + id); + pinCache.pinState = pinOriginalState = _getState(pin); + } else { + pinOriginalState = pinCache.pinState; + } + + vars.force3D !== false && gsap$1.set(pin, { + force3D: true + }); + self.spacer = spacer = pinCache.spacer; + cs = _getComputedStyle(pin); + spacingStart = cs[pinSpacing + direction.os2]; + pinGetter = gsap$1.getProperty(pin); + pinSetter = gsap$1.quickSetter(pin, direction.a, _px); + + _swapPinIn(pin, spacer, cs); + + pinState = _getState(pin); + } + + if (markers) { + markerVars = _isObject(markers) ? _setDefaults(markers, _markerDefaults) : _markerDefaults; + markerStartTrigger = _createMarker("scroller-start", id, scroller, direction, markerVars, 0); + markerEndTrigger = _createMarker("scroller-end", id, scroller, direction, markerVars, 0, markerStartTrigger); + offset = markerStartTrigger["offset" + direction.op.d2]; + + var content = _getTarget(_getProxyProp(scroller, "content") || scroller); + + markerStart = this.markerStart = _createMarker("start", id, content, direction, markerVars, offset, 0, containerAnimation); + markerEnd = this.markerEnd = _createMarker("end", id, content, direction, markerVars, offset, 0, containerAnimation); + containerAnimation && (caMarkerSetter = gsap$1.quickSetter([markerStart, markerEnd], direction.a, _px)); + + if (!useFixedPosition && !(_proxies.length && _getProxyProp(scroller, "fixedMarkers") === true)) { + _makePositionable(isViewport ? _body$1 : scroller); + + gsap$1.set([markerStartTrigger, markerEndTrigger], { + force3D: true + }); + markerStartSetter = gsap$1.quickSetter(markerStartTrigger, direction.a, _px); + markerEndSetter = gsap$1.quickSetter(markerEndTrigger, direction.a, _px); + } + } + + if (containerAnimation) { + var oldOnUpdate = containerAnimation.vars.onUpdate, + oldParams = containerAnimation.vars.onUpdateParams; + containerAnimation.eventCallback("onUpdate", function () { + self.update(0, 0, 1); + oldOnUpdate && oldOnUpdate.apply(containerAnimation, oldParams || []); + }); + } + + self.previous = function () { + return _triggers[_triggers.indexOf(self) - 1]; + }; + + self.next = function () { + return _triggers[_triggers.indexOf(self) + 1]; + }; + + self.revert = function (revert, temp) { + if (!temp) { + return self.kill(true); + } + + var r = revert !== false || !self.enabled, + prevRefreshing = _refreshing; + + if (r !== self.isReverted) { + if (r) { + prevScroll = Math.max(scrollFunc(), self.scroll.rec || 0); + prevProgress = self.progress; + prevAnimProgress = animation && animation.progress(); + } + + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(function (m) { + return m.style.display = r ? "none" : "block"; + }); + + if (r) { + _refreshing = self; + self.update(r); + } + + if (pin && (!pinReparent || !self.isActive)) { + if (r) { + _swapPinOut(pin, spacer, pinOriginalState); + } else { + _swapPinIn(pin, spacer, _getComputedStyle(pin), spacerState); + } + } + + r || self.update(r); + _refreshing = prevRefreshing; + self.isReverted = r; + } + }; + + self.refresh = function (soft, force, position, pinOffset) { + if ((_refreshing || !self.enabled) && !force) { + return; + } + + if (pin && soft && _lastScrollTime) { + _addListener$1(ScrollTrigger, "scrollEnd", _softRefresh); + + return; + } + + !_refreshingAll && onRefreshInit && onRefreshInit(self); + _refreshing = self; + + if (tweenTo.tween && !position) { + tweenTo.tween.kill(); + tweenTo.tween = 0; + } + + scrubTween && scrubTween.pause(); + invalidateOnRefresh && animation && animation.revert({ + kill: false + }).invalidate(); + self.isReverted || self.revert(true, true); + self._subPinOffset = false; + + var size = getScrollerSize(), + scrollerBounds = getScrollerOffsets(), + max = containerAnimation ? containerAnimation.duration() : _maxScroll(scroller, direction), + isFirstRefresh = change <= 0.01, + offset = 0, + otherPinOffset = pinOffset || 0, + parsedEnd = _isObject(position) ? position.end : vars.end, + parsedEndTrigger = vars.endTrigger || trigger, + parsedStart = _isObject(position) ? position.start : vars.start || (vars.start === 0 || !trigger ? 0 : pin ? "0 0" : "0 100%"), + pinnedContainer = self.pinnedContainer = vars.pinnedContainer && _getTarget(vars.pinnedContainer, self), + triggerIndex = trigger && Math.max(0, _triggers.indexOf(self)) || 0, + i = triggerIndex, + cs, + bounds, + scroll, + isVertical, + override, + curTrigger, + curPin, + oppositeScroll, + initted, + revertedPins, + forcedOverflow, + markerStartOffset, + markerEndOffset; + + if (markers && _isObject(position)) { + markerStartOffset = gsap$1.getProperty(markerStartTrigger, direction.p); + markerEndOffset = gsap$1.getProperty(markerEndTrigger, direction.p); + } + + while (i-- > 0) { + curTrigger = _triggers[i]; + curTrigger.end || curTrigger.refresh(0, 1) || (_refreshing = self); + curPin = curTrigger.pin; + + if (curPin && (curPin === trigger || curPin === pin || curPin === pinnedContainer) && !curTrigger.isReverted) { + revertedPins || (revertedPins = []); + revertedPins.unshift(curTrigger); + curTrigger.revert(true, true); + } + + if (curTrigger !== _triggers[i]) { + triggerIndex--; + i--; + } + } + + _isFunction(parsedStart) && (parsedStart = parsedStart(self)); + parsedStart = _parseClamp(parsedStart, "start", self); + start = _parsePosition(parsedStart, trigger, size, direction, scrollFunc(), markerStart, markerStartTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._startClamp && "_startClamp") || (pin ? -0.001 : 0); + _isFunction(parsedEnd) && (parsedEnd = parsedEnd(self)); + + if (_isString(parsedEnd) && !parsedEnd.indexOf("+=")) { + if (~parsedEnd.indexOf(" ")) { + parsedEnd = (_isString(parsedStart) ? parsedStart.split(" ")[0] : "") + parsedEnd; + } else { + offset = _offsetToPx(parsedEnd.substr(2), size); + parsedEnd = _isString(parsedStart) ? parsedStart : (containerAnimation ? gsap$1.utils.mapRange(0, containerAnimation.duration(), containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, start) : start) + offset; + parsedEndTrigger = trigger; + } + } + + parsedEnd = _parseClamp(parsedEnd, "end", self); + end = Math.max(start, _parsePosition(parsedEnd || (parsedEndTrigger ? "100% 0" : max), parsedEndTrigger, size, direction, scrollFunc() + offset, markerEnd, markerEndTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._endClamp && "_endClamp")) || -0.001; + offset = 0; + i = triggerIndex; + + while (i--) { + curTrigger = _triggers[i]; + curPin = curTrigger.pin; + + if (curPin && curTrigger.start - curTrigger._pinPush <= start && !containerAnimation && curTrigger.end > 0) { + cs = curTrigger.end - (self._startClamp ? Math.max(0, curTrigger.start) : curTrigger.start); + + if ((curPin === trigger && curTrigger.start - curTrigger._pinPush < start || curPin === pinnedContainer) && isNaN(parsedStart)) { + offset += cs * (1 - curTrigger.progress); + } + + curPin === pin && (otherPinOffset += cs); + } + } + + start += offset; + end += offset; + self._startClamp && (self._startClamp += offset); + + if (self._endClamp && !_refreshingAll) { + self._endClamp = end || -0.001; + end = Math.min(end, _maxScroll(scroller, direction)); + } + + change = end - start || (start -= 0.01) && 0.001; + + if (isFirstRefresh) { + prevProgress = gsap$1.utils.clamp(0, 1, gsap$1.utils.normalize(start, end, prevScroll)); + } + + self._pinPush = otherPinOffset; + + if (markerStart && offset) { + cs = {}; + cs[direction.a] = "+=" + offset; + pinnedContainer && (cs[direction.p] = "-=" + scrollFunc()); + gsap$1.set([markerStart, markerEnd], cs); + } + + if (pin && !(_clampingMax && self.end >= _maxScroll(scroller, direction))) { + cs = _getComputedStyle(pin); + isVertical = direction === _vertical; + scroll = scrollFunc(); + pinStart = parseFloat(pinGetter(direction.a)) + otherPinOffset; + + if (!max && end > 1) { + forcedOverflow = (isViewport ? _doc$1.scrollingElement || _docEl$1 : scroller).style; + forcedOverflow = { + style: forcedOverflow, + value: forcedOverflow["overflow" + direction.a.toUpperCase()] + }; + + if (isViewport && _getComputedStyle(_body$1)["overflow" + direction.a.toUpperCase()] !== "scroll") { + forcedOverflow.style["overflow" + direction.a.toUpperCase()] = "scroll"; + } + } + + _swapPinIn(pin, spacer, cs); + + pinState = _getState(pin); + bounds = _getBounds(pin, true); + oppositeScroll = useFixedPosition && _getScrollFunc(scroller, isVertical ? _horizontal : _vertical)(); + + if (pinSpacing) { + spacerState = [pinSpacing + direction.os2, change + otherPinOffset + _px]; + spacerState.t = spacer; + i = pinSpacing === _padding ? _getSize(pin, direction) + change + otherPinOffset : 0; + + if (i) { + spacerState.push(direction.d, i + _px); + spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + + _setState(spacerState); + + if (pinnedContainer) { + _triggers.forEach(function (t) { + if (t.pin === pinnedContainer && t.vars.pinSpacing !== false) { + t._subPinOffset = true; + } + }); + } + + useFixedPosition && scrollFunc(prevScroll); + } else { + i = _getSize(pin, direction); + i && spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + + if (useFixedPosition) { + override = { + top: bounds.top + (isVertical ? scroll - start : oppositeScroll) + _px, + left: bounds.left + (isVertical ? oppositeScroll : scroll - start) + _px, + boxSizing: "border-box", + position: "fixed" + }; + override[_width] = override["max" + _Width] = Math.ceil(bounds.width) + _px; + override[_height] = override["max" + _Height] = Math.ceil(bounds.height) + _px; + override[_margin] = override[_margin + _Top] = override[_margin + _Right] = override[_margin + _Bottom] = override[_margin + _Left] = "0"; + override[_padding] = cs[_padding]; + override[_padding + _Top] = cs[_padding + _Top]; + override[_padding + _Right] = cs[_padding + _Right]; + override[_padding + _Bottom] = cs[_padding + _Bottom]; + override[_padding + _Left] = cs[_padding + _Left]; + pinActiveState = _copyState(pinOriginalState, override, pinReparent); + _refreshingAll && scrollFunc(0); + } + + if (animation) { + initted = animation._initted; + + _suppressOverwrites(1); + + animation.render(animation.duration(), true, true); + pinChange = pinGetter(direction.a) - pinStart + change + otherPinOffset; + pinMoves = Math.abs(change - pinChange) > 1; + useFixedPosition && pinMoves && pinActiveState.splice(pinActiveState.length - 2, 2); + animation.render(0, true, true); + initted || animation.invalidate(true); + animation.parent || animation.totalTime(animation.totalTime()); + + _suppressOverwrites(0); + } else { + pinChange = change; + } + + forcedOverflow && (forcedOverflow.value ? forcedOverflow.style["overflow" + direction.a.toUpperCase()] = forcedOverflow.value : forcedOverflow.style.removeProperty("overflow-" + direction.a)); + } else if (trigger && scrollFunc() && !containerAnimation) { + bounds = trigger.parentNode; + + while (bounds && bounds !== _body$1) { + if (bounds._pinOffset) { + start -= bounds._pinOffset; + end -= bounds._pinOffset; + } + + bounds = bounds.parentNode; + } + } + + revertedPins && revertedPins.forEach(function (t) { + return t.revert(false, true); + }); + self.start = start; + self.end = end; + scroll1 = scroll2 = _refreshingAll ? prevScroll : scrollFunc(); + + if (!containerAnimation && !_refreshingAll) { + scroll1 < prevScroll && scrollFunc(prevScroll); + self.scroll.rec = 0; + } + + self.revert(false, true); + lastRefresh = _getTime$1(); + + if (snapDelayedCall) { + lastSnap = -1; + snapDelayedCall.restart(true); + } + + _refreshing = 0; + animation && isToggle && (animation._initted || prevAnimProgress) && animation.progress() !== prevAnimProgress && animation.progress(prevAnimProgress || 0, true).render(animation.time(), true, true); + + if (isFirstRefresh || prevProgress !== self.progress || containerAnimation || invalidateOnRefresh || animation && !animation._initted) { + animation && !isToggle && animation.totalProgress(containerAnimation && start < -0.001 && !prevProgress ? gsap$1.utils.normalize(start, end, 0) : prevProgress, true); + self.progress = isFirstRefresh || (scroll1 - start) / change === prevProgress ? 0 : prevProgress; + } + + pin && pinSpacing && (spacer._pinOffset = Math.round(self.progress * pinChange)); + scrubTween && scrubTween.invalidate(); + + if (!isNaN(markerStartOffset)) { + markerStartOffset -= gsap$1.getProperty(markerStartTrigger, direction.p); + markerEndOffset -= gsap$1.getProperty(markerEndTrigger, direction.p); + + _shiftMarker(markerStartTrigger, direction, markerStartOffset); + + _shiftMarker(markerStart, direction, markerStartOffset - (pinOffset || 0)); + + _shiftMarker(markerEndTrigger, direction, markerEndOffset); + + _shiftMarker(markerEnd, direction, markerEndOffset - (pinOffset || 0)); + } + + isFirstRefresh && !_refreshingAll && self.update(); + + if (onRefresh && !_refreshingAll && !executingOnRefresh) { + executingOnRefresh = true; + onRefresh(self); + executingOnRefresh = false; + } + }; + + self.getVelocity = function () { + return (scrollFunc() - scroll2) / (_getTime$1() - _time2) * 1000 || 0; + }; + + self.endAnimation = function () { + _endAnimation(self.callbackAnimation); + + if (animation) { + scrubTween ? scrubTween.progress(1) : !animation.paused() ? _endAnimation(animation, animation.reversed()) : isToggle || _endAnimation(animation, self.direction < 0, 1); + } + }; + + self.labelToScroll = function (label) { + return animation && animation.labels && (start || self.refresh() || start) + animation.labels[label] / animation.duration() * change || 0; + }; + + self.getTrailing = function (name) { + var i = _triggers.indexOf(self), + a = self.direction > 0 ? _triggers.slice(0, i).reverse() : _triggers.slice(i + 1); + + return (_isString(name) ? a.filter(function (t) { + return t.vars.preventOverlaps === name; + }) : a).filter(function (t) { + return self.direction > 0 ? t.end <= start : t.start >= end; + }); + }; + + self.update = function (reset, recordVelocity, forceFake) { + if (containerAnimation && !forceFake && !reset) { + return; + } + + var scroll = _refreshingAll === true ? prevScroll : self.scroll(), + p = reset ? 0 : (scroll - start) / change, + clipped = p < 0 ? 0 : p > 1 ? 1 : p || 0, + prevProgress = self.progress, + isActive, + wasActive, + toggleState, + action, + stateChanged, + toggled, + isAtMax, + isTakingAction; + + if (recordVelocity) { + scroll2 = scroll1; + scroll1 = containerAnimation ? scrollFunc() : scroll; + + if (snap) { + snap2 = snap1; + snap1 = animation && !isToggle ? animation.totalProgress() : clipped; + } + } + + if (anticipatePin && pin && !_refreshing && !_startup$1 && _lastScrollTime) { + if (!clipped && start < scroll + (scroll - scroll2) / (_getTime$1() - _time2) * anticipatePin) { + clipped = 0.0001; + } else if (clipped === 1 && end > scroll + (scroll - scroll2) / (_getTime$1() - _time2) * anticipatePin) { + clipped = 0.9999; + } + } + + if (clipped !== prevProgress && self.enabled) { + isActive = self.isActive = !!clipped && clipped < 1; + wasActive = !!prevProgress && prevProgress < 1; + toggled = isActive !== wasActive; + stateChanged = toggled || !!clipped !== !!prevProgress; + self.direction = clipped > prevProgress ? 1 : -1; + self.progress = clipped; + + if (stateChanged && !_refreshing) { + toggleState = clipped && !prevProgress ? 0 : clipped === 1 ? 1 : prevProgress === 1 ? 2 : 3; + + if (isToggle) { + action = !toggled && toggleActions[toggleState + 1] !== "none" && toggleActions[toggleState + 1] || toggleActions[toggleState]; + isTakingAction = animation && (action === "complete" || action === "reset" || action in animation); + } + } + + preventOverlaps && (toggled || isTakingAction) && (isTakingAction || scrub || !animation) && (_isFunction(preventOverlaps) ? preventOverlaps(self) : self.getTrailing(preventOverlaps).forEach(function (t) { + return t.endAnimation(); + })); + + if (!isToggle) { + if (scrubTween && !_refreshing && !_startup$1) { + scrubTween._dp._time - scrubTween._start !== scrubTween._time && scrubTween.render(scrubTween._dp._time - scrubTween._start); + + if (scrubTween.resetTo) { + scrubTween.resetTo("totalProgress", clipped, animation._tTime / animation._tDur); + } else { + scrubTween.vars.totalProgress = clipped; + scrubTween.invalidate().restart(); + } + } else if (animation) { + animation.totalProgress(clipped, !!(_refreshing && (lastRefresh || reset))); + } + } + + if (pin) { + reset && pinSpacing && (spacer.style[pinSpacing + direction.os2] = spacingStart); + + if (!useFixedPosition) { + pinSetter(_round(pinStart + pinChange * clipped)); + } else if (stateChanged) { + isAtMax = !reset && clipped > prevProgress && end + 1 > scroll && scroll + 1 >= _maxScroll(scroller, direction); + + if (pinReparent) { + if (!reset && (isActive || isAtMax)) { + var bounds = _getBounds(pin, true), + _offset = scroll - start; + + _reparent(pin, _body$1, bounds.top + (direction === _vertical ? _offset : 0) + _px, bounds.left + (direction === _vertical ? 0 : _offset) + _px); + } else { + _reparent(pin, spacer); + } + } + + _setState(isActive || isAtMax ? pinActiveState : pinState); + + pinMoves && clipped < 1 && isActive || pinSetter(pinStart + (clipped === 1 && !isAtMax ? pinChange : 0)); + } + } + + snap && !tweenTo.tween && !_refreshing && !_startup$1 && snapDelayedCall.restart(true); + toggleClass && (toggled || once && clipped && (clipped < 1 || !_limitCallbacks)) && _toArray(toggleClass.targets).forEach(function (el) { + return el.classList[isActive || once ? "add" : "remove"](toggleClass.className); + }); + onUpdate && !isToggle && !reset && onUpdate(self); + + if (stateChanged && !_refreshing) { + if (isToggle) { + if (isTakingAction) { + if (action === "complete") { + animation.pause().totalProgress(1); + } else if (action === "reset") { + animation.restart(true).pause(); + } else if (action === "restart") { + animation.restart(true); + } else { + animation[action](); + } + } + + onUpdate && onUpdate(self); + } + + if (toggled || !_limitCallbacks) { + onToggle && toggled && _callback(self, onToggle); + callbacks[toggleState] && _callback(self, callbacks[toggleState]); + once && (clipped === 1 ? self.kill(false, 1) : callbacks[toggleState] = 0); + + if (!toggled) { + toggleState = clipped === 1 ? 1 : 3; + callbacks[toggleState] && _callback(self, callbacks[toggleState]); + } + } + + if (fastScrollEnd && !isActive && Math.abs(self.getVelocity()) > (_isNumber(fastScrollEnd) ? fastScrollEnd : 2500)) { + _endAnimation(self.callbackAnimation); + + scrubTween ? scrubTween.progress(1) : _endAnimation(animation, action === "reverse" ? 1 : !clipped, 1); + } + } else if (isToggle && onUpdate && !_refreshing) { + onUpdate(self); + } + } + + if (markerEndSetter) { + var n = containerAnimation ? scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0) : scroll; + markerStartSetter(n + (markerStartTrigger._isFlipped ? 1 : 0)); + markerEndSetter(n); + } + + caMarkerSetter && caMarkerSetter(-scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0)); + }; + + self.enable = function (reset, refresh) { + if (!self.enabled) { + self.enabled = true; + + _addListener$1(scroller, "resize", _onResize); + + isViewport || _addListener$1(scroller, "scroll", _onScroll$1); + onRefreshInit && _addListener$1(ScrollTrigger, "refreshInit", onRefreshInit); + + if (reset !== false) { + self.progress = prevProgress = 0; + scroll1 = scroll2 = lastSnap = scrollFunc(); + } + + refresh !== false && self.refresh(); + } + }; + + self.getTween = function (snap) { + return snap && tweenTo ? tweenTo.tween : scrubTween; + }; + + self.setPositions = function (newStart, newEnd, keepClamp, pinOffset) { + if (containerAnimation) { + var st = containerAnimation.scrollTrigger, + duration = containerAnimation.duration(), + _change = st.end - st.start; + + newStart = st.start + _change * newStart / duration; + newEnd = st.start + _change * newEnd / duration; + } + + self.refresh(false, false, { + start: _keepClamp(newStart, keepClamp && !!self._startClamp), + end: _keepClamp(newEnd, keepClamp && !!self._endClamp) + }, pinOffset); + self.update(); + }; + + self.adjustPinSpacing = function (amount) { + if (spacerState && amount) { + var i = spacerState.indexOf(direction.d) + 1; + spacerState[i] = parseFloat(spacerState[i]) + amount + _px; + spacerState[1] = parseFloat(spacerState[1]) + amount + _px; + + _setState(spacerState); + } + }; + + self.disable = function (reset, allowAnimation) { + if (self.enabled) { + reset !== false && self.revert(true, true); + self.enabled = self.isActive = false; + allowAnimation || scrubTween && scrubTween.pause(); + prevScroll = 0; + pinCache && (pinCache.uncache = 1); + onRefreshInit && _removeListener$1(ScrollTrigger, "refreshInit", onRefreshInit); + + if (snapDelayedCall) { + snapDelayedCall.pause(); + tweenTo.tween && tweenTo.tween.kill() && (tweenTo.tween = 0); + } + + if (!isViewport) { + var i = _triggers.length; + + while (i--) { + if (_triggers[i].scroller === scroller && _triggers[i] !== self) { + return; + } + } + + _removeListener$1(scroller, "resize", _onResize); + + isViewport || _removeListener$1(scroller, "scroll", _onScroll$1); + } + } + }; + + self.kill = function (revert, allowAnimation) { + self.disable(revert, allowAnimation); + scrubTween && !allowAnimation && scrubTween.kill(); + id && delete _ids[id]; + + var i = _triggers.indexOf(self); + + i >= 0 && _triggers.splice(i, 1); + i === _i && _direction > 0 && _i--; + i = 0; + + _triggers.forEach(function (t) { + return t.scroller === self.scroller && (i = 1); + }); + + i || _refreshingAll || (self.scroll.rec = 0); + + if (animation) { + animation.scrollTrigger = null; + revert && animation.revert({ + kill: false + }); + allowAnimation || animation.kill(); + } + + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(function (m) { + return m.parentNode && m.parentNode.removeChild(m); + }); + _primary === self && (_primary = 0); + + if (pin) { + pinCache && (pinCache.uncache = 1); + i = 0; + + _triggers.forEach(function (t) { + return t.pin === pin && i++; + }); + + i || (pinCache.spacer = 0); + } + + vars.onKill && vars.onKill(self); + }; + + _triggers.push(self); + + self.enable(false, false); + customRevertReturn && customRevertReturn(self); + + if (animation && animation.add && !change) { + var updateFunc = self.update; + + self.update = function () { + self.update = updateFunc; + _scrollers.cache++; + start || end || self.refresh(); + }; + + gsap$1.delayedCall(0.01, self.update); + change = 0.01; + start = end = 0; + } else { + self.refresh(); + } + + pin && _queueRefreshAll(); + }; + + ScrollTrigger.register = function register(core) { + if (!_coreInitted$1) { + gsap$1 = core || _getGSAP$1(); + _windowExists() && window.document && ScrollTrigger.enable(); + _coreInitted$1 = _enabled; + } + + return _coreInitted$1; + }; + + ScrollTrigger.defaults = function defaults(config) { + if (config) { + for (var p in config) { + _defaults[p] = config[p]; + } + } + + return _defaults; + }; + + ScrollTrigger.disable = function disable(reset, kill) { + _enabled = 0; + + _triggers.forEach(function (trigger) { + return trigger[kill ? "kill" : "disable"](reset); + }); + + _removeListener$1(_win$1, "wheel", _onScroll$1); + + _removeListener$1(_doc$1, "scroll", _onScroll$1); + + clearInterval(_syncInterval); + + _removeListener$1(_doc$1, "touchcancel", _passThrough); + + _removeListener$1(_body$1, "touchstart", _passThrough); + + _multiListener(_removeListener$1, _doc$1, "pointerdown,touchstart,mousedown", _pointerDownHandler); + + _multiListener(_removeListener$1, _doc$1, "pointerup,touchend,mouseup", _pointerUpHandler); + + _resizeDelay.kill(); + + _iterateAutoRefresh(_removeListener$1); + + for (var i = 0; i < _scrollers.length; i += 3) { + _wheelListener(_removeListener$1, _scrollers[i], _scrollers[i + 1]); + + _wheelListener(_removeListener$1, _scrollers[i], _scrollers[i + 2]); + } + }; + + ScrollTrigger.enable = function enable() { + _win$1 = window; + _doc$1 = document; + _docEl$1 = _doc$1.documentElement; + _body$1 = _doc$1.body; + + if (gsap$1) { + _toArray = gsap$1.utils.toArray; + _clamp$1 = gsap$1.utils.clamp; + _context$1 = gsap$1.core.context || _passThrough; + _suppressOverwrites = gsap$1.core.suppressOverwrites || _passThrough; + _scrollRestoration = _win$1.history.scrollRestoration || "auto"; + _lastScroll = _win$1.pageYOffset || 0; + gsap$1.core.globals("ScrollTrigger", ScrollTrigger); + + if (_body$1) { + _enabled = 1; + _div100vh = document.createElement("div"); + _div100vh.style.height = "100vh"; + _div100vh.style.position = "absolute"; + + _refresh100vh(); + + _rafBugFix(); + + Observer.register(gsap$1); + ScrollTrigger.isTouch = Observer.isTouch; + _fixIOSBug = Observer.isTouch && /(iPad|iPhone|iPod|Mac)/g.test(navigator.userAgent); + _ignoreMobileResize = Observer.isTouch === 1; + + _addListener$1(_win$1, "wheel", _onScroll$1); + + _root$1 = [_win$1, _doc$1, _docEl$1, _body$1]; + + if (gsap$1.matchMedia) { + ScrollTrigger.matchMedia = function (vars) { + var mm = gsap$1.matchMedia(), + p; + + for (p in vars) { + mm.add(p, vars[p]); + } + + return mm; + }; + + gsap$1.addEventListener("matchMediaInit", function () { + return _revertAll(); + }); + gsap$1.addEventListener("matchMediaRevert", function () { + return _revertRecorded(); + }); + gsap$1.addEventListener("matchMedia", function () { + _refreshAll(0, 1); + + _dispatch("matchMedia"); + }); + gsap$1.matchMedia().add("(orientation: portrait)", function () { + _setBaseDimensions(); + + return _setBaseDimensions; + }); + } else { + console.warn("Requires GSAP 3.11.0 or later"); + } + + _setBaseDimensions(); + + _addListener$1(_doc$1, "scroll", _onScroll$1); + + var bodyHasStyle = _body$1.hasAttribute("style"), + bodyStyle = _body$1.style, + border = bodyStyle.borderTopStyle, + AnimationProto = gsap$1.core.Animation.prototype, + bounds, + i; + + AnimationProto.revert || Object.defineProperty(AnimationProto, "revert", { + value: function value() { + return this.time(-0.01, true); + } + }); + bodyStyle.borderTopStyle = "solid"; + bounds = _getBounds(_body$1); + _vertical.m = Math.round(bounds.top + _vertical.sc()) || 0; + _horizontal.m = Math.round(bounds.left + _horizontal.sc()) || 0; + border ? bodyStyle.borderTopStyle = border : bodyStyle.removeProperty("border-top-style"); + + if (!bodyHasStyle) { + _body$1.setAttribute("style", ""); + + _body$1.removeAttribute("style"); + } + + _syncInterval = setInterval(_sync, 250); + gsap$1.delayedCall(0.5, function () { + return _startup$1 = 0; + }); + + _addListener$1(_doc$1, "touchcancel", _passThrough); + + _addListener$1(_body$1, "touchstart", _passThrough); + + _multiListener(_addListener$1, _doc$1, "pointerdown,touchstart,mousedown", _pointerDownHandler); + + _multiListener(_addListener$1, _doc$1, "pointerup,touchend,mouseup", _pointerUpHandler); + + _transformProp = gsap$1.utils.checkPrefix("transform"); + + _stateProps.push(_transformProp); + + _coreInitted$1 = _getTime$1(); + _resizeDelay = gsap$1.delayedCall(0.2, _refreshAll).pause(); + _autoRefresh = [_doc$1, "visibilitychange", function () { + var w = _win$1.innerWidth, + h = _win$1.innerHeight; + + if (_doc$1.hidden) { + _prevWidth = w; + _prevHeight = h; + } else if (_prevWidth !== w || _prevHeight !== h) { + _onResize(); + } + }, _doc$1, "DOMContentLoaded", _refreshAll, _win$1, "load", _refreshAll, _win$1, "resize", _onResize]; + + _iterateAutoRefresh(_addListener$1); + + _triggers.forEach(function (trigger) { + return trigger.enable(0, 1); + }); + + for (i = 0; i < _scrollers.length; i += 3) { + _wheelListener(_removeListener$1, _scrollers[i], _scrollers[i + 1]); + + _wheelListener(_removeListener$1, _scrollers[i], _scrollers[i + 2]); + } + } + } + }; + + ScrollTrigger.config = function config(vars) { + "limitCallbacks" in vars && (_limitCallbacks = !!vars.limitCallbacks); + var ms = vars.syncInterval; + ms && clearInterval(_syncInterval) || (_syncInterval = ms) && setInterval(_sync, ms); + "ignoreMobileResize" in vars && (_ignoreMobileResize = ScrollTrigger.isTouch === 1 && vars.ignoreMobileResize); + + if ("autoRefreshEvents" in vars) { + _iterateAutoRefresh(_removeListener$1) || _iterateAutoRefresh(_addListener$1, vars.autoRefreshEvents || "none"); + _ignoreResize = (vars.autoRefreshEvents + "").indexOf("resize") === -1; + } + }; + + ScrollTrigger.scrollerProxy = function scrollerProxy(target, vars) { + var t = _getTarget(target), + i = _scrollers.indexOf(t), + isViewport = _isViewport$1(t); + + if (~i) { + _scrollers.splice(i, isViewport ? 6 : 2); + } + + if (vars) { + isViewport ? _proxies.unshift(_win$1, vars, _body$1, vars, _docEl$1, vars) : _proxies.unshift(t, vars); + } + }; + + ScrollTrigger.clearMatchMedia = function clearMatchMedia(query) { + _triggers.forEach(function (t) { + return t._ctx && t._ctx.query === query && t._ctx.kill(true, true); + }); + }; + + ScrollTrigger.isInViewport = function isInViewport(element, ratio, horizontal) { + var bounds = (_isString(element) ? _getTarget(element) : element).getBoundingClientRect(), + offset = bounds[horizontal ? _width : _height] * ratio || 0; + return horizontal ? bounds.right - offset > 0 && bounds.left + offset < _win$1.innerWidth : bounds.bottom - offset > 0 && bounds.top + offset < _win$1.innerHeight; + }; + + ScrollTrigger.positionInViewport = function positionInViewport(element, referencePoint, horizontal) { + _isString(element) && (element = _getTarget(element)); + var bounds = element.getBoundingClientRect(), + size = bounds[horizontal ? _width : _height], + offset = referencePoint == null ? size / 2 : referencePoint in _keywords ? _keywords[referencePoint] * size : ~referencePoint.indexOf("%") ? parseFloat(referencePoint) * size / 100 : parseFloat(referencePoint) || 0; + return horizontal ? (bounds.left + offset) / _win$1.innerWidth : (bounds.top + offset) / _win$1.innerHeight; + }; + + ScrollTrigger.killAll = function killAll(allowListeners) { + _triggers.slice(0).forEach(function (t) { + return t.vars.id !== "ScrollSmoother" && t.kill(); + }); + + if (allowListeners !== true) { + var listeners = _listeners.killAll || []; + _listeners = {}; + listeners.forEach(function (f) { + return f(); + }); + } + }; + + return ScrollTrigger; + }(); + ScrollTrigger$1.version = "3.12.7"; + + ScrollTrigger$1.saveStyles = function (targets) { + return targets ? _toArray(targets).forEach(function (target) { + if (target && target.style) { + var i = _savedStyles.indexOf(target); + + i >= 0 && _savedStyles.splice(i, 5); + + _savedStyles.push(target, target.style.cssText, target.getBBox && target.getAttribute("transform"), gsap$1.core.getCache(target), _context$1()); + } + }) : _savedStyles; + }; + + ScrollTrigger$1.revert = function (soft, media) { + return _revertAll(!soft, media); + }; + + ScrollTrigger$1.create = function (vars, animation) { + return new ScrollTrigger$1(vars, animation); + }; + + ScrollTrigger$1.refresh = function (safe) { + return safe ? _onResize(true) : (_coreInitted$1 || ScrollTrigger$1.register()) && _refreshAll(true); + }; + + ScrollTrigger$1.update = function (force) { + return ++_scrollers.cache && _updateAll(force === true ? 2 : 0); + }; + + ScrollTrigger$1.clearScrollMemory = _clearScrollMemory; + + ScrollTrigger$1.maxScroll = function (element, horizontal) { + return _maxScroll(element, horizontal ? _horizontal : _vertical); + }; + + ScrollTrigger$1.getScrollFunc = function (element, horizontal) { + return _getScrollFunc(_getTarget(element), horizontal ? _horizontal : _vertical); + }; + + ScrollTrigger$1.getById = function (id) { + return _ids[id]; + }; + + ScrollTrigger$1.getAll = function () { + return _triggers.filter(function (t) { + return t.vars.id !== "ScrollSmoother"; + }); + }; + + ScrollTrigger$1.isScrolling = function () { + return !!_lastScrollTime; + }; + + ScrollTrigger$1.snapDirectional = _snapDirectional; + + ScrollTrigger$1.addEventListener = function (type, callback) { + var a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); + }; + + ScrollTrigger$1.removeEventListener = function (type, callback) { + var a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); + }; + + ScrollTrigger$1.batch = function (targets, vars) { + var result = [], + varsCopy = {}, + interval = vars.interval || 0.016, + batchMax = vars.batchMax || 1e9, + proxyCallback = function proxyCallback(type, callback) { + var elements = [], + triggers = [], + delay = gsap$1.delayedCall(interval, function () { + callback(elements, triggers); + elements = []; + triggers = []; + }).pause(); + return function (self) { + elements.length || delay.restart(true); + elements.push(self.trigger); + triggers.push(self); + batchMax <= elements.length && delay.progress(1); + }; + }, + p; + + for (p in vars) { + varsCopy[p] = p.substr(0, 2) === "on" && _isFunction(vars[p]) && p !== "onRefreshInit" ? proxyCallback(p, vars[p]) : vars[p]; + } + + if (_isFunction(batchMax)) { + batchMax = batchMax(); + + _addListener$1(ScrollTrigger$1, "refresh", function () { + return batchMax = vars.batchMax(); + }); + } + + _toArray(targets).forEach(function (target) { + var config = {}; + + for (p in varsCopy) { + config[p] = varsCopy[p]; + } + + config.trigger = target; + result.push(ScrollTrigger$1.create(config)); + }); + + return result; + }; + + var _clampScrollAndGetDurationMultiplier = function _clampScrollAndGetDurationMultiplier(scrollFunc, current, end, max) { + current > max ? scrollFunc(max) : current < 0 && scrollFunc(0); + return end > max ? (max - current) / (end - current) : end < 0 ? current / (current - end) : 1; + }, + _allowNativePanning = function _allowNativePanning(target, direction) { + if (direction === true) { + target.style.removeProperty("touch-action"); + } else { + target.style.touchAction = direction === true ? "auto" : direction ? "pan-" + direction + (Observer.isTouch ? " pinch-zoom" : "") : "none"; + } + + target === _docEl$1 && _allowNativePanning(_body$1, direction); + }, + _overflow = { + auto: 1, + scroll: 1 + }, + _nestedScroll = function _nestedScroll(_ref5) { + var event = _ref5.event, + target = _ref5.target, + axis = _ref5.axis; + + var node = (event.changedTouches ? event.changedTouches[0] : event).target, + cache = node._gsap || gsap$1.core.getCache(node), + time = _getTime$1(), + cs; + + if (!cache._isScrollT || time - cache._isScrollT > 2000) { + while (node && node !== _body$1 && (node.scrollHeight <= node.clientHeight && node.scrollWidth <= node.clientWidth || !(_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]))) { + node = node.parentNode; + } + + cache._isScroll = node && node !== target && !_isViewport$1(node) && (_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]); + cache._isScrollT = time; + } + + if (cache._isScroll || axis === "x") { + event.stopPropagation(); + event._gsapAllow = true; + } + }, + _inputObserver = function _inputObserver(target, type, inputs, nested) { + return Observer.create({ + target: target, + capture: true, + debounce: false, + lockAxis: true, + type: type, + onWheel: nested = nested && _nestedScroll, + onPress: nested, + onDrag: nested, + onScroll: nested, + onEnable: function onEnable() { + return inputs && _addListener$1(_doc$1, Observer.eventTypes[0], _captureInputs, false, true); + }, + onDisable: function onDisable() { + return _removeListener$1(_doc$1, Observer.eventTypes[0], _captureInputs, true); + } + }); + }, + _inputExp = /(input|label|select|textarea)/i, + _inputIsFocused, + _captureInputs = function _captureInputs(e) { + var isInput = _inputExp.test(e.target.tagName); + + if (isInput || _inputIsFocused) { + e._gsapAllow = true; + _inputIsFocused = isInput; + } + }, + _getScrollNormalizer = function _getScrollNormalizer(vars) { + _isObject(vars) || (vars = {}); + vars.preventDefault = vars.isNormalizer = vars.allowClicks = true; + vars.type || (vars.type = "wheel,touch"); + vars.debounce = !!vars.debounce; + vars.id = vars.id || "normalizer"; + + var _vars2 = vars, + normalizeScrollX = _vars2.normalizeScrollX, + momentum = _vars2.momentum, + allowNestedScroll = _vars2.allowNestedScroll, + onRelease = _vars2.onRelease, + self, + maxY, + target = _getTarget(vars.target) || _docEl$1, + smoother = gsap$1.core.globals().ScrollSmoother, + smootherInstance = smoother && smoother.get(), + content = _fixIOSBug && (vars.content && _getTarget(vars.content) || smootherInstance && vars.content !== false && !smootherInstance.smooth() && smootherInstance.content()), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollFuncX = _getScrollFunc(target, _horizontal), + scale = 1, + initialScale = (Observer.isTouch && _win$1.visualViewport ? _win$1.visualViewport.scale * _win$1.visualViewport.width : _win$1.outerWidth) / _win$1.innerWidth, + wheelRefresh = 0, + resolveMomentumDuration = _isFunction(momentum) ? function () { + return momentum(self); + } : function () { + return momentum || 2.8; + }, + lastRefreshID, + skipTouchMove, + inputObserver = _inputObserver(target, vars.type, true, allowNestedScroll), + resumeTouchMove = function resumeTouchMove() { + return skipTouchMove = false; + }, + scrollClampX = _passThrough, + scrollClampY = _passThrough, + updateClamps = function updateClamps() { + maxY = _maxScroll(target, _vertical); + scrollClampY = _clamp$1(_fixIOSBug ? 1 : 0, maxY); + normalizeScrollX && (scrollClampX = _clamp$1(0, _maxScroll(target, _horizontal))); + lastRefreshID = _refreshID; + }, + removeContentOffset = function removeContentOffset() { + content._gsap.y = _round(parseFloat(content._gsap.y) + scrollFuncY.offset) + "px"; + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + parseFloat(content._gsap.y) + ", 0, 1)"; + scrollFuncY.offset = scrollFuncY.cacheID = 0; + }, + ignoreDrag = function ignoreDrag() { + if (skipTouchMove) { + requestAnimationFrame(resumeTouchMove); + + var offset = _round(self.deltaY / 2), + scroll = scrollClampY(scrollFuncY.v - offset); + + if (content && scroll !== scrollFuncY.v + scrollFuncY.offset) { + scrollFuncY.offset = scroll - scrollFuncY.v; + + var y = _round((parseFloat(content && content._gsap.y) || 0) - scrollFuncY.offset); + + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + y + ", 0, 1)"; + content._gsap.y = y + "px"; + scrollFuncY.cacheID = _scrollers.cache; + + _updateAll(); + } + + return true; + } + + scrollFuncY.offset && removeContentOffset(); + skipTouchMove = true; + }, + tween, + startScrollX, + startScrollY, + onStopDelayedCall, + onResize = function onResize() { + updateClamps(); + + if (tween.isActive() && tween.vars.scrollY > maxY) { + scrollFuncY() > maxY ? tween.progress(1) && scrollFuncY(maxY) : tween.resetTo("scrollY", maxY); + } + }; + + content && gsap$1.set(content, { + y: "+=0" + }); + + vars.ignoreCheck = function (e) { + return _fixIOSBug && e.type === "touchmove" && ignoreDrag() || scale > 1.05 && e.type !== "touchstart" || self.isGesturing || e.touches && e.touches.length > 1; + }; + + vars.onPress = function () { + skipTouchMove = false; + var prevScale = scale; + scale = _round((_win$1.visualViewport && _win$1.visualViewport.scale || 1) / initialScale); + tween.pause(); + prevScale !== scale && _allowNativePanning(target, scale > 1.01 ? true : normalizeScrollX ? false : "x"); + startScrollX = scrollFuncX(); + startScrollY = scrollFuncY(); + updateClamps(); + lastRefreshID = _refreshID; + }; + + vars.onRelease = vars.onGestureStart = function (self, wasDragging) { + scrollFuncY.offset && removeContentOffset(); + + if (!wasDragging) { + onStopDelayedCall.restart(true); + } else { + _scrollers.cache++; + var dur = resolveMomentumDuration(), + currentScroll, + endScroll; + + if (normalizeScrollX) { + currentScroll = scrollFuncX(); + endScroll = currentScroll + dur * 0.05 * -self.velocityX / 0.227; + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncX, currentScroll, endScroll, _maxScroll(target, _horizontal)); + tween.vars.scrollX = scrollClampX(endScroll); + } + + currentScroll = scrollFuncY(); + endScroll = currentScroll + dur * 0.05 * -self.velocityY / 0.227; + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncY, currentScroll, endScroll, _maxScroll(target, _vertical)); + tween.vars.scrollY = scrollClampY(endScroll); + tween.invalidate().duration(dur).play(0.01); + + if (_fixIOSBug && tween.vars.scrollY >= maxY || currentScroll >= maxY - 1) { + gsap$1.to({}, { + onUpdate: onResize, + duration: dur + }); + } + } + + onRelease && onRelease(self); + }; + + vars.onWheel = function () { + tween._ts && tween.pause(); + + if (_getTime$1() - wheelRefresh > 1000) { + lastRefreshID = 0; + wheelRefresh = _getTime$1(); + } + }; + + vars.onChange = function (self, dx, dy, xArray, yArray) { + _refreshID !== lastRefreshID && updateClamps(); + dx && normalizeScrollX && scrollFuncX(scrollClampX(xArray[2] === dx ? startScrollX + (self.startX - self.x) : scrollFuncX() + dx - xArray[1])); + + if (dy) { + scrollFuncY.offset && removeContentOffset(); + var isTouch = yArray[2] === dy, + y = isTouch ? startScrollY + self.startY - self.y : scrollFuncY() + dy - yArray[1], + yClamped = scrollClampY(y); + isTouch && y !== yClamped && (startScrollY += yClamped - y); + scrollFuncY(yClamped); + } + + (dy || dx) && _updateAll(); + }; + + vars.onEnable = function () { + _allowNativePanning(target, normalizeScrollX ? false : "x"); + + ScrollTrigger$1.addEventListener("refresh", onResize); + + _addListener$1(_win$1, "resize", onResize); + + if (scrollFuncY.smooth) { + scrollFuncY.target.style.scrollBehavior = "auto"; + scrollFuncY.smooth = scrollFuncX.smooth = false; + } + + inputObserver.enable(); + }; + + vars.onDisable = function () { + _allowNativePanning(target, true); + + _removeListener$1(_win$1, "resize", onResize); + + ScrollTrigger$1.removeEventListener("refresh", onResize); + inputObserver.kill(); + }; + + vars.lockAxis = vars.lockAxis !== false; + self = new Observer(vars); + self.iOS = _fixIOSBug; + _fixIOSBug && !scrollFuncY() && scrollFuncY(1); + _fixIOSBug && gsap$1.ticker.add(_passThrough); + onStopDelayedCall = self._dc; + tween = gsap$1.to(self, { + ease: "power4", + paused: true, + inherit: false, + scrollX: normalizeScrollX ? "+=0.1" : "+=0", + scrollY: "+=0.1", + modifiers: { + scrollY: _interruptionTracker(scrollFuncY, scrollFuncY(), function () { + return tween.pause(); + }) + }, + onUpdate: _updateAll, + onComplete: onStopDelayedCall.vars.onComplete + }); + return self; + }; + + ScrollTrigger$1.sort = function (func) { + if (_isFunction(func)) { + return _triggers.sort(func); + } + + var scroll = _win$1.pageYOffset || 0; + ScrollTrigger$1.getAll().forEach(function (t) { + return t._sortY = t.trigger ? scroll + t.trigger.getBoundingClientRect().top : t.start + _win$1.innerHeight; + }); + return _triggers.sort(func || function (a, b) { + return (a.vars.refreshPriority || 0) * -1e6 + (a.vars.containerAnimation ? 1e6 : a._sortY) - ((b.vars.containerAnimation ? 1e6 : b._sortY) + (b.vars.refreshPriority || 0) * -1e6); + }); + }; + + ScrollTrigger$1.observe = function (vars) { + return new Observer(vars); + }; + + ScrollTrigger$1.normalizeScroll = function (vars) { + if (typeof vars === "undefined") { + return _normalizer$1; + } + + if (vars === true && _normalizer$1) { + return _normalizer$1.enable(); + } + + if (vars === false) { + _normalizer$1 && _normalizer$1.kill(); + _normalizer$1 = vars; + return; + } + + var normalizer = vars instanceof Observer ? vars : _getScrollNormalizer(vars); + _normalizer$1 && _normalizer$1.target === normalizer.target && _normalizer$1.kill(); + _isViewport$1(normalizer.target) && (_normalizer$1 = normalizer); + return normalizer; + }; + + ScrollTrigger$1.core = { + _getVelocityProp: _getVelocityProp, + _inputObserver: _inputObserver, + _scrollers: _scrollers, + _proxies: _proxies, + bridge: { + ss: function ss() { + _lastScrollTime || _dispatch("scrollStart"); + _lastScrollTime = _getTime$1(); + }, + ref: function ref() { + return _refreshing; + } + } + }; + _getGSAP$1() && gsap$1.registerPlugin(ScrollTrigger$1); + + exports.ScrollTrigger = ScrollTrigger$1; + exports.default = ScrollTrigger$1; + + if (typeof(window) === 'undefined' || window !== exports) {Object.defineProperty(exports, '__esModule', { value: true });} else {delete window.default;} + +}))); diff --git a/node_modules/gsap/dist/ScrollTrigger.min.js b/node_modules/gsap/dist/ScrollTrigger.min.js new file mode 100644 index 0000000000000000000000000000000000000000..01c8533bb01e59eb5768aeff701f7269215f9299 --- /dev/null +++ b/node_modules/gsap/dist/ScrollTrigger.min.js @@ -0,0 +1,11 @@ +/*! + * ScrollTrigger 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e=e||self).window=e.window||{})}(this,function(e){"use strict";function _defineProperties(e,t){for(var r=0;r<t.length;r++){var n=t[r];n.enumerable=n.enumerable||!1,n.configurable=!0,"value"in n&&(n.writable=!0),Object.defineProperty(e,n.key,n)}}function r(){return Te||"undefined"!=typeof window&&(Te=window.gsap)&&Te.registerPlugin&&Te}function z(e,t){return~Ie.indexOf(e)&&Ie[Ie.indexOf(e)+1][t]}function A(e){return!!~t.indexOf(e)}function B(e,t,r,n,i){return e.addEventListener(t,r,{passive:!1!==n,capture:!!i})}function C(e,t,r,n){return e.removeEventListener(t,r,!!n)}function F(){return De&&De.isPressed||qe.cache++}function G(r,n){function dd(e){if(e||0===e){i&&(Se.history.scrollRestoration="manual");var t=De&&De.isPressed;e=dd.v=Math.round(e)||(De&&De.iOS?1:0),r(e),dd.cacheID=qe.cache,t&&o("ss",e)}else(n||qe.cache!==dd.cacheID||o("ref"))&&(dd.cacheID=qe.cache,dd.v=r());return dd.v+dd.offset}return dd.offset=0,r&&dd}function J(e,t){return(t&&t._ctx&&t._ctx.selector||Te.utils.toArray)(e)[0]||("string"==typeof e&&!1!==Te.config().nullTargetWarn?console.warn("Element not found:",e):null)}function K(t,e){var r=e.s,n=e.sc;A(t)&&(t=ke.scrollingElement||Ee);var i=qe.indexOf(t),o=n===ze.sc?1:2;~i||(i=qe.push(t)-1),qe[i+o]||B(t,"scroll",F);var a=qe[i+o],s=a||(qe[i+o]=G(z(t,r),!0)||(A(t)?n:G(function(e){return arguments.length?t[r]=e:t[r]})));return s.target=t,a||(s.smooth="smooth"===Te.getProperty(t,"scrollBehavior")),s}function L(e,t,i){function Cd(e,t){var r=Ye();t||n<r-s?(a=o,o=e,l=s,s=r):i?o+=e:o=a+(e-a)/(r-l)*(s-l)}var o=e,a=e,s=Ye(),l=s,n=t||50,c=Math.max(500,3*n);return{update:Cd,reset:function reset(){a=o=i?0:o,l=s=0},getVelocity:function getVelocity(e){var t=l,r=a,n=Ye();return!e&&0!==e||e===o||Cd(e),s===l||c<n-l?0:(o+(i?r:-r))/((i?n:s)-t)*1e3}}}function M(e,t){return t&&!e._gsapAllow&&e.preventDefault(),e.changedTouches?e.changedTouches[0]:e}function N(e){var t=Math.max.apply(Math,e),r=Math.min.apply(Math,e);return Math.abs(t)>=Math.abs(r)?t:r}function O(){(Ae=Te.core.globals().ScrollTrigger)&&Ae.core&&function _integrate(){var e=Ae.core,r=e.bridge||{},t=e._scrollers,n=e._proxies;t.push.apply(t,qe),n.push.apply(n,Ie),qe=t,Ie=n,o=function _bridge(e,t){return r[e](t)}}()}function P(e){return Te=e||r(),!Ce&&Te&&"undefined"!=typeof document&&document.body&&(Se=window,Ee=(ke=document).documentElement,Pe=ke.body,t=[Se,ke,Ee,Pe],Te.utils.clamp,Re=Te.core.context||function(){},Oe="onpointerenter"in Pe?"pointer":"mouse",Me=k.isTouch=Se.matchMedia&&Se.matchMedia("(hover: none), (pointer: coarse)").matches?1:"ontouchstart"in Se||0<navigator.maxTouchPoints||0<navigator.msMaxTouchPoints?2:0,Be=k.eventTypes=("ontouchstart"in Ee?"touchstart,touchmove,touchcancel,touchend":"onpointerdown"in Ee?"pointerdown,pointermove,pointercancel,pointerup":"mousedown,mousemove,mouseup,mouseup").split(","),setTimeout(function(){return i=0},500),O(),Ce=1),Ce}var Te,Ce,Se,ke,Ee,Pe,Me,Oe,Ae,t,De,Be,Re,i=1,Le=[],qe=[],Ie=[],Ye=Date.now,o=function _bridge(e,t){return t},n="scrollLeft",a="scrollTop",Fe={s:n,p:"left",p2:"Left",os:"right",os2:"Right",d:"width",d2:"Width",a:"x",sc:G(function(e){return arguments.length?Se.scrollTo(e,ze.sc()):Se.pageXOffset||ke[n]||Ee[n]||Pe[n]||0})},ze={s:a,p:"top",p2:"Top",os:"bottom",os2:"Bottom",d:"height",d2:"Height",a:"y",op:Fe,sc:G(function(e){return arguments.length?Se.scrollTo(Fe.sc(),e):Se.pageYOffset||ke[a]||Ee[a]||Pe[a]||0})};Fe.op=ze,qe.cache=0;var k=(Observer.prototype.init=function init(e){Ce||P(Te)||console.warn("Please gsap.registerPlugin(Observer)"),Ae||O();var i=e.tolerance,a=e.dragMinimum,t=e.type,o=e.target,r=e.lineHeight,n=e.debounce,s=e.preventDefault,l=e.onStop,c=e.onStopDelay,u=e.ignore,f=e.wheelSpeed,d=e.event,p=e.onDragStart,g=e.onDragEnd,h=e.onDrag,v=e.onPress,b=e.onRelease,m=e.onRight,y=e.onLeft,x=e.onUp,w=e.onDown,_=e.onChangeX,T=e.onChangeY,S=e.onChange,k=e.onToggleX,E=e.onToggleY,D=e.onHover,R=e.onHoverEnd,q=e.onMove,I=e.ignoreCheck,Y=e.isNormalizer,z=e.onGestureStart,H=e.onGestureEnd,X=e.onWheel,W=e.onEnable,V=e.onDisable,U=e.onClick,G=e.scrollSpeed,j=e.capture,Q=e.allowClicks,Z=e.lockAxis,$=e.onLockAxis;function cf(){return xe=Ye()}function df(e,t){return(se.event=e)&&u&&~u.indexOf(e.target)||t&&he&&"touch"!==e.pointerType||I&&I(e,t)}function ff(){var e=se.deltaX=N(me),t=se.deltaY=N(ye),r=Math.abs(e)>=i,n=Math.abs(t)>=i;S&&(r||n)&&S(se,e,t,me,ye),r&&(m&&0<se.deltaX&&m(se),y&&se.deltaX<0&&y(se),_&&_(se),k&&se.deltaX<0!=le<0&&k(se),le=se.deltaX,me[0]=me[1]=me[2]=0),n&&(w&&0<se.deltaY&&w(se),x&&se.deltaY<0&&x(se),T&&T(se),E&&se.deltaY<0!=ce<0&&E(se),ce=se.deltaY,ye[0]=ye[1]=ye[2]=0),(ne||re)&&(q&&q(se),re&&(p&&1===re&&p(se),h&&h(se),re=0),ne=!1),oe&&!(oe=!1)&&$&&$(se),ie&&(X(se),ie=!1),ee=0}function gf(e,t,r){me[r]+=e,ye[r]+=t,se._vx.update(e),se._vy.update(t),n?ee=ee||requestAnimationFrame(ff):ff()}function hf(e,t){Z&&!ae&&(se.axis=ae=Math.abs(e)>Math.abs(t)?"x":"y",oe=!0),"y"!==ae&&(me[2]+=e,se._vx.update(e,!0)),"x"!==ae&&(ye[2]+=t,se._vy.update(t,!0)),n?ee=ee||requestAnimationFrame(ff):ff()}function jf(e){if(!df(e,1)){var t=(e=M(e,s)).clientX,r=e.clientY,n=t-se.x,i=r-se.y,o=se.isDragging;se.x=t,se.y=r,(o||(n||i)&&(Math.abs(se.startX-t)>=a||Math.abs(se.startY-r)>=a))&&(re=o?2:1,o||(se.isDragging=!0),hf(n,i))}}function mf(e){return e.touches&&1<e.touches.length&&(se.isGesturing=!0)&&z(e,se.isDragging)}function nf(){return(se.isGesturing=!1)||H(se)}function of(e){if(!df(e)){var t=fe(),r=de();gf((t-pe)*G,(r-ge)*G,1),pe=t,ge=r,l&&te.restart(!0)}}function pf(e){if(!df(e)){e=M(e,s),X&&(ie=!0);var t=(1===e.deltaMode?r:2===e.deltaMode?Se.innerHeight:1)*f;gf(e.deltaX*t,e.deltaY*t,0),l&&!Y&&te.restart(!0)}}function qf(e){if(!df(e)){var t=e.clientX,r=e.clientY,n=t-se.x,i=r-se.y;se.x=t,se.y=r,ne=!0,l&&te.restart(!0),(n||i)&&hf(n,i)}}function rf(e){se.event=e,D(se)}function sf(e){se.event=e,R(se)}function tf(e){return df(e)||M(e,s)&&U(se)}this.target=o=J(o)||Ee,this.vars=e,u=u&&Te.utils.toArray(u),i=i||1e-9,a=a||0,f=f||1,G=G||1,t=t||"wheel,touch,pointer",n=!1!==n,r=r||parseFloat(Se.getComputedStyle(Pe).lineHeight)||22;var ee,te,re,ne,ie,oe,ae,se=this,le=0,ce=0,ue=e.passive||!s&&!1!==e.passive,fe=K(o,Fe),de=K(o,ze),pe=fe(),ge=de(),he=~t.indexOf("touch")&&!~t.indexOf("pointer")&&"pointerdown"===Be[0],ve=A(o),be=o.ownerDocument||ke,me=[0,0,0],ye=[0,0,0],xe=0,we=se.onPress=function(e){df(e,1)||e&&e.button||(se.axis=ae=null,te.pause(),se.isPressed=!0,e=M(e),le=ce=0,se.startX=se.x=e.clientX,se.startY=se.y=e.clientY,se._vx.reset(),se._vy.reset(),B(Y?o:be,Be[1],jf,ue,!0),se.deltaX=se.deltaY=0,v&&v(se))},_e=se.onRelease=function(t){if(!df(t,1)){C(Y?o:be,Be[1],jf,!0);var e=!isNaN(se.y-se.startY),r=se.isDragging,n=r&&(3<Math.abs(se.x-se.startX)||3<Math.abs(se.y-se.startY)),i=M(t);!n&&e&&(se._vx.reset(),se._vy.reset(),s&&Q&&Te.delayedCall(.08,function(){if(300<Ye()-xe&&!t.defaultPrevented)if(t.target.click)t.target.click();else if(be.createEvent){var e=be.createEvent("MouseEvents");e.initMouseEvent("click",!0,!0,Se,1,i.screenX,i.screenY,i.clientX,i.clientY,!1,!1,!1,!1,0,null),t.target.dispatchEvent(e)}})),se.isDragging=se.isGesturing=se.isPressed=!1,l&&r&&!Y&&te.restart(!0),re&&ff(),g&&r&&g(se),b&&b(se,n)}};te=se._dc=Te.delayedCall(c||.25,function onStopFunc(){se._vx.reset(),se._vy.reset(),te.pause(),l&&l(se)}).pause(),se.deltaX=se.deltaY=0,se._vx=L(0,50,!0),se._vy=L(0,50,!0),se.scrollX=fe,se.scrollY=de,se.isDragging=se.isGesturing=se.isPressed=!1,Re(this),se.enable=function(e){return se.isEnabled||(B(ve?be:o,"scroll",F),0<=t.indexOf("scroll")&&B(ve?be:o,"scroll",of,ue,j),0<=t.indexOf("wheel")&&B(o,"wheel",pf,ue,j),(0<=t.indexOf("touch")&&Me||0<=t.indexOf("pointer"))&&(B(o,Be[0],we,ue,j),B(be,Be[2],_e),B(be,Be[3],_e),Q&&B(o,"click",cf,!0,!0),U&&B(o,"click",tf),z&&B(be,"gesturestart",mf),H&&B(be,"gestureend",nf),D&&B(o,Oe+"enter",rf),R&&B(o,Oe+"leave",sf),q&&B(o,Oe+"move",qf)),se.isEnabled=!0,se.isDragging=se.isGesturing=se.isPressed=ne=re=!1,se._vx.reset(),se._vy.reset(),pe=fe(),ge=de(),e&&e.type&&we(e),W&&W(se)),se},se.disable=function(){se.isEnabled&&(Le.filter(function(e){return e!==se&&A(e.target)}).length||C(ve?be:o,"scroll",F),se.isPressed&&(se._vx.reset(),se._vy.reset(),C(Y?o:be,Be[1],jf,!0)),C(ve?be:o,"scroll",of,j),C(o,"wheel",pf,j),C(o,Be[0],we,j),C(be,Be[2],_e),C(be,Be[3],_e),C(o,"click",cf,!0),C(o,"click",tf),C(be,"gesturestart",mf),C(be,"gestureend",nf),C(o,Oe+"enter",rf),C(o,Oe+"leave",sf),C(o,Oe+"move",qf),se.isEnabled=se.isPressed=se.isDragging=!1,V&&V(se))},se.kill=se.revert=function(){se.disable();var e=Le.indexOf(se);0<=e&&Le.splice(e,1),De===se&&(De=0)},Le.push(se),Y&&A(o)&&(De=se),se.enable(d)},function _createClass(e,t,r){return t&&_defineProperties(e.prototype,t),r&&_defineProperties(e,r),e}(Observer,[{key:"velocityX",get:function get(){return this._vx.getVelocity()}},{key:"velocityY",get:function get(){return this._vy.getVelocity()}}]),Observer);function Observer(e){this.init(e)}k.version="3.12.7",k.create=function(e){return new k(e)},k.register=P,k.getAll=function(){return Le.slice()},k.getById=function(t){return Le.filter(function(e){return e.vars.id===t})[0]},r()&&Te.registerPlugin(k);function Ca(e,t,r){var n=ct(e)&&("clamp("===e.substr(0,6)||-1<e.indexOf("max"));return(r["_"+t+"Clamp"]=n)?e.substr(6,e.length-7):e}function Da(e,t){return!t||ct(e)&&"clamp("===e.substr(0,6)?e:"clamp("+e+")"}function Fa(){return je=1}function Ga(){return je=0}function Ha(e){return e}function Ia(e){return Math.round(1e5*e)/1e5||0}function Ja(){return"undefined"!=typeof window}function Ka(){return He||Ja()&&(He=window.gsap)&&He.registerPlugin&&He}function La(e){return!!~l.indexOf(e)}function Ma(e){return("Height"===e?T:Ne["inner"+e])||We["client"+e]||Je["client"+e]}function Na(e){return z(e,"getBoundingClientRect")||(La(e)?function(){return Ot.width=Ne.innerWidth,Ot.height=T,Ot}:function(){return wt(e)})}function Qa(e,t){var r=t.s,n=t.d2,i=t.d,o=t.a;return Math.max(0,(r="scroll"+n)&&(o=z(e,r))?o()-Na(e)()[i]:La(e)?(We[r]||Je[r])-Ma(n):e[r]-e["offset"+n])}function Ra(e,t){for(var r=0;r<g.length;r+=3)t&&!~t.indexOf(g[r+1])||e(g[r],g[r+1],g[r+2])}function Ta(e){return"function"==typeof e}function Ua(e){return"number"==typeof e}function Va(e){return"object"==typeof e}function Wa(e,t,r){return e&&e.progress(t?0:1)&&r&&e.pause()}function Xa(e,t){if(e.enabled){var r=e._ctx?e._ctx.add(function(){return t(e)}):t(e);r&&r.totalTime&&(e.callbackAnimation=r)}}function mb(e){return Ne.getComputedStyle(e)}function ob(e,t){for(var r in t)r in e||(e[r]=t[r]);return e}function qb(e,t){var r=t.d2;return e["offset"+r]||e["client"+r]||0}function rb(e){var t,r=[],n=e.labels,i=e.duration();for(t in n)r.push(n[t]/i);return r}function tb(i){var o=He.utils.snap(i),a=Array.isArray(i)&&i.slice(0).sort(function(e,t){return e-t});return a?function(e,t,r){var n;if(void 0===r&&(r=.001),!t)return o(e);if(0<t){for(e-=r,n=0;n<a.length;n++)if(a[n]>=e)return a[n];return a[n-1]}for(n=a.length,e+=r;n--;)if(a[n]<=e)return a[n];return a[0]}:function(e,t,r){void 0===r&&(r=.001);var n=o(e);return!t||Math.abs(n-e)<r||n-e<0==t<0?n:o(t<0?e-i:e+i)}}function vb(t,r,e,n){return e.split(",").forEach(function(e){return t(r,e,n)})}function wb(e,t,r,n,i){return e.addEventListener(t,r,{passive:!n,capture:!!i})}function xb(e,t,r,n){return e.removeEventListener(t,r,!!n)}function yb(e,t,r){(r=r&&r.wheelHandler)&&(e(t,"wheel",r),e(t,"touchmove",r))}function Cb(e,t){if(ct(e)){var r=e.indexOf("="),n=~r?(e.charAt(r-1)+1)*parseFloat(e.substr(r+1)):0;~r&&(e.indexOf("%")>r&&(n*=t/100),e=e.substr(0,r-1)),e=n+(e in H?H[e]*t:~e.indexOf("%")?parseFloat(e)*t/100:parseFloat(e)||0)}return e}function Db(e,t,r,n,i,o,a,s){var l=i.startColor,c=i.endColor,u=i.fontSize,f=i.indent,d=i.fontWeight,p=Xe.createElement("div"),g=La(r)||"fixed"===z(r,"pinType"),h=-1!==e.indexOf("scroller"),v=g?Je:r,b=-1!==e.indexOf("start"),m=b?l:c,y="border-color:"+m+";font-size:"+u+";color:"+m+";font-weight:"+d+";pointer-events:none;white-space:nowrap;font-family:sans-serif,Arial;z-index:1000;padding:4px 8px;border-width:0;border-style:solid;";return y+="position:"+((h||s)&&g?"fixed;":"absolute;"),!h&&!s&&g||(y+=(n===ze?q:I)+":"+(o+parseFloat(f))+"px;"),a&&(y+="box-sizing:border-box;text-align:left;width:"+a.offsetWidth+"px;"),p._isStart=b,p.setAttribute("class","gsap-marker-"+e+(t?" marker-"+t:"")),p.style.cssText=y,p.innerText=t||0===t?e+"-"+t:e,v.children[0]?v.insertBefore(p,v.children[0]):v.appendChild(p),p._offset=p["offset"+n.op.d2],X(p,0,n,b),p}function Ib(){return 34<at()-st&&(D=D||requestAnimationFrame(Z))}function Jb(){v&&v.isPressed&&!(v.startX>Je.clientWidth)||(qe.cache++,v?D=D||requestAnimationFrame(Z):Z(),st||U("scrollStart"),st=at())}function Kb(){y=Ne.innerWidth,m=Ne.innerHeight}function Lb(e){qe.cache++,!0!==e&&(Ke||h||Xe.fullscreenElement||Xe.webkitFullscreenElement||b&&y===Ne.innerWidth&&!(Math.abs(Ne.innerHeight-m)>.25*Ne.innerHeight))||c.restart(!0)}function Ob(){return xb(ne,"scrollEnd",Ob)||Et(!0)}function Rb(e){for(var t=0;t<j.length;t+=5)(!e||j[t+4]&&j[t+4].query===e)&&(j[t].style.cssText=j[t+1],j[t].getBBox&&j[t].setAttribute("transform",j[t+2]||""),j[t+3].uncache=1)}function Sb(e,t){var r;for(Qe=0;Qe<Ct.length;Qe++)!(r=Ct[Qe])||t&&r._ctx!==t||(e?r.kill(1):r.revert(!0,!0));S=!0,t&&Rb(t),t||U("revert")}function Tb(e,t){qe.cache++,!t&&rt||qe.forEach(function(e){return Ta(e)&&e.cacheID++&&(e.rec=0)}),ct(e)&&(Ne.history.scrollRestoration=w=e)}function Yb(){Je.appendChild(_),T=!v&&_.offsetHeight||Ne.innerHeight,Je.removeChild(_)}function Zb(t){return Ve(".gsap-marker-start, .gsap-marker-end, .gsap-marker-scroller-start, .gsap-marker-scroller-end").forEach(function(e){return e.style.display=t?"none":"block"})}function gc(e,t,r,n){if(!e._gsap.swappedIn){for(var i,o=$.length,a=t.style,s=e.style;o--;)a[i=$[o]]=r[i];a.position="absolute"===r.position?"absolute":"relative","inline"===r.display&&(a.display="inline-block"),s[I]=s[q]="auto",a.flexBasis=r.flexBasis||"auto",a.overflow="visible",a.boxSizing="border-box",a[ft]=qb(e,Fe)+xt,a[dt]=qb(e,ze)+xt,a[bt]=s[mt]=s.top=s.left="0",Mt(n),s[ft]=s.maxWidth=r[ft],s[dt]=s.maxHeight=r[dt],s[bt]=r[bt],e.parentNode!==t&&(e.parentNode.insertBefore(t,e),t.appendChild(e)),e._gsap.swappedIn=!0}}function jc(e){for(var t=ee.length,r=e.style,n=[],i=0;i<t;i++)n.push(ee[i],r[ee[i]]);return n.t=e,n}function mc(e,t,r,n,i,o,a,s,l,c,u,f,d,p){Ta(e)&&(e=e(s)),ct(e)&&"max"===e.substr(0,3)&&(e=f+("="===e.charAt(4)?Cb("0"+e.substr(3),r):0));var g,h,v,b=d?d.time():0;if(d&&d.seek(0),isNaN(e)||(e=+e),Ua(e))d&&(e=He.utils.mapRange(d.scrollTrigger.start,d.scrollTrigger.end,0,f,e)),a&&X(a,r,n,!0);else{Ta(t)&&(t=t(s));var m,y,x,w,_=(e||"0").split(" ");v=J(t,s)||Je,(m=wt(v)||{})&&(m.left||m.top)||"none"!==mb(v).display||(w=v.style.display,v.style.display="block",m=wt(v),w?v.style.display=w:v.style.removeProperty("display")),y=Cb(_[0],m[n.d]),x=Cb(_[1]||"0",r),e=m[n.p]-l[n.p]-c+y+i-x,a&&X(a,x,n,r-x<20||a._isStart&&20<x),r-=r-x}if(p&&(s[p]=e||-.001,e<0&&(e=0)),o){var T=e+r,C=o._isStart;g="scroll"+n.d2,X(o,T,n,C&&20<T||!C&&(u?Math.max(Je[g],We[g]):o.parentNode[g])<=T+1),u&&(l=wt(a),u&&(o.style[n.op.p]=l[n.op.p]-n.op.m-o._offset+xt))}return d&&v&&(g=wt(v),d.seek(f),h=wt(v),d._caScrollDist=g[n.p]-h[n.p],e=e/d._caScrollDist*f),d&&d.seek(b),d?e:Math.round(e)}function oc(e,t,r,n){if(e.parentNode!==t){var i,o,a=e.style;if(t===Je){for(i in e._stOrig=a.cssText,o=mb(e))+i||re.test(i)||!o[i]||"string"!=typeof a[i]||"0"===i||(a[i]=o[i]);a.top=r,a.left=n}else a.cssText=e._stOrig;He.core.getCache(e).uncache=1,t.appendChild(e)}}function pc(r,e,n){var i=e,o=i;return function(e){var t=Math.round(r());return t!==i&&t!==o&&3<Math.abs(t-i)&&3<Math.abs(t-o)&&(e=t,n&&n()),o=i,i=Math.round(e)}}function qc(e,t,r){var n={};n[t.p]="+="+r,He.set(e,n)}function rc(c,e){function Dk(e,t,r,n,i){var o=Dk.tween,a=t.onComplete,s={};r=r||u();var l=pc(u,r,function(){o.kill(),Dk.tween=0});return i=n&&i||0,n=n||e-r,o&&o.kill(),t[f]=e,t.inherit=!1,(t.modifiers=s)[f]=function(){return l(r+n*o.ratio+i*o.ratio*o.ratio)},t.onUpdate=function(){qe.cache++,Dk.tween&&Z()},t.onComplete=function(){Dk.tween=0,a&&a.call(o)},o=Dk.tween=He.to(c,t)}var u=K(c,e),f="_scroll"+e.p2;return(c[f]=u).wheelHandler=function(){return Dk.tween&&Dk.tween.kill()&&(Dk.tween=0)},wb(c,"wheel",u.wheelHandler),ne.isTouch&&wb(c,"touchmove",u.wheelHandler),Dk}var He,s,Ne,Xe,We,Je,l,c,Ve,Ue,Ge,u,Ke,je,f,Qe,d,p,g,Ze,$e,h,v,b,m,y,E,x,w,_,T,S,et,tt,D,rt,nt,it,ot=1,at=Date.now,R=at(),st=0,lt=0,ct=function _isString(e){return"string"==typeof e},ut=Math.abs,q="right",I="bottom",ft="width",dt="height",pt="Right",gt="Left",ht="Top",vt="Bottom",bt="padding",mt="margin",yt="Width",Y="Height",xt="px",wt=function _getBounds(e,t){var r=t&&"matrix(1, 0, 0, 1, 0, 0)"!==mb(e)[f]&&He.to(e,{x:0,y:0,xPercent:0,yPercent:0,rotation:0,rotationX:0,rotationY:0,scale:1,skewX:0,skewY:0}).progress(1),n=e.getBoundingClientRect();return r&&r.progress(0).kill(),n},_t={startColor:"green",endColor:"red",indent:0,fontSize:"16px",fontWeight:"normal"},Tt={toggleActions:"play",anticipatePin:0},H={top:0,left:0,center:.5,bottom:1,right:1},X=function _positionMarker(e,t,r,n){var i={display:"block"},o=r[n?"os2":"p2"],a=r[n?"p2":"os2"];e._isFlipped=n,i[r.a+"Percent"]=n?-100:0,i[r.a]=n?"1px":0,i["border"+o+yt]=1,i["border"+a+yt]=0,i[r.p]=t+"px",He.set(e,i)},Ct=[],St={},W={},V=[],U=function _dispatch(e){return W[e]&&W[e].map(function(e){return e()})||V},j=[],kt=0,Et=function _refreshAll(e,t){if(We=Xe.documentElement,Je=Xe.body,l=[Ne,Xe,We,Je],!st||e||S){Yb(),rt=ne.isRefreshing=!0,qe.forEach(function(e){return Ta(e)&&++e.cacheID&&(e.rec=e())});var r=U("refreshInit");Ze&&ne.sort(),t||Sb(),qe.forEach(function(e){Ta(e)&&(e.smooth&&(e.target.style.scrollBehavior="auto"),e(0))}),Ct.slice(0).forEach(function(e){return e.refresh()}),S=!1,Ct.forEach(function(e){if(e._subPinOffset&&e.pin){var t=e.vars.horizontal?"offsetWidth":"offsetHeight",r=e.pin[t];e.revert(!0,1),e.adjustPinSpacing(e.pin[t]-r),e.refresh()}}),et=1,Zb(!0),Ct.forEach(function(e){var t=Qa(e.scroller,e._dir),r="max"===e.vars.end||e._endClamp&&e.end>t,n=e._startClamp&&e.start>=t;(r||n)&&e.setPositions(n?t-1:e.start,r?Math.max(n?t:e.start+1,t):e.end,!0)}),Zb(!1),et=0,r.forEach(function(e){return e&&e.render&&e.render(-1)}),qe.forEach(function(e){Ta(e)&&(e.smooth&&requestAnimationFrame(function(){return e.target.style.scrollBehavior="smooth"}),e.rec&&e(e.rec))}),Tb(w,1),c.pause(),kt++,Z(rt=2),Ct.forEach(function(e){return Ta(e.vars.onRefresh)&&e.vars.onRefresh(e)}),rt=ne.isRefreshing=!1,U("refresh")}else wb(ne,"scrollEnd",Ob)},Q=0,Pt=1,Z=function _updateAll(e){if(2===e||!rt&&!S){ne.isUpdating=!0,it&&it.update(0);var t=Ct.length,r=at(),n=50<=r-R,i=t&&Ct[0].scroll();if(Pt=i<Q?-1:1,rt||(Q=i),n&&(st&&!je&&200<r-st&&(st=0,U("scrollEnd")),Ge=R,R=r),Pt<0){for(Qe=t;0<Qe--;)Ct[Qe]&&Ct[Qe].update(0,n);Pt=1}else for(Qe=0;Qe<t;Qe++)Ct[Qe]&&Ct[Qe].update(0,n);ne.isUpdating=!1}D=0},$=["left","top",I,q,mt+vt,mt+pt,mt+ht,mt+gt,"display","flexShrink","float","zIndex","gridColumnStart","gridColumnEnd","gridRowStart","gridRowEnd","gridArea","justifySelf","alignSelf","placeSelf","order"],ee=$.concat([ft,dt,"boxSizing","max"+yt,"max"+Y,"position",mt,bt,bt+ht,bt+pt,bt+vt,bt+gt]),te=/([A-Z])/g,Mt=function _setState(e){if(e){var t,r,n=e.t.style,i=e.length,o=0;for((e.t._gsap||He.core.getCache(e.t)).uncache=1;o<i;o+=2)r=e[o+1],t=e[o],r?n[t]=r:n[t]&&n.removeProperty(t.replace(te,"-$1").toLowerCase())}},Ot={left:0,top:0},re=/(webkit|moz|length|cssText|inset)/i,ne=(ScrollTrigger.prototype.init=function init(M,O){if(this.progress=this.start=0,this.vars&&this.kill(!0,!0),lt){var A,n,p,D,B,R,L,q,I,Y,F,e,H,N,X,W,V,U,t,G,b,j,Q,m,Z,y,$,x,r,w,_,ee,i,g,te,re,ne,T,o,C=(M=ob(ct(M)||Ua(M)||M.nodeType?{trigger:M}:M,Tt)).onUpdate,S=M.toggleClass,a=M.id,k=M.onToggle,ie=M.onRefresh,E=M.scrub,oe=M.trigger,ae=M.pin,se=M.pinSpacing,le=M.invalidateOnRefresh,P=M.anticipatePin,s=M.onScrubComplete,h=M.onSnapComplete,ce=M.once,ue=M.snap,fe=M.pinReparent,l=M.pinSpacer,de=M.containerAnimation,pe=M.fastScrollEnd,ge=M.preventOverlaps,he=M.horizontal||M.containerAnimation&&!1!==M.horizontal?Fe:ze,ve=!E&&0!==E,be=J(M.scroller||Ne),c=He.core.getCache(be),me=La(be),ye="fixed"===("pinType"in M?M.pinType:z(be,"pinType")||me&&"fixed"),xe=[M.onEnter,M.onLeave,M.onEnterBack,M.onLeaveBack],we=ve&&M.toggleActions.split(" "),_e="markers"in M?M.markers:Tt.markers,Te=me?0:parseFloat(mb(be)["border"+he.p2+yt])||0,Ce=this,Se=M.onRefreshInit&&function(){return M.onRefreshInit(Ce)},ke=function _getSizeFunc(e,t,r){var n=r.d,i=r.d2,o=r.a;return(o=z(e,"getBoundingClientRect"))?function(){return o()[n]}:function(){return(t?Ma(i):e["client"+i])||0}}(be,me,he),Ee=function _getOffsetsFunc(e,t){return!t||~Ie.indexOf(e)?Na(e):function(){return Ot}}(be,me),Pe=0,Me=0,Oe=0,Ae=K(be,he);if(Ce._startClamp=Ce._endClamp=!1,Ce._dir=he,P*=45,Ce.scroller=be,Ce.scroll=de?de.time.bind(de):Ae,D=Ae(),Ce.vars=M,O=O||M.animation,"refreshPriority"in M&&(Ze=1,-9999===M.refreshPriority&&(it=Ce)),c.tweenScroll=c.tweenScroll||{top:rc(be,ze),left:rc(be,Fe)},Ce.tweenTo=A=c.tweenScroll[he.p],Ce.scrubDuration=function(e){(i=Ua(e)&&e)?ee?ee.duration(e):ee=He.to(O,{ease:"expo",totalProgress:"+=0",inherit:!1,duration:i,paused:!0,onComplete:function onComplete(){return s&&s(Ce)}}):(ee&&ee.progress(1).kill(),ee=0)},O&&(O.vars.lazy=!1,O._initted&&!Ce.isReverted||!1!==O.vars.immediateRender&&!1!==M.immediateRender&&O.duration()&&O.render(0,!0,!0),Ce.animation=O.pause(),(O.scrollTrigger=Ce).scrubDuration(E),w=0,a=a||O.vars.id),ue&&(Va(ue)&&!ue.push||(ue={snapTo:ue}),"scrollBehavior"in Je.style&&He.set(me?[Je,We]:be,{scrollBehavior:"auto"}),qe.forEach(function(e){return Ta(e)&&e.target===(me?Xe.scrollingElement||We:be)&&(e.smooth=!1)}),p=Ta(ue.snapTo)?ue.snapTo:"labels"===ue.snapTo?function _getClosestLabel(t){return function(e){return He.utils.snap(rb(t),e)}}(O):"labelsDirectional"===ue.snapTo?function _getLabelAtDirection(r){return function(e,t){return tb(rb(r))(e,t.direction)}}(O):!1!==ue.directional?function(e,t){return tb(ue.snapTo)(e,at()-Me<500?0:t.direction)}:He.utils.snap(ue.snapTo),g=ue.duration||{min:.1,max:2},g=Va(g)?Ue(g.min,g.max):Ue(g,g),te=He.delayedCall(ue.delay||i/2||.1,function(){var e=Ae(),t=at()-Me<500,r=A.tween;if(!(t||Math.abs(Ce.getVelocity())<10)||r||je||Pe===e)Ce.isActive&&Pe!==e&&te.restart(!0);else{var n,i,o=(e-R)/N,a=O&&!ve?O.totalProgress():o,s=t?0:(a-_)/(at()-Ge)*1e3||0,l=He.utils.clamp(-o,1-o,ut(s/2)*s/.185),c=o+(!1===ue.inertia?0:l),u=ue.onStart,f=ue.onInterrupt,d=ue.onComplete;if(n=p(c,Ce),Ua(n)||(n=c),i=Math.max(0,Math.round(R+n*N)),e<=L&&R<=e&&i!==e){if(r&&!r._initted&&r.data<=ut(i-e))return;!1===ue.inertia&&(l=n-o),A(i,{duration:g(ut(.185*Math.max(ut(c-a),ut(n-a))/s/.05||0)),ease:ue.ease||"power3",data:ut(i-e),onInterrupt:function onInterrupt(){return te.restart(!0)&&f&&f(Ce)},onComplete:function onComplete(){Ce.update(),Pe=Ae(),O&&!ve&&(ee?ee.resetTo("totalProgress",n,O._tTime/O._tDur):O.progress(n)),w=_=O&&!ve?O.totalProgress():Ce.progress,h&&h(Ce),d&&d(Ce)}},e,l*N,i-e-l*N),u&&u(Ce,A.tween)}}}).pause()),a&&(St[a]=Ce),o=(o=(oe=Ce.trigger=J(oe||!0!==ae&&ae))&&oe._gsap&&oe._gsap.stRevert)&&o(Ce),ae=!0===ae?oe:J(ae),ct(S)&&(S={targets:oe,className:S}),ae&&(!1===se||se===mt||(se=!(!se&&ae.parentNode&&ae.parentNode.style&&"flex"===mb(ae.parentNode).display)&&bt),Ce.pin=ae,(n=He.core.getCache(ae)).spacer?X=n.pinState:(l&&((l=J(l))&&!l.nodeType&&(l=l.current||l.nativeElement),n.spacerIsNative=!!l,l&&(n.spacerState=jc(l))),n.spacer=U=l||Xe.createElement("div"),U.classList.add("pin-spacer"),a&&U.classList.add("pin-spacer-"+a),n.pinState=X=jc(ae)),!1!==M.force3D&&He.set(ae,{force3D:!0}),Ce.spacer=U=n.spacer,r=mb(ae),m=r[se+he.os2],G=He.getProperty(ae),b=He.quickSetter(ae,he.a,xt),gc(ae,U,r),V=jc(ae)),_e){e=Va(_e)?ob(_e,_t):_t,Y=Db("scroller-start",a,be,he,e,0),F=Db("scroller-end",a,be,he,e,0,Y),t=Y["offset"+he.op.d2];var u=J(z(be,"content")||be);q=this.markerStart=Db("start",a,u,he,e,t,0,de),I=this.markerEnd=Db("end",a,u,he,e,t,0,de),de&&(T=He.quickSetter([q,I],he.a,xt)),ye||Ie.length&&!0===z(be,"fixedMarkers")||(function _makePositionable(e){var t=mb(e).position;e.style.position="absolute"===t||"fixed"===t?t:"relative"}(me?Je:be),He.set([Y,F],{force3D:!0}),y=He.quickSetter(Y,he.a,xt),x=He.quickSetter(F,he.a,xt))}if(de){var f=de.vars.onUpdate,d=de.vars.onUpdateParams;de.eventCallback("onUpdate",function(){Ce.update(0,0,1),f&&f.apply(de,d||[])})}if(Ce.previous=function(){return Ct[Ct.indexOf(Ce)-1]},Ce.next=function(){return Ct[Ct.indexOf(Ce)+1]},Ce.revert=function(e,t){if(!t)return Ce.kill(!0);var r=!1!==e||!Ce.enabled,n=Ke;r!==Ce.isReverted&&(r&&(re=Math.max(Ae(),Ce.scroll.rec||0),Oe=Ce.progress,ne=O&&O.progress()),q&&[q,I,Y,F].forEach(function(e){return e.style.display=r?"none":"block"}),r&&(Ke=Ce).update(r),!ae||fe&&Ce.isActive||(r?function _swapPinOut(e,t,r){Mt(r);var n=e._gsap;if(n.spacerIsNative)Mt(n.spacerState);else if(e._gsap.swappedIn){var i=t.parentNode;i&&(i.insertBefore(e,t),i.removeChild(t))}e._gsap.swappedIn=!1}(ae,U,X):gc(ae,U,mb(ae),Z)),r||Ce.update(r),Ke=n,Ce.isReverted=r)},Ce.refresh=function(e,t,r,n){if(!Ke&&Ce.enabled||t)if(ae&&e&&st)wb(ScrollTrigger,"scrollEnd",Ob);else{!rt&&Se&&Se(Ce),Ke=Ce,A.tween&&!r&&(A.tween.kill(),A.tween=0),ee&&ee.pause(),le&&O&&O.revert({kill:!1}).invalidate(),Ce.isReverted||Ce.revert(!0,!0),Ce._subPinOffset=!1;var i,o,a,s,l,c,u,f,d,p,g,h,v,b=ke(),m=Ee(),y=de?de.duration():Qa(be,he),x=N<=.01,w=0,_=n||0,T=Va(r)?r.end:M.end,C=M.endTrigger||oe,S=Va(r)?r.start:M.start||(0!==M.start&&oe?ae?"0 0":"0 100%":0),k=Ce.pinnedContainer=M.pinnedContainer&&J(M.pinnedContainer,Ce),E=oe&&Math.max(0,Ct.indexOf(Ce))||0,P=E;for(_e&&Va(r)&&(h=He.getProperty(Y,he.p),v=He.getProperty(F,he.p));0<P--;)(c=Ct[P]).end||c.refresh(0,1)||(Ke=Ce),!(u=c.pin)||u!==oe&&u!==ae&&u!==k||c.isReverted||((p=p||[]).unshift(c),c.revert(!0,!0)),c!==Ct[P]&&(E--,P--);for(Ta(S)&&(S=S(Ce)),S=Ca(S,"start",Ce),R=mc(S,oe,b,he,Ae(),q,Y,Ce,m,Te,ye,y,de,Ce._startClamp&&"_startClamp")||(ae?-.001:0),Ta(T)&&(T=T(Ce)),ct(T)&&!T.indexOf("+=")&&(~T.indexOf(" ")?T=(ct(S)?S.split(" ")[0]:"")+T:(w=Cb(T.substr(2),b),T=ct(S)?S:(de?He.utils.mapRange(0,de.duration(),de.scrollTrigger.start,de.scrollTrigger.end,R):R)+w,C=oe)),T=Ca(T,"end",Ce),L=Math.max(R,mc(T||(C?"100% 0":y),C,b,he,Ae()+w,I,F,Ce,m,Te,ye,y,de,Ce._endClamp&&"_endClamp"))||-.001,w=0,P=E;P--;)(u=(c=Ct[P]).pin)&&c.start-c._pinPush<=R&&!de&&0<c.end&&(i=c.end-(Ce._startClamp?Math.max(0,c.start):c.start),(u===oe&&c.start-c._pinPush<R||u===k)&&isNaN(S)&&(w+=i*(1-c.progress)),u===ae&&(_+=i));if(R+=w,L+=w,Ce._startClamp&&(Ce._startClamp+=w),Ce._endClamp&&!rt&&(Ce._endClamp=L||-.001,L=Math.min(L,Qa(be,he))),N=L-R||(R-=.01)&&.001,x&&(Oe=He.utils.clamp(0,1,He.utils.normalize(R,L,re))),Ce._pinPush=_,q&&w&&((i={})[he.a]="+="+w,k&&(i[he.p]="-="+Ae()),He.set([q,I],i)),!ae||et&&Ce.end>=Qa(be,he)){if(oe&&Ae()&&!de)for(o=oe.parentNode;o&&o!==Je;)o._pinOffset&&(R-=o._pinOffset,L-=o._pinOffset),o=o.parentNode}else i=mb(ae),s=he===ze,a=Ae(),j=parseFloat(G(he.a))+_,!y&&1<L&&(g={style:g=(me?Xe.scrollingElement||We:be).style,value:g["overflow"+he.a.toUpperCase()]},me&&"scroll"!==mb(Je)["overflow"+he.a.toUpperCase()]&&(g.style["overflow"+he.a.toUpperCase()]="scroll")),gc(ae,U,i),V=jc(ae),o=wt(ae,!0),f=ye&&K(be,s?Fe:ze)(),se?((Z=[se+he.os2,N+_+xt]).t=U,(P=se===bt?qb(ae,he)+N+_:0)&&(Z.push(he.d,P+xt),"auto"!==U.style.flexBasis&&(U.style.flexBasis=P+xt)),Mt(Z),k&&Ct.forEach(function(e){e.pin===k&&!1!==e.vars.pinSpacing&&(e._subPinOffset=!0)}),ye&&Ae(re)):(P=qb(ae,he))&&"auto"!==U.style.flexBasis&&(U.style.flexBasis=P+xt),ye&&((l={top:o.top+(s?a-R:f)+xt,left:o.left+(s?f:a-R)+xt,boxSizing:"border-box",position:"fixed"})[ft]=l.maxWidth=Math.ceil(o.width)+xt,l[dt]=l.maxHeight=Math.ceil(o.height)+xt,l[mt]=l[mt+ht]=l[mt+pt]=l[mt+vt]=l[mt+gt]="0",l[bt]=i[bt],l[bt+ht]=i[bt+ht],l[bt+pt]=i[bt+pt],l[bt+vt]=i[bt+vt],l[bt+gt]=i[bt+gt],W=function _copyState(e,t,r){for(var n,i=[],o=e.length,a=r?8:0;a<o;a+=2)n=e[a],i.push(n,n in t?t[n]:e[a+1]);return i.t=e.t,i}(X,l,fe),rt&&Ae(0)),O?(d=O._initted,$e(1),O.render(O.duration(),!0,!0),Q=G(he.a)-j+N+_,$=1<Math.abs(N-Q),ye&&$&&W.splice(W.length-2,2),O.render(0,!0,!0),d||O.invalidate(!0),O.parent||O.totalTime(O.totalTime()),$e(0)):Q=N,g&&(g.value?g.style["overflow"+he.a.toUpperCase()]=g.value:g.style.removeProperty("overflow-"+he.a));p&&p.forEach(function(e){return e.revert(!1,!0)}),Ce.start=R,Ce.end=L,D=B=rt?re:Ae(),de||rt||(D<re&&Ae(re),Ce.scroll.rec=0),Ce.revert(!1,!0),Me=at(),te&&(Pe=-1,te.restart(!0)),Ke=0,O&&ve&&(O._initted||ne)&&O.progress()!==ne&&O.progress(ne||0,!0).render(O.time(),!0,!0),(x||Oe!==Ce.progress||de||le||O&&!O._initted)&&(O&&!ve&&O.totalProgress(de&&R<-.001&&!Oe?He.utils.normalize(R,L,0):Oe,!0),Ce.progress=x||(D-R)/N===Oe?0:Oe),ae&&se&&(U._pinOffset=Math.round(Ce.progress*Q)),ee&&ee.invalidate(),isNaN(h)||(h-=He.getProperty(Y,he.p),v-=He.getProperty(F,he.p),qc(Y,he,h),qc(q,he,h-(n||0)),qc(F,he,v),qc(I,he,v-(n||0))),x&&!rt&&Ce.update(),!ie||rt||H||(H=!0,ie(Ce),H=!1)}},Ce.getVelocity=function(){return(Ae()-B)/(at()-Ge)*1e3||0},Ce.endAnimation=function(){Wa(Ce.callbackAnimation),O&&(ee?ee.progress(1):O.paused()?ve||Wa(O,Ce.direction<0,1):Wa(O,O.reversed()))},Ce.labelToScroll=function(e){return O&&O.labels&&(R||Ce.refresh()||R)+O.labels[e]/O.duration()*N||0},Ce.getTrailing=function(t){var e=Ct.indexOf(Ce),r=0<Ce.direction?Ct.slice(0,e).reverse():Ct.slice(e+1);return(ct(t)?r.filter(function(e){return e.vars.preventOverlaps===t}):r).filter(function(e){return 0<Ce.direction?e.end<=R:e.start>=L})},Ce.update=function(e,t,r){if(!de||r||e){var n,i,o,a,s,l,c,u=!0===rt?re:Ce.scroll(),f=e?0:(u-R)/N,d=f<0?0:1<f?1:f||0,p=Ce.progress;if(t&&(B=D,D=de?Ae():u,ue&&(_=w,w=O&&!ve?O.totalProgress():d)),P&&ae&&!Ke&&!ot&&st&&(!d&&R<u+(u-B)/(at()-Ge)*P?d=1e-4:1===d&&L>u+(u-B)/(at()-Ge)*P&&(d=.9999)),d!==p&&Ce.enabled){if(a=(s=(n=Ce.isActive=!!d&&d<1)!=(!!p&&p<1))||!!d!=!!p,Ce.direction=p<d?1:-1,Ce.progress=d,a&&!Ke&&(i=d&&!p?0:1===d?1:1===p?2:3,ve&&(o=!s&&"none"!==we[i+1]&&we[i+1]||we[i],c=O&&("complete"===o||"reset"===o||o in O))),ge&&(s||c)&&(c||E||!O)&&(Ta(ge)?ge(Ce):Ce.getTrailing(ge).forEach(function(e){return e.endAnimation()})),ve||(!ee||Ke||ot?O&&O.totalProgress(d,!(!Ke||!Me&&!e)):(ee._dp._time-ee._start!==ee._time&&ee.render(ee._dp._time-ee._start),ee.resetTo?ee.resetTo("totalProgress",d,O._tTime/O._tDur):(ee.vars.totalProgress=d,ee.invalidate().restart()))),ae)if(e&&se&&(U.style[se+he.os2]=m),ye){if(a){if(l=!e&&p<d&&u<L+1&&u+1>=Qa(be,he),fe)if(e||!n&&!l)oc(ae,U);else{var g=wt(ae,!0),h=u-R;oc(ae,Je,g.top+(he===ze?h:0)+xt,g.left+(he===ze?0:h)+xt)}Mt(n||l?W:V),$&&d<1&&n||b(j+(1!==d||l?0:Q))}}else b(Ia(j+Q*d));!ue||A.tween||Ke||ot||te.restart(!0),S&&(s||ce&&d&&(d<1||!tt))&&Ve(S.targets).forEach(function(e){return e.classList[n||ce?"add":"remove"](S.className)}),!C||ve||e||C(Ce),a&&!Ke?(ve&&(c&&("complete"===o?O.pause().totalProgress(1):"reset"===o?O.restart(!0).pause():"restart"===o?O.restart(!0):O[o]()),C&&C(Ce)),!s&&tt||(k&&s&&Xa(Ce,k),xe[i]&&Xa(Ce,xe[i]),ce&&(1===d?Ce.kill(!1,1):xe[i]=0),s||xe[i=1===d?1:3]&&Xa(Ce,xe[i])),pe&&!n&&Math.abs(Ce.getVelocity())>(Ua(pe)?pe:2500)&&(Wa(Ce.callbackAnimation),ee?ee.progress(1):Wa(O,"reverse"===o?1:!d,1))):ve&&C&&!Ke&&C(Ce)}if(x){var v=de?u/de.duration()*(de._caScrollDist||0):u;y(v+(Y._isFlipped?1:0)),x(v)}T&&T(-u/de.duration()*(de._caScrollDist||0))}},Ce.enable=function(e,t){Ce.enabled||(Ce.enabled=!0,wb(be,"resize",Lb),me||wb(be,"scroll",Jb),Se&&wb(ScrollTrigger,"refreshInit",Se),!1!==e&&(Ce.progress=Oe=0,D=B=Pe=Ae()),!1!==t&&Ce.refresh())},Ce.getTween=function(e){return e&&A?A.tween:ee},Ce.setPositions=function(e,t,r,n){if(de){var i=de.scrollTrigger,o=de.duration(),a=i.end-i.start;e=i.start+a*e/o,t=i.start+a*t/o}Ce.refresh(!1,!1,{start:Da(e,r&&!!Ce._startClamp),end:Da(t,r&&!!Ce._endClamp)},n),Ce.update()},Ce.adjustPinSpacing=function(e){if(Z&&e){var t=Z.indexOf(he.d)+1;Z[t]=parseFloat(Z[t])+e+xt,Z[1]=parseFloat(Z[1])+e+xt,Mt(Z)}},Ce.disable=function(e,t){if(Ce.enabled&&(!1!==e&&Ce.revert(!0,!0),Ce.enabled=Ce.isActive=!1,t||ee&&ee.pause(),re=0,n&&(n.uncache=1),Se&&xb(ScrollTrigger,"refreshInit",Se),te&&(te.pause(),A.tween&&A.tween.kill()&&(A.tween=0)),!me)){for(var r=Ct.length;r--;)if(Ct[r].scroller===be&&Ct[r]!==Ce)return;xb(be,"resize",Lb),me||xb(be,"scroll",Jb)}},Ce.kill=function(e,t){Ce.disable(e,t),ee&&!t&&ee.kill(),a&&delete St[a];var r=Ct.indexOf(Ce);0<=r&&Ct.splice(r,1),r===Qe&&0<Pt&&Qe--,r=0,Ct.forEach(function(e){return e.scroller===Ce.scroller&&(r=1)}),r||rt||(Ce.scroll.rec=0),O&&(O.scrollTrigger=null,e&&O.revert({kill:!1}),t||O.kill()),q&&[q,I,Y,F].forEach(function(e){return e.parentNode&&e.parentNode.removeChild(e)}),it===Ce&&(it=0),ae&&(n&&(n.uncache=1),r=0,Ct.forEach(function(e){return e.pin===ae&&r++}),r||(n.spacer=0)),M.onKill&&M.onKill(Ce)},Ct.push(Ce),Ce.enable(!1,!1),o&&o(Ce),O&&O.add&&!N){var v=Ce.update;Ce.update=function(){Ce.update=v,qe.cache++,R||L||Ce.refresh()},He.delayedCall(.01,Ce.update),N=.01,R=L=0}else Ce.refresh();ae&&function _queueRefreshAll(){if(nt!==kt){var e=nt=kt;requestAnimationFrame(function(){return e===kt&&Et(!0)})}}()}else this.update=this.refresh=this.kill=Ha},ScrollTrigger.register=function register(e){return s||(He=e||Ka(),Ja()&&window.document&&ScrollTrigger.enable(),s=lt),s},ScrollTrigger.defaults=function defaults(e){if(e)for(var t in e)Tt[t]=e[t];return Tt},ScrollTrigger.disable=function disable(t,r){lt=0,Ct.forEach(function(e){return e[r?"kill":"disable"](t)}),xb(Ne,"wheel",Jb),xb(Xe,"scroll",Jb),clearInterval(u),xb(Xe,"touchcancel",Ha),xb(Je,"touchstart",Ha),vb(xb,Xe,"pointerdown,touchstart,mousedown",Fa),vb(xb,Xe,"pointerup,touchend,mouseup",Ga),c.kill(),Ra(xb);for(var e=0;e<qe.length;e+=3)yb(xb,qe[e],qe[e+1]),yb(xb,qe[e],qe[e+2])},ScrollTrigger.enable=function enable(){if(Ne=window,Xe=document,We=Xe.documentElement,Je=Xe.body,He&&(Ve=He.utils.toArray,Ue=He.utils.clamp,x=He.core.context||Ha,$e=He.core.suppressOverwrites||Ha,w=Ne.history.scrollRestoration||"auto",Q=Ne.pageYOffset||0,He.core.globals("ScrollTrigger",ScrollTrigger),Je)){lt=1,(_=document.createElement("div")).style.height="100vh",_.style.position="absolute",Yb(),function _rafBugFix(){return lt&&requestAnimationFrame(_rafBugFix)}(),k.register(He),ScrollTrigger.isTouch=k.isTouch,E=k.isTouch&&/(iPad|iPhone|iPod|Mac)/g.test(navigator.userAgent),b=1===k.isTouch,wb(Ne,"wheel",Jb),l=[Ne,Xe,We,Je],He.matchMedia?(ScrollTrigger.matchMedia=function(e){var t,r=He.matchMedia();for(t in e)r.add(t,e[t]);return r},He.addEventListener("matchMediaInit",function(){return Sb()}),He.addEventListener("matchMediaRevert",function(){return Rb()}),He.addEventListener("matchMedia",function(){Et(0,1),U("matchMedia")}),He.matchMedia().add("(orientation: portrait)",function(){return Kb(),Kb})):console.warn("Requires GSAP 3.11.0 or later"),Kb(),wb(Xe,"scroll",Jb);var e,t,r=Je.hasAttribute("style"),n=Je.style,i=n.borderTopStyle,o=He.core.Animation.prototype;for(o.revert||Object.defineProperty(o,"revert",{value:function value(){return this.time(-.01,!0)}}),n.borderTopStyle="solid",e=wt(Je),ze.m=Math.round(e.top+ze.sc())||0,Fe.m=Math.round(e.left+Fe.sc())||0,i?n.borderTopStyle=i:n.removeProperty("border-top-style"),r||(Je.setAttribute("style",""),Je.removeAttribute("style")),u=setInterval(Ib,250),He.delayedCall(.5,function(){return ot=0}),wb(Xe,"touchcancel",Ha),wb(Je,"touchstart",Ha),vb(wb,Xe,"pointerdown,touchstart,mousedown",Fa),vb(wb,Xe,"pointerup,touchend,mouseup",Ga),f=He.utils.checkPrefix("transform"),ee.push(f),s=at(),c=He.delayedCall(.2,Et).pause(),g=[Xe,"visibilitychange",function(){var e=Ne.innerWidth,t=Ne.innerHeight;Xe.hidden?(d=e,p=t):d===e&&p===t||Lb()},Xe,"DOMContentLoaded",Et,Ne,"load",Et,Ne,"resize",Lb],Ra(wb),Ct.forEach(function(e){return e.enable(0,1)}),t=0;t<qe.length;t+=3)yb(xb,qe[t],qe[t+1]),yb(xb,qe[t],qe[t+2])}},ScrollTrigger.config=function config(e){"limitCallbacks"in e&&(tt=!!e.limitCallbacks);var t=e.syncInterval;t&&clearInterval(u)||(u=t)&&setInterval(Ib,t),"ignoreMobileResize"in e&&(b=1===ScrollTrigger.isTouch&&e.ignoreMobileResize),"autoRefreshEvents"in e&&(Ra(xb)||Ra(wb,e.autoRefreshEvents||"none"),h=-1===(e.autoRefreshEvents+"").indexOf("resize"))},ScrollTrigger.scrollerProxy=function scrollerProxy(e,t){var r=J(e),n=qe.indexOf(r),i=La(r);~n&&qe.splice(n,i?6:2),t&&(i?Ie.unshift(Ne,t,Je,t,We,t):Ie.unshift(r,t))},ScrollTrigger.clearMatchMedia=function clearMatchMedia(t){Ct.forEach(function(e){return e._ctx&&e._ctx.query===t&&e._ctx.kill(!0,!0)})},ScrollTrigger.isInViewport=function isInViewport(e,t,r){var n=(ct(e)?J(e):e).getBoundingClientRect(),i=n[r?ft:dt]*t||0;return r?0<n.right-i&&n.left+i<Ne.innerWidth:0<n.bottom-i&&n.top+i<Ne.innerHeight},ScrollTrigger.positionInViewport=function positionInViewport(e,t,r){ct(e)&&(e=J(e));var n=e.getBoundingClientRect(),i=n[r?ft:dt],o=null==t?i/2:t in H?H[t]*i:~t.indexOf("%")?parseFloat(t)*i/100:parseFloat(t)||0;return r?(n.left+o)/Ne.innerWidth:(n.top+o)/Ne.innerHeight},ScrollTrigger.killAll=function killAll(e){if(Ct.slice(0).forEach(function(e){return"ScrollSmoother"!==e.vars.id&&e.kill()}),!0!==e){var t=W.killAll||[];W={},t.forEach(function(e){return e()})}},ScrollTrigger);function ScrollTrigger(e,t){s||ScrollTrigger.register(He)||console.warn("Please gsap.registerPlugin(ScrollTrigger)"),x(this),this.init(e,t)}ne.version="3.12.7",ne.saveStyles=function(e){return e?Ve(e).forEach(function(e){if(e&&e.style){var t=j.indexOf(e);0<=t&&j.splice(t,5),j.push(e,e.style.cssText,e.getBBox&&e.getAttribute("transform"),He.core.getCache(e),x())}}):j},ne.revert=function(e,t){return Sb(!e,t)},ne.create=function(e,t){return new ne(e,t)},ne.refresh=function(e){return e?Lb(!0):(s||ne.register())&&Et(!0)},ne.update=function(e){return++qe.cache&&Z(!0===e?2:0)},ne.clearScrollMemory=Tb,ne.maxScroll=function(e,t){return Qa(e,t?Fe:ze)},ne.getScrollFunc=function(e,t){return K(J(e),t?Fe:ze)},ne.getById=function(e){return St[e]},ne.getAll=function(){return Ct.filter(function(e){return"ScrollSmoother"!==e.vars.id})},ne.isScrolling=function(){return!!st},ne.snapDirectional=tb,ne.addEventListener=function(e,t){var r=W[e]||(W[e]=[]);~r.indexOf(t)||r.push(t)},ne.removeEventListener=function(e,t){var r=W[e],n=r&&r.indexOf(t);0<=n&&r.splice(n,1)},ne.batch=function(e,t){function Ep(e,t){var r=[],n=[],i=He.delayedCall(o,function(){t(r,n),r=[],n=[]}).pause();return function(e){r.length||i.restart(!0),r.push(e.trigger),n.push(e),a<=r.length&&i.progress(1)}}var r,n=[],i={},o=t.interval||.016,a=t.batchMax||1e9;for(r in t)i[r]="on"===r.substr(0,2)&&Ta(t[r])&&"onRefreshInit"!==r?Ep(0,t[r]):t[r];return Ta(a)&&(a=a(),wb(ne,"refresh",function(){return a=t.batchMax()})),Ve(e).forEach(function(e){var t={};for(r in i)t[r]=i[r];t.trigger=e,n.push(ne.create(t))}),n};function tc(e,t,r,n){return n<t?e(n):t<0&&e(0),n<r?(n-t)/(r-t):r<0?t/(t-r):1}function uc(e,t){!0===t?e.style.removeProperty("touch-action"):e.style.touchAction=!0===t?"auto":t?"pan-"+t+(k.isTouch?" pinch-zoom":""):"none",e===We&&uc(Je,t)}function wc(e){var t,r=e.event,n=e.target,i=e.axis,o=(r.changedTouches?r.changedTouches[0]:r).target,a=o._gsap||He.core.getCache(o),s=at();if(!a._isScrollT||2e3<s-a._isScrollT){for(;o&&o!==Je&&(o.scrollHeight<=o.clientHeight&&o.scrollWidth<=o.clientWidth||!oe[(t=mb(o)).overflowY]&&!oe[t.overflowX]);)o=o.parentNode;a._isScroll=o&&o!==n&&!La(o)&&(oe[(t=mb(o)).overflowY]||oe[t.overflowX]),a._isScrollT=s}!a._isScroll&&"x"!==i||(r.stopPropagation(),r._gsapAllow=!0)}function xc(e,t,r,n){return k.create({target:e,capture:!0,debounce:!1,lockAxis:!0,type:t,onWheel:n=n&&wc,onPress:n,onDrag:n,onScroll:n,onEnable:function onEnable(){return r&&wb(Xe,k.eventTypes[0],se,!1,!0)},onDisable:function onDisable(){return xb(Xe,k.eventTypes[0],se,!0)}})}function Bc(e){function Bq(){return i=!1}function Eq(){o=Qa(p,ze),S=Ue(E?1:0,o),f&&(C=Ue(0,Qa(p,Fe))),l=kt}function Fq(){v._gsap.y=Ia(parseFloat(v._gsap.y)+b.offset)+"px",v.style.transform="matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, "+parseFloat(v._gsap.y)+", 0, 1)",b.offset=b.cacheID=0}function Lq(){Eq(),a.isActive()&&a.vars.scrollY>o&&(b()>o?a.progress(1)&&b(o):a.resetTo("scrollY",o))}Va(e)||(e={}),e.preventDefault=e.isNormalizer=e.allowClicks=!0,e.type||(e.type="wheel,touch"),e.debounce=!!e.debounce,e.id=e.id||"normalizer";var n,o,l,i,a,c,u,s,f=e.normalizeScrollX,t=e.momentum,r=e.allowNestedScroll,d=e.onRelease,p=J(e.target)||We,g=He.core.globals().ScrollSmoother,h=g&&g.get(),v=E&&(e.content&&J(e.content)||h&&!1!==e.content&&!h.smooth()&&h.content()),b=K(p,ze),m=K(p,Fe),y=1,x=(k.isTouch&&Ne.visualViewport?Ne.visualViewport.scale*Ne.visualViewport.width:Ne.outerWidth)/Ne.innerWidth,w=0,_=Ta(t)?function(){return t(n)}:function(){return t||2.8},T=xc(p,e.type,!0,r),C=Ha,S=Ha;return v&&He.set(v,{y:"+=0"}),e.ignoreCheck=function(e){return E&&"touchmove"===e.type&&function ignoreDrag(){if(i){requestAnimationFrame(Bq);var e=Ia(n.deltaY/2),t=S(b.v-e);if(v&&t!==b.v+b.offset){b.offset=t-b.v;var r=Ia((parseFloat(v&&v._gsap.y)||0)-b.offset);v.style.transform="matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, "+r+", 0, 1)",v._gsap.y=r+"px",b.cacheID=qe.cache,Z()}return!0}b.offset&&Fq(),i=!0}()||1.05<y&&"touchstart"!==e.type||n.isGesturing||e.touches&&1<e.touches.length},e.onPress=function(){i=!1;var e=y;y=Ia((Ne.visualViewport&&Ne.visualViewport.scale||1)/x),a.pause(),e!==y&&uc(p,1.01<y||!f&&"x"),c=m(),u=b(),Eq(),l=kt},e.onRelease=e.onGestureStart=function(e,t){if(b.offset&&Fq(),t){qe.cache++;var r,n,i=_();f&&(n=(r=m())+.05*i*-e.velocityX/.227,i*=tc(m,r,n,Qa(p,Fe)),a.vars.scrollX=C(n)),n=(r=b())+.05*i*-e.velocityY/.227,i*=tc(b,r,n,Qa(p,ze)),a.vars.scrollY=S(n),a.invalidate().duration(i).play(.01),(E&&a.vars.scrollY>=o||o-1<=r)&&He.to({},{onUpdate:Lq,duration:i})}else s.restart(!0);d&&d(e)},e.onWheel=function(){a._ts&&a.pause(),1e3<at()-w&&(l=0,w=at())},e.onChange=function(e,t,r,n,i){if(kt!==l&&Eq(),t&&f&&m(C(n[2]===t?c+(e.startX-e.x):m()+t-n[1])),r){b.offset&&Fq();var o=i[2]===r,a=o?u+e.startY-e.y:b()+r-i[1],s=S(a);o&&a!==s&&(u+=s-a),b(s)}(r||t)&&Z()},e.onEnable=function(){uc(p,!f&&"x"),ne.addEventListener("refresh",Lq),wb(Ne,"resize",Lq),b.smooth&&(b.target.style.scrollBehavior="auto",b.smooth=m.smooth=!1),T.enable()},e.onDisable=function(){uc(p,!0),xb(Ne,"resize",Lq),ne.removeEventListener("refresh",Lq),T.kill()},e.lockAxis=!1!==e.lockAxis,((n=new k(e)).iOS=E)&&!b()&&b(1),E&&He.ticker.add(Ha),s=n._dc,a=He.to(n,{ease:"power4",paused:!0,inherit:!1,scrollX:f?"+=0.1":"+=0",scrollY:"+=0.1",modifiers:{scrollY:pc(b,b(),function(){return a.pause()})},onUpdate:Z,onComplete:s.vars.onComplete}),n}var ie,oe={auto:1,scroll:1},ae=/(input|label|select|textarea)/i,se=function _captureInputs(e){var t=ae.test(e.target.tagName);(t||ie)&&(e._gsapAllow=!0,ie=t)};ne.sort=function(e){if(Ta(e))return Ct.sort(e);var t=Ne.pageYOffset||0;return ne.getAll().forEach(function(e){return e._sortY=e.trigger?t+e.trigger.getBoundingClientRect().top:e.start+Ne.innerHeight}),Ct.sort(e||function(e,t){return-1e6*(e.vars.refreshPriority||0)+(e.vars.containerAnimation?1e6:e._sortY)-((t.vars.containerAnimation?1e6:t._sortY)+-1e6*(t.vars.refreshPriority||0))})},ne.observe=function(e){return new k(e)},ne.normalizeScroll=function(e){if(void 0===e)return v;if(!0===e&&v)return v.enable();if(!1===e)return v&&v.kill(),void(v=e);var t=e instanceof k?e:Bc(e);return v&&v.target===t.target&&v.kill(),La(t.target)&&(v=t),t},ne.core={_getVelocityProp:L,_inputObserver:xc,_scrollers:qe,_proxies:Ie,bridge:{ss:function ss(){st||U("scrollStart"),st=at()},ref:function ref(){return Ke}}},Ka()&&He.registerPlugin(ne),e.ScrollTrigger=ne,e.default=ne;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/ScrollTrigger.min.js.map b/node_modules/gsap/dist/ScrollTrigger.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..468b86f0680ce2474f1aba33bd0de06e0c001b09 --- /dev/null +++ b/node_modules/gsap/dist/ScrollTrigger.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"ScrollTrigger.min.js","sources":["../src/Observer.js","../src/ScrollTrigger.js"],"sourcesContent":["/*!\n * Observer 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet gsap, _coreInitted, _clamp, _win, _doc, _docEl, _body, _isTouch, _pointerType, ScrollTrigger, _root, _normalizer, _eventTypes, _context,\n\t_getGSAP = () => gsap || (typeof(window) !== \"undefined\" && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_startup = 1,\n\t_observers = [],\n\t_scrollers = [],\n\t_proxies = [],\n\t_getTime = Date.now,\n\t_bridge = (name, value) => value,\n\t_integrate = () => {\n\t\tlet core = ScrollTrigger.core,\n\t\t\tdata = core.bridge || {},\n\t\t\tscrollers = core._scrollers,\n\t\t\tproxies = core._proxies;\n\t\tscrollers.push(..._scrollers);\n\t\tproxies.push(..._proxies);\n\t\t_scrollers = scrollers;\n\t\t_proxies = proxies;\n\t\t_bridge = (name, value) => data[name](value);\n\t},\n\t_getProxyProp = (element, property) => ~_proxies.indexOf(element) && _proxies[_proxies.indexOf(element) + 1][property],\n\t_isViewport = el => !!~_root.indexOf(el),\n\t_addListener = (element, type, func, passive, capture) => element.addEventListener(type, func, {passive: passive !== false, capture: !!capture}),\n\t_removeListener = (element, type, func, capture) => element.removeEventListener(type, func, !!capture),\n\t_scrollLeft = \"scrollLeft\",\n\t_scrollTop = \"scrollTop\",\n\t_onScroll = () => (_normalizer && _normalizer.isPressed) || _scrollers.cache++,\n\t_scrollCacheFunc = (f, doNotCache) => {\n\t\tlet cachingFunc = value => { // since reading the scrollTop/scrollLeft/pageOffsetY/pageOffsetX can trigger a layout, this function allows us to cache the value so it only gets read fresh after a \"scroll\" event fires (or while we're refreshing because that can lengthen the page and alter the scroll position). when \"soft\" is true, that means don't actually set the scroll, but cache the new value instead (useful in ScrollSmoother)\n\t\t\tif (value || value === 0) {\n\t\t\t\t_startup && (_win.history.scrollRestoration = \"manual\"); // otherwise the new position will get overwritten by the browser onload.\n\t\t\t\tlet isNormalizing = _normalizer && _normalizer.isPressed;\n\t\t\t\tvalue = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); //TODO: iOS Bug: if you allow it to go to 0, Safari can start to report super strange (wildly inaccurate) touch positions!\n\t\t\t\tf(value);\n\t\t\t\tcachingFunc.cacheID = _scrollers.cache;\n\t\t\t\tisNormalizing && _bridge(\"ss\", value); // set scroll (notify ScrollTrigger so it can dispatch a \"scrollStart\" event if necessary\n\t\t\t} else if (doNotCache || _scrollers.cache !== cachingFunc.cacheID || _bridge(\"ref\")) {\n\t\t\t\tcachingFunc.cacheID = _scrollers.cache;\n\t\t\t\tcachingFunc.v = f();\n\t\t\t}\n\t\t\treturn cachingFunc.v + cachingFunc.offset;\n\t\t};\n\t\tcachingFunc.offset = 0;\n\t\treturn f && cachingFunc;\n\t},\n\t_horizontal = {s: _scrollLeft, p: \"left\", p2: \"Left\", os: \"right\", os2: \"Right\", d: \"width\", d2: \"Width\", a: \"x\", sc: _scrollCacheFunc(function(value) { return arguments.length ? _win.scrollTo(value, _vertical.sc()) : _win.pageXOffset || _doc[_scrollLeft] || _docEl[_scrollLeft] || _body[_scrollLeft] || 0})},\n\t_vertical = {s: _scrollTop, p: \"top\", p2: \"Top\", os: \"bottom\", os2: \"Bottom\", d: \"height\", d2: \"Height\", a: \"y\", op: _horizontal, sc: _scrollCacheFunc(function(value) { return arguments.length ? _win.scrollTo(_horizontal.sc(), value) : _win.pageYOffset || _doc[_scrollTop] || _docEl[_scrollTop] || _body[_scrollTop] || 0})},\n\t_getTarget = (t, self) => ((self && self._ctx && self._ctx.selector) || gsap.utils.toArray)(t)[0] || (typeof(t) === \"string\" && gsap.config().nullTargetWarn !== false ? console.warn(\"Element not found:\", t) : null),\n\n\t_getScrollFunc = (element, {s, sc}) => { // we store the scroller functions in an alternating sequenced Array like [element, verticalScrollFunc, horizontalScrollFunc, ...] so that we can minimize memory, maximize performance, and we also record the last position as a \".rec\" property in order to revert to that after refreshing to ensure things don't shift around.\n\t\t_isViewport(element) && (element = _doc.scrollingElement || _docEl);\n\t\tlet i = _scrollers.indexOf(element),\n\t\t\toffset = sc === _vertical.sc ? 1 : 2;\n\t\t!~i && (i = _scrollers.push(element) - 1);\n\t\t_scrollers[i + offset] || _addListener(element, \"scroll\", _onScroll); // clear the cache when a scroll occurs\n\t\tlet prev = _scrollers[i + offset],\n\t\t\tfunc = prev || (_scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function(value) { return arguments.length ? (element[s] = value) : element[s]; })));\n\t\tfunc.target = element;\n\t\tprev || (func.smooth = gsap.getProperty(element, \"scrollBehavior\") === \"smooth\"); // only set it the first time (don't reset every time a scrollFunc is requested because perhaps it happens during a refresh() when it's disabled in ScrollTrigger.\n\t\treturn func;\n\t},\n\t_getVelocityProp = (value, minTimeRefresh, useDelta) => {\n\t\tlet v1 = value,\n\t\t\tv2 = value,\n\t\t\tt1 = _getTime(),\n\t\t\tt2 = t1,\n\t\t\tmin = minTimeRefresh || 50,\n\t\t\tdropToZeroTime = Math.max(500, min * 3),\n\t\t\tupdate = (value, force) => {\n\t\t\t\tlet t = _getTime();\n\t\t\t\tif (force || t - t1 > min) {\n\t\t\t\t\tv2 = v1;\n\t\t\t\t\tv1 = value;\n\t\t\t\t\tt2 = t1;\n\t\t\t\t\tt1 = t;\n\t\t\t\t} else if (useDelta) {\n\t\t\t\t\tv1 += value;\n\t\t\t\t} else { // not totally necessary, but makes it a bit more accurate by adjusting the v1 value according to the new slope. This way we're not just ignoring the incoming data. Removing for now because it doesn't seem to make much practical difference and it's probably not worth the kb.\n\t\t\t\t\tv1 = v2 + (value - v2) / (t - t2) * (t1 - t2);\n\t\t\t\t}\n\t\t\t},\n\t\t\treset = () => { v2 = v1 = useDelta ? 0 : v1; t2 = t1 = 0; },\n\t\t\tgetVelocity = latestValue => {\n\t\t\t\tlet tOld = t2,\n\t\t\t\t\tvOld = v2,\n\t\t\t\t\tt = _getTime();\n\t\t\t\t(latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue);\n\t\t\t\treturn (t1 === t2 || t - t2 > dropToZeroTime) ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000;\n\t\t\t};\n\t\treturn {update, reset, getVelocity};\n\t},\n\t_getEvent = (e, preventDefault) => {\n\t\tpreventDefault && !e._gsapAllow && e.preventDefault();\n\t\treturn e.changedTouches ? e.changedTouches[0] : e;\n\t},\n\t_getAbsoluteMax = a => {\n\t\tlet max = Math.max(...a),\n\t\t\tmin = Math.min(...a);\n\t\treturn Math.abs(max) >= Math.abs(min) ? max : min;\n\t},\n\t_setScrollTrigger = () => {\n\t\tScrollTrigger = gsap.core.globals().ScrollTrigger;\n\t\tScrollTrigger && ScrollTrigger.core && _integrate();\n\t},\n\t_initCore = core => {\n\t\tgsap = core || _getGSAP();\n\t\tif (!_coreInitted && gsap && typeof(document) !== \"undefined\" && document.body) {\n\t\t\t_win = window;\n\t\t\t_doc = document;\n\t\t\t_docEl = _doc.documentElement;\n\t\t\t_body = _doc.body;\n\t\t\t_root = [_win, _doc, _docEl, _body];\n\t\t\t_clamp = gsap.utils.clamp;\n\t\t\t_context = gsap.core.context || function() {};\n\t\t\t_pointerType = \"onpointerenter\" in _body ? \"pointer\" : \"mouse\";\n\t\t\t// isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer.\n\t\t\t_isTouch = Observer.isTouch = _win.matchMedia && _win.matchMedia(\"(hover: none), (pointer: coarse)\").matches ? 1 : (\"ontouchstart\" in _win || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0) ? 2 : 0;\n\t\t\t_eventTypes = Observer.eventTypes = (\"ontouchstart\" in _docEl ? \"touchstart,touchmove,touchcancel,touchend\" : !(\"onpointerdown\" in _docEl) ? \"mousedown,mousemove,mouseup,mouseup\" : \"pointerdown,pointermove,pointercancel,pointerup\").split(\",\");\n\t\t\tsetTimeout(() => _startup = 0, 500);\n\t\t\t_setScrollTrigger();\n\t\t\t_coreInitted = 1;\n\t\t}\n\t\treturn _coreInitted;\n\t};\n\n_horizontal.op = _vertical;\n_scrollers.cache = 0;\n\nexport class Observer {\n\tconstructor(vars) {\n\t\tthis.init(vars);\n\t}\n\n\tinit(vars) {\n\t\t_coreInitted || _initCore(gsap) || console.warn(\"Please gsap.registerPlugin(Observer)\");\n\t\tScrollTrigger || _setScrollTrigger();\n\t\tlet {tolerance, dragMinimum, type, target, lineHeight, debounce, preventDefault, onStop, onStopDelay, ignore, wheelSpeed, event, onDragStart, onDragEnd, onDrag, onPress, onRelease, onRight, onLeft, onUp, onDown, onChangeX, onChangeY, onChange, onToggleX, onToggleY, onHover, onHoverEnd, onMove, ignoreCheck, isNormalizer, onGestureStart, onGestureEnd, onWheel, onEnable, onDisable, onClick, scrollSpeed, capture, allowClicks, lockAxis, onLockAxis} = vars;\n\t\tthis.target = target = _getTarget(target) || _docEl;\n\t\tthis.vars = vars;\n\t\tignore && (ignore = gsap.utils.toArray(ignore));\n\t\ttolerance = tolerance || 1e-9;\n\t\tdragMinimum = dragMinimum || 0;\n\t\twheelSpeed = wheelSpeed || 1;\n\t\tscrollSpeed = scrollSpeed || 1;\n\t\ttype = type || \"wheel,touch,pointer\";\n\t\tdebounce = debounce !== false;\n\t\tlineHeight || (lineHeight = parseFloat(_win.getComputedStyle(_body).lineHeight) || 22); // note: browser may report \"normal\", so default to 22.\n\t\tlet id, onStopDelayedCall, dragged, moved, wheeled, locked, axis,\n\t\t\tself = this,\n\t\t\tprevDeltaX = 0,\n\t\t\tprevDeltaY = 0,\n\t\t\tpassive = vars.passive || (!preventDefault && vars.passive !== false),\n\t\t\tscrollFuncX = _getScrollFunc(target, _horizontal),\n\t\t\tscrollFuncY = _getScrollFunc(target, _vertical),\n\t\t\tscrollX = scrollFuncX(),\n\t\t\tscrollY = scrollFuncY(),\n\t\t\tlimitToTouch = ~type.indexOf(\"touch\") && !~type.indexOf(\"pointer\") && _eventTypes[0] === \"pointerdown\", // for devices that accommodate mouse events and touch events, we need to distinguish.\n\t\t\tisViewport = _isViewport(target),\n\t\t\townerDoc = target.ownerDocument || _doc,\n\t\t\tdeltaX = [0, 0, 0], // wheel, scroll, pointer/touch\n\t\t\tdeltaY = [0, 0, 0],\n\t\t\tonClickTime = 0,\n\t\t\tclickCapture = () => onClickTime = _getTime(),\n\t\t\t_ignoreCheck = (e, isPointerOrTouch) => (self.event = e) && (ignore && ~ignore.indexOf(e.target)) || (isPointerOrTouch && limitToTouch && e.pointerType !== \"touch\") || (ignoreCheck && ignoreCheck(e, isPointerOrTouch)),\n\t\t\tonStopFunc = () => {\n\t\t\t\tself._vx.reset();\n\t\t\t\tself._vy.reset();\n\t\t\t\tonStopDelayedCall.pause();\n\t\t\t\tonStop && onStop(self);\n\t\t\t},\n\t\t\tupdate = () => {\n\t\t\t\tlet dx = self.deltaX = _getAbsoluteMax(deltaX),\n\t\t\t\t\tdy = self.deltaY = _getAbsoluteMax(deltaY),\n\t\t\t\t\tchangedX = Math.abs(dx) >= tolerance,\n\t\t\t\t\tchangedY = Math.abs(dy) >= tolerance;\n\t\t\t\tonChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); // in ScrollTrigger.normalizeScroll(), we need to know if it was touch/pointer so we need access to the deltaX/deltaY Arrays before we clear them out.\n\t\t\t\tif (changedX) {\n\t\t\t\t\tonRight && self.deltaX > 0 && onRight(self);\n\t\t\t\t\tonLeft && self.deltaX < 0 && onLeft(self);\n\t\t\t\t\tonChangeX && onChangeX(self);\n\t\t\t\t\tonToggleX && ((self.deltaX < 0) !== (prevDeltaX < 0)) && onToggleX(self);\n\t\t\t\t\tprevDeltaX = self.deltaX;\n\t\t\t\t\tdeltaX[0] = deltaX[1] = deltaX[2] = 0\n\t\t\t\t}\n\t\t\t\tif (changedY) {\n\t\t\t\t\tonDown && self.deltaY > 0 && onDown(self);\n\t\t\t\t\tonUp && self.deltaY < 0 && onUp(self);\n\t\t\t\t\tonChangeY && onChangeY(self);\n\t\t\t\t\tonToggleY && ((self.deltaY < 0) !== (prevDeltaY < 0)) && onToggleY(self);\n\t\t\t\t\tprevDeltaY = self.deltaY;\n\t\t\t\t\tdeltaY[0] = deltaY[1] = deltaY[2] = 0\n\t\t\t\t}\n\t\t\t\tif (moved || dragged) {\n\t\t\t\t\tonMove && onMove(self);\n\t\t\t\t\tif (dragged) {\n\t\t\t\t\t\tonDragStart && dragged === 1 && onDragStart(self);\n\t\t\t\t\t\tonDrag && onDrag(self);\n\t\t\t\t\t\tdragged = 0;\n\t\t\t\t\t}\n\t\t\t\t\tmoved = false;\n\t\t\t\t}\n\t\t\t\tlocked && !(locked = false) && onLockAxis && onLockAxis(self);\n\t\t\t\tif (wheeled) {\n\t\t\t\t\tonWheel(self);\n\t\t\t\t\twheeled = false;\n\t\t\t\t}\n\t\t\t\tid = 0;\n\t\t\t},\n\t\t\tonDelta = (x, y, index) => {\n\t\t\t\tdeltaX[index] += x;\n\t\t\t\tdeltaY[index] += y;\n\t\t\t\tself._vx.update(x);\n\t\t\t\tself._vy.update(y);\n\t\t\t\tdebounce ? id || (id = requestAnimationFrame(update)) : update();\n\t\t\t},\n\t\t\tonTouchOrPointerDelta = (x, y) => {\n\t\t\t\tif (lockAxis && !axis) {\n\t\t\t\t\tself.axis = axis = Math.abs(x) > Math.abs(y) ? \"x\" : \"y\";\n\t\t\t\t\tlocked = true;\n\t\t\t\t}\n\t\t\t\tif (axis !== \"y\") {\n\t\t\t\t\tdeltaX[2] += x;\n\t\t\t\t\tself._vx.update(x, true); // update the velocity as frequently as possible instead of in the debounced function so that very quick touch-scrolls (flicks) feel natural. If it's the mouse/touch/pointer, force it so that we get snappy/accurate momentum scroll.\n\t\t\t\t}\n\t\t\t\tif (axis !== \"x\") {\n\t\t\t\t\tdeltaY[2] += y;\n\t\t\t\t\tself._vy.update(y, true);\n\t\t\t\t}\n\t\t\t\tdebounce ? id || (id = requestAnimationFrame(update)) : update();\n\t\t\t},\n\t\t\t_onDrag = e => {\n\t\t\t\tif (_ignoreCheck(e, 1)) {return;}\n\t\t\t\te = _getEvent(e, preventDefault);\n\t\t\t\tlet x = e.clientX,\n\t\t\t\t\ty = e.clientY,\n\t\t\t\t\tdx = x - self.x,\n\t\t\t\t\tdy = y - self.y,\n\t\t\t\t\tisDragging = self.isDragging;\n\t\t\t\tself.x = x;\n\t\t\t\tself.y = y;\n\t\t\t\tif (isDragging || ((dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum))) {\n\t\t\t\t\tdragged = isDragging ? 2 : 1; // dragged: 0 = not dragging, 1 = first drag, 2 = normal drag\n\t\t\t\t\tisDragging || (self.isDragging = true);\n\t\t\t\t\tonTouchOrPointerDelta(dx, dy);\n\t\t\t\t}\n\t\t\t},\n\t\t\t_onPress = self.onPress = e => {\n\t\t\t\tif (_ignoreCheck(e, 1) || (e && e.button)) {return;}\n\t\t\t\tself.axis = axis = null;\n\t\t\t\tonStopDelayedCall.pause();\n\t\t\t\tself.isPressed = true;\n\t\t\t\te = _getEvent(e); // note: may need to preventDefault(?) Won't side-scroll on iOS Safari if we do, though.\n\t\t\t\tprevDeltaX = prevDeltaY = 0;\n\t\t\t\tself.startX = self.x = e.clientX;\n\t\t\t\tself.startY = self.y = e.clientY;\n\t\t\t\tself._vx.reset(); // otherwise the t2 may be stale if the user touches and flicks super fast and releases in less than 2 requestAnimationFrame ticks, causing velocity to be 0.\n\t\t\t\tself._vy.reset();\n\t\t\t\t_addListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true);\n\t\t\t\tself.deltaX = self.deltaY = 0;\n\t\t\t\tonPress && onPress(self);\n\t\t\t},\n\t\t\t_onRelease = self.onRelease = e => {\n\t\t\t\tif (_ignoreCheck(e, 1)) {return;}\n\t\t\t\t_removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true);\n\t\t\t\tlet isTrackingDrag = !isNaN(self.y - self.startY),\n\t\t\t\t\twasDragging = self.isDragging,\n\t\t\t\t\tisDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), // some touch devices need some wiggle room in terms of sensing clicks - the finger may move a few pixels.\n\t\t\t\t\teventData = _getEvent(e);\n\t\t\t\tif (!isDragNotClick && isTrackingDrag) {\n\t\t\t\t\tself._vx.reset();\n\t\t\t\t\tself._vy.reset();\n\t\t\t\t\t//if (preventDefault && allowClicks && self.isPressed) { // check isPressed because in a rare edge case, the inputObserver in ScrollTrigger may stopPropagation() on the press/drag, so the onRelease may get fired without the onPress/onDrag ever getting called, thus it could trigger a click to occur on a link after scroll-dragging it.\n\t\t\t\t\tif (preventDefault && allowClicks) {\n\t\t\t\t\t\tgsap.delayedCall(0.08, () => { // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular \"click\" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the \"real\"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the \"real\" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched.\n\t\t\t\t\t\t\tif (_getTime() - onClickTime > 300 && !e.defaultPrevented) {\n\t\t\t\t\t\t\t\tif (e.target.click) { //some browsers (like mobile Safari) don't properly trigger the click event\n\t\t\t\t\t\t\t\t\te.target.click();\n\t\t\t\t\t\t\t\t} else if (ownerDoc.createEvent) {\n\t\t\t\t\t\t\t\t\tlet syntheticEvent = ownerDoc.createEvent(\"MouseEvents\");\n\t\t\t\t\t\t\t\t\tsyntheticEvent.initMouseEvent(\"click\", true, true, _win, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null);\n\t\t\t\t\t\t\t\t\te.target.dispatchEvent(syntheticEvent);\n\t\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t});\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tself.isDragging = self.isGesturing = self.isPressed = false;\n\t\t\t\tonStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true);\n\t\t\t\tdragged && update(); // in case debouncing, we don't want onDrag to fire AFTER onDragEnd().\n\t\t\t\tonDragEnd && wasDragging && onDragEnd(self);\n\t\t\t\tonRelease && onRelease(self, isDragNotClick);\n\t\t\t},\n\t\t\t_onGestureStart = e => e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging),\n\t\t\t_onGestureEnd = () => (self.isGesturing = false) || onGestureEnd(self),\n\t\t\tonScroll = e => {\n\t\t\t\tif (_ignoreCheck(e)) {return;}\n\t\t\t\tlet x = scrollFuncX(),\n\t\t\t\t\ty = scrollFuncY();\n\t\t\t\tonDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1);\n\t\t\t\tscrollX = x;\n\t\t\t\tscrollY = y;\n\t\t\t\tonStop && onStopDelayedCall.restart(true);\n\t\t\t},\n\t\t\t_onWheel = e => {\n\t\t\t\tif (_ignoreCheck(e)) {return;}\n\t\t\t\te = _getEvent(e, preventDefault);\n\t\t\t\tonWheel && (wheeled = true);\n\t\t\t\tlet multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win.innerHeight : 1) * wheelSpeed;\n\t\t\t\tonDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0);\n\t\t\t\tonStop && !isNormalizer && onStopDelayedCall.restart(true);\n\t\t\t},\n\t\t\t_onMove = e => {\n\t\t\t\tif (_ignoreCheck(e)) {return;}\n\t\t\t\tlet x = e.clientX,\n\t\t\t\t\ty = e.clientY,\n\t\t\t\t\tdx = x - self.x,\n\t\t\t\t\tdy = y - self.y;\n\t\t\t\tself.x = x;\n\t\t\t\tself.y = y;\n\t\t\t\tmoved = true;\n\t\t\t\tonStop && onStopDelayedCall.restart(true);\n\t\t\t\t(dx || dy) && onTouchOrPointerDelta(dx, dy);\n\t\t\t},\n\t\t\t_onHover = e => {self.event = e; onHover(self);},\n\t\t\t_onHoverEnd = e => {self.event = e; onHoverEnd(self);},\n\t\t\t_onClick = e => _ignoreCheck(e) || (_getEvent(e, preventDefault) && onClick(self));\n\n\t\tonStopDelayedCall = self._dc = gsap.delayedCall(onStopDelay || 0.25, onStopFunc).pause();\n\n\t\tself.deltaX = self.deltaY = 0;\n\t\tself._vx = _getVelocityProp(0, 50, true);\n\t\tself._vy = _getVelocityProp(0, 50, true);\n\t\tself.scrollX = scrollFuncX;\n\t\tself.scrollY = scrollFuncY;\n\t\tself.isDragging = self.isGesturing = self.isPressed = false;\n\t\t_context(this);\n\t\tself.enable = e => {\n\t\t\tif (!self.isEnabled) {\n\t\t\t\t_addListener(isViewport ? ownerDoc : target, \"scroll\", _onScroll);\n\t\t\t\ttype.indexOf(\"scroll\") >= 0 && _addListener(isViewport ? ownerDoc : target, \"scroll\", onScroll, passive, capture);\n\t\t\t\ttype.indexOf(\"wheel\") >= 0 && _addListener(target, \"wheel\", _onWheel, passive, capture);\n\t\t\t\tif ((type.indexOf(\"touch\") >= 0 && _isTouch) || type.indexOf(\"pointer\") >= 0) {\n\t\t\t\t\t_addListener(target, _eventTypes[0], _onPress, passive, capture);\n\t\t\t\t\t_addListener(ownerDoc, _eventTypes[2], _onRelease);\n\t\t\t\t\t_addListener(ownerDoc, _eventTypes[3], _onRelease);\n\t\t\t\t\tallowClicks && _addListener(target, \"click\", clickCapture, true, true);\n\t\t\t\t\tonClick && _addListener(target, \"click\", _onClick);\n\t\t\t\t\tonGestureStart && _addListener(ownerDoc, \"gesturestart\", _onGestureStart);\n\t\t\t\t\tonGestureEnd && _addListener(ownerDoc, \"gestureend\", _onGestureEnd);\n\t\t\t\t\tonHover && _addListener(target, _pointerType + \"enter\", _onHover);\n\t\t\t\t\tonHoverEnd && _addListener(target, _pointerType + \"leave\", _onHoverEnd);\n\t\t\t\t\tonMove && _addListener(target, _pointerType + \"move\", _onMove);\n\t\t\t\t}\n\t\t\t\tself.isEnabled = true;\n\t\t\t\tself.isDragging = self.isGesturing = self.isPressed = moved = dragged = false;\n\t\t\t\tself._vx.reset();\n\t\t\t\tself._vy.reset();\n\t\t\t\tscrollX = scrollFuncX();\n\t\t\t\tscrollY = scrollFuncY();\n\t\t\t\te && e.type && _onPress(e);\n\t\t\t\tonEnable && onEnable(self);\n\t\t\t}\n\t\t\treturn self;\n\t\t};\n\t\tself.disable = () => {\n\t\t\tif (self.isEnabled) {\n\t\t\t\t// only remove the _onScroll listener if there aren't any others that rely on the functionality.\n\t\t\t\t_observers.filter(o => o !== self && _isViewport(o.target)).length || _removeListener(isViewport ? ownerDoc : target, \"scroll\", _onScroll);\n\t\t\t\tif (self.isPressed) {\n\t\t\t\t\tself._vx.reset();\n\t\t\t\t\tself._vy.reset();\n\t\t\t\t\t_removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true);\n\t\t\t\t}\n\t\t\t\t_removeListener(isViewport ? ownerDoc : target, \"scroll\", onScroll, capture);\n\t\t\t\t_removeListener(target, \"wheel\", _onWheel, capture);\n\t\t\t\t_removeListener(target, _eventTypes[0], _onPress, capture);\n\t\t\t\t_removeListener(ownerDoc, _eventTypes[2], _onRelease);\n\t\t\t\t_removeListener(ownerDoc, _eventTypes[3], _onRelease);\n\t\t\t\t_removeListener(target, \"click\", clickCapture, true);\n\t\t\t\t_removeListener(target, \"click\", _onClick);\n\t\t\t\t_removeListener(ownerDoc, \"gesturestart\", _onGestureStart);\n\t\t\t\t_removeListener(ownerDoc, \"gestureend\", _onGestureEnd);\n\t\t\t\t_removeListener(target, _pointerType + \"enter\", _onHover);\n\t\t\t\t_removeListener(target, _pointerType + \"leave\", _onHoverEnd);\n\t\t\t\t_removeListener(target, _pointerType + \"move\", _onMove);\n\t\t\t\tself.isEnabled = self.isPressed = self.isDragging = false;\n\t\t\t\tonDisable && onDisable(self);\n\t\t\t}\n\t\t};\n\n\t\tself.kill = self.revert = () => {\n\t\t\tself.disable();\n\t\t\tlet i = _observers.indexOf(self);\n\t\t\ti >= 0 && _observers.splice(i, 1);\n\t\t\t_normalizer === self && (_normalizer = 0);\n\t\t}\n\n\t\t_observers.push(self);\n\t\tisNormalizer && _isViewport(target) && (_normalizer = self);\n\n\t\tself.enable(event);\n\t}\n\n\tget velocityX() {\n\t\treturn this._vx.getVelocity();\n\t}\n\tget velocityY() {\n\t\treturn this._vy.getVelocity();\n\t}\n\n}\n\nObserver.version = \"3.12.7\";\nObserver.create = vars => new Observer(vars);\nObserver.register = _initCore;\nObserver.getAll = () => _observers.slice();\nObserver.getById = id => _observers.filter(o => o.vars.id === id)[0];\n\n_getGSAP() && gsap.registerPlugin(Observer);\n\nexport { Observer as default, _isViewport, _scrollers, _getScrollFunc, _getProxyProp, _proxies, _getVelocityProp, _vertical, _horizontal, _getTarget };","/*!\n * ScrollTrigger 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nimport { Observer, _getTarget, _vertical, _horizontal, _scrollers, _proxies, _getScrollFunc, _getProxyProp, _getVelocityProp } from \"./Observer.js\";\n\nlet gsap, _coreInitted, _win, _doc, _docEl, _body, _root, _resizeDelay, _toArray, _clamp, _time2, _syncInterval, _refreshing, _pointerIsDown, _transformProp, _i, _prevWidth, _prevHeight, _autoRefresh, _sort, _suppressOverwrites, _ignoreResize, _normalizer, _ignoreMobileResize, _baseScreenHeight, _baseScreenWidth, _fixIOSBug, _context, _scrollRestoration, _div100vh, _100vh, _isReverted, _clampingMax,\n\t_limitCallbacks, // if true, we'll only trigger callbacks if the active state toggles, so if you scroll immediately past both the start and end positions of a ScrollTrigger (thus inactive to inactive), neither its onEnter nor onLeave will be called. This is useful during startup.\n\t_startup = 1,\n\t_getTime = Date.now,\n\t_time1 = _getTime(),\n\t_lastScrollTime = 0,\n\t_enabled = 0,\n\t_parseClamp = (value, type, self) => {\n\t\tlet clamp = (_isString(value) && (value.substr(0, 6) === \"clamp(\" || value.indexOf(\"max\") > -1));\n\t\tself[\"_\" + type + \"Clamp\"] = clamp;\n\t\treturn clamp ? value.substr(6, value.length - 7) : value;\n\t},\n\t_keepClamp = (value, clamp) => clamp && (!_isString(value) || value.substr(0, 6) !== \"clamp(\") ? \"clamp(\" + value + \")\" : value,\n\t_rafBugFix = () => _enabled && requestAnimationFrame(_rafBugFix), // in some browsers (like Firefox), screen repaints weren't consistent unless we had SOMETHING queued up in requestAnimationFrame()! So this just creates a super simple loop to keep it alive and smooth out repaints.\n\t_pointerDownHandler = () => _pointerIsDown = 1,\n\t_pointerUpHandler = () => _pointerIsDown = 0,\n\t_passThrough = v => v,\n\t_round = value => Math.round(value * 100000) / 100000 || 0,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap),\n\t_isViewport = e => !!~_root.indexOf(e),\n\t_getViewportDimension = dimensionProperty => (dimensionProperty === \"Height\" ? _100vh : _win[\"inner\" + dimensionProperty]) || _docEl[\"client\" + dimensionProperty] || _body[\"client\" + dimensionProperty],\n\t_getBoundsFunc = element => _getProxyProp(element, \"getBoundingClientRect\") || (_isViewport(element) ? () => {_winOffsets.width = _win.innerWidth; _winOffsets.height = _100vh; return _winOffsets;} : () => _getBounds(element)),\n\t_getSizeFunc = (scroller, isViewport, {d, d2, a}) => (a = _getProxyProp(scroller, \"getBoundingClientRect\")) ? () => a()[d] : () => (isViewport ? _getViewportDimension(d2) : scroller[\"client\" + d2]) || 0,\n\t_getOffsetsFunc = (element, isViewport) => !isViewport || ~_proxies.indexOf(element) ? _getBoundsFunc(element) : () => _winOffsets,\n\t_maxScroll = (element, {s, d2, d, a}) => Math.max(0, (s = \"scroll\" + d2) && (a = _getProxyProp(element, s)) ? a() - _getBoundsFunc(element)()[d] : _isViewport(element) ? (_docEl[s] || _body[s]) - _getViewportDimension(d2) : element[s] - element[\"offset\" + d2]),\n\t_iterateAutoRefresh = (func, events) => {\n\t\tfor (let i = 0; i < _autoRefresh.length; i += 3) {\n\t\t\t(!events || ~events.indexOf(_autoRefresh[i+1])) && func(_autoRefresh[i], _autoRefresh[i+1], _autoRefresh[i+2]);\n\t\t}\n\t},\n\t_isString = value => typeof(value) === \"string\",\n\t_isFunction = value => typeof(value) === \"function\",\n\t_isNumber = value => typeof(value) === \"number\",\n\t_isObject = value => typeof(value) === \"object\",\n\t_endAnimation = (animation, reversed, pause) => animation && animation.progress(reversed ? 0 : 1) && pause && animation.pause(),\n\t_callback = (self, func) => {\n\t\tif (self.enabled) {\n\t\t\tlet result = self._ctx ? self._ctx.add(() => func(self)) : func(self);\n\t\t\tresult && result.totalTime && (self.callbackAnimation = result);\n\t\t}\n\t},\n\t_abs = Math.abs,\n\t_left = \"left\",\n\t_top = \"top\",\n\t_right = \"right\",\n\t_bottom = \"bottom\",\n\t_width = \"width\",\n\t_height = \"height\",\n\t_Right = \"Right\",\n\t_Left = \"Left\",\n\t_Top = \"Top\",\n\t_Bottom = \"Bottom\",\n\t_padding = \"padding\",\n\t_margin = \"margin\",\n\t_Width = \"Width\",\n\t_Height = \"Height\",\n\t_px = \"px\",\n\t_getComputedStyle = element => _win.getComputedStyle(element),\n\t_makePositionable = element => { // if the element already has position: absolute or fixed, leave that, otherwise make it position: relative\n\t\tlet position = _getComputedStyle(element).position;\n\t\telement.style.position = (position === \"absolute\" || position === \"fixed\") ? position : \"relative\";\n\t},\n\t_setDefaults = (obj, defaults) => {\n\t\tfor (let p in defaults) {\n\t\t\t(p in obj) || (obj[p] = defaults[p]);\n\t\t}\n\t\treturn obj;\n\t},\n\t_getBounds = (element, withoutTransforms) => {\n\t\tlet tween = withoutTransforms && _getComputedStyle(element)[_transformProp] !== \"matrix(1, 0, 0, 1, 0, 0)\" && gsap.to(element, {x: 0, y: 0, xPercent: 0, yPercent: 0, rotation: 0, rotationX: 0, rotationY: 0, scale: 1, skewX: 0, skewY: 0}).progress(1),\n\t\t\tbounds = element.getBoundingClientRect();\n\t\ttween && tween.progress(0).kill();\n\t\treturn bounds;\n\t},\n\t_getSize = (element, {d2}) => element[\"offset\" + d2] || element[\"client\" + d2] || 0,\n\t_getLabelRatioArray = timeline => {\n\t\tlet a = [],\n\t\t\tlabels = timeline.labels,\n\t\t\tduration = timeline.duration(),\n\t\t\tp;\n\t\tfor (p in labels) {\n\t\t\ta.push(labels[p] / duration);\n\t\t}\n\t\treturn a;\n\t},\n\t_getClosestLabel = animation => value => gsap.utils.snap(_getLabelRatioArray(animation), value),\n\t_snapDirectional = snapIncrementOrArray => {\n\t\tlet snap = gsap.utils.snap(snapIncrementOrArray),\n\t\t\ta = Array.isArray(snapIncrementOrArray) && snapIncrementOrArray.slice(0).sort((a, b) => a - b);\n\t\treturn a ? (value, direction, threshold= 1e-3) => {\n\t\t\tlet i;\n\t\t\tif (!direction) {\n\t\t\t\treturn snap(value);\n\t\t\t}\n\t\t\tif (direction > 0) {\n\t\t\t\tvalue -= threshold; // to avoid rounding errors. If we're too strict, it might snap forward, then immediately again, and again.\n\t\t\t\tfor (i = 0; i < a.length; i++) {\n\t\t\t\t\tif (a[i] >= value) {\n\t\t\t\t\t\treturn a[i];\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\treturn a[i-1];\n\t\t\t} else {\n\t\t\t\ti = a.length;\n\t\t\t\tvalue += threshold;\n\t\t\t\twhile (i--) {\n\t\t\t\t\tif (a[i] <= value) {\n\t\t\t\t\t\treturn a[i];\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn a[0];\n\t\t} : (value, direction, threshold= 1e-3) => {\n\t\t\tlet snapped = snap(value);\n\t\t\treturn !direction || Math.abs(snapped - value) < threshold || ((snapped - value < 0) === direction < 0) ? snapped : snap(direction < 0 ? value - snapIncrementOrArray : value + snapIncrementOrArray);\n\t\t};\n\t},\n\t_getLabelAtDirection = timeline => (value, st) => _snapDirectional(_getLabelRatioArray(timeline))(value, st.direction),\n\t_multiListener = (func, element, types, callback) => types.split(\",\").forEach(type => func(element, type, callback)),\n\t_addListener = (element, type, func, nonPassive, capture) => element.addEventListener(type, func, {passive: !nonPassive, capture: !!capture}),\n\t_removeListener = (element, type, func, capture) => element.removeEventListener(type, func, !!capture),\n\t_wheelListener = (func, el, scrollFunc) => {\n\t\tscrollFunc = scrollFunc && scrollFunc.wheelHandler\n\t\tif (scrollFunc) {\n\t\t\tfunc(el, \"wheel\", scrollFunc);\n\t\t\tfunc(el, \"touchmove\", scrollFunc);\n\t\t}\n\t},\n\t_markerDefaults = {startColor: \"green\", endColor: \"red\", indent: 0, fontSize: \"16px\", fontWeight:\"normal\"},\n\t_defaults = {toggleActions: \"play\", anticipatePin: 0},\n\t_keywords = {top: 0, left: 0, center: 0.5, bottom: 1, right: 1},\n\t_offsetToPx = (value, size) => {\n\t\tif (_isString(value)) {\n\t\t\tlet eqIndex = value.indexOf(\"=\"),\n\t\t\t\trelative = ~eqIndex ? +(value.charAt(eqIndex-1) + 1) * parseFloat(value.substr(eqIndex + 1)) : 0;\n\t\t\tif (~eqIndex) {\n\t\t\t\t(value.indexOf(\"%\") > eqIndex) && (relative *= size / 100);\n\t\t\t\tvalue = value.substr(0, eqIndex-1);\n\t\t\t}\n\t\t\tvalue = relative + ((value in _keywords) ? _keywords[value] * size : ~value.indexOf(\"%\") ? parseFloat(value) * size / 100 : parseFloat(value) || 0);\n\t\t}\n\t\treturn value;\n\t},\n\t_createMarker = (type, name, container, direction, {startColor, endColor, fontSize, indent, fontWeight}, offset, matchWidthEl, containerAnimation) => {\n\t\tlet e = _doc.createElement(\"div\"),\n\t\t\tuseFixedPosition = _isViewport(container) || _getProxyProp(container, \"pinType\") === \"fixed\",\n\t\t\tisScroller = type.indexOf(\"scroller\") !== -1,\n\t\t\tparent = useFixedPosition ? _body : container,\n\t\t\tisStart = type.indexOf(\"start\") !== -1,\n\t\t\tcolor = isStart ? startColor : endColor,\n\t\t\tcss = \"border-color:\" + color + \";font-size:\" + fontSize + \";color:\" + color + \";font-weight:\" + fontWeight + \";pointer-events:none;white-space:nowrap;font-family:sans-serif,Arial;z-index:1000;padding:4px 8px;border-width:0;border-style:solid;\";\n\t\tcss += \"position:\" + ((isScroller || containerAnimation) && useFixedPosition ? \"fixed;\" : \"absolute;\");\n\t\t(isScroller || containerAnimation || !useFixedPosition) && (css += (direction === _vertical ? _right : _bottom) + \":\" + (offset + parseFloat(indent)) + \"px;\");\n\t\tmatchWidthEl && (css += \"box-sizing:border-box;text-align:left;width:\" + matchWidthEl.offsetWidth + \"px;\");\n\t\te._isStart = isStart;\n\t\te.setAttribute(\"class\", \"gsap-marker-\" + type + (name ? \" marker-\" + name : \"\"));\n\t\te.style.cssText = css;\n\t\te.innerText = name || name === 0 ? type + \"-\" + name : type;\n\t\tparent.children[0] ? parent.insertBefore(e, parent.children[0]) : parent.appendChild(e);\n\t\te._offset = e[\"offset\" + direction.op.d2];\n\t\t_positionMarker(e, 0, direction, isStart);\n\t\treturn e;\n\t},\n\t_positionMarker = (marker, start, direction, flipped) => {\n\t\tlet vars = {display: \"block\"},\n\t\t\tside = direction[flipped ? \"os2\" : \"p2\"],\n\t\t\toppositeSide = direction[flipped ? \"p2\" : \"os2\"];\n\t\tmarker._isFlipped = flipped;\n\t\tvars[direction.a + \"Percent\"] = flipped ? -100 : 0;\n\t\tvars[direction.a] = flipped ? \"1px\" : 0;\n\t\tvars[\"border\" + side + _Width] = 1;\n\t\tvars[\"border\" + oppositeSide + _Width] = 0;\n\t\tvars[direction.p] = start + \"px\";\n\t\tgsap.set(marker, vars);\n\t},\n\t_triggers = [],\n\t_ids = {},\n\t_rafID,\n\t_sync = () => _getTime() - _lastScrollTime > 34 && (_rafID || (_rafID = requestAnimationFrame(_updateAll))),\n\t_onScroll = () => { // previously, we tried to optimize performance by batching/deferring to the next requestAnimationFrame(), but discovered that Safari has a few bugs that make this unworkable (especially on iOS). See https://codepen.io/GreenSock/pen/16c435b12ef09c38125204818e7b45fc?editors=0010 and https://codepen.io/GreenSock/pen/JjOxYpQ/3dd65ccec5a60f1d862c355d84d14562?editors=0010 and https://codepen.io/GreenSock/pen/ExbrPNa/087cef197dc35445a0951e8935c41503?editors=0010\n\t\tif (!_normalizer || !_normalizer.isPressed || _normalizer.startX > _body.clientWidth) { // if the user is dragging the scrollbar, allow it.\n\t\t\t_scrollers.cache++;\n\t\t\tif (_normalizer) {\n\t\t\t\t_rafID || (_rafID = requestAnimationFrame(_updateAll));\n\t\t\t} else {\n\t\t\t\t_updateAll(); // Safari in particular (on desktop) NEEDS the immediate update rather than waiting for a requestAnimationFrame() whereas iOS seems to benefit from waiting for the requestAnimationFrame() tick, at least when normalizing. See https://codepen.io/GreenSock/pen/qBYozqO?editors=0110\n\t\t\t}\n\t\t\t_lastScrollTime || _dispatch(\"scrollStart\");\n\t\t\t_lastScrollTime = _getTime();\n\t\t}\n\t},\n\t_setBaseDimensions = () => {\n\t\t_baseScreenWidth = _win.innerWidth;\n\t\t_baseScreenHeight = _win.innerHeight;\n\t},\n\t_onResize = (force) => {\n\t\t_scrollers.cache++;\n\t\t(force === true || (!_refreshing && !_ignoreResize && !_doc.fullscreenElement && !_doc.webkitFullscreenElement && (!_ignoreMobileResize || _baseScreenWidth !== _win.innerWidth || Math.abs(_win.innerHeight - _baseScreenHeight) > _win.innerHeight * 0.25))) && _resizeDelay.restart(true);\n\t}, // ignore resizes triggered by refresh()\n\t_listeners = {},\n\t_emptyArray = [],\n\t_softRefresh = () => _removeListener(ScrollTrigger, \"scrollEnd\", _softRefresh) || _refreshAll(true),\n\t_dispatch = type => (_listeners[type] && _listeners[type].map(f => f())) || _emptyArray,\n\t_savedStyles = [], // when ScrollTrigger.saveStyles() is called, the inline styles are recorded in this Array in a sequential format like [element, cssText, gsCache, media]. This keeps it very memory-efficient and fast to iterate through.\n\t_revertRecorded = media => {\n\t\tfor (let i = 0; i < _savedStyles.length; i+=5) {\n\t\t\tif (!media || _savedStyles[i+4] && _savedStyles[i+4].query === media) {\n\t\t\t\t_savedStyles[i].style.cssText = _savedStyles[i+1];\n\t\t\t\t_savedStyles[i].getBBox && _savedStyles[i].setAttribute(\"transform\", _savedStyles[i+2] || \"\");\n\t\t\t\t_savedStyles[i+3].uncache = 1;\n\t\t\t}\n\t\t}\n\t},\n\t_revertAll = (kill, media) => {\n\t\tlet trigger;\n\t\tfor (_i = 0; _i < _triggers.length; _i++) {\n\t\t\ttrigger = _triggers[_i];\n\t\t\tif (trigger && (!media || trigger._ctx === media)) {\n\t\t\t\tif (kill) {\n\t\t\t\t\ttrigger.kill(1);\n\t\t\t\t} else {\n\t\t\t\t\ttrigger.revert(true, true);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\t_isReverted = true;\n\t\tmedia && _revertRecorded(media);\n\t\tmedia || _dispatch(\"revert\");\n\t},\n\t_clearScrollMemory = (scrollRestoration, force) => { // zero-out all the recorded scroll positions. Don't use _triggers because if, for example, .matchMedia() is used to create some ScrollTriggers and then the user resizes and it removes ALL ScrollTriggers, and then go back to a size where there are ScrollTriggers, it would have kept the position(s) saved from the initial state.\n\t\t_scrollers.cache++;\n\t\t(force || !_refreshingAll) && _scrollers.forEach(obj => _isFunction(obj) && obj.cacheID++ && (obj.rec = 0));\n\t\t_isString(scrollRestoration) && (_win.history.scrollRestoration = _scrollRestoration = scrollRestoration);\n\t},\n\t_refreshingAll,\n\t_refreshID = 0,\n\t_queueRefreshID,\n\t_queueRefreshAll = () => { // we don't want to call _refreshAll() every time we create a new ScrollTrigger (for performance reasons) - it's better to batch them. Some frameworks dynamically load content and we can't rely on the window's \"load\" or \"DOMContentLoaded\" events to trigger it.\n\t\tif (_queueRefreshID !== _refreshID) {\n\t\t\tlet id = _queueRefreshID = _refreshID;\n\t\t\trequestAnimationFrame(() => id === _refreshID && _refreshAll(true));\n\t\t}\n\t},\n\t_refresh100vh = () => {\n\t\t_body.appendChild(_div100vh);\n\t\t_100vh = (!_normalizer && _div100vh.offsetHeight) || _win.innerHeight;\n\t\t_body.removeChild(_div100vh);\n\t},\n\t_hideAllMarkers = hide => _toArray(\".gsap-marker-start, .gsap-marker-end, .gsap-marker-scroller-start, .gsap-marker-scroller-end\").forEach(el => el.style.display = hide ? \"none\" : \"block\"),\n\t_refreshAll = (force, skipRevert) => {\n\t\t_docEl = _doc.documentElement; // some frameworks like Astro may cache the <body> and replace it during routing, so we'll just re-record the _docEl and _body for safety (otherwise, the markers may not get added properly).\n\t\t_body = _doc.body;\n\t\t_root = [_win, _doc, _docEl, _body];\n\t\tif (_lastScrollTime && !force && !_isReverted) {\n\t\t\t_addListener(ScrollTrigger, \"scrollEnd\", _softRefresh);\n\t\t\treturn;\n\t\t}\n\t\t_refresh100vh();\n\t\t_refreshingAll = ScrollTrigger.isRefreshing = true;\n\t\t_scrollers.forEach(obj => _isFunction(obj) && ++obj.cacheID && (obj.rec = obj())); // force the clearing of the cache because some browsers take a little while to dispatch the \"scroll\" event and the user may have changed the scroll position and then called ScrollTrigger.refresh() right away\n\t\tlet refreshInits = _dispatch(\"refreshInit\");\n\t\t_sort && ScrollTrigger.sort();\n\t\tskipRevert || _revertAll();\n\t\t_scrollers.forEach(obj => {\n\t\t\tif (_isFunction(obj)) {\n\t\t\t\tobj.smooth && (obj.target.style.scrollBehavior = \"auto\"); // smooth scrolling interferes\n\t\t\t\tobj(0);\n\t\t\t}\n\t\t});\n\t\t_triggers.slice(0).forEach(t => t.refresh()) // don't loop with _i because during a refresh() someone could call ScrollTrigger.update() which would iterate through _i resulting in a skip.\n\t\t_isReverted = false;\n\t\t_triggers.forEach((t) => { // nested pins (pinnedContainer) with pinSpacing may expand the container, so we must accommodate that here.\n\t\t\tif (t._subPinOffset && t.pin) {\n\t\t\t\tlet prop = t.vars.horizontal ? \"offsetWidth\" : \"offsetHeight\",\n\t\t\t\t\toriginal = t.pin[prop];\n\t\t\t\tt.revert(true, 1);\n\t\t\t\tt.adjustPinSpacing(t.pin[prop] - original);\n\t\t\t\tt.refresh();\n\t\t\t}\n\t\t});\n\t\t_clampingMax = 1; // pinSpacing might be propping a page open, thus when we .setPositions() to clamp a ScrollTrigger's end we should leave the pinSpacing alone. That's what this flag is for.\n\t\t_hideAllMarkers(true);\n\t\t_triggers.forEach(t => { // the scroller's max scroll position may change after all the ScrollTriggers refreshed (like pinning could push it down), so we need to loop back and correct any with end: \"max\". Same for anything with a clamped end\n\t\t\tlet max = _maxScroll(t.scroller, t._dir),\n\t\t\t\tendClamp = t.vars.end === \"max\" || (t._endClamp && t.end > max),\n\t\t\t\tstartClamp = t._startClamp && t.start >= max;\n\t\t\t(endClamp || startClamp) && t.setPositions(startClamp ? max - 1 : t.start, endClamp ? Math.max(startClamp ? max : t.start + 1, max) : t.end, true);\n\t\t});\n\t\t_hideAllMarkers(false);\n\t\t_clampingMax = 0;\n\t\trefreshInits.forEach(result => result && result.render && result.render(-1)); // if the onRefreshInit() returns an animation (typically a gsap.set()), revert it. This makes it easy to put things in a certain spot before refreshing for measurement purposes, and then put things back.\n\t\t_scrollers.forEach(obj => {\n\t\t\tif (_isFunction(obj)) {\n\t\t\t\tobj.smooth && requestAnimationFrame(() => obj.target.style.scrollBehavior = \"smooth\");\n\t\t\t\tobj.rec && obj(obj.rec);\n\t\t\t}\n\t\t});\n\t\t_clearScrollMemory(_scrollRestoration, 1);\n\t\t_resizeDelay.pause();\n\t\t_refreshID++;\n\t\t_refreshingAll = 2;\n\t\t_updateAll(2);\n\t\t_triggers.forEach(t => _isFunction(t.vars.onRefresh) && t.vars.onRefresh(t));\n\t\t_refreshingAll = ScrollTrigger.isRefreshing = false;\n\t\t_dispatch(\"refresh\");\n\t},\n\t_lastScroll = 0,\n\t_direction = 1,\n\t_primary,\n\t_updateAll = (force) => {\n\t\tif (force === 2 || (!_refreshingAll && !_isReverted)) { // _isReverted could be true if, for example, a matchMedia() is in the process of executing. We don't want to update during the time everything is reverted.\n\t\t\tScrollTrigger.isUpdating = true;\n\t\t\t_primary && _primary.update(0); // ScrollSmoother uses refreshPriority -9999 to become the primary that gets updated before all others because it affects the scroll position.\n\t\t\tlet l = _triggers.length,\n\t\t\t\ttime = _getTime(),\n\t\t\t\trecordVelocity = time - _time1 >= 50,\n\t\t\t\tscroll = l && _triggers[0].scroll();\n\t\t\t_direction = _lastScroll > scroll ? -1 : 1;\n\t\t\t_refreshingAll || (_lastScroll = scroll);\n\t\t\tif (recordVelocity) {\n\t\t\t\tif (_lastScrollTime && !_pointerIsDown && time - _lastScrollTime > 200) {\n\t\t\t\t\t_lastScrollTime = 0;\n\t\t\t\t\t_dispatch(\"scrollEnd\");\n\t\t\t\t}\n\t\t\t\t_time2 = _time1;\n\t\t\t\t_time1 = time;\n\t\t\t}\n\t\t\tif (_direction < 0) {\n\t\t\t\t_i = l;\n\t\t\t\twhile (_i-- > 0) {\n\t\t\t\t\t_triggers[_i] && _triggers[_i].update(0, recordVelocity);\n\t\t\t\t}\n\t\t\t\t_direction = 1;\n\t\t\t} else {\n\t\t\t\tfor (_i = 0; _i < l; _i++) {\n\t\t\t\t\t_triggers[_i] && _triggers[_i].update(0, recordVelocity);\n\t\t\t\t}\n\t\t\t}\n\t\t\tScrollTrigger.isUpdating = false;\n\t\t}\n\t\t_rafID = 0;\n\t},\n\t_propNamesToCopy = [_left, _top, _bottom, _right, _margin + _Bottom, _margin + _Right, _margin + _Top, _margin + _Left, \"display\", \"flexShrink\", \"float\", \"zIndex\", \"gridColumnStart\", \"gridColumnEnd\", \"gridRowStart\", \"gridRowEnd\", \"gridArea\", \"justifySelf\", \"alignSelf\", \"placeSelf\", \"order\"],\n\t_stateProps = _propNamesToCopy.concat([_width, _height, \"boxSizing\", \"max\" + _Width, \"max\" + _Height, \"position\", _margin, _padding, _padding + _Top, _padding + _Right, _padding + _Bottom, _padding + _Left]),\n\t_swapPinOut = (pin, spacer, state) => {\n\t\t_setState(state);\n\t\tlet cache = pin._gsap;\n\t\tif (cache.spacerIsNative) {\n\t\t\t_setState(cache.spacerState);\n\t\t} else if (pin._gsap.swappedIn) {\n\t\t\tlet parent = spacer.parentNode;\n\t\t\tif (parent) {\n\t\t\t\tparent.insertBefore(pin, spacer);\n\t\t\t\tparent.removeChild(spacer);\n\t\t\t}\n\t\t}\n\t\tpin._gsap.swappedIn = false;\n\t},\n\t_swapPinIn = (pin, spacer, cs, spacerState) => {\n\t\tif (!pin._gsap.swappedIn) {\n\t\t\tlet i = _propNamesToCopy.length,\n\t\t\t\tspacerStyle = spacer.style,\n\t\t\t\tpinStyle = pin.style,\n\t\t\t\tp;\n\t\t\twhile (i--) {\n\t\t\t\tp = _propNamesToCopy[i];\n\t\t\t\tspacerStyle[p] = cs[p];\n\t\t\t}\n\t\t\tspacerStyle.position = cs.position === \"absolute\" ? \"absolute\" : \"relative\";\n\t\t\t(cs.display === \"inline\") && (spacerStyle.display = \"inline-block\");\n\t\t\tpinStyle[_bottom] = pinStyle[_right] = \"auto\";\n\t\t\tspacerStyle.flexBasis = cs.flexBasis || \"auto\";\n\t\t\tspacerStyle.overflow = \"visible\";\n\t\t\tspacerStyle.boxSizing = \"border-box\";\n\t\t\tspacerStyle[_width] = _getSize(pin, _horizontal) + _px;\n\t\t\tspacerStyle[_height] = _getSize(pin, _vertical) + _px;\n\t\t\tspacerStyle[_padding] = pinStyle[_margin] = pinStyle[_top] = pinStyle[_left] = \"0\";\n\t\t\t_setState(spacerState);\n\t\t\tpinStyle[_width] = pinStyle[\"max\" + _Width] = cs[_width];\n\t\t\tpinStyle[_height] = pinStyle[\"max\" + _Height] = cs[_height];\n\t\t\tpinStyle[_padding] = cs[_padding];\n\t\t\tif (pin.parentNode !== spacer) {\n\t\t\t\tpin.parentNode.insertBefore(spacer, pin);\n\t\t\t\tspacer.appendChild(pin);\n\t\t\t}\n\t\t\tpin._gsap.swappedIn = true;\n\t\t}\n\t},\n\t_capsExp = /([A-Z])/g,\n\t_setState = state => {\n\t\tif (state) {\n\t\t\tlet style = state.t.style,\n\t\t\t\tl = state.length,\n\t\t\t\ti = 0,\n\t\t\t\tp, value;\n\t\t\t(state.t._gsap || gsap.core.getCache(state.t)).uncache = 1; // otherwise transforms may be off\n\t\t\tfor (; i < l; i +=2) {\n\t\t\t\tvalue = state[i+1];\n\t\t\t\tp = state[i];\n\t\t\t\tif (value) {\n\t\t\t\t\tstyle[p] = value;\n\t\t\t\t} else if (style[p]) {\n\t\t\t\t\tstyle.removeProperty(p.replace(_capsExp, \"-$1\").toLowerCase());\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t},\n\t_getState = element => { // returns an Array with alternating values like [property, value, property, value] and a \"t\" property pointing to the target (element). Makes it fast and cheap.\n\t\tlet l = _stateProps.length,\n\t\t\tstyle = element.style,\n\t\t\tstate = [],\n\t\t\ti = 0;\n\t\tfor (; i < l; i++) {\n\t\t\tstate.push(_stateProps[i], style[_stateProps[i]]);\n\t\t}\n\t\tstate.t = element;\n\t\treturn state;\n\t},\n\t_copyState = (state, override, omitOffsets) => {\n\t\tlet result = [],\n\t\t\tl = state.length,\n\t\t\ti = omitOffsets ? 8 : 0, // skip top, left, right, bottom if omitOffsets is true\n\t\t\tp;\n\t\tfor (; i < l; i += 2) {\n\t\t\tp = state[i];\n\t\t\tresult.push(p, (p in override) ? override[p] : state[i+1]);\n\t\t}\n\t\tresult.t = state.t;\n\t\treturn result;\n\t},\n\t_winOffsets = {left:0, top:0},\n\t// // potential future feature (?) Allow users to calculate where a trigger hits (scroll position) like getScrollPosition(\"#id\", \"top bottom\")\n\t// _getScrollPosition = (trigger, position, {scroller, containerAnimation, horizontal}) => {\n\t// \tscroller = _getTarget(scroller || _win);\n\t// \tlet direction = horizontal ? _horizontal : _vertical,\n\t// \t\tisViewport = _isViewport(scroller);\n\t// \t_getSizeFunc(scroller, isViewport, direction);\n\t// \treturn _parsePosition(position, _getTarget(trigger), _getSizeFunc(scroller, isViewport, direction)(), direction, _getScrollFunc(scroller, direction)(), 0, 0, 0, _getOffsetsFunc(scroller, isViewport)(), isViewport ? 0 : parseFloat(_getComputedStyle(scroller)[\"border\" + direction.p2 + _Width]) || 0, 0, containerAnimation ? containerAnimation.duration() : _maxScroll(scroller), containerAnimation);\n\t// },\n\t_parsePosition = (value, trigger, scrollerSize, direction, scroll, marker, markerScroller, self, scrollerBounds, borderWidth, useFixedPosition, scrollerMax, containerAnimation, clampZeroProp) => {\n\t\t_isFunction(value) && (value = value(self));\n\t\tif (_isString(value) && value.substr(0,3) === \"max\") {\n\t\t\tvalue = scrollerMax + (value.charAt(4) === \"=\" ? _offsetToPx(\"0\" + value.substr(3), scrollerSize) : 0);\n\t\t}\n\t\tlet time = containerAnimation ? containerAnimation.time() : 0,\n\t\t\tp1, p2, element;\n\t\tcontainerAnimation && containerAnimation.seek(0);\n\t\tisNaN(value) || (value = +value); // convert a string number like \"45\" to an actual number\n\t\tif (!_isNumber(value)) {\n\t\t\t_isFunction(trigger) && (trigger = trigger(self));\n\t\t\tlet offsets = (value || \"0\").split(\" \"),\n\t\t\t\tbounds, localOffset, globalOffset, display;\n\t\t\telement = _getTarget(trigger, self) || _body;\n\t\t\tbounds = _getBounds(element) || {};\n\t\t\tif ((!bounds || (!bounds.left && !bounds.top)) && _getComputedStyle(element).display === \"none\") { // if display is \"none\", it won't report getBoundingClientRect() properly\n\t\t\t\tdisplay = element.style.display;\n\t\t\t\telement.style.display = \"block\";\n\t\t\t\tbounds = _getBounds(element);\n\t\t\t\tdisplay ? (element.style.display = display) : element.style.removeProperty(\"display\");\n\t\t\t}\n\t\t\tlocalOffset = _offsetToPx(offsets[0], bounds[direction.d]);\n\t\t\tglobalOffset = _offsetToPx(offsets[1] || \"0\", scrollerSize);\n\t\t\tvalue = bounds[direction.p] - scrollerBounds[direction.p] - borderWidth + localOffset + scroll - globalOffset;\n\t\t\tmarkerScroller && _positionMarker(markerScroller, globalOffset, direction, (scrollerSize - globalOffset < 20 || (markerScroller._isStart && globalOffset > 20)));\n\t\t\tscrollerSize -= scrollerSize - globalOffset; // adjust for the marker\n\t\t} else {\n\t\t\tcontainerAnimation && (value = gsap.utils.mapRange(containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, 0, scrollerMax, value));\n\t\t\tmarkerScroller && _positionMarker(markerScroller, scrollerSize, direction, true);\n\t\t}\n\t\tif (clampZeroProp) {\n\t\t\tself[clampZeroProp] = value || -0.001;\n\t\t\tvalue < 0 && (value = 0);\n\t\t}\n\t\tif (marker) {\n\t\t\tlet position = value + scrollerSize,\n\t\t\t\tisStart = marker._isStart;\n\t\t\tp1 = \"scroll\" + direction.d2;\n\t\t\t_positionMarker(marker, position, direction, (isStart && position > 20) || (!isStart && (useFixedPosition ? Math.max(_body[p1], _docEl[p1]) : marker.parentNode[p1]) <= position + 1));\n\t\t\tif (useFixedPosition) {\n\t\t\t\tscrollerBounds = _getBounds(markerScroller);\n\t\t\t\tuseFixedPosition && (marker.style[direction.op.p] = (scrollerBounds[direction.op.p] - direction.op.m - marker._offset) + _px);\n\t\t\t}\n\t\t}\n\t\tif (containerAnimation && element) {\n\t\t\tp1 = _getBounds(element);\n\t\t\tcontainerAnimation.seek(scrollerMax);\n\t\t\tp2 = _getBounds(element);\n\t\t\tcontainerAnimation._caScrollDist = p1[direction.p] - p2[direction.p];\n\t\t\tvalue = value / (containerAnimation._caScrollDist) * scrollerMax;\n\t\t}\n\t\tcontainerAnimation && containerAnimation.seek(time);\n\t\treturn containerAnimation ? value : Math.round(value);\n\t},\n\t_prefixExp = /(webkit|moz|length|cssText|inset)/i,\n\t_reparent = (element, parent, top, left) => {\n\t\tif (element.parentNode !== parent) {\n\t\t\tlet style = element.style,\n\t\t\t\tp, cs;\n\t\t\tif (parent === _body) {\n\t\t\t\telement._stOrig = style.cssText; // record original inline styles so we can revert them later\n\t\t\t\tcs = _getComputedStyle(element);\n\t\t\t\tfor (p in cs) { // must copy all relevant styles to ensure that nothing changes visually when we reparent to the <body>. Skip the vendor prefixed ones.\n\t\t\t\t\tif (!+p && !_prefixExp.test(p) && cs[p] && typeof style[p] === \"string\" && p !== \"0\") {\n\t\t\t\t\t\tstyle[p] = cs[p];\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tstyle.top = top;\n\t\t\t\tstyle.left = left;\n\t\t\t} else {\n\t\t\t\tstyle.cssText = element._stOrig;\n\t\t\t}\n\t\t\tgsap.core.getCache(element).uncache = 1;\n\t\t\tparent.appendChild(element);\n\t\t}\n\t},\n\t_interruptionTracker = (getValueFunc, initialValue, onInterrupt) => {\n\t\tlet last1 = initialValue,\n\t\t\tlast2 = last1;\n\t\treturn value => {\n\t\t\tlet current = Math.round(getValueFunc()); // round because in some [very uncommon] Windows environments, scroll can get reported with decimals even though it was set without.\n\t\t\tif (current !== last1 && current !== last2 && Math.abs(current - last1) > 3 && Math.abs(current - last2) > 3) { // if the user scrolls, kill the tween. iOS Safari intermittently misreports the scroll position, it may be the most recently-set one or the one before that! When Safari is zoomed (CMD-+), it often misreports as 1 pixel off too! So if we set the scroll position to 125, for example, it'll actually report it as 124.\n\t\t\t\tvalue = current;\n\t\t\t\tonInterrupt && onInterrupt();\n\t\t\t}\n\t\t\tlast2 = last1;\n\t\t\tlast1 = Math.round(value);\n\t\t\treturn last1;\n\t\t};\n\t},\n\t_shiftMarker = (marker, direction, value) => {\n\t\tlet vars = {};\n\t\tvars[direction.p] = \"+=\" + value;\n\t\tgsap.set(marker, vars);\n\t},\n\t// _mergeAnimations = animations => {\n\t// \tlet tl = gsap.timeline({smoothChildTiming: true}).startTime(Math.min(...animations.map(a => a.globalTime(0))));\n\t// \tanimations.forEach(a => {let time = a.totalTime(); tl.add(a); a.totalTime(time); });\n\t// \ttl.smoothChildTiming = false;\n\t// \treturn tl;\n\t// },\n\n\t// returns a function that can be used to tween the scroll position in the direction provided, and when doing so it'll add a .tween property to the FUNCTION itself, and remove it when the tween completes or gets killed. This gives us a way to have multiple ScrollTriggers use a central function for any given scroller and see if there's a scroll tween running (which would affect if/how things get updated)\n\t_getTweenCreator = (scroller, direction) => {\n\t\tlet getScroll = _getScrollFunc(scroller, direction),\n\t\t\tprop = \"_scroll\" + direction.p2, // add a tweenable property to the scroller that's a getter/setter function, like _scrollTop or _scrollLeft. This way, if someone does gsap.killTweensOf(scroller) it'll kill the scroll tween.\n\t\t\tgetTween = (scrollTo, vars, initialValue, change1, change2) => {\n\t\t\t\tlet tween = getTween.tween,\n\t\t\t\t\tonComplete = vars.onComplete,\n\t\t\t\t\tmodifiers = {};\n\t\t\t\tinitialValue = initialValue || getScroll();\n\t\t\t\tlet checkForInterruption = _interruptionTracker(getScroll, initialValue, () => {\n\t\t\t\t\ttween.kill();\n\t\t\t\t\tgetTween.tween = 0;\n\t\t\t\t});\n\t\t\t\tchange2 = (change1 && change2) || 0; // if change1 is 0, we set that to the difference and ignore change2. Otherwise, there would be a compound effect.\n\t\t\t\tchange1 = change1 || (scrollTo - initialValue);\n\t\t\t\ttween && tween.kill();\n\t\t\t\tvars[prop] = scrollTo;\n\t\t\t\tvars.inherit = false;\n\t\t\t\tvars.modifiers = modifiers;\n\t\t\t\tmodifiers[prop] = () => checkForInterruption(initialValue + change1 * tween.ratio + change2 * tween.ratio * tween.ratio);\n\t\t\t\tvars.onUpdate = () => {\n\t\t\t\t\t_scrollers.cache++;\n\t\t\t\t\tgetTween.tween && _updateAll(); // if it was interrupted/killed, like in a context.revert(), don't force an updateAll()\n\t\t\t\t};\n\t\t\t\tvars.onComplete = () => {\n\t\t\t\t\tgetTween.tween = 0;\n\t\t\t\t\tonComplete && onComplete.call(tween);\n\t\t\t\t};\n\t\t\t\ttween = getTween.tween = gsap.to(scroller, vars);\n\t\t\t\treturn tween;\n\t\t\t};\n\t\tscroller[prop] = getScroll;\n\t\tgetScroll.wheelHandler = () => getTween.tween && getTween.tween.kill() && (getTween.tween = 0);\n\t\t_addListener(scroller, \"wheel\", getScroll.wheelHandler); // Windows machines handle mousewheel scrolling in chunks (like \"3 lines per scroll\") meaning the typical strategy for cancelling the scroll isn't as sensitive. It's much more likely to match one of the previous 2 scroll event positions. So we kill any snapping as soon as there's a wheel event.\n\t\tScrollTrigger.isTouch && _addListener(scroller, \"touchmove\", getScroll.wheelHandler);\n\t\treturn getTween;\n\t};\n\n\n\n\nexport class ScrollTrigger {\n\n\tconstructor(vars, animation) {\n\t\t_coreInitted || ScrollTrigger.register(gsap) || console.warn(\"Please gsap.registerPlugin(ScrollTrigger)\");\n\t\t_context(this);\n\t\tthis.init(vars, animation);\n\t}\n\n\tinit(vars, animation) {\n\t\tthis.progress = this.start = 0;\n\t\tthis.vars && this.kill(true, true); // in case it's being initted again\n\t\tif (!_enabled) {\n\t\t\tthis.update = this.refresh = this.kill = _passThrough;\n\t\t\treturn;\n\t\t}\n\t\tvars = _setDefaults((_isString(vars) || _isNumber(vars) || vars.nodeType) ? {trigger: vars} : vars, _defaults);\n\t\tlet {onUpdate, toggleClass, id, onToggle, onRefresh, scrub, trigger, pin, pinSpacing, invalidateOnRefresh, anticipatePin, onScrubComplete, onSnapComplete, once, snap, pinReparent, pinSpacer, containerAnimation, fastScrollEnd, preventOverlaps} = vars,\n\t\t\tdirection = vars.horizontal || (vars.containerAnimation && vars.horizontal !== false) ? _horizontal : _vertical,\n\t\t\tisToggle = !scrub && scrub !== 0,\n\t\t\tscroller = _getTarget(vars.scroller || _win),\n\t\t\tscrollerCache = gsap.core.getCache(scroller),\n\t\t\tisViewport = _isViewport(scroller),\n\t\t\tuseFixedPosition = (\"pinType\" in vars ? vars.pinType : _getProxyProp(scroller, \"pinType\") || (isViewport && \"fixed\")) === \"fixed\",\n\t\t\tcallbacks = [vars.onEnter, vars.onLeave, vars.onEnterBack, vars.onLeaveBack],\n\t\t\ttoggleActions = isToggle && vars.toggleActions.split(\" \"),\n\t\t\tmarkers = \"markers\" in vars ? vars.markers : _defaults.markers,\n\t\t\tborderWidth = isViewport ? 0 : parseFloat(_getComputedStyle(scroller)[\"border\" + direction.p2 + _Width]) || 0,\n\t\t\tself = this,\n\t\t\tonRefreshInit = vars.onRefreshInit && (() => vars.onRefreshInit(self)),\n\t\t\tgetScrollerSize = _getSizeFunc(scroller, isViewport, direction),\n\t\t\tgetScrollerOffsets = _getOffsetsFunc(scroller, isViewport),\n\t\t\tlastSnap = 0,\n\t\t\tlastRefresh = 0,\n\t\t\tprevProgress = 0,\n\t\t\tscrollFunc = _getScrollFunc(scroller, direction),\n\t\t\ttweenTo, pinCache, snapFunc, scroll1, scroll2, start, end, markerStart, markerEnd, markerStartTrigger, markerEndTrigger, markerVars, executingOnRefresh,\n\t\t\tchange, pinOriginalState, pinActiveState, pinState, spacer, offset, pinGetter, pinSetter, pinStart, pinChange, spacingStart, spacerState, markerStartSetter, pinMoves,\n\t\t\tmarkerEndSetter, cs, snap1, snap2, scrubTween, scrubSmooth, snapDurClamp, snapDelayedCall, prevScroll, prevAnimProgress, caMarkerSetter, customRevertReturn;\n\n\t\t// for the sake of efficiency, _startClamp/_endClamp serve like a truthy value indicating that clamping was enabled on the start/end, and ALSO store the actual pre-clamped numeric value. We tap into that in ScrollSmoother for speed effects. So for example, if start=\"clamp(top bottom)\" results in a start of -100 naturally, it would get clamped to 0 but -100 would be stored in _startClamp.\n\t\tself._startClamp = self._endClamp = false;\n\t\tself._dir = direction;\n\t\tanticipatePin *= 45;\n\t\tself.scroller = scroller;\n\t\tself.scroll = containerAnimation ? containerAnimation.time.bind(containerAnimation) : scrollFunc;\n\t\tscroll1 = scrollFunc();\n\t\tself.vars = vars;\n\t\tanimation = animation || vars.animation;\n\t\tif (\"refreshPriority\" in vars) {\n\t\t\t_sort = 1;\n\t\t\tvars.refreshPriority === -9999 && (_primary = self); // used by ScrollSmoother\n\t\t}\n\t\tscrollerCache.tweenScroll = scrollerCache.tweenScroll || {\n\t\t\ttop: _getTweenCreator(scroller, _vertical),\n\t\t\tleft: _getTweenCreator(scroller, _horizontal)\n\t\t};\n\t\tself.tweenTo = tweenTo = scrollerCache.tweenScroll[direction.p];\n\t\tself.scrubDuration = value => {\n\t\t\tscrubSmooth = _isNumber(value) && value;\n\t\t\tif (!scrubSmooth) {\n\t\t\t\tscrubTween && scrubTween.progress(1).kill();\n\t\t\t\tscrubTween = 0;\n\t\t\t} else {\n\t\t\t\tscrubTween ? scrubTween.duration(value) : (scrubTween = gsap.to(animation, {ease: \"expo\", totalProgress: \"+=0\", inherit: false, duration: scrubSmooth, paused: true, onComplete: () => onScrubComplete && onScrubComplete(self)}));\n\t\t\t}\n\t\t};\n\t\tif (animation) {\n\t\t\tanimation.vars.lazy = false;\n\t\t\t(animation._initted && !self.isReverted) || (animation.vars.immediateRender !== false && vars.immediateRender !== false && animation.duration() && animation.render(0, true, true)); // special case: if this ScrollTrigger gets re-initted, a from() tween with a stagger could get initted initially and then reverted on the re-init which means it'll need to get rendered again here to properly display things. Otherwise, See https://gsap.com/forums/topic/36777-scrollsmoother-splittext-nextjs/ and https://codepen.io/GreenSock/pen/eYPyPpd?editors=0010\n\t\t\tself.animation = animation.pause();\n\t\t\tanimation.scrollTrigger = self;\n\t\t\tself.scrubDuration(scrub);\n\t\t\tsnap1 = 0;\n\t\t\tid || (id = animation.vars.id);\n\t\t}\n\n\t\tif (snap) {\n\t\t\t// TODO: potential idea: use legitimate CSS scroll snapping by pushing invisible elements into the DOM that serve as snap positions, and toggle the document.scrollingElement.style.scrollSnapType onToggle. See https://codepen.io/GreenSock/pen/JjLrgWM for a quick proof of concept.\n\t\t\tif (!_isObject(snap) || snap.push) {\n\t\t\t\tsnap = {snapTo: snap};\n\t\t\t}\n\t\t\t(\"scrollBehavior\" in _body.style) && gsap.set(isViewport ? [_body, _docEl] : scroller, {scrollBehavior: \"auto\"}); // smooth scrolling doesn't work with snap.\n\t\t\t_scrollers.forEach(o => _isFunction(o) && o.target === (isViewport ? _doc.scrollingElement || _docEl : scroller) && (o.smooth = false)); // note: set smooth to false on both the vertical and horizontal scroll getters/setters\n\t\t\tsnapFunc = _isFunction(snap.snapTo) ? snap.snapTo : snap.snapTo === \"labels\" ? _getClosestLabel(animation) : snap.snapTo === \"labelsDirectional\" ? _getLabelAtDirection(animation) : snap.directional !== false ? (value, st) => _snapDirectional(snap.snapTo)(value, _getTime() - lastRefresh < 500 ? 0 : st.direction) : gsap.utils.snap(snap.snapTo);\n\t\t\tsnapDurClamp = snap.duration || {min: 0.1, max: 2};\n\t\t\tsnapDurClamp = _isObject(snapDurClamp) ? _clamp(snapDurClamp.min, snapDurClamp.max) : _clamp(snapDurClamp, snapDurClamp);\n\t\t\tsnapDelayedCall = gsap.delayedCall(snap.delay || (scrubSmooth / 2) || 0.1, () => {\n\t\t\t\tlet scroll = scrollFunc(),\n\t\t\t\t\trefreshedRecently = _getTime() - lastRefresh < 500,\n\t\t\t\t\ttween = tweenTo.tween;\n\t\t\t\tif ((refreshedRecently || Math.abs(self.getVelocity()) < 10) && !tween && !_pointerIsDown && lastSnap !== scroll) {\n\t\t\t\t\tlet progress = (scroll - start) / change, // don't use self.progress because this might run between the refresh() and when the scroll position updates and self.progress is set properly in the update() method.\n\t\t\t\t\t\ttotalProgress = animation && !isToggle ? animation.totalProgress() : progress,\n\t\t\t\t\t\tvelocity = refreshedRecently ? 0 : ((totalProgress - snap2) / (_getTime() - _time2) * 1000) || 0,\n\t\t\t\t\t\tchange1 = gsap.utils.clamp(-progress, 1 - progress, _abs(velocity / 2) * velocity / 0.185),\n\t\t\t\t\t\tnaturalEnd = progress + (snap.inertia === false ? 0 : change1),\n\t\t\t\t\t\tendValue, endScroll,\n\t\t\t\t\t\t{ onStart, onInterrupt, onComplete } = snap;\n\t\t\t\t\tendValue = snapFunc(naturalEnd, self);\n\t\t\t\t\t_isNumber(endValue) || (endValue = naturalEnd); // in case the function didn't return a number, fall back to using the naturalEnd\n\t\t\t\t\tendScroll = Math.max(0, Math.round(start + endValue * change));\n\t\t\t\t\tif (scroll <= end && scroll >= start && endScroll !== scroll) {\n\t\t\t\t\t\tif (tween && !tween._initted && tween.data <= _abs(endScroll - scroll)) { // there's an overlapping snap! So we must figure out which one is closer and let that tween live.\n\t\t\t\t\t\t\treturn;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tif (snap.inertia === false) {\n\t\t\t\t\t\t\tchange1 = endValue - progress;\n\t\t\t\t\t\t}\n\t\t\t\t\t\ttweenTo(endScroll, {\n\t\t\t\t\t\t\tduration: snapDurClamp(_abs( (Math.max(_abs(naturalEnd - totalProgress), _abs(endValue - totalProgress)) * 0.185 / velocity / 0.05) || 0)),\n\t\t\t\t\t\t\tease: snap.ease || \"power3\",\n\t\t\t\t\t\t\tdata: _abs(endScroll - scroll), // record the distance so that if another snap tween occurs (conflict) we can prioritize the closest snap.\n\t\t\t\t\t\t\tonInterrupt: () => snapDelayedCall.restart(true) && onInterrupt && onInterrupt(self),\n\t\t\t\t\t\t\tonComplete() {\n\t\t\t\t\t\t\t\tself.update();\n\t\t\t\t\t\t\t\tlastSnap = scrollFunc();\n\t\t\t\t\t\t\t\tif (animation && !isToggle) { // the resolution of the scrollbar is limited, so we should correct the scrubbed animation's playhead at the end to match EXACTLY where it was supposed to snap\n\t\t\t\t\t\t\t\t\tscrubTween ? scrubTween.resetTo(\"totalProgress\", endValue, animation._tTime / animation._tDur) : animation.progress(endValue);\n\t\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\t\tsnap1 = snap2 = animation && !isToggle ? animation.totalProgress() : self.progress;\n\t\t\t\t\t\t\t\tonSnapComplete && onSnapComplete(self);\n\t\t\t\t\t\t\t\tonComplete && onComplete(self);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}, scroll, change1 * change, endScroll - scroll - change1 * change);\n\t\t\t\t\t\tonStart && onStart(self, tweenTo.tween);\n\t\t\t\t\t}\n\t\t\t\t} else if (self.isActive && lastSnap !== scroll) {\n\t\t\t\t\tsnapDelayedCall.restart(true);\n\t\t\t\t}\n\t\t\t}).pause();\n\t\t}\n\t\tid && (_ids[id] = self);\n\t\ttrigger = self.trigger = _getTarget(trigger || (pin !== true && pin));\n\n\t\t// if a trigger has some kind of scroll-related effect applied that could contaminate the \"y\" or \"x\" position (like a ScrollSmoother effect), we needed a way to temporarily revert it, so we use the stRevert property of the gsCache. It can return another function that we'll call at the end so it can return to its normal state.\n\t\tcustomRevertReturn = trigger && trigger._gsap && trigger._gsap.stRevert;\n\t\tcustomRevertReturn && (customRevertReturn = customRevertReturn(self));\n\n\t\tpin = pin === true ? trigger : _getTarget(pin);\n\t\t_isString(toggleClass) && (toggleClass = {targets: trigger, className: toggleClass});\n\t\tif (pin) {\n\t\t\t(pinSpacing === false || pinSpacing === _margin) || (pinSpacing = !pinSpacing && pin.parentNode && pin.parentNode.style && _getComputedStyle(pin.parentNode).display === \"flex\" ? false : _padding); // if the parent is display: flex, don't apply pinSpacing by default. We should check that pin.parentNode is an element (not shadow dom window)\n\t\t\tself.pin = pin;\n\t\t\tpinCache = gsap.core.getCache(pin);\n\t\t\tif (!pinCache.spacer) { // record the spacer and pinOriginalState on the cache in case someone tries pinning the same element with MULTIPLE ScrollTriggers - we don't want to have multiple spacers or record the \"original\" pin state after it has already been affected by another ScrollTrigger.\n\t\t\t\tif (pinSpacer) {\n\t\t\t\t\tpinSpacer = _getTarget(pinSpacer);\n\t\t\t\t\tpinSpacer && !pinSpacer.nodeType && (pinSpacer = pinSpacer.current || pinSpacer.nativeElement); // for React & Angular\n\t\t\t\t\tpinCache.spacerIsNative = !!pinSpacer;\n\t\t\t\t\tpinSpacer && (pinCache.spacerState = _getState(pinSpacer));\n\t\t\t\t}\n\t\t\t\tpinCache.spacer = spacer = pinSpacer || _doc.createElement(\"div\");\n\t\t\t\tspacer.classList.add(\"pin-spacer\");\n\t\t\t\tid && spacer.classList.add(\"pin-spacer-\" + id);\n\t\t\t\tpinCache.pinState = pinOriginalState = _getState(pin);\n\t\t\t} else {\n\t\t\t\tpinOriginalState = pinCache.pinState;\n\t\t\t}\n\t\t\tvars.force3D !== false && gsap.set(pin, {force3D: true});\n\t\t\tself.spacer = spacer = pinCache.spacer;\n\t\t\tcs = _getComputedStyle(pin);\n\t\t\tspacingStart = cs[pinSpacing + direction.os2];\n\t\t\tpinGetter = gsap.getProperty(pin);\n\t\t\tpinSetter = gsap.quickSetter(pin, direction.a, _px);\n\t\t\t// pin.firstChild && !_maxScroll(pin, direction) && (pin.style.overflow = \"hidden\"); // protects from collapsing margins, but can have unintended consequences as demonstrated here: https://codepen.io/GreenSock/pen/1e42c7a73bfa409d2cf1e184e7a4248d so it was removed in favor of just telling people to set up their CSS to avoid the collapsing margins (overflow: hidden | auto is just one option. Another is border-top: 1px solid transparent).\n\t\t\t_swapPinIn(pin, spacer, cs);\n\t\t\tpinState = _getState(pin);\n\t\t}\n\t\tif (markers) {\n\t\t\tmarkerVars = _isObject(markers) ? _setDefaults(markers, _markerDefaults) : _markerDefaults;\n\t\t\tmarkerStartTrigger = _createMarker(\"scroller-start\", id, scroller, direction, markerVars, 0);\n\t\t\tmarkerEndTrigger = _createMarker(\"scroller-end\", id, scroller, direction, markerVars, 0, markerStartTrigger);\n\t\t\toffset = markerStartTrigger[\"offset\" + direction.op.d2];\n\t\t\tlet content = _getTarget(_getProxyProp(scroller, \"content\") || scroller);\n\t\t\tmarkerStart = this.markerStart = _createMarker(\"start\", id, content, direction, markerVars, offset, 0, containerAnimation);\n\t\t\tmarkerEnd = this.markerEnd = _createMarker(\"end\", id, content, direction, markerVars, offset, 0, containerAnimation);\n\t\t\tcontainerAnimation && (caMarkerSetter = gsap.quickSetter([markerStart, markerEnd], direction.a, _px));\n\t\t\tif ((!useFixedPosition && !(_proxies.length && _getProxyProp(scroller, \"fixedMarkers\") === true))) {\n\t\t\t\t_makePositionable(isViewport ? _body : scroller);\n\t\t\t\tgsap.set([markerStartTrigger, markerEndTrigger], {force3D: true});\n\t\t\t\tmarkerStartSetter = gsap.quickSetter(markerStartTrigger, direction.a, _px);\n\t\t\t\tmarkerEndSetter = gsap.quickSetter(markerEndTrigger, direction.a, _px);\n\t\t\t}\n\t\t}\n\n\t\tif (containerAnimation) {\n\t\t\tlet oldOnUpdate = containerAnimation.vars.onUpdate,\n\t\t\t\toldParams = containerAnimation.vars.onUpdateParams;\n\t\t\tcontainerAnimation.eventCallback(\"onUpdate\", () => {\n\t\t\t\tself.update(0, 0, 1);\n\t\t\t\toldOnUpdate && oldOnUpdate.apply(containerAnimation, oldParams || []);\n\t\t\t});\n\t\t}\n\n\t\tself.previous = () => _triggers[_triggers.indexOf(self) - 1];\n\t\tself.next = () => _triggers[_triggers.indexOf(self) + 1];\n\n\t\tself.revert = (revert, temp) => {\n\t\t\tif (!temp) { return self.kill(true); } // for compatibility with gsap.context() and gsap.matchMedia() which call revert()\n\t\t\tlet r = revert !== false || !self.enabled,\n\t\t\t\tprevRefreshing = _refreshing;\n\t\t\tif (r !== self.isReverted) {\n\t\t\t\tif (r) {\n\t\t\t\t\tprevScroll = Math.max(scrollFunc(), self.scroll.rec || 0); // record the scroll so we can revert later (repositioning/pinning things can affect scroll position). In the static refresh() method, we first record all the scroll positions as a reference.\n\t\t\t\t\tprevProgress = self.progress;\n\t\t\t\t\tprevAnimProgress = animation && animation.progress();\n\t\t\t\t}\n\t\t\t\tmarkerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(m => m.style.display = r ? \"none\" : \"block\");\n\t\t\t\tif (r) {\n\t\t\t\t\t_refreshing = self;\n\t\t\t\t\tself.update(r); // make sure the pin is back in its original position so that all the measurements are correct. do this BEFORE swapping the pin out\n\t\t\t\t}\n\t\t\t\tif (pin && (!pinReparent || !self.isActive)) {\n\t\t\t\t\tif (r) {\n\t\t\t\t\t\t_swapPinOut(pin, spacer, pinOriginalState);\n\t\t\t\t\t} else {\n\t\t\t\t\t\t_swapPinIn(pin, spacer, _getComputedStyle(pin), spacerState);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tr || self.update(r); // when we're restoring, the update should run AFTER swapping the pin into its pin-spacer.\n\t\t\t\t_refreshing = prevRefreshing; // restore. We set it to true during the update() so that things fire properly in there.\n\t\t\t\tself.isReverted = r;\n\t\t\t}\n\t\t}\n\n\t\tself.refresh = (soft, force, position, pinOffset) => { // position is typically only defined if it's coming from setPositions() - it's a way to skip the normal parsing. pinOffset is also only from setPositions() and is mostly related to fancy stuff we need to do in ScrollSmoother with effects\n\t\t\tif ((_refreshing || !self.enabled) && !force) {\n\t\t\t\treturn;\n\t\t\t}\n\t\t\tif (pin && soft && _lastScrollTime) {\n\t\t\t\t_addListener(ScrollTrigger, \"scrollEnd\", _softRefresh);\n\t\t\t\treturn;\n\t\t\t}\n\t\t\t!_refreshingAll && onRefreshInit && onRefreshInit(self);\n\t\t\t_refreshing = self;\n\t\t\tif (tweenTo.tween && !position) { // we skip this if a position is passed in because typically that's from .setPositions() and it's best to allow in-progress snapping to continue.\n\t\t\t\ttweenTo.tween.kill();\n\t\t\t\ttweenTo.tween = 0;\n\t\t\t}\n\t\t\tscrubTween && scrubTween.pause();\n\t\t\tinvalidateOnRefresh && animation && animation.revert({kill: false}).invalidate();\n\t\t\tself.isReverted || self.revert(true, true);\n\t\t\tself._subPinOffset = false; // we'll set this to true in the sub-pins if we find any\n\t\t\tlet size = getScrollerSize(),\n\t\t\t\tscrollerBounds = getScrollerOffsets(),\n\t\t\t\tmax = containerAnimation ? containerAnimation.duration() : _maxScroll(scroller, direction),\n\t\t\t\tisFirstRefresh = change <= 0.01,\n\t\t\t\toffset = 0,\n\t\t\t\totherPinOffset = pinOffset || 0,\n\t\t\t\tparsedEnd = _isObject(position) ? position.end : vars.end,\n\t\t\t\tparsedEndTrigger = vars.endTrigger || trigger,\n\t\t\t\tparsedStart = _isObject(position) ? position.start : (vars.start || (vars.start === 0 || !trigger ? 0 : (pin ? \"0 0\" : \"0 100%\"))),\n\t\t\t\tpinnedContainer = self.pinnedContainer = vars.pinnedContainer && _getTarget(vars.pinnedContainer, self),\n\t\t\t\ttriggerIndex = (trigger && Math.max(0, _triggers.indexOf(self))) || 0,\n\t\t\t\ti = triggerIndex,\n\t\t\t\tcs, bounds, scroll, isVertical, override, curTrigger, curPin, oppositeScroll, initted, revertedPins, forcedOverflow, markerStartOffset, markerEndOffset;\n\t\t\tif (markers && _isObject(position)) { // if we alter the start/end positions with .setPositions(), it generally feeds in absolute NUMBERS which don't convey information about where to line up the markers, so to keep it intuitive, we record how far the trigger positions shift after applying the new numbers and then offset by that much in the opposite direction. We do the same to the associated trigger markers too of course.\n\t\t\t\tmarkerStartOffset = gsap.getProperty(markerStartTrigger, direction.p);\n\t\t\t\tmarkerEndOffset = gsap.getProperty(markerEndTrigger, direction.p);\n\t\t\t}\n\t\t\twhile (i-- > 0) { // user might try to pin the same element more than once, so we must find any prior triggers with the same pin, revert them, and determine how long they're pinning so that we can offset things appropriately. Make sure we revert from last to first so that things \"rewind\" properly.\n\t\t\t\tcurTrigger = _triggers[i];\n\t\t\t\tcurTrigger.end || curTrigger.refresh(0, 1) || (_refreshing = self); // if it's a timeline-based trigger that hasn't been fully initialized yet because it's waiting for 1 tick, just force the refresh() here, otherwise if it contains a pin that's supposed to affect other ScrollTriggers further down the page, they won't be adjusted properly.\n\t\t\t\tcurPin = curTrigger.pin;\n\t\t\t\tif (curPin && (curPin === trigger || curPin === pin || curPin === pinnedContainer) && !curTrigger.isReverted) {\n\t\t\t\t\trevertedPins || (revertedPins = []);\n\t\t\t\t\trevertedPins.unshift(curTrigger); // we'll revert from first to last to make sure things reach their end state properly\n\t\t\t\t\tcurTrigger.revert(true, true);\n\t\t\t\t}\n\t\t\t\tif (curTrigger !== _triggers[i]) { // in case it got removed.\n\t\t\t\t\ttriggerIndex--;\n\t\t\t\t\ti--;\n\t\t\t\t}\n\t\t\t}\n\t\t\t_isFunction(parsedStart) && (parsedStart = parsedStart(self));\n\t\t\tparsedStart = _parseClamp(parsedStart, \"start\", self);\n\t\t\tstart = _parsePosition(parsedStart, trigger, size, direction, scrollFunc(), markerStart, markerStartTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._startClamp && \"_startClamp\") || (pin ? -0.001 : 0);\n\t\t\t_isFunction(parsedEnd) && (parsedEnd = parsedEnd(self));\n\t\t\tif (_isString(parsedEnd) && !parsedEnd.indexOf(\"+=\")) {\n\t\t\t\tif (~parsedEnd.indexOf(\" \")) {\n\t\t\t\t\tparsedEnd = (_isString(parsedStart) ? parsedStart.split(\" \")[0] : \"\") + parsedEnd;\n\t\t\t\t} else {\n\t\t\t\t\toffset = _offsetToPx(parsedEnd.substr(2), size);\n\t\t\t\t\tparsedEnd = _isString(parsedStart) ? parsedStart : (containerAnimation ? gsap.utils.mapRange(0, containerAnimation.duration(), containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, start) : start) + offset; // _parsePosition won't factor in the offset if the start is a number, so do it here.\n\t\t\t\t\tparsedEndTrigger = trigger;\n\t\t\t\t}\n\t\t\t}\n\t\t\tparsedEnd = _parseClamp(parsedEnd, \"end\", self);\n\t\t\tend = Math.max(start, _parsePosition(parsedEnd || (parsedEndTrigger ? \"100% 0\" : max), parsedEndTrigger, size, direction, scrollFunc() + offset, markerEnd, markerEndTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._endClamp && \"_endClamp\")) || -0.001;\n\n\t\t\toffset = 0;\n\t\t\ti = triggerIndex;\n\t\t\twhile (i--) {\n\t\t\t\tcurTrigger = _triggers[i];\n\t\t\t\tcurPin = curTrigger.pin;\n\t\t\t\tif (curPin && curTrigger.start - curTrigger._pinPush <= start && !containerAnimation && curTrigger.end > 0) {\n\t\t\t\t\tcs = curTrigger.end - (self._startClamp ? Math.max(0, curTrigger.start) : curTrigger.start);\n\t\t\t\t\tif (((curPin === trigger && curTrigger.start - curTrigger._pinPush < start) || curPin === pinnedContainer) && isNaN(parsedStart)) { // numeric start values shouldn't be offset at all - treat them as absolute\n\t\t\t\t\t\toffset += cs * (1 - curTrigger.progress);\n\t\t\t\t\t}\n\t\t\t\t\tcurPin === pin && (otherPinOffset += cs);\n\t\t\t\t}\n\t\t\t}\n\t\t\tstart += offset;\n\t\t\tend += offset;\n\t\t\tself._startClamp && (self._startClamp += offset);\n\n\t\t\tif (self._endClamp && !_refreshingAll) {\n\t\t\t\tself._endClamp = end || -0.001;\n\t\t\t\tend = Math.min(end, _maxScroll(scroller, direction));\n\t\t\t}\n\t\t\tchange = (end - start) || ((start -= 0.01) && 0.001);\n\n\t\t\tif (isFirstRefresh) { // on the very first refresh(), the prevProgress couldn't have been accurate yet because the start/end were never calculated, so we set it here. Before 3.11.5, it could lead to an inaccurate scroll position restoration with snapping.\n\t\t\t\tprevProgress = gsap.utils.clamp(0, 1, gsap.utils.normalize(start, end, prevScroll));\n\t\t\t}\n\t\t\tself._pinPush = otherPinOffset;\n\t\t\tif (markerStart && offset) { // offset the markers if necessary\n\t\t\t\tcs = {};\n\t\t\t\tcs[direction.a] = \"+=\" + offset;\n\t\t\t\tpinnedContainer && (cs[direction.p] = \"-=\" + scrollFunc());\n\t\t\t\tgsap.set([markerStart, markerEnd], cs);\n\t\t\t}\n\n\t\t\tif (pin && !(_clampingMax && self.end >= _maxScroll(scroller, direction))) {\n\t\t\t\tcs = _getComputedStyle(pin);\n\t\t\t\tisVertical = direction === _vertical;\n\t\t\t\tscroll = scrollFunc(); // recalculate because the triggers can affect the scroll\n\t\t\t\tpinStart = parseFloat(pinGetter(direction.a)) + otherPinOffset;\n\t\t\t\tif (!max && end > 1) { // makes sure the scroller has a scrollbar, otherwise if something has width: 100%, for example, it would be too big (exclude the scrollbar). See https://gsap.com/forums/topic/25182-scrolltrigger-width-of-page-increase-where-markers-are-set-to-false/\n\t\t\t\t\tforcedOverflow = (isViewport ? (_doc.scrollingElement || _docEl) : scroller).style;\n\t\t\t\t\tforcedOverflow = {style: forcedOverflow, value: forcedOverflow[\"overflow\" + direction.a.toUpperCase()]};\n\t\t\t\t\tif (isViewport && _getComputedStyle(_body)[\"overflow\" + direction.a.toUpperCase()] !== \"scroll\") { // avoid an extra scrollbar if BOTH <html> and <body> have overflow set to \"scroll\"\n\t\t\t\t\t\tforcedOverflow.style[\"overflow\" + direction.a.toUpperCase()] = \"scroll\";\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\t_swapPinIn(pin, spacer, cs);\n\t\t\t\tpinState = _getState(pin);\n\t\t\t\t// transforms will interfere with the top/left/right/bottom placement, so remove them temporarily. getBoundingClientRect() factors in transforms.\n\t\t\t\tbounds = _getBounds(pin, true);\n\t\t\t\toppositeScroll = useFixedPosition && _getScrollFunc(scroller, isVertical ? _horizontal : _vertical)();\n\t\t\t\tif (pinSpacing) {\n\t\t\t\t\tspacerState = [pinSpacing + direction.os2, change + otherPinOffset + _px];\n\t\t\t\t\tspacerState.t = spacer;\n\t\t\t\t\ti = (pinSpacing === _padding) ? _getSize(pin, direction) + change + otherPinOffset : 0;\n\t\t\t\t\tif (i) {\n\t\t\t\t\t\tspacerState.push(direction.d, i + _px); // for box-sizing: border-box (must include padding).\n\t\t\t\t\t\tspacer.style.flexBasis !== \"auto\" && (spacer.style.flexBasis = i + _px);\n\t\t\t\t\t}\n\t\t\t\t\t_setState(spacerState);\n\t\t\t\t\tif (pinnedContainer) { // in ScrollTrigger.refresh(), we need to re-evaluate the pinContainer's size because this pinSpacing may stretch it out, but we can't just add the exact distance because depending on layout, it may not push things down or it may only do so partially.\n\t\t\t\t\t\t_triggers.forEach(t => {\n\t\t\t\t\t\t\tif (t.pin === pinnedContainer && t.vars.pinSpacing !== false) {\n\t\t\t\t\t\t\t\tt._subPinOffset = true;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t});\n\t\t\t\t\t}\n\t\t\t\t\tuseFixedPosition && scrollFunc(prevScroll);\n\t\t\t\t} else {\n\t\t\t\t\ti = _getSize(pin, direction);\n\t\t\t\t\ti && spacer.style.flexBasis !== \"auto\" && (spacer.style.flexBasis = i + _px);\n\t\t\t\t}\n\t\t\t\tif (useFixedPosition) {\n\t\t\t\t\toverride = {\n\t\t\t\t\t\ttop: (bounds.top + (isVertical ? scroll - start : oppositeScroll)) + _px,\n\t\t\t\t\t\tleft: (bounds.left + (isVertical ? oppositeScroll : scroll - start)) + _px,\n\t\t\t\t\t\tboxSizing: \"border-box\",\n\t\t\t\t\t\tposition: \"fixed\"\n\t\t\t\t\t};\n\t\t\t\t\toverride[_width] = override[\"max\" + _Width] = Math.ceil(bounds.width) + _px;\n\t\t\t\t\toverride[_height] = override[\"max\" + _Height] = Math.ceil(bounds.height) + _px;\n\t\t\t\t\toverride[_margin] = override[_margin + _Top] = override[_margin + _Right] = override[_margin + _Bottom] = override[_margin + _Left] = \"0\";\n\t\t\t\t\toverride[_padding] = cs[_padding];\n\t\t\t\t\toverride[_padding + _Top] = cs[_padding + _Top];\n\t\t\t\t\toverride[_padding + _Right] = cs[_padding + _Right];\n\t\t\t\t\toverride[_padding + _Bottom] = cs[_padding + _Bottom];\n\t\t\t\t\toverride[_padding + _Left] = cs[_padding + _Left];\n\t\t\t\t\tpinActiveState = _copyState(pinOriginalState, override, pinReparent);\n\t\t\t\t\t_refreshingAll && scrollFunc(0);\n\t\t\t\t}\n\t\t\t\tif (animation) { // the animation might be affecting the transform, so we must jump to the end, check the value, and compensate accordingly. Otherwise, when it becomes unpinned, the pinSetter() will get set to a value that doesn't include whatever the animation did.\n\t\t\t\t\tinitted = animation._initted; // if not, we must invalidate() after this step, otherwise it could lock in starting values prematurely.\n\t\t\t\t\t_suppressOverwrites(1);\n\t\t\t\t\tanimation.render(animation.duration(), true, true);\n\t\t\t\t\tpinChange = pinGetter(direction.a) - pinStart + change + otherPinOffset;\n\t\t\t\t\tpinMoves = Math.abs(change - pinChange) > 1;\n\t\t\t\t\tuseFixedPosition && pinMoves && pinActiveState.splice(pinActiveState.length - 2, 2); // transform is the last property/value set in the state Array. Since the animation is controlling that, we should omit it.\n\t\t\t\t\tanimation.render(0, true, true);\n\t\t\t\t\tinitted || animation.invalidate(true);\n\t\t\t\t\tanimation.parent || animation.totalTime(animation.totalTime()); // if, for example, a toggleAction called play() and then refresh() happens and when we render(1) above, it would cause the animation to complete and get removed from its parent, so this makes sure it gets put back in.\n\t\t\t\t\t_suppressOverwrites(0);\n\t\t\t\t} else {\n\t\t\t\t\tpinChange = change\n\t\t\t\t}\n\t\t\t\tforcedOverflow && (forcedOverflow.value ? (forcedOverflow.style[\"overflow\" + direction.a.toUpperCase()] = forcedOverflow.value) : forcedOverflow.style.removeProperty(\"overflow-\" + direction.a));\n\t\t\t} else if (trigger && scrollFunc() && !containerAnimation) { // it may be INSIDE a pinned element, so walk up the tree and look for any elements with _pinOffset to compensate because anything with pinSpacing that's already scrolled would throw off the measurements in getBoundingClientRect()\n\t\t\t\tbounds = trigger.parentNode;\n\t\t\t\twhile (bounds && bounds !== _body) {\n\t\t\t\t\tif (bounds._pinOffset) {\n\t\t\t\t\t\tstart -= bounds._pinOffset;\n\t\t\t\t\t\tend -= bounds._pinOffset;\n\t\t\t\t\t}\n\t\t\t\t\tbounds = bounds.parentNode;\n\t\t\t\t}\n\t\t\t}\n\t\t\trevertedPins && revertedPins.forEach(t => t.revert(false, true));\n\t\t\tself.start = start;\n\t\t\tself.end = end;\n\t\t\tscroll1 = scroll2 = _refreshingAll ? prevScroll : scrollFunc(); // reset velocity\n\t\t\tif (!containerAnimation && !_refreshingAll) {\n\t\t\t\tscroll1 < prevScroll && scrollFunc(prevScroll);\n\t\t\t\tself.scroll.rec = 0;\n\t\t\t}\n\t\t\tself.revert(false, true);\n\t\t\tlastRefresh = _getTime();\n\t\t\tif (snapDelayedCall) {\n\t\t\t\tlastSnap = -1; // just so snapping gets re-enabled, clear out any recorded last value\n\t\t\t\t// self.isActive && scrollFunc(start + change * prevProgress); // previously this line was here to ensure that when snapping kicks in, it's from the previous progress but in some cases that's not desirable, like an all-page ScrollTrigger when new content gets added to the page, that'd totally change the progress.\n\t\t\t\tsnapDelayedCall.restart(true);\n\t\t\t}\n\t\t\t_refreshing = 0;\n\t\t\tanimation && isToggle && (animation._initted || prevAnimProgress) && animation.progress() !== prevAnimProgress && animation.progress(prevAnimProgress || 0, true).render(animation.time(), true, true); // must force a re-render because if saveStyles() was used on the target(s), the styles could have been wiped out during the refresh().\n\t\t\tif (isFirstRefresh || prevProgress !== self.progress || containerAnimation || invalidateOnRefresh || (animation && !animation._initted)) { // ensures that the direction is set properly (when refreshing, progress is set back to 0 initially, then back again to wherever it needs to be) and that callbacks are triggered.\n\t\t\t\tanimation && !isToggle && animation.totalProgress(containerAnimation && start < -0.001 && !prevProgress ? gsap.utils.normalize(start, end, 0) : prevProgress, true); // to avoid issues where animation callbacks like onStart aren't triggered.\n\t\t\t\tself.progress = isFirstRefresh || ((scroll1 - start) / change === prevProgress) ? 0 : prevProgress;\n\t\t\t}\n\t\t\tpin && pinSpacing && (spacer._pinOffset = Math.round(self.progress * pinChange));\n\t\t\tscrubTween && scrubTween.invalidate();\n\n\t\t\tif (!isNaN(markerStartOffset)) { // numbers were passed in for the position which are absolute, so instead of just putting the markers at the very bottom of the viewport, we figure out how far they shifted down (it's safe to assume they were originally positioned in closer relation to the trigger element with values like \"top\", \"center\", a percentage or whatever, so we offset that much in the opposite direction to basically revert them to the relative position thy were at previously.\n\t\t\t\tmarkerStartOffset -= gsap.getProperty(markerStartTrigger, direction.p);\n\t\t\t\tmarkerEndOffset -= gsap.getProperty(markerEndTrigger, direction.p);\n\t\t\t\t_shiftMarker(markerStartTrigger, direction, markerStartOffset);\n\t\t\t\t_shiftMarker(markerStart, direction, markerStartOffset - (pinOffset || 0));\n\t\t\t\t_shiftMarker(markerEndTrigger, direction, markerEndOffset);\n\t\t\t\t_shiftMarker(markerEnd, direction, markerEndOffset - (pinOffset || 0));\n\t\t\t}\n\n\t\t\tisFirstRefresh && !_refreshingAll && self.update(); // edge case - when you reload a page when it's already scrolled down, some browsers fire a \"scroll\" event before DOMContentLoaded, triggering an updateAll(). If we don't update the self.progress as part of refresh(), then when it happens next, it may record prevProgress as 0 when it really shouldn't, potentially causing a callback in an animation to fire again.\n\n\t\t\tif (onRefresh && !_refreshingAll && !executingOnRefresh) { // when refreshing all, we do extra work to correct pinnedContainer sizes and ensure things don't exceed the maxScroll, so we should do all the refreshes at the end after all that work so that the start/end values are corrected.\n\t\t\t\texecutingOnRefresh = true;\n\t\t\t\tonRefresh(self);\n\t\t\t\texecutingOnRefresh = false;\n\t\t\t}\n\t\t};\n\n\t\tself.getVelocity = () => ((scrollFunc() - scroll2) / (_getTime() - _time2) * 1000) || 0;\n\n\t\tself.endAnimation = () => {\n\t\t\t_endAnimation(self.callbackAnimation);\n\t\t\tif (animation) {\n\t\t\t\tscrubTween ? scrubTween.progress(1) : (!animation.paused() ? _endAnimation(animation, animation.reversed()) : isToggle || _endAnimation(animation, self.direction < 0, 1));\n\t\t\t}\n\t\t};\n\n\t\tself.labelToScroll = label => animation && animation.labels && ((start || self.refresh() || start) + (animation.labels[label] / animation.duration()) * change) || 0;\n\n\t\tself.getTrailing = name => {\n\t\t\tlet i = _triggers.indexOf(self),\n\t\t\t\ta = self.direction > 0 ? _triggers.slice(0, i).reverse() : _triggers.slice(i+1);\n\t\t\treturn (_isString(name) ? a.filter(t => t.vars.preventOverlaps === name) : a).filter(t => self.direction > 0 ? t.end <= start : t.start >= end);\n\t\t};\n\n\n\t\tself.update = (reset, recordVelocity, forceFake) => {\n\t\t\tif (containerAnimation && !forceFake && !reset) {\n\t\t\t\treturn;\n\t\t\t}\n\t\t\tlet scroll = _refreshingAll === true ? prevScroll : self.scroll(),\n\t\t\t\tp = reset ? 0 : (scroll - start) / change,\n\t\t\t\tclipped = p < 0 ? 0 : p > 1 ? 1 : p || 0,\n\t\t\t\tprevProgress = self.progress,\n\t\t\t\tisActive, wasActive, toggleState, action, stateChanged, toggled, isAtMax, isTakingAction;\n\t\t\tif (recordVelocity) {\n\t\t\t\tscroll2 = scroll1;\n\t\t\t\tscroll1 = containerAnimation ? scrollFunc() : scroll;\n\t\t\t\tif (snap) {\n\t\t\t\t\tsnap2 = snap1;\n\t\t\t\t\tsnap1 = animation && !isToggle ? animation.totalProgress() : clipped;\n\t\t\t\t}\n\t\t\t}\n\t\t\t// anticipate the pinning a few ticks ahead of time based on velocity to avoid a visual glitch due to the fact that most browsers do scrolling on a separate thread (not synced with requestAnimationFrame).\n\t\t\tif (anticipatePin && pin && !_refreshing && !_startup && _lastScrollTime) {\n\t\t\t\tif (!clipped && start < scroll + ((scroll - scroll2) / (_getTime() - _time2)) * anticipatePin) {\n\t\t\t\t\tclipped = 0.0001;\n\t\t\t\t} else if (clipped === 1 && end > scroll + ((scroll - scroll2) / (_getTime() - _time2)) * anticipatePin) {\n\t\t\t\t\tclipped = 0.9999;\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (clipped !== prevProgress && self.enabled) {\n\t\t\t\tisActive = self.isActive = !!clipped && clipped < 1;\n\t\t\t\twasActive = !!prevProgress && prevProgress < 1;\n\t\t\t\ttoggled = isActive !== wasActive;\n\t\t\t\tstateChanged = toggled || !!clipped !== !!prevProgress; // could go from start all the way to end, thus it didn't toggle but it did change state in a sense (may need to fire a callback)\n\t\t\t\tself.direction = clipped > prevProgress ? 1 : -1;\n\t\t\t\tself.progress = clipped;\n\n\t\t\t\tif (stateChanged && !_refreshing) {\n\t\t\t\t\ttoggleState = clipped && !prevProgress ? 0 : clipped === 1 ? 1 : prevProgress === 1 ? 2 : 3; // 0 = enter, 1 = leave, 2 = enterBack, 3 = leaveBack (we prioritize the FIRST encounter, thus if you scroll really fast past the onEnter and onLeave in one tick, it'd prioritize onEnter.\n\t\t\t\t\tif (isToggle) {\n\t\t\t\t\t\taction = (!toggled && toggleActions[toggleState + 1] !== \"none\" && toggleActions[toggleState + 1]) || toggleActions[toggleState]; // if it didn't toggle, that means it shot right past and since we prioritize the \"enter\" action, we should switch to the \"leave\" in this case (but only if one is defined)\n\t\t\t\t\t\tisTakingAction = animation && (action === \"complete\" || action === \"reset\" || action in animation);\n\t\t\t\t\t}\n\t\t\t\t}\n\n\t\t\t\tpreventOverlaps && (toggled || isTakingAction) && (isTakingAction || scrub || !animation) && (_isFunction(preventOverlaps) ? preventOverlaps(self) : self.getTrailing(preventOverlaps).forEach(t => t.endAnimation()));\n\n\t\t\t\tif (!isToggle) {\n\t\t\t\t\tif (scrubTween && !_refreshing && !_startup) {\n\t\t\t\t\t\t(scrubTween._dp._time - scrubTween._start !== scrubTween._time) && scrubTween.render(scrubTween._dp._time - scrubTween._start); // if there's a scrub on both the container animation and this one (or a ScrollSmoother), the update order would cause this one not to have rendered yet, so it wouldn't make any progress before we .restart() it heading toward the new progress so it'd appear stuck thus we force a render here.\n\t\t\t\t\t\tif (scrubTween.resetTo) {\n\t\t\t\t\t\t\tscrubTween.resetTo(\"totalProgress\", clipped, animation._tTime / animation._tDur);\n\t\t\t\t\t\t} else { // legacy support (courtesy), before 3.10.0\n\t\t\t\t\t\t\tscrubTween.vars.totalProgress = clipped;\n\t\t\t\t\t\t\tscrubTween.invalidate().restart();\n\t\t\t\t\t\t}\n\t\t\t\t\t} else if (animation) {\n\t\t\t\t\t\tanimation.totalProgress(clipped, !!(_refreshing && (lastRefresh || reset)));\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (pin) {\n\t\t\t\t\treset && pinSpacing && (spacer.style[pinSpacing + direction.os2] = spacingStart);\n\t\t\t\t\tif (!useFixedPosition) {\n\t\t\t\t\t\tpinSetter(_round(pinStart + pinChange * clipped));\n\t\t\t\t\t} else if (stateChanged) {\n\t\t\t\t\t\tisAtMax = !reset && clipped > prevProgress && end + 1 > scroll && scroll + 1 >= _maxScroll(scroller, direction); // if it's at the VERY end of the page, don't switch away from position: fixed because it's pointless and it could cause a brief flash when the user scrolls back up (when it gets pinned again)\n\t\t\t\t\t\tif (pinReparent) {\n\t\t\t\t\t\t\tif (!reset && (isActive || isAtMax)) {\n\t\t\t\t\t\t\t\tlet bounds = _getBounds(pin, true),\n\t\t\t\t\t\t\t\t\toffset = scroll - start;\n\t\t\t\t\t\t\t\t_reparent(pin, _body, (bounds.top + (direction === _vertical ? offset : 0)) + _px, (bounds.left + (direction === _vertical ? 0 : offset)) + _px);\n\t\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\t\t_reparent(pin, spacer);\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\t_setState(isActive || isAtMax ? pinActiveState : pinState);\n\t\t\t\t\t\t(pinMoves && clipped < 1 && isActive) || pinSetter(pinStart + (clipped === 1 && !isAtMax ? pinChange : 0));\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tsnap && !tweenTo.tween && !_refreshing && !_startup && snapDelayedCall.restart(true);\n\t\t\t\ttoggleClass && (toggled || (once && clipped && (clipped < 1 || !_limitCallbacks))) && _toArray(toggleClass.targets).forEach(el => el.classList[isActive || once ? \"add\" : \"remove\"](toggleClass.className)); // classes could affect positioning, so do it even if reset or refreshing is true.\n\t\t\t\tonUpdate && !isToggle && !reset && onUpdate(self);\n\t\t\t\tif (stateChanged && !_refreshing) {\n\t\t\t\t\tif (isToggle) {\n\t\t\t\t\t\tif (isTakingAction) {\n\t\t\t\t\t\t\tif (action === \"complete\") {\n\t\t\t\t\t\t\t\tanimation.pause().totalProgress(1);\n\t\t\t\t\t\t\t} else if (action === \"reset\") {\n\t\t\t\t\t\t\t\tanimation.restart(true).pause();\n\t\t\t\t\t\t\t} else if (action === \"restart\") {\n\t\t\t\t\t\t\t\tanimation.restart(true);\n\t\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\t\tanimation[action]();\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t}\n\t\t\t\t\t\tonUpdate && onUpdate(self);\n\t\t\t\t\t}\n\t\t\t\t\tif (toggled || !_limitCallbacks) { // on startup, the page could be scrolled and we don't want to fire callbacks that didn't toggle. For example onEnter shouldn't fire if the ScrollTrigger isn't actually entered.\n\t\t\t\t\t\tonToggle && toggled && _callback(self, onToggle);\n\t\t\t\t\t\tcallbacks[toggleState] && _callback(self, callbacks[toggleState]);\n\t\t\t\t\t\tonce && (clipped === 1 ? self.kill(false, 1) : (callbacks[toggleState] = 0)); // a callback shouldn't be called again if once is true.\n\t\t\t\t\t\tif (!toggled) { // it's possible to go completely past, like from before the start to after the end (or vice-versa) in which case BOTH callbacks should be fired in that order\n\t\t\t\t\t\t\ttoggleState = clipped === 1 ? 1 : 3;\n\t\t\t\t\t\t\tcallbacks[toggleState] && _callback(self, callbacks[toggleState]);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (fastScrollEnd && !isActive && Math.abs(self.getVelocity()) > (_isNumber(fastScrollEnd) ? fastScrollEnd : 2500)) {\n\t\t\t\t\t\t_endAnimation(self.callbackAnimation);\n\t\t\t\t\t\tscrubTween ? scrubTween.progress(1) : _endAnimation(animation, action === \"reverse\" ? 1 : !clipped, 1);\n\t\t\t\t\t}\n\t\t\t\t} else if (isToggle && onUpdate && !_refreshing) {\n\t\t\t\t\tonUpdate(self);\n\t\t\t\t}\n\t\t\t}\n\t\t\t// update absolutely-positioned markers (only if the scroller isn't the viewport)\n\t\t\tif (markerEndSetter) {\n\t\t\t\tlet n = containerAnimation ? scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0) : scroll;\n\t\t\t\tmarkerStartSetter(n + (markerStartTrigger._isFlipped ? 1 : 0));\n\t\t\t\tmarkerEndSetter(n);\n\t\t\t}\n\t\t\tcaMarkerSetter && caMarkerSetter(-scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0));\n\t\t};\n\n\t\tself.enable = (reset, refresh) => {\n\t\t\tif (!self.enabled) {\n\t\t\t\tself.enabled = true;\n\t\t\t\t_addListener(scroller, \"resize\", _onResize);\n\t\t\t\tisViewport || _addListener(scroller, \"scroll\", _onScroll);\n\t\t\t\tonRefreshInit && _addListener(ScrollTrigger, \"refreshInit\", onRefreshInit);\n\t\t\t\tif (reset !== false) {\n\t\t\t\t\tself.progress = prevProgress = 0;\n\t\t\t\t\tscroll1 = scroll2 = lastSnap = scrollFunc();\n\t\t\t\t}\n\t\t\t\trefresh !== false && self.refresh();\n\t\t\t}\n\t\t};\n\n\t\tself.getTween = snap => snap && tweenTo ? tweenTo.tween : scrubTween;\n\n\t\tself.setPositions = (newStart, newEnd, keepClamp, pinOffset) => { // doesn't persist after refresh()! Intended to be a way to override values that were set during refresh(), like you could set it in onRefresh()\n\t\t\tif (containerAnimation) { // convert ratios into scroll positions. Remember, start/end values on ScrollTriggers that have a containerAnimation refer to the time (in seconds), NOT scroll positions.\n\t\t\t\tlet st = containerAnimation.scrollTrigger,\n\t\t\t\t\tduration = containerAnimation.duration(),\n\t\t\t\t\tchange = st.end - st.start;\n\t\t\t\tnewStart = st.start + change * newStart / duration;\n\t\t\t\tnewEnd = st.start + change * newEnd / duration;\n\t\t\t}\n\t\t\tself.refresh(false, false, {start: _keepClamp(newStart, keepClamp && !!self._startClamp), end: _keepClamp(newEnd, keepClamp && !!self._endClamp)}, pinOffset);\n\t\t\tself.update();\n\t\t};\n\n\t\tself.adjustPinSpacing = amount => {\n\t\t\tif (spacerState && amount) {\n\t\t\t\tlet i = spacerState.indexOf(direction.d) + 1;\n\t\t\t\tspacerState[i] = (parseFloat(spacerState[i]) + amount) + _px;\n\t\t\t\tspacerState[1] = (parseFloat(spacerState[1]) + amount) + _px;\n\t\t\t\t_setState(spacerState);\n\t\t\t}\n\t\t};\n\n\t\tself.disable = (reset, allowAnimation) => {\n\t\t\tif (self.enabled) {\n\t\t\t\treset !== false && self.revert(true, true);\n\t\t\t\tself.enabled = self.isActive = false;\n\t\t\t\tallowAnimation || (scrubTween && scrubTween.pause());\n\t\t\t\tprevScroll = 0;\n\t\t\t\tpinCache && (pinCache.uncache = 1);\n\t\t\t\tonRefreshInit && _removeListener(ScrollTrigger, \"refreshInit\", onRefreshInit);\n\t\t\t\tif (snapDelayedCall) {\n\t\t\t\t\tsnapDelayedCall.pause();\n\t\t\t\t\ttweenTo.tween && tweenTo.tween.kill() && (tweenTo.tween = 0);\n\t\t\t\t}\n\t\t\t\tif (!isViewport) {\n\t\t\t\t\tlet i = _triggers.length;\n\t\t\t\t\twhile (i--) {\n\t\t\t\t\t\tif (_triggers[i].scroller === scroller && _triggers[i] !== self) {\n\t\t\t\t\t\t\treturn; //don't remove the listeners if there are still other triggers referencing it.\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\t_removeListener(scroller, \"resize\", _onResize);\n\t\t\t\t\tisViewport || _removeListener(scroller, \"scroll\", _onScroll);\n\t\t\t\t}\n\t\t\t}\n\t\t};\n\n\t\tself.kill = (revert, allowAnimation) => {\n\t\t\tself.disable(revert, allowAnimation);\n\t\t\tscrubTween && !allowAnimation && scrubTween.kill();\n\t\t\tid && (delete _ids[id]);\n\t\t\tlet i = _triggers.indexOf(self);\n\t\t\ti >= 0 && _triggers.splice(i, 1);\n\t\t\ti === _i && _direction > 0 && _i--; // if we're in the middle of a refresh() or update(), splicing would cause skips in the index, so adjust...\n\n\t\t\t// if no other ScrollTrigger instances of the same scroller are found, wipe out any recorded scroll position. Otherwise, in a single page application, for example, it could maintain scroll position when it really shouldn't.\n\t\t\ti = 0;\n\t\t\t_triggers.forEach(t => t.scroller === self.scroller && (i = 1));\n\t\t\ti || _refreshingAll || (self.scroll.rec = 0);\n\n\t\t\tif (animation) {\n\t\t\t\tanimation.scrollTrigger = null;\n\t\t\t\trevert && animation.revert({kill: false});\n\t\t\t\tallowAnimation || animation.kill();\n\t\t\t}\n\t\t\tmarkerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(m => m.parentNode && m.parentNode.removeChild(m));\n\t\t\t_primary === self && (_primary = 0);\n\t\t\tif (pin) {\n\t\t\t\tpinCache && (pinCache.uncache = 1);\n\t\t\t\ti = 0;\n\t\t\t\t_triggers.forEach(t => t.pin === pin && i++);\n\t\t\t\ti || (pinCache.spacer = 0); // if there aren't any more ScrollTriggers with the same pin, remove the spacer, otherwise it could be contaminated with old/stale values if the user re-creates a ScrollTrigger for the same element.\n\t\t\t}\n\t\t\tvars.onKill && vars.onKill(self);\n\t\t};\n\n\t\t_triggers.push(self);\n\t\tself.enable(false, false);\n\t\tcustomRevertReturn && customRevertReturn(self);\n\n\t\tif (animation && animation.add && !change) { // if the animation is a timeline, it may not have been populated yet, so it wouldn't render at the proper place on the first refresh(), thus we should schedule one for the next tick. If \"change\" is defined, we know it must be re-enabling, thus we can refresh() right away.\n\t\t\tlet updateFunc = self.update; // some browsers may fire a scroll event BEFORE a tick elapses and/or the DOMContentLoaded fires. So there's a chance update() will be called BEFORE a refresh() has happened on a Timeline-attached ScrollTrigger which means the start/end won't be calculated yet. We don't want to add conditional logic inside the update() method (like check to see if end is defined and if not, force a refresh()) because that's a function that gets hit a LOT (performance). So we swap out the real update() method for this one that'll re-attach it the first time it gets called and of course forces a refresh().\n\t\t\tself.update = () => {\n\t\t\t\tself.update = updateFunc;\n\t\t\t\t_scrollers.cache++; // otherwise a cached scroll position may get used in the refresh() in a very rare scenario, like if ScrollTriggers are created inside a DOMContentLoaded event and the queued requestAnimationFrame() fires beforehand. See https://gsap.com/community/forums/topic/41267-scrolltrigger-breaks-on-refresh-when-using-domcontentloaded/\n\t\t\t\tstart || end || self.refresh();\n\t\t\t};\n\t\t\tgsap.delayedCall(0.01, self.update);\n\t\t\tchange = 0.01;\n\t\t\tstart = end = 0;\n\t\t} else {\n\t\t\tself.refresh();\n\t\t}\n\t\tpin && _queueRefreshAll(); // pinning could affect the positions of other things, so make sure we queue a full refresh()\n\t}\n\n\n\tstatic register(core) {\n\t\tif (!_coreInitted) {\n\t\t\tgsap = core || _getGSAP();\n\t\t\t_windowExists() && window.document && ScrollTrigger.enable();\n\t\t\t_coreInitted = _enabled;\n\t\t}\n\t\treturn _coreInitted;\n\t}\n\n\tstatic defaults(config) {\n\t\tif (config) {\n\t\t\tfor (let p in config) {\n\t\t\t\t_defaults[p] = config[p];\n\t\t\t}\n\t\t}\n\t\treturn _defaults;\n\t}\n\n\tstatic disable(reset, kill) {\n\t\t_enabled = 0;\n\t\t_triggers.forEach(trigger => trigger[kill ? \"kill\" : \"disable\"](reset));\n\t\t_removeListener(_win, \"wheel\", _onScroll);\n\t\t_removeListener(_doc, \"scroll\", _onScroll);\n\t\tclearInterval(_syncInterval);\n\t\t_removeListener(_doc, \"touchcancel\", _passThrough);\n\t\t_removeListener(_body, \"touchstart\", _passThrough);\n\t\t_multiListener(_removeListener, _doc, \"pointerdown,touchstart,mousedown\", _pointerDownHandler);\n\t\t_multiListener(_removeListener, _doc, \"pointerup,touchend,mouseup\", _pointerUpHandler);\n\t\t_resizeDelay.kill();\n\t\t_iterateAutoRefresh(_removeListener);\n\t\tfor (let i = 0; i < _scrollers.length; i+=3) {\n\t\t\t_wheelListener(_removeListener, _scrollers[i], _scrollers[i+1]);\n\t\t\t_wheelListener(_removeListener, _scrollers[i], _scrollers[i+2]);\n\t\t}\n\t}\n\n\tstatic enable() {\n\t\t_win = window;\n\t\t_doc = document;\n\t\t_docEl = _doc.documentElement;\n\t\t_body = _doc.body;\n\t\tif (gsap) {\n\t\t\t_toArray = gsap.utils.toArray;\n\t\t\t_clamp = gsap.utils.clamp;\n\t\t\t_context = gsap.core.context || _passThrough;\n\t\t\t_suppressOverwrites = gsap.core.suppressOverwrites || _passThrough;\n\t\t\t_scrollRestoration = _win.history.scrollRestoration || \"auto\";\n\t\t\t_lastScroll = _win.pageYOffset || 0;\n\t\t\tgsap.core.globals(\"ScrollTrigger\", ScrollTrigger); // must register the global manually because in Internet Explorer, functions (classes) don't have a \"name\" property.\n\t\t\tif (_body) {\n\t\t\t\t_enabled = 1;\n\t\t\t\t_div100vh = document.createElement(\"div\"); // to solve mobile browser address bar show/hide resizing, we shouldn't rely on window.innerHeight. Instead, use a <div> with its height set to 100vh and measure that since that's what the scrolling is based on anyway and it's not affected by address bar showing/hiding.\n\t\t\t\t_div100vh.style.height = \"100vh\";\n\t\t\t\t_div100vh.style.position = \"absolute\";\n\t\t\t\t_refresh100vh();\n\t\t\t\t_rafBugFix();\n\t\t\t\tObserver.register(gsap);\n\t\t\t\t// isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer.\n\t\t\t\tScrollTrigger.isTouch = Observer.isTouch;\n\t\t\t\t_fixIOSBug = Observer.isTouch && /(iPad|iPhone|iPod|Mac)/g.test(navigator.userAgent); // since 2017, iOS has had a bug that causes event.clientX/Y to be inaccurate when a scroll occurs, thus we must alternate ignoring every other touchmove event to work around it. See https://bugs.webkit.org/show_bug.cgi?id=181954 and https://codepen.io/GreenSock/pen/ExbrPNa/087cef197dc35445a0951e8935c41503\n\t\t\t\t_ignoreMobileResize = Observer.isTouch === 1;\n\t\t\t\t_addListener(_win, \"wheel\", _onScroll); // mostly for 3rd party smooth scrolling libraries.\n\t\t\t\t_root = [_win, _doc, _docEl, _body];\n\t\t\t\tif (gsap.matchMedia) {\n\t\t\t\t\tScrollTrigger.matchMedia = vars => {\n\t\t\t\t\t\tlet mm = gsap.matchMedia(),\n\t\t\t\t\t\t\tp;\n\t\t\t\t\t\tfor (p in vars) {\n\t\t\t\t\t\t\tmm.add(p, vars[p]);\n\t\t\t\t\t\t}\n\t\t\t\t\t\treturn mm;\n\t\t\t\t\t};\n\t\t\t\t\tgsap.addEventListener(\"matchMediaInit\", () => _revertAll());\n\t\t\t\t\tgsap.addEventListener(\"matchMediaRevert\", () => _revertRecorded());\n\t\t\t\t\tgsap.addEventListener(\"matchMedia\", () => {\n\t\t\t\t\t\t_refreshAll(0, 1);\n\t\t\t\t\t\t_dispatch(\"matchMedia\");\n\t\t\t\t\t});\n\t\t\t\t\tgsap.matchMedia().add(\"(orientation: portrait)\", () => { // when orientation changes, we should take new base measurements for the ignoreMobileResize feature.\n\t\t\t\t\t\t_setBaseDimensions();\n\t\t\t\t\t\treturn _setBaseDimensions;\n\t\t\t\t\t});\n\t\t\t\t} else {\n\t\t\t\t\tconsole.warn(\"Requires GSAP 3.11.0 or later\");\n\t\t\t\t}\n\t\t\t\t_setBaseDimensions();\n\t\t\t\t_addListener(_doc, \"scroll\", _onScroll); // some browsers (like Chrome), the window stops dispatching scroll events on the window if you scroll really fast, but it's consistent on the document!\n\t\t\t\tlet bodyHasStyle = _body.hasAttribute(\"style\"),\n\t\t\t\t\tbodyStyle = _body.style,\n\t\t\t\t\tborder = bodyStyle.borderTopStyle,\n\t\t\t\t\tAnimationProto = gsap.core.Animation.prototype,\n\t\t\t\t\tbounds, i;\n\t\t\t\tAnimationProto.revert || Object.defineProperty(AnimationProto, \"revert\", { value: function() { return this.time(-0.01, true); }}); // only for backwards compatibility (Animation.revert() was added after 3.10.4)\n\t\t\t\tbodyStyle.borderTopStyle = \"solid\"; // works around an issue where a margin of a child element could throw off the bounds of the _body, making it seem like there's a margin when there actually isn't. The border ensures that the bounds are accurate.\n\t\t\t\tbounds = _getBounds(_body);\n\t\t\t\t_vertical.m = Math.round(bounds.top + _vertical.sc()) || 0; // accommodate the offset of the <body> caused by margins and/or padding\n\t\t\t\t_horizontal.m = Math.round(bounds.left + _horizontal.sc()) || 0;\n\t\t\t\tborder ? (bodyStyle.borderTopStyle = border) : bodyStyle.removeProperty(\"border-top-style\");\n\t\t\t\tif (!bodyHasStyle) { // SSR frameworks like Next.js complain if this attribute gets added.\n\t\t\t\t\t_body.setAttribute(\"style\", \"\"); // it's not enough to just removeAttribute() - we must first set it to empty, otherwise Next.js complains.\n\t\t\t\t\t_body.removeAttribute(\"style\");\n\t\t\t\t}\n\t\t\t\t// TODO: (?) maybe move to leveraging the velocity mechanism in Observer and skip intervals.\n\t\t\t\t_syncInterval = setInterval(_sync, 250);\n\t\t\t\tgsap.delayedCall(0.5, () => _startup = 0);\n\t\t\t\t_addListener(_doc, \"touchcancel\", _passThrough); // some older Android devices intermittently stop dispatching \"touchmove\" events if we don't listen for \"touchcancel\" on the document.\n\t\t\t\t_addListener(_body, \"touchstart\", _passThrough); //works around Safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/\n\t\t\t\t_multiListener(_addListener, _doc, \"pointerdown,touchstart,mousedown\", _pointerDownHandler);\n\t\t\t\t_multiListener(_addListener, _doc, \"pointerup,touchend,mouseup\", _pointerUpHandler);\n\t\t\t\t_transformProp = gsap.utils.checkPrefix(\"transform\");\n\t\t\t\t_stateProps.push(_transformProp);\n\t\t\t\t_coreInitted = _getTime();\n\t\t\t\t_resizeDelay = gsap.delayedCall(0.2, _refreshAll).pause();\n\t\t\t\t_autoRefresh = [_doc, \"visibilitychange\", () => {\n\t\t\t\t\tlet w = _win.innerWidth,\n\t\t\t\t\t\th = _win.innerHeight;\n\t\t\t\t\tif (_doc.hidden) {\n\t\t\t\t\t\t_prevWidth = w;\n\t\t\t\t\t\t_prevHeight = h;\n\t\t\t\t\t} else if (_prevWidth !== w || _prevHeight !== h) {\n\t\t\t\t\t\t_onResize();\n\t\t\t\t\t}\n\t\t\t\t}, _doc, \"DOMContentLoaded\", _refreshAll, _win, \"load\", _refreshAll, _win, \"resize\", _onResize];\n\t\t\t\t_iterateAutoRefresh(_addListener);\n\t\t\t\t_triggers.forEach(trigger => trigger.enable(0, 1));\n\t\t\t\tfor (i = 0; i < _scrollers.length; i+=3) {\n\t\t\t\t\t_wheelListener(_removeListener, _scrollers[i], _scrollers[i+1]);\n\t\t\t\t\t_wheelListener(_removeListener, _scrollers[i], _scrollers[i+2]);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t}\n\n\tstatic config(vars) {\n\t\t(\"limitCallbacks\" in vars) && (_limitCallbacks = !!vars.limitCallbacks);\n\t\tlet ms = vars.syncInterval;\n\t\tms && clearInterval(_syncInterval) || ((_syncInterval = ms) && setInterval(_sync, ms));\n\t\t(\"ignoreMobileResize\" in vars) && (_ignoreMobileResize = ScrollTrigger.isTouch === 1 && vars.ignoreMobileResize);\n\t\tif (\"autoRefreshEvents\" in vars) {\n\t\t\t_iterateAutoRefresh(_removeListener) || _iterateAutoRefresh(_addListener, vars.autoRefreshEvents || \"none\");\n\t\t\t_ignoreResize = (vars.autoRefreshEvents + \"\").indexOf(\"resize\") === -1;\n\t\t}\n\t}\n\n\tstatic scrollerProxy(target, vars) {\n\t\tlet t = _getTarget(target),\n\t\t\ti = _scrollers.indexOf(t),\n\t\t\tisViewport = _isViewport(t);\n\t\tif (~i) {\n\t\t\t_scrollers.splice(i, isViewport ? 6 : 2);\n\t\t}\n\t\tif (vars) {\n\t\t\tisViewport ? _proxies.unshift(_win, vars, _body, vars, _docEl, vars) : _proxies.unshift(t, vars);\n\t\t}\n\t}\n\n\tstatic clearMatchMedia(query) {\n\t\t_triggers.forEach(t => t._ctx && t._ctx.query === query && t._ctx.kill(true, true));\n\t}\n\n\tstatic isInViewport(element, ratio, horizontal) {\n\t\tlet bounds = (_isString(element) ? _getTarget(element) : element).getBoundingClientRect(),\n\t\t\toffset = bounds[horizontal ? _width : _height] * ratio || 0;\n\t\treturn horizontal ? bounds.right - offset > 0 && bounds.left + offset < _win.innerWidth : bounds.bottom - offset > 0 && bounds.top + offset < _win.innerHeight;\n\t}\n\n\tstatic positionInViewport(element, referencePoint, horizontal) {\n\t\t_isString(element) && (element = _getTarget(element));\n\t\tlet bounds = element.getBoundingClientRect(),\n\t\t\tsize = bounds[horizontal ? _width : _height],\n\t\t\toffset = referencePoint == null ? size / 2 : ((referencePoint in _keywords) ? _keywords[referencePoint] * size : ~referencePoint.indexOf(\"%\") ? parseFloat(referencePoint) * size / 100 : parseFloat(referencePoint) || 0);\n\t\treturn horizontal ? (bounds.left + offset) / _win.innerWidth : (bounds.top + offset) / _win.innerHeight;\n\t}\n\n\tstatic killAll(allowListeners) {\n\t\t_triggers.slice(0).forEach(t => t.vars.id !== \"ScrollSmoother\" && t.kill());\n\t\tif (allowListeners !== true) {\n\t\t\tlet listeners = _listeners.killAll || [];\n\t\t\t_listeners = {};\n\t\t\tlisteners.forEach(f => f());\n\t\t}\n\t}\n\n}\n\nScrollTrigger.version = \"3.12.7\";\nScrollTrigger.saveStyles = targets => targets ? _toArray(targets).forEach(target => { // saved styles are recorded in a consecutive alternating Array, like [element, cssText, transform attribute, cache, matchMedia, ...]\n\tif (target && target.style) {\n\t\tlet i = _savedStyles.indexOf(target);\n\t\ti >= 0 && _savedStyles.splice(i, 5);\n\t\t_savedStyles.push(target, target.style.cssText, target.getBBox && target.getAttribute(\"transform\"), gsap.core.getCache(target), _context());\n\t}\n}) : _savedStyles;\nScrollTrigger.revert = (soft, media) => _revertAll(!soft, media);\nScrollTrigger.create = (vars, animation) => new ScrollTrigger(vars, animation);\nScrollTrigger.refresh = safe => safe ? _onResize(true) : (_coreInitted || ScrollTrigger.register()) && _refreshAll(true);\nScrollTrigger.update = force => ++_scrollers.cache && _updateAll(force === true ? 2 : 0);\nScrollTrigger.clearScrollMemory = _clearScrollMemory;\nScrollTrigger.maxScroll = (element, horizontal) => _maxScroll(element, horizontal ? _horizontal : _vertical);\nScrollTrigger.getScrollFunc = (element, horizontal) => _getScrollFunc(_getTarget(element), horizontal ? _horizontal : _vertical);\nScrollTrigger.getById = id => _ids[id];\nScrollTrigger.getAll = () => _triggers.filter(t => t.vars.id !== \"ScrollSmoother\"); // it's common for people to ScrollTrigger.getAll(t => t.kill()) on page routes, for example, and we don't want it to ruin smooth scrolling by killing the main ScrollSmoother one.\nScrollTrigger.isScrolling = () => !!_lastScrollTime;\nScrollTrigger.snapDirectional = _snapDirectional;\nScrollTrigger.addEventListener = (type, callback) => {\n\tlet a = _listeners[type] || (_listeners[type] = []);\n\t~a.indexOf(callback) || a.push(callback);\n};\nScrollTrigger.removeEventListener = (type, callback) => {\n\tlet a = _listeners[type],\n\t\ti = a && a.indexOf(callback);\n\ti >= 0 && a.splice(i, 1);\n};\nScrollTrigger.batch = (targets, vars) => {\n\tlet result = [],\n\t\tvarsCopy = {},\n\t\tinterval = vars.interval || 0.016,\n\t\tbatchMax = vars.batchMax || 1e9,\n\t\tproxyCallback = (type, callback) => {\n\t\t\tlet elements = [],\n\t\t\t\ttriggers = [],\n\t\t\t\tdelay = gsap.delayedCall(interval, () => {callback(elements, triggers); elements = []; triggers = [];}).pause();\n\t\t\treturn self => {\n\t\t\t\telements.length || delay.restart(true);\n\t\t\t\telements.push(self.trigger);\n\t\t\t\ttriggers.push(self);\n\t\t\t\tbatchMax <= elements.length && delay.progress(1);\n\t\t\t};\n\t\t},\n\t\tp;\n\tfor (p in vars) {\n\t\tvarsCopy[p] = (p.substr(0, 2) === \"on\" && _isFunction(vars[p]) && p !== \"onRefreshInit\") ? proxyCallback(p, vars[p]) : vars[p];\n\t}\n\tif (_isFunction(batchMax)) {\n\t\tbatchMax = batchMax();\n\t\t_addListener(ScrollTrigger, \"refresh\", () => batchMax = vars.batchMax());\n\t}\n\t_toArray(targets).forEach(target => {\n\t\tlet config = {};\n\t\tfor (p in varsCopy) {\n\t\t\tconfig[p] = varsCopy[p];\n\t\t}\n\t\tconfig.trigger = target;\n\t\tresult.push(ScrollTrigger.create(config));\n\t});\n\treturn result;\n}\n\n\n// to reduce file size. clamps the scroll and also returns a duration multiplier so that if the scroll gets chopped shorter, the duration gets curtailed as well (otherwise if you're very close to the top of the page, for example, and swipe up really fast, it'll suddenly slow down and take a long time to reach the top).\nlet _clampScrollAndGetDurationMultiplier = (scrollFunc, current, end, max) => {\n\t\tcurrent > max ? scrollFunc(max) : current < 0 && scrollFunc(0);\n\t\treturn end > max ? (max - current) / (end - current) : end < 0 ? current / (current - end) : 1;\n\t},\n\t_allowNativePanning = (target, direction) => {\n\t\tif (direction === true) {\n\t\t\ttarget.style.removeProperty(\"touch-action\");\n\t\t} else {\n\t\t\ttarget.style.touchAction = direction === true ? \"auto\" : direction ? \"pan-\" + direction + (Observer.isTouch ? \" pinch-zoom\" : \"\") : \"none\"; // note: Firefox doesn't support it pinch-zoom properly, at least in addition to a pan-x or pan-y.\n\t\t}\n\t\ttarget === _docEl && _allowNativePanning(_body, direction);\n\t},\n\t_overflow = {auto: 1, scroll: 1},\n\t_nestedScroll = ({event, target, axis}) => {\n\t\tlet node = (event.changedTouches ? event.changedTouches[0] : event).target,\n\t\t\tcache = node._gsap || gsap.core.getCache(node),\n\t\t\ttime = _getTime(), cs;\n\t\tif (!cache._isScrollT || time - cache._isScrollT > 2000) { // cache for 2 seconds to improve performance.\n\t\t\twhile (node && node !== _body && ((node.scrollHeight <= node.clientHeight && node.scrollWidth <= node.clientWidth) || !(_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]))) node = node.parentNode;\n\t\t\tcache._isScroll = node && node !== target && !_isViewport(node) && (_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]);\n\t\t\tcache._isScrollT = time;\n\t\t}\n\t\tif (cache._isScroll || axis === \"x\") {\n\t\t\tevent.stopPropagation();\n\t\t\tevent._gsapAllow = true;\n\t\t}\n\t},\n\t// capture events on scrollable elements INSIDE the <body> and allow those by calling stopPropagation() when we find a scrollable ancestor\n\t_inputObserver = (target, type, inputs, nested) => Observer.create({\n\t\ttarget: target,\n\t\tcapture: true,\n\t\tdebounce: false,\n\t\tlockAxis: true,\n\t\ttype: type,\n\t\tonWheel: (nested = nested && _nestedScroll),\n\t\tonPress: nested,\n\t\tonDrag: nested,\n\t\tonScroll: nested,\n\t\tonEnable: () => inputs && _addListener(_doc, Observer.eventTypes[0], _captureInputs, false, true),\n\t\tonDisable: () => _removeListener(_doc, Observer.eventTypes[0], _captureInputs, true)\n\t}),\n\t_inputExp = /(input|label|select|textarea)/i,\n\t_inputIsFocused,\n\t_captureInputs = e => {\n\t\tlet isInput = _inputExp.test(e.target.tagName);\n\t\tif (isInput || _inputIsFocused) {\n\t\t\te._gsapAllow = true;\n\t\t\t_inputIsFocused = isInput;\n\t\t}\n\t},\n\t_getScrollNormalizer = vars => {\n\t\t_isObject(vars) || (vars = {});\n\t\tvars.preventDefault = vars.isNormalizer = vars.allowClicks = true;\n\t\tvars.type || (vars.type = \"wheel,touch\");\n\t\tvars.debounce = !!vars.debounce;\n\t\tvars.id = vars.id || \"normalizer\";\n\t\tlet {normalizeScrollX, momentum, allowNestedScroll, onRelease} = vars,\n\t\t\tself, maxY,\n\t\t\ttarget = _getTarget(vars.target) || _docEl,\n\t\t\tsmoother = gsap.core.globals().ScrollSmoother,\n\t\t\tsmootherInstance = smoother && smoother.get(),\n\t\t\tcontent = _fixIOSBug && ((vars.content && _getTarget(vars.content)) || (smootherInstance && vars.content !== false && !smootherInstance.smooth() && smootherInstance.content())),\n\t\t\tscrollFuncY = _getScrollFunc(target, _vertical),\n\t\t\tscrollFuncX = _getScrollFunc(target, _horizontal),\n\t\t\tscale = 1,\n\t\t\tinitialScale = (Observer.isTouch && _win.visualViewport ? _win.visualViewport.scale * _win.visualViewport.width : _win.outerWidth) / _win.innerWidth,\n\t\t\twheelRefresh = 0,\n\t\t\tresolveMomentumDuration = _isFunction(momentum) ? () => momentum(self) : () => momentum || 2.8,\n\t\t\tlastRefreshID, skipTouchMove,\n\t\t\tinputObserver = _inputObserver(target, vars.type, true, allowNestedScroll),\n\t\t\tresumeTouchMove = () => skipTouchMove = false,\n\t\t\tscrollClampX = _passThrough,\n\t\t\tscrollClampY = _passThrough,\n\t\t\tupdateClamps = () => {\n\t\t\t\tmaxY = _maxScroll(target, _vertical);\n\t\t\t\tscrollClampY = _clamp(_fixIOSBug ? 1 : 0, maxY);\n\t\t\t\tnormalizeScrollX && (scrollClampX = _clamp(0, _maxScroll(target, _horizontal)));\n\t\t\t\tlastRefreshID = _refreshID;\n\t\t\t},\n\t\t\tremoveContentOffset = () => {\n\t\t\t\tcontent._gsap.y = _round(parseFloat(content._gsap.y) + scrollFuncY.offset) + \"px\";\n\t\t\t\tcontent.style.transform = \"matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, \" + parseFloat(content._gsap.y) + \", 0, 1)\";\n\t\t\t\tscrollFuncY.offset = scrollFuncY.cacheID = 0;\n\t\t\t},\n\t\t\tignoreDrag = () => {\n\t\t\t\tif (skipTouchMove) {\n\t\t\t\t\trequestAnimationFrame(resumeTouchMove);\n\t\t\t\t\tlet offset = _round(self.deltaY / 2),\n\t\t\t\t\t\tscroll = scrollClampY(scrollFuncY.v - offset);\n\t\t\t\t\tif (content && scroll !== scrollFuncY.v + scrollFuncY.offset) {\n\t\t\t\t\t\tscrollFuncY.offset = scroll - scrollFuncY.v;\n\t\t\t\t\t\tlet y = _round((parseFloat(content && content._gsap.y) || 0) - scrollFuncY.offset);\n\t\t\t\t\t\tcontent.style.transform = \"matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, \" + y + \", 0, 1)\";\n\t\t\t\t\t\tcontent._gsap.y = y + \"px\";\n\t\t\t\t\t\tscrollFuncY.cacheID = _scrollers.cache;\n\t\t\t\t\t\t_updateAll();\n\t\t\t\t\t}\n\t\t\t\t\treturn true;\n\t\t\t\t}\n\t\t\t\tscrollFuncY.offset && removeContentOffset();\n\t\t\t\tskipTouchMove = true;\n\t\t\t},\n\t\t\ttween, startScrollX, startScrollY, onStopDelayedCall,\n\t\t\tonResize = () => { // if the window resizes, like on an iPhone which Apple FORCES the address bar to show/hide even if we event.preventDefault(), it may be scrolling too far now that the address bar is showing, so we must dynamically adjust the momentum tween.\n\t\t\t\tupdateClamps();\n\t\t\t\tif (tween.isActive() && tween.vars.scrollY > maxY) {\n\t\t\t\t\tscrollFuncY() > maxY ? tween.progress(1) && scrollFuncY(maxY) : tween.resetTo(\"scrollY\", maxY);\n\t\t\t\t}\n\t\t\t};\n\t\tcontent && gsap.set(content, {y: \"+=0\"}); // to ensure there's a cache (element._gsap)\n\t\tvars.ignoreCheck = e => (_fixIOSBug && e.type === \"touchmove\" && ignoreDrag(e)) || (scale > 1.05 && e.type !== \"touchstart\") || self.isGesturing || (e.touches && e.touches.length > 1);\n\t\tvars.onPress = () => {\n\t\t\tskipTouchMove = false;\n\t\t\tlet prevScale = scale;\n\t\t\tscale = _round(((_win.visualViewport && _win.visualViewport.scale) || 1) / initialScale);\n\t\t\ttween.pause();\n\t\t\tprevScale !== scale && _allowNativePanning(target, scale > 1.01 ? true : normalizeScrollX ? false : \"x\");\n\t\t\tstartScrollX = scrollFuncX();\n\t\t\tstartScrollY = scrollFuncY();\n\t\t\tupdateClamps();\n\t\t\tlastRefreshID = _refreshID;\n\t\t}\n\t\tvars.onRelease = vars.onGestureStart = (self, wasDragging) => {\n\t\t\tscrollFuncY.offset && removeContentOffset();\n\t\t\tif (!wasDragging) {\n\t\t\t\tonStopDelayedCall.restart(true);\n\t\t\t} else {\n\t\t\t\t_scrollers.cache++; // make sure we're pulling the non-cached value\n\t\t\t\t// alternate algorithm: durX = Math.min(6, Math.abs(self.velocityX / 800)),\tdur = Math.max(durX, Math.min(6, Math.abs(self.velocityY / 800))); dur = dur * (0.4 + (1 - _power4In(dur / 6)) * 0.6)) * (momentumSpeed || 1)\n\t\t\t\tlet dur = resolveMomentumDuration(),\n\t\t\t\t\tcurrentScroll, endScroll;\n\t\t\t\tif (normalizeScrollX) {\n\t\t\t\t\tcurrentScroll = scrollFuncX();\n\t\t\t\t\tendScroll = currentScroll + (dur * 0.05 * -self.velocityX) / 0.227; // the constant .227 is from power4(0.05). velocity is inverted because scrolling goes in the opposite direction.\n\t\t\t\t\tdur *= _clampScrollAndGetDurationMultiplier(scrollFuncX, currentScroll, endScroll, _maxScroll(target, _horizontal));\n\t\t\t\t\ttween.vars.scrollX = scrollClampX(endScroll);\n\t\t\t\t}\n\t\t\t\tcurrentScroll = scrollFuncY();\n\t\t\t\tendScroll = currentScroll + (dur * 0.05 * -self.velocityY) / 0.227; // the constant .227 is from power4(0.05)\n\t\t\t\tdur *= _clampScrollAndGetDurationMultiplier(scrollFuncY, currentScroll, endScroll, _maxScroll(target, _vertical));\n\t\t\t\ttween.vars.scrollY = scrollClampY(endScroll);\n\t\t\t\ttween.invalidate().duration(dur).play(0.01);\n\t\t\t\tif (_fixIOSBug && tween.vars.scrollY >= maxY || currentScroll >= maxY-1) { // iOS bug: it'll show the address bar but NOT fire the window \"resize\" event until the animation is done but we must protect against overshoot so we leverage an onUpdate to do so.\n\t\t\t\t\tgsap.to({}, {onUpdate: onResize, duration: dur});\n\t\t\t\t}\n\t\t\t}\n\t\t\tonRelease && onRelease(self);\n\t\t};\n\t\tvars.onWheel = () => {\n\t\t\ttween._ts && tween.pause();\n\t\t\tif (_getTime() - wheelRefresh > 1000) { // after 1 second, refresh the clamps otherwise that'll only happen when ScrollTrigger.refresh() is called or for touch-scrolling.\n\t\t\t\tlastRefreshID = 0;\n\t\t\t\twheelRefresh = _getTime();\n\t\t\t}\n\t\t};\n\t\tvars.onChange = (self, dx, dy, xArray, yArray) => {\n\t\t\t_refreshID !== lastRefreshID && updateClamps();\n\t\t\tdx && normalizeScrollX && scrollFuncX(scrollClampX(xArray[2] === dx ? startScrollX + (self.startX - self.x) : scrollFuncX() + dx - xArray[1])); // for more precision, we track pointer/touch movement from the start, otherwise it'll drift.\n\t\t\tif (dy) {\n\t\t\t\tscrollFuncY.offset && removeContentOffset();\n\t\t\t\tlet isTouch = yArray[2] === dy,\n\t\t\t\t\ty = isTouch ? startScrollY + self.startY - self.y : scrollFuncY() + dy - yArray[1],\n\t\t\t\t\tyClamped = scrollClampY(y);\n\t\t\t\tisTouch && y !== yClamped && (startScrollY += yClamped - y);\n\t\t\t\tscrollFuncY(yClamped);\n\t\t\t}\n\t\t\t(dy || dx) && _updateAll();\n\t\t};\n\t\tvars.onEnable = () => {\n\t\t\t_allowNativePanning(target, normalizeScrollX ? false : \"x\");\n\t\t\tScrollTrigger.addEventListener(\"refresh\", onResize);\n\t\t\t_addListener(_win, \"resize\", onResize);\n\t\t\tif (scrollFuncY.smooth) {\n\t\t\t\tscrollFuncY.target.style.scrollBehavior = \"auto\";\n\t\t\t\tscrollFuncY.smooth = scrollFuncX.smooth = false;\n\t\t\t}\n\t\t\tinputObserver.enable();\n\t\t};\n\t\tvars.onDisable = () => {\n\t\t\t_allowNativePanning(target, true);\n\t\t\t_removeListener(_win, \"resize\", onResize);\n\t\t\tScrollTrigger.removeEventListener(\"refresh\", onResize);\n\t\t\tinputObserver.kill();\n\t\t};\n\t\tvars.lockAxis = vars.lockAxis !== false;\n\t\tself = new Observer(vars);\n\t\tself.iOS = _fixIOSBug; // used in the Observer getCachedScroll() function to work around an iOS bug that wreaks havoc with TouchEvent.clientY if we allow scroll to go all the way back to 0.\n\t\t_fixIOSBug && !scrollFuncY() && scrollFuncY(1); // iOS bug causes event.clientY values to freak out (wildly inaccurate) if the scroll position is exactly 0.\n\t\t_fixIOSBug && gsap.ticker.add(_passThrough); // prevent the ticker from sleeping\n\t\tonStopDelayedCall = self._dc;\n\t\ttween = gsap.to(self, {ease: \"power4\", paused: true, inherit: false, scrollX: normalizeScrollX ? \"+=0.1\" : \"+=0\", scrollY: \"+=0.1\", modifiers: {scrollY: _interruptionTracker(scrollFuncY, scrollFuncY(), () => tween.pause())\t}, onUpdate: _updateAll, onComplete: onStopDelayedCall.vars.onComplete}); // we need the modifier to sense if the scroll position is altered outside of the momentum tween (like with a scrollTo tween) so we can pause() it to prevent conflicts.\n\t\treturn self;\n\t};\n\nScrollTrigger.sort = func => {\n\tif (_isFunction(func)) {\n\t\treturn _triggers.sort(func);\n\t}\n\tlet scroll = _win.pageYOffset || 0;\n\tScrollTrigger.getAll().forEach(t => t._sortY = t.trigger ? scroll + t.trigger.getBoundingClientRect().top : t.start + _win.innerHeight);\n\treturn _triggers.sort(func || ((a, b) => (a.vars.refreshPriority || 0) * -1e6 + (a.vars.containerAnimation ? 1e6 : a._sortY) - ((b.vars.containerAnimation ? 1e6 : b._sortY) + (b.vars.refreshPriority || 0) * -1e6))); // anything with a containerAnimation should refresh last.\n}\nScrollTrigger.observe = vars => new Observer(vars);\nScrollTrigger.normalizeScroll = vars => {\n\tif (typeof(vars) === \"undefined\") {\n\t\treturn _normalizer;\n\t}\n\tif (vars === true && _normalizer) {\n\t\treturn _normalizer.enable();\n\t}\n\tif (vars === false) {\n\t\t_normalizer && _normalizer.kill();\n\t\t_normalizer = vars;\n\t\treturn;\n\t}\n\tlet normalizer = vars instanceof Observer ? vars : _getScrollNormalizer(vars);\n\t_normalizer && _normalizer.target === normalizer.target && _normalizer.kill();\n\t_isViewport(normalizer.target) && (_normalizer = normalizer);\n\treturn normalizer;\n};\n\n\nScrollTrigger.core = { // smaller file size way to leverage in ScrollSmoother and Observer\n\t_getVelocityProp,\n\t_inputObserver,\n\t_scrollers,\n\t_proxies,\n\tbridge: {\n\t\t// when normalizeScroll sets the scroll position (ss = setScroll)\n\t\tss: () => {\n\t\t\t_lastScrollTime || _dispatch(\"scrollStart\");\n\t\t\t_lastScrollTime = _getTime();\n\t\t},\n\t\t// a way to get the _refreshing value in Observer\n\t\tref: () => _refreshing\n\t}\n};\n\n_getGSAP() && gsap.registerPlugin(ScrollTrigger);\n\nexport { ScrollTrigger as default };"],"names":["_getGSAP","gsap","window","registerPlugin","_getProxyProp","element","property","_proxies","indexOf","_isViewport","el","_root","_addListener","type","func","passive","capture","addEventListener","_removeListener","removeEventListener","_onScroll","_normalizer","isPressed","_scrollers","cache","_scrollCacheFunc","f","doNotCache","cachingFunc","value","_startup","_win","history","scrollRestoration","isNormalizing","v","Math","round","iOS","cacheID","_bridge","offset","_getTarget","t","self","_ctx","selector","utils","toArray","config","nullTargetWarn","console","warn","_getScrollFunc","s","sc","_doc","scrollingElement","_docEl","i","_vertical","push","prev","arguments","length","target","smooth","getProperty","_getVelocityProp","minTimeRefresh","useDelta","update","force","_getTime","min","t1","v2","v1","t2","dropToZeroTime","max","reset","getVelocity","latestValue","tOld","vOld","_getEvent","e","preventDefault","_gsapAllow","changedTouches","_getAbsoluteMax","a","abs","_setScrollTrigger","ScrollTrigger","core","globals","_integrate","data","bridge","scrollers","proxies","name","_initCore","_coreInitted","document","body","documentElement","_body","clamp","_context","context","_pointerType","_isTouch","Observer","isTouch","matchMedia","matches","navigator","maxTouchPoints","msMaxTouchPoints","_eventTypes","eventTypes","split","setTimeout","_observers","Date","now","_scrollLeft","_scrollTop","_horizontal","p","p2","os","os2","d","d2","scrollTo","pageXOffset","op","pageYOffset","init","vars","tolerance","dragMinimum","lineHeight","debounce","onStop","onStopDelay","ignore","wheelSpeed","event","onDragStart","onDragEnd","onDrag","onPress","onRelease","onRight","onLeft","onUp","onDown","onChangeX","onChangeY","onChange","onToggleX","onToggleY","onHover","onHoverEnd","onMove","ignoreCheck","isNormalizer","onGestureStart","onGestureEnd","onWheel","onEnable","onDisable","onClick","scrollSpeed","allowClicks","lockAxis","onLockAxis","clickCapture","onClickTime","_ignoreCheck","isPointerOrTouch","limitToTouch","pointerType","dx","deltaX","dy","deltaY","changedX","changedY","prevDeltaX","prevDeltaY","moved","dragged","locked","wheeled","id","onDelta","x","y","index","_vx","_vy","requestAnimationFrame","onTouchOrPointerDelta","axis","_onDrag","clientX","clientY","isDragging","startX","startY","_onGestureStart","touches","isGesturing","_onGestureEnd","onScroll","scrollFuncX","scrollFuncY","scrollX","scrollY","onStopDelayedCall","restart","_onWheel","multiplier","deltaMode","innerHeight","_onMove","_onHover","_onHoverEnd","_onClick","parseFloat","getComputedStyle","this","isViewport","ownerDoc","ownerDocument","_onPress","button","pause","_onRelease","isTrackingDrag","isNaN","wasDragging","isDragNotClick","eventData","delayedCall","defaultPrevented","click","createEvent","syntheticEvent","initMouseEvent","screenX","screenY","dispatchEvent","_dc","onStopFunc","enable","isEnabled","disable","filter","o","kill","revert","splice","version","create","register","getAll","slice","getById","_parseClamp","_isString","substr","_keepClamp","_pointerDownHandler","_pointerIsDown","_pointerUpHandler","_passThrough","_round","_windowExists","_getViewportDimension","dimensionProperty","_100vh","_getBoundsFunc","_winOffsets","width","innerWidth","height","_getBounds","_maxScroll","_iterateAutoRefresh","events","_autoRefresh","_isFunction","_isNumber","_isObject","_endAnimation","animation","reversed","progress","_callback","enabled","result","add","totalTime","callbackAnimation","_getComputedStyle","_setDefaults","obj","defaults","_getSize","_getLabelRatioArray","timeline","labels","duration","_snapDirectional","snapIncrementOrArray","snap","Array","isArray","sort","b","direction","threshold","snapped","_multiListener","types","callback","forEach","nonPassive","_wheelListener","scrollFunc","wheelHandler","_offsetToPx","size","eqIndex","relative","charAt","_keywords","_createMarker","container","matchWidthEl","containerAnimation","startColor","endColor","fontSize","indent","fontWeight","createElement","useFixedPosition","isScroller","parent","isStart","color","css","_right","_bottom","offsetWidth","_isStart","setAttribute","style","cssText","innerText","children","insertBefore","appendChild","_offset","_positionMarker","_sync","_lastScrollTime","_rafID","_updateAll","clientWidth","_dispatch","_setBaseDimensions","_baseScreenWidth","_baseScreenHeight","_onResize","_refreshing","_ignoreResize","fullscreenElement","webkitFullscreenElement","_ignoreMobileResize","_resizeDelay","_softRefresh","_refreshAll","_revertRecorded","media","_savedStyles","query","getBBox","uncache","_revertAll","trigger","_i","_triggers","_isReverted","_clearScrollMemory","_refreshingAll","rec","_scrollRestoration","_refresh100vh","_div100vh","offsetHeight","removeChild","_hideAllMarkers","hide","_toArray","display","_swapPinIn","pin","spacer","cs","spacerState","_gsap","swappedIn","_propNamesToCopy","spacerStyle","pinStyle","position","flexBasis","overflow","boxSizing","_width","_px","_height","_padding","_margin","_setState","parentNode","_getState","l","_stateProps","state","_parsePosition","scrollerSize","scroll","marker","markerScroller","scrollerBounds","borderWidth","scrollerMax","clampZeroProp","p1","time","seek","mapRange","scrollTrigger","start","end","bounds","localOffset","globalOffset","offsets","left","top","removeProperty","m","_caScrollDist","_reparent","_stOrig","_prefixExp","test","getCache","_interruptionTracker","getValueFunc","initialValue","onInterrupt","last1","last2","current","_shiftMarker","set","_getTweenCreator","scroller","getTween","change1","change2","tween","onComplete","modifiers","getScroll","checkForInterruption","prop","inherit","ratio","onUpdate","call","to","_clamp","_time2","_syncInterval","_transformProp","_prevWidth","_prevHeight","_sort","_suppressOverwrites","_fixIOSBug","_clampingMax","_limitCallbacks","_queueRefreshID","_primary","_time1","_enabled","_abs","_Right","_Left","_Top","_Bottom","_Width","_Height","withoutTransforms","xPercent","yPercent","rotation","rotationX","rotationY","scale","skewX","skewY","getBoundingClientRect","_markerDefaults","_defaults","toggleActions","anticipatePin","center","bottom","right","flipped","side","oppositeSide","_isFlipped","_ids","_listeners","_emptyArray","map","_refreshID","skipRevert","isRefreshing","refreshInits","scrollBehavior","refresh","_subPinOffset","horizontal","original","adjustPinSpacing","_dir","endClamp","_endClamp","startClamp","_startClamp","setPositions","render","onRefresh","_lastScroll","_direction","isUpdating","recordVelocity","concat","_capsExp","replace","toLowerCase","tweenTo","pinCache","snapFunc","scroll1","scroll2","markerStart","markerEnd","markerStartTrigger","markerEndTrigger","markerVars","executingOnRefresh","change","pinOriginalState","pinActiveState","pinState","pinGetter","pinSetter","pinStart","pinChange","spacingStart","markerStartSetter","pinMoves","markerEndSetter","snap1","snap2","scrubTween","scrubSmooth","snapDurClamp","snapDelayedCall","prevScroll","prevAnimProgress","caMarkerSetter","customRevertReturn","nodeType","toggleClass","onToggle","scrub","pinSpacing","invalidateOnRefresh","onScrubComplete","onSnapComplete","once","pinReparent","pinSpacer","fastScrollEnd","preventOverlaps","isToggle","scrollerCache","pinType","callbacks","onEnter","onLeave","onEnterBack","onLeaveBack","markers","onRefreshInit","getScrollerSize","_getSizeFunc","getScrollerOffsets","_getOffsetsFunc","lastSnap","lastRefresh","prevProgress","bind","refreshPriority","tweenScroll","scrubDuration","ease","totalProgress","paused","lazy","_initted","isReverted","immediateRender","snapTo","_getClosestLabel","_getLabelAtDirection","st","directional","delay","refreshedRecently","isActive","endValue","endScroll","velocity","naturalEnd","inertia","onStart","resetTo","_tTime","_tDur","stRevert","targets","className","nativeElement","spacerIsNative","classList","force3D","quickSetter","content","_makePositionable","oldOnUpdate","oldParams","onUpdateParams","eventCallback","apply","previous","next","temp","r","prevRefreshing","_swapPinOut","soft","pinOffset","invalidate","isVertical","override","curTrigger","curPin","oppositeScroll","initted","revertedPins","forcedOverflow","markerStartOffset","markerEndOffset","isFirstRefresh","otherPinOffset","parsedEnd","parsedEndTrigger","endTrigger","parsedStart","pinnedContainer","triggerIndex","unshift","_pinPush","normalize","_pinOffset","toUpperCase","ceil","_copyState","omitOffsets","endAnimation","labelToScroll","label","getTrailing","reverse","forceFake","toggleState","action","stateChanged","toggled","isAtMax","isTakingAction","clipped","_dp","_time","_start","n","newStart","newEnd","keepClamp","amount","allowAnimation","onKill","updateFunc","_queueRefreshAll","clearInterval","suppressOverwrites","_rafBugFix","userAgent","mm","bodyHasStyle","hasAttribute","bodyStyle","border","borderTopStyle","AnimationProto","Animation","prototype","Object","defineProperty","removeAttribute","setInterval","checkPrefix","w","h","hidden","limitCallbacks","ms","syncInterval","ignoreMobileResize","autoRefreshEvents","scrollerProxy","clearMatchMedia","isInViewport","positionInViewport","referencePoint","killAll","allowListeners","listeners","saveStyles","getAttribute","safe","clearScrollMemory","maxScroll","getScrollFunc","isScrolling","snapDirectional","batch","proxyCallback","elements","triggers","interval","batchMax","varsCopy","_clampScrollAndGetDurationMultiplier","_allowNativePanning","touchAction","_nestedScroll","node","_isScrollT","scrollHeight","clientHeight","scrollWidth","_overflow","overflowY","overflowX","_isScroll","stopPropagation","_inputObserver","inputs","nested","_captureInputs","_getScrollNormalizer","resumeTouchMove","skipTouchMove","updateClamps","maxY","scrollClampY","normalizeScrollX","scrollClampX","lastRefreshID","removeContentOffset","transform","onResize","startScrollX","startScrollY","momentum","allowNestedScroll","smoother","ScrollSmoother","smootherInstance","get","initialScale","visualViewport","outerWidth","wheelRefresh","resolveMomentumDuration","inputObserver","ignoreDrag","prevScale","currentScroll","dur","velocityX","velocityY","play","_ts","xArray","yArray","yClamped","ticker","_inputIsFocused","auto","_inputExp","isInput","tagName","_sortY","observe","normalizeScroll","normalizer","ss","ref"],"mappings":";;;;;;;;;mYAYY,SAAXA,WAAiBC,IAA4B,oBAAZC,SAA4BD,GAAOC,OAAOD,OAASA,GAAKE,gBAAkBF,GAkB3F,SAAhBG,EAAiBC,EAASC,UAAcC,GAASC,QAAQH,IAAYE,GAASA,GAASC,QAAQH,GAAW,GAAGC,GAC/F,SAAdG,EAAcC,YAASC,EAAMH,QAAQE,GACtB,SAAfE,EAAgBP,EAASQ,EAAMC,EAAMC,EAASC,UAAYX,EAAQY,iBAAiBJ,EAAMC,EAAM,CAACC,SAAqB,IAAZA,EAAmBC,UAAWA,IACrH,SAAlBE,EAAmBb,EAASQ,EAAMC,EAAME,UAAYX,EAAQc,oBAAoBN,EAAMC,IAAQE,GAGlF,SAAZI,WAAmBC,IAAeA,GAAYC,WAAcC,GAAWC,QACpD,SAAnBC,EAAoBC,EAAGC,GACJ,SAAdC,GAAcC,MACbA,GAAmB,IAAVA,EAAa,CACzBC,IAAaC,GAAKC,QAAQC,kBAAoB,cAC1CC,EAAgBb,IAAeA,GAAYC,UAC/CO,EAAQD,GAAYO,EAAIC,KAAKC,MAAMR,KAAWR,IAAeA,GAAYiB,IAAM,EAAI,GACnFZ,EAAEG,GACFD,GAAYW,QAAUhB,GAAWC,MACjCU,GAAiBM,EAAQ,KAAMX,QACrBF,GAAcJ,GAAWC,QAAUI,GAAYW,SAAWC,EAAQ,UAC5EZ,GAAYW,QAAUhB,GAAWC,MACjCI,GAAYO,EAAIT,YAEVE,GAAYO,EAAIP,GAAYa,cAEpCb,GAAYa,OAAS,EACdf,GAAKE,GAIA,SAAbc,EAAcC,EAAGC,UAAWA,GAAQA,EAAKC,MAAQD,EAAKC,KAAKC,UAAa7C,GAAK8C,MAAMC,SAASL,GAAG,KAAqB,iBAAPA,IAAoD,IAAjC1C,GAAKgD,SAASC,eAA2BC,QAAQC,KAAK,qBAAsBT,GAAK,MAEhM,SAAjBU,EAAkBhD,SAAUiD,IAAAA,EAAGC,IAAAA,GAC9B9C,EAAYJ,KAAaA,EAAUmD,GAAKC,kBAAoBC,QACxDC,EAAIpC,GAAWf,QAAQH,GAC1BoC,EAASc,IAAOK,GAAUL,GAAK,EAAI,GAClCI,IAAMA,EAAIpC,GAAWsC,KAAKxD,GAAW,GACvCkB,GAAWoC,EAAIlB,IAAW7B,EAAaP,EAAS,SAAUe,OACtD0C,EAAOvC,GAAWoC,EAAIlB,GACzB3B,EAAOgD,IAASvC,GAAWoC,EAAIlB,GAAUhB,EAAiBrB,EAAcC,EAASiD,IAAI,KAAU7C,EAAYJ,GAAWkD,EAAK9B,EAAiB,SAASI,UAAgBkC,UAAUC,OAAU3D,EAAQiD,GAAKzB,EAASxB,EAAQiD,cACxNxC,EAAKmD,OAAS5D,EACdyD,IAAShD,EAAKoD,OAAyD,WAAhDjE,GAAKkE,YAAY9D,EAAS,mBAC1CS,EAEW,SAAnBsD,EAAoBvC,EAAOwC,EAAgBC,GAOhC,SAATC,GAAU1C,EAAO2C,OACZ7B,EAAI8B,KACJD,GAAkBE,EAAT/B,EAAIgC,GAChBC,EAAKC,EACLA,EAAKhD,EACLiD,EAAKH,EACLA,EAAKhC,GACK2B,EACVO,GAAMhD,EAENgD,EAAKD,GAAM/C,EAAQ+C,IAAOjC,EAAImC,IAAOH,EAAKG,OAhBzCD,EAAKhD,EACR+C,EAAK/C,EACL8C,EAAKF,KACLK,EAAKH,EACLD,EAAML,GAAkB,GACxBU,EAAiB3C,KAAK4C,IAAI,IAAW,EAANN,SAsBzB,CAACH,OAAAA,GAAQU,MARP,SAARA,QAAgBL,EAAKC,EAAKP,EAAW,EAAIO,EAAIC,EAAKH,EAAK,GAQjCO,YAPR,SAAdA,YAAcC,OACTC,EAAON,EACVO,EAAOT,EACPjC,EAAI8B,YACJU,GAA+B,IAAhBA,GAAsBA,IAAgBN,GAAMN,GAAOY,GAC3DR,IAAOG,GAAeC,EAATpC,EAAImC,EAAuB,GAAKD,GAAMP,EAAWe,GAAQA,MAAWf,EAAW3B,EAAIgC,GAAMS,GAAQ,MAI7G,SAAZE,EAAaC,EAAGC,UACfA,IAAmBD,EAAEE,YAAcF,EAAEC,iBAC9BD,EAAEG,eAAiBH,EAAEG,eAAe,GAAKH,EAE/B,SAAlBI,EAAkBC,OACbZ,EAAM5C,KAAK4C,UAAL5C,KAAYwD,GACrBlB,EAAMtC,KAAKsC,UAALtC,KAAYwD,UACZxD,KAAKyD,IAAIb,IAAQ5C,KAAKyD,IAAInB,GAAOM,EAAMN,EAE3B,SAApBoB,KACCC,GAAgB9F,GAAK+F,KAAKC,UAAUF,gBACnBA,GAAcC,MA7FnB,SAAbE,iBACKF,EAAOD,GAAcC,KACxBG,EAAOH,EAAKI,QAAU,GACtBC,EAAYL,EAAKzE,WACjB+E,EAAUN,EAAKzF,SAChB8F,EAAUxC,WAAVwC,EAAkB9E,IAClB+E,EAAQzC,WAARyC,EAAgB/F,IAChBgB,GAAa8E,EACb9F,GAAW+F,EACX9D,EAAU,iBAAC+D,EAAM1E,UAAUsE,EAAKI,GAAM1E,IAoFCqE,GAE5B,SAAZM,EAAYR,UACX/F,GAAO+F,GAAQhG,KACVyG,IAAgBxG,IAA6B,oBAAdyG,UAA6BA,SAASC,OACzE5E,GAAO7B,OAEPwD,IADAF,GAAOkD,UACOE,gBACdC,GAAQrD,GAAKmD,KACbhG,EAAQ,CAACoB,GAAMyB,GAAME,GAAQmD,IACpB5G,GAAK8C,MAAM+D,MACpBC,GAAW9G,GAAK+F,KAAKgB,SAAW,aAChCC,GAAe,mBAAoBJ,GAAQ,UAAY,QAEvDK,GAAWC,EAASC,QAAUrF,GAAKsF,YAActF,GAAKsF,WAAW,oCAAoCC,QAAU,EAAK,iBAAkBvF,IAAmC,EAA3BwF,UAAUC,gBAAmD,EAA7BD,UAAUE,iBAAwB,EAAI,EACpNC,GAAcP,EAASQ,YAAc,iBAAkBjE,GAAS,4CAAgD,kBAAmBA,GAAkD,kDAAxC,uCAA2FkE,MAAM,KAC9OC,WAAW,kBAAM/F,EAAW,GAAG,KAC/BgE,IACAW,GAAe,GAETA,GAzHT,IAAIxG,GAAMwG,GAAsB1E,GAAMyB,GAAME,GAAQmD,GAAOK,GAAUD,GAAclB,GAAepF,EAAOU,GAAaqG,GAAaX,GAElIjF,EAAW,EACXgG,GAAa,GACbvG,GAAa,GACbhB,GAAW,GACXkE,GAAWsD,KAAKC,IAChBxF,EAAU,iBAAC+D,EAAM1E,UAAUA,GAgB3BoG,EAAc,aACdC,EAAa,YAoBbC,GAAc,CAAC7E,EAAG2E,EAAaG,EAAG,OAAQC,GAAI,OAAQC,GAAI,QAASC,IAAK,QAASC,EAAG,QAASC,GAAI,QAAS7C,EAAG,IAAKrC,GAAI9B,EAAiB,SAASI,UAAgBkC,UAAUC,OAASjC,GAAK2G,SAAS7G,EAAO+B,GAAUL,MAAQxB,GAAK4G,aAAenF,GAAKyE,IAAgBvE,GAAOuE,IAAgBpB,GAAMoB,IAAgB,KAChTrE,GAAY,CAACN,EAAG4E,EAAYE,EAAG,MAAOC,GAAI,MAAOC,GAAI,SAAUC,IAAK,SAAUC,EAAG,SAAUC,GAAI,SAAU7C,EAAG,IAAKgD,GAAIT,GAAa5E,GAAI9B,EAAiB,SAASI,UAAgBkC,UAAUC,OAASjC,GAAK2G,SAASP,GAAY5E,KAAM1B,GAASE,GAAK8G,aAAerF,GAAK0E,IAAexE,GAAOwE,IAAerB,GAAMqB,IAAe,KA+EhUC,GAAYS,GAAKhF,GACjBrC,GAAWC,MAAQ,MAEN2F,sBAKZ2B,KAAA,cAAKC,GACJtC,IAAgBD,EAAUvG,KAASkD,QAAQC,KAAK,wCAChD2C,IAAiBD,QACZkD,EAA6bD,EAA7bC,UAAWC,EAAkbF,EAAlbE,YAAapI,EAAqakI,EAAralI,KAAMoD,EAA+Z8E,EAA/Z9E,OAAQiF,EAAuZH,EAAvZG,WAAYC,EAA2YJ,EAA3YI,SAAU3D,EAAiYuD,EAAjYvD,eAAgB4D,EAAiXL,EAAjXK,OAAQC,EAAyWN,EAAzWM,YAAaC,EAA4VP,EAA5VO,OAAQC,EAAoVR,EAApVQ,WAAYC,EAAwUT,EAAxUS,MAAOC,EAAiUV,EAAjUU,YAAaC,EAAoTX,EAApTW,UAAWC,EAAySZ,EAAzSY,OAAQC,EAAiSb,EAAjSa,QAASC,EAAwRd,EAAxRc,UAAWC,EAA6Qf,EAA7Qe,QAASC,EAAoQhB,EAApQgB,OAAQC,EAA4PjB,EAA5PiB,KAAMC,EAAsPlB,EAAtPkB,OAAQC,EAA8OnB,EAA9OmB,UAAWC,EAAmOpB,EAAnOoB,UAAWC,EAAwNrB,EAAxNqB,SAAUC,EAA8MtB,EAA9MsB,UAAWC,EAAmMvB,EAAnMuB,UAAWC,EAAwLxB,EAAxLwB,QAASC,EAA+KzB,EAA/KyB,WAAYC,EAAmK1B,EAAnK0B,OAAQC,EAA2J3B,EAA3J2B,YAAaC,EAA8I5B,EAA9I4B,aAAcC,EAAgI7B,EAAhI6B,eAAgBC,EAAgH9B,EAAhH8B,aAAcC,EAAkG/B,EAAlG+B,QAASC,EAAyFhC,EAAzFgC,SAAUC,EAA+EjC,EAA/EiC,UAAWC,EAAoElC,EAApEkC,QAASC,EAA2DnC,EAA3DmC,YAAalK,EAA8C+H,EAA9C/H,QAASmK,EAAqCpC,EAArCoC,YAAaC,EAAwBrC,EAAxBqC,SAAUC,EAActC,EAAdsC,WA0Bpa,SAAfC,YAAqBC,GAAc9G,KACpB,SAAf+G,GAAgBjG,EAAGkG,UAAsB7I,GAAK4G,MAAQjE,IAAO+D,IAAWA,EAAO9I,QAAQ+E,EAAEtB,SAAawH,GAAoBC,IAAkC,UAAlBnG,EAAEoG,aAA6BjB,GAAeA,EAAYnF,EAAGkG,GAO9L,SAATlH,SACKqH,EAAKhJ,GAAKiJ,OAASlG,EAAgBkG,IACtCC,EAAKlJ,GAAKmJ,OAASpG,EAAgBoG,IACnCC,EAAW5J,KAAKyD,IAAI+F,IAAO5C,EAC3BiD,EAAW7J,KAAKyD,IAAIiG,IAAO9C,EAC5BoB,IAAa4B,GAAYC,IAAa7B,EAASxH,GAAMgJ,EAAIE,EAAID,GAAQE,IACjEC,IACHlC,GAAyB,EAAdlH,GAAKiJ,QAAc/B,EAAQlH,IACtCmH,GAAUnH,GAAKiJ,OAAS,GAAK9B,EAAOnH,IACpCsH,GAAaA,EAAUtH,IACvByH,GAAezH,GAAKiJ,OAAS,GAAQK,GAAa,GAAO7B,EAAUzH,IACnEsJ,GAAatJ,GAAKiJ,OAClBA,GAAO,GAAKA,GAAO,GAAKA,GAAO,GAAK,GAEjCI,IACHhC,GAAwB,EAAdrH,GAAKmJ,QAAc9B,EAAOrH,IACpCoH,GAAQpH,GAAKmJ,OAAS,GAAK/B,EAAKpH,IAChCuH,GAAaA,EAAUvH,IACvB0H,GAAe1H,GAAKmJ,OAAS,GAAQI,GAAa,GAAO7B,EAAU1H,IACnEuJ,GAAavJ,GAAKmJ,OAClBA,GAAO,GAAKA,GAAO,GAAKA,GAAO,GAAK,IAEjCK,IAASC,MACZ5B,GAAUA,EAAO7H,IACbyJ,KACH5C,GAA2B,IAAZ4C,IAAiB5C,EAAY7G,IAC5C+G,GAAUA,EAAO/G,IACjByJ,GAAU,GAEXD,IAAQ,GAETE,MAAYA,IAAS,IAAUjB,GAAcA,EAAWzI,IACpD2J,KACHzB,EAAQlI,IACR2J,IAAU,GAEXC,GAAK,EAEI,SAAVC,GAAWC,EAAGC,EAAGC,GAChBf,GAAOe,IAAUF,EACjBX,GAAOa,IAAUD,EACjB/J,GAAKiK,IAAItI,OAAOmI,GAChB9J,GAAKkK,IAAIvI,OAAOoI,GAChBxD,EAAkBqD,GAAPA,IAAYO,sBAAsBxI,IAAWA,KAEjC,SAAxByI,GAAyBN,EAAGC,GACvBvB,IAAa6B,KAChBrK,GAAKqK,KAAOA,GAAO7K,KAAKyD,IAAI6G,GAAKtK,KAAKyD,IAAI8G,GAAK,IAAM,IACrDL,IAAS,GAEG,MAATW,KACHpB,GAAO,IAAMa,EACb9J,GAAKiK,IAAItI,OAAOmI,GAAG,IAEP,MAATO,KACHlB,GAAO,IAAMY,EACb/J,GAAKkK,IAAIvI,OAAOoI,GAAG,IAEpBxD,EAAkBqD,GAAPA,IAAYO,sBAAsBxI,IAAWA,KAE/C,SAAV2I,GAAU3H,OACLiG,GAAajG,EAAG,QAEhBmH,GADJnH,EAAID,EAAUC,EAAGC,IACP2H,QACTR,EAAIpH,EAAE6H,QACNxB,EAAKc,EAAI9J,GAAK8J,EACdZ,EAAKa,EAAI/J,GAAK+J,EACdU,EAAazK,GAAKyK,WACnBzK,GAAK8J,EAAIA,EACT9J,GAAK+J,EAAIA,GACLU,IAAgBzB,GAAME,KAAQ1J,KAAKyD,IAAIjD,GAAK0K,OAASZ,IAAMzD,GAAe7G,KAAKyD,IAAIjD,GAAK2K,OAASZ,IAAM1D,MAC1GoD,GAAUgB,EAAa,EAAI,EAC3BA,IAAezK,GAAKyK,YAAa,GACjCL,GAAsBpB,EAAIE,KAiDV,SAAlB0B,GAAkBjI,UAAKA,EAAEkI,SAA8B,EAAnBlI,EAAEkI,QAAQzJ,SAAepB,GAAK8K,aAAc,IAAS9C,EAAerF,EAAG3C,GAAKyK,YAChG,SAAhBM,YAAuB/K,GAAK8K,aAAc,IAAU7C,EAAajI,IACtD,SAAXgL,GAAWrI,OACNiG,GAAajG,QACbmH,EAAImB,KACPlB,EAAImB,KACLrB,IAASC,EAAIqB,IAAW7C,GAAcyB,EAAIqB,IAAW9C,EAAa,GAClE6C,GAAUrB,EACVsB,GAAUrB,EACVvD,GAAU6E,GAAkBC,SAAQ,IAE1B,SAAXC,GAAW5I,OACNiG,GAAajG,IACjBA,EAAID,EAAUC,EAAGC,GACjBsF,IAAYyB,IAAU,OAClB6B,GAA8B,IAAhB7I,EAAE8I,UAAkBnF,EAA6B,IAAhB3D,EAAE8I,UAAkBtM,GAAKuM,YAAc,GAAK/E,EAC/FkD,GAAQlH,EAAEsG,OAASuC,EAAY7I,EAAEwG,OAASqC,EAAY,GACtDhF,IAAWuB,GAAgBsD,GAAkBC,SAAQ,IAE5C,SAAVK,GAAUhJ,OACLiG,GAAajG,QACbmH,EAAInH,EAAE4H,QACTR,EAAIpH,EAAE6H,QACNxB,EAAKc,EAAI9J,GAAK8J,EACdZ,EAAKa,EAAI/J,GAAK+J,EACf/J,GAAK8J,EAAIA,EACT9J,GAAK+J,EAAIA,EACTP,IAAQ,EACRhD,GAAU6E,GAAkBC,SAAQ,IACnCtC,GAAME,IAAOkB,GAAsBpB,EAAIE,IAE9B,SAAX0C,GAAWjJ,GAAM3C,GAAK4G,MAAQjE,EAAGgF,EAAQ3H,IAC3B,SAAd6L,GAAclJ,GAAM3C,GAAK4G,MAAQjE,EAAGiF,EAAW5H,IACpC,SAAX8L,GAAWnJ,UAAKiG,GAAajG,IAAOD,EAAUC,EAAGC,IAAmByF,EAAQrI,SA5LxEqB,OAASA,EAASvB,EAAWuB,IAAWP,QACxCqF,KAAOA,EACDO,EAAXA,GAAoBrJ,GAAK8C,MAAMC,QAAQsG,GACvCN,EAAYA,GAAa,KACzBC,EAAcA,GAAe,EAC7BM,EAAaA,GAAc,EAC3B2B,EAAcA,GAAe,EAC7BrK,EAAOA,GAAQ,sBACfsI,GAAwB,IAAbA,EACID,EAAfA,GAA4ByF,WAAW5M,GAAK6M,iBAAiB/H,IAAOqC,aAAe,OAC/EsD,GAAIyB,GAAmB5B,GAASD,GAAOG,GAASD,GAAQW,GAC3DrK,GAAOiM,KACP3C,GAAa,EACbC,GAAa,EACbpL,GAAUgI,EAAKhI,UAAayE,IAAmC,IAAjBuD,EAAKhI,QACnD8M,GAAcxK,EAAeY,EAAQkE,IACrC2F,GAAczK,EAAeY,EAAQL,IACrCmK,GAAUF,KACVG,GAAUF,KACVpC,IAAgB7K,EAAKL,QAAQ,YAAcK,EAAKL,QAAQ,YAAiC,gBAAnBkH,GAAY,GAClFoH,GAAarO,EAAYwD,GACzB8K,GAAW9K,EAAO+K,eAAiBxL,GACnCqI,GAAS,CAAC,EAAG,EAAG,GAChBE,GAAS,CAAC,EAAG,EAAG,GAChBR,GAAc,EAqFd0D,GAAWrM,GAAKgH,QAAU,SAAArE,GACrBiG,GAAajG,EAAG,IAAOA,GAAKA,EAAE2J,SAClCtM,GAAKqK,KAAOA,GAAO,KACnBgB,GAAkBkB,QAClBvM,GAAKtB,WAAY,EACjBiE,EAAID,EAAUC,GACd2G,GAAaC,GAAa,EAC1BvJ,GAAK0K,OAAS1K,GAAK8J,EAAInH,EAAE4H,QACzBvK,GAAK2K,OAAS3K,GAAK+J,EAAIpH,EAAE6H,QACzBxK,GAAKiK,IAAI5H,QACTrC,GAAKkK,IAAI7H,QACTrE,EAAa+J,EAAe1G,EAAS8K,GAAUrH,GAAY,GAAIwF,GAASnM,IAAS,GACjF6B,GAAKiJ,OAASjJ,GAAKmJ,OAAS,EAC5BnC,GAAWA,EAAQhH,MAEpBwM,GAAaxM,GAAKiH,UAAY,SAAAtE,OACzBiG,GAAajG,EAAG,IACpBrE,EAAgByJ,EAAe1G,EAAS8K,GAAUrH,GAAY,GAAIwF,IAAS,OACvEmC,GAAkBC,MAAM1M,GAAK+J,EAAI/J,GAAK2K,QACzCgC,EAAc3M,GAAKyK,WACnBmC,EAAiBD,IAAiD,EAAjCnN,KAAKyD,IAAIjD,GAAK8J,EAAI9J,GAAK0K,SAAgD,EAAjClL,KAAKyD,IAAIjD,GAAK+J,EAAI/J,GAAK2K,SAC9FkC,EAAYnK,EAAUC,IAClBiK,GAAkBH,IACtBzM,GAAKiK,IAAI5H,QACTrC,GAAKkK,IAAI7H,QAELO,GAAkB2F,GACrBlL,GAAKyP,YAAY,IAAM,cACS,IAA3BjL,KAAa8G,KAAsBhG,EAAEoK,oBACpCpK,EAAEtB,OAAO2L,MACZrK,EAAEtB,OAAO2L,aACH,GAAIb,GAASc,YAAa,KAC5BC,EAAiBf,GAASc,YAAY,eAC1CC,EAAeC,eAAe,SAAS,GAAM,EAAMhO,GAAM,EAAG0N,EAAUO,QAASP,EAAUQ,QAASR,EAAUtC,QAASsC,EAAUrC,SAAS,GAAO,GAAO,GAAO,EAAO,EAAG,MACvK7H,EAAEtB,OAAOiM,cAAcJ,OAM5BlN,GAAKyK,WAAazK,GAAK8K,YAAc9K,GAAKtB,WAAY,EACtD8H,GAAUmG,IAAgB5E,GAAgBsD,GAAkBC,SAAQ,GACpE7B,IAAW9H,KACXmF,GAAa6F,GAAe7F,EAAU9G,IACtCiH,GAAaA,EAAUjH,GAAM4M,KAqC/BvB,GAAoBrL,GAAKuN,IAAMlQ,GAAKyP,YAAYrG,GAAe,IAnKjD,SAAb+G,aACCxN,GAAKiK,IAAI5H,QACTrC,GAAKkK,IAAI7H,QACTgJ,GAAkBkB,QAClB/F,GAAUA,EAAOxG,MA+J8DuM,QAEjFvM,GAAKiJ,OAASjJ,GAAKmJ,OAAS,EAC5BnJ,GAAKiK,IAAMzI,EAAiB,EAAG,IAAI,GACnCxB,GAAKkK,IAAM1I,EAAiB,EAAG,IAAI,GACnCxB,GAAKmL,QAAUF,GACfjL,GAAKoL,QAAUF,GACflL,GAAKyK,WAAazK,GAAK8K,YAAc9K,GAAKtB,WAAY,EACtDyF,GAAS8H,MACTjM,GAAKyN,OAAS,SAAA9K,UACR3C,GAAK0N,YACT1P,EAAakO,GAAaC,GAAW9K,EAAQ,SAAU7C,GAC7B,GAA1BP,EAAKL,QAAQ,WAAkBI,EAAakO,GAAaC,GAAW9K,EAAQ,SAAU2J,GAAU7M,GAASC,GAChF,GAAzBH,EAAKL,QAAQ,UAAiBI,EAAaqD,EAAQ,QAASkK,GAAUpN,GAASC,IACjD,GAAzBH,EAAKL,QAAQ,UAAiB0G,IAAwC,GAA3BrG,EAAKL,QAAQ,cAC5DI,EAAaqD,EAAQyD,GAAY,GAAIuH,GAAUlO,GAASC,GACxDJ,EAAamO,GAAUrH,GAAY,GAAI0H,IACvCxO,EAAamO,GAAUrH,GAAY,GAAI0H,IACvCjE,GAAevK,EAAaqD,EAAQ,QAASqH,IAAc,GAAM,GACjEL,GAAWrK,EAAaqD,EAAQ,QAASyK,IACzC9D,GAAkBhK,EAAamO,GAAU,eAAgBvB,IACzD3C,GAAgBjK,EAAamO,GAAU,aAAcpB,IACrDpD,GAAW3J,EAAaqD,EAAQgD,GAAe,QAASuH,IACxDhE,GAAc5J,EAAaqD,EAAQgD,GAAe,QAASwH,IAC3DhE,GAAU7J,EAAaqD,EAAQgD,GAAe,OAAQsH,KAEvD3L,GAAK0N,WAAY,EACjB1N,GAAKyK,WAAazK,GAAK8K,YAAc9K,GAAKtB,UAAY8K,GAAQC,IAAU,EACxEzJ,GAAKiK,IAAI5H,QACTrC,GAAKkK,IAAI7H,QACT8I,GAAUF,KACVG,GAAUF,KACVvI,GAAKA,EAAE1E,MAAQoO,GAAS1J,GACxBwF,GAAYA,EAASnI,KAEfA,IAERA,GAAK2N,QAAU,WACV3N,GAAK0N,YAERxI,GAAW0I,OAAO,SAAAC,UAAKA,IAAM7N,IAAQnC,EAAYgQ,EAAExM,UAASD,QAAU9C,EAAgB4N,GAAaC,GAAW9K,EAAQ,SAAU7C,GAC5HwB,GAAKtB,YACRsB,GAAKiK,IAAI5H,QACTrC,GAAKkK,IAAI7H,QACT/D,EAAgByJ,EAAe1G,EAAS8K,GAAUrH,GAAY,GAAIwF,IAAS,IAE5EhM,EAAgB4N,GAAaC,GAAW9K,EAAQ,SAAU2J,GAAU5M,GACpEE,EAAgB+C,EAAQ,QAASkK,GAAUnN,GAC3CE,EAAgB+C,EAAQyD,GAAY,GAAIuH,GAAUjO,GAClDE,EAAgB6N,GAAUrH,GAAY,GAAI0H,IAC1ClO,EAAgB6N,GAAUrH,GAAY,GAAI0H,IAC1ClO,EAAgB+C,EAAQ,QAASqH,IAAc,GAC/CpK,EAAgB+C,EAAQ,QAASyK,IACjCxN,EAAgB6N,GAAU,eAAgBvB,IAC1CtM,EAAgB6N,GAAU,aAAcpB,IACxCzM,EAAgB+C,EAAQgD,GAAe,QAASuH,IAChDtN,EAAgB+C,EAAQgD,GAAe,QAASwH,IAChDvN,EAAgB+C,EAAQgD,GAAe,OAAQsH,IAC/C3L,GAAK0N,UAAY1N,GAAKtB,UAAYsB,GAAKyK,YAAa,EACpDrC,GAAaA,EAAUpI,MAIzBA,GAAK8N,KAAO9N,GAAK+N,OAAS,WACzB/N,GAAK2N,cACD5M,EAAImE,GAAWtH,QAAQoC,IACtB,GAALe,GAAUmE,GAAW8I,OAAOjN,EAAG,GAC/BtC,KAAgBuB,KAASvB,GAAc,IAGxCyG,GAAWjE,KAAKjB,IAChB+H,GAAgBlK,EAAYwD,KAAY5C,GAAcuB,IAEtDA,GAAKyN,OAAO7G,8JAILqF,KAAKhC,IAAI3H,2DAGT2J,KAAK/B,IAAI5H,8CAtRL6D,QACND,KAAKC,GA0RZ5B,EAAS0J,QAAU,SACnB1J,EAAS2J,OAAS,SAAA/H,UAAQ,IAAI5B,EAAS4B,IACvC5B,EAAS4J,SAAWvK,EACpBW,EAAS6J,OAAS,kBAAMlJ,GAAWmJ,SACnC9J,EAAS+J,QAAU,SAAA1E,UAAM1E,GAAW0I,OAAO,SAAAC,UAAKA,EAAE1H,KAAKyD,KAAOA,IAAI,IAElExM,KAAcC,GAAKE,eAAegH,GCxZnB,SAAdgK,GAAetP,EAAOhB,EAAM+B,OACvBkE,EAASsK,GAAUvP,KAAkC,WAAvBA,EAAMwP,OAAO,EAAG,KAA2C,EAAxBxP,EAAMrB,QAAQ,eACnFoC,EAAK,IAAM/B,EAAO,SAAWiG,GACdjF,EAAMwP,OAAO,EAAGxP,EAAMmC,OAAS,GAAKnC,EAEvC,SAAbyP,GAAczP,EAAOiF,UAAUA,GAAWsK,GAAUvP,IAAiC,WAAvBA,EAAMwP,OAAO,EAAG,GAA4CxP,EAAzB,SAAWA,EAAQ,IAE9F,SAAtB0P,YAA4BC,GAAiB,EACzB,SAApBC,YAA0BD,GAAiB,EAC5B,SAAfE,GAAevP,UAAKA,EACX,SAATwP,GAAS9P,UAASO,KAAKC,MAAc,IAARR,GAAkB,KAAU,EACzC,SAAhB+P,WAAyC,oBAAZ1R,OAClB,SAAXF,YAAiBC,IAAS2R,OAAoB3R,GAAOC,OAAOD,OAASA,GAAKE,gBAAkBF,GAC9E,SAAdQ,GAAc8E,YAAQ5E,EAAMH,QAAQ+E,GACZ,SAAxBsM,GAAwBC,UAA4C,WAAtBA,EAAiCC,EAAShQ,GAAK,QAAU+P,KAAuBpO,GAAO,SAAWoO,IAAsBjL,GAAM,SAAWiL,GACtK,SAAjBE,GAAiB3R,UAAWD,EAAcC,EAAS,2BAA6BI,GAAYJ,GAAW,kBAAO4R,GAAYC,MAAQnQ,GAAKoQ,WAAYF,GAAYG,OAASL,EAAeE,IAAgB,kBAAMI,GAAWhS,KAG3M,SAAbiS,GAAcjS,SAAUiD,IAAAA,EAAGmF,IAAAA,GAAID,IAAAA,EAAG5C,IAAAA,SAAOxD,KAAK4C,IAAI,GAAI1B,EAAI,SAAWmF,KAAQ7C,EAAIxF,EAAcC,EAASiD,IAAMsC,IAAMoM,GAAe3R,EAAf2R,GAA0BxJ,GAAK/H,GAAYJ,IAAYqD,GAAOJ,IAAMuD,GAAMvD,IAAMuO,GAAsBpJ,GAAMpI,EAAQiD,GAAKjD,EAAQ,SAAWoI,IAC1O,SAAtB8J,GAAuBzR,EAAM0R,OACvB,IAAI7O,EAAI,EAAGA,EAAI8O,EAAazO,OAAQL,GAAK,EAC3C6O,KAAWA,EAAOhS,QAAQiS,EAAa9O,EAAE,KAAQ7C,EAAK2R,EAAa9O,GAAI8O,EAAa9O,EAAE,GAAI8O,EAAa9O,EAAE,IAI/F,SAAd+O,GAAc7Q,SAA2B,mBAAXA,EAClB,SAAZ8Q,GAAY9Q,SAA2B,iBAAXA,EAChB,SAAZ+Q,GAAY/Q,SAA2B,iBAAXA,EACZ,SAAhBgR,GAAiBC,EAAWC,EAAU5D,UAAU2D,GAAaA,EAAUE,SAASD,EAAW,EAAI,IAAM5D,GAAS2D,EAAU3D,QAC5G,SAAZ8D,GAAarQ,EAAM9B,MACd8B,EAAKsQ,QAAS,KACbC,EAASvQ,EAAKC,KAAOD,EAAKC,KAAKuQ,IAAI,kBAAMtS,EAAK8B,KAAS9B,EAAK8B,GAChEuQ,GAAUA,EAAOE,YAAczQ,EAAK0Q,kBAAoBH,IAmBtC,SAApBI,GAAoBlT,UAAW0B,GAAK6M,iBAAiBvO,GAKtC,SAAfmT,GAAgBC,EAAKC,OACf,IAAItL,KAAKsL,EACZtL,KAAKqL,IAASA,EAAIrL,GAAKsL,EAAStL,WAE3BqL,EAQG,SAAXE,GAAYtT,SAAUoI,IAAAA,UAAQpI,EAAQ,SAAWoI,IAAOpI,EAAQ,SAAWoI,IAAO,EAC5D,SAAtBmL,GAAsBC,OAIpBzL,EAHGxC,EAAI,GACPkO,EAASD,EAASC,OAClBC,EAAWF,EAASE,eAEhB3L,KAAK0L,EACTlO,EAAE/B,KAAKiQ,EAAO1L,GAAK2L,UAEbnO,EAGW,SAAnBoO,GAAmBC,OACdC,EAAOjU,GAAK8C,MAAMmR,KAAKD,GAC1BrO,EAAIuO,MAAMC,QAAQH,IAAyBA,EAAqBhD,MAAM,GAAGoD,KAAK,SAACzO,EAAG0O,UAAM1O,EAAI0O,WACtF1O,EAAI,SAAC/D,EAAO0S,EAAWC,OACzB7Q,cADyB6Q,IAAAA,EAAW,OAEnCD,SACGL,EAAKrS,MAEG,EAAZ0S,EAAe,KAClB1S,GAAS2S,EACJ7Q,EAAI,EAAGA,EAAIiC,EAAE5B,OAAQL,OACrBiC,EAAEjC,IAAM9B,SACJ+D,EAAEjC,UAGJiC,EAAEjC,EAAE,OAEXA,EAAIiC,EAAE5B,OACNnC,GAAS2S,EACF7Q,QACFiC,EAAEjC,IAAM9B,SACJ+D,EAAEjC,UAILiC,EAAE,IACN,SAAC/D,EAAO0S,EAAWC,YAAAA,IAAAA,EAAW,UAC7BC,EAAUP,EAAKrS,UACX0S,GAAanS,KAAKyD,IAAI4O,EAAU5S,GAAS2S,GAAeC,EAAU5S,EAAQ,GAAO0S,EAAY,EAAKE,EAAUP,EAAKK,EAAY,EAAI1S,EAAQoS,EAAuBpS,EAAQoS,IAIjK,SAAjBS,GAAkB5T,EAAMT,EAASsU,EAAOC,UAAaD,EAAM/M,MAAM,KAAKiN,QAAQ,SAAAhU,UAAQC,EAAKT,EAASQ,EAAM+T,KAC3F,SAAfhU,GAAgBP,EAASQ,EAAMC,EAAMgU,EAAY9T,UAAYX,EAAQY,iBAAiBJ,EAAMC,EAAM,CAACC,SAAU+T,EAAY9T,UAAWA,IAClH,SAAlBE,GAAmBb,EAASQ,EAAMC,EAAME,UAAYX,EAAQc,oBAAoBN,EAAMC,IAAQE,GAC7E,SAAjB+T,GAAkBjU,EAAMJ,EAAIsU,IAC3BA,EAAaA,GAAcA,EAAWC,gBAErCnU,EAAKJ,EAAI,QAASsU,GAClBlU,EAAKJ,EAAI,YAAasU,IAMV,SAAdE,GAAerT,EAAOsT,MACjB/D,GAAUvP,GAAQ,KACjBuT,EAAUvT,EAAMrB,QAAQ,KAC3B6U,GAAYD,GAAYvT,EAAMyT,OAAOF,EAAQ,GAAK,GAAKzG,WAAW9M,EAAMwP,OAAO+D,EAAU,IAAM,GAC3FA,IACHvT,EAAMrB,QAAQ,KAAO4U,IAAaC,GAAYF,EAAO,KACtDtT,EAAQA,EAAMwP,OAAO,EAAG+D,EAAQ,IAEjCvT,EAAQwT,GAAaxT,KAAS0T,EAAaA,EAAU1T,GAASsT,GAAQtT,EAAMrB,QAAQ,KAAOmO,WAAW9M,GAASsT,EAAO,IAAMxG,WAAW9M,IAAU,UAE3IA,EAEQ,SAAhB2T,GAAiB3U,EAAM0F,EAAMkP,EAAWlB,IAAiE9R,EAAQiT,EAAcC,OAA3EC,IAAAA,WAAYC,IAAAA,SAAUC,IAAAA,SAAUC,IAAAA,OAAQC,IAAAA,WACvFzQ,EAAI/B,GAAKyS,cAAc,OAC1BC,EAAmBzV,GAAYgV,IAAsD,UAAxCrV,EAAcqV,EAAW,WACtEU,GAA2C,IAA9BtV,EAAKL,QAAQ,YAC1B4V,EAASF,EAAmBrP,GAAQ4O,EACpCY,GAAqC,IAA3BxV,EAAKL,QAAQ,SACvB8V,EAAQD,EAAUT,EAAaC,EAC/BU,EAAM,gBAAkBD,EAAQ,cAAgBR,EAAW,UAAYQ,EAAQ,gBAAkBN,EAAa,8IAC/GO,GAAO,cAAgBJ,GAAcR,IAAuBO,EAAmB,SAAW,cACzFC,IAAcR,GAAuBO,IAAsBK,IAAQhC,IAAc3Q,GAAY4S,EAASC,GAAW,KAAOhU,EAASkM,WAAWoH,IAAW,OACxJL,IAAiBa,GAAO,+CAAiDb,EAAagB,YAAc,OACpGnR,EAAEoR,SAAWN,EACb9Q,EAAEqR,aAAa,QAAS,eAAiB/V,GAAQ0F,EAAO,WAAaA,EAAO,KAC5EhB,EAAEsR,MAAMC,QAAUP,EAClBhR,EAAEwR,UAAYxQ,GAAiB,IAATA,EAAa1F,EAAO,IAAM0F,EAAO1F,EACvDuV,EAAOY,SAAS,GAAKZ,EAAOa,aAAa1R,EAAG6Q,EAAOY,SAAS,IAAMZ,EAAOc,YAAY3R,GACrFA,EAAE4R,QAAU5R,EAAE,SAAWgP,EAAU3L,GAAGH,IACtC2O,EAAgB7R,EAAG,EAAGgP,EAAW8B,GAC1B9Q,EAiBA,SAAR8R,YAA6C,GAA/B5S,KAAa6S,KAAoCC,EAAXA,GAAoBxK,sBAAsByK,IAClF,SAAZpW,KACMC,GAAgBA,EAAYC,aAAaD,EAAYiM,OAASzG,GAAM4Q,eACxElW,GAAWC,QACPH,EACQkW,EAAXA,GAAoBxK,sBAAsByK,GAE1CA,IAEDF,IAAmBI,EAAU,eAC7BJ,GAAkB7S,MAGC,SAArBkT,KACCC,EAAmB7V,GAAKoQ,WACxB0F,EAAoB9V,GAAKuM,YAEd,SAAZwJ,GAAatT,GACZjD,GAAWC,SACA,IAAVgD,IAAoBuT,IAAgBC,GAAkBxU,GAAKyU,mBAAsBzU,GAAK0U,yBAA6BC,GAAuBP,IAAqB7V,GAAKoQ,cAAc/P,KAAKyD,IAAI9D,GAAKuM,YAAcuJ,GAAwC,IAAnB9V,GAAKuM,eAAyB8J,EAAalK,SAAQ,GAIzQ,SAAfmK,YAAqBnX,GAAgB6E,GAAe,YAAasS,KAAiBC,IAAY,GAG5E,SAAlBC,GAAkBC,OACZ,IAAI7U,EAAI,EAAGA,EAAI8U,EAAazU,OAAQL,GAAG,IACtC6U,GAASC,EAAa9U,EAAE,IAAM8U,EAAa9U,EAAE,GAAG+U,QAAUF,KAC9DC,EAAa9U,GAAGkT,MAAMC,QAAU2B,EAAa9U,EAAE,GAC/C8U,EAAa9U,GAAGgV,SAAWF,EAAa9U,GAAGiT,aAAa,YAAa6B,EAAa9U,EAAE,IAAM,IAC1F8U,EAAa9U,EAAE,GAAGiV,QAAU,GAIlB,SAAbC,GAAcnI,EAAM8H,OACfM,MACCC,GAAK,EAAGA,GAAKC,GAAUhV,OAAQ+U,OACnCD,EAAUE,GAAUD,MACHP,GAASM,EAAQjW,OAAS2V,IACtC9H,EACHoI,EAAQpI,KAAK,GAEboI,EAAQnI,QAAO,GAAM,IAIxBsI,GAAc,EACdT,GAASD,GAAgBC,GACzBA,GAASd,EAAU,UAEC,SAArBwB,GAAsBjX,EAAmBuC,GACxCjD,GAAWC,SACVgD,GAAU2U,IAAmB5X,GAAWsT,QAAQ,SAAApB,UAAOf,GAAYe,IAAQA,EAAIlR,YAAckR,EAAI2F,IAAM,KACxGhI,GAAUnP,KAAuBF,GAAKC,QAAQC,kBAAoBoX,EAAqBpX,GAWxE,SAAhBqX,KACCzS,GAAMqQ,YAAYqC,GAClBxH,GAAW1Q,GAAekY,EAAUC,cAAiBzX,GAAKuM,YAC1DzH,GAAM4S,YAAYF,GAED,SAAlBG,GAAkBC,UAAQC,GAAS,gGAAgG/E,QAAQ,SAAAnU,UAAMA,EAAGmW,MAAMgD,QAAUF,EAAO,OAAS,UA8GvK,SAAbG,GAAcC,EAAKC,EAAQC,EAAIC,OACzBH,EAAII,MAAMC,UAAW,SAIxBhS,EAHGzE,EAAI0W,EAAiBrW,OACxBsW,EAAcN,EAAOnD,MACrB0D,EAAWR,EAAIlD,MAETlT,KAEN2W,EADAlS,EAAIiS,EAAiB1W,IACJsW,EAAG7R,GAErBkS,EAAYE,SAA2B,aAAhBP,EAAGO,SAA0B,WAAa,WACjD,WAAfP,EAAGJ,UAA0BS,EAAYT,QAAU,gBACpDU,EAAS9D,GAAW8D,EAAS/D,GAAU,OACvC8D,EAAYG,UAAYR,EAAGQ,WAAa,OACxCH,EAAYI,SAAW,UACvBJ,EAAYK,UAAY,aACxBL,EAAYM,IAAUjH,GAASoG,EAAK5R,IAAe0S,GACnDP,EAAYQ,IAAWnH,GAASoG,EAAKnW,IAAaiX,GAClDP,EAAYS,IAAYR,EAASS,IAAWT,EAAQ,IAASA,EAAQ,KAAU,IAC/EU,GAAUf,GACVK,EAASK,IAAUL,EAAQ,SAAmBN,EAAGW,IACjDL,EAASO,IAAWP,EAAQ,UAAoBN,EAAGa,IACnDP,EAASQ,IAAYd,EAAGc,IACpBhB,EAAImB,aAAelB,IACtBD,EAAImB,WAAWjE,aAAa+C,EAAQD,GACpCC,EAAO9C,YAAY6C,IAEpBA,EAAII,MAAMC,WAAY,GAsBZ,SAAZe,GAAY9a,WACP+a,EAAIC,GAAYrX,OACnB6S,EAAQxW,EAAQwW,MAChByE,EAAQ,GACR3X,EAAI,EACEA,EAAIyX,EAAGzX,IACb2X,EAAMzX,KAAKwX,GAAY1X,GAAIkT,EAAMwE,GAAY1X,YAE9C2X,EAAM3Y,EAAItC,EACHib,EAuBS,SAAjBC,GAAkB1Z,EAAOiX,EAAS0C,EAAcjH,EAAWkH,EAAQC,EAAQC,EAAgB/Y,EAAMgZ,EAAgBC,EAAa3F,EAAkB4F,EAAanG,EAAoBoG,GAChLrJ,GAAY7Q,KAAWA,EAAQA,EAAMe,IACjCwO,GAAUvP,IAAgC,QAAtBA,EAAMwP,OAAO,EAAE,KACtCxP,EAAQia,GAAmC,MAApBja,EAAMyT,OAAO,GAAaJ,GAAY,IAAMrT,EAAMwP,OAAO,GAAImK,GAAgB,QAGpGQ,EAAI3T,EAAIhI,EADL4b,EAAOtG,EAAqBA,EAAmBsG,OAAS,KAE5DtG,GAAsBA,EAAmBuG,KAAK,GAC9C5M,MAAMzN,KAAWA,GAASA,GACrB8Q,GAAU9Q,GAkBd8T,IAAuB9T,EAAQ5B,GAAK8C,MAAMoZ,SAASxG,EAAmByG,cAAcC,MAAO1G,EAAmByG,cAAcE,IAAK,EAAGR,EAAaja,IACjJ8Z,GAAkBvE,EAAgBuE,EAAgBH,EAAcjH,GAAW,OAnBrD,CACtB7B,GAAYoG,KAAaA,EAAUA,EAAQlW,QAE1C2Z,EAAQC,EAAaC,EAAc5C,EADhC6C,GAAW7a,GAAS,KAAK+F,MAAM,KAEnCvH,EAAUqC,EAAWoW,EAASlW,IAASiE,IACvC0V,EAASlK,GAAWhS,IAAY,MACdkc,EAAOI,MAASJ,EAAOK,MAAgD,SAAvCrJ,GAAkBlT,GAASwZ,UAC5EA,EAAUxZ,EAAQwW,MAAMgD,QACxBxZ,EAAQwW,MAAMgD,QAAU,QACxB0C,EAASlK,GAAWhS,GACpBwZ,EAAWxZ,EAAQwW,MAAMgD,QAAUA,EAAWxZ,EAAQwW,MAAMgG,eAAe,YAE5EL,EAActH,GAAYwH,EAAQ,GAAIH,EAAOhI,EAAU/L,IACvDiU,EAAevH,GAAYwH,EAAQ,IAAM,IAAKlB,GAC9C3Z,EAAQ0a,EAAOhI,EAAUnM,GAAKwT,EAAerH,EAAUnM,GAAKyT,EAAcW,EAAcf,EAASgB,EACjGd,GAAkBvE,EAAgBuE,EAAgBc,EAAclI,EAAYiH,EAAeiB,EAAe,IAAOd,EAAehF,UAA2B,GAAf8F,GAC5IjB,GAAgBA,EAAeiB,KAK5BV,IACHnZ,EAAKmZ,GAAiBla,IAAU,KAChCA,EAAQ,IAAMA,EAAQ,IAEnB6Z,EAAQ,KACPlB,EAAW3Y,EAAQ2Z,EACtBnF,EAAUqF,EAAO/E,SAClBqF,EAAK,SAAWzH,EAAU9L,GAC1B2O,EAAgBsE,EAAQlB,EAAUjG,EAAY8B,GAAsB,GAAXmE,IAAoBnE,IAAYH,EAAmB9T,KAAK4C,IAAI6B,GAAMmV,GAAKtY,GAAOsY,IAAON,EAAOR,WAAWc,KAAQxB,EAAW,GAC/KtE,IACH0F,EAAiBvJ,GAAWsJ,GAC5BzF,IAAqBwF,EAAO7E,MAAMtC,EAAU3L,GAAGR,GAAMwT,EAAerH,EAAU3L,GAAGR,GAAKmM,EAAU3L,GAAGkU,EAAIpB,EAAOvE,QAAW0D,YAGvHlF,GAAsBtV,IACzB2b,EAAK3J,GAAWhS,GAChBsV,EAAmBuG,KAAKJ,GACxBzT,EAAKgK,GAAWhS,GAChBsV,EAAmBoH,cAAgBf,EAAGzH,EAAUnM,GAAKC,EAAGkM,EAAUnM,GAClEvG,EAAQA,EAAS8T,EAAmBoH,cAAiBjB,GAEtDnG,GAAsBA,EAAmBuG,KAAKD,GACvCtG,EAAqB9T,EAAQO,KAAKC,MAAMR,GAGpC,SAAZmb,GAAa3c,EAAS+V,EAAQwG,EAAKD,MAC9Btc,EAAQ6a,aAAe9E,EAAQ,KAEjChO,EAAG6R,EADApD,EAAQxW,EAAQwW,SAEhBT,IAAWvP,GAAO,KAGhBuB,KAFL/H,EAAQ4c,QAAUpG,EAAMC,QACxBmD,EAAK1G,GAAkBlT,IAEhB+H,GAAM8U,GAAWC,KAAK/U,KAAM6R,EAAG7R,IAA0B,iBAAbyO,EAAMzO,IAAyB,MAANA,IAC1EyO,EAAMzO,GAAK6R,EAAG7R,IAGhByO,EAAM+F,IAAMA,EACZ/F,EAAM8F,KAAOA,OAEb9F,EAAMC,QAAUzW,EAAQ4c,QAEzBhd,GAAK+F,KAAKoX,SAAS/c,GAASuY,QAAU,EACtCxC,EAAOc,YAAY7W,IAGE,SAAvBgd,GAAwBC,EAAcC,EAAcC,OAC/CC,EAAQF,EACXG,EAAQD,SACF,SAAA5b,OACF8b,EAAUvb,KAAKC,MAAMib,YACrBK,IAAYF,GAASE,IAAYD,GAAqC,EAA5Btb,KAAKyD,IAAI8X,EAAUF,IAA0C,EAA5Brb,KAAKyD,IAAI8X,EAAUD,KACjG7b,EAAQ8b,EACRH,GAAeA,KAEhBE,EAAQD,EACRA,EAAQrb,KAAKC,MAAMR,IAIN,SAAf+b,GAAgBlC,EAAQnH,EAAW1S,OAC9BkH,EAAO,GACXA,EAAKwL,EAAUnM,GAAK,KAAOvG,EAC3B5B,GAAK4d,IAAInC,EAAQ3S,GAUC,SAAnB+U,GAAoBC,EAAUxJ,GAGjB,SAAXyJ,GAAYtV,EAAUK,EAAMwU,EAAcU,EAASC,OAC9CC,EAAQH,GAASG,MACpBC,EAAarV,EAAKqV,WAClBC,EAAY,GACbd,EAAeA,GAAgBe,QAC3BC,EAAuBlB,GAAqBiB,EAAWf,EAAc,WACxEY,EAAMzN,OACNsN,GAASG,MAAQ,WAElBD,EAAWD,GAAWC,GAAY,EAClCD,EAAUA,GAAYvV,EAAW6U,EACjCY,GAASA,EAAMzN,OACf3H,EAAKyV,GAAQ9V,EACbK,EAAK0V,SAAU,GACf1V,EAAKsV,UAAYA,GACPG,GAAQ,kBAAMD,EAAqBhB,EAAeU,EAAUE,EAAMO,MAAQR,EAAUC,EAAMO,MAAQP,EAAMO,QAClH3V,EAAK4V,SAAW,WACfpd,GAAWC,QACXwc,GAASG,OAAS3G,KAEnBzO,EAAKqV,WAAa,WACjBJ,GAASG,MAAQ,EACjBC,GAAcA,EAAWQ,KAAKT,IAE/BA,EAAQH,GAASG,MAAQle,GAAK4e,GAAGd,EAAUhV,OA1BzCuV,EAAYjb,EAAe0a,EAAUxJ,GACxCiK,EAAO,UAAYjK,EAAUlM,UA4B9B0V,EAASS,GAAQF,GACPrJ,aAAe,kBAAM+I,GAASG,OAASH,GAASG,MAAMzN,SAAWsN,GAASG,MAAQ,IAC5Fvd,GAAamd,EAAU,QAASO,EAAUrJ,cAC1ClP,GAAcqB,SAAWxG,GAAamd,EAAU,YAAaO,EAAUrJ,cAChE+I,GAjkBT,IAAI/d,GAAMwG,EAAc1E,GAAMyB,GAAME,GAAQmD,GAAOlG,EAAOyX,EAAcwB,GAAUkF,GAAQC,GAAQC,EAAejH,GAAavG,GAAgByN,EAAgBlG,GAAImG,EAAYC,EAAa1M,EAAc2M,GAAOC,GAAqBrH,EAAe3W,EAAa8W,EAAqBN,EAAmBD,EAAkB0H,EAAYvY,EAAUsS,EAAoBE,EAAWxH,EAAQkH,EAAasG,GACpYC,GAiLAjI,EAyDA4B,GAEAsG,GAwEAC,GAnTA5d,GAAW,EACX2C,GAAWsD,KAAKC,IAChB2X,EAASlb,KACT6S,GAAkB,EAClBsI,GAAW,EAyBXxO,GAAY,SAAZA,UAAYvP,SAA2B,iBAAXA,GAW5Bge,GAAOzd,KAAKyD,IAGZ2Q,EAAS,QACTC,EAAU,SACVmE,GAAS,QACTE,GAAU,SACVgF,GAAS,QACTC,GAAQ,OACRC,GAAO,MACPC,GAAU,SACVlF,GAAW,UACXC,GAAU,SACVkF,GAAS,QACTC,EAAU,SACVtF,GAAM,KAYNxI,GAAa,SAAbA,WAAchS,EAAS+f,OAClBjC,EAAQiC,GAAoE,6BAA/C7M,GAAkBlT,GAAS4e,IAAkDhf,GAAK4e,GAAGxe,EAAS,CAACqM,EAAG,EAAGC,EAAG,EAAG0T,SAAU,EAAGC,SAAU,EAAGC,SAAU,EAAGC,UAAW,EAAGC,UAAW,EAAGC,MAAO,EAAGC,MAAO,EAAGC,MAAO,IAAI5N,SAAS,GACtPuJ,EAASlc,EAAQwgB,+BAClB1C,GAASA,EAAMnL,SAAS,GAAGtC,OACpB6L,GAwDRuE,GAAkB,CAAClL,WAAY,QAASC,SAAU,MAAOE,OAAQ,EAAGD,SAAU,OAAQE,WAAW,UACjG+K,GAAY,CAACC,cAAe,OAAQC,cAAe,GACnD1L,EAAY,CAACqH,IAAK,EAAGD,KAAM,EAAGuE,OAAQ,GAAKC,OAAQ,EAAGC,MAAO,GAiC7DhK,EAAkB,SAAlBA,gBAAmBsE,EAAQW,EAAO9H,EAAW8M,OACxCtY,EAAO,CAAC8Q,QAAS,SACpByH,EAAO/M,EAAU8M,EAAU,MAAQ,MACnCE,EAAehN,EAAU8M,EAAU,KAAO,OAC3C3F,EAAO8F,WAAaH,EACpBtY,EAAKwL,EAAU3O,EAAI,WAAayb,GAAW,IAAM,EACjDtY,EAAKwL,EAAU3O,GAAKyb,EAAU,MAAQ,EACtCtY,EAAK,SAAWuY,EAAOpB,IAAU,EACjCnX,EAAK,SAAWwY,EAAerB,IAAU,EACzCnX,EAAKwL,EAAUnM,GAAKiU,EAAQ,KAC5Bpc,GAAK4d,IAAInC,EAAQ3S,IAElBiQ,GAAY,GACZyI,GAAO,GAuBPC,EAAa,GACbC,EAAc,GAEdjK,EAAY,SAAZA,UAAY7W,UAAS6gB,EAAW7gB,IAAS6gB,EAAW7gB,GAAM+gB,IAAI,SAAAlgB,UAAKA,OAASigB,GAC5ElJ,EAAe,GAgCfoJ,GAAa,EAcbvJ,GAAc,SAAdA,YAAe9T,EAAOsd,MACrBpe,GAASF,GAAKoD,gBACdC,GAAQrD,GAAKmD,KACbhG,EAAQ,CAACoB,GAAMyB,GAAME,GAAQmD,KACzByQ,IAAoB9S,GAAUyU,GAIlCK,KACAH,GAAiBpT,GAAcgc,cAAe,EAC9CxgB,GAAWsT,QAAQ,SAAApB,UAAOf,GAAYe,MAAUA,EAAIlR,UAAYkR,EAAI2F,IAAM3F,WACtEuO,EAAetK,EAAU,eAC7B0H,IAASrZ,GAAcsO,OACvByN,GAAcjJ,KACdtX,GAAWsT,QAAQ,SAAApB,GACdf,GAAYe,KACfA,EAAIvP,SAAWuP,EAAIxP,OAAO4S,MAAMoL,eAAiB,QACjDxO,EAAI,MAGNuF,GAAU/H,MAAM,GAAG4D,QAAQ,SAAAlS,UAAKA,EAAEuf,YAClCjJ,GAAc,EACdD,GAAUnE,QAAQ,SAAClS,MACdA,EAAEwf,eAAiBxf,EAAEoX,IAAK,KACzByE,EAAO7b,EAAEoG,KAAKqZ,WAAa,cAAgB,eAC9CC,EAAW1f,EAAEoX,IAAIyE,GAClB7b,EAAEgO,QAAO,EAAM,GACfhO,EAAE2f,iBAAiB3f,EAAEoX,IAAIyE,GAAQ6D,GACjC1f,EAAEuf,aAGJ3C,GAAe,EACf7F,IAAgB,GAChBV,GAAUnE,QAAQ,SAAAlS,OACbqC,EAAMsN,GAAW3P,EAAEob,SAAUpb,EAAE4f,MAClCC,EAA0B,QAAf7f,EAAEoG,KAAKuT,KAAkB3Z,EAAE8f,WAAa9f,EAAE2Z,IAAMtX,EAC3D0d,EAAa/f,EAAEggB,aAAehgB,EAAE0Z,OAASrX,GACzCwd,GAAYE,IAAe/f,EAAEigB,aAAaF,EAAa1d,EAAM,EAAIrC,EAAE0Z,MAAOmG,EAAWpgB,KAAK4C,IAAI0d,EAAa1d,EAAMrC,EAAE0Z,MAAQ,EAAGrX,GAAOrC,EAAE2Z,KAAK,KAE9I5C,IAAgB,GAChB6F,GAAe,EACfyC,EAAanN,QAAQ,SAAA1B,UAAUA,GAAUA,EAAO0P,QAAU1P,EAAO0P,QAAQ,KACzEthB,GAAWsT,QAAQ,SAAApB,GACdf,GAAYe,KACfA,EAAIvP,QAAU6I,sBAAsB,kBAAM0G,EAAIxP,OAAO4S,MAAMoL,eAAiB,WAC5ExO,EAAI2F,KAAO3F,EAAIA,EAAI2F,QAGrBF,GAAmBG,EAAoB,GACvCjB,EAAajJ,QACb0S,KAEArK,EADA2B,GAAiB,GAEjBH,GAAUnE,QAAQ,SAAAlS,UAAK+P,GAAY/P,EAAEoG,KAAK+Z,YAAcngB,EAAEoG,KAAK+Z,UAAUngB,KACzEwW,GAAiBpT,GAAcgc,cAAe,EAC9CrK,EAAU,gBAlDT9W,GAAamF,GAAe,YAAasS,KAoD3C0K,EAAc,EACdC,GAAa,EAEbxL,EAAa,SAAbA,WAAchT,MACC,IAAVA,IAAiB2U,KAAmBF,EAAc,CACrDlT,GAAckd,YAAa,EAC3BvD,IAAYA,GAASnb,OAAO,OACxB6W,EAAIpC,GAAUhV,OACjBiY,EAAOxX,KACPye,EAAkC,IAAjBjH,EAAO0D,EACxBlE,EAASL,GAAKpC,GAAU,GAAGyC,YAC5BuH,GAA2BvH,EAAdsH,GAAwB,EAAI,EACzC5J,KAAmB4J,EAActH,GAC7ByH,IACC5L,KAAoB9F,IAA2C,IAAzByK,EAAO3E,KAChDA,GAAkB,EAClBI,EAAU,cAEXqH,GAASY,EACTA,EAAS1D,GAEN+G,GAAa,EAAG,KACnBjK,GAAKqC,EACS,EAAPrC,MACNC,GAAUD,KAAOC,GAAUD,IAAIxU,OAAO,EAAG2e,GAE1CF,GAAa,WAERjK,GAAK,EAAGA,GAAKqC,EAAGrC,KACpBC,GAAUD,KAAOC,GAAUD,IAAIxU,OAAO,EAAG2e,GAG3Cnd,GAAckd,YAAa,EAE5B1L,EAAS,GAEV8C,EAAmB,CA5SX,OACD,MA2S0B5D,EAASD,EAAQwE,GAAUiF,GAASjF,GAAU8E,GAAQ9E,GAAUgF,GAAMhF,GAAU+E,GAAO,UAAW,aAAc,QAAS,SAAU,kBAAmB,gBAAiB,eAAgB,aAAc,WAAY,cAAe,YAAa,YAAa,SAC3R1E,GAAchB,EAAiB8I,OAAO,CAACvI,GAAQE,GAAS,YAAa,MAAQoF,GAAQ,MAAQC,EAAS,WAAYnF,GAASD,GAAUA,GAAWiF,GAAMjF,GAAW+E,GAAQ/E,GAAWkF,GAASlF,GAAWgF,KA6CxMqD,GAAW,WACXnI,GAAY,SAAZA,UAAYK,MACPA,EAAO,KAITlT,EAAGvG,EAHAgV,EAAQyE,EAAM3Y,EAAEkU,MACnBuE,EAAIE,EAAMtX,OACVL,EAAI,OAEJ2X,EAAM3Y,EAAEwX,OAASla,GAAK+F,KAAKoX,SAAS9B,EAAM3Y,IAAIiW,QAAU,EAClDjV,EAAIyX,EAAGzX,GAAI,EACjB9B,EAAQyZ,EAAM3X,EAAE,GAChByE,EAAIkT,EAAM3X,GACN9B,EACHgV,EAAMzO,GAAKvG,EACDgV,EAAMzO,IAChByO,EAAMgG,eAAezU,EAAEib,QAAQD,GAAU,OAAOE,iBA4BpDrR,GAAc,CAAC0K,KAAK,EAAGC,IAAI,GA+D3BM,GAAa,qCAyFDnX,4BAQZ+C,KAAA,cAAKC,EAAM+J,WACLE,SAAWnE,KAAKwN,MAAQ,OACxBtT,MAAQ8F,KAAK6B,MAAK,GAAM,GACxBkP,QAwBJ2D,EAASC,EAAUC,EAAUC,EAASC,EAAStH,EAAOC,EAAKsH,EAAaC,EAAWC,EAAoBC,EAAkBC,EAAYC,EACrIC,EAAQC,EAAkBC,EAAgBC,EAAUrK,EAAQvX,EAAQ6hB,EAAWC,EAAWC,EAAUC,EAAWC,EAAcxK,EAAayK,EAAmBC,EAC7JC,EAAiB5K,EAAI6K,EAAOC,EAAOC,GAAYC,EAAaC,EAAcC,GAAiBC,GAAYC,GAAkBC,EAAgBC,EArBrI5G,GADL5V,EAAOyK,GAAcpC,GAAUrI,IAAS4J,GAAU5J,IAASA,EAAKyc,SAAY,CAAC1M,QAAS/P,GAAQA,EAAMgY,KAC/FpC,SAAU8G,EAAsO1c,EAAtO0c,YAAajZ,EAAyNzD,EAAzNyD,GAAIkZ,EAAqN3c,EAArN2c,SAAU5C,GAA2M/Z,EAA3M+Z,UAAW6C,EAAgM5c,EAAhM4c,MAAO7M,GAAyL/P,EAAzL+P,QAASiB,GAAgLhR,EAAhLgR,IAAK6L,GAA2K7c,EAA3K6c,WAAYC,GAA+J9c,EAA/J8c,oBAAqB5E,EAA0IlY,EAA1IkY,cAAe6E,EAA2H/c,EAA3H+c,gBAAiBC,EAA0Ghd,EAA1Ggd,eAAgBC,GAA0Fjd,EAA1Fid,KAAM9R,GAAoFnL,EAApFmL,KAAM+R,GAA8Eld,EAA9Ekd,YAAaC,EAAiEnd,EAAjEmd,UAAWvQ,GAAsD5M,EAAtD4M,mBAAoBwQ,GAAkCpd,EAAlCod,cAAeC,GAAmBrd,EAAnBqd,gBACjO7R,GAAYxL,EAAKqZ,YAAerZ,EAAK4M,qBAA0C,IAApB5M,EAAKqZ,WAAwBja,GAAcvE,GACtGyiB,IAAYV,GAAmB,IAAVA,EACrB5H,GAAWrb,EAAWqG,EAAKgV,UAAYhc,IACvCukB,EAAgBrmB,GAAK+F,KAAKoX,SAASW,IACnCjP,GAAarO,GAAYsd,IACzB7H,GAA0H,WAAtG,YAAanN,EAAOA,EAAKwd,QAAUnmB,EAAc2d,GAAU,YAAejP,IAAc,SAC5G0X,GAAY,CAACzd,EAAK0d,QAAS1d,EAAK2d,QAAS3d,EAAK4d,YAAa5d,EAAK6d,aAChE5F,GAAgBqF,IAAYtd,EAAKiY,cAAcpZ,MAAM,KACrDif,GAAU,YAAa9d,EAAOA,EAAK8d,QAAU9F,GAAU8F,QACvDhL,GAAc/M,GAAa,EAAIH,WAAW4E,GAAkBwK,IAAU,SAAWxJ,GAAUlM,GAAK6X,MAAY,EAC5Gtd,GAAOiM,KACPiY,GAAgB/d,EAAK+d,eAAkB,kBAAM/d,EAAK+d,cAAclkB,KAChEmkB,GA7kBa,SAAfC,aAAgBjJ,EAAUjP,SAAatG,IAAAA,EAAGC,IAAAA,GAAI7C,IAAAA,SAAQA,EAAIxF,EAAc2d,EAAU,0BAA4B,kBAAMnY,IAAI4C,IAAK,kBAAOsG,EAAa+C,GAAsBpJ,GAAMsV,EAAS,SAAWtV,KAAQ,GA6kBrLue,CAAajJ,GAAUjP,GAAYyF,IACrD0S,GA7kBgB,SAAlBC,gBAAmB7mB,EAASyO,UAAgBA,IAAevO,GAASC,QAAQH,GAAW2R,GAAe3R,GAAW,kBAAM4R,IA6kBhGiV,CAAgBnJ,GAAUjP,IAC/CqY,GAAW,EACXC,GAAc,EACdC,GAAe,EACfrS,GAAa3R,EAAe0a,GAAUxJ,OAMvC3R,GAAK+f,YAAc/f,GAAK6f,WAAY,EACpC7f,GAAK2f,KAAOhO,GACZ0M,GAAiB,GACjBre,GAAKmb,SAAWA,GAChBnb,GAAK6Y,OAAS9F,GAAqBA,GAAmBsG,KAAKqL,KAAK3R,IAAsBX,GACtF0O,EAAU1O,KACVpS,GAAKmG,KAAOA,EACZ+J,EAAYA,GAAa/J,EAAK+J,UAC1B,oBAAqB/J,IACxBqW,GAAQ,GACkB,OAA1BrW,EAAKwe,kBAA8B7H,GAAW9c,KAE/C0jB,EAAckB,YAAclB,EAAckB,aAAe,CACxD5K,IAAKkB,GAAiBC,GAAUna,IAChC+Y,KAAMmB,GAAiBC,GAAU5V,KAElCvF,GAAK2gB,QAAUA,EAAU+C,EAAckB,YAAYjT,GAAUnM,GAC7DxF,GAAK6kB,cAAgB,SAAA5lB,IACpBojB,EAActS,GAAU9Q,IAAUA,GAKjCmjB,GAAaA,GAAWjR,SAASlS,GAAUmjB,GAAa/kB,GAAK4e,GAAG/L,EAAW,CAAC4U,KAAM,OAAQC,cAAe,MAAOlJ,SAAS,EAAO1K,SAAUkR,EAAa2C,QAAQ,EAAMxJ,WAAY,6BAAM0H,GAAmBA,EAAgBljB,QAH1NoiB,IAAcA,GAAWhS,SAAS,GAAGtC,OACrCsU,GAAa,IAKXlS,IACHA,EAAU/J,KAAK8e,MAAO,EACrB/U,EAAUgV,WAAallB,GAAKmlB,aAAmD,IAAnCjV,EAAU/J,KAAKif,kBAAsD,IAAzBjf,EAAKif,iBAA6BlV,EAAUiB,YAAcjB,EAAU+P,OAAO,GAAG,GAAM,GAC7KjgB,GAAKkQ,UAAYA,EAAU3D,SAC3B2D,EAAUsJ,cAAgBxZ,IACrB6kB,cAAc9B,GACnBb,EAAQ,EACDtY,EAAPA,GAAYsG,EAAU/J,KAAKyD,IAGxB0H,KAEEtB,GAAUsB,MAASA,GAAKrQ,OAC5BqQ,GAAO,CAAC+T,OAAQ/T,wBAEIrN,GAAMgQ,OAAU5W,GAAK4d,IAAI/O,GAAa,CAACjI,GAAOnD,IAAUqa,GAAU,CAACkE,eAAgB,SACxG1gB,GAAWsT,QAAQ,SAAApE,UAAKiC,GAAYjC,IAAMA,EAAExM,UAAY6K,GAAatL,GAAKC,kBAAoBC,GAASqa,MAActN,EAAEvM,QAAS,KAChIuf,EAAW/Q,GAAYwB,GAAK+T,QAAU/T,GAAK+T,OAAyB,WAAhB/T,GAAK+T,OApkBxC,SAAnBC,iBAAmBpV,UAAa,SAAAjR,UAAS5B,GAAK8C,MAAMmR,KAAKN,GAAoBd,GAAYjR,IAokBRqmB,CAAiBpV,GAA6B,sBAAhBoB,GAAK+T,OApiB7F,SAAvBE,qBAAuBtU,UAAY,SAAChS,EAAOumB,UAAOpU,GAAiBJ,GAAoBC,GAArCG,CAAgDnS,EAAOumB,EAAG7T,YAoiByC4T,CAAqBrV,IAAkC,IAArBoB,GAAKmU,YAAwB,SAACxmB,EAAOumB,UAAOpU,GAAiBE,GAAK+T,OAAtBjU,CAA8BnS,EAAO4C,KAAa2iB,GAAc,IAAM,EAAIgB,EAAG7T,YAAatU,GAAK8C,MAAMmR,KAAKA,GAAK+T,QAChV/C,EAAehR,GAAKH,UAAY,CAACrP,IAAK,GAAKM,IAAK,GAChDkgB,EAAetS,GAAUsS,GAAgBpG,GAAOoG,EAAaxgB,IAAKwgB,EAAalgB,KAAO8Z,GAAOoG,EAAcA,GAC3GC,GAAkBllB,GAAKyP,YAAYwE,GAAKoU,OAAUrD,EAAc,GAAM,GAAK,eACtExJ,EAASzG,KACZuT,EAAoB9jB,KAAa2iB,GAAc,IAC/CjJ,EAAQoF,EAAQpF,WACZoK,GAAqBnmB,KAAKyD,IAAIjD,GAAKsC,eAAiB,KAAQiZ,GAAU3M,IAAkB2V,KAAa1L,EAoC/F7Y,GAAK4lB,UAAYrB,KAAa1L,GACxC0J,GAAgBjX,SAAQ,OArCyF,KAMhHua,EAAUC,EALP1V,GAAYyI,EAASY,GAAS6H,EACjCyD,EAAgB7U,IAAcuT,GAAWvT,EAAU6U,gBAAkB3U,EACrE2V,EAAWJ,EAAoB,GAAMZ,EAAgB5C,IAAUtgB,KAAasa,IAAU,KAAS,EAC/Fd,EAAUhe,GAAK8C,MAAM+D,OAAOkM,EAAU,EAAIA,EAAU6M,GAAK8I,EAAW,GAAKA,EAAW,MACpFC,EAAa5V,IAA6B,IAAjBkB,GAAK2U,QAAoB,EAAI5K,GAEpD6K,EAAqC5U,GAArC4U,QAAStL,EAA4BtJ,GAA5BsJ,YAAaY,EAAelK,GAAfkK,cACzBqK,EAAWhF,EAASmF,EAAYhmB,IAChC+P,GAAU8V,KAAcA,EAAWG,GACnCF,EAAYtmB,KAAK4C,IAAI,EAAG5C,KAAKC,MAAMga,EAAQoM,EAAWvE,IAClDzI,GAAUa,GAAiBD,GAAVZ,GAAmBiN,IAAcjN,EAAQ,IACzD0C,IAAUA,EAAM2J,UAAY3J,EAAMhY,MAAQ0Z,GAAK6I,EAAYjN,WAG1C,IAAjBvH,GAAK2U,UACR5K,EAAUwK,EAAWzV,GAEtBuQ,EAAQmF,EAAW,CAClB3U,SAAUmR,EAAarF,GAAoF,KAA7Ezd,KAAK4C,IAAI6a,GAAK+I,EAAajB,GAAgB9H,GAAK4I,EAAWd,IAA0BgB,EAAW,KAAS,IACvIjB,KAAMxT,GAAKwT,MAAQ,SACnBvhB,KAAM0Z,GAAK6I,EAAYjN,GACvB+B,YAAa,8BAAM2H,GAAgBjX,SAAQ,IAASsP,GAAeA,EAAY5a,KAC/Ewb,iCACCxb,GAAK2B,SACL4iB,GAAWnS,KACPlC,IAAcuT,KACjBrB,GAAaA,GAAW+D,QAAQ,gBAAiBN,EAAU3V,EAAUkW,OAASlW,EAAUmW,OAASnW,EAAUE,SAASyV,IAErH3D,EAAQC,EAAQjS,IAAcuT,GAAWvT,EAAU6U,gBAAkB/kB,GAAKoQ,SAC1E+S,GAAkBA,EAAenjB,IACjCwb,GAAcA,EAAWxb,MAExB6Y,EAAQwC,EAAUiG,EAAQwE,EAAYjN,EAASwC,EAAUiG,GAC5D4E,GAAWA,EAAQlmB,GAAM2gB,EAAQpF,WAKjChP,SAEJ3C,IAAOiV,GAAKjV,GAAM5J,IAKK2iB,GADvBA,GAHAzM,GAAUlW,GAAKkW,QAAUpW,EAAWoW,KAAoB,IAARiB,IAAgBA,MAGhCjB,GAAQqB,OAASrB,GAAQqB,MAAM+O,WACnB3D,EAAmB3iB,IAE/DmX,IAAc,IAARA,GAAejB,GAAUpW,EAAWqX,IAC1C3I,GAAUqU,KAAiBA,EAAc,CAAC0D,QAASrQ,GAASsQ,UAAW3D,IACnE1L,MACa,IAAf6L,IAAwBA,KAAe5K,KAAa4K,MAAcA,IAAc7L,GAAImB,YAAcnB,GAAImB,WAAWrE,OAAuD,SAA9CtD,GAAkBwG,GAAImB,YAAYrB,UAA6BkB,IAC1LnY,GAAKmX,IAAMA,IACXyJ,EAAWvjB,GAAK+F,KAAKoX,SAASrD,KAChBC,OAYbmK,EAAmBX,EAASa,UAXxB6B,KACHA,EAAYxjB,EAAWwjB,MACTA,EAAUV,WAAaU,EAAYA,EAAUvI,SAAWuI,EAAUmD,eAChF7F,EAAS8F,iBAAmBpD,EAC5BA,IAAc1C,EAAStJ,YAAciB,GAAU+K,KAEhD1C,EAASxJ,OAASA,EAASkM,GAAa1iB,GAAKyS,cAAc,OAC3D+D,EAAOuP,UAAUnW,IAAI,cACrB5G,GAAMwN,EAAOuP,UAAUnW,IAAI,cAAgB5G,GAC3CgX,EAASa,SAAWF,EAAmBhJ,GAAUpB,MAIjC,IAAjBhR,EAAKygB,SAAqBvpB,GAAK4d,IAAI9D,GAAK,CAACyP,SAAS,IAClD5mB,GAAKoX,OAASA,EAASwJ,EAASxJ,OAChCC,EAAK1G,GAAkBwG,IACvB2K,EAAezK,EAAG2L,GAAarR,GAAUhM,KACzC+b,EAAYrkB,GAAKkE,YAAY4V,IAC7BwK,EAAYtkB,GAAKwpB,YAAY1P,GAAKxF,GAAU3O,EAAGiV,IAE/Cf,GAAWC,GAAKC,EAAQC,GACxBoK,EAAWlJ,GAAUpB,KAElB8M,GAAS,CACZ7C,EAAapR,GAAUiU,IAAWrT,GAAaqT,GAAS/F,IAAmBA,GAC3EgD,EAAqBtO,GAAc,iBAAkBhJ,EAAIuR,GAAUxJ,GAAWyP,EAAY,GAC1FD,EAAmBvO,GAAc,eAAgBhJ,EAAIuR,GAAUxJ,GAAWyP,EAAY,EAAGF,GACzFrhB,EAASqhB,EAAmB,SAAWvP,GAAU3L,GAAGH,QAChDihB,EAAUhnB,EAAWtC,EAAc2d,GAAU,YAAcA,IAC/D6F,EAAc/U,KAAK+U,YAAcpO,GAAc,QAAShJ,EAAIkd,EAASnV,GAAWyP,EAAYvhB,EAAQ,EAAGkT,IACvGkO,EAAYhV,KAAKgV,UAAYrO,GAAc,MAAOhJ,EAAIkd,EAASnV,GAAWyP,EAAYvhB,EAAQ,EAAGkT,IACjGA,KAAuB2P,EAAiBrlB,GAAKwpB,YAAY,CAAC7F,EAAaC,GAAYtP,GAAU3O,EAAGiV,KAC1F3E,IAAsB3V,GAASyD,SAAsD,IAA5C5D,EAAc2d,GAAU,kBA7rBrD,SAApB4L,kBAAoBtpB,OACfma,EAAWjH,GAAkBlT,GAASma,SAC1Cna,EAAQwW,MAAM2D,SAAyB,aAAbA,GAAwC,UAAbA,EAAwBA,EAAW,WA4rBtFmP,CAAkB7a,GAAajI,GAAQkX,IACvC9d,GAAK4d,IAAI,CAACiG,EAAoBC,GAAmB,CAACyF,SAAS,IAC3D7E,EAAoB1kB,GAAKwpB,YAAY3F,EAAoBvP,GAAU3O,EAAGiV,IACtEgK,EAAkB5kB,GAAKwpB,YAAY1F,EAAkBxP,GAAU3O,EAAGiV,QAIhElF,GAAoB,KACnBiU,EAAcjU,GAAmB5M,KAAK4V,SACzCkL,EAAYlU,GAAmB5M,KAAK+gB,eACrCnU,GAAmBoU,cAAc,WAAY,WAC5CnnB,GAAK2B,OAAO,EAAG,EAAG,GAClBqlB,GAAeA,EAAYI,MAAMrU,GAAoBkU,GAAa,SAIpEjnB,GAAKqnB,SAAW,kBAAMjR,GAAUA,GAAUxY,QAAQoC,IAAQ,IAC1DA,GAAKsnB,KAAO,kBAAMlR,GAAUA,GAAUxY,QAAQoC,IAAQ,IAEtDA,GAAK+N,OAAS,SAACA,EAAQwZ,OACjBA,SAAevnB,GAAK8N,MAAK,OAC1B0Z,GAAe,IAAXzZ,IAAqB/N,GAAKsQ,QACjCmX,EAAiBtS,GACdqS,IAAMxnB,GAAKmlB,aACVqC,IACHhF,GAAahjB,KAAK4C,IAAIgQ,KAAcpS,GAAK6Y,OAAOrC,KAAO,GACvDiO,GAAezkB,GAAKoQ,SACpBqS,GAAmBvS,GAAaA,EAAUE,YAE3C4Q,GAAe,CAACA,EAAaC,EAAWC,EAAoBC,GAAkBlP,QAAQ,SAAAiI,UAAKA,EAAEjG,MAAMgD,QAAUuQ,EAAI,OAAS,UACtHA,IACHrS,GAAcnV,IACT2B,OAAO6lB,IAETrQ,IAASkM,IAAgBrjB,GAAK4lB,WAC7B4B,EAncM,SAAdE,YAAevQ,EAAKC,EAAQsB,GAC3BL,GAAUK,OACN9Z,EAAQuY,EAAII,SACZ3Y,EAAM8nB,eACTrO,GAAUzZ,EAAM0Y,kBACV,GAAIH,EAAII,MAAMC,UAAW,KAC3BhE,EAAS4D,EAAOkB,WAChB9E,IACHA,EAAOa,aAAa8C,EAAKC,GACzB5D,EAAOqD,YAAYO,IAGrBD,EAAII,MAAMC,WAAY,EAwblBkQ,CAAYvQ,GAAKC,EAAQmK,GAEzBrK,GAAWC,GAAKC,EAAQzG,GAAkBwG,IAAMG,IAGlDkQ,GAAKxnB,GAAK2B,OAAO6lB,GACjBrS,GAAcsS,EACdznB,GAAKmlB,WAAaqC,IAIpBxnB,GAAKsf,QAAU,SAACqI,EAAM/lB,EAAOgW,EAAUgQ,OACjCzS,IAAgBnV,GAAKsQ,SAAa1O,KAGnCuV,IAAOwQ,GAAQjT,GAClB1W,GAAamF,cAAe,YAAasS,UAGzCc,IAAkB2N,IAAiBA,GAAclkB,IAClDmV,GAAcnV,GACV2gB,EAAQpF,QAAU3D,IACrB+I,EAAQpF,MAAMzN,OACd6S,EAAQpF,MAAQ,GAEjB6G,IAAcA,GAAW7V,QACzB0W,IAAuB/S,GAAaA,EAAUnC,OAAO,CAACD,MAAM,IAAQ+Z,aACpE7nB,GAAKmlB,YAAcnlB,GAAK+N,QAAO,GAAM,GACrC/N,GAAKuf,eAAgB,MAapBlI,EAAIsC,EAAQd,EAAQiP,EAAYC,EAAUC,EAAYC,EAAQC,EAAgBC,EAASC,EAAcC,EAAgBC,EAAmBC,EAZrIhW,EAAO4R,KACVnL,EAAiBqL,KACjBjiB,EAAM2Q,GAAqBA,GAAmB5B,WAAazB,GAAWyL,GAAUxJ,IAChF6W,EAAiBlH,GAAU,IAC3BzhB,EAAS,EACT4oB,EAAiBb,GAAa,EAC9Bc,EAAY1Y,GAAU4H,GAAYA,EAAS8B,IAAMvT,EAAKuT,IACtDiP,EAAmBxiB,EAAKyiB,YAAc1S,GACtC2S,EAAc7Y,GAAU4H,GAAYA,EAAS6B,MAAStT,EAAKsT,QAAyB,IAAftT,EAAKsT,OAAgBvD,GAAeiB,GAAM,MAAQ,SAAnB,GACpG2R,EAAkB9oB,GAAK8oB,gBAAkB3iB,EAAK2iB,iBAAmBhpB,EAAWqG,EAAK2iB,gBAAiB9oB,IAClG+oB,EAAgB7S,IAAW1W,KAAK4C,IAAI,EAAGgU,GAAUxY,QAAQoC,MAAW,EACpEe,EAAIgoB,MAED9E,IAAWjU,GAAU4H,KACxB0Q,EAAoBjrB,GAAKkE,YAAY2f,EAAoBvP,GAAUnM,GACnE+iB,EAAkBlrB,GAAKkE,YAAY4f,EAAkBxP,GAAUnM,IAEnD,EAANzE,MACNinB,EAAa5R,GAAUrV,IACZ2Y,KAAOsO,EAAW1I,QAAQ,EAAG,KAAOnK,GAAcnV,MAC7DioB,EAASD,EAAW7Q,MACL8Q,IAAW/R,IAAW+R,IAAW9Q,IAAO8Q,IAAWa,GAAqBd,EAAW7C,cAChFiD,EAAjBA,GAAgC,IACnBY,QAAQhB,GACrBA,EAAWja,QAAO,GAAM,IAErBia,IAAe5R,GAAUrV,KAC5BgoB,IACAhoB,SAGF+O,GAAY+Y,KAAiBA,EAAcA,EAAY7oB,KACvD6oB,EAActa,GAAYsa,EAAa,QAAS7oB,IAChDyZ,EAAQd,GAAekQ,EAAa3S,GAAS3D,EAAMZ,GAAWS,KAAc4O,EAAaE,EAAoBlhB,GAAMgZ,EAAgBC,GAAa3F,GAAkBlR,EAAK2Q,GAAoB/S,GAAK+f,aAAe,iBAAmB5I,IAAO,KAAQ,GACjPrH,GAAY4Y,KAAeA,EAAYA,EAAU1oB,KAC7CwO,GAAUka,KAAeA,EAAU9qB,QAAQ,SACzC8qB,EAAU9qB,QAAQ,KACtB8qB,GAAala,GAAUqa,GAAeA,EAAY7jB,MAAM,KAAK,GAAK,IAAM0jB,GAExE7oB,EAASyS,GAAYoW,EAAUja,OAAO,GAAI8D,GAC1CmW,EAAYla,GAAUqa,GAAeA,GAAe9V,GAAqB1V,GAAK8C,MAAMoZ,SAAS,EAAGxG,GAAmB5B,WAAY4B,GAAmByG,cAAcC,MAAO1G,GAAmByG,cAAcE,IAAKD,GAASA,GAAS5Z,EAC/N8oB,EAAmBzS,KAGrBwS,EAAYna,GAAYma,EAAW,MAAO1oB,IAC1C0Z,EAAMla,KAAK4C,IAAIqX,EAAOd,GAAe+P,IAAcC,EAAmB,SAAWvmB,GAAMumB,EAAkBpW,EAAMZ,GAAWS,KAAevS,EAAQohB,EAAWE,EAAkBnhB,GAAMgZ,EAAgBC,GAAa3F,GAAkBlR,EAAK2Q,GAAoB/S,GAAK6f,WAAa,gBAAkB,KAEhShgB,EAAS,EACTkB,EAAIgoB,EACGhoB,MAENknB,GADAD,EAAa5R,GAAUrV,IACHoW,MACN6Q,EAAWvO,MAAQuO,EAAWiB,UAAYxP,IAAU1G,IAAuC,EAAjBiV,EAAWtO,MAClGrC,EAAK2Q,EAAWtO,KAAO1Z,GAAK+f,YAAcvgB,KAAK4C,IAAI,EAAG4lB,EAAWvO,OAASuO,EAAWvO,QAC/EwO,IAAW/R,IAAW8R,EAAWvO,MAAQuO,EAAWiB,SAAWxP,GAAUwO,IAAWa,IAAoBpc,MAAMmc,KACnHhpB,GAAUwX,GAAM,EAAI2Q,EAAW5X,WAEhC6X,IAAW9Q,KAAQsR,GAAkBpR,OAGvCoC,GAAS5Z,EACT6Z,GAAO7Z,EACPG,GAAK+f,cAAgB/f,GAAK+f,aAAelgB,GAErCG,GAAK6f,YAActJ,KACtBvW,GAAK6f,UAAYnG,IAAQ,KACzBA,EAAMla,KAAKsC,IAAI4X,EAAKhK,GAAWyL,GAAUxJ,MAE1C2P,EAAU5H,EAAMD,IAAYA,GAAS,MAAS,KAE1C+O,IACH/D,GAAepnB,GAAK8C,MAAM+D,MAAM,EAAG,EAAG7G,GAAK8C,MAAM+oB,UAAUzP,EAAOC,EAAK8I,MAExExiB,GAAKipB,SAAWR,EACZzH,GAAenhB,KAClBwX,EAAK,IACF1F,GAAU3O,GAAK,KAAOnD,EACzBipB,IAAoBzR,EAAG1F,GAAUnM,GAAK,KAAO4M,MAC7C/U,GAAK4d,IAAI,CAAC+F,EAAaC,GAAY5J,KAGhCF,IAASwF,IAAgB3c,GAAK0Z,KAAOhK,GAAWyL,GAAUxJ,KAuEvD,GAAIuE,IAAW9D,OAAiBW,OACtC4G,EAASzD,GAAQoC,WACVqB,GAAUA,IAAW1V,IACvB0V,EAAOwP,aACV1P,GAASE,EAAOwP,WAChBzP,GAAOC,EAAOwP,YAEfxP,EAASA,EAAOrB,gBA7EjBjB,EAAK1G,GAAkBwG,IACvB2Q,EAAanW,KAAc3Q,GAC3B6X,EAASzG,KACTwP,EAAW7V,WAAW2V,EAAU/P,GAAU3O,IAAMylB,GAC3CrmB,GAAa,EAANsX,IAEX2O,EAAiB,CAACpU,MADlBoU,GAAkBnc,GAActL,GAAKC,kBAAoBC,GAAUqa,IAAUlH,MACpChV,MAAOopB,EAAe,WAAa1W,GAAU3O,EAAEomB,gBACpFld,IAAmF,WAArEyE,GAAkB1M,IAAO,WAAa0N,GAAU3O,EAAEomB,iBACnEf,EAAepU,MAAM,WAAatC,GAAU3O,EAAEomB,eAAiB,WAGjElS,GAAWC,GAAKC,EAAQC,GACxBoK,EAAWlJ,GAAUpB,IAErBwC,EAASlK,GAAW0H,IAAK,GACzB+Q,EAAiB5U,IAAoB7S,EAAe0a,GAAU2M,EAAaviB,GAAcvE,GAApDP,GACjCuiB,KACH1L,EAAc,CAAC0L,GAAarR,GAAUhM,IAAK2b,EAASmH,EAAiBxQ,KACzDlY,EAAIqX,GAChBrW,EAAKiiB,KAAe7K,GAAYpH,GAASoG,GAAKxF,IAAa2P,EAASmH,EAAiB,KAEpFnR,EAAYrW,KAAK0Q,GAAU/L,EAAG7E,EAAIkX,IACP,SAA3Bb,EAAOnD,MAAM4D,YAAyBT,EAAOnD,MAAM4D,UAAY9W,EAAIkX,KAEpEI,GAAUf,GACNwR,GACH1S,GAAUnE,QAAQ,SAAAlS,GACbA,EAAEoX,MAAQ2R,IAAyC,IAAtB/oB,EAAEoG,KAAK6c,aACvCjjB,EAAEwf,eAAgB,KAIrBjM,IAAoBlB,GAAWoQ,MAE/BzhB,EAAIgQ,GAASoG,GAAKxF,MACc,SAA3ByF,EAAOnD,MAAM4D,YAAyBT,EAAOnD,MAAM4D,UAAY9W,EAAIkX,IAErE3E,MACHyU,EAAW,CACV/N,IAAML,EAAOK,KAAO8N,EAAajP,EAASY,EAAQyO,GAAmBjQ,GACrE8B,KAAOJ,EAAOI,MAAQ+N,EAAaI,EAAiBrP,EAASY,GAAUxB,GACvEF,UAAW,aACXH,SAAU,UAEFI,IAAU+P,EAAQ,SAAmBvoB,KAAK6pB,KAAK1P,EAAOrK,OAAS2I,GACxE8P,EAAS7P,IAAW6P,EAAQ,UAAoBvoB,KAAK6pB,KAAK1P,EAAOnK,QAAUyI,GAC3E8P,EAAS3P,IAAW2P,EAAS3P,GAAUgF,IAAQ2K,EAAS3P,GAAU8E,IAAU6K,EAAS3P,GAAUiF,IAAW0K,EAAS3P,GAAU+E,IAAS,IACtI4K,EAAS5P,IAAYd,EAAGc,IACxB4P,EAAS5P,GAAWiF,IAAQ/F,EAAGc,GAAWiF,IAC1C2K,EAAS5P,GAAW+E,IAAU7F,EAAGc,GAAW+E,IAC5C6K,EAAS5P,GAAWkF,IAAWhG,EAAGc,GAAWkF,IAC7C0K,EAAS5P,GAAWgF,IAAS9F,EAAGc,GAAWgF,IAC3CqE,EA7hBS,SAAb8H,WAAc5Q,EAAOqP,EAAUwB,WAI7B/jB,EAHG+K,EAAS,GACZiI,EAAIE,EAAMtX,OACVL,EAAIwoB,EAAc,EAAI,EAEhBxoB,EAAIyX,EAAGzX,GAAK,EAClByE,EAAIkT,EAAM3X,GACVwP,EAAOtP,KAAKuE,EAAIA,KAAKuiB,EAAYA,EAASviB,GAAKkT,EAAM3X,EAAE,WAExDwP,EAAOxQ,EAAI2Y,EAAM3Y,EACVwQ,EAmhBa+Y,CAAW/H,EAAkBwG,EAAU1E,IACxD9M,IAAkBnE,GAAW,IAE1BlC,GACHiY,EAAUjY,EAAUgV,SACpBzI,GAAoB,GACpBvM,EAAU+P,OAAO/P,EAAUiB,YAAY,GAAM,GAC7C0Q,EAAYH,EAAU/P,GAAU3O,GAAK4e,EAAWN,EAASmH,EACzDzG,EAA0C,EAA/BxiB,KAAKyD,IAAIqe,EAASO,GAC7BvO,IAAoB0O,GAAYR,EAAexT,OAAOwT,EAAepgB,OAAS,EAAG,GACjF8O,EAAU+P,OAAO,GAAG,GAAM,GAC1BkI,GAAWjY,EAAU2X,YAAW,GAChC3X,EAAUsD,QAAUtD,EAAUO,UAAUP,EAAUO,aAClDgM,GAAoB,IAEpBoF,EAAYP,EAEb+G,IAAmBA,EAAeppB,MAASopB,EAAepU,MAAM,WAAatC,GAAU3O,EAAEomB,eAAiBf,EAAeppB,MAASopB,EAAepU,MAAMgG,eAAe,YAActI,GAAU3O,IAW/LolB,GAAgBA,EAAanW,QAAQ,SAAAlS,UAAKA,EAAEgO,QAAO,GAAO,KAC1D/N,GAAKyZ,MAAQA,EACbzZ,GAAK0Z,IAAMA,EACXoH,EAAUC,EAAUxK,GAAiBiM,GAAapQ,KAC7CW,IAAuBwD,KAC3BuK,EAAU0B,IAAcpQ,GAAWoQ,IACnCxiB,GAAK6Y,OAAOrC,IAAM,GAEnBxW,GAAK+N,QAAO,GAAO,GACnByW,GAAc3iB,KACV0gB,KACHgC,IAAY,EAEZhC,GAAgBjX,SAAQ,IAEzB6J,GAAc,EACdjF,GAAauT,KAAavT,EAAUgV,UAAYzC,KAAqBvS,EAAUE,aAAeqS,IAAoBvS,EAAUE,SAASqS,IAAoB,GAAG,GAAMxC,OAAO/P,EAAUmJ,QAAQ,GAAM,IAC7LmP,GAAkB/D,KAAiBzkB,GAAKoQ,UAAY2C,IAAsBkQ,IAAwB/S,IAAcA,EAAUgV,YAC7HhV,IAAcuT,IAAYvT,EAAU6U,cAAchS,IAAsB0G,GAAS,OAAUgL,GAAepnB,GAAK8C,MAAM+oB,UAAUzP,EAAOC,EAAK,GAAK+K,IAAc,GAC9JzkB,GAAKoQ,SAAWoY,IAAoB1H,EAAUrH,GAAS6H,IAAWmD,GAAgB,EAAIA,IAEvFtN,IAAO6L,KAAe5L,EAAO+R,WAAa3pB,KAAKC,MAAMO,GAAKoQ,SAAWyR,IACrEO,IAAcA,GAAWyF,aAEpBnb,MAAM4b,KACVA,GAAqBjrB,GAAKkE,YAAY2f,EAAoBvP,GAAUnM,GACpE+iB,GAAmBlrB,GAAKkE,YAAY4f,EAAkBxP,GAAUnM,GAChEwV,GAAakG,EAAoBvP,GAAW2W,GAC5CtN,GAAagG,EAAarP,GAAW2W,GAAqBV,GAAa,IACvE5M,GAAamG,EAAkBxP,GAAW4W,GAC1CvN,GAAaiG,EAAWtP,GAAW4W,GAAmBX,GAAa,KAGpEY,IAAmBjS,IAAkBvW,GAAK2B,UAEtCue,IAAc3J,IAAmB8K,IACpCA,GAAqB,EACrBnB,GAAUlgB,IACVqhB,GAAqB,KAIvBrhB,GAAKsC,YAAc,kBAAQ8P,KAAe2O,IAAYlf,KAAasa,IAAU,KAAS,GAEtFnc,GAAKwpB,aAAe,WACnBvZ,GAAcjQ,GAAK0Q,mBACfR,IACHkS,GAAaA,GAAWhS,SAAS,GAAOF,EAAU8U,SAA4DvB,IAAYxT,GAAcC,EAAWlQ,GAAK2R,UAAY,EAAG,GAA1G1B,GAAcC,EAAWA,EAAUC,cAIlGnQ,GAAKypB,cAAgB,SAAAC,UAASxZ,GAAaA,EAAUgB,SAAYuI,GAASzZ,GAAKsf,WAAa7F,GAAUvJ,EAAUgB,OAAOwY,GAASxZ,EAAUiB,WAAcmQ,GAAW,GAEnKthB,GAAK2pB,YAAc,SAAAhmB,OACd5C,EAAIqV,GAAUxY,QAAQoC,IACzBgD,EAAqB,EAAjBhD,GAAK2R,UAAgByE,GAAU/H,MAAM,EAAGtN,GAAG6oB,UAAYxT,GAAU/H,MAAMtN,EAAE,UACtEyN,GAAU7K,GAAQX,EAAE4K,OAAO,SAAA7N,UAAKA,EAAEoG,KAAKqd,kBAAoB7f,IAAQX,GAAG4K,OAAO,SAAA7N,UAAsB,EAAjBC,GAAK2R,UAAgB5R,EAAE2Z,KAAOD,EAAQ1Z,EAAE0Z,OAASC,KAI5I1Z,GAAK2B,OAAS,SAACU,EAAOie,EAAgBuJ,OACjC9W,IAAuB8W,GAAcxnB,OAOxCujB,EAAqBkE,EAAaC,EAAQC,EAAcC,EAASC,EAASC,EAJvEtR,GAA4B,IAAnBtC,GAA0BiM,GAAaxiB,GAAK6Y,SACxDrT,EAAInD,EAAQ,GAAKwW,EAASY,GAAS6H,EACnC8I,EAAU5kB,EAAI,EAAI,EAAQ,EAAJA,EAAQ,EAAIA,GAAK,EACvCif,EAAezkB,GAAKoQ,YAEjBkQ,IACHS,EAAUD,EACVA,EAAU/N,GAAqBX,KAAeyG,EAC1CvH,KACH6Q,EAAQD,EACRA,EAAQhS,IAAcuT,GAAWvT,EAAU6U,gBAAkBqF,IAI3D/L,GAAiBlH,KAAQhC,KAAgBjW,IAAYwV,MACnD0V,GAAW3Q,EAAQZ,GAAWA,EAASkI,IAAYlf,KAAasa,IAAWkC,EAC/E+L,EAAU,KACY,IAAZA,GAAiB1Q,EAAMb,GAAWA,EAASkI,IAAYlf,KAAasa,IAAWkC,IACzF+L,EAAU,QAGRA,IAAY3F,GAAgBzkB,GAAKsQ,QAAS,IAI7C0Z,GADAC,GAFArE,EAAW5lB,GAAK4lB,WAAawE,GAAWA,EAAU,OACpC3F,GAAgBA,EAAe,OAEjB2F,KAAc3F,EAC1CzkB,GAAK2R,UAAsB8S,EAAV2F,EAAyB,GAAK,EAC/CpqB,GAAKoQ,SAAWga,EAEZJ,IAAiB7U,KACpB2U,EAAcM,IAAY3F,EAAe,EAAgB,IAAZ2F,EAAgB,EAAqB,IAAjB3F,EAAqB,EAAI,EACtFhB,KACHsG,GAAWE,GAA8C,SAAnC7L,GAAc0L,EAAc,IAAiB1L,GAAc0L,EAAc,IAAO1L,GAAc0L,GACpHK,EAAiBja,IAAyB,aAAX6Z,GAAoC,UAAXA,GAAsBA,KAAU7Z,KAI1FsT,KAAoByG,GAAWE,KAAoBA,GAAkBpH,IAAU7S,KAAeJ,GAAY0T,IAAmBA,GAAgBxjB,IAAQA,GAAK2pB,YAAYnG,IAAiBvR,QAAQ,SAAAlS,UAAKA,EAAEypB,kBAEjM/F,MACArB,IAAejN,IAAgBjW,GAQxBgR,GACVA,EAAU6U,cAAcqF,KAAYjV,KAAgBqP,KAAeniB,KARlE+f,GAAWiI,IAAIC,MAAQlI,GAAWmI,SAAWnI,GAAWkI,OAAUlI,GAAWnC,OAAOmC,GAAWiI,IAAIC,MAAQlI,GAAWmI,QACnHnI,GAAW+D,QACd/D,GAAW+D,QAAQ,gBAAiBiE,EAASla,EAAUkW,OAASlW,EAAUmW,QAE1EjE,GAAWjc,KAAK4e,cAAgBqF,EAChChI,GAAWyF,aAAavc,aAMvB6L,MACH9U,GAAS2gB,KAAe5L,EAAOnD,MAAM+O,GAAarR,GAAUhM,KAAOmc,GAC9DxO,IAEE,GAAI0W,EAAc,IACxBE,GAAW7nB,GAAmBoiB,EAAV2F,GAAoCvR,EAAVa,EAAM,GAAcb,EAAS,GAAKnJ,GAAWyL,GAAUxJ,IACjG0R,MACEhhB,IAAUujB,IAAYsE,EAK1B9P,GAAUjD,GAAKC,OALqB,KAChCuC,EAASlK,GAAW0H,IAAK,GAC5BtX,EAASgZ,EAASY,EACnBW,GAAUjD,GAAKlT,GAAQ0V,EAAOK,KAAOrI,KAAc3Q,GAAYnB,EAAS,GAAMoY,GAAM0B,EAAOI,MAAQpI,KAAc3Q,GAAY,EAAInB,GAAWoY,IAK9II,GAAUuN,GAAYsE,EAAU1I,EAAiBC,GAChDO,GAAYoI,EAAU,GAAKxE,GAAajE,EAAUC,GAAwB,IAAZwI,GAAkBF,EAAsB,EAAZrI,UAb3FF,EAAU5S,GAAO6S,EAAWC,EAAYuI,KAgB1C9Y,IAASqP,EAAQpF,OAAUpG,IAAgBjW,IAAYqjB,GAAgBjX,SAAQ,GAC/EuX,IAAgBoH,GAAY7G,IAAQgH,IAAYA,EAAU,IAAMxN,MAAsB5F,GAAS6L,EAAY0D,SAAStU,QAAQ,SAAAnU,UAAMA,EAAG6oB,UAAUf,GAAYxC,GAAO,MAAQ,UAAUP,EAAY2D,cAChMzK,GAAa0H,IAAaphB,GAAS0Z,EAAS/b,IACxCgqB,IAAiB7U,IAChBsO,KACC0G,IACY,aAAXJ,EACH7Z,EAAU3D,QAAQwY,cAAc,GACX,UAAXgF,EACV7Z,EAAU5E,SAAQ,GAAMiB,QACH,YAAXwd,EACV7Z,EAAU5E,SAAQ,GAElB4E,EAAU6Z,MAGZhO,GAAYA,EAAS/b,MAElBiqB,GAAYrN,KACfkG,GAAYmH,GAAW5Z,GAAUrQ,GAAM8iB,GACvCc,GAAUkG,IAAgBzZ,GAAUrQ,GAAM4jB,GAAUkG,IACpD1G,KAAqB,IAAZgH,EAAgBpqB,GAAK8N,MAAK,EAAO,GAAM8V,GAAUkG,GAAe,GACpEG,GAEJrG,GADAkG,EAA0B,IAAZM,EAAgB,EAAI,IACR/Z,GAAUrQ,GAAM4jB,GAAUkG,KAGlDvG,KAAkBqC,GAAYpmB,KAAKyD,IAAIjD,GAAKsC,gBAAkByN,GAAUwT,IAAiBA,GAAgB,QAC5GtT,GAAcjQ,GAAK0Q,mBACnB0R,GAAaA,GAAWhS,SAAS,GAAKH,GAAcC,EAAsB,YAAX6Z,EAAuB,GAAKK,EAAS,KAE3F3G,IAAY1H,IAAa5G,IACnC4G,EAAS/b,OAIPiiB,EAAiB,KAChBuI,EAAIzX,GAAqB8F,EAAS9F,GAAmB5B,YAAc4B,GAAmBoH,eAAiB,GAAKtB,EAChHkJ,EAAkByI,GAAKtJ,EAAmBtC,WAAa,EAAI,IAC3DqD,EAAgBuI,GAEjB9H,GAAkBA,GAAgB7J,EAAS9F,GAAmB5B,YAAc4B,GAAmBoH,eAAiB,MAGjHna,GAAKyN,OAAS,SAACpL,EAAOid,GAChBtf,GAAKsQ,UACTtQ,GAAKsQ,SAAU,EACftS,GAAamd,GAAU,SAAUjG,IACjChJ,IAAclO,GAAamd,GAAU,SAAU3c,IAC/C0lB,IAAiBlmB,GAAamF,cAAe,cAAe+gB,KAC9C,IAAV7hB,IACHrC,GAAKoQ,SAAWqU,GAAe,EAC/B3D,EAAUC,EAAUwD,GAAWnS,OAEpB,IAAZkN,GAAqBtf,GAAKsf,YAI5Btf,GAAKob,SAAW,SAAA9J,UAAQA,GAAQqP,EAAUA,EAAQpF,MAAQ6G,IAE1DpiB,GAAKggB,aAAe,SAACyK,EAAUC,EAAQC,EAAW/C,MAC7C7U,GAAoB,KACnByS,EAAKzS,GAAmByG,cAC3BrI,EAAW4B,GAAmB5B,WAC9BmQ,EAASkE,EAAG9L,IAAM8L,EAAG/L,MACtBgR,EAAWjF,EAAG/L,MAAQ6H,EAASmJ,EAAWtZ,EAC1CuZ,EAASlF,EAAG/L,MAAQ6H,EAASoJ,EAASvZ,EAEvCnR,GAAKsf,SAAQ,GAAO,EAAO,CAAC7F,MAAO/K,GAAW+b,EAAUE,KAAe3qB,GAAK+f,aAAcrG,IAAKhL,GAAWgc,EAAQC,KAAe3qB,GAAK6f,YAAa+H,GACnJ5nB,GAAK2B,UAGN3B,GAAK0f,iBAAmB,SAAAkL,MACnBtT,GAAesT,EAAQ,KACtB7pB,EAAIuW,EAAY1Z,QAAQ+T,GAAU/L,GAAK,EAC3C0R,EAAYvW,GAAMgL,WAAWuL,EAAYvW,IAAM6pB,EAAU3S,GACzDX,EAAY,GAAMvL,WAAWuL,EAAY,IAAMsT,EAAU3S,GACzDI,GAAUf,KAIZtX,GAAK2N,QAAU,SAACtL,EAAOwoB,MAClB7qB,GAAKsQ,WACE,IAAVjO,GAAmBrC,GAAK+N,QAAO,GAAM,GACrC/N,GAAKsQ,QAAUtQ,GAAK4lB,UAAW,EAC/BiF,GAAmBzI,IAAcA,GAAW7V,QAC5CiW,GAAa,EACb5B,IAAaA,EAAS5K,QAAU,GAChCkO,IAAiB5lB,GAAgB6E,cAAe,cAAe+gB,IAC3D3B,KACHA,GAAgBhW,QAChBoU,EAAQpF,OAASoF,EAAQpF,MAAMzN,SAAW6S,EAAQpF,MAAQ,KAEtDrP,IAAY,SACZnL,EAAIqV,GAAUhV,OACXL,QACFqV,GAAUrV,GAAGoa,WAAaA,IAAY/E,GAAUrV,KAAOf,UAI5D1B,GAAgB6c,GAAU,SAAUjG,IACpChJ,IAAc5N,GAAgB6c,GAAU,SAAU3c,MAKrDwB,GAAK8N,KAAO,SAACC,EAAQ8c,GACpB7qB,GAAK2N,QAAQI,EAAQ8c,GACrBzI,KAAeyI,GAAkBzI,GAAWtU,OAC5ClE,UAAciV,GAAKjV,OACf7I,EAAIqV,GAAUxY,QAAQoC,IACrB,GAALe,GAAUqV,GAAUpI,OAAOjN,EAAG,GAC9BA,IAAMoV,IAAmB,EAAbiK,IAAkBjK,KAG9BpV,EAAI,EACJqV,GAAUnE,QAAQ,SAAAlS,UAAKA,EAAEob,WAAanb,GAAKmb,WAAapa,EAAI,KAC5DA,GAAKwV,KAAmBvW,GAAK6Y,OAAOrC,IAAM,GAEtCtG,IACHA,EAAUsJ,cAAgB,KAC1BzL,GAAUmC,EAAUnC,OAAO,CAACD,MAAM,IAClC+c,GAAkB3a,EAAUpC,QAE7BkT,GAAe,CAACA,EAAaC,EAAWC,EAAoBC,GAAkBlP,QAAQ,SAAAiI,UAAKA,EAAE5B,YAAc4B,EAAE5B,WAAWzB,YAAYqD,KACpI4C,KAAa9c,KAAS8c,GAAW,GAC7B3F,KACHyJ,IAAaA,EAAS5K,QAAU,GAChCjV,EAAI,EACJqV,GAAUnE,QAAQ,SAAAlS,UAAKA,EAAEoX,MAAQA,IAAOpW,MACxCA,IAAM6f,EAASxJ,OAAS,IAEzBjR,EAAK2kB,QAAU3kB,EAAK2kB,OAAO9qB,KAG5BoW,GAAUnV,KAAKjB,IACfA,GAAKyN,QAAO,GAAO,GACnBkV,GAAsBA,EAAmB3iB,IAErCkQ,GAAaA,EAAUM,MAAQ8Q,EAAQ,KACtCyJ,EAAa/qB,GAAK2B,OACtB3B,GAAK2B,OAAS,WACb3B,GAAK2B,OAASopB,EACdpsB,GAAWC,QACX6a,GAASC,GAAO1Z,GAAKsf,WAEtBjiB,GAAKyP,YAAY,IAAM9M,GAAK2B,QAC5B2f,EAAS,IACT7H,EAAQC,EAAM,OAEd1Z,GAAKsf,UAENnI,IA7gCkB,SAAnB6T,sBACKnO,KAAoBoC,GAAY,KAC/BrV,EAAKiT,GAAkBoC,GAC3B9U,sBAAsB,kBAAMP,IAAOqV,IAAcvJ,IAAY,MA0gCvDsV,aAxqBDrpB,OAASsK,KAAKqT,QAAUrT,KAAK6B,KAAOgB,kBA4qBpCX,SAAP,kBAAgB/K,UACVS,IACJxG,GAAO+F,GAAQhG,KACf4R,MAAmB1R,OAAOwG,UAAYX,cAAcsK,SACpD5J,EAAemZ,IAETnZ,iBAGDiN,SAAP,kBAAgBzQ,MACXA,MACE,IAAImF,KAAKnF,EACb8d,GAAU3Y,GAAKnF,EAAOmF,UAGjB2Y,kBAGDxQ,QAAP,iBAAetL,EAAOyL,GACrBkP,GAAW,EACX5G,GAAUnE,QAAQ,SAAAiE,UAAWA,EAAQpI,EAAO,OAAS,WAAWzL,KAChE/D,GAAgBa,GAAM,QAASX,IAC/BF,GAAgBsC,GAAM,SAAUpC,IAChCysB,cAAc7O,GACd9d,GAAgBsC,GAAM,cAAekO,IACrCxQ,GAAgB2F,GAAO,aAAc6K,IACrCgD,GAAexT,GAAiBsC,GAAM,mCAAoC+N,IAC1EmD,GAAexT,GAAiBsC,GAAM,6BAA8BiO,IACpE2G,EAAa1H,OACb6B,GAAoBrR,QACf,IAAIyC,EAAI,EAAGA,EAAIpC,GAAWyC,OAAQL,GAAG,EACzCoR,GAAe7T,GAAiBK,GAAWoC,GAAIpC,GAAWoC,EAAE,IAC5DoR,GAAe7T,GAAiBK,GAAWoC,GAAIpC,GAAWoC,EAAE,mBAIvD0M,OAAP,qBACCtO,GAAO7B,OACPsD,GAAOkD,SACPhD,GAASF,GAAKoD,gBACdC,GAAQrD,GAAKmD,KACT1G,KACH2Z,GAAW3Z,GAAK8C,MAAMC,QACtB8b,GAAS7e,GAAK8C,MAAM+D,MACpBC,EAAW9G,GAAK+F,KAAKgB,SAAW0K,GAChC2N,GAAsBpf,GAAK+F,KAAK8nB,oBAAsBpc,GACtD2H,EAAqBtX,GAAKC,QAAQC,mBAAqB,OACvD8gB,EAAchhB,GAAK8G,aAAe,EAClC5I,GAAK+F,KAAKC,QAAQ,gBAAiBF,eAC/Bc,IAAO,CACV+Y,GAAW,GACXrG,EAAY7S,SAASuP,cAAc,QACzBY,MAAMzE,OAAS,QACzBmH,EAAU1C,MAAM2D,SAAW,WAC3BlB,KAxyCU,SAAbyU,oBAAmBnO,IAAY7S,sBAAsBghB,YAyyClDA,GACA5mB,EAAS4J,SAAS9Q,IAElB8F,cAAcqB,QAAUD,EAASC,QACjCkY,EAAanY,EAASC,SAAW,0BAA0B+V,KAAK5V,UAAUymB,WAC1E7V,EAA2C,IAArBhR,EAASC,QAC/BxG,GAAamB,GAAM,QAASX,IAC5BT,EAAQ,CAACoB,GAAMyB,GAAME,GAAQmD,IACzB5G,GAAKoH,YACRtB,cAAcsB,WAAa,SAAA0B,OAEzBX,EADG6lB,EAAKhuB,GAAKoH,iBAETe,KAAKW,EACTklB,EAAG7a,IAAIhL,EAAGW,EAAKX,WAET6lB,GAERhuB,GAAKgB,iBAAiB,iBAAkB,kBAAM4X,OAC9C5Y,GAAKgB,iBAAiB,mBAAoB,kBAAMsX,OAChDtY,GAAKgB,iBAAiB,aAAc,WACnCqX,GAAY,EAAG,GACfZ,EAAU,gBAEXzX,GAAKoH,aAAa+L,IAAI,0BAA2B,kBAChDuE,KACOA,MAGRxU,QAAQC,KAAK,iCAEduU,KACA/W,GAAa4C,GAAM,SAAUpC,QAK5Bmb,EAAQ5Y,EAJLuqB,EAAernB,GAAMsnB,aAAa,SACrCC,EAAYvnB,GAAMgQ,MAClBwX,EAASD,EAAUE,eACnBC,EAAiBtuB,GAAK+F,KAAKwoB,UAAUC,cAEtCF,EAAe5d,QAAU+d,OAAOC,eAAeJ,EAAgB,SAAU,CAAE1sB,MAAO,wBAAoBgN,KAAKoN,MAAM,KAAM,MACvHmS,EAAUE,eAAiB,QAC3B/R,EAASlK,GAAWxL,IACpBjD,GAAUkZ,EAAI1a,KAAKC,MAAMka,EAAOK,IAAMhZ,GAAUL,OAAS,EACzD4E,GAAY2U,EAAI1a,KAAKC,MAAMka,EAAOI,KAAOxU,GAAY5E,OAAS,EAC9D8qB,EAAUD,EAAUE,eAAiBD,EAAUD,EAAUvR,eAAe,oBACnEqR,IACJrnB,GAAM+P,aAAa,QAAS,IAC5B/P,GAAM+nB,gBAAgB,UAGvB5P,EAAgB6P,YAAYxX,GAAO,KACnCpX,GAAKyP,YAAY,GAAK,kBAAM5N,GAAW,IACvClB,GAAa4C,GAAM,cAAekO,IAClC9Q,GAAaiG,GAAO,aAAc6K,IAClCgD,GAAe9T,GAAc4C,GAAM,mCAAoC+N,IACvEmD,GAAe9T,GAAc4C,GAAM,6BAA8BiO,IACjEwN,EAAiBhf,GAAK8C,MAAM+rB,YAAY,aACxCzT,GAAYxX,KAAKob,GACjBxY,EAAehC,KACf2T,EAAenY,GAAKyP,YAAY,GAAK4I,IAAanJ,QAClDsD,EAAe,CAACjP,GAAM,mBAAoB,eACrCurB,EAAIhtB,GAAKoQ,WACZ6c,EAAIjtB,GAAKuM,YACN9K,GAAKyrB,QACR/P,EAAa6P,EACb5P,EAAc6P,GACJ9P,IAAe6P,GAAK5P,IAAgB6P,GAC9ClX,MAECtU,GAAM,mBAAoB8U,GAAavW,GAAM,OAAQuW,GAAavW,GAAM,SAAU+V,IACrFvF,GAAoB3R,IACpBoY,GAAUnE,QAAQ,SAAAiE,UAAWA,EAAQzI,OAAO,EAAG,KAC1C1M,EAAI,EAAGA,EAAIpC,GAAWyC,OAAQL,GAAG,EACrCoR,GAAe7T,GAAiBK,GAAWoC,GAAIpC,GAAWoC,EAAE,IAC5DoR,GAAe7T,GAAiBK,GAAWoC,GAAIpC,GAAWoC,EAAE,oBAMzDV,OAAP,gBAAc8F,sBACQA,IAAUyW,KAAoBzW,EAAKmmB,oBACpDC,EAAKpmB,EAAKqmB,aACdD,GAAMtB,cAAc7O,KAAoBA,EAAgBmQ,IAAON,YAAYxX,GAAO8X,0BACzDpmB,IAAUoP,EAAgD,IAA1BpS,cAAcqB,SAAiB2B,EAAKsmB,oBACzF,sBAAuBtmB,IAC1BwJ,GAAoBrR,KAAoBqR,GAAoB3R,GAAcmI,EAAKumB,mBAAqB,QACpGtX,GAAqE,KAApDjP,EAAKumB,kBAAoB,IAAI9uB,QAAQ,0BAIjD+uB,cAAP,uBAAqBtrB,EAAQ8E,OACxBpG,EAAID,EAAWuB,GAClBN,EAAIpC,GAAWf,QAAQmC,GACvBmM,EAAarO,GAAYkC,IACrBgB,GACJpC,GAAWqP,OAAOjN,EAAGmL,EAAa,EAAI,GAEnC/F,IACH+F,EAAavO,GAASqrB,QAAQ7pB,GAAMgH,EAAMlC,GAAOkC,EAAMrF,GAAQqF,GAAQxI,GAASqrB,QAAQjpB,EAAGoG,mBAItFymB,gBAAP,yBAAuB9W,GACtBM,GAAUnE,QAAQ,SAAAlS,UAAKA,EAAEE,MAAQF,EAAEE,KAAK6V,QAAUA,GAAS/V,EAAEE,KAAK6N,MAAK,GAAM,oBAGvE+e,aAAP,sBAAoBpvB,EAASqe,EAAO0D,OAC/B7F,GAAUnL,GAAU/Q,GAAWqC,EAAWrC,GAAWA,GAASwgB,wBACjEpe,EAAS8Z,EAAO6F,EAAaxH,GAASE,IAAW4D,GAAS,SACpD0D,EAAqC,EAAxB7F,EAAO6E,MAAQ3e,GAAc8Z,EAAOI,KAAOla,EAASV,GAAKoQ,WAAsC,EAAzBoK,EAAO4E,OAAS1e,GAAc8Z,EAAOK,IAAMna,EAASV,GAAKuM,2BAG7IohB,mBAAP,4BAA0BrvB,EAASsvB,EAAgBvN,GAClDhR,GAAU/Q,KAAaA,EAAUqC,EAAWrC,QACxCkc,EAASlc,EAAQwgB,wBACpB1L,EAAOoH,EAAO6F,EAAaxH,GAASE,IACpCrY,EAA2B,MAAlBktB,EAAyBxa,EAAO,EAAMwa,KAAkBpa,EAAaA,EAAUoa,GAAkBxa,GAAQwa,EAAenvB,QAAQ,KAAOmO,WAAWghB,GAAkBxa,EAAO,IAAMxG,WAAWghB,IAAmB,SAClNvN,GAAc7F,EAAOI,KAAOla,GAAUV,GAAKoQ,YAAcoK,EAAOK,IAAMna,GAAUV,GAAKuM,2BAGtFshB,QAAP,iBAAeC,MACd7W,GAAU/H,MAAM,GAAG4D,QAAQ,SAAAlS,SAAmB,mBAAdA,EAAEoG,KAAKyD,IAA2B7J,EAAE+N,UAC7C,IAAnBmf,EAAyB,KACxBC,EAAYpO,EAAWkO,SAAW,GACtClO,EAAa,GACboO,EAAUjb,QAAQ,SAAAnT,UAAKA,8CAz2BbqH,EAAM+J,GACjBrM,GAAgBV,cAAcgL,SAAS9Q,KAASkD,QAAQC,KAAK,6CAC7D2D,EAAS8H,WACJ/F,KAAKC,EAAM+J,MA42BJjC,QAAU,YACVkf,WAAa,SAAA5G,UAAWA,EAAUvP,GAASuP,GAAStU,QAAQ,SAAA5Q,MACrEA,GAAUA,EAAO4S,MAAO,KACvBlT,EAAI8U,EAAajY,QAAQyD,GACxB,GAALN,GAAU8U,EAAa7H,OAAOjN,EAAG,GACjC8U,EAAa5U,KAAKI,EAAQA,EAAO4S,MAAMC,QAAS7S,EAAO0U,SAAW1U,EAAO+rB,aAAa,aAAc/vB,GAAK+F,KAAKoX,SAASnZ,GAAS8C,QAE7H0R,MACS9H,OAAS,SAAC4Z,EAAM/R,UAAUK,IAAY0R,EAAM/R,OAC5C1H,OAAS,SAAC/H,EAAM+J,UAAc,IAAI/M,GAAcgD,EAAM+J,OACtDoP,QAAU,SAAA+N,UAAQA,EAAOnY,IAAU,IAASrR,GAAgBV,GAAcgL,aAAeuH,IAAY,OACrG/T,OAAS,SAAAC,WAAWjD,GAAWC,OAASgW,GAAqB,IAAVhT,EAAiB,EAAI,OACxE0rB,kBAAoBhX,MACpBiX,UAAY,SAAC9vB,EAAS+hB,UAAe9P,GAAWjS,EAAS+hB,EAAaja,GAAcvE,QACpFwsB,cAAgB,SAAC/vB,EAAS+hB,UAAe/e,EAAeX,EAAWrC,GAAU+hB,EAAaja,GAAcvE,QACxGsN,QAAU,SAAA1E,UAAMiV,GAAKjV,OACrBwE,OAAS,kBAAMgI,GAAUxI,OAAO,SAAA7N,SAAmB,mBAAdA,EAAEoG,KAAKyD,SAC5C6jB,YAAc,mBAAQ/Y,OACtBgZ,gBAAkBtc,MAClB/S,iBAAmB,SAACJ,EAAM+T,OACnChP,EAAI8b,EAAW7gB,KAAU6gB,EAAW7gB,GAAQ,KAC/C+E,EAAEpF,QAAQoU,IAAahP,EAAE/B,KAAK+Q,OAElBzT,oBAAsB,SAACN,EAAM+T,OACtChP,EAAI8b,EAAW7gB,GAClB8C,EAAIiC,GAAKA,EAAEpF,QAAQoU,GACf,GAALjR,GAAUiC,EAAEgL,OAAOjN,EAAG,OAET4sB,MAAQ,SAACpH,EAASpgB,GAKd,SAAhBynB,GAAiB3vB,EAAM+T,OAClB6b,EAAW,GACdC,EAAW,GACXpI,EAAQroB,GAAKyP,YAAYihB,EAAU,WAAO/b,EAAS6b,EAAUC,GAAWD,EAAW,GAAIC,EAAW,KAAMvhB,eAClG,SAAAvM,GACN6tB,EAASzsB,QAAUskB,EAAMpa,SAAQ,GACjCuiB,EAAS5sB,KAAKjB,EAAKkW,SACnB4X,EAAS7sB,KAAKjB,GACdguB,GAAYH,EAASzsB,QAAUskB,EAAMtV,SAAS,QAGhD5K,EAfG+K,EAAS,GACZ0d,EAAW,GACXF,EAAW5nB,EAAK4nB,UAAY,KAC5BC,EAAW7nB,EAAK6nB,UAAY,QAaxBxoB,KAAKW,EACT8nB,EAASzoB,GAAyB,OAAnBA,EAAEiJ,OAAO,EAAG,IAAeqB,GAAY3J,EAAKX,KAAa,kBAANA,EAAyBooB,GAAcpoB,EAAGW,EAAKX,IAAMW,EAAKX,UAEzHsK,GAAYke,KACfA,EAAWA,IACXhwB,GAAamF,GAAe,UAAW,kBAAM6qB,EAAW7nB,EAAK6nB,cAE9DhX,GAASuP,GAAStU,QAAQ,SAAA5Q,OACrBhB,EAAS,OACRmF,KAAKyoB,EACT5tB,EAAOmF,GAAKyoB,EAASzoB,GAEtBnF,EAAO6V,QAAU7U,EACjBkP,EAAOtP,KAAKkC,GAAc+K,OAAO7N,MAE3BkQ,GAKmC,SAAvC2d,GAAwC9b,EAAY2I,EAASrB,EAAKtX,UAC1DA,EAAV2Y,EAAgB3I,EAAWhQ,GAAO2Y,EAAU,GAAK3I,EAAW,GAC/ChQ,EAANsX,GAAatX,EAAM2Y,IAAYrB,EAAMqB,GAAWrB,EAAM,EAAIqB,GAAWA,EAAUrB,GAAO,EAExE,SAAtByU,GAAuB9sB,EAAQsQ,IACZ,IAAdA,EACHtQ,EAAO4S,MAAMgG,eAAe,gBAE5B5Y,EAAO4S,MAAMma,aAA4B,IAAdzc,EAAqB,OAASA,EAAY,OAASA,GAAapN,EAASC,QAAU,cAAgB,IAAM,OAErInD,IAAWP,IAAUqtB,GAAoBlqB,GAAO0N,GAGjC,SAAhB0c,UAGqBhX,EAHHzQ,IAAAA,MAAOvF,IAAAA,OAAQgJ,IAAAA,KAC5BikB,GAAQ1nB,EAAM9D,eAAiB8D,EAAM9D,eAAe,GAAK8D,GAAOvF,OACnEzC,EAAQ0vB,EAAK/W,OAASla,GAAK+F,KAAKoX,SAAS8T,GACzCjV,EAAOxX,SACHjD,EAAM2vB,YAAwC,IAA1BlV,EAAOza,EAAM2vB,WAAmB,MACjDD,GAAQA,IAASrqB,KAAWqqB,EAAKE,cAAgBF,EAAKG,cAAgBH,EAAKI,aAAeJ,EAAKzZ,cAAkB8Z,IAAWtX,EAAK1G,GAAkB2d,IAAOM,aAAcD,GAAUtX,EAAGwX,aAAcP,EAAOA,EAAKhW,WACtN1Z,EAAMkwB,UAAYR,GAAQA,IAASjtB,IAAWxD,GAAYywB,KAAUK,IAAWtX,EAAK1G,GAAkB2d,IAAOM,YAAcD,GAAUtX,EAAGwX,YACxIjwB,EAAM2vB,WAAalV,GAEhBza,EAAMkwB,WAAsB,MAATzkB,IACtBzD,EAAMmoB,kBACNnoB,EAAM/D,YAAa,GAIJ,SAAjBmsB,GAAkB3tB,EAAQpD,EAAMgxB,EAAQC,UAAW3qB,EAAS2J,OAAO,CAClE7M,OAAQA,EACRjD,SAAS,EACTmI,UAAU,EACViC,UAAU,EACVvK,KAAMA,EACNiK,QAAUgnB,EAASA,GAAUb,GAC7BrnB,QAASkoB,EACTnoB,OAAQmoB,EACRlkB,SAAUkkB,EACV/mB,SAAU,2BAAM8mB,GAAUjxB,GAAa4C,GAAM2D,EAASQ,WAAW,GAAIoqB,IAAgB,GAAO,IAC5F/mB,UAAW,4BAAM9J,GAAgBsC,GAAM2D,EAASQ,WAAW,GAAIoqB,IAAgB,MAWzD,SAAvBC,GAAuBjpB,GAoBH,SAAlBkpB,YAAwBC,GAAgB,EAGzB,SAAfC,KACCC,EAAO9f,GAAWrO,EAAQL,IAC1ByuB,EAAevT,GAAOQ,EAAa,EAAI,EAAG8S,GAC1CE,IAAqBC,EAAezT,GAAO,EAAGxM,GAAWrO,EAAQkE,MACjEqqB,EAAgB3Q,GAEK,SAAtB4Q,KACC/I,EAAQvP,MAAMxN,EAAIgF,GAAOhD,WAAW+a,EAAQvP,MAAMxN,GAAKmB,EAAYrL,QAAU,KAC7EinB,EAAQ7S,MAAM6b,UAAY,mDAAqD/jB,WAAW+a,EAAQvP,MAAMxN,GAAK,UAC7GmB,EAAYrL,OAASqL,EAAYvL,QAAU,EAqBjC,SAAXowB,KACCR,KACIhU,EAAMqK,YAAcrK,EAAMpV,KAAKiF,QAAUokB,IAC5CtkB,IAAgBskB,EAAOjU,EAAMnL,SAAS,IAAMlF,EAAYskB,GAAQjU,EAAM4K,QAAQ,UAAWqJ,IAvD5Fxf,GAAU7J,KAAUA,EAAO,IAC3BA,EAAKvD,eAAiBuD,EAAK4B,aAAe5B,EAAKoC,aAAc,EAC7DpC,EAAKlI,OAASkI,EAAKlI,KAAO,eAC1BkI,EAAKI,WAAaJ,EAAKI,SACvBJ,EAAKyD,GAAKzD,EAAKyD,IAAM,iBAEpB5J,EAAMwvB,EAWNI,EAAeN,EAkCf/T,EAAOyU,EAAcC,EAAc5kB,EA9C/BqkB,EAA4DvpB,EAA5DupB,iBAAkBQ,EAA0C/pB,EAA1C+pB,SAAUC,EAAgChqB,EAAhCgqB,kBAAmBlpB,EAAad,EAAbc,UAEnD5F,EAASvB,EAAWqG,EAAK9E,SAAWP,GACpCsvB,EAAW/yB,GAAK+F,KAAKC,UAAUgtB,eAC/BC,EAAmBF,GAAYA,EAASG,MACxCzJ,EAAUpK,IAAgBvW,EAAK2gB,SAAWhnB,EAAWqG,EAAK2gB,UAAcwJ,IAAqC,IAAjBnqB,EAAK2gB,UAAsBwJ,EAAiBhvB,UAAYgvB,EAAiBxJ,WACrK5b,EAAczK,EAAeY,EAAQL,IACrCiK,EAAcxK,EAAeY,EAAQkE,IACrCuY,EAAQ,EACR0S,GAAgBjsB,EAASC,SAAWrF,GAAKsxB,eAAiBtxB,GAAKsxB,eAAe3S,MAAQ3e,GAAKsxB,eAAenhB,MAAQnQ,GAAKuxB,YAAcvxB,GAAKoQ,WAC1IohB,EAAe,EACfC,EAA0B9gB,GAAYogB,GAAY,kBAAMA,EAASlwB,IAAQ,kBAAMkwB,GAAY,KAE3FW,EAAgB7B,GAAe3tB,EAAQ8E,EAAKlI,MAAM,EAAMkyB,GAExDR,EAAe7gB,GACf2gB,EAAe3gB,UAqChBgY,GAAWzpB,GAAK4d,IAAI6L,EAAS,CAAC/c,EAAG,QACjC5D,EAAK2B,YAAc,SAAAnF,UAAM+Z,GAAyB,cAAX/Z,EAAE1E,MA1B3B,SAAb6yB,gBACKxB,EAAe,CAClBnlB,sBAAsBklB,QAClBxvB,EAASkP,GAAO/O,EAAKmJ,OAAS,GACjC0P,EAAS4W,EAAavkB,EAAY3L,EAAIM,MACnCinB,GAAWjO,IAAW3N,EAAY3L,EAAI2L,EAAYrL,OAAQ,CAC7DqL,EAAYrL,OAASgZ,EAAS3N,EAAY3L,MACtCwK,EAAIgF,IAAQhD,WAAW+a,GAAWA,EAAQvP,MAAMxN,IAAM,GAAKmB,EAAYrL,QAC3EinB,EAAQ7S,MAAM6b,UAAY,mDAAqD/lB,EAAI,UACnF+c,EAAQvP,MAAMxN,EAAIA,EAAI,KACtBmB,EAAYvL,QAAUhB,GAAWC,MACjCgW,WAEM,EAER1J,EAAYrL,QAAUgwB,KACtBP,GAAgB,EAU+CwB,IAA2B,KAARhT,GAA2B,eAAXnb,EAAE1E,MAA0B+B,EAAK8K,aAAgBnI,EAAEkI,SAA8B,EAAnBlI,EAAEkI,QAAQzJ,QAC5K+E,EAAKa,QAAU,WACdsoB,GAAgB,MACZyB,EAAYjT,EAChBA,EAAQ/O,IAAS5P,GAAKsxB,gBAAkBtxB,GAAKsxB,eAAe3S,OAAU,GAAK0S,GAC3EjV,EAAMhP,QACNwkB,IAAcjT,GAASqQ,GAAoB9sB,EAAgB,KAARyc,IAAsB4R,GAA2B,KACpGM,EAAe/kB,IACfglB,EAAe/kB,IACfqkB,KACAK,EAAgB3Q,IAEjB9Y,EAAKc,UAAYd,EAAK6B,eAAiB,SAAChI,EAAM2M,MAC7CzB,EAAYrL,QAAUgwB,KACjBljB,EAEE,CACNhO,GAAWC,YAGVoyB,EAAelL,EADZmL,EAAML,IAENlB,IAEH5J,GADAkL,EAAgB/lB,KACmB,IAANgmB,GAAcjxB,EAAKkxB,UAAa,KAC7DD,GAAO/C,GAAqCjjB,EAAa+lB,EAAelL,EAAWpW,GAAWrO,EAAQkE,KACtGgW,EAAMpV,KAAKgF,QAAUwkB,EAAa7J,IAGnCA,GADAkL,EAAgB9lB,KACmB,IAAN+lB,GAAcjxB,EAAKmxB,UAAa,KAC7DF,GAAO/C,GAAqChjB,EAAa8lB,EAAelL,EAAWpW,GAAWrO,EAAQL,KACtGua,EAAMpV,KAAKiF,QAAUqkB,EAAa3J,GAClCvK,EAAMsM,aAAa1W,SAAS8f,GAAKG,KAAK,MAClC1U,GAAcnB,EAAMpV,KAAKiF,SAAWokB,GAAyBA,EAAK,GAAtBwB,IAC/C3zB,GAAK4e,GAAG,GAAI,CAACF,SAAUgU,GAAU5e,SAAU8f,SAlB5C5lB,EAAkBC,SAAQ,GAqB3BrE,GAAaA,EAAUjH,IAExBmG,EAAK+B,QAAU,WACdqT,EAAM8V,KAAO9V,EAAMhP,QACa,IAA5B1K,KAAa8uB,IAChBf,EAAgB,EAChBe,EAAe9uB,OAGjBsE,EAAKqB,SAAW,SAACxH,EAAMgJ,EAAIE,EAAIooB,EAAQC,MACtCtS,KAAe2Q,GAAiBL,KAChCvmB,GAAM0mB,GAAoBzkB,EAAY0kB,EAAa2B,EAAO,KAAOtoB,EAAKgnB,GAAgBhwB,EAAK0K,OAAS1K,EAAK8J,GAAKmB,IAAgBjC,EAAKsoB,EAAO,KACtIpoB,EAAI,CACPgC,EAAYrL,QAAUgwB,SAClBrrB,EAAU+sB,EAAO,KAAOroB,EAC3Ba,EAAIvF,EAAUyrB,EAAejwB,EAAK2K,OAAS3K,EAAK+J,EAAImB,IAAgBhC,EAAKqoB,EAAO,GAChFC,EAAW/B,EAAa1lB,GACzBvF,GAAWuF,IAAMynB,IAAavB,GAAgBuB,EAAWznB,GACzDmB,EAAYsmB,IAEZtoB,GAAMF,IAAO4L,KAEfzO,EAAKgC,SAAW,WACfgmB,GAAoB9sB,GAAQquB,GAA2B,KACvDvsB,GAAc9E,iBAAiB,UAAW0xB,IAC1C/xB,GAAamB,GAAM,SAAU4wB,IACzB7kB,EAAY5J,SACf4J,EAAY7J,OAAO4S,MAAMoL,eAAiB,OAC1CnU,EAAY5J,OAAS2J,EAAY3J,QAAS,GAE3CuvB,EAAcpjB,UAEftH,EAAKiC,UAAY,WAChB+lB,GAAoB9sB,GAAQ,GAC5B/C,GAAgBa,GAAM,SAAU4wB,IAChC5sB,GAAc5E,oBAAoB,UAAWwxB,IAC7Cc,EAAc/iB,QAEf3H,EAAKqC,UAA6B,IAAlBrC,EAAKqC,WACrBxI,EAAO,IAAIuE,EAAS4B,IACfzG,IAAMgd,KACIxR,KAAiBA,EAAY,GAC5CwR,GAAcrf,GAAKo0B,OAAOjhB,IAAI1B,IAC9BzD,EAAoBrL,EAAKuN,IACzBgO,EAAQle,GAAK4e,GAAGjc,EAAM,CAAC8kB,KAAM,SAAUE,QAAQ,EAAMnJ,SAAS,EAAO1Q,QAASukB,EAAmB,QAAU,MAAOtkB,QAAS,QAASqQ,UAAW,CAACrQ,QAASqP,GAAqBvP,EAAaA,IAAe,kBAAMqQ,EAAMhP,WAAYwP,SAAUnH,EAAY4G,WAAYnQ,EAAkBlF,KAAKqV,aACpRxb,EA/LT,IA0CC0xB,GA9BA/C,GAAY,CAACgD,KAAM,EAAG9Y,OAAQ,GA6B9B+Y,GAAY,iCAEZzC,GAAiB,SAAjBA,eAAiBxsB,OACZkvB,EAAUD,GAAUrX,KAAK5X,EAAEtB,OAAOywB,UAClCD,GAAWH,MACd/uB,EAAEE,YAAa,EACf6uB,GAAkBG,OAmJPpgB,KAAO,SAAAvT,MAChB4R,GAAY5R,UACRkY,GAAU3E,KAAKvT,OAEnB2a,EAAS1Z,GAAK8G,aAAe,SACjC9C,GAAciL,SAAS6D,QAAQ,SAAAlS,UAAKA,EAAEgyB,OAAShyB,EAAEmW,QAAU2C,EAAS9Y,EAAEmW,QAAQ+H,wBAAwBjE,IAAMja,EAAE0Z,MAAQta,GAAKuM,cACpH0K,GAAU3E,KAAKvT,GAAS,SAAC8E,EAAG0O,UAAuC,KAAhC1O,EAAEmD,KAAKwe,iBAAmB,IAAa3hB,EAAEmD,KAAK4M,mBAAqB,IAAM/P,EAAE+uB,UAAYrgB,EAAEvL,KAAK4M,mBAAqB,IAAMrB,EAAEqgB,SAA2C,KAAhCrgB,EAAEvL,KAAKwe,iBAAmB,UAE7LqN,QAAU,SAAA7rB,UAAQ,IAAI5B,EAAS4B,OAC/B8rB,gBAAkB,SAAA9rB,WACV,IAAVA,SACH1H,MAEK,IAAT0H,GAAiB1H,SACbA,EAAYgP,aAEP,IAATtH,SACH1H,GAAeA,EAAYqP,YAC3BrP,EAAc0H,OAGX+rB,EAAa/rB,aAAgB5B,EAAW4B,EAAOipB,GAAqBjpB,UACxE1H,GAAeA,EAAY4C,SAAW6wB,EAAW7wB,QAAU5C,EAAYqP,OACvEjQ,GAAYq0B,EAAW7wB,UAAY5C,EAAcyzB,GAC1CA,MAIM9uB,KAAO,CACpB5B,iBAAAA,EACAwtB,eAAAA,GACArwB,WAAAA,GACAhB,SAAAA,GACA6F,OAAQ,CAEP2uB,GAAI,cACHzd,IAAmBI,EAAU,eAC7BJ,GAAkB7S,MAGnBuwB,IAAK,sBAAMjd,YAIC9X,GAAKE,eAAe4F"} \ No newline at end of file diff --git a/node_modules/gsap/dist/TextPlugin.js b/node_modules/gsap/dist/TextPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..4ae6f993ae647ca48fdcc54da1e03ff491e48d0f --- /dev/null +++ b/node_modules/gsap/dist/TextPlugin.js @@ -0,0 +1,256 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + var _trimExp = /(?:^\s+|\s+$)/g; + var emojiExp = /([\uD800-\uDBFF][\uDC00-\uDFFF](?:[\u200D\uFE0F][\uD800-\uDBFF][\uDC00-\uDFFF]){2,}|\uD83D\uDC69(?:\u200D(?:(?:\uD83D\uDC69\u200D)?\uD83D\uDC67|(?:\uD83D\uDC69\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2642\u2640]\uFE0F|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDD27\uDCBC\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC6F\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3C-\uDD3E\uDDD6-\uDDDF])\u200D[\u2640\u2642]\uFE0F|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|(?:\u26F9|\uD83C[\uDFCC\uDFCB]|\uD83D\uDD75)(?:\uFE0F\u200D[\u2640\u2642]|(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642])\uFE0F|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\uD83D\uDC69\u200D[\u2695\u2696\u2708]|\uD83D\uDC68(?:(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708]))\uFE0F|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83D\uDC69\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|[#\*0-9]\uFE0F\u20E3|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|\uD83D\uDC68(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]))|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uD83C[\uDFFB-\uDFFF])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270A-\u270D]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC70\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDCAA\uDD74\uDD7A\uDD90\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD36\uDDD1-\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\u200D(?:(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC69\uDC6E\uDC70-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD26\uDD30-\uDD39\uDD3D\uDD3E\uDDD1-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])?|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDEEB\uDEEC\uDEF4-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u2660\u2663\u2665\u2666\u2668\u267B\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])\uFE0F)/; + function getText(e) { + var type = e.nodeType, + result = ""; + + if (type === 1 || type === 9 || type === 11) { + if (typeof e.textContent === "string") { + return e.textContent; + } else { + for (e = e.firstChild; e; e = e.nextSibling) { + result += getText(e); + } + } + } else if (type === 3 || type === 4) { + return e.nodeValue; + } + + return result; + } + function splitInnerHTML(element, delimiter, trim, preserveSpaces, unescapedCharCodes) { + var node = element.firstChild, + result = [], + s; + + while (node) { + if (node.nodeType === 3) { + s = (node.nodeValue + "").replace(/^\n+/g, ""); + + if (!preserveSpaces) { + s = s.replace(/\s+/g, " "); + } + + result.push.apply(result, emojiSafeSplit(s, delimiter, trim, preserveSpaces, unescapedCharCodes)); + } else if ((node.nodeName + "").toLowerCase() === "br") { + result[result.length - 1] += "<br>"; + } else { + result.push(node.outerHTML); + } + + node = node.nextSibling; + } + + if (!unescapedCharCodes) { + s = result.length; + + while (s--) { + result[s] === "&" && result.splice(s, 1, "&"); + } + } + + return result; + } + function emojiSafeSplit(text, delimiter, trim, preserveSpaces, unescapedCharCodes) { + text += ""; + trim && (text = text.trim ? text.trim() : text.replace(_trimExp, "")); + + if (delimiter && delimiter !== "") { + return text.replace(/>/g, ">").replace(/</g, "<").split(delimiter); + } + + var result = [], + l = text.length, + i = 0, + j, + character; + + for (; i < l; i++) { + character = text.charAt(i); + + if (character.charCodeAt(0) >= 0xD800 && character.charCodeAt(0) <= 0xDBFF || text.charCodeAt(i + 1) >= 0xFE00 && text.charCodeAt(i + 1) <= 0xFE0F) { + j = ((text.substr(i, 12).split(emojiExp) || [])[1] || "").length || 2; + character = text.substr(i, j); + result.emoji = 1; + i += j - 1; + } + + result.push(unescapedCharCodes ? character : character === ">" ? ">" : character === "<" ? "<" : preserveSpaces && character === " " && (text.charAt(i - 1) === " " || text.charAt(i + 1) === " ") ? " " : character); + } + + return result; + } + + /*! + * TextPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var gsap, + _tempDiv, + _getGSAP = function _getGSAP() { + return gsap || typeof window !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap; + }; + + var TextPlugin = { + version: "3.12.7", + name: "text", + init: function init(target, value, tween) { + typeof value !== "object" && (value = { + value: value + }); + + var i = target.nodeName.toUpperCase(), + data = this, + _value = value, + newClass = _value.newClass, + oldClass = _value.oldClass, + preserveSpaces = _value.preserveSpaces, + rtl = _value.rtl, + delimiter = data.delimiter = value.delimiter || "", + fillChar = data.fillChar = value.fillChar || (value.padSpace ? " " : ""), + _short, + text, + original, + j, + condensedText, + condensedOriginal, + aggregate, + s; + + data.svg = target.getBBox && (i === "TEXT" || i === "TSPAN"); + + if (!("innerHTML" in target) && !data.svg) { + return false; + } + + data.target = target; + + if (!("value" in value)) { + data.text = data.original = [""]; + return; + } + + original = splitInnerHTML(target, delimiter, false, preserveSpaces, data.svg); + _tempDiv || (_tempDiv = document.createElement("div")); + _tempDiv.innerHTML = value.value; + text = splitInnerHTML(_tempDiv, delimiter, false, preserveSpaces, data.svg); + data.from = tween._from; + + if ((data.from || rtl) && !(rtl && data.from)) { + i = original; + original = text; + text = i; + } + + data.hasClass = !!(newClass || oldClass); + data.newClass = rtl ? oldClass : newClass; + data.oldClass = rtl ? newClass : oldClass; + i = original.length - text.length; + _short = i < 0 ? original : text; + + if (i < 0) { + i = -i; + } + + while (--i > -1) { + _short.push(fillChar); + } + + if (value.type === "diff") { + j = 0; + condensedText = []; + condensedOriginal = []; + aggregate = ""; + + for (i = 0; i < text.length; i++) { + s = text[i]; + + if (s === original[i]) { + aggregate += s; + } else { + condensedText[j] = aggregate + s; + condensedOriginal[j++] = aggregate + original[i]; + aggregate = ""; + } + } + + text = condensedText; + original = condensedOriginal; + + if (aggregate) { + text.push(aggregate); + original.push(aggregate); + } + } + + value.speed && tween.duration(Math.min(0.05 / value.speed * _short.length, value.maxDuration || 9999)); + data.rtl = rtl; + data.original = original; + data.text = text; + + data._props.push("text"); + }, + render: function render(ratio, data) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + + if (data.from) { + ratio = 1 - ratio; + } + + var text = data.text, + hasClass = data.hasClass, + newClass = data.newClass, + oldClass = data.oldClass, + delimiter = data.delimiter, + target = data.target, + fillChar = data.fillChar, + original = data.original, + rtl = data.rtl, + l = text.length, + i = (rtl ? 1 - ratio : ratio) * l + 0.5 | 0, + applyNew, + applyOld, + str; + + if (hasClass && ratio) { + applyNew = newClass && i; + applyOld = oldClass && i !== l; + str = (applyNew ? "<span class='" + newClass + "'>" : "") + text.slice(0, i).join(delimiter) + (applyNew ? "</span>" : "") + (applyOld ? "<span class='" + oldClass + "'>" : "") + delimiter + original.slice(i).join(delimiter) + (applyOld ? "</span>" : ""); + } else { + str = text.slice(0, i).join(delimiter) + delimiter + original.slice(i).join(delimiter); + } + + if (data.svg) { + target.textContent = str; + } else { + target.innerHTML = fillChar === " " && ~str.indexOf(" ") ? str.split(" ").join(" ") : str; + } + } + }; + TextPlugin.splitInnerHTML = splitInnerHTML; + TextPlugin.emojiSafeSplit = emojiSafeSplit; + TextPlugin.getText = getText; + _getGSAP() && gsap.registerPlugin(TextPlugin); + + exports.TextPlugin = TextPlugin; + exports.default = TextPlugin; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/TextPlugin.min.js b/node_modules/gsap/dist/TextPlugin.min.js new file mode 100644 index 0000000000000000000000000000000000000000..b13dc477b38d0defc34cbdf1204fff415fc054c8 --- /dev/null +++ b/node_modules/gsap/dist/TextPlugin.min.js @@ -0,0 +1,11 @@ +/*! + * TextPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(D,u){"object"==typeof exports&&"undefined"!=typeof module?u(exports):"function"==typeof define&&define.amd?define(["exports"],u):u((D=D||self).window=D.window||{})}(this,function(D){"use strict";var r=/(?:^\s+|\s+$)/g,A=/([\uD800-\uDBFF][\uDC00-\uDFFF](?:[\u200D\uFE0F][\uD800-\uDBFF][\uDC00-\uDFFF]){2,}|\uD83D\uDC69(?:\u200D(?:(?:\uD83D\uDC69\u200D)?\uD83D\uDC67|(?:\uD83D\uDC69\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2642\u2640]\uFE0F|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDD27\uDCBC\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC6F\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3C-\uDD3E\uDDD6-\uDDDF])\u200D[\u2640\u2642]\uFE0F|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|(?:\u26F9|\uD83C[\uDFCC\uDFCB]|\uD83D\uDD75)(?:\uFE0F\u200D[\u2640\u2642]|(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642])\uFE0F|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\uD83D\uDC69\u200D[\u2695\u2696\u2708]|\uD83D\uDC68(?:(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708]))\uFE0F|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83D\uDC69\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|[#\*0-9]\uFE0F\u20E3|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|\uD83D\uDC68(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]))|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uD83C[\uDFFB-\uDFFF])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270A-\u270D]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC70\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDCAA\uDD74\uDD7A\uDD90\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD36\uDDD1-\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\u200D(?:(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC69\uDC6E\uDC70-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD26\uDD30-\uDD39\uDD3D\uDD3E\uDDD1-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])?|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDEEB\uDEEC\uDEF4-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u2660\u2663\u2665\u2666\u2668\u267B\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])\uFE0F)/;function splitInnerHTML(D,u,F,C,E){for(var e,t=D.firstChild,n=[];t;)3===t.nodeType?(e=(t.nodeValue+"").replace(/^\n+/g,""),C||(e=e.replace(/\s+/g," ")),n.push.apply(n,emojiSafeSplit(e,u,F,C,E))):"br"===(t.nodeName+"").toLowerCase()?n[n.length-1]+="<br>":n.push(t.outerHTML),t=t.nextSibling;if(!E)for(e=n.length;e--;)"&"===n[e]&&n.splice(e,1,"&");return n}function emojiSafeSplit(D,u,F,C,E){if(D+="",F&&(D=D.trim?D.trim():D.replace(r,"")),u&&""!==u)return D.replace(/>/g,">").replace(/</g,"<").split(u);for(var e,t,n=[],B=D.length,i=0;i<B;i++)(55296<=(t=D.charAt(i)).charCodeAt(0)&&t.charCodeAt(0)<=56319||65024<=D.charCodeAt(i+1)&&D.charCodeAt(i+1)<=65039)&&(e=((D.substr(i,12).split(A)||[])[1]||"").length||2,t=D.substr(i,e),i+=e-(n.emoji=1)),n.push(E?t:">"===t?">":"<"===t?"<":!C||" "!==t||" "!==D.charAt(i-1)&&" "!==D.charAt(i+1)?t:" ");return n}var u,g,F={version:"3.12.7",name:"text",init:function init(D,u,F){"object"!=typeof u&&(u={value:u});var C,E,e,t,n,B,i,r,A=D.nodeName.toUpperCase(),s=this,l=u.newClass,o=u.oldClass,a=u.preserveSpaces,p=u.rtl,f=s.delimiter=u.delimiter||"",d=s.fillChar=u.fillChar||(u.padSpace?" ":"");if(s.svg=D.getBBox&&("TEXT"===A||"TSPAN"===A),!("innerHTML"in D||s.svg))return!1;if(s.target=D,"value"in u){for(e=splitInnerHTML(D,f,!1,a,s.svg),(g=g||document.createElement("div")).innerHTML=u.value,E=splitInnerHTML(g,f,!1,a,s.svg),s.from=F._from,!s.from&&!p||p&&s.from||(A=e,e=E,E=A),s.hasClass=!(!l&&!o),s.newClass=p?o:l,s.oldClass=p?l:o,C=(A=e.length-E.length)<0?e:E,A<0&&(A=-A);-1<--A;)C.push(d);if("diff"===u.type){for(n=[],B=[],i="",A=t=0;A<E.length;A++)(r=E[A])===e[A]?i+=r:(n[t]=i+r,B[t++]=i+e[A],i="");E=n,e=B,i&&(E.push(i),e.push(i))}u.speed&&F.duration(Math.min(.05/u.speed*C.length,u.maxDuration||9999)),s.rtl=p,s.original=e,s.text=E,s._props.push("text")}else s.text=s.original=[""]},render:function render(D,u){1<D?D=1:D<0&&(D=0),u.from&&(D=1-D);var F,C,E,e=u.text,t=u.hasClass,n=u.newClass,B=u.oldClass,i=u.delimiter,r=u.target,A=u.fillChar,s=u.original,l=u.rtl,o=e.length,a=(l?1-D:D)*o+.5|0;E=t&&D?(C=B&&a!==o,((F=n&&a)?"<span class='"+n+"'>":"")+e.slice(0,a).join(i)+(F?"</span>":"")+(C?"<span class='"+B+"'>":"")+i+s.slice(a).join(i)+(C?"</span>":"")):e.slice(0,a).join(i)+i+s.slice(a).join(i),u.svg?r.textContent=E:r.innerHTML=" "===A&&~E.indexOf(" ")?E.split(" ").join(" "):E}};F.splitInnerHTML=splitInnerHTML,F.emojiSafeSplit=emojiSafeSplit,F.getText=function getText(D){var u=D.nodeType,F="";if(1===u||9===u||11===u){if("string"==typeof D.textContent)return D.textContent;for(D=D.firstChild;D;D=D.nextSibling)F+=getText(D)}else if(3===u||4===u)return D.nodeValue;return F},function _getGSAP(){return u||"undefined"!=typeof window&&(u=window.gsap)&&u.registerPlugin&&u}()&&u.registerPlugin(F),D.TextPlugin=F,D.default=F;if (typeof(window)==="undefined"||window!==D){Object.defineProperty(D,"__esModule",{value:!0})} else {delete D.default}}); + diff --git a/node_modules/gsap/dist/TextPlugin.min.js.map b/node_modules/gsap/dist/TextPlugin.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..559dc7d18c7cd06b0c473ab7f7a9fe31a314cf7b --- /dev/null +++ b/node_modules/gsap/dist/TextPlugin.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"TextPlugin.min.js","sources":["../src/utils/strings.js","../src/TextPlugin.js"],"sourcesContent":["/*!\n * strings: 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _trimExp = /(?:^\\s+|\\s+$)/g;\n\nexport const emojiExp = /([\\uD800-\\uDBFF][\\uDC00-\\uDFFF](?:[\\u200D\\uFE0F][\\uD800-\\uDBFF][\\uDC00-\\uDFFF]){2,}|\\uD83D\\uDC69(?:\\u200D(?:(?:\\uD83D\\uDC69\\u200D)?\\uD83D\\uDC67|(?:\\uD83D\\uDC69\\u200D)?\\uD83D\\uDC66)|\\uD83C[\\uDFFB-\\uDFFF])|\\uD83D\\uDC69\\u200D(?:\\uD83D\\uDC69\\u200D)?\\uD83D\\uDC66\\u200D\\uD83D\\uDC66|\\uD83D\\uDC69\\u200D(?:\\uD83D\\uDC69\\u200D)?\\uD83D\\uDC67\\u200D(?:\\uD83D[\\uDC66\\uDC67])|\\uD83C\\uDFF3\\uFE0F\\u200D\\uD83C\\uDF08|(?:\\uD83C[\\uDFC3\\uDFC4\\uDFCA]|\\uD83D[\\uDC6E\\uDC71\\uDC73\\uDC77\\uDC81\\uDC82\\uDC86\\uDC87\\uDE45-\\uDE47\\uDE4B\\uDE4D\\uDE4E\\uDEA3\\uDEB4-\\uDEB6]|\\uD83E[\\uDD26\\uDD37-\\uDD39\\uDD3D\\uDD3E\\uDDD6-\\uDDDD])(?:\\uD83C[\\uDFFB-\\uDFFF])\\u200D[\\u2642\\u2640]\\uFE0F|\\uD83D\\uDC69(?:\\uD83C[\\uDFFB-\\uDFFF])\\u200D(?:\\uD83C[\\uDF3E\\uDF73\\uDF93\\uDFA4\\uDFA8\\uDFEB\\uDFED]|\\uD83D[\\uDCBB\\uDD27\\uDCBC\\uDD2C\\uDE80\\uDE92])|(?:\\uD83C[\\uDFC3\\uDFC4\\uDFCA]|\\uD83D[\\uDC6E\\uDC6F\\uDC71\\uDC73\\uDC77\\uDC81\\uDC82\\uDC86\\uDC87\\uDE45-\\uDE47\\uDE4B\\uDE4D\\uDE4E\\uDEA3\\uDEB4-\\uDEB6]|\\uD83E[\\uDD26\\uDD37-\\uDD39\\uDD3C-\\uDD3E\\uDDD6-\\uDDDF])\\u200D[\\u2640\\u2642]\\uFE0F|\\uD83C\\uDDFD\\uD83C\\uDDF0|\\uD83C\\uDDF6\\uD83C\\uDDE6|\\uD83C\\uDDF4\\uD83C\\uDDF2|\\uD83C\\uDDE9(?:\\uD83C[\\uDDEA\\uDDEC\\uDDEF\\uDDF0\\uDDF2\\uDDF4\\uDDFF])|\\uD83C\\uDDF7(?:\\uD83C[\\uDDEA\\uDDF4\\uDDF8\\uDDFA\\uDDFC])|\\uD83C\\uDDE8(?:\\uD83C[\\uDDE6\\uDDE8\\uDDE9\\uDDEB-\\uDDEE\\uDDF0-\\uDDF5\\uDDF7\\uDDFA-\\uDDFF])|(?:\\u26F9|\\uD83C[\\uDFCC\\uDFCB]|\\uD83D\\uDD75)(?:\\uFE0F\\u200D[\\u2640\\u2642]|(?:\\uD83C[\\uDFFB-\\uDFFF])\\u200D[\\u2640\\u2642])\\uFE0F|(?:\\uD83D\\uDC41\\uFE0F\\u200D\\uD83D\\uDDE8|\\uD83D\\uDC69(?:\\uD83C[\\uDFFB-\\uDFFF])\\u200D[\\u2695\\u2696\\u2708]|\\uD83D\\uDC69\\u200D[\\u2695\\u2696\\u2708]|\\uD83D\\uDC68(?:(?:\\uD83C[\\uDFFB-\\uDFFF])\\u200D[\\u2695\\u2696\\u2708]|\\u200D[\\u2695\\u2696\\u2708]))\\uFE0F|\\uD83C\\uDDF2(?:\\uD83C[\\uDDE6\\uDDE8-\\uDDED\\uDDF0-\\uDDFF])|\\uD83D\\uDC69\\u200D(?:\\uD83C[\\uDF3E\\uDF73\\uDF93\\uDFA4\\uDFA8\\uDFEB\\uDFED]|\\uD83D[\\uDCBB\\uDCBC\\uDD27\\uDD2C\\uDE80\\uDE92]|\\u2764\\uFE0F\\u200D(?:\\uD83D\\uDC8B\\u200D(?:\\uD83D[\\uDC68\\uDC69])|\\uD83D[\\uDC68\\uDC69]))|\\uD83C\\uDDF1(?:\\uD83C[\\uDDE6-\\uDDE8\\uDDEE\\uDDF0\\uDDF7-\\uDDFB\\uDDFE])|\\uD83C\\uDDEF(?:\\uD83C[\\uDDEA\\uDDF2\\uDDF4\\uDDF5])|\\uD83C\\uDDED(?:\\uD83C[\\uDDF0\\uDDF2\\uDDF3\\uDDF7\\uDDF9\\uDDFA])|\\uD83C\\uDDEB(?:\\uD83C[\\uDDEE-\\uDDF0\\uDDF2\\uDDF4\\uDDF7])|[#\\*0-9]\\uFE0F\\u20E3|\\uD83C\\uDDE7(?:\\uD83C[\\uDDE6\\uDDE7\\uDDE9-\\uDDEF\\uDDF1-\\uDDF4\\uDDF6-\\uDDF9\\uDDFB\\uDDFC\\uDDFE\\uDDFF])|\\uD83C\\uDDE6(?:\\uD83C[\\uDDE8-\\uDDEC\\uDDEE\\uDDF1\\uDDF2\\uDDF4\\uDDF6-\\uDDFA\\uDDFC\\uDDFD\\uDDFF])|\\uD83C\\uDDFF(?:\\uD83C[\\uDDE6\\uDDF2\\uDDFC])|\\uD83C\\uDDF5(?:\\uD83C[\\uDDE6\\uDDEA-\\uDDED\\uDDF0-\\uDDF3\\uDDF7-\\uDDF9\\uDDFC\\uDDFE])|\\uD83C\\uDDFB(?:\\uD83C[\\uDDE6\\uDDE8\\uDDEA\\uDDEC\\uDDEE\\uDDF3\\uDDFA])|\\uD83C\\uDDF3(?:\\uD83C[\\uDDE6\\uDDE8\\uDDEA-\\uDDEC\\uDDEE\\uDDF1\\uDDF4\\uDDF5\\uDDF7\\uDDFA\\uDDFF])|\\uD83C\\uDFF4\\uDB40\\uDC67\\uDB40\\uDC62(?:\\uDB40\\uDC77\\uDB40\\uDC6C\\uDB40\\uDC73|\\uDB40\\uDC73\\uDB40\\uDC63\\uDB40\\uDC74|\\uDB40\\uDC65\\uDB40\\uDC6E\\uDB40\\uDC67)\\uDB40\\uDC7F|\\uD83D\\uDC68(?:\\u200D(?:\\u2764\\uFE0F\\u200D(?:\\uD83D\\uDC8B\\u200D)?\\uD83D\\uDC68|(?:(?:\\uD83D[\\uDC68\\uDC69])\\u200D)?\\uD83D\\uDC66\\u200D\\uD83D\\uDC66|(?:(?:\\uD83D[\\uDC68\\uDC69])\\u200D)?\\uD83D\\uDC67\\u200D(?:\\uD83D[\\uDC66\\uDC67])|\\uD83C[\\uDF3E\\uDF73\\uDF93\\uDFA4\\uDFA8\\uDFEB\\uDFED]|\\uD83D[\\uDCBB\\uDCBC\\uDD27\\uDD2C\\uDE80\\uDE92])|(?:\\uD83C[\\uDFFB-\\uDFFF])\\u200D(?:\\uD83C[\\uDF3E\\uDF73\\uDF93\\uDFA4\\uDFA8\\uDFEB\\uDFED]|\\uD83D[\\uDCBB\\uDCBC\\uDD27\\uDD2C\\uDE80\\uDE92]))|\\uD83C\\uDDF8(?:\\uD83C[\\uDDE6-\\uDDEA\\uDDEC-\\uDDF4\\uDDF7-\\uDDF9\\uDDFB\\uDDFD-\\uDDFF])|\\uD83C\\uDDF0(?:\\uD83C[\\uDDEA\\uDDEC-\\uDDEE\\uDDF2\\uDDF3\\uDDF5\\uDDF7\\uDDFC\\uDDFE\\uDDFF])|\\uD83C\\uDDFE(?:\\uD83C[\\uDDEA\\uDDF9])|\\uD83C\\uDDEE(?:\\uD83C[\\uDDE8-\\uDDEA\\uDDF1-\\uDDF4\\uDDF6-\\uDDF9])|\\uD83C\\uDDF9(?:\\uD83C[\\uDDE6\\uDDE8\\uDDE9\\uDDEB-\\uDDED\\uDDEF-\\uDDF4\\uDDF7\\uDDF9\\uDDFB\\uDDFC\\uDDFF])|\\uD83C\\uDDEC(?:\\uD83C[\\uDDE6\\uDDE7\\uDDE9-\\uDDEE\\uDDF1-\\uDDF3\\uDDF5-\\uDDFA\\uDDFC\\uDDFE])|\\uD83C\\uDDFA(?:\\uD83C[\\uDDE6\\uDDEC\\uDDF2\\uDDF3\\uDDF8\\uDDFE\\uDDFF])|\\uD83C\\uDDEA(?:\\uD83C[\\uDDE6\\uDDE8\\uDDEA\\uDDEC\\uDDED\\uDDF7-\\uDDFA])|\\uD83C\\uDDFC(?:\\uD83C[\\uDDEB\\uDDF8])|(?:\\u26F9|\\uD83C[\\uDFCB\\uDFCC]|\\uD83D\\uDD75)(?:\\uD83C[\\uDFFB-\\uDFFF])|(?:\\uD83C[\\uDFC3\\uDFC4\\uDFCA]|\\uD83D[\\uDC6E\\uDC71\\uDC73\\uDC77\\uDC81\\uDC82\\uDC86\\uDC87\\uDE45-\\uDE47\\uDE4B\\uDE4D\\uDE4E\\uDEA3\\uDEB4-\\uDEB6]|\\uD83E[\\uDD26\\uDD37-\\uDD39\\uDD3D\\uDD3E\\uDDD6-\\uDDDD])(?:\\uD83C[\\uDFFB-\\uDFFF])|(?:[\\u261D\\u270A-\\u270D]|\\uD83C[\\uDF85\\uDFC2\\uDFC7]|\\uD83D[\\uDC42\\uDC43\\uDC46-\\uDC50\\uDC66\\uDC67\\uDC70\\uDC72\\uDC74-\\uDC76\\uDC78\\uDC7C\\uDC83\\uDC85\\uDCAA\\uDD74\\uDD7A\\uDD90\\uDD95\\uDD96\\uDE4C\\uDE4F\\uDEC0\\uDECC]|\\uD83E[\\uDD18-\\uDD1C\\uDD1E\\uDD1F\\uDD30-\\uDD36\\uDDD1-\\uDDD5])(?:\\uD83C[\\uDFFB-\\uDFFF])|\\uD83D\\uDC68(?:\\u200D(?:(?:(?:\\uD83D[\\uDC68\\uDC69])\\u200D)?\\uD83D\\uDC67|(?:(?:\\uD83D[\\uDC68\\uDC69])\\u200D)?\\uD83D\\uDC66)|\\uD83C[\\uDFFB-\\uDFFF])|(?:[\\u261D\\u26F9\\u270A-\\u270D]|\\uD83C[\\uDF85\\uDFC2-\\uDFC4\\uDFC7\\uDFCA-\\uDFCC]|\\uD83D[\\uDC42\\uDC43\\uDC46-\\uDC50\\uDC66-\\uDC69\\uDC6E\\uDC70-\\uDC78\\uDC7C\\uDC81-\\uDC83\\uDC85-\\uDC87\\uDCAA\\uDD74\\uDD75\\uDD7A\\uDD90\\uDD95\\uDD96\\uDE45-\\uDE47\\uDE4B-\\uDE4F\\uDEA3\\uDEB4-\\uDEB6\\uDEC0\\uDECC]|\\uD83E[\\uDD18-\\uDD1C\\uDD1E\\uDD1F\\uDD26\\uDD30-\\uDD39\\uDD3D\\uDD3E\\uDDD1-\\uDDDD])(?:\\uD83C[\\uDFFB-\\uDFFF])?|(?:[\\u231A\\u231B\\u23E9-\\u23EC\\u23F0\\u23F3\\u25FD\\u25FE\\u2614\\u2615\\u2648-\\u2653\\u267F\\u2693\\u26A1\\u26AA\\u26AB\\u26BD\\u26BE\\u26C4\\u26C5\\u26CE\\u26D4\\u26EA\\u26F2\\u26F3\\u26F5\\u26FA\\u26FD\\u2705\\u270A\\u270B\\u2728\\u274C\\u274E\\u2753-\\u2755\\u2757\\u2795-\\u2797\\u27B0\\u27BF\\u2B1B\\u2B1C\\u2B50\\u2B55]|\\uD83C[\\uDC04\\uDCCF\\uDD8E\\uDD91-\\uDD9A\\uDDE6-\\uDDFF\\uDE01\\uDE1A\\uDE2F\\uDE32-\\uDE36\\uDE38-\\uDE3A\\uDE50\\uDE51\\uDF00-\\uDF20\\uDF2D-\\uDF35\\uDF37-\\uDF7C\\uDF7E-\\uDF93\\uDFA0-\\uDFCA\\uDFCF-\\uDFD3\\uDFE0-\\uDFF0\\uDFF4\\uDFF8-\\uDFFF]|\\uD83D[\\uDC00-\\uDC3E\\uDC40\\uDC42-\\uDCFC\\uDCFF-\\uDD3D\\uDD4B-\\uDD4E\\uDD50-\\uDD67\\uDD7A\\uDD95\\uDD96\\uDDA4\\uDDFB-\\uDE4F\\uDE80-\\uDEC5\\uDECC\\uDED0-\\uDED2\\uDEEB\\uDEEC\\uDEF4-\\uDEF8]|\\uD83E[\\uDD10-\\uDD3A\\uDD3C-\\uDD3E\\uDD40-\\uDD45\\uDD47-\\uDD4C\\uDD50-\\uDD6B\\uDD80-\\uDD97\\uDDC0\\uDDD0-\\uDDE6])|(?:[#\\*0-9\\xA9\\xAE\\u203C\\u2049\\u2122\\u2139\\u2194-\\u2199\\u21A9\\u21AA\\u231A\\u231B\\u2328\\u23CF\\u23E9-\\u23F3\\u23F8-\\u23FA\\u24C2\\u25AA\\u25AB\\u25B6\\u25C0\\u25FB-\\u25FE\\u2600-\\u2604\\u260E\\u2611\\u2614\\u2615\\u2618\\u261D\\u2620\\u2622\\u2623\\u2626\\u262A\\u262E\\u262F\\u2638-\\u263A\\u2640\\u2642\\u2648-\\u2653\\u2660\\u2663\\u2665\\u2666\\u2668\\u267B\\u267F\\u2692-\\u2697\\u2699\\u269B\\u269C\\u26A0\\u26A1\\u26AA\\u26AB\\u26B0\\u26B1\\u26BD\\u26BE\\u26C4\\u26C5\\u26C8\\u26CE\\u26CF\\u26D1\\u26D3\\u26D4\\u26E9\\u26EA\\u26F0-\\u26F5\\u26F7-\\u26FA\\u26FD\\u2702\\u2705\\u2708-\\u270D\\u270F\\u2712\\u2714\\u2716\\u271D\\u2721\\u2728\\u2733\\u2734\\u2744\\u2747\\u274C\\u274E\\u2753-\\u2755\\u2757\\u2763\\u2764\\u2795-\\u2797\\u27A1\\u27B0\\u27BF\\u2934\\u2935\\u2B05-\\u2B07\\u2B1B\\u2B1C\\u2B50\\u2B55\\u3030\\u303D\\u3297\\u3299]|\\uD83C[\\uDC04\\uDCCF\\uDD70\\uDD71\\uDD7E\\uDD7F\\uDD8E\\uDD91-\\uDD9A\\uDDE6-\\uDDFF\\uDE01\\uDE02\\uDE1A\\uDE2F\\uDE32-\\uDE3A\\uDE50\\uDE51\\uDF00-\\uDF21\\uDF24-\\uDF93\\uDF96\\uDF97\\uDF99-\\uDF9B\\uDF9E-\\uDFF0\\uDFF3-\\uDFF5\\uDFF7-\\uDFFF]|\\uD83D[\\uDC00-\\uDCFD\\uDCFF-\\uDD3D\\uDD49-\\uDD4E\\uDD50-\\uDD67\\uDD6F\\uDD70\\uDD73-\\uDD7A\\uDD87\\uDD8A-\\uDD8D\\uDD90\\uDD95\\uDD96\\uDDA4\\uDDA5\\uDDA8\\uDDB1\\uDDB2\\uDDBC\\uDDC2-\\uDDC4\\uDDD1-\\uDDD3\\uDDDC-\\uDDDE\\uDDE1\\uDDE3\\uDDE8\\uDDEF\\uDDF3\\uDDFA-\\uDE4F\\uDE80-\\uDEC5\\uDECB-\\uDED2\\uDEE0-\\uDEE5\\uDEE9\\uDEEB\\uDEEC\\uDEF0\\uDEF3-\\uDEF8]|\\uD83E[\\uDD10-\\uDD3A\\uDD3C-\\uDD3E\\uDD40-\\uDD45\\uDD47-\\uDD4C\\uDD50-\\uDD6B\\uDD80-\\uDD97\\uDDC0\\uDDD0-\\uDDE6])\\uFE0F)/;\n\nexport function getText(e) {\n\tlet type = e.nodeType,\n\t\tresult = \"\";\n\tif (type === 1 || type === 9 || type === 11) {\n\t\tif (typeof(e.textContent) === \"string\") {\n\t\t\treturn e.textContent;\n\t\t} else {\n\t\t\tfor (e = e.firstChild; e; e = e.nextSibling ) {\n\t\t\t\tresult += getText(e);\n\t\t\t}\n\t\t}\n\t} else if (type === 3 || type === 4) {\n\t\treturn e.nodeValue;\n\t}\n\treturn result;\n}\n\nexport function splitInnerHTML(element, delimiter, trim, preserveSpaces, unescapedCharCodes) {\n\tlet node = element.firstChild,\n\t\tresult = [], s;\n\twhile (node) {\n\t\tif (node.nodeType === 3) {\n\t\t\ts = (node.nodeValue + \"\").replace(/^\\n+/g, \"\");\n\t\t\tif (!preserveSpaces) {\n\t\t\t\ts = s.replace(/\\s+/g, \" \");\n\t\t\t}\n\t\t\tresult.push(...emojiSafeSplit(s, delimiter, trim, preserveSpaces, unescapedCharCodes));\n\t\t} else if ((node.nodeName + \"\").toLowerCase() === \"br\") {\n\t\t\tresult[result.length-1] += \"<br>\";\n\t\t} else {\n\t\t\tresult.push(node.outerHTML);\n\t\t}\n\t\tnode = node.nextSibling;\n\t}\n\tif (!unescapedCharCodes) {\n\t\ts = result.length;\n\t\twhile (s--) {\n\t\t\tresult[s] === \"&\" && result.splice(s, 1, \"&\");\n\t\t}\n\t}\n\treturn result;\n}\n\n/*\n//smaller kb version that only handles the simpler emoji's, which is often perfectly adequate.\n\nlet _emoji = \"[\\uE000-\\uF8FF]|\\uD83C[\\uDC00-\\uDFFF]|\\uD83D[\\uDC00-\\uDFFF]|[\\u2694-\\u2697]|\\uD83E[\\uDD10-\\uDD5D]|[\\uD800-\\uDBFF][\\uDC00-\\uDFFF]\",\n\t_emojiExp = new RegExp(_emoji),\n\t_emojiAndCharsExp = new RegExp(_emoji + \"|.\", \"g\"),\n\t_emojiSafeSplit = (text, delimiter, trim) => {\n\t\tif (trim) {\n\t\t\ttext = text.replace(_trimExp, \"\");\n\t\t}\n\t\treturn ((delimiter === \"\" || !delimiter) && _emojiExp.test(text)) ? text.match(_emojiAndCharsExp) : text.split(delimiter || \"\");\n\t};\n */\nexport function emojiSafeSplit(text, delimiter, trim, preserveSpaces, unescapedCharCodes) {\n\ttext += \"\"; // make sure it's cast as a string. Someone may pass in a number.\n\ttrim && (text = text.trim ? text.trim() : text.replace(_trimExp, \"\")); // IE9 and earlier compatibility\n\tif (delimiter && delimiter !== \"\") {\n\t\treturn text.replace(/>/g, \">\").replace(/</g, \"<\").split(delimiter);\n\t}\n\tlet result = [],\n\t\tl = text.length,\n\t\ti = 0,\n\t\tj, character;\n\tfor (; i < l; i++) {\n\t\tcharacter = text.charAt(i);\n\t\tif ((character.charCodeAt(0) >= 0xD800 && character.charCodeAt(0) <= 0xDBFF) || (text.charCodeAt(i+1) >= 0xFE00 && text.charCodeAt(i+1) <= 0xFE0F)) { //special emoji characters use 2 or 4 unicode characters that we must keep together.\n\t\t\tj = ((text.substr(i, 12).split(emojiExp) || [])[1] || \"\").length || 2;\n\t\t\tcharacter = text.substr(i, j);\n\t\t\tresult.emoji = 1;\n\t\t\ti += j - 1;\n\t\t}\n\t\tresult.push(unescapedCharCodes ? character : character === \">\" ? \">\" : (character === \"<\") ? \"<\" : preserveSpaces && character === \" \" && (text.charAt(i-1) === \" \" || text.charAt(i+1) === \" \") ? \" \" : character);\n\t}\n\treturn result;\n}","/*!\n * TextPlugin 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nimport { emojiSafeSplit, getText, splitInnerHTML } from \"./utils/strings.js\";\n\nlet gsap, _tempDiv,\n\t_getGSAP = () => gsap || (typeof(window) !== \"undefined\" && (gsap = window.gsap) && gsap.registerPlugin && gsap);\n\n\nexport const TextPlugin = {\n\tversion:\"3.12.7\",\n\tname:\"text\",\n\tinit(target, value, tween) {\n\t\ttypeof(value) !== \"object\" && (value = {value:value});\n\t\tlet i = target.nodeName.toUpperCase(),\n\t\t\tdata = this,\n\t\t\t{ newClass, oldClass, preserveSpaces, rtl } = value,\n\t\t\tdelimiter = data.delimiter = value.delimiter || \"\",\n\t\t\tfillChar = data.fillChar = value.fillChar || (value.padSpace ? \" \" : \"\"),\n\t\t\tshort, text, original, j, condensedText, condensedOriginal, aggregate, s;\n\t\tdata.svg = (target.getBBox && (i === \"TEXT\" || i === \"TSPAN\"));\n\t\tif (!(\"innerHTML\" in target) && !data.svg) {\n\t\t\treturn false;\n\t\t}\n\t\tdata.target = target;\n\t\tif (!(\"value\" in value)) {\n\t\t\tdata.text = data.original = [\"\"];\n\t\t\treturn;\n\t\t}\n\t\toriginal = splitInnerHTML(target, delimiter, false, preserveSpaces, data.svg);\n\t\t_tempDiv || (_tempDiv = document.createElement(\"div\"));\n\t\t_tempDiv.innerHTML = value.value;\n\t\ttext = splitInnerHTML(_tempDiv, delimiter, false, preserveSpaces, data.svg);\n\t\tdata.from = tween._from;\n\t\tif ((data.from || rtl) && !(rtl && data.from)) { // right-to-left or \"from()\" tweens should invert things (but if it's BOTH .from() and rtl, inverting twice equals not inverting at all :)\n\t\t\ti = original;\n\t\t\toriginal = text;\n\t\t\ttext = i;\n\t\t}\n\t\tdata.hasClass = !!(newClass || oldClass);\n\t\tdata.newClass = rtl ? oldClass : newClass;\n\t\tdata.oldClass = rtl ? newClass : oldClass;\n\t\ti = original.length - text.length;\n\t\tshort = i < 0 ? original : text;\n\t\tif (i < 0) {\n\t\t\ti = -i;\n\t\t}\n\t\twhile (--i > -1) {\n\t\t\tshort.push(fillChar);\n\t\t}\n\t\tif (value.type === \"diff\") {\n\t\t\tj = 0;\n\t\t\tcondensedText = [];\n\t\t\tcondensedOriginal = [];\n\t\t\taggregate = \"\";\n\t\t\tfor (i = 0; i < text.length; i++) {\n\t\t\t\ts = text[i];\n\t\t\t\tif (s === original[i]) {\n\t\t\t\t\taggregate += s;\n\t\t\t\t} else {\n\t\t\t\t\tcondensedText[j] = aggregate + s;\n\t\t\t\t\tcondensedOriginal[j++] = aggregate + original[i];\n\t\t\t\t\taggregate = \"\";\n\t\t\t\t}\n\t\t\t}\n\t\t\ttext = condensedText;\n\t\t\toriginal = condensedOriginal;\n\t\t\tif (aggregate) {\n\t\t\t\ttext.push(aggregate);\n\t\t\t\toriginal.push(aggregate);\n\t\t\t}\n\t\t}\n\t\tvalue.speed && tween.duration(Math.min(0.05 / value.speed * short.length, value.maxDuration || 9999));\n\t\tdata.rtl = rtl;\n\t\tdata.original = original;\n\t\tdata.text = text;\n\t\tdata._props.push(\"text\");\n\t},\n\trender(ratio, data) {\n\t\tif (ratio > 1) {\n\t\t\tratio = 1;\n\t\t} else if (ratio < 0) {\n\t\t\tratio = 0;\n\t\t}\n\t\tif (data.from) {\n\t\t\tratio = 1 - ratio;\n\t\t}\n\t\tlet { text, hasClass, newClass, oldClass, delimiter, target, fillChar, original, rtl } = data,\n\t\t\tl = text.length,\n\t\t\ti = ((rtl ? 1 - ratio : ratio) * l + 0.5) | 0,\n\t\t\tapplyNew, applyOld, str;\n\t\tif (hasClass && ratio) {\n\t\t\tapplyNew = (newClass && i);\n\t\t\tapplyOld = (oldClass && i !== l);\n\t\t\tstr = (applyNew ? \"<span class='\" + newClass + \"'>\" : \"\") + text.slice(0, i).join(delimiter) + (applyNew ? \"</span>\" : \"\") + (applyOld ? \"<span class='\" + oldClass + \"'>\" : \"\") + delimiter + original.slice(i).join(delimiter) + (applyOld ? \"</span>\" : \"\");\n\t\t} else {\n\t\t\tstr = text.slice(0, i).join(delimiter) + delimiter + original.slice(i).join(delimiter);\n\t\t}\n\t\tif (data.svg) { //SVG text elements don't have an \"innerHTML\" in Microsoft browsers.\n\t\t\ttarget.textContent = str;\n\t\t} else {\n\t\t\ttarget.innerHTML = (fillChar === \" \" && ~str.indexOf(\" \")) ? str.split(\" \").join(\" \") : str;\n\t\t}\n\t}\n};\n\nTextPlugin.splitInnerHTML = splitInnerHTML;\nTextPlugin.emojiSafeSplit = emojiSafeSplit;\nTextPlugin.getText = getText;\n\n_getGSAP() && gsap.registerPlugin(TextPlugin);\n\nexport { TextPlugin as default };"],"names":["_trimExp","emojiExp","splitInnerHTML","element","delimiter","trim","preserveSpaces","unescapedCharCodes","s","node","firstChild","result","nodeType","nodeValue","replace","push","emojiSafeSplit","nodeName","toLowerCase","length","outerHTML","nextSibling","splice","text","split","j","character","l","i","charAt","charCodeAt","substr","emoji","gsap","_tempDiv","TextPlugin","version","name","init","target","value","tween","short","original","condensedText","condensedOriginal","aggregate","toUpperCase","data","this","newClass","oldClass","rtl","fillChar","padSpace","svg","getBBox","document","createElement","innerHTML","from","_from","hasClass","type","speed","duration","Math","min","maxDuration","_props","render","ratio","applyNew","applyOld","str","slice","join","textContent","indexOf","getText","e","_getGSAP","window","registerPlugin"],"mappings":";;;;;;;;;6MAWA,IAAIA,EAAW,iBAEFC,EAAW,4gOAmBjB,SAASC,eAAeC,EAASC,EAAWC,EAAMC,EAAgBC,WAE1DC,EADVC,EAAON,EAAQO,WAClBC,EAAS,GACHF,GACgB,IAAlBA,EAAKG,UACRJ,GAAKC,EAAKI,UAAY,IAAIC,QAAQ,QAAS,IACtCR,IACJE,EAAIA,EAAEM,QAAQ,OAAQ,MAEvBH,EAAOI,WAAPJ,EAAeK,eAAeR,EAAGJ,EAAWC,EAAMC,EAAgBC,KACjB,QAAtCE,EAAKQ,SAAW,IAAIC,cAC/BP,EAAOA,EAAOQ,OAAO,IAAM,OAE3BR,EAAOI,KAAKN,EAAKW,WAElBX,EAAOA,EAAKY,gBAERd,MACJC,EAAIG,EAAOQ,OACJX,KACQ,MAAdG,EAAOH,IAAcG,EAAOW,OAAOd,EAAG,EAAG,gBAGpCG,EAgBD,SAASK,eAAeO,EAAMnB,EAAWC,EAAMC,EAAgBC,MACrEgB,GAAQ,GACRlB,IAASkB,EAAOA,EAAKlB,KAAOkB,EAAKlB,OAASkB,EAAKT,QAAQd,EAAU,KAC7DI,GAA2B,KAAdA,SACTmB,EAAKT,QAAQ,KAAM,QAAQA,QAAQ,KAAM,QAAQU,MAAMpB,WAK9DqB,EAAGC,EAHAf,EAAS,GACZgB,EAAIJ,EAAKJ,OACTS,EAAI,EAEEA,EAAID,EAAGC,KAEmB,QADhCF,EAAYH,EAAKM,OAAOD,IACTE,WAAW,IAAgBJ,EAAUI,WAAW,IAAM,OAAoC,OAAxBP,EAAKO,WAAWF,EAAE,IAAgBL,EAAKO,WAAWF,EAAE,IAAM,SAC1IH,IAAMF,EAAKQ,OAAOH,EAAG,IAAIJ,MAAMvB,IAAa,IAAI,IAAM,IAAIkB,QAAU,EACpEO,EAAYH,EAAKQ,OAAOH,EAAGH,GAE3BG,GAAKH,GADLd,EAAOqB,MAAQ,IAGhBrB,EAAOI,KAAKR,EAAqBmB,EAA0B,MAAdA,EAAoB,OAAwB,MAAdA,EAAqB,QAASpB,GAAgC,MAAdoB,GAA2C,MAArBH,EAAKM,OAAOD,EAAE,IAAmC,MAArBL,EAAKM,OAAOD,EAAE,GAAyBF,EAAX,iBAEnMf,EC9ER,IAAIsB,EAAMC,EAIGC,EAAa,CACzBC,QAAQ,SACRC,KAAK,OACLC,mBAAKC,EAAQC,EAAOC,GACD,iBAAXD,IAAwBA,EAAQ,CAACA,MAAMA,QAM7CE,EAAOnB,EAAMoB,EAAUlB,EAAGmB,EAAeC,EAAmBC,EAAWtC,EALpEoB,EAAIW,EAAOtB,SAAS8B,cACvBC,EAAOC,KACLC,EAA4CV,EAA5CU,SAAUC,EAAkCX,EAAlCW,SAAU7C,EAAwBkC,EAAxBlC,eAAgB8C,EAAQZ,EAARY,IACtChD,EAAY4C,EAAK5C,UAAYoC,EAAMpC,WAAa,GAChDiD,EAAWL,EAAKK,SAAWb,EAAMa,WAAab,EAAMc,SAAW,SAAW,OAE3EN,EAAKO,IAAOhB,EAAOiB,UAAkB,SAAN5B,GAAsB,UAANA,KACzC,cAAeW,GAAYS,EAAKO,YAC9B,KAERP,EAAKT,OAASA,EACR,UAAWC,OAIjBG,EAAWzC,eAAeqC,EAAQnC,GAAW,EAAOE,EAAgB0C,EAAKO,MAC5DrB,EAAbA,GAAwBuB,SAASC,cAAc,QACtCC,UAAYnB,EAAMA,MAC3BjB,EAAOrB,eAAegC,EAAU9B,GAAW,EAAOE,EAAgB0C,EAAKO,KACvEP,EAAKY,KAAOnB,EAAMoB,OACbb,EAAKY,OAAQR,GAAUA,GAAOJ,EAAKY,OACvChC,EAAIe,EACJA,EAAWpB,EACXA,EAAOK,GAERoB,EAAKc,YAAcZ,IAAYC,GAC/BH,EAAKE,SAAWE,EAAMD,EAAWD,EACjCF,EAAKG,SAAWC,EAAMF,EAAWC,EAEjCT,GADAd,EAAIe,EAASxB,OAASI,EAAKJ,QACf,EAAIwB,EAAWpB,EACvBK,EAAI,IACPA,GAAKA,IAEQ,IAALA,GACRc,EAAM3B,KAAKsC,MAEO,SAAfb,EAAMuB,KAAiB,KAE1BnB,EAAgB,GAChBC,EAAoB,GACpBC,EAAY,GACPlB,EAJLH,EAAI,EAIQG,EAAIL,EAAKJ,OAAQS,KAC5BpB,EAAIe,EAAKK,MACCe,EAASf,GAClBkB,GAAatC,GAEboC,EAAcnB,GAAKqB,EAAYtC,EAC/BqC,EAAkBpB,KAAOqB,EAAYH,EAASf,GAC9CkB,EAAY,IAGdvB,EAAOqB,EACPD,EAAWE,EACPC,IACHvB,EAAKR,KAAK+B,GACVH,EAAS5B,KAAK+B,IAGhBN,EAAMwB,OAASvB,EAAMwB,SAASC,KAAKC,IAAI,IAAO3B,EAAMwB,MAAQtB,EAAMvB,OAAQqB,EAAM4B,aAAe,OAC/FpB,EAAKI,IAAMA,EACXJ,EAAKL,SAAWA,EAChBK,EAAKzB,KAAOA,EACZyB,EAAKqB,OAAOtD,KAAK,aAlDhBiC,EAAKzB,KAAOyB,EAAKL,SAAW,CAAC,KAoD/B2B,uBAAOC,EAAOvB,GACD,EAARuB,EACHA,EAAQ,EACEA,EAAQ,IAClBA,EAAQ,GAELvB,EAAKY,OACRW,EAAQ,EAAIA,OAKZC,EAAUC,EAAUC,EAHfnD,EAAmFyB,EAAnFzB,KAAMuC,EAA6Ed,EAA7Ec,SAAUZ,EAAmEF,EAAnEE,SAAUC,EAAyDH,EAAzDG,SAAU/C,EAA+C4C,EAA/C5C,UAAWmC,EAAoCS,EAApCT,OAAQc,EAA4BL,EAA5BK,SAAUV,EAAkBK,EAAlBL,SAAUS,EAAQJ,EAARI,IAChFzB,EAAIJ,EAAKJ,OACTS,GAAMwB,EAAM,EAAImB,EAAQA,GAAS5C,EAAI,GAAO,EAK5C+C,EAHGZ,GAAYS,GAEfE,EAAYtB,GAAYvB,IAAMD,IAD9B6C,EAAYtB,GAAYtB,GAEN,gBAAkBsB,EAAW,KAAO,IAAM3B,EAAKoD,MAAM,EAAG/C,GAAGgD,KAAKxE,IAAcoE,EAAW,UAAY,KAAOC,EAAW,gBAAkBtB,EAAW,KAAO,IAAM/C,EAAYuC,EAASgC,MAAM/C,GAAGgD,KAAKxE,IAAcqE,EAAW,UAAY,KAErPlD,EAAKoD,MAAM,EAAG/C,GAAGgD,KAAKxE,GAAaA,EAAYuC,EAASgC,MAAM/C,GAAGgD,KAAKxE,GAEzE4C,EAAKO,IACRhB,EAAOsC,YAAcH,EAErBnC,EAAOoB,UAA0B,WAAbN,IAA0BqB,EAAII,QAAQ,MAASJ,EAAIlD,MAAM,MAAMoD,KAAK,gBAAkBF,IAK7GvC,EAAWjC,eAAiBA,eAC5BiC,EAAWnB,eAAiBA,eAC5BmB,EAAW4C,QDrGJ,SAASA,QAAQC,OACnBjB,EAAOiB,EAAEpE,SACZD,EAAS,MACG,IAAToD,GAAuB,IAATA,GAAuB,KAATA,EAAa,IACd,iBAAnBiB,EAAEH,mBACLG,EAAEH,gBAEJG,EAAIA,EAAEtE,WAAYsE,EAAGA,EAAIA,EAAE3D,YAC/BV,GAAUoE,QAAQC,QAGd,GAAa,IAATjB,GAAuB,IAATA,SACjBiB,EAAEnE,iBAEHF,GCfI,SAAXsE,kBAAiBhD,GAA4B,oBAAZiD,SAA4BjD,EAAOiD,OAAOjD,OAASA,EAAKkD,gBAAkBlD,EAwG5GgD,IAAchD,EAAKkD,eAAehD"} \ No newline at end of file diff --git a/node_modules/gsap/dist/all.js b/node_modules/gsap/dist/all.js new file mode 100644 index 0000000000000000000000000000000000000000..88fb2cfd16fce94072f6afe2695a43c5770d8e30 --- /dev/null +++ b/node_modules/gsap/dist/all.js @@ -0,0 +1,16725 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + function _defineProperties(target, props) { + for (var i = 0; i < props.length; i++) { + var descriptor = props[i]; + descriptor.enumerable = descriptor.enumerable || false; + descriptor.configurable = true; + if ("value" in descriptor) descriptor.writable = true; + Object.defineProperty(target, descriptor.key, descriptor); + } + } + + function _createClass(Constructor, protoProps, staticProps) { + if (protoProps) _defineProperties(Constructor.prototype, protoProps); + if (staticProps) _defineProperties(Constructor, staticProps); + return Constructor; + } + + function _inheritsLoose(subClass, superClass) { + subClass.prototype = Object.create(superClass.prototype); + subClass.prototype.constructor = subClass; + subClass.__proto__ = superClass; + } + + function _assertThisInitialized(self) { + if (self === void 0) { + throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); + } + + return self; + } + + /*! + * GSAP 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var _config = { + autoSleep: 120, + force3D: "auto", + nullTargetWarn: 1, + units: { + lineHeight: "" + } + }, + _defaults = { + duration: .5, + overwrite: false, + delay: 0 + }, + _suppressOverwrites, + _reverting, + _context, + _bigNum = 1e8, + _tinyNum = 1 / _bigNum, + _2PI = Math.PI * 2, + _HALF_PI = _2PI / 4, + _gsID = 0, + _sqrt = Math.sqrt, + _cos = Math.cos, + _sin = Math.sin, + _isString = function _isString(value) { + return typeof value === "string"; + }, + _isFunction = function _isFunction(value) { + return typeof value === "function"; + }, + _isNumber = function _isNumber(value) { + return typeof value === "number"; + }, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; + }, + _isObject = function _isObject(value) { + return typeof value === "object"; + }, + _isNotFalse = function _isNotFalse(value) { + return value !== false; + }, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _isFuncOrString = function _isFuncOrString(value) { + return _isFunction(value) || _isString(value); + }, + _isTypedArray = typeof ArrayBuffer === "function" && ArrayBuffer.isView || function () {}, + _isArray = Array.isArray, + _strictNumExp = /(?:-?\.?\d|\.)+/gi, + _numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/g, + _numWithUnitExp = /[-+=.]*\d+[.e-]*\d*[a-z%]*/g, + _complexStringNumExp = /[-+=.]*\d+\.?\d*(?:e-|e\+)?\d*/gi, + _relExp = /[+-]=-?[.\d]+/, + _delimitedValueExp = /[^,'"\[\]\s]+/gi, + _unitExp = /^[+\-=e\s\d]*\d+[.\d]*([a-z]*|%)\s*$/i, + _globalTimeline, + _win, + _coreInitted, + _doc, + _globals = {}, + _installScope = {}, + _coreReady, + _install = function _install(scope) { + return (_installScope = _merge(scope, _globals)) && gsap; + }, + _missingPlugin = function _missingPlugin(property, value) { + return console.warn("Invalid property", property, "set to", value, "Missing plugin? gsap.registerPlugin()"); + }, + _warn = function _warn(message, suppress) { + return !suppress && console.warn(message); + }, + _addGlobal = function _addGlobal(name, obj) { + return name && (_globals[name] = obj) && _installScope && (_installScope[name] = obj) || _globals; + }, + _emptyFunc = function _emptyFunc() { + return 0; + }, + _startAtRevertConfig = { + suppressEvents: true, + isStart: true, + kill: false + }, + _revertConfigNoKill = { + suppressEvents: true, + kill: false + }, + _revertConfig = { + suppressEvents: true + }, + _reservedProps = {}, + _lazyTweens = [], + _lazyLookup = {}, + _lastRenderedFrame, + _plugins = {}, + _effects = {}, + _nextGCFrame = 30, + _harnessPlugins = [], + _callbackNames = "", + _harness = function _harness(targets) { + var target = targets[0], + harnessPlugin, + i; + _isObject(target) || _isFunction(target) || (targets = [targets]); + + if (!(harnessPlugin = (target._gsap || {}).harness)) { + i = _harnessPlugins.length; + + while (i-- && !_harnessPlugins[i].targetTest(target)) {} + + harnessPlugin = _harnessPlugins[i]; + } + + i = targets.length; + + while (i--) { + targets[i] && (targets[i]._gsap || (targets[i]._gsap = new GSCache(targets[i], harnessPlugin))) || targets.splice(i, 1); + } + + return targets; + }, + _getCache = function _getCache(target) { + return target._gsap || _harness(toArray(target))[0]._gsap; + }, + _getProperty = function _getProperty(target, property, v) { + return (v = target[property]) && _isFunction(v) ? target[property]() : _isUndefined(v) && target.getAttribute && target.getAttribute(property) || v; + }, + _forEachName = function _forEachName(names, func) { + return (names = names.split(",")).forEach(func) || names; + }, + _round = function _round(value) { + return Math.round(value * 100000) / 100000 || 0; + }, + _roundPrecise = function _roundPrecise(value) { + return Math.round(value * 10000000) / 10000000 || 0; + }, + _parseRelative = function _parseRelative(start, value) { + var operator = value.charAt(0), + end = parseFloat(value.substr(2)); + start = parseFloat(start); + return operator === "+" ? start + end : operator === "-" ? start - end : operator === "*" ? start * end : start / end; + }, + _arrayContainsAny = function _arrayContainsAny(toSearch, toFind) { + var l = toFind.length, + i = 0; + + for (; toSearch.indexOf(toFind[i]) < 0 && ++i < l;) {} + + return i < l; + }, + _lazyRender = function _lazyRender() { + var l = _lazyTweens.length, + a = _lazyTweens.slice(0), + i, + tween; + + _lazyLookup = {}; + _lazyTweens.length = 0; + + for (i = 0; i < l; i++) { + tween = a[i]; + tween && tween._lazy && (tween.render(tween._lazy[0], tween._lazy[1], true)._lazy = 0); + } + }, + _lazySafeRender = function _lazySafeRender(animation, time, suppressEvents, force) { + _lazyTweens.length && !_reverting && _lazyRender(); + animation.render(time, suppressEvents, force || _reverting && time < 0 && (animation._initted || animation._startAt)); + _lazyTweens.length && !_reverting && _lazyRender(); + }, + _numericIfPossible = function _numericIfPossible(value) { + var n = parseFloat(value); + return (n || n === 0) && (value + "").match(_delimitedValueExp).length < 2 ? n : _isString(value) ? value.trim() : value; + }, + _passThrough = function _passThrough(p) { + return p; + }, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + p in obj || (obj[p] = defaults[p]); + } + + return obj; + }, + _setKeyframeDefaults = function _setKeyframeDefaults(excludeDuration) { + return function (obj, defaults) { + for (var p in defaults) { + p in obj || p === "duration" && excludeDuration || p === "ease" || (obj[p] = defaults[p]); + } + }; + }, + _merge = function _merge(base, toMerge) { + for (var p in toMerge) { + base[p] = toMerge[p]; + } + + return base; + }, + _mergeDeep = function _mergeDeep(base, toMerge) { + for (var p in toMerge) { + p !== "__proto__" && p !== "constructor" && p !== "prototype" && (base[p] = _isObject(toMerge[p]) ? _mergeDeep(base[p] || (base[p] = {}), toMerge[p]) : toMerge[p]); + } + + return base; + }, + _copyExcluding = function _copyExcluding(obj, excluding) { + var copy = {}, + p; + + for (p in obj) { + p in excluding || (copy[p] = obj[p]); + } + + return copy; + }, + _inheritDefaults = function _inheritDefaults(vars) { + var parent = vars.parent || _globalTimeline, + func = vars.keyframes ? _setKeyframeDefaults(_isArray(vars.keyframes)) : _setDefaults; + + if (_isNotFalse(vars.inherit)) { + while (parent) { + func(vars, parent.vars.defaults); + parent = parent.parent || parent._dp; + } + } + + return vars; + }, + _arraysMatch = function _arraysMatch(a1, a2) { + var i = a1.length, + match = i === a2.length; + + while (match && i-- && a1[i] === a2[i]) {} + + return i < 0; + }, + _addLinkedListItem = function _addLinkedListItem(parent, child, firstProp, lastProp, sortBy) { + if (firstProp === void 0) { + firstProp = "_first"; + } + + if (lastProp === void 0) { + lastProp = "_last"; + } + + var prev = parent[lastProp], + t; + + if (sortBy) { + t = child[sortBy]; + + while (prev && prev[sortBy] > t) { + prev = prev._prev; + } + } + + if (prev) { + child._next = prev._next; + prev._next = child; + } else { + child._next = parent[firstProp]; + parent[firstProp] = child; + } + + if (child._next) { + child._next._prev = child; + } else { + parent[lastProp] = child; + } + + child._prev = prev; + child.parent = child._dp = parent; + return child; + }, + _removeLinkedListItem = function _removeLinkedListItem(parent, child, firstProp, lastProp) { + if (firstProp === void 0) { + firstProp = "_first"; + } + + if (lastProp === void 0) { + lastProp = "_last"; + } + + var prev = child._prev, + next = child._next; + + if (prev) { + prev._next = next; + } else if (parent[firstProp] === child) { + parent[firstProp] = next; + } + + if (next) { + next._prev = prev; + } else if (parent[lastProp] === child) { + parent[lastProp] = prev; + } + + child._next = child._prev = child.parent = null; + }, + _removeFromParent = function _removeFromParent(child, onlyIfParentHasAutoRemove) { + child.parent && (!onlyIfParentHasAutoRemove || child.parent.autoRemoveChildren) && child.parent.remove && child.parent.remove(child); + child._act = 0; + }, + _uncache = function _uncache(animation, child) { + if (animation && (!child || child._end > animation._dur || child._start < 0)) { + var a = animation; + + while (a) { + a._dirty = 1; + a = a.parent; + } + } + + return animation; + }, + _recacheAncestors = function _recacheAncestors(animation) { + var parent = animation.parent; + + while (parent && parent.parent) { + parent._dirty = 1; + parent.totalDuration(); + parent = parent.parent; + } + + return animation; + }, + _rewindStartAt = function _rewindStartAt(tween, totalTime, suppressEvents, force) { + return tween._startAt && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween.vars.immediateRender && !tween.vars.autoRevert || tween._startAt.render(totalTime, true, force)); + }, + _hasNoPausedAncestors = function _hasNoPausedAncestors(animation) { + return !animation || animation._ts && _hasNoPausedAncestors(animation.parent); + }, + _elapsedCycleDuration = function _elapsedCycleDuration(animation) { + return animation._repeat ? _animationCycle(animation._tTime, animation = animation.duration() + animation._rDelay) * animation : 0; + }, + _animationCycle = function _animationCycle(tTime, cycleDuration) { + var whole = Math.floor(tTime = _roundPrecise(tTime / cycleDuration)); + return tTime && whole === tTime ? whole - 1 : whole; + }, + _parentToChildTotalTime = function _parentToChildTotalTime(parentTime, child) { + return (parentTime - child._start) * child._ts + (child._ts >= 0 ? 0 : child._dirty ? child.totalDuration() : child._tDur); + }, + _setEnd = function _setEnd(animation) { + return animation._end = _roundPrecise(animation._start + (animation._tDur / Math.abs(animation._ts || animation._rts || _tinyNum) || 0)); + }, + _alignPlayhead = function _alignPlayhead(animation, totalTime) { + var parent = animation._dp; + + if (parent && parent.smoothChildTiming && animation._ts) { + animation._start = _roundPrecise(parent._time - (animation._ts > 0 ? totalTime / animation._ts : ((animation._dirty ? animation.totalDuration() : animation._tDur) - totalTime) / -animation._ts)); + + _setEnd(animation); + + parent._dirty || _uncache(parent, animation); + } + + return animation; + }, + _postAddChecks = function _postAddChecks(timeline, child) { + var t; + + if (child._time || !child._dur && child._initted || child._start < timeline._time && (child._dur || !child.add)) { + t = _parentToChildTotalTime(timeline.rawTime(), child); + + if (!child._dur || _clamp(0, child.totalDuration(), t) - child._tTime > _tinyNum) { + child.render(t, true); + } + } + + if (_uncache(timeline, child)._dp && timeline._initted && timeline._time >= timeline._dur && timeline._ts) { + if (timeline._dur < timeline.duration()) { + t = timeline; + + while (t._dp) { + t.rawTime() >= 0 && t.totalTime(t._tTime); + t = t._dp; + } + } + + timeline._zTime = -_tinyNum; + } + }, + _addToTimeline = function _addToTimeline(timeline, child, position, skipChecks) { + child.parent && _removeFromParent(child); + child._start = _roundPrecise((_isNumber(position) ? position : position || timeline !== _globalTimeline ? _parsePosition(timeline, position, child) : timeline._time) + child._delay); + child._end = _roundPrecise(child._start + (child.totalDuration() / Math.abs(child.timeScale()) || 0)); + + _addLinkedListItem(timeline, child, "_first", "_last", timeline._sort ? "_start" : 0); + + _isFromOrFromStart(child) || (timeline._recent = child); + skipChecks || _postAddChecks(timeline, child); + timeline._ts < 0 && _alignPlayhead(timeline, timeline._tTime); + return timeline; + }, + _scrollTrigger = function _scrollTrigger(animation, trigger) { + return (_globals.ScrollTrigger || _missingPlugin("scrollTrigger", trigger)) && _globals.ScrollTrigger.create(trigger, animation); + }, + _attemptInitTween = function _attemptInitTween(tween, time, force, suppressEvents, tTime) { + _initTween(tween, time, tTime); + + if (!tween._initted) { + return 1; + } + + if (!force && tween._pt && !_reverting && (tween._dur && tween.vars.lazy !== false || !tween._dur && tween.vars.lazy) && _lastRenderedFrame !== _ticker.frame) { + _lazyTweens.push(tween); + + tween._lazy = [tTime, suppressEvents]; + return 1; + } + }, + _parentPlayheadIsBeforeStart = function _parentPlayheadIsBeforeStart(_ref) { + var parent = _ref.parent; + return parent && parent._ts && parent._initted && !parent._lock && (parent.rawTime() < 0 || _parentPlayheadIsBeforeStart(parent)); + }, + _isFromOrFromStart = function _isFromOrFromStart(_ref2) { + var data = _ref2.data; + return data === "isFromStart" || data === "isStart"; + }, + _renderZeroDurationTween = function _renderZeroDurationTween(tween, totalTime, suppressEvents, force) { + var prevRatio = tween.ratio, + ratio = totalTime < 0 || !totalTime && (!tween._start && _parentPlayheadIsBeforeStart(tween) && !(!tween._initted && _isFromOrFromStart(tween)) || (tween._ts < 0 || tween._dp._ts < 0) && !_isFromOrFromStart(tween)) ? 0 : 1, + repeatDelay = tween._rDelay, + tTime = 0, + pt, + iteration, + prevIteration; + + if (repeatDelay && tween._repeat) { + tTime = _clamp(0, tween._tDur, totalTime); + iteration = _animationCycle(tTime, repeatDelay); + tween._yoyo && iteration & 1 && (ratio = 1 - ratio); + + if (iteration !== _animationCycle(tween._tTime, repeatDelay)) { + prevRatio = 1 - ratio; + tween.vars.repeatRefresh && tween._initted && tween.invalidate(); + } + } + + if (ratio !== prevRatio || _reverting || force || tween._zTime === _tinyNum || !totalTime && tween._zTime) { + if (!tween._initted && _attemptInitTween(tween, totalTime, force, suppressEvents, tTime)) { + return; + } + + prevIteration = tween._zTime; + tween._zTime = totalTime || (suppressEvents ? _tinyNum : 0); + suppressEvents || (suppressEvents = totalTime && !prevIteration); + tween.ratio = ratio; + tween._from && (ratio = 1 - ratio); + tween._time = 0; + tween._tTime = tTime; + pt = tween._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + totalTime < 0 && _rewindStartAt(tween, totalTime, suppressEvents, true); + tween._onUpdate && !suppressEvents && _callback(tween, "onUpdate"); + tTime && tween._repeat && !suppressEvents && tween.parent && _callback(tween, "onRepeat"); + + if ((totalTime >= tween._tDur || totalTime < 0) && tween.ratio === ratio) { + ratio && _removeFromParent(tween, 1); + + if (!suppressEvents && !_reverting) { + _callback(tween, ratio ? "onComplete" : "onReverseComplete", true); + + tween._prom && tween._prom(); + } + } + } else if (!tween._zTime) { + tween._zTime = totalTime; + } + }, + _findNextPauseTween = function _findNextPauseTween(animation, prevTime, time) { + var child; + + if (time > prevTime) { + child = animation._first; + + while (child && child._start <= time) { + if (child.data === "isPause" && child._start > prevTime) { + return child; + } + + child = child._next; + } + } else { + child = animation._last; + + while (child && child._start >= time) { + if (child.data === "isPause" && child._start < prevTime) { + return child; + } + + child = child._prev; + } + } + }, + _setDuration = function _setDuration(animation, duration, skipUncache, leavePlayhead) { + var repeat = animation._repeat, + dur = _roundPrecise(duration) || 0, + totalProgress = animation._tTime / animation._tDur; + totalProgress && !leavePlayhead && (animation._time *= dur / animation._dur); + animation._dur = dur; + animation._tDur = !repeat ? dur : repeat < 0 ? 1e10 : _roundPrecise(dur * (repeat + 1) + animation._rDelay * repeat); + totalProgress > 0 && !leavePlayhead && _alignPlayhead(animation, animation._tTime = animation._tDur * totalProgress); + animation.parent && _setEnd(animation); + skipUncache || _uncache(animation.parent, animation); + return animation; + }, + _onUpdateTotalDuration = function _onUpdateTotalDuration(animation) { + return animation instanceof Timeline ? _uncache(animation) : _setDuration(animation, animation._dur); + }, + _zeroPosition = { + _start: 0, + endTime: _emptyFunc, + totalDuration: _emptyFunc + }, + _parsePosition = function _parsePosition(animation, position, percentAnimation) { + var labels = animation.labels, + recent = animation._recent || _zeroPosition, + clippedDuration = animation.duration() >= _bigNum ? recent.endTime(false) : animation._dur, + i, + offset, + isPercent; + + if (_isString(position) && (isNaN(position) || position in labels)) { + offset = position.charAt(0); + isPercent = position.substr(-1) === "%"; + i = position.indexOf("="); + + if (offset === "<" || offset === ">") { + i >= 0 && (position = position.replace(/=/, "")); + return (offset === "<" ? recent._start : recent.endTime(recent._repeat >= 0)) + (parseFloat(position.substr(1)) || 0) * (isPercent ? (i < 0 ? recent : percentAnimation).totalDuration() / 100 : 1); + } + + if (i < 0) { + position in labels || (labels[position] = clippedDuration); + return labels[position]; + } + + offset = parseFloat(position.charAt(i - 1) + position.substr(i + 1)); + + if (isPercent && percentAnimation) { + offset = offset / 100 * (_isArray(percentAnimation) ? percentAnimation[0] : percentAnimation).totalDuration(); + } + + return i > 1 ? _parsePosition(animation, position.substr(0, i - 1), percentAnimation) + offset : clippedDuration + offset; + } + + return position == null ? clippedDuration : +position; + }, + _createTweenType = function _createTweenType(type, params, timeline) { + var isLegacy = _isNumber(params[1]), + varsIndex = (isLegacy ? 2 : 1) + (type < 2 ? 0 : 1), + vars = params[varsIndex], + irVars, + parent; + + isLegacy && (vars.duration = params[1]); + vars.parent = timeline; + + if (type) { + irVars = vars; + parent = timeline; + + while (parent && !("immediateRender" in irVars)) { + irVars = parent.vars.defaults || {}; + parent = _isNotFalse(parent.vars.inherit) && parent.parent; + } + + vars.immediateRender = _isNotFalse(irVars.immediateRender); + type < 2 ? vars.runBackwards = 1 : vars.startAt = params[varsIndex - 1]; + } + + return new Tween(params[0], vars, params[varsIndex + 1]); + }, + _conditionalReturn = function _conditionalReturn(value, func) { + return value || value === 0 ? func(value) : func; + }, + _clamp = function _clamp(min, max, value) { + return value < min ? min : value > max ? max : value; + }, + getUnit = function getUnit(value, v) { + return !_isString(value) || !(v = _unitExp.exec(value)) ? "" : v[1]; + }, + clamp = function clamp(min, max, value) { + return _conditionalReturn(value, function (v) { + return _clamp(min, max, v); + }); + }, + _slice = [].slice, + _isArrayLike = function _isArrayLike(value, nonEmpty) { + return value && _isObject(value) && "length" in value && (!nonEmpty && !value.length || value.length - 1 in value && _isObject(value[0])) && !value.nodeType && value !== _win; + }, + _flatten = function _flatten(ar, leaveStrings, accumulator) { + if (accumulator === void 0) { + accumulator = []; + } + + return ar.forEach(function (value) { + var _accumulator; + + return _isString(value) && !leaveStrings || _isArrayLike(value, 1) ? (_accumulator = accumulator).push.apply(_accumulator, toArray(value)) : accumulator.push(value); + }) || accumulator; + }, + toArray = function toArray(value, scope, leaveStrings) { + return _context && !scope && _context.selector ? _context.selector(value) : _isString(value) && !leaveStrings && (_coreInitted || !_wake()) ? _slice.call((scope || _doc).querySelectorAll(value), 0) : _isArray(value) ? _flatten(value, leaveStrings) : _isArrayLike(value) ? _slice.call(value, 0) : value ? [value] : []; + }, + selector = function selector(value) { + value = toArray(value)[0] || _warn("Invalid scope") || {}; + return function (v) { + var el = value.current || value.nativeElement || value; + return toArray(v, el.querySelectorAll ? el : el === value ? _warn("Invalid scope") || _doc.createElement("div") : value); + }; + }, + shuffle = function shuffle(a) { + return a.sort(function () { + return .5 - Math.random(); + }); + }, + distribute = function distribute(v) { + if (_isFunction(v)) { + return v; + } + + var vars = _isObject(v) ? v : { + each: v + }, + ease = _parseEase(vars.ease), + from = vars.from || 0, + base = parseFloat(vars.base) || 0, + cache = {}, + isDecimal = from > 0 && from < 1, + ratios = isNaN(from) || isDecimal, + axis = vars.axis, + ratioX = from, + ratioY = from; + + if (_isString(from)) { + ratioX = ratioY = { + center: .5, + edges: .5, + end: 1 + }[from] || 0; + } else if (!isDecimal && ratios) { + ratioX = from[0]; + ratioY = from[1]; + } + + return function (i, target, a) { + var l = (a || vars).length, + distances = cache[l], + originX, + originY, + x, + y, + d, + j, + max, + min, + wrapAt; + + if (!distances) { + wrapAt = vars.grid === "auto" ? 0 : (vars.grid || [1, _bigNum])[1]; + + if (!wrapAt) { + max = -_bigNum; + + while (max < (max = a[wrapAt++].getBoundingClientRect().left) && wrapAt < l) {} + + wrapAt < l && wrapAt--; + } + + distances = cache[l] = []; + originX = ratios ? Math.min(wrapAt, l) * ratioX - .5 : from % wrapAt; + originY = wrapAt === _bigNum ? 0 : ratios ? l * ratioY / wrapAt - .5 : from / wrapAt | 0; + max = 0; + min = _bigNum; + + for (j = 0; j < l; j++) { + x = j % wrapAt - originX; + y = originY - (j / wrapAt | 0); + distances[j] = d = !axis ? _sqrt(x * x + y * y) : Math.abs(axis === "y" ? y : x); + d > max && (max = d); + d < min && (min = d); + } + + from === "random" && shuffle(distances); + distances.max = max - min; + distances.min = min; + distances.v = l = (parseFloat(vars.amount) || parseFloat(vars.each) * (wrapAt > l ? l - 1 : !axis ? Math.max(wrapAt, l / wrapAt) : axis === "y" ? l / wrapAt : wrapAt) || 0) * (from === "edges" ? -1 : 1); + distances.b = l < 0 ? base - l : base; + distances.u = getUnit(vars.amount || vars.each) || 0; + ease = ease && l < 0 ? _invertEase(ease) : ease; + } + + l = (distances[i] - distances.min) / distances.max || 0; + return _roundPrecise(distances.b + (ease ? ease(l) : l) * distances.v) + distances.u; + }; + }, + _roundModifier = function _roundModifier(v) { + var p = Math.pow(10, ((v + "").split(".")[1] || "").length); + return function (raw) { + var n = _roundPrecise(Math.round(parseFloat(raw) / v) * v * p); + + return (n - n % 1) / p + (_isNumber(raw) ? 0 : getUnit(raw)); + }; + }, + snap = function snap(snapTo, value) { + var isArray = _isArray(snapTo), + radius, + is2D; + + if (!isArray && _isObject(snapTo)) { + radius = isArray = snapTo.radius || _bigNum; + + if (snapTo.values) { + snapTo = toArray(snapTo.values); + + if (is2D = !_isNumber(snapTo[0])) { + radius *= radius; + } + } else { + snapTo = _roundModifier(snapTo.increment); + } + } + + return _conditionalReturn(value, !isArray ? _roundModifier(snapTo) : _isFunction(snapTo) ? function (raw) { + is2D = snapTo(raw); + return Math.abs(is2D - raw) <= radius ? is2D : raw; + } : function (raw) { + var x = parseFloat(is2D ? raw.x : raw), + y = parseFloat(is2D ? raw.y : 0), + min = _bigNum, + closest = 0, + i = snapTo.length, + dx, + dy; + + while (i--) { + if (is2D) { + dx = snapTo[i].x - x; + dy = snapTo[i].y - y; + dx = dx * dx + dy * dy; + } else { + dx = Math.abs(snapTo[i] - x); + } + + if (dx < min) { + min = dx; + closest = i; + } + } + + closest = !radius || min <= radius ? snapTo[closest] : raw; + return is2D || closest === raw || _isNumber(raw) ? closest : closest + getUnit(raw); + }); + }, + random = function random(min, max, roundingIncrement, returnFunction) { + return _conditionalReturn(_isArray(min) ? !max : roundingIncrement === true ? !!(roundingIncrement = 0) : !returnFunction, function () { + return _isArray(min) ? min[~~(Math.random() * min.length)] : (roundingIncrement = roundingIncrement || 1e-5) && (returnFunction = roundingIncrement < 1 ? Math.pow(10, (roundingIncrement + "").length - 2) : 1) && Math.floor(Math.round((min - roundingIncrement / 2 + Math.random() * (max - min + roundingIncrement * .99)) / roundingIncrement) * roundingIncrement * returnFunction) / returnFunction; + }); + }, + pipe = function pipe() { + for (var _len = arguments.length, functions = new Array(_len), _key = 0; _key < _len; _key++) { + functions[_key] = arguments[_key]; + } + + return function (value) { + return functions.reduce(function (v, f) { + return f(v); + }, value); + }; + }, + unitize = function unitize(func, unit) { + return function (value) { + return func(parseFloat(value)) + (unit || getUnit(value)); + }; + }, + normalize = function normalize(min, max, value) { + return mapRange(min, max, 0, 1, value); + }, + _wrapArray = function _wrapArray(a, wrapper, value) { + return _conditionalReturn(value, function (index) { + return a[~~wrapper(index)]; + }); + }, + wrap = function wrap(min, max, value) { + var range = max - min; + return _isArray(min) ? _wrapArray(min, wrap(0, min.length), max) : _conditionalReturn(value, function (value) { + return (range + (value - min) % range) % range + min; + }); + }, + wrapYoyo = function wrapYoyo(min, max, value) { + var range = max - min, + total = range * 2; + return _isArray(min) ? _wrapArray(min, wrapYoyo(0, min.length - 1), max) : _conditionalReturn(value, function (value) { + value = (total + (value - min) % total) % total || 0; + return min + (value > range ? total - value : value); + }); + }, + _replaceRandom = function _replaceRandom(value) { + var prev = 0, + s = "", + i, + nums, + end, + isArray; + + while (~(i = value.indexOf("random(", prev))) { + end = value.indexOf(")", i); + isArray = value.charAt(i + 7) === "["; + nums = value.substr(i + 7, end - i - 7).match(isArray ? _delimitedValueExp : _strictNumExp); + s += value.substr(prev, i - prev) + random(isArray ? nums : +nums[0], isArray ? 0 : +nums[1], +nums[2] || 1e-5); + prev = end + 1; + } + + return s + value.substr(prev, value.length - prev); + }, + mapRange = function mapRange(inMin, inMax, outMin, outMax, value) { + var inRange = inMax - inMin, + outRange = outMax - outMin; + return _conditionalReturn(value, function (value) { + return outMin + ((value - inMin) / inRange * outRange || 0); + }); + }, + interpolate = function interpolate(start, end, progress, mutate) { + var func = isNaN(start + end) ? 0 : function (p) { + return (1 - p) * start + p * end; + }; + + if (!func) { + var isString = _isString(start), + master = {}, + p, + i, + interpolators, + l, + il; + + progress === true && (mutate = 1) && (progress = null); + + if (isString) { + start = { + p: start + }; + end = { + p: end + }; + } else if (_isArray(start) && !_isArray(end)) { + interpolators = []; + l = start.length; + il = l - 2; + + for (i = 1; i < l; i++) { + interpolators.push(interpolate(start[i - 1], start[i])); + } + + l--; + + func = function func(p) { + p *= l; + var i = Math.min(il, ~~p); + return interpolators[i](p - i); + }; + + progress = end; + } else if (!mutate) { + start = _merge(_isArray(start) ? [] : {}, start); + } + + if (!interpolators) { + for (p in end) { + _addPropTween.call(master, start, p, "get", end[p]); + } + + func = function func(p) { + return _renderPropTweens(p, master) || (isString ? start.p : start); + }; + } + } + + return _conditionalReturn(progress, func); + }, + _getLabelInDirection = function _getLabelInDirection(timeline, fromTime, backward) { + var labels = timeline.labels, + min = _bigNum, + p, + distance, + label; + + for (p in labels) { + distance = labels[p] - fromTime; + + if (distance < 0 === !!backward && distance && min > (distance = Math.abs(distance))) { + label = p; + min = distance; + } + } + + return label; + }, + _callback = function _callback(animation, type, executeLazyFirst) { + var v = animation.vars, + callback = v[type], + prevContext = _context, + context = animation._ctx, + params, + scope, + result; + + if (!callback) { + return; + } + + params = v[type + "Params"]; + scope = v.callbackScope || animation; + executeLazyFirst && _lazyTweens.length && _lazyRender(); + context && (_context = context); + result = params ? callback.apply(scope, params) : callback.call(scope); + _context = prevContext; + return result; + }, + _interrupt = function _interrupt(animation) { + _removeFromParent(animation); + + animation.scrollTrigger && animation.scrollTrigger.kill(!!_reverting); + animation.progress() < 1 && _callback(animation, "onInterrupt"); + return animation; + }, + _quickTween, + _registerPluginQueue = [], + _createPlugin = function _createPlugin(config) { + if (!config) return; + config = !config.name && config["default"] || config; + + if (_windowExists() || config.headless) { + var name = config.name, + isFunc = _isFunction(config), + Plugin = name && !isFunc && config.init ? function () { + this._props = []; + } : config, + instanceDefaults = { + init: _emptyFunc, + render: _renderPropTweens, + add: _addPropTween, + kill: _killPropTweensOf, + modifier: _addPluginModifier, + rawVars: 0 + }, + statics = { + targetTest: 0, + get: 0, + getSetter: _getSetter, + aliases: {}, + register: 0 + }; + + _wake(); + + if (config !== Plugin) { + if (_plugins[name]) { + return; + } + + _setDefaults(Plugin, _setDefaults(_copyExcluding(config, instanceDefaults), statics)); + + _merge(Plugin.prototype, _merge(instanceDefaults, _copyExcluding(config, statics))); + + _plugins[Plugin.prop = name] = Plugin; + + if (config.targetTest) { + _harnessPlugins.push(Plugin); + + _reservedProps[name] = 1; + } + + name = (name === "css" ? "CSS" : name.charAt(0).toUpperCase() + name.substr(1)) + "Plugin"; + } + + _addGlobal(name, Plugin); + + config.register && config.register(gsap, Plugin, PropTween); + } else { + _registerPluginQueue.push(config); + } + }, + _255 = 255, + _colorLookup = { + aqua: [0, _255, _255], + lime: [0, _255, 0], + silver: [192, 192, 192], + black: [0, 0, 0], + maroon: [128, 0, 0], + teal: [0, 128, 128], + blue: [0, 0, _255], + navy: [0, 0, 128], + white: [_255, _255, _255], + olive: [128, 128, 0], + yellow: [_255, _255, 0], + orange: [_255, 165, 0], + gray: [128, 128, 128], + purple: [128, 0, 128], + green: [0, 128, 0], + red: [_255, 0, 0], + pink: [_255, 192, 203], + cyan: [0, _255, _255], + transparent: [_255, _255, _255, 0] + }, + _hue = function _hue(h, m1, m2) { + h += h < 0 ? 1 : h > 1 ? -1 : 0; + return (h * 6 < 1 ? m1 + (m2 - m1) * h * 6 : h < .5 ? m2 : h * 3 < 2 ? m1 + (m2 - m1) * (2 / 3 - h) * 6 : m1) * _255 + .5 | 0; + }, + splitColor = function splitColor(v, toHSL, forceAlpha) { + var a = !v ? _colorLookup.black : _isNumber(v) ? [v >> 16, v >> 8 & _255, v & _255] : 0, + r, + g, + b, + h, + s, + l, + max, + min, + d, + wasHSL; + + if (!a) { + if (v.substr(-1) === ",") { + v = v.substr(0, v.length - 1); + } + + if (_colorLookup[v]) { + a = _colorLookup[v]; + } else if (v.charAt(0) === "#") { + if (v.length < 6) { + r = v.charAt(1); + g = v.charAt(2); + b = v.charAt(3); + v = "#" + r + r + g + g + b + b + (v.length === 5 ? v.charAt(4) + v.charAt(4) : ""); + } + + if (v.length === 9) { + a = parseInt(v.substr(1, 6), 16); + return [a >> 16, a >> 8 & _255, a & _255, parseInt(v.substr(7), 16) / 255]; + } + + v = parseInt(v.substr(1), 16); + a = [v >> 16, v >> 8 & _255, v & _255]; + } else if (v.substr(0, 3) === "hsl") { + a = wasHSL = v.match(_strictNumExp); + + if (!toHSL) { + h = +a[0] % 360 / 360; + s = +a[1] / 100; + l = +a[2] / 100; + g = l <= .5 ? l * (s + 1) : l + s - l * s; + r = l * 2 - g; + a.length > 3 && (a[3] *= 1); + a[0] = _hue(h + 1 / 3, r, g); + a[1] = _hue(h, r, g); + a[2] = _hue(h - 1 / 3, r, g); + } else if (~v.indexOf("=")) { + a = v.match(_numExp); + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + } + } else { + a = v.match(_strictNumExp) || _colorLookup.transparent; + } + + a = a.map(Number); + } + + if (toHSL && !wasHSL) { + r = a[0] / _255; + g = a[1] / _255; + b = a[2] / _255; + max = Math.max(r, g, b); + min = Math.min(r, g, b); + l = (max + min) / 2; + + if (max === min) { + h = s = 0; + } else { + d = max - min; + s = l > 0.5 ? d / (2 - max - min) : d / (max + min); + h = max === r ? (g - b) / d + (g < b ? 6 : 0) : max === g ? (b - r) / d + 2 : (r - g) / d + 4; + h *= 60; + } + + a[0] = ~~(h + .5); + a[1] = ~~(s * 100 + .5); + a[2] = ~~(l * 100 + .5); + } + + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + }, + _colorOrderData = function _colorOrderData(v) { + var values = [], + c = [], + i = -1; + v.split(_colorExp).forEach(function (v) { + var a = v.match(_numWithUnitExp) || []; + values.push.apply(values, a); + c.push(i += a.length + 1); + }); + values.c = c; + return values; + }, + _formatColors = function _formatColors(s, toHSL, orderMatchData) { + var result = "", + colors = (s + result).match(_colorExp), + type = toHSL ? "hsla(" : "rgba(", + i = 0, + c, + shell, + d, + l; + + if (!colors) { + return s; + } + + colors = colors.map(function (color) { + return (color = splitColor(color, toHSL, 1)) && type + (toHSL ? color[0] + "," + color[1] + "%," + color[2] + "%," + color[3] : color.join(",")) + ")"; + }); + + if (orderMatchData) { + d = _colorOrderData(s); + c = orderMatchData.c; + + if (c.join(result) !== d.c.join(result)) { + shell = s.replace(_colorExp, "1").split(_numWithUnitExp); + l = shell.length - 1; + + for (; i < l; i++) { + result += shell[i] + (~c.indexOf(i) ? colors.shift() || type + "0,0,0,0)" : (d.length ? d : colors.length ? colors : orderMatchData).shift()); + } + } + } + + if (!shell) { + shell = s.split(_colorExp); + l = shell.length - 1; + + for (; i < l; i++) { + result += shell[i] + colors[i]; + } + } + + return result + shell[l]; + }, + _colorExp = function () { + var s = "(?:\\b(?:(?:rgb|rgba|hsl|hsla)\\(.+?\\))|\\B#(?:[0-9a-f]{3,4}){1,2}\\b", + p; + + for (p in _colorLookup) { + s += "|" + p + "\\b"; + } + + return new RegExp(s + ")", "gi"); + }(), + _hslExp = /hsl[a]?\(/, + _colorStringFilter = function _colorStringFilter(a) { + var combined = a.join(" "), + toHSL; + _colorExp.lastIndex = 0; + + if (_colorExp.test(combined)) { + toHSL = _hslExp.test(combined); + a[1] = _formatColors(a[1], toHSL); + a[0] = _formatColors(a[0], toHSL, _colorOrderData(a[1])); + return true; + } + }, + _tickerActive, + _ticker = function () { + var _getTime = Date.now, + _lagThreshold = 500, + _adjustedLag = 33, + _startTime = _getTime(), + _lastUpdate = _startTime, + _gap = 1000 / 240, + _nextTime = _gap, + _listeners = [], + _id, + _req, + _raf, + _self, + _delta, + _i, + _tick = function _tick(v) { + var elapsed = _getTime() - _lastUpdate, + manual = v === true, + overlap, + dispatch, + time, + frame; + + (elapsed > _lagThreshold || elapsed < 0) && (_startTime += elapsed - _adjustedLag); + _lastUpdate += elapsed; + time = _lastUpdate - _startTime; + overlap = time - _nextTime; + + if (overlap > 0 || manual) { + frame = ++_self.frame; + _delta = time - _self.time * 1000; + _self.time = time = time / 1000; + _nextTime += overlap + (overlap >= _gap ? 4 : _gap - overlap); + dispatch = 1; + } + + manual || (_id = _req(_tick)); + + if (dispatch) { + for (_i = 0; _i < _listeners.length; _i++) { + _listeners[_i](time, _delta, frame, v); + } + } + }; + + _self = { + time: 0, + frame: 0, + tick: function tick() { + _tick(true); + }, + deltaRatio: function deltaRatio(fps) { + return _delta / (1000 / (fps || 60)); + }, + wake: function wake() { + if (_coreReady) { + if (!_coreInitted && _windowExists()) { + _win = _coreInitted = window; + _doc = _win.document || {}; + _globals.gsap = gsap; + (_win.gsapVersions || (_win.gsapVersions = [])).push(gsap.version); + + _install(_installScope || _win.GreenSockGlobals || !_win.gsap && _win || {}); + + _registerPluginQueue.forEach(_createPlugin); + } + + _raf = typeof requestAnimationFrame !== "undefined" && requestAnimationFrame; + _id && _self.sleep(); + + _req = _raf || function (f) { + return setTimeout(f, _nextTime - _self.time * 1000 + 1 | 0); + }; + + _tickerActive = 1; + + _tick(2); + } + }, + sleep: function sleep() { + (_raf ? cancelAnimationFrame : clearTimeout)(_id); + _tickerActive = 0; + _req = _emptyFunc; + }, + lagSmoothing: function lagSmoothing(threshold, adjustedLag) { + _lagThreshold = threshold || Infinity; + _adjustedLag = Math.min(adjustedLag || 33, _lagThreshold); + }, + fps: function fps(_fps) { + _gap = 1000 / (_fps || 240); + _nextTime = _self.time * 1000 + _gap; + }, + add: function add(callback, once, prioritize) { + var func = once ? function (t, d, f, v) { + callback(t, d, f, v); + + _self.remove(func); + } : callback; + + _self.remove(callback); + + _listeners[prioritize ? "unshift" : "push"](func); + + _wake(); + + return func; + }, + remove: function remove(callback, i) { + ~(i = _listeners.indexOf(callback)) && _listeners.splice(i, 1) && _i >= i && _i--; + }, + _listeners: _listeners + }; + return _self; + }(), + _wake = function _wake() { + return !_tickerActive && _ticker.wake(); + }, + _easeMap = {}, + _customEaseExp = /^[\d.\-M][\d.\-,\s]/, + _quotesExp = /["']/g, + _parseObjectInString = function _parseObjectInString(value) { + var obj = {}, + split = value.substr(1, value.length - 3).split(":"), + key = split[0], + i = 1, + l = split.length, + index, + val, + parsedVal; + + for (; i < l; i++) { + val = split[i]; + index = i !== l - 1 ? val.lastIndexOf(",") : val.length; + parsedVal = val.substr(0, index); + obj[key] = isNaN(parsedVal) ? parsedVal.replace(_quotesExp, "").trim() : +parsedVal; + key = val.substr(index + 1).trim(); + } + + return obj; + }, + _valueInParentheses = function _valueInParentheses(value) { + var open = value.indexOf("(") + 1, + close = value.indexOf(")"), + nested = value.indexOf("(", open); + return value.substring(open, ~nested && nested < close ? value.indexOf(")", close + 1) : close); + }, + _configEaseFromString = function _configEaseFromString(name) { + var split = (name + "").split("("), + ease = _easeMap[split[0]]; + return ease && split.length > 1 && ease.config ? ease.config.apply(null, ~name.indexOf("{") ? [_parseObjectInString(split[1])] : _valueInParentheses(name).split(",").map(_numericIfPossible)) : _easeMap._CE && _customEaseExp.test(name) ? _easeMap._CE("", name) : ease; + }, + _invertEase = function _invertEase(ease) { + return function (p) { + return 1 - ease(1 - p); + }; + }, + _propagateYoyoEase = function _propagateYoyoEase(timeline, isYoyo) { + var child = timeline._first, + ease; + + while (child) { + if (child instanceof Timeline) { + _propagateYoyoEase(child, isYoyo); + } else if (child.vars.yoyoEase && (!child._yoyo || !child._repeat) && child._yoyo !== isYoyo) { + if (child.timeline) { + _propagateYoyoEase(child.timeline, isYoyo); + } else { + ease = child._ease; + child._ease = child._yEase; + child._yEase = ease; + child._yoyo = isYoyo; + } + } + + child = child._next; + } + }, + _parseEase = function _parseEase(ease, defaultEase) { + return !ease ? defaultEase : (_isFunction(ease) ? ease : _easeMap[ease] || _configEaseFromString(ease)) || defaultEase; + }, + _insertEase = function _insertEase(names, easeIn, easeOut, easeInOut) { + if (easeOut === void 0) { + easeOut = function easeOut(p) { + return 1 - easeIn(1 - p); + }; + } + + if (easeInOut === void 0) { + easeInOut = function easeInOut(p) { + return p < .5 ? easeIn(p * 2) / 2 : 1 - easeIn((1 - p) * 2) / 2; + }; + } + + var ease = { + easeIn: easeIn, + easeOut: easeOut, + easeInOut: easeInOut + }, + lowercaseName; + + _forEachName(names, function (name) { + _easeMap[name] = _globals[name] = ease; + _easeMap[lowercaseName = name.toLowerCase()] = easeOut; + + for (var p in ease) { + _easeMap[lowercaseName + (p === "easeIn" ? ".in" : p === "easeOut" ? ".out" : ".inOut")] = _easeMap[name + "." + p] = ease[p]; + } + }); + + return ease; + }, + _easeInOutFromOut = function _easeInOutFromOut(easeOut) { + return function (p) { + return p < .5 ? (1 - easeOut(1 - p * 2)) / 2 : .5 + easeOut((p - .5) * 2) / 2; + }; + }, + _configElastic = function _configElastic(type, amplitude, period) { + var p1 = amplitude >= 1 ? amplitude : 1, + p2 = (period || (type ? .3 : .45)) / (amplitude < 1 ? amplitude : 1), + p3 = p2 / _2PI * (Math.asin(1 / p1) || 0), + easeOut = function easeOut(p) { + return p === 1 ? 1 : p1 * Math.pow(2, -10 * p) * _sin((p - p3) * p2) + 1; + }, + ease = type === "out" ? easeOut : type === "in" ? function (p) { + return 1 - easeOut(1 - p); + } : _easeInOutFromOut(easeOut); + + p2 = _2PI / p2; + + ease.config = function (amplitude, period) { + return _configElastic(type, amplitude, period); + }; + + return ease; + }, + _configBack = function _configBack(type, overshoot) { + if (overshoot === void 0) { + overshoot = 1.70158; + } + + var easeOut = function easeOut(p) { + return p ? --p * p * ((overshoot + 1) * p + overshoot) + 1 : 0; + }, + ease = type === "out" ? easeOut : type === "in" ? function (p) { + return 1 - easeOut(1 - p); + } : _easeInOutFromOut(easeOut); + + ease.config = function (overshoot) { + return _configBack(type, overshoot); + }; + + return ease; + }; + + _forEachName("Linear,Quad,Cubic,Quart,Quint,Strong", function (name, i) { + var power = i < 5 ? i + 1 : i; + + _insertEase(name + ",Power" + (power - 1), i ? function (p) { + return Math.pow(p, power); + } : function (p) { + return p; + }, function (p) { + return 1 - Math.pow(1 - p, power); + }, function (p) { + return p < .5 ? Math.pow(p * 2, power) / 2 : 1 - Math.pow((1 - p) * 2, power) / 2; + }); + }); + + _easeMap.Linear.easeNone = _easeMap.none = _easeMap.Linear.easeIn; + + _insertEase("Elastic", _configElastic("in"), _configElastic("out"), _configElastic()); + + (function (n, c) { + var n1 = 1 / c, + n2 = 2 * n1, + n3 = 2.5 * n1, + easeOut = function easeOut(p) { + return p < n1 ? n * p * p : p < n2 ? n * Math.pow(p - 1.5 / c, 2) + .75 : p < n3 ? n * (p -= 2.25 / c) * p + .9375 : n * Math.pow(p - 2.625 / c, 2) + .984375; + }; + + _insertEase("Bounce", function (p) { + return 1 - easeOut(1 - p); + }, easeOut); + })(7.5625, 2.75); + + _insertEase("Expo", function (p) { + return Math.pow(2, 10 * (p - 1)) * p + p * p * p * p * p * p * (1 - p); + }); + + _insertEase("Circ", function (p) { + return -(_sqrt(1 - p * p) - 1); + }); + + _insertEase("Sine", function (p) { + return p === 1 ? 1 : -_cos(p * _HALF_PI) + 1; + }); + + _insertEase("Back", _configBack("in"), _configBack("out"), _configBack()); + + _easeMap.SteppedEase = _easeMap.steps = _globals.SteppedEase = { + config: function config(steps, immediateStart) { + if (steps === void 0) { + steps = 1; + } + + var p1 = 1 / steps, + p2 = steps + (immediateStart ? 0 : 1), + p3 = immediateStart ? 1 : 0, + max = 1 - _tinyNum; + return function (p) { + return ((p2 * _clamp(0, max, p) | 0) + p3) * p1; + }; + } + }; + _defaults.ease = _easeMap["quad.out"]; + + _forEachName("onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt", function (name) { + return _callbackNames += name + "," + name + "Params,"; + }); + + var GSCache = function GSCache(target, harness) { + this.id = _gsID++; + target._gsap = this; + this.target = target; + this.harness = harness; + this.get = harness ? harness.get : _getProperty; + this.set = harness ? harness.getSetter : _getSetter; + }; + var Animation = function () { + function Animation(vars) { + this.vars = vars; + this._delay = +vars.delay || 0; + + if (this._repeat = vars.repeat === Infinity ? -2 : vars.repeat || 0) { + this._rDelay = vars.repeatDelay || 0; + this._yoyo = !!vars.yoyo || !!vars.yoyoEase; + } + + this._ts = 1; + + _setDuration(this, +vars.duration, 1, 1); + + this.data = vars.data; + + if (_context) { + this._ctx = _context; + + _context.data.push(this); + } + + _tickerActive || _ticker.wake(); + } + + var _proto = Animation.prototype; + + _proto.delay = function delay(value) { + if (value || value === 0) { + this.parent && this.parent.smoothChildTiming && this.startTime(this._start + value - this._delay); + this._delay = value; + return this; + } + + return this._delay; + }; + + _proto.duration = function duration(value) { + return arguments.length ? this.totalDuration(this._repeat > 0 ? value + (value + this._rDelay) * this._repeat : value) : this.totalDuration() && this._dur; + }; + + _proto.totalDuration = function totalDuration(value) { + if (!arguments.length) { + return this._tDur; + } + + this._dirty = 0; + return _setDuration(this, this._repeat < 0 ? value : (value - this._repeat * this._rDelay) / (this._repeat + 1)); + }; + + _proto.totalTime = function totalTime(_totalTime, suppressEvents) { + _wake(); + + if (!arguments.length) { + return this._tTime; + } + + var parent = this._dp; + + if (parent && parent.smoothChildTiming && this._ts) { + _alignPlayhead(this, _totalTime); + + !parent._dp || parent.parent || _postAddChecks(parent, this); + + while (parent && parent.parent) { + if (parent.parent._time !== parent._start + (parent._ts >= 0 ? parent._tTime / parent._ts : (parent.totalDuration() - parent._tTime) / -parent._ts)) { + parent.totalTime(parent._tTime, true); + } + + parent = parent.parent; + } + + if (!this.parent && this._dp.autoRemoveChildren && (this._ts > 0 && _totalTime < this._tDur || this._ts < 0 && _totalTime > 0 || !this._tDur && !_totalTime)) { + _addToTimeline(this._dp, this, this._start - this._delay); + } + } + + if (this._tTime !== _totalTime || !this._dur && !suppressEvents || this._initted && Math.abs(this._zTime) === _tinyNum || !_totalTime && !this._initted && (this.add || this._ptLookup)) { + this._ts || (this._pTime = _totalTime); + + _lazySafeRender(this, _totalTime, suppressEvents); + } + + return this; + }; + + _proto.time = function time(value, suppressEvents) { + return arguments.length ? this.totalTime(Math.min(this.totalDuration(), value + _elapsedCycleDuration(this)) % (this._dur + this._rDelay) || (value ? this._dur : 0), suppressEvents) : this._time; + }; + + _proto.totalProgress = function totalProgress(value, suppressEvents) { + return arguments.length ? this.totalTime(this.totalDuration() * value, suppressEvents) : this.totalDuration() ? Math.min(1, this._tTime / this._tDur) : this.rawTime() >= 0 && this._initted ? 1 : 0; + }; + + _proto.progress = function progress(value, suppressEvents) { + return arguments.length ? this.totalTime(this.duration() * (this._yoyo && !(this.iteration() & 1) ? 1 - value : value) + _elapsedCycleDuration(this), suppressEvents) : this.duration() ? Math.min(1, this._time / this._dur) : this.rawTime() > 0 ? 1 : 0; + }; + + _proto.iteration = function iteration(value, suppressEvents) { + var cycleDuration = this.duration() + this._rDelay; + + return arguments.length ? this.totalTime(this._time + (value - 1) * cycleDuration, suppressEvents) : this._repeat ? _animationCycle(this._tTime, cycleDuration) + 1 : 1; + }; + + _proto.timeScale = function timeScale(value, suppressEvents) { + if (!arguments.length) { + return this._rts === -_tinyNum ? 0 : this._rts; + } + + if (this._rts === value) { + return this; + } + + var tTime = this.parent && this._ts ? _parentToChildTotalTime(this.parent._time, this) : this._tTime; + this._rts = +value || 0; + this._ts = this._ps || value === -_tinyNum ? 0 : this._rts; + this.totalTime(_clamp(-Math.abs(this._delay), this._tDur, tTime), suppressEvents !== false); + + _setEnd(this); + + return _recacheAncestors(this); + }; + + _proto.paused = function paused(value) { + if (!arguments.length) { + return this._ps; + } + + if (this._ps !== value) { + this._ps = value; + + if (value) { + this._pTime = this._tTime || Math.max(-this._delay, this.rawTime()); + this._ts = this._act = 0; + } else { + _wake(); + + this._ts = this._rts; + this.totalTime(this.parent && !this.parent.smoothChildTiming ? this.rawTime() : this._tTime || this._pTime, this.progress() === 1 && Math.abs(this._zTime) !== _tinyNum && (this._tTime -= _tinyNum)); + } + } + + return this; + }; + + _proto.startTime = function startTime(value) { + if (arguments.length) { + this._start = value; + var parent = this.parent || this._dp; + parent && (parent._sort || !this.parent) && _addToTimeline(parent, this, value - this._delay); + return this; + } + + return this._start; + }; + + _proto.endTime = function endTime(includeRepeats) { + return this._start + (_isNotFalse(includeRepeats) ? this.totalDuration() : this.duration()) / Math.abs(this._ts || 1); + }; + + _proto.rawTime = function rawTime(wrapRepeats) { + var parent = this.parent || this._dp; + return !parent ? this._tTime : wrapRepeats && (!this._ts || this._repeat && this._time && this.totalProgress() < 1) ? this._tTime % (this._dur + this._rDelay) : !this._ts ? this._tTime : _parentToChildTotalTime(parent.rawTime(wrapRepeats), this); + }; + + _proto.revert = function revert(config) { + if (config === void 0) { + config = _revertConfig; + } + + var prevIsReverting = _reverting; + _reverting = config; + + if (this._initted || this._startAt) { + this.timeline && this.timeline.revert(config); + this.totalTime(-0.01, config.suppressEvents); + } + + this.data !== "nested" && config.kill !== false && this.kill(); + _reverting = prevIsReverting; + return this; + }; + + _proto.globalTime = function globalTime(rawTime) { + var animation = this, + time = arguments.length ? rawTime : animation.rawTime(); + + while (animation) { + time = animation._start + time / (Math.abs(animation._ts) || 1); + animation = animation._dp; + } + + return !this.parent && this._sat ? this._sat.globalTime(rawTime) : time; + }; + + _proto.repeat = function repeat(value) { + if (arguments.length) { + this._repeat = value === Infinity ? -2 : value; + return _onUpdateTotalDuration(this); + } + + return this._repeat === -2 ? Infinity : this._repeat; + }; + + _proto.repeatDelay = function repeatDelay(value) { + if (arguments.length) { + var time = this._time; + this._rDelay = value; + + _onUpdateTotalDuration(this); + + return time ? this.time(time) : this; + } + + return this._rDelay; + }; + + _proto.yoyo = function yoyo(value) { + if (arguments.length) { + this._yoyo = value; + return this; + } + + return this._yoyo; + }; + + _proto.seek = function seek(position, suppressEvents) { + return this.totalTime(_parsePosition(this, position), _isNotFalse(suppressEvents)); + }; + + _proto.restart = function restart(includeDelay, suppressEvents) { + this.play().totalTime(includeDelay ? -this._delay : 0, _isNotFalse(suppressEvents)); + this._dur || (this._zTime = -_tinyNum); + return this; + }; + + _proto.play = function play(from, suppressEvents) { + from != null && this.seek(from, suppressEvents); + return this.reversed(false).paused(false); + }; + + _proto.reverse = function reverse(from, suppressEvents) { + from != null && this.seek(from || this.totalDuration(), suppressEvents); + return this.reversed(true).paused(false); + }; + + _proto.pause = function pause(atTime, suppressEvents) { + atTime != null && this.seek(atTime, suppressEvents); + return this.paused(true); + }; + + _proto.resume = function resume() { + return this.paused(false); + }; + + _proto.reversed = function reversed(value) { + if (arguments.length) { + !!value !== this.reversed() && this.timeScale(-this._rts || (value ? -_tinyNum : 0)); + return this; + } + + return this._rts < 0; + }; + + _proto.invalidate = function invalidate() { + this._initted = this._act = 0; + this._zTime = -_tinyNum; + return this; + }; + + _proto.isActive = function isActive() { + var parent = this.parent || this._dp, + start = this._start, + rawTime; + return !!(!parent || this._ts && this._initted && parent.isActive() && (rawTime = parent.rawTime(true)) >= start && rawTime < this.endTime(true) - _tinyNum); + }; + + _proto.eventCallback = function eventCallback(type, callback, params) { + var vars = this.vars; + + if (arguments.length > 1) { + if (!callback) { + delete vars[type]; + } else { + vars[type] = callback; + params && (vars[type + "Params"] = params); + type === "onUpdate" && (this._onUpdate = callback); + } + + return this; + } + + return vars[type]; + }; + + _proto.then = function then(onFulfilled) { + var self = this; + return new Promise(function (resolve) { + var f = _isFunction(onFulfilled) ? onFulfilled : _passThrough, + _resolve = function _resolve() { + var _then = self.then; + self.then = null; + _isFunction(f) && (f = f(self)) && (f.then || f === self) && (self.then = _then); + resolve(f); + self.then = _then; + }; + + if (self._initted && self.totalProgress() === 1 && self._ts >= 0 || !self._tTime && self._ts < 0) { + _resolve(); + } else { + self._prom = _resolve; + } + }); + }; + + _proto.kill = function kill() { + _interrupt(this); + }; + + return Animation; + }(); + + _setDefaults(Animation.prototype, { + _time: 0, + _start: 0, + _end: 0, + _tTime: 0, + _tDur: 0, + _dirty: 0, + _repeat: 0, + _yoyo: false, + parent: null, + _initted: false, + _rDelay: 0, + _ts: 1, + _dp: 0, + ratio: 0, + _zTime: -_tinyNum, + _prom: 0, + _ps: false, + _rts: 1 + }); + + var Timeline = function (_Animation) { + _inheritsLoose(Timeline, _Animation); + + function Timeline(vars, position) { + var _this; + + if (vars === void 0) { + vars = {}; + } + + _this = _Animation.call(this, vars) || this; + _this.labels = {}; + _this.smoothChildTiming = !!vars.smoothChildTiming; + _this.autoRemoveChildren = !!vars.autoRemoveChildren; + _this._sort = _isNotFalse(vars.sortChildren); + _globalTimeline && _addToTimeline(vars.parent || _globalTimeline, _assertThisInitialized(_this), position); + vars.reversed && _this.reverse(); + vars.paused && _this.paused(true); + vars.scrollTrigger && _scrollTrigger(_assertThisInitialized(_this), vars.scrollTrigger); + return _this; + } + + var _proto2 = Timeline.prototype; + + _proto2.to = function to(targets, vars, position) { + _createTweenType(0, arguments, this); + + return this; + }; + + _proto2.from = function from(targets, vars, position) { + _createTweenType(1, arguments, this); + + return this; + }; + + _proto2.fromTo = function fromTo(targets, fromVars, toVars, position) { + _createTweenType(2, arguments, this); + + return this; + }; + + _proto2.set = function set(targets, vars, position) { + vars.duration = 0; + vars.parent = this; + _inheritDefaults(vars).repeatDelay || (vars.repeat = 0); + vars.immediateRender = !!vars.immediateRender; + new Tween(targets, vars, _parsePosition(this, position), 1); + return this; + }; + + _proto2.call = function call(callback, params, position) { + return _addToTimeline(this, Tween.delayedCall(0, callback, params), position); + }; + + _proto2.staggerTo = function staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.duration = duration; + vars.stagger = vars.stagger || stagger; + vars.onComplete = onCompleteAll; + vars.onCompleteParams = onCompleteAllParams; + vars.parent = this; + new Tween(targets, vars, _parsePosition(this, position)); + return this; + }; + + _proto2.staggerFrom = function staggerFrom(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.runBackwards = 1; + _inheritDefaults(vars).immediateRender = _isNotFalse(vars.immediateRender); + return this.staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams); + }; + + _proto2.staggerFromTo = function staggerFromTo(targets, duration, fromVars, toVars, stagger, position, onCompleteAll, onCompleteAllParams) { + toVars.startAt = fromVars; + _inheritDefaults(toVars).immediateRender = _isNotFalse(toVars.immediateRender); + return this.staggerTo(targets, duration, toVars, stagger, position, onCompleteAll, onCompleteAllParams); + }; + + _proto2.render = function render(totalTime, suppressEvents, force) { + var prevTime = this._time, + tDur = this._dirty ? this.totalDuration() : this._tDur, + dur = this._dur, + tTime = totalTime <= 0 ? 0 : _roundPrecise(totalTime), + crossingStart = this._zTime < 0 !== totalTime < 0 && (this._initted || !dur), + time, + child, + next, + iteration, + cycleDuration, + prevPaused, + pauseTween, + timeScale, + prevStart, + prevIteration, + yoyo, + isYoyo; + this !== _globalTimeline && tTime > tDur && totalTime >= 0 && (tTime = tDur); + + if (tTime !== this._tTime || force || crossingStart) { + if (prevTime !== this._time && dur) { + tTime += this._time - prevTime; + totalTime += this._time - prevTime; + } + + time = tTime; + prevStart = this._start; + timeScale = this._ts; + prevPaused = !timeScale; + + if (crossingStart) { + dur || (prevTime = this._zTime); + (totalTime || !suppressEvents) && (this._zTime = totalTime); + } + + if (this._repeat) { + yoyo = this._yoyo; + cycleDuration = dur + this._rDelay; + + if (this._repeat < -1 && totalTime < 0) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + + time = _roundPrecise(tTime % cycleDuration); + + if (tTime === tDur) { + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); + iteration = ~~prevIteration; + + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } + + time > dur && (time = dur); + } + + prevIteration = _animationCycle(this._tTime, cycleDuration); + !prevTime && this._tTime && prevIteration !== iteration && this._tTime - prevIteration * cycleDuration - this._dur <= 0 && (prevIteration = iteration); + + if (yoyo && iteration & 1) { + time = dur - time; + isYoyo = 1; + } + + if (iteration !== prevIteration && !this._lock) { + var rewinding = yoyo && prevIteration & 1, + doesWrap = rewinding === (yoyo && iteration & 1); + iteration < prevIteration && (rewinding = !rewinding); + prevTime = rewinding ? 0 : tTime % dur ? dur : tTime; + this._lock = 1; + this.render(prevTime || (isYoyo ? 0 : _roundPrecise(iteration * cycleDuration)), suppressEvents, !dur)._lock = 0; + this._tTime = tTime; + !suppressEvents && this.parent && _callback(this, "onRepeat"); + this.vars.repeatRefresh && !isYoyo && (this.invalidate()._lock = 1); + + if (prevTime && prevTime !== this._time || prevPaused !== !this._ts || this.vars.onRepeat && !this.parent && !this._act) { + return this; + } + + dur = this._dur; + tDur = this._tDur; + + if (doesWrap) { + this._lock = 2; + prevTime = rewinding ? dur : -0.0001; + this.render(prevTime, true); + this.vars.repeatRefresh && !isYoyo && this.invalidate(); + } + + this._lock = 0; + + if (!this._ts && !prevPaused) { + return this; + } + + _propagateYoyoEase(this, isYoyo); + } + } + + if (this._hasPause && !this._forcing && this._lock < 2) { + pauseTween = _findNextPauseTween(this, _roundPrecise(prevTime), _roundPrecise(time)); + + if (pauseTween) { + tTime -= time - (time = pauseTween._start); + } + } + + this._tTime = tTime; + this._time = time; + this._act = !timeScale; + + if (!this._initted) { + this._onUpdate = this.vars.onUpdate; + this._initted = 1; + this._zTime = totalTime; + prevTime = 0; + } + + if (!prevTime && time && !suppressEvents && !iteration) { + _callback(this, "onStart"); + + if (this._tTime !== tTime) { + return this; + } + } + + if (time >= prevTime && totalTime >= 0) { + child = this._first; + + while (child) { + next = child._next; + + if ((child._act || time >= child._start) && child._ts && pauseTween !== child) { + if (child.parent !== this) { + return this.render(totalTime, suppressEvents, force); + } + + child.render(child._ts > 0 ? (time - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (time - child._start) * child._ts, suppressEvents, force); + + if (time !== this._time || !this._ts && !prevPaused) { + pauseTween = 0; + next && (tTime += this._zTime = -_tinyNum); + break; + } + } + + child = next; + } + } else { + child = this._last; + var adjustedTime = totalTime < 0 ? totalTime : time; + + while (child) { + next = child._prev; + + if ((child._act || adjustedTime <= child._end) && child._ts && pauseTween !== child) { + if (child.parent !== this) { + return this.render(totalTime, suppressEvents, force); + } + + child.render(child._ts > 0 ? (adjustedTime - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (adjustedTime - child._start) * child._ts, suppressEvents, force || _reverting && (child._initted || child._startAt)); + + if (time !== this._time || !this._ts && !prevPaused) { + pauseTween = 0; + next && (tTime += this._zTime = adjustedTime ? -_tinyNum : _tinyNum); + break; + } + } + + child = next; + } + } + + if (pauseTween && !suppressEvents) { + this.pause(); + pauseTween.render(time >= prevTime ? 0 : -_tinyNum)._zTime = time >= prevTime ? 1 : -1; + + if (this._ts) { + this._start = prevStart; + + _setEnd(this); + + return this.render(totalTime, suppressEvents, force); + } + } + + this._onUpdate && !suppressEvents && _callback(this, "onUpdate", true); + if (tTime === tDur && this._tTime >= this.totalDuration() || !tTime && prevTime) if (prevStart === this._start || Math.abs(timeScale) !== Math.abs(this._ts)) if (!this._lock) { + (totalTime || !dur) && (tTime === tDur && this._ts > 0 || !tTime && this._ts < 0) && _removeFromParent(this, 1); + + if (!suppressEvents && !(totalTime < 0 && !prevTime) && (tTime || prevTime || !tDur)) { + _callback(this, tTime === tDur && totalTime >= 0 ? "onComplete" : "onReverseComplete", true); + + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + + return this; + }; + + _proto2.add = function add(child, position) { + var _this2 = this; + + _isNumber(position) || (position = _parsePosition(this, position, child)); + + if (!(child instanceof Animation)) { + if (_isArray(child)) { + child.forEach(function (obj) { + return _this2.add(obj, position); + }); + return this; + } + + if (_isString(child)) { + return this.addLabel(child, position); + } + + if (_isFunction(child)) { + child = Tween.delayedCall(0, child); + } else { + return this; + } + } + + return this !== child ? _addToTimeline(this, child, position) : this; + }; + + _proto2.getChildren = function getChildren(nested, tweens, timelines, ignoreBeforeTime) { + if (nested === void 0) { + nested = true; + } + + if (tweens === void 0) { + tweens = true; + } + + if (timelines === void 0) { + timelines = true; + } + + if (ignoreBeforeTime === void 0) { + ignoreBeforeTime = -_bigNum; + } + + var a = [], + child = this._first; + + while (child) { + if (child._start >= ignoreBeforeTime) { + if (child instanceof Tween) { + tweens && a.push(child); + } else { + timelines && a.push(child); + nested && a.push.apply(a, child.getChildren(true, tweens, timelines)); + } + } + + child = child._next; + } + + return a; + }; + + _proto2.getById = function getById(id) { + var animations = this.getChildren(1, 1, 1), + i = animations.length; + + while (i--) { + if (animations[i].vars.id === id) { + return animations[i]; + } + } + }; + + _proto2.remove = function remove(child) { + if (_isString(child)) { + return this.removeLabel(child); + } + + if (_isFunction(child)) { + return this.killTweensOf(child); + } + + child.parent === this && _removeLinkedListItem(this, child); + + if (child === this._recent) { + this._recent = this._last; + } + + return _uncache(this); + }; + + _proto2.totalTime = function totalTime(_totalTime2, suppressEvents) { + if (!arguments.length) { + return this._tTime; + } + + this._forcing = 1; + + if (!this._dp && this._ts) { + this._start = _roundPrecise(_ticker.time - (this._ts > 0 ? _totalTime2 / this._ts : (this.totalDuration() - _totalTime2) / -this._ts)); + } + + _Animation.prototype.totalTime.call(this, _totalTime2, suppressEvents); + + this._forcing = 0; + return this; + }; + + _proto2.addLabel = function addLabel(label, position) { + this.labels[label] = _parsePosition(this, position); + return this; + }; + + _proto2.removeLabel = function removeLabel(label) { + delete this.labels[label]; + return this; + }; + + _proto2.addPause = function addPause(position, callback, params) { + var t = Tween.delayedCall(0, callback || _emptyFunc, params); + t.data = "isPause"; + this._hasPause = 1; + return _addToTimeline(this, t, _parsePosition(this, position)); + }; + + _proto2.removePause = function removePause(position) { + var child = this._first; + position = _parsePosition(this, position); + + while (child) { + if (child._start === position && child.data === "isPause") { + _removeFromParent(child); + } + + child = child._next; + } + }; + + _proto2.killTweensOf = function killTweensOf(targets, props, onlyActive) { + var tweens = this.getTweensOf(targets, onlyActive), + i = tweens.length; + + while (i--) { + _overwritingTween !== tweens[i] && tweens[i].kill(targets, props); + } + + return this; + }; + + _proto2.getTweensOf = function getTweensOf(targets, onlyActive) { + var a = [], + parsedTargets = toArray(targets), + child = this._first, + isGlobalTime = _isNumber(onlyActive), + children; + + while (child) { + if (child instanceof Tween) { + if (_arrayContainsAny(child._targets, parsedTargets) && (isGlobalTime ? (!_overwritingTween || child._initted && child._ts) && child.globalTime(0) <= onlyActive && child.globalTime(child.totalDuration()) > onlyActive : !onlyActive || child.isActive())) { + a.push(child); + } + } else if ((children = child.getTweensOf(parsedTargets, onlyActive)).length) { + a.push.apply(a, children); + } + + child = child._next; + } + + return a; + }; + + _proto2.tweenTo = function tweenTo(position, vars) { + vars = vars || {}; + + var tl = this, + endTime = _parsePosition(tl, position), + _vars = vars, + startAt = _vars.startAt, + _onStart = _vars.onStart, + onStartParams = _vars.onStartParams, + immediateRender = _vars.immediateRender, + initted, + tween = Tween.to(tl, _setDefaults({ + ease: vars.ease || "none", + lazy: false, + immediateRender: false, + time: endTime, + overwrite: "auto", + duration: vars.duration || Math.abs((endTime - (startAt && "time" in startAt ? startAt.time : tl._time)) / tl.timeScale()) || _tinyNum, + onStart: function onStart() { + tl.pause(); + + if (!initted) { + var duration = vars.duration || Math.abs((endTime - (startAt && "time" in startAt ? startAt.time : tl._time)) / tl.timeScale()); + tween._dur !== duration && _setDuration(tween, duration, 0, 1).render(tween._time, true, true); + initted = 1; + } + + _onStart && _onStart.apply(tween, onStartParams || []); + } + }, vars)); + + return immediateRender ? tween.render(0) : tween; + }; + + _proto2.tweenFromTo = function tweenFromTo(fromPosition, toPosition, vars) { + return this.tweenTo(toPosition, _setDefaults({ + startAt: { + time: _parsePosition(this, fromPosition) + } + }, vars)); + }; + + _proto2.recent = function recent() { + return this._recent; + }; + + _proto2.nextLabel = function nextLabel(afterTime) { + if (afterTime === void 0) { + afterTime = this._time; + } + + return _getLabelInDirection(this, _parsePosition(this, afterTime)); + }; + + _proto2.previousLabel = function previousLabel(beforeTime) { + if (beforeTime === void 0) { + beforeTime = this._time; + } + + return _getLabelInDirection(this, _parsePosition(this, beforeTime), 1); + }; + + _proto2.currentLabel = function currentLabel(value) { + return arguments.length ? this.seek(value, true) : this.previousLabel(this._time + _tinyNum); + }; + + _proto2.shiftChildren = function shiftChildren(amount, adjustLabels, ignoreBeforeTime) { + if (ignoreBeforeTime === void 0) { + ignoreBeforeTime = 0; + } + + var child = this._first, + labels = this.labels, + p; + + while (child) { + if (child._start >= ignoreBeforeTime) { + child._start += amount; + child._end += amount; + } + + child = child._next; + } + + if (adjustLabels) { + for (p in labels) { + if (labels[p] >= ignoreBeforeTime) { + labels[p] += amount; + } + } + } + + return _uncache(this); + }; + + _proto2.invalidate = function invalidate(soft) { + var child = this._first; + this._lock = 0; + + while (child) { + child.invalidate(soft); + child = child._next; + } + + return _Animation.prototype.invalidate.call(this, soft); + }; + + _proto2.clear = function clear(includeLabels) { + if (includeLabels === void 0) { + includeLabels = true; + } + + var child = this._first, + next; + + while (child) { + next = child._next; + this.remove(child); + child = next; + } + + this._dp && (this._time = this._tTime = this._pTime = 0); + includeLabels && (this.labels = {}); + return _uncache(this); + }; + + _proto2.totalDuration = function totalDuration(value) { + var max = 0, + self = this, + child = self._last, + prevStart = _bigNum, + prev, + start, + parent; + + if (arguments.length) { + return self.timeScale((self._repeat < 0 ? self.duration() : self.totalDuration()) / (self.reversed() ? -value : value)); + } + + if (self._dirty) { + parent = self.parent; + + while (child) { + prev = child._prev; + child._dirty && child.totalDuration(); + start = child._start; + + if (start > prevStart && self._sort && child._ts && !self._lock) { + self._lock = 1; + _addToTimeline(self, child, start - child._delay, 1)._lock = 0; + } else { + prevStart = start; + } + + if (start < 0 && child._ts) { + max -= start; + + if (!parent && !self._dp || parent && parent.smoothChildTiming) { + self._start += start / self._ts; + self._time -= start; + self._tTime -= start; + } + + self.shiftChildren(-start, false, -1e999); + prevStart = 0; + } + + child._end > max && child._ts && (max = child._end); + child = prev; + } + + _setDuration(self, self === _globalTimeline && self._time > max ? self._time : max, 1, 1); + + self._dirty = 0; + } + + return self._tDur; + }; + + Timeline.updateRoot = function updateRoot(time) { + if (_globalTimeline._ts) { + _lazySafeRender(_globalTimeline, _parentToChildTotalTime(time, _globalTimeline)); + + _lastRenderedFrame = _ticker.frame; + } + + if (_ticker.frame >= _nextGCFrame) { + _nextGCFrame += _config.autoSleep || 120; + var child = _globalTimeline._first; + if (!child || !child._ts) if (_config.autoSleep && _ticker._listeners.length < 2) { + while (child && !child._ts) { + child = child._next; + } + + child || _ticker.sleep(); + } + } + }; + + return Timeline; + }(Animation); + + _setDefaults(Timeline.prototype, { + _lock: 0, + _hasPause: 0, + _forcing: 0 + }); + + var _addComplexStringPropTween = function _addComplexStringPropTween(target, prop, start, end, setter, stringFilter, funcParam) { + var pt = new PropTween(this._pt, target, prop, 0, 1, _renderComplexString, null, setter), + index = 0, + matchIndex = 0, + result, + startNums, + color, + endNum, + chunk, + startNum, + hasRandom, + a; + pt.b = start; + pt.e = end; + start += ""; + end += ""; + + if (hasRandom = ~end.indexOf("random(")) { + end = _replaceRandom(end); + } + + if (stringFilter) { + a = [start, end]; + stringFilter(a, target, prop); + start = a[0]; + end = a[1]; + } + + startNums = start.match(_complexStringNumExp) || []; + + while (result = _complexStringNumExp.exec(end)) { + endNum = result[0]; + chunk = end.substring(index, result.index); + + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(") { + color = 1; + } + + if (endNum !== startNums[matchIndex++]) { + startNum = parseFloat(startNums[matchIndex - 1]) || 0; + pt._pt = { + _next: pt._pt, + p: chunk || matchIndex === 1 ? chunk : ",", + s: startNum, + c: endNum.charAt(1) === "=" ? _parseRelative(startNum, endNum) - startNum : parseFloat(endNum) - startNum, + m: color && color < 4 ? Math.round : 0 + }; + index = _complexStringNumExp.lastIndex; + } + } + + pt.c = index < end.length ? end.substring(index, end.length) : ""; + pt.fp = funcParam; + + if (_relExp.test(end) || hasRandom) { + pt.e = 0; + } + + this._pt = pt; + return pt; + }, + _addPropTween = function _addPropTween(target, prop, start, end, index, targets, modifier, stringFilter, funcParam, optional) { + _isFunction(end) && (end = end(index || 0, target, targets)); + var currentValue = target[prop], + parsedStart = start !== "get" ? start : !_isFunction(currentValue) ? currentValue : funcParam ? target[prop.indexOf("set") || !_isFunction(target["get" + prop.substr(3)]) ? prop : "get" + prop.substr(3)](funcParam) : target[prop](), + setter = !_isFunction(currentValue) ? _setterPlain : funcParam ? _setterFuncWithParam : _setterFunc, + pt; + + if (_isString(end)) { + if (~end.indexOf("random(")) { + end = _replaceRandom(end); + } + + if (end.charAt(1) === "=") { + pt = _parseRelative(parsedStart, end) + (getUnit(parsedStart) || 0); + + if (pt || pt === 0) { + end = pt; + } + } + } + + if (!optional || parsedStart !== end || _forceAllPropTweens) { + if (!isNaN(parsedStart * end) && end !== "") { + pt = new PropTween(this._pt, target, prop, +parsedStart || 0, end - (parsedStart || 0), typeof currentValue === "boolean" ? _renderBoolean : _renderPlain, 0, setter); + funcParam && (pt.fp = funcParam); + modifier && pt.modifier(modifier, this, target); + return this._pt = pt; + } + + !currentValue && !(prop in target) && _missingPlugin(prop, end); + return _addComplexStringPropTween.call(this, target, prop, parsedStart, end, setter, stringFilter || _config.stringFilter, funcParam); + } + }, + _processVars = function _processVars(vars, index, target, targets, tween) { + _isFunction(vars) && (vars = _parseFuncOrString(vars, tween, index, target, targets)); + + if (!_isObject(vars) || vars.style && vars.nodeType || _isArray(vars) || _isTypedArray(vars)) { + return _isString(vars) ? _parseFuncOrString(vars, tween, index, target, targets) : vars; + } + + var copy = {}, + p; + + for (p in vars) { + copy[p] = _parseFuncOrString(vars[p], tween, index, target, targets); + } + + return copy; + }, + _checkPlugin = function _checkPlugin(property, vars, tween, index, target, targets) { + var plugin, pt, ptLookup, i; + + if (_plugins[property] && (plugin = new _plugins[property]()).init(target, plugin.rawVars ? vars[property] : _processVars(vars[property], index, target, targets, tween), tween, index, targets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, property, 0, 1, plugin.render, plugin, 0, plugin.priority); + + if (tween !== _quickTween) { + ptLookup = tween._ptLookup[tween._targets.indexOf(target)]; + i = plugin._props.length; + + while (i--) { + ptLookup[plugin._props[i]] = pt; + } + } + } + + return plugin; + }, + _overwritingTween, + _forceAllPropTweens, + _initTween = function _initTween(tween, time, tTime) { + var vars = tween.vars, + ease = vars.ease, + startAt = vars.startAt, + immediateRender = vars.immediateRender, + lazy = vars.lazy, + onUpdate = vars.onUpdate, + runBackwards = vars.runBackwards, + yoyoEase = vars.yoyoEase, + keyframes = vars.keyframes, + autoRevert = vars.autoRevert, + dur = tween._dur, + prevStartAt = tween._startAt, + targets = tween._targets, + parent = tween.parent, + fullTargets = parent && parent.data === "nested" ? parent.vars.targets : targets, + autoOverwrite = tween._overwrite === "auto" && !_suppressOverwrites, + tl = tween.timeline, + cleanVars, + i, + p, + pt, + target, + hasPriority, + gsData, + harness, + plugin, + ptLookup, + index, + harnessVars, + overwritten; + tl && (!keyframes || !ease) && (ease = "none"); + tween._ease = _parseEase(ease, _defaults.ease); + tween._yEase = yoyoEase ? _invertEase(_parseEase(yoyoEase === true ? ease : yoyoEase, _defaults.ease)) : 0; + + if (yoyoEase && tween._yoyo && !tween._repeat) { + yoyoEase = tween._yEase; + tween._yEase = tween._ease; + tween._ease = yoyoEase; + } + + tween._from = !tl && !!vars.runBackwards; + + if (!tl || keyframes && !vars.stagger) { + harness = targets[0] ? _getCache(targets[0]).harness : 0; + harnessVars = harness && vars[harness.prop]; + cleanVars = _copyExcluding(vars, _reservedProps); + + if (prevStartAt) { + prevStartAt._zTime < 0 && prevStartAt.progress(1); + time < 0 && runBackwards && immediateRender && !autoRevert ? prevStartAt.render(-1, true) : prevStartAt.revert(runBackwards && dur ? _revertConfigNoKill : _startAtRevertConfig); + prevStartAt._lazy = 0; + } + + if (startAt) { + _removeFromParent(tween._startAt = Tween.set(targets, _setDefaults({ + data: "isStart", + overwrite: false, + parent: parent, + immediateRender: true, + lazy: !prevStartAt && _isNotFalse(lazy), + startAt: null, + delay: 0, + onUpdate: onUpdate && function () { + return _callback(tween, "onUpdate"); + }, + stagger: 0 + }, startAt))); + + tween._startAt._dp = 0; + tween._startAt._sat = tween; + time < 0 && (_reverting || !immediateRender && !autoRevert) && tween._startAt.revert(_revertConfigNoKill); + + if (immediateRender) { + if (dur && time <= 0 && tTime <= 0) { + time && (tween._zTime = time); + return; + } + } + } else if (runBackwards && dur) { + if (!prevStartAt) { + time && (immediateRender = false); + p = _setDefaults({ + overwrite: false, + data: "isFromStart", + lazy: immediateRender && !prevStartAt && _isNotFalse(lazy), + immediateRender: immediateRender, + stagger: 0, + parent: parent + }, cleanVars); + harnessVars && (p[harness.prop] = harnessVars); + + _removeFromParent(tween._startAt = Tween.set(targets, p)); + + tween._startAt._dp = 0; + tween._startAt._sat = tween; + time < 0 && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween._startAt.render(-1, true)); + tween._zTime = time; + + if (!immediateRender) { + _initTween(tween._startAt, _tinyNum, _tinyNum); + } else if (!time) { + return; + } + } + } + + tween._pt = tween._ptCache = 0; + lazy = dur && _isNotFalse(lazy) || lazy && !dur; + + for (i = 0; i < targets.length; i++) { + target = targets[i]; + gsData = target._gsap || _harness(targets)[i]._gsap; + tween._ptLookup[i] = ptLookup = {}; + _lazyLookup[gsData.id] && _lazyTweens.length && _lazyRender(); + index = fullTargets === targets ? i : fullTargets.indexOf(target); + + if (harness && (plugin = new harness()).init(target, harnessVars || cleanVars, tween, index, fullTargets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, plugin.name, 0, 1, plugin.render, plugin, 0, plugin.priority); + + plugin._props.forEach(function (name) { + ptLookup[name] = pt; + }); + + plugin.priority && (hasPriority = 1); + } + + if (!harness || harnessVars) { + for (p in cleanVars) { + if (_plugins[p] && (plugin = _checkPlugin(p, cleanVars, tween, index, target, fullTargets))) { + plugin.priority && (hasPriority = 1); + } else { + ptLookup[p] = pt = _addPropTween.call(tween, target, p, "get", cleanVars[p], index, fullTargets, 0, vars.stringFilter); + } + } + } + + tween._op && tween._op[i] && tween.kill(target, tween._op[i]); + + if (autoOverwrite && tween._pt) { + _overwritingTween = tween; + + _globalTimeline.killTweensOf(target, ptLookup, tween.globalTime(time)); + + overwritten = !tween.parent; + _overwritingTween = 0; + } + + tween._pt && lazy && (_lazyLookup[gsData.id] = 1); + } + + hasPriority && _sortPropTweensByPriority(tween); + tween._onInit && tween._onInit(tween); + } + + tween._onUpdate = onUpdate; + tween._initted = (!tween._op || tween._pt) && !overwritten; + keyframes && time <= 0 && tl.render(_bigNum, true, true); + }, + _updatePropTweens = function _updatePropTweens(tween, property, value, start, startIsRelative, ratio, time, skipRecursion) { + var ptCache = (tween._pt && tween._ptCache || (tween._ptCache = {}))[property], + pt, + rootPT, + lookup, + i; + + if (!ptCache) { + ptCache = tween._ptCache[property] = []; + lookup = tween._ptLookup; + i = tween._targets.length; + + while (i--) { + pt = lookup[i][property]; + + if (pt && pt.d && pt.d._pt) { + pt = pt.d._pt; + + while (pt && pt.p !== property && pt.fp !== property) { + pt = pt._next; + } + } + + if (!pt) { + _forceAllPropTweens = 1; + tween.vars[property] = "+=0"; + + _initTween(tween, time); + + _forceAllPropTweens = 0; + return skipRecursion ? _warn(property + " not eligible for reset") : 1; + } + + ptCache.push(pt); + } + } + + i = ptCache.length; + + while (i--) { + rootPT = ptCache[i]; + pt = rootPT._pt || rootPT; + pt.s = (start || start === 0) && !startIsRelative ? start : pt.s + (start || 0) + ratio * pt.c; + pt.c = value - pt.s; + rootPT.e && (rootPT.e = _round(value) + getUnit(rootPT.e)); + rootPT.b && (rootPT.b = pt.s + getUnit(rootPT.b)); + } + }, + _addAliasesToVars = function _addAliasesToVars(targets, vars) { + var harness = targets[0] ? _getCache(targets[0]).harness : 0, + propertyAliases = harness && harness.aliases, + copy, + p, + i, + aliases; + + if (!propertyAliases) { + return vars; + } + + copy = _merge({}, vars); + + for (p in propertyAliases) { + if (p in copy) { + aliases = propertyAliases[p].split(","); + i = aliases.length; + + while (i--) { + copy[aliases[i]] = copy[p]; + } + } + } + + return copy; + }, + _parseKeyframe = function _parseKeyframe(prop, obj, allProps, easeEach) { + var ease = obj.ease || easeEach || "power1.inOut", + p, + a; + + if (_isArray(obj)) { + a = allProps[prop] || (allProps[prop] = []); + obj.forEach(function (value, i) { + return a.push({ + t: i / (obj.length - 1) * 100, + v: value, + e: ease + }); + }); + } else { + for (p in obj) { + a = allProps[p] || (allProps[p] = []); + p === "ease" || a.push({ + t: parseFloat(prop), + v: obj[p], + e: ease + }); + } + } + }, + _parseFuncOrString = function _parseFuncOrString(value, tween, i, target, targets) { + return _isFunction(value) ? value.call(tween, i, target, targets) : _isString(value) && ~value.indexOf("random(") ? _replaceRandom(value) : value; + }, + _staggerTweenProps = _callbackNames + "repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase,autoRevert", + _staggerPropsToSkip = {}; + + _forEachName(_staggerTweenProps + ",id,stagger,delay,duration,paused,scrollTrigger", function (name) { + return _staggerPropsToSkip[name] = 1; + }); + + var Tween = function (_Animation2) { + _inheritsLoose(Tween, _Animation2); + + function Tween(targets, vars, position, skipInherit) { + var _this3; + + if (typeof vars === "number") { + position.duration = vars; + vars = position; + position = null; + } + + _this3 = _Animation2.call(this, skipInherit ? vars : _inheritDefaults(vars)) || this; + var _this3$vars = _this3.vars, + duration = _this3$vars.duration, + delay = _this3$vars.delay, + immediateRender = _this3$vars.immediateRender, + stagger = _this3$vars.stagger, + overwrite = _this3$vars.overwrite, + keyframes = _this3$vars.keyframes, + defaults = _this3$vars.defaults, + scrollTrigger = _this3$vars.scrollTrigger, + yoyoEase = _this3$vars.yoyoEase, + parent = vars.parent || _globalTimeline, + parsedTargets = (_isArray(targets) || _isTypedArray(targets) ? _isNumber(targets[0]) : "length" in vars) ? [targets] : toArray(targets), + tl, + i, + copy, + l, + p, + curTarget, + staggerFunc, + staggerVarsToMerge; + _this3._targets = parsedTargets.length ? _harness(parsedTargets) : _warn("GSAP target " + targets + " not found. https://gsap.com", !_config.nullTargetWarn) || []; + _this3._ptLookup = []; + _this3._overwrite = overwrite; + + if (keyframes || stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + vars = _this3.vars; + tl = _this3.timeline = new Timeline({ + data: "nested", + defaults: defaults || {}, + targets: parent && parent.data === "nested" ? parent.vars.targets : parsedTargets + }); + tl.kill(); + tl.parent = tl._dp = _assertThisInitialized(_this3); + tl._start = 0; + + if (stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + l = parsedTargets.length; + staggerFunc = stagger && distribute(stagger); + + if (_isObject(stagger)) { + for (p in stagger) { + if (~_staggerTweenProps.indexOf(p)) { + staggerVarsToMerge || (staggerVarsToMerge = {}); + staggerVarsToMerge[p] = stagger[p]; + } + } + } + + for (i = 0; i < l; i++) { + copy = _copyExcluding(vars, _staggerPropsToSkip); + copy.stagger = 0; + yoyoEase && (copy.yoyoEase = yoyoEase); + staggerVarsToMerge && _merge(copy, staggerVarsToMerge); + curTarget = parsedTargets[i]; + copy.duration = +_parseFuncOrString(duration, _assertThisInitialized(_this3), i, curTarget, parsedTargets); + copy.delay = (+_parseFuncOrString(delay, _assertThisInitialized(_this3), i, curTarget, parsedTargets) || 0) - _this3._delay; + + if (!stagger && l === 1 && copy.delay) { + _this3._delay = delay = copy.delay; + _this3._start += delay; + copy.delay = 0; + } + + tl.to(curTarget, copy, staggerFunc ? staggerFunc(i, curTarget, parsedTargets) : 0); + tl._ease = _easeMap.none; + } + + tl.duration() ? duration = delay = 0 : _this3.timeline = 0; + } else if (keyframes) { + _inheritDefaults(_setDefaults(tl.vars.defaults, { + ease: "none" + })); + + tl._ease = _parseEase(keyframes.ease || vars.ease || "none"); + var time = 0, + a, + kf, + v; + + if (_isArray(keyframes)) { + keyframes.forEach(function (frame) { + return tl.to(parsedTargets, frame, ">"); + }); + tl.duration(); + } else { + copy = {}; + + for (p in keyframes) { + p === "ease" || p === "easeEach" || _parseKeyframe(p, keyframes[p], copy, keyframes.easeEach); + } + + for (p in copy) { + a = copy[p].sort(function (a, b) { + return a.t - b.t; + }); + time = 0; + + for (i = 0; i < a.length; i++) { + kf = a[i]; + v = { + ease: kf.e, + duration: (kf.t - (i ? a[i - 1].t : 0)) / 100 * duration + }; + v[p] = kf.v; + tl.to(parsedTargets, v, time); + time += v.duration; + } + } + + tl.duration() < duration && tl.to({}, { + duration: duration - tl.duration() + }); + } + } + + duration || _this3.duration(duration = tl.duration()); + } else { + _this3.timeline = 0; + } + + if (overwrite === true && !_suppressOverwrites) { + _overwritingTween = _assertThisInitialized(_this3); + + _globalTimeline.killTweensOf(parsedTargets); + + _overwritingTween = 0; + } + + _addToTimeline(parent, _assertThisInitialized(_this3), position); + + vars.reversed && _this3.reverse(); + vars.paused && _this3.paused(true); + + if (immediateRender || !duration && !keyframes && _this3._start === _roundPrecise(parent._time) && _isNotFalse(immediateRender) && _hasNoPausedAncestors(_assertThisInitialized(_this3)) && parent.data !== "nested") { + _this3._tTime = -_tinyNum; + + _this3.render(Math.max(0, -delay) || 0); + } + + scrollTrigger && _scrollTrigger(_assertThisInitialized(_this3), scrollTrigger); + return _this3; + } + + var _proto3 = Tween.prototype; + + _proto3.render = function render(totalTime, suppressEvents, force) { + var prevTime = this._time, + tDur = this._tDur, + dur = this._dur, + isNegative = totalTime < 0, + tTime = totalTime > tDur - _tinyNum && !isNegative ? tDur : totalTime < _tinyNum ? 0 : totalTime, + time, + pt, + iteration, + cycleDuration, + prevIteration, + isYoyo, + ratio, + timeline, + yoyoEase; + + if (!dur) { + _renderZeroDurationTween(this, totalTime, suppressEvents, force); + } else if (tTime !== this._tTime || !totalTime || force || !this._initted && this._tTime || this._startAt && this._zTime < 0 !== isNegative || this._lazy) { + time = tTime; + timeline = this.timeline; + + if (this._repeat) { + cycleDuration = dur + this._rDelay; + + if (this._repeat < -1 && isNegative) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + + time = _roundPrecise(tTime % cycleDuration); + + if (tTime === tDur) { + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); + iteration = ~~prevIteration; + + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } else if (time > dur) { + time = dur; + } + } + + isYoyo = this._yoyo && iteration & 1; + + if (isYoyo) { + yoyoEase = this._yEase; + time = dur - time; + } + + prevIteration = _animationCycle(this._tTime, cycleDuration); + + if (time === prevTime && !force && this._initted && iteration === prevIteration) { + this._tTime = tTime; + return this; + } + + if (iteration !== prevIteration) { + timeline && this._yEase && _propagateYoyoEase(timeline, isYoyo); + + if (this.vars.repeatRefresh && !isYoyo && !this._lock && time !== cycleDuration && this._initted) { + this._lock = force = 1; + this.render(_roundPrecise(cycleDuration * iteration), true).invalidate()._lock = 0; + } + } + } + + if (!this._initted) { + if (_attemptInitTween(this, isNegative ? totalTime : time, force, suppressEvents, tTime)) { + this._tTime = 0; + return this; + } + + if (prevTime !== this._time && !(force && this.vars.repeatRefresh && iteration !== prevIteration)) { + return this; + } + + if (dur !== this._dur) { + return this.render(totalTime, suppressEvents, force); + } + } + + this._tTime = tTime; + this._time = time; + + if (!this._act && this._ts) { + this._act = 1; + this._lazy = 0; + } + + this.ratio = ratio = (yoyoEase || this._ease)(time / dur); + + if (this._from) { + this.ratio = ratio = 1 - ratio; + } + + if (time && !prevTime && !suppressEvents && !iteration) { + _callback(this, "onStart"); + + if (this._tTime !== tTime) { + return this; + } + } + + pt = this._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + timeline && timeline.render(totalTime < 0 ? totalTime : timeline._dur * timeline._ease(time / this._dur), suppressEvents, force) || this._startAt && (this._zTime = totalTime); + + if (this._onUpdate && !suppressEvents) { + isNegative && _rewindStartAt(this, totalTime, suppressEvents, force); + + _callback(this, "onUpdate"); + } + + this._repeat && iteration !== prevIteration && this.vars.onRepeat && !suppressEvents && this.parent && _callback(this, "onRepeat"); + + if ((tTime === this._tDur || !tTime) && this._tTime === tTime) { + isNegative && !this._onUpdate && _rewindStartAt(this, totalTime, true, true); + (totalTime || !dur) && (tTime === this._tDur && this._ts > 0 || !tTime && this._ts < 0) && _removeFromParent(this, 1); + + if (!suppressEvents && !(isNegative && !prevTime) && (tTime || prevTime || isYoyo)) { + _callback(this, tTime === tDur ? "onComplete" : "onReverseComplete", true); + + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + + return this; + }; + + _proto3.targets = function targets() { + return this._targets; + }; + + _proto3.invalidate = function invalidate(soft) { + (!soft || !this.vars.runBackwards) && (this._startAt = 0); + this._pt = this._op = this._onUpdate = this._lazy = this.ratio = 0; + this._ptLookup = []; + this.timeline && this.timeline.invalidate(soft); + return _Animation2.prototype.invalidate.call(this, soft); + }; + + _proto3.resetTo = function resetTo(property, value, start, startIsRelative, skipRecursion) { + _tickerActive || _ticker.wake(); + this._ts || this.play(); + var time = Math.min(this._dur, (this._dp._time - this._start) * this._ts), + ratio; + this._initted || _initTween(this, time); + ratio = this._ease(time / this._dur); + + if (_updatePropTweens(this, property, value, start, startIsRelative, ratio, time, skipRecursion)) { + return this.resetTo(property, value, start, startIsRelative, 1); + } + + _alignPlayhead(this, 0); + + this.parent || _addLinkedListItem(this._dp, this, "_first", "_last", this._dp._sort ? "_start" : 0); + return this.render(0); + }; + + _proto3.kill = function kill(targets, vars) { + if (vars === void 0) { + vars = "all"; + } + + if (!targets && (!vars || vars === "all")) { + this._lazy = this._pt = 0; + this.parent ? _interrupt(this) : this.scrollTrigger && this.scrollTrigger.kill(!!_reverting); + return this; + } + + if (this.timeline) { + var tDur = this.timeline.totalDuration(); + this.timeline.killTweensOf(targets, vars, _overwritingTween && _overwritingTween.vars.overwrite !== true)._first || _interrupt(this); + this.parent && tDur !== this.timeline.totalDuration() && _setDuration(this, this._dur * this.timeline._tDur / tDur, 0, 1); + return this; + } + + var parsedTargets = this._targets, + killingTargets = targets ? toArray(targets) : parsedTargets, + propTweenLookup = this._ptLookup, + firstPT = this._pt, + overwrittenProps, + curLookup, + curOverwriteProps, + props, + p, + pt, + i; + + if ((!vars || vars === "all") && _arraysMatch(parsedTargets, killingTargets)) { + vars === "all" && (this._pt = 0); + return _interrupt(this); + } + + overwrittenProps = this._op = this._op || []; + + if (vars !== "all") { + if (_isString(vars)) { + p = {}; + + _forEachName(vars, function (name) { + return p[name] = 1; + }); + + vars = p; + } + + vars = _addAliasesToVars(parsedTargets, vars); + } + + i = parsedTargets.length; + + while (i--) { + if (~killingTargets.indexOf(parsedTargets[i])) { + curLookup = propTweenLookup[i]; + + if (vars === "all") { + overwrittenProps[i] = vars; + props = curLookup; + curOverwriteProps = {}; + } else { + curOverwriteProps = overwrittenProps[i] = overwrittenProps[i] || {}; + props = vars; + } + + for (p in props) { + pt = curLookup && curLookup[p]; + + if (pt) { + if (!("kill" in pt.d) || pt.d.kill(p) === true) { + _removeLinkedListItem(this, pt, "_pt"); + } + + delete curLookup[p]; + } + + if (curOverwriteProps !== "all") { + curOverwriteProps[p] = 1; + } + } + } + } + + this._initted && !this._pt && firstPT && _interrupt(this); + return this; + }; + + Tween.to = function to(targets, vars) { + return new Tween(targets, vars, arguments[2]); + }; + + Tween.from = function from(targets, vars) { + return _createTweenType(1, arguments); + }; + + Tween.delayedCall = function delayedCall(delay, callback, params, scope) { + return new Tween(callback, 0, { + immediateRender: false, + lazy: false, + overwrite: false, + delay: delay, + onComplete: callback, + onReverseComplete: callback, + onCompleteParams: params, + onReverseCompleteParams: params, + callbackScope: scope + }); + }; + + Tween.fromTo = function fromTo(targets, fromVars, toVars) { + return _createTweenType(2, arguments); + }; + + Tween.set = function set(targets, vars) { + vars.duration = 0; + vars.repeatDelay || (vars.repeat = 0); + return new Tween(targets, vars); + }; + + Tween.killTweensOf = function killTweensOf(targets, props, onlyActive) { + return _globalTimeline.killTweensOf(targets, props, onlyActive); + }; + + return Tween; + }(Animation); + + _setDefaults(Tween.prototype, { + _targets: [], + _lazy: 0, + _startAt: 0, + _op: 0, + _onInit: 0 + }); + + _forEachName("staggerTo,staggerFrom,staggerFromTo", function (name) { + Tween[name] = function () { + var tl = new Timeline(), + params = _slice.call(arguments, 0); + + params.splice(name === "staggerFromTo" ? 5 : 4, 0, 0); + return tl[name].apply(tl, params); + }; + }); + + var _setterPlain = function _setterPlain(target, property, value) { + return target[property] = value; + }, + _setterFunc = function _setterFunc(target, property, value) { + return target[property](value); + }, + _setterFuncWithParam = function _setterFuncWithParam(target, property, value, data) { + return target[property](data.fp, value); + }, + _setterAttribute = function _setterAttribute(target, property, value) { + return target.setAttribute(property, value); + }, + _getSetter = function _getSetter(target, property) { + return _isFunction(target[property]) ? _setterFunc : _isUndefined(target[property]) && target.setAttribute ? _setterAttribute : _setterPlain; + }, + _renderPlain = function _renderPlain(ratio, data) { + return data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 1000000) / 1000000, data); + }, + _renderBoolean = function _renderBoolean(ratio, data) { + return data.set(data.t, data.p, !!(data.s + data.c * ratio), data); + }, + _renderComplexString = function _renderComplexString(ratio, data) { + var pt = data._pt, + s = ""; + + if (!ratio && data.b) { + s = data.b; + } else if (ratio === 1 && data.e) { + s = data.e; + } else { + while (pt) { + s = pt.p + (pt.m ? pt.m(pt.s + pt.c * ratio) : Math.round((pt.s + pt.c * ratio) * 10000) / 10000) + s; + pt = pt._next; + } + + s += data.c; + } + + data.set(data.t, data.p, s, data); + }, + _renderPropTweens = function _renderPropTweens(ratio, data) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + }, + _addPluginModifier = function _addPluginModifier(modifier, tween, target, property) { + var pt = this._pt, + next; + + while (pt) { + next = pt._next; + pt.p === property && pt.modifier(modifier, tween, target); + pt = next; + } + }, + _killPropTweensOf = function _killPropTweensOf(property) { + var pt = this._pt, + hasNonDependentRemaining, + next; + + while (pt) { + next = pt._next; + + if (pt.p === property && !pt.op || pt.op === property) { + _removeLinkedListItem(this, pt, "_pt"); + } else if (!pt.dep) { + hasNonDependentRemaining = 1; + } + + pt = next; + } + + return !hasNonDependentRemaining; + }, + _setterWithModifier = function _setterWithModifier(target, property, value, data) { + data.mSet(target, property, data.m.call(data.tween, value, data.mt), data); + }, + _sortPropTweensByPriority = function _sortPropTweensByPriority(parent) { + var pt = parent._pt, + next, + pt2, + first, + last; + + while (pt) { + next = pt._next; + pt2 = first; + + while (pt2 && pt2.pr > pt.pr) { + pt2 = pt2._next; + } + + if (pt._prev = pt2 ? pt2._prev : last) { + pt._prev._next = pt; + } else { + first = pt; + } + + if (pt._next = pt2) { + pt2._prev = pt; + } else { + last = pt; + } + + pt = next; + } + + parent._pt = first; + }; + + var PropTween = function () { + function PropTween(next, target, prop, start, change, renderer, data, setter, priority) { + this.t = target; + this.s = start; + this.c = change; + this.p = prop; + this.r = renderer || _renderPlain; + this.d = data || this; + this.set = setter || _setterPlain; + this.pr = priority || 0; + this._next = next; + + if (next) { + next._prev = this; + } + } + + var _proto4 = PropTween.prototype; + + _proto4.modifier = function modifier(func, tween, target) { + this.mSet = this.mSet || this.set; + this.set = _setterWithModifier; + this.m = func; + this.mt = target; + this.tween = tween; + }; + + return PropTween; + }(); + + _forEachName(_callbackNames + "parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger", function (name) { + return _reservedProps[name] = 1; + }); + + _globals.TweenMax = _globals.TweenLite = Tween; + _globals.TimelineLite = _globals.TimelineMax = Timeline; + _globalTimeline = new Timeline({ + sortChildren: false, + defaults: _defaults, + autoRemoveChildren: true, + id: "root", + smoothChildTiming: true + }); + _config.stringFilter = _colorStringFilter; + + var _media = [], + _listeners = {}, + _emptyArray = [], + _lastMediaTime = 0, + _contextID = 0, + _dispatch = function _dispatch(type) { + return (_listeners[type] || _emptyArray).map(function (f) { + return f(); + }); + }, + _onMediaChange = function _onMediaChange() { + var time = Date.now(), + matches = []; + + if (time - _lastMediaTime > 2) { + _dispatch("matchMediaInit"); + + _media.forEach(function (c) { + var queries = c.queries, + conditions = c.conditions, + match, + p, + anyMatch, + toggled; + + for (p in queries) { + match = _win.matchMedia(queries[p]).matches; + match && (anyMatch = 1); + + if (match !== conditions[p]) { + conditions[p] = match; + toggled = 1; + } + } + + if (toggled) { + c.revert(); + anyMatch && matches.push(c); + } + }); + + _dispatch("matchMediaRevert"); + + matches.forEach(function (c) { + return c.onMatch(c, function (func) { + return c.add(null, func); + }); + }); + _lastMediaTime = time; + + _dispatch("matchMedia"); + } + }; + + var Context = function () { + function Context(func, scope) { + this.selector = scope && selector(scope); + this.data = []; + this._r = []; + this.isReverted = false; + this.id = _contextID++; + func && this.add(func); + } + + var _proto5 = Context.prototype; + + _proto5.add = function add(name, func, scope) { + if (_isFunction(name)) { + scope = func; + func = name; + name = _isFunction; + } + + var self = this, + f = function f() { + var prev = _context, + prevSelector = self.selector, + result; + prev && prev !== self && prev.data.push(self); + scope && (self.selector = selector(scope)); + _context = self; + result = func.apply(self, arguments); + _isFunction(result) && self._r.push(result); + _context = prev; + self.selector = prevSelector; + self.isReverted = false; + return result; + }; + + self.last = f; + return name === _isFunction ? f(self, function (func) { + return self.add(null, func); + }) : name ? self[name] = f : f; + }; + + _proto5.ignore = function ignore(func) { + var prev = _context; + _context = null; + func(this); + _context = prev; + }; + + _proto5.getTweens = function getTweens() { + var a = []; + this.data.forEach(function (e) { + return e instanceof Context ? a.push.apply(a, e.getTweens()) : e instanceof Tween && !(e.parent && e.parent.data === "nested") && a.push(e); + }); + return a; + }; + + _proto5.clear = function clear() { + this._r.length = this.data.length = 0; + }; + + _proto5.kill = function kill(revert, matchMedia) { + var _this4 = this; + + if (revert) { + (function () { + var tweens = _this4.getTweens(), + i = _this4.data.length, + t; + + while (i--) { + t = _this4.data[i]; + + if (t.data === "isFlip") { + t.revert(); + t.getChildren(true, true, false).forEach(function (tween) { + return tweens.splice(tweens.indexOf(tween), 1); + }); + } + } + + tweens.map(function (t) { + return { + g: t._dur || t._delay || t._sat && !t._sat.vars.immediateRender ? t.globalTime(0) : -Infinity, + t: t + }; + }).sort(function (a, b) { + return b.g - a.g || -Infinity; + }).forEach(function (o) { + return o.t.revert(revert); + }); + i = _this4.data.length; + + while (i--) { + t = _this4.data[i]; + + if (t instanceof Timeline) { + if (t.data !== "nested") { + t.scrollTrigger && t.scrollTrigger.revert(); + t.kill(); + } + } else { + !(t instanceof Tween) && t.revert && t.revert(revert); + } + } + + _this4._r.forEach(function (f) { + return f(revert, _this4); + }); + + _this4.isReverted = true; + })(); + } else { + this.data.forEach(function (e) { + return e.kill && e.kill(); + }); + } + + this.clear(); + + if (matchMedia) { + var i = _media.length; + + while (i--) { + _media[i].id === this.id && _media.splice(i, 1); + } + } + }; + + _proto5.revert = function revert(config) { + this.kill(config || {}); + }; + + return Context; + }(); + + var MatchMedia = function () { + function MatchMedia(scope) { + this.contexts = []; + this.scope = scope; + _context && _context.data.push(this); + } + + var _proto6 = MatchMedia.prototype; + + _proto6.add = function add(conditions, func, scope) { + _isObject(conditions) || (conditions = { + matches: conditions + }); + var context = new Context(0, scope || this.scope), + cond = context.conditions = {}, + mq, + p, + active; + _context && !context.selector && (context.selector = _context.selector); + this.contexts.push(context); + func = context.add("onMatch", func); + context.queries = conditions; + + for (p in conditions) { + if (p === "all") { + active = 1; + } else { + mq = _win.matchMedia(conditions[p]); + + if (mq) { + _media.indexOf(context) < 0 && _media.push(context); + (cond[p] = mq.matches) && (active = 1); + mq.addListener ? mq.addListener(_onMediaChange) : mq.addEventListener("change", _onMediaChange); + } + } + } + + active && func(context, function (f) { + return context.add(null, f); + }); + return this; + }; + + _proto6.revert = function revert(config) { + this.kill(config || {}); + }; + + _proto6.kill = function kill(revert) { + this.contexts.forEach(function (c) { + return c.kill(revert, true); + }); + }; + + return MatchMedia; + }(); + + var _gsap = { + registerPlugin: function registerPlugin() { + for (var _len2 = arguments.length, args = new Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { + args[_key2] = arguments[_key2]; + } + + args.forEach(function (config) { + return _createPlugin(config); + }); + }, + timeline: function timeline(vars) { + return new Timeline(vars); + }, + getTweensOf: function getTweensOf(targets, onlyActive) { + return _globalTimeline.getTweensOf(targets, onlyActive); + }, + getProperty: function getProperty(target, property, unit, uncache) { + _isString(target) && (target = toArray(target)[0]); + + var getter = _getCache(target || {}).get, + format = unit ? _passThrough : _numericIfPossible; + + unit === "native" && (unit = ""); + return !target ? target : !property ? function (property, unit, uncache) { + return format((_plugins[property] && _plugins[property].get || getter)(target, property, unit, uncache)); + } : format((_plugins[property] && _plugins[property].get || getter)(target, property, unit, uncache)); + }, + quickSetter: function quickSetter(target, property, unit) { + target = toArray(target); + + if (target.length > 1) { + var setters = target.map(function (t) { + return gsap.quickSetter(t, property, unit); + }), + l = setters.length; + return function (value) { + var i = l; + + while (i--) { + setters[i](value); + } + }; + } + + target = target[0] || {}; + + var Plugin = _plugins[property], + cache = _getCache(target), + p = cache.harness && (cache.harness.aliases || {})[property] || property, + setter = Plugin ? function (value) { + var p = new Plugin(); + _quickTween._pt = 0; + p.init(target, unit ? value + unit : value, _quickTween, 0, [target]); + p.render(1, p); + _quickTween._pt && _renderPropTweens(1, _quickTween); + } : cache.set(target, p); + + return Plugin ? setter : function (value) { + return setter(target, p, unit ? value + unit : value, cache, 1); + }; + }, + quickTo: function quickTo(target, property, vars) { + var _setDefaults2; + + var tween = gsap.to(target, _setDefaults((_setDefaults2 = {}, _setDefaults2[property] = "+=0.1", _setDefaults2.paused = true, _setDefaults2.stagger = 0, _setDefaults2), vars || {})), + func = function func(value, start, startIsRelative) { + return tween.resetTo(property, value, start, startIsRelative); + }; + + func.tween = tween; + return func; + }, + isTweening: function isTweening(targets) { + return _globalTimeline.getTweensOf(targets, true).length > 0; + }, + defaults: function defaults(value) { + value && value.ease && (value.ease = _parseEase(value.ease, _defaults.ease)); + return _mergeDeep(_defaults, value || {}); + }, + config: function config(value) { + return _mergeDeep(_config, value || {}); + }, + registerEffect: function registerEffect(_ref3) { + var name = _ref3.name, + effect = _ref3.effect, + plugins = _ref3.plugins, + defaults = _ref3.defaults, + extendTimeline = _ref3.extendTimeline; + (plugins || "").split(",").forEach(function (pluginName) { + return pluginName && !_plugins[pluginName] && !_globals[pluginName] && _warn(name + " effect requires " + pluginName + " plugin."); + }); + + _effects[name] = function (targets, vars, tl) { + return effect(toArray(targets), _setDefaults(vars || {}, defaults), tl); + }; + + if (extendTimeline) { + Timeline.prototype[name] = function (targets, vars, position) { + return this.add(_effects[name](targets, _isObject(vars) ? vars : (position = vars) && {}, this), position); + }; + } + }, + registerEase: function registerEase(name, ease) { + _easeMap[name] = _parseEase(ease); + }, + parseEase: function parseEase(ease, defaultEase) { + return arguments.length ? _parseEase(ease, defaultEase) : _easeMap; + }, + getById: function getById(id) { + return _globalTimeline.getById(id); + }, + exportRoot: function exportRoot(vars, includeDelayedCalls) { + if (vars === void 0) { + vars = {}; + } + + var tl = new Timeline(vars), + child, + next; + tl.smoothChildTiming = _isNotFalse(vars.smoothChildTiming); + + _globalTimeline.remove(tl); + + tl._dp = 0; + tl._time = tl._tTime = _globalTimeline._time; + child = _globalTimeline._first; + + while (child) { + next = child._next; + + if (includeDelayedCalls || !(!child._dur && child instanceof Tween && child.vars.onComplete === child._targets[0])) { + _addToTimeline(tl, child, child._start - child._delay); + } + + child = next; + } + + _addToTimeline(_globalTimeline, tl, 0); + + return tl; + }, + context: function context(func, scope) { + return func ? new Context(func, scope) : _context; + }, + matchMedia: function matchMedia(scope) { + return new MatchMedia(scope); + }, + matchMediaRefresh: function matchMediaRefresh() { + return _media.forEach(function (c) { + var cond = c.conditions, + found, + p; + + for (p in cond) { + if (cond[p]) { + cond[p] = false; + found = 1; + } + } + + found && c.revert(); + }) || _onMediaChange(); + }, + addEventListener: function addEventListener(type, callback) { + var a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); + }, + removeEventListener: function removeEventListener(type, callback) { + var a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); + }, + utils: { + wrap: wrap, + wrapYoyo: wrapYoyo, + distribute: distribute, + random: random, + snap: snap, + normalize: normalize, + getUnit: getUnit, + clamp: clamp, + splitColor: splitColor, + toArray: toArray, + selector: selector, + mapRange: mapRange, + pipe: pipe, + unitize: unitize, + interpolate: interpolate, + shuffle: shuffle + }, + install: _install, + effects: _effects, + ticker: _ticker, + updateRoot: Timeline.updateRoot, + plugins: _plugins, + globalTimeline: _globalTimeline, + core: { + PropTween: PropTween, + globals: _addGlobal, + Tween: Tween, + Timeline: Timeline, + Animation: Animation, + getCache: _getCache, + _removeLinkedListItem: _removeLinkedListItem, + reverting: function reverting() { + return _reverting; + }, + context: function context(toAdd) { + if (toAdd && _context) { + _context.data.push(toAdd); + + toAdd._ctx = _context; + } + + return _context; + }, + suppressOverwrites: function suppressOverwrites(value) { + return _suppressOverwrites = value; + } + } + }; + + _forEachName("to,from,fromTo,delayedCall,set,killTweensOf", function (name) { + return _gsap[name] = Tween[name]; + }); + + _ticker.add(Timeline.updateRoot); + + _quickTween = _gsap.to({}, { + duration: 0 + }); + + var _getPluginPropTween = function _getPluginPropTween(plugin, prop) { + var pt = plugin._pt; + + while (pt && pt.p !== prop && pt.op !== prop && pt.fp !== prop) { + pt = pt._next; + } + + return pt; + }, + _addModifiers = function _addModifiers(tween, modifiers) { + var targets = tween._targets, + p, + i, + pt; + + for (p in modifiers) { + i = targets.length; + + while (i--) { + pt = tween._ptLookup[i][p]; + + if (pt && (pt = pt.d)) { + if (pt._pt) { + pt = _getPluginPropTween(pt, p); + } + + pt && pt.modifier && pt.modifier(modifiers[p], tween, targets[i], p); + } + } + } + }, + _buildModifierPlugin = function _buildModifierPlugin(name, modifier) { + return { + name: name, + rawVars: 1, + init: function init(target, vars, tween) { + tween._onInit = function (tween) { + var temp, p; + + if (_isString(vars)) { + temp = {}; + + _forEachName(vars, function (name) { + return temp[name] = 1; + }); + + vars = temp; + } + + if (modifier) { + temp = {}; + + for (p in vars) { + temp[p] = modifier(vars[p]); + } + + vars = temp; + } + + _addModifiers(tween, vars); + }; + } + }; + }; + + var gsap = _gsap.registerPlugin({ + name: "attr", + init: function init(target, vars, tween, index, targets) { + var p, pt, v; + this.tween = tween; + + for (p in vars) { + v = target.getAttribute(p) || ""; + pt = this.add(target, "setAttribute", (v || 0) + "", vars[p], index, targets, 0, 0, p); + pt.op = p; + pt.b = v; + + this._props.push(p); + } + }, + render: function render(ratio, data) { + var pt = data._pt; + + while (pt) { + _reverting ? pt.set(pt.t, pt.p, pt.b, pt) : pt.r(ratio, pt.d); + pt = pt._next; + } + } + }, { + name: "endArray", + init: function init(target, value) { + var i = value.length; + + while (i--) { + this.add(target, i, target[i] || 0, value[i], 0, 0, 0, 0, 0, 1); + } + } + }, _buildModifierPlugin("roundProps", _roundModifier), _buildModifierPlugin("modifiers"), _buildModifierPlugin("snap", snap)) || _gsap; + Tween.version = Timeline.version = gsap.version = "3.12.7"; + _coreReady = 1; + _windowExists() && _wake(); + var Power0 = _easeMap.Power0, + Power1 = _easeMap.Power1, + Power2 = _easeMap.Power2, + Power3 = _easeMap.Power3, + Power4 = _easeMap.Power4, + Linear = _easeMap.Linear, + Quad = _easeMap.Quad, + Cubic = _easeMap.Cubic, + Quart = _easeMap.Quart, + Quint = _easeMap.Quint, + Strong = _easeMap.Strong, + Elastic = _easeMap.Elastic, + Back = _easeMap.Back, + SteppedEase = _easeMap.SteppedEase, + Bounce = _easeMap.Bounce, + Sine = _easeMap.Sine, + Expo = _easeMap.Expo, + Circ = _easeMap.Circ; + + var _win$1, + _doc$1, + _docElement, + _pluginInitted, + _tempDiv, + _tempDivStyler, + _recentSetterPlugin, + _reverting$1, + _windowExists$1 = function _windowExists() { + return typeof window !== "undefined"; + }, + _transformProps = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _atan2 = Math.atan2, + _bigNum$1 = 1e8, + _capsExp = /([A-Z])/g, + _horizontalExp = /(left|right|width|margin|padding|x)/i, + _complexExp = /[\s,\(]\S/, + _propertyAliases = { + autoAlpha: "opacity,visibility", + scale: "scaleX,scaleY", + alpha: "opacity" + }, + _renderCSSProp = function _renderCSSProp(ratio, data) { + return data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u, data); + }, + _renderPropWithEnd = function _renderPropWithEnd(ratio, data) { + return data.set(data.t, data.p, ratio === 1 ? data.e : Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u, data); + }, + _renderCSSPropWithBeginning = function _renderCSSPropWithBeginning(ratio, data) { + return data.set(data.t, data.p, ratio ? Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u : data.b, data); + }, + _renderRoundedCSSProp = function _renderRoundedCSSProp(ratio, data) { + var value = data.s + data.c * ratio; + data.set(data.t, data.p, ~~(value + (value < 0 ? -.5 : .5)) + data.u, data); + }, + _renderNonTweeningValue = function _renderNonTweeningValue(ratio, data) { + return data.set(data.t, data.p, ratio ? data.e : data.b, data); + }, + _renderNonTweeningValueOnlyAtEnd = function _renderNonTweeningValueOnlyAtEnd(ratio, data) { + return data.set(data.t, data.p, ratio !== 1 ? data.b : data.e, data); + }, + _setterCSSStyle = function _setterCSSStyle(target, property, value) { + return target.style[property] = value; + }, + _setterCSSProp = function _setterCSSProp(target, property, value) { + return target.style.setProperty(property, value); + }, + _setterTransform = function _setterTransform(target, property, value) { + return target._gsap[property] = value; + }, + _setterScale = function _setterScale(target, property, value) { + return target._gsap.scaleX = target._gsap.scaleY = value; + }, + _setterScaleWithRender = function _setterScaleWithRender(target, property, value, data, ratio) { + var cache = target._gsap; + cache.scaleX = cache.scaleY = value; + cache.renderTransform(ratio, cache); + }, + _setterTransformWithRender = function _setterTransformWithRender(target, property, value, data, ratio) { + var cache = target._gsap; + cache[property] = value; + cache.renderTransform(ratio, cache); + }, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _saveStyle = function _saveStyle(property, isNotCSS) { + var _this = this; + + var target = this.target, + style = target.style, + cache = target._gsap; + + if (property in _transformProps && style) { + this.tfm = this.tfm || {}; + + if (property !== "transform") { + property = _propertyAliases[property] || property; + ~property.indexOf(",") ? property.split(",").forEach(function (a) { + return _this.tfm[a] = _get(target, a); + }) : this.tfm[property] = cache.x ? cache[property] : _get(target, property); + property === _transformOriginProp && (this.tfm.zOrigin = cache.zOrigin); + } else { + return _propertyAliases.transform.split(",").forEach(function (p) { + return _saveStyle.call(_this, p, isNotCSS); + }); + } + + if (this.props.indexOf(_transformProp) >= 0) { + return; + } + + if (cache.svg) { + this.svgo = target.getAttribute("data-svg-origin"); + this.props.push(_transformOriginProp, isNotCSS, ""); + } + + property = _transformProp; + } + + (style || isNotCSS) && this.props.push(property, isNotCSS, style[property]); + }, + _removeIndependentTransforms = function _removeIndependentTransforms(style) { + if (style.translate) { + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } + }, + _revertStyle = function _revertStyle() { + var props = this.props, + target = this.target, + style = target.style, + cache = target._gsap, + i, + p; + + for (i = 0; i < props.length; i += 3) { + if (!props[i + 1]) { + props[i + 2] ? style[props[i]] = props[i + 2] : style.removeProperty(props[i].substr(0, 2) === "--" ? props[i] : props[i].replace(_capsExp, "-$1").toLowerCase()); + } else if (props[i + 1] === 2) { + target[props[i]](props[i + 2]); + } else { + target[props[i]] = props[i + 2]; + } + } + + if (this.tfm) { + for (p in this.tfm) { + cache[p] = this.tfm[p]; + } + + if (cache.svg) { + cache.renderTransform(); + target.setAttribute("data-svg-origin", this.svgo || ""); + } + + i = _reverting$1(); + + if ((!i || !i.isStart) && !style[_transformProp]) { + _removeIndependentTransforms(style); + + if (cache.zOrigin && style[_transformOriginProp]) { + style[_transformOriginProp] += " " + cache.zOrigin + "px"; + cache.zOrigin = 0; + cache.renderTransform(); + } + + cache.uncache = 1; + } + } + }, + _getStyleSaver = function _getStyleSaver(target, properties) { + var saver = { + target: target, + props: [], + revert: _revertStyle, + save: _saveStyle + }; + target._gsap || gsap.core.getCache(target); + properties && target.style && target.nodeType && properties.split(",").forEach(function (p) { + return saver.save(p); + }); + return saver; + }, + _supports3D, + _createElement = function _createElement(type, ns) { + var e = _doc$1.createElementNS ? _doc$1.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc$1.createElement(type); + return e && e.style ? e : _doc$1.createElement(type); + }, + _getComputedProperty = function _getComputedProperty(target, property, skipPrefixFallback) { + var cs = getComputedStyle(target); + return cs[property] || cs.getPropertyValue(property.replace(_capsExp, "-$1").toLowerCase()) || cs.getPropertyValue(property) || !skipPrefixFallback && _getComputedProperty(target, _checkPropPrefix(property) || property, 1) || ""; + }, + _prefixes = "O,Moz,ms,Ms,Webkit".split(","), + _checkPropPrefix = function _checkPropPrefix(property, element, preferPrefix) { + var e = element || _tempDiv, + s = e.style, + i = 5; + + if (property in s && !preferPrefix) { + return property; + } + + property = property.charAt(0).toUpperCase() + property.substr(1); + + while (i-- && !(_prefixes[i] + property in s)) {} + + return i < 0 ? null : (i === 3 ? "ms" : i >= 0 ? _prefixes[i] : "") + property; + }, + _initCore = function _initCore() { + if (_windowExists$1() && window.document) { + _win$1 = window; + _doc$1 = _win$1.document; + _docElement = _doc$1.documentElement; + _tempDiv = _createElement("div") || { + style: {} + }; + _tempDivStyler = _createElement("div"); + _transformProp = _checkPropPrefix(_transformProp); + _transformOriginProp = _transformProp + "Origin"; + _tempDiv.style.cssText = "border-width:0;line-height:0;position:absolute;padding:0"; + _supports3D = !!_checkPropPrefix("perspective"); + _reverting$1 = gsap.core.reverting; + _pluginInitted = 1; + } + }, + _getReparentedCloneBBox = function _getReparentedCloneBBox(target) { + var owner = target.ownerSVGElement, + svg = _createElement("svg", owner && owner.getAttribute("xmlns") || "http://www.w3.org/2000/svg"), + clone = target.cloneNode(true), + bbox; + + clone.style.display = "block"; + svg.appendChild(clone); + + _docElement.appendChild(svg); + + try { + bbox = clone.getBBox(); + } catch (e) {} + + svg.removeChild(clone); + + _docElement.removeChild(svg); + + return bbox; + }, + _getAttributeFallbacks = function _getAttributeFallbacks(target, attributesArray) { + var i = attributesArray.length; + + while (i--) { + if (target.hasAttribute(attributesArray[i])) { + return target.getAttribute(attributesArray[i]); + } + } + }, + _getBBox = function _getBBox(target) { + var bounds, cloned; + + try { + bounds = target.getBBox(); + } catch (error) { + bounds = _getReparentedCloneBBox(target); + cloned = 1; + } + + bounds && (bounds.width || bounds.height) || cloned || (bounds = _getReparentedCloneBBox(target)); + return bounds && !bounds.width && !bounds.x && !bounds.y ? { + x: +_getAttributeFallbacks(target, ["x", "cx", "x1"]) || 0, + y: +_getAttributeFallbacks(target, ["y", "cy", "y1"]) || 0, + width: 0, + height: 0 + } : bounds; + }, + _isSVG = function _isSVG(e) { + return !!(e.getCTM && (!e.parentNode || e.ownerSVGElement) && _getBBox(e)); + }, + _removeProperty = function _removeProperty(target, property) { + if (property) { + var style = target.style, + first2Chars; + + if (property in _transformProps && property !== _transformOriginProp) { + property = _transformProp; + } + + if (style.removeProperty) { + first2Chars = property.substr(0, 2); + + if (first2Chars === "ms" || property.substr(0, 6) === "webkit") { + property = "-" + property; + } + + style.removeProperty(first2Chars === "--" ? property : property.replace(_capsExp, "-$1").toLowerCase()); + } else { + style.removeAttribute(property); + } + } + }, + _addNonTweeningPT = function _addNonTweeningPT(plugin, target, property, beginning, end, onlySetAtEnd) { + var pt = new PropTween(plugin._pt, target, property, 0, 1, onlySetAtEnd ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue); + plugin._pt = pt; + pt.b = beginning; + pt.e = end; + + plugin._props.push(property); + + return pt; + }, + _nonConvertibleUnits = { + deg: 1, + rad: 1, + turn: 1 + }, + _nonStandardLayouts = { + grid: 1, + flex: 1 + }, + _convertToUnit = function _convertToUnit(target, property, value, unit) { + var curValue = parseFloat(value) || 0, + curUnit = (value + "").trim().substr((curValue + "").length) || "px", + style = _tempDiv.style, + horizontal = _horizontalExp.test(property), + isRootSVG = target.tagName.toLowerCase() === "svg", + measureProperty = (isRootSVG ? "client" : "offset") + (horizontal ? "Width" : "Height"), + amount = 100, + toPixels = unit === "px", + toPercent = unit === "%", + px, + parent, + cache, + isSVG; + + if (unit === curUnit || !curValue || _nonConvertibleUnits[unit] || _nonConvertibleUnits[curUnit]) { + return curValue; + } + + curUnit !== "px" && !toPixels && (curValue = _convertToUnit(target, property, value, "px")); + isSVG = target.getCTM && _isSVG(target); + + if ((toPercent || curUnit === "%") && (_transformProps[property] || ~property.indexOf("adius"))) { + px = isSVG ? target.getBBox()[horizontal ? "width" : "height"] : target[measureProperty]; + return _round(toPercent ? curValue / px * amount : curValue / 100 * px); + } + + style[horizontal ? "width" : "height"] = amount + (toPixels ? curUnit : unit); + parent = unit !== "rem" && ~property.indexOf("adius") || unit === "em" && target.appendChild && !isRootSVG ? target : target.parentNode; + + if (isSVG) { + parent = (target.ownerSVGElement || {}).parentNode; + } + + if (!parent || parent === _doc$1 || !parent.appendChild) { + parent = _doc$1.body; + } + + cache = parent._gsap; + + if (cache && toPercent && cache.width && horizontal && cache.time === _ticker.time && !cache.uncache) { + return _round(curValue / cache.width * amount); + } else { + if (toPercent && (property === "height" || property === "width")) { + var v = target.style[property]; + target.style[property] = amount + unit; + px = target[measureProperty]; + v ? target.style[property] = v : _removeProperty(target, property); + } else { + (toPercent || curUnit === "%") && !_nonStandardLayouts[_getComputedProperty(parent, "display")] && (style.position = _getComputedProperty(target, "position")); + parent === target && (style.position = "static"); + parent.appendChild(_tempDiv); + px = _tempDiv[measureProperty]; + parent.removeChild(_tempDiv); + style.position = "absolute"; + } + + if (horizontal && toPercent) { + cache = _getCache(parent); + cache.time = _ticker.time; + cache.width = parent[measureProperty]; + } + } + + return _round(toPixels ? px * curValue / amount : px && curValue ? amount / px * curValue : 0); + }, + _get = function _get(target, property, unit, uncache) { + var value; + _pluginInitted || _initCore(); + + if (property in _propertyAliases && property !== "transform") { + property = _propertyAliases[property]; + + if (~property.indexOf(",")) { + property = property.split(",")[0]; + } + } + + if (_transformProps[property] && property !== "transform") { + value = _parseTransform(target, uncache); + value = property !== "transformOrigin" ? value[property] : value.svg ? value.origin : _firstTwoOnly(_getComputedProperty(target, _transformOriginProp)) + " " + value.zOrigin + "px"; + } else { + value = target.style[property]; + + if (!value || value === "auto" || uncache || ~(value + "").indexOf("calc(")) { + value = _specialProps[property] && _specialProps[property](target, property, unit) || _getComputedProperty(target, property) || _getProperty(target, property) || (property === "opacity" ? 1 : 0); + } + } + + return unit && !~(value + "").trim().indexOf(" ") ? _convertToUnit(target, property, value, unit) + unit : value; + }, + _tweenComplexCSSString = function _tweenComplexCSSString(target, prop, start, end) { + if (!start || start === "none") { + var p = _checkPropPrefix(prop, target, 1), + s = p && _getComputedProperty(target, p, 1); + + if (s && s !== start) { + prop = p; + start = s; + } else if (prop === "borderColor") { + start = _getComputedProperty(target, "borderTopColor"); + } + } + + var pt = new PropTween(this._pt, target.style, prop, 0, 1, _renderComplexString), + index = 0, + matchIndex = 0, + a, + result, + startValues, + startNum, + color, + startValue, + endValue, + endNum, + chunk, + endUnit, + startUnit, + endValues; + pt.b = start; + pt.e = end; + start += ""; + end += ""; + + if (end === "auto") { + startValue = target.style[prop]; + target.style[prop] = end; + end = _getComputedProperty(target, prop) || end; + startValue ? target.style[prop] = startValue : _removeProperty(target, prop); + } + + a = [start, end]; + + _colorStringFilter(a); + + start = a[0]; + end = a[1]; + startValues = start.match(_numWithUnitExp) || []; + endValues = end.match(_numWithUnitExp) || []; + + if (endValues.length) { + while (result = _numWithUnitExp.exec(end)) { + endValue = result[0]; + chunk = end.substring(index, result.index); + + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(" || chunk.substr(-5) === "hsla(") { + color = 1; + } + + if (endValue !== (startValue = startValues[matchIndex++] || "")) { + startNum = parseFloat(startValue) || 0; + startUnit = startValue.substr((startNum + "").length); + endValue.charAt(1) === "=" && (endValue = _parseRelative(startNum, endValue) + startUnit); + endNum = parseFloat(endValue); + endUnit = endValue.substr((endNum + "").length); + index = _numWithUnitExp.lastIndex - endUnit.length; + + if (!endUnit) { + endUnit = endUnit || _config.units[prop] || startUnit; + + if (index === end.length) { + end += endUnit; + pt.e += endUnit; + } + } + + if (startUnit !== endUnit) { + startNum = _convertToUnit(target, prop, startValue, endUnit) || 0; + } + + pt._pt = { + _next: pt._pt, + p: chunk || matchIndex === 1 ? chunk : ",", + s: startNum, + c: endNum - startNum, + m: color && color < 4 || prop === "zIndex" ? Math.round : 0 + }; + } + } + + pt.c = index < end.length ? end.substring(index, end.length) : ""; + } else { + pt.r = prop === "display" && end === "none" ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue; + } + + _relExp.test(end) && (pt.e = 0); + this._pt = pt; + return pt; + }, + _keywordToPercent = { + top: "0%", + bottom: "100%", + left: "0%", + right: "100%", + center: "50%" + }, + _convertKeywordsToPercentages = function _convertKeywordsToPercentages(value) { + var split = value.split(" "), + x = split[0], + y = split[1] || "50%"; + + if (x === "top" || x === "bottom" || y === "left" || y === "right") { + value = x; + x = y; + y = value; + } + + split[0] = _keywordToPercent[x] || x; + split[1] = _keywordToPercent[y] || y; + return split.join(" "); + }, + _renderClearProps = function _renderClearProps(ratio, data) { + if (data.tween && data.tween._time === data.tween._dur) { + var target = data.t, + style = target.style, + props = data.u, + cache = target._gsap, + prop, + clearTransforms, + i; + + if (props === "all" || props === true) { + style.cssText = ""; + clearTransforms = 1; + } else { + props = props.split(","); + i = props.length; + + while (--i > -1) { + prop = props[i]; + + if (_transformProps[prop]) { + clearTransforms = 1; + prop = prop === "transformOrigin" ? _transformOriginProp : _transformProp; + } + + _removeProperty(target, prop); + } + } + + if (clearTransforms) { + _removeProperty(target, _transformProp); + + if (cache) { + cache.svg && target.removeAttribute("transform"); + style.scale = style.rotate = style.translate = "none"; + + _parseTransform(target, 1); + + cache.uncache = 1; + + _removeIndependentTransforms(style); + } + } + } + }, + _specialProps = { + clearProps: function clearProps(plugin, target, property, endValue, tween) { + if (tween.data !== "isFromStart") { + var pt = plugin._pt = new PropTween(plugin._pt, target, property, 0, 0, _renderClearProps); + pt.u = endValue; + pt.pr = -10; + pt.tween = tween; + + plugin._props.push(property); + + return 1; + } + } + }, + _identity2DMatrix = [1, 0, 0, 1, 0, 0], + _rotationalProperties = {}, + _isNullTransform = function _isNullTransform(value) { + return value === "matrix(1, 0, 0, 1, 0, 0)" || value === "none" || !value; + }, + _getComputedTransformMatrixAsArray = function _getComputedTransformMatrixAsArray(target) { + var matrixString = _getComputedProperty(target, _transformProp); + + return _isNullTransform(matrixString) ? _identity2DMatrix : matrixString.substr(7).match(_numExp).map(_round); + }, + _getMatrix = function _getMatrix(target, force2D) { + var cache = target._gsap || _getCache(target), + style = target.style, + matrix = _getComputedTransformMatrixAsArray(target), + parent, + nextSibling, + temp, + addedToDOM; + + if (cache.svg && target.getAttribute("transform")) { + temp = target.transform.baseVal.consolidate().matrix; + matrix = [temp.a, temp.b, temp.c, temp.d, temp.e, temp.f]; + return matrix.join(",") === "1,0,0,1,0,0" ? _identity2DMatrix : matrix; + } else if (matrix === _identity2DMatrix && !target.offsetParent && target !== _docElement && !cache.svg) { + temp = style.display; + style.display = "block"; + parent = target.parentNode; + + if (!parent || !target.offsetParent && !target.getBoundingClientRect().width) { + addedToDOM = 1; + nextSibling = target.nextElementSibling; + + _docElement.appendChild(target); + } + + matrix = _getComputedTransformMatrixAsArray(target); + temp ? style.display = temp : _removeProperty(target, "display"); + + if (addedToDOM) { + nextSibling ? parent.insertBefore(target, nextSibling) : parent ? parent.appendChild(target) : _docElement.removeChild(target); + } + } + + return force2D && matrix.length > 6 ? [matrix[0], matrix[1], matrix[4], matrix[5], matrix[12], matrix[13]] : matrix; + }, + _applySVGOrigin = function _applySVGOrigin(target, origin, originIsAbsolute, smooth, matrixArray, pluginToAddPropTweensTo) { + var cache = target._gsap, + matrix = matrixArray || _getMatrix(target, true), + xOriginOld = cache.xOrigin || 0, + yOriginOld = cache.yOrigin || 0, + xOffsetOld = cache.xOffset || 0, + yOffsetOld = cache.yOffset || 0, + a = matrix[0], + b = matrix[1], + c = matrix[2], + d = matrix[3], + tx = matrix[4], + ty = matrix[5], + originSplit = origin.split(" "), + xOrigin = parseFloat(originSplit[0]) || 0, + yOrigin = parseFloat(originSplit[1]) || 0, + bounds, + determinant, + x, + y; + + if (!originIsAbsolute) { + bounds = _getBBox(target); + xOrigin = bounds.x + (~originSplit[0].indexOf("%") ? xOrigin / 100 * bounds.width : xOrigin); + yOrigin = bounds.y + (~(originSplit[1] || originSplit[0]).indexOf("%") ? yOrigin / 100 * bounds.height : yOrigin); + } else if (matrix !== _identity2DMatrix && (determinant = a * d - b * c)) { + x = xOrigin * (d / determinant) + yOrigin * (-c / determinant) + (c * ty - d * tx) / determinant; + y = xOrigin * (-b / determinant) + yOrigin * (a / determinant) - (a * ty - b * tx) / determinant; + xOrigin = x; + yOrigin = y; + } + + if (smooth || smooth !== false && cache.smooth) { + tx = xOrigin - xOriginOld; + ty = yOrigin - yOriginOld; + cache.xOffset = xOffsetOld + (tx * a + ty * c) - tx; + cache.yOffset = yOffsetOld + (tx * b + ty * d) - ty; + } else { + cache.xOffset = cache.yOffset = 0; + } + + cache.xOrigin = xOrigin; + cache.yOrigin = yOrigin; + cache.smooth = !!smooth; + cache.origin = origin; + cache.originIsAbsolute = !!originIsAbsolute; + target.style[_transformOriginProp] = "0px 0px"; + + if (pluginToAddPropTweensTo) { + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOrigin", xOriginOld, xOrigin); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOrigin", yOriginOld, yOrigin); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOffset", xOffsetOld, cache.xOffset); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOffset", yOffsetOld, cache.yOffset); + } + + target.setAttribute("data-svg-origin", xOrigin + " " + yOrigin); + }, + _parseTransform = function _parseTransform(target, uncache) { + var cache = target._gsap || new GSCache(target); + + if ("x" in cache && !uncache && !cache.uncache) { + return cache; + } + + var style = target.style, + invertedScaleX = cache.scaleX < 0, + px = "px", + deg = "deg", + cs = getComputedStyle(target), + origin = _getComputedProperty(target, _transformOriginProp) || "0", + x, + y, + z, + scaleX, + scaleY, + rotation, + rotationX, + rotationY, + skewX, + skewY, + perspective, + xOrigin, + yOrigin, + matrix, + angle, + cos, + sin, + a, + b, + c, + d, + a12, + a22, + t1, + t2, + t3, + a13, + a23, + a33, + a42, + a43, + a32; + x = y = z = rotation = rotationX = rotationY = skewX = skewY = perspective = 0; + scaleX = scaleY = 1; + cache.svg = !!(target.getCTM && _isSVG(target)); + + if (cs.translate) { + if (cs.translate !== "none" || cs.scale !== "none" || cs.rotate !== "none") { + style[_transformProp] = (cs.translate !== "none" ? "translate3d(" + (cs.translate + " 0 0").split(" ").slice(0, 3).join(", ") + ") " : "") + (cs.rotate !== "none" ? "rotate(" + cs.rotate + ") " : "") + (cs.scale !== "none" ? "scale(" + cs.scale.split(" ").join(",") + ") " : "") + (cs[_transformProp] !== "none" ? cs[_transformProp] : ""); + } + + style.scale = style.rotate = style.translate = "none"; + } + + matrix = _getMatrix(target, cache.svg); + + if (cache.svg) { + if (cache.uncache) { + t2 = target.getBBox(); + origin = cache.xOrigin - t2.x + "px " + (cache.yOrigin - t2.y) + "px"; + t1 = ""; + } else { + t1 = !uncache && target.getAttribute("data-svg-origin"); + } + + _applySVGOrigin(target, t1 || origin, !!t1 || cache.originIsAbsolute, cache.smooth !== false, matrix); + } + + xOrigin = cache.xOrigin || 0; + yOrigin = cache.yOrigin || 0; + + if (matrix !== _identity2DMatrix) { + a = matrix[0]; + b = matrix[1]; + c = matrix[2]; + d = matrix[3]; + x = a12 = matrix[4]; + y = a22 = matrix[5]; + + if (matrix.length === 6) { + scaleX = Math.sqrt(a * a + b * b); + scaleY = Math.sqrt(d * d + c * c); + rotation = a || b ? _atan2(b, a) * _RAD2DEG : 0; + skewX = c || d ? _atan2(c, d) * _RAD2DEG + rotation : 0; + skewX && (scaleY *= Math.abs(Math.cos(skewX * _DEG2RAD))); + + if (cache.svg) { + x -= xOrigin - (xOrigin * a + yOrigin * c); + y -= yOrigin - (xOrigin * b + yOrigin * d); + } + } else { + a32 = matrix[6]; + a42 = matrix[7]; + a13 = matrix[8]; + a23 = matrix[9]; + a33 = matrix[10]; + a43 = matrix[11]; + x = matrix[12]; + y = matrix[13]; + z = matrix[14]; + angle = _atan2(a32, a33); + rotationX = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a12 * cos + a13 * sin; + t2 = a22 * cos + a23 * sin; + t3 = a32 * cos + a33 * sin; + a13 = a12 * -sin + a13 * cos; + a23 = a22 * -sin + a23 * cos; + a33 = a32 * -sin + a33 * cos; + a43 = a42 * -sin + a43 * cos; + a12 = t1; + a22 = t2; + a32 = t3; + } + + angle = _atan2(-c, a33); + rotationY = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a * cos - a13 * sin; + t2 = b * cos - a23 * sin; + t3 = c * cos - a33 * sin; + a43 = d * sin + a43 * cos; + a = t1; + b = t2; + c = t3; + } + + angle = _atan2(b, a); + rotation = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(angle); + sin = Math.sin(angle); + t1 = a * cos + b * sin; + t2 = a12 * cos + a22 * sin; + b = b * cos - a * sin; + a22 = a22 * cos - a12 * sin; + a = t1; + a12 = t2; + } + + if (rotationX && Math.abs(rotationX) + Math.abs(rotation) > 359.9) { + rotationX = rotation = 0; + rotationY = 180 - rotationY; + } + + scaleX = _round(Math.sqrt(a * a + b * b + c * c)); + scaleY = _round(Math.sqrt(a22 * a22 + a32 * a32)); + angle = _atan2(a12, a22); + skewX = Math.abs(angle) > 0.0002 ? angle * _RAD2DEG : 0; + perspective = a43 ? 1 / (a43 < 0 ? -a43 : a43) : 0; + } + + if (cache.svg) { + t1 = target.getAttribute("transform"); + cache.forceCSS = target.setAttribute("transform", "") || !_isNullTransform(_getComputedProperty(target, _transformProp)); + t1 && target.setAttribute("transform", t1); + } + } + + if (Math.abs(skewX) > 90 && Math.abs(skewX) < 270) { + if (invertedScaleX) { + scaleX *= -1; + skewX += rotation <= 0 ? 180 : -180; + rotation += rotation <= 0 ? 180 : -180; + } else { + scaleY *= -1; + skewX += skewX <= 0 ? 180 : -180; + } + } + + uncache = uncache || cache.uncache; + cache.x = x - ((cache.xPercent = x && (!uncache && cache.xPercent || (Math.round(target.offsetWidth / 2) === Math.round(-x) ? -50 : 0))) ? target.offsetWidth * cache.xPercent / 100 : 0) + px; + cache.y = y - ((cache.yPercent = y && (!uncache && cache.yPercent || (Math.round(target.offsetHeight / 2) === Math.round(-y) ? -50 : 0))) ? target.offsetHeight * cache.yPercent / 100 : 0) + px; + cache.z = z + px; + cache.scaleX = _round(scaleX); + cache.scaleY = _round(scaleY); + cache.rotation = _round(rotation) + deg; + cache.rotationX = _round(rotationX) + deg; + cache.rotationY = _round(rotationY) + deg; + cache.skewX = skewX + deg; + cache.skewY = skewY + deg; + cache.transformPerspective = perspective + px; + + if (cache.zOrigin = parseFloat(origin.split(" ")[2]) || !uncache && cache.zOrigin || 0) { + style[_transformOriginProp] = _firstTwoOnly(origin); + } + + cache.xOffset = cache.yOffset = 0; + cache.force3D = _config.force3D; + cache.renderTransform = cache.svg ? _renderSVGTransforms : _supports3D ? _renderCSSTransforms : _renderNon3DTransforms; + cache.uncache = 0; + return cache; + }, + _firstTwoOnly = function _firstTwoOnly(value) { + return (value = value.split(" "))[0] + " " + value[1]; + }, + _addPxTranslate = function _addPxTranslate(target, start, value) { + var unit = getUnit(start); + return _round(parseFloat(start) + parseFloat(_convertToUnit(target, "x", value + "px", unit))) + unit; + }, + _renderNon3DTransforms = function _renderNon3DTransforms(ratio, cache) { + cache.z = "0px"; + cache.rotationY = cache.rotationX = "0deg"; + cache.force3D = 0; + + _renderCSSTransforms(ratio, cache); + }, + _zeroDeg = "0deg", + _zeroPx = "0px", + _endParenthesis = ") ", + _renderCSSTransforms = function _renderCSSTransforms(ratio, cache) { + var _ref = cache || this, + xPercent = _ref.xPercent, + yPercent = _ref.yPercent, + x = _ref.x, + y = _ref.y, + z = _ref.z, + rotation = _ref.rotation, + rotationY = _ref.rotationY, + rotationX = _ref.rotationX, + skewX = _ref.skewX, + skewY = _ref.skewY, + scaleX = _ref.scaleX, + scaleY = _ref.scaleY, + transformPerspective = _ref.transformPerspective, + force3D = _ref.force3D, + target = _ref.target, + zOrigin = _ref.zOrigin, + transforms = "", + use3D = force3D === "auto" && ratio && ratio !== 1 || force3D === true; + + if (zOrigin && (rotationX !== _zeroDeg || rotationY !== _zeroDeg)) { + var angle = parseFloat(rotationY) * _DEG2RAD, + a13 = Math.sin(angle), + a33 = Math.cos(angle), + cos; + + angle = parseFloat(rotationX) * _DEG2RAD; + cos = Math.cos(angle); + x = _addPxTranslate(target, x, a13 * cos * -zOrigin); + y = _addPxTranslate(target, y, -Math.sin(angle) * -zOrigin); + z = _addPxTranslate(target, z, a33 * cos * -zOrigin + zOrigin); + } + + if (transformPerspective !== _zeroPx) { + transforms += "perspective(" + transformPerspective + _endParenthesis; + } + + if (xPercent || yPercent) { + transforms += "translate(" + xPercent + "%, " + yPercent + "%) "; + } + + if (use3D || x !== _zeroPx || y !== _zeroPx || z !== _zeroPx) { + transforms += z !== _zeroPx || use3D ? "translate3d(" + x + ", " + y + ", " + z + ") " : "translate(" + x + ", " + y + _endParenthesis; + } + + if (rotation !== _zeroDeg) { + transforms += "rotate(" + rotation + _endParenthesis; + } + + if (rotationY !== _zeroDeg) { + transforms += "rotateY(" + rotationY + _endParenthesis; + } + + if (rotationX !== _zeroDeg) { + transforms += "rotateX(" + rotationX + _endParenthesis; + } + + if (skewX !== _zeroDeg || skewY !== _zeroDeg) { + transforms += "skew(" + skewX + ", " + skewY + _endParenthesis; + } + + if (scaleX !== 1 || scaleY !== 1) { + transforms += "scale(" + scaleX + ", " + scaleY + _endParenthesis; + } + + target.style[_transformProp] = transforms || "translate(0, 0)"; + }, + _renderSVGTransforms = function _renderSVGTransforms(ratio, cache) { + var _ref2 = cache || this, + xPercent = _ref2.xPercent, + yPercent = _ref2.yPercent, + x = _ref2.x, + y = _ref2.y, + rotation = _ref2.rotation, + skewX = _ref2.skewX, + skewY = _ref2.skewY, + scaleX = _ref2.scaleX, + scaleY = _ref2.scaleY, + target = _ref2.target, + xOrigin = _ref2.xOrigin, + yOrigin = _ref2.yOrigin, + xOffset = _ref2.xOffset, + yOffset = _ref2.yOffset, + forceCSS = _ref2.forceCSS, + tx = parseFloat(x), + ty = parseFloat(y), + a11, + a21, + a12, + a22, + temp; + + rotation = parseFloat(rotation); + skewX = parseFloat(skewX); + skewY = parseFloat(skewY); + + if (skewY) { + skewY = parseFloat(skewY); + skewX += skewY; + rotation += skewY; + } + + if (rotation || skewX) { + rotation *= _DEG2RAD; + skewX *= _DEG2RAD; + a11 = Math.cos(rotation) * scaleX; + a21 = Math.sin(rotation) * scaleX; + a12 = Math.sin(rotation - skewX) * -scaleY; + a22 = Math.cos(rotation - skewX) * scaleY; + + if (skewX) { + skewY *= _DEG2RAD; + temp = Math.tan(skewX - skewY); + temp = Math.sqrt(1 + temp * temp); + a12 *= temp; + a22 *= temp; + + if (skewY) { + temp = Math.tan(skewY); + temp = Math.sqrt(1 + temp * temp); + a11 *= temp; + a21 *= temp; + } + } + + a11 = _round(a11); + a21 = _round(a21); + a12 = _round(a12); + a22 = _round(a22); + } else { + a11 = scaleX; + a22 = scaleY; + a21 = a12 = 0; + } + + if (tx && !~(x + "").indexOf("px") || ty && !~(y + "").indexOf("px")) { + tx = _convertToUnit(target, "x", x, "px"); + ty = _convertToUnit(target, "y", y, "px"); + } + + if (xOrigin || yOrigin || xOffset || yOffset) { + tx = _round(tx + xOrigin - (xOrigin * a11 + yOrigin * a12) + xOffset); + ty = _round(ty + yOrigin - (xOrigin * a21 + yOrigin * a22) + yOffset); + } + + if (xPercent || yPercent) { + temp = target.getBBox(); + tx = _round(tx + xPercent / 100 * temp.width); + ty = _round(ty + yPercent / 100 * temp.height); + } + + temp = "matrix(" + a11 + "," + a21 + "," + a12 + "," + a22 + "," + tx + "," + ty + ")"; + target.setAttribute("transform", temp); + forceCSS && (target.style[_transformProp] = temp); + }, + _addRotationalPropTween = function _addRotationalPropTween(plugin, target, property, startNum, endValue) { + var cap = 360, + isString = _isString(endValue), + endNum = parseFloat(endValue) * (isString && ~endValue.indexOf("rad") ? _RAD2DEG : 1), + change = endNum - startNum, + finalValue = startNum + change + "deg", + direction, + pt; + + if (isString) { + direction = endValue.split("_")[1]; + + if (direction === "short") { + change %= cap; + + if (change !== change % (cap / 2)) { + change += change < 0 ? cap : -cap; + } + } + + if (direction === "cw" && change < 0) { + change = (change + cap * _bigNum$1) % cap - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = (change - cap * _bigNum$1) % cap - ~~(change / cap) * cap; + } + } + + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + pt.u = "deg"; + + plugin._props.push(property); + + return pt; + }, + _assign = function _assign(target, source) { + for (var p in source) { + target[p] = source[p]; + } + + return target; + }, + _addRawTransformPTs = function _addRawTransformPTs(plugin, transforms, target) { + var startCache = _assign({}, target._gsap), + exclude = "perspective,force3D,transformOrigin,svgOrigin", + style = target.style, + endCache, + p, + startValue, + endValue, + startNum, + endNum, + startUnit, + endUnit; + + if (startCache.svg) { + startValue = target.getAttribute("transform"); + target.setAttribute("transform", ""); + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + + _removeProperty(target, _transformProp); + + target.setAttribute("transform", startValue); + } else { + startValue = getComputedStyle(target)[_transformProp]; + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + style[_transformProp] = startValue; + } + + for (p in _transformProps) { + startValue = startCache[p]; + endValue = endCache[p]; + + if (startValue !== endValue && exclude.indexOf(p) < 0) { + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + startNum = startUnit !== endUnit ? _convertToUnit(target, p, startValue, endUnit) : parseFloat(startValue); + endNum = parseFloat(endValue); + plugin._pt = new PropTween(plugin._pt, endCache, p, startNum, endNum - startNum, _renderCSSProp); + plugin._pt.u = endUnit || 0; + + plugin._props.push(p); + } + } + + _assign(endCache, startCache); + }; + + _forEachName("padding,margin,Width,Radius", function (name, index) { + var t = "Top", + r = "Right", + b = "Bottom", + l = "Left", + props = (index < 3 ? [t, r, b, l] : [t + l, t + r, b + r, b + l]).map(function (side) { + return index < 2 ? name + side : "border" + side + name; + }); + + _specialProps[index > 1 ? "border" + name : name] = function (plugin, target, property, endValue, tween) { + var a, vars; + + if (arguments.length < 4) { + a = props.map(function (prop) { + return _get(plugin, prop, property); + }); + vars = a.join(" "); + return vars.split(a[0]).length === 5 ? a[0] : vars; + } + + a = (endValue + "").split(" "); + vars = {}; + props.forEach(function (prop, i) { + return vars[prop] = a[i] = a[i] || a[(i - 1) / 2 | 0]; + }); + plugin.init(target, vars, tween); + }; + }); + + var CSSPlugin = { + name: "css", + register: _initCore, + targetTest: function targetTest(target) { + return target.style && target.nodeType; + }, + init: function init(target, vars, tween, index, targets) { + var props = this._props, + style = target.style, + startAt = tween.vars.startAt, + startValue, + endValue, + endNum, + startNum, + type, + specialProp, + p, + startUnit, + endUnit, + relative, + isTransformRelated, + transformPropTween, + cache, + smooth, + hasPriority, + inlineProps; + _pluginInitted || _initCore(); + this.styles = this.styles || _getStyleSaver(target); + inlineProps = this.styles.props; + this.tween = tween; + + for (p in vars) { + if (p === "autoRound") { + continue; + } + + endValue = vars[p]; + + if (_plugins[p] && _checkPlugin(p, vars, tween, index, target, targets)) { + continue; + } + + type = typeof endValue; + specialProp = _specialProps[p]; + + if (type === "function") { + endValue = endValue.call(tween, index, target, targets); + type = typeof endValue; + } + + if (type === "string" && ~endValue.indexOf("random(")) { + endValue = _replaceRandom(endValue); + } + + if (specialProp) { + specialProp(this, target, p, endValue, tween) && (hasPriority = 1); + } else if (p.substr(0, 2) === "--") { + startValue = (getComputedStyle(target).getPropertyValue(p) + "").trim(); + endValue += ""; + _colorExp.lastIndex = 0; + + if (!_colorExp.test(startValue)) { + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + } + + endUnit ? startUnit !== endUnit && (startValue = _convertToUnit(target, p, startValue, endUnit) + endUnit) : startUnit && (endValue += startUnit); + this.add(style, "setProperty", startValue, endValue, index, targets, 0, 0, p); + props.push(p); + inlineProps.push(p, 0, style[p]); + } else if (type !== "undefined") { + if (startAt && p in startAt) { + startValue = typeof startAt[p] === "function" ? startAt[p].call(tween, index, target, targets) : startAt[p]; + _isString(startValue) && ~startValue.indexOf("random(") && (startValue = _replaceRandom(startValue)); + getUnit(startValue + "") || startValue === "auto" || (startValue += _config.units[p] || getUnit(_get(target, p)) || ""); + (startValue + "").charAt(1) === "=" && (startValue = _get(target, p)); + } else { + startValue = _get(target, p); + } + + startNum = parseFloat(startValue); + relative = type === "string" && endValue.charAt(1) === "=" && endValue.substr(0, 2); + relative && (endValue = endValue.substr(2)); + endNum = parseFloat(endValue); + + if (p in _propertyAliases) { + if (p === "autoAlpha") { + if (startNum === 1 && _get(target, "visibility") === "hidden" && endNum) { + startNum = 0; + } + + inlineProps.push("visibility", 0, style.visibility); + + _addNonTweeningPT(this, style, "visibility", startNum ? "inherit" : "hidden", endNum ? "inherit" : "hidden", !endNum); + } + + if (p !== "scale" && p !== "transform") { + p = _propertyAliases[p]; + ~p.indexOf(",") && (p = p.split(",")[0]); + } + } + + isTransformRelated = p in _transformProps; + + if (isTransformRelated) { + this.styles.save(p); + + if (!transformPropTween) { + cache = target._gsap; + cache.renderTransform && !vars.parseTransform || _parseTransform(target, vars.parseTransform); + smooth = vars.smoothOrigin !== false && cache.smooth; + transformPropTween = this._pt = new PropTween(this._pt, style, _transformProp, 0, 1, cache.renderTransform, cache, 0, -1); + transformPropTween.dep = 1; + } + + if (p === "scale") { + this._pt = new PropTween(this._pt, cache, "scaleY", cache.scaleY, (relative ? _parseRelative(cache.scaleY, relative + endNum) : endNum) - cache.scaleY || 0, _renderCSSProp); + this._pt.u = 0; + props.push("scaleY", p); + p += "X"; + } else if (p === "transformOrigin") { + inlineProps.push(_transformOriginProp, 0, style[_transformOriginProp]); + endValue = _convertKeywordsToPercentages(endValue); + + if (cache.svg) { + _applySVGOrigin(target, endValue, 0, smooth, 0, this); + } else { + endUnit = parseFloat(endValue.split(" ")[2]) || 0; + endUnit !== cache.zOrigin && _addNonTweeningPT(this, cache, "zOrigin", cache.zOrigin, endUnit); + + _addNonTweeningPT(this, style, p, _firstTwoOnly(startValue), _firstTwoOnly(endValue)); + } + + continue; + } else if (p === "svgOrigin") { + _applySVGOrigin(target, endValue, 1, smooth, 0, this); + + continue; + } else if (p in _rotationalProperties) { + _addRotationalPropTween(this, cache, p, startNum, relative ? _parseRelative(startNum, relative + endValue) : endValue); + + continue; + } else if (p === "smoothOrigin") { + _addNonTweeningPT(this, cache, "smooth", cache.smooth, endValue); + + continue; + } else if (p === "force3D") { + cache[p] = endValue; + continue; + } else if (p === "transform") { + _addRawTransformPTs(this, endValue, target); + + continue; + } + } else if (!(p in style)) { + p = _checkPropPrefix(p) || p; + } + + if (isTransformRelated || (endNum || endNum === 0) && (startNum || startNum === 0) && !_complexExp.test(endValue) && p in style) { + startUnit = (startValue + "").substr((startNum + "").length); + endNum || (endNum = 0); + endUnit = getUnit(endValue) || (p in _config.units ? _config.units[p] : startUnit); + startUnit !== endUnit && (startNum = _convertToUnit(target, p, startValue, endUnit)); + this._pt = new PropTween(this._pt, isTransformRelated ? cache : style, p, startNum, (relative ? _parseRelative(startNum, relative + endNum) : endNum) - startNum, !isTransformRelated && (endUnit === "px" || p === "zIndex") && vars.autoRound !== false ? _renderRoundedCSSProp : _renderCSSProp); + this._pt.u = endUnit || 0; + + if (startUnit !== endUnit && endUnit !== "%") { + this._pt.b = startValue; + this._pt.r = _renderCSSPropWithBeginning; + } + } else if (!(p in style)) { + if (p in target) { + this.add(target, p, startValue || target[p], relative ? relative + endValue : endValue, index, targets); + } else if (p !== "parseTransform") { + _missingPlugin(p, endValue); + + continue; + } + } else { + _tweenComplexCSSString.call(this, target, p, startValue, relative ? relative + endValue : endValue); + } + + isTransformRelated || (p in style ? inlineProps.push(p, 0, style[p]) : typeof target[p] === "function" ? inlineProps.push(p, 2, target[p]()) : inlineProps.push(p, 1, startValue || target[p])); + props.push(p); + } + } + + hasPriority && _sortPropTweensByPriority(this); + }, + render: function render(ratio, data) { + if (data.tween._time || !_reverting$1()) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + } else { + data.styles.revert(); + } + }, + get: _get, + aliases: _propertyAliases, + getSetter: function getSetter(target, property, plugin) { + var p = _propertyAliases[property]; + p && p.indexOf(",") < 0 && (property = p); + return property in _transformProps && property !== _transformOriginProp && (target._gsap.x || _get(target, "x")) ? plugin && _recentSetterPlugin === plugin ? property === "scale" ? _setterScale : _setterTransform : (_recentSetterPlugin = plugin || {}) && (property === "scale" ? _setterScaleWithRender : _setterTransformWithRender) : target.style && !_isUndefined(target.style[property]) ? _setterCSSStyle : ~property.indexOf("-") ? _setterCSSProp : _getSetter(target, property); + }, + core: { + _removeProperty: _removeProperty, + _getMatrix: _getMatrix + } + }; + gsap.utils.checkPrefix = _checkPropPrefix; + gsap.core.getStyleSaver = _getStyleSaver; + + (function (positionAndScale, rotation, others, aliases) { + var all = _forEachName(positionAndScale + "," + rotation + "," + others, function (name) { + _transformProps[name] = 1; + }); + + _forEachName(rotation, function (name) { + _config.units[name] = "deg"; + _rotationalProperties[name] = 1; + }); + + _propertyAliases[all[13]] = positionAndScale + "," + rotation; + + _forEachName(aliases, function (name) { + var split = name.split(":"); + _propertyAliases[split[1]] = all[split[0]]; + }); + })("x,y,z,scale,scaleX,scaleY,xPercent,yPercent", "rotation,rotationX,rotationY,skewX,skewY", "transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective", "0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY"); + + _forEachName("x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective", function (name) { + _config.units[name] = "px"; + }); + + gsap.registerPlugin(CSSPlugin); + + var _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _numbersExp = /(?:(-)?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + _selectorExp = /(^[#\.][a-z]|[a-y][a-z])/i, + _DEG2RAD$1 = Math.PI / 180, + _RAD2DEG$1 = 180 / Math.PI, + _sin$1 = Math.sin, + _cos$1 = Math.cos, + _abs = Math.abs, + _sqrt$1 = Math.sqrt, + _atan2$1 = Math.atan2, + _largeNum = 1e8, + _isString$1 = function _isString(value) { + return typeof value === "string"; + }, + _isNumber$1 = function _isNumber(value) { + return typeof value === "number"; + }, + _isUndefined$1 = function _isUndefined(value) { + return typeof value === "undefined"; + }, + _temp = {}, + _temp2 = {}, + _roundingNum = 1e5, + _wrapProgress = function _wrapProgress(progress) { + return Math.round((progress + _largeNum) % 1 * _roundingNum) / _roundingNum || (progress < 0 ? 0 : 1); + }, + _round$1 = function _round(value) { + return Math.round(value * _roundingNum) / _roundingNum || 0; + }, + _roundPrecise$1 = function _roundPrecise(value) { + return Math.round(value * 1e10) / 1e10 || 0; + }, + _splitSegment = function _splitSegment(rawPath, segIndex, i, t) { + var segment = rawPath[segIndex], + shift = t === 1 ? 6 : subdivideSegment(segment, i, t); + + if ((shift || !t) && shift + i + 2 < segment.length) { + rawPath.splice(segIndex, 0, segment.slice(0, i + shift + 2)); + segment.splice(0, i + shift); + return 1; + } + }, + _getSampleIndex = function _getSampleIndex(samples, length, progress) { + var l = samples.length, + i = ~~(progress * l); + + if (samples[i] > length) { + while (--i && samples[i] > length) {} + + i < 0 && (i = 0); + } else { + while (samples[++i] < length && i < l) {} + } + + return i < l ? i : l - 1; + }, + _reverseRawPath = function _reverseRawPath(rawPath, skipOuter) { + var i = rawPath.length; + skipOuter || rawPath.reverse(); + + while (i--) { + rawPath[i].reversed || reverseSegment(rawPath[i]); + } + }, + _copyMetaData = function _copyMetaData(source, copy) { + copy.totalLength = source.totalLength; + + if (source.samples) { + copy.samples = source.samples.slice(0); + copy.lookup = source.lookup.slice(0); + copy.minLength = source.minLength; + copy.resolution = source.resolution; + } else if (source.totalPoints) { + copy.totalPoints = source.totalPoints; + } + + return copy; + }, + _appendOrMerge = function _appendOrMerge(rawPath, segment) { + var index = rawPath.length, + prevSeg = rawPath[index - 1] || [], + l = prevSeg.length; + + if (index && segment[0] === prevSeg[l - 2] && segment[1] === prevSeg[l - 1]) { + segment = prevSeg.concat(segment.slice(2)); + index--; + } + + rawPath[index] = segment; + }; + + function getRawPath(value) { + value = _isString$1(value) && _selectorExp.test(value) ? document.querySelector(value) || value : value; + var e = value.getAttribute ? value : 0, + rawPath; + + if (e && (value = value.getAttribute("d"))) { + if (!e._gsPath) { + e._gsPath = {}; + } + + rawPath = e._gsPath[value]; + return rawPath && !rawPath._dirty ? rawPath : e._gsPath[value] = stringToRawPath(value); + } + + return !value ? console.warn("Expecting a <path> element or an SVG path data string") : _isString$1(value) ? stringToRawPath(value) : _isNumber$1(value[0]) ? [value] : value; + } + function copyRawPath(rawPath) { + var a = [], + i = 0; + + for (; i < rawPath.length; i++) { + a[i] = _copyMetaData(rawPath[i], rawPath[i].slice(0)); + } + + return _copyMetaData(rawPath, a); + } + function reverseSegment(segment) { + var i = 0, + y; + segment.reverse(); + + for (; i < segment.length; i += 2) { + y = segment[i]; + segment[i] = segment[i + 1]; + segment[i + 1] = y; + } + + segment.reversed = !segment.reversed; + } + + var _createPath = function _createPath(e, ignore) { + var path = document.createElementNS("http://www.w3.org/2000/svg", "path"), + attr = [].slice.call(e.attributes), + i = attr.length, + name; + ignore = "," + ignore + ","; + + while (--i > -1) { + name = attr[i].nodeName.toLowerCase(); + + if (ignore.indexOf("," + name + ",") < 0) { + path.setAttributeNS(null, name, attr[i].nodeValue); + } + } + + return path; + }, + _typeAttrs = { + rect: "rx,ry,x,y,width,height", + circle: "r,cx,cy", + ellipse: "rx,ry,cx,cy", + line: "x1,x2,y1,y2" + }, + _attrToObj = function _attrToObj(e, attrs) { + var props = attrs ? attrs.split(",") : [], + obj = {}, + i = props.length; + + while (--i > -1) { + obj[props[i]] = +e.getAttribute(props[i]) || 0; + } + + return obj; + }; + + function convertToPath(element, swap) { + var type = element.tagName.toLowerCase(), + circ = 0.552284749831, + data, + x, + y, + r, + ry, + path, + rcirc, + rycirc, + points, + w, + h, + x2, + x3, + x4, + x5, + x6, + y2, + y3, + y4, + y5, + y6, + attr; + + if (type === "path" || !element.getBBox) { + return element; + } + + path = _createPath(element, "x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points"); + attr = _attrToObj(element, _typeAttrs[type]); + + if (type === "rect") { + r = attr.rx; + ry = attr.ry || r; + x = attr.x; + y = attr.y; + w = attr.width - r * 2; + h = attr.height - ry * 2; + + if (r || ry) { + x2 = x + r * (1 - circ); + x3 = x + r; + x4 = x3 + w; + x5 = x4 + r * circ; + x6 = x4 + r; + y2 = y + ry * (1 - circ); + y3 = y + ry; + y4 = y3 + h; + y5 = y4 + ry * circ; + y6 = y4 + ry; + data = "M" + x6 + "," + y3 + " V" + y4 + " C" + [x6, y5, x5, y6, x4, y6, x4 - (x4 - x3) / 3, y6, x3 + (x4 - x3) / 3, y6, x3, y6, x2, y6, x, y5, x, y4, x, y4 - (y4 - y3) / 3, x, y3 + (y4 - y3) / 3, x, y3, x, y2, x2, y, x3, y, x3 + (x4 - x3) / 3, y, x4 - (x4 - x3) / 3, y, x4, y, x5, y, x6, y2, x6, y3].join(",") + "z"; + } else { + data = "M" + (x + w) + "," + y + " v" + h + " h" + -w + " v" + -h + " h" + w + "z"; + } + } else if (type === "circle" || type === "ellipse") { + if (type === "circle") { + r = ry = attr.r; + rycirc = r * circ; + } else { + r = attr.rx; + ry = attr.ry; + rycirc = ry * circ; + } + + x = attr.cx; + y = attr.cy; + rcirc = r * circ; + data = "M" + (x + r) + "," + y + " C" + [x + r, y + rycirc, x + rcirc, y + ry, x, y + ry, x - rcirc, y + ry, x - r, y + rycirc, x - r, y, x - r, y - rycirc, x - rcirc, y - ry, x, y - ry, x + rcirc, y - ry, x + r, y - rycirc, x + r, y].join(",") + "z"; + } else if (type === "line") { + data = "M" + attr.x1 + "," + attr.y1 + " L" + attr.x2 + "," + attr.y2; + } else if (type === "polyline" || type === "polygon") { + points = (element.getAttribute("points") + "").match(_numbersExp) || []; + x = points.shift(); + y = points.shift(); + data = "M" + x + "," + y + " L" + points.join(","); + + if (type === "polygon") { + data += "," + x + "," + y + "z"; + } + } + + path.setAttribute("d", rawPathToString(path._gsRawPath = stringToRawPath(data))); + + if (swap && element.parentNode) { + element.parentNode.insertBefore(path, element); + element.parentNode.removeChild(element); + } + + return path; + } + + function getRotationAtBezierT(segment, i, t) { + var a = segment[i], + b = segment[i + 2], + c = segment[i + 4], + x; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + x = b + (c + (segment[i + 6] - c) * t - b) * t - a; + a = segment[i + 1]; + b = segment[i + 3]; + c = segment[i + 5]; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + return _round$1(_atan2$1(b + (c + (segment[i + 7] - c) * t - b) * t - a, x) * _RAD2DEG$1); + } + + function sliceRawPath(rawPath, start, end) { + end = _isUndefined$1(end) ? 1 : _roundPrecise$1(end) || 0; + start = _roundPrecise$1(start) || 0; + var loops = Math.max(0, ~~(_abs(end - start) - 1e-8)), + path = copyRawPath(rawPath); + + if (start > end) { + start = 1 - start; + end = 1 - end; + + _reverseRawPath(path); + + path.totalLength = 0; + } + + if (start < 0 || end < 0) { + var offset = Math.abs(~~Math.min(start, end)) + 1; + start += offset; + end += offset; + } + + path.totalLength || cacheRawPathMeasurements(path); + var wrap = end > 1, + s = getProgressData(path, start, _temp, true), + e = getProgressData(path, end, _temp2), + eSeg = e.segment, + sSeg = s.segment, + eSegIndex = e.segIndex, + sSegIndex = s.segIndex, + ei = e.i, + si = s.i, + sameSegment = sSegIndex === eSegIndex, + sameBezier = ei === si && sameSegment, + wrapsBehind, + sShift, + eShift, + i, + copy, + totalSegments, + l, + j; + + if (wrap || loops) { + wrapsBehind = eSegIndex < sSegIndex || sameSegment && ei < si || sameBezier && e.t < s.t; + + if (_splitSegment(path, sSegIndex, si, s.t)) { + sSegIndex++; + + if (!wrapsBehind) { + eSegIndex++; + + if (sameBezier) { + e.t = (e.t - s.t) / (1 - s.t); + ei = 0; + } else if (sameSegment) { + ei -= si; + } + } + } + + if (Math.abs(1 - (end - start)) < 1e-5) { + eSegIndex = sSegIndex - 1; + } else if (!e.t && eSegIndex) { + eSegIndex--; + } else if (_splitSegment(path, eSegIndex, ei, e.t) && wrapsBehind) { + sSegIndex++; + } + + if (s.t === 1) { + sSegIndex = (sSegIndex + 1) % path.length; + } + + copy = []; + totalSegments = path.length; + l = 1 + totalSegments * loops; + j = sSegIndex; + l += (totalSegments - sSegIndex + eSegIndex) % totalSegments; + + for (i = 0; i < l; i++) { + _appendOrMerge(copy, path[j++ % totalSegments]); + } + + path = copy; + } else { + eShift = e.t === 1 ? 6 : subdivideSegment(eSeg, ei, e.t); + + if (start !== end) { + sShift = subdivideSegment(sSeg, si, sameBezier ? s.t / e.t : s.t); + sameSegment && (eShift += sShift); + eSeg.splice(ei + eShift + 2); + (sShift || si) && sSeg.splice(0, si + sShift); + i = path.length; + + while (i--) { + (i < sSegIndex || i > eSegIndex) && path.splice(i, 1); + } + } else { + eSeg.angle = getRotationAtBezierT(eSeg, ei + eShift, 0); + ei += eShift; + s = eSeg[ei]; + e = eSeg[ei + 1]; + eSeg.length = eSeg.totalLength = 0; + eSeg.totalPoints = path.totalPoints = 8; + eSeg.push(s, e, s, e, s, e, s, e); + } + } + + path.totalLength = 0; + return path; + } + + function measureSegment(segment, startIndex, bezierQty) { + startIndex = startIndex || 0; + + if (!segment.samples) { + segment.samples = []; + segment.lookup = []; + } + + var resolution = ~~segment.resolution || 12, + inc = 1 / resolution, + endIndex = bezierQty ? startIndex + bezierQty * 6 + 1 : segment.length, + x1 = segment[startIndex], + y1 = segment[startIndex + 1], + samplesIndex = startIndex ? startIndex / 6 * resolution : 0, + samples = segment.samples, + lookup = segment.lookup, + min = (startIndex ? segment.minLength : _largeNum) || _largeNum, + prevLength = samples[samplesIndex + bezierQty * resolution - 1], + length = startIndex ? samples[samplesIndex - 1] : 0, + i, + j, + x4, + x3, + x2, + xd, + xd1, + y4, + y3, + y2, + yd, + yd1, + inv, + t, + lengthIndex, + l, + segLength; + samples.length = lookup.length = 0; + + for (j = startIndex + 2; j < endIndex; j += 6) { + x4 = segment[j + 4] - x1; + x3 = segment[j + 2] - x1; + x2 = segment[j] - x1; + y4 = segment[j + 5] - y1; + y3 = segment[j + 3] - y1; + y2 = segment[j + 1] - y1; + xd = xd1 = yd = yd1 = 0; + + if (_abs(x4) < .01 && _abs(y4) < .01 && _abs(x2) + _abs(y2) < .01) { + if (segment.length > 8) { + segment.splice(j, 6); + j -= 6; + endIndex -= 6; + } + } else { + for (i = 1; i <= resolution; i++) { + t = inc * i; + inv = 1 - t; + xd = xd1 - (xd1 = (t * t * x4 + 3 * inv * (t * x3 + inv * x2)) * t); + yd = yd1 - (yd1 = (t * t * y4 + 3 * inv * (t * y3 + inv * y2)) * t); + l = _sqrt$1(yd * yd + xd * xd); + + if (l < min) { + min = l; + } + + length += l; + samples[samplesIndex++] = length; + } + } + + x1 += x4; + y1 += y4; + } + + if (prevLength) { + prevLength -= length; + + for (; samplesIndex < samples.length; samplesIndex++) { + samples[samplesIndex] += prevLength; + } + } + + if (samples.length && min) { + segment.totalLength = segLength = samples[samples.length - 1] || 0; + segment.minLength = min; + + if (segLength / min < 9999) { + l = lengthIndex = 0; + + for (i = 0; i < segLength; i += min) { + lookup[l++] = samples[lengthIndex] < i ? ++lengthIndex : lengthIndex; + } + } + } else { + segment.totalLength = samples[0] = 0; + } + + return startIndex ? length - samples[startIndex / 2 - 1] : length; + } + + function cacheRawPathMeasurements(rawPath, resolution) { + var pathLength, points, i; + + for (i = pathLength = points = 0; i < rawPath.length; i++) { + rawPath[i].resolution = ~~resolution || 12; + points += rawPath[i].length; + pathLength += measureSegment(rawPath[i]); + } + + rawPath.totalPoints = points; + rawPath.totalLength = pathLength; + return rawPath; + } + function subdivideSegment(segment, i, t) { + if (t <= 0 || t >= 1) { + return 0; + } + + var ax = segment[i], + ay = segment[i + 1], + cp1x = segment[i + 2], + cp1y = segment[i + 3], + cp2x = segment[i + 4], + cp2y = segment[i + 5], + bx = segment[i + 6], + by = segment[i + 7], + x1a = ax + (cp1x - ax) * t, + x2 = cp1x + (cp2x - cp1x) * t, + y1a = ay + (cp1y - ay) * t, + y2 = cp1y + (cp2y - cp1y) * t, + x1 = x1a + (x2 - x1a) * t, + y1 = y1a + (y2 - y1a) * t, + x2a = cp2x + (bx - cp2x) * t, + y2a = cp2y + (by - cp2y) * t; + x2 += (x2a - x2) * t; + y2 += (y2a - y2) * t; + segment.splice(i + 2, 4, _round$1(x1a), _round$1(y1a), _round$1(x1), _round$1(y1), _round$1(x1 + (x2 - x1) * t), _round$1(y1 + (y2 - y1) * t), _round$1(x2), _round$1(y2), _round$1(x2a), _round$1(y2a)); + segment.samples && segment.samples.splice(i / 6 * segment.resolution | 0, 0, 0, 0, 0, 0, 0, 0); + return 6; + } + + function getProgressData(rawPath, progress, decoratee, pushToNextIfAtEnd) { + decoratee = decoratee || {}; + rawPath.totalLength || cacheRawPathMeasurements(rawPath); + + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + + var segIndex = 0, + segment = rawPath[0], + samples, + resolution, + length, + min, + max, + i, + t; + + if (!progress) { + t = i = segIndex = 0; + segment = rawPath[0]; + } else if (progress === 1) { + t = 1; + segIndex = rawPath.length - 1; + segment = rawPath[segIndex]; + i = segment.length - 8; + } else { + if (rawPath.length > 1) { + length = rawPath.totalLength * progress; + max = i = 0; + + while ((max += rawPath[i++].totalLength) < length) { + segIndex = i; + } + + segment = rawPath[segIndex]; + min = max - segment.totalLength; + progress = (length - min) / (max - min) || 0; + } + + samples = segment.samples; + resolution = segment.resolution; + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[~~(length / segment.minLength)] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i - 1] : 0; + max = samples[i]; + + if (max < length) { + min = max; + max = samples[++i]; + } + + t = 1 / resolution * ((length - min) / (max - min) + i % resolution); + i = ~~(i / resolution) * 6; + + if (pushToNextIfAtEnd && t === 1) { + if (i + 6 < segment.length) { + i += 6; + t = 0; + } else if (segIndex + 1 < rawPath.length) { + i = t = 0; + segment = rawPath[++segIndex]; + } + } + } + + decoratee.t = t; + decoratee.i = i; + decoratee.path = rawPath; + decoratee.segment = segment; + decoratee.segIndex = segIndex; + return decoratee; + } + + function getPositionOnPath(rawPath, progress, includeAngle, point) { + var segment = rawPath[0], + result = point || {}, + samples, + resolution, + length, + min, + max, + i, + t, + a, + inv; + + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + + segment.lookup || cacheRawPathMeasurements(rawPath); + + if (rawPath.length > 1) { + length = rawPath.totalLength * progress; + max = i = 0; + + while ((max += rawPath[i++].totalLength) < length) { + segment = rawPath[i]; + } + + min = max - segment.totalLength; + progress = (length - min) / (max - min) || 0; + } + + samples = segment.samples; + resolution = segment.resolution; + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i - 1] : 0; + max = samples[i]; + + if (max < length) { + min = max; + max = samples[++i]; + } + + t = 1 / resolution * ((length - min) / (max - min) + i % resolution) || 0; + inv = 1 - t; + i = ~~(i / resolution) * 6; + a = segment[i]; + result.x = _round$1((t * t * (segment[i + 6] - a) + 3 * inv * (t * (segment[i + 4] - a) + inv * (segment[i + 2] - a))) * t + a); + result.y = _round$1((t * t * (segment[i + 7] - (a = segment[i + 1])) + 3 * inv * (t * (segment[i + 5] - a) + inv * (segment[i + 3] - a))) * t + a); + + if (includeAngle) { + result.angle = segment.totalLength ? getRotationAtBezierT(segment, i, t >= 1 ? 1 - 1e-9 : t ? t : 1e-9) : segment.angle || 0; + } + + return result; + } + function transformRawPath(rawPath, a, b, c, d, tx, ty) { + var j = rawPath.length, + segment, + l, + i, + x, + y; + + while (--j > -1) { + segment = rawPath[j]; + l = segment.length; + + for (i = 0; i < l; i += 2) { + x = segment[i]; + y = segment[i + 1]; + segment[i] = x * a + y * c + tx; + segment[i + 1] = x * b + y * d + ty; + } + } + + rawPath._dirty = 1; + return rawPath; + } + + function arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) { + if (lastX === x && lastY === y) { + return; + } + + rx = _abs(rx); + ry = _abs(ry); + + var angleRad = angle % 360 * _DEG2RAD$1, + cosAngle = _cos$1(angleRad), + sinAngle = _sin$1(angleRad), + PI = Math.PI, + TWOPI = PI * 2, + dx2 = (lastX - x) / 2, + dy2 = (lastY - y) / 2, + x1 = cosAngle * dx2 + sinAngle * dy2, + y1 = -sinAngle * dx2 + cosAngle * dy2, + x1_sq = x1 * x1, + y1_sq = y1 * y1, + radiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry); + + if (radiiCheck > 1) { + rx = _sqrt$1(radiiCheck) * rx; + ry = _sqrt$1(radiiCheck) * ry; + } + + var rx_sq = rx * rx, + ry_sq = ry * ry, + sq = (rx_sq * ry_sq - rx_sq * y1_sq - ry_sq * x1_sq) / (rx_sq * y1_sq + ry_sq * x1_sq); + + if (sq < 0) { + sq = 0; + } + + var coef = (largeArcFlag === sweepFlag ? -1 : 1) * _sqrt$1(sq), + cx1 = coef * (rx * y1 / ry), + cy1 = coef * -(ry * x1 / rx), + sx2 = (lastX + x) / 2, + sy2 = (lastY + y) / 2, + cx = sx2 + (cosAngle * cx1 - sinAngle * cy1), + cy = sy2 + (sinAngle * cx1 + cosAngle * cy1), + ux = (x1 - cx1) / rx, + uy = (y1 - cy1) / ry, + vx = (-x1 - cx1) / rx, + vy = (-y1 - cy1) / ry, + temp = ux * ux + uy * uy, + angleStart = (uy < 0 ? -1 : 1) * Math.acos(ux / _sqrt$1(temp)), + angleExtent = (ux * vy - uy * vx < 0 ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt$1(temp * (vx * vx + vy * vy))); + + isNaN(angleExtent) && (angleExtent = PI); + + if (!sweepFlag && angleExtent > 0) { + angleExtent -= TWOPI; + } else if (sweepFlag && angleExtent < 0) { + angleExtent += TWOPI; + } + + angleStart %= TWOPI; + angleExtent %= TWOPI; + + var segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)), + rawPath = [], + angleIncrement = angleExtent / segments, + controlLength = 4 / 3 * _sin$1(angleIncrement / 2) / (1 + _cos$1(angleIncrement / 2)), + ma = cosAngle * rx, + mb = sinAngle * rx, + mc = sinAngle * -ry, + md = cosAngle * ry, + i; + + for (i = 0; i < segments; i++) { + angle = angleStart + i * angleIncrement; + x1 = _cos$1(angle); + y1 = _sin$1(angle); + ux = _cos$1(angle += angleIncrement); + uy = _sin$1(angle); + rawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy); + } + + for (i = 0; i < rawPath.length; i += 2) { + x1 = rawPath[i]; + y1 = rawPath[i + 1]; + rawPath[i] = x1 * ma + y1 * mc + cx; + rawPath[i + 1] = x1 * mb + y1 * md + cy; + } + + rawPath[i - 2] = x; + rawPath[i - 1] = y; + return rawPath; + } + + function stringToRawPath(d) { + var a = (d + "").replace(_scientific, function (m) { + var n = +m; + return n < 0.0001 && n > -0.0001 ? 0 : n; + }).match(_svgPathExp) || [], + path = [], + relativeX = 0, + relativeY = 0, + twoThirds = 2 / 3, + elements = a.length, + points = 0, + errorMessage = "ERROR: malformed path: " + d, + i, + j, + x, + y, + command, + isRelative, + segment, + startX, + startY, + difX, + difY, + beziers, + prevCommand, + flag1, + flag2, + line = function line(sx, sy, ex, ey) { + difX = (ex - sx) / 3; + difY = (ey - sy) / 3; + segment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey); + }; + + if (!d || !isNaN(a[0]) || isNaN(a[1])) { + console.log(errorMessage); + return path; + } + + for (i = 0; i < elements; i++) { + prevCommand = command; + + if (isNaN(a[i])) { + command = a[i].toUpperCase(); + isRelative = command !== a[i]; + } else { + i--; + } + + x = +a[i + 1]; + y = +a[i + 2]; + + if (isRelative) { + x += relativeX; + y += relativeY; + } + + if (!i) { + startX = x; + startY = y; + } + + if (command === "M") { + if (segment) { + if (segment.length < 8) { + path.length -= 1; + } else { + points += segment.length; + } + } + + relativeX = startX = x; + relativeY = startY = y; + segment = [x, y]; + path.push(segment); + i += 2; + command = "L"; + } else if (command === "C") { + if (!segment) { + segment = [0, 0]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(x, y, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, relativeX += a[i + 5] * 1, relativeY += a[i + 6] * 1); + i += 6; + } else if (command === "S") { + difX = relativeX; + difY = relativeY; + + if (prevCommand === "C" || prevCommand === "S") { + difX += relativeX - segment[segment.length - 4]; + difY += relativeY - segment[segment.length - 3]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(difX, difY, x, y, relativeX += a[i + 3] * 1, relativeY += a[i + 4] * 1); + i += 4; + } else if (command === "Q") { + difX = relativeX + (x - relativeX) * twoThirds; + difY = relativeY + (y - relativeY) * twoThirds; + + if (!isRelative) { + relativeX = relativeY = 0; + } + + relativeX += a[i + 3] * 1; + relativeY += a[i + 4] * 1; + segment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY); + i += 4; + } else if (command === "T") { + difX = relativeX - segment[segment.length - 4]; + difY = relativeY - segment[segment.length - 3]; + segment.push(relativeX + difX, relativeY + difY, x + (relativeX + difX * 1.5 - x) * twoThirds, y + (relativeY + difY * 1.5 - y) * twoThirds, relativeX = x, relativeY = y); + i += 2; + } else if (command === "H") { + line(relativeX, relativeY, relativeX = x, relativeY); + i += 1; + } else if (command === "V") { + line(relativeX, relativeY, relativeX, relativeY = x + (isRelative ? relativeY - relativeX : 0)); + i += 1; + } else if (command === "L" || command === "Z") { + if (command === "Z") { + x = startX; + y = startY; + segment.closed = true; + } + + if (command === "L" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) { + line(relativeX, relativeY, x, y); + + if (command === "L") { + i += 2; + } + } + + relativeX = x; + relativeY = y; + } else if (command === "A") { + flag1 = a[i + 4]; + flag2 = a[i + 5]; + difX = a[i + 6]; + difY = a[i + 7]; + j = 7; + + if (flag1.length > 1) { + if (flag1.length < 3) { + difY = difX; + difX = flag2; + j--; + } else { + difY = flag2; + difX = flag1.substr(2); + j -= 2; + } + + flag2 = flag1.charAt(1); + flag1 = flag1.charAt(0); + } + + beziers = arcToSegment(relativeX, relativeY, +a[i + 1], +a[i + 2], +a[i + 3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX * 1, (isRelative ? relativeY : 0) + difY * 1); + i += j; + + if (beziers) { + for (j = 0; j < beziers.length; j++) { + segment.push(beziers[j]); + } + } + + relativeX = segment[segment.length - 2]; + relativeY = segment[segment.length - 1]; + } else { + console.log(errorMessage); + } + } + + i = segment.length; + + if (i < 6) { + path.pop(); + i = 0; + } else if (segment[0] === segment[i - 2] && segment[1] === segment[i - 1]) { + segment.closed = true; + } + + path.totalPoints = points + i; + return path; + } + function flatPointsToSegment(points, curviness) { + if (curviness === void 0) { + curviness = 1; + } + + var x = points[0], + y = 0, + segment = [x, y], + i = 2; + + for (; i < points.length; i += 2) { + segment.push(x, y, points[i], y = (points[i] - x) * curviness / 2, x = points[i], -y); + } + + return segment; + } + function pointsToSegment(points, curviness) { + _abs(points[0] - points[2]) < 1e-4 && _abs(points[1] - points[3]) < 1e-4 && (points = points.slice(2)); + var l = points.length - 2, + x = +points[0], + y = +points[1], + nextX = +points[2], + nextY = +points[3], + segment = [x, y, x, y], + dx2 = nextX - x, + dy2 = nextY - y, + closed = Math.abs(points[l] - x) < 0.001 && Math.abs(points[l + 1] - y) < 0.001, + prevX, + prevY, + i, + dx1, + dy1, + r1, + r2, + r3, + tl, + mx1, + mx2, + mxm, + my1, + my2, + mym; + + if (closed) { + points.push(nextX, nextY); + nextX = x; + nextY = y; + x = points[l - 2]; + y = points[l - 1]; + points.unshift(x, y); + l += 4; + } + + curviness = curviness || curviness === 0 ? +curviness : 1; + + for (i = 2; i < l; i += 2) { + prevX = x; + prevY = y; + x = nextX; + y = nextY; + nextX = +points[i + 2]; + nextY = +points[i + 3]; + + if (x === nextX && y === nextY) { + continue; + } + + dx1 = dx2; + dy1 = dy2; + dx2 = nextX - x; + dy2 = nextY - y; + r1 = _sqrt$1(dx1 * dx1 + dy1 * dy1); + r2 = _sqrt$1(dx2 * dx2 + dy2 * dy2); + r3 = _sqrt$1(Math.pow(dx2 / r2 + dx1 / r1, 2) + Math.pow(dy2 / r2 + dy1 / r1, 2)); + tl = (r1 + r2) * curviness * 0.25 / r3; + mx1 = x - (x - prevX) * (r1 ? tl / r1 : 0); + mx2 = x + (nextX - x) * (r2 ? tl / r2 : 0); + mxm = x - (mx1 + ((mx2 - mx1) * (r1 * 3 / (r1 + r2) + 0.5) / 4 || 0)); + my1 = y - (y - prevY) * (r1 ? tl / r1 : 0); + my2 = y + (nextY - y) * (r2 ? tl / r2 : 0); + mym = y - (my1 + ((my2 - my1) * (r1 * 3 / (r1 + r2) + 0.5) / 4 || 0)); + + if (x !== prevX || y !== prevY) { + segment.push(_round$1(mx1 + mxm), _round$1(my1 + mym), _round$1(x), _round$1(y), _round$1(mx2 + mxm), _round$1(my2 + mym)); + } + } + + x !== nextX || y !== nextY || segment.length < 4 ? segment.push(_round$1(nextX), _round$1(nextY), _round$1(nextX), _round$1(nextY)) : segment.length -= 2; + + if (segment.length === 2) { + segment.push(x, y, x, y, x, y); + } else if (closed) { + segment.splice(0, 6); + segment.length = segment.length - 6; + } + + return segment; + } + function rawPathToString(rawPath) { + if (_isNumber$1(rawPath[0])) { + rawPath = [rawPath]; + } + + var result = "", + l = rawPath.length, + sl, + s, + i, + segment; + + for (s = 0; s < l; s++) { + segment = rawPath[s]; + result += "M" + _round$1(segment[0]) + "," + _round$1(segment[1]) + " C"; + sl = segment.length; + + for (i = 2; i < sl; i++) { + result += _round$1(segment[i++]) + "," + _round$1(segment[i++]) + " " + _round$1(segment[i++]) + "," + _round$1(segment[i++]) + " " + _round$1(segment[i++]) + "," + _round$1(segment[i]) + " "; + } + + if (segment.closed) { + result += "z"; + } + } + + return result; + } + + /*! + * CustomEase 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var gsap$1, + _coreInitted$1, + _getGSAP = function _getGSAP() { + return gsap$1 || typeof window !== "undefined" && (gsap$1 = window.gsap) && gsap$1.registerPlugin && gsap$1; + }, + _initCore$1 = function _initCore() { + gsap$1 = _getGSAP(); + + if (gsap$1) { + gsap$1.registerEase("_CE", CustomEase.create); + _coreInitted$1 = 1; + } else { + console.warn("Please gsap.registerPlugin(CustomEase)"); + } + }, + _bigNum$2 = 1e20, + _round$2 = function _round(value) { + return ~~(value * 1000 + (value < 0 ? -.5 : .5)) / 1000; + }, + _numExp$1 = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/gi, + _needsParsingExp = /[cLlsSaAhHvVtTqQ]/g, + _findMinimum = function _findMinimum(values) { + var l = values.length, + min = _bigNum$2, + i; + + for (i = 1; i < l; i += 6) { + +values[i] < min && (min = +values[i]); + } + + return min; + }, + _normalize = function _normalize(values, height, originY) { + if (!originY && originY !== 0) { + originY = Math.max(+values[values.length - 1], +values[1]); + } + + var tx = +values[0] * -1, + ty = -originY, + l = values.length, + sx = 1 / (+values[l - 2] + tx), + sy = -height || (Math.abs(+values[l - 1] - +values[1]) < 0.01 * (+values[l - 2] - +values[0]) ? _findMinimum(values) + ty : +values[l - 1] + ty), + i; + + if (sy) { + sy = 1 / sy; + } else { + sy = -sx; + } + + for (i = 0; i < l; i += 2) { + values[i] = (+values[i] + tx) * sx; + values[i + 1] = (+values[i + 1] + ty) * sy; + } + }, + _bezierToPoints = function _bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) { + var x12 = (x1 + x2) / 2, + y12 = (y1 + y2) / 2, + x23 = (x2 + x3) / 2, + y23 = (y2 + y3) / 2, + x34 = (x3 + x4) / 2, + y34 = (y3 + y4) / 2, + x123 = (x12 + x23) / 2, + y123 = (y12 + y23) / 2, + x234 = (x23 + x34) / 2, + y234 = (y23 + y34) / 2, + x1234 = (x123 + x234) / 2, + y1234 = (y123 + y234) / 2, + dx = x4 - x1, + dy = y4 - y1, + d2 = Math.abs((x2 - x4) * dy - (y2 - y4) * dx), + d3 = Math.abs((x3 - x4) * dy - (y3 - y4) * dx), + length; + + if (!points) { + points = [{ + x: x1, + y: y1 + }, { + x: x4, + y: y4 + }]; + index = 1; + } + + points.splice(index || points.length - 1, 0, { + x: x1234, + y: y1234 + }); + + if ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) { + length = points.length; + + _bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index); + + _bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 1 + (points.length - length)); + } + + return points; + }; + + var CustomEase = function () { + function CustomEase(id, data, config) { + _coreInitted$1 || _initCore$1(); + this.id = id; + this.setData(data, config); + } + + var _proto = CustomEase.prototype; + + _proto.setData = function setData(data, config) { + config = config || {}; + data = data || "0,0,1,1"; + var values = data.match(_numExp$1), + closest = 1, + points = [], + lookup = [], + precision = config.precision || 1, + fast = precision <= 1, + l, + a1, + a2, + i, + inc, + j, + point, + prevPoint, + p; + this.data = data; + + if (_needsParsingExp.test(data) || ~data.indexOf("M") && data.indexOf("C") < 0) { + values = stringToRawPath(data)[0]; + } + + l = values.length; + + if (l === 4) { + values.unshift(0, 0); + values.push(1, 1); + l = 8; + } else if ((l - 2) % 6) { + throw "Invalid CustomEase"; + } + + if (+values[0] !== 0 || +values[l - 2] !== 1) { + _normalize(values, config.height, config.originY); + } + + this.segment = values; + + for (i = 2; i < l; i += 6) { + a1 = { + x: +values[i - 2], + y: +values[i - 1] + }; + a2 = { + x: +values[i + 4], + y: +values[i + 5] + }; + points.push(a1, a2); + + _bezierToPoints(a1.x, a1.y, +values[i], +values[i + 1], +values[i + 2], +values[i + 3], a2.x, a2.y, 1 / (precision * 200000), points, points.length - 1); + } + + l = points.length; + + for (i = 0; i < l; i++) { + point = points[i]; + prevPoint = points[i - 1] || point; + + if ((point.x > prevPoint.x || prevPoint.y !== point.y && prevPoint.x === point.x || point === prevPoint) && point.x <= 1) { + prevPoint.cx = point.x - prevPoint.x; + prevPoint.cy = point.y - prevPoint.y; + prevPoint.n = point; + prevPoint.nx = point.x; + + if (fast && i > 1 && Math.abs(prevPoint.cy / prevPoint.cx - points[i - 2].cy / points[i - 2].cx) > 2) { + fast = 0; + } + + if (prevPoint.cx < closest) { + if (!prevPoint.cx) { + prevPoint.cx = 0.001; + + if (i === l - 1) { + prevPoint.x -= 0.001; + closest = Math.min(closest, 0.001); + fast = 0; + } + } else { + closest = prevPoint.cx; + } + } + } else { + points.splice(i--, 1); + l--; + } + } + + l = 1 / closest + 1 | 0; + inc = 1 / l; + j = 0; + point = points[0]; + + if (fast) { + for (i = 0; i < l; i++) { + p = i * inc; + + if (point.nx < p) { + point = points[++j]; + } + + a1 = point.y + (p - point.x) / point.cx * point.cy; + lookup[i] = { + x: p, + cx: inc, + y: a1, + cy: 0, + nx: 9 + }; + + if (i) { + lookup[i - 1].cy = a1 - lookup[i - 1].y; + } + } + + j = points[points.length - 1]; + lookup[l - 1].cy = j.y - a1; + lookup[l - 1].cx = j.x - lookup[lookup.length - 1].x; + } else { + for (i = 0; i < l; i++) { + if (point.nx < i * inc) { + point = points[++j]; + } + + lookup[i] = point; + } + + if (j < points.length - 1) { + lookup[i - 1] = points[points.length - 2]; + } + } + + this.ease = function (p) { + var point = lookup[p * l | 0] || lookup[l - 1]; + + if (point.nx < p) { + point = point.n; + } + + return point.y + (p - point.x) / point.cx * point.cy; + }; + + this.ease.custom = this; + this.id && gsap$1 && gsap$1.registerEase(this.id, this.ease); + return this; + }; + + _proto.getSVGData = function getSVGData(config) { + return CustomEase.getSVGData(this, config); + }; + + CustomEase.create = function create(id, data, config) { + return new CustomEase(id, data, config).ease; + }; + + CustomEase.register = function register(core) { + gsap$1 = core; + + _initCore$1(); + }; + + CustomEase.get = function get(id) { + return gsap$1.parseEase(id); + }; + + CustomEase.getSVGData = function getSVGData(ease, config) { + config = config || {}; + var width = config.width || 100, + height = config.height || 100, + x = config.x || 0, + y = (config.y || 0) + height, + e = gsap$1.utils.toArray(config.path)[0], + a, + slope, + i, + inc, + tx, + ty, + precision, + threshold, + prevX, + prevY; + + if (config.invert) { + height = -height; + y = 0; + } + + if (typeof ease === "string") { + ease = gsap$1.parseEase(ease); + } + + if (ease.custom) { + ease = ease.custom; + } + + if (ease instanceof CustomEase) { + a = rawPathToString(transformRawPath([ease.segment], width, 0, 0, -height, x, y)); + } else { + a = [x, y]; + precision = Math.max(5, (config.precision || 1) * 200); + inc = 1 / precision; + precision += 2; + threshold = 5 / precision; + prevX = _round$2(x + inc * width); + prevY = _round$2(y + ease(inc) * -height); + slope = (prevY - y) / (prevX - x); + + for (i = 2; i < precision; i++) { + tx = _round$2(x + i * inc * width); + ty = _round$2(y + ease(i * inc) * -height); + + if (Math.abs((ty - prevY) / (tx - prevX) - slope) > threshold || i === precision - 1) { + a.push(prevX, prevY); + slope = (ty - prevY) / (tx - prevX); + } + + prevX = tx; + prevY = ty; + } + + a = "M" + a.join(","); + } + + e && e.setAttribute("d", a); + return a; + }; + + return CustomEase; + }(); + CustomEase.version = "3.12.7"; + CustomEase.headless = true; + _getGSAP() && gsap$1.registerPlugin(CustomEase); + + var _doc$2, + _win$2, + _docElement$1, + _body, + _divContainer, + _svgContainer, + _identityMatrix, + _gEl, + _transformProp$1 = "transform", + _transformOriginProp$1 = _transformProp$1 + "Origin", + _hasOffsetBug, + _setDoc = function _setDoc(element) { + var doc = element.ownerDocument || element; + + if (!(_transformProp$1 in element.style) && "msTransform" in element.style) { + _transformProp$1 = "msTransform"; + _transformOriginProp$1 = _transformProp$1 + "Origin"; + } + + while (doc.parentNode && (doc = doc.parentNode)) {} + + _win$2 = window; + _identityMatrix = new Matrix2D(); + + if (doc) { + _doc$2 = doc; + _docElement$1 = doc.documentElement; + _body = doc.body; + _gEl = _doc$2.createElementNS("http://www.w3.org/2000/svg", "g"); + _gEl.style.transform = "none"; + var d1 = doc.createElement("div"), + d2 = doc.createElement("div"), + root = doc && (doc.body || doc.firstElementChild); + + if (root && root.appendChild) { + root.appendChild(d1); + d1.appendChild(d2); + d1.setAttribute("style", "position:static;transform:translate3d(0,0,1px)"); + _hasOffsetBug = d2.offsetParent !== d1; + root.removeChild(d1); + } + } + + return doc; + }, + _forceNonZeroScale = function _forceNonZeroScale(e) { + var a, cache; + + while (e && e !== _body) { + cache = e._gsap; + cache && cache.uncache && cache.get(e, "x"); + + if (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) { + cache.scaleX = cache.scaleY = 1e-4; + cache.renderTransform(1, cache); + a ? a.push(cache) : a = [cache]; + } + + e = e.parentNode; + } + + return a; + }, + _svgTemps = [], + _divTemps = [], + _getDocScrollTop = function _getDocScrollTop() { + return _win$2.pageYOffset || _doc$2.scrollTop || _docElement$1.scrollTop || _body.scrollTop || 0; + }, + _getDocScrollLeft = function _getDocScrollLeft() { + return _win$2.pageXOffset || _doc$2.scrollLeft || _docElement$1.scrollLeft || _body.scrollLeft || 0; + }, + _svgOwner = function _svgOwner(element) { + return element.ownerSVGElement || ((element.tagName + "").toLowerCase() === "svg" ? element : null); + }, + _isFixed = function _isFixed(element) { + if (_win$2.getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + return _isFixed(element); + } + }, + _createSibling = function _createSibling(element, i) { + if (element.parentNode && (_doc$2 || _setDoc(element))) { + var svg = _svgOwner(element), + ns = svg ? svg.getAttribute("xmlns") || "http://www.w3.org/2000/svg" : "http://www.w3.org/1999/xhtml", + type = svg ? i ? "rect" : "g" : "div", + x = i !== 2 ? 0 : 100, + y = i === 3 ? 100 : 0, + css = "position:absolute;display:block;pointer-events:none;margin:0;padding:0;", + e = _doc$2.createElementNS ? _doc$2.createElementNS(ns.replace(/^https/, "http"), type) : _doc$2.createElement(type); + + if (i) { + if (!svg) { + if (!_divContainer) { + _divContainer = _createSibling(element); + _divContainer.style.cssText = css; + } + + e.style.cssText = css + "width:0.1px;height:0.1px;top:" + y + "px;left:" + x + "px"; + + _divContainer.appendChild(e); + } else { + _svgContainer || (_svgContainer = _createSibling(element)); + e.setAttribute("width", 0.01); + e.setAttribute("height", 0.01); + e.setAttribute("transform", "translate(" + x + "," + y + ")"); + + _svgContainer.appendChild(e); + } + } + + return e; + } + + throw "Need document and parent."; + }, + _consolidate = function _consolidate(m) { + var c = new Matrix2D(), + i = 0; + + for (; i < m.numberOfItems; i++) { + c.multiply(m.getItem(i).matrix); + } + + return c; + }, + _getCTM = function _getCTM(svg) { + var m = svg.getCTM(), + transform; + + if (!m) { + transform = svg.style[_transformProp$1]; + svg.style[_transformProp$1] = "none"; + svg.appendChild(_gEl); + m = _gEl.getCTM(); + svg.removeChild(_gEl); + transform ? svg.style[_transformProp$1] = transform : svg.style.removeProperty(_transformProp$1.replace(/([A-Z])/g, "-$1").toLowerCase()); + } + + return m || _identityMatrix.clone(); + }, + _placeSiblings = function _placeSiblings(element, adjustGOffset) { + var svg = _svgOwner(element), + isRootSVG = element === svg, + siblings = svg ? _svgTemps : _divTemps, + parent = element.parentNode, + container, + m, + b, + x, + y, + cs; + + if (element === _win$2) { + return element; + } + + siblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3)); + container = svg ? _svgContainer : _divContainer; + + if (svg) { + if (isRootSVG) { + b = _getCTM(element); + x = -b.e / b.a; + y = -b.f / b.d; + m = _identityMatrix; + } else if (element.getBBox) { + b = element.getBBox(); + m = element.transform ? element.transform.baseVal : {}; + m = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; + x = m.a * b.x + m.c * b.y; + y = m.b * b.x + m.d * b.y; + } else { + m = new Matrix2D(); + x = y = 0; + } + + if (adjustGOffset && element.tagName.toLowerCase() === "g") { + x = y = 0; + } + + (isRootSVG ? svg : parent).appendChild(container); + container.setAttribute("transform", "matrix(" + m.a + "," + m.b + "," + m.c + "," + m.d + "," + (m.e + x) + "," + (m.f + y) + ")"); + } else { + x = y = 0; + + if (_hasOffsetBug) { + m = element.offsetParent; + b = element; + + while (b && (b = b.parentNode) && b !== m && b.parentNode) { + if ((_win$2.getComputedStyle(b)[_transformProp$1] + "").length > 4) { + x = b.offsetLeft; + y = b.offsetTop; + b = 0; + } + } + } + + cs = _win$2.getComputedStyle(element); + + if (cs.position !== "absolute" && cs.position !== "fixed") { + m = element.offsetParent; + + while (parent && parent !== m) { + x += parent.scrollLeft || 0; + y += parent.scrollTop || 0; + parent = parent.parentNode; + } + } + + b = container.style; + b.top = element.offsetTop - y + "px"; + b.left = element.offsetLeft - x + "px"; + b[_transformProp$1] = cs[_transformProp$1]; + b[_transformOriginProp$1] = cs[_transformOriginProp$1]; + b.position = cs.position === "fixed" ? "fixed" : "absolute"; + element.parentNode.appendChild(container); + } + + return container; + }, + _setMatrix = function _setMatrix(m, a, b, c, d, e, f) { + m.a = a; + m.b = b; + m.c = c; + m.d = d; + m.e = e; + m.f = f; + return m; + }; + + var Matrix2D = function () { + function Matrix2D(a, b, c, d, e, f) { + if (a === void 0) { + a = 1; + } + + if (b === void 0) { + b = 0; + } + + if (c === void 0) { + c = 0; + } + + if (d === void 0) { + d = 1; + } + + if (e === void 0) { + e = 0; + } + + if (f === void 0) { + f = 0; + } + + _setMatrix(this, a, b, c, d, e, f); + } + + var _proto = Matrix2D.prototype; + + _proto.inverse = function inverse() { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + determinant = a * d - b * c || 1e-10; + return _setMatrix(this, d / determinant, -b / determinant, -c / determinant, a / determinant, (c * f - d * e) / determinant, -(a * f - b * e) / determinant); + }; + + _proto.multiply = function multiply(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + a2 = matrix.a, + b2 = matrix.c, + c2 = matrix.b, + d2 = matrix.d, + e2 = matrix.e, + f2 = matrix.f; + return _setMatrix(this, a2 * a + c2 * c, a2 * b + c2 * d, b2 * a + d2 * c, b2 * b + d2 * d, e + e2 * a + f2 * c, f + e2 * b + f2 * d); + }; + + _proto.clone = function clone() { + return new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f); + }; + + _proto.equals = function equals(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + return a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f; + }; + + _proto.apply = function apply(point, decoratee) { + if (decoratee === void 0) { + decoratee = {}; + } + + var x = point.x, + y = point.y, + a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + decoratee.x = x * a + y * c + e || 0; + decoratee.y = x * b + y * d + f || 0; + return decoratee; + }; + + return Matrix2D; + }(); + function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { + if (!element || !element.parentNode || (_doc$2 || _setDoc(element)).documentElement === element) { + return new Matrix2D(); + } + + var zeroScales = _forceNonZeroScale(element), + svg = _svgOwner(element), + temps = svg ? _svgTemps : _divTemps, + container = _placeSiblings(element, adjustGOffset), + b1 = temps[0].getBoundingClientRect(), + b2 = temps[1].getBoundingClientRect(), + b3 = temps[2].getBoundingClientRect(), + parent = container.parentNode, + isFixed = !includeScrollInFixed && _isFixed(element), + m = new Matrix2D((b2.left - b1.left) / 100, (b2.top - b1.top) / 100, (b3.left - b1.left) / 100, (b3.top - b1.top) / 100, b1.left + (isFixed ? 0 : _getDocScrollLeft()), b1.top + (isFixed ? 0 : _getDocScrollTop())); + + parent.removeChild(container); + + if (zeroScales) { + b1 = zeroScales.length; + + while (b1--) { + b2 = zeroScales[b1]; + b2.scaleX = b2.scaleY = 0; + b2.renderTransform(1, b2); + } + } + + return inverse ? m.inverse() : m; + } + + var gsap$2, + _win$3, + _doc$3, + _docElement$2, + _body$1, + _tempDiv$1, + _placeholderDiv, + _coreInitted$2, + _checkPrefix, + _toArray, + _supportsPassive, + _isTouchDevice, + _touchEventLookup, + _isMultiTouching, + _isAndroid, + InertiaPlugin, + _defaultCursor, + _supportsPointer, + _context$1, + _getStyleSaver$1, + _dragCount = 0, + _windowExists$2 = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$1 = function _getGSAP() { + return gsap$2 || _windowExists$2() && (gsap$2 = window.gsap) && gsap$2.registerPlugin && gsap$2; + }, + _isFunction$1 = function _isFunction(value) { + return typeof value === "function"; + }, + _isObject$1 = function _isObject(value) { + return typeof value === "object"; + }, + _isUndefined$2 = function _isUndefined(value) { + return typeof value === "undefined"; + }, + _emptyFunc$1 = function _emptyFunc() { + return false; + }, + _transformProp$2 = "transform", + _transformOriginProp$2 = "transformOrigin", + _round$3 = function _round(value) { + return Math.round(value * 10000) / 10000; + }, + _isArray$1 = Array.isArray, + _createElement$1 = function _createElement(type, ns) { + var e = _doc$3.createElementNS ? _doc$3.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc$3.createElement(type); + return e.style ? e : _doc$3.createElement(type); + }, + _RAD2DEG$2 = 180 / Math.PI, + _bigNum$3 = 1e20, + _identityMatrix$1 = new Matrix2D(), + _getTime = Date.now || function () { + return new Date().getTime(); + }, + _renderQueue = [], + _lookup = {}, + _lookupCount = 0, + _clickableTagExp = /^(?:a|input|textarea|button|select)$/i, + _lastDragTime = 0, + _temp1 = {}, + _windowProxy = {}, + _copy = function _copy(obj, factor) { + var copy = {}, + p; + + for (p in obj) { + copy[p] = factor ? obj[p] * factor : obj[p]; + } + + return copy; + }, + _extend = function _extend(obj, defaults) { + for (var p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + + return obj; + }, + _setTouchActionForAllDescendants = function _setTouchActionForAllDescendants(elements, value) { + var i = elements.length, + children; + + while (i--) { + value ? elements[i].style.touchAction = value : elements[i].style.removeProperty("touch-action"); + children = elements[i].children; + children && children.length && _setTouchActionForAllDescendants(children, value); + } + }, + _renderQueueTick = function _renderQueueTick() { + return _renderQueue.forEach(function (func) { + return func(); + }); + }, + _addToRenderQueue = function _addToRenderQueue(func) { + _renderQueue.push(func); + + if (_renderQueue.length === 1) { + gsap$2.ticker.add(_renderQueueTick); + } + }, + _renderQueueTimeout = function _renderQueueTimeout() { + return !_renderQueue.length && gsap$2.ticker.remove(_renderQueueTick); + }, + _removeFromRenderQueue = function _removeFromRenderQueue(func) { + var i = _renderQueue.length; + + while (i--) { + if (_renderQueue[i] === func) { + _renderQueue.splice(i, 1); + } + } + + gsap$2.to(_renderQueueTimeout, { + overwrite: true, + delay: 15, + duration: 0, + onComplete: _renderQueueTimeout, + data: "_draggable" + }); + }, + _setDefaults$1 = function _setDefaults(obj, defaults) { + for (var p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + + return obj; + }, + _addListener = function _addListener(element, type, func, capture) { + if (element.addEventListener) { + var touchType = _touchEventLookup[type]; + capture = capture || (_supportsPassive ? { + passive: false + } : null); + element.addEventListener(touchType || type, func, capture); + touchType && type !== touchType && element.addEventListener(type, func, capture); + } + }, + _removeListener = function _removeListener(element, type, func, capture) { + if (element.removeEventListener) { + var touchType = _touchEventLookup[type]; + element.removeEventListener(touchType || type, func, capture); + touchType && type !== touchType && element.removeEventListener(type, func, capture); + } + }, + _preventDefault = function _preventDefault(event) { + event.preventDefault && event.preventDefault(); + event.preventManipulation && event.preventManipulation(); + }, + _hasTouchID = function _hasTouchID(list, ID) { + var i = list.length; + + while (i--) { + if (list[i].identifier === ID) { + return true; + } + } + }, + _onMultiTouchDocumentEnd = function _onMultiTouchDocumentEnd(event) { + _isMultiTouching = event.touches && _dragCount < event.touches.length; + + _removeListener(event.target, "touchend", _onMultiTouchDocumentEnd); + }, + _onMultiTouchDocument = function _onMultiTouchDocument(event) { + _isMultiTouching = event.touches && _dragCount < event.touches.length; + + _addListener(event.target, "touchend", _onMultiTouchDocumentEnd); + }, + _getDocScrollTop$1 = function _getDocScrollTop(doc) { + return _win$3.pageYOffset || doc.scrollTop || doc.documentElement.scrollTop || doc.body.scrollTop || 0; + }, + _getDocScrollLeft$1 = function _getDocScrollLeft(doc) { + return _win$3.pageXOffset || doc.scrollLeft || doc.documentElement.scrollLeft || doc.body.scrollLeft || 0; + }, + _addScrollListener = function _addScrollListener(e, callback) { + _addListener(e, "scroll", callback); + + if (!_isRoot(e.parentNode)) { + _addScrollListener(e.parentNode, callback); + } + }, + _removeScrollListener = function _removeScrollListener(e, callback) { + _removeListener(e, "scroll", callback); + + if (!_isRoot(e.parentNode)) { + _removeScrollListener(e.parentNode, callback); + } + }, + _isRoot = function _isRoot(e) { + return !!(!e || e === _docElement$2 || e.nodeType === 9 || e === _doc$3.body || e === _win$3 || !e.nodeType || !e.parentNode); + }, + _getMaxScroll = function _getMaxScroll(element, axis) { + var dim = axis === "x" ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return Math.max(0, _isRoot(element) ? Math.max(_docElement$2[scroll], _body$1[scroll]) - (_win$3["inner" + dim] || _docElement$2[client] || _body$1[client]) : element[scroll] - element[client]); + }, + _recordMaxScrolls = function _recordMaxScrolls(e, skipCurrent) { + var x = _getMaxScroll(e, "x"), + y = _getMaxScroll(e, "y"); + + if (_isRoot(e)) { + e = _windowProxy; + } else { + _recordMaxScrolls(e.parentNode, skipCurrent); + } + + e._gsMaxScrollX = x; + e._gsMaxScrollY = y; + + if (!skipCurrent) { + e._gsScrollX = e.scrollLeft || 0; + e._gsScrollY = e.scrollTop || 0; + } + }, + _setStyle = function _setStyle(element, property, value) { + var style = element.style; + + if (!style) { + return; + } + + if (_isUndefined$2(style[property])) { + property = _checkPrefix(property, element) || property; + } + + if (value == null) { + style.removeProperty && style.removeProperty(property.replace(/([A-Z])/g, "-$1").toLowerCase()); + } else { + style[property] = value; + } + }, + _getComputedStyle = function _getComputedStyle(element) { + return _win$3.getComputedStyle(element instanceof Element ? element : element.host || (element.parentNode || {}).host || element); + }, + _tempRect = {}, + _parseRect = function _parseRect(e) { + if (e === _win$3) { + _tempRect.left = _tempRect.top = 0; + _tempRect.width = _tempRect.right = _docElement$2.clientWidth || e.innerWidth || _body$1.clientWidth || 0; + _tempRect.height = _tempRect.bottom = (e.innerHeight || 0) - 20 < _docElement$2.clientHeight ? _docElement$2.clientHeight : e.innerHeight || _body$1.clientHeight || 0; + return _tempRect; + } + + var doc = e.ownerDocument || _doc$3, + r = !_isUndefined$2(e.pageX) ? { + left: e.pageX - _getDocScrollLeft$1(doc), + top: e.pageY - _getDocScrollTop$1(doc), + right: e.pageX - _getDocScrollLeft$1(doc) + 1, + bottom: e.pageY - _getDocScrollTop$1(doc) + 1 + } : !e.nodeType && !_isUndefined$2(e.left) && !_isUndefined$2(e.top) ? e : _toArray(e)[0].getBoundingClientRect(); + + if (_isUndefined$2(r.right) && !_isUndefined$2(r.width)) { + r.right = r.left + r.width; + r.bottom = r.top + r.height; + } else if (_isUndefined$2(r.width)) { + r = { + width: r.right - r.left, + height: r.bottom - r.top, + right: r.right, + left: r.left, + bottom: r.bottom, + top: r.top + }; + } + + return r; + }, + _dispatchEvent = function _dispatchEvent(target, type, callbackName) { + var vars = target.vars, + callback = vars[callbackName], + listeners = target._listeners[type], + result; + + if (_isFunction$1(callback)) { + result = callback.apply(vars.callbackScope || target, vars[callbackName + "Params"] || [target.pointerEvent]); + } + + if (listeners && target.dispatchEvent(type) === false) { + result = false; + } + + return result; + }, + _getBounds = function _getBounds(target, context) { + var e = _toArray(target)[0], + top, + left, + offset; + + if (!e.nodeType && e !== _win$3) { + if (!_isUndefined$2(target.left)) { + offset = { + x: 0, + y: 0 + }; + return { + left: target.left - offset.x, + top: target.top - offset.y, + width: target.width, + height: target.height + }; + } + + left = target.min || target.minX || target.minRotation || 0; + top = target.min || target.minY || 0; + return { + left: left, + top: top, + width: (target.max || target.maxX || target.maxRotation || 0) - left, + height: (target.max || target.maxY || 0) - top + }; + } + + return _getElementBounds(e, context); + }, + _point1 = {}, + _getElementBounds = function _getElementBounds(element, context) { + context = _toArray(context)[0]; + var isSVG = element.getBBox && element.ownerSVGElement, + doc = element.ownerDocument || _doc$3, + left, + right, + top, + bottom, + matrix, + p1, + p2, + p3, + p4, + bbox, + width, + height, + cs; + + if (element === _win$3) { + top = _getDocScrollTop$1(doc); + left = _getDocScrollLeft$1(doc); + right = left + (doc.documentElement.clientWidth || element.innerWidth || doc.body.clientWidth || 0); + bottom = top + ((element.innerHeight || 0) - 20 < doc.documentElement.clientHeight ? doc.documentElement.clientHeight : element.innerHeight || doc.body.clientHeight || 0); + } else if (context === _win$3 || _isUndefined$2(context)) { + return element.getBoundingClientRect(); + } else { + left = top = 0; + + if (isSVG) { + bbox = element.getBBox(); + width = bbox.width; + height = bbox.height; + } else { + if (element.viewBox && (bbox = element.viewBox.baseVal)) { + left = bbox.x || 0; + top = bbox.y || 0; + width = bbox.width; + height = bbox.height; + } + + if (!width) { + cs = _getComputedStyle(element); + bbox = cs.boxSizing === "border-box"; + width = (parseFloat(cs.width) || element.clientWidth || 0) + (bbox ? 0 : parseFloat(cs.borderLeftWidth) + parseFloat(cs.borderRightWidth)); + height = (parseFloat(cs.height) || element.clientHeight || 0) + (bbox ? 0 : parseFloat(cs.borderTopWidth) + parseFloat(cs.borderBottomWidth)); + } + } + + right = width; + bottom = height; + } + + if (element === context) { + return { + left: left, + top: top, + width: right - left, + height: bottom - top + }; + } + + matrix = getGlobalMatrix(context, true).multiply(getGlobalMatrix(element)); + p1 = matrix.apply({ + x: left, + y: top + }); + p2 = matrix.apply({ + x: right, + y: top + }); + p3 = matrix.apply({ + x: right, + y: bottom + }); + p4 = matrix.apply({ + x: left, + y: bottom + }); + left = Math.min(p1.x, p2.x, p3.x, p4.x); + top = Math.min(p1.y, p2.y, p3.y, p4.y); + return { + left: left, + top: top, + width: Math.max(p1.x, p2.x, p3.x, p4.x) - left, + height: Math.max(p1.y, p2.y, p3.y, p4.y) - top + }; + }, + _parseInertia = function _parseInertia(draggable, snap, max, min, factor, forceZeroVelocity) { + var vars = {}, + a, + i, + l; + + if (snap) { + if (factor !== 1 && snap instanceof Array) { + vars.end = a = []; + l = snap.length; + + if (_isObject$1(snap[0])) { + for (i = 0; i < l; i++) { + a[i] = _copy(snap[i], factor); + } + } else { + for (i = 0; i < l; i++) { + a[i] = snap[i] * factor; + } + } + + max += 1.1; + min -= 1.1; + } else if (_isFunction$1(snap)) { + vars.end = function (value) { + var result = snap.call(draggable, value), + copy, + p; + + if (factor !== 1) { + if (_isObject$1(result)) { + copy = {}; + + for (p in result) { + copy[p] = result[p] * factor; + } + + result = copy; + } else { + result *= factor; + } + } + + return result; + }; + } else { + vars.end = snap; + } + } + + if (max || max === 0) { + vars.max = max; + } + + if (min || min === 0) { + vars.min = min; + } + + if (forceZeroVelocity) { + vars.velocity = 0; + } + + return vars; + }, + _isClickable = function _isClickable(element) { + var data; + return !element || !element.getAttribute || element === _body$1 ? false : (data = element.getAttribute("data-clickable")) === "true" || data !== "false" && (_clickableTagExp.test(element.nodeName + "") || element.getAttribute("contentEditable") === "true") ? true : _isClickable(element.parentNode); + }, + _setSelectable = function _setSelectable(elements, selectable) { + var i = elements.length, + e; + + while (i--) { + e = elements[i]; + e.ondragstart = e.onselectstart = selectable ? null : _emptyFunc$1; + gsap$2.set(e, { + lazy: true, + userSelect: selectable ? "text" : "none" + }); + } + }, + _isFixed$1 = function _isFixed(element) { + if (_getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + return _isFixed(element); + } + }, + _supports3D$1, + _addPaddingBR, + ScrollProxy = function ScrollProxy(element, vars) { + element = gsap$2.utils.toArray(element)[0]; + vars = vars || {}; + var content = document.createElement("div"), + style = content.style, + node = element.firstChild, + offsetTop = 0, + offsetLeft = 0, + prevTop = element.scrollTop, + prevLeft = element.scrollLeft, + scrollWidth = element.scrollWidth, + scrollHeight = element.scrollHeight, + extraPadRight = 0, + maxLeft = 0, + maxTop = 0, + elementWidth, + elementHeight, + contentHeight, + nextNode, + transformStart, + transformEnd; + + if (_supports3D$1 && vars.force3D !== false) { + transformStart = "translate3d("; + transformEnd = "px,0px)"; + } else if (_transformProp$2) { + transformStart = "translate("; + transformEnd = "px)"; + } + + this.scrollTop = function (value, force) { + if (!arguments.length) { + return -this.top(); + } + + this.top(-value, force); + }; + + this.scrollLeft = function (value, force) { + if (!arguments.length) { + return -this.left(); + } + + this.left(-value, force); + }; + + this.left = function (value, force) { + if (!arguments.length) { + return -(element.scrollLeft + offsetLeft); + } + + var dif = element.scrollLeft - prevLeft, + oldOffset = offsetLeft; + + if ((dif > 2 || dif < -2) && !force) { + prevLeft = element.scrollLeft; + gsap$2.killTweensOf(this, { + left: 1, + scrollLeft: 1 + }); + this.left(-prevLeft); + + if (vars.onKill) { + vars.onKill(); + } + + return; + } + + value = -value; + + if (value < 0) { + offsetLeft = value - 0.5 | 0; + value = 0; + } else if (value > maxLeft) { + offsetLeft = value - maxLeft | 0; + value = maxLeft; + } else { + offsetLeft = 0; + } + + if (offsetLeft || oldOffset) { + if (!this._skip) { + style[_transformProp$2] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + + if (offsetLeft + extraPadRight >= 0) { + style.paddingRight = offsetLeft + extraPadRight + "px"; + } + } + + element.scrollLeft = value | 0; + prevLeft = element.scrollLeft; + }; + + this.top = function (value, force) { + if (!arguments.length) { + return -(element.scrollTop + offsetTop); + } + + var dif = element.scrollTop - prevTop, + oldOffset = offsetTop; + + if ((dif > 2 || dif < -2) && !force) { + prevTop = element.scrollTop; + gsap$2.killTweensOf(this, { + top: 1, + scrollTop: 1 + }); + this.top(-prevTop); + + if (vars.onKill) { + vars.onKill(); + } + + return; + } + + value = -value; + + if (value < 0) { + offsetTop = value - 0.5 | 0; + value = 0; + } else if (value > maxTop) { + offsetTop = value - maxTop | 0; + value = maxTop; + } else { + offsetTop = 0; + } + + if (offsetTop || oldOffset) { + if (!this._skip) { + style[_transformProp$2] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + } + + element.scrollTop = value | 0; + prevTop = element.scrollTop; + }; + + this.maxScrollTop = function () { + return maxTop; + }; + + this.maxScrollLeft = function () { + return maxLeft; + }; + + this.disable = function () { + node = content.firstChild; + + while (node) { + nextNode = node.nextSibling; + element.appendChild(node); + node = nextNode; + } + + if (element === content.parentNode) { + element.removeChild(content); + } + }; + + this.enable = function () { + node = element.firstChild; + + if (node === content) { + return; + } + + while (node) { + nextNode = node.nextSibling; + content.appendChild(node); + node = nextNode; + } + + element.appendChild(content); + this.calibrate(); + }; + + this.calibrate = function (force) { + var widthMatches = element.clientWidth === elementWidth, + cs, + x, + y; + prevTop = element.scrollTop; + prevLeft = element.scrollLeft; + + if (widthMatches && element.clientHeight === elementHeight && content.offsetHeight === contentHeight && scrollWidth === element.scrollWidth && scrollHeight === element.scrollHeight && !force) { + return; + } + + if (offsetTop || offsetLeft) { + x = this.left(); + y = this.top(); + this.left(-element.scrollLeft); + this.top(-element.scrollTop); + } + + cs = _getComputedStyle(element); + + if (!widthMatches || force) { + style.display = "block"; + style.width = "auto"; + style.paddingRight = "0px"; + extraPadRight = Math.max(0, element.scrollWidth - element.clientWidth); + + if (extraPadRight) { + extraPadRight += parseFloat(cs.paddingLeft) + (_addPaddingBR ? parseFloat(cs.paddingRight) : 0); + } + } + + style.display = "inline-block"; + style.position = "relative"; + style.overflow = "visible"; + style.verticalAlign = "top"; + style.boxSizing = "content-box"; + style.width = "100%"; + style.paddingRight = extraPadRight + "px"; + + if (_addPaddingBR) { + style.paddingBottom = cs.paddingBottom; + } + + elementWidth = element.clientWidth; + elementHeight = element.clientHeight; + scrollWidth = element.scrollWidth; + scrollHeight = element.scrollHeight; + maxLeft = element.scrollWidth - elementWidth; + maxTop = element.scrollHeight - elementHeight; + contentHeight = content.offsetHeight; + style.display = "block"; + + if (x || y) { + this.left(x); + this.top(y); + } + }; + + this.content = content; + this.element = element; + this._skip = false; + this.enable(); + }, + _initCore$2 = function _initCore(required) { + if (_windowExists$2() && document.body) { + var nav = window && window.navigator; + _win$3 = window; + _doc$3 = document; + _docElement$2 = _doc$3.documentElement; + _body$1 = _doc$3.body; + _tempDiv$1 = _createElement$1("div"); + _supportsPointer = !!window.PointerEvent; + _placeholderDiv = _createElement$1("div"); + _placeholderDiv.style.cssText = "visibility:hidden;height:1px;top:-1px;pointer-events:none;position:relative;clear:both;cursor:grab"; + _defaultCursor = _placeholderDiv.style.cursor === "grab" ? "grab" : "move"; + _isAndroid = nav && nav.userAgent.toLowerCase().indexOf("android") !== -1; + _isTouchDevice = "ontouchstart" in _docElement$2 && "orientation" in _win$3 || nav && (nav.MaxTouchPoints > 0 || nav.msMaxTouchPoints > 0); + + _addPaddingBR = function () { + var div = _createElement$1("div"), + child = _createElement$1("div"), + childStyle = child.style, + parent = _body$1, + val; + + childStyle.display = "inline-block"; + childStyle.position = "relative"; + div.style.cssText = "width:90px;height:40px;padding:10px;overflow:auto;visibility:hidden"; + div.appendChild(child); + parent.appendChild(div); + val = child.offsetHeight + 18 > div.scrollHeight; + parent.removeChild(div); + return val; + }(); + + _touchEventLookup = function (types) { + var standard = types.split(","), + converted = ("onpointerdown" in _tempDiv$1 ? "pointerdown,pointermove,pointerup,pointercancel" : "onmspointerdown" in _tempDiv$1 ? "MSPointerDown,MSPointerMove,MSPointerUp,MSPointerCancel" : types).split(","), + obj = {}, + i = 4; + + while (--i > -1) { + obj[standard[i]] = converted[i]; + obj[converted[i]] = standard[i]; + } + + try { + _docElement$2.addEventListener("test", null, Object.defineProperty({}, "passive", { + get: function get() { + _supportsPassive = 1; + } + })); + } catch (e) {} + + return obj; + }("touchstart,touchmove,touchend,touchcancel"); + + _addListener(_doc$3, "touchcancel", _emptyFunc$1); + + _addListener(_win$3, "touchmove", _emptyFunc$1); + + _body$1 && _body$1.addEventListener("touchstart", _emptyFunc$1); + + _addListener(_doc$3, "contextmenu", function () { + for (var p in _lookup) { + if (_lookup[p].isPressed) { + _lookup[p].endDrag(); + } + } + }); + + gsap$2 = _coreInitted$2 = _getGSAP$1(); + } + + if (gsap$2) { + InertiaPlugin = gsap$2.plugins.inertia; + + _context$1 = gsap$2.core.context || function () {}; + + _checkPrefix = gsap$2.utils.checkPrefix; + _transformProp$2 = _checkPrefix(_transformProp$2); + _transformOriginProp$2 = _checkPrefix(_transformOriginProp$2); + _toArray = gsap$2.utils.toArray; + _getStyleSaver$1 = gsap$2.core.getStyleSaver; + _supports3D$1 = !!_checkPrefix("perspective"); + } else if (required) { + console.warn("Please gsap.registerPlugin(Draggable)"); + } + }; + + var EventDispatcher = function () { + function EventDispatcher(target) { + this._listeners = {}; + this.target = target || this; + } + + var _proto = EventDispatcher.prototype; + + _proto.addEventListener = function addEventListener(type, callback) { + var list = this._listeners[type] || (this._listeners[type] = []); + + if (!~list.indexOf(callback)) { + list.push(callback); + } + }; + + _proto.removeEventListener = function removeEventListener(type, callback) { + var list = this._listeners[type], + i = list && list.indexOf(callback); + i >= 0 && list.splice(i, 1); + }; + + _proto.dispatchEvent = function dispatchEvent(type) { + var _this = this; + + var result; + (this._listeners[type] || []).forEach(function (callback) { + return callback.call(_this, { + type: type, + target: _this.target + }) === false && (result = false); + }); + return result; + }; + + return EventDispatcher; + }(); + + var Draggable = function (_EventDispatcher) { + _inheritsLoose(Draggable, _EventDispatcher); + + function Draggable(target, vars) { + var _this2; + + _this2 = _EventDispatcher.call(this) || this; + _coreInitted$2 || _initCore$2(1); + target = _toArray(target)[0]; + _this2.styles = _getStyleSaver$1 && _getStyleSaver$1(target, "transform,left,top"); + + if (!InertiaPlugin) { + InertiaPlugin = gsap$2.plugins.inertia; + } + + _this2.vars = vars = _copy(vars || {}); + _this2.target = target; + _this2.x = _this2.y = _this2.rotation = 0; + _this2.dragResistance = parseFloat(vars.dragResistance) || 0; + _this2.edgeResistance = isNaN(vars.edgeResistance) ? 1 : parseFloat(vars.edgeResistance) || 0; + _this2.lockAxis = vars.lockAxis; + _this2.autoScroll = vars.autoScroll || 0; + _this2.lockedAxis = null; + _this2.allowEventDefault = !!vars.allowEventDefault; + gsap$2.getProperty(target, "x"); + + var type = (vars.type || "x,y").toLowerCase(), + xyMode = ~type.indexOf("x") || ~type.indexOf("y"), + rotationMode = type.indexOf("rotation") !== -1, + xProp = rotationMode ? "rotation" : xyMode ? "x" : "left", + yProp = xyMode ? "y" : "top", + allowX = !!(~type.indexOf("x") || ~type.indexOf("left") || type === "scroll"), + allowY = !!(~type.indexOf("y") || ~type.indexOf("top") || type === "scroll"), + minimumMovement = vars.minimumMovement || 2, + self = _assertThisInitialized(_this2), + triggers = _toArray(vars.trigger || vars.handle || target), + killProps = {}, + dragEndTime = 0, + checkAutoScrollBounds = false, + autoScrollMarginTop = vars.autoScrollMarginTop || 40, + autoScrollMarginRight = vars.autoScrollMarginRight || 40, + autoScrollMarginBottom = vars.autoScrollMarginBottom || 40, + autoScrollMarginLeft = vars.autoScrollMarginLeft || 40, + isClickable = vars.clickableTest || _isClickable, + clickTime = 0, + gsCache = target._gsap || gsap$2.core.getCache(target), + isFixed = _isFixed$1(target), + getPropAsNum = function getPropAsNum(property, unit) { + return parseFloat(gsCache.get(target, property, unit)); + }, + ownerDoc = target.ownerDocument || _doc$3, + enabled, + scrollProxy, + startPointerX, + startPointerY, + startElementX, + startElementY, + hasBounds, + hasDragCallback, + hasMoveCallback, + maxX, + minX, + maxY, + minY, + touch, + touchID, + rotationOrigin, + dirty, + old, + snapX, + snapY, + snapXY, + isClicking, + touchEventTarget, + matrix, + interrupted, + allowNativeTouchScrolling, + touchDragAxis, + isDispatching, + clickDispatch, + trustedClickDispatch, + isPreventingDefault, + innerMatrix, + dragged, + onContextMenu = function onContextMenu(e) { + _preventDefault(e); + + e.stopImmediatePropagation && e.stopImmediatePropagation(); + return false; + }, + render = function render(suppressEvents) { + if (self.autoScroll && self.isDragging && (checkAutoScrollBounds || dirty)) { + var e = target, + autoScrollFactor = self.autoScroll * 15, + parent, + isRoot, + rect, + pointerX, + pointerY, + changeX, + changeY, + gap; + checkAutoScrollBounds = false; + _windowProxy.scrollTop = _win$3.pageYOffset != null ? _win$3.pageYOffset : ownerDoc.documentElement.scrollTop != null ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop; + _windowProxy.scrollLeft = _win$3.pageXOffset != null ? _win$3.pageXOffset : ownerDoc.documentElement.scrollLeft != null ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft; + pointerX = self.pointerX - _windowProxy.scrollLeft; + pointerY = self.pointerY - _windowProxy.scrollTop; + + while (e && !isRoot) { + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + rect = isRoot ? { + bottom: Math.max(_docElement$2.clientHeight, _win$3.innerHeight || 0), + right: Math.max(_docElement$2.clientWidth, _win$3.innerWidth || 0), + left: 0, + top: 0 + } : parent.getBoundingClientRect(); + changeX = changeY = 0; + + if (allowY) { + gap = parent._gsMaxScrollY - parent.scrollTop; + + if (gap < 0) { + changeY = gap; + } else if (pointerY > rect.bottom - autoScrollMarginBottom && gap) { + checkAutoScrollBounds = true; + changeY = Math.min(gap, autoScrollFactor * (1 - Math.max(0, rect.bottom - pointerY) / autoScrollMarginBottom) | 0); + } else if (pointerY < rect.top + autoScrollMarginTop && parent.scrollTop) { + checkAutoScrollBounds = true; + changeY = -Math.min(parent.scrollTop, autoScrollFactor * (1 - Math.max(0, pointerY - rect.top) / autoScrollMarginTop) | 0); + } + + if (changeY) { + parent.scrollTop += changeY; + } + } + + if (allowX) { + gap = parent._gsMaxScrollX - parent.scrollLeft; + + if (gap < 0) { + changeX = gap; + } else if (pointerX > rect.right - autoScrollMarginRight && gap) { + checkAutoScrollBounds = true; + changeX = Math.min(gap, autoScrollFactor * (1 - Math.max(0, rect.right - pointerX) / autoScrollMarginRight) | 0); + } else if (pointerX < rect.left + autoScrollMarginLeft && parent.scrollLeft) { + checkAutoScrollBounds = true; + changeX = -Math.min(parent.scrollLeft, autoScrollFactor * (1 - Math.max(0, pointerX - rect.left) / autoScrollMarginLeft) | 0); + } + + if (changeX) { + parent.scrollLeft += changeX; + } + } + + if (isRoot && (changeX || changeY)) { + _win$3.scrollTo(parent.scrollLeft, parent.scrollTop); + + setPointerPosition(self.pointerX + changeX, self.pointerY + changeY); + } + + e = parent; + } + } + + if (dirty) { + var x = self.x, + y = self.y; + + if (rotationMode) { + self.deltaX = x - parseFloat(gsCache.rotation); + self.rotation = x; + gsCache.rotation = x + "deg"; + gsCache.renderTransform(1, gsCache); + } else { + if (scrollProxy) { + if (allowY) { + self.deltaY = y - scrollProxy.top(); + scrollProxy.top(y); + } + + if (allowX) { + self.deltaX = x - scrollProxy.left(); + scrollProxy.left(x); + } + } else if (xyMode) { + if (allowY) { + self.deltaY = y - parseFloat(gsCache.y); + gsCache.y = y + "px"; + } + + if (allowX) { + self.deltaX = x - parseFloat(gsCache.x); + gsCache.x = x + "px"; + } + + gsCache.renderTransform(1, gsCache); + } else { + if (allowY) { + self.deltaY = y - parseFloat(target.style.top || 0); + target.style.top = y + "px"; + } + + if (allowX) { + self.deltaX = x - parseFloat(target.style.left || 0); + target.style.left = x + "px"; + } + } + } + + if (hasDragCallback && !suppressEvents && !isDispatching) { + isDispatching = true; + + if (_dispatchEvent(self, "drag", "onDrag") === false) { + if (allowX) { + self.x -= self.deltaX; + } + + if (allowY) { + self.y -= self.deltaY; + } + + render(true); + } + + isDispatching = false; + } + } + + dirty = false; + }, + syncXY = function syncXY(skipOnUpdate, skipSnap) { + var x = self.x, + y = self.y, + snappedValue, + cs; + + if (!target._gsap) { + gsCache = gsap$2.core.getCache(target); + } + + gsCache.uncache && gsap$2.getProperty(target, "x"); + + if (xyMode) { + self.x = parseFloat(gsCache.x); + self.y = parseFloat(gsCache.y); + } else if (rotationMode) { + self.x = self.rotation = parseFloat(gsCache.rotation); + } else if (scrollProxy) { + self.y = scrollProxy.top(); + self.x = scrollProxy.left(); + } else { + self.y = parseFloat(target.style.top || (cs = _getComputedStyle(target)) && cs.top) || 0; + self.x = parseFloat(target.style.left || (cs || {}).left) || 0; + } + + if ((snapX || snapY || snapXY) && !skipSnap && (self.isDragging || self.isThrowing)) { + if (snapXY) { + _temp1.x = self.x; + _temp1.y = self.y; + snappedValue = snapXY(_temp1); + + if (snappedValue.x !== self.x) { + self.x = snappedValue.x; + dirty = true; + } + + if (snappedValue.y !== self.y) { + self.y = snappedValue.y; + dirty = true; + } + } + + if (snapX) { + snappedValue = snapX(self.x); + + if (snappedValue !== self.x) { + self.x = snappedValue; + + if (rotationMode) { + self.rotation = snappedValue; + } + + dirty = true; + } + } + + if (snapY) { + snappedValue = snapY(self.y); + + if (snappedValue !== self.y) { + self.y = snappedValue; + } + + dirty = true; + } + } + + dirty && render(true); + + if (!skipOnUpdate) { + self.deltaX = self.x - x; + self.deltaY = self.y - y; + + _dispatchEvent(self, "throwupdate", "onThrowUpdate"); + } + }, + buildSnapFunc = function buildSnapFunc(snap, min, max, factor) { + if (min == null) { + min = -_bigNum$3; + } + + if (max == null) { + max = _bigNum$3; + } + + if (_isFunction$1(snap)) { + return function (n) { + var edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance; + return snap.call(self, (n > max ? max + (n - max) * edgeTolerance : n < min ? min + (n - min) * edgeTolerance : n) * factor) * factor; + }; + } + + if (_isArray$1(snap)) { + return function (n) { + var i = snap.length, + closest = 0, + absDif = _bigNum$3, + val, + dif; + + while (--i > -1) { + val = snap[i]; + dif = val - n; + + if (dif < 0) { + dif = -dif; + } + + if (dif < absDif && val >= min && val <= max) { + closest = i; + absDif = dif; + } + } + + return snap[closest]; + }; + } + + return isNaN(snap) ? function (n) { + return n; + } : function () { + return snap * factor; + }; + }, + buildPointSnapFunc = function buildPointSnapFunc(snap, minX, maxX, minY, maxY, radius, factor) { + radius = radius && radius < _bigNum$3 ? radius * radius : _bigNum$3; + + if (_isFunction$1(snap)) { + return function (point) { + var edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance, + x = point.x, + y = point.y, + result, + dx, + dy; + point.x = x = x > maxX ? maxX + (x - maxX) * edgeTolerance : x < minX ? minX + (x - minX) * edgeTolerance : x; + point.y = y = y > maxY ? maxY + (y - maxY) * edgeTolerance : y < minY ? minY + (y - minY) * edgeTolerance : y; + result = snap.call(self, point); + + if (result !== point) { + point.x = result.x; + point.y = result.y; + } + + if (factor !== 1) { + point.x *= factor; + point.y *= factor; + } + + if (radius < _bigNum$3) { + dx = point.x - x; + dy = point.y - y; + + if (dx * dx + dy * dy > radius) { + point.x = x; + point.y = y; + } + } + + return point; + }; + } + + if (_isArray$1(snap)) { + return function (p) { + var i = snap.length, + closest = 0, + minDist = _bigNum$3, + x, + y, + point, + dist; + + while (--i > -1) { + point = snap[i]; + x = point.x - p.x; + y = point.y - p.y; + dist = x * x + y * y; + + if (dist < minDist) { + closest = i; + minDist = dist; + } + } + + return minDist <= radius ? snap[closest] : p; + }; + } + + return function (n) { + return n; + }; + }, + calculateBounds = function calculateBounds() { + var bounds, targetBounds, snap, snapIsRaw; + hasBounds = false; + + if (scrollProxy) { + scrollProxy.calibrate(); + self.minX = minX = -scrollProxy.maxScrollLeft(); + self.minY = minY = -scrollProxy.maxScrollTop(); + self.maxX = maxX = self.maxY = maxY = 0; + hasBounds = true; + } else if (!!vars.bounds) { + bounds = _getBounds(vars.bounds, target.parentNode); + + if (rotationMode) { + self.minX = minX = bounds.left; + self.maxX = maxX = bounds.left + bounds.width; + self.minY = minY = self.maxY = maxY = 0; + } else if (!_isUndefined$2(vars.bounds.maxX) || !_isUndefined$2(vars.bounds.maxY)) { + bounds = vars.bounds; + self.minX = minX = bounds.minX; + self.minY = minY = bounds.minY; + self.maxX = maxX = bounds.maxX; + self.maxY = maxY = bounds.maxY; + } else { + targetBounds = _getBounds(target, target.parentNode); + self.minX = minX = Math.round(getPropAsNum(xProp, "px") + bounds.left - targetBounds.left); + self.minY = minY = Math.round(getPropAsNum(yProp, "px") + bounds.top - targetBounds.top); + self.maxX = maxX = Math.round(minX + (bounds.width - targetBounds.width)); + self.maxY = maxY = Math.round(minY + (bounds.height - targetBounds.height)); + } + + if (minX > maxX) { + self.minX = maxX; + self.maxX = maxX = minX; + minX = self.minX; + } + + if (minY > maxY) { + self.minY = maxY; + self.maxY = maxY = minY; + minY = self.minY; + } + + if (rotationMode) { + self.minRotation = minX; + self.maxRotation = maxX; + } + + hasBounds = true; + } + + if (vars.liveSnap) { + snap = vars.liveSnap === true ? vars.snap || {} : vars.liveSnap; + snapIsRaw = _isArray$1(snap) || _isFunction$1(snap); + + if (rotationMode) { + snapX = buildSnapFunc(snapIsRaw ? snap : snap.rotation, minX, maxX, 1); + snapY = null; + } else { + if (snap.points) { + snapXY = buildPointSnapFunc(snapIsRaw ? snap : snap.points, minX, maxX, minY, maxY, snap.radius, scrollProxy ? -1 : 1); + } else { + if (allowX) { + snapX = buildSnapFunc(snapIsRaw ? snap : snap.x || snap.left || snap.scrollLeft, minX, maxX, scrollProxy ? -1 : 1); + } + + if (allowY) { + snapY = buildSnapFunc(snapIsRaw ? snap : snap.y || snap.top || snap.scrollTop, minY, maxY, scrollProxy ? -1 : 1); + } + } + } + } + }, + onThrowComplete = function onThrowComplete() { + self.isThrowing = false; + + _dispatchEvent(self, "throwcomplete", "onThrowComplete"); + }, + onThrowInterrupt = function onThrowInterrupt() { + self.isThrowing = false; + }, + animate = function animate(inertia, forceZeroVelocity) { + var snap, snapIsRaw, tween, overshootTolerance; + + if (inertia && InertiaPlugin) { + if (inertia === true) { + snap = vars.snap || vars.liveSnap || {}; + snapIsRaw = _isArray$1(snap) || _isFunction$1(snap); + inertia = { + resistance: (vars.throwResistance || vars.resistance || 1000) / (rotationMode ? 10 : 1) + }; + + if (rotationMode) { + inertia.rotation = _parseInertia(self, snapIsRaw ? snap : snap.rotation, maxX, minX, 1, forceZeroVelocity); + } else { + if (allowX) { + inertia[xProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.x || snap.left, maxX, minX, scrollProxy ? -1 : 1, forceZeroVelocity || self.lockedAxis === "x"); + } + + if (allowY) { + inertia[yProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.y || snap.top, maxY, minY, scrollProxy ? -1 : 1, forceZeroVelocity || self.lockedAxis === "y"); + } + + if (snap.points || _isArray$1(snap) && _isObject$1(snap[0])) { + inertia.linkedProps = xProp + "," + yProp; + inertia.radius = snap.radius; + } + } + } + + self.isThrowing = true; + overshootTolerance = !isNaN(vars.overshootTolerance) ? vars.overshootTolerance : vars.edgeResistance === 1 ? 0 : 1 - self.edgeResistance + 0.2; + + if (!inertia.duration) { + inertia.duration = { + max: Math.max(vars.minDuration || 0, "maxDuration" in vars ? vars.maxDuration : 2), + min: !isNaN(vars.minDuration) ? vars.minDuration : overshootTolerance === 0 || _isObject$1(inertia) && inertia.resistance > 1000 ? 0 : 0.5, + overshoot: overshootTolerance + }; + } + + self.tween = tween = gsap$2.to(scrollProxy || target, { + inertia: inertia, + data: "_draggable", + inherit: false, + onComplete: onThrowComplete, + onInterrupt: onThrowInterrupt, + onUpdate: vars.fastMode ? _dispatchEvent : syncXY, + onUpdateParams: vars.fastMode ? [self, "onthrowupdate", "onThrowUpdate"] : snap && snap.radius ? [false, true] : [] + }); + + if (!vars.fastMode) { + if (scrollProxy) { + scrollProxy._skip = true; + } + + tween.render(1e9, true, true); + syncXY(true, true); + self.endX = self.x; + self.endY = self.y; + + if (rotationMode) { + self.endRotation = self.x; + } + + tween.play(0); + syncXY(true, true); + + if (scrollProxy) { + scrollProxy._skip = false; + } + } + } else if (hasBounds) { + self.applyBounds(); + } + }, + updateMatrix = function updateMatrix(shiftStart) { + var start = matrix, + p; + matrix = getGlobalMatrix(target.parentNode, true); + + if (shiftStart && self.isPressed && !matrix.equals(start || new Matrix2D())) { + p = start.inverse().apply({ + x: startPointerX, + y: startPointerY + }); + matrix.apply(p, p); + startPointerX = p.x; + startPointerY = p.y; + } + + if (matrix.equals(_identityMatrix$1)) { + matrix = null; + } + }, + recordStartPositions = function recordStartPositions() { + var edgeTolerance = 1 - self.edgeResistance, + offsetX = isFixed ? _getDocScrollLeft$1(ownerDoc) : 0, + offsetY = isFixed ? _getDocScrollTop$1(ownerDoc) : 0, + parsedOrigin, + x, + y; + + if (xyMode) { + gsCache.x = getPropAsNum(xProp, "px") + "px"; + gsCache.y = getPropAsNum(yProp, "px") + "px"; + gsCache.renderTransform(); + } + + updateMatrix(false); + _point1.x = self.pointerX - offsetX; + _point1.y = self.pointerY - offsetY; + matrix && matrix.apply(_point1, _point1); + startPointerX = _point1.x; + startPointerY = _point1.y; + + if (dirty) { + setPointerPosition(self.pointerX, self.pointerY); + render(true); + } + + innerMatrix = getGlobalMatrix(target); + + if (scrollProxy) { + calculateBounds(); + startElementY = scrollProxy.top(); + startElementX = scrollProxy.left(); + } else { + if (isTweening()) { + syncXY(true, true); + calculateBounds(); + } else { + self.applyBounds(); + } + + if (rotationMode) { + parsedOrigin = target.ownerSVGElement ? [gsCache.xOrigin - target.getBBox().x, gsCache.yOrigin - target.getBBox().y] : (_getComputedStyle(target)[_transformOriginProp$2] || "0 0").split(" "); + rotationOrigin = self.rotationOrigin = getGlobalMatrix(target).apply({ + x: parseFloat(parsedOrigin[0]) || 0, + y: parseFloat(parsedOrigin[1]) || 0 + }); + syncXY(true, true); + x = self.pointerX - rotationOrigin.x - offsetX; + y = rotationOrigin.y - self.pointerY + offsetY; + startElementX = self.x; + startElementY = self.y = Math.atan2(y, x) * _RAD2DEG$2; + } else { + startElementY = getPropAsNum(yProp, "px"); + startElementX = getPropAsNum(xProp, "px"); + } + } + + if (hasBounds && edgeTolerance) { + if (startElementX > maxX) { + startElementX = maxX + (startElementX - maxX) / edgeTolerance; + } else if (startElementX < minX) { + startElementX = minX - (minX - startElementX) / edgeTolerance; + } + + if (!rotationMode) { + if (startElementY > maxY) { + startElementY = maxY + (startElementY - maxY) / edgeTolerance; + } else if (startElementY < minY) { + startElementY = minY - (minY - startElementY) / edgeTolerance; + } + } + } + + self.startX = startElementX = _round$3(startElementX); + self.startY = startElementY = _round$3(startElementY); + }, + isTweening = function isTweening() { + return self.tween && self.tween.isActive(); + }, + removePlaceholder = function removePlaceholder() { + if (_placeholderDiv.parentNode && !isTweening() && !self.isDragging) { + _placeholderDiv.parentNode.removeChild(_placeholderDiv); + } + }, + onPress = function onPress(e, force) { + var i; + + if (!enabled || self.isPressed || !e || (e.type === "mousedown" || e.type === "pointerdown") && !force && _getTime() - clickTime < 30 && _touchEventLookup[self.pointerEvent.type]) { + isPreventingDefault && e && enabled && _preventDefault(e); + return; + } + + interrupted = isTweening(); + dragged = false; + self.pointerEvent = e; + + if (_touchEventLookup[e.type]) { + touchEventTarget = ~e.type.indexOf("touch") ? e.currentTarget || e.target : ownerDoc; + + _addListener(touchEventTarget, "touchend", onRelease); + + _addListener(touchEventTarget, "touchmove", onMove); + + _addListener(touchEventTarget, "touchcancel", onRelease); + + _addListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + touchEventTarget = null; + + _addListener(ownerDoc, "mousemove", onMove); + } + + touchDragAxis = null; + + if (!_supportsPointer || !touchEventTarget) { + _addListener(ownerDoc, "mouseup", onRelease); + + e && e.target && _addListener(e.target, "mouseup", onRelease); + } + + isClicking = isClickable.call(self, e.target) && vars.dragClickables === false && !force; + + if (isClicking) { + _addListener(e.target, "change", onRelease); + + _dispatchEvent(self, "pressInit", "onPressInit"); + + _dispatchEvent(self, "press", "onPress"); + + _setSelectable(triggers, true); + + isPreventingDefault = false; + return; + } + + allowNativeTouchScrolling = !touchEventTarget || allowX === allowY || self.vars.allowNativeTouchScrolling === false || self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2) ? false : allowX ? "y" : "x"; + isPreventingDefault = !allowNativeTouchScrolling && !self.allowEventDefault; + + if (isPreventingDefault) { + _preventDefault(e); + + _addListener(_win$3, "touchforcechange", _preventDefault); + } + + if (e.changedTouches) { + e = touch = e.changedTouches[0]; + touchID = e.identifier; + } else if (e.pointerId) { + touchID = e.pointerId; + } else { + touch = touchID = null; + } + + _dragCount++; + + _addToRenderQueue(render); + + startPointerY = self.pointerY = e.pageY; + startPointerX = self.pointerX = e.pageX; + + _dispatchEvent(self, "pressInit", "onPressInit"); + + if (allowNativeTouchScrolling || self.autoScroll) { + _recordMaxScrolls(target.parentNode); + } + + if (target.parentNode && self.autoScroll && !scrollProxy && !rotationMode && target.parentNode._gsMaxScrollX && !_placeholderDiv.parentNode && !target.getBBox) { + _placeholderDiv.style.width = target.parentNode.scrollWidth + "px"; + target.parentNode.appendChild(_placeholderDiv); + } + + recordStartPositions(); + self.tween && self.tween.kill(); + self.isThrowing = false; + gsap$2.killTweensOf(scrollProxy || target, killProps, true); + scrollProxy && gsap$2.killTweensOf(target, { + scrollTo: 1 + }, true); + self.tween = self.lockedAxis = null; + + if (vars.zIndexBoost || !rotationMode && !scrollProxy && vars.zIndexBoost !== false) { + target.style.zIndex = Draggable.zIndex++; + } + + self.isPressed = true; + hasDragCallback = !!(vars.onDrag || self._listeners.drag); + hasMoveCallback = !!(vars.onMove || self._listeners.move); + + if (vars.cursor !== false || vars.activeCursor) { + i = triggers.length; + + while (--i > -1) { + gsap$2.set(triggers[i], { + cursor: vars.activeCursor || vars.cursor || (_defaultCursor === "grab" ? "grabbing" : _defaultCursor) + }); + } + } + + _dispatchEvent(self, "press", "onPress"); + }, + onMove = function onMove(e) { + var originalEvent = e, + touches, + pointerX, + pointerY, + i, + dx, + dy; + + if (!enabled || _isMultiTouching || !self.isPressed || !e) { + isPreventingDefault && e && enabled && _preventDefault(e); + return; + } + + self.pointerEvent = e; + touches = e.changedTouches; + + if (touches) { + e = touches[0]; + + if (e !== touch && e.identifier !== touchID) { + i = touches.length; + + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} + + if (i < 0) { + return; + } + } + } else if (e.pointerId && touchID && e.pointerId !== touchID) { + return; + } + + if (touchEventTarget && allowNativeTouchScrolling && !touchDragAxis) { + _point1.x = e.pageX - (isFixed ? _getDocScrollLeft$1(ownerDoc) : 0); + _point1.y = e.pageY - (isFixed ? _getDocScrollTop$1(ownerDoc) : 0); + matrix && matrix.apply(_point1, _point1); + pointerX = _point1.x; + pointerY = _point1.y; + dx = Math.abs(pointerX - startPointerX); + dy = Math.abs(pointerY - startPointerY); + + if (dx !== dy && (dx > minimumMovement || dy > minimumMovement) || _isAndroid && allowNativeTouchScrolling === touchDragAxis) { + touchDragAxis = dx > dy && allowX ? "x" : "y"; + + if (allowNativeTouchScrolling && touchDragAxis !== allowNativeTouchScrolling) { + _addListener(_win$3, "touchforcechange", _preventDefault); + } + + if (self.vars.lockAxisOnTouchScroll !== false && allowX && allowY) { + self.lockedAxis = touchDragAxis === "x" ? "y" : "x"; + _isFunction$1(self.vars.onLockAxis) && self.vars.onLockAxis.call(self, originalEvent); + } + + if (_isAndroid && allowNativeTouchScrolling === touchDragAxis) { + onRelease(originalEvent); + return; + } + } + } + + if (!self.allowEventDefault && (!allowNativeTouchScrolling || touchDragAxis && allowNativeTouchScrolling !== touchDragAxis) && originalEvent.cancelable !== false) { + _preventDefault(originalEvent); + + isPreventingDefault = true; + } else if (isPreventingDefault) { + isPreventingDefault = false; + } + + if (self.autoScroll) { + checkAutoScrollBounds = true; + } + + setPointerPosition(e.pageX, e.pageY, hasMoveCallback); + }, + setPointerPosition = function setPointerPosition(pointerX, pointerY, invokeOnMove) { + var dragTolerance = 1 - self.dragResistance, + edgeTolerance = 1 - self.edgeResistance, + prevPointerX = self.pointerX, + prevPointerY = self.pointerY, + prevStartElementY = startElementY, + prevX = self.x, + prevY = self.y, + prevEndX = self.endX, + prevEndY = self.endY, + prevEndRotation = self.endRotation, + prevDirty = dirty, + xChange, + yChange, + x, + y, + dif, + temp; + self.pointerX = pointerX; + self.pointerY = pointerY; + + if (isFixed) { + pointerX -= _getDocScrollLeft$1(ownerDoc); + pointerY -= _getDocScrollTop$1(ownerDoc); + } + + if (rotationMode) { + y = Math.atan2(rotationOrigin.y - pointerY, pointerX - rotationOrigin.x) * _RAD2DEG$2; + dif = self.y - y; + + if (dif > 180) { + startElementY -= 360; + self.y = y; + } else if (dif < -180) { + startElementY += 360; + self.y = y; + } + + if (self.x !== startElementX || Math.max(Math.abs(startPointerX - pointerX), Math.abs(startPointerY - pointerY)) > minimumMovement) { + self.y = y; + x = startElementX + (startElementY - y) * dragTolerance; + } else { + x = startElementX; + } + } else { + if (matrix) { + temp = pointerX * matrix.a + pointerY * matrix.c + matrix.e; + pointerY = pointerX * matrix.b + pointerY * matrix.d + matrix.f; + pointerX = temp; + } + + yChange = pointerY - startPointerY; + xChange = pointerX - startPointerX; + + if (yChange < minimumMovement && yChange > -minimumMovement) { + yChange = 0; + } + + if (xChange < minimumMovement && xChange > -minimumMovement) { + xChange = 0; + } + + if ((self.lockAxis || self.lockedAxis) && (xChange || yChange)) { + temp = self.lockedAxis; + + if (!temp) { + self.lockedAxis = temp = allowX && Math.abs(xChange) > Math.abs(yChange) ? "y" : allowY ? "x" : null; + + if (temp && _isFunction$1(self.vars.onLockAxis)) { + self.vars.onLockAxis.call(self, self.pointerEvent); + } + } + + if (temp === "y") { + yChange = 0; + } else if (temp === "x") { + xChange = 0; + } + } + + x = _round$3(startElementX + xChange * dragTolerance); + y = _round$3(startElementY + yChange * dragTolerance); + } + + if ((snapX || snapY || snapXY) && (self.x !== x || self.y !== y && !rotationMode)) { + if (snapXY) { + _temp1.x = x; + _temp1.y = y; + temp = snapXY(_temp1); + x = _round$3(temp.x); + y = _round$3(temp.y); + } + + if (snapX) { + x = _round$3(snapX(x)); + } + + if (snapY) { + y = _round$3(snapY(y)); + } + } + + if (hasBounds) { + if (x > maxX) { + x = maxX + Math.round((x - maxX) * edgeTolerance); + } else if (x < minX) { + x = minX + Math.round((x - minX) * edgeTolerance); + } + + if (!rotationMode) { + if (y > maxY) { + y = Math.round(maxY + (y - maxY) * edgeTolerance); + } else if (y < minY) { + y = Math.round(minY + (y - minY) * edgeTolerance); + } + } + } + + if (self.x !== x || self.y !== y && !rotationMode) { + if (rotationMode) { + self.endRotation = self.x = self.endX = x; + dirty = true; + } else { + if (allowY) { + self.y = self.endY = y; + dirty = true; + } + + if (allowX) { + self.x = self.endX = x; + dirty = true; + } + } + + if (!invokeOnMove || _dispatchEvent(self, "move", "onMove") !== false) { + if (!self.isDragging && self.isPressed) { + self.isDragging = dragged = true; + + _dispatchEvent(self, "dragstart", "onDragStart"); + } + } else { + self.pointerX = prevPointerX; + self.pointerY = prevPointerY; + startElementY = prevStartElementY; + self.x = prevX; + self.y = prevY; + self.endX = prevEndX; + self.endY = prevEndY; + self.endRotation = prevEndRotation; + dirty = prevDirty; + } + } + }, + onRelease = function onRelease(e, force) { + if (!enabled || !self.isPressed || e && touchID != null && !force && (e.pointerId && e.pointerId !== touchID && e.target !== target || e.changedTouches && !_hasTouchID(e.changedTouches, touchID))) { + isPreventingDefault && e && enabled && _preventDefault(e); + return; + } + + self.isPressed = false; + var originalEvent = e, + wasDragging = self.isDragging, + isContextMenuRelease = self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2), + placeholderDelayedCall = gsap$2.delayedCall(0.001, removePlaceholder), + touches, + i, + syntheticEvent, + eventTarget, + syntheticClick; + + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchend", onRelease); + + _removeListener(touchEventTarget, "touchmove", onMove); + + _removeListener(touchEventTarget, "touchcancel", onRelease); + + _removeListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + _removeListener(ownerDoc, "mousemove", onMove); + } + + _removeListener(_win$3, "touchforcechange", _preventDefault); + + if (!_supportsPointer || !touchEventTarget) { + _removeListener(ownerDoc, "mouseup", onRelease); + + e && e.target && _removeListener(e.target, "mouseup", onRelease); + } + + dirty = false; + + if (wasDragging) { + dragEndTime = _lastDragTime = _getTime(); + self.isDragging = false; + } + + _removeFromRenderQueue(render); + + if (isClicking && !isContextMenuRelease) { + if (e) { + _removeListener(e.target, "change", onRelease); + + self.pointerEvent = originalEvent; + } + + _setSelectable(triggers, false); + + _dispatchEvent(self, "release", "onRelease"); + + _dispatchEvent(self, "click", "onClick"); + + isClicking = false; + return; + } + + i = triggers.length; + + while (--i > -1) { + _setStyle(triggers[i], "cursor", vars.cursor || (vars.cursor !== false ? _defaultCursor : null)); + } + + _dragCount--; + + if (e) { + touches = e.changedTouches; + + if (touches) { + e = touches[0]; + + if (e !== touch && e.identifier !== touchID) { + i = touches.length; + + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} + + if (i < 0 && !force) { + return; + } + } + } + + self.pointerEvent = originalEvent; + self.pointerX = e.pageX; + self.pointerY = e.pageY; + } + + if (isContextMenuRelease && originalEvent) { + _preventDefault(originalEvent); + + isPreventingDefault = true; + + _dispatchEvent(self, "release", "onRelease"); + } else if (originalEvent && !wasDragging) { + isPreventingDefault = false; + + if (interrupted && (vars.snap || vars.bounds)) { + animate(vars.inertia || vars.throwProps); + } + + _dispatchEvent(self, "release", "onRelease"); + + if ((!_isAndroid || originalEvent.type !== "touchmove") && originalEvent.type.indexOf("cancel") === -1) { + _dispatchEvent(self, "click", "onClick"); + + if (_getTime() - clickTime < 300) { + _dispatchEvent(self, "doubleclick", "onDoubleClick"); + } + + eventTarget = originalEvent.target || target; + clickTime = _getTime(); + + syntheticClick = function syntheticClick() { + if (clickTime !== clickDispatch && self.enabled() && !self.isPressed && !originalEvent.defaultPrevented) { + if (eventTarget.click) { + eventTarget.click(); + } else if (ownerDoc.createEvent) { + syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win$3, 1, self.pointerEvent.screenX, self.pointerEvent.screenY, self.pointerX, self.pointerY, false, false, false, false, 0, null); + eventTarget.dispatchEvent(syntheticEvent); + } + } + }; + + if (!_isAndroid && !originalEvent.defaultPrevented) { + gsap$2.delayedCall(0.05, syntheticClick); + } + } + } else { + animate(vars.inertia || vars.throwProps); + + if (!self.allowEventDefault && originalEvent && (vars.dragClickables !== false || !isClickable.call(self, originalEvent.target)) && wasDragging && (!allowNativeTouchScrolling || touchDragAxis && allowNativeTouchScrolling === touchDragAxis) && originalEvent.cancelable !== false) { + isPreventingDefault = true; + + _preventDefault(originalEvent); + } else { + isPreventingDefault = false; + } + + _dispatchEvent(self, "release", "onRelease"); + } + + isTweening() && placeholderDelayedCall.duration(self.tween.duration()); + wasDragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return true; + }, + updateScroll = function updateScroll(e) { + if (e && self.isDragging && !scrollProxy) { + var parent = e.target || target.parentNode, + deltaX = parent.scrollLeft - parent._gsScrollX, + deltaY = parent.scrollTop - parent._gsScrollY; + + if (deltaX || deltaY) { + if (matrix) { + startPointerX -= deltaX * matrix.a + deltaY * matrix.c; + startPointerY -= deltaY * matrix.d + deltaX * matrix.b; + } else { + startPointerX -= deltaX; + startPointerY -= deltaY; + } + + parent._gsScrollX += deltaX; + parent._gsScrollY += deltaY; + setPointerPosition(self.pointerX, self.pointerY); + } + } + }, + onClick = function onClick(e) { + var time = _getTime(), + recentlyClicked = time - clickTime < 100, + recentlyDragged = time - dragEndTime < 50, + alreadyDispatched = recentlyClicked && clickDispatch === clickTime, + defaultPrevented = self.pointerEvent && self.pointerEvent.defaultPrevented, + alreadyDispatchedTrusted = recentlyClicked && trustedClickDispatch === clickTime, + trusted = e.isTrusted || e.isTrusted == null && recentlyClicked && alreadyDispatched; + + if ((alreadyDispatched || recentlyDragged && self.vars.suppressClickOnDrag !== false) && e.stopImmediatePropagation) { + e.stopImmediatePropagation(); + } + + if (recentlyClicked && !(self.pointerEvent && self.pointerEvent.defaultPrevented) && (!alreadyDispatched || trusted && !alreadyDispatchedTrusted)) { + if (trusted && alreadyDispatched) { + trustedClickDispatch = clickTime; + } + + clickDispatch = clickTime; + return; + } + + if (self.isPressed || recentlyDragged || recentlyClicked) { + if (!trusted || !e.detail || !recentlyClicked || defaultPrevented) { + _preventDefault(e); + } + } + + if (!recentlyClicked && !recentlyDragged && !dragged) { + e && e.target && (self.pointerEvent = e); + + _dispatchEvent(self, "click", "onClick"); + } + }, + localizePoint = function localizePoint(p) { + return matrix ? { + x: p.x * matrix.a + p.y * matrix.c + matrix.e, + y: p.x * matrix.b + p.y * matrix.d + matrix.f + } : { + x: p.x, + y: p.y + }; + }; + + old = Draggable.get(target); + old && old.kill(); + + _this2.startDrag = function (event, align) { + var r1, r2, p1, p2; + onPress(event || self.pointerEvent, true); + + if (align && !self.hitTest(event || self.pointerEvent)) { + r1 = _parseRect(event || self.pointerEvent); + r2 = _parseRect(target); + p1 = localizePoint({ + x: r1.left + r1.width / 2, + y: r1.top + r1.height / 2 + }); + p2 = localizePoint({ + x: r2.left + r2.width / 2, + y: r2.top + r2.height / 2 + }); + startPointerX -= p1.x - p2.x; + startPointerY -= p1.y - p2.y; + } + + if (!self.isDragging) { + self.isDragging = dragged = true; + + _dispatchEvent(self, "dragstart", "onDragStart"); + } + }; + + _this2.drag = onMove; + + _this2.endDrag = function (e) { + return onRelease(e || self.pointerEvent, true); + }; + + _this2.timeSinceDrag = function () { + return self.isDragging ? 0 : (_getTime() - dragEndTime) / 1000; + }; + + _this2.timeSinceClick = function () { + return (_getTime() - clickTime) / 1000; + }; + + _this2.hitTest = function (target, threshold) { + return Draggable.hitTest(self.target, target, threshold); + }; + + _this2.getDirection = function (from, diagonalThreshold) { + var mode = from === "velocity" && InertiaPlugin ? from : _isObject$1(from) && !rotationMode ? "element" : "start", + xChange, + yChange, + ratio, + direction, + r1, + r2; + + if (mode === "element") { + r1 = _parseRect(self.target); + r2 = _parseRect(from); + } + + xChange = mode === "start" ? self.x - startElementX : mode === "velocity" ? InertiaPlugin.getVelocity(target, xProp) : r1.left + r1.width / 2 - (r2.left + r2.width / 2); + + if (rotationMode) { + return xChange < 0 ? "counter-clockwise" : "clockwise"; + } else { + diagonalThreshold = diagonalThreshold || 2; + yChange = mode === "start" ? self.y - startElementY : mode === "velocity" ? InertiaPlugin.getVelocity(target, yProp) : r1.top + r1.height / 2 - (r2.top + r2.height / 2); + ratio = Math.abs(xChange / yChange); + direction = ratio < 1 / diagonalThreshold ? "" : xChange < 0 ? "left" : "right"; + + if (ratio < diagonalThreshold) { + if (direction !== "") { + direction += "-"; + } + + direction += yChange < 0 ? "up" : "down"; + } + } + + return direction; + }; + + _this2.applyBounds = function (newBounds, sticky) { + var x, y, forceZeroVelocity, e, parent, isRoot; + + if (newBounds && vars.bounds !== newBounds) { + vars.bounds = newBounds; + return self.update(true, sticky); + } + + syncXY(true); + calculateBounds(); + + if (hasBounds && !isTweening()) { + x = self.x; + y = self.y; + + if (x > maxX) { + x = maxX; + } else if (x < minX) { + x = minX; + } + + if (y > maxY) { + y = maxY; + } else if (y < minY) { + y = minY; + } + + if (self.x !== x || self.y !== y) { + forceZeroVelocity = true; + self.x = self.endX = x; + + if (rotationMode) { + self.endRotation = x; + } else { + self.y = self.endY = y; + } + + dirty = true; + render(true); + + if (self.autoScroll && !self.isDragging) { + _recordMaxScrolls(target.parentNode); + + e = target; + _windowProxy.scrollTop = _win$3.pageYOffset != null ? _win$3.pageYOffset : ownerDoc.documentElement.scrollTop != null ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop; + _windowProxy.scrollLeft = _win$3.pageXOffset != null ? _win$3.pageXOffset : ownerDoc.documentElement.scrollLeft != null ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft; + + while (e && !isRoot) { + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + + if (allowY && parent.scrollTop > parent._gsMaxScrollY) { + parent.scrollTop = parent._gsMaxScrollY; + } + + if (allowX && parent.scrollLeft > parent._gsMaxScrollX) { + parent.scrollLeft = parent._gsMaxScrollX; + } + + e = parent; + } + } + } + + if (self.isThrowing && (forceZeroVelocity || self.endX > maxX || self.endX < minX || self.endY > maxY || self.endY < minY)) { + animate(vars.inertia || vars.throwProps, forceZeroVelocity); + } + } + + return self; + }; + + _this2.update = function (applyBounds, sticky, ignoreExternalChanges) { + if (sticky && self.isPressed) { + var m = getGlobalMatrix(target), + p = innerMatrix.apply({ + x: self.x - startElementX, + y: self.y - startElementY + }), + m2 = getGlobalMatrix(target.parentNode, true); + m2.apply({ + x: m.e - p.x, + y: m.f - p.y + }, p); + self.x -= p.x - m2.e; + self.y -= p.y - m2.f; + render(true); + recordStartPositions(); + } + + var x = self.x, + y = self.y; + updateMatrix(!sticky); + + if (applyBounds) { + self.applyBounds(); + } else { + dirty && ignoreExternalChanges && render(true); + syncXY(true); + } + + if (sticky) { + setPointerPosition(self.pointerX, self.pointerY); + dirty && render(true); + } + + if (self.isPressed && !sticky && (allowX && Math.abs(x - self.x) > 0.01 || allowY && Math.abs(y - self.y) > 0.01 && !rotationMode)) { + recordStartPositions(); + } + + if (self.autoScroll) { + _recordMaxScrolls(target.parentNode, self.isDragging); + + checkAutoScrollBounds = self.isDragging; + render(true); + + _removeScrollListener(target, updateScroll); + + _addScrollListener(target, updateScroll); + } + + return self; + }; + + _this2.enable = function (type) { + var setVars = { + lazy: true + }, + id, + i, + trigger; + + if (vars.cursor !== false) { + setVars.cursor = vars.cursor || _defaultCursor; + } + + if (gsap$2.utils.checkPrefix("touchCallout")) { + setVars.touchCallout = "none"; + } + + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, allowX === allowY ? "none" : vars.allowNativeTouchScrolling && target.scrollHeight === target.clientHeight === (target.scrollWidth === target.clientHeight) || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x"); + + i = triggers.length; + + while (--i > -1) { + trigger = triggers[i]; + _supportsPointer || _addListener(trigger, "mousedown", onPress); + + _addListener(trigger, "touchstart", onPress); + + _addListener(trigger, "click", onClick, true); + + gsap$2.set(trigger, setVars); + + if (trigger.getBBox && trigger.ownerSVGElement && allowX !== allowY) { + gsap$2.set(trigger.ownerSVGElement, { + touchAction: vars.allowNativeTouchScrolling || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x" + }); + } + + vars.allowContextMenu || _addListener(trigger, "contextmenu", onContextMenu); + } + + _setSelectable(triggers, false); + } + + _addScrollListener(target, updateScroll); + + enabled = true; + + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.track(scrollProxy || target, xyMode ? "x,y" : rotationMode ? "rotation" : "top,left"); + } + + target._gsDragID = id = target._gsDragID || "d" + _lookupCount++; + _lookup[id] = self; + + if (scrollProxy) { + scrollProxy.enable(); + scrollProxy.element._gsDragID = id; + } + + (vars.bounds || rotationMode) && recordStartPositions(); + vars.bounds && self.applyBounds(); + return self; + }; + + _this2.disable = function (type) { + var dragging = self.isDragging, + i = triggers.length, + trigger; + + while (--i > -1) { + _setStyle(triggers[i], "cursor", null); + } + + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, null); + + i = triggers.length; + + while (--i > -1) { + trigger = triggers[i]; + + _setStyle(trigger, "touchCallout", null); + + _removeListener(trigger, "mousedown", onPress); + + _removeListener(trigger, "touchstart", onPress); + + _removeListener(trigger, "click", onClick, true); + + _removeListener(trigger, "contextmenu", onContextMenu); + } + + _setSelectable(triggers, true); + + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchcancel", onRelease); + + _removeListener(touchEventTarget, "touchend", onRelease); + + _removeListener(touchEventTarget, "touchmove", onMove); + } + + _removeListener(ownerDoc, "mouseup", onRelease); + + _removeListener(ownerDoc, "mousemove", onMove); + } + + _removeScrollListener(target, updateScroll); + + enabled = false; + + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.untrack(scrollProxy || target, xyMode ? "x,y" : rotationMode ? "rotation" : "top,left"); + self.tween && self.tween.kill(); + } + + scrollProxy && scrollProxy.disable(); + + _removeFromRenderQueue(render); + + self.isDragging = self.isPressed = isClicking = false; + dragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return self; + }; + + _this2.enabled = function (value, type) { + return arguments.length ? value ? self.enable(type) : self.disable(type) : enabled; + }; + + _this2.kill = function () { + self.isThrowing = false; + self.tween && self.tween.kill(); + self.disable(); + gsap$2.set(triggers, { + clearProps: "userSelect" + }); + delete _lookup[target._gsDragID]; + return self; + }; + + _this2.revert = function () { + this.kill(); + this.styles && this.styles.revert(); + }; + + if (~type.indexOf("scroll")) { + scrollProxy = _this2.scrollProxy = new ScrollProxy(target, _extend({ + onKill: function onKill() { + self.isPressed && onRelease(null); + } + }, vars)); + target.style.overflowY = allowY && !_isTouchDevice ? "auto" : "hidden"; + target.style.overflowX = allowX && !_isTouchDevice ? "auto" : "hidden"; + target = scrollProxy.content; + } + + if (rotationMode) { + killProps.rotation = 1; + } else { + if (allowX) { + killProps[xProp] = 1; + } + + if (allowY) { + killProps[yProp] = 1; + } + } + + gsCache.force3D = "force3D" in vars ? vars.force3D : true; + + _context$1(_assertThisInitialized(_this2)); + + _this2.enable(); + + return _this2; + } + + Draggable.register = function register(core) { + gsap$2 = core; + + _initCore$2(); + }; + + Draggable.create = function create(targets, vars) { + _coreInitted$2 || _initCore$2(true); + return _toArray(targets).map(function (target) { + return new Draggable(target, vars); + }); + }; + + Draggable.get = function get(target) { + return _lookup[(_toArray(target)[0] || {})._gsDragID]; + }; + + Draggable.timeSinceDrag = function timeSinceDrag() { + return (_getTime() - _lastDragTime) / 1000; + }; + + Draggable.hitTest = function hitTest(obj1, obj2, threshold) { + if (obj1 === obj2) { + return false; + } + + var r1 = _parseRect(obj1), + r2 = _parseRect(obj2), + top = r1.top, + left = r1.left, + right = r1.right, + bottom = r1.bottom, + width = r1.width, + height = r1.height, + isOutside = r2.left > right || r2.right < left || r2.top > bottom || r2.bottom < top, + overlap, + area, + isRatio; + + if (isOutside || !threshold) { + return !isOutside; + } + + isRatio = (threshold + "").indexOf("%") !== -1; + threshold = parseFloat(threshold) || 0; + overlap = { + left: Math.max(left, r2.left), + top: Math.max(top, r2.top) + }; + overlap.width = Math.min(right, r2.right) - overlap.left; + overlap.height = Math.min(bottom, r2.bottom) - overlap.top; + + if (overlap.width < 0 || overlap.height < 0) { + return false; + } + + if (isRatio) { + threshold *= 0.01; + area = overlap.width * overlap.height; + return area >= width * height * threshold || area >= r2.width * r2.height * threshold; + } + + return overlap.width > threshold && overlap.height > threshold; + }; + + return Draggable; + }(EventDispatcher); + + _setDefaults$1(Draggable.prototype, { + pointerX: 0, + pointerY: 0, + startX: 0, + startY: 0, + deltaX: 0, + deltaY: 0, + isDragging: false, + isPressed: false + }); + + Draggable.zIndex = 1000; + Draggable.version = "3.12.7"; + _getGSAP$1() && gsap$2.registerPlugin(Draggable); + + /*! + * CSSRulePlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap$3, + _coreInitted$3, + _doc$4, + CSSPlugin$1, + _windowExists$3 = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$2 = function _getGSAP() { + return gsap$3 || _windowExists$3() && (gsap$3 = window.gsap) && gsap$3.registerPlugin && gsap$3; + }, + _checkRegister = function _checkRegister() { + if (!_coreInitted$3) { + _initCore$3(); + + if (!CSSPlugin$1) { + console.warn("Please gsap.registerPlugin(CSSPlugin, CSSRulePlugin)"); + } + } + + return _coreInitted$3; + }, + _initCore$3 = function _initCore(core) { + gsap$3 = core || _getGSAP$2(); + + if (_windowExists$3()) { + _doc$4 = document; + } + + if (gsap$3) { + CSSPlugin$1 = gsap$3.plugins.css; + + if (CSSPlugin$1) { + _coreInitted$3 = 1; + } + } + }; + + var CSSRulePlugin = { + version: "3.12.7", + name: "cssRule", + init: function init(target, value, tween, index, targets) { + if (!_checkRegister() || typeof target.cssText === "undefined") { + return false; + } + + var div = target._gsProxy = target._gsProxy || _doc$4.createElement("div"); + + this.ss = target; + this.style = div.style; + div.style.cssText = target.cssText; + CSSPlugin$1.prototype.init.call(this, div, value, tween, index, targets); + }, + render: function render(ratio, data) { + var pt = data._pt, + style = data.style, + ss = data.ss, + i; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + i = style.length; + + while (--i > -1) { + ss[style[i]] = style[style[i]]; + } + }, + getRule: function getRule(selector) { + _checkRegister(); + + var ruleProp = _doc$4.all ? "rules" : "cssRules", + styleSheets = _doc$4.styleSheets, + i = styleSheets.length, + pseudo = selector.charAt(0) === ":", + j, + curSS, + cs, + a; + selector = (pseudo ? "" : ",") + selector.split("::").join(":").toLowerCase() + ","; + + if (pseudo) { + a = []; + } + + while (i--) { + try { + curSS = styleSheets[i][ruleProp]; + + if (!curSS) { + continue; + } + + j = curSS.length; + } catch (e) { + console.warn(e); + continue; + } + + while (--j > -1) { + cs = curSS[j]; + + if (cs.selectorText && ("," + cs.selectorText.split("::").join(":").toLowerCase() + ",").indexOf(selector) !== -1) { + if (pseudo) { + a.push(cs.style); + } else { + return cs.style; + } + } + } + } + + return a; + }, + register: _initCore$3 + }; + _getGSAP$2() && gsap$3.registerPlugin(CSSRulePlugin); + + /*! + * EaselPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap$4, + _coreInitted$4, + _win$4, + _createJS, + _ColorFilter, + _ColorMatrixFilter, + _colorProps = "redMultiplier,greenMultiplier,blueMultiplier,alphaMultiplier,redOffset,greenOffset,blueOffset,alphaOffset".split(","), + _windowExists$4 = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$3 = function _getGSAP() { + return gsap$4 || _windowExists$4() && (gsap$4 = window.gsap) && gsap$4.registerPlugin && gsap$4; + }, + _getCreateJS = function _getCreateJS() { + return _createJS || _win$4 && _win$4.createjs || _win$4 || {}; + }, + _warn$1 = function _warn(message) { + return console.warn(message); + }, + _cache = function _cache(target) { + var b = target.getBounds && target.getBounds(); + + if (!b) { + b = target.nominalBounds || { + x: 0, + y: 0, + width: 100, + height: 100 + }; + target.setBounds && target.setBounds(b.x, b.y, b.width, b.height); + } + + target.cache && target.cache(b.x, b.y, b.width, b.height); + + _warn$1("EaselPlugin: for filters to display in EaselJS, you must call the object's cache() method first. GSAP attempted to use the target's getBounds() for the cache but that may not be completely accurate. " + target); + }, + _parseColorFilter = function _parseColorFilter(target, v, plugin) { + if (!_ColorFilter) { + _ColorFilter = _getCreateJS().ColorFilter; + + if (!_ColorFilter) { + _warn$1("EaselPlugin error: The EaselJS ColorFilter JavaScript file wasn't loaded."); + } + } + + var filters = target.filters || [], + i = filters.length, + c, + s, + e, + a, + p, + pt; + + while (i--) { + if (filters[i] instanceof _ColorFilter) { + s = filters[i]; + break; + } + } + + if (!s) { + s = new _ColorFilter(); + filters.push(s); + target.filters = filters; + } + + e = s.clone(); + + if (v.tint != null) { + c = gsap$4.utils.splitColor(v.tint); + a = v.tintAmount != null ? +v.tintAmount : 1; + e.redOffset = +c[0] * a; + e.greenOffset = +c[1] * a; + e.blueOffset = +c[2] * a; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - a; + } else { + for (p in v) { + if (p !== "exposure") if (p !== "brightness") { + e[p] = +v[p]; + } + } + } + + if (v.exposure != null) { + e.redOffset = e.greenOffset = e.blueOffset = 255 * (+v.exposure - 1); + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1; + } else if (v.brightness != null) { + a = +v.brightness - 1; + e.redOffset = e.greenOffset = e.blueOffset = a > 0 ? a * 255 : 0; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - Math.abs(a); + } + + i = 8; + + while (i--) { + p = _colorProps[i]; + + if (s[p] !== e[p]) { + pt = plugin.add(s, p, s[p], e[p], 0, 0, 0, 0, 0, 1); + + if (pt) { + pt.op = "easel_colorFilter"; + } + } + } + + plugin._props.push("easel_colorFilter"); + + if (!target.cacheID) { + _cache(target); + } + }, + _idMatrix = [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + _applyMatrix = function _applyMatrix(m, m2) { + if (!(m instanceof Array) || !(m2 instanceof Array)) { + return m2; + } + + var temp = [], + i = 0, + z = 0, + y, + x; + + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = x === 4 ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i + 1] * m2[x + 5] + m[i + 2] * m2[x + 10] + m[i + 3] * m2[x + 15] + z; + } + + i += 5; + } + + return temp; + }, + _setSaturation = function _setSaturation(m, n) { + if (isNaN(n)) { + return m; + } + + var inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); + }, + _colorize = function _colorize(m, color, amount) { + if (isNaN(amount)) { + amount = 1; + } + + var c = gsap$4.utils.splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); + }, + _setHue = function _setHue(m, n) { + if (isNaN(n)) { + return m; + } + + n *= Math.PI / 180; + var c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([_lumR + c * (1 - _lumR) + s * -_lumR, _lumG + c * -_lumG + s * -_lumG, _lumB + c * -_lumB + s * (1 - _lumB), 0, 0, _lumR + c * -_lumR + s * 0.143, _lumG + c * (1 - _lumG) + s * 0.14, _lumB + c * -_lumB + s * -0.283, 0, 0, _lumR + c * -_lumR + s * -(1 - _lumR), _lumG + c * -_lumG + s * _lumG, _lumB + c * (1 - _lumB) + s * _lumB, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); + }, + _setContrast = function _setContrast(m, n) { + if (isNaN(n)) { + return m; + } + + n += 0.01; + return _applyMatrix([n, 0, 0, 0, 128 * (1 - n), 0, n, 0, 0, 128 * (1 - n), 0, 0, n, 0, 128 * (1 - n), 0, 0, 0, 1, 0], m); + }, + _parseColorMatrixFilter = function _parseColorMatrixFilter(target, v, plugin) { + if (!_ColorMatrixFilter) { + _ColorMatrixFilter = _getCreateJS().ColorMatrixFilter; + + if (!_ColorMatrixFilter) { + _warn$1("EaselPlugin: The EaselJS ColorMatrixFilter JavaScript file wasn't loaded."); + } + } + + var filters = target.filters || [], + i = filters.length, + matrix, + startMatrix, + s, + pg; + + while (--i > -1) { + if (filters[i] instanceof _ColorMatrixFilter) { + s = filters[i]; + break; + } + } + + if (!s) { + s = new _ColorMatrixFilter(_idMatrix.slice()); + filters.push(s); + target.filters = filters; + } + + startMatrix = s.matrix; + matrix = _idMatrix.slice(); + + if (v.colorize != null) { + matrix = _colorize(matrix, v.colorize, Number(v.colorizeAmount)); + } + + if (v.contrast != null) { + matrix = _setContrast(matrix, Number(v.contrast)); + } + + if (v.hue != null) { + matrix = _setHue(matrix, Number(v.hue)); + } + + if (v.saturation != null) { + matrix = _setSaturation(matrix, Number(v.saturation)); + } + + i = matrix.length; + + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg = plugin.add(startMatrix, i, startMatrix[i], matrix[i], 0, 0, 0, 0, 0, 1); + + if (pg) { + pg.op = "easel_colorMatrixFilter"; + } + } + } + + plugin._props.push("easel_colorMatrixFilter"); + + if (!target.cacheID) { + _cache(); + } + + plugin._matrix = startMatrix; + }, + _initCore$4 = function _initCore(core) { + gsap$4 = core || _getGSAP$3(); + + if (_windowExists$4()) { + _win$4 = window; + } + + if (gsap$4) { + _coreInitted$4 = 1; + } + }; + + var EaselPlugin = { + version: "3.12.7", + name: "easel", + init: function init(target, value, tween, index, targets) { + if (!_coreInitted$4) { + _initCore$4(); + + if (!gsap$4) { + _warn$1("Please gsap.registerPlugin(EaselPlugin)"); + } + } + + this.target = target; + var p, pt, tint, colorMatrix, end, labels, i; + + for (p in value) { + end = value[p]; + + if (p === "colorFilter" || p === "tint" || p === "tintAmount" || p === "exposure" || p === "brightness") { + if (!tint) { + _parseColorFilter(target, value.colorFilter || value, this); + + tint = true; + } + } else if (p === "saturation" || p === "contrast" || p === "hue" || p === "colorize" || p === "colorizeAmount") { + if (!colorMatrix) { + _parseColorMatrixFilter(target, value.colorMatrixFilter || value, this); + + colorMatrix = true; + } + } else if (p === "frame") { + if (typeof end === "string" && end.charAt(1) !== "=" && (labels = target.labels)) { + for (i = 0; i < labels.length; i++) { + if (labels[i].label === end) { + end = labels[i].position; + } + } + } + + pt = this.add(target, "gotoAndStop", target.currentFrame, end, index, targets, Math.round, 0, 0, 1); + + if (pt) { + pt.op = p; + } + } else if (target[p] != null) { + this.add(target, p, "get", end); + } + } + }, + render: function render(ratio, data) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + if (data.target.cacheID) { + data.target.updateCache(); + } + }, + register: _initCore$4 + }; + + EaselPlugin.registerCreateJS = function (createjs) { + _createJS = createjs; + }; + + _getGSAP$3() && gsap$4.registerPlugin(EaselPlugin); + + /*! + * EasePack 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap$5, + _registerEase, + _getGSAP$4 = function _getGSAP() { + return gsap$5 || typeof window !== "undefined" && (gsap$5 = window.gsap) && gsap$5.registerPlugin && gsap$5; + }, + _boolean = function _boolean(value, defaultValue) { + return !!(typeof value === "undefined" ? defaultValue : value && !~(value + "").indexOf("false")); + }, + _initCore$5 = function _initCore(core) { + gsap$5 = core || _getGSAP$4(); + + if (gsap$5) { + _registerEase = gsap$5.registerEase; + + var eases = gsap$5.parseEase(), + createConfig = function createConfig(ease) { + return function (ratio) { + var y = 0.5 + ratio / 2; + + ease.config = function (p) { + return ease(2 * (1 - p) * p * y + p * p); + }; + }; + }, + p; + + for (p in eases) { + if (!eases[p].config) { + createConfig(eases[p]); + } + } + + _registerEase("slow", SlowMo); + + _registerEase("expoScale", ExpoScaleEase); + + _registerEase("rough", RoughEase); + + for (p in EasePack) { + p !== "version" && gsap$5.core.globals(p, EasePack[p]); + } + } + }, + _createSlowMo = function _createSlowMo(linearRatio, power, yoyoMode) { + linearRatio = Math.min(1, linearRatio || 0.7); + + var pow = linearRatio < 1 ? power || power === 0 ? power : 0.7 : 0, + p1 = (1 - linearRatio) / 2, + p3 = p1 + linearRatio, + calcEnd = _boolean(yoyoMode); + + return function (p) { + var r = p + (0.5 - p) * pow; + return p < p1 ? calcEnd ? 1 - (p = 1 - p / p1) * p : r - (p = 1 - p / p1) * p * p * p * r : p > p3 ? calcEnd ? p === 1 ? 0 : 1 - (p = (p - p3) / p1) * p : r + (p - r) * (p = (p - p3) / p1) * p * p * p : calcEnd ? 1 : r; + }; + }, + _createExpoScale = function _createExpoScale(start, end, ease) { + var p1 = Math.log(end / start), + p2 = end - start; + ease && (ease = gsap$5.parseEase(ease)); + return function (p) { + return (start * Math.exp(p1 * (ease ? ease(p) : p)) - start) / p2; + }; + }, + EasePoint = function EasePoint(time, value, next) { + this.t = time; + this.v = value; + + if (next) { + this.next = next; + next.prev = this; + this.c = next.v - value; + this.gap = next.t - time; + } + }, + _createRoughEase = function _createRoughEase(vars) { + if (typeof vars !== "object") { + vars = { + points: +vars || 20 + }; + } + + var taper = vars.taper || "none", + a = [], + cnt = 0, + points = (+vars.points || 20) | 0, + i = points, + randomize = _boolean(vars.randomize, true), + clamp = _boolean(vars.clamp), + template = gsap$5 ? gsap$5.parseEase(vars.template) : 0, + strength = (+vars.strength || 1) * 0.4, + x, + y, + bump, + invX, + obj, + pnt, + recent; + + while (--i > -1) { + x = randomize ? Math.random() : 1 / points * i; + y = template ? template(x) : x; + + if (taper === "none") { + bump = strength; + } else if (taper === "out") { + invX = 1 - x; + bump = invX * invX * strength; + } else if (taper === "in") { + bump = x * x * strength; + } else if (x < 0.5) { + invX = x * 2; + bump = invX * invX * 0.5 * strength; + } else { + invX = (1 - x) * 2; + bump = invX * invX * 0.5 * strength; + } + + if (randomize) { + y += Math.random() * bump - bump * 0.5; + } else if (i % 2) { + y += bump * 0.5; + } else { + y -= bump * 0.5; + } + + if (clamp) { + if (y > 1) { + y = 1; + } else if (y < 0) { + y = 0; + } + } + + a[cnt++] = { + x: x, + y: y + }; + } + + a.sort(function (a, b) { + return a.x - b.x; + }); + pnt = new EasePoint(1, 1, null); + i = points; + + while (i--) { + obj = a[i]; + pnt = new EasePoint(obj.x, obj.y, pnt); + } + + recent = new EasePoint(0, 0, pnt.t ? pnt : pnt.next); + return function (p) { + var pnt = recent; + + if (p > pnt.t) { + while (pnt.next && p >= pnt.t) { + pnt = pnt.next; + } + + pnt = pnt.prev; + } else { + while (pnt.prev && p <= pnt.t) { + pnt = pnt.prev; + } + } + + recent = pnt; + return pnt.v + (p - pnt.t) / pnt.gap * pnt.c; + }; + }; + + var SlowMo = _createSlowMo(0.7); + SlowMo.ease = SlowMo; + SlowMo.config = _createSlowMo; + var ExpoScaleEase = _createExpoScale(1, 2); + ExpoScaleEase.config = _createExpoScale; + var RoughEase = _createRoughEase(); + RoughEase.ease = RoughEase; + RoughEase.config = _createRoughEase; + var EasePack = { + SlowMo: SlowMo, + RoughEase: RoughEase, + ExpoScaleEase: ExpoScaleEase + }; + + for (var p in EasePack) { + EasePack[p].register = _initCore$5; + EasePack[p].version = "3.12.7"; + } + + _getGSAP$4() && gsap$5.registerPlugin(SlowMo); + + /*! + * Flip 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var _id = 1, + _toArray$1, + gsap$6, + _batch, + _batchAction, + _body$2, + _closestTenth, + _getStyleSaver$2, + _forEachBatch = function _forEachBatch(batch, name) { + return batch.actions.forEach(function (a) { + return a.vars[name] && a.vars[name](a); + }); + }, + _batchLookup = {}, + _RAD2DEG$3 = 180 / Math.PI, + _DEG2RAD$2 = Math.PI / 180, + _emptyObj = {}, + _dashedNameLookup = {}, + _memoizedRemoveProps = {}, + _listToArray = function _listToArray(list) { + return typeof list === "string" ? list.split(" ").join("").split(",") : list; + }, + _callbacks = _listToArray("onStart,onUpdate,onComplete,onReverseComplete,onInterrupt"), + _removeProps = _listToArray("transform,transformOrigin,width,height,position,top,left,opacity,zIndex,maxWidth,maxHeight,minWidth,minHeight"), + _getEl = function _getEl(target) { + return _toArray$1(target)[0] || console.warn("Element not found:", target); + }, + _round$4 = function _round(value) { + return Math.round(value * 10000) / 10000 || 0; + }, + _toggleClass = function _toggleClass(targets, className, action) { + return targets.forEach(function (el) { + return el.classList[action](className); + }); + }, + _reserved = { + zIndex: 1, + kill: 1, + simple: 1, + spin: 1, + clearProps: 1, + targets: 1, + toggleClass: 1, + onComplete: 1, + onUpdate: 1, + onInterrupt: 1, + onStart: 1, + delay: 1, + repeat: 1, + repeatDelay: 1, + yoyo: 1, + scale: 1, + fade: 1, + absolute: 1, + props: 1, + onEnter: 1, + onLeave: 1, + custom: 1, + paused: 1, + nested: 1, + prune: 1, + absoluteOnLeave: 1 + }, + _fitReserved = { + zIndex: 1, + simple: 1, + clearProps: 1, + scale: 1, + absolute: 1, + fitChild: 1, + getVars: 1, + props: 1 + }, + _camelToDashed = function _camelToDashed(p) { + return p.replace(/([A-Z])/g, "-$1").toLowerCase(); + }, + _copy$1 = function _copy(obj, exclude) { + var result = {}, + p; + + for (p in obj) { + exclude[p] || (result[p] = obj[p]); + } + + return result; + }, + _memoizedProps = {}, + _memoizeProps = function _memoizeProps(props) { + var p = _memoizedProps[props] = _listToArray(props); + + _memoizedRemoveProps[props] = p.concat(_removeProps); + return p; + }, + _getInverseGlobalMatrix = function _getInverseGlobalMatrix(el) { + var cache = el._gsap || gsap$6.core.getCache(el); + + if (cache.gmCache === gsap$6.ticker.frame) { + return cache.gMatrix; + } + + cache.gmCache = gsap$6.ticker.frame; + return cache.gMatrix = getGlobalMatrix(el, true, false, true); + }, + _getDOMDepth = function _getDOMDepth(el, invert, level) { + if (level === void 0) { + level = 0; + } + + var parent = el.parentNode, + inc = 1000 * Math.pow(10, level) * (invert ? -1 : 1), + l = invert ? -inc * 900 : 0; + + while (el) { + l += inc; + el = el.previousSibling; + } + + return parent ? l + _getDOMDepth(parent, invert, level + 1) : l; + }, + _orderByDOMDepth = function _orderByDOMDepth(comps, invert, isElStates) { + comps.forEach(function (comp) { + return comp.d = _getDOMDepth(isElStates ? comp.element : comp.t, invert); + }); + comps.sort(function (c1, c2) { + return c1.d - c2.d; + }); + return comps; + }, + _recordInlineStyles = function _recordInlineStyles(elState, props) { + var style = elState.element.style, + a = elState.css = elState.css || [], + i = props.length, + p, + v; + + while (i--) { + p = props[i]; + v = style[p] || style.getPropertyValue(p); + a.push(v ? p : _dashedNameLookup[p] || (_dashedNameLookup[p] = _camelToDashed(p)), v); + } + + return style; + }, + _applyInlineStyles = function _applyInlineStyles(state) { + var css = state.css, + style = state.element.style, + i = 0; + state.cache.uncache = 1; + + for (; i < css.length; i += 2) { + css[i + 1] ? style[css[i]] = css[i + 1] : style.removeProperty(css[i]); + } + + if (!css[css.indexOf("transform") + 1] && style.translate) { + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } + }, + _setFinalStates = function _setFinalStates(comps, onlyTransforms) { + comps.forEach(function (c) { + return c.a.cache.uncache = 1; + }); + onlyTransforms || comps.finalStates.forEach(_applyInlineStyles); + }, + _absoluteProps = "paddingTop,paddingRight,paddingBottom,paddingLeft,gridArea,transition".split(","), + _makeAbsolute = function _makeAbsolute(elState, fallbackNode, ignoreBatch) { + var element = elState.element, + width = elState.width, + height = elState.height, + uncache = elState.uncache, + getProp = elState.getProp, + style = element.style, + i = 4, + result, + displayIsNone, + cs; + typeof fallbackNode !== "object" && (fallbackNode = elState); + + if (_batch && ignoreBatch !== 1) { + _batch._abs.push({ + t: element, + b: elState, + a: elState, + sd: 0 + }); + + _batch._final.push(function () { + return (elState.cache.uncache = 1) && _applyInlineStyles(elState); + }); + + return element; + } + + displayIsNone = getProp("display") === "none"; + + if (!elState.isVisible || displayIsNone) { + displayIsNone && (_recordInlineStyles(elState, ["display"]).display = fallbackNode.display); + elState.matrix = fallbackNode.matrix; + elState.width = width = elState.width || fallbackNode.width; + elState.height = height = elState.height || fallbackNode.height; + } + + _recordInlineStyles(elState, _absoluteProps); + + cs = window.getComputedStyle(element); + + while (i--) { + style[_absoluteProps[i]] = cs[_absoluteProps[i]]; + } + + style.gridArea = "1 / 1 / 1 / 1"; + style.transition = "none"; + style.position = "absolute"; + style.width = width + "px"; + style.height = height + "px"; + style.top || (style.top = "0px"); + style.left || (style.left = "0px"); + + if (uncache) { + result = new ElementState(element); + } else { + result = _copy$1(elState, _emptyObj); + result.position = "absolute"; + + if (elState.simple) { + var bounds = element.getBoundingClientRect(); + result.matrix = new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()); + } else { + result.matrix = getGlobalMatrix(element, false, false, true); + } + } + + result = _fit(result, elState, true); + elState.x = _closestTenth(result.x, 0.01); + elState.y = _closestTenth(result.y, 0.01); + return element; + }, + _filterComps = function _filterComps(comps, targets) { + if (targets !== true) { + targets = _toArray$1(targets); + comps = comps.filter(function (c) { + if (targets.indexOf((c.sd < 0 ? c.b : c.a).element) !== -1) { + return true; + } else { + c.t._gsap.renderTransform(1); + + if (c.b.isVisible) { + c.t.style.width = c.b.width + "px"; + c.t.style.height = c.b.height + "px"; + } + } + }); + } + + return comps; + }, + _makeCompsAbsolute = function _makeCompsAbsolute(comps) { + return _orderByDOMDepth(comps, true).forEach(function (c) { + return (c.a.isVisible || c.b.isVisible) && _makeAbsolute(c.sd < 0 ? c.b : c.a, c.b, 1); + }); + }, + _findElStateInState = function _findElStateInState(state, other) { + return other && state.idLookup[_parseElementState(other).id] || state.elementStates[0]; + }, + _parseElementState = function _parseElementState(elOrNode, props, simple, other) { + return elOrNode instanceof ElementState ? elOrNode : elOrNode instanceof FlipState ? _findElStateInState(elOrNode, other) : new ElementState(typeof elOrNode === "string" ? _getEl(elOrNode) || console.warn(elOrNode + " not found") : elOrNode, props, simple); + }, + _recordProps = function _recordProps(elState, props) { + var getProp = gsap$6.getProperty(elState.element, null, "native"), + obj = elState.props = {}, + i = props.length; + + while (i--) { + obj[props[i]] = (getProp(props[i]) + "").trim(); + } + + obj.zIndex && (obj.zIndex = parseFloat(obj.zIndex) || 0); + return elState; + }, + _applyProps = function _applyProps(element, props) { + var style = element.style || element, + p; + + for (p in props) { + style[p] = props[p]; + } + }, + _getID = function _getID(el) { + var id = el.getAttribute("data-flip-id"); + id || el.setAttribute("data-flip-id", id = "auto-" + _id++); + return id; + }, + _elementsFromElementStates = function _elementsFromElementStates(elStates) { + return elStates.map(function (elState) { + return elState.element; + }); + }, + _handleCallback = function _handleCallback(callback, elStates, tl) { + return callback && elStates.length && tl.add(callback(_elementsFromElementStates(elStates), tl, new FlipState(elStates, 0, true)), 0); + }, + _fit = function _fit(fromState, toState, scale, applyProps, fitChild, vars) { + var element = fromState.element, + cache = fromState.cache, + parent = fromState.parent, + x = fromState.x, + y = fromState.y, + width = toState.width, + height = toState.height, + scaleX = toState.scaleX, + scaleY = toState.scaleY, + rotation = toState.rotation, + bounds = toState.bounds, + styles = vars && _getStyleSaver$2 && _getStyleSaver$2(element, "transform,width,height"), + dimensionState = fromState, + _toState$matrix = toState.matrix, + e = _toState$matrix.e, + f = _toState$matrix.f, + deep = fromState.bounds.width !== bounds.width || fromState.bounds.height !== bounds.height || fromState.scaleX !== scaleX || fromState.scaleY !== scaleY || fromState.rotation !== rotation, + simple = !deep && fromState.simple && toState.simple && !fitChild, + skewX, + fromPoint, + toPoint, + getProp, + parentMatrix, + matrix, + bbox; + + if (simple || !parent) { + scaleX = scaleY = 1; + rotation = skewX = 0; + } else { + parentMatrix = _getInverseGlobalMatrix(parent); + matrix = parentMatrix.clone().multiply(toState.ctm ? toState.matrix.clone().multiply(toState.ctm) : toState.matrix); + rotation = _round$4(Math.atan2(matrix.b, matrix.a) * _RAD2DEG$3); + skewX = _round$4(Math.atan2(matrix.c, matrix.d) * _RAD2DEG$3 + rotation) % 360; + scaleX = Math.sqrt(Math.pow(matrix.a, 2) + Math.pow(matrix.b, 2)); + scaleY = Math.sqrt(Math.pow(matrix.c, 2) + Math.pow(matrix.d, 2)) * Math.cos(skewX * _DEG2RAD$2); + + if (fitChild) { + fitChild = _toArray$1(fitChild)[0]; + getProp = gsap$6.getProperty(fitChild); + bbox = fitChild.getBBox && typeof fitChild.getBBox === "function" && fitChild.getBBox(); + dimensionState = { + scaleX: getProp("scaleX"), + scaleY: getProp("scaleY"), + width: bbox ? bbox.width : Math.ceil(parseFloat(getProp("width", "px"))), + height: bbox ? bbox.height : parseFloat(getProp("height", "px")) + }; + } + + cache.rotation = rotation + "deg"; + cache.skewX = skewX + "deg"; + } + + if (scale) { + scaleX *= width === dimensionState.width || !dimensionState.width ? 1 : width / dimensionState.width; + scaleY *= height === dimensionState.height || !dimensionState.height ? 1 : height / dimensionState.height; + cache.scaleX = scaleX; + cache.scaleY = scaleY; + } else { + width = _closestTenth(width * scaleX / dimensionState.scaleX, 0); + height = _closestTenth(height * scaleY / dimensionState.scaleY, 0); + element.style.width = width + "px"; + element.style.height = height + "px"; + } + + applyProps && _applyProps(element, toState.props); + + if (simple || !parent) { + x += e - fromState.matrix.e; + y += f - fromState.matrix.f; + } else if (deep || parent !== toState.parent) { + cache.renderTransform(1, cache); + matrix = getGlobalMatrix(fitChild || element, false, false, true); + fromPoint = parentMatrix.apply({ + x: matrix.e, + y: matrix.f + }); + toPoint = parentMatrix.apply({ + x: e, + y: f + }); + x += toPoint.x - fromPoint.x; + y += toPoint.y - fromPoint.y; + } else { + parentMatrix.e = parentMatrix.f = 0; + toPoint = parentMatrix.apply({ + x: e - fromState.matrix.e, + y: f - fromState.matrix.f + }); + x += toPoint.x; + y += toPoint.y; + } + + x = _closestTenth(x, 0.02); + y = _closestTenth(y, 0.02); + + if (vars && !(vars instanceof ElementState)) { + styles && styles.revert(); + } else { + cache.x = x + "px"; + cache.y = y + "px"; + cache.renderTransform(1, cache); + } + + if (vars) { + vars.x = x; + vars.y = y; + vars.rotation = rotation; + vars.skewX = skewX; + + if (scale) { + vars.scaleX = scaleX; + vars.scaleY = scaleY; + } else { + vars.width = width; + vars.height = height; + } + } + + return vars || cache; + }, + _parseState = function _parseState(targetsOrState, vars) { + return targetsOrState instanceof FlipState ? targetsOrState : new FlipState(targetsOrState, vars); + }, + _getChangingElState = function _getChangingElState(toState, fromState, id) { + var to1 = toState.idLookup[id], + to2 = toState.alt[id]; + return to2.isVisible && (!(fromState.getElementState(to2.element) || to2).isVisible || !to1.isVisible) ? to2 : to1; + }, + _bodyMetrics = [], + _bodyProps = "width,height,overflowX,overflowY".split(","), + _bodyLocked, + _lockBodyScroll = function _lockBodyScroll(lock) { + if (lock !== _bodyLocked) { + var s = _body$2.style, + w = _body$2.clientWidth === window.outerWidth, + h = _body$2.clientHeight === window.outerHeight, + i = 4; + + if (lock && (w || h)) { + while (i--) { + _bodyMetrics[i] = s[_bodyProps[i]]; + } + + if (w) { + s.width = _body$2.clientWidth + "px"; + s.overflowY = "hidden"; + } + + if (h) { + s.height = _body$2.clientHeight + "px"; + s.overflowX = "hidden"; + } + + _bodyLocked = lock; + } else if (_bodyLocked) { + while (i--) { + _bodyMetrics[i] ? s[_bodyProps[i]] = _bodyMetrics[i] : s.removeProperty(_camelToDashed(_bodyProps[i])); + } + + _bodyLocked = lock; + } + } + }, + _fromTo = function _fromTo(fromState, toState, vars, relative) { + fromState instanceof FlipState && toState instanceof FlipState || console.warn("Not a valid state object."); + vars = vars || {}; + + var _vars = vars, + clearProps = _vars.clearProps, + onEnter = _vars.onEnter, + onLeave = _vars.onLeave, + absolute = _vars.absolute, + absoluteOnLeave = _vars.absoluteOnLeave, + custom = _vars.custom, + delay = _vars.delay, + paused = _vars.paused, + repeat = _vars.repeat, + repeatDelay = _vars.repeatDelay, + yoyo = _vars.yoyo, + toggleClass = _vars.toggleClass, + nested = _vars.nested, + _zIndex = _vars.zIndex, + scale = _vars.scale, + fade = _vars.fade, + stagger = _vars.stagger, + spin = _vars.spin, + prune = _vars.prune, + props = ("props" in vars ? vars : fromState).props, + tweenVars = _copy$1(vars, _reserved), + animation = gsap$6.timeline({ + delay: delay, + paused: paused, + repeat: repeat, + repeatDelay: repeatDelay, + yoyo: yoyo, + data: "isFlip" + }), + remainingProps = tweenVars, + entering = [], + leaving = [], + comps = [], + swapOutTargets = [], + spinNum = spin === true ? 1 : spin || 0, + spinFunc = typeof spin === "function" ? spin : function () { + return spinNum; + }, + interrupted = fromState.interrupted || toState.interrupted, + addFunc = animation[relative !== 1 ? "to" : "from"], + v, + p, + endTime, + i, + el, + comp, + state, + targets, + finalStates, + fromNode, + toNode, + run, + a, + b; + + for (p in toState.idLookup) { + toNode = !toState.alt[p] ? toState.idLookup[p] : _getChangingElState(toState, fromState, p); + el = toNode.element; + fromNode = fromState.idLookup[p]; + fromState.alt[p] && el === fromNode.element && (fromState.alt[p].isVisible || !toNode.isVisible) && (fromNode = fromState.alt[p]); + + if (fromNode) { + comp = { + t: el, + b: fromNode, + a: toNode, + sd: fromNode.element === el ? 0 : toNode.isVisible ? 1 : -1 + }; + comps.push(comp); + + if (comp.sd) { + if (comp.sd < 0) { + comp.b = toNode; + comp.a = fromNode; + } + + interrupted && _recordInlineStyles(comp.b, props ? _memoizedRemoveProps[props] : _removeProps); + fade && comps.push(comp.swap = { + t: fromNode.element, + b: comp.b, + a: comp.a, + sd: -comp.sd, + swap: comp + }); + } + + el._flip = fromNode.element._flip = _batch ? _batch.timeline : animation; + } else if (toNode.isVisible) { + comps.push({ + t: el, + b: _copy$1(toNode, { + isVisible: 1 + }), + a: toNode, + sd: 0, + entering: 1 + }); + el._flip = _batch ? _batch.timeline : animation; + } + } + + props && (_memoizedProps[props] || _memoizeProps(props)).forEach(function (p) { + return tweenVars[p] = function (i) { + return comps[i].a.props[p]; + }; + }); + comps.finalStates = finalStates = []; + + run = function run() { + _orderByDOMDepth(comps); + + _lockBodyScroll(true); + + for (i = 0; i < comps.length; i++) { + comp = comps[i]; + a = comp.a; + b = comp.b; + + if (prune && !a.isDifferent(b) && !comp.entering) { + comps.splice(i--, 1); + } else { + el = comp.t; + nested && !(comp.sd < 0) && i && (a.matrix = getGlobalMatrix(el, false, false, true)); + + if (b.isVisible && a.isVisible) { + if (comp.sd < 0) { + state = new ElementState(el, props, fromState.simple); + + _fit(state, a, scale, 0, 0, state); + + state.matrix = getGlobalMatrix(el, false, false, true); + state.css = comp.b.css; + comp.a = a = state; + fade && (el.style.opacity = interrupted ? b.opacity : a.opacity); + stagger && swapOutTargets.push(el); + } else if (comp.sd > 0 && fade) { + el.style.opacity = interrupted ? a.opacity - b.opacity : "0"; + } + + _fit(a, b, scale, props); + } else if (b.isVisible !== a.isVisible) { + if (!b.isVisible) { + a.isVisible && entering.push(a); + comps.splice(i--, 1); + } else if (!a.isVisible) { + b.css = a.css; + leaving.push(b); + comps.splice(i--, 1); + absolute && nested && _fit(a, b, scale, props); + } + } + + if (!scale) { + el.style.maxWidth = Math.max(a.width, b.width) + "px"; + el.style.maxHeight = Math.max(a.height, b.height) + "px"; + el.style.minWidth = Math.min(a.width, b.width) + "px"; + el.style.minHeight = Math.min(a.height, b.height) + "px"; + } + + nested && toggleClass && el.classList.add(toggleClass); + } + + finalStates.push(a); + } + + var classTargets; + + if (toggleClass) { + classTargets = finalStates.map(function (s) { + return s.element; + }); + nested && classTargets.forEach(function (e) { + return e.classList.remove(toggleClass); + }); + } + + _lockBodyScroll(false); + + if (scale) { + tweenVars.scaleX = function (i) { + return comps[i].a.scaleX; + }; + + tweenVars.scaleY = function (i) { + return comps[i].a.scaleY; + }; + } else { + tweenVars.width = function (i) { + return comps[i].a.width + "px"; + }; + + tweenVars.height = function (i) { + return comps[i].a.height + "px"; + }; + + tweenVars.autoRound = vars.autoRound || false; + } + + tweenVars.x = function (i) { + return comps[i].a.x + "px"; + }; + + tweenVars.y = function (i) { + return comps[i].a.y + "px"; + }; + + tweenVars.rotation = function (i) { + return comps[i].a.rotation + (spin ? spinFunc(i, targets[i], targets) * 360 : 0); + }; + + tweenVars.skewX = function (i) { + return comps[i].a.skewX; + }; + + targets = comps.map(function (c) { + return c.t; + }); + + if (_zIndex || _zIndex === 0) { + tweenVars.modifiers = { + zIndex: function zIndex() { + return _zIndex; + } + }; + tweenVars.zIndex = _zIndex; + tweenVars.immediateRender = vars.immediateRender !== false; + } + + fade && (tweenVars.opacity = function (i) { + return comps[i].sd < 0 ? 0 : comps[i].sd > 0 ? comps[i].a.opacity : "+=0"; + }); + + if (swapOutTargets.length) { + stagger = gsap$6.utils.distribute(stagger); + var dummyArray = targets.slice(swapOutTargets.length); + + tweenVars.stagger = function (i, el) { + return stagger(~swapOutTargets.indexOf(el) ? targets.indexOf(comps[i].swap.t) : i, el, dummyArray); + }; + } + + _callbacks.forEach(function (name) { + return vars[name] && animation.eventCallback(name, vars[name], vars[name + "Params"]); + }); + + if (custom && targets.length) { + remainingProps = _copy$1(tweenVars, _reserved); + + if ("scale" in custom) { + custom.scaleX = custom.scaleY = custom.scale; + delete custom.scale; + } + + for (p in custom) { + v = _copy$1(custom[p], _fitReserved); + v[p] = tweenVars[p]; + !("duration" in v) && "duration" in tweenVars && (v.duration = tweenVars.duration); + v.stagger = tweenVars.stagger; + addFunc.call(animation, targets, v, 0); + delete remainingProps[p]; + } + } + + if (targets.length || leaving.length || entering.length) { + toggleClass && animation.add(function () { + return _toggleClass(classTargets, toggleClass, animation._zTime < 0 ? "remove" : "add"); + }, 0) && !paused && _toggleClass(classTargets, toggleClass, "add"); + targets.length && addFunc.call(animation, targets, remainingProps, 0); + } + + _handleCallback(onEnter, entering, animation); + + _handleCallback(onLeave, leaving, animation); + + var batchTl = _batch && _batch.timeline; + + if (batchTl) { + batchTl.add(animation, 0); + + _batch._final.push(function () { + return _setFinalStates(comps, !clearProps); + }); + } + + endTime = animation.duration(); + animation.call(function () { + var forward = animation.time() >= endTime; + forward && !batchTl && _setFinalStates(comps, !clearProps); + toggleClass && _toggleClass(classTargets, toggleClass, forward ? "remove" : "add"); + }); + }; + + absoluteOnLeave && (absolute = comps.filter(function (comp) { + return !comp.sd && !comp.a.isVisible && comp.b.isVisible; + }).map(function (comp) { + return comp.a.element; + })); + + if (_batch) { + var _batch$_abs; + + absolute && (_batch$_abs = _batch._abs).push.apply(_batch$_abs, _filterComps(comps, absolute)); + + _batch._run.push(run); + } else { + absolute && _makeCompsAbsolute(_filterComps(comps, absolute)); + run(); + } + + var anim = _batch ? _batch.timeline : animation; + + anim.revert = function () { + return _killFlip(anim, 1, 1); + }; + + return anim; + }, + _interrupt$1 = function _interrupt(tl) { + tl.vars.onInterrupt && tl.vars.onInterrupt.apply(tl, tl.vars.onInterruptParams || []); + tl.getChildren(true, false, true).forEach(_interrupt); + }, + _killFlip = function _killFlip(tl, action, force) { + if (tl && tl.progress() < 1 && (!tl.paused() || force)) { + if (action) { + _interrupt$1(tl); + + action < 2 && tl.progress(1); + tl.kill(); + } + + return true; + } + }, + _createLookup = function _createLookup(state) { + var lookup = state.idLookup = {}, + alt = state.alt = {}, + elStates = state.elementStates, + i = elStates.length, + elState; + + while (i--) { + elState = elStates[i]; + lookup[elState.id] ? alt[elState.id] = elState : lookup[elState.id] = elState; + } + }; + + var FlipState = function () { + function FlipState(targets, vars, targetsAreElementStates) { + this.props = vars && vars.props; + this.simple = !!(vars && vars.simple); + + if (targetsAreElementStates) { + this.targets = _elementsFromElementStates(targets); + this.elementStates = targets; + + _createLookup(this); + } else { + this.targets = _toArray$1(targets); + var soft = vars && (vars.kill === false || vars.batch && !vars.kill); + _batch && !soft && _batch._kill.push(this); + this.update(soft || !!_batch); + } + } + + var _proto = FlipState.prototype; + + _proto.update = function update(soft) { + var _this = this; + + this.elementStates = this.targets.map(function (el) { + return new ElementState(el, _this.props, _this.simple); + }); + + _createLookup(this); + + this.interrupt(soft); + this.recordInlineStyles(); + return this; + }; + + _proto.clear = function clear() { + this.targets.length = this.elementStates.length = 0; + + _createLookup(this); + + return this; + }; + + _proto.fit = function fit(state, scale, nested) { + var elStatesInOrder = _orderByDOMDepth(this.elementStates.slice(0), false, true), + toElStates = (state || this).idLookup, + i = 0, + fromNode, + toNode; + + for (; i < elStatesInOrder.length; i++) { + fromNode = elStatesInOrder[i]; + nested && (fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true)); + toNode = toElStates[fromNode.id]; + toNode && _fit(fromNode, toNode, scale, true, 0, fromNode); + fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true); + } + + return this; + }; + + _proto.getProperty = function getProperty(element, property) { + var es = this.getElementState(element) || _emptyObj; + + return (property in es ? es : es.props || _emptyObj)[property]; + }; + + _proto.add = function add(state) { + var i = state.targets.length, + lookup = this.idLookup, + alt = this.alt, + index, + es, + es2; + + while (i--) { + es = state.elementStates[i]; + es2 = lookup[es.id]; + + if (es2 && (es.element === es2.element || alt[es.id] && alt[es.id].element === es.element)) { + index = this.elementStates.indexOf(es.element === es2.element ? es2 : alt[es.id]); + this.targets.splice(index, 1, state.targets[i]); + this.elementStates.splice(index, 1, es); + } else { + this.targets.push(state.targets[i]); + this.elementStates.push(es); + } + } + + state.interrupted && (this.interrupted = true); + state.simple || (this.simple = false); + + _createLookup(this); + + return this; + }; + + _proto.compare = function compare(state) { + var l1 = state.idLookup, + l2 = this.idLookup, + unchanged = [], + changed = [], + enter = [], + leave = [], + targets = [], + a1 = state.alt, + a2 = this.alt, + place = function place(s1, s2, el) { + return (s1.isVisible !== s2.isVisible ? s1.isVisible ? enter : leave : s1.isVisible ? changed : unchanged).push(el) && targets.push(el); + }, + placeIfDoesNotExist = function placeIfDoesNotExist(s1, s2, el) { + return targets.indexOf(el) < 0 && place(s1, s2, el); + }, + s1, + s2, + p, + el, + s1Alt, + s2Alt, + c1, + c2; + + for (p in l1) { + s1Alt = a1[p]; + s2Alt = a2[p]; + s1 = !s1Alt ? l1[p] : _getChangingElState(state, this, p); + el = s1.element; + s2 = l2[p]; + + if (s2Alt) { + c2 = s2.isVisible || !s2Alt.isVisible && el === s2.element ? s2 : s2Alt; + c1 = s1Alt && !s1.isVisible && !s1Alt.isVisible && c2.element === s1Alt.element ? s1Alt : s1; + + if (c1.isVisible && c2.isVisible && c1.element !== c2.element) { + (c1.isDifferent(c2) ? changed : unchanged).push(c1.element, c2.element); + targets.push(c1.element, c2.element); + } else { + place(c1, c2, c1.element); + } + + s1Alt && c1.element === s1Alt.element && (s1Alt = l1[p]); + placeIfDoesNotExist(c1.element !== s2.element && s1Alt ? s1Alt : c1, s2, s2.element); + placeIfDoesNotExist(s1Alt && s1Alt.element === s2Alt.element ? s1Alt : c1, s2Alt, s2Alt.element); + s1Alt && placeIfDoesNotExist(s1Alt, s2Alt.element === s1Alt.element ? s2Alt : s2, s1Alt.element); + } else { + !s2 ? enter.push(el) : !s2.isDifferent(s1) ? unchanged.push(el) : place(s1, s2, el); + s1Alt && placeIfDoesNotExist(s1Alt, s2, s1Alt.element); + } + } + + for (p in l2) { + if (!l1[p]) { + leave.push(l2[p].element); + a2[p] && leave.push(a2[p].element); + } + } + + return { + changed: changed, + unchanged: unchanged, + enter: enter, + leave: leave + }; + }; + + _proto.recordInlineStyles = function recordInlineStyles() { + var props = _memoizedRemoveProps[this.props] || _removeProps, + i = this.elementStates.length; + + while (i--) { + _recordInlineStyles(this.elementStates[i], props); + } + }; + + _proto.interrupt = function interrupt(soft) { + var _this2 = this; + + var timelines = []; + this.targets.forEach(function (t) { + var tl = t._flip, + foundInProgress = _killFlip(tl, soft ? 0 : 1); + + soft && foundInProgress && timelines.indexOf(tl) < 0 && tl.add(function () { + return _this2.updateVisibility(); + }); + foundInProgress && timelines.push(tl); + }); + !soft && timelines.length && this.updateVisibility(); + this.interrupted || (this.interrupted = !!timelines.length); + }; + + _proto.updateVisibility = function updateVisibility() { + this.elementStates.forEach(function (es) { + var b = es.element.getBoundingClientRect(); + es.isVisible = !!(b.width || b.height || b.top || b.left); + es.uncache = 1; + }); + }; + + _proto.getElementState = function getElementState(element) { + return this.elementStates[this.targets.indexOf(_getEl(element))]; + }; + + _proto.makeAbsolute = function makeAbsolute() { + return _orderByDOMDepth(this.elementStates.slice(0), true, true).map(_makeAbsolute); + }; + + return FlipState; + }(); + + var ElementState = function () { + function ElementState(element, props, simple) { + this.element = element; + this.update(props, simple); + } + + var _proto2 = ElementState.prototype; + + _proto2.isDifferent = function isDifferent(state) { + var b1 = this.bounds, + b2 = state.bounds; + return b1.top !== b2.top || b1.left !== b2.left || b1.width !== b2.width || b1.height !== b2.height || !this.matrix.equals(state.matrix) || this.opacity !== state.opacity || this.props && state.props && JSON.stringify(this.props) !== JSON.stringify(state.props); + }; + + _proto2.update = function update(props, simple) { + var self = this, + element = self.element, + getProp = gsap$6.getProperty(element), + cache = gsap$6.core.getCache(element), + bounds = element.getBoundingClientRect(), + bbox = element.getBBox && typeof element.getBBox === "function" && element.nodeName.toLowerCase() !== "svg" && element.getBBox(), + m = simple ? new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()) : getGlobalMatrix(element, false, false, true); + self.getProp = getProp; + self.element = element; + self.id = _getID(element); + self.matrix = m; + self.cache = cache; + self.bounds = bounds; + self.isVisible = !!(bounds.width || bounds.height || bounds.left || bounds.top); + self.display = getProp("display"); + self.position = getProp("position"); + self.parent = element.parentNode; + self.x = getProp("x"); + self.y = getProp("y"); + self.scaleX = cache.scaleX; + self.scaleY = cache.scaleY; + self.rotation = getProp("rotation"); + self.skewX = getProp("skewX"); + self.opacity = getProp("opacity"); + self.width = bbox ? bbox.width : _closestTenth(getProp("width", "px"), 0.04); + self.height = bbox ? bbox.height : _closestTenth(getProp("height", "px"), 0.04); + props && _recordProps(self, _memoizedProps[props] || _memoizeProps(props)); + self.ctm = element.getCTM && element.nodeName.toLowerCase() === "svg" && _getCTM(element).inverse(); + self.simple = simple || _round$4(m.a) === 1 && !_round$4(m.b) && !_round$4(m.c) && _round$4(m.d) === 1; + self.uncache = 0; + }; + + return ElementState; + }(); + + var FlipAction = function () { + function FlipAction(vars, batch) { + this.vars = vars; + this.batch = batch; + this.states = []; + this.timeline = batch.timeline; + } + + var _proto3 = FlipAction.prototype; + + _proto3.getStateById = function getStateById(id) { + var i = this.states.length; + + while (i--) { + if (this.states[i].idLookup[id]) { + return this.states[i]; + } + } + }; + + _proto3.kill = function kill() { + this.batch.remove(this); + }; + + return FlipAction; + }(); + + var FlipBatch = function () { + function FlipBatch(id) { + this.id = id; + this.actions = []; + this._kill = []; + this._final = []; + this._abs = []; + this._run = []; + this.data = {}; + this.state = new FlipState(); + this.timeline = gsap$6.timeline(); + } + + var _proto4 = FlipBatch.prototype; + + _proto4.add = function add(config) { + var result = this.actions.filter(function (action) { + return action.vars === config; + }); + + if (result.length) { + return result[0]; + } + + result = new FlipAction(typeof config === "function" ? { + animate: config + } : config, this); + this.actions.push(result); + return result; + }; + + _proto4.remove = function remove(action) { + var i = this.actions.indexOf(action); + i >= 0 && this.actions.splice(i, 1); + return this; + }; + + _proto4.getState = function getState(merge) { + var _this3 = this; + + var prevBatch = _batch, + prevAction = _batchAction; + _batch = this; + this.state.clear(); + this._kill.length = 0; + this.actions.forEach(function (action) { + if (action.vars.getState) { + action.states.length = 0; + _batchAction = action; + action.state = action.vars.getState(action); + } + + merge && action.states.forEach(function (s) { + return _this3.state.add(s); + }); + }); + _batchAction = prevAction; + _batch = prevBatch; + this.killConflicts(); + return this; + }; + + _proto4.animate = function animate() { + var _this4 = this; + + var prevBatch = _batch, + tl = this.timeline, + i = this.actions.length, + finalStates, + endTime; + _batch = this; + tl.clear(); + this._abs.length = this._final.length = this._run.length = 0; + this.actions.forEach(function (a) { + a.vars.animate && a.vars.animate(a); + var onEnter = a.vars.onEnter, + onLeave = a.vars.onLeave, + targets = a.targets, + s, + result; + + if (targets && targets.length && (onEnter || onLeave)) { + s = new FlipState(); + a.states.forEach(function (state) { + return s.add(state); + }); + result = s.compare(Flip.getState(targets)); + result.enter.length && onEnter && onEnter(result.enter); + result.leave.length && onLeave && onLeave(result.leave); + } + }); + + _makeCompsAbsolute(this._abs); + + this._run.forEach(function (f) { + return f(); + }); + + endTime = tl.duration(); + finalStates = this._final.slice(0); + tl.add(function () { + if (endTime <= tl.time()) { + finalStates.forEach(function (f) { + return f(); + }); + + _forEachBatch(_this4, "onComplete"); + } + }); + _batch = prevBatch; + + while (i--) { + this.actions[i].vars.once && this.actions[i].kill(); + } + + _forEachBatch(this, "onStart"); + + tl.restart(); + return this; + }; + + _proto4.loadState = function loadState(done) { + done || (done = function done() { + return 0; + }); + var queue = []; + this.actions.forEach(function (c) { + if (c.vars.loadState) { + var i, + f = function f(targets) { + targets && (c.targets = targets); + i = queue.indexOf(f); + + if (~i) { + queue.splice(i, 1); + queue.length || done(); + } + }; + + queue.push(f); + c.vars.loadState(f); + } + }); + queue.length || done(); + return this; + }; + + _proto4.setState = function setState() { + this.actions.forEach(function (c) { + return c.targets = c.vars.setState && c.vars.setState(c); + }); + return this; + }; + + _proto4.killConflicts = function killConflicts(soft) { + this.state.interrupt(soft); + + this._kill.forEach(function (state) { + return state.interrupt(soft); + }); + + return this; + }; + + _proto4.run = function run(skipGetState, merge) { + var _this5 = this; + + if (this !== _batch) { + skipGetState || this.getState(merge); + this.loadState(function () { + if (!_this5._killed) { + _this5.setState(); + + _this5.animate(); + } + }); + } + + return this; + }; + + _proto4.clear = function clear(stateOnly) { + this.state.clear(); + stateOnly || (this.actions.length = 0); + }; + + _proto4.getStateById = function getStateById(id) { + var i = this.actions.length, + s; + + while (i--) { + s = this.actions[i].getStateById(id); + + if (s) { + return s; + } + } + + return this.state.idLookup[id] && this.state; + }; + + _proto4.kill = function kill() { + this._killed = 1; + this.clear(); + delete _batchLookup[this.id]; + }; + + return FlipBatch; + }(); + + var Flip = function () { + function Flip() {} + + Flip.getState = function getState(targets, vars) { + var state = _parseState(targets, vars); + + _batchAction && _batchAction.states.push(state); + vars && vars.batch && Flip.batch(vars.batch).state.add(state); + return state; + }; + + Flip.from = function from(state, vars) { + vars = vars || {}; + "clearProps" in vars || (vars.clearProps = true); + return _fromTo(state, _parseState(vars.targets || state.targets, { + props: vars.props || state.props, + simple: vars.simple, + kill: !!vars.kill + }), vars, -1); + }; + + Flip.to = function to(state, vars) { + return _fromTo(state, _parseState(vars.targets || state.targets, { + props: vars.props || state.props, + simple: vars.simple, + kill: !!vars.kill + }), vars, 1); + }; + + Flip.fromTo = function fromTo(fromState, toState, vars) { + return _fromTo(fromState, toState, vars); + }; + + Flip.fit = function fit(fromEl, toEl, vars) { + var v = vars ? _copy$1(vars, _fitReserved) : {}, + _ref = vars || v, + absolute = _ref.absolute, + scale = _ref.scale, + getVars = _ref.getVars, + props = _ref.props, + runBackwards = _ref.runBackwards, + onComplete = _ref.onComplete, + simple = _ref.simple, + fitChild = vars && vars.fitChild && _getEl(vars.fitChild), + before = _parseElementState(toEl, props, simple, fromEl), + after = _parseElementState(fromEl, 0, simple, before), + inlineProps = props ? _memoizedRemoveProps[props] : _removeProps, + ctx = gsap$6.context(); + + props && _applyProps(v, before.props); + + _recordInlineStyles(after, inlineProps); + + if (runBackwards) { + "immediateRender" in v || (v.immediateRender = true); + + v.onComplete = function () { + _applyInlineStyles(after); + + onComplete && onComplete.apply(this, arguments); + }; + } + + absolute && _makeAbsolute(after, before); + v = _fit(after, before, scale || fitChild, props, fitChild, v.duration || getVars ? v : 0); + typeof vars === "object" && "zIndex" in vars && (v.zIndex = vars.zIndex); + ctx && !getVars && ctx.add(function () { + return function () { + return _applyInlineStyles(after); + }; + }); + return getVars ? v : v.duration ? gsap$6.to(after.element, v) : null; + }; + + Flip.makeAbsolute = function makeAbsolute(targetsOrStates, vars) { + return (targetsOrStates instanceof FlipState ? targetsOrStates : new FlipState(targetsOrStates, vars)).makeAbsolute(); + }; + + Flip.batch = function batch(id) { + id || (id = "default"); + return _batchLookup[id] || (_batchLookup[id] = new FlipBatch(id)); + }; + + Flip.killFlipsOf = function killFlipsOf(targets, complete) { + (targets instanceof FlipState ? targets.targets : _toArray$1(targets)).forEach(function (t) { + return t && _killFlip(t._flip, complete !== false ? 1 : 2); + }); + }; + + Flip.isFlipping = function isFlipping(target) { + var f = Flip.getByTarget(target); + return !!f && f.isActive(); + }; + + Flip.getByTarget = function getByTarget(target) { + return (_getEl(target) || _emptyObj)._flip; + }; + + Flip.getElementState = function getElementState(target, props) { + return new ElementState(_getEl(target), props); + }; + + Flip.convertCoordinates = function convertCoordinates(fromElement, toElement, point) { + var m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }; + + Flip.register = function register(core) { + _body$2 = typeof document !== "undefined" && document.body; + + if (_body$2) { + gsap$6 = core; + + _setDoc(_body$2); + + _toArray$1 = gsap$6.utils.toArray; + _getStyleSaver$2 = gsap$6.core.getStyleSaver; + var snap = gsap$6.utils.snap(0.1); + + _closestTenth = function _closestTenth(value, add) { + return snap(parseFloat(value) + add); + }; + } + }; + + return Flip; + }(); + Flip.version = "3.12.7"; + typeof window !== "undefined" && window.gsap && window.gsap.registerPlugin(Flip); + + /*! + * MotionPathPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var _xProps = "x,translateX,left,marginLeft,xPercent".split(","), + _yProps = "y,translateY,top,marginTop,yPercent".split(","), + _DEG2RAD$3 = Math.PI / 180, + gsap$7, + PropTween$1, + _getUnit, + _toArray$2, + _getStyleSaver$3, + _reverting$2, + _getGSAP$5 = function _getGSAP() { + return gsap$7 || typeof window !== "undefined" && (gsap$7 = window.gsap) && gsap$7.registerPlugin && gsap$7; + }, + _populateSegmentFromArray = function _populateSegmentFromArray(segment, values, property, mode) { + var l = values.length, + si = mode === 2 ? 0 : mode, + i = 0, + v; + + for (; i < l; i++) { + segment[si] = v = parseFloat(values[i][property]); + mode === 2 && (segment[si + 1] = 0); + si += 2; + } + + return segment; + }, + _getPropNum = function _getPropNum(target, prop, unit) { + return parseFloat(target._gsap.get(target, prop, unit || "px")) || 0; + }, + _relativize = function _relativize(segment) { + var x = segment[0], + y = segment[1], + i; + + for (i = 2; i < segment.length; i += 2) { + x = segment[i] += x; + y = segment[i + 1] += y; + } + }, + _segmentToRawPath = function _segmentToRawPath(plugin, segment, target, x, y, slicer, vars, unitX, unitY) { + if (vars.type === "cubic") { + segment = [segment]; + } else { + vars.fromCurrent !== false && segment.unshift(_getPropNum(target, x, unitX), y ? _getPropNum(target, y, unitY) : 0); + vars.relative && _relativize(segment); + var pointFunc = y ? pointsToSegment : flatPointsToSegment; + segment = [pointFunc(segment, vars.curviness)]; + } + + segment = slicer(_align(segment, target, vars)); + + _addDimensionalPropTween(plugin, target, x, segment, "x", unitX); + + y && _addDimensionalPropTween(plugin, target, y, segment, "y", unitY); + return cacheRawPathMeasurements(segment, vars.resolution || (vars.curviness === 0 ? 20 : 12)); + }, + _emptyFunc$2 = function _emptyFunc(v) { + return v; + }, + _numExp$2 = /[-+\.]*\d+\.?(?:e-|e\+)?\d*/g, + _originToPoint = function _originToPoint(element, origin, parentMatrix) { + var m = getGlobalMatrix(element), + x = 0, + y = 0, + svg; + + if ((element.tagName + "").toLowerCase() === "svg") { + svg = element.viewBox.baseVal; + svg.width || (svg = { + width: +element.getAttribute("width"), + height: +element.getAttribute("height") + }); + } else { + svg = origin && element.getBBox && element.getBBox(); + } + + if (origin && origin !== "auto") { + x = origin.push ? origin[0] * (svg ? svg.width : element.offsetWidth || 0) : origin.x; + y = origin.push ? origin[1] * (svg ? svg.height : element.offsetHeight || 0) : origin.y; + } + + return parentMatrix.apply(x || y ? m.apply({ + x: x, + y: y + }) : { + x: m.e, + y: m.f + }); + }, + _getAlignMatrix = function _getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin) { + var parentMatrix = getGlobalMatrix(fromElement.parentNode, true, true), + m = parentMatrix.clone().multiply(getGlobalMatrix(toElement)), + fromPoint = _originToPoint(fromElement, fromOrigin, parentMatrix), + _originToPoint2 = _originToPoint(toElement, toOrigin, parentMatrix), + x = _originToPoint2.x, + y = _originToPoint2.y, + p; + + m.e = m.f = 0; + + if (toOrigin === "auto" && toElement.getTotalLength && toElement.tagName.toLowerCase() === "path") { + p = toElement.getAttribute("d").match(_numExp$2) || []; + p = m.apply({ + x: +p[0], + y: +p[1] + }); + x += p.x; + y += p.y; + } + + if (p) { + p = m.apply(toElement.getBBox()); + x -= p.x; + y -= p.y; + } + + m.e = x - fromPoint.x; + m.f = y - fromPoint.y; + return m; + }, + _align = function _align(rawPath, target, _ref) { + var align = _ref.align, + matrix = _ref.matrix, + offsetX = _ref.offsetX, + offsetY = _ref.offsetY, + alignOrigin = _ref.alignOrigin; + + var x = rawPath[0][0], + y = rawPath[0][1], + curX = _getPropNum(target, "x"), + curY = _getPropNum(target, "y"), + alignTarget, + m, + p; + + if (!rawPath || !rawPath.length) { + return getRawPath("M0,0L0,0"); + } + + if (align) { + if (align === "self" || (alignTarget = _toArray$2(align)[0] || target) === target) { + transformRawPath(rawPath, 1, 0, 0, 1, curX - x, curY - y); + } else { + if (alignOrigin && alignOrigin[2] !== false) { + gsap$7.set(target, { + transformOrigin: alignOrigin[0] * 100 + "% " + alignOrigin[1] * 100 + "%" + }); + } else { + alignOrigin = [_getPropNum(target, "xPercent") / -100, _getPropNum(target, "yPercent") / -100]; + } + + m = _getAlignMatrix(target, alignTarget, alignOrigin, "auto"); + p = m.apply({ + x: x, + y: y + }); + transformRawPath(rawPath, m.a, m.b, m.c, m.d, curX + m.e - (p.x - m.e), curY + m.f - (p.y - m.f)); + } + } + + if (matrix) { + transformRawPath(rawPath, matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f); + } else if (offsetX || offsetY) { + transformRawPath(rawPath, 1, 0, 0, 1, offsetX || 0, offsetY || 0); + } + + return rawPath; + }, + _addDimensionalPropTween = function _addDimensionalPropTween(plugin, target, property, rawPath, pathProperty, forceUnit) { + var cache = target._gsap, + harness = cache.harness, + alias = harness && harness.aliases && harness.aliases[property], + prop = alias && alias.indexOf(",") < 0 ? alias : property, + pt = plugin._pt = new PropTween$1(plugin._pt, target, prop, 0, 0, _emptyFunc$2, 0, cache.set(target, prop, plugin)); + pt.u = _getUnit(cache.get(target, prop, forceUnit)) || 0; + pt.path = rawPath; + pt.pp = pathProperty; + + plugin._props.push(prop); + }, + _sliceModifier = function _sliceModifier(start, end) { + return function (rawPath) { + return start || end !== 1 ? sliceRawPath(rawPath, start, end) : rawPath; + }; + }; + + var MotionPathPlugin = { + version: "3.12.7", + name: "motionPath", + register: function register(core, Plugin, propTween) { + gsap$7 = core; + _getUnit = gsap$7.utils.getUnit; + _toArray$2 = gsap$7.utils.toArray; + _getStyleSaver$3 = gsap$7.core.getStyleSaver; + + _reverting$2 = gsap$7.core.reverting || function () {}; + + PropTween$1 = propTween; + }, + init: function init(target, vars, tween) { + if (!gsap$7) { + console.warn("Please gsap.registerPlugin(MotionPathPlugin)"); + return false; + } + + if (!(typeof vars === "object" && !vars.style) || !vars.path) { + vars = { + path: vars + }; + } + + var rawPaths = [], + _vars = vars, + path = _vars.path, + autoRotate = _vars.autoRotate, + unitX = _vars.unitX, + unitY = _vars.unitY, + x = _vars.x, + y = _vars.y, + firstObj = path[0], + slicer = _sliceModifier(vars.start, "end" in vars ? vars.end : 1), + rawPath, + p; + + this.rawPaths = rawPaths; + this.target = target; + this.tween = tween; + this.styles = _getStyleSaver$3 && _getStyleSaver$3(target, "transform"); + + if (this.rotate = autoRotate || autoRotate === 0) { + this.rOffset = parseFloat(autoRotate) || 0; + this.radians = !!vars.useRadians; + this.rProp = vars.rotation || "rotation"; + this.rSet = target._gsap.set(target, this.rProp, this); + this.ru = _getUnit(target._gsap.get(target, this.rProp)) || 0; + } + + if (Array.isArray(path) && !("closed" in path) && typeof firstObj !== "number") { + for (p in firstObj) { + if (!x && ~_xProps.indexOf(p)) { + x = p; + } else if (!y && ~_yProps.indexOf(p)) { + y = p; + } + } + + if (x && y) { + rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray(_populateSegmentFromArray([], path, x, 0), path, y, 1), target, x, y, slicer, vars, unitX || _getUnit(path[0][x]), unitY || _getUnit(path[0][y]))); + } else { + x = y = 0; + } + + for (p in firstObj) { + p !== x && p !== y && rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray([], path, p, 2), target, p, 0, slicer, vars, _getUnit(path[0][p]))); + } + } else { + rawPath = slicer(_align(getRawPath(vars.path), target, vars)); + cacheRawPathMeasurements(rawPath, vars.resolution); + rawPaths.push(rawPath); + + _addDimensionalPropTween(this, target, vars.x || "x", rawPath, "x", vars.unitX || "px"); + + _addDimensionalPropTween(this, target, vars.y || "y", rawPath, "y", vars.unitY || "px"); + } + + tween.vars.immediateRender && this.render(tween.progress(), this); + }, + render: function render(ratio, data) { + var rawPaths = data.rawPaths, + i = rawPaths.length, + pt = data._pt; + + if (data.tween._time || !_reverting$2()) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + + while (i--) { + getPositionOnPath(rawPaths[i], ratio, !i && data.rotate, rawPaths[i]); + } + + while (pt) { + pt.set(pt.t, pt.p, pt.path[pt.pp] + pt.u, pt.d, ratio); + pt = pt._next; + } + + data.rotate && data.rSet(data.target, data.rProp, rawPaths[0].angle * (data.radians ? _DEG2RAD$3 : 1) + data.rOffset + data.ru, data, ratio); + } else { + data.styles.revert(); + } + }, + getLength: function getLength(path) { + return cacheRawPathMeasurements(getRawPath(path)).totalLength; + }, + sliceRawPath: sliceRawPath, + getRawPath: getRawPath, + pointsToSegment: pointsToSegment, + stringToRawPath: stringToRawPath, + rawPathToString: rawPathToString, + transformRawPath: transformRawPath, + getGlobalMatrix: getGlobalMatrix, + getPositionOnPath: getPositionOnPath, + cacheRawPathMeasurements: cacheRawPathMeasurements, + convertToPath: function convertToPath$1(targets, swap) { + return _toArray$2(targets).map(function (target) { + return convertToPath(target, swap !== false); + }); + }, + convertCoordinates: function convertCoordinates(fromElement, toElement, point) { + var m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }, + getAlignMatrix: _getAlignMatrix, + getRelativePosition: function getRelativePosition(fromElement, toElement, fromOrigin, toOrigin) { + var m = _getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin); + + return { + x: m.e, + y: m.f + }; + }, + arrayToRawPath: function arrayToRawPath(value, vars) { + vars = vars || {}; + + var segment = _populateSegmentFromArray(_populateSegmentFromArray([], value, vars.x || "x", 0), value, vars.y || "y", 1); + + vars.relative && _relativize(segment); + return [vars.type === "cubic" ? segment : pointsToSegment(segment, vars.curviness)]; + } + }; + _getGSAP$5() && gsap$7.registerPlugin(MotionPathPlugin); + + /*! + * Observer 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap$8, + _coreInitted$5, + _clamp$1, + _win$5, + _doc$5, + _docEl, + _body$3, + _isTouch, + _pointerType, + ScrollTrigger, + _root, + _normalizer, + _eventTypes, + _context$2, + _getGSAP$6 = function _getGSAP() { + return gsap$8 || typeof window !== "undefined" && (gsap$8 = window.gsap) && gsap$8.registerPlugin && gsap$8; + }, + _startup = 1, + _observers = []; + exports._scrollers = []; + exports._proxies = []; + var _getTime$1 = Date.now, + _bridge = function _bridge(name, value) { + return value; + }, + _integrate = function _integrate() { + var core = ScrollTrigger.core, + data = core.bridge || {}, + scrollers = core._scrollers, + proxies = core._proxies; + scrollers.push.apply(scrollers, exports._scrollers); + proxies.push.apply(proxies, exports._proxies); + exports._scrollers = scrollers; + exports._proxies = proxies; + + _bridge = function _bridge(name, value) { + return data[name](value); + }; + }, + _getProxyProp = function _getProxyProp(element, property) { + return ~exports._proxies.indexOf(element) && exports._proxies[exports._proxies.indexOf(element) + 1][property]; + }, + _isViewport = function _isViewport(el) { + return !!~_root.indexOf(el); + }, + _addListener$1 = function _addListener(element, type, func, passive, capture) { + return element.addEventListener(type, func, { + passive: passive !== false, + capture: !!capture + }); + }, + _removeListener$1 = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); + }, + _scrollLeft = "scrollLeft", + _scrollTop = "scrollTop", + _onScroll = function _onScroll() { + return _normalizer && _normalizer.isPressed || exports._scrollers.cache++; + }, + _scrollCacheFunc = function _scrollCacheFunc(f, doNotCache) { + var cachingFunc = function cachingFunc(value) { + if (value || value === 0) { + _startup && (_win$5.history.scrollRestoration = "manual"); + var isNormalizing = _normalizer && _normalizer.isPressed; + value = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); + f(value); + cachingFunc.cacheID = exports._scrollers.cache; + isNormalizing && _bridge("ss", value); + } else if (doNotCache || exports._scrollers.cache !== cachingFunc.cacheID || _bridge("ref")) { + cachingFunc.cacheID = exports._scrollers.cache; + cachingFunc.v = f(); + } + + return cachingFunc.v + cachingFunc.offset; + }; + + cachingFunc.offset = 0; + return f && cachingFunc; + }, + _horizontal = { + s: _scrollLeft, + p: "left", + p2: "Left", + os: "right", + os2: "Right", + d: "width", + d2: "Width", + a: "x", + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win$5.scrollTo(value, _vertical.sc()) : _win$5.pageXOffset || _doc$5[_scrollLeft] || _docEl[_scrollLeft] || _body$3[_scrollLeft] || 0; + }) + }, + _vertical = { + s: _scrollTop, + p: "top", + p2: "Top", + os: "bottom", + os2: "Bottom", + d: "height", + d2: "Height", + a: "y", + op: _horizontal, + sc: _scrollCacheFunc(function (value) { + return arguments.length ? _win$5.scrollTo(_horizontal.sc(), value) : _win$5.pageYOffset || _doc$5[_scrollTop] || _docEl[_scrollTop] || _body$3[_scrollTop] || 0; + }) + }, + _getTarget = function _getTarget(t, self) { + return (self && self._ctx && self._ctx.selector || gsap$8.utils.toArray)(t)[0] || (typeof t === "string" && gsap$8.config().nullTargetWarn !== false ? console.warn("Element not found:", t) : null); + }, + _getScrollFunc = function _getScrollFunc(element, _ref) { + var s = _ref.s, + sc = _ref.sc; + _isViewport(element) && (element = _doc$5.scrollingElement || _docEl); + + var i = exports._scrollers.indexOf(element), + offset = sc === _vertical.sc ? 1 : 2; + + !~i && (i = exports._scrollers.push(element) - 1); + exports._scrollers[i + offset] || _addListener$1(element, "scroll", _onScroll); + var prev = exports._scrollers[i + offset], + func = prev || (exports._scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function (value) { + return arguments.length ? element[s] = value : element[s]; + }))); + func.target = element; + prev || (func.smooth = gsap$8.getProperty(element, "scrollBehavior") === "smooth"); + return func; + }, + _getVelocityProp = function _getVelocityProp(value, minTimeRefresh, useDelta) { + var v1 = value, + v2 = value, + t1 = _getTime$1(), + t2 = t1, + min = minTimeRefresh || 50, + dropToZeroTime = Math.max(500, min * 3), + update = function update(value, force) { + var t = _getTime$1(); + + if (force || t - t1 > min) { + v2 = v1; + v1 = value; + t2 = t1; + t1 = t; + } else if (useDelta) { + v1 += value; + } else { + v1 = v2 + (value - v2) / (t - t2) * (t1 - t2); + } + }, + reset = function reset() { + v2 = v1 = useDelta ? 0 : v1; + t2 = t1 = 0; + }, + getVelocity = function getVelocity(latestValue) { + var tOld = t2, + vOld = v2, + t = _getTime$1(); + + (latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue); + return t1 === t2 || t - t2 > dropToZeroTime ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000; + }; + + return { + update: update, + reset: reset, + getVelocity: getVelocity + }; + }, + _getEvent = function _getEvent(e, preventDefault) { + preventDefault && !e._gsapAllow && e.preventDefault(); + return e.changedTouches ? e.changedTouches[0] : e; + }, + _getAbsoluteMax = function _getAbsoluteMax(a) { + var max = Math.max.apply(Math, a), + min = Math.min.apply(Math, a); + return Math.abs(max) >= Math.abs(min) ? max : min; + }, + _setScrollTrigger = function _setScrollTrigger() { + ScrollTrigger = gsap$8.core.globals().ScrollTrigger; + ScrollTrigger && ScrollTrigger.core && _integrate(); + }, + _initCore$6 = function _initCore(core) { + gsap$8 = core || _getGSAP$6(); + + if (!_coreInitted$5 && gsap$8 && typeof document !== "undefined" && document.body) { + _win$5 = window; + _doc$5 = document; + _docEl = _doc$5.documentElement; + _body$3 = _doc$5.body; + _root = [_win$5, _doc$5, _docEl, _body$3]; + _clamp$1 = gsap$8.utils.clamp; + + _context$2 = gsap$8.core.context || function () {}; + + _pointerType = "onpointerenter" in _body$3 ? "pointer" : "mouse"; + _isTouch = Observer.isTouch = _win$5.matchMedia && _win$5.matchMedia("(hover: none), (pointer: coarse)").matches ? 1 : "ontouchstart" in _win$5 || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0 ? 2 : 0; + _eventTypes = Observer.eventTypes = ("ontouchstart" in _docEl ? "touchstart,touchmove,touchcancel,touchend" : !("onpointerdown" in _docEl) ? "mousedown,mousemove,mouseup,mouseup" : "pointerdown,pointermove,pointercancel,pointerup").split(","); + setTimeout(function () { + return _startup = 0; + }, 500); + + _setScrollTrigger(); + + _coreInitted$5 = 1; + } + + return _coreInitted$5; + }; + + _horizontal.op = _vertical; + exports._scrollers.cache = 0; + var Observer = function () { + function Observer(vars) { + this.init(vars); + } + + var _proto = Observer.prototype; + + _proto.init = function init(vars) { + _coreInitted$5 || _initCore$6(gsap$8) || console.warn("Please gsap.registerPlugin(Observer)"); + ScrollTrigger || _setScrollTrigger(); + var tolerance = vars.tolerance, + dragMinimum = vars.dragMinimum, + type = vars.type, + target = vars.target, + lineHeight = vars.lineHeight, + debounce = vars.debounce, + preventDefault = vars.preventDefault, + onStop = vars.onStop, + onStopDelay = vars.onStopDelay, + ignore = vars.ignore, + wheelSpeed = vars.wheelSpeed, + event = vars.event, + onDragStart = vars.onDragStart, + onDragEnd = vars.onDragEnd, + onDrag = vars.onDrag, + onPress = vars.onPress, + onRelease = vars.onRelease, + onRight = vars.onRight, + onLeft = vars.onLeft, + onUp = vars.onUp, + onDown = vars.onDown, + onChangeX = vars.onChangeX, + onChangeY = vars.onChangeY, + onChange = vars.onChange, + onToggleX = vars.onToggleX, + onToggleY = vars.onToggleY, + onHover = vars.onHover, + onHoverEnd = vars.onHoverEnd, + onMove = vars.onMove, + ignoreCheck = vars.ignoreCheck, + isNormalizer = vars.isNormalizer, + onGestureStart = vars.onGestureStart, + onGestureEnd = vars.onGestureEnd, + onWheel = vars.onWheel, + onEnable = vars.onEnable, + onDisable = vars.onDisable, + onClick = vars.onClick, + scrollSpeed = vars.scrollSpeed, + capture = vars.capture, + allowClicks = vars.allowClicks, + lockAxis = vars.lockAxis, + onLockAxis = vars.onLockAxis; + this.target = target = _getTarget(target) || _docEl; + this.vars = vars; + ignore && (ignore = gsap$8.utils.toArray(ignore)); + tolerance = tolerance || 1e-9; + dragMinimum = dragMinimum || 0; + wheelSpeed = wheelSpeed || 1; + scrollSpeed = scrollSpeed || 1; + type = type || "wheel,touch,pointer"; + debounce = debounce !== false; + lineHeight || (lineHeight = parseFloat(_win$5.getComputedStyle(_body$3).lineHeight) || 22); + + var id, + onStopDelayedCall, + dragged, + moved, + wheeled, + locked, + axis, + self = this, + prevDeltaX = 0, + prevDeltaY = 0, + passive = vars.passive || !preventDefault && vars.passive !== false, + scrollFuncX = _getScrollFunc(target, _horizontal), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollX = scrollFuncX(), + scrollY = scrollFuncY(), + limitToTouch = ~type.indexOf("touch") && !~type.indexOf("pointer") && _eventTypes[0] === "pointerdown", + isViewport = _isViewport(target), + ownerDoc = target.ownerDocument || _doc$5, + deltaX = [0, 0, 0], + deltaY = [0, 0, 0], + onClickTime = 0, + clickCapture = function clickCapture() { + return onClickTime = _getTime$1(); + }, + _ignoreCheck = function _ignoreCheck(e, isPointerOrTouch) { + return (self.event = e) && ignore && ~ignore.indexOf(e.target) || isPointerOrTouch && limitToTouch && e.pointerType !== "touch" || ignoreCheck && ignoreCheck(e, isPointerOrTouch); + }, + onStopFunc = function onStopFunc() { + self._vx.reset(); + + self._vy.reset(); + + onStopDelayedCall.pause(); + onStop && onStop(self); + }, + update = function update() { + var dx = self.deltaX = _getAbsoluteMax(deltaX), + dy = self.deltaY = _getAbsoluteMax(deltaY), + changedX = Math.abs(dx) >= tolerance, + changedY = Math.abs(dy) >= tolerance; + + onChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); + + if (changedX) { + onRight && self.deltaX > 0 && onRight(self); + onLeft && self.deltaX < 0 && onLeft(self); + onChangeX && onChangeX(self); + onToggleX && self.deltaX < 0 !== prevDeltaX < 0 && onToggleX(self); + prevDeltaX = self.deltaX; + deltaX[0] = deltaX[1] = deltaX[2] = 0; + } + + if (changedY) { + onDown && self.deltaY > 0 && onDown(self); + onUp && self.deltaY < 0 && onUp(self); + onChangeY && onChangeY(self); + onToggleY && self.deltaY < 0 !== prevDeltaY < 0 && onToggleY(self); + prevDeltaY = self.deltaY; + deltaY[0] = deltaY[1] = deltaY[2] = 0; + } + + if (moved || dragged) { + onMove && onMove(self); + + if (dragged) { + onDragStart && dragged === 1 && onDragStart(self); + onDrag && onDrag(self); + dragged = 0; + } + + moved = false; + } + + locked && !(locked = false) && onLockAxis && onLockAxis(self); + + if (wheeled) { + onWheel(self); + wheeled = false; + } + + id = 0; + }, + onDelta = function onDelta(x, y, index) { + deltaX[index] += x; + deltaY[index] += y; + + self._vx.update(x); + + self._vy.update(y); + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + onTouchOrPointerDelta = function onTouchOrPointerDelta(x, y) { + if (lockAxis && !axis) { + self.axis = axis = Math.abs(x) > Math.abs(y) ? "x" : "y"; + locked = true; + } + + if (axis !== "y") { + deltaX[2] += x; + + self._vx.update(x, true); + } + + if (axis !== "x") { + deltaY[2] += y; + + self._vy.update(y, true); + } + + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + _onDrag = function _onDrag(e) { + if (_ignoreCheck(e, 1)) { + return; + } + + e = _getEvent(e, preventDefault); + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y, + isDragging = self.isDragging; + self.x = x; + self.y = y; + + if (isDragging || (dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum)) { + dragged = isDragging ? 2 : 1; + isDragging || (self.isDragging = true); + onTouchOrPointerDelta(dx, dy); + } + }, + _onPress = self.onPress = function (e) { + if (_ignoreCheck(e, 1) || e && e.button) { + return; + } + + self.axis = axis = null; + onStopDelayedCall.pause(); + self.isPressed = true; + e = _getEvent(e); + prevDeltaX = prevDeltaY = 0; + self.startX = self.x = e.clientX; + self.startY = self.y = e.clientY; + + self._vx.reset(); + + self._vy.reset(); + + _addListener$1(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true); + + self.deltaX = self.deltaY = 0; + onPress && onPress(self); + }, + _onRelease = self.onRelease = function (e) { + if (_ignoreCheck(e, 1)) { + return; + } + + _removeListener$1(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + + var isTrackingDrag = !isNaN(self.y - self.startY), + wasDragging = self.isDragging, + isDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), + eventData = _getEvent(e); + + if (!isDragNotClick && isTrackingDrag) { + self._vx.reset(); + + self._vy.reset(); + + if (preventDefault && allowClicks) { + gsap$8.delayedCall(0.08, function () { + if (_getTime$1() - onClickTime > 300 && !e.defaultPrevented) { + if (e.target.click) { + e.target.click(); + } else if (ownerDoc.createEvent) { + var syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win$5, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null); + e.target.dispatchEvent(syntheticEvent); + } + } + }); + } + } + + self.isDragging = self.isGesturing = self.isPressed = false; + onStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true); + dragged && update(); + onDragEnd && wasDragging && onDragEnd(self); + onRelease && onRelease(self, isDragNotClick); + }, + _onGestureStart = function _onGestureStart(e) { + return e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging); + }, + _onGestureEnd = function _onGestureEnd() { + return (self.isGesturing = false) || onGestureEnd(self); + }, + onScroll = function onScroll(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = scrollFuncX(), + y = scrollFuncY(); + onDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1); + scrollX = x; + scrollY = y; + onStop && onStopDelayedCall.restart(true); + }, + _onWheel = function _onWheel(e) { + if (_ignoreCheck(e)) { + return; + } + + e = _getEvent(e, preventDefault); + onWheel && (wheeled = true); + var multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win$5.innerHeight : 1) * wheelSpeed; + onDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0); + onStop && !isNormalizer && onStopDelayedCall.restart(true); + }, + _onMove = function _onMove(e) { + if (_ignoreCheck(e)) { + return; + } + + var x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y; + self.x = x; + self.y = y; + moved = true; + onStop && onStopDelayedCall.restart(true); + (dx || dy) && onTouchOrPointerDelta(dx, dy); + }, + _onHover = function _onHover(e) { + self.event = e; + onHover(self); + }, + _onHoverEnd = function _onHoverEnd(e) { + self.event = e; + onHoverEnd(self); + }, + _onClick = function _onClick(e) { + return _ignoreCheck(e) || _getEvent(e, preventDefault) && onClick(self); + }; + + onStopDelayedCall = self._dc = gsap$8.delayedCall(onStopDelay || 0.25, onStopFunc).pause(); + self.deltaX = self.deltaY = 0; + self._vx = _getVelocityProp(0, 50, true); + self._vy = _getVelocityProp(0, 50, true); + self.scrollX = scrollFuncX; + self.scrollY = scrollFuncY; + self.isDragging = self.isGesturing = self.isPressed = false; + + _context$2(this); + + self.enable = function (e) { + if (!self.isEnabled) { + _addListener$1(isViewport ? ownerDoc : target, "scroll", _onScroll); + + type.indexOf("scroll") >= 0 && _addListener$1(isViewport ? ownerDoc : target, "scroll", onScroll, passive, capture); + type.indexOf("wheel") >= 0 && _addListener$1(target, "wheel", _onWheel, passive, capture); + + if (type.indexOf("touch") >= 0 && _isTouch || type.indexOf("pointer") >= 0) { + _addListener$1(target, _eventTypes[0], _onPress, passive, capture); + + _addListener$1(ownerDoc, _eventTypes[2], _onRelease); + + _addListener$1(ownerDoc, _eventTypes[3], _onRelease); + + allowClicks && _addListener$1(target, "click", clickCapture, true, true); + onClick && _addListener$1(target, "click", _onClick); + onGestureStart && _addListener$1(ownerDoc, "gesturestart", _onGestureStart); + onGestureEnd && _addListener$1(ownerDoc, "gestureend", _onGestureEnd); + onHover && _addListener$1(target, _pointerType + "enter", _onHover); + onHoverEnd && _addListener$1(target, _pointerType + "leave", _onHoverEnd); + onMove && _addListener$1(target, _pointerType + "move", _onMove); + } + + self.isEnabled = true; + self.isDragging = self.isGesturing = self.isPressed = moved = dragged = false; + + self._vx.reset(); + + self._vy.reset(); + + scrollX = scrollFuncX(); + scrollY = scrollFuncY(); + e && e.type && _onPress(e); + onEnable && onEnable(self); + } + + return self; + }; + + self.disable = function () { + if (self.isEnabled) { + _observers.filter(function (o) { + return o !== self && _isViewport(o.target); + }).length || _removeListener$1(isViewport ? ownerDoc : target, "scroll", _onScroll); + + if (self.isPressed) { + self._vx.reset(); + + self._vy.reset(); + + _removeListener$1(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + } + + _removeListener$1(isViewport ? ownerDoc : target, "scroll", onScroll, capture); + + _removeListener$1(target, "wheel", _onWheel, capture); + + _removeListener$1(target, _eventTypes[0], _onPress, capture); + + _removeListener$1(ownerDoc, _eventTypes[2], _onRelease); + + _removeListener$1(ownerDoc, _eventTypes[3], _onRelease); + + _removeListener$1(target, "click", clickCapture, true); + + _removeListener$1(target, "click", _onClick); + + _removeListener$1(ownerDoc, "gesturestart", _onGestureStart); + + _removeListener$1(ownerDoc, "gestureend", _onGestureEnd); + + _removeListener$1(target, _pointerType + "enter", _onHover); + + _removeListener$1(target, _pointerType + "leave", _onHoverEnd); + + _removeListener$1(target, _pointerType + "move", _onMove); + + self.isEnabled = self.isPressed = self.isDragging = false; + onDisable && onDisable(self); + } + }; + + self.kill = self.revert = function () { + self.disable(); + + var i = _observers.indexOf(self); + + i >= 0 && _observers.splice(i, 1); + _normalizer === self && (_normalizer = 0); + }; + + _observers.push(self); + + isNormalizer && _isViewport(target) && (_normalizer = self); + self.enable(event); + }; + + _createClass(Observer, [{ + key: "velocityX", + get: function get() { + return this._vx.getVelocity(); + } + }, { + key: "velocityY", + get: function get() { + return this._vy.getVelocity(); + } + }]); + + return Observer; + }(); + Observer.version = "3.12.7"; + + Observer.create = function (vars) { + return new Observer(vars); + }; + + Observer.register = _initCore$6; + + Observer.getAll = function () { + return _observers.slice(); + }; + + Observer.getById = function (id) { + return _observers.filter(function (o) { + return o.vars.id === id; + })[0]; + }; + + _getGSAP$6() && gsap$8.registerPlugin(Observer); + + /*! + * PixiPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap$9, + _splitColor, + _coreInitted$6, + _PIXI, + PropTween$2, + _getSetter$1, + _isV4, + _isV8Plus, + _windowExists$5 = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$7 = function _getGSAP() { + return gsap$9 || _windowExists$5() && (gsap$9 = window.gsap) && gsap$9.registerPlugin && gsap$9; + }, + _isFunction$2 = function _isFunction(value) { + return typeof value === "function"; + }, + _warn$2 = function _warn(message) { + return console.warn(message); + }, + _idMatrix$1 = [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0], + _lumR$1 = 0.212671, + _lumG$1 = 0.715160, + _lumB$1 = 0.072169, + _filterClass = function _filterClass(name) { + return _isFunction$2(_PIXI[name]) ? _PIXI[name] : _PIXI.filters[name]; + }, + _applyMatrix$1 = function _applyMatrix(m, m2) { + var temp = [], + i = 0, + z = 0, + y, + x; + + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = x === 4 ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i + 1] * m2[x + 5] + m[i + 2] * m2[x + 10] + m[i + 3] * m2[x + 15] + z; + } + + i += 5; + } + + return temp; + }, + _setSaturation$1 = function _setSaturation(m, n) { + var inv = 1 - n, + r = inv * _lumR$1, + g = inv * _lumG$1, + b = inv * _lumB$1; + return _applyMatrix$1([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); + }, + _colorize$1 = function _colorize(m, color, amount) { + var c = _splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + + return _applyMatrix$1([inv + amount * r * _lumR$1, amount * r * _lumG$1, amount * r * _lumB$1, 0, 0, amount * g * _lumR$1, inv + amount * g * _lumG$1, amount * g * _lumB$1, 0, 0, amount * b * _lumR$1, amount * b * _lumG$1, inv + amount * b * _lumB$1, 0, 0, 0, 0, 0, 1, 0], m); + }, + _setHue$1 = function _setHue(m, n) { + n *= Math.PI / 180; + var c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix$1([_lumR$1 + c * (1 - _lumR$1) + s * -_lumR$1, _lumG$1 + c * -_lumG$1 + s * -_lumG$1, _lumB$1 + c * -_lumB$1 + s * (1 - _lumB$1), 0, 0, _lumR$1 + c * -_lumR$1 + s * 0.143, _lumG$1 + c * (1 - _lumG$1) + s * 0.14, _lumB$1 + c * -_lumB$1 + s * -0.283, 0, 0, _lumR$1 + c * -_lumR$1 + s * -(1 - _lumR$1), _lumG$1 + c * -_lumG$1 + s * _lumG$1, _lumB$1 + c * (1 - _lumB$1) + s * _lumB$1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); + }, + _setContrast$1 = function _setContrast(m, n) { + return _applyMatrix$1([n, 0, 0, 0, 0.5 * (1 - n), 0, n, 0, 0, 0.5 * (1 - n), 0, 0, n, 0, 0.5 * (1 - n), 0, 0, 0, 1, 0], m); + }, + _getFilter = function _getFilter(target, type) { + var filterClass = _filterClass(type), + filters = target.filters || [], + i = filters.length, + filter; + + filterClass || _warn$2(type + " not found. PixiPlugin.registerPIXI(PIXI)"); + + while (--i > -1) { + if (filters[i] instanceof filterClass) { + return filters[i]; + } + } + + filter = new filterClass(); + + if (type === "BlurFilter") { + filter.blur = 0; + } + + filters.push(filter); + target.filters = filters; + return filter; + }, + _addColorMatrixFilterCacheTween = function _addColorMatrixFilterCacheTween(p, plugin, cache, vars) { + plugin.add(cache, p, cache[p], vars[p]); + + plugin._props.push(p); + }, + _applyBrightnessToMatrix = function _applyBrightnessToMatrix(brightness, matrix) { + var filterClass = _filterClass("ColorMatrixFilter"), + temp = new filterClass(); + + temp.matrix = matrix; + temp.brightness(brightness, true); + return temp.matrix; + }, + _copy$2 = function _copy(obj) { + var copy = {}, + p; + + for (p in obj) { + copy[p] = obj[p]; + } + + return copy; + }, + _CMFdefaults = { + contrast: 1, + saturation: 1, + colorizeAmount: 0, + colorize: "rgb(255,255,255)", + hue: 0, + brightness: 1 + }, + _parseColorMatrixFilter$1 = function _parseColorMatrixFilter(target, v, pg) { + var filter = _getFilter(target, "ColorMatrixFilter"), + cache = target._gsColorMatrixFilter = target._gsColorMatrixFilter || _copy$2(_CMFdefaults), + combine = v.combineCMF && !("colorMatrixFilter" in v && !v.colorMatrixFilter), + i, + matrix, + startMatrix; + + startMatrix = filter.matrix; + + if (v.resolution) { + filter.resolution = v.resolution; + } + + if (v.matrix && v.matrix.length === startMatrix.length) { + matrix = v.matrix; + + if (cache.contrast !== 1) { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + + if (cache.hue) { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + + if (cache.brightness !== 1) { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + + if (cache.colorizeAmount) { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + + if (cache.saturation !== 1) { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } else { + matrix = _idMatrix$1.slice(); + + if (v.contrast != null) { + matrix = _setContrast$1(matrix, +v.contrast); + + _addColorMatrixFilterCacheTween("contrast", pg, cache, v); + } else if (cache.contrast !== 1) { + if (combine) { + matrix = _setContrast$1(matrix, cache.contrast); + } else { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + } + + if (v.hue != null) { + matrix = _setHue$1(matrix, +v.hue); + + _addColorMatrixFilterCacheTween("hue", pg, cache, v); + } else if (cache.hue) { + if (combine) { + matrix = _setHue$1(matrix, cache.hue); + } else { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + } + + if (v.brightness != null) { + matrix = _applyBrightnessToMatrix(+v.brightness, matrix); + + _addColorMatrixFilterCacheTween("brightness", pg, cache, v); + } else if (cache.brightness !== 1) { + if (combine) { + matrix = _applyBrightnessToMatrix(cache.brightness, matrix); + } else { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + } + + if (v.colorize != null) { + v.colorizeAmount = "colorizeAmount" in v ? +v.colorizeAmount : 1; + matrix = _colorize$1(matrix, v.colorize, v.colorizeAmount); + + _addColorMatrixFilterCacheTween("colorize", pg, cache, v); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, v); + } else if (cache.colorizeAmount) { + if (combine) { + matrix = _colorize$1(matrix, cache.colorize, cache.colorizeAmount); + } else { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + } + + if (v.saturation != null) { + matrix = _setSaturation$1(matrix, +v.saturation); + + _addColorMatrixFilterCacheTween("saturation", pg, cache, v); + } else if (cache.saturation !== 1) { + if (combine) { + matrix = _setSaturation$1(matrix, cache.saturation); + } else { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } + } + + i = matrix.length; + + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg.add(startMatrix, i, startMatrix[i], matrix[i], "colorMatrixFilter"); + } + } + + pg._props.push("colorMatrixFilter"); + }, + _renderColor = function _renderColor(ratio, _ref) { + var t = _ref.t, + p = _ref.p, + color = _ref.color, + set = _ref.set; + set(t, p, color[0] << 16 | color[1] << 8 | color[2]); + }, + _renderDirtyCache = function _renderDirtyCache(ratio, _ref2) { + var g = _ref2.g; + + if (_isV8Plus) { + g.fill(); + g.stroke(); + } else if (g) { + g.dirty++; + g.clearDirty++; + } + }, + _renderAutoAlpha = function _renderAutoAlpha(ratio, data) { + data.t.visible = !!data.t.alpha; + }, + _addColorTween = function _addColorTween(target, p, value, plugin) { + var currentValue = target[p], + startColor = _splitColor(_isFunction$2(currentValue) ? target[p.indexOf("set") || !_isFunction$2(target["get" + p.substr(3)]) ? p : "get" + p.substr(3)]() : currentValue), + endColor = _splitColor(value); + + plugin._pt = new PropTween$2(plugin._pt, target, p, 0, 0, _renderColor, { + t: target, + p: p, + color: startColor, + set: _getSetter$1(target, p) + }); + plugin.add(startColor, 0, startColor[0], endColor[0]); + plugin.add(startColor, 1, startColor[1], endColor[1]); + plugin.add(startColor, 2, startColor[2], endColor[2]); + }, + _colorProps$1 = { + tint: 1, + lineColor: 1, + fillColor: 1, + strokeColor: 1 + }, + _xyContexts = "position,scale,skew,pivot,anchor,tilePosition,tileScale".split(","), + _contexts = { + x: "position", + y: "position", + tileX: "tilePosition", + tileY: "tilePosition" + }, + _colorMatrixFilterProps = { + colorMatrixFilter: 1, + saturation: 1, + contrast: 1, + hue: 1, + colorize: 1, + colorizeAmount: 1, + brightness: 1, + combineCMF: 1 + }, + _DEG2RAD$4 = Math.PI / 180, + _isString$2 = function _isString(value) { + return typeof value === "string"; + }, + _degreesToRadians = function _degreesToRadians(value) { + return _isString$2(value) && value.charAt(1) === "=" ? value.substr(0, 2) + parseFloat(value.substr(2)) * _DEG2RAD$4 : value * _DEG2RAD$4; + }, + _renderPropWithEnd$1 = function _renderPropWithEnd(ratio, data) { + return data.set(data.t, data.p, ratio === 1 ? data.e : Math.round((data.s + data.c * ratio) * 100000) / 100000, data); + }, + _addRotationalPropTween$1 = function _addRotationalPropTween(plugin, target, property, startNum, endValue, radians) { + var cap = 360 * (radians ? _DEG2RAD$4 : 1), + isString = _isString$2(endValue), + relative = isString && endValue.charAt(1) === "=" ? +(endValue.charAt(0) + "1") : 0, + endNum = parseFloat(relative ? endValue.substr(2) : endValue) * (radians ? _DEG2RAD$4 : 1), + change = relative ? endNum * relative : endNum - startNum, + finalValue = startNum + change, + direction, + pt; + + if (isString) { + direction = endValue.split("_")[1]; + + if (direction === "short") { + change %= cap; + + if (change !== change % (cap / 2)) { + change += change < 0 ? cap : -cap; + } + } + + if (direction === "cw" && change < 0) { + change = (change + cap * 1e10) % cap - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = (change - cap * 1e10) % cap - ~~(change / cap) * cap; + } + } + + plugin._pt = pt = new PropTween$2(plugin._pt, target, property, startNum, change, _renderPropWithEnd$1); + pt.e = finalValue; + return pt; + }, + _initCore$7 = function _initCore() { + if (!_coreInitted$6) { + gsap$9 = _getGSAP$7(); + _PIXI = _coreInitted$6 = _PIXI || _windowExists$5() && window.PIXI; + var version = _PIXI && _PIXI.VERSION && parseFloat(_PIXI.VERSION.split(".")[0]) || 0; + _isV4 = version === 4; + _isV8Plus = version >= 8; + + _splitColor = function _splitColor(color) { + return gsap$9.utils.splitColor((color + "").substr(0, 2) === "0x" ? "#" + color.substr(2) : color); + }; + } + }, + i, + p$1; + + for (i = 0; i < _xyContexts.length; i++) { + p$1 = _xyContexts[i]; + _contexts[p$1 + "X"] = p$1; + _contexts[p$1 + "Y"] = p$1; + } + + var PixiPlugin = { + version: "3.12.7", + name: "pixi", + register: function register(core, Plugin, propTween) { + gsap$9 = core; + PropTween$2 = propTween; + _getSetter$1 = Plugin.getSetter; + + _initCore$7(); + }, + headless: true, + registerPIXI: function registerPIXI(pixi) { + _PIXI = pixi; + }, + init: function init(target, values, tween, index, targets) { + _PIXI || _initCore$7(); + + if (!_PIXI) { + _warn$2("PIXI was not found. PixiPlugin.registerPIXI(PIXI);"); + + return false; + } + + var context, axis, value, colorMatrix, filter, p, padding, i, data, subProp; + + for (p in values) { + context = _contexts[p]; + value = values[p]; + + if (context) { + axis = ~p.charAt(p.length - 1).toLowerCase().indexOf("x") ? "x" : "y"; + this.add(target[context], axis, target[context][axis], context === "skew" ? _degreesToRadians(value) : value, 0, 0, 0, 0, 0, 1); + } else if (p === "scale" || p === "anchor" || p === "pivot" || p === "tileScale") { + this.add(target[p], "x", target[p].x, value); + this.add(target[p], "y", target[p].y, value); + } else if (p === "rotation" || p === "angle") { + _addRotationalPropTween$1(this, target, p, target[p], value, p === "rotation"); + } else if (_colorMatrixFilterProps[p]) { + if (!colorMatrix) { + _parseColorMatrixFilter$1(target, values.colorMatrixFilter || values, this); + + colorMatrix = true; + } + } else if (p === "blur" || p === "blurX" || p === "blurY" || p === "blurPadding") { + filter = _getFilter(target, "BlurFilter"); + this.add(filter, p, filter[p], value); + + if (values.blurPadding !== 0) { + padding = values.blurPadding || Math.max(filter[p], value) * 2; + i = target.filters.length; + + while (--i > -1) { + target.filters[i].padding = Math.max(target.filters[i].padding, padding); + } + } + } else if (_colorProps$1[p]) { + if ((p === "lineColor" || p === "fillColor" || p === "strokeColor") && target instanceof _PIXI.Graphics) { + data = "fillStyle" in target ? [target] : (target.geometry || target).graphicsData; + subProp = p.substr(0, p.length - 5); + _isV8Plus && subProp === "line" && (subProp = "stroke"); + this._pt = new PropTween$2(this._pt, target, p, 0, 0, _renderDirtyCache, { + g: target.geometry || target + }); + i = data.length; + + while (--i > -1) { + _addColorTween(_isV4 ? data[i] : data[i][subProp + "Style"], _isV4 ? p : "color", value, this); + } + } else { + _addColorTween(target, p, value, this); + } + } else if (p === "autoAlpha") { + this._pt = new PropTween$2(this._pt, target, "visible", 0, 0, _renderAutoAlpha); + this.add(target, "alpha", target.alpha, value); + + this._props.push("alpha", "visible"); + } else if (p !== "resolution") { + this.add(target, p, "get", value); + } + + this._props.push(p); + } + } + }; + _getGSAP$7() && gsap$9.registerPlugin(PixiPlugin); + + /*! + * ScrollToPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var gsap$a, + _coreInitted$7, + _window, + _docEl$1, + _body$4, + _toArray$3, + _config$1, + ScrollTrigger$1, + _windowExists$6 = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$8 = function _getGSAP() { + return gsap$a || _windowExists$6() && (gsap$a = window.gsap) && gsap$a.registerPlugin && gsap$a; + }, + _isString$3 = function _isString(value) { + return typeof value === "string"; + }, + _isFunction$3 = function _isFunction(value) { + return typeof value === "function"; + }, + _max = function _max(element, axis) { + var dim = axis === "x" ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return element === _window || element === _docEl$1 || element === _body$4 ? Math.max(_docEl$1[scroll], _body$4[scroll]) - (_window["inner" + dim] || _docEl$1[client] || _body$4[client]) : element[scroll] - element["offset" + dim]; + }, + _buildGetter = function _buildGetter(e, axis) { + var p = "scroll" + (axis === "x" ? "Left" : "Top"); + + if (e === _window) { + if (e.pageXOffset != null) { + p = "page" + axis.toUpperCase() + "Offset"; + } else { + e = _docEl$1[p] != null ? _docEl$1 : _body$4; + } + } + + return function () { + return e[p]; + }; + }, + _clean = function _clean(value, index, target, targets) { + _isFunction$3(value) && (value = value(index, target, targets)); + + if (typeof value !== "object") { + return _isString$3(value) && value !== "max" && value.charAt(1) !== "=" ? { + x: value, + y: value + } : { + y: value + }; + } else if (value.nodeType) { + return { + y: value, + x: value + }; + } else { + var result = {}, + p; + + for (p in value) { + result[p] = p !== "onAutoKill" && _isFunction$3(value[p]) ? value[p](index, target, targets) : value[p]; + } + + return result; + } + }, + _getOffset = function _getOffset(element, container) { + element = _toArray$3(element)[0]; + + if (!element || !element.getBoundingClientRect) { + return console.warn("scrollTo target doesn't exist. Using 0") || { + x: 0, + y: 0 + }; + } + + var rect = element.getBoundingClientRect(), + isRoot = !container || container === _window || container === _body$4, + cRect = isRoot ? { + top: _docEl$1.clientTop - (_window.pageYOffset || _docEl$1.scrollTop || _body$4.scrollTop || 0), + left: _docEl$1.clientLeft - (_window.pageXOffset || _docEl$1.scrollLeft || _body$4.scrollLeft || 0) + } : container.getBoundingClientRect(), + offsets = { + x: rect.left - cRect.left, + y: rect.top - cRect.top + }; + + if (!isRoot && container) { + offsets.x += _buildGetter(container, "x")(); + offsets.y += _buildGetter(container, "y")(); + } + + return offsets; + }, + _parseVal = function _parseVal(value, target, axis, currentVal, offset) { + return !isNaN(value) && typeof value !== "object" ? parseFloat(value) - offset : _isString$3(value) && value.charAt(1) === "=" ? parseFloat(value.substr(2)) * (value.charAt(0) === "-" ? -1 : 1) + currentVal - offset : value === "max" ? _max(target, axis) - offset : Math.min(_max(target, axis), _getOffset(value, target)[axis] - offset); + }, + _initCore$8 = function _initCore() { + gsap$a = _getGSAP$8(); + + if (_windowExists$6() && gsap$a && typeof document !== "undefined" && document.body) { + _window = window; + _body$4 = document.body; + _docEl$1 = document.documentElement; + _toArray$3 = gsap$a.utils.toArray; + gsap$a.config({ + autoKillThreshold: 7 + }); + _config$1 = gsap$a.config(); + _coreInitted$7 = 1; + } + }; + + var ScrollToPlugin = { + version: "3.12.7", + name: "scrollTo", + rawVars: 1, + register: function register(core) { + gsap$a = core; + + _initCore$8(); + }, + init: function init(target, value, tween, index, targets) { + _coreInitted$7 || _initCore$8(); + var data = this, + snapType = gsap$a.getProperty(target, "scrollSnapType"); + data.isWin = target === _window; + data.target = target; + data.tween = tween; + value = _clean(value, index, target, targets); + data.vars = value; + data.autoKill = !!("autoKill" in value ? value : _config$1).autoKill; + data.getX = _buildGetter(target, "x"); + data.getY = _buildGetter(target, "y"); + data.x = data.xPrev = data.getX(); + data.y = data.yPrev = data.getY(); + ScrollTrigger$1 || (ScrollTrigger$1 = gsap$a.core.globals().ScrollTrigger); + gsap$a.getProperty(target, "scrollBehavior") === "smooth" && gsap$a.set(target, { + scrollBehavior: "auto" + }); + + if (snapType && snapType !== "none") { + data.snap = 1; + data.snapInline = target.style.scrollSnapType; + target.style.scrollSnapType = "none"; + } + + if (value.x != null) { + data.add(data, "x", data.x, _parseVal(value.x, target, "x", data.x, value.offsetX || 0), index, targets); + + data._props.push("scrollTo_x"); + } else { + data.skipX = 1; + } + + if (value.y != null) { + data.add(data, "y", data.y, _parseVal(value.y, target, "y", data.y, value.offsetY || 0), index, targets); + + data._props.push("scrollTo_y"); + } else { + data.skipY = 1; + } + }, + render: function render(ratio, data) { + var pt = data._pt, + target = data.target, + tween = data.tween, + autoKill = data.autoKill, + xPrev = data.xPrev, + yPrev = data.yPrev, + isWin = data.isWin, + snap = data.snap, + snapInline = data.snapInline, + x, + y, + yDif, + xDif, + threshold; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + x = isWin || !data.skipX ? data.getX() : xPrev; + y = isWin || !data.skipY ? data.getY() : yPrev; + yDif = y - yPrev; + xDif = x - xPrev; + threshold = _config$1.autoKillThreshold; + + if (data.x < 0) { + data.x = 0; + } + + if (data.y < 0) { + data.y = 0; + } + + if (autoKill) { + if (!data.skipX && (xDif > threshold || xDif < -threshold) && x < _max(target, "x")) { + data.skipX = 1; + } + + if (!data.skipY && (yDif > threshold || yDif < -threshold) && y < _max(target, "y")) { + data.skipY = 1; + } + + if (data.skipX && data.skipY) { + tween.kill(); + data.vars.onAutoKill && data.vars.onAutoKill.apply(tween, data.vars.onAutoKillParams || []); + } + } + + if (isWin) { + _window.scrollTo(!data.skipX ? data.x : x, !data.skipY ? data.y : y); + } else { + data.skipY || (target.scrollTop = data.y); + data.skipX || (target.scrollLeft = data.x); + } + + if (snap && (ratio === 1 || ratio === 0)) { + y = target.scrollTop; + x = target.scrollLeft; + snapInline ? target.style.scrollSnapType = snapInline : target.style.removeProperty("scroll-snap-type"); + target.scrollTop = y + 1; + target.scrollLeft = x + 1; + target.scrollTop = y; + target.scrollLeft = x; + } + + data.xPrev = data.x; + data.yPrev = data.y; + ScrollTrigger$1 && ScrollTrigger$1.update(); + }, + kill: function kill(property) { + var both = property === "scrollTo", + i = this._props.indexOf(property); + + if (both || property === "scrollTo_x") { + this.skipX = 1; + } + + if (both || property === "scrollTo_y") { + this.skipY = 1; + } + + i > -1 && this._props.splice(i, 1); + return !this._props.length; + } + }; + ScrollToPlugin.max = _max; + ScrollToPlugin.getOffset = _getOffset; + ScrollToPlugin.buildGetter = _buildGetter; + + ScrollToPlugin.config = function (vars) { + _config$1 || _initCore$8() || (_config$1 = gsap$a.config()); + + for (var p in vars) { + _config$1[p] = vars[p]; + } + }; + + _getGSAP$8() && gsap$a.registerPlugin(ScrollToPlugin); + + /*! + * ScrollTrigger 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var gsap$b, + _coreInitted$8, + _win$6, + _doc$6, + _docEl$2, + _body$5, + _root$1, + _resizeDelay, + _toArray$4, + _clamp$2, + _time2, + _syncInterval, + _refreshing, + _pointerIsDown, + _transformProp$3, + _i, + _prevWidth, + _prevHeight, + _autoRefresh, + _sort, + _suppressOverwrites$1, + _ignoreResize, + _normalizer$1, + _ignoreMobileResize, + _baseScreenHeight, + _baseScreenWidth, + _fixIOSBug, + _context$3, + _scrollRestoration, + _div100vh, + _100vh, + _isReverted, + _clampingMax, + _limitCallbacks, + _startup$1 = 1, + _getTime$2 = Date.now, + _time1 = _getTime$2(), + _lastScrollTime = 0, + _enabled = 0, + _parseClamp = function _parseClamp(value, type, self) { + var clamp = _isString$4(value) && (value.substr(0, 6) === "clamp(" || value.indexOf("max") > -1); + self["_" + type + "Clamp"] = clamp; + return clamp ? value.substr(6, value.length - 7) : value; + }, + _keepClamp = function _keepClamp(value, clamp) { + return clamp && (!_isString$4(value) || value.substr(0, 6) !== "clamp(") ? "clamp(" + value + ")" : value; + }, + _rafBugFix = function _rafBugFix() { + return _enabled && requestAnimationFrame(_rafBugFix); + }, + _pointerDownHandler = function _pointerDownHandler() { + return _pointerIsDown = 1; + }, + _pointerUpHandler = function _pointerUpHandler() { + return _pointerIsDown = 0; + }, + _passThrough$1 = function _passThrough(v) { + return v; + }, + _round$5 = function _round(value) { + return Math.round(value * 100000) / 100000 || 0; + }, + _windowExists$7 = function _windowExists() { + return typeof window !== "undefined"; + }, + _getGSAP$9 = function _getGSAP() { + return gsap$b || _windowExists$7() && (gsap$b = window.gsap) && gsap$b.registerPlugin && gsap$b; + }, + _isViewport$1 = function _isViewport(e) { + return !!~_root$1.indexOf(e); + }, + _getViewportDimension = function _getViewportDimension(dimensionProperty) { + return (dimensionProperty === "Height" ? _100vh : _win$6["inner" + dimensionProperty]) || _docEl$2["client" + dimensionProperty] || _body$5["client" + dimensionProperty]; + }, + _getBoundsFunc = function _getBoundsFunc(element) { + return _getProxyProp(element, "getBoundingClientRect") || (_isViewport$1(element) ? function () { + _winOffsets.width = _win$6.innerWidth; + _winOffsets.height = _100vh; + return _winOffsets; + } : function () { + return _getBounds$1(element); + }); + }, + _getSizeFunc = function _getSizeFunc(scroller, isViewport, _ref) { + var d = _ref.d, + d2 = _ref.d2, + a = _ref.a; + return (a = _getProxyProp(scroller, "getBoundingClientRect")) ? function () { + return a()[d]; + } : function () { + return (isViewport ? _getViewportDimension(d2) : scroller["client" + d2]) || 0; + }; + }, + _getOffsetsFunc = function _getOffsetsFunc(element, isViewport) { + return !isViewport || ~exports._proxies.indexOf(element) ? _getBoundsFunc(element) : function () { + return _winOffsets; + }; + }, + _maxScroll = function _maxScroll(element, _ref2) { + var s = _ref2.s, + d2 = _ref2.d2, + d = _ref2.d, + a = _ref2.a; + return Math.max(0, (s = "scroll" + d2) && (a = _getProxyProp(element, s)) ? a() - _getBoundsFunc(element)()[d] : _isViewport$1(element) ? (_docEl$2[s] || _body$5[s]) - _getViewportDimension(d2) : element[s] - element["offset" + d2]); + }, + _iterateAutoRefresh = function _iterateAutoRefresh(func, events) { + for (var i = 0; i < _autoRefresh.length; i += 3) { + (!events || ~events.indexOf(_autoRefresh[i + 1])) && func(_autoRefresh[i], _autoRefresh[i + 1], _autoRefresh[i + 2]); + } + }, + _isString$4 = function _isString(value) { + return typeof value === "string"; + }, + _isFunction$4 = function _isFunction(value) { + return typeof value === "function"; + }, + _isNumber$2 = function _isNumber(value) { + return typeof value === "number"; + }, + _isObject$2 = function _isObject(value) { + return typeof value === "object"; + }, + _endAnimation = function _endAnimation(animation, reversed, pause) { + return animation && animation.progress(reversed ? 0 : 1) && pause && animation.pause(); + }, + _callback$1 = function _callback(self, func) { + if (self.enabled) { + var result = self._ctx ? self._ctx.add(function () { + return func(self); + }) : func(self); + result && result.totalTime && (self.callbackAnimation = result); + } + }, + _abs$1 = Math.abs, + _left = "left", + _top = "top", + _right = "right", + _bottom = "bottom", + _width = "width", + _height = "height", + _Right = "Right", + _Left = "Left", + _Top = "Top", + _Bottom = "Bottom", + _padding = "padding", + _margin = "margin", + _Width = "Width", + _Height = "Height", + _px = "px", + _getComputedStyle$1 = function _getComputedStyle(element) { + return _win$6.getComputedStyle(element); + }, + _makePositionable = function _makePositionable(element) { + var position = _getComputedStyle$1(element).position; + + element.style.position = position === "absolute" || position === "fixed" ? position : "relative"; + }, + _setDefaults$2 = function _setDefaults(obj, defaults) { + for (var p in defaults) { + p in obj || (obj[p] = defaults[p]); + } + + return obj; + }, + _getBounds$1 = function _getBounds(element, withoutTransforms) { + var tween = withoutTransforms && _getComputedStyle$1(element)[_transformProp$3] !== "matrix(1, 0, 0, 1, 0, 0)" && gsap$b.to(element, { + x: 0, + y: 0, + xPercent: 0, + yPercent: 0, + rotation: 0, + rotationX: 0, + rotationY: 0, + scale: 1, + skewX: 0, + skewY: 0 + }).progress(1), + bounds = element.getBoundingClientRect(); + tween && tween.progress(0).kill(); + return bounds; + }, + _getSize = function _getSize(element, _ref3) { + var d2 = _ref3.d2; + return element["offset" + d2] || element["client" + d2] || 0; + }, + _getLabelRatioArray = function _getLabelRatioArray(timeline) { + var a = [], + labels = timeline.labels, + duration = timeline.duration(), + p; + + for (p in labels) { + a.push(labels[p] / duration); + } + + return a; + }, + _getClosestLabel = function _getClosestLabel(animation) { + return function (value) { + return gsap$b.utils.snap(_getLabelRatioArray(animation), value); + }; + }, + _snapDirectional = function _snapDirectional(snapIncrementOrArray) { + var snap = gsap$b.utils.snap(snapIncrementOrArray), + a = Array.isArray(snapIncrementOrArray) && snapIncrementOrArray.slice(0).sort(function (a, b) { + return a - b; + }); + return a ? function (value, direction, threshold) { + if (threshold === void 0) { + threshold = 1e-3; + } + + var i; + + if (!direction) { + return snap(value); + } + + if (direction > 0) { + value -= threshold; + + for (i = 0; i < a.length; i++) { + if (a[i] >= value) { + return a[i]; + } + } + + return a[i - 1]; + } else { + i = a.length; + value += threshold; + + while (i--) { + if (a[i] <= value) { + return a[i]; + } + } + } + + return a[0]; + } : function (value, direction, threshold) { + if (threshold === void 0) { + threshold = 1e-3; + } + + var snapped = snap(value); + return !direction || Math.abs(snapped - value) < threshold || snapped - value < 0 === direction < 0 ? snapped : snap(direction < 0 ? value - snapIncrementOrArray : value + snapIncrementOrArray); + }; + }, + _getLabelAtDirection = function _getLabelAtDirection(timeline) { + return function (value, st) { + return _snapDirectional(_getLabelRatioArray(timeline))(value, st.direction); + }; + }, + _multiListener = function _multiListener(func, element, types, callback) { + return types.split(",").forEach(function (type) { + return func(element, type, callback); + }); + }, + _addListener$2 = function _addListener(element, type, func, nonPassive, capture) { + return element.addEventListener(type, func, { + passive: !nonPassive, + capture: !!capture + }); + }, + _removeListener$2 = function _removeListener(element, type, func, capture) { + return element.removeEventListener(type, func, !!capture); + }, + _wheelListener = function _wheelListener(func, el, scrollFunc) { + scrollFunc = scrollFunc && scrollFunc.wheelHandler; + + if (scrollFunc) { + func(el, "wheel", scrollFunc); + func(el, "touchmove", scrollFunc); + } + }, + _markerDefaults = { + startColor: "green", + endColor: "red", + indent: 0, + fontSize: "16px", + fontWeight: "normal" + }, + _defaults$1 = { + toggleActions: "play", + anticipatePin: 0 + }, + _keywords = { + top: 0, + left: 0, + center: 0.5, + bottom: 1, + right: 1 + }, + _offsetToPx = function _offsetToPx(value, size) { + if (_isString$4(value)) { + var eqIndex = value.indexOf("="), + relative = ~eqIndex ? +(value.charAt(eqIndex - 1) + 1) * parseFloat(value.substr(eqIndex + 1)) : 0; + + if (~eqIndex) { + value.indexOf("%") > eqIndex && (relative *= size / 100); + value = value.substr(0, eqIndex - 1); + } + + value = relative + (value in _keywords ? _keywords[value] * size : ~value.indexOf("%") ? parseFloat(value) * size / 100 : parseFloat(value) || 0); + } + + return value; + }, + _createMarker = function _createMarker(type, name, container, direction, _ref4, offset, matchWidthEl, containerAnimation) { + var startColor = _ref4.startColor, + endColor = _ref4.endColor, + fontSize = _ref4.fontSize, + indent = _ref4.indent, + fontWeight = _ref4.fontWeight; + + var e = _doc$6.createElement("div"), + useFixedPosition = _isViewport$1(container) || _getProxyProp(container, "pinType") === "fixed", + isScroller = type.indexOf("scroller") !== -1, + parent = useFixedPosition ? _body$5 : container, + isStart = type.indexOf("start") !== -1, + color = isStart ? startColor : endColor, + css = "border-color:" + color + ";font-size:" + fontSize + ";color:" + color + ";font-weight:" + fontWeight + ";pointer-events:none;white-space:nowrap;font-family:sans-serif,Arial;z-index:1000;padding:4px 8px;border-width:0;border-style:solid;"; + + css += "position:" + ((isScroller || containerAnimation) && useFixedPosition ? "fixed;" : "absolute;"); + (isScroller || containerAnimation || !useFixedPosition) && (css += (direction === _vertical ? _right : _bottom) + ":" + (offset + parseFloat(indent)) + "px;"); + matchWidthEl && (css += "box-sizing:border-box;text-align:left;width:" + matchWidthEl.offsetWidth + "px;"); + e._isStart = isStart; + e.setAttribute("class", "gsap-marker-" + type + (name ? " marker-" + name : "")); + e.style.cssText = css; + e.innerText = name || name === 0 ? type + "-" + name : type; + parent.children[0] ? parent.insertBefore(e, parent.children[0]) : parent.appendChild(e); + e._offset = e["offset" + direction.op.d2]; + + _positionMarker(e, 0, direction, isStart); + + return e; + }, + _positionMarker = function _positionMarker(marker, start, direction, flipped) { + var vars = { + display: "block" + }, + side = direction[flipped ? "os2" : "p2"], + oppositeSide = direction[flipped ? "p2" : "os2"]; + marker._isFlipped = flipped; + vars[direction.a + "Percent"] = flipped ? -100 : 0; + vars[direction.a] = flipped ? "1px" : 0; + vars["border" + side + _Width] = 1; + vars["border" + oppositeSide + _Width] = 0; + vars[direction.p] = start + "px"; + gsap$b.set(marker, vars); + }, + _triggers = [], + _ids = {}, + _rafID, + _sync = function _sync() { + return _getTime$2() - _lastScrollTime > 34 && (_rafID || (_rafID = requestAnimationFrame(_updateAll))); + }, + _onScroll$1 = function _onScroll() { + if (!_normalizer$1 || !_normalizer$1.isPressed || _normalizer$1.startX > _body$5.clientWidth) { + exports._scrollers.cache++; + + if (_normalizer$1) { + _rafID || (_rafID = requestAnimationFrame(_updateAll)); + } else { + _updateAll(); + } + + _lastScrollTime || _dispatch$1("scrollStart"); + _lastScrollTime = _getTime$2(); + } + }, + _setBaseDimensions = function _setBaseDimensions() { + _baseScreenWidth = _win$6.innerWidth; + _baseScreenHeight = _win$6.innerHeight; + }, + _onResize = function _onResize(force) { + exports._scrollers.cache++; + (force === true || !_refreshing && !_ignoreResize && !_doc$6.fullscreenElement && !_doc$6.webkitFullscreenElement && (!_ignoreMobileResize || _baseScreenWidth !== _win$6.innerWidth || Math.abs(_win$6.innerHeight - _baseScreenHeight) > _win$6.innerHeight * 0.25)) && _resizeDelay.restart(true); + }, + _listeners$1 = {}, + _emptyArray$1 = [], + _softRefresh = function _softRefresh() { + return _removeListener$2(ScrollTrigger$2, "scrollEnd", _softRefresh) || _refreshAll(true); + }, + _dispatch$1 = function _dispatch(type) { + return _listeners$1[type] && _listeners$1[type].map(function (f) { + return f(); + }) || _emptyArray$1; + }, + _savedStyles = [], + _revertRecorded = function _revertRecorded(media) { + for (var i = 0; i < _savedStyles.length; i += 5) { + if (!media || _savedStyles[i + 4] && _savedStyles[i + 4].query === media) { + _savedStyles[i].style.cssText = _savedStyles[i + 1]; + _savedStyles[i].getBBox && _savedStyles[i].setAttribute("transform", _savedStyles[i + 2] || ""); + _savedStyles[i + 3].uncache = 1; + } + } + }, + _revertAll = function _revertAll(kill, media) { + var trigger; + + for (_i = 0; _i < _triggers.length; _i++) { + trigger = _triggers[_i]; + + if (trigger && (!media || trigger._ctx === media)) { + if (kill) { + trigger.kill(1); + } else { + trigger.revert(true, true); + } + } + } + + _isReverted = true; + media && _revertRecorded(media); + media || _dispatch$1("revert"); + }, + _clearScrollMemory = function _clearScrollMemory(scrollRestoration, force) { + exports._scrollers.cache++; + (force || !_refreshingAll) && exports._scrollers.forEach(function (obj) { + return _isFunction$4(obj) && obj.cacheID++ && (obj.rec = 0); + }); + _isString$4(scrollRestoration) && (_win$6.history.scrollRestoration = _scrollRestoration = scrollRestoration); + }, + _refreshingAll, + _refreshID = 0, + _queueRefreshID, + _queueRefreshAll = function _queueRefreshAll() { + if (_queueRefreshID !== _refreshID) { + var id = _queueRefreshID = _refreshID; + requestAnimationFrame(function () { + return id === _refreshID && _refreshAll(true); + }); + } + }, + _refresh100vh = function _refresh100vh() { + _body$5.appendChild(_div100vh); + + _100vh = !_normalizer$1 && _div100vh.offsetHeight || _win$6.innerHeight; + + _body$5.removeChild(_div100vh); + }, + _hideAllMarkers = function _hideAllMarkers(hide) { + return _toArray$4(".gsap-marker-start, .gsap-marker-end, .gsap-marker-scroller-start, .gsap-marker-scroller-end").forEach(function (el) { + return el.style.display = hide ? "none" : "block"; + }); + }, + _refreshAll = function _refreshAll(force, skipRevert) { + _docEl$2 = _doc$6.documentElement; + _body$5 = _doc$6.body; + _root$1 = [_win$6, _doc$6, _docEl$2, _body$5]; + + if (_lastScrollTime && !force && !_isReverted) { + _addListener$2(ScrollTrigger$2, "scrollEnd", _softRefresh); + + return; + } + + _refresh100vh(); + + _refreshingAll = ScrollTrigger$2.isRefreshing = true; + + exports._scrollers.forEach(function (obj) { + return _isFunction$4(obj) && ++obj.cacheID && (obj.rec = obj()); + }); + + var refreshInits = _dispatch$1("refreshInit"); + + _sort && ScrollTrigger$2.sort(); + skipRevert || _revertAll(); + + exports._scrollers.forEach(function (obj) { + if (_isFunction$4(obj)) { + obj.smooth && (obj.target.style.scrollBehavior = "auto"); + obj(0); + } + }); + + _triggers.slice(0).forEach(function (t) { + return t.refresh(); + }); + + _isReverted = false; + + _triggers.forEach(function (t) { + if (t._subPinOffset && t.pin) { + var prop = t.vars.horizontal ? "offsetWidth" : "offsetHeight", + original = t.pin[prop]; + t.revert(true, 1); + t.adjustPinSpacing(t.pin[prop] - original); + t.refresh(); + } + }); + + _clampingMax = 1; + + _hideAllMarkers(true); + + _triggers.forEach(function (t) { + var max = _maxScroll(t.scroller, t._dir), + endClamp = t.vars.end === "max" || t._endClamp && t.end > max, + startClamp = t._startClamp && t.start >= max; + + (endClamp || startClamp) && t.setPositions(startClamp ? max - 1 : t.start, endClamp ? Math.max(startClamp ? max : t.start + 1, max) : t.end, true); + }); + + _hideAllMarkers(false); + + _clampingMax = 0; + refreshInits.forEach(function (result) { + return result && result.render && result.render(-1); + }); + + exports._scrollers.forEach(function (obj) { + if (_isFunction$4(obj)) { + obj.smooth && requestAnimationFrame(function () { + return obj.target.style.scrollBehavior = "smooth"; + }); + obj.rec && obj(obj.rec); + } + }); + + _clearScrollMemory(_scrollRestoration, 1); + + _resizeDelay.pause(); + + _refreshID++; + _refreshingAll = 2; + + _updateAll(2); + + _triggers.forEach(function (t) { + return _isFunction$4(t.vars.onRefresh) && t.vars.onRefresh(t); + }); + + _refreshingAll = ScrollTrigger$2.isRefreshing = false; + + _dispatch$1("refresh"); + }, + _lastScroll = 0, + _direction = 1, + _primary, + _updateAll = function _updateAll(force) { + if (force === 2 || !_refreshingAll && !_isReverted) { + ScrollTrigger$2.isUpdating = true; + _primary && _primary.update(0); + + var l = _triggers.length, + time = _getTime$2(), + recordVelocity = time - _time1 >= 50, + scroll = l && _triggers[0].scroll(); + + _direction = _lastScroll > scroll ? -1 : 1; + _refreshingAll || (_lastScroll = scroll); + + if (recordVelocity) { + if (_lastScrollTime && !_pointerIsDown && time - _lastScrollTime > 200) { + _lastScrollTime = 0; + + _dispatch$1("scrollEnd"); + } + + _time2 = _time1; + _time1 = time; + } + + if (_direction < 0) { + _i = l; + + while (_i-- > 0) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + + _direction = 1; + } else { + for (_i = 0; _i < l; _i++) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + } + + ScrollTrigger$2.isUpdating = false; + } + + _rafID = 0; + }, + _propNamesToCopy = [_left, _top, _bottom, _right, _margin + _Bottom, _margin + _Right, _margin + _Top, _margin + _Left, "display", "flexShrink", "float", "zIndex", "gridColumnStart", "gridColumnEnd", "gridRowStart", "gridRowEnd", "gridArea", "justifySelf", "alignSelf", "placeSelf", "order"], + _stateProps = _propNamesToCopy.concat([_width, _height, "boxSizing", "max" + _Width, "max" + _Height, "position", _margin, _padding, _padding + _Top, _padding + _Right, _padding + _Bottom, _padding + _Left]), + _swapPinOut = function _swapPinOut(pin, spacer, state) { + _setState(state); + + var cache = pin._gsap; + + if (cache.spacerIsNative) { + _setState(cache.spacerState); + } else if (pin._gsap.swappedIn) { + var parent = spacer.parentNode; + + if (parent) { + parent.insertBefore(pin, spacer); + parent.removeChild(spacer); + } + } + + pin._gsap.swappedIn = false; + }, + _swapPinIn = function _swapPinIn(pin, spacer, cs, spacerState) { + if (!pin._gsap.swappedIn) { + var i = _propNamesToCopy.length, + spacerStyle = spacer.style, + pinStyle = pin.style, + p; + + while (i--) { + p = _propNamesToCopy[i]; + spacerStyle[p] = cs[p]; + } + + spacerStyle.position = cs.position === "absolute" ? "absolute" : "relative"; + cs.display === "inline" && (spacerStyle.display = "inline-block"); + pinStyle[_bottom] = pinStyle[_right] = "auto"; + spacerStyle.flexBasis = cs.flexBasis || "auto"; + spacerStyle.overflow = "visible"; + spacerStyle.boxSizing = "border-box"; + spacerStyle[_width] = _getSize(pin, _horizontal) + _px; + spacerStyle[_height] = _getSize(pin, _vertical) + _px; + spacerStyle[_padding] = pinStyle[_margin] = pinStyle[_top] = pinStyle[_left] = "0"; + + _setState(spacerState); + + pinStyle[_width] = pinStyle["max" + _Width] = cs[_width]; + pinStyle[_height] = pinStyle["max" + _Height] = cs[_height]; + pinStyle[_padding] = cs[_padding]; + + if (pin.parentNode !== spacer) { + pin.parentNode.insertBefore(spacer, pin); + spacer.appendChild(pin); + } + + pin._gsap.swappedIn = true; + } + }, + _capsExp$1 = /([A-Z])/g, + _setState = function _setState(state) { + if (state) { + var style = state.t.style, + l = state.length, + i = 0, + p, + value; + (state.t._gsap || gsap$b.core.getCache(state.t)).uncache = 1; + + for (; i < l; i += 2) { + value = state[i + 1]; + p = state[i]; + + if (value) { + style[p] = value; + } else if (style[p]) { + style.removeProperty(p.replace(_capsExp$1, "-$1").toLowerCase()); + } + } + } + }, + _getState = function _getState(element) { + var l = _stateProps.length, + style = element.style, + state = [], + i = 0; + + for (; i < l; i++) { + state.push(_stateProps[i], style[_stateProps[i]]); + } + + state.t = element; + return state; + }, + _copyState = function _copyState(state, override, omitOffsets) { + var result = [], + l = state.length, + i = omitOffsets ? 8 : 0, + p; + + for (; i < l; i += 2) { + p = state[i]; + result.push(p, p in override ? override[p] : state[i + 1]); + } + + result.t = state.t; + return result; + }, + _winOffsets = { + left: 0, + top: 0 + }, + _parsePosition$1 = function _parsePosition(value, trigger, scrollerSize, direction, scroll, marker, markerScroller, self, scrollerBounds, borderWidth, useFixedPosition, scrollerMax, containerAnimation, clampZeroProp) { + _isFunction$4(value) && (value = value(self)); + + if (_isString$4(value) && value.substr(0, 3) === "max") { + value = scrollerMax + (value.charAt(4) === "=" ? _offsetToPx("0" + value.substr(3), scrollerSize) : 0); + } + + var time = containerAnimation ? containerAnimation.time() : 0, + p1, + p2, + element; + containerAnimation && containerAnimation.seek(0); + isNaN(value) || (value = +value); + + if (!_isNumber$2(value)) { + _isFunction$4(trigger) && (trigger = trigger(self)); + var offsets = (value || "0").split(" "), + bounds, + localOffset, + globalOffset, + display; + element = _getTarget(trigger, self) || _body$5; + bounds = _getBounds$1(element) || {}; + + if ((!bounds || !bounds.left && !bounds.top) && _getComputedStyle$1(element).display === "none") { + display = element.style.display; + element.style.display = "block"; + bounds = _getBounds$1(element); + display ? element.style.display = display : element.style.removeProperty("display"); + } + + localOffset = _offsetToPx(offsets[0], bounds[direction.d]); + globalOffset = _offsetToPx(offsets[1] || "0", scrollerSize); + value = bounds[direction.p] - scrollerBounds[direction.p] - borderWidth + localOffset + scroll - globalOffset; + markerScroller && _positionMarker(markerScroller, globalOffset, direction, scrollerSize - globalOffset < 20 || markerScroller._isStart && globalOffset > 20); + scrollerSize -= scrollerSize - globalOffset; + } else { + containerAnimation && (value = gsap$b.utils.mapRange(containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, 0, scrollerMax, value)); + markerScroller && _positionMarker(markerScroller, scrollerSize, direction, true); + } + + if (clampZeroProp) { + self[clampZeroProp] = value || -0.001; + value < 0 && (value = 0); + } + + if (marker) { + var position = value + scrollerSize, + isStart = marker._isStart; + p1 = "scroll" + direction.d2; + + _positionMarker(marker, position, direction, isStart && position > 20 || !isStart && (useFixedPosition ? Math.max(_body$5[p1], _docEl$2[p1]) : marker.parentNode[p1]) <= position + 1); + + if (useFixedPosition) { + scrollerBounds = _getBounds$1(markerScroller); + useFixedPosition && (marker.style[direction.op.p] = scrollerBounds[direction.op.p] - direction.op.m - marker._offset + _px); + } + } + + if (containerAnimation && element) { + p1 = _getBounds$1(element); + containerAnimation.seek(scrollerMax); + p2 = _getBounds$1(element); + containerAnimation._caScrollDist = p1[direction.p] - p2[direction.p]; + value = value / containerAnimation._caScrollDist * scrollerMax; + } + + containerAnimation && containerAnimation.seek(time); + return containerAnimation ? value : Math.round(value); + }, + _prefixExp = /(webkit|moz|length|cssText|inset)/i, + _reparent = function _reparent(element, parent, top, left) { + if (element.parentNode !== parent) { + var style = element.style, + p, + cs; + + if (parent === _body$5) { + element._stOrig = style.cssText; + cs = _getComputedStyle$1(element); + + for (p in cs) { + if (!+p && !_prefixExp.test(p) && cs[p] && typeof style[p] === "string" && p !== "0") { + style[p] = cs[p]; + } + } + + style.top = top; + style.left = left; + } else { + style.cssText = element._stOrig; + } + + gsap$b.core.getCache(element).uncache = 1; + parent.appendChild(element); + } + }, + _interruptionTracker = function _interruptionTracker(getValueFunc, initialValue, onInterrupt) { + var last1 = initialValue, + last2 = last1; + return function (value) { + var current = Math.round(getValueFunc()); + + if (current !== last1 && current !== last2 && Math.abs(current - last1) > 3 && Math.abs(current - last2) > 3) { + value = current; + onInterrupt && onInterrupt(); + } + + last2 = last1; + last1 = Math.round(value); + return last1; + }; + }, + _shiftMarker = function _shiftMarker(marker, direction, value) { + var vars = {}; + vars[direction.p] = "+=" + value; + gsap$b.set(marker, vars); + }, + _getTweenCreator = function _getTweenCreator(scroller, direction) { + var getScroll = _getScrollFunc(scroller, direction), + prop = "_scroll" + direction.p2, + getTween = function getTween(scrollTo, vars, initialValue, change1, change2) { + var tween = getTween.tween, + onComplete = vars.onComplete, + modifiers = {}; + initialValue = initialValue || getScroll(); + + var checkForInterruption = _interruptionTracker(getScroll, initialValue, function () { + tween.kill(); + getTween.tween = 0; + }); + + change2 = change1 && change2 || 0; + change1 = change1 || scrollTo - initialValue; + tween && tween.kill(); + vars[prop] = scrollTo; + vars.inherit = false; + vars.modifiers = modifiers; + + modifiers[prop] = function () { + return checkForInterruption(initialValue + change1 * tween.ratio + change2 * tween.ratio * tween.ratio); + }; + + vars.onUpdate = function () { + exports._scrollers.cache++; + getTween.tween && _updateAll(); + }; + + vars.onComplete = function () { + getTween.tween = 0; + onComplete && onComplete.call(tween); + }; + + tween = getTween.tween = gsap$b.to(scroller, vars); + return tween; + }; + + scroller[prop] = getScroll; + + getScroll.wheelHandler = function () { + return getTween.tween && getTween.tween.kill() && (getTween.tween = 0); + }; + + _addListener$2(scroller, "wheel", getScroll.wheelHandler); + + ScrollTrigger$2.isTouch && _addListener$2(scroller, "touchmove", getScroll.wheelHandler); + return getTween; + }; + + var ScrollTrigger$2 = function () { + function ScrollTrigger(vars, animation) { + _coreInitted$8 || ScrollTrigger.register(gsap$b) || console.warn("Please gsap.registerPlugin(ScrollTrigger)"); + + _context$3(this); + + this.init(vars, animation); + } + + var _proto = ScrollTrigger.prototype; + + _proto.init = function init(vars, animation) { + this.progress = this.start = 0; + this.vars && this.kill(true, true); + + if (!_enabled) { + this.update = this.refresh = this.kill = _passThrough$1; + return; + } + + vars = _setDefaults$2(_isString$4(vars) || _isNumber$2(vars) || vars.nodeType ? { + trigger: vars + } : vars, _defaults$1); + + var _vars = vars, + onUpdate = _vars.onUpdate, + toggleClass = _vars.toggleClass, + id = _vars.id, + onToggle = _vars.onToggle, + onRefresh = _vars.onRefresh, + scrub = _vars.scrub, + trigger = _vars.trigger, + pin = _vars.pin, + pinSpacing = _vars.pinSpacing, + invalidateOnRefresh = _vars.invalidateOnRefresh, + anticipatePin = _vars.anticipatePin, + onScrubComplete = _vars.onScrubComplete, + onSnapComplete = _vars.onSnapComplete, + once = _vars.once, + snap = _vars.snap, + pinReparent = _vars.pinReparent, + pinSpacer = _vars.pinSpacer, + containerAnimation = _vars.containerAnimation, + fastScrollEnd = _vars.fastScrollEnd, + preventOverlaps = _vars.preventOverlaps, + direction = vars.horizontal || vars.containerAnimation && vars.horizontal !== false ? _horizontal : _vertical, + isToggle = !scrub && scrub !== 0, + scroller = _getTarget(vars.scroller || _win$6), + scrollerCache = gsap$b.core.getCache(scroller), + isViewport = _isViewport$1(scroller), + useFixedPosition = ("pinType" in vars ? vars.pinType : _getProxyProp(scroller, "pinType") || isViewport && "fixed") === "fixed", + callbacks = [vars.onEnter, vars.onLeave, vars.onEnterBack, vars.onLeaveBack], + toggleActions = isToggle && vars.toggleActions.split(" "), + markers = "markers" in vars ? vars.markers : _defaults$1.markers, + borderWidth = isViewport ? 0 : parseFloat(_getComputedStyle$1(scroller)["border" + direction.p2 + _Width]) || 0, + self = this, + onRefreshInit = vars.onRefreshInit && function () { + return vars.onRefreshInit(self); + }, + getScrollerSize = _getSizeFunc(scroller, isViewport, direction), + getScrollerOffsets = _getOffsetsFunc(scroller, isViewport), + lastSnap = 0, + lastRefresh = 0, + prevProgress = 0, + scrollFunc = _getScrollFunc(scroller, direction), + tweenTo, + pinCache, + snapFunc, + scroll1, + scroll2, + start, + end, + markerStart, + markerEnd, + markerStartTrigger, + markerEndTrigger, + markerVars, + executingOnRefresh, + change, + pinOriginalState, + pinActiveState, + pinState, + spacer, + offset, + pinGetter, + pinSetter, + pinStart, + pinChange, + spacingStart, + spacerState, + markerStartSetter, + pinMoves, + markerEndSetter, + cs, + snap1, + snap2, + scrubTween, + scrubSmooth, + snapDurClamp, + snapDelayedCall, + prevScroll, + prevAnimProgress, + caMarkerSetter, + customRevertReturn; + + self._startClamp = self._endClamp = false; + self._dir = direction; + anticipatePin *= 45; + self.scroller = scroller; + self.scroll = containerAnimation ? containerAnimation.time.bind(containerAnimation) : scrollFunc; + scroll1 = scrollFunc(); + self.vars = vars; + animation = animation || vars.animation; + + if ("refreshPriority" in vars) { + _sort = 1; + vars.refreshPriority === -9999 && (_primary = self); + } + + scrollerCache.tweenScroll = scrollerCache.tweenScroll || { + top: _getTweenCreator(scroller, _vertical), + left: _getTweenCreator(scroller, _horizontal) + }; + self.tweenTo = tweenTo = scrollerCache.tweenScroll[direction.p]; + + self.scrubDuration = function (value) { + scrubSmooth = _isNumber$2(value) && value; + + if (!scrubSmooth) { + scrubTween && scrubTween.progress(1).kill(); + scrubTween = 0; + } else { + scrubTween ? scrubTween.duration(value) : scrubTween = gsap$b.to(animation, { + ease: "expo", + totalProgress: "+=0", + inherit: false, + duration: scrubSmooth, + paused: true, + onComplete: function onComplete() { + return onScrubComplete && onScrubComplete(self); + } + }); + } + }; + + if (animation) { + animation.vars.lazy = false; + animation._initted && !self.isReverted || animation.vars.immediateRender !== false && vars.immediateRender !== false && animation.duration() && animation.render(0, true, true); + self.animation = animation.pause(); + animation.scrollTrigger = self; + self.scrubDuration(scrub); + snap1 = 0; + id || (id = animation.vars.id); + } + + if (snap) { + if (!_isObject$2(snap) || snap.push) { + snap = { + snapTo: snap + }; + } + + "scrollBehavior" in _body$5.style && gsap$b.set(isViewport ? [_body$5, _docEl$2] : scroller, { + scrollBehavior: "auto" + }); + + exports._scrollers.forEach(function (o) { + return _isFunction$4(o) && o.target === (isViewport ? _doc$6.scrollingElement || _docEl$2 : scroller) && (o.smooth = false); + }); + + snapFunc = _isFunction$4(snap.snapTo) ? snap.snapTo : snap.snapTo === "labels" ? _getClosestLabel(animation) : snap.snapTo === "labelsDirectional" ? _getLabelAtDirection(animation) : snap.directional !== false ? function (value, st) { + return _snapDirectional(snap.snapTo)(value, _getTime$2() - lastRefresh < 500 ? 0 : st.direction); + } : gsap$b.utils.snap(snap.snapTo); + snapDurClamp = snap.duration || { + min: 0.1, + max: 2 + }; + snapDurClamp = _isObject$2(snapDurClamp) ? _clamp$2(snapDurClamp.min, snapDurClamp.max) : _clamp$2(snapDurClamp, snapDurClamp); + snapDelayedCall = gsap$b.delayedCall(snap.delay || scrubSmooth / 2 || 0.1, function () { + var scroll = scrollFunc(), + refreshedRecently = _getTime$2() - lastRefresh < 500, + tween = tweenTo.tween; + + if ((refreshedRecently || Math.abs(self.getVelocity()) < 10) && !tween && !_pointerIsDown && lastSnap !== scroll) { + var progress = (scroll - start) / change, + totalProgress = animation && !isToggle ? animation.totalProgress() : progress, + velocity = refreshedRecently ? 0 : (totalProgress - snap2) / (_getTime$2() - _time2) * 1000 || 0, + change1 = gsap$b.utils.clamp(-progress, 1 - progress, _abs$1(velocity / 2) * velocity / 0.185), + naturalEnd = progress + (snap.inertia === false ? 0 : change1), + endValue, + endScroll, + _snap = snap, + onStart = _snap.onStart, + _onInterrupt = _snap.onInterrupt, + _onComplete = _snap.onComplete; + endValue = snapFunc(naturalEnd, self); + _isNumber$2(endValue) || (endValue = naturalEnd); + endScroll = Math.max(0, Math.round(start + endValue * change)); + + if (scroll <= end && scroll >= start && endScroll !== scroll) { + if (tween && !tween._initted && tween.data <= _abs$1(endScroll - scroll)) { + return; + } + + if (snap.inertia === false) { + change1 = endValue - progress; + } + + tweenTo(endScroll, { + duration: snapDurClamp(_abs$1(Math.max(_abs$1(naturalEnd - totalProgress), _abs$1(endValue - totalProgress)) * 0.185 / velocity / 0.05 || 0)), + ease: snap.ease || "power3", + data: _abs$1(endScroll - scroll), + onInterrupt: function onInterrupt() { + return snapDelayedCall.restart(true) && _onInterrupt && _onInterrupt(self); + }, + onComplete: function onComplete() { + self.update(); + lastSnap = scrollFunc(); + + if (animation && !isToggle) { + scrubTween ? scrubTween.resetTo("totalProgress", endValue, animation._tTime / animation._tDur) : animation.progress(endValue); + } + + snap1 = snap2 = animation && !isToggle ? animation.totalProgress() : self.progress; + onSnapComplete && onSnapComplete(self); + _onComplete && _onComplete(self); + } + }, scroll, change1 * change, endScroll - scroll - change1 * change); + onStart && onStart(self, tweenTo.tween); + } + } else if (self.isActive && lastSnap !== scroll) { + snapDelayedCall.restart(true); + } + }).pause(); + } + + id && (_ids[id] = self); + trigger = self.trigger = _getTarget(trigger || pin !== true && pin); + customRevertReturn = trigger && trigger._gsap && trigger._gsap.stRevert; + customRevertReturn && (customRevertReturn = customRevertReturn(self)); + pin = pin === true ? trigger : _getTarget(pin); + _isString$4(toggleClass) && (toggleClass = { + targets: trigger, + className: toggleClass + }); + + if (pin) { + pinSpacing === false || pinSpacing === _margin || (pinSpacing = !pinSpacing && pin.parentNode && pin.parentNode.style && _getComputedStyle$1(pin.parentNode).display === "flex" ? false : _padding); + self.pin = pin; + pinCache = gsap$b.core.getCache(pin); + + if (!pinCache.spacer) { + if (pinSpacer) { + pinSpacer = _getTarget(pinSpacer); + pinSpacer && !pinSpacer.nodeType && (pinSpacer = pinSpacer.current || pinSpacer.nativeElement); + pinCache.spacerIsNative = !!pinSpacer; + pinSpacer && (pinCache.spacerState = _getState(pinSpacer)); + } + + pinCache.spacer = spacer = pinSpacer || _doc$6.createElement("div"); + spacer.classList.add("pin-spacer"); + id && spacer.classList.add("pin-spacer-" + id); + pinCache.pinState = pinOriginalState = _getState(pin); + } else { + pinOriginalState = pinCache.pinState; + } + + vars.force3D !== false && gsap$b.set(pin, { + force3D: true + }); + self.spacer = spacer = pinCache.spacer; + cs = _getComputedStyle$1(pin); + spacingStart = cs[pinSpacing + direction.os2]; + pinGetter = gsap$b.getProperty(pin); + pinSetter = gsap$b.quickSetter(pin, direction.a, _px); + + _swapPinIn(pin, spacer, cs); + + pinState = _getState(pin); + } + + if (markers) { + markerVars = _isObject$2(markers) ? _setDefaults$2(markers, _markerDefaults) : _markerDefaults; + markerStartTrigger = _createMarker("scroller-start", id, scroller, direction, markerVars, 0); + markerEndTrigger = _createMarker("scroller-end", id, scroller, direction, markerVars, 0, markerStartTrigger); + offset = markerStartTrigger["offset" + direction.op.d2]; + + var content = _getTarget(_getProxyProp(scroller, "content") || scroller); + + markerStart = this.markerStart = _createMarker("start", id, content, direction, markerVars, offset, 0, containerAnimation); + markerEnd = this.markerEnd = _createMarker("end", id, content, direction, markerVars, offset, 0, containerAnimation); + containerAnimation && (caMarkerSetter = gsap$b.quickSetter([markerStart, markerEnd], direction.a, _px)); + + if (!useFixedPosition && !(exports._proxies.length && _getProxyProp(scroller, "fixedMarkers") === true)) { + _makePositionable(isViewport ? _body$5 : scroller); + + gsap$b.set([markerStartTrigger, markerEndTrigger], { + force3D: true + }); + markerStartSetter = gsap$b.quickSetter(markerStartTrigger, direction.a, _px); + markerEndSetter = gsap$b.quickSetter(markerEndTrigger, direction.a, _px); + } + } + + if (containerAnimation) { + var oldOnUpdate = containerAnimation.vars.onUpdate, + oldParams = containerAnimation.vars.onUpdateParams; + containerAnimation.eventCallback("onUpdate", function () { + self.update(0, 0, 1); + oldOnUpdate && oldOnUpdate.apply(containerAnimation, oldParams || []); + }); + } + + self.previous = function () { + return _triggers[_triggers.indexOf(self) - 1]; + }; + + self.next = function () { + return _triggers[_triggers.indexOf(self) + 1]; + }; + + self.revert = function (revert, temp) { + if (!temp) { + return self.kill(true); + } + + var r = revert !== false || !self.enabled, + prevRefreshing = _refreshing; + + if (r !== self.isReverted) { + if (r) { + prevScroll = Math.max(scrollFunc(), self.scroll.rec || 0); + prevProgress = self.progress; + prevAnimProgress = animation && animation.progress(); + } + + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(function (m) { + return m.style.display = r ? "none" : "block"; + }); + + if (r) { + _refreshing = self; + self.update(r); + } + + if (pin && (!pinReparent || !self.isActive)) { + if (r) { + _swapPinOut(pin, spacer, pinOriginalState); + } else { + _swapPinIn(pin, spacer, _getComputedStyle$1(pin), spacerState); + } + } + + r || self.update(r); + _refreshing = prevRefreshing; + self.isReverted = r; + } + }; + + self.refresh = function (soft, force, position, pinOffset) { + if ((_refreshing || !self.enabled) && !force) { + return; + } + + if (pin && soft && _lastScrollTime) { + _addListener$2(ScrollTrigger, "scrollEnd", _softRefresh); + + return; + } + + !_refreshingAll && onRefreshInit && onRefreshInit(self); + _refreshing = self; + + if (tweenTo.tween && !position) { + tweenTo.tween.kill(); + tweenTo.tween = 0; + } + + scrubTween && scrubTween.pause(); + invalidateOnRefresh && animation && animation.revert({ + kill: false + }).invalidate(); + self.isReverted || self.revert(true, true); + self._subPinOffset = false; + + var size = getScrollerSize(), + scrollerBounds = getScrollerOffsets(), + max = containerAnimation ? containerAnimation.duration() : _maxScroll(scroller, direction), + isFirstRefresh = change <= 0.01, + offset = 0, + otherPinOffset = pinOffset || 0, + parsedEnd = _isObject$2(position) ? position.end : vars.end, + parsedEndTrigger = vars.endTrigger || trigger, + parsedStart = _isObject$2(position) ? position.start : vars.start || (vars.start === 0 || !trigger ? 0 : pin ? "0 0" : "0 100%"), + pinnedContainer = self.pinnedContainer = vars.pinnedContainer && _getTarget(vars.pinnedContainer, self), + triggerIndex = trigger && Math.max(0, _triggers.indexOf(self)) || 0, + i = triggerIndex, + cs, + bounds, + scroll, + isVertical, + override, + curTrigger, + curPin, + oppositeScroll, + initted, + revertedPins, + forcedOverflow, + markerStartOffset, + markerEndOffset; + + if (markers && _isObject$2(position)) { + markerStartOffset = gsap$b.getProperty(markerStartTrigger, direction.p); + markerEndOffset = gsap$b.getProperty(markerEndTrigger, direction.p); + } + + while (i-- > 0) { + curTrigger = _triggers[i]; + curTrigger.end || curTrigger.refresh(0, 1) || (_refreshing = self); + curPin = curTrigger.pin; + + if (curPin && (curPin === trigger || curPin === pin || curPin === pinnedContainer) && !curTrigger.isReverted) { + revertedPins || (revertedPins = []); + revertedPins.unshift(curTrigger); + curTrigger.revert(true, true); + } + + if (curTrigger !== _triggers[i]) { + triggerIndex--; + i--; + } + } + + _isFunction$4(parsedStart) && (parsedStart = parsedStart(self)); + parsedStart = _parseClamp(parsedStart, "start", self); + start = _parsePosition$1(parsedStart, trigger, size, direction, scrollFunc(), markerStart, markerStartTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._startClamp && "_startClamp") || (pin ? -0.001 : 0); + _isFunction$4(parsedEnd) && (parsedEnd = parsedEnd(self)); + + if (_isString$4(parsedEnd) && !parsedEnd.indexOf("+=")) { + if (~parsedEnd.indexOf(" ")) { + parsedEnd = (_isString$4(parsedStart) ? parsedStart.split(" ")[0] : "") + parsedEnd; + } else { + offset = _offsetToPx(parsedEnd.substr(2), size); + parsedEnd = _isString$4(parsedStart) ? parsedStart : (containerAnimation ? gsap$b.utils.mapRange(0, containerAnimation.duration(), containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, start) : start) + offset; + parsedEndTrigger = trigger; + } + } + + parsedEnd = _parseClamp(parsedEnd, "end", self); + end = Math.max(start, _parsePosition$1(parsedEnd || (parsedEndTrigger ? "100% 0" : max), parsedEndTrigger, size, direction, scrollFunc() + offset, markerEnd, markerEndTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._endClamp && "_endClamp")) || -0.001; + offset = 0; + i = triggerIndex; + + while (i--) { + curTrigger = _triggers[i]; + curPin = curTrigger.pin; + + if (curPin && curTrigger.start - curTrigger._pinPush <= start && !containerAnimation && curTrigger.end > 0) { + cs = curTrigger.end - (self._startClamp ? Math.max(0, curTrigger.start) : curTrigger.start); + + if ((curPin === trigger && curTrigger.start - curTrigger._pinPush < start || curPin === pinnedContainer) && isNaN(parsedStart)) { + offset += cs * (1 - curTrigger.progress); + } + + curPin === pin && (otherPinOffset += cs); + } + } + + start += offset; + end += offset; + self._startClamp && (self._startClamp += offset); + + if (self._endClamp && !_refreshingAll) { + self._endClamp = end || -0.001; + end = Math.min(end, _maxScroll(scroller, direction)); + } + + change = end - start || (start -= 0.01) && 0.001; + + if (isFirstRefresh) { + prevProgress = gsap$b.utils.clamp(0, 1, gsap$b.utils.normalize(start, end, prevScroll)); + } + + self._pinPush = otherPinOffset; + + if (markerStart && offset) { + cs = {}; + cs[direction.a] = "+=" + offset; + pinnedContainer && (cs[direction.p] = "-=" + scrollFunc()); + gsap$b.set([markerStart, markerEnd], cs); + } + + if (pin && !(_clampingMax && self.end >= _maxScroll(scroller, direction))) { + cs = _getComputedStyle$1(pin); + isVertical = direction === _vertical; + scroll = scrollFunc(); + pinStart = parseFloat(pinGetter(direction.a)) + otherPinOffset; + + if (!max && end > 1) { + forcedOverflow = (isViewport ? _doc$6.scrollingElement || _docEl$2 : scroller).style; + forcedOverflow = { + style: forcedOverflow, + value: forcedOverflow["overflow" + direction.a.toUpperCase()] + }; + + if (isViewport && _getComputedStyle$1(_body$5)["overflow" + direction.a.toUpperCase()] !== "scroll") { + forcedOverflow.style["overflow" + direction.a.toUpperCase()] = "scroll"; + } + } + + _swapPinIn(pin, spacer, cs); + + pinState = _getState(pin); + bounds = _getBounds$1(pin, true); + oppositeScroll = useFixedPosition && _getScrollFunc(scroller, isVertical ? _horizontal : _vertical)(); + + if (pinSpacing) { + spacerState = [pinSpacing + direction.os2, change + otherPinOffset + _px]; + spacerState.t = spacer; + i = pinSpacing === _padding ? _getSize(pin, direction) + change + otherPinOffset : 0; + + if (i) { + spacerState.push(direction.d, i + _px); + spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + + _setState(spacerState); + + if (pinnedContainer) { + _triggers.forEach(function (t) { + if (t.pin === pinnedContainer && t.vars.pinSpacing !== false) { + t._subPinOffset = true; + } + }); + } + + useFixedPosition && scrollFunc(prevScroll); + } else { + i = _getSize(pin, direction); + i && spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + + if (useFixedPosition) { + override = { + top: bounds.top + (isVertical ? scroll - start : oppositeScroll) + _px, + left: bounds.left + (isVertical ? oppositeScroll : scroll - start) + _px, + boxSizing: "border-box", + position: "fixed" + }; + override[_width] = override["max" + _Width] = Math.ceil(bounds.width) + _px; + override[_height] = override["max" + _Height] = Math.ceil(bounds.height) + _px; + override[_margin] = override[_margin + _Top] = override[_margin + _Right] = override[_margin + _Bottom] = override[_margin + _Left] = "0"; + override[_padding] = cs[_padding]; + override[_padding + _Top] = cs[_padding + _Top]; + override[_padding + _Right] = cs[_padding + _Right]; + override[_padding + _Bottom] = cs[_padding + _Bottom]; + override[_padding + _Left] = cs[_padding + _Left]; + pinActiveState = _copyState(pinOriginalState, override, pinReparent); + _refreshingAll && scrollFunc(0); + } + + if (animation) { + initted = animation._initted; + + _suppressOverwrites$1(1); + + animation.render(animation.duration(), true, true); + pinChange = pinGetter(direction.a) - pinStart + change + otherPinOffset; + pinMoves = Math.abs(change - pinChange) > 1; + useFixedPosition && pinMoves && pinActiveState.splice(pinActiveState.length - 2, 2); + animation.render(0, true, true); + initted || animation.invalidate(true); + animation.parent || animation.totalTime(animation.totalTime()); + + _suppressOverwrites$1(0); + } else { + pinChange = change; + } + + forcedOverflow && (forcedOverflow.value ? forcedOverflow.style["overflow" + direction.a.toUpperCase()] = forcedOverflow.value : forcedOverflow.style.removeProperty("overflow-" + direction.a)); + } else if (trigger && scrollFunc() && !containerAnimation) { + bounds = trigger.parentNode; + + while (bounds && bounds !== _body$5) { + if (bounds._pinOffset) { + start -= bounds._pinOffset; + end -= bounds._pinOffset; + } + + bounds = bounds.parentNode; + } + } + + revertedPins && revertedPins.forEach(function (t) { + return t.revert(false, true); + }); + self.start = start; + self.end = end; + scroll1 = scroll2 = _refreshingAll ? prevScroll : scrollFunc(); + + if (!containerAnimation && !_refreshingAll) { + scroll1 < prevScroll && scrollFunc(prevScroll); + self.scroll.rec = 0; + } + + self.revert(false, true); + lastRefresh = _getTime$2(); + + if (snapDelayedCall) { + lastSnap = -1; + snapDelayedCall.restart(true); + } + + _refreshing = 0; + animation && isToggle && (animation._initted || prevAnimProgress) && animation.progress() !== prevAnimProgress && animation.progress(prevAnimProgress || 0, true).render(animation.time(), true, true); + + if (isFirstRefresh || prevProgress !== self.progress || containerAnimation || invalidateOnRefresh || animation && !animation._initted) { + animation && !isToggle && animation.totalProgress(containerAnimation && start < -0.001 && !prevProgress ? gsap$b.utils.normalize(start, end, 0) : prevProgress, true); + self.progress = isFirstRefresh || (scroll1 - start) / change === prevProgress ? 0 : prevProgress; + } + + pin && pinSpacing && (spacer._pinOffset = Math.round(self.progress * pinChange)); + scrubTween && scrubTween.invalidate(); + + if (!isNaN(markerStartOffset)) { + markerStartOffset -= gsap$b.getProperty(markerStartTrigger, direction.p); + markerEndOffset -= gsap$b.getProperty(markerEndTrigger, direction.p); + + _shiftMarker(markerStartTrigger, direction, markerStartOffset); + + _shiftMarker(markerStart, direction, markerStartOffset - (pinOffset || 0)); + + _shiftMarker(markerEndTrigger, direction, markerEndOffset); + + _shiftMarker(markerEnd, direction, markerEndOffset - (pinOffset || 0)); + } + + isFirstRefresh && !_refreshingAll && self.update(); + + if (onRefresh && !_refreshingAll && !executingOnRefresh) { + executingOnRefresh = true; + onRefresh(self); + executingOnRefresh = false; + } + }; + + self.getVelocity = function () { + return (scrollFunc() - scroll2) / (_getTime$2() - _time2) * 1000 || 0; + }; + + self.endAnimation = function () { + _endAnimation(self.callbackAnimation); + + if (animation) { + scrubTween ? scrubTween.progress(1) : !animation.paused() ? _endAnimation(animation, animation.reversed()) : isToggle || _endAnimation(animation, self.direction < 0, 1); + } + }; + + self.labelToScroll = function (label) { + return animation && animation.labels && (start || self.refresh() || start) + animation.labels[label] / animation.duration() * change || 0; + }; + + self.getTrailing = function (name) { + var i = _triggers.indexOf(self), + a = self.direction > 0 ? _triggers.slice(0, i).reverse() : _triggers.slice(i + 1); + + return (_isString$4(name) ? a.filter(function (t) { + return t.vars.preventOverlaps === name; + }) : a).filter(function (t) { + return self.direction > 0 ? t.end <= start : t.start >= end; + }); + }; + + self.update = function (reset, recordVelocity, forceFake) { + if (containerAnimation && !forceFake && !reset) { + return; + } + + var scroll = _refreshingAll === true ? prevScroll : self.scroll(), + p = reset ? 0 : (scroll - start) / change, + clipped = p < 0 ? 0 : p > 1 ? 1 : p || 0, + prevProgress = self.progress, + isActive, + wasActive, + toggleState, + action, + stateChanged, + toggled, + isAtMax, + isTakingAction; + + if (recordVelocity) { + scroll2 = scroll1; + scroll1 = containerAnimation ? scrollFunc() : scroll; + + if (snap) { + snap2 = snap1; + snap1 = animation && !isToggle ? animation.totalProgress() : clipped; + } + } + + if (anticipatePin && pin && !_refreshing && !_startup$1 && _lastScrollTime) { + if (!clipped && start < scroll + (scroll - scroll2) / (_getTime$2() - _time2) * anticipatePin) { + clipped = 0.0001; + } else if (clipped === 1 && end > scroll + (scroll - scroll2) / (_getTime$2() - _time2) * anticipatePin) { + clipped = 0.9999; + } + } + + if (clipped !== prevProgress && self.enabled) { + isActive = self.isActive = !!clipped && clipped < 1; + wasActive = !!prevProgress && prevProgress < 1; + toggled = isActive !== wasActive; + stateChanged = toggled || !!clipped !== !!prevProgress; + self.direction = clipped > prevProgress ? 1 : -1; + self.progress = clipped; + + if (stateChanged && !_refreshing) { + toggleState = clipped && !prevProgress ? 0 : clipped === 1 ? 1 : prevProgress === 1 ? 2 : 3; + + if (isToggle) { + action = !toggled && toggleActions[toggleState + 1] !== "none" && toggleActions[toggleState + 1] || toggleActions[toggleState]; + isTakingAction = animation && (action === "complete" || action === "reset" || action in animation); + } + } + + preventOverlaps && (toggled || isTakingAction) && (isTakingAction || scrub || !animation) && (_isFunction$4(preventOverlaps) ? preventOverlaps(self) : self.getTrailing(preventOverlaps).forEach(function (t) { + return t.endAnimation(); + })); + + if (!isToggle) { + if (scrubTween && !_refreshing && !_startup$1) { + scrubTween._dp._time - scrubTween._start !== scrubTween._time && scrubTween.render(scrubTween._dp._time - scrubTween._start); + + if (scrubTween.resetTo) { + scrubTween.resetTo("totalProgress", clipped, animation._tTime / animation._tDur); + } else { + scrubTween.vars.totalProgress = clipped; + scrubTween.invalidate().restart(); + } + } else if (animation) { + animation.totalProgress(clipped, !!(_refreshing && (lastRefresh || reset))); + } + } + + if (pin) { + reset && pinSpacing && (spacer.style[pinSpacing + direction.os2] = spacingStart); + + if (!useFixedPosition) { + pinSetter(_round$5(pinStart + pinChange * clipped)); + } else if (stateChanged) { + isAtMax = !reset && clipped > prevProgress && end + 1 > scroll && scroll + 1 >= _maxScroll(scroller, direction); + + if (pinReparent) { + if (!reset && (isActive || isAtMax)) { + var bounds = _getBounds$1(pin, true), + _offset = scroll - start; + + _reparent(pin, _body$5, bounds.top + (direction === _vertical ? _offset : 0) + _px, bounds.left + (direction === _vertical ? 0 : _offset) + _px); + } else { + _reparent(pin, spacer); + } + } + + _setState(isActive || isAtMax ? pinActiveState : pinState); + + pinMoves && clipped < 1 && isActive || pinSetter(pinStart + (clipped === 1 && !isAtMax ? pinChange : 0)); + } + } + + snap && !tweenTo.tween && !_refreshing && !_startup$1 && snapDelayedCall.restart(true); + toggleClass && (toggled || once && clipped && (clipped < 1 || !_limitCallbacks)) && _toArray$4(toggleClass.targets).forEach(function (el) { + return el.classList[isActive || once ? "add" : "remove"](toggleClass.className); + }); + onUpdate && !isToggle && !reset && onUpdate(self); + + if (stateChanged && !_refreshing) { + if (isToggle) { + if (isTakingAction) { + if (action === "complete") { + animation.pause().totalProgress(1); + } else if (action === "reset") { + animation.restart(true).pause(); + } else if (action === "restart") { + animation.restart(true); + } else { + animation[action](); + } + } + + onUpdate && onUpdate(self); + } + + if (toggled || !_limitCallbacks) { + onToggle && toggled && _callback$1(self, onToggle); + callbacks[toggleState] && _callback$1(self, callbacks[toggleState]); + once && (clipped === 1 ? self.kill(false, 1) : callbacks[toggleState] = 0); + + if (!toggled) { + toggleState = clipped === 1 ? 1 : 3; + callbacks[toggleState] && _callback$1(self, callbacks[toggleState]); + } + } + + if (fastScrollEnd && !isActive && Math.abs(self.getVelocity()) > (_isNumber$2(fastScrollEnd) ? fastScrollEnd : 2500)) { + _endAnimation(self.callbackAnimation); + + scrubTween ? scrubTween.progress(1) : _endAnimation(animation, action === "reverse" ? 1 : !clipped, 1); + } + } else if (isToggle && onUpdate && !_refreshing) { + onUpdate(self); + } + } + + if (markerEndSetter) { + var n = containerAnimation ? scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0) : scroll; + markerStartSetter(n + (markerStartTrigger._isFlipped ? 1 : 0)); + markerEndSetter(n); + } + + caMarkerSetter && caMarkerSetter(-scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0)); + }; + + self.enable = function (reset, refresh) { + if (!self.enabled) { + self.enabled = true; + + _addListener$2(scroller, "resize", _onResize); + + isViewport || _addListener$2(scroller, "scroll", _onScroll$1); + onRefreshInit && _addListener$2(ScrollTrigger, "refreshInit", onRefreshInit); + + if (reset !== false) { + self.progress = prevProgress = 0; + scroll1 = scroll2 = lastSnap = scrollFunc(); + } + + refresh !== false && self.refresh(); + } + }; + + self.getTween = function (snap) { + return snap && tweenTo ? tweenTo.tween : scrubTween; + }; + + self.setPositions = function (newStart, newEnd, keepClamp, pinOffset) { + if (containerAnimation) { + var st = containerAnimation.scrollTrigger, + duration = containerAnimation.duration(), + _change = st.end - st.start; + + newStart = st.start + _change * newStart / duration; + newEnd = st.start + _change * newEnd / duration; + } + + self.refresh(false, false, { + start: _keepClamp(newStart, keepClamp && !!self._startClamp), + end: _keepClamp(newEnd, keepClamp && !!self._endClamp) + }, pinOffset); + self.update(); + }; + + self.adjustPinSpacing = function (amount) { + if (spacerState && amount) { + var i = spacerState.indexOf(direction.d) + 1; + spacerState[i] = parseFloat(spacerState[i]) + amount + _px; + spacerState[1] = parseFloat(spacerState[1]) + amount + _px; + + _setState(spacerState); + } + }; + + self.disable = function (reset, allowAnimation) { + if (self.enabled) { + reset !== false && self.revert(true, true); + self.enabled = self.isActive = false; + allowAnimation || scrubTween && scrubTween.pause(); + prevScroll = 0; + pinCache && (pinCache.uncache = 1); + onRefreshInit && _removeListener$2(ScrollTrigger, "refreshInit", onRefreshInit); + + if (snapDelayedCall) { + snapDelayedCall.pause(); + tweenTo.tween && tweenTo.tween.kill() && (tweenTo.tween = 0); + } + + if (!isViewport) { + var i = _triggers.length; + + while (i--) { + if (_triggers[i].scroller === scroller && _triggers[i] !== self) { + return; + } + } + + _removeListener$2(scroller, "resize", _onResize); + + isViewport || _removeListener$2(scroller, "scroll", _onScroll$1); + } + } + }; + + self.kill = function (revert, allowAnimation) { + self.disable(revert, allowAnimation); + scrubTween && !allowAnimation && scrubTween.kill(); + id && delete _ids[id]; + + var i = _triggers.indexOf(self); + + i >= 0 && _triggers.splice(i, 1); + i === _i && _direction > 0 && _i--; + i = 0; + + _triggers.forEach(function (t) { + return t.scroller === self.scroller && (i = 1); + }); + + i || _refreshingAll || (self.scroll.rec = 0); + + if (animation) { + animation.scrollTrigger = null; + revert && animation.revert({ + kill: false + }); + allowAnimation || animation.kill(); + } + + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(function (m) { + return m.parentNode && m.parentNode.removeChild(m); + }); + _primary === self && (_primary = 0); + + if (pin) { + pinCache && (pinCache.uncache = 1); + i = 0; + + _triggers.forEach(function (t) { + return t.pin === pin && i++; + }); + + i || (pinCache.spacer = 0); + } + + vars.onKill && vars.onKill(self); + }; + + _triggers.push(self); + + self.enable(false, false); + customRevertReturn && customRevertReturn(self); + + if (animation && animation.add && !change) { + var updateFunc = self.update; + + self.update = function () { + self.update = updateFunc; + exports._scrollers.cache++; + start || end || self.refresh(); + }; + + gsap$b.delayedCall(0.01, self.update); + change = 0.01; + start = end = 0; + } else { + self.refresh(); + } + + pin && _queueRefreshAll(); + }; + + ScrollTrigger.register = function register(core) { + if (!_coreInitted$8) { + gsap$b = core || _getGSAP$9(); + _windowExists$7() && window.document && ScrollTrigger.enable(); + _coreInitted$8 = _enabled; + } + + return _coreInitted$8; + }; + + ScrollTrigger.defaults = function defaults(config) { + if (config) { + for (var p in config) { + _defaults$1[p] = config[p]; + } + } + + return _defaults$1; + }; + + ScrollTrigger.disable = function disable(reset, kill) { + _enabled = 0; + + _triggers.forEach(function (trigger) { + return trigger[kill ? "kill" : "disable"](reset); + }); + + _removeListener$2(_win$6, "wheel", _onScroll$1); + + _removeListener$2(_doc$6, "scroll", _onScroll$1); + + clearInterval(_syncInterval); + + _removeListener$2(_doc$6, "touchcancel", _passThrough$1); + + _removeListener$2(_body$5, "touchstart", _passThrough$1); + + _multiListener(_removeListener$2, _doc$6, "pointerdown,touchstart,mousedown", _pointerDownHandler); + + _multiListener(_removeListener$2, _doc$6, "pointerup,touchend,mouseup", _pointerUpHandler); + + _resizeDelay.kill(); + + _iterateAutoRefresh(_removeListener$2); + + for (var i = 0; i < exports._scrollers.length; i += 3) { + _wheelListener(_removeListener$2, exports._scrollers[i], exports._scrollers[i + 1]); + + _wheelListener(_removeListener$2, exports._scrollers[i], exports._scrollers[i + 2]); + } + }; + + ScrollTrigger.enable = function enable() { + _win$6 = window; + _doc$6 = document; + _docEl$2 = _doc$6.documentElement; + _body$5 = _doc$6.body; + + if (gsap$b) { + _toArray$4 = gsap$b.utils.toArray; + _clamp$2 = gsap$b.utils.clamp; + _context$3 = gsap$b.core.context || _passThrough$1; + _suppressOverwrites$1 = gsap$b.core.suppressOverwrites || _passThrough$1; + _scrollRestoration = _win$6.history.scrollRestoration || "auto"; + _lastScroll = _win$6.pageYOffset || 0; + gsap$b.core.globals("ScrollTrigger", ScrollTrigger); + + if (_body$5) { + _enabled = 1; + _div100vh = document.createElement("div"); + _div100vh.style.height = "100vh"; + _div100vh.style.position = "absolute"; + + _refresh100vh(); + + _rafBugFix(); + + Observer.register(gsap$b); + ScrollTrigger.isTouch = Observer.isTouch; + _fixIOSBug = Observer.isTouch && /(iPad|iPhone|iPod|Mac)/g.test(navigator.userAgent); + _ignoreMobileResize = Observer.isTouch === 1; + + _addListener$2(_win$6, "wheel", _onScroll$1); + + _root$1 = [_win$6, _doc$6, _docEl$2, _body$5]; + + if (gsap$b.matchMedia) { + ScrollTrigger.matchMedia = function (vars) { + var mm = gsap$b.matchMedia(), + p; + + for (p in vars) { + mm.add(p, vars[p]); + } + + return mm; + }; + + gsap$b.addEventListener("matchMediaInit", function () { + return _revertAll(); + }); + gsap$b.addEventListener("matchMediaRevert", function () { + return _revertRecorded(); + }); + gsap$b.addEventListener("matchMedia", function () { + _refreshAll(0, 1); + + _dispatch$1("matchMedia"); + }); + gsap$b.matchMedia().add("(orientation: portrait)", function () { + _setBaseDimensions(); + + return _setBaseDimensions; + }); + } else { + console.warn("Requires GSAP 3.11.0 or later"); + } + + _setBaseDimensions(); + + _addListener$2(_doc$6, "scroll", _onScroll$1); + + var bodyHasStyle = _body$5.hasAttribute("style"), + bodyStyle = _body$5.style, + border = bodyStyle.borderTopStyle, + AnimationProto = gsap$b.core.Animation.prototype, + bounds, + i; + + AnimationProto.revert || Object.defineProperty(AnimationProto, "revert", { + value: function value() { + return this.time(-0.01, true); + } + }); + bodyStyle.borderTopStyle = "solid"; + bounds = _getBounds$1(_body$5); + _vertical.m = Math.round(bounds.top + _vertical.sc()) || 0; + _horizontal.m = Math.round(bounds.left + _horizontal.sc()) || 0; + border ? bodyStyle.borderTopStyle = border : bodyStyle.removeProperty("border-top-style"); + + if (!bodyHasStyle) { + _body$5.setAttribute("style", ""); + + _body$5.removeAttribute("style"); + } + + _syncInterval = setInterval(_sync, 250); + gsap$b.delayedCall(0.5, function () { + return _startup$1 = 0; + }); + + _addListener$2(_doc$6, "touchcancel", _passThrough$1); + + _addListener$2(_body$5, "touchstart", _passThrough$1); + + _multiListener(_addListener$2, _doc$6, "pointerdown,touchstart,mousedown", _pointerDownHandler); + + _multiListener(_addListener$2, _doc$6, "pointerup,touchend,mouseup", _pointerUpHandler); + + _transformProp$3 = gsap$b.utils.checkPrefix("transform"); + + _stateProps.push(_transformProp$3); + + _coreInitted$8 = _getTime$2(); + _resizeDelay = gsap$b.delayedCall(0.2, _refreshAll).pause(); + _autoRefresh = [_doc$6, "visibilitychange", function () { + var w = _win$6.innerWidth, + h = _win$6.innerHeight; + + if (_doc$6.hidden) { + _prevWidth = w; + _prevHeight = h; + } else if (_prevWidth !== w || _prevHeight !== h) { + _onResize(); + } + }, _doc$6, "DOMContentLoaded", _refreshAll, _win$6, "load", _refreshAll, _win$6, "resize", _onResize]; + + _iterateAutoRefresh(_addListener$2); + + _triggers.forEach(function (trigger) { + return trigger.enable(0, 1); + }); + + for (i = 0; i < exports._scrollers.length; i += 3) { + _wheelListener(_removeListener$2, exports._scrollers[i], exports._scrollers[i + 1]); + + _wheelListener(_removeListener$2, exports._scrollers[i], exports._scrollers[i + 2]); + } + } + } + }; + + ScrollTrigger.config = function config(vars) { + "limitCallbacks" in vars && (_limitCallbacks = !!vars.limitCallbacks); + var ms = vars.syncInterval; + ms && clearInterval(_syncInterval) || (_syncInterval = ms) && setInterval(_sync, ms); + "ignoreMobileResize" in vars && (_ignoreMobileResize = ScrollTrigger.isTouch === 1 && vars.ignoreMobileResize); + + if ("autoRefreshEvents" in vars) { + _iterateAutoRefresh(_removeListener$2) || _iterateAutoRefresh(_addListener$2, vars.autoRefreshEvents || "none"); + _ignoreResize = (vars.autoRefreshEvents + "").indexOf("resize") === -1; + } + }; + + ScrollTrigger.scrollerProxy = function scrollerProxy(target, vars) { + var t = _getTarget(target), + i = exports._scrollers.indexOf(t), + isViewport = _isViewport$1(t); + + if (~i) { + exports._scrollers.splice(i, isViewport ? 6 : 2); + } + + if (vars) { + isViewport ? exports._proxies.unshift(_win$6, vars, _body$5, vars, _docEl$2, vars) : exports._proxies.unshift(t, vars); + } + }; + + ScrollTrigger.clearMatchMedia = function clearMatchMedia(query) { + _triggers.forEach(function (t) { + return t._ctx && t._ctx.query === query && t._ctx.kill(true, true); + }); + }; + + ScrollTrigger.isInViewport = function isInViewport(element, ratio, horizontal) { + var bounds = (_isString$4(element) ? _getTarget(element) : element).getBoundingClientRect(), + offset = bounds[horizontal ? _width : _height] * ratio || 0; + return horizontal ? bounds.right - offset > 0 && bounds.left + offset < _win$6.innerWidth : bounds.bottom - offset > 0 && bounds.top + offset < _win$6.innerHeight; + }; + + ScrollTrigger.positionInViewport = function positionInViewport(element, referencePoint, horizontal) { + _isString$4(element) && (element = _getTarget(element)); + var bounds = element.getBoundingClientRect(), + size = bounds[horizontal ? _width : _height], + offset = referencePoint == null ? size / 2 : referencePoint in _keywords ? _keywords[referencePoint] * size : ~referencePoint.indexOf("%") ? parseFloat(referencePoint) * size / 100 : parseFloat(referencePoint) || 0; + return horizontal ? (bounds.left + offset) / _win$6.innerWidth : (bounds.top + offset) / _win$6.innerHeight; + }; + + ScrollTrigger.killAll = function killAll(allowListeners) { + _triggers.slice(0).forEach(function (t) { + return t.vars.id !== "ScrollSmoother" && t.kill(); + }); + + if (allowListeners !== true) { + var listeners = _listeners$1.killAll || []; + _listeners$1 = {}; + listeners.forEach(function (f) { + return f(); + }); + } + }; + + return ScrollTrigger; + }(); + ScrollTrigger$2.version = "3.12.7"; + + ScrollTrigger$2.saveStyles = function (targets) { + return targets ? _toArray$4(targets).forEach(function (target) { + if (target && target.style) { + var i = _savedStyles.indexOf(target); + + i >= 0 && _savedStyles.splice(i, 5); + + _savedStyles.push(target, target.style.cssText, target.getBBox && target.getAttribute("transform"), gsap$b.core.getCache(target), _context$3()); + } + }) : _savedStyles; + }; + + ScrollTrigger$2.revert = function (soft, media) { + return _revertAll(!soft, media); + }; + + ScrollTrigger$2.create = function (vars, animation) { + return new ScrollTrigger$2(vars, animation); + }; + + ScrollTrigger$2.refresh = function (safe) { + return safe ? _onResize(true) : (_coreInitted$8 || ScrollTrigger$2.register()) && _refreshAll(true); + }; + + ScrollTrigger$2.update = function (force) { + return ++exports._scrollers.cache && _updateAll(force === true ? 2 : 0); + }; + + ScrollTrigger$2.clearScrollMemory = _clearScrollMemory; + + ScrollTrigger$2.maxScroll = function (element, horizontal) { + return _maxScroll(element, horizontal ? _horizontal : _vertical); + }; + + ScrollTrigger$2.getScrollFunc = function (element, horizontal) { + return _getScrollFunc(_getTarget(element), horizontal ? _horizontal : _vertical); + }; + + ScrollTrigger$2.getById = function (id) { + return _ids[id]; + }; + + ScrollTrigger$2.getAll = function () { + return _triggers.filter(function (t) { + return t.vars.id !== "ScrollSmoother"; + }); + }; + + ScrollTrigger$2.isScrolling = function () { + return !!_lastScrollTime; + }; + + ScrollTrigger$2.snapDirectional = _snapDirectional; + + ScrollTrigger$2.addEventListener = function (type, callback) { + var a = _listeners$1[type] || (_listeners$1[type] = []); + ~a.indexOf(callback) || a.push(callback); + }; + + ScrollTrigger$2.removeEventListener = function (type, callback) { + var a = _listeners$1[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); + }; + + ScrollTrigger$2.batch = function (targets, vars) { + var result = [], + varsCopy = {}, + interval = vars.interval || 0.016, + batchMax = vars.batchMax || 1e9, + proxyCallback = function proxyCallback(type, callback) { + var elements = [], + triggers = [], + delay = gsap$b.delayedCall(interval, function () { + callback(elements, triggers); + elements = []; + triggers = []; + }).pause(); + return function (self) { + elements.length || delay.restart(true); + elements.push(self.trigger); + triggers.push(self); + batchMax <= elements.length && delay.progress(1); + }; + }, + p; + + for (p in vars) { + varsCopy[p] = p.substr(0, 2) === "on" && _isFunction$4(vars[p]) && p !== "onRefreshInit" ? proxyCallback(p, vars[p]) : vars[p]; + } + + if (_isFunction$4(batchMax)) { + batchMax = batchMax(); + + _addListener$2(ScrollTrigger$2, "refresh", function () { + return batchMax = vars.batchMax(); + }); + } + + _toArray$4(targets).forEach(function (target) { + var config = {}; + + for (p in varsCopy) { + config[p] = varsCopy[p]; + } + + config.trigger = target; + result.push(ScrollTrigger$2.create(config)); + }); + + return result; + }; + + var _clampScrollAndGetDurationMultiplier = function _clampScrollAndGetDurationMultiplier(scrollFunc, current, end, max) { + current > max ? scrollFunc(max) : current < 0 && scrollFunc(0); + return end > max ? (max - current) / (end - current) : end < 0 ? current / (current - end) : 1; + }, + _allowNativePanning = function _allowNativePanning(target, direction) { + if (direction === true) { + target.style.removeProperty("touch-action"); + } else { + target.style.touchAction = direction === true ? "auto" : direction ? "pan-" + direction + (Observer.isTouch ? " pinch-zoom" : "") : "none"; + } + + target === _docEl$2 && _allowNativePanning(_body$5, direction); + }, + _overflow = { + auto: 1, + scroll: 1 + }, + _nestedScroll = function _nestedScroll(_ref5) { + var event = _ref5.event, + target = _ref5.target, + axis = _ref5.axis; + + var node = (event.changedTouches ? event.changedTouches[0] : event).target, + cache = node._gsap || gsap$b.core.getCache(node), + time = _getTime$2(), + cs; + + if (!cache._isScrollT || time - cache._isScrollT > 2000) { + while (node && node !== _body$5 && (node.scrollHeight <= node.clientHeight && node.scrollWidth <= node.clientWidth || !(_overflow[(cs = _getComputedStyle$1(node)).overflowY] || _overflow[cs.overflowX]))) { + node = node.parentNode; + } + + cache._isScroll = node && node !== target && !_isViewport$1(node) && (_overflow[(cs = _getComputedStyle$1(node)).overflowY] || _overflow[cs.overflowX]); + cache._isScrollT = time; + } + + if (cache._isScroll || axis === "x") { + event.stopPropagation(); + event._gsapAllow = true; + } + }, + _inputObserver = function _inputObserver(target, type, inputs, nested) { + return Observer.create({ + target: target, + capture: true, + debounce: false, + lockAxis: true, + type: type, + onWheel: nested = nested && _nestedScroll, + onPress: nested, + onDrag: nested, + onScroll: nested, + onEnable: function onEnable() { + return inputs && _addListener$2(_doc$6, Observer.eventTypes[0], _captureInputs, false, true); + }, + onDisable: function onDisable() { + return _removeListener$2(_doc$6, Observer.eventTypes[0], _captureInputs, true); + } + }); + }, + _inputExp = /(input|label|select|textarea)/i, + _inputIsFocused, + _captureInputs = function _captureInputs(e) { + var isInput = _inputExp.test(e.target.tagName); + + if (isInput || _inputIsFocused) { + e._gsapAllow = true; + _inputIsFocused = isInput; + } + }, + _getScrollNormalizer = function _getScrollNormalizer(vars) { + _isObject$2(vars) || (vars = {}); + vars.preventDefault = vars.isNormalizer = vars.allowClicks = true; + vars.type || (vars.type = "wheel,touch"); + vars.debounce = !!vars.debounce; + vars.id = vars.id || "normalizer"; + + var _vars2 = vars, + normalizeScrollX = _vars2.normalizeScrollX, + momentum = _vars2.momentum, + allowNestedScroll = _vars2.allowNestedScroll, + onRelease = _vars2.onRelease, + self, + maxY, + target = _getTarget(vars.target) || _docEl$2, + smoother = gsap$b.core.globals().ScrollSmoother, + smootherInstance = smoother && smoother.get(), + content = _fixIOSBug && (vars.content && _getTarget(vars.content) || smootherInstance && vars.content !== false && !smootherInstance.smooth() && smootherInstance.content()), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollFuncX = _getScrollFunc(target, _horizontal), + scale = 1, + initialScale = (Observer.isTouch && _win$6.visualViewport ? _win$6.visualViewport.scale * _win$6.visualViewport.width : _win$6.outerWidth) / _win$6.innerWidth, + wheelRefresh = 0, + resolveMomentumDuration = _isFunction$4(momentum) ? function () { + return momentum(self); + } : function () { + return momentum || 2.8; + }, + lastRefreshID, + skipTouchMove, + inputObserver = _inputObserver(target, vars.type, true, allowNestedScroll), + resumeTouchMove = function resumeTouchMove() { + return skipTouchMove = false; + }, + scrollClampX = _passThrough$1, + scrollClampY = _passThrough$1, + updateClamps = function updateClamps() { + maxY = _maxScroll(target, _vertical); + scrollClampY = _clamp$2(_fixIOSBug ? 1 : 0, maxY); + normalizeScrollX && (scrollClampX = _clamp$2(0, _maxScroll(target, _horizontal))); + lastRefreshID = _refreshID; + }, + removeContentOffset = function removeContentOffset() { + content._gsap.y = _round$5(parseFloat(content._gsap.y) + scrollFuncY.offset) + "px"; + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + parseFloat(content._gsap.y) + ", 0, 1)"; + scrollFuncY.offset = scrollFuncY.cacheID = 0; + }, + ignoreDrag = function ignoreDrag() { + if (skipTouchMove) { + requestAnimationFrame(resumeTouchMove); + + var offset = _round$5(self.deltaY / 2), + scroll = scrollClampY(scrollFuncY.v - offset); + + if (content && scroll !== scrollFuncY.v + scrollFuncY.offset) { + scrollFuncY.offset = scroll - scrollFuncY.v; + + var y = _round$5((parseFloat(content && content._gsap.y) || 0) - scrollFuncY.offset); + + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + y + ", 0, 1)"; + content._gsap.y = y + "px"; + scrollFuncY.cacheID = exports._scrollers.cache; + + _updateAll(); + } + + return true; + } + + scrollFuncY.offset && removeContentOffset(); + skipTouchMove = true; + }, + tween, + startScrollX, + startScrollY, + onStopDelayedCall, + onResize = function onResize() { + updateClamps(); + + if (tween.isActive() && tween.vars.scrollY > maxY) { + scrollFuncY() > maxY ? tween.progress(1) && scrollFuncY(maxY) : tween.resetTo("scrollY", maxY); + } + }; + + content && gsap$b.set(content, { + y: "+=0" + }); + + vars.ignoreCheck = function (e) { + return _fixIOSBug && e.type === "touchmove" && ignoreDrag() || scale > 1.05 && e.type !== "touchstart" || self.isGesturing || e.touches && e.touches.length > 1; + }; + + vars.onPress = function () { + skipTouchMove = false; + var prevScale = scale; + scale = _round$5((_win$6.visualViewport && _win$6.visualViewport.scale || 1) / initialScale); + tween.pause(); + prevScale !== scale && _allowNativePanning(target, scale > 1.01 ? true : normalizeScrollX ? false : "x"); + startScrollX = scrollFuncX(); + startScrollY = scrollFuncY(); + updateClamps(); + lastRefreshID = _refreshID; + }; + + vars.onRelease = vars.onGestureStart = function (self, wasDragging) { + scrollFuncY.offset && removeContentOffset(); + + if (!wasDragging) { + onStopDelayedCall.restart(true); + } else { + exports._scrollers.cache++; + var dur = resolveMomentumDuration(), + currentScroll, + endScroll; + + if (normalizeScrollX) { + currentScroll = scrollFuncX(); + endScroll = currentScroll + dur * 0.05 * -self.velocityX / 0.227; + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncX, currentScroll, endScroll, _maxScroll(target, _horizontal)); + tween.vars.scrollX = scrollClampX(endScroll); + } + + currentScroll = scrollFuncY(); + endScroll = currentScroll + dur * 0.05 * -self.velocityY / 0.227; + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncY, currentScroll, endScroll, _maxScroll(target, _vertical)); + tween.vars.scrollY = scrollClampY(endScroll); + tween.invalidate().duration(dur).play(0.01); + + if (_fixIOSBug && tween.vars.scrollY >= maxY || currentScroll >= maxY - 1) { + gsap$b.to({}, { + onUpdate: onResize, + duration: dur + }); + } + } + + onRelease && onRelease(self); + }; + + vars.onWheel = function () { + tween._ts && tween.pause(); + + if (_getTime$2() - wheelRefresh > 1000) { + lastRefreshID = 0; + wheelRefresh = _getTime$2(); + } + }; + + vars.onChange = function (self, dx, dy, xArray, yArray) { + _refreshID !== lastRefreshID && updateClamps(); + dx && normalizeScrollX && scrollFuncX(scrollClampX(xArray[2] === dx ? startScrollX + (self.startX - self.x) : scrollFuncX() + dx - xArray[1])); + + if (dy) { + scrollFuncY.offset && removeContentOffset(); + var isTouch = yArray[2] === dy, + y = isTouch ? startScrollY + self.startY - self.y : scrollFuncY() + dy - yArray[1], + yClamped = scrollClampY(y); + isTouch && y !== yClamped && (startScrollY += yClamped - y); + scrollFuncY(yClamped); + } + + (dy || dx) && _updateAll(); + }; + + vars.onEnable = function () { + _allowNativePanning(target, normalizeScrollX ? false : "x"); + + ScrollTrigger$2.addEventListener("refresh", onResize); + + _addListener$2(_win$6, "resize", onResize); + + if (scrollFuncY.smooth) { + scrollFuncY.target.style.scrollBehavior = "auto"; + scrollFuncY.smooth = scrollFuncX.smooth = false; + } + + inputObserver.enable(); + }; + + vars.onDisable = function () { + _allowNativePanning(target, true); + + _removeListener$2(_win$6, "resize", onResize); + + ScrollTrigger$2.removeEventListener("refresh", onResize); + inputObserver.kill(); + }; + + vars.lockAxis = vars.lockAxis !== false; + self = new Observer(vars); + self.iOS = _fixIOSBug; + _fixIOSBug && !scrollFuncY() && scrollFuncY(1); + _fixIOSBug && gsap$b.ticker.add(_passThrough$1); + onStopDelayedCall = self._dc; + tween = gsap$b.to(self, { + ease: "power4", + paused: true, + inherit: false, + scrollX: normalizeScrollX ? "+=0.1" : "+=0", + scrollY: "+=0.1", + modifiers: { + scrollY: _interruptionTracker(scrollFuncY, scrollFuncY(), function () { + return tween.pause(); + }) + }, + onUpdate: _updateAll, + onComplete: onStopDelayedCall.vars.onComplete + }); + return self; + }; + + ScrollTrigger$2.sort = function (func) { + if (_isFunction$4(func)) { + return _triggers.sort(func); + } + + var scroll = _win$6.pageYOffset || 0; + ScrollTrigger$2.getAll().forEach(function (t) { + return t._sortY = t.trigger ? scroll + t.trigger.getBoundingClientRect().top : t.start + _win$6.innerHeight; + }); + return _triggers.sort(func || function (a, b) { + return (a.vars.refreshPriority || 0) * -1e6 + (a.vars.containerAnimation ? 1e6 : a._sortY) - ((b.vars.containerAnimation ? 1e6 : b._sortY) + (b.vars.refreshPriority || 0) * -1e6); + }); + }; + + ScrollTrigger$2.observe = function (vars) { + return new Observer(vars); + }; + + ScrollTrigger$2.normalizeScroll = function (vars) { + if (typeof vars === "undefined") { + return _normalizer$1; + } + + if (vars === true && _normalizer$1) { + return _normalizer$1.enable(); + } + + if (vars === false) { + _normalizer$1 && _normalizer$1.kill(); + _normalizer$1 = vars; + return; + } + + var normalizer = vars instanceof Observer ? vars : _getScrollNormalizer(vars); + _normalizer$1 && _normalizer$1.target === normalizer.target && _normalizer$1.kill(); + _isViewport$1(normalizer.target) && (_normalizer$1 = normalizer); + return normalizer; + }; + + ScrollTrigger$2.core = { + _getVelocityProp: _getVelocityProp, + _inputObserver: _inputObserver, + _scrollers: exports._scrollers, + _proxies: exports._proxies, + bridge: { + ss: function ss() { + _lastScrollTime || _dispatch$1("scrollStart"); + _lastScrollTime = _getTime$2(); + }, + ref: function ref() { + return _refreshing; + } + } + }; + _getGSAP$9() && gsap$b.registerPlugin(ScrollTrigger$2); + + var _trimExp = /(?:^\s+|\s+$)/g; + var emojiExp = /([\uD800-\uDBFF][\uDC00-\uDFFF](?:[\u200D\uFE0F][\uD800-\uDBFF][\uDC00-\uDFFF]){2,}|\uD83D\uDC69(?:\u200D(?:(?:\uD83D\uDC69\u200D)?\uD83D\uDC67|(?:\uD83D\uDC69\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2642\u2640]\uFE0F|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDD27\uDCBC\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC6F\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3C-\uDD3E\uDDD6-\uDDDF])\u200D[\u2640\u2642]\uFE0F|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|(?:\u26F9|\uD83C[\uDFCC\uDFCB]|\uD83D\uDD75)(?:\uFE0F\u200D[\u2640\u2642]|(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642])\uFE0F|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\uD83D\uDC69\u200D[\u2695\u2696\u2708]|\uD83D\uDC68(?:(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708]))\uFE0F|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83D\uDC69\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|[#\*0-9]\uFE0F\u20E3|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|\uD83D\uDC68(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]))|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uD83C[\uDFFB-\uDFFF])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270A-\u270D]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC70\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDCAA\uDD74\uDD7A\uDD90\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD36\uDDD1-\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\u200D(?:(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC69\uDC6E\uDC70-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD26\uDD30-\uDD39\uDD3D\uDD3E\uDDD1-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])?|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDEEB\uDEEC\uDEF4-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u2660\u2663\u2665\u2666\u2668\u267B\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])\uFE0F)/; + function getText(e) { + var type = e.nodeType, + result = ""; + + if (type === 1 || type === 9 || type === 11) { + if (typeof e.textContent === "string") { + return e.textContent; + } else { + for (e = e.firstChild; e; e = e.nextSibling) { + result += getText(e); + } + } + } else if (type === 3 || type === 4) { + return e.nodeValue; + } + + return result; + } + function splitInnerHTML(element, delimiter, trim, preserveSpaces, unescapedCharCodes) { + var node = element.firstChild, + result = [], + s; + + while (node) { + if (node.nodeType === 3) { + s = (node.nodeValue + "").replace(/^\n+/g, ""); + + if (!preserveSpaces) { + s = s.replace(/\s+/g, " "); + } + + result.push.apply(result, emojiSafeSplit(s, delimiter, trim, preserveSpaces, unescapedCharCodes)); + } else if ((node.nodeName + "").toLowerCase() === "br") { + result[result.length - 1] += "<br>"; + } else { + result.push(node.outerHTML); + } + + node = node.nextSibling; + } + + if (!unescapedCharCodes) { + s = result.length; + + while (s--) { + result[s] === "&" && result.splice(s, 1, "&"); + } + } + + return result; + } + function emojiSafeSplit(text, delimiter, trim, preserveSpaces, unescapedCharCodes) { + text += ""; + trim && (text = text.trim ? text.trim() : text.replace(_trimExp, "")); + + if (delimiter && delimiter !== "") { + return text.replace(/>/g, ">").replace(/</g, "<").split(delimiter); + } + + var result = [], + l = text.length, + i = 0, + j, + character; + + for (; i < l; i++) { + character = text.charAt(i); + + if (character.charCodeAt(0) >= 0xD800 && character.charCodeAt(0) <= 0xDBFF || text.charCodeAt(i + 1) >= 0xFE00 && text.charCodeAt(i + 1) <= 0xFE0F) { + j = ((text.substr(i, 12).split(emojiExp) || [])[1] || "").length || 2; + character = text.substr(i, j); + result.emoji = 1; + i += j - 1; + } + + result.push(unescapedCharCodes ? character : character === ">" ? ">" : character === "<" ? "<" : preserveSpaces && character === " " && (text.charAt(i - 1) === " " || text.charAt(i + 1) === " ") ? " " : character); + } + + return result; + } + + /*! + * TextPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + + var gsap$c, + _tempDiv$2, + _getGSAP$a = function _getGSAP() { + return gsap$c || typeof window !== "undefined" && (gsap$c = window.gsap) && gsap$c.registerPlugin && gsap$c; + }; + + var TextPlugin = { + version: "3.12.7", + name: "text", + init: function init(target, value, tween) { + typeof value !== "object" && (value = { + value: value + }); + + var i = target.nodeName.toUpperCase(), + data = this, + _value = value, + newClass = _value.newClass, + oldClass = _value.oldClass, + preserveSpaces = _value.preserveSpaces, + rtl = _value.rtl, + delimiter = data.delimiter = value.delimiter || "", + fillChar = data.fillChar = value.fillChar || (value.padSpace ? " " : ""), + _short, + text, + original, + j, + condensedText, + condensedOriginal, + aggregate, + s; + + data.svg = target.getBBox && (i === "TEXT" || i === "TSPAN"); + + if (!("innerHTML" in target) && !data.svg) { + return false; + } + + data.target = target; + + if (!("value" in value)) { + data.text = data.original = [""]; + return; + } + + original = splitInnerHTML(target, delimiter, false, preserveSpaces, data.svg); + _tempDiv$2 || (_tempDiv$2 = document.createElement("div")); + _tempDiv$2.innerHTML = value.value; + text = splitInnerHTML(_tempDiv$2, delimiter, false, preserveSpaces, data.svg); + data.from = tween._from; + + if ((data.from || rtl) && !(rtl && data.from)) { + i = original; + original = text; + text = i; + } + + data.hasClass = !!(newClass || oldClass); + data.newClass = rtl ? oldClass : newClass; + data.oldClass = rtl ? newClass : oldClass; + i = original.length - text.length; + _short = i < 0 ? original : text; + + if (i < 0) { + i = -i; + } + + while (--i > -1) { + _short.push(fillChar); + } + + if (value.type === "diff") { + j = 0; + condensedText = []; + condensedOriginal = []; + aggregate = ""; + + for (i = 0; i < text.length; i++) { + s = text[i]; + + if (s === original[i]) { + aggregate += s; + } else { + condensedText[j] = aggregate + s; + condensedOriginal[j++] = aggregate + original[i]; + aggregate = ""; + } + } + + text = condensedText; + original = condensedOriginal; + + if (aggregate) { + text.push(aggregate); + original.push(aggregate); + } + } + + value.speed && tween.duration(Math.min(0.05 / value.speed * _short.length, value.maxDuration || 9999)); + data.rtl = rtl; + data.original = original; + data.text = text; + + data._props.push("text"); + }, + render: function render(ratio, data) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + + if (data.from) { + ratio = 1 - ratio; + } + + var text = data.text, + hasClass = data.hasClass, + newClass = data.newClass, + oldClass = data.oldClass, + delimiter = data.delimiter, + target = data.target, + fillChar = data.fillChar, + original = data.original, + rtl = data.rtl, + l = text.length, + i = (rtl ? 1 - ratio : ratio) * l + 0.5 | 0, + applyNew, + applyOld, + str; + + if (hasClass && ratio) { + applyNew = newClass && i; + applyOld = oldClass && i !== l; + str = (applyNew ? "<span class='" + newClass + "'>" : "") + text.slice(0, i).join(delimiter) + (applyNew ? "</span>" : "") + (applyOld ? "<span class='" + oldClass + "'>" : "") + delimiter + original.slice(i).join(delimiter) + (applyOld ? "</span>" : ""); + } else { + str = text.slice(0, i).join(delimiter) + delimiter + original.slice(i).join(delimiter); + } + + if (data.svg) { + target.textContent = str; + } else { + target.innerHTML = fillChar === " " && ~str.indexOf(" ") ? str.split(" ").join(" ") : str; + } + } + }; + TextPlugin.splitInnerHTML = splitInnerHTML; + TextPlugin.emojiSafeSplit = emojiSafeSplit; + TextPlugin.getText = getText; + _getGSAP$a() && gsap$c.registerPlugin(TextPlugin); + + var gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, + TweenMaxWithCSS = gsapWithCSS.core.Tween; + + exports.Back = Back; + exports.Bounce = Bounce; + exports.CSSPlugin = CSSPlugin; + exports.CSSRulePlugin = CSSRulePlugin; + exports.Circ = Circ; + exports.Cubic = Cubic; + exports.CustomEase = CustomEase; + exports.Draggable = Draggable; + exports.EasePack = EasePack; + exports.EaselPlugin = EaselPlugin; + exports.Elastic = Elastic; + exports.Expo = Expo; + exports.ExpoScaleEase = ExpoScaleEase; + exports.Flip = Flip; + exports.Linear = Linear; + exports.MotionPathPlugin = MotionPathPlugin; + exports.Observer = Observer; + exports.PixiPlugin = PixiPlugin; + exports.Power0 = Power0; + exports.Power1 = Power1; + exports.Power2 = Power2; + exports.Power3 = Power3; + exports.Power4 = Power4; + exports.Quad = Quad; + exports.Quart = Quart; + exports.Quint = Quint; + exports.RoughEase = RoughEase; + exports.ScrollToPlugin = ScrollToPlugin; + exports.ScrollTrigger = ScrollTrigger$2; + exports.Sine = Sine; + exports.SlowMo = SlowMo; + exports.SteppedEase = SteppedEase; + exports.Strong = Strong; + exports.TextPlugin = TextPlugin; + exports.TimelineLite = Timeline; + exports.TimelineMax = Timeline; + exports.TweenLite = Tween; + exports.TweenMax = TweenMaxWithCSS; + exports._getProxyProp = _getProxyProp; + exports._getScrollFunc = _getScrollFunc; + exports._getTarget = _getTarget; + exports._getVelocityProp = _getVelocityProp; + exports._horizontal = _horizontal; + exports._isViewport = _isViewport; + exports._vertical = _vertical; + exports.clamp = clamp; + exports.default = gsapWithCSS; + exports.distribute = distribute; + exports.getUnit = getUnit; + exports.gsap = gsapWithCSS; + exports.interpolate = interpolate; + exports.mapRange = mapRange; + exports.normalize = normalize; + exports.pipe = pipe; + exports.random = random; + exports.selector = selector; + exports.shuffle = shuffle; + exports.snap = snap; + exports.splitColor = splitColor; + exports.toArray = toArray; + exports.unitize = unitize; + exports.wrap = wrap; + exports.wrapYoyo = wrapYoyo; + + Object.defineProperty(exports, '__esModule', { value: true }); + +}))); diff --git a/node_modules/gsap/dist/gsap.js b/node_modules/gsap/dist/gsap.js new file mode 100644 index 0000000000000000000000000000000000000000..90293392382713081d0e4d5f3e19791cca5c7f5e --- /dev/null +++ b/node_modules/gsap/dist/gsap.js @@ -0,0 +1,5616 @@ +(function (global, factory) { + typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) : + typeof define === 'function' && define.amd ? define(['exports'], factory) : + (global = global || self, factory(global.window = global.window || {})); +}(this, (function (exports) { 'use strict'; + + function _inheritsLoose(subClass, superClass) { + subClass.prototype = Object.create(superClass.prototype); + subClass.prototype.constructor = subClass; + subClass.__proto__ = superClass; + } + + function _assertThisInitialized(self) { + if (self === void 0) { + throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); + } + + return self; + } + + /*! + * GSAP 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + var _config = { + autoSleep: 120, + force3D: "auto", + nullTargetWarn: 1, + units: { + lineHeight: "" + } + }, + _defaults = { + duration: .5, + overwrite: false, + delay: 0 + }, + _suppressOverwrites, + _reverting, + _context, + _bigNum = 1e8, + _tinyNum = 1 / _bigNum, + _2PI = Math.PI * 2, + _HALF_PI = _2PI / 4, + _gsID = 0, + _sqrt = Math.sqrt, + _cos = Math.cos, + _sin = Math.sin, + _isString = function _isString(value) { + return typeof value === "string"; + }, + _isFunction = function _isFunction(value) { + return typeof value === "function"; + }, + _isNumber = function _isNumber(value) { + return typeof value === "number"; + }, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; + }, + _isObject = function _isObject(value) { + return typeof value === "object"; + }, + _isNotFalse = function _isNotFalse(value) { + return value !== false; + }, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; + }, + _isFuncOrString = function _isFuncOrString(value) { + return _isFunction(value) || _isString(value); + }, + _isTypedArray = typeof ArrayBuffer === "function" && ArrayBuffer.isView || function () {}, + _isArray = Array.isArray, + _strictNumExp = /(?:-?\.?\d|\.)+/gi, + _numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/g, + _numWithUnitExp = /[-+=.]*\d+[.e-]*\d*[a-z%]*/g, + _complexStringNumExp = /[-+=.]*\d+\.?\d*(?:e-|e\+)?\d*/gi, + _relExp = /[+-]=-?[.\d]+/, + _delimitedValueExp = /[^,'"\[\]\s]+/gi, + _unitExp = /^[+\-=e\s\d]*\d+[.\d]*([a-z]*|%)\s*$/i, + _globalTimeline, + _win, + _coreInitted, + _doc, + _globals = {}, + _installScope = {}, + _coreReady, + _install = function _install(scope) { + return (_installScope = _merge(scope, _globals)) && gsap; + }, + _missingPlugin = function _missingPlugin(property, value) { + return console.warn("Invalid property", property, "set to", value, "Missing plugin? gsap.registerPlugin()"); + }, + _warn = function _warn(message, suppress) { + return !suppress && console.warn(message); + }, + _addGlobal = function _addGlobal(name, obj) { + return name && (_globals[name] = obj) && _installScope && (_installScope[name] = obj) || _globals; + }, + _emptyFunc = function _emptyFunc() { + return 0; + }, + _startAtRevertConfig = { + suppressEvents: true, + isStart: true, + kill: false + }, + _revertConfigNoKill = { + suppressEvents: true, + kill: false + }, + _revertConfig = { + suppressEvents: true + }, + _reservedProps = {}, + _lazyTweens = [], + _lazyLookup = {}, + _lastRenderedFrame, + _plugins = {}, + _effects = {}, + _nextGCFrame = 30, + _harnessPlugins = [], + _callbackNames = "", + _harness = function _harness(targets) { + var target = targets[0], + harnessPlugin, + i; + _isObject(target) || _isFunction(target) || (targets = [targets]); + + if (!(harnessPlugin = (target._gsap || {}).harness)) { + i = _harnessPlugins.length; + + while (i-- && !_harnessPlugins[i].targetTest(target)) {} + + harnessPlugin = _harnessPlugins[i]; + } + + i = targets.length; + + while (i--) { + targets[i] && (targets[i]._gsap || (targets[i]._gsap = new GSCache(targets[i], harnessPlugin))) || targets.splice(i, 1); + } + + return targets; + }, + _getCache = function _getCache(target) { + return target._gsap || _harness(toArray(target))[0]._gsap; + }, + _getProperty = function _getProperty(target, property, v) { + return (v = target[property]) && _isFunction(v) ? target[property]() : _isUndefined(v) && target.getAttribute && target.getAttribute(property) || v; + }, + _forEachName = function _forEachName(names, func) { + return (names = names.split(",")).forEach(func) || names; + }, + _round = function _round(value) { + return Math.round(value * 100000) / 100000 || 0; + }, + _roundPrecise = function _roundPrecise(value) { + return Math.round(value * 10000000) / 10000000 || 0; + }, + _parseRelative = function _parseRelative(start, value) { + var operator = value.charAt(0), + end = parseFloat(value.substr(2)); + start = parseFloat(start); + return operator === "+" ? start + end : operator === "-" ? start - end : operator === "*" ? start * end : start / end; + }, + _arrayContainsAny = function _arrayContainsAny(toSearch, toFind) { + var l = toFind.length, + i = 0; + + for (; toSearch.indexOf(toFind[i]) < 0 && ++i < l;) {} + + return i < l; + }, + _lazyRender = function _lazyRender() { + var l = _lazyTweens.length, + a = _lazyTweens.slice(0), + i, + tween; + + _lazyLookup = {}; + _lazyTweens.length = 0; + + for (i = 0; i < l; i++) { + tween = a[i]; + tween && tween._lazy && (tween.render(tween._lazy[0], tween._lazy[1], true)._lazy = 0); + } + }, + _lazySafeRender = function _lazySafeRender(animation, time, suppressEvents, force) { + _lazyTweens.length && !_reverting && _lazyRender(); + animation.render(time, suppressEvents, force || _reverting && time < 0 && (animation._initted || animation._startAt)); + _lazyTweens.length && !_reverting && _lazyRender(); + }, + _numericIfPossible = function _numericIfPossible(value) { + var n = parseFloat(value); + return (n || n === 0) && (value + "").match(_delimitedValueExp).length < 2 ? n : _isString(value) ? value.trim() : value; + }, + _passThrough = function _passThrough(p) { + return p; + }, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + p in obj || (obj[p] = defaults[p]); + } + + return obj; + }, + _setKeyframeDefaults = function _setKeyframeDefaults(excludeDuration) { + return function (obj, defaults) { + for (var p in defaults) { + p in obj || p === "duration" && excludeDuration || p === "ease" || (obj[p] = defaults[p]); + } + }; + }, + _merge = function _merge(base, toMerge) { + for (var p in toMerge) { + base[p] = toMerge[p]; + } + + return base; + }, + _mergeDeep = function _mergeDeep(base, toMerge) { + for (var p in toMerge) { + p !== "__proto__" && p !== "constructor" && p !== "prototype" && (base[p] = _isObject(toMerge[p]) ? _mergeDeep(base[p] || (base[p] = {}), toMerge[p]) : toMerge[p]); + } + + return base; + }, + _copyExcluding = function _copyExcluding(obj, excluding) { + var copy = {}, + p; + + for (p in obj) { + p in excluding || (copy[p] = obj[p]); + } + + return copy; + }, + _inheritDefaults = function _inheritDefaults(vars) { + var parent = vars.parent || _globalTimeline, + func = vars.keyframes ? _setKeyframeDefaults(_isArray(vars.keyframes)) : _setDefaults; + + if (_isNotFalse(vars.inherit)) { + while (parent) { + func(vars, parent.vars.defaults); + parent = parent.parent || parent._dp; + } + } + + return vars; + }, + _arraysMatch = function _arraysMatch(a1, a2) { + var i = a1.length, + match = i === a2.length; + + while (match && i-- && a1[i] === a2[i]) {} + + return i < 0; + }, + _addLinkedListItem = function _addLinkedListItem(parent, child, firstProp, lastProp, sortBy) { + if (firstProp === void 0) { + firstProp = "_first"; + } + + if (lastProp === void 0) { + lastProp = "_last"; + } + + var prev = parent[lastProp], + t; + + if (sortBy) { + t = child[sortBy]; + + while (prev && prev[sortBy] > t) { + prev = prev._prev; + } + } + + if (prev) { + child._next = prev._next; + prev._next = child; + } else { + child._next = parent[firstProp]; + parent[firstProp] = child; + } + + if (child._next) { + child._next._prev = child; + } else { + parent[lastProp] = child; + } + + child._prev = prev; + child.parent = child._dp = parent; + return child; + }, + _removeLinkedListItem = function _removeLinkedListItem(parent, child, firstProp, lastProp) { + if (firstProp === void 0) { + firstProp = "_first"; + } + + if (lastProp === void 0) { + lastProp = "_last"; + } + + var prev = child._prev, + next = child._next; + + if (prev) { + prev._next = next; + } else if (parent[firstProp] === child) { + parent[firstProp] = next; + } + + if (next) { + next._prev = prev; + } else if (parent[lastProp] === child) { + parent[lastProp] = prev; + } + + child._next = child._prev = child.parent = null; + }, + _removeFromParent = function _removeFromParent(child, onlyIfParentHasAutoRemove) { + child.parent && (!onlyIfParentHasAutoRemove || child.parent.autoRemoveChildren) && child.parent.remove && child.parent.remove(child); + child._act = 0; + }, + _uncache = function _uncache(animation, child) { + if (animation && (!child || child._end > animation._dur || child._start < 0)) { + var a = animation; + + while (a) { + a._dirty = 1; + a = a.parent; + } + } + + return animation; + }, + _recacheAncestors = function _recacheAncestors(animation) { + var parent = animation.parent; + + while (parent && parent.parent) { + parent._dirty = 1; + parent.totalDuration(); + parent = parent.parent; + } + + return animation; + }, + _rewindStartAt = function _rewindStartAt(tween, totalTime, suppressEvents, force) { + return tween._startAt && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween.vars.immediateRender && !tween.vars.autoRevert || tween._startAt.render(totalTime, true, force)); + }, + _hasNoPausedAncestors = function _hasNoPausedAncestors(animation) { + return !animation || animation._ts && _hasNoPausedAncestors(animation.parent); + }, + _elapsedCycleDuration = function _elapsedCycleDuration(animation) { + return animation._repeat ? _animationCycle(animation._tTime, animation = animation.duration() + animation._rDelay) * animation : 0; + }, + _animationCycle = function _animationCycle(tTime, cycleDuration) { + var whole = Math.floor(tTime = _roundPrecise(tTime / cycleDuration)); + return tTime && whole === tTime ? whole - 1 : whole; + }, + _parentToChildTotalTime = function _parentToChildTotalTime(parentTime, child) { + return (parentTime - child._start) * child._ts + (child._ts >= 0 ? 0 : child._dirty ? child.totalDuration() : child._tDur); + }, + _setEnd = function _setEnd(animation) { + return animation._end = _roundPrecise(animation._start + (animation._tDur / Math.abs(animation._ts || animation._rts || _tinyNum) || 0)); + }, + _alignPlayhead = function _alignPlayhead(animation, totalTime) { + var parent = animation._dp; + + if (parent && parent.smoothChildTiming && animation._ts) { + animation._start = _roundPrecise(parent._time - (animation._ts > 0 ? totalTime / animation._ts : ((animation._dirty ? animation.totalDuration() : animation._tDur) - totalTime) / -animation._ts)); + + _setEnd(animation); + + parent._dirty || _uncache(parent, animation); + } + + return animation; + }, + _postAddChecks = function _postAddChecks(timeline, child) { + var t; + + if (child._time || !child._dur && child._initted || child._start < timeline._time && (child._dur || !child.add)) { + t = _parentToChildTotalTime(timeline.rawTime(), child); + + if (!child._dur || _clamp(0, child.totalDuration(), t) - child._tTime > _tinyNum) { + child.render(t, true); + } + } + + if (_uncache(timeline, child)._dp && timeline._initted && timeline._time >= timeline._dur && timeline._ts) { + if (timeline._dur < timeline.duration()) { + t = timeline; + + while (t._dp) { + t.rawTime() >= 0 && t.totalTime(t._tTime); + t = t._dp; + } + } + + timeline._zTime = -_tinyNum; + } + }, + _addToTimeline = function _addToTimeline(timeline, child, position, skipChecks) { + child.parent && _removeFromParent(child); + child._start = _roundPrecise((_isNumber(position) ? position : position || timeline !== _globalTimeline ? _parsePosition(timeline, position, child) : timeline._time) + child._delay); + child._end = _roundPrecise(child._start + (child.totalDuration() / Math.abs(child.timeScale()) || 0)); + + _addLinkedListItem(timeline, child, "_first", "_last", timeline._sort ? "_start" : 0); + + _isFromOrFromStart(child) || (timeline._recent = child); + skipChecks || _postAddChecks(timeline, child); + timeline._ts < 0 && _alignPlayhead(timeline, timeline._tTime); + return timeline; + }, + _scrollTrigger = function _scrollTrigger(animation, trigger) { + return (_globals.ScrollTrigger || _missingPlugin("scrollTrigger", trigger)) && _globals.ScrollTrigger.create(trigger, animation); + }, + _attemptInitTween = function _attemptInitTween(tween, time, force, suppressEvents, tTime) { + _initTween(tween, time, tTime); + + if (!tween._initted) { + return 1; + } + + if (!force && tween._pt && !_reverting && (tween._dur && tween.vars.lazy !== false || !tween._dur && tween.vars.lazy) && _lastRenderedFrame !== _ticker.frame) { + _lazyTweens.push(tween); + + tween._lazy = [tTime, suppressEvents]; + return 1; + } + }, + _parentPlayheadIsBeforeStart = function _parentPlayheadIsBeforeStart(_ref) { + var parent = _ref.parent; + return parent && parent._ts && parent._initted && !parent._lock && (parent.rawTime() < 0 || _parentPlayheadIsBeforeStart(parent)); + }, + _isFromOrFromStart = function _isFromOrFromStart(_ref2) { + var data = _ref2.data; + return data === "isFromStart" || data === "isStart"; + }, + _renderZeroDurationTween = function _renderZeroDurationTween(tween, totalTime, suppressEvents, force) { + var prevRatio = tween.ratio, + ratio = totalTime < 0 || !totalTime && (!tween._start && _parentPlayheadIsBeforeStart(tween) && !(!tween._initted && _isFromOrFromStart(tween)) || (tween._ts < 0 || tween._dp._ts < 0) && !_isFromOrFromStart(tween)) ? 0 : 1, + repeatDelay = tween._rDelay, + tTime = 0, + pt, + iteration, + prevIteration; + + if (repeatDelay && tween._repeat) { + tTime = _clamp(0, tween._tDur, totalTime); + iteration = _animationCycle(tTime, repeatDelay); + tween._yoyo && iteration & 1 && (ratio = 1 - ratio); + + if (iteration !== _animationCycle(tween._tTime, repeatDelay)) { + prevRatio = 1 - ratio; + tween.vars.repeatRefresh && tween._initted && tween.invalidate(); + } + } + + if (ratio !== prevRatio || _reverting || force || tween._zTime === _tinyNum || !totalTime && tween._zTime) { + if (!tween._initted && _attemptInitTween(tween, totalTime, force, suppressEvents, tTime)) { + return; + } + + prevIteration = tween._zTime; + tween._zTime = totalTime || (suppressEvents ? _tinyNum : 0); + suppressEvents || (suppressEvents = totalTime && !prevIteration); + tween.ratio = ratio; + tween._from && (ratio = 1 - ratio); + tween._time = 0; + tween._tTime = tTime; + pt = tween._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + totalTime < 0 && _rewindStartAt(tween, totalTime, suppressEvents, true); + tween._onUpdate && !suppressEvents && _callback(tween, "onUpdate"); + tTime && tween._repeat && !suppressEvents && tween.parent && _callback(tween, "onRepeat"); + + if ((totalTime >= tween._tDur || totalTime < 0) && tween.ratio === ratio) { + ratio && _removeFromParent(tween, 1); + + if (!suppressEvents && !_reverting) { + _callback(tween, ratio ? "onComplete" : "onReverseComplete", true); + + tween._prom && tween._prom(); + } + } + } else if (!tween._zTime) { + tween._zTime = totalTime; + } + }, + _findNextPauseTween = function _findNextPauseTween(animation, prevTime, time) { + var child; + + if (time > prevTime) { + child = animation._first; + + while (child && child._start <= time) { + if (child.data === "isPause" && child._start > prevTime) { + return child; + } + + child = child._next; + } + } else { + child = animation._last; + + while (child && child._start >= time) { + if (child.data === "isPause" && child._start < prevTime) { + return child; + } + + child = child._prev; + } + } + }, + _setDuration = function _setDuration(animation, duration, skipUncache, leavePlayhead) { + var repeat = animation._repeat, + dur = _roundPrecise(duration) || 0, + totalProgress = animation._tTime / animation._tDur; + totalProgress && !leavePlayhead && (animation._time *= dur / animation._dur); + animation._dur = dur; + animation._tDur = !repeat ? dur : repeat < 0 ? 1e10 : _roundPrecise(dur * (repeat + 1) + animation._rDelay * repeat); + totalProgress > 0 && !leavePlayhead && _alignPlayhead(animation, animation._tTime = animation._tDur * totalProgress); + animation.parent && _setEnd(animation); + skipUncache || _uncache(animation.parent, animation); + return animation; + }, + _onUpdateTotalDuration = function _onUpdateTotalDuration(animation) { + return animation instanceof Timeline ? _uncache(animation) : _setDuration(animation, animation._dur); + }, + _zeroPosition = { + _start: 0, + endTime: _emptyFunc, + totalDuration: _emptyFunc + }, + _parsePosition = function _parsePosition(animation, position, percentAnimation) { + var labels = animation.labels, + recent = animation._recent || _zeroPosition, + clippedDuration = animation.duration() >= _bigNum ? recent.endTime(false) : animation._dur, + i, + offset, + isPercent; + + if (_isString(position) && (isNaN(position) || position in labels)) { + offset = position.charAt(0); + isPercent = position.substr(-1) === "%"; + i = position.indexOf("="); + + if (offset === "<" || offset === ">") { + i >= 0 && (position = position.replace(/=/, "")); + return (offset === "<" ? recent._start : recent.endTime(recent._repeat >= 0)) + (parseFloat(position.substr(1)) || 0) * (isPercent ? (i < 0 ? recent : percentAnimation).totalDuration() / 100 : 1); + } + + if (i < 0) { + position in labels || (labels[position] = clippedDuration); + return labels[position]; + } + + offset = parseFloat(position.charAt(i - 1) + position.substr(i + 1)); + + if (isPercent && percentAnimation) { + offset = offset / 100 * (_isArray(percentAnimation) ? percentAnimation[0] : percentAnimation).totalDuration(); + } + + return i > 1 ? _parsePosition(animation, position.substr(0, i - 1), percentAnimation) + offset : clippedDuration + offset; + } + + return position == null ? clippedDuration : +position; + }, + _createTweenType = function _createTweenType(type, params, timeline) { + var isLegacy = _isNumber(params[1]), + varsIndex = (isLegacy ? 2 : 1) + (type < 2 ? 0 : 1), + vars = params[varsIndex], + irVars, + parent; + + isLegacy && (vars.duration = params[1]); + vars.parent = timeline; + + if (type) { + irVars = vars; + parent = timeline; + + while (parent && !("immediateRender" in irVars)) { + irVars = parent.vars.defaults || {}; + parent = _isNotFalse(parent.vars.inherit) && parent.parent; + } + + vars.immediateRender = _isNotFalse(irVars.immediateRender); + type < 2 ? vars.runBackwards = 1 : vars.startAt = params[varsIndex - 1]; + } + + return new Tween(params[0], vars, params[varsIndex + 1]); + }, + _conditionalReturn = function _conditionalReturn(value, func) { + return value || value === 0 ? func(value) : func; + }, + _clamp = function _clamp(min, max, value) { + return value < min ? min : value > max ? max : value; + }, + getUnit = function getUnit(value, v) { + return !_isString(value) || !(v = _unitExp.exec(value)) ? "" : v[1]; + }, + clamp = function clamp(min, max, value) { + return _conditionalReturn(value, function (v) { + return _clamp(min, max, v); + }); + }, + _slice = [].slice, + _isArrayLike = function _isArrayLike(value, nonEmpty) { + return value && _isObject(value) && "length" in value && (!nonEmpty && !value.length || value.length - 1 in value && _isObject(value[0])) && !value.nodeType && value !== _win; + }, + _flatten = function _flatten(ar, leaveStrings, accumulator) { + if (accumulator === void 0) { + accumulator = []; + } + + return ar.forEach(function (value) { + var _accumulator; + + return _isString(value) && !leaveStrings || _isArrayLike(value, 1) ? (_accumulator = accumulator).push.apply(_accumulator, toArray(value)) : accumulator.push(value); + }) || accumulator; + }, + toArray = function toArray(value, scope, leaveStrings) { + return _context && !scope && _context.selector ? _context.selector(value) : _isString(value) && !leaveStrings && (_coreInitted || !_wake()) ? _slice.call((scope || _doc).querySelectorAll(value), 0) : _isArray(value) ? _flatten(value, leaveStrings) : _isArrayLike(value) ? _slice.call(value, 0) : value ? [value] : []; + }, + selector = function selector(value) { + value = toArray(value)[0] || _warn("Invalid scope") || {}; + return function (v) { + var el = value.current || value.nativeElement || value; + return toArray(v, el.querySelectorAll ? el : el === value ? _warn("Invalid scope") || _doc.createElement("div") : value); + }; + }, + shuffle = function shuffle(a) { + return a.sort(function () { + return .5 - Math.random(); + }); + }, + distribute = function distribute(v) { + if (_isFunction(v)) { + return v; + } + + var vars = _isObject(v) ? v : { + each: v + }, + ease = _parseEase(vars.ease), + from = vars.from || 0, + base = parseFloat(vars.base) || 0, + cache = {}, + isDecimal = from > 0 && from < 1, + ratios = isNaN(from) || isDecimal, + axis = vars.axis, + ratioX = from, + ratioY = from; + + if (_isString(from)) { + ratioX = ratioY = { + center: .5, + edges: .5, + end: 1 + }[from] || 0; + } else if (!isDecimal && ratios) { + ratioX = from[0]; + ratioY = from[1]; + } + + return function (i, target, a) { + var l = (a || vars).length, + distances = cache[l], + originX, + originY, + x, + y, + d, + j, + max, + min, + wrapAt; + + if (!distances) { + wrapAt = vars.grid === "auto" ? 0 : (vars.grid || [1, _bigNum])[1]; + + if (!wrapAt) { + max = -_bigNum; + + while (max < (max = a[wrapAt++].getBoundingClientRect().left) && wrapAt < l) {} + + wrapAt < l && wrapAt--; + } + + distances = cache[l] = []; + originX = ratios ? Math.min(wrapAt, l) * ratioX - .5 : from % wrapAt; + originY = wrapAt === _bigNum ? 0 : ratios ? l * ratioY / wrapAt - .5 : from / wrapAt | 0; + max = 0; + min = _bigNum; + + for (j = 0; j < l; j++) { + x = j % wrapAt - originX; + y = originY - (j / wrapAt | 0); + distances[j] = d = !axis ? _sqrt(x * x + y * y) : Math.abs(axis === "y" ? y : x); + d > max && (max = d); + d < min && (min = d); + } + + from === "random" && shuffle(distances); + distances.max = max - min; + distances.min = min; + distances.v = l = (parseFloat(vars.amount) || parseFloat(vars.each) * (wrapAt > l ? l - 1 : !axis ? Math.max(wrapAt, l / wrapAt) : axis === "y" ? l / wrapAt : wrapAt) || 0) * (from === "edges" ? -1 : 1); + distances.b = l < 0 ? base - l : base; + distances.u = getUnit(vars.amount || vars.each) || 0; + ease = ease && l < 0 ? _invertEase(ease) : ease; + } + + l = (distances[i] - distances.min) / distances.max || 0; + return _roundPrecise(distances.b + (ease ? ease(l) : l) * distances.v) + distances.u; + }; + }, + _roundModifier = function _roundModifier(v) { + var p = Math.pow(10, ((v + "").split(".")[1] || "").length); + return function (raw) { + var n = _roundPrecise(Math.round(parseFloat(raw) / v) * v * p); + + return (n - n % 1) / p + (_isNumber(raw) ? 0 : getUnit(raw)); + }; + }, + snap = function snap(snapTo, value) { + var isArray = _isArray(snapTo), + radius, + is2D; + + if (!isArray && _isObject(snapTo)) { + radius = isArray = snapTo.radius || _bigNum; + + if (snapTo.values) { + snapTo = toArray(snapTo.values); + + if (is2D = !_isNumber(snapTo[0])) { + radius *= radius; + } + } else { + snapTo = _roundModifier(snapTo.increment); + } + } + + return _conditionalReturn(value, !isArray ? _roundModifier(snapTo) : _isFunction(snapTo) ? function (raw) { + is2D = snapTo(raw); + return Math.abs(is2D - raw) <= radius ? is2D : raw; + } : function (raw) { + var x = parseFloat(is2D ? raw.x : raw), + y = parseFloat(is2D ? raw.y : 0), + min = _bigNum, + closest = 0, + i = snapTo.length, + dx, + dy; + + while (i--) { + if (is2D) { + dx = snapTo[i].x - x; + dy = snapTo[i].y - y; + dx = dx * dx + dy * dy; + } else { + dx = Math.abs(snapTo[i] - x); + } + + if (dx < min) { + min = dx; + closest = i; + } + } + + closest = !radius || min <= radius ? snapTo[closest] : raw; + return is2D || closest === raw || _isNumber(raw) ? closest : closest + getUnit(raw); + }); + }, + random = function random(min, max, roundingIncrement, returnFunction) { + return _conditionalReturn(_isArray(min) ? !max : roundingIncrement === true ? !!(roundingIncrement = 0) : !returnFunction, function () { + return _isArray(min) ? min[~~(Math.random() * min.length)] : (roundingIncrement = roundingIncrement || 1e-5) && (returnFunction = roundingIncrement < 1 ? Math.pow(10, (roundingIncrement + "").length - 2) : 1) && Math.floor(Math.round((min - roundingIncrement / 2 + Math.random() * (max - min + roundingIncrement * .99)) / roundingIncrement) * roundingIncrement * returnFunction) / returnFunction; + }); + }, + pipe = function pipe() { + for (var _len = arguments.length, functions = new Array(_len), _key = 0; _key < _len; _key++) { + functions[_key] = arguments[_key]; + } + + return function (value) { + return functions.reduce(function (v, f) { + return f(v); + }, value); + }; + }, + unitize = function unitize(func, unit) { + return function (value) { + return func(parseFloat(value)) + (unit || getUnit(value)); + }; + }, + normalize = function normalize(min, max, value) { + return mapRange(min, max, 0, 1, value); + }, + _wrapArray = function _wrapArray(a, wrapper, value) { + return _conditionalReturn(value, function (index) { + return a[~~wrapper(index)]; + }); + }, + wrap = function wrap(min, max, value) { + var range = max - min; + return _isArray(min) ? _wrapArray(min, wrap(0, min.length), max) : _conditionalReturn(value, function (value) { + return (range + (value - min) % range) % range + min; + }); + }, + wrapYoyo = function wrapYoyo(min, max, value) { + var range = max - min, + total = range * 2; + return _isArray(min) ? _wrapArray(min, wrapYoyo(0, min.length - 1), max) : _conditionalReturn(value, function (value) { + value = (total + (value - min) % total) % total || 0; + return min + (value > range ? total - value : value); + }); + }, + _replaceRandom = function _replaceRandom(value) { + var prev = 0, + s = "", + i, + nums, + end, + isArray; + + while (~(i = value.indexOf("random(", prev))) { + end = value.indexOf(")", i); + isArray = value.charAt(i + 7) === "["; + nums = value.substr(i + 7, end - i - 7).match(isArray ? _delimitedValueExp : _strictNumExp); + s += value.substr(prev, i - prev) + random(isArray ? nums : +nums[0], isArray ? 0 : +nums[1], +nums[2] || 1e-5); + prev = end + 1; + } + + return s + value.substr(prev, value.length - prev); + }, + mapRange = function mapRange(inMin, inMax, outMin, outMax, value) { + var inRange = inMax - inMin, + outRange = outMax - outMin; + return _conditionalReturn(value, function (value) { + return outMin + ((value - inMin) / inRange * outRange || 0); + }); + }, + interpolate = function interpolate(start, end, progress, mutate) { + var func = isNaN(start + end) ? 0 : function (p) { + return (1 - p) * start + p * end; + }; + + if (!func) { + var isString = _isString(start), + master = {}, + p, + i, + interpolators, + l, + il; + + progress === true && (mutate = 1) && (progress = null); + + if (isString) { + start = { + p: start + }; + end = { + p: end + }; + } else if (_isArray(start) && !_isArray(end)) { + interpolators = []; + l = start.length; + il = l - 2; + + for (i = 1; i < l; i++) { + interpolators.push(interpolate(start[i - 1], start[i])); + } + + l--; + + func = function func(p) { + p *= l; + var i = Math.min(il, ~~p); + return interpolators[i](p - i); + }; + + progress = end; + } else if (!mutate) { + start = _merge(_isArray(start) ? [] : {}, start); + } + + if (!interpolators) { + for (p in end) { + _addPropTween.call(master, start, p, "get", end[p]); + } + + func = function func(p) { + return _renderPropTweens(p, master) || (isString ? start.p : start); + }; + } + } + + return _conditionalReturn(progress, func); + }, + _getLabelInDirection = function _getLabelInDirection(timeline, fromTime, backward) { + var labels = timeline.labels, + min = _bigNum, + p, + distance, + label; + + for (p in labels) { + distance = labels[p] - fromTime; + + if (distance < 0 === !!backward && distance && min > (distance = Math.abs(distance))) { + label = p; + min = distance; + } + } + + return label; + }, + _callback = function _callback(animation, type, executeLazyFirst) { + var v = animation.vars, + callback = v[type], + prevContext = _context, + context = animation._ctx, + params, + scope, + result; + + if (!callback) { + return; + } + + params = v[type + "Params"]; + scope = v.callbackScope || animation; + executeLazyFirst && _lazyTweens.length && _lazyRender(); + context && (_context = context); + result = params ? callback.apply(scope, params) : callback.call(scope); + _context = prevContext; + return result; + }, + _interrupt = function _interrupt(animation) { + _removeFromParent(animation); + + animation.scrollTrigger && animation.scrollTrigger.kill(!!_reverting); + animation.progress() < 1 && _callback(animation, "onInterrupt"); + return animation; + }, + _quickTween, + _registerPluginQueue = [], + _createPlugin = function _createPlugin(config) { + if (!config) return; + config = !config.name && config["default"] || config; + + if (_windowExists() || config.headless) { + var name = config.name, + isFunc = _isFunction(config), + Plugin = name && !isFunc && config.init ? function () { + this._props = []; + } : config, + instanceDefaults = { + init: _emptyFunc, + render: _renderPropTweens, + add: _addPropTween, + kill: _killPropTweensOf, + modifier: _addPluginModifier, + rawVars: 0 + }, + statics = { + targetTest: 0, + get: 0, + getSetter: _getSetter, + aliases: {}, + register: 0 + }; + + _wake(); + + if (config !== Plugin) { + if (_plugins[name]) { + return; + } + + _setDefaults(Plugin, _setDefaults(_copyExcluding(config, instanceDefaults), statics)); + + _merge(Plugin.prototype, _merge(instanceDefaults, _copyExcluding(config, statics))); + + _plugins[Plugin.prop = name] = Plugin; + + if (config.targetTest) { + _harnessPlugins.push(Plugin); + + _reservedProps[name] = 1; + } + + name = (name === "css" ? "CSS" : name.charAt(0).toUpperCase() + name.substr(1)) + "Plugin"; + } + + _addGlobal(name, Plugin); + + config.register && config.register(gsap, Plugin, PropTween); + } else { + _registerPluginQueue.push(config); + } + }, + _255 = 255, + _colorLookup = { + aqua: [0, _255, _255], + lime: [0, _255, 0], + silver: [192, 192, 192], + black: [0, 0, 0], + maroon: [128, 0, 0], + teal: [0, 128, 128], + blue: [0, 0, _255], + navy: [0, 0, 128], + white: [_255, _255, _255], + olive: [128, 128, 0], + yellow: [_255, _255, 0], + orange: [_255, 165, 0], + gray: [128, 128, 128], + purple: [128, 0, 128], + green: [0, 128, 0], + red: [_255, 0, 0], + pink: [_255, 192, 203], + cyan: [0, _255, _255], + transparent: [_255, _255, _255, 0] + }, + _hue = function _hue(h, m1, m2) { + h += h < 0 ? 1 : h > 1 ? -1 : 0; + return (h * 6 < 1 ? m1 + (m2 - m1) * h * 6 : h < .5 ? m2 : h * 3 < 2 ? m1 + (m2 - m1) * (2 / 3 - h) * 6 : m1) * _255 + .5 | 0; + }, + splitColor = function splitColor(v, toHSL, forceAlpha) { + var a = !v ? _colorLookup.black : _isNumber(v) ? [v >> 16, v >> 8 & _255, v & _255] : 0, + r, + g, + b, + h, + s, + l, + max, + min, + d, + wasHSL; + + if (!a) { + if (v.substr(-1) === ",") { + v = v.substr(0, v.length - 1); + } + + if (_colorLookup[v]) { + a = _colorLookup[v]; + } else if (v.charAt(0) === "#") { + if (v.length < 6) { + r = v.charAt(1); + g = v.charAt(2); + b = v.charAt(3); + v = "#" + r + r + g + g + b + b + (v.length === 5 ? v.charAt(4) + v.charAt(4) : ""); + } + + if (v.length === 9) { + a = parseInt(v.substr(1, 6), 16); + return [a >> 16, a >> 8 & _255, a & _255, parseInt(v.substr(7), 16) / 255]; + } + + v = parseInt(v.substr(1), 16); + a = [v >> 16, v >> 8 & _255, v & _255]; + } else if (v.substr(0, 3) === "hsl") { + a = wasHSL = v.match(_strictNumExp); + + if (!toHSL) { + h = +a[0] % 360 / 360; + s = +a[1] / 100; + l = +a[2] / 100; + g = l <= .5 ? l * (s + 1) : l + s - l * s; + r = l * 2 - g; + a.length > 3 && (a[3] *= 1); + a[0] = _hue(h + 1 / 3, r, g); + a[1] = _hue(h, r, g); + a[2] = _hue(h - 1 / 3, r, g); + } else if (~v.indexOf("=")) { + a = v.match(_numExp); + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + } + } else { + a = v.match(_strictNumExp) || _colorLookup.transparent; + } + + a = a.map(Number); + } + + if (toHSL && !wasHSL) { + r = a[0] / _255; + g = a[1] / _255; + b = a[2] / _255; + max = Math.max(r, g, b); + min = Math.min(r, g, b); + l = (max + min) / 2; + + if (max === min) { + h = s = 0; + } else { + d = max - min; + s = l > 0.5 ? d / (2 - max - min) : d / (max + min); + h = max === r ? (g - b) / d + (g < b ? 6 : 0) : max === g ? (b - r) / d + 2 : (r - g) / d + 4; + h *= 60; + } + + a[0] = ~~(h + .5); + a[1] = ~~(s * 100 + .5); + a[2] = ~~(l * 100 + .5); + } + + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + }, + _colorOrderData = function _colorOrderData(v) { + var values = [], + c = [], + i = -1; + v.split(_colorExp).forEach(function (v) { + var a = v.match(_numWithUnitExp) || []; + values.push.apply(values, a); + c.push(i += a.length + 1); + }); + values.c = c; + return values; + }, + _formatColors = function _formatColors(s, toHSL, orderMatchData) { + var result = "", + colors = (s + result).match(_colorExp), + type = toHSL ? "hsla(" : "rgba(", + i = 0, + c, + shell, + d, + l; + + if (!colors) { + return s; + } + + colors = colors.map(function (color) { + return (color = splitColor(color, toHSL, 1)) && type + (toHSL ? color[0] + "," + color[1] + "%," + color[2] + "%," + color[3] : color.join(",")) + ")"; + }); + + if (orderMatchData) { + d = _colorOrderData(s); + c = orderMatchData.c; + + if (c.join(result) !== d.c.join(result)) { + shell = s.replace(_colorExp, "1").split(_numWithUnitExp); + l = shell.length - 1; + + for (; i < l; i++) { + result += shell[i] + (~c.indexOf(i) ? colors.shift() || type + "0,0,0,0)" : (d.length ? d : colors.length ? colors : orderMatchData).shift()); + } + } + } + + if (!shell) { + shell = s.split(_colorExp); + l = shell.length - 1; + + for (; i < l; i++) { + result += shell[i] + colors[i]; + } + } + + return result + shell[l]; + }, + _colorExp = function () { + var s = "(?:\\b(?:(?:rgb|rgba|hsl|hsla)\\(.+?\\))|\\B#(?:[0-9a-f]{3,4}){1,2}\\b", + p; + + for (p in _colorLookup) { + s += "|" + p + "\\b"; + } + + return new RegExp(s + ")", "gi"); + }(), + _hslExp = /hsl[a]?\(/, + _colorStringFilter = function _colorStringFilter(a) { + var combined = a.join(" "), + toHSL; + _colorExp.lastIndex = 0; + + if (_colorExp.test(combined)) { + toHSL = _hslExp.test(combined); + a[1] = _formatColors(a[1], toHSL); + a[0] = _formatColors(a[0], toHSL, _colorOrderData(a[1])); + return true; + } + }, + _tickerActive, + _ticker = function () { + var _getTime = Date.now, + _lagThreshold = 500, + _adjustedLag = 33, + _startTime = _getTime(), + _lastUpdate = _startTime, + _gap = 1000 / 240, + _nextTime = _gap, + _listeners = [], + _id, + _req, + _raf, + _self, + _delta, + _i, + _tick = function _tick(v) { + var elapsed = _getTime() - _lastUpdate, + manual = v === true, + overlap, + dispatch, + time, + frame; + + (elapsed > _lagThreshold || elapsed < 0) && (_startTime += elapsed - _adjustedLag); + _lastUpdate += elapsed; + time = _lastUpdate - _startTime; + overlap = time - _nextTime; + + if (overlap > 0 || manual) { + frame = ++_self.frame; + _delta = time - _self.time * 1000; + _self.time = time = time / 1000; + _nextTime += overlap + (overlap >= _gap ? 4 : _gap - overlap); + dispatch = 1; + } + + manual || (_id = _req(_tick)); + + if (dispatch) { + for (_i = 0; _i < _listeners.length; _i++) { + _listeners[_i](time, _delta, frame, v); + } + } + }; + + _self = { + time: 0, + frame: 0, + tick: function tick() { + _tick(true); + }, + deltaRatio: function deltaRatio(fps) { + return _delta / (1000 / (fps || 60)); + }, + wake: function wake() { + if (_coreReady) { + if (!_coreInitted && _windowExists()) { + _win = _coreInitted = window; + _doc = _win.document || {}; + _globals.gsap = gsap; + (_win.gsapVersions || (_win.gsapVersions = [])).push(gsap.version); + + _install(_installScope || _win.GreenSockGlobals || !_win.gsap && _win || {}); + + _registerPluginQueue.forEach(_createPlugin); + } + + _raf = typeof requestAnimationFrame !== "undefined" && requestAnimationFrame; + _id && _self.sleep(); + + _req = _raf || function (f) { + return setTimeout(f, _nextTime - _self.time * 1000 + 1 | 0); + }; + + _tickerActive = 1; + + _tick(2); + } + }, + sleep: function sleep() { + (_raf ? cancelAnimationFrame : clearTimeout)(_id); + _tickerActive = 0; + _req = _emptyFunc; + }, + lagSmoothing: function lagSmoothing(threshold, adjustedLag) { + _lagThreshold = threshold || Infinity; + _adjustedLag = Math.min(adjustedLag || 33, _lagThreshold); + }, + fps: function fps(_fps) { + _gap = 1000 / (_fps || 240); + _nextTime = _self.time * 1000 + _gap; + }, + add: function add(callback, once, prioritize) { + var func = once ? function (t, d, f, v) { + callback(t, d, f, v); + + _self.remove(func); + } : callback; + + _self.remove(callback); + + _listeners[prioritize ? "unshift" : "push"](func); + + _wake(); + + return func; + }, + remove: function remove(callback, i) { + ~(i = _listeners.indexOf(callback)) && _listeners.splice(i, 1) && _i >= i && _i--; + }, + _listeners: _listeners + }; + return _self; + }(), + _wake = function _wake() { + return !_tickerActive && _ticker.wake(); + }, + _easeMap = {}, + _customEaseExp = /^[\d.\-M][\d.\-,\s]/, + _quotesExp = /["']/g, + _parseObjectInString = function _parseObjectInString(value) { + var obj = {}, + split = value.substr(1, value.length - 3).split(":"), + key = split[0], + i = 1, + l = split.length, + index, + val, + parsedVal; + + for (; i < l; i++) { + val = split[i]; + index = i !== l - 1 ? val.lastIndexOf(",") : val.length; + parsedVal = val.substr(0, index); + obj[key] = isNaN(parsedVal) ? parsedVal.replace(_quotesExp, "").trim() : +parsedVal; + key = val.substr(index + 1).trim(); + } + + return obj; + }, + _valueInParentheses = function _valueInParentheses(value) { + var open = value.indexOf("(") + 1, + close = value.indexOf(")"), + nested = value.indexOf("(", open); + return value.substring(open, ~nested && nested < close ? value.indexOf(")", close + 1) : close); + }, + _configEaseFromString = function _configEaseFromString(name) { + var split = (name + "").split("("), + ease = _easeMap[split[0]]; + return ease && split.length > 1 && ease.config ? ease.config.apply(null, ~name.indexOf("{") ? [_parseObjectInString(split[1])] : _valueInParentheses(name).split(",").map(_numericIfPossible)) : _easeMap._CE && _customEaseExp.test(name) ? _easeMap._CE("", name) : ease; + }, + _invertEase = function _invertEase(ease) { + return function (p) { + return 1 - ease(1 - p); + }; + }, + _propagateYoyoEase = function _propagateYoyoEase(timeline, isYoyo) { + var child = timeline._first, + ease; + + while (child) { + if (child instanceof Timeline) { + _propagateYoyoEase(child, isYoyo); + } else if (child.vars.yoyoEase && (!child._yoyo || !child._repeat) && child._yoyo !== isYoyo) { + if (child.timeline) { + _propagateYoyoEase(child.timeline, isYoyo); + } else { + ease = child._ease; + child._ease = child._yEase; + child._yEase = ease; + child._yoyo = isYoyo; + } + } + + child = child._next; + } + }, + _parseEase = function _parseEase(ease, defaultEase) { + return !ease ? defaultEase : (_isFunction(ease) ? ease : _easeMap[ease] || _configEaseFromString(ease)) || defaultEase; + }, + _insertEase = function _insertEase(names, easeIn, easeOut, easeInOut) { + if (easeOut === void 0) { + easeOut = function easeOut(p) { + return 1 - easeIn(1 - p); + }; + } + + if (easeInOut === void 0) { + easeInOut = function easeInOut(p) { + return p < .5 ? easeIn(p * 2) / 2 : 1 - easeIn((1 - p) * 2) / 2; + }; + } + + var ease = { + easeIn: easeIn, + easeOut: easeOut, + easeInOut: easeInOut + }, + lowercaseName; + + _forEachName(names, function (name) { + _easeMap[name] = _globals[name] = ease; + _easeMap[lowercaseName = name.toLowerCase()] = easeOut; + + for (var p in ease) { + _easeMap[lowercaseName + (p === "easeIn" ? ".in" : p === "easeOut" ? ".out" : ".inOut")] = _easeMap[name + "." + p] = ease[p]; + } + }); + + return ease; + }, + _easeInOutFromOut = function _easeInOutFromOut(easeOut) { + return function (p) { + return p < .5 ? (1 - easeOut(1 - p * 2)) / 2 : .5 + easeOut((p - .5) * 2) / 2; + }; + }, + _configElastic = function _configElastic(type, amplitude, period) { + var p1 = amplitude >= 1 ? amplitude : 1, + p2 = (period || (type ? .3 : .45)) / (amplitude < 1 ? amplitude : 1), + p3 = p2 / _2PI * (Math.asin(1 / p1) || 0), + easeOut = function easeOut(p) { + return p === 1 ? 1 : p1 * Math.pow(2, -10 * p) * _sin((p - p3) * p2) + 1; + }, + ease = type === "out" ? easeOut : type === "in" ? function (p) { + return 1 - easeOut(1 - p); + } : _easeInOutFromOut(easeOut); + + p2 = _2PI / p2; + + ease.config = function (amplitude, period) { + return _configElastic(type, amplitude, period); + }; + + return ease; + }, + _configBack = function _configBack(type, overshoot) { + if (overshoot === void 0) { + overshoot = 1.70158; + } + + var easeOut = function easeOut(p) { + return p ? --p * p * ((overshoot + 1) * p + overshoot) + 1 : 0; + }, + ease = type === "out" ? easeOut : type === "in" ? function (p) { + return 1 - easeOut(1 - p); + } : _easeInOutFromOut(easeOut); + + ease.config = function (overshoot) { + return _configBack(type, overshoot); + }; + + return ease; + }; + + _forEachName("Linear,Quad,Cubic,Quart,Quint,Strong", function (name, i) { + var power = i < 5 ? i + 1 : i; + + _insertEase(name + ",Power" + (power - 1), i ? function (p) { + return Math.pow(p, power); + } : function (p) { + return p; + }, function (p) { + return 1 - Math.pow(1 - p, power); + }, function (p) { + return p < .5 ? Math.pow(p * 2, power) / 2 : 1 - Math.pow((1 - p) * 2, power) / 2; + }); + }); + + _easeMap.Linear.easeNone = _easeMap.none = _easeMap.Linear.easeIn; + + _insertEase("Elastic", _configElastic("in"), _configElastic("out"), _configElastic()); + + (function (n, c) { + var n1 = 1 / c, + n2 = 2 * n1, + n3 = 2.5 * n1, + easeOut = function easeOut(p) { + return p < n1 ? n * p * p : p < n2 ? n * Math.pow(p - 1.5 / c, 2) + .75 : p < n3 ? n * (p -= 2.25 / c) * p + .9375 : n * Math.pow(p - 2.625 / c, 2) + .984375; + }; + + _insertEase("Bounce", function (p) { + return 1 - easeOut(1 - p); + }, easeOut); + })(7.5625, 2.75); + + _insertEase("Expo", function (p) { + return Math.pow(2, 10 * (p - 1)) * p + p * p * p * p * p * p * (1 - p); + }); + + _insertEase("Circ", function (p) { + return -(_sqrt(1 - p * p) - 1); + }); + + _insertEase("Sine", function (p) { + return p === 1 ? 1 : -_cos(p * _HALF_PI) + 1; + }); + + _insertEase("Back", _configBack("in"), _configBack("out"), _configBack()); + + _easeMap.SteppedEase = _easeMap.steps = _globals.SteppedEase = { + config: function config(steps, immediateStart) { + if (steps === void 0) { + steps = 1; + } + + var p1 = 1 / steps, + p2 = steps + (immediateStart ? 0 : 1), + p3 = immediateStart ? 1 : 0, + max = 1 - _tinyNum; + return function (p) { + return ((p2 * _clamp(0, max, p) | 0) + p3) * p1; + }; + } + }; + _defaults.ease = _easeMap["quad.out"]; + + _forEachName("onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt", function (name) { + return _callbackNames += name + "," + name + "Params,"; + }); + + var GSCache = function GSCache(target, harness) { + this.id = _gsID++; + target._gsap = this; + this.target = target; + this.harness = harness; + this.get = harness ? harness.get : _getProperty; + this.set = harness ? harness.getSetter : _getSetter; + }; + var Animation = function () { + function Animation(vars) { + this.vars = vars; + this._delay = +vars.delay || 0; + + if (this._repeat = vars.repeat === Infinity ? -2 : vars.repeat || 0) { + this._rDelay = vars.repeatDelay || 0; + this._yoyo = !!vars.yoyo || !!vars.yoyoEase; + } + + this._ts = 1; + + _setDuration(this, +vars.duration, 1, 1); + + this.data = vars.data; + + if (_context) { + this._ctx = _context; + + _context.data.push(this); + } + + _tickerActive || _ticker.wake(); + } + + var _proto = Animation.prototype; + + _proto.delay = function delay(value) { + if (value || value === 0) { + this.parent && this.parent.smoothChildTiming && this.startTime(this._start + value - this._delay); + this._delay = value; + return this; + } + + return this._delay; + }; + + _proto.duration = function duration(value) { + return arguments.length ? this.totalDuration(this._repeat > 0 ? value + (value + this._rDelay) * this._repeat : value) : this.totalDuration() && this._dur; + }; + + _proto.totalDuration = function totalDuration(value) { + if (!arguments.length) { + return this._tDur; + } + + this._dirty = 0; + return _setDuration(this, this._repeat < 0 ? value : (value - this._repeat * this._rDelay) / (this._repeat + 1)); + }; + + _proto.totalTime = function totalTime(_totalTime, suppressEvents) { + _wake(); + + if (!arguments.length) { + return this._tTime; + } + + var parent = this._dp; + + if (parent && parent.smoothChildTiming && this._ts) { + _alignPlayhead(this, _totalTime); + + !parent._dp || parent.parent || _postAddChecks(parent, this); + + while (parent && parent.parent) { + if (parent.parent._time !== parent._start + (parent._ts >= 0 ? parent._tTime / parent._ts : (parent.totalDuration() - parent._tTime) / -parent._ts)) { + parent.totalTime(parent._tTime, true); + } + + parent = parent.parent; + } + + if (!this.parent && this._dp.autoRemoveChildren && (this._ts > 0 && _totalTime < this._tDur || this._ts < 0 && _totalTime > 0 || !this._tDur && !_totalTime)) { + _addToTimeline(this._dp, this, this._start - this._delay); + } + } + + if (this._tTime !== _totalTime || !this._dur && !suppressEvents || this._initted && Math.abs(this._zTime) === _tinyNum || !_totalTime && !this._initted && (this.add || this._ptLookup)) { + this._ts || (this._pTime = _totalTime); + + _lazySafeRender(this, _totalTime, suppressEvents); + } + + return this; + }; + + _proto.time = function time(value, suppressEvents) { + return arguments.length ? this.totalTime(Math.min(this.totalDuration(), value + _elapsedCycleDuration(this)) % (this._dur + this._rDelay) || (value ? this._dur : 0), suppressEvents) : this._time; + }; + + _proto.totalProgress = function totalProgress(value, suppressEvents) { + return arguments.length ? this.totalTime(this.totalDuration() * value, suppressEvents) : this.totalDuration() ? Math.min(1, this._tTime / this._tDur) : this.rawTime() >= 0 && this._initted ? 1 : 0; + }; + + _proto.progress = function progress(value, suppressEvents) { + return arguments.length ? this.totalTime(this.duration() * (this._yoyo && !(this.iteration() & 1) ? 1 - value : value) + _elapsedCycleDuration(this), suppressEvents) : this.duration() ? Math.min(1, this._time / this._dur) : this.rawTime() > 0 ? 1 : 0; + }; + + _proto.iteration = function iteration(value, suppressEvents) { + var cycleDuration = this.duration() + this._rDelay; + + return arguments.length ? this.totalTime(this._time + (value - 1) * cycleDuration, suppressEvents) : this._repeat ? _animationCycle(this._tTime, cycleDuration) + 1 : 1; + }; + + _proto.timeScale = function timeScale(value, suppressEvents) { + if (!arguments.length) { + return this._rts === -_tinyNum ? 0 : this._rts; + } + + if (this._rts === value) { + return this; + } + + var tTime = this.parent && this._ts ? _parentToChildTotalTime(this.parent._time, this) : this._tTime; + this._rts = +value || 0; + this._ts = this._ps || value === -_tinyNum ? 0 : this._rts; + this.totalTime(_clamp(-Math.abs(this._delay), this._tDur, tTime), suppressEvents !== false); + + _setEnd(this); + + return _recacheAncestors(this); + }; + + _proto.paused = function paused(value) { + if (!arguments.length) { + return this._ps; + } + + if (this._ps !== value) { + this._ps = value; + + if (value) { + this._pTime = this._tTime || Math.max(-this._delay, this.rawTime()); + this._ts = this._act = 0; + } else { + _wake(); + + this._ts = this._rts; + this.totalTime(this.parent && !this.parent.smoothChildTiming ? this.rawTime() : this._tTime || this._pTime, this.progress() === 1 && Math.abs(this._zTime) !== _tinyNum && (this._tTime -= _tinyNum)); + } + } + + return this; + }; + + _proto.startTime = function startTime(value) { + if (arguments.length) { + this._start = value; + var parent = this.parent || this._dp; + parent && (parent._sort || !this.parent) && _addToTimeline(parent, this, value - this._delay); + return this; + } + + return this._start; + }; + + _proto.endTime = function endTime(includeRepeats) { + return this._start + (_isNotFalse(includeRepeats) ? this.totalDuration() : this.duration()) / Math.abs(this._ts || 1); + }; + + _proto.rawTime = function rawTime(wrapRepeats) { + var parent = this.parent || this._dp; + return !parent ? this._tTime : wrapRepeats && (!this._ts || this._repeat && this._time && this.totalProgress() < 1) ? this._tTime % (this._dur + this._rDelay) : !this._ts ? this._tTime : _parentToChildTotalTime(parent.rawTime(wrapRepeats), this); + }; + + _proto.revert = function revert(config) { + if (config === void 0) { + config = _revertConfig; + } + + var prevIsReverting = _reverting; + _reverting = config; + + if (this._initted || this._startAt) { + this.timeline && this.timeline.revert(config); + this.totalTime(-0.01, config.suppressEvents); + } + + this.data !== "nested" && config.kill !== false && this.kill(); + _reverting = prevIsReverting; + return this; + }; + + _proto.globalTime = function globalTime(rawTime) { + var animation = this, + time = arguments.length ? rawTime : animation.rawTime(); + + while (animation) { + time = animation._start + time / (Math.abs(animation._ts) || 1); + animation = animation._dp; + } + + return !this.parent && this._sat ? this._sat.globalTime(rawTime) : time; + }; + + _proto.repeat = function repeat(value) { + if (arguments.length) { + this._repeat = value === Infinity ? -2 : value; + return _onUpdateTotalDuration(this); + } + + return this._repeat === -2 ? Infinity : this._repeat; + }; + + _proto.repeatDelay = function repeatDelay(value) { + if (arguments.length) { + var time = this._time; + this._rDelay = value; + + _onUpdateTotalDuration(this); + + return time ? this.time(time) : this; + } + + return this._rDelay; + }; + + _proto.yoyo = function yoyo(value) { + if (arguments.length) { + this._yoyo = value; + return this; + } + + return this._yoyo; + }; + + _proto.seek = function seek(position, suppressEvents) { + return this.totalTime(_parsePosition(this, position), _isNotFalse(suppressEvents)); + }; + + _proto.restart = function restart(includeDelay, suppressEvents) { + this.play().totalTime(includeDelay ? -this._delay : 0, _isNotFalse(suppressEvents)); + this._dur || (this._zTime = -_tinyNum); + return this; + }; + + _proto.play = function play(from, suppressEvents) { + from != null && this.seek(from, suppressEvents); + return this.reversed(false).paused(false); + }; + + _proto.reverse = function reverse(from, suppressEvents) { + from != null && this.seek(from || this.totalDuration(), suppressEvents); + return this.reversed(true).paused(false); + }; + + _proto.pause = function pause(atTime, suppressEvents) { + atTime != null && this.seek(atTime, suppressEvents); + return this.paused(true); + }; + + _proto.resume = function resume() { + return this.paused(false); + }; + + _proto.reversed = function reversed(value) { + if (arguments.length) { + !!value !== this.reversed() && this.timeScale(-this._rts || (value ? -_tinyNum : 0)); + return this; + } + + return this._rts < 0; + }; + + _proto.invalidate = function invalidate() { + this._initted = this._act = 0; + this._zTime = -_tinyNum; + return this; + }; + + _proto.isActive = function isActive() { + var parent = this.parent || this._dp, + start = this._start, + rawTime; + return !!(!parent || this._ts && this._initted && parent.isActive() && (rawTime = parent.rawTime(true)) >= start && rawTime < this.endTime(true) - _tinyNum); + }; + + _proto.eventCallback = function eventCallback(type, callback, params) { + var vars = this.vars; + + if (arguments.length > 1) { + if (!callback) { + delete vars[type]; + } else { + vars[type] = callback; + params && (vars[type + "Params"] = params); + type === "onUpdate" && (this._onUpdate = callback); + } + + return this; + } + + return vars[type]; + }; + + _proto.then = function then(onFulfilled) { + var self = this; + return new Promise(function (resolve) { + var f = _isFunction(onFulfilled) ? onFulfilled : _passThrough, + _resolve = function _resolve() { + var _then = self.then; + self.then = null; + _isFunction(f) && (f = f(self)) && (f.then || f === self) && (self.then = _then); + resolve(f); + self.then = _then; + }; + + if (self._initted && self.totalProgress() === 1 && self._ts >= 0 || !self._tTime && self._ts < 0) { + _resolve(); + } else { + self._prom = _resolve; + } + }); + }; + + _proto.kill = function kill() { + _interrupt(this); + }; + + return Animation; + }(); + + _setDefaults(Animation.prototype, { + _time: 0, + _start: 0, + _end: 0, + _tTime: 0, + _tDur: 0, + _dirty: 0, + _repeat: 0, + _yoyo: false, + parent: null, + _initted: false, + _rDelay: 0, + _ts: 1, + _dp: 0, + ratio: 0, + _zTime: -_tinyNum, + _prom: 0, + _ps: false, + _rts: 1 + }); + + var Timeline = function (_Animation) { + _inheritsLoose(Timeline, _Animation); + + function Timeline(vars, position) { + var _this; + + if (vars === void 0) { + vars = {}; + } + + _this = _Animation.call(this, vars) || this; + _this.labels = {}; + _this.smoothChildTiming = !!vars.smoothChildTiming; + _this.autoRemoveChildren = !!vars.autoRemoveChildren; + _this._sort = _isNotFalse(vars.sortChildren); + _globalTimeline && _addToTimeline(vars.parent || _globalTimeline, _assertThisInitialized(_this), position); + vars.reversed && _this.reverse(); + vars.paused && _this.paused(true); + vars.scrollTrigger && _scrollTrigger(_assertThisInitialized(_this), vars.scrollTrigger); + return _this; + } + + var _proto2 = Timeline.prototype; + + _proto2.to = function to(targets, vars, position) { + _createTweenType(0, arguments, this); + + return this; + }; + + _proto2.from = function from(targets, vars, position) { + _createTweenType(1, arguments, this); + + return this; + }; + + _proto2.fromTo = function fromTo(targets, fromVars, toVars, position) { + _createTweenType(2, arguments, this); + + return this; + }; + + _proto2.set = function set(targets, vars, position) { + vars.duration = 0; + vars.parent = this; + _inheritDefaults(vars).repeatDelay || (vars.repeat = 0); + vars.immediateRender = !!vars.immediateRender; + new Tween(targets, vars, _parsePosition(this, position), 1); + return this; + }; + + _proto2.call = function call(callback, params, position) { + return _addToTimeline(this, Tween.delayedCall(0, callback, params), position); + }; + + _proto2.staggerTo = function staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.duration = duration; + vars.stagger = vars.stagger || stagger; + vars.onComplete = onCompleteAll; + vars.onCompleteParams = onCompleteAllParams; + vars.parent = this; + new Tween(targets, vars, _parsePosition(this, position)); + return this; + }; + + _proto2.staggerFrom = function staggerFrom(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.runBackwards = 1; + _inheritDefaults(vars).immediateRender = _isNotFalse(vars.immediateRender); + return this.staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams); + }; + + _proto2.staggerFromTo = function staggerFromTo(targets, duration, fromVars, toVars, stagger, position, onCompleteAll, onCompleteAllParams) { + toVars.startAt = fromVars; + _inheritDefaults(toVars).immediateRender = _isNotFalse(toVars.immediateRender); + return this.staggerTo(targets, duration, toVars, stagger, position, onCompleteAll, onCompleteAllParams); + }; + + _proto2.render = function render(totalTime, suppressEvents, force) { + var prevTime = this._time, + tDur = this._dirty ? this.totalDuration() : this._tDur, + dur = this._dur, + tTime = totalTime <= 0 ? 0 : _roundPrecise(totalTime), + crossingStart = this._zTime < 0 !== totalTime < 0 && (this._initted || !dur), + time, + child, + next, + iteration, + cycleDuration, + prevPaused, + pauseTween, + timeScale, + prevStart, + prevIteration, + yoyo, + isYoyo; + this !== _globalTimeline && tTime > tDur && totalTime >= 0 && (tTime = tDur); + + if (tTime !== this._tTime || force || crossingStart) { + if (prevTime !== this._time && dur) { + tTime += this._time - prevTime; + totalTime += this._time - prevTime; + } + + time = tTime; + prevStart = this._start; + timeScale = this._ts; + prevPaused = !timeScale; + + if (crossingStart) { + dur || (prevTime = this._zTime); + (totalTime || !suppressEvents) && (this._zTime = totalTime); + } + + if (this._repeat) { + yoyo = this._yoyo; + cycleDuration = dur + this._rDelay; + + if (this._repeat < -1 && totalTime < 0) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + + time = _roundPrecise(tTime % cycleDuration); + + if (tTime === tDur) { + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); + iteration = ~~prevIteration; + + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } + + time > dur && (time = dur); + } + + prevIteration = _animationCycle(this._tTime, cycleDuration); + !prevTime && this._tTime && prevIteration !== iteration && this._tTime - prevIteration * cycleDuration - this._dur <= 0 && (prevIteration = iteration); + + if (yoyo && iteration & 1) { + time = dur - time; + isYoyo = 1; + } + + if (iteration !== prevIteration && !this._lock) { + var rewinding = yoyo && prevIteration & 1, + doesWrap = rewinding === (yoyo && iteration & 1); + iteration < prevIteration && (rewinding = !rewinding); + prevTime = rewinding ? 0 : tTime % dur ? dur : tTime; + this._lock = 1; + this.render(prevTime || (isYoyo ? 0 : _roundPrecise(iteration * cycleDuration)), suppressEvents, !dur)._lock = 0; + this._tTime = tTime; + !suppressEvents && this.parent && _callback(this, "onRepeat"); + this.vars.repeatRefresh && !isYoyo && (this.invalidate()._lock = 1); + + if (prevTime && prevTime !== this._time || prevPaused !== !this._ts || this.vars.onRepeat && !this.parent && !this._act) { + return this; + } + + dur = this._dur; + tDur = this._tDur; + + if (doesWrap) { + this._lock = 2; + prevTime = rewinding ? dur : -0.0001; + this.render(prevTime, true); + this.vars.repeatRefresh && !isYoyo && this.invalidate(); + } + + this._lock = 0; + + if (!this._ts && !prevPaused) { + return this; + } + + _propagateYoyoEase(this, isYoyo); + } + } + + if (this._hasPause && !this._forcing && this._lock < 2) { + pauseTween = _findNextPauseTween(this, _roundPrecise(prevTime), _roundPrecise(time)); + + if (pauseTween) { + tTime -= time - (time = pauseTween._start); + } + } + + this._tTime = tTime; + this._time = time; + this._act = !timeScale; + + if (!this._initted) { + this._onUpdate = this.vars.onUpdate; + this._initted = 1; + this._zTime = totalTime; + prevTime = 0; + } + + if (!prevTime && time && !suppressEvents && !iteration) { + _callback(this, "onStart"); + + if (this._tTime !== tTime) { + return this; + } + } + + if (time >= prevTime && totalTime >= 0) { + child = this._first; + + while (child) { + next = child._next; + + if ((child._act || time >= child._start) && child._ts && pauseTween !== child) { + if (child.parent !== this) { + return this.render(totalTime, suppressEvents, force); + } + + child.render(child._ts > 0 ? (time - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (time - child._start) * child._ts, suppressEvents, force); + + if (time !== this._time || !this._ts && !prevPaused) { + pauseTween = 0; + next && (tTime += this._zTime = -_tinyNum); + break; + } + } + + child = next; + } + } else { + child = this._last; + var adjustedTime = totalTime < 0 ? totalTime : time; + + while (child) { + next = child._prev; + + if ((child._act || adjustedTime <= child._end) && child._ts && pauseTween !== child) { + if (child.parent !== this) { + return this.render(totalTime, suppressEvents, force); + } + + child.render(child._ts > 0 ? (adjustedTime - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (adjustedTime - child._start) * child._ts, suppressEvents, force || _reverting && (child._initted || child._startAt)); + + if (time !== this._time || !this._ts && !prevPaused) { + pauseTween = 0; + next && (tTime += this._zTime = adjustedTime ? -_tinyNum : _tinyNum); + break; + } + } + + child = next; + } + } + + if (pauseTween && !suppressEvents) { + this.pause(); + pauseTween.render(time >= prevTime ? 0 : -_tinyNum)._zTime = time >= prevTime ? 1 : -1; + + if (this._ts) { + this._start = prevStart; + + _setEnd(this); + + return this.render(totalTime, suppressEvents, force); + } + } + + this._onUpdate && !suppressEvents && _callback(this, "onUpdate", true); + if (tTime === tDur && this._tTime >= this.totalDuration() || !tTime && prevTime) if (prevStart === this._start || Math.abs(timeScale) !== Math.abs(this._ts)) if (!this._lock) { + (totalTime || !dur) && (tTime === tDur && this._ts > 0 || !tTime && this._ts < 0) && _removeFromParent(this, 1); + + if (!suppressEvents && !(totalTime < 0 && !prevTime) && (tTime || prevTime || !tDur)) { + _callback(this, tTime === tDur && totalTime >= 0 ? "onComplete" : "onReverseComplete", true); + + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + + return this; + }; + + _proto2.add = function add(child, position) { + var _this2 = this; + + _isNumber(position) || (position = _parsePosition(this, position, child)); + + if (!(child instanceof Animation)) { + if (_isArray(child)) { + child.forEach(function (obj) { + return _this2.add(obj, position); + }); + return this; + } + + if (_isString(child)) { + return this.addLabel(child, position); + } + + if (_isFunction(child)) { + child = Tween.delayedCall(0, child); + } else { + return this; + } + } + + return this !== child ? _addToTimeline(this, child, position) : this; + }; + + _proto2.getChildren = function getChildren(nested, tweens, timelines, ignoreBeforeTime) { + if (nested === void 0) { + nested = true; + } + + if (tweens === void 0) { + tweens = true; + } + + if (timelines === void 0) { + timelines = true; + } + + if (ignoreBeforeTime === void 0) { + ignoreBeforeTime = -_bigNum; + } + + var a = [], + child = this._first; + + while (child) { + if (child._start >= ignoreBeforeTime) { + if (child instanceof Tween) { + tweens && a.push(child); + } else { + timelines && a.push(child); + nested && a.push.apply(a, child.getChildren(true, tweens, timelines)); + } + } + + child = child._next; + } + + return a; + }; + + _proto2.getById = function getById(id) { + var animations = this.getChildren(1, 1, 1), + i = animations.length; + + while (i--) { + if (animations[i].vars.id === id) { + return animations[i]; + } + } + }; + + _proto2.remove = function remove(child) { + if (_isString(child)) { + return this.removeLabel(child); + } + + if (_isFunction(child)) { + return this.killTweensOf(child); + } + + child.parent === this && _removeLinkedListItem(this, child); + + if (child === this._recent) { + this._recent = this._last; + } + + return _uncache(this); + }; + + _proto2.totalTime = function totalTime(_totalTime2, suppressEvents) { + if (!arguments.length) { + return this._tTime; + } + + this._forcing = 1; + + if (!this._dp && this._ts) { + this._start = _roundPrecise(_ticker.time - (this._ts > 0 ? _totalTime2 / this._ts : (this.totalDuration() - _totalTime2) / -this._ts)); + } + + _Animation.prototype.totalTime.call(this, _totalTime2, suppressEvents); + + this._forcing = 0; + return this; + }; + + _proto2.addLabel = function addLabel(label, position) { + this.labels[label] = _parsePosition(this, position); + return this; + }; + + _proto2.removeLabel = function removeLabel(label) { + delete this.labels[label]; + return this; + }; + + _proto2.addPause = function addPause(position, callback, params) { + var t = Tween.delayedCall(0, callback || _emptyFunc, params); + t.data = "isPause"; + this._hasPause = 1; + return _addToTimeline(this, t, _parsePosition(this, position)); + }; + + _proto2.removePause = function removePause(position) { + var child = this._first; + position = _parsePosition(this, position); + + while (child) { + if (child._start === position && child.data === "isPause") { + _removeFromParent(child); + } + + child = child._next; + } + }; + + _proto2.killTweensOf = function killTweensOf(targets, props, onlyActive) { + var tweens = this.getTweensOf(targets, onlyActive), + i = tweens.length; + + while (i--) { + _overwritingTween !== tweens[i] && tweens[i].kill(targets, props); + } + + return this; + }; + + _proto2.getTweensOf = function getTweensOf(targets, onlyActive) { + var a = [], + parsedTargets = toArray(targets), + child = this._first, + isGlobalTime = _isNumber(onlyActive), + children; + + while (child) { + if (child instanceof Tween) { + if (_arrayContainsAny(child._targets, parsedTargets) && (isGlobalTime ? (!_overwritingTween || child._initted && child._ts) && child.globalTime(0) <= onlyActive && child.globalTime(child.totalDuration()) > onlyActive : !onlyActive || child.isActive())) { + a.push(child); + } + } else if ((children = child.getTweensOf(parsedTargets, onlyActive)).length) { + a.push.apply(a, children); + } + + child = child._next; + } + + return a; + }; + + _proto2.tweenTo = function tweenTo(position, vars) { + vars = vars || {}; + + var tl = this, + endTime = _parsePosition(tl, position), + _vars = vars, + startAt = _vars.startAt, + _onStart = _vars.onStart, + onStartParams = _vars.onStartParams, + immediateRender = _vars.immediateRender, + initted, + tween = Tween.to(tl, _setDefaults({ + ease: vars.ease || "none", + lazy: false, + immediateRender: false, + time: endTime, + overwrite: "auto", + duration: vars.duration || Math.abs((endTime - (startAt && "time" in startAt ? startAt.time : tl._time)) / tl.timeScale()) || _tinyNum, + onStart: function onStart() { + tl.pause(); + + if (!initted) { + var duration = vars.duration || Math.abs((endTime - (startAt && "time" in startAt ? startAt.time : tl._time)) / tl.timeScale()); + tween._dur !== duration && _setDuration(tween, duration, 0, 1).render(tween._time, true, true); + initted = 1; + } + + _onStart && _onStart.apply(tween, onStartParams || []); + } + }, vars)); + + return immediateRender ? tween.render(0) : tween; + }; + + _proto2.tweenFromTo = function tweenFromTo(fromPosition, toPosition, vars) { + return this.tweenTo(toPosition, _setDefaults({ + startAt: { + time: _parsePosition(this, fromPosition) + } + }, vars)); + }; + + _proto2.recent = function recent() { + return this._recent; + }; + + _proto2.nextLabel = function nextLabel(afterTime) { + if (afterTime === void 0) { + afterTime = this._time; + } + + return _getLabelInDirection(this, _parsePosition(this, afterTime)); + }; + + _proto2.previousLabel = function previousLabel(beforeTime) { + if (beforeTime === void 0) { + beforeTime = this._time; + } + + return _getLabelInDirection(this, _parsePosition(this, beforeTime), 1); + }; + + _proto2.currentLabel = function currentLabel(value) { + return arguments.length ? this.seek(value, true) : this.previousLabel(this._time + _tinyNum); + }; + + _proto2.shiftChildren = function shiftChildren(amount, adjustLabels, ignoreBeforeTime) { + if (ignoreBeforeTime === void 0) { + ignoreBeforeTime = 0; + } + + var child = this._first, + labels = this.labels, + p; + + while (child) { + if (child._start >= ignoreBeforeTime) { + child._start += amount; + child._end += amount; + } + + child = child._next; + } + + if (adjustLabels) { + for (p in labels) { + if (labels[p] >= ignoreBeforeTime) { + labels[p] += amount; + } + } + } + + return _uncache(this); + }; + + _proto2.invalidate = function invalidate(soft) { + var child = this._first; + this._lock = 0; + + while (child) { + child.invalidate(soft); + child = child._next; + } + + return _Animation.prototype.invalidate.call(this, soft); + }; + + _proto2.clear = function clear(includeLabels) { + if (includeLabels === void 0) { + includeLabels = true; + } + + var child = this._first, + next; + + while (child) { + next = child._next; + this.remove(child); + child = next; + } + + this._dp && (this._time = this._tTime = this._pTime = 0); + includeLabels && (this.labels = {}); + return _uncache(this); + }; + + _proto2.totalDuration = function totalDuration(value) { + var max = 0, + self = this, + child = self._last, + prevStart = _bigNum, + prev, + start, + parent; + + if (arguments.length) { + return self.timeScale((self._repeat < 0 ? self.duration() : self.totalDuration()) / (self.reversed() ? -value : value)); + } + + if (self._dirty) { + parent = self.parent; + + while (child) { + prev = child._prev; + child._dirty && child.totalDuration(); + start = child._start; + + if (start > prevStart && self._sort && child._ts && !self._lock) { + self._lock = 1; + _addToTimeline(self, child, start - child._delay, 1)._lock = 0; + } else { + prevStart = start; + } + + if (start < 0 && child._ts) { + max -= start; + + if (!parent && !self._dp || parent && parent.smoothChildTiming) { + self._start += start / self._ts; + self._time -= start; + self._tTime -= start; + } + + self.shiftChildren(-start, false, -1e999); + prevStart = 0; + } + + child._end > max && child._ts && (max = child._end); + child = prev; + } + + _setDuration(self, self === _globalTimeline && self._time > max ? self._time : max, 1, 1); + + self._dirty = 0; + } + + return self._tDur; + }; + + Timeline.updateRoot = function updateRoot(time) { + if (_globalTimeline._ts) { + _lazySafeRender(_globalTimeline, _parentToChildTotalTime(time, _globalTimeline)); + + _lastRenderedFrame = _ticker.frame; + } + + if (_ticker.frame >= _nextGCFrame) { + _nextGCFrame += _config.autoSleep || 120; + var child = _globalTimeline._first; + if (!child || !child._ts) if (_config.autoSleep && _ticker._listeners.length < 2) { + while (child && !child._ts) { + child = child._next; + } + + child || _ticker.sleep(); + } + } + }; + + return Timeline; + }(Animation); + + _setDefaults(Timeline.prototype, { + _lock: 0, + _hasPause: 0, + _forcing: 0 + }); + + var _addComplexStringPropTween = function _addComplexStringPropTween(target, prop, start, end, setter, stringFilter, funcParam) { + var pt = new PropTween(this._pt, target, prop, 0, 1, _renderComplexString, null, setter), + index = 0, + matchIndex = 0, + result, + startNums, + color, + endNum, + chunk, + startNum, + hasRandom, + a; + pt.b = start; + pt.e = end; + start += ""; + end += ""; + + if (hasRandom = ~end.indexOf("random(")) { + end = _replaceRandom(end); + } + + if (stringFilter) { + a = [start, end]; + stringFilter(a, target, prop); + start = a[0]; + end = a[1]; + } + + startNums = start.match(_complexStringNumExp) || []; + + while (result = _complexStringNumExp.exec(end)) { + endNum = result[0]; + chunk = end.substring(index, result.index); + + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(") { + color = 1; + } + + if (endNum !== startNums[matchIndex++]) { + startNum = parseFloat(startNums[matchIndex - 1]) || 0; + pt._pt = { + _next: pt._pt, + p: chunk || matchIndex === 1 ? chunk : ",", + s: startNum, + c: endNum.charAt(1) === "=" ? _parseRelative(startNum, endNum) - startNum : parseFloat(endNum) - startNum, + m: color && color < 4 ? Math.round : 0 + }; + index = _complexStringNumExp.lastIndex; + } + } + + pt.c = index < end.length ? end.substring(index, end.length) : ""; + pt.fp = funcParam; + + if (_relExp.test(end) || hasRandom) { + pt.e = 0; + } + + this._pt = pt; + return pt; + }, + _addPropTween = function _addPropTween(target, prop, start, end, index, targets, modifier, stringFilter, funcParam, optional) { + _isFunction(end) && (end = end(index || 0, target, targets)); + var currentValue = target[prop], + parsedStart = start !== "get" ? start : !_isFunction(currentValue) ? currentValue : funcParam ? target[prop.indexOf("set") || !_isFunction(target["get" + prop.substr(3)]) ? prop : "get" + prop.substr(3)](funcParam) : target[prop](), + setter = !_isFunction(currentValue) ? _setterPlain : funcParam ? _setterFuncWithParam : _setterFunc, + pt; + + if (_isString(end)) { + if (~end.indexOf("random(")) { + end = _replaceRandom(end); + } + + if (end.charAt(1) === "=") { + pt = _parseRelative(parsedStart, end) + (getUnit(parsedStart) || 0); + + if (pt || pt === 0) { + end = pt; + } + } + } + + if (!optional || parsedStart !== end || _forceAllPropTweens) { + if (!isNaN(parsedStart * end) && end !== "") { + pt = new PropTween(this._pt, target, prop, +parsedStart || 0, end - (parsedStart || 0), typeof currentValue === "boolean" ? _renderBoolean : _renderPlain, 0, setter); + funcParam && (pt.fp = funcParam); + modifier && pt.modifier(modifier, this, target); + return this._pt = pt; + } + + !currentValue && !(prop in target) && _missingPlugin(prop, end); + return _addComplexStringPropTween.call(this, target, prop, parsedStart, end, setter, stringFilter || _config.stringFilter, funcParam); + } + }, + _processVars = function _processVars(vars, index, target, targets, tween) { + _isFunction(vars) && (vars = _parseFuncOrString(vars, tween, index, target, targets)); + + if (!_isObject(vars) || vars.style && vars.nodeType || _isArray(vars) || _isTypedArray(vars)) { + return _isString(vars) ? _parseFuncOrString(vars, tween, index, target, targets) : vars; + } + + var copy = {}, + p; + + for (p in vars) { + copy[p] = _parseFuncOrString(vars[p], tween, index, target, targets); + } + + return copy; + }, + _checkPlugin = function _checkPlugin(property, vars, tween, index, target, targets) { + var plugin, pt, ptLookup, i; + + if (_plugins[property] && (plugin = new _plugins[property]()).init(target, plugin.rawVars ? vars[property] : _processVars(vars[property], index, target, targets, tween), tween, index, targets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, property, 0, 1, plugin.render, plugin, 0, plugin.priority); + + if (tween !== _quickTween) { + ptLookup = tween._ptLookup[tween._targets.indexOf(target)]; + i = plugin._props.length; + + while (i--) { + ptLookup[plugin._props[i]] = pt; + } + } + } + + return plugin; + }, + _overwritingTween, + _forceAllPropTweens, + _initTween = function _initTween(tween, time, tTime) { + var vars = tween.vars, + ease = vars.ease, + startAt = vars.startAt, + immediateRender = vars.immediateRender, + lazy = vars.lazy, + onUpdate = vars.onUpdate, + runBackwards = vars.runBackwards, + yoyoEase = vars.yoyoEase, + keyframes = vars.keyframes, + autoRevert = vars.autoRevert, + dur = tween._dur, + prevStartAt = tween._startAt, + targets = tween._targets, + parent = tween.parent, + fullTargets = parent && parent.data === "nested" ? parent.vars.targets : targets, + autoOverwrite = tween._overwrite === "auto" && !_suppressOverwrites, + tl = tween.timeline, + cleanVars, + i, + p, + pt, + target, + hasPriority, + gsData, + harness, + plugin, + ptLookup, + index, + harnessVars, + overwritten; + tl && (!keyframes || !ease) && (ease = "none"); + tween._ease = _parseEase(ease, _defaults.ease); + tween._yEase = yoyoEase ? _invertEase(_parseEase(yoyoEase === true ? ease : yoyoEase, _defaults.ease)) : 0; + + if (yoyoEase && tween._yoyo && !tween._repeat) { + yoyoEase = tween._yEase; + tween._yEase = tween._ease; + tween._ease = yoyoEase; + } + + tween._from = !tl && !!vars.runBackwards; + + if (!tl || keyframes && !vars.stagger) { + harness = targets[0] ? _getCache(targets[0]).harness : 0; + harnessVars = harness && vars[harness.prop]; + cleanVars = _copyExcluding(vars, _reservedProps); + + if (prevStartAt) { + prevStartAt._zTime < 0 && prevStartAt.progress(1); + time < 0 && runBackwards && immediateRender && !autoRevert ? prevStartAt.render(-1, true) : prevStartAt.revert(runBackwards && dur ? _revertConfigNoKill : _startAtRevertConfig); + prevStartAt._lazy = 0; + } + + if (startAt) { + _removeFromParent(tween._startAt = Tween.set(targets, _setDefaults({ + data: "isStart", + overwrite: false, + parent: parent, + immediateRender: true, + lazy: !prevStartAt && _isNotFalse(lazy), + startAt: null, + delay: 0, + onUpdate: onUpdate && function () { + return _callback(tween, "onUpdate"); + }, + stagger: 0 + }, startAt))); + + tween._startAt._dp = 0; + tween._startAt._sat = tween; + time < 0 && (_reverting || !immediateRender && !autoRevert) && tween._startAt.revert(_revertConfigNoKill); + + if (immediateRender) { + if (dur && time <= 0 && tTime <= 0) { + time && (tween._zTime = time); + return; + } + } + } else if (runBackwards && dur) { + if (!prevStartAt) { + time && (immediateRender = false); + p = _setDefaults({ + overwrite: false, + data: "isFromStart", + lazy: immediateRender && !prevStartAt && _isNotFalse(lazy), + immediateRender: immediateRender, + stagger: 0, + parent: parent + }, cleanVars); + harnessVars && (p[harness.prop] = harnessVars); + + _removeFromParent(tween._startAt = Tween.set(targets, p)); + + tween._startAt._dp = 0; + tween._startAt._sat = tween; + time < 0 && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween._startAt.render(-1, true)); + tween._zTime = time; + + if (!immediateRender) { + _initTween(tween._startAt, _tinyNum, _tinyNum); + } else if (!time) { + return; + } + } + } + + tween._pt = tween._ptCache = 0; + lazy = dur && _isNotFalse(lazy) || lazy && !dur; + + for (i = 0; i < targets.length; i++) { + target = targets[i]; + gsData = target._gsap || _harness(targets)[i]._gsap; + tween._ptLookup[i] = ptLookup = {}; + _lazyLookup[gsData.id] && _lazyTweens.length && _lazyRender(); + index = fullTargets === targets ? i : fullTargets.indexOf(target); + + if (harness && (plugin = new harness()).init(target, harnessVars || cleanVars, tween, index, fullTargets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, plugin.name, 0, 1, plugin.render, plugin, 0, plugin.priority); + + plugin._props.forEach(function (name) { + ptLookup[name] = pt; + }); + + plugin.priority && (hasPriority = 1); + } + + if (!harness || harnessVars) { + for (p in cleanVars) { + if (_plugins[p] && (plugin = _checkPlugin(p, cleanVars, tween, index, target, fullTargets))) { + plugin.priority && (hasPriority = 1); + } else { + ptLookup[p] = pt = _addPropTween.call(tween, target, p, "get", cleanVars[p], index, fullTargets, 0, vars.stringFilter); + } + } + } + + tween._op && tween._op[i] && tween.kill(target, tween._op[i]); + + if (autoOverwrite && tween._pt) { + _overwritingTween = tween; + + _globalTimeline.killTweensOf(target, ptLookup, tween.globalTime(time)); + + overwritten = !tween.parent; + _overwritingTween = 0; + } + + tween._pt && lazy && (_lazyLookup[gsData.id] = 1); + } + + hasPriority && _sortPropTweensByPriority(tween); + tween._onInit && tween._onInit(tween); + } + + tween._onUpdate = onUpdate; + tween._initted = (!tween._op || tween._pt) && !overwritten; + keyframes && time <= 0 && tl.render(_bigNum, true, true); + }, + _updatePropTweens = function _updatePropTweens(tween, property, value, start, startIsRelative, ratio, time, skipRecursion) { + var ptCache = (tween._pt && tween._ptCache || (tween._ptCache = {}))[property], + pt, + rootPT, + lookup, + i; + + if (!ptCache) { + ptCache = tween._ptCache[property] = []; + lookup = tween._ptLookup; + i = tween._targets.length; + + while (i--) { + pt = lookup[i][property]; + + if (pt && pt.d && pt.d._pt) { + pt = pt.d._pt; + + while (pt && pt.p !== property && pt.fp !== property) { + pt = pt._next; + } + } + + if (!pt) { + _forceAllPropTweens = 1; + tween.vars[property] = "+=0"; + + _initTween(tween, time); + + _forceAllPropTweens = 0; + return skipRecursion ? _warn(property + " not eligible for reset") : 1; + } + + ptCache.push(pt); + } + } + + i = ptCache.length; + + while (i--) { + rootPT = ptCache[i]; + pt = rootPT._pt || rootPT; + pt.s = (start || start === 0) && !startIsRelative ? start : pt.s + (start || 0) + ratio * pt.c; + pt.c = value - pt.s; + rootPT.e && (rootPT.e = _round(value) + getUnit(rootPT.e)); + rootPT.b && (rootPT.b = pt.s + getUnit(rootPT.b)); + } + }, + _addAliasesToVars = function _addAliasesToVars(targets, vars) { + var harness = targets[0] ? _getCache(targets[0]).harness : 0, + propertyAliases = harness && harness.aliases, + copy, + p, + i, + aliases; + + if (!propertyAliases) { + return vars; + } + + copy = _merge({}, vars); + + for (p in propertyAliases) { + if (p in copy) { + aliases = propertyAliases[p].split(","); + i = aliases.length; + + while (i--) { + copy[aliases[i]] = copy[p]; + } + } + } + + return copy; + }, + _parseKeyframe = function _parseKeyframe(prop, obj, allProps, easeEach) { + var ease = obj.ease || easeEach || "power1.inOut", + p, + a; + + if (_isArray(obj)) { + a = allProps[prop] || (allProps[prop] = []); + obj.forEach(function (value, i) { + return a.push({ + t: i / (obj.length - 1) * 100, + v: value, + e: ease + }); + }); + } else { + for (p in obj) { + a = allProps[p] || (allProps[p] = []); + p === "ease" || a.push({ + t: parseFloat(prop), + v: obj[p], + e: ease + }); + } + } + }, + _parseFuncOrString = function _parseFuncOrString(value, tween, i, target, targets) { + return _isFunction(value) ? value.call(tween, i, target, targets) : _isString(value) && ~value.indexOf("random(") ? _replaceRandom(value) : value; + }, + _staggerTweenProps = _callbackNames + "repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase,autoRevert", + _staggerPropsToSkip = {}; + + _forEachName(_staggerTweenProps + ",id,stagger,delay,duration,paused,scrollTrigger", function (name) { + return _staggerPropsToSkip[name] = 1; + }); + + var Tween = function (_Animation2) { + _inheritsLoose(Tween, _Animation2); + + function Tween(targets, vars, position, skipInherit) { + var _this3; + + if (typeof vars === "number") { + position.duration = vars; + vars = position; + position = null; + } + + _this3 = _Animation2.call(this, skipInherit ? vars : _inheritDefaults(vars)) || this; + var _this3$vars = _this3.vars, + duration = _this3$vars.duration, + delay = _this3$vars.delay, + immediateRender = _this3$vars.immediateRender, + stagger = _this3$vars.stagger, + overwrite = _this3$vars.overwrite, + keyframes = _this3$vars.keyframes, + defaults = _this3$vars.defaults, + scrollTrigger = _this3$vars.scrollTrigger, + yoyoEase = _this3$vars.yoyoEase, + parent = vars.parent || _globalTimeline, + parsedTargets = (_isArray(targets) || _isTypedArray(targets) ? _isNumber(targets[0]) : "length" in vars) ? [targets] : toArray(targets), + tl, + i, + copy, + l, + p, + curTarget, + staggerFunc, + staggerVarsToMerge; + _this3._targets = parsedTargets.length ? _harness(parsedTargets) : _warn("GSAP target " + targets + " not found. https://gsap.com", !_config.nullTargetWarn) || []; + _this3._ptLookup = []; + _this3._overwrite = overwrite; + + if (keyframes || stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + vars = _this3.vars; + tl = _this3.timeline = new Timeline({ + data: "nested", + defaults: defaults || {}, + targets: parent && parent.data === "nested" ? parent.vars.targets : parsedTargets + }); + tl.kill(); + tl.parent = tl._dp = _assertThisInitialized(_this3); + tl._start = 0; + + if (stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + l = parsedTargets.length; + staggerFunc = stagger && distribute(stagger); + + if (_isObject(stagger)) { + for (p in stagger) { + if (~_staggerTweenProps.indexOf(p)) { + staggerVarsToMerge || (staggerVarsToMerge = {}); + staggerVarsToMerge[p] = stagger[p]; + } + } + } + + for (i = 0; i < l; i++) { + copy = _copyExcluding(vars, _staggerPropsToSkip); + copy.stagger = 0; + yoyoEase && (copy.yoyoEase = yoyoEase); + staggerVarsToMerge && _merge(copy, staggerVarsToMerge); + curTarget = parsedTargets[i]; + copy.duration = +_parseFuncOrString(duration, _assertThisInitialized(_this3), i, curTarget, parsedTargets); + copy.delay = (+_parseFuncOrString(delay, _assertThisInitialized(_this3), i, curTarget, parsedTargets) || 0) - _this3._delay; + + if (!stagger && l === 1 && copy.delay) { + _this3._delay = delay = copy.delay; + _this3._start += delay; + copy.delay = 0; + } + + tl.to(curTarget, copy, staggerFunc ? staggerFunc(i, curTarget, parsedTargets) : 0); + tl._ease = _easeMap.none; + } + + tl.duration() ? duration = delay = 0 : _this3.timeline = 0; + } else if (keyframes) { + _inheritDefaults(_setDefaults(tl.vars.defaults, { + ease: "none" + })); + + tl._ease = _parseEase(keyframes.ease || vars.ease || "none"); + var time = 0, + a, + kf, + v; + + if (_isArray(keyframes)) { + keyframes.forEach(function (frame) { + return tl.to(parsedTargets, frame, ">"); + }); + tl.duration(); + } else { + copy = {}; + + for (p in keyframes) { + p === "ease" || p === "easeEach" || _parseKeyframe(p, keyframes[p], copy, keyframes.easeEach); + } + + for (p in copy) { + a = copy[p].sort(function (a, b) { + return a.t - b.t; + }); + time = 0; + + for (i = 0; i < a.length; i++) { + kf = a[i]; + v = { + ease: kf.e, + duration: (kf.t - (i ? a[i - 1].t : 0)) / 100 * duration + }; + v[p] = kf.v; + tl.to(parsedTargets, v, time); + time += v.duration; + } + } + + tl.duration() < duration && tl.to({}, { + duration: duration - tl.duration() + }); + } + } + + duration || _this3.duration(duration = tl.duration()); + } else { + _this3.timeline = 0; + } + + if (overwrite === true && !_suppressOverwrites) { + _overwritingTween = _assertThisInitialized(_this3); + + _globalTimeline.killTweensOf(parsedTargets); + + _overwritingTween = 0; + } + + _addToTimeline(parent, _assertThisInitialized(_this3), position); + + vars.reversed && _this3.reverse(); + vars.paused && _this3.paused(true); + + if (immediateRender || !duration && !keyframes && _this3._start === _roundPrecise(parent._time) && _isNotFalse(immediateRender) && _hasNoPausedAncestors(_assertThisInitialized(_this3)) && parent.data !== "nested") { + _this3._tTime = -_tinyNum; + + _this3.render(Math.max(0, -delay) || 0); + } + + scrollTrigger && _scrollTrigger(_assertThisInitialized(_this3), scrollTrigger); + return _this3; + } + + var _proto3 = Tween.prototype; + + _proto3.render = function render(totalTime, suppressEvents, force) { + var prevTime = this._time, + tDur = this._tDur, + dur = this._dur, + isNegative = totalTime < 0, + tTime = totalTime > tDur - _tinyNum && !isNegative ? tDur : totalTime < _tinyNum ? 0 : totalTime, + time, + pt, + iteration, + cycleDuration, + prevIteration, + isYoyo, + ratio, + timeline, + yoyoEase; + + if (!dur) { + _renderZeroDurationTween(this, totalTime, suppressEvents, force); + } else if (tTime !== this._tTime || !totalTime || force || !this._initted && this._tTime || this._startAt && this._zTime < 0 !== isNegative || this._lazy) { + time = tTime; + timeline = this.timeline; + + if (this._repeat) { + cycleDuration = dur + this._rDelay; + + if (this._repeat < -1 && isNegative) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + + time = _roundPrecise(tTime % cycleDuration); + + if (tTime === tDur) { + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); + iteration = ~~prevIteration; + + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } else if (time > dur) { + time = dur; + } + } + + isYoyo = this._yoyo && iteration & 1; + + if (isYoyo) { + yoyoEase = this._yEase; + time = dur - time; + } + + prevIteration = _animationCycle(this._tTime, cycleDuration); + + if (time === prevTime && !force && this._initted && iteration === prevIteration) { + this._tTime = tTime; + return this; + } + + if (iteration !== prevIteration) { + timeline && this._yEase && _propagateYoyoEase(timeline, isYoyo); + + if (this.vars.repeatRefresh && !isYoyo && !this._lock && time !== cycleDuration && this._initted) { + this._lock = force = 1; + this.render(_roundPrecise(cycleDuration * iteration), true).invalidate()._lock = 0; + } + } + } + + if (!this._initted) { + if (_attemptInitTween(this, isNegative ? totalTime : time, force, suppressEvents, tTime)) { + this._tTime = 0; + return this; + } + + if (prevTime !== this._time && !(force && this.vars.repeatRefresh && iteration !== prevIteration)) { + return this; + } + + if (dur !== this._dur) { + return this.render(totalTime, suppressEvents, force); + } + } + + this._tTime = tTime; + this._time = time; + + if (!this._act && this._ts) { + this._act = 1; + this._lazy = 0; + } + + this.ratio = ratio = (yoyoEase || this._ease)(time / dur); + + if (this._from) { + this.ratio = ratio = 1 - ratio; + } + + if (time && !prevTime && !suppressEvents && !iteration) { + _callback(this, "onStart"); + + if (this._tTime !== tTime) { + return this; + } + } + + pt = this._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + timeline && timeline.render(totalTime < 0 ? totalTime : timeline._dur * timeline._ease(time / this._dur), suppressEvents, force) || this._startAt && (this._zTime = totalTime); + + if (this._onUpdate && !suppressEvents) { + isNegative && _rewindStartAt(this, totalTime, suppressEvents, force); + + _callback(this, "onUpdate"); + } + + this._repeat && iteration !== prevIteration && this.vars.onRepeat && !suppressEvents && this.parent && _callback(this, "onRepeat"); + + if ((tTime === this._tDur || !tTime) && this._tTime === tTime) { + isNegative && !this._onUpdate && _rewindStartAt(this, totalTime, true, true); + (totalTime || !dur) && (tTime === this._tDur && this._ts > 0 || !tTime && this._ts < 0) && _removeFromParent(this, 1); + + if (!suppressEvents && !(isNegative && !prevTime) && (tTime || prevTime || isYoyo)) { + _callback(this, tTime === tDur ? "onComplete" : "onReverseComplete", true); + + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + + return this; + }; + + _proto3.targets = function targets() { + return this._targets; + }; + + _proto3.invalidate = function invalidate(soft) { + (!soft || !this.vars.runBackwards) && (this._startAt = 0); + this._pt = this._op = this._onUpdate = this._lazy = this.ratio = 0; + this._ptLookup = []; + this.timeline && this.timeline.invalidate(soft); + return _Animation2.prototype.invalidate.call(this, soft); + }; + + _proto3.resetTo = function resetTo(property, value, start, startIsRelative, skipRecursion) { + _tickerActive || _ticker.wake(); + this._ts || this.play(); + var time = Math.min(this._dur, (this._dp._time - this._start) * this._ts), + ratio; + this._initted || _initTween(this, time); + ratio = this._ease(time / this._dur); + + if (_updatePropTweens(this, property, value, start, startIsRelative, ratio, time, skipRecursion)) { + return this.resetTo(property, value, start, startIsRelative, 1); + } + + _alignPlayhead(this, 0); + + this.parent || _addLinkedListItem(this._dp, this, "_first", "_last", this._dp._sort ? "_start" : 0); + return this.render(0); + }; + + _proto3.kill = function kill(targets, vars) { + if (vars === void 0) { + vars = "all"; + } + + if (!targets && (!vars || vars === "all")) { + this._lazy = this._pt = 0; + this.parent ? _interrupt(this) : this.scrollTrigger && this.scrollTrigger.kill(!!_reverting); + return this; + } + + if (this.timeline) { + var tDur = this.timeline.totalDuration(); + this.timeline.killTweensOf(targets, vars, _overwritingTween && _overwritingTween.vars.overwrite !== true)._first || _interrupt(this); + this.parent && tDur !== this.timeline.totalDuration() && _setDuration(this, this._dur * this.timeline._tDur / tDur, 0, 1); + return this; + } + + var parsedTargets = this._targets, + killingTargets = targets ? toArray(targets) : parsedTargets, + propTweenLookup = this._ptLookup, + firstPT = this._pt, + overwrittenProps, + curLookup, + curOverwriteProps, + props, + p, + pt, + i; + + if ((!vars || vars === "all") && _arraysMatch(parsedTargets, killingTargets)) { + vars === "all" && (this._pt = 0); + return _interrupt(this); + } + + overwrittenProps = this._op = this._op || []; + + if (vars !== "all") { + if (_isString(vars)) { + p = {}; + + _forEachName(vars, function (name) { + return p[name] = 1; + }); + + vars = p; + } + + vars = _addAliasesToVars(parsedTargets, vars); + } + + i = parsedTargets.length; + + while (i--) { + if (~killingTargets.indexOf(parsedTargets[i])) { + curLookup = propTweenLookup[i]; + + if (vars === "all") { + overwrittenProps[i] = vars; + props = curLookup; + curOverwriteProps = {}; + } else { + curOverwriteProps = overwrittenProps[i] = overwrittenProps[i] || {}; + props = vars; + } + + for (p in props) { + pt = curLookup && curLookup[p]; + + if (pt) { + if (!("kill" in pt.d) || pt.d.kill(p) === true) { + _removeLinkedListItem(this, pt, "_pt"); + } + + delete curLookup[p]; + } + + if (curOverwriteProps !== "all") { + curOverwriteProps[p] = 1; + } + } + } + } + + this._initted && !this._pt && firstPT && _interrupt(this); + return this; + }; + + Tween.to = function to(targets, vars) { + return new Tween(targets, vars, arguments[2]); + }; + + Tween.from = function from(targets, vars) { + return _createTweenType(1, arguments); + }; + + Tween.delayedCall = function delayedCall(delay, callback, params, scope) { + return new Tween(callback, 0, { + immediateRender: false, + lazy: false, + overwrite: false, + delay: delay, + onComplete: callback, + onReverseComplete: callback, + onCompleteParams: params, + onReverseCompleteParams: params, + callbackScope: scope + }); + }; + + Tween.fromTo = function fromTo(targets, fromVars, toVars) { + return _createTweenType(2, arguments); + }; + + Tween.set = function set(targets, vars) { + vars.duration = 0; + vars.repeatDelay || (vars.repeat = 0); + return new Tween(targets, vars); + }; + + Tween.killTweensOf = function killTweensOf(targets, props, onlyActive) { + return _globalTimeline.killTweensOf(targets, props, onlyActive); + }; + + return Tween; + }(Animation); + + _setDefaults(Tween.prototype, { + _targets: [], + _lazy: 0, + _startAt: 0, + _op: 0, + _onInit: 0 + }); + + _forEachName("staggerTo,staggerFrom,staggerFromTo", function (name) { + Tween[name] = function () { + var tl = new Timeline(), + params = _slice.call(arguments, 0); + + params.splice(name === "staggerFromTo" ? 5 : 4, 0, 0); + return tl[name].apply(tl, params); + }; + }); + + var _setterPlain = function _setterPlain(target, property, value) { + return target[property] = value; + }, + _setterFunc = function _setterFunc(target, property, value) { + return target[property](value); + }, + _setterFuncWithParam = function _setterFuncWithParam(target, property, value, data) { + return target[property](data.fp, value); + }, + _setterAttribute = function _setterAttribute(target, property, value) { + return target.setAttribute(property, value); + }, + _getSetter = function _getSetter(target, property) { + return _isFunction(target[property]) ? _setterFunc : _isUndefined(target[property]) && target.setAttribute ? _setterAttribute : _setterPlain; + }, + _renderPlain = function _renderPlain(ratio, data) { + return data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 1000000) / 1000000, data); + }, + _renderBoolean = function _renderBoolean(ratio, data) { + return data.set(data.t, data.p, !!(data.s + data.c * ratio), data); + }, + _renderComplexString = function _renderComplexString(ratio, data) { + var pt = data._pt, + s = ""; + + if (!ratio && data.b) { + s = data.b; + } else if (ratio === 1 && data.e) { + s = data.e; + } else { + while (pt) { + s = pt.p + (pt.m ? pt.m(pt.s + pt.c * ratio) : Math.round((pt.s + pt.c * ratio) * 10000) / 10000) + s; + pt = pt._next; + } + + s += data.c; + } + + data.set(data.t, data.p, s, data); + }, + _renderPropTweens = function _renderPropTweens(ratio, data) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + }, + _addPluginModifier = function _addPluginModifier(modifier, tween, target, property) { + var pt = this._pt, + next; + + while (pt) { + next = pt._next; + pt.p === property && pt.modifier(modifier, tween, target); + pt = next; + } + }, + _killPropTweensOf = function _killPropTweensOf(property) { + var pt = this._pt, + hasNonDependentRemaining, + next; + + while (pt) { + next = pt._next; + + if (pt.p === property && !pt.op || pt.op === property) { + _removeLinkedListItem(this, pt, "_pt"); + } else if (!pt.dep) { + hasNonDependentRemaining = 1; + } + + pt = next; + } + + return !hasNonDependentRemaining; + }, + _setterWithModifier = function _setterWithModifier(target, property, value, data) { + data.mSet(target, property, data.m.call(data.tween, value, data.mt), data); + }, + _sortPropTweensByPriority = function _sortPropTweensByPriority(parent) { + var pt = parent._pt, + next, + pt2, + first, + last; + + while (pt) { + next = pt._next; + pt2 = first; + + while (pt2 && pt2.pr > pt.pr) { + pt2 = pt2._next; + } + + if (pt._prev = pt2 ? pt2._prev : last) { + pt._prev._next = pt; + } else { + first = pt; + } + + if (pt._next = pt2) { + pt2._prev = pt; + } else { + last = pt; + } + + pt = next; + } + + parent._pt = first; + }; + + var PropTween = function () { + function PropTween(next, target, prop, start, change, renderer, data, setter, priority) { + this.t = target; + this.s = start; + this.c = change; + this.p = prop; + this.r = renderer || _renderPlain; + this.d = data || this; + this.set = setter || _setterPlain; + this.pr = priority || 0; + this._next = next; + + if (next) { + next._prev = this; + } + } + + var _proto4 = PropTween.prototype; + + _proto4.modifier = function modifier(func, tween, target) { + this.mSet = this.mSet || this.set; + this.set = _setterWithModifier; + this.m = func; + this.mt = target; + this.tween = tween; + }; + + return PropTween; + }(); + + _forEachName(_callbackNames + "parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger", function (name) { + return _reservedProps[name] = 1; + }); + + _globals.TweenMax = _globals.TweenLite = Tween; + _globals.TimelineLite = _globals.TimelineMax = Timeline; + _globalTimeline = new Timeline({ + sortChildren: false, + defaults: _defaults, + autoRemoveChildren: true, + id: "root", + smoothChildTiming: true + }); + _config.stringFilter = _colorStringFilter; + + var _media = [], + _listeners = {}, + _emptyArray = [], + _lastMediaTime = 0, + _contextID = 0, + _dispatch = function _dispatch(type) { + return (_listeners[type] || _emptyArray).map(function (f) { + return f(); + }); + }, + _onMediaChange = function _onMediaChange() { + var time = Date.now(), + matches = []; + + if (time - _lastMediaTime > 2) { + _dispatch("matchMediaInit"); + + _media.forEach(function (c) { + var queries = c.queries, + conditions = c.conditions, + match, + p, + anyMatch, + toggled; + + for (p in queries) { + match = _win.matchMedia(queries[p]).matches; + match && (anyMatch = 1); + + if (match !== conditions[p]) { + conditions[p] = match; + toggled = 1; + } + } + + if (toggled) { + c.revert(); + anyMatch && matches.push(c); + } + }); + + _dispatch("matchMediaRevert"); + + matches.forEach(function (c) { + return c.onMatch(c, function (func) { + return c.add(null, func); + }); + }); + _lastMediaTime = time; + + _dispatch("matchMedia"); + } + }; + + var Context = function () { + function Context(func, scope) { + this.selector = scope && selector(scope); + this.data = []; + this._r = []; + this.isReverted = false; + this.id = _contextID++; + func && this.add(func); + } + + var _proto5 = Context.prototype; + + _proto5.add = function add(name, func, scope) { + if (_isFunction(name)) { + scope = func; + func = name; + name = _isFunction; + } + + var self = this, + f = function f() { + var prev = _context, + prevSelector = self.selector, + result; + prev && prev !== self && prev.data.push(self); + scope && (self.selector = selector(scope)); + _context = self; + result = func.apply(self, arguments); + _isFunction(result) && self._r.push(result); + _context = prev; + self.selector = prevSelector; + self.isReverted = false; + return result; + }; + + self.last = f; + return name === _isFunction ? f(self, function (func) { + return self.add(null, func); + }) : name ? self[name] = f : f; + }; + + _proto5.ignore = function ignore(func) { + var prev = _context; + _context = null; + func(this); + _context = prev; + }; + + _proto5.getTweens = function getTweens() { + var a = []; + this.data.forEach(function (e) { + return e instanceof Context ? a.push.apply(a, e.getTweens()) : e instanceof Tween && !(e.parent && e.parent.data === "nested") && a.push(e); + }); + return a; + }; + + _proto5.clear = function clear() { + this._r.length = this.data.length = 0; + }; + + _proto5.kill = function kill(revert, matchMedia) { + var _this4 = this; + + if (revert) { + (function () { + var tweens = _this4.getTweens(), + i = _this4.data.length, + t; + + while (i--) { + t = _this4.data[i]; + + if (t.data === "isFlip") { + t.revert(); + t.getChildren(true, true, false).forEach(function (tween) { + return tweens.splice(tweens.indexOf(tween), 1); + }); + } + } + + tweens.map(function (t) { + return { + g: t._dur || t._delay || t._sat && !t._sat.vars.immediateRender ? t.globalTime(0) : -Infinity, + t: t + }; + }).sort(function (a, b) { + return b.g - a.g || -Infinity; + }).forEach(function (o) { + return o.t.revert(revert); + }); + i = _this4.data.length; + + while (i--) { + t = _this4.data[i]; + + if (t instanceof Timeline) { + if (t.data !== "nested") { + t.scrollTrigger && t.scrollTrigger.revert(); + t.kill(); + } + } else { + !(t instanceof Tween) && t.revert && t.revert(revert); + } + } + + _this4._r.forEach(function (f) { + return f(revert, _this4); + }); + + _this4.isReverted = true; + })(); + } else { + this.data.forEach(function (e) { + return e.kill && e.kill(); + }); + } + + this.clear(); + + if (matchMedia) { + var i = _media.length; + + while (i--) { + _media[i].id === this.id && _media.splice(i, 1); + } + } + }; + + _proto5.revert = function revert(config) { + this.kill(config || {}); + }; + + return Context; + }(); + + var MatchMedia = function () { + function MatchMedia(scope) { + this.contexts = []; + this.scope = scope; + _context && _context.data.push(this); + } + + var _proto6 = MatchMedia.prototype; + + _proto6.add = function add(conditions, func, scope) { + _isObject(conditions) || (conditions = { + matches: conditions + }); + var context = new Context(0, scope || this.scope), + cond = context.conditions = {}, + mq, + p, + active; + _context && !context.selector && (context.selector = _context.selector); + this.contexts.push(context); + func = context.add("onMatch", func); + context.queries = conditions; + + for (p in conditions) { + if (p === "all") { + active = 1; + } else { + mq = _win.matchMedia(conditions[p]); + + if (mq) { + _media.indexOf(context) < 0 && _media.push(context); + (cond[p] = mq.matches) && (active = 1); + mq.addListener ? mq.addListener(_onMediaChange) : mq.addEventListener("change", _onMediaChange); + } + } + } + + active && func(context, function (f) { + return context.add(null, f); + }); + return this; + }; + + _proto6.revert = function revert(config) { + this.kill(config || {}); + }; + + _proto6.kill = function kill(revert) { + this.contexts.forEach(function (c) { + return c.kill(revert, true); + }); + }; + + return MatchMedia; + }(); + + var _gsap = { + registerPlugin: function registerPlugin() { + for (var _len2 = arguments.length, args = new Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { + args[_key2] = arguments[_key2]; + } + + args.forEach(function (config) { + return _createPlugin(config); + }); + }, + timeline: function timeline(vars) { + return new Timeline(vars); + }, + getTweensOf: function getTweensOf(targets, onlyActive) { + return _globalTimeline.getTweensOf(targets, onlyActive); + }, + getProperty: function getProperty(target, property, unit, uncache) { + _isString(target) && (target = toArray(target)[0]); + + var getter = _getCache(target || {}).get, + format = unit ? _passThrough : _numericIfPossible; + + unit === "native" && (unit = ""); + return !target ? target : !property ? function (property, unit, uncache) { + return format((_plugins[property] && _plugins[property].get || getter)(target, property, unit, uncache)); + } : format((_plugins[property] && _plugins[property].get || getter)(target, property, unit, uncache)); + }, + quickSetter: function quickSetter(target, property, unit) { + target = toArray(target); + + if (target.length > 1) { + var setters = target.map(function (t) { + return gsap.quickSetter(t, property, unit); + }), + l = setters.length; + return function (value) { + var i = l; + + while (i--) { + setters[i](value); + } + }; + } + + target = target[0] || {}; + + var Plugin = _plugins[property], + cache = _getCache(target), + p = cache.harness && (cache.harness.aliases || {})[property] || property, + setter = Plugin ? function (value) { + var p = new Plugin(); + _quickTween._pt = 0; + p.init(target, unit ? value + unit : value, _quickTween, 0, [target]); + p.render(1, p); + _quickTween._pt && _renderPropTweens(1, _quickTween); + } : cache.set(target, p); + + return Plugin ? setter : function (value) { + return setter(target, p, unit ? value + unit : value, cache, 1); + }; + }, + quickTo: function quickTo(target, property, vars) { + var _setDefaults2; + + var tween = gsap.to(target, _setDefaults((_setDefaults2 = {}, _setDefaults2[property] = "+=0.1", _setDefaults2.paused = true, _setDefaults2.stagger = 0, _setDefaults2), vars || {})), + func = function func(value, start, startIsRelative) { + return tween.resetTo(property, value, start, startIsRelative); + }; + + func.tween = tween; + return func; + }, + isTweening: function isTweening(targets) { + return _globalTimeline.getTweensOf(targets, true).length > 0; + }, + defaults: function defaults(value) { + value && value.ease && (value.ease = _parseEase(value.ease, _defaults.ease)); + return _mergeDeep(_defaults, value || {}); + }, + config: function config(value) { + return _mergeDeep(_config, value || {}); + }, + registerEffect: function registerEffect(_ref3) { + var name = _ref3.name, + effect = _ref3.effect, + plugins = _ref3.plugins, + defaults = _ref3.defaults, + extendTimeline = _ref3.extendTimeline; + (plugins || "").split(",").forEach(function (pluginName) { + return pluginName && !_plugins[pluginName] && !_globals[pluginName] && _warn(name + " effect requires " + pluginName + " plugin."); + }); + + _effects[name] = function (targets, vars, tl) { + return effect(toArray(targets), _setDefaults(vars || {}, defaults), tl); + }; + + if (extendTimeline) { + Timeline.prototype[name] = function (targets, vars, position) { + return this.add(_effects[name](targets, _isObject(vars) ? vars : (position = vars) && {}, this), position); + }; + } + }, + registerEase: function registerEase(name, ease) { + _easeMap[name] = _parseEase(ease); + }, + parseEase: function parseEase(ease, defaultEase) { + return arguments.length ? _parseEase(ease, defaultEase) : _easeMap; + }, + getById: function getById(id) { + return _globalTimeline.getById(id); + }, + exportRoot: function exportRoot(vars, includeDelayedCalls) { + if (vars === void 0) { + vars = {}; + } + + var tl = new Timeline(vars), + child, + next; + tl.smoothChildTiming = _isNotFalse(vars.smoothChildTiming); + + _globalTimeline.remove(tl); + + tl._dp = 0; + tl._time = tl._tTime = _globalTimeline._time; + child = _globalTimeline._first; + + while (child) { + next = child._next; + + if (includeDelayedCalls || !(!child._dur && child instanceof Tween && child.vars.onComplete === child._targets[0])) { + _addToTimeline(tl, child, child._start - child._delay); + } + + child = next; + } + + _addToTimeline(_globalTimeline, tl, 0); + + return tl; + }, + context: function context(func, scope) { + return func ? new Context(func, scope) : _context; + }, + matchMedia: function matchMedia(scope) { + return new MatchMedia(scope); + }, + matchMediaRefresh: function matchMediaRefresh() { + return _media.forEach(function (c) { + var cond = c.conditions, + found, + p; + + for (p in cond) { + if (cond[p]) { + cond[p] = false; + found = 1; + } + } + + found && c.revert(); + }) || _onMediaChange(); + }, + addEventListener: function addEventListener(type, callback) { + var a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); + }, + removeEventListener: function removeEventListener(type, callback) { + var a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); + }, + utils: { + wrap: wrap, + wrapYoyo: wrapYoyo, + distribute: distribute, + random: random, + snap: snap, + normalize: normalize, + getUnit: getUnit, + clamp: clamp, + splitColor: splitColor, + toArray: toArray, + selector: selector, + mapRange: mapRange, + pipe: pipe, + unitize: unitize, + interpolate: interpolate, + shuffle: shuffle + }, + install: _install, + effects: _effects, + ticker: _ticker, + updateRoot: Timeline.updateRoot, + plugins: _plugins, + globalTimeline: _globalTimeline, + core: { + PropTween: PropTween, + globals: _addGlobal, + Tween: Tween, + Timeline: Timeline, + Animation: Animation, + getCache: _getCache, + _removeLinkedListItem: _removeLinkedListItem, + reverting: function reverting() { + return _reverting; + }, + context: function context(toAdd) { + if (toAdd && _context) { + _context.data.push(toAdd); + + toAdd._ctx = _context; + } + + return _context; + }, + suppressOverwrites: function suppressOverwrites(value) { + return _suppressOverwrites = value; + } + } + }; + + _forEachName("to,from,fromTo,delayedCall,set,killTweensOf", function (name) { + return _gsap[name] = Tween[name]; + }); + + _ticker.add(Timeline.updateRoot); + + _quickTween = _gsap.to({}, { + duration: 0 + }); + + var _getPluginPropTween = function _getPluginPropTween(plugin, prop) { + var pt = plugin._pt; + + while (pt && pt.p !== prop && pt.op !== prop && pt.fp !== prop) { + pt = pt._next; + } + + return pt; + }, + _addModifiers = function _addModifiers(tween, modifiers) { + var targets = tween._targets, + p, + i, + pt; + + for (p in modifiers) { + i = targets.length; + + while (i--) { + pt = tween._ptLookup[i][p]; + + if (pt && (pt = pt.d)) { + if (pt._pt) { + pt = _getPluginPropTween(pt, p); + } + + pt && pt.modifier && pt.modifier(modifiers[p], tween, targets[i], p); + } + } + } + }, + _buildModifierPlugin = function _buildModifierPlugin(name, modifier) { + return { + name: name, + rawVars: 1, + init: function init(target, vars, tween) { + tween._onInit = function (tween) { + var temp, p; + + if (_isString(vars)) { + temp = {}; + + _forEachName(vars, function (name) { + return temp[name] = 1; + }); + + vars = temp; + } + + if (modifier) { + temp = {}; + + for (p in vars) { + temp[p] = modifier(vars[p]); + } + + vars = temp; + } + + _addModifiers(tween, vars); + }; + } + }; + }; + + var gsap = _gsap.registerPlugin({ + name: "attr", + init: function init(target, vars, tween, index, targets) { + var p, pt, v; + this.tween = tween; + + for (p in vars) { + v = target.getAttribute(p) || ""; + pt = this.add(target, "setAttribute", (v || 0) + "", vars[p], index, targets, 0, 0, p); + pt.op = p; + pt.b = v; + + this._props.push(p); + } + }, + render: function render(ratio, data) { + var pt = data._pt; + + while (pt) { + _reverting ? pt.set(pt.t, pt.p, pt.b, pt) : pt.r(ratio, pt.d); + pt = pt._next; + } + } + }, { + name: "endArray", + init: function init(target, value) { + var i = value.length; + + while (i--) { + this.add(target, i, target[i] || 0, value[i], 0, 0, 0, 0, 0, 1); + } + } + }, _buildModifierPlugin("roundProps", _roundModifier), _buildModifierPlugin("modifiers"), _buildModifierPlugin("snap", snap)) || _gsap; + Tween.version = Timeline.version = gsap.version = "3.12.7"; + _coreReady = 1; + _windowExists() && _wake(); + var Power0 = _easeMap.Power0, + Power1 = _easeMap.Power1, + Power2 = _easeMap.Power2, + Power3 = _easeMap.Power3, + Power4 = _easeMap.Power4, + Linear = _easeMap.Linear, + Quad = _easeMap.Quad, + Cubic = _easeMap.Cubic, + Quart = _easeMap.Quart, + Quint = _easeMap.Quint, + Strong = _easeMap.Strong, + Elastic = _easeMap.Elastic, + Back = _easeMap.Back, + SteppedEase = _easeMap.SteppedEase, + Bounce = _easeMap.Bounce, + Sine = _easeMap.Sine, + Expo = _easeMap.Expo, + Circ = _easeMap.Circ; + + var _win$1, + _doc$1, + _docElement, + _pluginInitted, + _tempDiv, + _tempDivStyler, + _recentSetterPlugin, + _reverting$1, + _windowExists$1 = function _windowExists() { + return typeof window !== "undefined"; + }, + _transformProps = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _atan2 = Math.atan2, + _bigNum$1 = 1e8, + _capsExp = /([A-Z])/g, + _horizontalExp = /(left|right|width|margin|padding|x)/i, + _complexExp = /[\s,\(]\S/, + _propertyAliases = { + autoAlpha: "opacity,visibility", + scale: "scaleX,scaleY", + alpha: "opacity" + }, + _renderCSSProp = function _renderCSSProp(ratio, data) { + return data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u, data); + }, + _renderPropWithEnd = function _renderPropWithEnd(ratio, data) { + return data.set(data.t, data.p, ratio === 1 ? data.e : Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u, data); + }, + _renderCSSPropWithBeginning = function _renderCSSPropWithBeginning(ratio, data) { + return data.set(data.t, data.p, ratio ? Math.round((data.s + data.c * ratio) * 10000) / 10000 + data.u : data.b, data); + }, + _renderRoundedCSSProp = function _renderRoundedCSSProp(ratio, data) { + var value = data.s + data.c * ratio; + data.set(data.t, data.p, ~~(value + (value < 0 ? -.5 : .5)) + data.u, data); + }, + _renderNonTweeningValue = function _renderNonTweeningValue(ratio, data) { + return data.set(data.t, data.p, ratio ? data.e : data.b, data); + }, + _renderNonTweeningValueOnlyAtEnd = function _renderNonTweeningValueOnlyAtEnd(ratio, data) { + return data.set(data.t, data.p, ratio !== 1 ? data.b : data.e, data); + }, + _setterCSSStyle = function _setterCSSStyle(target, property, value) { + return target.style[property] = value; + }, + _setterCSSProp = function _setterCSSProp(target, property, value) { + return target.style.setProperty(property, value); + }, + _setterTransform = function _setterTransform(target, property, value) { + return target._gsap[property] = value; + }, + _setterScale = function _setterScale(target, property, value) { + return target._gsap.scaleX = target._gsap.scaleY = value; + }, + _setterScaleWithRender = function _setterScaleWithRender(target, property, value, data, ratio) { + var cache = target._gsap; + cache.scaleX = cache.scaleY = value; + cache.renderTransform(ratio, cache); + }, + _setterTransformWithRender = function _setterTransformWithRender(target, property, value, data, ratio) { + var cache = target._gsap; + cache[property] = value; + cache.renderTransform(ratio, cache); + }, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _saveStyle = function _saveStyle(property, isNotCSS) { + var _this = this; + + var target = this.target, + style = target.style, + cache = target._gsap; + + if (property in _transformProps && style) { + this.tfm = this.tfm || {}; + + if (property !== "transform") { + property = _propertyAliases[property] || property; + ~property.indexOf(",") ? property.split(",").forEach(function (a) { + return _this.tfm[a] = _get(target, a); + }) : this.tfm[property] = cache.x ? cache[property] : _get(target, property); + property === _transformOriginProp && (this.tfm.zOrigin = cache.zOrigin); + } else { + return _propertyAliases.transform.split(",").forEach(function (p) { + return _saveStyle.call(_this, p, isNotCSS); + }); + } + + if (this.props.indexOf(_transformProp) >= 0) { + return; + } + + if (cache.svg) { + this.svgo = target.getAttribute("data-svg-origin"); + this.props.push(_transformOriginProp, isNotCSS, ""); + } + + property = _transformProp; + } + + (style || isNotCSS) && this.props.push(property, isNotCSS, style[property]); + }, + _removeIndependentTransforms = function _removeIndependentTransforms(style) { + if (style.translate) { + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } + }, + _revertStyle = function _revertStyle() { + var props = this.props, + target = this.target, + style = target.style, + cache = target._gsap, + i, + p; + + for (i = 0; i < props.length; i += 3) { + if (!props[i + 1]) { + props[i + 2] ? style[props[i]] = props[i + 2] : style.removeProperty(props[i].substr(0, 2) === "--" ? props[i] : props[i].replace(_capsExp, "-$1").toLowerCase()); + } else if (props[i + 1] === 2) { + target[props[i]](props[i + 2]); + } else { + target[props[i]] = props[i + 2]; + } + } + + if (this.tfm) { + for (p in this.tfm) { + cache[p] = this.tfm[p]; + } + + if (cache.svg) { + cache.renderTransform(); + target.setAttribute("data-svg-origin", this.svgo || ""); + } + + i = _reverting$1(); + + if ((!i || !i.isStart) && !style[_transformProp]) { + _removeIndependentTransforms(style); + + if (cache.zOrigin && style[_transformOriginProp]) { + style[_transformOriginProp] += " " + cache.zOrigin + "px"; + cache.zOrigin = 0; + cache.renderTransform(); + } + + cache.uncache = 1; + } + } + }, + _getStyleSaver = function _getStyleSaver(target, properties) { + var saver = { + target: target, + props: [], + revert: _revertStyle, + save: _saveStyle + }; + target._gsap || gsap.core.getCache(target); + properties && target.style && target.nodeType && properties.split(",").forEach(function (p) { + return saver.save(p); + }); + return saver; + }, + _supports3D, + _createElement = function _createElement(type, ns) { + var e = _doc$1.createElementNS ? _doc$1.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc$1.createElement(type); + return e && e.style ? e : _doc$1.createElement(type); + }, + _getComputedProperty = function _getComputedProperty(target, property, skipPrefixFallback) { + var cs = getComputedStyle(target); + return cs[property] || cs.getPropertyValue(property.replace(_capsExp, "-$1").toLowerCase()) || cs.getPropertyValue(property) || !skipPrefixFallback && _getComputedProperty(target, _checkPropPrefix(property) || property, 1) || ""; + }, + _prefixes = "O,Moz,ms,Ms,Webkit".split(","), + _checkPropPrefix = function _checkPropPrefix(property, element, preferPrefix) { + var e = element || _tempDiv, + s = e.style, + i = 5; + + if (property in s && !preferPrefix) { + return property; + } + + property = property.charAt(0).toUpperCase() + property.substr(1); + + while (i-- && !(_prefixes[i] + property in s)) {} + + return i < 0 ? null : (i === 3 ? "ms" : i >= 0 ? _prefixes[i] : "") + property; + }, + _initCore = function _initCore() { + if (_windowExists$1() && window.document) { + _win$1 = window; + _doc$1 = _win$1.document; + _docElement = _doc$1.documentElement; + _tempDiv = _createElement("div") || { + style: {} + }; + _tempDivStyler = _createElement("div"); + _transformProp = _checkPropPrefix(_transformProp); + _transformOriginProp = _transformProp + "Origin"; + _tempDiv.style.cssText = "border-width:0;line-height:0;position:absolute;padding:0"; + _supports3D = !!_checkPropPrefix("perspective"); + _reverting$1 = gsap.core.reverting; + _pluginInitted = 1; + } + }, + _getReparentedCloneBBox = function _getReparentedCloneBBox(target) { + var owner = target.ownerSVGElement, + svg = _createElement("svg", owner && owner.getAttribute("xmlns") || "http://www.w3.org/2000/svg"), + clone = target.cloneNode(true), + bbox; + + clone.style.display = "block"; + svg.appendChild(clone); + + _docElement.appendChild(svg); + + try { + bbox = clone.getBBox(); + } catch (e) {} + + svg.removeChild(clone); + + _docElement.removeChild(svg); + + return bbox; + }, + _getAttributeFallbacks = function _getAttributeFallbacks(target, attributesArray) { + var i = attributesArray.length; + + while (i--) { + if (target.hasAttribute(attributesArray[i])) { + return target.getAttribute(attributesArray[i]); + } + } + }, + _getBBox = function _getBBox(target) { + var bounds, cloned; + + try { + bounds = target.getBBox(); + } catch (error) { + bounds = _getReparentedCloneBBox(target); + cloned = 1; + } + + bounds && (bounds.width || bounds.height) || cloned || (bounds = _getReparentedCloneBBox(target)); + return bounds && !bounds.width && !bounds.x && !bounds.y ? { + x: +_getAttributeFallbacks(target, ["x", "cx", "x1"]) || 0, + y: +_getAttributeFallbacks(target, ["y", "cy", "y1"]) || 0, + width: 0, + height: 0 + } : bounds; + }, + _isSVG = function _isSVG(e) { + return !!(e.getCTM && (!e.parentNode || e.ownerSVGElement) && _getBBox(e)); + }, + _removeProperty = function _removeProperty(target, property) { + if (property) { + var style = target.style, + first2Chars; + + if (property in _transformProps && property !== _transformOriginProp) { + property = _transformProp; + } + + if (style.removeProperty) { + first2Chars = property.substr(0, 2); + + if (first2Chars === "ms" || property.substr(0, 6) === "webkit") { + property = "-" + property; + } + + style.removeProperty(first2Chars === "--" ? property : property.replace(_capsExp, "-$1").toLowerCase()); + } else { + style.removeAttribute(property); + } + } + }, + _addNonTweeningPT = function _addNonTweeningPT(plugin, target, property, beginning, end, onlySetAtEnd) { + var pt = new PropTween(plugin._pt, target, property, 0, 1, onlySetAtEnd ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue); + plugin._pt = pt; + pt.b = beginning; + pt.e = end; + + plugin._props.push(property); + + return pt; + }, + _nonConvertibleUnits = { + deg: 1, + rad: 1, + turn: 1 + }, + _nonStandardLayouts = { + grid: 1, + flex: 1 + }, + _convertToUnit = function _convertToUnit(target, property, value, unit) { + var curValue = parseFloat(value) || 0, + curUnit = (value + "").trim().substr((curValue + "").length) || "px", + style = _tempDiv.style, + horizontal = _horizontalExp.test(property), + isRootSVG = target.tagName.toLowerCase() === "svg", + measureProperty = (isRootSVG ? "client" : "offset") + (horizontal ? "Width" : "Height"), + amount = 100, + toPixels = unit === "px", + toPercent = unit === "%", + px, + parent, + cache, + isSVG; + + if (unit === curUnit || !curValue || _nonConvertibleUnits[unit] || _nonConvertibleUnits[curUnit]) { + return curValue; + } + + curUnit !== "px" && !toPixels && (curValue = _convertToUnit(target, property, value, "px")); + isSVG = target.getCTM && _isSVG(target); + + if ((toPercent || curUnit === "%") && (_transformProps[property] || ~property.indexOf("adius"))) { + px = isSVG ? target.getBBox()[horizontal ? "width" : "height"] : target[measureProperty]; + return _round(toPercent ? curValue / px * amount : curValue / 100 * px); + } + + style[horizontal ? "width" : "height"] = amount + (toPixels ? curUnit : unit); + parent = unit !== "rem" && ~property.indexOf("adius") || unit === "em" && target.appendChild && !isRootSVG ? target : target.parentNode; + + if (isSVG) { + parent = (target.ownerSVGElement || {}).parentNode; + } + + if (!parent || parent === _doc$1 || !parent.appendChild) { + parent = _doc$1.body; + } + + cache = parent._gsap; + + if (cache && toPercent && cache.width && horizontal && cache.time === _ticker.time && !cache.uncache) { + return _round(curValue / cache.width * amount); + } else { + if (toPercent && (property === "height" || property === "width")) { + var v = target.style[property]; + target.style[property] = amount + unit; + px = target[measureProperty]; + v ? target.style[property] = v : _removeProperty(target, property); + } else { + (toPercent || curUnit === "%") && !_nonStandardLayouts[_getComputedProperty(parent, "display")] && (style.position = _getComputedProperty(target, "position")); + parent === target && (style.position = "static"); + parent.appendChild(_tempDiv); + px = _tempDiv[measureProperty]; + parent.removeChild(_tempDiv); + style.position = "absolute"; + } + + if (horizontal && toPercent) { + cache = _getCache(parent); + cache.time = _ticker.time; + cache.width = parent[measureProperty]; + } + } + + return _round(toPixels ? px * curValue / amount : px && curValue ? amount / px * curValue : 0); + }, + _get = function _get(target, property, unit, uncache) { + var value; + _pluginInitted || _initCore(); + + if (property in _propertyAliases && property !== "transform") { + property = _propertyAliases[property]; + + if (~property.indexOf(",")) { + property = property.split(",")[0]; + } + } + + if (_transformProps[property] && property !== "transform") { + value = _parseTransform(target, uncache); + value = property !== "transformOrigin" ? value[property] : value.svg ? value.origin : _firstTwoOnly(_getComputedProperty(target, _transformOriginProp)) + " " + value.zOrigin + "px"; + } else { + value = target.style[property]; + + if (!value || value === "auto" || uncache || ~(value + "").indexOf("calc(")) { + value = _specialProps[property] && _specialProps[property](target, property, unit) || _getComputedProperty(target, property) || _getProperty(target, property) || (property === "opacity" ? 1 : 0); + } + } + + return unit && !~(value + "").trim().indexOf(" ") ? _convertToUnit(target, property, value, unit) + unit : value; + }, + _tweenComplexCSSString = function _tweenComplexCSSString(target, prop, start, end) { + if (!start || start === "none") { + var p = _checkPropPrefix(prop, target, 1), + s = p && _getComputedProperty(target, p, 1); + + if (s && s !== start) { + prop = p; + start = s; + } else if (prop === "borderColor") { + start = _getComputedProperty(target, "borderTopColor"); + } + } + + var pt = new PropTween(this._pt, target.style, prop, 0, 1, _renderComplexString), + index = 0, + matchIndex = 0, + a, + result, + startValues, + startNum, + color, + startValue, + endValue, + endNum, + chunk, + endUnit, + startUnit, + endValues; + pt.b = start; + pt.e = end; + start += ""; + end += ""; + + if (end === "auto") { + startValue = target.style[prop]; + target.style[prop] = end; + end = _getComputedProperty(target, prop) || end; + startValue ? target.style[prop] = startValue : _removeProperty(target, prop); + } + + a = [start, end]; + + _colorStringFilter(a); + + start = a[0]; + end = a[1]; + startValues = start.match(_numWithUnitExp) || []; + endValues = end.match(_numWithUnitExp) || []; + + if (endValues.length) { + while (result = _numWithUnitExp.exec(end)) { + endValue = result[0]; + chunk = end.substring(index, result.index); + + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(" || chunk.substr(-5) === "hsla(") { + color = 1; + } + + if (endValue !== (startValue = startValues[matchIndex++] || "")) { + startNum = parseFloat(startValue) || 0; + startUnit = startValue.substr((startNum + "").length); + endValue.charAt(1) === "=" && (endValue = _parseRelative(startNum, endValue) + startUnit); + endNum = parseFloat(endValue); + endUnit = endValue.substr((endNum + "").length); + index = _numWithUnitExp.lastIndex - endUnit.length; + + if (!endUnit) { + endUnit = endUnit || _config.units[prop] || startUnit; + + if (index === end.length) { + end += endUnit; + pt.e += endUnit; + } + } + + if (startUnit !== endUnit) { + startNum = _convertToUnit(target, prop, startValue, endUnit) || 0; + } + + pt._pt = { + _next: pt._pt, + p: chunk || matchIndex === 1 ? chunk : ",", + s: startNum, + c: endNum - startNum, + m: color && color < 4 || prop === "zIndex" ? Math.round : 0 + }; + } + } + + pt.c = index < end.length ? end.substring(index, end.length) : ""; + } else { + pt.r = prop === "display" && end === "none" ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue; + } + + _relExp.test(end) && (pt.e = 0); + this._pt = pt; + return pt; + }, + _keywordToPercent = { + top: "0%", + bottom: "100%", + left: "0%", + right: "100%", + center: "50%" + }, + _convertKeywordsToPercentages = function _convertKeywordsToPercentages(value) { + var split = value.split(" "), + x = split[0], + y = split[1] || "50%"; + + if (x === "top" || x === "bottom" || y === "left" || y === "right") { + value = x; + x = y; + y = value; + } + + split[0] = _keywordToPercent[x] || x; + split[1] = _keywordToPercent[y] || y; + return split.join(" "); + }, + _renderClearProps = function _renderClearProps(ratio, data) { + if (data.tween && data.tween._time === data.tween._dur) { + var target = data.t, + style = target.style, + props = data.u, + cache = target._gsap, + prop, + clearTransforms, + i; + + if (props === "all" || props === true) { + style.cssText = ""; + clearTransforms = 1; + } else { + props = props.split(","); + i = props.length; + + while (--i > -1) { + prop = props[i]; + + if (_transformProps[prop]) { + clearTransforms = 1; + prop = prop === "transformOrigin" ? _transformOriginProp : _transformProp; + } + + _removeProperty(target, prop); + } + } + + if (clearTransforms) { + _removeProperty(target, _transformProp); + + if (cache) { + cache.svg && target.removeAttribute("transform"); + style.scale = style.rotate = style.translate = "none"; + + _parseTransform(target, 1); + + cache.uncache = 1; + + _removeIndependentTransforms(style); + } + } + } + }, + _specialProps = { + clearProps: function clearProps(plugin, target, property, endValue, tween) { + if (tween.data !== "isFromStart") { + var pt = plugin._pt = new PropTween(plugin._pt, target, property, 0, 0, _renderClearProps); + pt.u = endValue; + pt.pr = -10; + pt.tween = tween; + + plugin._props.push(property); + + return 1; + } + } + }, + _identity2DMatrix = [1, 0, 0, 1, 0, 0], + _rotationalProperties = {}, + _isNullTransform = function _isNullTransform(value) { + return value === "matrix(1, 0, 0, 1, 0, 0)" || value === "none" || !value; + }, + _getComputedTransformMatrixAsArray = function _getComputedTransformMatrixAsArray(target) { + var matrixString = _getComputedProperty(target, _transformProp); + + return _isNullTransform(matrixString) ? _identity2DMatrix : matrixString.substr(7).match(_numExp).map(_round); + }, + _getMatrix = function _getMatrix(target, force2D) { + var cache = target._gsap || _getCache(target), + style = target.style, + matrix = _getComputedTransformMatrixAsArray(target), + parent, + nextSibling, + temp, + addedToDOM; + + if (cache.svg && target.getAttribute("transform")) { + temp = target.transform.baseVal.consolidate().matrix; + matrix = [temp.a, temp.b, temp.c, temp.d, temp.e, temp.f]; + return matrix.join(",") === "1,0,0,1,0,0" ? _identity2DMatrix : matrix; + } else if (matrix === _identity2DMatrix && !target.offsetParent && target !== _docElement && !cache.svg) { + temp = style.display; + style.display = "block"; + parent = target.parentNode; + + if (!parent || !target.offsetParent && !target.getBoundingClientRect().width) { + addedToDOM = 1; + nextSibling = target.nextElementSibling; + + _docElement.appendChild(target); + } + + matrix = _getComputedTransformMatrixAsArray(target); + temp ? style.display = temp : _removeProperty(target, "display"); + + if (addedToDOM) { + nextSibling ? parent.insertBefore(target, nextSibling) : parent ? parent.appendChild(target) : _docElement.removeChild(target); + } + } + + return force2D && matrix.length > 6 ? [matrix[0], matrix[1], matrix[4], matrix[5], matrix[12], matrix[13]] : matrix; + }, + _applySVGOrigin = function _applySVGOrigin(target, origin, originIsAbsolute, smooth, matrixArray, pluginToAddPropTweensTo) { + var cache = target._gsap, + matrix = matrixArray || _getMatrix(target, true), + xOriginOld = cache.xOrigin || 0, + yOriginOld = cache.yOrigin || 0, + xOffsetOld = cache.xOffset || 0, + yOffsetOld = cache.yOffset || 0, + a = matrix[0], + b = matrix[1], + c = matrix[2], + d = matrix[3], + tx = matrix[4], + ty = matrix[5], + originSplit = origin.split(" "), + xOrigin = parseFloat(originSplit[0]) || 0, + yOrigin = parseFloat(originSplit[1]) || 0, + bounds, + determinant, + x, + y; + + if (!originIsAbsolute) { + bounds = _getBBox(target); + xOrigin = bounds.x + (~originSplit[0].indexOf("%") ? xOrigin / 100 * bounds.width : xOrigin); + yOrigin = bounds.y + (~(originSplit[1] || originSplit[0]).indexOf("%") ? yOrigin / 100 * bounds.height : yOrigin); + } else if (matrix !== _identity2DMatrix && (determinant = a * d - b * c)) { + x = xOrigin * (d / determinant) + yOrigin * (-c / determinant) + (c * ty - d * tx) / determinant; + y = xOrigin * (-b / determinant) + yOrigin * (a / determinant) - (a * ty - b * tx) / determinant; + xOrigin = x; + yOrigin = y; + } + + if (smooth || smooth !== false && cache.smooth) { + tx = xOrigin - xOriginOld; + ty = yOrigin - yOriginOld; + cache.xOffset = xOffsetOld + (tx * a + ty * c) - tx; + cache.yOffset = yOffsetOld + (tx * b + ty * d) - ty; + } else { + cache.xOffset = cache.yOffset = 0; + } + + cache.xOrigin = xOrigin; + cache.yOrigin = yOrigin; + cache.smooth = !!smooth; + cache.origin = origin; + cache.originIsAbsolute = !!originIsAbsolute; + target.style[_transformOriginProp] = "0px 0px"; + + if (pluginToAddPropTweensTo) { + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOrigin", xOriginOld, xOrigin); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOrigin", yOriginOld, yOrigin); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOffset", xOffsetOld, cache.xOffset); + + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOffset", yOffsetOld, cache.yOffset); + } + + target.setAttribute("data-svg-origin", xOrigin + " " + yOrigin); + }, + _parseTransform = function _parseTransform(target, uncache) { + var cache = target._gsap || new GSCache(target); + + if ("x" in cache && !uncache && !cache.uncache) { + return cache; + } + + var style = target.style, + invertedScaleX = cache.scaleX < 0, + px = "px", + deg = "deg", + cs = getComputedStyle(target), + origin = _getComputedProperty(target, _transformOriginProp) || "0", + x, + y, + z, + scaleX, + scaleY, + rotation, + rotationX, + rotationY, + skewX, + skewY, + perspective, + xOrigin, + yOrigin, + matrix, + angle, + cos, + sin, + a, + b, + c, + d, + a12, + a22, + t1, + t2, + t3, + a13, + a23, + a33, + a42, + a43, + a32; + x = y = z = rotation = rotationX = rotationY = skewX = skewY = perspective = 0; + scaleX = scaleY = 1; + cache.svg = !!(target.getCTM && _isSVG(target)); + + if (cs.translate) { + if (cs.translate !== "none" || cs.scale !== "none" || cs.rotate !== "none") { + style[_transformProp] = (cs.translate !== "none" ? "translate3d(" + (cs.translate + " 0 0").split(" ").slice(0, 3).join(", ") + ") " : "") + (cs.rotate !== "none" ? "rotate(" + cs.rotate + ") " : "") + (cs.scale !== "none" ? "scale(" + cs.scale.split(" ").join(",") + ") " : "") + (cs[_transformProp] !== "none" ? cs[_transformProp] : ""); + } + + style.scale = style.rotate = style.translate = "none"; + } + + matrix = _getMatrix(target, cache.svg); + + if (cache.svg) { + if (cache.uncache) { + t2 = target.getBBox(); + origin = cache.xOrigin - t2.x + "px " + (cache.yOrigin - t2.y) + "px"; + t1 = ""; + } else { + t1 = !uncache && target.getAttribute("data-svg-origin"); + } + + _applySVGOrigin(target, t1 || origin, !!t1 || cache.originIsAbsolute, cache.smooth !== false, matrix); + } + + xOrigin = cache.xOrigin || 0; + yOrigin = cache.yOrigin || 0; + + if (matrix !== _identity2DMatrix) { + a = matrix[0]; + b = matrix[1]; + c = matrix[2]; + d = matrix[3]; + x = a12 = matrix[4]; + y = a22 = matrix[5]; + + if (matrix.length === 6) { + scaleX = Math.sqrt(a * a + b * b); + scaleY = Math.sqrt(d * d + c * c); + rotation = a || b ? _atan2(b, a) * _RAD2DEG : 0; + skewX = c || d ? _atan2(c, d) * _RAD2DEG + rotation : 0; + skewX && (scaleY *= Math.abs(Math.cos(skewX * _DEG2RAD))); + + if (cache.svg) { + x -= xOrigin - (xOrigin * a + yOrigin * c); + y -= yOrigin - (xOrigin * b + yOrigin * d); + } + } else { + a32 = matrix[6]; + a42 = matrix[7]; + a13 = matrix[8]; + a23 = matrix[9]; + a33 = matrix[10]; + a43 = matrix[11]; + x = matrix[12]; + y = matrix[13]; + z = matrix[14]; + angle = _atan2(a32, a33); + rotationX = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a12 * cos + a13 * sin; + t2 = a22 * cos + a23 * sin; + t3 = a32 * cos + a33 * sin; + a13 = a12 * -sin + a13 * cos; + a23 = a22 * -sin + a23 * cos; + a33 = a32 * -sin + a33 * cos; + a43 = a42 * -sin + a43 * cos; + a12 = t1; + a22 = t2; + a32 = t3; + } + + angle = _atan2(-c, a33); + rotationY = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a * cos - a13 * sin; + t2 = b * cos - a23 * sin; + t3 = c * cos - a33 * sin; + a43 = d * sin + a43 * cos; + a = t1; + b = t2; + c = t3; + } + + angle = _atan2(b, a); + rotation = angle * _RAD2DEG; + + if (angle) { + cos = Math.cos(angle); + sin = Math.sin(angle); + t1 = a * cos + b * sin; + t2 = a12 * cos + a22 * sin; + b = b * cos - a * sin; + a22 = a22 * cos - a12 * sin; + a = t1; + a12 = t2; + } + + if (rotationX && Math.abs(rotationX) + Math.abs(rotation) > 359.9) { + rotationX = rotation = 0; + rotationY = 180 - rotationY; + } + + scaleX = _round(Math.sqrt(a * a + b * b + c * c)); + scaleY = _round(Math.sqrt(a22 * a22 + a32 * a32)); + angle = _atan2(a12, a22); + skewX = Math.abs(angle) > 0.0002 ? angle * _RAD2DEG : 0; + perspective = a43 ? 1 / (a43 < 0 ? -a43 : a43) : 0; + } + + if (cache.svg) { + t1 = target.getAttribute("transform"); + cache.forceCSS = target.setAttribute("transform", "") || !_isNullTransform(_getComputedProperty(target, _transformProp)); + t1 && target.setAttribute("transform", t1); + } + } + + if (Math.abs(skewX) > 90 && Math.abs(skewX) < 270) { + if (invertedScaleX) { + scaleX *= -1; + skewX += rotation <= 0 ? 180 : -180; + rotation += rotation <= 0 ? 180 : -180; + } else { + scaleY *= -1; + skewX += skewX <= 0 ? 180 : -180; + } + } + + uncache = uncache || cache.uncache; + cache.x = x - ((cache.xPercent = x && (!uncache && cache.xPercent || (Math.round(target.offsetWidth / 2) === Math.round(-x) ? -50 : 0))) ? target.offsetWidth * cache.xPercent / 100 : 0) + px; + cache.y = y - ((cache.yPercent = y && (!uncache && cache.yPercent || (Math.round(target.offsetHeight / 2) === Math.round(-y) ? -50 : 0))) ? target.offsetHeight * cache.yPercent / 100 : 0) + px; + cache.z = z + px; + cache.scaleX = _round(scaleX); + cache.scaleY = _round(scaleY); + cache.rotation = _round(rotation) + deg; + cache.rotationX = _round(rotationX) + deg; + cache.rotationY = _round(rotationY) + deg; + cache.skewX = skewX + deg; + cache.skewY = skewY + deg; + cache.transformPerspective = perspective + px; + + if (cache.zOrigin = parseFloat(origin.split(" ")[2]) || !uncache && cache.zOrigin || 0) { + style[_transformOriginProp] = _firstTwoOnly(origin); + } + + cache.xOffset = cache.yOffset = 0; + cache.force3D = _config.force3D; + cache.renderTransform = cache.svg ? _renderSVGTransforms : _supports3D ? _renderCSSTransforms : _renderNon3DTransforms; + cache.uncache = 0; + return cache; + }, + _firstTwoOnly = function _firstTwoOnly(value) { + return (value = value.split(" "))[0] + " " + value[1]; + }, + _addPxTranslate = function _addPxTranslate(target, start, value) { + var unit = getUnit(start); + return _round(parseFloat(start) + parseFloat(_convertToUnit(target, "x", value + "px", unit))) + unit; + }, + _renderNon3DTransforms = function _renderNon3DTransforms(ratio, cache) { + cache.z = "0px"; + cache.rotationY = cache.rotationX = "0deg"; + cache.force3D = 0; + + _renderCSSTransforms(ratio, cache); + }, + _zeroDeg = "0deg", + _zeroPx = "0px", + _endParenthesis = ") ", + _renderCSSTransforms = function _renderCSSTransforms(ratio, cache) { + var _ref = cache || this, + xPercent = _ref.xPercent, + yPercent = _ref.yPercent, + x = _ref.x, + y = _ref.y, + z = _ref.z, + rotation = _ref.rotation, + rotationY = _ref.rotationY, + rotationX = _ref.rotationX, + skewX = _ref.skewX, + skewY = _ref.skewY, + scaleX = _ref.scaleX, + scaleY = _ref.scaleY, + transformPerspective = _ref.transformPerspective, + force3D = _ref.force3D, + target = _ref.target, + zOrigin = _ref.zOrigin, + transforms = "", + use3D = force3D === "auto" && ratio && ratio !== 1 || force3D === true; + + if (zOrigin && (rotationX !== _zeroDeg || rotationY !== _zeroDeg)) { + var angle = parseFloat(rotationY) * _DEG2RAD, + a13 = Math.sin(angle), + a33 = Math.cos(angle), + cos; + + angle = parseFloat(rotationX) * _DEG2RAD; + cos = Math.cos(angle); + x = _addPxTranslate(target, x, a13 * cos * -zOrigin); + y = _addPxTranslate(target, y, -Math.sin(angle) * -zOrigin); + z = _addPxTranslate(target, z, a33 * cos * -zOrigin + zOrigin); + } + + if (transformPerspective !== _zeroPx) { + transforms += "perspective(" + transformPerspective + _endParenthesis; + } + + if (xPercent || yPercent) { + transforms += "translate(" + xPercent + "%, " + yPercent + "%) "; + } + + if (use3D || x !== _zeroPx || y !== _zeroPx || z !== _zeroPx) { + transforms += z !== _zeroPx || use3D ? "translate3d(" + x + ", " + y + ", " + z + ") " : "translate(" + x + ", " + y + _endParenthesis; + } + + if (rotation !== _zeroDeg) { + transforms += "rotate(" + rotation + _endParenthesis; + } + + if (rotationY !== _zeroDeg) { + transforms += "rotateY(" + rotationY + _endParenthesis; + } + + if (rotationX !== _zeroDeg) { + transforms += "rotateX(" + rotationX + _endParenthesis; + } + + if (skewX !== _zeroDeg || skewY !== _zeroDeg) { + transforms += "skew(" + skewX + ", " + skewY + _endParenthesis; + } + + if (scaleX !== 1 || scaleY !== 1) { + transforms += "scale(" + scaleX + ", " + scaleY + _endParenthesis; + } + + target.style[_transformProp] = transforms || "translate(0, 0)"; + }, + _renderSVGTransforms = function _renderSVGTransforms(ratio, cache) { + var _ref2 = cache || this, + xPercent = _ref2.xPercent, + yPercent = _ref2.yPercent, + x = _ref2.x, + y = _ref2.y, + rotation = _ref2.rotation, + skewX = _ref2.skewX, + skewY = _ref2.skewY, + scaleX = _ref2.scaleX, + scaleY = _ref2.scaleY, + target = _ref2.target, + xOrigin = _ref2.xOrigin, + yOrigin = _ref2.yOrigin, + xOffset = _ref2.xOffset, + yOffset = _ref2.yOffset, + forceCSS = _ref2.forceCSS, + tx = parseFloat(x), + ty = parseFloat(y), + a11, + a21, + a12, + a22, + temp; + + rotation = parseFloat(rotation); + skewX = parseFloat(skewX); + skewY = parseFloat(skewY); + + if (skewY) { + skewY = parseFloat(skewY); + skewX += skewY; + rotation += skewY; + } + + if (rotation || skewX) { + rotation *= _DEG2RAD; + skewX *= _DEG2RAD; + a11 = Math.cos(rotation) * scaleX; + a21 = Math.sin(rotation) * scaleX; + a12 = Math.sin(rotation - skewX) * -scaleY; + a22 = Math.cos(rotation - skewX) * scaleY; + + if (skewX) { + skewY *= _DEG2RAD; + temp = Math.tan(skewX - skewY); + temp = Math.sqrt(1 + temp * temp); + a12 *= temp; + a22 *= temp; + + if (skewY) { + temp = Math.tan(skewY); + temp = Math.sqrt(1 + temp * temp); + a11 *= temp; + a21 *= temp; + } + } + + a11 = _round(a11); + a21 = _round(a21); + a12 = _round(a12); + a22 = _round(a22); + } else { + a11 = scaleX; + a22 = scaleY; + a21 = a12 = 0; + } + + if (tx && !~(x + "").indexOf("px") || ty && !~(y + "").indexOf("px")) { + tx = _convertToUnit(target, "x", x, "px"); + ty = _convertToUnit(target, "y", y, "px"); + } + + if (xOrigin || yOrigin || xOffset || yOffset) { + tx = _round(tx + xOrigin - (xOrigin * a11 + yOrigin * a12) + xOffset); + ty = _round(ty + yOrigin - (xOrigin * a21 + yOrigin * a22) + yOffset); + } + + if (xPercent || yPercent) { + temp = target.getBBox(); + tx = _round(tx + xPercent / 100 * temp.width); + ty = _round(ty + yPercent / 100 * temp.height); + } + + temp = "matrix(" + a11 + "," + a21 + "," + a12 + "," + a22 + "," + tx + "," + ty + ")"; + target.setAttribute("transform", temp); + forceCSS && (target.style[_transformProp] = temp); + }, + _addRotationalPropTween = function _addRotationalPropTween(plugin, target, property, startNum, endValue) { + var cap = 360, + isString = _isString(endValue), + endNum = parseFloat(endValue) * (isString && ~endValue.indexOf("rad") ? _RAD2DEG : 1), + change = endNum - startNum, + finalValue = startNum + change + "deg", + direction, + pt; + + if (isString) { + direction = endValue.split("_")[1]; + + if (direction === "short") { + change %= cap; + + if (change !== change % (cap / 2)) { + change += change < 0 ? cap : -cap; + } + } + + if (direction === "cw" && change < 0) { + change = (change + cap * _bigNum$1) % cap - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = (change - cap * _bigNum$1) % cap - ~~(change / cap) * cap; + } + } + + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + pt.u = "deg"; + + plugin._props.push(property); + + return pt; + }, + _assign = function _assign(target, source) { + for (var p in source) { + target[p] = source[p]; + } + + return target; + }, + _addRawTransformPTs = function _addRawTransformPTs(plugin, transforms, target) { + var startCache = _assign({}, target._gsap), + exclude = "perspective,force3D,transformOrigin,svgOrigin", + style = target.style, + endCache, + p, + startValue, + endValue, + startNum, + endNum, + startUnit, + endUnit; + + if (startCache.svg) { + startValue = target.getAttribute("transform"); + target.setAttribute("transform", ""); + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + + _removeProperty(target, _transformProp); + + target.setAttribute("transform", startValue); + } else { + startValue = getComputedStyle(target)[_transformProp]; + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + style[_transformProp] = startValue; + } + + for (p in _transformProps) { + startValue = startCache[p]; + endValue = endCache[p]; + + if (startValue !== endValue && exclude.indexOf(p) < 0) { + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + startNum = startUnit !== endUnit ? _convertToUnit(target, p, startValue, endUnit) : parseFloat(startValue); + endNum = parseFloat(endValue); + plugin._pt = new PropTween(plugin._pt, endCache, p, startNum, endNum - startNum, _renderCSSProp); + plugin._pt.u = endUnit || 0; + + plugin._props.push(p); + } + } + + _assign(endCache, startCache); + }; + + _forEachName("padding,margin,Width,Radius", function (name, index) { + var t = "Top", + r = "Right", + b = "Bottom", + l = "Left", + props = (index < 3 ? [t, r, b, l] : [t + l, t + r, b + r, b + l]).map(function (side) { + return index < 2 ? name + side : "border" + side + name; + }); + + _specialProps[index > 1 ? "border" + name : name] = function (plugin, target, property, endValue, tween) { + var a, vars; + + if (arguments.length < 4) { + a = props.map(function (prop) { + return _get(plugin, prop, property); + }); + vars = a.join(" "); + return vars.split(a[0]).length === 5 ? a[0] : vars; + } + + a = (endValue + "").split(" "); + vars = {}; + props.forEach(function (prop, i) { + return vars[prop] = a[i] = a[i] || a[(i - 1) / 2 | 0]; + }); + plugin.init(target, vars, tween); + }; + }); + + var CSSPlugin = { + name: "css", + register: _initCore, + targetTest: function targetTest(target) { + return target.style && target.nodeType; + }, + init: function init(target, vars, tween, index, targets) { + var props = this._props, + style = target.style, + startAt = tween.vars.startAt, + startValue, + endValue, + endNum, + startNum, + type, + specialProp, + p, + startUnit, + endUnit, + relative, + isTransformRelated, + transformPropTween, + cache, + smooth, + hasPriority, + inlineProps; + _pluginInitted || _initCore(); + this.styles = this.styles || _getStyleSaver(target); + inlineProps = this.styles.props; + this.tween = tween; + + for (p in vars) { + if (p === "autoRound") { + continue; + } + + endValue = vars[p]; + + if (_plugins[p] && _checkPlugin(p, vars, tween, index, target, targets)) { + continue; + } + + type = typeof endValue; + specialProp = _specialProps[p]; + + if (type === "function") { + endValue = endValue.call(tween, index, target, targets); + type = typeof endValue; + } + + if (type === "string" && ~endValue.indexOf("random(")) { + endValue = _replaceRandom(endValue); + } + + if (specialProp) { + specialProp(this, target, p, endValue, tween) && (hasPriority = 1); + } else if (p.substr(0, 2) === "--") { + startValue = (getComputedStyle(target).getPropertyValue(p) + "").trim(); + endValue += ""; + _colorExp.lastIndex = 0; + + if (!_colorExp.test(startValue)) { + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + } + + endUnit ? startUnit !== endUnit && (startValue = _convertToUnit(target, p, startValue, endUnit) + endUnit) : startUnit && (endValue += startUnit); + this.add(style, "setProperty", startValue, endValue, index, targets, 0, 0, p); + props.push(p); + inlineProps.push(p, 0, style[p]); + } else if (type !== "undefined") { + if (startAt && p in startAt) { + startValue = typeof startAt[p] === "function" ? startAt[p].call(tween, index, target, targets) : startAt[p]; + _isString(startValue) && ~startValue.indexOf("random(") && (startValue = _replaceRandom(startValue)); + getUnit(startValue + "") || startValue === "auto" || (startValue += _config.units[p] || getUnit(_get(target, p)) || ""); + (startValue + "").charAt(1) === "=" && (startValue = _get(target, p)); + } else { + startValue = _get(target, p); + } + + startNum = parseFloat(startValue); + relative = type === "string" && endValue.charAt(1) === "=" && endValue.substr(0, 2); + relative && (endValue = endValue.substr(2)); + endNum = parseFloat(endValue); + + if (p in _propertyAliases) { + if (p === "autoAlpha") { + if (startNum === 1 && _get(target, "visibility") === "hidden" && endNum) { + startNum = 0; + } + + inlineProps.push("visibility", 0, style.visibility); + + _addNonTweeningPT(this, style, "visibility", startNum ? "inherit" : "hidden", endNum ? "inherit" : "hidden", !endNum); + } + + if (p !== "scale" && p !== "transform") { + p = _propertyAliases[p]; + ~p.indexOf(",") && (p = p.split(",")[0]); + } + } + + isTransformRelated = p in _transformProps; + + if (isTransformRelated) { + this.styles.save(p); + + if (!transformPropTween) { + cache = target._gsap; + cache.renderTransform && !vars.parseTransform || _parseTransform(target, vars.parseTransform); + smooth = vars.smoothOrigin !== false && cache.smooth; + transformPropTween = this._pt = new PropTween(this._pt, style, _transformProp, 0, 1, cache.renderTransform, cache, 0, -1); + transformPropTween.dep = 1; + } + + if (p === "scale") { + this._pt = new PropTween(this._pt, cache, "scaleY", cache.scaleY, (relative ? _parseRelative(cache.scaleY, relative + endNum) : endNum) - cache.scaleY || 0, _renderCSSProp); + this._pt.u = 0; + props.push("scaleY", p); + p += "X"; + } else if (p === "transformOrigin") { + inlineProps.push(_transformOriginProp, 0, style[_transformOriginProp]); + endValue = _convertKeywordsToPercentages(endValue); + + if (cache.svg) { + _applySVGOrigin(target, endValue, 0, smooth, 0, this); + } else { + endUnit = parseFloat(endValue.split(" ")[2]) || 0; + endUnit !== cache.zOrigin && _addNonTweeningPT(this, cache, "zOrigin", cache.zOrigin, endUnit); + + _addNonTweeningPT(this, style, p, _firstTwoOnly(startValue), _firstTwoOnly(endValue)); + } + + continue; + } else if (p === "svgOrigin") { + _applySVGOrigin(target, endValue, 1, smooth, 0, this); + + continue; + } else if (p in _rotationalProperties) { + _addRotationalPropTween(this, cache, p, startNum, relative ? _parseRelative(startNum, relative + endValue) : endValue); + + continue; + } else if (p === "smoothOrigin") { + _addNonTweeningPT(this, cache, "smooth", cache.smooth, endValue); + + continue; + } else if (p === "force3D") { + cache[p] = endValue; + continue; + } else if (p === "transform") { + _addRawTransformPTs(this, endValue, target); + + continue; + } + } else if (!(p in style)) { + p = _checkPropPrefix(p) || p; + } + + if (isTransformRelated || (endNum || endNum === 0) && (startNum || startNum === 0) && !_complexExp.test(endValue) && p in style) { + startUnit = (startValue + "").substr((startNum + "").length); + endNum || (endNum = 0); + endUnit = getUnit(endValue) || (p in _config.units ? _config.units[p] : startUnit); + startUnit !== endUnit && (startNum = _convertToUnit(target, p, startValue, endUnit)); + this._pt = new PropTween(this._pt, isTransformRelated ? cache : style, p, startNum, (relative ? _parseRelative(startNum, relative + endNum) : endNum) - startNum, !isTransformRelated && (endUnit === "px" || p === "zIndex") && vars.autoRound !== false ? _renderRoundedCSSProp : _renderCSSProp); + this._pt.u = endUnit || 0; + + if (startUnit !== endUnit && endUnit !== "%") { + this._pt.b = startValue; + this._pt.r = _renderCSSPropWithBeginning; + } + } else if (!(p in style)) { + if (p in target) { + this.add(target, p, startValue || target[p], relative ? relative + endValue : endValue, index, targets); + } else if (p !== "parseTransform") { + _missingPlugin(p, endValue); + + continue; + } + } else { + _tweenComplexCSSString.call(this, target, p, startValue, relative ? relative + endValue : endValue); + } + + isTransformRelated || (p in style ? inlineProps.push(p, 0, style[p]) : typeof target[p] === "function" ? inlineProps.push(p, 2, target[p]()) : inlineProps.push(p, 1, startValue || target[p])); + props.push(p); + } + } + + hasPriority && _sortPropTweensByPriority(this); + }, + render: function render(ratio, data) { + if (data.tween._time || !_reverting$1()) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + } else { + data.styles.revert(); + } + }, + get: _get, + aliases: _propertyAliases, + getSetter: function getSetter(target, property, plugin) { + var p = _propertyAliases[property]; + p && p.indexOf(",") < 0 && (property = p); + return property in _transformProps && property !== _transformOriginProp && (target._gsap.x || _get(target, "x")) ? plugin && _recentSetterPlugin === plugin ? property === "scale" ? _setterScale : _setterTransform : (_recentSetterPlugin = plugin || {}) && (property === "scale" ? _setterScaleWithRender : _setterTransformWithRender) : target.style && !_isUndefined(target.style[property]) ? _setterCSSStyle : ~property.indexOf("-") ? _setterCSSProp : _getSetter(target, property); + }, + core: { + _removeProperty: _removeProperty, + _getMatrix: _getMatrix + } + }; + gsap.utils.checkPrefix = _checkPropPrefix; + gsap.core.getStyleSaver = _getStyleSaver; + + (function (positionAndScale, rotation, others, aliases) { + var all = _forEachName(positionAndScale + "," + rotation + "," + others, function (name) { + _transformProps[name] = 1; + }); + + _forEachName(rotation, function (name) { + _config.units[name] = "deg"; + _rotationalProperties[name] = 1; + }); + + _propertyAliases[all[13]] = positionAndScale + "," + rotation; + + _forEachName(aliases, function (name) { + var split = name.split(":"); + _propertyAliases[split[1]] = all[split[0]]; + }); + })("x,y,z,scale,scaleX,scaleY,xPercent,yPercent", "rotation,rotationX,rotationY,skewX,skewY", "transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective", "0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY"); + + _forEachName("x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective", function (name) { + _config.units[name] = "px"; + }); + + gsap.registerPlugin(CSSPlugin); + + var gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, + TweenMaxWithCSS = gsapWithCSS.core.Tween; + + exports.Back = Back; + exports.Bounce = Bounce; + exports.CSSPlugin = CSSPlugin; + exports.Circ = Circ; + exports.Cubic = Cubic; + exports.Elastic = Elastic; + exports.Expo = Expo; + exports.Linear = Linear; + exports.Power0 = Power0; + exports.Power1 = Power1; + exports.Power2 = Power2; + exports.Power3 = Power3; + exports.Power4 = Power4; + exports.Quad = Quad; + exports.Quart = Quart; + exports.Quint = Quint; + exports.Sine = Sine; + exports.SteppedEase = SteppedEase; + exports.Strong = Strong; + exports.TimelineLite = Timeline; + exports.TimelineMax = Timeline; + exports.TweenLite = Tween; + exports.TweenMax = TweenMaxWithCSS; + exports.default = gsapWithCSS; + exports.gsap = gsapWithCSS; + + if (typeof(window) === 'undefined' || window !== exports) {Object.defineProperty(exports, '__esModule', { value: true });} else {delete window.default;} + +}))); diff --git a/node_modules/gsap/dist/gsap.min.js b/node_modules/gsap/dist/gsap.min.js new file mode 100644 index 0000000000000000000000000000000000000000..c0f9bfa86aa4382bb4f20fca99c2faee31ca5954 --- /dev/null +++ b/node_modules/gsap/dist/gsap.min.js @@ -0,0 +1,11 @@ +/*! + * GSAP 3.12.7 + * https://gsap.com + * + * @license Copyright 2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ + +!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?e(exports):"function"==typeof define&&define.amd?define(["exports"],e):e((t=t||self).window=t.window||{})}(this,function(e){"use strict";function _inheritsLoose(t,e){t.prototype=Object.create(e.prototype),(t.prototype.constructor=t).__proto__=e}function _assertThisInitialized(t){if(void 0===t)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return t}function r(t){return"string"==typeof t}function s(t){return"function"==typeof t}function t(t){return"number"==typeof t}function u(t){return void 0===t}function v(t){return"object"==typeof t}function w(t){return!1!==t}function x(){return"undefined"!=typeof window}function y(t){return s(t)||r(t)}function P(t){return(i=yt(t,ot))&&ze}function Q(t,e){return console.warn("Invalid property",t,"set to",e,"Missing plugin? gsap.registerPlugin()")}function R(t,e){return!e&&console.warn(t)}function S(t,e){return t&&(ot[t]=e)&&i&&(i[t]=e)||ot}function T(){return 0}function ea(t){var e,r,i=t[0];if(v(i)||s(i)||(t=[t]),!(e=(i._gsap||{}).harness)){for(r=gt.length;r--&&!gt[r].targetTest(i););e=gt[r]}for(r=t.length;r--;)t[r]&&(t[r]._gsap||(t[r]._gsap=new Vt(t[r],e)))||t.splice(r,1);return t}function fa(t){return t._gsap||ea(Mt(t))[0]._gsap}function ga(t,e,r){return(r=t[e])&&s(r)?t[e]():u(r)&&t.getAttribute&&t.getAttribute(e)||r}function ha(t,e){return(t=t.split(",")).forEach(e)||t}function ia(t){return Math.round(1e5*t)/1e5||0}function ja(t){return Math.round(1e7*t)/1e7||0}function ka(t,e){var r=e.charAt(0),i=parseFloat(e.substr(2));return t=parseFloat(t),"+"===r?t+i:"-"===r?t-i:"*"===r?t*i:t/i}function la(t,e){for(var r=e.length,i=0;t.indexOf(e[i])<0&&++i<r;);return i<r}function ma(){var t,e,r=dt.length,i=dt.slice(0);for(ct={},t=dt.length=0;t<r;t++)(e=i[t])&&e._lazy&&(e.render(e._lazy[0],e._lazy[1],!0)._lazy=0)}function na(t,e,r,i){dt.length&&!L&&ma(),t.render(e,r,i||L&&e<0&&(t._initted||t._startAt)),dt.length&&!L&&ma()}function oa(t){var e=parseFloat(t);return(e||0===e)&&(t+"").match(at).length<2?e:r(t)?t.trim():t}function pa(t){return t}function qa(t,e){for(var r in e)r in t||(t[r]=e[r]);return t}function ta(t,e){for(var r in e)"__proto__"!==r&&"constructor"!==r&&"prototype"!==r&&(t[r]=v(e[r])?ta(t[r]||(t[r]={}),e[r]):e[r]);return t}function ua(t,e){var r,i={};for(r in t)r in e||(i[r]=t[r]);return i}function va(t){var e=t.parent||I,r=t.keyframes?function _setKeyframeDefaults(i){return function(t,e){for(var r in e)r in t||"duration"===r&&i||"ease"===r||(t[r]=e[r])}}(Z(t.keyframes)):qa;if(w(t.inherit))for(;e;)r(t,e.vars.defaults),e=e.parent||e._dp;return t}function xa(t,e,r,i,n){void 0===r&&(r="_first"),void 0===i&&(i="_last");var a,s=t[i];if(n)for(a=e[n];s&&s[n]>a;)s=s._prev;return s?(e._next=s._next,s._next=e):(e._next=t[r],t[r]=e),e._next?e._next._prev=e:t[i]=e,e._prev=s,e.parent=e._dp=t,e}function ya(t,e,r,i){void 0===r&&(r="_first"),void 0===i&&(i="_last");var n=e._prev,a=e._next;n?n._next=a:t[r]===e&&(t[r]=a),a?a._prev=n:t[i]===e&&(t[i]=n),e._next=e._prev=e.parent=null}function za(t,e){t.parent&&(!e||t.parent.autoRemoveChildren)&&t.parent.remove&&t.parent.remove(t),t._act=0}function Aa(t,e){if(t&&(!e||e._end>t._dur||e._start<0))for(var r=t;r;)r._dirty=1,r=r.parent;return t}function Ca(t,e,r,i){return t._startAt&&(L?t._startAt.revert(ht):t.vars.immediateRender&&!t.vars.autoRevert||t._startAt.render(e,!0,i))}function Ea(t){return t._repeat?Tt(t._tTime,t=t.duration()+t._rDelay)*t:0}function Ga(t,e){return(t-e._start)*e._ts+(0<=e._ts?0:e._dirty?e.totalDuration():e._tDur)}function Ha(t){return t._end=ja(t._start+(t._tDur/Math.abs(t._ts||t._rts||X)||0))}function Ia(t,e){var r=t._dp;return r&&r.smoothChildTiming&&t._ts&&(t._start=ja(r._time-(0<t._ts?e/t._ts:((t._dirty?t.totalDuration():t._tDur)-e)/-t._ts)),Ha(t),r._dirty||Aa(r,t)),t}function Ja(t,e){var r;if((e._time||!e._dur&&e._initted||e._start<t._time&&(e._dur||!e.add))&&(r=Ga(t.rawTime(),e),(!e._dur||kt(0,e.totalDuration(),r)-e._tTime>X)&&e.render(r,!0)),Aa(t,e)._dp&&t._initted&&t._time>=t._dur&&t._ts){if(t._dur<t.duration())for(r=t;r._dp;)0<=r.rawTime()&&r.totalTime(r._tTime),r=r._dp;t._zTime=-X}}function Ka(e,r,i,n){return r.parent&&za(r),r._start=ja((t(i)?i:i||e!==I?xt(e,i,r):e._time)+r._delay),r._end=ja(r._start+(r.totalDuration()/Math.abs(r.timeScale())||0)),xa(e,r,"_first","_last",e._sort?"_start":0),bt(r)||(e._recent=r),n||Ja(e,r),e._ts<0&&Ia(e,e._tTime),e}function La(t,e){return(ot.ScrollTrigger||Q("scrollTrigger",e))&&ot.ScrollTrigger.create(e,t)}function Ma(t,e,r,i,n){return Qt(t,e,n),t._initted?!r&&t._pt&&!L&&(t._dur&&!1!==t.vars.lazy||!t._dur&&t.vars.lazy)&&f!==Rt.frame?(dt.push(t),t._lazy=[n,i],1):void 0:1}function Ra(t,e,r,i){var n=t._repeat,a=ja(e)||0,s=t._tTime/t._tDur;return s&&!i&&(t._time*=a/t._dur),t._dur=a,t._tDur=n?n<0?1e10:ja(a*(n+1)+t._rDelay*n):a,0<s&&!i&&Ia(t,t._tTime=t._tDur*s),t.parent&&Ha(t),r||Aa(t.parent,t),t}function Sa(t){return t instanceof Xt?Aa(t):Ra(t,t._dur)}function Va(e,r,i){var n,a,s=t(r[1]),o=(s?2:1)+(e<2?0:1),u=r[o];if(s&&(u.duration=r[1]),u.parent=i,e){for(n=u,a=i;a&&!("immediateRender"in n);)n=a.vars.defaults||{},a=w(a.vars.inherit)&&a.parent;u.immediateRender=w(n.immediateRender),e<2?u.runBackwards=1:u.startAt=r[o-1]}return new $t(r[0],u,r[1+o])}function Wa(t,e){return t||0===t?e(t):e}function Ya(t,e){return r(t)&&(e=st.exec(t))?e[1]:""}function _a(t,e){return t&&v(t)&&"length"in t&&(!e&&!t.length||t.length-1 in t&&v(t[0]))&&!t.nodeType&&t!==h}function cb(r){return r=Mt(r)[0]||R("Invalid scope")||{},function(t){var e=r.current||r.nativeElement||r;return Mt(t,e.querySelectorAll?e:e===r?R("Invalid scope")||a.createElement("div"):r)}}function db(t){return t.sort(function(){return.5-Math.random()})}function eb(t){if(s(t))return t;var p=v(t)?t:{each:t},_=jt(p.ease),m=p.from||0,g=parseFloat(p.base)||0,y={},e=0<m&&m<1,T=isNaN(m)||e,b=p.axis,w=m,x=m;return r(m)?w=x={center:.5,edges:.5,end:1}[m]||0:!e&&T&&(w=m[0],x=m[1]),function(t,e,r){var i,n,a,s,o,u,h,l,f,d=(r||p).length,c=y[d];if(!c){if(!(f="auto"===p.grid?0:(p.grid||[1,U])[1])){for(h=-U;h<(h=r[f++].getBoundingClientRect().left)&&f<d;);f<d&&f--}for(c=y[d]=[],i=T?Math.min(f,d)*w-.5:m%f,n=f===U?0:T?d*x/f-.5:m/f|0,l=U,u=h=0;u<d;u++)a=u%f-i,s=n-(u/f|0),c[u]=o=b?Math.abs("y"===b?s:a):K(a*a+s*s),h<o&&(h=o),o<l&&(l=o);"random"===m&&db(c),c.max=h-l,c.min=l,c.v=d=(parseFloat(p.amount)||parseFloat(p.each)*(d<f?d-1:b?"y"===b?d/f:f:Math.max(f,d/f))||0)*("edges"===m?-1:1),c.b=d<0?g-d:g,c.u=Ya(p.amount||p.each)||0,_=_&&d<0?Bt(_):_}return d=(c[t]-c.min)/c.max||0,ja(c.b+(_?_(d):d)*c.v)+c.u}}function fb(i){var n=Math.pow(10,((i+"").split(".")[1]||"").length);return function(e){var r=ja(Math.round(parseFloat(e)/i)*i*n);return(r-r%1)/n+(t(e)?0:Ya(e))}}function gb(h,e){var l,f,r=Z(h);return!r&&v(h)&&(l=r=h.radius||U,h.values?(h=Mt(h.values),(f=!t(h[0]))&&(l*=l)):h=fb(h.increment)),Wa(e,r?s(h)?function(t){return f=h(t),Math.abs(f-t)<=l?f:t}:function(e){for(var r,i,n=parseFloat(f?e.x:e),a=parseFloat(f?e.y:0),s=U,o=0,u=h.length;u--;)(r=f?(r=h[u].x-n)*r+(i=h[u].y-a)*i:Math.abs(h[u]-n))<s&&(s=r,o=u);return o=!l||s<=l?h[o]:e,f||o===e||t(e)?o:o+Ya(e)}:fb(h))}function hb(t,e,r,i){return Wa(Z(t)?!e:!0===r?!!(r=0):!i,function(){return Z(t)?t[~~(Math.random()*t.length)]:(r=r||1e-5)&&(i=r<1?Math.pow(10,(r+"").length-2):1)&&Math.floor(Math.round((t-r/2+Math.random()*(e-t+.99*r))/r)*r*i)/i})}function lb(e,r,t){return Wa(t,function(t){return e[~~r(t)]})}function ob(t){for(var e,r,i,n,a=0,s="";~(e=t.indexOf("random(",a));)i=t.indexOf(")",e),n="["===t.charAt(e+7),r=t.substr(e+7,i-e-7).match(n?at:tt),s+=t.substr(a,e-a)+hb(n?r:+r[0],n?0:+r[1],+r[2]||1e-5),a=i+1;return s+t.substr(a,t.length-a)}function rb(t,e,r){var i,n,a,s=t.labels,o=U;for(i in s)(n=s[i]-e)<0==!!r&&n&&o>(n=Math.abs(n))&&(a=i,o=n);return a}function tb(t){return za(t),t.scrollTrigger&&t.scrollTrigger.kill(!!L),t.progress()<1&&Ct(t,"onInterrupt"),t}function wb(t){if(t)if(t=!t.name&&t.default||t,x()||t.headless){var e=t.name,r=s(t),i=e&&!r&&t.init?function(){this._props=[]}:t,n={init:T,render:he,add:Wt,kill:ce,modifier:fe,rawVars:0},a={targetTest:0,get:0,getSetter:ne,aliases:{},register:0};if(Ft(),t!==i){if(pt[e])return;qa(i,qa(ua(t,n),a)),yt(i.prototype,yt(n,ua(t,a))),pt[i.prop=e]=i,t.targetTest&&(gt.push(i),ft[e]=1),e=("css"===e?"CSS":e.charAt(0).toUpperCase()+e.substr(1))+"Plugin"}S(e,i),t.register&&t.register(ze,i,_e)}else At.push(t)}function zb(t,e,r){return(6*(t+=t<0?1:1<t?-1:0)<1?e+(r-e)*t*6:t<.5?r:3*t<2?e+(r-e)*(2/3-t)*6:e)*St+.5|0}function Ab(e,r,i){var n,a,s,o,u,h,l,f,d,c,p=e?t(e)?[e>>16,e>>8&St,e&St]:0:zt.black;if(!p){if(","===e.substr(-1)&&(e=e.substr(0,e.length-1)),zt[e])p=zt[e];else if("#"===e.charAt(0)){if(e.length<6&&(e="#"+(n=e.charAt(1))+n+(a=e.charAt(2))+a+(s=e.charAt(3))+s+(5===e.length?e.charAt(4)+e.charAt(4):"")),9===e.length)return[(p=parseInt(e.substr(1,6),16))>>16,p>>8&St,p&St,parseInt(e.substr(7),16)/255];p=[(e=parseInt(e.substr(1),16))>>16,e>>8&St,e&St]}else if("hsl"===e.substr(0,3))if(p=c=e.match(tt),r){if(~e.indexOf("="))return p=e.match(et),i&&p.length<4&&(p[3]=1),p}else o=+p[0]%360/360,u=p[1]/100,n=2*(h=p[2]/100)-(a=h<=.5?h*(u+1):h+u-h*u),3<p.length&&(p[3]*=1),p[0]=zb(o+1/3,n,a),p[1]=zb(o,n,a),p[2]=zb(o-1/3,n,a);else p=e.match(tt)||zt.transparent;p=p.map(Number)}return r&&!c&&(n=p[0]/St,a=p[1]/St,s=p[2]/St,h=((l=Math.max(n,a,s))+(f=Math.min(n,a,s)))/2,l===f?o=u=0:(d=l-f,u=.5<h?d/(2-l-f):d/(l+f),o=l===n?(a-s)/d+(a<s?6:0):l===a?(s-n)/d+2:(n-a)/d+4,o*=60),p[0]=~~(o+.5),p[1]=~~(100*u+.5),p[2]=~~(100*h+.5)),i&&p.length<4&&(p[3]=1),p}function Bb(t){var r=[],i=[],n=-1;return t.split(Et).forEach(function(t){var e=t.match(rt)||[];r.push.apply(r,e),i.push(n+=e.length+1)}),r.c=i,r}function Cb(t,e,r){var i,n,a,s,o="",u=(t+o).match(Et),h=e?"hsla(":"rgba(",l=0;if(!u)return t;if(u=u.map(function(t){return(t=Ab(t,e,1))&&h+(e?t[0]+","+t[1]+"%,"+t[2]+"%,"+t[3]:t.join(","))+")"}),r&&(a=Bb(t),(i=r.c).join(o)!==a.c.join(o)))for(s=(n=t.replace(Et,"1").split(rt)).length-1;l<s;l++)o+=n[l]+(~i.indexOf(l)?u.shift()||h+"0,0,0,0)":(a.length?a:u.length?u:r).shift());if(!n)for(s=(n=t.split(Et)).length-1;l<s;l++)o+=n[l]+u[l];return o+n[s]}function Fb(t){var e,r=t.join(" ");if(Et.lastIndex=0,Et.test(r))return e=Dt.test(r),t[1]=Cb(t[1],e),t[0]=Cb(t[0],e,Bb(t[1])),!0}function Ob(t){var e=(t+"").split("("),r=Lt[e[0]];return r&&1<e.length&&r.config?r.config.apply(null,~t.indexOf("{")?[function _parseObjectInString(t){for(var e,r,i,n={},a=t.substr(1,t.length-3).split(":"),s=a[0],o=1,u=a.length;o<u;o++)r=a[o],e=o!==u-1?r.lastIndexOf(","):r.length,i=r.substr(0,e),n[s]=isNaN(i)?i.replace(Yt,"").trim():+i,s=r.substr(e+1).trim();return n}(e[1])]:function _valueInParentheses(t){var e=t.indexOf("(")+1,r=t.indexOf(")"),i=t.indexOf("(",e);return t.substring(e,~i&&i<r?t.indexOf(")",r+1):r)}(t).split(",").map(oa)):Lt._CE&&It.test(t)?Lt._CE("",t):r}function Qb(t,e){for(var r,i=t._first;i;)i instanceof Xt?Qb(i,e):!i.vars.yoyoEase||i._yoyo&&i._repeat||i._yoyo===e||(i.timeline?Qb(i.timeline,e):(r=i._ease,i._ease=i._yEase,i._yEase=r,i._yoyo=e)),i=i._next}function Sb(t,e,r,i){void 0===r&&(r=function easeOut(t){return 1-e(1-t)}),void 0===i&&(i=function easeInOut(t){return t<.5?e(2*t)/2:1-e(2*(1-t))/2});var n,a={easeIn:e,easeOut:r,easeInOut:i};return ha(t,function(t){for(var e in Lt[t]=ot[t]=a,Lt[n=t.toLowerCase()]=r,a)Lt[n+("easeIn"===e?".in":"easeOut"===e?".out":".inOut")]=Lt[t+"."+e]=a[e]}),a}function Tb(e){return function(t){return t<.5?(1-e(1-2*t))/2:.5+e(2*(t-.5))/2}}function Ub(r,t,e){function Jm(t){return 1===t?1:i*Math.pow(2,-10*t)*H((t-a)*n)+1}var i=1<=t?t:1,n=(e||(r?.3:.45))/(t<1?t:1),a=n/N*(Math.asin(1/i)||0),s="out"===r?Jm:"in"===r?function(t){return 1-Jm(1-t)}:Tb(Jm);return n=N/n,s.config=function(t,e){return Ub(r,t,e)},s}function Vb(e,r){function Rm(t){return t?--t*t*((r+1)*t+r)+1:0}void 0===r&&(r=1.70158);var t="out"===e?Rm:"in"===e?function(t){return 1-Rm(1-t)}:Tb(Rm);return t.config=function(t){return Vb(e,t)},t}var F,L,l,I,h,n,a,i,o,f,d,c,p,_,m,g,b,k,O,M,C,A,z,E,D,Y,B,j,q={autoSleep:120,force3D:"auto",nullTargetWarn:1,units:{lineHeight:""}},V={duration:.5,overwrite:!1,delay:0},U=1e8,X=1/U,N=2*Math.PI,G=N/4,W=0,K=Math.sqrt,J=Math.cos,H=Math.sin,$="function"==typeof ArrayBuffer&&ArrayBuffer.isView||function(){},Z=Array.isArray,tt=/(?:-?\.?\d|\.)+/gi,et=/[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/g,rt=/[-+=.]*\d+[.e-]*\d*[a-z%]*/g,it=/[-+=.]*\d+\.?\d*(?:e-|e\+)?\d*/gi,nt=/[+-]=-?[.\d]+/,at=/[^,'"\[\]\s]+/gi,st=/^[+\-=e\s\d]*\d+[.\d]*([a-z]*|%)\s*$/i,ot={},ut={suppressEvents:!0,isStart:!0,kill:!1},ht={suppressEvents:!0,kill:!1},lt={suppressEvents:!0},ft={},dt=[],ct={},pt={},_t={},mt=30,gt=[],vt="",yt=function _merge(t,e){for(var r in e)t[r]=e[r];return t},Tt=function _animationCycle(t,e){var r=Math.floor(t=ja(t/e));return t&&r===t?r-1:r},bt=function _isFromOrFromStart(t){var e=t.data;return"isFromStart"===e||"isStart"===e},wt={_start:0,endTime:T,totalDuration:T},xt=function _parsePosition(t,e,i){var n,a,s,o=t.labels,u=t._recent||wt,h=t.duration()>=U?u.endTime(!1):t._dur;return r(e)&&(isNaN(e)||e in o)?(a=e.charAt(0),s="%"===e.substr(-1),n=e.indexOf("="),"<"===a||">"===a?(0<=n&&(e=e.replace(/=/,"")),("<"===a?u._start:u.endTime(0<=u._repeat))+(parseFloat(e.substr(1))||0)*(s?(n<0?u:i).totalDuration()/100:1)):n<0?(e in o||(o[e]=h),o[e]):(a=parseFloat(e.charAt(n-1)+e.substr(n+1)),s&&i&&(a=a/100*(Z(i)?i[0]:i).totalDuration()),1<n?_parsePosition(t,e.substr(0,n-1),i)+a:h+a)):null==e?h:+e},kt=function _clamp(t,e,r){return r<t?t:e<r?e:r},Ot=[].slice,Mt=function toArray(t,e,i){return l&&!e&&l.selector?l.selector(t):!r(t)||i||!n&&Ft()?Z(t)?function _flatten(t,e,i){return void 0===i&&(i=[]),t.forEach(function(t){return r(t)&&!e||_a(t,1)?i.push.apply(i,Mt(t)):i.push(t)})||i}(t,i):_a(t)?Ot.call(t,0):t?[t]:[]:Ot.call((e||a).querySelectorAll(t),0)},Pt=function mapRange(e,t,r,i,n){var a=t-e,s=i-r;return Wa(n,function(t){return r+((t-e)/a*s||0)})},Ct=function _callback(t,e,r){var i,n,a,s=t.vars,o=s[e],u=l,h=t._ctx;if(o)return i=s[e+"Params"],n=s.callbackScope||t,r&&dt.length&&ma(),h&&(l=h),a=i?o.apply(n,i):o.call(n),l=u,a},At=[],St=255,zt={aqua:[0,St,St],lime:[0,St,0],silver:[192,192,192],black:[0,0,0],maroon:[128,0,0],teal:[0,128,128],blue:[0,0,St],navy:[0,0,128],white:[St,St,St],olive:[128,128,0],yellow:[St,St,0],orange:[St,165,0],gray:[128,128,128],purple:[128,0,128],green:[0,128,0],red:[St,0,0],pink:[St,192,203],cyan:[0,St,St],transparent:[St,St,St,0]},Et=function(){var t,e="(?:\\b(?:(?:rgb|rgba|hsl|hsla)\\(.+?\\))|\\B#(?:[0-9a-f]{3,4}){1,2}\\b";for(t in zt)e+="|"+t+"\\b";return new RegExp(e+")","gi")}(),Dt=/hsl[a]?\(/,Rt=(O=Date.now,M=500,C=33,A=O(),z=A,D=E=1e3/240,g={time:0,frame:0,tick:function tick(){yl(!0)},deltaRatio:function deltaRatio(t){return b/(1e3/(t||60))},wake:function wake(){o&&(!n&&x()&&(h=n=window,a=h.document||{},ot.gsap=ze,(h.gsapVersions||(h.gsapVersions=[])).push(ze.version),P(i||h.GreenSockGlobals||!h.gsap&&h||{}),At.forEach(wb)),m="undefined"!=typeof requestAnimationFrame&&requestAnimationFrame,p&&g.sleep(),_=m||function(t){return setTimeout(t,D-1e3*g.time+1|0)},c=1,yl(2))},sleep:function sleep(){(m?cancelAnimationFrame:clearTimeout)(p),c=0,_=T},lagSmoothing:function lagSmoothing(t,e){M=t||1/0,C=Math.min(e||33,M)},fps:function fps(t){E=1e3/(t||240),D=1e3*g.time+E},add:function add(n,t,e){var a=t?function(t,e,r,i){n(t,e,r,i),g.remove(a)}:n;return g.remove(n),Y[e?"unshift":"push"](a),Ft(),a},remove:function remove(t,e){~(e=Y.indexOf(t))&&Y.splice(e,1)&&e<=k&&k--},_listeners:Y=[]}),Ft=function _wake(){return!c&&Rt.wake()},Lt={},It=/^[\d.\-M][\d.\-,\s]/,Yt=/["']/g,Bt=function _invertEase(e){return function(t){return 1-e(1-t)}},jt=function _parseEase(t,e){return t&&(s(t)?t:Lt[t]||Ob(t))||e};function yl(t){var e,r,i,n,a=O()-z,s=!0===t;if((M<a||a<0)&&(A+=a-C),(0<(e=(i=(z+=a)-A)-D)||s)&&(n=++g.frame,b=i-1e3*g.time,g.time=i/=1e3,D+=e+(E<=e?4:E-e),r=1),s||(p=_(yl)),r)for(k=0;k<Y.length;k++)Y[k](i,b,n,t)}function gn(t){return t<j?B*t*t:t<.7272727272727273?B*Math.pow(t-1.5/2.75,2)+.75:t<.9090909090909092?B*(t-=2.25/2.75)*t+.9375:B*Math.pow(t-2.625/2.75,2)+.984375}ha("Linear,Quad,Cubic,Quart,Quint,Strong",function(t,e){var r=e<5?e+1:e;Sb(t+",Power"+(r-1),e?function(t){return Math.pow(t,r)}:function(t){return t},function(t){return 1-Math.pow(1-t,r)},function(t){return t<.5?Math.pow(2*t,r)/2:1-Math.pow(2*(1-t),r)/2})}),Lt.Linear.easeNone=Lt.none=Lt.Linear.easeIn,Sb("Elastic",Ub("in"),Ub("out"),Ub()),B=7.5625,j=1/2.75,Sb("Bounce",function(t){return 1-gn(1-t)},gn),Sb("Expo",function(t){return Math.pow(2,10*(t-1))*t+t*t*t*t*t*t*(1-t)}),Sb("Circ",function(t){return-(K(1-t*t)-1)}),Sb("Sine",function(t){return 1===t?1:1-J(t*G)}),Sb("Back",Vb("in"),Vb("out"),Vb()),Lt.SteppedEase=Lt.steps=ot.SteppedEase={config:function config(t,e){void 0===t&&(t=1);var r=1/t,i=t+(e?0:1),n=e?1:0;return function(t){return((i*kt(0,.99999999,t)|0)+n)*r}}},V.ease=Lt["quad.out"],ha("onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt",function(t){return vt+=t+","+t+"Params,"});var qt,Vt=function GSCache(t,e){this.id=W++,(t._gsap=this).target=t,this.harness=e,this.get=e?e.get:ga,this.set=e?e.getSetter:ne},Ut=((qt=Animation.prototype).delay=function delay(t){return t||0===t?(this.parent&&this.parent.smoothChildTiming&&this.startTime(this._start+t-this._delay),this._delay=t,this):this._delay},qt.duration=function duration(t){return arguments.length?this.totalDuration(0<this._repeat?t+(t+this._rDelay)*this._repeat:t):this.totalDuration()&&this._dur},qt.totalDuration=function totalDuration(t){return arguments.length?(this._dirty=0,Ra(this,this._repeat<0?t:(t-this._repeat*this._rDelay)/(this._repeat+1))):this._tDur},qt.totalTime=function totalTime(t,e){if(Ft(),!arguments.length)return this._tTime;var r=this._dp;if(r&&r.smoothChildTiming&&this._ts){for(Ia(this,t),!r._dp||r.parent||Ja(r,this);r&&r.parent;)r.parent._time!==r._start+(0<=r._ts?r._tTime/r._ts:(r.totalDuration()-r._tTime)/-r._ts)&&r.totalTime(r._tTime,!0),r=r.parent;!this.parent&&this._dp.autoRemoveChildren&&(0<this._ts&&t<this._tDur||this._ts<0&&0<t||!this._tDur&&!t)&&Ka(this._dp,this,this._start-this._delay)}return(this._tTime!==t||!this._dur&&!e||this._initted&&Math.abs(this._zTime)===X||!t&&!this._initted&&(this.add||this._ptLookup))&&(this._ts||(this._pTime=t),na(this,t,e)),this},qt.time=function time(t,e){return arguments.length?this.totalTime(Math.min(this.totalDuration(),t+Ea(this))%(this._dur+this._rDelay)||(t?this._dur:0),e):this._time},qt.totalProgress=function totalProgress(t,e){return arguments.length?this.totalTime(this.totalDuration()*t,e):this.totalDuration()?Math.min(1,this._tTime/this._tDur):0<=this.rawTime()&&this._initted?1:0},qt.progress=function progress(t,e){return arguments.length?this.totalTime(this.duration()*(!this._yoyo||1&this.iteration()?t:1-t)+Ea(this),e):this.duration()?Math.min(1,this._time/this._dur):0<this.rawTime()?1:0},qt.iteration=function iteration(t,e){var r=this.duration()+this._rDelay;return arguments.length?this.totalTime(this._time+(t-1)*r,e):this._repeat?Tt(this._tTime,r)+1:1},qt.timeScale=function timeScale(t,e){if(!arguments.length)return this._rts===-X?0:this._rts;if(this._rts===t)return this;var r=this.parent&&this._ts?Ga(this.parent._time,this):this._tTime;return this._rts=+t||0,this._ts=this._ps||t===-X?0:this._rts,this.totalTime(kt(-Math.abs(this._delay),this._tDur,r),!1!==e),Ha(this),function _recacheAncestors(t){for(var e=t.parent;e&&e.parent;)e._dirty=1,e.totalDuration(),e=e.parent;return t}(this)},qt.paused=function paused(t){return arguments.length?(this._ps!==t&&((this._ps=t)?(this._pTime=this._tTime||Math.max(-this._delay,this.rawTime()),this._ts=this._act=0):(Ft(),this._ts=this._rts,this.totalTime(this.parent&&!this.parent.smoothChildTiming?this.rawTime():this._tTime||this._pTime,1===this.progress()&&Math.abs(this._zTime)!==X&&(this._tTime-=X)))),this):this._ps},qt.startTime=function startTime(t){if(arguments.length){this._start=t;var e=this.parent||this._dp;return!e||!e._sort&&this.parent||Ka(e,this,t-this._delay),this}return this._start},qt.endTime=function endTime(t){return this._start+(w(t)?this.totalDuration():this.duration())/Math.abs(this._ts||1)},qt.rawTime=function rawTime(t){var e=this.parent||this._dp;return e?t&&(!this._ts||this._repeat&&this._time&&this.totalProgress()<1)?this._tTime%(this._dur+this._rDelay):this._ts?Ga(e.rawTime(t),this):this._tTime:this._tTime},qt.revert=function revert(t){void 0===t&&(t=lt);var e=L;return L=t,(this._initted||this._startAt)&&(this.timeline&&this.timeline.revert(t),this.totalTime(-.01,t.suppressEvents)),"nested"!==this.data&&!1!==t.kill&&this.kill(),L=e,this},qt.globalTime=function globalTime(t){for(var e=this,r=arguments.length?t:e.rawTime();e;)r=e._start+r/(Math.abs(e._ts)||1),e=e._dp;return!this.parent&&this._sat?this._sat.globalTime(t):r},qt.repeat=function repeat(t){return arguments.length?(this._repeat=t===1/0?-2:t,Sa(this)):-2===this._repeat?1/0:this._repeat},qt.repeatDelay=function repeatDelay(t){if(arguments.length){var e=this._time;return this._rDelay=t,Sa(this),e?this.time(e):this}return this._rDelay},qt.yoyo=function yoyo(t){return arguments.length?(this._yoyo=t,this):this._yoyo},qt.seek=function seek(t,e){return this.totalTime(xt(this,t),w(e))},qt.restart=function restart(t,e){return this.play().totalTime(t?-this._delay:0,w(e)),this._dur||(this._zTime=-X),this},qt.play=function play(t,e){return null!=t&&this.seek(t,e),this.reversed(!1).paused(!1)},qt.reverse=function reverse(t,e){return null!=t&&this.seek(t||this.totalDuration(),e),this.reversed(!0).paused(!1)},qt.pause=function pause(t,e){return null!=t&&this.seek(t,e),this.paused(!0)},qt.resume=function resume(){return this.paused(!1)},qt.reversed=function reversed(t){return arguments.length?(!!t!==this.reversed()&&this.timeScale(-this._rts||(t?-X:0)),this):this._rts<0},qt.invalidate=function invalidate(){return this._initted=this._act=0,this._zTime=-X,this},qt.isActive=function isActive(){var t,e=this.parent||this._dp,r=this._start;return!(e&&!(this._ts&&this._initted&&e.isActive()&&(t=e.rawTime(!0))>=r&&t<this.endTime(!0)-X))},qt.eventCallback=function eventCallback(t,e,r){var i=this.vars;return 1<arguments.length?(e?(i[t]=e,r&&(i[t+"Params"]=r),"onUpdate"===t&&(this._onUpdate=e)):delete i[t],this):i[t]},qt.then=function then(t){var i=this;return new Promise(function(e){function Co(){var t=i.then;i.then=null,s(r)&&(r=r(i))&&(r.then||r===i)&&(i.then=t),e(r),i.then=t}var r=s(t)?t:pa;i._initted&&1===i.totalProgress()&&0<=i._ts||!i._tTime&&i._ts<0?Co():i._prom=Co})},qt.kill=function kill(){tb(this)},Animation);function Animation(t){this.vars=t,this._delay=+t.delay||0,(this._repeat=t.repeat===1/0?-2:t.repeat||0)&&(this._rDelay=t.repeatDelay||0,this._yoyo=!!t.yoyo||!!t.yoyoEase),this._ts=1,Ra(this,+t.duration,1,1),this.data=t.data,l&&(this._ctx=l).data.push(this),c||Rt.wake()}qa(Ut.prototype,{_time:0,_start:0,_end:0,_tTime:0,_tDur:0,_dirty:0,_repeat:0,_yoyo:!1,parent:null,_initted:!1,_rDelay:0,_ts:1,_dp:0,ratio:0,_zTime:-X,_prom:0,_ps:!1,_rts:1});var Xt=function(i){function Timeline(t,e){var r;return void 0===t&&(t={}),(r=i.call(this,t)||this).labels={},r.smoothChildTiming=!!t.smoothChildTiming,r.autoRemoveChildren=!!t.autoRemoveChildren,r._sort=w(t.sortChildren),I&&Ka(t.parent||I,_assertThisInitialized(r),e),t.reversed&&r.reverse(),t.paused&&r.paused(!0),t.scrollTrigger&&La(_assertThisInitialized(r),t.scrollTrigger),r}_inheritsLoose(Timeline,i);var e=Timeline.prototype;return e.to=function to(t,e,r){return Va(0,arguments,this),this},e.from=function from(t,e,r){return Va(1,arguments,this),this},e.fromTo=function fromTo(t,e,r,i){return Va(2,arguments,this),this},e.set=function set(t,e,r){return e.duration=0,e.parent=this,va(e).repeatDelay||(e.repeat=0),e.immediateRender=!!e.immediateRender,new $t(t,e,xt(this,r),1),this},e.call=function call(t,e,r){return Ka(this,$t.delayedCall(0,t,e),r)},e.staggerTo=function staggerTo(t,e,r,i,n,a,s){return r.duration=e,r.stagger=r.stagger||i,r.onComplete=a,r.onCompleteParams=s,r.parent=this,new $t(t,r,xt(this,n)),this},e.staggerFrom=function staggerFrom(t,e,r,i,n,a,s){return r.runBackwards=1,va(r).immediateRender=w(r.immediateRender),this.staggerTo(t,e,r,i,n,a,s)},e.staggerFromTo=function staggerFromTo(t,e,r,i,n,a,s,o){return i.startAt=r,va(i).immediateRender=w(i.immediateRender),this.staggerTo(t,e,i,n,a,s,o)},e.render=function render(t,e,r){var i,n,a,s,o,u,h,l,f,d,c,p,_=this._time,m=this._dirty?this.totalDuration():this._tDur,g=this._dur,v=t<=0?0:ja(t),y=this._zTime<0!=t<0&&(this._initted||!g);if(this!==I&&m<v&&0<=t&&(v=m),v!==this._tTime||r||y){if(_!==this._time&&g&&(v+=this._time-_,t+=this._time-_),i=v,f=this._start,u=!(l=this._ts),y&&(g||(_=this._zTime),!t&&e||(this._zTime=t)),this._repeat){if(c=this._yoyo,o=g+this._rDelay,this._repeat<-1&&t<0)return this.totalTime(100*o+t,e,r);if(i=ja(v%o),v===m?(s=this._repeat,i=g):((s=~~(d=ja(v/o)))&&s===d&&(i=g,s--),g<i&&(i=g)),d=Tt(this._tTime,o),!_&&this._tTime&&d!==s&&this._tTime-d*o-this._dur<=0&&(d=s),c&&1&s&&(i=g-i,p=1),s!==d&&!this._lock){var T=c&&1&d,b=T===(c&&1&s);if(s<d&&(T=!T),_=T?0:v%g?g:v,this._lock=1,this.render(_||(p?0:ja(s*o)),e,!g)._lock=0,this._tTime=v,!e&&this.parent&&Ct(this,"onRepeat"),this.vars.repeatRefresh&&!p&&(this.invalidate()._lock=1),_&&_!==this._time||u!=!this._ts||this.vars.onRepeat&&!this.parent&&!this._act)return this;if(g=this._dur,m=this._tDur,b&&(this._lock=2,_=T?g:-1e-4,this.render(_,!0),this.vars.repeatRefresh&&!p&&this.invalidate()),this._lock=0,!this._ts&&!u)return this;Qb(this,p)}}if(this._hasPause&&!this._forcing&&this._lock<2&&(h=function _findNextPauseTween(t,e,r){var i;if(e<r)for(i=t._first;i&&i._start<=r;){if("isPause"===i.data&&i._start>e)return i;i=i._next}else for(i=t._last;i&&i._start>=r;){if("isPause"===i.data&&i._start<e)return i;i=i._prev}}(this,ja(_),ja(i)))&&(v-=i-(i=h._start)),this._tTime=v,this._time=i,this._act=!l,this._initted||(this._onUpdate=this.vars.onUpdate,this._initted=1,this._zTime=t,_=0),!_&&i&&!e&&!s&&(Ct(this,"onStart"),this._tTime!==v))return this;if(_<=i&&0<=t)for(n=this._first;n;){if(a=n._next,(n._act||i>=n._start)&&n._ts&&h!==n){if(n.parent!==this)return this.render(t,e,r);if(n.render(0<n._ts?(i-n._start)*n._ts:(n._dirty?n.totalDuration():n._tDur)+(i-n._start)*n._ts,e,r),i!==this._time||!this._ts&&!u){h=0,a&&(v+=this._zTime=-X);break}}n=a}else{n=this._last;for(var w=t<0?t:i;n;){if(a=n._prev,(n._act||w<=n._end)&&n._ts&&h!==n){if(n.parent!==this)return this.render(t,e,r);if(n.render(0<n._ts?(w-n._start)*n._ts:(n._dirty?n.totalDuration():n._tDur)+(w-n._start)*n._ts,e,r||L&&(n._initted||n._startAt)),i!==this._time||!this._ts&&!u){h=0,a&&(v+=this._zTime=w?-X:X);break}}n=a}}if(h&&!e&&(this.pause(),h.render(_<=i?0:-X)._zTime=_<=i?1:-1,this._ts))return this._start=f,Ha(this),this.render(t,e,r);this._onUpdate&&!e&&Ct(this,"onUpdate",!0),(v===m&&this._tTime>=this.totalDuration()||!v&&_)&&(f!==this._start&&Math.abs(l)===Math.abs(this._ts)||this._lock||(!t&&g||!(v===m&&0<this._ts||!v&&this._ts<0)||za(this,1),e||t<0&&!_||!v&&!_&&m||(Ct(this,v===m&&0<=t?"onComplete":"onReverseComplete",!0),!this._prom||v<m&&0<this.timeScale()||this._prom())))}return this},e.add=function add(e,i){var n=this;if(t(i)||(i=xt(this,i,e)),!(e instanceof Ut)){if(Z(e))return e.forEach(function(t){return n.add(t,i)}),this;if(r(e))return this.addLabel(e,i);if(!s(e))return this;e=$t.delayedCall(0,e)}return this!==e?Ka(this,e,i):this},e.getChildren=function getChildren(t,e,r,i){void 0===t&&(t=!0),void 0===e&&(e=!0),void 0===r&&(r=!0),void 0===i&&(i=-U);for(var n=[],a=this._first;a;)a._start>=i&&(a instanceof $t?e&&n.push(a):(r&&n.push(a),t&&n.push.apply(n,a.getChildren(!0,e,r)))),a=a._next;return n},e.getById=function getById(t){for(var e=this.getChildren(1,1,1),r=e.length;r--;)if(e[r].vars.id===t)return e[r]},e.remove=function remove(t){return r(t)?this.removeLabel(t):s(t)?this.killTweensOf(t):(t.parent===this&&ya(this,t),t===this._recent&&(this._recent=this._last),Aa(this))},e.totalTime=function totalTime(t,e){return arguments.length?(this._forcing=1,!this._dp&&this._ts&&(this._start=ja(Rt.time-(0<this._ts?t/this._ts:(this.totalDuration()-t)/-this._ts))),i.prototype.totalTime.call(this,t,e),this._forcing=0,this):this._tTime},e.addLabel=function addLabel(t,e){return this.labels[t]=xt(this,e),this},e.removeLabel=function removeLabel(t){return delete this.labels[t],this},e.addPause=function addPause(t,e,r){var i=$t.delayedCall(0,e||T,r);return i.data="isPause",this._hasPause=1,Ka(this,i,xt(this,t))},e.removePause=function removePause(t){var e=this._first;for(t=xt(this,t);e;)e._start===t&&"isPause"===e.data&&za(e),e=e._next},e.killTweensOf=function killTweensOf(t,e,r){for(var i=this.getTweensOf(t,r),n=i.length;n--;)Nt!==i[n]&&i[n].kill(t,e);return this},e.getTweensOf=function getTweensOf(e,r){for(var i,n=[],a=Mt(e),s=this._first,o=t(r);s;)s instanceof $t?la(s._targets,a)&&(o?(!Nt||s._initted&&s._ts)&&s.globalTime(0)<=r&&s.globalTime(s.totalDuration())>r:!r||s.isActive())&&n.push(s):(i=s.getTweensOf(a,r)).length&&n.push.apply(n,i),s=s._next;return n},e.tweenTo=function tweenTo(t,e){e=e||{};var r,i=this,n=xt(i,t),a=e.startAt,s=e.onStart,o=e.onStartParams,u=e.immediateRender,h=$t.to(i,qa({ease:e.ease||"none",lazy:!1,immediateRender:!1,time:n,overwrite:"auto",duration:e.duration||Math.abs((n-(a&&"time"in a?a.time:i._time))/i.timeScale())||X,onStart:function onStart(){if(i.pause(),!r){var t=e.duration||Math.abs((n-(a&&"time"in a?a.time:i._time))/i.timeScale());h._dur!==t&&Ra(h,t,0,1).render(h._time,!0,!0),r=1}s&&s.apply(h,o||[])}},e));return u?h.render(0):h},e.tweenFromTo=function tweenFromTo(t,e,r){return this.tweenTo(e,qa({startAt:{time:xt(this,t)}},r))},e.recent=function recent(){return this._recent},e.nextLabel=function nextLabel(t){return void 0===t&&(t=this._time),rb(this,xt(this,t))},e.previousLabel=function previousLabel(t){return void 0===t&&(t=this._time),rb(this,xt(this,t),1)},e.currentLabel=function currentLabel(t){return arguments.length?this.seek(t,!0):this.previousLabel(this._time+X)},e.shiftChildren=function shiftChildren(t,e,r){void 0===r&&(r=0);for(var i,n=this._first,a=this.labels;n;)n._start>=r&&(n._start+=t,n._end+=t),n=n._next;if(e)for(i in a)a[i]>=r&&(a[i]+=t);return Aa(this)},e.invalidate=function invalidate(t){var e=this._first;for(this._lock=0;e;)e.invalidate(t),e=e._next;return i.prototype.invalidate.call(this,t)},e.clear=function clear(t){void 0===t&&(t=!0);for(var e,r=this._first;r;)e=r._next,this.remove(r),r=e;return this._dp&&(this._time=this._tTime=this._pTime=0),t&&(this.labels={}),Aa(this)},e.totalDuration=function totalDuration(t){var e,r,i,n=0,a=this,s=a._last,o=U;if(arguments.length)return a.timeScale((a._repeat<0?a.duration():a.totalDuration())/(a.reversed()?-t:t));if(a._dirty){for(i=a.parent;s;)e=s._prev,s._dirty&&s.totalDuration(),o<(r=s._start)&&a._sort&&s._ts&&!a._lock?(a._lock=1,Ka(a,s,r-s._delay,1)._lock=0):o=r,r<0&&s._ts&&(n-=r,(!i&&!a._dp||i&&i.smoothChildTiming)&&(a._start+=r/a._ts,a._time-=r,a._tTime-=r),a.shiftChildren(-r,!1,-Infinity),o=0),s._end>n&&s._ts&&(n=s._end),s=e;Ra(a,a===I&&a._time>n?a._time:n,1,1),a._dirty=0}return a._tDur},Timeline.updateRoot=function updateRoot(t){if(I._ts&&(na(I,Ga(t,I)),f=Rt.frame),Rt.frame>=mt){mt+=q.autoSleep||120;var e=I._first;if((!e||!e._ts)&&q.autoSleep&&Rt._listeners.length<2){for(;e&&!e._ts;)e=e._next;e||Rt.sleep()}}},Timeline}(Ut);qa(Xt.prototype,{_lock:0,_hasPause:0,_forcing:0});function ac(t,e,i,n,a,o){var u,h,l,f;if(pt[t]&&!1!==(u=new pt[t]).init(a,u.rawVars?e[t]:function _processVars(t,e,i,n,a){if(s(t)&&(t=Kt(t,a,e,i,n)),!v(t)||t.style&&t.nodeType||Z(t)||$(t))return r(t)?Kt(t,a,e,i,n):t;var o,u={};for(o in t)u[o]=Kt(t[o],a,e,i,n);return u}(e[t],n,a,o,i),i,n,o)&&(i._pt=h=new _e(i._pt,a,t,0,1,u.render,u,0,u.priority),i!==d))for(l=i._ptLookup[i._targets.indexOf(a)],f=u._props.length;f--;)l[u._props[f]]=h;return u}function gc(t,r,e,i){var n,a,s=r.ease||i||"power1.inOut";if(Z(r))a=e[t]||(e[t]=[]),r.forEach(function(t,e){return a.push({t:e/(r.length-1)*100,v:t,e:s})});else for(n in r)a=e[n]||(e[n]=[]),"ease"===n||a.push({t:parseFloat(t),v:r[n],e:s})}var Nt,Gt,Wt=function _addPropTween(t,e,i,n,a,o,u,h,l,f){s(n)&&(n=n(a||0,t,o));var d,c=t[e],p="get"!==i?i:s(c)?l?t[e.indexOf("set")||!s(t["get"+e.substr(3)])?e:"get"+e.substr(3)](l):t[e]():c,_=s(c)?l?re:te:Zt;if(r(n)&&(~n.indexOf("random(")&&(n=ob(n)),"="===n.charAt(1)&&(!(d=ka(p,n)+(Ya(p)||0))&&0!==d||(n=d))),!f||p!==n||Gt)return isNaN(p*n)||""===n?(c||e in t||Q(e,n),function _addComplexStringPropTween(t,e,r,i,n,a,s){var o,u,h,l,f,d,c,p,_=new _e(this._pt,t,e,0,1,ue,null,n),m=0,g=0;for(_.b=r,_.e=i,r+="",(c=~(i+="").indexOf("random("))&&(i=ob(i)),a&&(a(p=[r,i],t,e),r=p[0],i=p[1]),u=r.match(it)||[];o=it.exec(i);)l=o[0],f=i.substring(m,o.index),h?h=(h+1)%5:"rgba("===f.substr(-5)&&(h=1),l!==u[g++]&&(d=parseFloat(u[g-1])||0,_._pt={_next:_._pt,p:f||1===g?f:",",s:d,c:"="===l.charAt(1)?ka(d,l)-d:parseFloat(l)-d,m:h&&h<4?Math.round:0},m=it.lastIndex);return _.c=m<i.length?i.substring(m,i.length):"",_.fp=s,(nt.test(i)||c)&&(_.e=0),this._pt=_}.call(this,t,e,p,n,_,h||q.stringFilter,l)):(d=new _e(this._pt,t,e,+p||0,n-(p||0),"boolean"==typeof c?se:ae,0,_),l&&(d.fp=l),u&&d.modifier(u,this,t),this._pt=d)},Qt=function _initTween(t,e,r){var i,n,a,s,o,u,h,l,f,d,c,p,_,m=t.vars,g=m.ease,v=m.startAt,y=m.immediateRender,T=m.lazy,b=m.onUpdate,x=m.runBackwards,k=m.yoyoEase,O=m.keyframes,M=m.autoRevert,P=t._dur,C=t._startAt,A=t._targets,S=t.parent,z=S&&"nested"===S.data?S.vars.targets:A,E="auto"===t._overwrite&&!F,D=t.timeline;if(!D||O&&g||(g="none"),t._ease=jt(g,V.ease),t._yEase=k?Bt(jt(!0===k?g:k,V.ease)):0,k&&t._yoyo&&!t._repeat&&(k=t._yEase,t._yEase=t._ease,t._ease=k),t._from=!D&&!!m.runBackwards,!D||O&&!m.stagger){if(p=(l=A[0]?fa(A[0]).harness:0)&&m[l.prop],i=ua(m,ft),C&&(C._zTime<0&&C.progress(1),e<0&&x&&y&&!M?C.render(-1,!0):C.revert(x&&P?ht:ut),C._lazy=0),v){if(za(t._startAt=$t.set(A,qa({data:"isStart",overwrite:!1,parent:S,immediateRender:!0,lazy:!C&&w(T),startAt:null,delay:0,onUpdate:b&&function(){return Ct(t,"onUpdate")},stagger:0},v))),t._startAt._dp=0,t._startAt._sat=t,e<0&&(L||!y&&!M)&&t._startAt.revert(ht),y&&P&&e<=0&&r<=0)return void(e&&(t._zTime=e))}else if(x&&P&&!C)if(e&&(y=!1),a=qa({overwrite:!1,data:"isFromStart",lazy:y&&!C&&w(T),immediateRender:y,stagger:0,parent:S},i),p&&(a[l.prop]=p),za(t._startAt=$t.set(A,a)),t._startAt._dp=0,t._startAt._sat=t,e<0&&(L?t._startAt.revert(ht):t._startAt.render(-1,!0)),t._zTime=e,y){if(!e)return}else _initTween(t._startAt,X,X);for(t._pt=t._ptCache=0,T=P&&w(T)||T&&!P,n=0;n<A.length;n++){if(h=(o=A[n])._gsap||ea(A)[n]._gsap,t._ptLookup[n]=d={},ct[h.id]&&dt.length&&ma(),c=z===A?n:z.indexOf(o),l&&!1!==(f=new l).init(o,p||i,t,c,z)&&(t._pt=s=new _e(t._pt,o,f.name,0,1,f.render,f,0,f.priority),f._props.forEach(function(t){d[t]=s}),f.priority&&(u=1)),!l||p)for(a in i)pt[a]&&(f=ac(a,i,t,c,o,z))?f.priority&&(u=1):d[a]=s=Wt.call(t,o,a,"get",i[a],c,z,0,m.stringFilter);t._op&&t._op[n]&&t.kill(o,t._op[n]),E&&t._pt&&(Nt=t,I.killTweensOf(o,d,t.globalTime(e)),_=!t.parent,Nt=0),t._pt&&T&&(ct[h.id]=1)}u&&pe(t),t._onInit&&t._onInit(t)}t._onUpdate=b,t._initted=(!t._op||t._pt)&&!_,O&&e<=0&&D.render(U,!0,!0)},Kt=function _parseFuncOrString(t,e,i,n,a){return s(t)?t.call(e,i,n,a):r(t)&&~t.indexOf("random(")?ob(t):t},Jt=vt+"repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase,autoRevert",Ht={};ha(Jt+",id,stagger,delay,duration,paused,scrollTrigger",function(t){return Ht[t]=1});var $t=function(D){function Tween(e,r,i,n){var a;"number"==typeof r&&(i.duration=r,r=i,i=null);var s,o,u,h,l,f,d,c,p=(a=D.call(this,n?r:va(r))||this).vars,_=p.duration,m=p.delay,g=p.immediateRender,T=p.stagger,b=p.overwrite,x=p.keyframes,k=p.defaults,O=p.scrollTrigger,M=p.yoyoEase,P=r.parent||I,C=(Z(e)||$(e)?t(e[0]):"length"in r)?[e]:Mt(e);if(a._targets=C.length?ea(C):R("GSAP target "+e+" not found. https://gsap.com",!q.nullTargetWarn)||[],a._ptLookup=[],a._overwrite=b,x||T||y(_)||y(m)){if(r=a.vars,(s=a.timeline=new Xt({data:"nested",defaults:k||{},targets:P&&"nested"===P.data?P.vars.targets:C})).kill(),s.parent=s._dp=_assertThisInitialized(a),s._start=0,T||y(_)||y(m)){if(h=C.length,d=T&&eb(T),v(T))for(l in T)~Jt.indexOf(l)&&((c=c||{})[l]=T[l]);for(o=0;o<h;o++)(u=ua(r,Ht)).stagger=0,M&&(u.yoyoEase=M),c&&yt(u,c),f=C[o],u.duration=+Kt(_,_assertThisInitialized(a),o,f,C),u.delay=(+Kt(m,_assertThisInitialized(a),o,f,C)||0)-a._delay,!T&&1===h&&u.delay&&(a._delay=m=u.delay,a._start+=m,u.delay=0),s.to(f,u,d?d(o,f,C):0),s._ease=Lt.none;s.duration()?_=m=0:a.timeline=0}else if(x){va(qa(s.vars.defaults,{ease:"none"})),s._ease=jt(x.ease||r.ease||"none");var A,S,z,E=0;if(Z(x))x.forEach(function(t){return s.to(C,t,">")}),s.duration();else{for(l in u={},x)"ease"===l||"easeEach"===l||gc(l,x[l],u,x.easeEach);for(l in u)for(A=u[l].sort(function(t,e){return t.t-e.t}),o=E=0;o<A.length;o++)(z={ease:(S=A[o]).e,duration:(S.t-(o?A[o-1].t:0))/100*_})[l]=S.v,s.to(C,z,E),E+=z.duration;s.duration()<_&&s.to({},{duration:_-s.duration()})}}_||a.duration(_=s.duration())}else a.timeline=0;return!0!==b||F||(Nt=_assertThisInitialized(a),I.killTweensOf(C),Nt=0),Ka(P,_assertThisInitialized(a),i),r.reversed&&a.reverse(),r.paused&&a.paused(!0),(g||!_&&!x&&a._start===ja(P._time)&&w(g)&&function _hasNoPausedAncestors(t){return!t||t._ts&&_hasNoPausedAncestors(t.parent)}(_assertThisInitialized(a))&&"nested"!==P.data)&&(a._tTime=-X,a.render(Math.max(0,-m)||0)),O&&La(_assertThisInitialized(a),O),a}_inheritsLoose(Tween,D);var e=Tween.prototype;return e.render=function render(t,e,r){var i,n,a,s,o,u,h,l,f,d=this._time,c=this._tDur,p=this._dur,_=t<0,m=c-X<t&&!_?c:t<X?0:t;if(p){if(m!==this._tTime||!t||r||!this._initted&&this._tTime||this._startAt&&this._zTime<0!=_||this._lazy){if(i=m,l=this.timeline,this._repeat){if(s=p+this._rDelay,this._repeat<-1&&_)return this.totalTime(100*s+t,e,r);if(i=ja(m%s),m===c?(a=this._repeat,i=p):(a=~~(o=ja(m/s)))&&a===o?(i=p,a--):p<i&&(i=p),(u=this._yoyo&&1&a)&&(f=this._yEase,i=p-i),o=Tt(this._tTime,s),i===d&&!r&&this._initted&&a===o)return this._tTime=m,this;a!==o&&(l&&this._yEase&&Qb(l,u),this.vars.repeatRefresh&&!u&&!this._lock&&i!==s&&this._initted&&(this._lock=r=1,this.render(ja(s*a),!0).invalidate()._lock=0))}if(!this._initted){if(Ma(this,_?t:i,r,e,m))return this._tTime=0,this;if(!(d===this._time||r&&this.vars.repeatRefresh&&a!==o))return this;if(p!==this._dur)return this.render(t,e,r)}if(this._tTime=m,this._time=i,!this._act&&this._ts&&(this._act=1,this._lazy=0),this.ratio=h=(f||this._ease)(i/p),this._from&&(this.ratio=h=1-h),i&&!d&&!e&&!a&&(Ct(this,"onStart"),this._tTime!==m))return this;for(n=this._pt;n;)n.r(h,n.d),n=n._next;l&&l.render(t<0?t:l._dur*l._ease(i/this._dur),e,r)||this._startAt&&(this._zTime=t),this._onUpdate&&!e&&(_&&Ca(this,t,0,r),Ct(this,"onUpdate")),this._repeat&&a!==o&&this.vars.onRepeat&&!e&&this.parent&&Ct(this,"onRepeat"),m!==this._tDur&&m||this._tTime!==m||(_&&!this._onUpdate&&Ca(this,t,0,!0),!t&&p||!(m===this._tDur&&0<this._ts||!m&&this._ts<0)||za(this,1),e||_&&!d||!(m||d||u)||(Ct(this,m===c?"onComplete":"onReverseComplete",!0),!this._prom||m<c&&0<this.timeScale()||this._prom()))}}else!function _renderZeroDurationTween(t,e,r,i){var n,a,s,o=t.ratio,u=e<0||!e&&(!t._start&&function _parentPlayheadIsBeforeStart(t){var e=t.parent;return e&&e._ts&&e._initted&&!e._lock&&(e.rawTime()<0||_parentPlayheadIsBeforeStart(e))}(t)&&(t._initted||!bt(t))||(t._ts<0||t._dp._ts<0)&&!bt(t))?0:1,h=t._rDelay,l=0;if(h&&t._repeat&&(l=kt(0,t._tDur,e),a=Tt(l,h),t._yoyo&&1&a&&(u=1-u),a!==Tt(t._tTime,h)&&(o=1-u,t.vars.repeatRefresh&&t._initted&&t.invalidate())),u!==o||L||i||t._zTime===X||!e&&t._zTime){if(!t._initted&&Ma(t,e,i,r,l))return;for(s=t._zTime,t._zTime=e||(r?X:0),r=r||e&&!s,t.ratio=u,t._from&&(u=1-u),t._time=0,t._tTime=l,n=t._pt;n;)n.r(u,n.d),n=n._next;e<0&&Ca(t,e,0,!0),t._onUpdate&&!r&&Ct(t,"onUpdate"),l&&t._repeat&&!r&&t.parent&&Ct(t,"onRepeat"),(e>=t._tDur||e<0)&&t.ratio===u&&(u&&za(t,1),r||L||(Ct(t,u?"onComplete":"onReverseComplete",!0),t._prom&&t._prom()))}else t._zTime||(t._zTime=e)}(this,t,e,r);return this},e.targets=function targets(){return this._targets},e.invalidate=function invalidate(t){return t&&this.vars.runBackwards||(this._startAt=0),this._pt=this._op=this._onUpdate=this._lazy=this.ratio=0,this._ptLookup=[],this.timeline&&this.timeline.invalidate(t),D.prototype.invalidate.call(this,t)},e.resetTo=function resetTo(t,e,r,i,n){c||Rt.wake(),this._ts||this.play();var a,s=Math.min(this._dur,(this._dp._time-this._start)*this._ts);return this._initted||Qt(this,s),a=this._ease(s/this._dur),function _updatePropTweens(t,e,r,i,n,a,s,o){var u,h,l,f,d=(t._pt&&t._ptCache||(t._ptCache={}))[e];if(!d)for(d=t._ptCache[e]=[],l=t._ptLookup,f=t._targets.length;f--;){if((u=l[f][e])&&u.d&&u.d._pt)for(u=u.d._pt;u&&u.p!==e&&u.fp!==e;)u=u._next;if(!u)return Gt=1,t.vars[e]="+=0",Qt(t,s),Gt=0,o?R(e+" not eligible for reset"):1;d.push(u)}for(f=d.length;f--;)(u=(h=d[f])._pt||h).s=!i&&0!==i||n?u.s+(i||0)+a*u.c:i,u.c=r-u.s,h.e&&(h.e=ia(r)+Ya(h.e)),h.b&&(h.b=u.s+Ya(h.b))}(this,t,e,r,i,a,s,n)?this.resetTo(t,e,r,i,1):(Ia(this,0),this.parent||xa(this._dp,this,"_first","_last",this._dp._sort?"_start":0),this.render(0))},e.kill=function kill(t,e){if(void 0===e&&(e="all"),!(t||e&&"all"!==e))return this._lazy=this._pt=0,this.parent?tb(this):this.scrollTrigger&&this.scrollTrigger.kill(!!L),this;if(this.timeline){var i=this.timeline.totalDuration();return this.timeline.killTweensOf(t,e,Nt&&!0!==Nt.vars.overwrite)._first||tb(this),this.parent&&i!==this.timeline.totalDuration()&&Ra(this,this._dur*this.timeline._tDur/i,0,1),this}var n,a,s,o,u,h,l,f=this._targets,d=t?Mt(t):f,c=this._ptLookup,p=this._pt;if((!e||"all"===e)&&function _arraysMatch(t,e){for(var r=t.length,i=r===e.length;i&&r--&&t[r]===e[r];);return r<0}(f,d))return"all"===e&&(this._pt=0),tb(this);for(n=this._op=this._op||[],"all"!==e&&(r(e)&&(u={},ha(e,function(t){return u[t]=1}),e=u),e=function _addAliasesToVars(t,e){var r,i,n,a,s=t[0]?fa(t[0]).harness:0,o=s&&s.aliases;if(!o)return e;for(i in r=yt({},e),o)if(i in r)for(n=(a=o[i].split(",")).length;n--;)r[a[n]]=r[i];return r}(f,e)),l=f.length;l--;)if(~d.indexOf(f[l]))for(u in a=c[l],"all"===e?(n[l]=e,o=a,s={}):(s=n[l]=n[l]||{},o=e),o)(h=a&&a[u])&&("kill"in h.d&&!0!==h.d.kill(u)||ya(this,h,"_pt"),delete a[u]),"all"!==s&&(s[u]=1);return this._initted&&!this._pt&&p&&tb(this),this},Tween.to=function to(t,e,r){return new Tween(t,e,r)},Tween.from=function from(t,e){return Va(1,arguments)},Tween.delayedCall=function delayedCall(t,e,r,i){return new Tween(e,0,{immediateRender:!1,lazy:!1,overwrite:!1,delay:t,onComplete:e,onReverseComplete:e,onCompleteParams:r,onReverseCompleteParams:r,callbackScope:i})},Tween.fromTo=function fromTo(t,e,r){return Va(2,arguments)},Tween.set=function set(t,e){return e.duration=0,e.repeatDelay||(e.repeat=0),new Tween(t,e)},Tween.killTweensOf=function killTweensOf(t,e,r){return I.killTweensOf(t,e,r)},Tween}(Ut);qa($t.prototype,{_targets:[],_lazy:0,_startAt:0,_op:0,_onInit:0}),ha("staggerTo,staggerFrom,staggerFromTo",function(r){$t[r]=function(){var t=new Xt,e=Ot.call(arguments,0);return e.splice("staggerFromTo"===r?5:4,0,0),t[r].apply(t,e)}});function oc(t,e,r){return t.setAttribute(e,r)}function wc(t,e,r,i){i.mSet(t,e,i.m.call(i.tween,r,i.mt),i)}var Zt=function _setterPlain(t,e,r){return t[e]=r},te=function _setterFunc(t,e,r){return t[e](r)},re=function _setterFuncWithParam(t,e,r,i){return t[e](i.fp,r)},ne=function _getSetter(t,e){return s(t[e])?te:u(t[e])&&t.setAttribute?oc:Zt},ae=function _renderPlain(t,e){return e.set(e.t,e.p,Math.round(1e6*(e.s+e.c*t))/1e6,e)},se=function _renderBoolean(t,e){return e.set(e.t,e.p,!!(e.s+e.c*t),e)},ue=function _renderComplexString(t,e){var r=e._pt,i="";if(!t&&e.b)i=e.b;else if(1===t&&e.e)i=e.e;else{for(;r;)i=r.p+(r.m?r.m(r.s+r.c*t):Math.round(1e4*(r.s+r.c*t))/1e4)+i,r=r._next;i+=e.c}e.set(e.t,e.p,i,e)},he=function _renderPropTweens(t,e){for(var r=e._pt;r;)r.r(t,r.d),r=r._next},fe=function _addPluginModifier(t,e,r,i){for(var n,a=this._pt;a;)n=a._next,a.p===i&&a.modifier(t,e,r),a=n},ce=function _killPropTweensOf(t){for(var e,r,i=this._pt;i;)r=i._next,i.p===t&&!i.op||i.op===t?ya(this,i,"_pt"):i.dep||(e=1),i=r;return!e},pe=function _sortPropTweensByPriority(t){for(var e,r,i,n,a=t._pt;a;){for(e=a._next,r=i;r&&r.pr>a.pr;)r=r._next;(a._prev=r?r._prev:n)?a._prev._next=a:i=a,(a._next=r)?r._prev=a:n=a,a=e}t._pt=i},_e=(PropTween.prototype.modifier=function modifier(t,e,r){this.mSet=this.mSet||this.set,this.set=wc,this.m=t,this.mt=r,this.tween=e},PropTween);function PropTween(t,e,r,i,n,a,s,o,u){this.t=e,this.s=i,this.c=n,this.p=r,this.r=a||ae,this.d=s||this,this.set=o||Zt,this.pr=u||0,(this._next=t)&&(t._prev=this)}ha(vt+"parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger",function(t){return ft[t]=1}),ot.TweenMax=ot.TweenLite=$t,ot.TimelineLite=ot.TimelineMax=Xt,I=new Xt({sortChildren:!1,defaults:V,autoRemoveChildren:!0,id:"root",smoothChildTiming:!0}),q.stringFilter=Fb;function Ec(t){return(ye[t]||Te).map(function(t){return t()})}function Fc(){var t=Date.now(),o=[];2<t-Oe&&(Ec("matchMediaInit"),ge.forEach(function(t){var e,r,i,n,a=t.queries,s=t.conditions;for(r in a)(e=h.matchMedia(a[r]).matches)&&(i=1),e!==s[r]&&(s[r]=e,n=1);n&&(t.revert(),i&&o.push(t))}),Ec("matchMediaRevert"),o.forEach(function(e){return e.onMatch(e,function(t){return e.add(null,t)})}),Oe=t,Ec("matchMedia"))}var me,ge=[],ye={},Te=[],Oe=0,Me=0,Pe=((me=Context.prototype).add=function add(t,i,n){function Gw(){var t,e=l,r=a.selector;return e&&e!==a&&e.data.push(a),n&&(a.selector=cb(n)),l=a,t=i.apply(a,arguments),s(t)&&a._r.push(t),l=e,a.selector=r,a.isReverted=!1,t}s(t)&&(n=i,i=t,t=s);var a=this;return a.last=Gw,t===s?Gw(a,function(t){return a.add(null,t)}):t?a[t]=Gw:Gw},me.ignore=function ignore(t){var e=l;l=null,t(this),l=e},me.getTweens=function getTweens(){var e=[];return this.data.forEach(function(t){return t instanceof Context?e.push.apply(e,t.getTweens()):t instanceof $t&&!(t.parent&&"nested"===t.parent.data)&&e.push(t)}),e},me.clear=function clear(){this._r.length=this.data.length=0},me.kill=function kill(i,t){var n=this;if(i?function(){for(var t,e=n.getTweens(),r=n.data.length;r--;)"isFlip"===(t=n.data[r]).data&&(t.revert(),t.getChildren(!0,!0,!1).forEach(function(t){return e.splice(e.indexOf(t),1)}));for(e.map(function(t){return{g:t._dur||t._delay||t._sat&&!t._sat.vars.immediateRender?t.globalTime(0):-1/0,t:t}}).sort(function(t,e){return e.g-t.g||-1/0}).forEach(function(t){return t.t.revert(i)}),r=n.data.length;r--;)(t=n.data[r])instanceof Xt?"nested"!==t.data&&(t.scrollTrigger&&t.scrollTrigger.revert(),t.kill()):t instanceof $t||!t.revert||t.revert(i);n._r.forEach(function(t){return t(i,n)}),n.isReverted=!0}():this.data.forEach(function(t){return t.kill&&t.kill()}),this.clear(),t)for(var e=ge.length;e--;)ge[e].id===this.id&&ge.splice(e,1)},me.revert=function revert(t){this.kill(t||{})},Context);function Context(t,e){this.selector=e&&cb(e),this.data=[],this._r=[],this.isReverted=!1,this.id=Me++,t&&this.add(t)}var Ce,Ae=((Ce=MatchMedia.prototype).add=function add(t,e,r){v(t)||(t={matches:t});var i,n,a,s=new Pe(0,r||this.scope),o=s.conditions={};for(n in l&&!s.selector&&(s.selector=l.selector),this.contexts.push(s),e=s.add("onMatch",e),s.queries=t)"all"===n?a=1:(i=h.matchMedia(t[n]))&&(ge.indexOf(s)<0&&ge.push(s),(o[n]=i.matches)&&(a=1),i.addListener?i.addListener(Fc):i.addEventListener("change",Fc));return a&&e(s,function(t){return s.add(null,t)}),this},Ce.revert=function revert(t){this.kill(t||{})},Ce.kill=function kill(e){this.contexts.forEach(function(t){return t.kill(e,!0)})},MatchMedia);function MatchMedia(t){this.contexts=[],this.scope=t,l&&l.data.push(this)}var Se={registerPlugin:function registerPlugin(){for(var t=arguments.length,e=new Array(t),r=0;r<t;r++)e[r]=arguments[r];e.forEach(function(t){return wb(t)})},timeline:function timeline(t){return new Xt(t)},getTweensOf:function getTweensOf(t,e){return I.getTweensOf(t,e)},getProperty:function getProperty(i,t,e,n){r(i)&&(i=Mt(i)[0]);var a=fa(i||{}).get,s=e?pa:oa;return"native"===e&&(e=""),i?t?s((pt[t]&&pt[t].get||a)(i,t,e,n)):function(t,e,r){return s((pt[t]&&pt[t].get||a)(i,t,e,r))}:i},quickSetter:function quickSetter(r,e,i){if(1<(r=Mt(r)).length){var n=r.map(function(t){return ze.quickSetter(t,e,i)}),a=n.length;return function(t){for(var e=a;e--;)n[e](t)}}r=r[0]||{};var s=pt[e],o=fa(r),u=o.harness&&(o.harness.aliases||{})[e]||e,h=s?function(t){var e=new s;d._pt=0,e.init(r,i?t+i:t,d,0,[r]),e.render(1,e),d._pt&&he(1,d)}:o.set(r,u);return s?h:function(t){return h(r,u,i?t+i:t,o,1)}},quickTo:function quickTo(t,i,e){function $x(t,e,r){return n.resetTo(i,t,e,r)}var r,n=ze.to(t,qa(((r={})[i]="+=0.1",r.paused=!0,r.stagger=0,r),e||{}));return $x.tween=n,$x},isTweening:function isTweening(t){return 0<I.getTweensOf(t,!0).length},defaults:function defaults(t){return t&&t.ease&&(t.ease=jt(t.ease,V.ease)),ta(V,t||{})},config:function config(t){return ta(q,t||{})},registerEffect:function registerEffect(t){var i=t.name,n=t.effect,e=t.plugins,a=t.defaults,r=t.extendTimeline;(e||"").split(",").forEach(function(t){return t&&!pt[t]&&!ot[t]&&R(i+" effect requires "+t+" plugin.")}),_t[i]=function(t,e,r){return n(Mt(t),qa(e||{},a),r)},r&&(Xt.prototype[i]=function(t,e,r){return this.add(_t[i](t,v(e)?e:(r=e)&&{},this),r)})},registerEase:function registerEase(t,e){Lt[t]=jt(e)},parseEase:function parseEase(t,e){return arguments.length?jt(t,e):Lt},getById:function getById(t){return I.getById(t)},exportRoot:function exportRoot(t,e){void 0===t&&(t={});var r,i,n=new Xt(t);for(n.smoothChildTiming=w(t.smoothChildTiming),I.remove(n),n._dp=0,n._time=n._tTime=I._time,r=I._first;r;)i=r._next,!e&&!r._dur&&r instanceof $t&&r.vars.onComplete===r._targets[0]||Ka(n,r,r._start-r._delay),r=i;return Ka(I,n,0),n},context:function context(t,e){return t?new Pe(t,e):l},matchMedia:function matchMedia(t){return new Ae(t)},matchMediaRefresh:function matchMediaRefresh(){return ge.forEach(function(t){var e,r,i=t.conditions;for(r in i)i[r]&&(i[r]=!1,e=1);e&&t.revert()})||Fc()},addEventListener:function addEventListener(t,e){var r=ye[t]||(ye[t]=[]);~r.indexOf(e)||r.push(e)},removeEventListener:function removeEventListener(t,e){var r=ye[t],i=r&&r.indexOf(e);0<=i&&r.splice(i,1)},utils:{wrap:function wrap(e,t,r){var i=t-e;return Z(e)?lb(e,wrap(0,e.length),t):Wa(r,function(t){return(i+(t-e)%i)%i+e})},wrapYoyo:function wrapYoyo(e,t,r){var i=t-e,n=2*i;return Z(e)?lb(e,wrapYoyo(0,e.length-1),t):Wa(r,function(t){return e+(i<(t=(n+(t-e)%n)%n||0)?n-t:t)})},distribute:eb,random:hb,snap:gb,normalize:function normalize(t,e,r){return Pt(t,e,0,1,r)},getUnit:Ya,clamp:function clamp(e,r,t){return Wa(t,function(t){return kt(e,r,t)})},splitColor:Ab,toArray:Mt,selector:cb,mapRange:Pt,pipe:function pipe(){for(var t=arguments.length,e=new Array(t),r=0;r<t;r++)e[r]=arguments[r];return function(t){return e.reduce(function(t,e){return e(t)},t)}},unitize:function unitize(e,r){return function(t){return e(parseFloat(t))+(r||Ya(t))}},interpolate:function interpolate(e,i,t,n){var a=isNaN(e+i)?0:function(t){return(1-t)*e+t*i};if(!a){var s,o,u,h,l,f=r(e),d={};if(!0===t&&(n=1)&&(t=null),f)e={p:e},i={p:i};else if(Z(e)&&!Z(i)){for(u=[],h=e.length,l=h-2,o=1;o<h;o++)u.push(interpolate(e[o-1],e[o]));h--,a=function func(t){t*=h;var e=Math.min(l,~~t);return u[e](t-e)},t=i}else n||(e=yt(Z(e)?[]:{},e));if(!u){for(s in i)Wt.call(d,e,s,"get",i[s]);a=function func(t){return he(t,d)||(f?e.p:e)}}}return Wa(t,a)},shuffle:db},install:P,effects:_t,ticker:Rt,updateRoot:Xt.updateRoot,plugins:pt,globalTimeline:I,core:{PropTween:_e,globals:S,Tween:$t,Timeline:Xt,Animation:Ut,getCache:fa,_removeLinkedListItem:ya,reverting:function reverting(){return L},context:function context(t){return t&&l&&(l.data.push(t),t._ctx=l),l},suppressOverwrites:function suppressOverwrites(t){return F=t}}};ha("to,from,fromTo,delayedCall,set,killTweensOf",function(t){return Se[t]=$t[t]}),Rt.add(Xt.updateRoot),d=Se.to({},{duration:0});function Jc(t,e){for(var r=t._pt;r&&r.p!==e&&r.op!==e&&r.fp!==e;)r=r._next;return r}function Lc(t,a){return{name:t,rawVars:1,init:function init(t,n,e){e._onInit=function(t){var e,i;if(r(n)&&(e={},ha(n,function(t){return e[t]=1}),n=e),a){for(i in e={},n)e[i]=a(n[i]);n=e}!function _addModifiers(t,e){var r,i,n,a=t._targets;for(r in e)for(i=a.length;i--;)(n=(n=t._ptLookup[i][r])&&n.d)&&(n._pt&&(n=Jc(n,r)),n&&n.modifier&&n.modifier(e[r],t,a[i],r))}(t,n)}}}}var ze=Se.registerPlugin({name:"attr",init:function init(t,e,r,i,n){var a,s,o;for(a in this.tween=r,e)o=t.getAttribute(a)||"",(s=this.add(t,"setAttribute",(o||0)+"",e[a],i,n,0,0,a)).op=a,s.b=o,this._props.push(a)},render:function render(t,e){for(var r=e._pt;r;)L?r.set(r.t,r.p,r.b,r):r.r(t,r.d),r=r._next}},{name:"endArray",init:function init(t,e){for(var r=e.length;r--;)this.add(t,r,t[r]||0,e[r],0,0,0,0,0,1)}},Lc("roundProps",fb),Lc("modifiers"),Lc("snap",gb))||Se;$t.version=Xt.version=ze.version="3.12.7",o=1,x()&&Ft();function vd(t,e){return e.set(e.t,e.p,Math.round(1e4*(e.s+e.c*t))/1e4+e.u,e)}function wd(t,e){return e.set(e.t,e.p,1===t?e.e:Math.round(1e4*(e.s+e.c*t))/1e4+e.u,e)}function xd(t,e){return e.set(e.t,e.p,t?Math.round(1e4*(e.s+e.c*t))/1e4+e.u:e.b,e)}function yd(t,e){var r=e.s+e.c*t;e.set(e.t,e.p,~~(r+(r<0?-.5:.5))+e.u,e)}function zd(t,e){return e.set(e.t,e.p,t?e.e:e.b,e)}function Ad(t,e){return e.set(e.t,e.p,1!==t?e.b:e.e,e)}function Bd(t,e,r){return t.style[e]=r}function Cd(t,e,r){return t.style.setProperty(e,r)}function Dd(t,e,r){return t._gsap[e]=r}function Ed(t,e,r){return t._gsap.scaleX=t._gsap.scaleY=r}function Fd(t,e,r,i,n){var a=t._gsap;a.scaleX=a.scaleY=r,a.renderTransform(n,a)}function Gd(t,e,r,i,n){var a=t._gsap;a[e]=r,a.renderTransform(n,a)}function Jd(t,e){var r=this,i=this.target,n=i.style,a=i._gsap;if(t in ar&&n){if(this.tfm=this.tfm||{},"transform"===t)return dr.transform.split(",").forEach(function(t){return Jd.call(r,t,e)});if(~(t=dr[t]||t).indexOf(",")?t.split(",").forEach(function(t){return r.tfm[t]=yr(i,t)}):this.tfm[t]=a.x?a[t]:yr(i,t),t===pr&&(this.tfm.zOrigin=a.zOrigin),0<=this.props.indexOf(cr))return;a.svg&&(this.svgo=i.getAttribute("data-svg-origin"),this.props.push(pr,e,"")),t=cr}(n||e)&&this.props.push(t,e,n[t])}function Kd(t){t.translate&&(t.removeProperty("translate"),t.removeProperty("scale"),t.removeProperty("rotate"))}function Ld(){var t,e,r=this.props,i=this.target,n=i.style,a=i._gsap;for(t=0;t<r.length;t+=3)r[t+1]?2===r[t+1]?i[r[t]](r[t+2]):i[r[t]]=r[t+2]:r[t+2]?n[r[t]]=r[t+2]:n.removeProperty("--"===r[t].substr(0,2)?r[t]:r[t].replace(hr,"-$1").toLowerCase());if(this.tfm){for(e in this.tfm)a[e]=this.tfm[e];a.svg&&(a.renderTransform(),i.setAttribute("data-svg-origin",this.svgo||"")),(t=Ye())&&t.isStart||n[cr]||(Kd(n),a.zOrigin&&n[pr]&&(n[pr]+=" "+a.zOrigin+"px",a.zOrigin=0,a.renderTransform()),a.uncache=1)}}function Md(t,e){var r={target:t,props:[],revert:Ld,save:Jd};return t._gsap||ze.core.getCache(t),e&&t.style&&t.nodeType&&e.split(",").forEach(function(t){return r.save(t)}),r}function Od(t,e){var r=De.createElementNS?De.createElementNS((e||"http://www.w3.org/1999/xhtml").replace(/^https/,"http"),t):De.createElement(t);return r&&r.style?r:De.createElement(t)}function Pd(t,e,r){var i=getComputedStyle(t);return i[e]||i.getPropertyValue(e.replace(hr,"-$1").toLowerCase())||i.getPropertyValue(e)||!r&&Pd(t,mr(e)||e,1)||""}function Sd(){(function _windowExists(){return"undefined"!=typeof window})()&&window.document&&(Ee=window,De=Ee.document,Re=De.documentElement,Le=Od("div")||{style:{}},Od("div"),cr=mr(cr),pr=cr+"Origin",Le.style.cssText="border-width:0;line-height:0;position:absolute;padding:0",Be=!!mr("perspective"),Ye=ze.core.reverting,Fe=1)}function Td(t){var e,r=t.ownerSVGElement,i=Od("svg",r&&r.getAttribute("xmlns")||"http://www.w3.org/2000/svg"),n=t.cloneNode(!0);n.style.display="block",i.appendChild(n),Re.appendChild(i);try{e=n.getBBox()}catch(t){}return i.removeChild(n),Re.removeChild(i),e}function Ud(t,e){for(var r=e.length;r--;)if(t.hasAttribute(e[r]))return t.getAttribute(e[r])}function Vd(e){var r,i;try{r=e.getBBox()}catch(t){r=Td(e),i=1}return r&&(r.width||r.height)||i||(r=Td(e)),!r||r.width||r.x||r.y?r:{x:+Ud(e,["x","cx","x1"])||0,y:+Ud(e,["y","cy","y1"])||0,width:0,height:0}}function Wd(t){return!(!t.getCTM||t.parentNode&&!t.ownerSVGElement||!Vd(t))}function Xd(t,e){if(e){var r,i=t.style;e in ar&&e!==pr&&(e=cr),i.removeProperty?("ms"!==(r=e.substr(0,2))&&"webkit"!==e.substr(0,6)||(e="-"+e),i.removeProperty("--"===r?e:e.replace(hr,"-$1").toLowerCase())):i.removeAttribute(e)}}function Yd(t,e,r,i,n,a){var s=new _e(t._pt,e,r,0,1,a?Ad:zd);return(t._pt=s).b=i,s.e=n,t._props.push(r),s}function _d(t,e,r,i){var n,a,s,o,u=parseFloat(r)||0,h=(r+"").trim().substr((u+"").length)||"px",l=Le.style,f=lr.test(e),d="svg"===t.tagName.toLowerCase(),c=(d?"client":"offset")+(f?"Width":"Height"),p="px"===i,_="%"===i;if(i===h||!u||gr[i]||gr[h])return u;if("px"===h||p||(u=_d(t,e,r,"px")),o=t.getCTM&&Wd(t),(_||"%"===h)&&(ar[e]||~e.indexOf("adius")))return n=o?t.getBBox()[f?"width":"height"]:t[c],ia(_?u/n*100:u/100*n);if(l[f?"width":"height"]=100+(p?h:i),a="rem"!==i&&~e.indexOf("adius")||"em"===i&&t.appendChild&&!d?t:t.parentNode,o&&(a=(t.ownerSVGElement||{}).parentNode),a&&a!==De&&a.appendChild||(a=De.body),(s=a._gsap)&&_&&s.width&&f&&s.time===Rt.time&&!s.uncache)return ia(u/s.width*100);if(!_||"height"!==e&&"width"!==e)!_&&"%"!==h||vr[Pd(a,"display")]||(l.position=Pd(t,"position")),a===t&&(l.position="static"),a.appendChild(Le),n=Le[c],a.removeChild(Le),l.position="absolute";else{var m=t.style[e];t.style[e]=100+i,n=t[c],m?t.style[e]=m:Xd(t,e)}return f&&_&&((s=fa(a)).time=Rt.time,s.width=a[c]),ia(p?n*u/100:n&&u?100/n*u:0)}function be(t,e,r,i){if(!r||"none"===r){var n=mr(e,t,1),a=n&&Pd(t,n,1);a&&a!==r?(e=n,r=a):"borderColor"===e&&(r=Pd(t,"borderTopColor"))}var s,o,u,h,l,f,d,c,p,_,m,g=new _e(this._pt,t.style,e,0,1,ue),v=0,y=0;if(g.b=r,g.e=i,r+="","auto"===(i+="")&&(f=t.style[e],t.style[e]=i,i=Pd(t,e)||i,f?t.style[e]=f:Xd(t,e)),Fb(s=[r,i]),i=s[1],u=(r=s[0]).match(rt)||[],(i.match(rt)||[]).length){for(;o=rt.exec(i);)d=o[0],p=i.substring(v,o.index),l?l=(l+1)%5:"rgba("!==p.substr(-5)&&"hsla("!==p.substr(-5)||(l=1),d!==(f=u[y++]||"")&&(h=parseFloat(f)||0,m=f.substr((h+"").length),"="===d.charAt(1)&&(d=ka(h,d)+m),c=parseFloat(d),_=d.substr((c+"").length),v=rt.lastIndex-_.length,_||(_=_||q.units[e]||m,v===i.length&&(i+=_,g.e+=_)),m!==_&&(h=_d(t,e,f,_)||0),g._pt={_next:g._pt,p:p||1===y?p:",",s:h,c:c-h,m:l&&l<4||"zIndex"===e?Math.round:0});g.c=v<i.length?i.substring(v,i.length):""}else g.r="display"===e&&"none"===i?Ad:zd;return nt.test(i)&&(g.e=0),this._pt=g}function de(t){var e=t.split(" "),r=e[0],i=e[1]||"50%";return"top"!==r&&"bottom"!==r&&"left"!==i&&"right"!==i||(t=r,r=i,i=t),e[0]=Tr[r]||r,e[1]=Tr[i]||i,e.join(" ")}function ee(t,e){if(e.tween&&e.tween._time===e.tween._dur){var r,i,n,a=e.t,s=a.style,o=e.u,u=a._gsap;if("all"===o||!0===o)s.cssText="",i=1;else for(n=(o=o.split(",")).length;-1<--n;)r=o[n],ar[r]&&(i=1,r="transformOrigin"===r?pr:cr),Xd(a,r);i&&(Xd(a,cr),u&&(u.svg&&a.removeAttribute("transform"),s.scale=s.rotate=s.translate="none",kr(a,1),u.uncache=1,Kd(s)))}}function ie(t){return"matrix(1, 0, 0, 1, 0, 0)"===t||"none"===t||!t}function je(t){var e=Pd(t,cr);return ie(e)?wr:e.substr(7).match(et).map(ia)}function ke(t,e){var r,i,n,a,s=t._gsap||fa(t),o=t.style,u=je(t);return s.svg&&t.getAttribute("transform")?"1,0,0,1,0,0"===(u=[(n=t.transform.baseVal.consolidate().matrix).a,n.b,n.c,n.d,n.e,n.f]).join(",")?wr:u:(u!==wr||t.offsetParent||t===Re||s.svg||(n=o.display,o.display="block",(r=t.parentNode)&&(t.offsetParent||t.getBoundingClientRect().width)||(a=1,i=t.nextElementSibling,Re.appendChild(t)),u=je(t),n?o.display=n:Xd(t,"display"),a&&(i?r.insertBefore(t,i):r?r.appendChild(t):Re.removeChild(t))),e&&6<u.length?[u[0],u[1],u[4],u[5],u[12],u[13]]:u)}function le(t,e,r,i,n,a){var s,o,u,h=t._gsap,l=n||ke(t,!0),f=h.xOrigin||0,d=h.yOrigin||0,c=h.xOffset||0,p=h.yOffset||0,_=l[0],m=l[1],g=l[2],v=l[3],y=l[4],T=l[5],b=e.split(" "),w=parseFloat(b[0])||0,x=parseFloat(b[1])||0;r?l!==wr&&(o=_*v-m*g)&&(u=w*(-m/o)+x*(_/o)-(_*T-m*y)/o,w=w*(v/o)+x*(-g/o)+(g*T-v*y)/o,x=u):(w=(s=Vd(t)).x+(~b[0].indexOf("%")?w/100*s.width:w),x=s.y+(~(b[1]||b[0]).indexOf("%")?x/100*s.height:x)),i||!1!==i&&h.smooth?(y=w-f,T=x-d,h.xOffset=c+(y*_+T*g)-y,h.yOffset=p+(y*m+T*v)-T):h.xOffset=h.yOffset=0,h.xOrigin=w,h.yOrigin=x,h.smooth=!!i,h.origin=e,h.originIsAbsolute=!!r,t.style[pr]="0px 0px",a&&(Yd(a,h,"xOrigin",f,w),Yd(a,h,"yOrigin",d,x),Yd(a,h,"xOffset",c,h.xOffset),Yd(a,h,"yOffset",p,h.yOffset)),t.setAttribute("data-svg-origin",w+" "+x)}function oe(t,e,r){var i=Ya(e);return ia(parseFloat(e)+parseFloat(_d(t,"x",r+"px",i)))+i}function ve(t,e,i,n,a){var s,o,u=360,h=r(a),l=parseFloat(a)*(h&&~a.indexOf("rad")?sr:1)-n,f=n+l+"deg";return h&&("short"===(s=a.split("_")[1])&&(l%=u)!==l%180&&(l+=l<0?u:-u),"cw"===s&&l<0?l=(l+36e9)%u-~~(l/u)*u:"ccw"===s&&0<l&&(l=(l-36e9)%u-~~(l/u)*u)),t._pt=o=new _e(t._pt,e,i,n,l,wd),o.e=f,o.u="deg",t._props.push(i),o}function we(t,e){for(var r in e)t[r]=e[r];return t}function xe(t,e,r){var i,n,a,s,o,u,h,l=we({},r._gsap),f=r.style;for(n in l.svg?(a=r.getAttribute("transform"),r.setAttribute("transform",""),f[cr]=e,i=kr(r,1),Xd(r,cr),r.setAttribute("transform",a)):(a=getComputedStyle(r)[cr],f[cr]=e,i=kr(r,1),f[cr]=a),ar)(a=l[n])!==(s=i[n])&&"perspective,force3D,transformOrigin,svgOrigin".indexOf(n)<0&&(o=Ya(a)!==(h=Ya(s))?_d(r,n,a,h):parseFloat(a),u=parseFloat(s),t._pt=new _e(t._pt,i,n,o,u-o,vd),t._pt.u=h||0,t._props.push(n));we(i,l)}var Ee,De,Re,Fe,Le,Ie,Ye,Be,qe=Lt.Power0,Ve=Lt.Power1,Ue=Lt.Power2,Xe=Lt.Power3,Ne=Lt.Power4,Ge=Lt.Linear,We=Lt.Quad,Qe=Lt.Cubic,Ke=Lt.Quart,Je=Lt.Quint,He=Lt.Strong,$e=Lt.Elastic,Ze=Lt.Back,tr=Lt.SteppedEase,er=Lt.Bounce,rr=Lt.Sine,ir=Lt.Expo,nr=Lt.Circ,ar={},sr=180/Math.PI,or=Math.PI/180,ur=Math.atan2,hr=/([A-Z])/g,lr=/(left|right|width|margin|padding|x)/i,fr=/[\s,\(]\S/,dr={autoAlpha:"opacity,visibility",scale:"scaleX,scaleY",alpha:"opacity"},cr="transform",pr=cr+"Origin",_r="O,Moz,ms,Ms,Webkit".split(","),mr=function _checkPropPrefix(t,e,r){var i=(e||Le).style,n=5;if(t in i&&!r)return t;for(t=t.charAt(0).toUpperCase()+t.substr(1);n--&&!(_r[n]+t in i););return n<0?null:(3===n?"ms":0<=n?_r[n]:"")+t},gr={deg:1,rad:1,turn:1},vr={grid:1,flex:1},yr=function _get(t,e,r,i){var n;return Fe||Sd(),e in dr&&"transform"!==e&&~(e=dr[e]).indexOf(",")&&(e=e.split(",")[0]),ar[e]&&"transform"!==e?(n=kr(t,i),n="transformOrigin"!==e?n[e]:n.svg?n.origin:Or(Pd(t,pr))+" "+n.zOrigin+"px"):(n=t.style[e])&&"auto"!==n&&!i&&!~(n+"").indexOf("calc(")||(n=br[e]&&br[e](t,e,r)||Pd(t,e)||ga(t,e)||("opacity"===e?1:0)),r&&!~(n+"").trim().indexOf(" ")?_d(t,e,n,r)+r:n},Tr={top:"0%",bottom:"100%",left:"0%",right:"100%",center:"50%"},br={clearProps:function clearProps(t,e,r,i,n){if("isFromStart"!==n.data){var a=t._pt=new _e(t._pt,e,r,0,0,ee);return a.u=i,a.pr=-10,a.tween=n,t._props.push(r),1}}},wr=[1,0,0,1,0,0],xr={},kr=function _parseTransform(t,e){var r=t._gsap||new Vt(t);if("x"in r&&!e&&!r.uncache)return r;var i,n,a,s,o,u,h,l,f,d,c,p,_,m,g,v,y,T,b,w,x,k,O,M,P,C,A,S,z,E,D,R,F=t.style,L=r.scaleX<0,I="deg",Y=getComputedStyle(t),B=Pd(t,pr)||"0";return i=n=a=u=h=l=f=d=c=0,s=o=1,r.svg=!(!t.getCTM||!Wd(t)),Y.translate&&("none"===Y.translate&&"none"===Y.scale&&"none"===Y.rotate||(F[cr]=("none"!==Y.translate?"translate3d("+(Y.translate+" 0 0").split(" ").slice(0,3).join(", ")+") ":"")+("none"!==Y.rotate?"rotate("+Y.rotate+") ":"")+("none"!==Y.scale?"scale("+Y.scale.split(" ").join(",")+") ":"")+("none"!==Y[cr]?Y[cr]:"")),F.scale=F.rotate=F.translate="none"),m=ke(t,r.svg),r.svg&&(M=r.uncache?(P=t.getBBox(),B=r.xOrigin-P.x+"px "+(r.yOrigin-P.y)+"px",""):!e&&t.getAttribute("data-svg-origin"),le(t,M||B,!!M||r.originIsAbsolute,!1!==r.smooth,m)),p=r.xOrigin||0,_=r.yOrigin||0,m!==wr&&(T=m[0],b=m[1],w=m[2],x=m[3],i=k=m[4],n=O=m[5],6===m.length?(s=Math.sqrt(T*T+b*b),o=Math.sqrt(x*x+w*w),u=T||b?ur(b,T)*sr:0,(f=w||x?ur(w,x)*sr+u:0)&&(o*=Math.abs(Math.cos(f*or))),r.svg&&(i-=p-(p*T+_*w),n-=_-(p*b+_*x))):(R=m[6],E=m[7],A=m[8],S=m[9],z=m[10],D=m[11],i=m[12],n=m[13],a=m[14],h=(g=ur(R,z))*sr,g&&(M=k*(v=Math.cos(-g))+A*(y=Math.sin(-g)),P=O*v+S*y,C=R*v+z*y,A=k*-y+A*v,S=O*-y+S*v,z=R*-y+z*v,D=E*-y+D*v,k=M,O=P,R=C),l=(g=ur(-w,z))*sr,g&&(v=Math.cos(-g),D=x*(y=Math.sin(-g))+D*v,T=M=T*v-A*y,b=P=b*v-S*y,w=C=w*v-z*y),u=(g=ur(b,T))*sr,g&&(M=T*(v=Math.cos(g))+b*(y=Math.sin(g)),P=k*v+O*y,b=b*v-T*y,O=O*v-k*y,T=M,k=P),h&&359.9<Math.abs(h)+Math.abs(u)&&(h=u=0,l=180-l),s=ia(Math.sqrt(T*T+b*b+w*w)),o=ia(Math.sqrt(O*O+R*R)),g=ur(k,O),f=2e-4<Math.abs(g)?g*sr:0,c=D?1/(D<0?-D:D):0),r.svg&&(M=t.getAttribute("transform"),r.forceCSS=t.setAttribute("transform","")||!ie(Pd(t,cr)),M&&t.setAttribute("transform",M))),90<Math.abs(f)&&Math.abs(f)<270&&(L?(s*=-1,f+=u<=0?180:-180,u+=u<=0?180:-180):(o*=-1,f+=f<=0?180:-180)),e=e||r.uncache,r.x=i-((r.xPercent=i&&(!e&&r.xPercent||(Math.round(t.offsetWidth/2)===Math.round(-i)?-50:0)))?t.offsetWidth*r.xPercent/100:0)+"px",r.y=n-((r.yPercent=n&&(!e&&r.yPercent||(Math.round(t.offsetHeight/2)===Math.round(-n)?-50:0)))?t.offsetHeight*r.yPercent/100:0)+"px",r.z=a+"px",r.scaleX=ia(s),r.scaleY=ia(o),r.rotation=ia(u)+I,r.rotationX=ia(h)+I,r.rotationY=ia(l)+I,r.skewX=f+I,r.skewY=d+I,r.transformPerspective=c+"px",(r.zOrigin=parseFloat(B.split(" ")[2])||!e&&r.zOrigin||0)&&(F[pr]=Or(B)),r.xOffset=r.yOffset=0,r.force3D=q.force3D,r.renderTransform=r.svg?zr:Be?Sr:Mr,r.uncache=0,r},Or=function _firstTwoOnly(t){return(t=t.split(" "))[0]+" "+t[1]},Mr=function _renderNon3DTransforms(t,e){e.z="0px",e.rotationY=e.rotationX="0deg",e.force3D=0,Sr(t,e)},Pr="0deg",Cr="0px",Ar=") ",Sr=function _renderCSSTransforms(t,e){var r=e||this,i=r.xPercent,n=r.yPercent,a=r.x,s=r.y,o=r.z,u=r.rotation,h=r.rotationY,l=r.rotationX,f=r.skewX,d=r.skewY,c=r.scaleX,p=r.scaleY,_=r.transformPerspective,m=r.force3D,g=r.target,v=r.zOrigin,y="",T="auto"===m&&t&&1!==t||!0===m;if(v&&(l!==Pr||h!==Pr)){var b,w=parseFloat(h)*or,x=Math.sin(w),k=Math.cos(w);w=parseFloat(l)*or,b=Math.cos(w),a=oe(g,a,x*b*-v),s=oe(g,s,-Math.sin(w)*-v),o=oe(g,o,k*b*-v+v)}_!==Cr&&(y+="perspective("+_+Ar),(i||n)&&(y+="translate("+i+"%, "+n+"%) "),!T&&a===Cr&&s===Cr&&o===Cr||(y+=o!==Cr||T?"translate3d("+a+", "+s+", "+o+") ":"translate("+a+", "+s+Ar),u!==Pr&&(y+="rotate("+u+Ar),h!==Pr&&(y+="rotateY("+h+Ar),l!==Pr&&(y+="rotateX("+l+Ar),f===Pr&&d===Pr||(y+="skew("+f+", "+d+Ar),1===c&&1===p||(y+="scale("+c+", "+p+Ar),g.style[cr]=y||"translate(0, 0)"},zr=function _renderSVGTransforms(t,e){var r,i,n,a,s,o=e||this,u=o.xPercent,h=o.yPercent,l=o.x,f=o.y,d=o.rotation,c=o.skewX,p=o.skewY,_=o.scaleX,m=o.scaleY,g=o.target,v=o.xOrigin,y=o.yOrigin,T=o.xOffset,b=o.yOffset,w=o.forceCSS,x=parseFloat(l),k=parseFloat(f);d=parseFloat(d),c=parseFloat(c),(p=parseFloat(p))&&(c+=p=parseFloat(p),d+=p),d||c?(d*=or,c*=or,r=Math.cos(d)*_,i=Math.sin(d)*_,n=Math.sin(d-c)*-m,a=Math.cos(d-c)*m,c&&(p*=or,s=Math.tan(c-p),n*=s=Math.sqrt(1+s*s),a*=s,p&&(s=Math.tan(p),r*=s=Math.sqrt(1+s*s),i*=s)),r=ia(r),i=ia(i),n=ia(n),a=ia(a)):(r=_,a=m,i=n=0),(x&&!~(l+"").indexOf("px")||k&&!~(f+"").indexOf("px"))&&(x=_d(g,"x",l,"px"),k=_d(g,"y",f,"px")),(v||y||T||b)&&(x=ia(x+v-(v*r+y*n)+T),k=ia(k+y-(v*i+y*a)+b)),(u||h)&&(s=g.getBBox(),x=ia(x+u/100*s.width),k=ia(k+h/100*s.height)),s="matrix("+r+","+i+","+n+","+a+","+x+","+k+")",g.setAttribute("transform",s),w&&(g.style[cr]=s)};ha("padding,margin,Width,Radius",function(e,r){var t="Right",i="Bottom",n="Left",o=(r<3?["Top",t,i,n]:["Top"+n,"Top"+t,i+t,i+n]).map(function(t){return r<2?e+t:"border"+t+e});br[1<r?"border"+e:e]=function(e,t,r,i,n){var a,s;if(arguments.length<4)return a=o.map(function(t){return yr(e,t,r)}),5===(s=a.join(" ")).split(a[0]).length?a[0]:s;a=(i+"").split(" "),s={},o.forEach(function(t,e){return s[t]=a[e]=a[e]||a[(e-1)/2|0]}),e.init(t,s,n)}});var Er,Dr,Rr,Fr={name:"css",register:Sd,targetTest:function targetTest(t){return t.style&&t.nodeType},init:function init(t,e,i,n,a){var s,o,u,h,l,f,d,c,p,_,m,g,v,y,T,b,w=this._props,x=t.style,k=i.vars.startAt;for(d in Fe||Sd(),this.styles=this.styles||Md(t),b=this.styles.props,this.tween=i,e)if("autoRound"!==d&&(o=e[d],!pt[d]||!ac(d,e,i,n,t,a)))if(l=typeof o,f=br[d],"function"===l&&(l=typeof(o=o.call(i,n,t,a))),"string"===l&&~o.indexOf("random(")&&(o=ob(o)),f)f(this,t,d,o,i)&&(T=1);else if("--"===d.substr(0,2))s=(getComputedStyle(t).getPropertyValue(d)+"").trim(),o+="",Et.lastIndex=0,Et.test(s)||(c=Ya(s),p=Ya(o)),p?c!==p&&(s=_d(t,d,s,p)+p):c&&(o+=c),this.add(x,"setProperty",s,o,n,a,0,0,d),w.push(d),b.push(d,0,x[d]);else if("undefined"!==l){if(k&&d in k?(s="function"==typeof k[d]?k[d].call(i,n,t,a):k[d],r(s)&&~s.indexOf("random(")&&(s=ob(s)),Ya(s+"")||"auto"===s||(s+=q.units[d]||Ya(yr(t,d))||""),"="===(s+"").charAt(1)&&(s=yr(t,d))):s=yr(t,d),h=parseFloat(s),(_="string"===l&&"="===o.charAt(1)&&o.substr(0,2))&&(o=o.substr(2)),u=parseFloat(o),d in dr&&("autoAlpha"===d&&(1===h&&"hidden"===yr(t,"visibility")&&u&&(h=0),b.push("visibility",0,x.visibility),Yd(this,x,"visibility",h?"inherit":"hidden",u?"inherit":"hidden",!u)),"scale"!==d&&"transform"!==d&&~(d=dr[d]).indexOf(",")&&(d=d.split(",")[0])),m=d in ar)if(this.styles.save(d),g||((v=t._gsap).renderTransform&&!e.parseTransform||kr(t,e.parseTransform),y=!1!==e.smoothOrigin&&v.smooth,(g=this._pt=new _e(this._pt,x,cr,0,1,v.renderTransform,v,0,-1)).dep=1),"scale"===d)this._pt=new _e(this._pt,v,"scaleY",v.scaleY,(_?ka(v.scaleY,_+u):u)-v.scaleY||0,vd),this._pt.u=0,w.push("scaleY",d),d+="X";else{if("transformOrigin"===d){b.push(pr,0,x[pr]),o=de(o),v.svg?le(t,o,0,y,0,this):((p=parseFloat(o.split(" ")[2])||0)!==v.zOrigin&&Yd(this,v,"zOrigin",v.zOrigin,p),Yd(this,x,d,Or(s),Or(o)));continue}if("svgOrigin"===d){le(t,o,1,y,0,this);continue}if(d in xr){ve(this,v,d,h,_?ka(h,_+o):o);continue}if("smoothOrigin"===d){Yd(this,v,"smooth",v.smooth,o);continue}if("force3D"===d){v[d]=o;continue}if("transform"===d){xe(this,o,t);continue}}else d in x||(d=mr(d)||d);if(m||(u||0===u)&&(h||0===h)&&!fr.test(o)&&d in x)u=u||0,(c=(s+"").substr((h+"").length))!==(p=Ya(o)||(d in q.units?q.units[d]:c))&&(h=_d(t,d,s,p)),this._pt=new _e(this._pt,m?v:x,d,h,(_?ka(h,_+u):u)-h,m||"px"!==p&&"zIndex"!==d||!1===e.autoRound?vd:yd),this._pt.u=p||0,c!==p&&"%"!==p&&(this._pt.b=s,this._pt.r=xd);else if(d in x)be.call(this,t,d,s,_?_+o:o);else if(d in t)this.add(t,d,s||t[d],_?_+o:o,n,a);else if("parseTransform"!==d){Q(d,o);continue}m||(d in x?b.push(d,0,x[d]):"function"==typeof t[d]?b.push(d,2,t[d]()):b.push(d,1,s||t[d])),w.push(d)}T&&pe(this)},render:function render(t,e){if(e.tween._time||!Ye())for(var r=e._pt;r;)r.r(t,r.d),r=r._next;else e.styles.revert()},get:yr,aliases:dr,getSetter:function getSetter(t,e,r){var i=dr[e];return i&&i.indexOf(",")<0&&(e=i),e in ar&&e!==pr&&(t._gsap.x||yr(t,"x"))?r&&Ie===r?"scale"===e?Ed:Dd:(Ie=r||{})&&("scale"===e?Fd:Gd):t.style&&!u(t.style[e])?Bd:~e.indexOf("-")?Cd:ne(t,e)},core:{_removeProperty:Xd,_getMatrix:ke}};ze.utils.checkPrefix=mr,ze.core.getStyleSaver=Md,Rr=ha((Er="x,y,z,scale,scaleX,scaleY,xPercent,yPercent")+","+(Dr="rotation,rotationX,rotationY,skewX,skewY")+",transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective",function(t){ar[t]=1}),ha(Dr,function(t){q.units[t]="deg",xr[t]=1}),dr[Rr[13]]=Er+","+Dr,ha("0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY",function(t){var e=t.split(":");dr[e[1]]=Rr[e[0]]}),ha("x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective",function(t){q.units[t]="px"}),ze.registerPlugin(Fr);var Lr=ze.registerPlugin(Fr)||ze,Ir=Lr.core.Tween;e.Back=Ze,e.Bounce=er,e.CSSPlugin=Fr,e.Circ=nr,e.Cubic=Qe,e.Elastic=$e,e.Expo=ir,e.Linear=Ge,e.Power0=qe,e.Power1=Ve,e.Power2=Ue,e.Power3=Xe,e.Power4=Ne,e.Quad=We,e.Quart=Ke,e.Quint=Je,e.Sine=rr,e.SteppedEase=tr,e.Strong=He,e.TimelineLite=Xt,e.TimelineMax=Xt,e.TweenLite=$t,e.TweenMax=Ir,e.default=Lr,e.gsap=Lr;if (typeof(window)==="undefined"||window!==e){Object.defineProperty(e,"__esModule",{value:!0})} else {delete e.default}}); + diff --git a/node_modules/gsap/dist/gsap.min.js.map b/node_modules/gsap/dist/gsap.min.js.map new file mode 100644 index 0000000000000000000000000000000000000000..206ceb208ba79deecc50b00819e06b572d58c0a2 --- /dev/null +++ b/node_modules/gsap/dist/gsap.min.js.map @@ -0,0 +1 @@ +{"version":3,"file":"gsap.min.js","sources":["../src/gsap-core.js","../src/CSSPlugin.js","../src/index.js"],"sourcesContent":["/*!\n * GSAP 3.12.7\n * https://gsap.com\n *\n * @license Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nlet _config = {\n\t\tautoSleep: 120,\n\t\tforce3D: \"auto\",\n\t\tnullTargetWarn: 1,\n\t\tunits: {lineHeight:\"\"}\n\t},\n\t_defaults = {\n\t\tduration: .5,\n\t\toverwrite: false,\n\t\tdelay: 0\n\t},\n\t_suppressOverwrites,\n\t_reverting, _context,\n\t_bigNum = 1e8,\n\t_tinyNum = 1 / _bigNum,\n\t_2PI = Math.PI * 2,\n\t_HALF_PI = _2PI / 4,\n\t_gsID = 0,\n\t_sqrt = Math.sqrt,\n\t_cos = Math.cos,\n\t_sin = Math.sin,\n\t_isString = value => typeof(value) === \"string\",\n\t_isFunction = value => typeof(value) === \"function\",\n\t_isNumber = value => typeof(value) === \"number\",\n\t_isUndefined = value => typeof(value) === \"undefined\",\n\t_isObject = value => typeof(value) === \"object\",\n\t_isNotFalse = value => value !== false,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_isFuncOrString = value => _isFunction(value) || _isString(value),\n\t_isTypedArray = (typeof ArrayBuffer === \"function\" && ArrayBuffer.isView) || function() {}, // note: IE10 has ArrayBuffer, but NOT ArrayBuffer.isView().\n\t_isArray = Array.isArray,\n\t_strictNumExp = /(?:-?\\.?\\d|\\.)+/gi, //only numbers (including negatives and decimals) but NOT relative values.\n\t_numExp = /[-+=.]*\\d+[.e\\-+]*\\d*[e\\-+]*\\d*/g, //finds any numbers, including ones that start with += or -=, negative numbers, and ones in scientific notation like 1e-8.\n\t_numWithUnitExp = /[-+=.]*\\d+[.e-]*\\d*[a-z%]*/g,\n\t_complexStringNumExp = /[-+=.]*\\d+\\.?\\d*(?:e-|e\\+)?\\d*/gi, //duplicate so that while we're looping through matches from exec(), it doesn't contaminate the lastIndex of _numExp which we use to search for colors too.\n\t_relExp = /[+-]=-?[.\\d]+/,\n\t_delimitedValueExp = /[^,'\"\\[\\]\\s]+/gi, // previously /[#\\-+.]*\\b[a-z\\d\\-=+%.]+/gi but didn't catch special characters.\n\t_unitExp = /^[+\\-=e\\s\\d]*\\d+[.\\d]*([a-z]*|%)\\s*$/i,\n\t_globalTimeline, _win, _coreInitted, _doc,\n\t_globals = {},\n\t_installScope = {},\n\t_coreReady,\n\t_install = scope => (_installScope = _merge(scope, _globals)) && gsap,\n\t_missingPlugin = (property, value) => console.warn(\"Invalid property\", property, \"set to\", value, \"Missing plugin? gsap.registerPlugin()\"),\n\t_warn = (message, suppress) => !suppress && console.warn(message),\n\t_addGlobal = (name, obj) => (name && (_globals[name] = obj) && (_installScope && (_installScope[name] = obj))) || _globals,\n\t_emptyFunc = () => 0,\n\t_startAtRevertConfig = {suppressEvents: true, isStart: true, kill: false},\n\t_revertConfigNoKill = {suppressEvents: true, kill: false},\n\t_revertConfig = {suppressEvents: true},\n\t_reservedProps = {},\n\t_lazyTweens = [],\n\t_lazyLookup = {},\n\t_lastRenderedFrame,\n\t_plugins = {},\n\t_effects = {},\n\t_nextGCFrame = 30,\n\t_harnessPlugins = [],\n\t_callbackNames = \"\",\n\t_harness = targets => {\n\t\tlet target = targets[0],\n\t\t\tharnessPlugin, i;\n\t\t_isObject(target) || _isFunction(target) || (targets = [targets]);\n\t\tif (!(harnessPlugin = (target._gsap || {}).harness)) { // find the first target with a harness. We assume targets passed into an animation will be of similar type, meaning the same kind of harness can be used for them all (performance optimization)\n\t\t\ti = _harnessPlugins.length;\n\t\t\twhile (i-- && !_harnessPlugins[i].targetTest(target)) {\t}\n\t\t\tharnessPlugin = _harnessPlugins[i];\n\t\t}\n\t\ti = targets.length;\n\t\twhile (i--) {\n\t\t\t(targets[i] && (targets[i]._gsap || (targets[i]._gsap = new GSCache(targets[i], harnessPlugin)))) || targets.splice(i, 1);\n\t\t}\n\t\treturn targets;\n\t},\n\t_getCache = target => target._gsap || _harness(toArray(target))[0]._gsap,\n\t_getProperty = (target, property, v) => (v = target[property]) && _isFunction(v) ? target[property]() : (_isUndefined(v) && target.getAttribute && target.getAttribute(property)) || v,\n\t_forEachName = (names, func) => ((names = names.split(\",\")).forEach(func)) || names, //split a comma-delimited list of names into an array, then run a forEach() function and return the split array (this is just a way to consolidate/shorten some code).\n\t_round = value => Math.round(value * 100000) / 100000 || 0,\n\t_roundPrecise = value => Math.round(value * 10000000) / 10000000 || 0, // increased precision mostly for timing values.\n\t_parseRelative = (start, value) => {\n\t\tlet operator = value.charAt(0),\n\t\t\tend = parseFloat(value.substr(2));\n\t\tstart = parseFloat(start);\n\t\treturn operator === \"+\" ? start + end : operator === \"-\" ? start - end : operator === \"*\" ? start * end : start / end;\n\t},\n\t_arrayContainsAny = (toSearch, toFind) => { //searches one array to find matches for any of the items in the toFind array. As soon as one is found, it returns true. It does NOT return all the matches; it's simply a boolean search.\n\t\tlet l = toFind.length,\n\t\t\ti = 0;\n\t\tfor (; toSearch.indexOf(toFind[i]) < 0 && ++i < l;) { }\n\t\treturn (i < l);\n\t},\n\t_lazyRender = () => {\n\t\tlet l = _lazyTweens.length,\n\t\t\ta = _lazyTweens.slice(0),\n\t\t\ti, tween;\n\t\t_lazyLookup = {};\n\t\t_lazyTweens.length = 0;\n\t\tfor (i = 0; i < l; i++) {\n\t\t\ttween = a[i];\n\t\t\ttween && tween._lazy && (tween.render(tween._lazy[0], tween._lazy[1], true)._lazy = 0);\n\t\t}\n\t},\n\t_lazySafeRender = (animation, time, suppressEvents, force) => {\n\t\t_lazyTweens.length && !_reverting && _lazyRender();\n\t\tanimation.render(time, suppressEvents, force || (_reverting && time < 0 && (animation._initted || animation._startAt)));\n\t\t_lazyTweens.length && !_reverting && _lazyRender(); //in case rendering caused any tweens to lazy-init, we should render them because typically when someone calls seek() or time() or progress(), they expect an immediate render.\n\t},\n\t_numericIfPossible = value => {\n\t\tlet n = parseFloat(value);\n\t\treturn (n || n === 0) && (value + \"\").match(_delimitedValueExp).length < 2 ? n : _isString(value) ? value.trim() : value;\n\t},\n\t_passThrough = p => p,\n\t_setDefaults = (obj, defaults) => {\n\t\tfor (let p in defaults) {\n\t\t\t(p in obj) || (obj[p] = defaults[p]);\n\t\t}\n\t\treturn obj;\n\t},\n\t_setKeyframeDefaults = excludeDuration => (obj, defaults) => {\n\t\tfor (let p in defaults) {\n\t\t\t(p in obj) || (p === \"duration\" && excludeDuration) || p === \"ease\" || (obj[p] = defaults[p]);\n\t\t}\n\t},\n\t_merge = (base, toMerge) => {\n\t\tfor (let p in toMerge) {\n\t\t\tbase[p] = toMerge[p];\n\t\t}\n\t\treturn base;\n\t},\n\t_mergeDeep = (base, toMerge) => {\n\t\tfor (let p in toMerge) {\n\t\t\tp !== \"__proto__\" && p !== \"constructor\" && p !== \"prototype\" && (base[p] = _isObject(toMerge[p]) ? _mergeDeep(base[p] || (base[p] = {}), toMerge[p]) : toMerge[p]);\n\t\t}\n\t\treturn base;\n\t},\n\t_copyExcluding = (obj, excluding) => {\n\t\tlet copy = {},\n\t\t\tp;\n\t\tfor (p in obj) {\n\t\t\t(p in excluding) || (copy[p] = obj[p]);\n\t\t}\n\t\treturn copy;\n\t},\n\t_inheritDefaults = vars => {\n\t\tlet parent = vars.parent || _globalTimeline,\n\t\t\tfunc = vars.keyframes ? _setKeyframeDefaults(_isArray(vars.keyframes)) : _setDefaults;\n\t\tif (_isNotFalse(vars.inherit)) {\n\t\t\twhile (parent) {\n\t\t\t\tfunc(vars, parent.vars.defaults);\n\t\t\t\tparent = parent.parent || parent._dp;\n\t\t\t}\n\t\t}\n\t\treturn vars;\n\t},\n\t_arraysMatch = (a1, a2) => {\n\t\tlet i = a1.length,\n\t\t\tmatch = i === a2.length;\n\t\twhile (match && i-- && a1[i] === a2[i]) { }\n\t\treturn i < 0;\n\t},\n\t_addLinkedListItem = (parent, child, firstProp = \"_first\", lastProp = \"_last\", sortBy) => {\n\t\tlet prev = parent[lastProp],\n\t\t\tt;\n\t\tif (sortBy) {\n\t\t\tt = child[sortBy];\n\t\t\twhile (prev && prev[sortBy] > t) {\n\t\t\t\tprev = prev._prev;\n\t\t\t}\n\t\t}\n\t\tif (prev) {\n\t\t\tchild._next = prev._next;\n\t\t\tprev._next = child;\n\t\t} else {\n\t\t\tchild._next = parent[firstProp];\n\t\t\tparent[firstProp] = child;\n\t\t}\n\t\tif (child._next) {\n\t\t\tchild._next._prev = child;\n\t\t} else {\n\t\t\tparent[lastProp] = child;\n\t\t}\n\t\tchild._prev = prev;\n\t\tchild.parent = child._dp = parent;\n\t\treturn child;\n\t},\n\t_removeLinkedListItem = (parent, child, firstProp = \"_first\", lastProp = \"_last\") => {\n\t\tlet prev = child._prev,\n\t\t\tnext = child._next;\n\t\tif (prev) {\n\t\t\tprev._next = next;\n\t\t} else if (parent[firstProp] === child) {\n\t\t\tparent[firstProp] = next;\n\t\t}\n\t\tif (next) {\n\t\t\tnext._prev = prev;\n\t\t} else if (parent[lastProp] === child) {\n\t\t\tparent[lastProp] = prev;\n\t\t}\n\t\tchild._next = child._prev = child.parent = null; // don't delete the _dp just so we can revert if necessary. But parent should be null to indicate the item isn't in a linked list.\n\t},\n\t_removeFromParent = (child, onlyIfParentHasAutoRemove) => {\n\t\tchild.parent && (!onlyIfParentHasAutoRemove || child.parent.autoRemoveChildren) && child.parent.remove && child.parent.remove(child);\n\t\tchild._act = 0;\n\t},\n\t_uncache = (animation, child) => {\n\t\tif (animation && (!child || child._end > animation._dur || child._start < 0)) { // performance optimization: if a child animation is passed in we should only uncache if that child EXTENDS the animation (its end time is beyond the end)\n\t\t\tlet a = animation;\n\t\t\twhile (a) {\n\t\t\t\ta._dirty = 1;\n\t\t\t\ta = a.parent;\n\t\t\t}\n\t\t}\n\t\treturn animation;\n\t},\n\t_recacheAncestors = animation => {\n\t\tlet parent = animation.parent;\n\t\twhile (parent && parent.parent) { //sometimes we must force a re-sort of all children and update the duration/totalDuration of all ancestor timelines immediately in case, for example, in the middle of a render loop, one tween alters another tween's timeScale which shoves its startTime before 0, forcing the parent timeline to shift around and shiftChildren() which could affect that next tween's render (startTime). Doesn't matter for the root timeline though.\n\t\t\tparent._dirty = 1;\n\t\t\tparent.totalDuration();\n\t\t\tparent = parent.parent;\n\t\t}\n\t\treturn animation;\n\t},\n\t_rewindStartAt = (tween, totalTime, suppressEvents, force) => tween._startAt && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : (tween.vars.immediateRender && !tween.vars.autoRevert) || tween._startAt.render(totalTime, true, force)),\n\t_hasNoPausedAncestors = animation => !animation || (animation._ts && _hasNoPausedAncestors(animation.parent)),\n\t_elapsedCycleDuration = animation => animation._repeat ? _animationCycle(animation._tTime, (animation = animation.duration() + animation._rDelay)) * animation : 0,\n\t// feed in the totalTime and cycleDuration and it'll return the cycle (iteration minus 1) and if the playhead is exactly at the very END, it will NOT bump up to the next cycle.\n\t_animationCycle = (tTime, cycleDuration) => {\n\t\tlet whole = Math.floor(tTime = _roundPrecise(tTime / cycleDuration));\n\t\treturn tTime && (whole === tTime) ? whole - 1 : whole;\n\t},\n\t_parentToChildTotalTime = (parentTime, child) => (parentTime - child._start) * child._ts + (child._ts >= 0 ? 0 : (child._dirty ? child.totalDuration() : child._tDur)),\n\t_setEnd = animation => (animation._end = _roundPrecise(animation._start + ((animation._tDur / Math.abs(animation._ts || animation._rts || _tinyNum)) || 0))),\n\t_alignPlayhead = (animation, totalTime) => { // adjusts the animation's _start and _end according to the provided totalTime (only if the parent's smoothChildTiming is true and the animation isn't paused). It doesn't do any rendering or forcing things back into parent timelines, etc. - that's what totalTime() is for.\n\t\tlet parent = animation._dp;\n\t\tif (parent && parent.smoothChildTiming && animation._ts) {\n\t\t\tanimation._start = _roundPrecise(parent._time - (animation._ts > 0 ? totalTime / animation._ts : ((animation._dirty ? animation.totalDuration() : animation._tDur) - totalTime) / -animation._ts));\n\t\t\t_setEnd(animation);\n\t\t\tparent._dirty || _uncache(parent, animation); //for performance improvement. If the parent's cache is already dirty, it already took care of marking the ancestors as dirty too, so skip the function call here.\n\t\t}\n\t\treturn animation;\n\t},\n\t/*\n\t_totalTimeToTime = (clampedTotalTime, duration, repeat, repeatDelay, yoyo) => {\n\t\tlet cycleDuration = duration + repeatDelay,\n\t\t\ttime = _round(clampedTotalTime % cycleDuration);\n\t\tif (time > duration) {\n\t\t\ttime = duration;\n\t\t}\n\t\treturn (yoyo && (~~(clampedTotalTime / cycleDuration) & 1)) ? duration - time : time;\n\t},\n\t*/\n\t_postAddChecks = (timeline, child) => {\n\t\tlet t;\n\t\tif (child._time || (!child._dur && child._initted) || (child._start < timeline._time && (child._dur || !child.add))) { // in case, for example, the _start is moved on a tween that has already rendered, or if it's being inserted into a timeline BEFORE where the playhead is currently. Imagine it's at its end state, then the startTime is moved WAY later (after the end of this timeline), it should render at its beginning. Special case: if it's a timeline (has .add() method) and no duration, we can skip rendering because the user may be populating it AFTER adding it to a parent timeline (unconventional, but possible, and we wouldn't want it to get removed if the parent's autoRemoveChildren is true).\n\t\t\tt = _parentToChildTotalTime(timeline.rawTime(), child);\n\t\t\tif (!child._dur || _clamp(0, child.totalDuration(), t) - child._tTime > _tinyNum) {\n\t\t\t\tchild.render(t, true);\n\t\t\t}\n\t\t}\n\t\t//if the timeline has already ended but the inserted tween/timeline extends the duration, we should enable this timeline again so that it renders properly. We should also align the playhead with the parent timeline's when appropriate.\n\t\tif (_uncache(timeline, child)._dp && timeline._initted && timeline._time >= timeline._dur && timeline._ts) {\n\t\t\t//in case any of the ancestors had completed but should now be enabled...\n\t\t\tif (timeline._dur < timeline.duration()) {\n\t\t\t\tt = timeline;\n\t\t\t\twhile (t._dp) {\n\t\t\t\t\t(t.rawTime() >= 0) && t.totalTime(t._tTime); //moves the timeline (shifts its startTime) if necessary, and also enables it. If it's currently zero, though, it may not be scheduled to render until later so there's no need to force it to align with the current playhead position. Only move to catch up with the playhead.\n\t\t\t\t\tt = t._dp;\n\t\t\t\t}\n\t\t\t}\n\t\t\ttimeline._zTime = -_tinyNum; // helps ensure that the next render() will be forced (crossingStart = true in render()), even if the duration hasn't changed (we're adding a child which would need to get rendered). Definitely an edge case. Note: we MUST do this AFTER the loop above where the totalTime() might trigger a render() because this _addToTimeline() method gets called from the Animation constructor, BEFORE tweens even record their targets, etc. so we wouldn't want things to get triggered in the wrong order.\n\t\t}\n\t},\n\t_addToTimeline = (timeline, child, position, skipChecks) => {\n\t\tchild.parent && _removeFromParent(child);\n\t\tchild._start = _roundPrecise((_isNumber(position) ? position : position || timeline !== _globalTimeline ? _parsePosition(timeline, position, child) : timeline._time) + child._delay);\n\t\tchild._end = _roundPrecise(child._start + ((child.totalDuration() / Math.abs(child.timeScale())) || 0));\n\t\t_addLinkedListItem(timeline, child, \"_first\", \"_last\", timeline._sort ? \"_start\" : 0);\n\t\t_isFromOrFromStart(child) || (timeline._recent = child);\n\t\tskipChecks || _postAddChecks(timeline, child);\n\t\ttimeline._ts < 0 && _alignPlayhead(timeline, timeline._tTime); // if the timeline is reversed and the new child makes it longer, we may need to adjust the parent's _start (push it back)\n\t\treturn timeline;\n\t},\n\t_scrollTrigger = (animation, trigger) => (_globals.ScrollTrigger || _missingPlugin(\"scrollTrigger\", trigger)) && _globals.ScrollTrigger.create(trigger, animation),\n\t_attemptInitTween = (tween, time, force, suppressEvents, tTime) => {\n\t\t_initTween(tween, time, tTime);\n\t\tif (!tween._initted) {\n\t\t\treturn 1;\n\t\t}\n\t\tif (!force && tween._pt && !_reverting && ((tween._dur && tween.vars.lazy !== false) || (!tween._dur && tween.vars.lazy)) && _lastRenderedFrame !== _ticker.frame) {\n\t\t\t_lazyTweens.push(tween);\n\t\t\ttween._lazy = [tTime, suppressEvents];\n\t\t\treturn 1;\n\t\t}\n\t},\n\t_parentPlayheadIsBeforeStart = ({parent}) => parent && parent._ts && parent._initted && !parent._lock && (parent.rawTime() < 0 || _parentPlayheadIsBeforeStart(parent)), // check parent's _lock because when a timeline repeats/yoyos and does its artificial wrapping, we shouldn't force the ratio back to 0\n\t_isFromOrFromStart = ({data}) => data === \"isFromStart\" || data === \"isStart\",\n\t_renderZeroDurationTween = (tween, totalTime, suppressEvents, force) => {\n\t\tlet prevRatio = tween.ratio,\n\t\t\tratio = totalTime < 0 || (!totalTime && ((!tween._start && _parentPlayheadIsBeforeStart(tween) && !(!tween._initted && _isFromOrFromStart(tween))) || ((tween._ts < 0 || tween._dp._ts < 0) && !_isFromOrFromStart(tween)))) ? 0 : 1, // if the tween or its parent is reversed and the totalTime is 0, we should go to a ratio of 0. Edge case: if a from() or fromTo() stagger tween is placed later in a timeline, the \"startAt\" zero-duration tween could initially render at a time when the parent timeline's playhead is technically BEFORE where this tween is, so make sure that any \"from\" and \"fromTo\" startAt tweens are rendered the first time at a ratio of 1.\n\t\t\trepeatDelay = tween._rDelay,\n\t\t\ttTime = 0,\n\t\t\tpt, iteration, prevIteration;\n\t\tif (repeatDelay && tween._repeat) { // in case there's a zero-duration tween that has a repeat with a repeatDelay\n\t\t\ttTime = _clamp(0, tween._tDur, totalTime);\n\t\t\titeration = _animationCycle(tTime, repeatDelay);\n\t\t\ttween._yoyo && (iteration & 1) && (ratio = 1 - ratio);\n\t\t\tif (iteration !== _animationCycle(tween._tTime, repeatDelay)) { // if iteration changed\n\t\t\t\tprevRatio = 1 - ratio;\n\t\t\t\ttween.vars.repeatRefresh && tween._initted && tween.invalidate();\n\t\t\t}\n\t\t}\n\t\tif (ratio !== prevRatio || _reverting || force || tween._zTime === _tinyNum || (!totalTime && tween._zTime)) {\n\t\t\tif (!tween._initted && _attemptInitTween(tween, totalTime, force, suppressEvents, tTime)) { // if we render the very beginning (time == 0) of a fromTo(), we must force the render (normal tweens wouldn't need to render at a time of 0 when the prevTime was also 0). This is also mandatory to make sure overwriting kicks in immediately.\n\t\t\t\treturn;\n\t\t\t}\n\t\t\tprevIteration = tween._zTime;\n\t\t\ttween._zTime = totalTime || (suppressEvents ? _tinyNum : 0); // when the playhead arrives at EXACTLY time 0 (right on top) of a zero-duration tween, we need to discern if events are suppressed so that when the playhead moves again (next time), it'll trigger the callback. If events are NOT suppressed, obviously the callback would be triggered in this render. Basically, the callback should fire either when the playhead ARRIVES or LEAVES this exact spot, not both. Imagine doing a timeline.seek(0) and there's a callback that sits at 0. Since events are suppressed on that seek() by default, nothing will fire, but when the playhead moves off of that position, the callback should fire. This behavior is what people intuitively expect.\n\t\t\tsuppressEvents || (suppressEvents = totalTime && !prevIteration); // if it was rendered previously at exactly 0 (_zTime) and now the playhead is moving away, DON'T fire callbacks otherwise they'll seem like duplicates.\n\t\t\ttween.ratio = ratio;\n\t\t\ttween._from && (ratio = 1 - ratio);\n\t\t\ttween._time = 0;\n\t\t\ttween._tTime = tTime;\n\t\t\tpt = tween._pt;\n\t\t\twhile (pt) {\n\t\t\t\tpt.r(ratio, pt.d);\n\t\t\t\tpt = pt._next;\n\t\t\t}\n\t\t\ttotalTime < 0 && _rewindStartAt(tween, totalTime, suppressEvents, true);\n\t\t\ttween._onUpdate && !suppressEvents && _callback(tween, \"onUpdate\");\n\t\t\ttTime && tween._repeat && !suppressEvents && tween.parent && _callback(tween, \"onRepeat\");\n\t\t\tif ((totalTime >= tween._tDur || totalTime < 0) && tween.ratio === ratio) {\n\t\t\t\tratio && _removeFromParent(tween, 1);\n\t\t\t\tif (!suppressEvents && !_reverting) {\n\t\t\t\t\t_callback(tween, (ratio ? \"onComplete\" : \"onReverseComplete\"), true);\n\t\t\t\t\ttween._prom && tween._prom();\n\t\t\t\t}\n\t\t\t}\n\t\t} else if (!tween._zTime) {\n\t\t\ttween._zTime = totalTime;\n\t\t}\n\t},\n\t_findNextPauseTween = (animation, prevTime, time) => {\n\t\tlet child;\n\t\tif (time > prevTime) {\n\t\t\tchild = animation._first;\n\t\t\twhile (child && child._start <= time) {\n\t\t\t\tif (child.data === \"isPause\" && child._start > prevTime) {\n\t\t\t\t\treturn child;\n\t\t\t\t}\n\t\t\t\tchild = child._next;\n\t\t\t}\n\t\t} else {\n\t\t\tchild = animation._last;\n\t\t\twhile (child && child._start >= time) {\n\t\t\t\tif (child.data === \"isPause\" && child._start < prevTime) {\n\t\t\t\t\treturn child;\n\t\t\t\t}\n\t\t\t\tchild = child._prev;\n\t\t\t}\n\t\t}\n\t},\n\t_setDuration = (animation, duration, skipUncache, leavePlayhead) => {\n\t\tlet repeat = animation._repeat,\n\t\t\tdur = _roundPrecise(duration) || 0,\n\t\t\ttotalProgress = animation._tTime / animation._tDur;\n\t\ttotalProgress && !leavePlayhead && (animation._time *= dur / animation._dur);\n\t\tanimation._dur = dur;\n\t\tanimation._tDur = !repeat ? dur : repeat < 0 ? 1e10 : _roundPrecise(dur * (repeat + 1) + (animation._rDelay * repeat));\n\t\ttotalProgress > 0 && !leavePlayhead && _alignPlayhead(animation, (animation._tTime = animation._tDur * totalProgress));\n\t\tanimation.parent && _setEnd(animation);\n\t\tskipUncache || _uncache(animation.parent, animation);\n\t\treturn animation;\n\t},\n\t_onUpdateTotalDuration = animation => (animation instanceof Timeline) ? _uncache(animation) : _setDuration(animation, animation._dur),\n\t_zeroPosition = {_start:0, endTime:_emptyFunc, totalDuration:_emptyFunc},\n\t_parsePosition = (animation, position, percentAnimation) => {\n\t\tlet labels = animation.labels,\n\t\t\trecent = animation._recent || _zeroPosition,\n\t\t\tclippedDuration = animation.duration() >= _bigNum ? recent.endTime(false) : animation._dur, //in case there's a child that infinitely repeats, users almost never intend for the insertion point of a new child to be based on a SUPER long value like that so we clip it and assume the most recently-added child's endTime should be used instead.\n\t\t\ti, offset, isPercent;\n\t\tif (_isString(position) && (isNaN(position) || (position in labels))) { //if the string is a number like \"1\", check to see if there's a label with that name, otherwise interpret it as a number (absolute value).\n\t\t\toffset = position.charAt(0);\n\t\t\tisPercent = position.substr(-1) === \"%\";\n\t\t\ti = position.indexOf(\"=\");\n\t\t\tif (offset === \"<\" || offset === \">\") {\n\t\t\t\ti >= 0 && (position = position.replace(/=/, \"\"));\n\t\t\t\treturn (offset === \"<\" ? recent._start : recent.endTime(recent._repeat >= 0)) + (parseFloat(position.substr(1)) || 0) * (isPercent ? (i < 0 ? recent : percentAnimation).totalDuration() / 100 : 1);\n\t\t\t}\n\t\t\tif (i < 0) {\n\t\t\t\t(position in labels) || (labels[position] = clippedDuration);\n\t\t\t\treturn labels[position];\n\t\t\t}\n\t\t\toffset = parseFloat(position.charAt(i-1) + position.substr(i+1));\n\t\t\tif (isPercent && percentAnimation) {\n\t\t\t\toffset = offset / 100 * (_isArray(percentAnimation) ? percentAnimation[0] : percentAnimation).totalDuration();\n\t\t\t}\n\t\t\treturn (i > 1) ? _parsePosition(animation, position.substr(0, i-1), percentAnimation) + offset : clippedDuration + offset;\n\t\t}\n\t\treturn (position == null) ? clippedDuration : +position;\n\t},\n\t_createTweenType = (type, params, timeline) => {\n\t\tlet isLegacy = _isNumber(params[1]),\n\t\t\tvarsIndex = (isLegacy ? 2 : 1) + (type < 2 ? 0 : 1),\n\t\t\tvars = params[varsIndex],\n\t\t\tirVars, parent;\n\t\tisLegacy && (vars.duration = params[1]);\n\t\tvars.parent = timeline;\n\t\tif (type) {\n\t\t\tirVars = vars;\n\t\t\tparent = timeline;\n\t\t\twhile (parent && !(\"immediateRender\" in irVars)) { // inheritance hasn't happened yet, but someone may have set a default in an ancestor timeline. We could do vars.immediateRender = _isNotFalse(_inheritDefaults(vars).immediateRender) but that'd exact a slight performance penalty because _inheritDefaults() also runs in the Tween constructor. We're paying a small kb price here to gain speed.\n\t\t\t\tirVars = parent.vars.defaults || {};\n\t\t\t\tparent = _isNotFalse(parent.vars.inherit) && parent.parent;\n\t\t\t}\n\t\t\tvars.immediateRender = _isNotFalse(irVars.immediateRender);\n\t\t\ttype < 2 ? (vars.runBackwards = 1) : (vars.startAt = params[varsIndex - 1]); // \"from\" vars\n\t\t}\n\t\treturn new Tween(params[0], vars, params[varsIndex + 1]);\n\t},\n\t_conditionalReturn = (value, func) => value || value === 0 ? func(value) : func,\n\t_clamp = (min, max, value) => value < min ? min : value > max ? max : value,\n\tgetUnit = (value, v) => !_isString(value) || !(v = _unitExp.exec(value)) ? \"\" : v[1], // note: protect against padded numbers as strings, like \"100.100\". That shouldn't return \"00\" as the unit. If it's numeric, return no unit.\n\tclamp = (min, max, value) => _conditionalReturn(value, v => _clamp(min, max, v)),\n\t_slice = [].slice,\n\t_isArrayLike = (value, nonEmpty) => value && (_isObject(value) && \"length\" in value && ((!nonEmpty && !value.length) || ((value.length - 1) in value && _isObject(value[0]))) && !value.nodeType && value !== _win),\n\t_flatten = (ar, leaveStrings, accumulator = []) => ar.forEach(value => (_isString(value) && !leaveStrings) || _isArrayLike(value, 1) ? accumulator.push(...toArray(value)) : accumulator.push(value)) || accumulator,\n\t//takes any value and returns an array. If it's a string (and leaveStrings isn't true), it'll use document.querySelectorAll() and convert that to an array. It'll also accept iterables like jQuery objects.\n\ttoArray = (value, scope, leaveStrings) => _context && !scope && _context.selector ? _context.selector(value) : _isString(value) && !leaveStrings && (_coreInitted || !_wake()) ? _slice.call((scope || _doc).querySelectorAll(value), 0) : _isArray(value) ? _flatten(value, leaveStrings) : _isArrayLike(value) ? _slice.call(value, 0) : value ? [value] : [],\n\tselector = value => {\n\t\tvalue = toArray(value)[0] || _warn(\"Invalid scope\") || {};\n\t\treturn v => {\n\t\t\tlet el = value.current || value.nativeElement || value;\n\t\t\treturn toArray(v, el.querySelectorAll ? el : el === value ? _warn(\"Invalid scope\") || _doc.createElement(\"div\") : value);\n\t\t};\n\t},\n\tshuffle = a => a.sort(() => .5 - Math.random()), // alternative that's a bit faster and more reliably diverse but bigger: for (let j, v, i = a.length; i; j = (Math.random() * i) | 0, v = a[--i], a[i] = a[j], a[j] = v); return a;\n\t//for distributing values across an array. Can accept a number, a function or (most commonly) a function which can contain the following properties: {base, amount, from, ease, grid, axis, length, each}. Returns a function that expects the following parameters: index, target, array. Recognizes the following\n\tdistribute = v => {\n\t\tif (_isFunction(v)) {\n\t\t\treturn v;\n\t\t}\n\t\tlet vars = _isObject(v) ? v : {each:v}, //n:1 is just to indicate v was a number; we leverage that later to set v according to the length we get. If a number is passed in, we treat it like the old stagger value where 0.1, for example, would mean that things would be distributed with 0.1 between each element in the array rather than a total \"amount\" that's chunked out among them all.\n\t\t\tease = _parseEase(vars.ease),\n\t\t\tfrom = vars.from || 0,\n\t\t\tbase = parseFloat(vars.base) || 0,\n\t\t\tcache = {},\n\t\t\tisDecimal = (from > 0 && from < 1),\n\t\t\tratios = isNaN(from) || isDecimal,\n\t\t\taxis = vars.axis,\n\t\t\tratioX = from,\n\t\t\tratioY = from;\n\t\tif (_isString(from)) {\n\t\t\tratioX = ratioY = {center:.5, edges:.5, end:1}[from] || 0;\n\t\t} else if (!isDecimal && ratios) {\n\t\t\tratioX = from[0];\n\t\t\tratioY = from[1];\n\t\t}\n\t\treturn (i, target, a) => {\n\t\t\tlet l = (a || vars).length,\n\t\t\t\tdistances = cache[l],\n\t\t\t\toriginX, originY, x, y, d, j, max, min, wrapAt;\n\t\t\tif (!distances) {\n\t\t\t\twrapAt = (vars.grid === \"auto\") ? 0 : (vars.grid || [1, _bigNum])[1];\n\t\t\t\tif (!wrapAt) {\n\t\t\t\t\tmax = -_bigNum;\n\t\t\t\t\twhile (max < (max = a[wrapAt++].getBoundingClientRect().left) && wrapAt < l) { }\n\t\t\t\t\twrapAt < l && wrapAt--;\n\t\t\t\t}\n\t\t\t\tdistances = cache[l] = [];\n\t\t\t\toriginX = ratios ? (Math.min(wrapAt, l) * ratioX) - .5 : from % wrapAt;\n\t\t\t\toriginY = wrapAt === _bigNum ? 0 : ratios ? l * ratioY / wrapAt - .5 : (from / wrapAt) | 0;\n\t\t\t\tmax = 0;\n\t\t\t\tmin = _bigNum;\n\t\t\t\tfor (j = 0; j < l; j++) {\n\t\t\t\t\tx = (j % wrapAt) - originX;\n\t\t\t\t\ty = originY - ((j / wrapAt) | 0);\n\t\t\t\t\tdistances[j] = d = !axis ? _sqrt(x * x + y * y) : Math.abs((axis === \"y\") ? y : x);\n\t\t\t\t\t(d > max) && (max = d);\n\t\t\t\t\t(d < min) && (min = d);\n\t\t\t\t}\n\t\t\t\t(from === \"random\") && shuffle(distances);\n\t\t\t\tdistances.max = max - min;\n\t\t\t\tdistances.min = min;\n\t\t\t\tdistances.v = l = (parseFloat(vars.amount) || (parseFloat(vars.each) * (wrapAt > l ? l - 1 : !axis ? Math.max(wrapAt, l / wrapAt) : axis === \"y\" ? l / wrapAt : wrapAt)) || 0) * (from === \"edges\" ? -1 : 1);\n\t\t\t\tdistances.b = (l < 0) ? base - l : base;\n\t\t\t\tdistances.u = getUnit(vars.amount || vars.each) || 0; //unit\n\t\t\t\tease = (ease && l < 0) ? _invertEase(ease) : ease;\n\t\t\t}\n\t\t\tl = ((distances[i] - distances.min) / distances.max) || 0;\n\t\t\treturn _roundPrecise(distances.b + (ease ? ease(l) : l) * distances.v) + distances.u; //round in order to work around floating point errors\n\t\t};\n\t},\n\t_roundModifier = v => { //pass in 0.1 get a function that'll round to the nearest tenth, or 5 to round to the closest 5, or 0.001 to the closest 1000th, etc.\n\t\tlet p = Math.pow(10, ((v + \"\").split(\".\")[1] || \"\").length); //to avoid floating point math errors (like 24 * 0.1 == 2.4000000000000004), we chop off at a specific number of decimal places (much faster than toFixed())\n\t\treturn raw => {\n\t\t\tlet n = _roundPrecise(Math.round(parseFloat(raw) / v) * v * p);\n\t\t\treturn (n - n % 1) / p + (_isNumber(raw) ? 0 : getUnit(raw)); // n - n % 1 replaces Math.floor() in order to handle negative values properly. For example, Math.floor(-150.00000000000003) is 151!\n\t\t};\n\t},\n\tsnap = (snapTo, value) => {\n\t\tlet isArray = _isArray(snapTo),\n\t\t\tradius, is2D;\n\t\tif (!isArray && _isObject(snapTo)) {\n\t\t\tradius = isArray = snapTo.radius || _bigNum;\n\t\t\tif (snapTo.values) {\n\t\t\t\tsnapTo = toArray(snapTo.values);\n\t\t\t\tif ((is2D = !_isNumber(snapTo[0]))) {\n\t\t\t\t\tradius *= radius; //performance optimization so we don't have to Math.sqrt() in the loop.\n\t\t\t\t}\n\t\t\t} else {\n\t\t\t\tsnapTo = _roundModifier(snapTo.increment);\n\t\t\t}\n\t\t}\n\t\treturn _conditionalReturn(value, !isArray ? _roundModifier(snapTo) : _isFunction(snapTo) ? raw => {is2D = snapTo(raw); return Math.abs(is2D - raw) <= radius ? is2D : raw; } : raw => {\n\t\t\tlet x = parseFloat(is2D ? raw.x : raw),\n\t\t\t\ty = parseFloat(is2D ? raw.y : 0),\n\t\t\t\tmin = _bigNum,\n\t\t\t\tclosest = 0,\n\t\t\t\ti = snapTo.length,\n\t\t\t\tdx, dy;\n\t\t\twhile (i--) {\n\t\t\t\tif (is2D) {\n\t\t\t\t\tdx = snapTo[i].x - x;\n\t\t\t\t\tdy = snapTo[i].y - y;\n\t\t\t\t\tdx = dx * dx + dy * dy;\n\t\t\t\t} else {\n\t\t\t\t\tdx = Math.abs(snapTo[i] - x);\n\t\t\t\t}\n\t\t\t\tif (dx < min) {\n\t\t\t\t\tmin = dx;\n\t\t\t\t\tclosest = i;\n\t\t\t\t}\n\t\t\t}\n\t\t\tclosest = (!radius || min <= radius) ? snapTo[closest] : raw;\n\t\t\treturn (is2D || closest === raw || _isNumber(raw)) ? closest : closest + getUnit(raw);\n\t\t});\n\t},\n\trandom = (min, max, roundingIncrement, returnFunction) => _conditionalReturn(_isArray(min) ? !max : roundingIncrement === true ? !!(roundingIncrement = 0) : !returnFunction, () => _isArray(min) ? min[~~(Math.random() * min.length)] : (roundingIncrement = roundingIncrement || 1e-5) && (returnFunction = roundingIncrement < 1 ? 10 ** ((roundingIncrement + \"\").length - 2) : 1) && (Math.floor(Math.round((min - roundingIncrement / 2 + Math.random() * (max - min + roundingIncrement * .99)) / roundingIncrement) * roundingIncrement * returnFunction) / returnFunction)),\n\tpipe = (...functions) => value => functions.reduce((v, f) => f(v), value),\n\tunitize = (func, unit) => value => func(parseFloat(value)) + (unit || getUnit(value)),\n\tnormalize = (min, max, value) => mapRange(min, max, 0, 1, value),\n\t_wrapArray = (a, wrapper, value) => _conditionalReturn(value, index => a[~~wrapper(index)]),\n\twrap = function(min, max, value) { // NOTE: wrap() CANNOT be an arrow function! A very odd compiling bug causes problems (unrelated to GSAP).\n\t\tlet range = max - min;\n\t\treturn _isArray(min) ? _wrapArray(min, wrap(0, min.length), max) : _conditionalReturn(value, value => ((range + (value - min) % range) % range) + min);\n\t},\n\twrapYoyo = (min, max, value) => {\n\t\tlet range = max - min,\n\t\t\ttotal = range * 2;\n\t\treturn _isArray(min) ? _wrapArray(min, wrapYoyo(0, min.length - 1), max) : _conditionalReturn(value, value => {\n\t\t\tvalue = (total + (value - min) % total) % total || 0;\n\t\t\treturn min + ((value > range) ? (total - value) : value);\n\t\t});\n\t},\n\t_replaceRandom = value => { //replaces all occurrences of random(...) in a string with the calculated random value. can be a range like random(-100, 100, 5) or an array like random([0, 100, 500])\n\t\tlet prev = 0,\n\t\t\ts = \"\",\n\t\t\ti, nums, end, isArray;\n\t\twhile (~(i = value.indexOf(\"random(\", prev))) {\n\t\t\tend = value.indexOf(\")\", i);\n\t\t\tisArray = value.charAt(i + 7) === \"[\";\n\t\t\tnums = value.substr(i + 7, end - i - 7).match(isArray ? _delimitedValueExp : _strictNumExp);\n\t\t\ts += value.substr(prev, i - prev) + random(isArray ? nums : +nums[0], isArray ? 0 : +nums[1], +nums[2] || 1e-5);\n\t\t\tprev = end + 1;\n\t\t}\n\t\treturn s + value.substr(prev, value.length - prev);\n\t},\n\tmapRange = (inMin, inMax, outMin, outMax, value) => {\n\t\tlet inRange = inMax - inMin,\n\t\t\toutRange = outMax - outMin;\n\t\treturn _conditionalReturn(value, value => outMin + ((((value - inMin) / inRange) * outRange) || 0));\n\t},\n\tinterpolate = (start, end, progress, mutate) => {\n\t\tlet func = isNaN(start + end) ? 0 : p => (1 - p) * start + p * end;\n\t\tif (!func) {\n\t\t\tlet isString = _isString(start),\n\t\t\t\tmaster = {},\n\t\t\t\tp, i, interpolators, l, il;\n\t\t\tprogress === true && (mutate = 1) && (progress = null);\n\t\t\tif (isString) {\n\t\t\t\tstart = {p: start};\n\t\t\t\tend = {p: end};\n\n\t\t\t} else if (_isArray(start) && !_isArray(end)) {\n\t\t\t\tinterpolators = [];\n\t\t\t\tl = start.length;\n\t\t\t\til = l - 2;\n\t\t\t\tfor (i = 1; i < l; i++) {\n\t\t\t\t\tinterpolators.push(interpolate(start[i-1], start[i])); //build the interpolators up front as a performance optimization so that when the function is called many times, it can just reuse them.\n\t\t\t\t}\n\t\t\t\tl--;\n\t\t\t\tfunc = p => {\n\t\t\t\t\tp *= l;\n\t\t\t\t\tlet i = Math.min(il, ~~p);\n\t\t\t\t\treturn interpolators[i](p - i);\n\t\t\t\t};\n\t\t\t\tprogress = end;\n\t\t\t} else if (!mutate) {\n\t\t\t\tstart = _merge(_isArray(start) ? [] : {}, start);\n\t\t\t}\n\t\t\tif (!interpolators) {\n\t\t\t\tfor (p in end) {\n\t\t\t\t\t_addPropTween.call(master, start, p, \"get\", end[p]);\n\t\t\t\t}\n\t\t\t\tfunc = p => _renderPropTweens(p, master) || (isString ? start.p : start);\n\t\t\t}\n\t\t}\n\t\treturn _conditionalReturn(progress, func);\n\t},\n\t_getLabelInDirection = (timeline, fromTime, backward) => { //used for nextLabel() and previousLabel()\n\t\tlet labels = timeline.labels,\n\t\t\tmin = _bigNum,\n\t\t\tp, distance, label;\n\t\tfor (p in labels) {\n\t\t\tdistance = labels[p] - fromTime;\n\t\t\tif ((distance < 0) === !!backward && distance && min > (distance = Math.abs(distance))) {\n\t\t\t\tlabel = p;\n\t\t\t\tmin = distance;\n\t\t\t}\n\t\t}\n\t\treturn label;\n\t},\n\t_callback = (animation, type, executeLazyFirst) => {\n\t\tlet v = animation.vars,\n\t\t\tcallback = v[type],\n\t\t\tprevContext = _context,\n\t\t\tcontext = animation._ctx,\n\t\t\tparams, scope, result;\n\t\tif (!callback) {\n\t\t\treturn;\n\t\t}\n\t\tparams = v[type + \"Params\"];\n\t\tscope = v.callbackScope || animation;\n\t\texecuteLazyFirst && _lazyTweens.length && _lazyRender(); //in case rendering caused any tweens to lazy-init, we should render them because typically when a timeline finishes, users expect things to have rendered fully. Imagine an onUpdate on a timeline that reports/checks tweened values.\n\t\tcontext && (_context = context);\n\t\tresult = params ? callback.apply(scope, params) : callback.call(scope);\n\t\t_context = prevContext;\n\t\treturn result;\n\t},\n\t_interrupt = animation => {\n\t\t_removeFromParent(animation);\n\t\tanimation.scrollTrigger && animation.scrollTrigger.kill(!!_reverting);\n\t\tanimation.progress() < 1 && _callback(animation, \"onInterrupt\");\n\t\treturn animation;\n\t},\n\t_quickTween,\n\t_registerPluginQueue = [],\n\t_createPlugin = config => {\n\t\tif (!config) return;\n\t\tconfig = (!config.name && config.default) || config; // UMD packaging wraps things oddly, so for example MotionPathHelper becomes {MotionPathHelper:MotionPathHelper, default:MotionPathHelper}.\n\t\tif (_windowExists() || config.headless) { // edge case: some build tools may pass in a null/undefined value\n\t\t\tlet name = config.name,\n\t\t\t\tisFunc = _isFunction(config),\n\t\t\t\tPlugin = (name && !isFunc && config.init) ? function () {\n\t\t\t\t\tthis._props = [];\n\t\t\t\t} : config, //in case someone passes in an object that's not a plugin, like CustomEase\n\t\t\t\tinstanceDefaults = {init: _emptyFunc, render: _renderPropTweens, add: _addPropTween, kill: _killPropTweensOf, modifier: _addPluginModifier, rawVars: 0},\n\t\t\t\tstatics = {targetTest: 0, get: 0, getSetter: _getSetter, aliases: {}, register: 0};\n\t\t\t_wake();\n\t\t\tif (config !== Plugin) {\n\t\t\t\tif (_plugins[name]) {\n\t\t\t\t\treturn;\n\t\t\t\t}\n\t\t\t\t_setDefaults(Plugin, _setDefaults(_copyExcluding(config, instanceDefaults), statics)); //static methods\n\t\t\t\t_merge(Plugin.prototype, _merge(instanceDefaults, _copyExcluding(config, statics))); //instance methods\n\t\t\t\t_plugins[(Plugin.prop = name)] = Plugin;\n\t\t\t\tif (config.targetTest) {\n\t\t\t\t\t_harnessPlugins.push(Plugin);\n\t\t\t\t\t_reservedProps[name] = 1;\n\t\t\t\t}\n\t\t\t\tname = (name === \"css\" ? \"CSS\" : name.charAt(0).toUpperCase() + name.substr(1)) + \"Plugin\"; //for the global name. \"motionPath\" should become MotionPathPlugin\n\t\t\t}\n\t\t\t_addGlobal(name, Plugin);\n\t\t\tconfig.register && config.register(gsap, Plugin, PropTween);\n\t\t} else {\n\t\t\t_registerPluginQueue.push(config);\n\t\t}\n\t},\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * COLORS\n * --------------------------------------------------------------------------------------\n */\n\n\t_255 = 255,\n\t_colorLookup = {\n\t\taqua:[0,_255,_255],\n\t\tlime:[0,_255,0],\n\t\tsilver:[192,192,192],\n\t\tblack:[0,0,0],\n\t\tmaroon:[128,0,0],\n\t\tteal:[0,128,128],\n\t\tblue:[0,0,_255],\n\t\tnavy:[0,0,128],\n\t\twhite:[_255,_255,_255],\n\t\tolive:[128,128,0],\n\t\tyellow:[_255,_255,0],\n\t\torange:[_255,165,0],\n\t\tgray:[128,128,128],\n\t\tpurple:[128,0,128],\n\t\tgreen:[0,128,0],\n\t\tred:[_255,0,0],\n\t\tpink:[_255,192,203],\n\t\tcyan:[0,_255,_255],\n\t\ttransparent:[_255,_255,_255,0]\n\t},\n\t// possible future idea to replace the hard-coded color name values - put this in the ticker.wake() where we set the _doc:\n\t// let ctx = _doc.createElement(\"canvas\").getContext(\"2d\");\n\t// _forEachName(\"aqua,lime,silver,black,maroon,teal,blue,navy,white,olive,yellow,orange,gray,purple,green,red,pink,cyan\", color => {ctx.fillStyle = color; _colorLookup[color] = splitColor(ctx.fillStyle)});\n\t_hue = (h, m1, m2) => {\n\t\th += h < 0 ? 1 : h > 1 ? -1 : 0;\n\t\treturn ((((h * 6 < 1) ? m1 + (m2 - m1) * h * 6 : h < .5 ? m2 : (h * 3 < 2) ? m1 + (m2 - m1) * (2 / 3 - h) * 6 : m1) * _255) + .5) | 0;\n\t},\n\tsplitColor = (v, toHSL, forceAlpha) => {\n\t\tlet a = !v ? _colorLookup.black : _isNumber(v) ? [v >> 16, (v >> 8) & _255, v & _255] : 0,\n\t\t\tr, g, b, h, s, l, max, min, d, wasHSL;\n\t\tif (!a) {\n\t\t\tif (v.substr(-1) === \",\") { //sometimes a trailing comma is included and we should chop it off (typically from a comma-delimited list of values like a textShadow:\"2px 2px 2px blue, 5px 5px 5px rgb(255,0,0)\" - in this example \"blue,\" has a trailing comma. We could strip it out inside parseComplex() but we'd need to do it to the beginning and ending values plus it wouldn't provide protection from other potential scenarios like if the user passes in a similar value.\n\t\t\t\tv = v.substr(0, v.length - 1);\n\t\t\t}\n\t\t\tif (_colorLookup[v]) {\n\t\t\t\ta = _colorLookup[v];\n\t\t\t} else if (v.charAt(0) === \"#\") {\n\t\t\t\tif (v.length < 6) { //for shorthand like #9F0 or #9F0F (could have alpha)\n\t\t\t\t\tr = v.charAt(1);\n\t\t\t\t\tg = v.charAt(2);\n\t\t\t\t\tb = v.charAt(3);\n\t\t\t\t\tv = \"#\" + r + r + g + g + b + b + (v.length === 5 ? v.charAt(4) + v.charAt(4) : \"\");\n\t\t\t\t}\n\t\t\t\tif (v.length === 9) { // hex with alpha, like #fd5e53ff\n\t\t\t\t\ta = parseInt(v.substr(1, 6), 16);\n\t\t\t\t\treturn [a >> 16, (a >> 8) & _255, a & _255, parseInt(v.substr(7), 16) / 255];\n\t\t\t\t}\n\t\t\t\tv = parseInt(v.substr(1), 16);\n\t\t\t\ta = [v >> 16, (v >> 8) & _255, v & _255];\n\t\t\t} else if (v.substr(0, 3) === \"hsl\") {\n\t\t\t\ta = wasHSL = v.match(_strictNumExp);\n\t\t\t\tif (!toHSL) {\n\t\t\t\t\th = (+a[0] % 360) / 360;\n\t\t\t\t\ts = +a[1] / 100;\n\t\t\t\t\tl = +a[2] / 100;\n\t\t\t\t\tg = (l <= .5) ? l * (s + 1) : l + s - l * s;\n\t\t\t\t\tr = l * 2 - g;\n\t\t\t\t\ta.length > 3 && (a[3] *= 1); //cast as number\n\t\t\t\t\ta[0] = _hue(h + 1 / 3, r, g);\n\t\t\t\t\ta[1] = _hue(h, r, g);\n\t\t\t\t\ta[2] = _hue(h - 1 / 3, r, g);\n\t\t\t\t} else if (~v.indexOf(\"=\")) { //if relative values are found, just return the raw strings with the relative prefixes in place.\n\t\t\t\t\ta = v.match(_numExp);\n\t\t\t\t\tforceAlpha && a.length < 4 && (a[3] = 1);\n\t\t\t\t\treturn a;\n\t\t\t\t}\n\t\t\t} else {\n\t\t\t\ta = v.match(_strictNumExp) || _colorLookup.transparent;\n\t\t\t}\n\t\t\ta = a.map(Number);\n\t\t}\n\t\tif (toHSL && !wasHSL) {\n\t\t\tr = a[0] / _255;\n\t\t\tg = a[1] / _255;\n\t\t\tb = a[2] / _255;\n\t\t\tmax = Math.max(r, g, b);\n\t\t\tmin = Math.min(r, g, b);\n\t\t\tl = (max + min) / 2;\n\t\t\tif (max === min) {\n\t\t\t\th = s = 0;\n\t\t\t} else {\n\t\t\t\td = max - min;\n\t\t\t\ts = l > 0.5 ? d / (2 - max - min) : d / (max + min);\n\t\t\t\th = max === r ? (g - b) / d + (g < b ? 6 : 0) : max === g ? (b - r) / d + 2 : (r - g) / d + 4;\n\t\t\t\th *= 60;\n\t\t\t}\n\t\t\ta[0] = ~~(h + .5);\n\t\t\ta[1] = ~~(s * 100 + .5);\n\t\t\ta[2] = ~~(l * 100 + .5);\n\t\t}\n\t\tforceAlpha && a.length < 4 && (a[3] = 1);\n\t\treturn a;\n\t},\n\t_colorOrderData = v => { // strips out the colors from the string, finds all the numeric slots (with units) and returns an array of those. The Array also has a \"c\" property which is an Array of the index values where the colors belong. This is to help work around issues where there's a mis-matched order of color/numeric data like drop-shadow(#f00 0px 1px 2px) and drop-shadow(0x 1px 2px #f00). This is basically a helper function used in _formatColors()\n\t\tlet values = [],\n\t\t\tc = [],\n\t\t\ti = -1;\n\t\tv.split(_colorExp).forEach(v => {\n\t\t\tlet a = v.match(_numWithUnitExp) || [];\n\t\t\tvalues.push(...a);\n\t\t\tc.push(i += a.length + 1);\n\t\t});\n\t\tvalues.c = c;\n\t\treturn values;\n\t},\n\t_formatColors = (s, toHSL, orderMatchData) => {\n\t\tlet result = \"\",\n\t\t\tcolors = (s + result).match(_colorExp),\n\t\t\ttype = toHSL ? \"hsla(\" : \"rgba(\",\n\t\t\ti = 0,\n\t\t\tc, shell, d, l;\n\t\tif (!colors) {\n\t\t\treturn s;\n\t\t}\n\t\tcolors = colors.map(color => (color = splitColor(color, toHSL, 1)) && type + (toHSL ? color[0] + \",\" + color[1] + \"%,\" + color[2] + \"%,\" + color[3] : color.join(\",\")) + \")\");\n\t\tif (orderMatchData) {\n\t\t\td = _colorOrderData(s);\n\t\t\tc = orderMatchData.c;\n\t\t\tif (c.join(result) !== d.c.join(result)) {\n\t\t\t\tshell = s.replace(_colorExp, \"1\").split(_numWithUnitExp);\n\t\t\t\tl = shell.length - 1;\n\t\t\t\tfor (; i < l; i++) {\n\t\t\t\t\tresult += shell[i] + (~c.indexOf(i) ? colors.shift() || type + \"0,0,0,0)\" : (d.length ? d : colors.length ? colors : orderMatchData).shift());\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tif (!shell) {\n\t\t\tshell = s.split(_colorExp);\n\t\t\tl = shell.length - 1;\n\t\t\tfor (; i < l; i++) {\n\t\t\t\tresult += shell[i] + colors[i];\n\t\t\t}\n\t\t}\n\t\treturn result + shell[l];\n\t},\n\t_colorExp = (function() {\n\t\tlet s = \"(?:\\\\b(?:(?:rgb|rgba|hsl|hsla)\\\\(.+?\\\\))|\\\\B#(?:[0-9a-f]{3,4}){1,2}\\\\b\", //we'll dynamically build this Regular Expression to conserve file size. After building it, it will be able to find rgb(), rgba(), # (hexadecimal), and named color values like red, blue, purple, etc.,\n\t\t\tp;\n\t\tfor (p in _colorLookup) {\n\t\t\ts += \"|\" + p + \"\\\\b\";\n\t\t}\n\t\treturn new RegExp(s + \")\", \"gi\");\n\t})(),\n\t_hslExp = /hsl[a]?\\(/,\n\t_colorStringFilter = a => {\n\t\tlet combined = a.join(\" \"),\n\t\t\ttoHSL;\n\t\t_colorExp.lastIndex = 0;\n\t\tif (_colorExp.test(combined)) {\n\t\t\ttoHSL = _hslExp.test(combined);\n\t\t\ta[1] = _formatColors(a[1], toHSL);\n\t\t\ta[0] = _formatColors(a[0], toHSL, _colorOrderData(a[1])); // make sure the order of numbers/colors match with the END value.\n\t\t\treturn true;\n\t\t}\n\t},\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * TICKER\n * --------------------------------------------------------------------------------------\n */\n\t_tickerActive,\n\t_ticker = (function() {\n\t\tlet _getTime = Date.now,\n\t\t\t_lagThreshold = 500,\n\t\t\t_adjustedLag = 33,\n\t\t\t_startTime = _getTime(),\n\t\t\t_lastUpdate = _startTime,\n\t\t\t_gap = 1000 / 240,\n\t\t\t_nextTime = _gap,\n\t\t\t_listeners = [],\n\t\t\t_id, _req, _raf, _self, _delta, _i,\n\t\t\t_tick = v => {\n\t\t\t\tlet elapsed = _getTime() - _lastUpdate,\n\t\t\t\t\tmanual = v === true,\n\t\t\t\t\toverlap, dispatch, time, frame;\n\t\t\t\t(elapsed > _lagThreshold || elapsed < 0) && (_startTime += elapsed - _adjustedLag);\n\t\t\t\t_lastUpdate += elapsed;\n\t\t\t\ttime = _lastUpdate - _startTime;\n\t\t\t\toverlap = time - _nextTime;\n\t\t\t\tif (overlap > 0 || manual) {\n\t\t\t\t\tframe = ++_self.frame;\n\t\t\t\t\t_delta = time - _self.time * 1000;\n\t\t\t\t\t_self.time = time = time / 1000;\n\t\t\t\t\t_nextTime += overlap + (overlap >= _gap ? 4 : _gap - overlap);\n\t\t\t\t\tdispatch = 1;\n\t\t\t\t}\n\t\t\t\tmanual || (_id = _req(_tick)); //make sure the request is made before we dispatch the \"tick\" event so that timing is maintained. Otherwise, if processing the \"tick\" requires a bunch of time (like 15ms) and we're using a setTimeout() that's based on 16.7ms, it'd technically take 31.7ms between frames otherwise.\n\t\t\t\tif (dispatch) {\n\t\t\t\t\tfor (_i = 0; _i < _listeners.length; _i++) { // use _i and check _listeners.length instead of a variable because a listener could get removed during the loop, and if that happens to an element less than the current index, it'd throw things off in the loop.\n\t\t\t\t\t\t_listeners[_i](time, _delta, frame, v);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t};\n\t\t_self = {\n\t\t\ttime:0,\n\t\t\tframe:0,\n\t\t\ttick() {\n\t\t\t\t_tick(true);\n\t\t\t},\n\t\t\tdeltaRatio(fps) {\n\t\t\t\treturn _delta / (1000 / (fps || 60));\n\t\t\t},\n\t\t\twake() {\n\t\t\t\tif (_coreReady) {\n\t\t\t\t\tif (!_coreInitted && _windowExists()) {\n\t\t\t\t\t\t_win = _coreInitted = window;\n\t\t\t\t\t\t_doc = _win.document || {};\n\t\t\t\t\t\t_globals.gsap = gsap;\n\t\t\t\t\t\t(_win.gsapVersions || (_win.gsapVersions = [])).push(gsap.version);\n\t\t\t\t\t\t_install(_installScope || _win.GreenSockGlobals || (!_win.gsap && _win) || {});\n\t\t\t\t\t\t_registerPluginQueue.forEach(_createPlugin);\n\t\t\t\t\t}\n\t\t\t\t\t_raf = typeof(requestAnimationFrame) !== \"undefined\" && requestAnimationFrame;\n\t\t\t\t\t_id && _self.sleep();\n\t\t\t\t\t_req = _raf || (f => setTimeout(f, (_nextTime - _self.time * 1000 + 1) | 0));\n\t\t\t\t\t_tickerActive = 1;\n\t\t\t\t\t_tick(2);\n\t\t\t\t}\n\t\t\t},\n\t\t\tsleep() {\n\t\t\t\t(_raf ? cancelAnimationFrame : clearTimeout)(_id);\n\t\t\t\t_tickerActive = 0;\n\t\t\t\t_req = _emptyFunc;\n\t\t\t},\n\t\t\tlagSmoothing(threshold, adjustedLag) {\n\t\t\t\t_lagThreshold = threshold || Infinity; // zero should be interpreted as basically unlimited\n\t\t\t\t_adjustedLag = Math.min(adjustedLag || 33, _lagThreshold);\n\t\t\t},\n\t\t\tfps(fps) {\n\t\t\t\t_gap = 1000 / (fps || 240);\n\t\t\t\t_nextTime = _self.time * 1000 + _gap;\n\t\t\t},\n\t\t\tadd(callback, once, prioritize) {\n\t\t\t\tlet func = once ? (t, d, f, v) => {callback(t, d, f, v); _self.remove(func);} : callback;\n\t\t\t\t_self.remove(callback);\n\t\t\t\t_listeners[prioritize ? \"unshift\" : \"push\"](func);\n\t\t\t\t_wake();\n\t\t\t\treturn func;\n\t\t\t},\n\t\t\tremove(callback, i) {\n\t\t\t\t~(i = _listeners.indexOf(callback)) && _listeners.splice(i, 1) && _i >= i && _i--;\n\t\t\t},\n\t\t\t_listeners:_listeners\n\t\t};\n\t\treturn _self;\n\t})(),\n\t_wake = () => !_tickerActive && _ticker.wake(), //also ensures the core classes are initialized.\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n* -------------------------------------------------\n* EASING\n* -------------------------------------------------\n*/\n\t_easeMap = {},\n\t_customEaseExp = /^[\\d.\\-M][\\d.\\-,\\s]/,\n\t_quotesExp = /[\"']/g,\n\t_parseObjectInString = value => { //takes a string like \"{wiggles:10, type:anticipate})\" and turns it into a real object. Notice it ends in \")\" and includes the {} wrappers. This is because we only use this function for parsing ease configs and prioritized optimization rather than reusability.\n\t\tlet obj = {},\n\t\t\tsplit = value.substr(1, value.length-3).split(\":\"),\n\t\t\tkey = split[0],\n\t\t\ti = 1,\n\t\t\tl = split.length,\n\t\t\tindex, val, parsedVal;\n\t\tfor (; i < l; i++) {\n\t\t\tval = split[i];\n\t\t\tindex = i !== l-1 ? val.lastIndexOf(\",\") : val.length;\n\t\t\tparsedVal = val.substr(0, index);\n\t\t\tobj[key] = isNaN(parsedVal) ? parsedVal.replace(_quotesExp, \"\").trim() : +parsedVal;\n\t\t\tkey = val.substr(index+1).trim();\n\t\t}\n\t\treturn obj;\n\t},\n\t_valueInParentheses = value => {\n\t\tlet open = value.indexOf(\"(\") + 1,\n\t\t\tclose = value.indexOf(\")\"),\n\t\t\tnested = value.indexOf(\"(\", open);\n\t\treturn value.substring(open, ~nested && nested < close ? value.indexOf(\")\", close + 1) : close);\n\t},\n\t_configEaseFromString = name => { //name can be a string like \"elastic.out(1,0.5)\", and pass in _easeMap as obj and it'll parse it out and call the actual function like _easeMap.Elastic.easeOut.config(1,0.5). It will also parse custom ease strings as long as CustomEase is loaded and registered (internally as _easeMap._CE).\n\t\tlet split = (name + \"\").split(\"(\"),\n\t\t\tease = _easeMap[split[0]];\n\t\treturn (ease && split.length > 1 && ease.config) ? ease.config.apply(null, ~name.indexOf(\"{\") ? [_parseObjectInString(split[1])] : _valueInParentheses(name).split(\",\").map(_numericIfPossible)) : (_easeMap._CE && _customEaseExp.test(name)) ? _easeMap._CE(\"\", name) : ease;\n\t},\n\t_invertEase = ease => p => 1 - ease(1 - p),\n\t// allow yoyoEase to be set in children and have those affected when the parent/ancestor timeline yoyos.\n\t_propagateYoyoEase = (timeline, isYoyo) => {\n\t\tlet child = timeline._first, ease;\n\t\twhile (child) {\n\t\t\tif (child instanceof Timeline) {\n\t\t\t\t_propagateYoyoEase(child, isYoyo);\n\t\t\t} else if (child.vars.yoyoEase && (!child._yoyo || !child._repeat) && child._yoyo !== isYoyo) {\n\t\t\t\tif (child.timeline) {\n\t\t\t\t\t_propagateYoyoEase(child.timeline, isYoyo);\n\t\t\t\t} else {\n\t\t\t\t\tease = child._ease;\n\t\t\t\t\tchild._ease = child._yEase;\n\t\t\t\t\tchild._yEase = ease;\n\t\t\t\t\tchild._yoyo = isYoyo;\n\t\t\t\t}\n\t\t\t}\n\t\t\tchild = child._next;\n\t\t}\n\t},\n\t_parseEase = (ease, defaultEase) => !ease ? defaultEase : (_isFunction(ease) ? ease : _easeMap[ease] || _configEaseFromString(ease)) || defaultEase,\n\t_insertEase = (names, easeIn, easeOut = p => 1 - easeIn(1 - p), easeInOut = (p => p < .5 ? easeIn(p * 2) / 2 : 1 - easeIn((1 - p) * 2) / 2)) => {\n\t\tlet ease = {easeIn, easeOut, easeInOut},\n\t\t\tlowercaseName;\n\t\t_forEachName(names, name => {\n\t\t\t_easeMap[name] = _globals[name] = ease;\n\t\t\t_easeMap[(lowercaseName = name.toLowerCase())] = easeOut;\n\t\t\tfor (let p in ease) {\n\t\t\t\t_easeMap[lowercaseName + (p === \"easeIn\" ? \".in\" : p === \"easeOut\" ? \".out\" : \".inOut\")] = _easeMap[name + \".\" + p] = ease[p];\n\t\t\t}\n\t\t});\n\t\treturn ease;\n\t},\n\t_easeInOutFromOut = easeOut => (p => p < .5 ? (1 - easeOut(1 - (p * 2))) / 2 : .5 + easeOut((p - .5) * 2) / 2),\n\t_configElastic = (type, amplitude, period) => {\n\t\tlet p1 = (amplitude >= 1) ? amplitude : 1, //note: if amplitude is < 1, we simply adjust the period for a more natural feel. Otherwise the math doesn't work right and the curve starts at 1.\n\t\t\tp2 = (period || (type ? .3 : .45)) / (amplitude < 1 ? amplitude : 1),\n\t\t\tp3 = p2 / _2PI * (Math.asin(1 / p1) || 0),\n\t\t\teaseOut = p => p === 1 ? 1 : p1 * (2 ** (-10 * p)) * _sin((p - p3) * p2) + 1,\n\t\t\tease = (type === \"out\") ? easeOut : (type === \"in\") ? p => 1 - easeOut(1 - p) : _easeInOutFromOut(easeOut);\n\t\tp2 = _2PI / p2; //precalculate to optimize\n\t\tease.config = (amplitude, period) => _configElastic(type, amplitude, period);\n\t\treturn ease;\n\t},\n\t_configBack = (type, overshoot = 1.70158) => {\n\t\tlet easeOut = p => p ? ((--p) * p * ((overshoot + 1) * p + overshoot) + 1) : 0,\n\t\t\tease = type === \"out\" ? easeOut : type === \"in\" ? p => 1 - easeOut(1 - p) : _easeInOutFromOut(easeOut);\n\t\tease.config = overshoot => _configBack(type, overshoot);\n\t\treturn ease;\n\t};\n\t// a cheaper (kb and cpu) but more mild way to get a parameterized weighted ease by feeding in a value between -1 (easeIn) and 1 (easeOut) where 0 is linear.\n\t// _weightedEase = ratio => {\n\t// \tlet y = 0.5 + ratio / 2;\n\t// \treturn p => (2 * (1 - p) * p * y + p * p);\n\t// },\n\t// a stronger (but more expensive kb/cpu) parameterized weighted ease that lets you feed in a value between -1 (easeIn) and 1 (easeOut) where 0 is linear.\n\t// _weightedEaseStrong = ratio => {\n\t// \tratio = .5 + ratio / 2;\n\t// \tlet o = 1 / 3 * (ratio < .5 ? ratio : 1 - ratio),\n\t// \t\tb = ratio - o,\n\t// \t\tc = ratio + o;\n\t// \treturn p => p === 1 ? p : 3 * b * (1 - p) * (1 - p) * p + 3 * c * (1 - p) * p * p + p * p * p;\n\t// };\n\n_forEachName(\"Linear,Quad,Cubic,Quart,Quint,Strong\", (name, i) => {\n\tlet power = i < 5 ? i + 1 : i;\n\t_insertEase(name + \",Power\" + (power - 1), i ? p => p ** power : p => p, p => 1 - (1 - p) ** power, p => p < .5 ? (p * 2) ** power / 2 : 1 - ((1 - p) * 2) ** power / 2);\n});\n_easeMap.Linear.easeNone = _easeMap.none = _easeMap.Linear.easeIn;\n_insertEase(\"Elastic\", _configElastic(\"in\"), _configElastic(\"out\"), _configElastic());\n((n, c) => {\n\tlet n1 = 1 / c,\n\t\tn2 = 2 * n1,\n\t\tn3 = 2.5 * n1,\n\t\teaseOut = p => (p < n1) ? n * p * p : (p < n2) ? n * (p - 1.5 / c) ** 2 + .75 : (p < n3) ? n * (p -= 2.25 / c) * p + .9375 : n * (p - 2.625 / c) ** 2 + .984375;\n\t_insertEase(\"Bounce\", p => 1 - easeOut(1 - p), easeOut);\n})(7.5625, 2.75);\n_insertEase(\"Expo\", p => (2 ** (10 * (p - 1))) * p + p * p * p * p * p * p * (1-p)); // previously 2 ** (10 * (p - 1)) but that doesn't end up with the value quite at the right spot so we do a blended ease to ensure it lands where it should perfectly.\n_insertEase(\"Circ\", p => -(_sqrt(1 - (p * p)) - 1));\n_insertEase(\"Sine\", p => p === 1 ? 1 : -_cos(p * _HALF_PI) + 1);\n_insertEase(\"Back\", _configBack(\"in\"), _configBack(\"out\"), _configBack());\n_easeMap.SteppedEase = _easeMap.steps = _globals.SteppedEase = {\n\tconfig(steps = 1, immediateStart) {\n\t\tlet p1 = 1 / steps,\n\t\t\tp2 = steps + (immediateStart ? 0 : 1),\n\t\t\tp3 = immediateStart ? 1 : 0,\n\t\t\tmax = 1 - _tinyNum;\n\t\treturn p => (((p2 * _clamp(0, max, p)) | 0) + p3) * p1;\n\t}\n};\n_defaults.ease = _easeMap[\"quad.out\"];\n\n\n_forEachName(\"onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt\", name => _callbackNames += name + \",\" + name + \"Params,\");\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * CACHE\n * --------------------------------------------------------------------------------------\n */\nexport class GSCache {\n\n\tconstructor(target, harness) {\n\t\tthis.id = _gsID++;\n\t\ttarget._gsap = this;\n\t\tthis.target = target;\n\t\tthis.harness = harness;\n\t\tthis.get = harness ? harness.get : _getProperty;\n\t\tthis.set = harness ? harness.getSetter : _getSetter;\n\t}\n\n}\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * ANIMATION\n * --------------------------------------------------------------------------------------\n */\n\nexport class Animation {\n\n\tconstructor(vars) {\n\t\tthis.vars = vars;\n\t\tthis._delay = +vars.delay || 0;\n\t\tif ((this._repeat = vars.repeat === Infinity ? -2 : vars.repeat || 0)) { // TODO: repeat: Infinity on a timeline's children must flag that timeline internally and affect its totalDuration, otherwise it'll stop in the negative direction when reaching the start.\n\t\t\tthis._rDelay = vars.repeatDelay || 0;\n\t\t\tthis._yoyo = !!vars.yoyo || !!vars.yoyoEase;\n\t\t}\n\t\tthis._ts = 1;\n\t\t_setDuration(this, +vars.duration, 1, 1);\n\t\tthis.data = vars.data;\n\t\tif (_context) {\n\t\t\tthis._ctx = _context;\n\t\t\t_context.data.push(this);\n\t\t}\n\t\t_tickerActive || _ticker.wake();\n\t}\n\n\tdelay(value) {\n\t\tif (value || value === 0) {\n\t\t\tthis.parent && this.parent.smoothChildTiming && (this.startTime(this._start + value - this._delay));\n\t\t\tthis._delay = value;\n\t\t\treturn this;\n\t\t}\n\t\treturn this._delay;\n\t}\n\n\tduration(value) {\n\t\treturn arguments.length ? this.totalDuration(this._repeat > 0 ? value + (value + this._rDelay) * this._repeat : value) : this.totalDuration() && this._dur;\n\t}\n\n\ttotalDuration(value) {\n\t\tif (!arguments.length) {\n\t\t\treturn this._tDur;\n\t\t}\n\t\tthis._dirty = 0;\n\t\treturn _setDuration(this, this._repeat < 0 ? value : (value - (this._repeat * this._rDelay)) / (this._repeat + 1));\n\t}\n\n\ttotalTime(totalTime, suppressEvents) {\n\t\t_wake();\n\t\tif (!arguments.length) {\n\t\t\treturn this._tTime;\n\t\t}\n\t\tlet parent = this._dp;\n\t\tif (parent && parent.smoothChildTiming && this._ts) {\n\t\t\t_alignPlayhead(this, totalTime);\n\t\t\t!parent._dp || parent.parent || _postAddChecks(parent, this); // edge case: if this is a child of a timeline that already completed, for example, we must re-activate the parent.\n\t\t\t//in case any of the ancestor timelines had completed but should now be enabled, we should reset their totalTime() which will also ensure that they're lined up properly and enabled. Skip for animations that are on the root (wasteful). Example: a TimelineLite.exportRoot() is performed when there's a paused tween on the root, the export will not complete until that tween is unpaused, but imagine a child gets restarted later, after all [unpaused] tweens have completed. The start of that child would get pushed out, but one of the ancestors may have completed.\n\t\t\twhile (parent && parent.parent) {\n\t\t\t\tif (parent.parent._time !== parent._start + (parent._ts >= 0 ? parent._tTime / parent._ts : (parent.totalDuration() - parent._tTime) / -parent._ts)) {\n\t\t\t\t\tparent.totalTime(parent._tTime, true);\n\t\t\t\t}\n\t\t\t\tparent = parent.parent;\n\t\t\t}\n\t\t\tif (!this.parent && this._dp.autoRemoveChildren && ((this._ts > 0 && totalTime < this._tDur) || (this._ts < 0 && totalTime > 0) || (!this._tDur && !totalTime) )) { //if the animation doesn't have a parent, put it back into its last parent (recorded as _dp for exactly cases like this). Limit to parents with autoRemoveChildren (like globalTimeline) so that if the user manually removes an animation from a timeline and then alters its playhead, it doesn't get added back in.\n\t\t\t\t_addToTimeline(this._dp, this, this._start - this._delay);\n\t\t\t}\n\t\t}\n if (this._tTime !== totalTime || (!this._dur && !suppressEvents) || (this._initted && Math.abs(this._zTime) === _tinyNum) || (!totalTime && !this._initted && (this.add || this._ptLookup))) { // check for _ptLookup on a Tween instance to ensure it has actually finished being instantiated, otherwise if this.reverse() gets called in the Animation constructor, it could trigger a render() here even though the _targets weren't populated, thus when _init() is called there won't be any PropTweens (it'll act like the tween is non-functional)\n \tthis._ts || (this._pTime = totalTime); // otherwise, if an animation is paused, then the playhead is moved back to zero, then resumed, it'd revert back to the original time at the pause\n\t //if (!this._lock) { // avoid endless recursion (not sure we need this yet or if it's worth the performance hit)\n\t\t // this._lock = 1;\n\t\t _lazySafeRender(this, totalTime, suppressEvents);\n\t\t // this._lock = 0;\n\t //}\n\t\t}\n\t\treturn this;\n\t}\n\n\ttime(value, suppressEvents) {\n\t\treturn arguments.length ? this.totalTime((Math.min(this.totalDuration(), value + _elapsedCycleDuration(this)) % (this._dur + this._rDelay)) || (value ? this._dur : 0), suppressEvents) : this._time; // note: if the modulus results in 0, the playhead could be exactly at the end or the beginning, and we always defer to the END with a non-zero value, otherwise if you set the time() to the very end (duration()), it would render at the START!\n\t}\n\n\ttotalProgress(value, suppressEvents) {\n\t\treturn arguments.length ? this.totalTime( this.totalDuration() * value, suppressEvents) : this.totalDuration() ? Math.min(1, this._tTime / this._tDur) : this.rawTime() >= 0 && this._initted ? 1 : 0;\n\t}\n\n\tprogress(value, suppressEvents) {\n\t\treturn arguments.length ? this.totalTime( this.duration() * (this._yoyo && !(this.iteration() & 1) ? 1 - value : value) + _elapsedCycleDuration(this), suppressEvents) : (this.duration() ? Math.min(1, this._time / this._dur) : this.rawTime() > 0 ? 1 : 0);\n\t}\n\n\titeration(value, suppressEvents) {\n\t\tlet cycleDuration = this.duration() + this._rDelay;\n\t\treturn arguments.length ? this.totalTime(this._time + (value - 1) * cycleDuration, suppressEvents) : this._repeat ? _animationCycle(this._tTime, cycleDuration) + 1 : 1;\n\t}\n\n\t// potential future addition:\n\t// isPlayingBackwards() {\n\t// \tlet animation = this,\n\t// \t\torientation = 1; // 1 = forward, -1 = backward\n\t// \twhile (animation) {\n\t// \t\torientation *= animation.reversed() || (animation.repeat() && !(animation.iteration() & 1)) ? -1 : 1;\n\t// \t\tanimation = animation.parent;\n\t// \t}\n\t// \treturn orientation < 0;\n\t// }\n\n\ttimeScale(value, suppressEvents) {\n\t\tif (!arguments.length) {\n\t\t\treturn this._rts === -_tinyNum ? 0 : this._rts; // recorded timeScale. Special case: if someone calls reverse() on an animation with timeScale of 0, we assign it -_tinyNum to remember it's reversed.\n\t\t}\n\t\tif (this._rts === value) {\n\t\t\treturn this;\n\t\t}\n\t\tlet tTime = this.parent && this._ts ? _parentToChildTotalTime(this.parent._time, this) : this._tTime; // make sure to do the parentToChildTotalTime() BEFORE setting the new _ts because the old one must be used in that calculation.\n\n\t\t// future addition? Up side: fast and minimal file size. Down side: only works on this animation; if a timeline is reversed, for example, its childrens' onReverse wouldn't get called.\n\t\t//(+value < 0 && this._rts >= 0) && _callback(this, \"onReverse\", true);\n\n\t\t// prioritize rendering where the parent's playhead lines up instead of this._tTime because there could be a tween that's animating another tween's timeScale in the same rendering loop (same parent), thus if the timeScale tween renders first, it would alter _start BEFORE _tTime was set on that tick (in the rendering loop), effectively freezing it until the timeScale tween finishes.\n\t\tthis._rts = +value || 0;\n\t\tthis._ts = (this._ps || value === -_tinyNum) ? 0 : this._rts; // _ts is the functional timeScale which would be 0 if the animation is paused.\n\t\tthis.totalTime(_clamp(-Math.abs(this._delay), this._tDur, tTime), suppressEvents !== false);\n\t\t_setEnd(this); // if parent.smoothChildTiming was false, the end time didn't get updated in the _alignPlayhead() method, so do it here.\n\t\treturn _recacheAncestors(this);\n\t}\n\n\tpaused(value) {\n\t\tif (!arguments.length) {\n\t\t\treturn this._ps;\n\t\t}\n\t\t// possible future addition - if an animation is removed from its parent and then .restart() or .play() or .resume() is called, perhaps we should force it back into the globalTimeline but be careful because what if it's already at its end? We don't want it to just persist forever and not get released for GC.\n\t\t// !this.parent && !value && this._tTime < this._tDur && this !== _globalTimeline && _globalTimeline.add(this);\n\t\tif (this._ps !== value) {\n\t\t\tthis._ps = value;\n\t\t\tif (value) {\n\t\t\t\tthis._pTime = this._tTime || Math.max(-this._delay, this.rawTime()); // if the pause occurs during the delay phase, make sure that's factored in when resuming.\n\t\t\t\tthis._ts = this._act = 0; // _ts is the functional timeScale, so a paused tween would effectively have a timeScale of 0. We record the \"real\" timeScale as _rts (recorded time scale)\n\t\t\t} else {\n\t\t\t\t_wake();\n\t\t\t\tthis._ts = this._rts;\n\t\t\t\t//only defer to _pTime (pauseTime) if tTime is zero. Remember, someone could pause() an animation, then scrub the playhead and resume(). If the parent doesn't have smoothChildTiming, we render at the rawTime() because the startTime won't get updated.\n\t\t\t\tthis.totalTime(this.parent && !this.parent.smoothChildTiming ? this.rawTime() : this._tTime || this._pTime, (this.progress() === 1) && Math.abs(this._zTime) !== _tinyNum && (this._tTime -= _tinyNum)); // edge case: animation.progress(1).pause().play() wouldn't render again because the playhead is already at the end, but the call to totalTime() below will add it back to its parent...and not remove it again (since removing only happens upon rendering at a new time). Offsetting the _tTime slightly is done simply to cause the final render in totalTime() that'll pop it off its timeline (if autoRemoveChildren is true, of course). Check to make sure _zTime isn't -_tinyNum to avoid an edge case where the playhead is pushed to the end but INSIDE a tween/callback, the timeline itself is paused thus halting rendering and leaving a few unrendered. When resuming, it wouldn't render those otherwise.\n\t\t\t}\n\t\t}\n\t\treturn this;\n\t}\n\n\tstartTime(value) {\n\t\tif (arguments.length) {\n\t\t\tthis._start = value;\n\t\t\tlet parent = this.parent || this._dp;\n\t\t\tparent && (parent._sort || !this.parent) && _addToTimeline(parent, this, value - this._delay);\n\t\t\treturn this;\n\t\t}\n\t\treturn this._start;\n\t}\n\n\tendTime(includeRepeats) {\n\t\treturn this._start + (_isNotFalse(includeRepeats) ? this.totalDuration() : this.duration()) / Math.abs(this._ts || 1);\n\t}\n\n\trawTime(wrapRepeats) {\n\t\tlet parent = this.parent || this._dp; // _dp = detached parent\n\t\treturn !parent ? this._tTime : (wrapRepeats && (!this._ts || (this._repeat && this._time && this.totalProgress() < 1))) ? this._tTime % (this._dur + this._rDelay) : !this._ts ? this._tTime : _parentToChildTotalTime(parent.rawTime(wrapRepeats), this);\n\t}\n\n\trevert(config= _revertConfig) {\n\t\tlet prevIsReverting = _reverting;\n\t\t_reverting = config;\n\t\tif (this._initted || this._startAt) {\n\t\t\tthis.timeline && this.timeline.revert(config);\n\t\t\tthis.totalTime(-0.01, config.suppressEvents);\n\t\t}\n\t\tthis.data !== \"nested\" && config.kill !== false && this.kill();\n\t\t_reverting = prevIsReverting;\n\t\treturn this;\n\t}\n\n\tglobalTime(rawTime) {\n\t\tlet animation = this,\n\t\t\ttime = arguments.length ? rawTime : animation.rawTime();\n\t\twhile (animation) {\n\t\t\ttime = animation._start + time / (Math.abs(animation._ts) || 1);\n\t\t\tanimation = animation._dp;\n\t\t}\n\t\treturn !this.parent && this._sat ? this._sat.globalTime(rawTime) : time; // the _startAt tweens for .fromTo() and .from() that have immediateRender should always be FIRST in the timeline (important for context.revert()). \"_sat\" stands for _startAtTween, referring to the parent tween that created the _startAt. We must discern if that tween had immediateRender so that we can know whether or not to prioritize it in revert().\n\t}\n\n\trepeat(value) {\n\t\tif (arguments.length) {\n\t\t\tthis._repeat = value === Infinity ? -2 : value;\n\t\t\treturn _onUpdateTotalDuration(this);\n\t\t}\n\t\treturn this._repeat === -2 ? Infinity : this._repeat;\n\t}\n\n\trepeatDelay(value) {\n\t\tif (arguments.length) {\n\t\t\tlet time = this._time;\n\t\t\tthis._rDelay = value;\n\t\t\t_onUpdateTotalDuration(this);\n\t\t\treturn time ? this.time(time) : this;\n\t\t}\n\t\treturn this._rDelay;\n\t}\n\n\tyoyo(value) {\n\t\tif (arguments.length) {\n\t\t\tthis._yoyo = value;\n\t\t\treturn this;\n\t\t}\n\t\treturn this._yoyo;\n\t}\n\n\tseek(position, suppressEvents) {\n\t\treturn this.totalTime(_parsePosition(this, position), _isNotFalse(suppressEvents));\n\t}\n\n\trestart(includeDelay, suppressEvents) {\n\t\tthis.play().totalTime(includeDelay ? -this._delay : 0, _isNotFalse(suppressEvents));\n\t\tthis._dur || (this._zTime = -_tinyNum); // ensures onComplete fires on a zero-duration animation that gets restarted.\n\t\treturn this;\n\t}\n\n\tplay(from, suppressEvents) {\n\t\tfrom != null && this.seek(from, suppressEvents);\n\t\treturn this.reversed(false).paused(false);\n\t}\n\n\treverse(from, suppressEvents) {\n\t\tfrom != null && this.seek(from || this.totalDuration(), suppressEvents);\n\t\treturn this.reversed(true).paused(false);\n\t}\n\n\tpause(atTime, suppressEvents) {\n\t\tatTime != null && this.seek(atTime, suppressEvents);\n\t\treturn this.paused(true);\n\t}\n\n\tresume() {\n\t\treturn this.paused(false);\n\t}\n\n\treversed(value) {\n\t\tif (arguments.length) {\n\t\t\t!!value !== this.reversed() && this.timeScale(-this._rts || (value ? -_tinyNum : 0)); // in case timeScale is zero, reversing would have no effect so we use _tinyNum.\n\t\t\treturn this;\n\t\t}\n\t\treturn this._rts < 0;\n\t}\n\n\tinvalidate() {\n\t\tthis._initted = this._act = 0;\n\t\tthis._zTime = -_tinyNum;\n\t\treturn this;\n\t}\n\n\tisActive() {\n\t\tlet parent = this.parent || this._dp,\n\t\t\tstart = this._start,\n\t\t\trawTime;\n\t\treturn !!(!parent || (this._ts && this._initted && parent.isActive() && (rawTime = parent.rawTime(true)) >= start && rawTime < this.endTime(true) - _tinyNum));\n\t}\n\n\teventCallback(type, callback, params) {\n\t\tlet vars = this.vars;\n\t\tif (arguments.length > 1) {\n\t\t\tif (!callback) {\n\t\t\t\tdelete vars[type];\n\t\t\t} else {\n\t\t\t\tvars[type] = callback;\n\t\t\t\tparams && (vars[type + \"Params\"] = params);\n\t\t\t\ttype === \"onUpdate\" && (this._onUpdate = callback);\n\t\t\t}\n\t\t\treturn this;\n\t\t}\n\t\treturn vars[type];\n\t}\n\n\tthen(onFulfilled) {\n\t\tlet self = this;\n\t\treturn new Promise(resolve => {\n\t\t\tlet f = _isFunction(onFulfilled) ? onFulfilled : _passThrough,\n\t\t\t\t_resolve = () => {\n\t\t\t\t\tlet _then = self.then;\n\t\t\t\t\tself.then = null; // temporarily null the then() method to avoid an infinite loop (see https://github.com/greensock/GSAP/issues/322)\n\t\t\t\t\t_isFunction(f) && (f = f(self)) && (f.then || f === self) && (self.then = _then);\n\t\t\t\t\tresolve(f);\n\t\t\t\t\tself.then = _then;\n\t\t\t\t};\n\t\t\tif (self._initted && (self.totalProgress() === 1 && self._ts >= 0) || (!self._tTime && self._ts < 0)) {\n\t\t\t\t_resolve();\n\t\t\t} else {\n\t\t\t\tself._prom = _resolve;\n\t\t\t}\n\t\t});\n\t}\n\n\tkill() {\n\t\t_interrupt(this);\n\t}\n\n}\n\n_setDefaults(Animation.prototype, {_time:0, _start:0, _end:0, _tTime:0, _tDur:0, _dirty:0, _repeat:0, _yoyo:false, parent:null, _initted:false, _rDelay:0, _ts:1, _dp:0, ratio:0, _zTime:-_tinyNum, _prom:0, _ps:false, _rts:1});\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * -------------------------------------------------\n * TIMELINE\n * -------------------------------------------------\n */\n\nexport class Timeline extends Animation {\n\n\tconstructor(vars = {}, position) {\n\t\tsuper(vars);\n\t\tthis.labels = {};\n\t\tthis.smoothChildTiming = !!vars.smoothChildTiming;\n\t\tthis.autoRemoveChildren = !!vars.autoRemoveChildren;\n\t\tthis._sort = _isNotFalse(vars.sortChildren);\n\t\t_globalTimeline && _addToTimeline(vars.parent || _globalTimeline, this, position);\n\t\tvars.reversed && this.reverse();\n\t\tvars.paused && this.paused(true);\n\t\tvars.scrollTrigger && _scrollTrigger(this, vars.scrollTrigger);\n\t}\n\n\tto(targets, vars, position) {\n\t\t_createTweenType(0, arguments, this);\n\t\treturn this;\n\t}\n\n\tfrom(targets, vars, position) {\n\t\t_createTweenType(1, arguments, this);\n\t\treturn this;\n\t}\n\n\tfromTo(targets, fromVars, toVars, position) {\n\t\t_createTweenType(2, arguments, this);\n\t\treturn this;\n\t}\n\n\tset(targets, vars, position) {\n\t\tvars.duration = 0;\n\t\tvars.parent = this;\n\t\t_inheritDefaults(vars).repeatDelay || (vars.repeat = 0);\n\t\tvars.immediateRender = !!vars.immediateRender;\n\t\tnew Tween(targets, vars, _parsePosition(this, position), 1);\n\t\treturn this;\n\t}\n\n\tcall(callback, params, position) {\n\t\treturn _addToTimeline(this, Tween.delayedCall(0, callback, params), position);\n\t}\n\n\t//ONLY for backward compatibility! Maybe delete?\n\tstaggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) {\n\t\tvars.duration = duration;\n\t\tvars.stagger = vars.stagger || stagger;\n\t\tvars.onComplete = onCompleteAll;\n\t\tvars.onCompleteParams = onCompleteAllParams;\n\t\tvars.parent = this;\n\t\tnew Tween(targets, vars, _parsePosition(this, position));\n\t\treturn this;\n\t}\n\n\tstaggerFrom(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) {\n\t\tvars.runBackwards = 1;\n\t\t_inheritDefaults(vars).immediateRender = _isNotFalse(vars.immediateRender);\n\t\treturn this.staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams);\n\t}\n\n\tstaggerFromTo(targets, duration, fromVars, toVars, stagger, position, onCompleteAll, onCompleteAllParams) {\n\t\ttoVars.startAt = fromVars;\n\t\t_inheritDefaults(toVars).immediateRender = _isNotFalse(toVars.immediateRender);\n\t\treturn this.staggerTo(targets, duration, toVars, stagger, position, onCompleteAll, onCompleteAllParams);\n\t}\n\n\trender(totalTime, suppressEvents, force) {\n\t\tlet prevTime = this._time,\n\t\t\ttDur = this._dirty ? this.totalDuration() : this._tDur,\n\t\t\tdur = this._dur,\n\t\t\ttTime = totalTime <= 0 ? 0 : _roundPrecise(totalTime), // if a paused timeline is resumed (or its _start is updated for another reason...which rounds it), that could result in the playhead shifting a **tiny** amount and a zero-duration child at that spot may get rendered at a different ratio, like its totalTime in render() may be 1e-17 instead of 0, for example.\n\t\t\tcrossingStart = (this._zTime < 0) !== (totalTime < 0) && (this._initted || !dur),\n\t\t\ttime, child, next, iteration, cycleDuration, prevPaused, pauseTween, timeScale, prevStart, prevIteration, yoyo, isYoyo;\n\t\tthis !== _globalTimeline && tTime > tDur && totalTime >= 0 && (tTime = tDur);\n\t\tif (tTime !== this._tTime || force || crossingStart) {\n\t\t\tif (prevTime !== this._time && dur) { //if totalDuration() finds a child with a negative startTime and smoothChildTiming is true, things get shifted around internally so we need to adjust the time accordingly. For example, if a tween starts at -30 we must shift EVERYTHING forward 30 seconds and move this timeline's startTime backward by 30 seconds so that things align with the playhead (no jump).\n\t\t\t\ttTime += this._time - prevTime;\n\t\t\t\ttotalTime += this._time - prevTime;\n\t\t\t}\n\t\t\ttime = tTime;\n\t\t\tprevStart = this._start;\n\t\t\ttimeScale = this._ts;\n\t\t\tprevPaused = !timeScale;\n\t\t\tif (crossingStart) {\n\t\t\t\tdur || (prevTime = this._zTime);\n\t\t\t\t //when the playhead arrives at EXACTLY time 0 (right on top) of a zero-duration timeline, we need to discern if events are suppressed so that when the playhead moves again (next time), it'll trigger the callback. If events are NOT suppressed, obviously the callback would be triggered in this render. Basically, the callback should fire either when the playhead ARRIVES or LEAVES this exact spot, not both. Imagine doing a timeline.seek(0) and there's a callback that sits at 0. Since events are suppressed on that seek() by default, nothing will fire, but when the playhead moves off of that position, the callback should fire. This behavior is what people intuitively expect.\n\t\t\t\t(totalTime || !suppressEvents) && (this._zTime = totalTime);\n\t\t\t}\n\t\t\tif (this._repeat) { //adjust the time for repeats and yoyos\n\t\t\t\tyoyo = this._yoyo;\n\t\t\t\tcycleDuration = dur + this._rDelay;\n\t\t\t\tif (this._repeat < -1 && totalTime < 0) {\n\t\t\t\t\treturn this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force);\n\t\t\t\t}\n\t\t\t\ttime = _roundPrecise(tTime % cycleDuration); //round to avoid floating point errors. (4 % 0.8 should be 0 but some browsers report it as 0.79999999!)\n\t\t\t\tif (tTime === tDur) { // the tDur === tTime is for edge cases where there's a lengthy decimal on the duration and it may reach the very end but the time is rendered as not-quite-there (remember, tDur is rounded to 4 decimals whereas dur isn't)\n\t\t\t\t\titeration = this._repeat;\n\t\t\t\t\ttime = dur;\n\t\t\t\t} else {\n\t\t\t\t\tprevIteration = _roundPrecise(tTime / cycleDuration); // full decimal version of iterations, not the previous iteration (we're reusing prevIteration variable for efficiency)\n\t\t\t\t\titeration = ~~prevIteration;\n\t\t\t\t\tif (iteration && iteration === prevIteration) {\n\t\t\t\t\t\ttime = dur;\n\t\t\t\t\t\titeration--;\n\t\t\t\t\t}\n\t\t\t\t\ttime > dur && (time = dur);\n\t\t\t\t}\n\t\t\t\tprevIteration = _animationCycle(this._tTime, cycleDuration);\n\t\t\t\t!prevTime && this._tTime && prevIteration !== iteration && this._tTime - prevIteration * cycleDuration - this._dur <= 0 && (prevIteration = iteration); // edge case - if someone does addPause() at the very beginning of a repeating timeline, that pause is technically at the same spot as the end which causes this._time to get set to 0 when the totalTime would normally place the playhead at the end. See https://gsap.com/forums/topic/23823-closing-nav-animation-not-working-on-ie-and-iphone-6-maybe-other-older-browser/?tab=comments#comment-113005 also, this._tTime - prevIteration * cycleDuration - this._dur <= 0 just checks to make sure it wasn't previously in the \"repeatDelay\" portion\n\t\t\t\tif (yoyo && (iteration & 1)) {\n\t\t\t\t\ttime = dur - time;\n\t\t\t\t\tisYoyo = 1;\n\t\t\t\t}\n\t\t\t\t/*\n\t\t\t\tmake sure children at the end/beginning of the timeline are rendered properly. If, for example,\n\t\t\t\ta 3-second long timeline rendered at 2.9 seconds previously, and now renders at 3.2 seconds (which\n\t\t\t\twould get translated to 2.8 seconds if the timeline yoyos or 0.2 seconds if it just repeats), there\n\t\t\t\tcould be a callback or a short tween that's at 2.95 or 3 seconds in which wouldn't render. So\n\t\t\t\twe need to push the timeline to the end (and/or beginning depending on its yoyo value). Also we must\n\t\t\t\tensure that zero-duration tweens at the very beginning or end of the Timeline work.\n\t\t\t\t*/\n\t\t\t\tif (iteration !== prevIteration && !this._lock) {\n\t\t\t\t\tlet rewinding = (yoyo && (prevIteration & 1)),\n\t\t\t\t\t\tdoesWrap = (rewinding === (yoyo && (iteration & 1)));\n\t\t\t\t\titeration < prevIteration && (rewinding = !rewinding);\n\t\t\t\t\tprevTime = rewinding ? 0 : tTime % dur ? dur : tTime; // if the playhead is landing exactly at the end of an iteration, use that totalTime rather than only the duration, otherwise it'll skip the 2nd render since it's effectively at the same time.\n\t\t\t\t\tthis._lock = 1;\n\t\t\t\t\tthis.render(prevTime || (isYoyo ? 0 : _roundPrecise(iteration * cycleDuration)), suppressEvents, !dur)._lock = 0;\n\t\t\t\t\tthis._tTime = tTime; // if a user gets the iteration() inside the onRepeat, for example, it should be accurate.\n\t\t\t\t\t!suppressEvents && this.parent && _callback(this, \"onRepeat\");\n\t\t\t\t\tthis.vars.repeatRefresh && !isYoyo && (this.invalidate()._lock = 1);\n\t\t\t\t\tif ((prevTime && prevTime !== this._time) || prevPaused !== !this._ts || (this.vars.onRepeat && !this.parent && !this._act)) { // if prevTime is 0 and we render at the very end, _time will be the end, thus won't match. So in this edge case, prevTime won't match _time but that's okay. If it gets killed in the onRepeat, eject as well.\n\t\t\t\t\t\treturn this;\n\t\t\t\t\t}\n\t\t\t\t\tdur = this._dur; // in case the duration changed in the onRepeat\n\t\t\t\t\ttDur = this._tDur;\n\t\t\t\t\tif (doesWrap) {\n\t\t\t\t\t\tthis._lock = 2;\n\t\t\t\t\t\tprevTime = rewinding ? dur : -0.0001;\n\t\t\t\t\t\tthis.render(prevTime, true);\n\t\t\t\t\t\tthis.vars.repeatRefresh && !isYoyo && this.invalidate();\n\t\t\t\t\t}\n\t\t\t\t\tthis._lock = 0;\n\t\t\t\t\tif (!this._ts && !prevPaused) {\n\t\t\t\t\t\treturn this;\n\t\t\t\t\t}\n\t\t\t\t\t//in order for yoyoEase to work properly when there's a stagger, we must swap out the ease in each sub-tween.\n\t\t\t\t\t_propagateYoyoEase(this, isYoyo);\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (this._hasPause && !this._forcing && this._lock < 2) {\n\t\t\t\tpauseTween = _findNextPauseTween(this, _roundPrecise(prevTime), _roundPrecise(time));\n\t\t\t\tif (pauseTween) {\n\t\t\t\t\ttTime -= time - (time = pauseTween._start);\n\t\t\t\t}\n\t\t\t}\n\n\t\t\tthis._tTime = tTime;\n\t\t\tthis._time = time;\n\t\t\tthis._act = !timeScale; //as long as it's not paused, force it to be active so that if the user renders independent of the parent timeline, it'll be forced to re-render on the next tick.\n\n\t\t\tif (!this._initted) {\n\t\t\t\tthis._onUpdate = this.vars.onUpdate;\n\t\t\t\tthis._initted = 1;\n\t\t\t\tthis._zTime = totalTime;\n\t\t\t\tprevTime = 0; // upon init, the playhead should always go forward; someone could invalidate() a completed timeline and then if they restart(), that would make child tweens render in reverse order which could lock in the wrong starting values if they build on each other, like tl.to(obj, {x: 100}).to(obj, {x: 0}).\n\t\t\t}\n\t\t\tif (!prevTime && time && !suppressEvents && !iteration) {\n\t\t\t\t_callback(this, \"onStart\");\n\t\t\t\tif (this._tTime !== tTime) { // in case the onStart triggered a render at a different spot, eject. Like if someone did animation.pause(0.5) or something inside the onStart.\n\t\t\t\t\treturn this;\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (time >= prevTime && totalTime >= 0) {\n\t\t\t\tchild = this._first;\n\t\t\t\twhile (child) {\n\t\t\t\t\tnext = child._next;\n\t\t\t\t\tif ((child._act || time >= child._start) && child._ts && pauseTween !== child) {\n\t\t\t\t\t\tif (child.parent !== this) { // an extreme edge case - the child's render could do something like kill() the \"next\" one in the linked list, or reparent it. In that case we must re-initiate the whole render to be safe.\n\t\t\t\t\t\t\treturn this.render(totalTime, suppressEvents, force);\n\t\t\t\t\t\t}\n\t\t\t\t\t\tchild.render(child._ts > 0 ? (time - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (time - child._start) * child._ts, suppressEvents, force);\n\t\t\t\t\t\tif (time !== this._time || (!this._ts && !prevPaused)) { //in case a tween pauses or seeks the timeline when rendering, like inside of an onUpdate/onComplete\n\t\t\t\t\t\t\tpauseTween = 0;\n\t\t\t\t\t\t\tnext && (tTime += (this._zTime = -_tinyNum)); // it didn't finish rendering, so flag zTime as negative so that the next time render() is called it'll be forced (to render any remaining children)\n\t\t\t\t\t\t\tbreak;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tchild = next;\n\t\t\t\t}\n\t\t\t} else {\n\t\t\t\tchild = this._last;\n\t\t\t\tlet adjustedTime = totalTime < 0 ? totalTime : time; //when the playhead goes backward beyond the start of this timeline, we must pass that information down to the child animations so that zero-duration tweens know whether to render their starting or ending values.\n\t\t\t\twhile (child) {\n\t\t\t\t\tnext = child._prev;\n\t\t\t\t\tif ((child._act || adjustedTime <= child._end) && child._ts && pauseTween !== child) {\n\t\t\t\t\t\tif (child.parent !== this) { // an extreme edge case - the child's render could do something like kill() the \"next\" one in the linked list, or reparent it. In that case we must re-initiate the whole render to be safe.\n\t\t\t\t\t\t\treturn this.render(totalTime, suppressEvents, force);\n\t\t\t\t\t\t}\n\t\t\t\t\t\tchild.render(child._ts > 0 ? (adjustedTime - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (adjustedTime - child._start) * child._ts, suppressEvents, force || (_reverting && (child._initted || child._startAt))); // if reverting, we should always force renders of initted tweens (but remember that .fromTo() or .from() may have a _startAt but not _initted yet). If, for example, a .fromTo() tween with a stagger (which creates an internal timeline) gets reverted BEFORE some of its child tweens render for the first time, it may not properly trigger them to revert.\n\t\t\t\t\t\tif (time !== this._time || (!this._ts && !prevPaused)) { //in case a tween pauses or seeks the timeline when rendering, like inside of an onUpdate/onComplete\n\t\t\t\t\t\t\tpauseTween = 0;\n\t\t\t\t\t\t\tnext && (tTime += (this._zTime = adjustedTime ? -_tinyNum : _tinyNum)); // it didn't finish rendering, so adjust zTime so that so that the next time render() is called it'll be forced (to render any remaining children)\n\t\t\t\t\t\t\tbreak;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tchild = next;\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (pauseTween && !suppressEvents) {\n\t\t\t\tthis.pause();\n\t\t\t\tpauseTween.render(time >= prevTime ? 0 : -_tinyNum)._zTime = time >= prevTime ? 1 : -1;\n\t\t\t\tif (this._ts) { //the callback resumed playback! So since we may have held back the playhead due to where the pause is positioned, go ahead and jump to where it's SUPPOSED to be (if no pause happened).\n\t\t\t\t\tthis._start = prevStart; //if the pause was at an earlier time and the user resumed in the callback, it could reposition the timeline (changing its startTime), throwing things off slightly, so we make sure the _start doesn't shift.\n\t\t\t\t\t_setEnd(this);\n\t\t\t\t\treturn this.render(totalTime, suppressEvents, force);\n\t\t\t\t}\n\t\t\t}\n\t\t\tthis._onUpdate && !suppressEvents && _callback(this, \"onUpdate\", true);\n\t\t\tif ((tTime === tDur && this._tTime >= this.totalDuration()) || (!tTime && prevTime)) if (prevStart === this._start || Math.abs(timeScale) !== Math.abs(this._ts)) if (!this._lock) { // remember, a child's callback may alter this timeline's playhead or timeScale which is why we need to add some of these checks.\n\t\t\t\t(totalTime || !dur) && ((tTime === tDur && this._ts > 0) || (!tTime && this._ts < 0)) && _removeFromParent(this, 1); // don't remove if the timeline is reversed and the playhead isn't at 0, otherwise tl.progress(1).reverse() won't work. Only remove if the playhead is at the end and timeScale is positive, or if the playhead is at 0 and the timeScale is negative.\n\t\t\t\tif (!suppressEvents && !(totalTime < 0 && !prevTime) && (tTime || prevTime || !tDur)) {\n\t\t\t\t\t_callback(this, (tTime === tDur && totalTime >= 0 ? \"onComplete\" : \"onReverseComplete\"), true);\n\t\t\t\t\tthis._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom();\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\treturn this;\n\t}\n\n\tadd(child, position) {\n\t\t_isNumber(position) || (position = _parsePosition(this, position, child));\n\t\tif (!(child instanceof Animation)) {\n\t\t\tif (_isArray(child)) {\n\t\t\t\tchild.forEach(obj => this.add(obj, position));\n\t\t\t\treturn this;\n\t\t\t}\n\t\t\tif (_isString(child)) {\n\t\t\t\treturn this.addLabel(child, position);\n\t\t\t}\n\t\t\tif (_isFunction(child)) {\n\t\t\t\tchild = Tween.delayedCall(0, child);\n\t\t\t} else {\n\t\t\t\treturn this;\n\t\t\t}\n\t\t}\n\t\treturn this !== child ? _addToTimeline(this, child, position) : this; //don't allow a timeline to be added to itself as a child!\n\t}\n\n\tgetChildren(nested = true, tweens = true, timelines = true, ignoreBeforeTime = -_bigNum) {\n\t\tlet a = [],\n\t\t\tchild = this._first;\n\t\twhile (child) {\n\t\t\tif (child._start >= ignoreBeforeTime) {\n\t\t\t\tif (child instanceof Tween) {\n\t\t\t\t\ttweens && a.push(child);\n\t\t\t\t} else {\n\t\t\t\t\ttimelines && a.push(child);\n\t\t\t\t\tnested && a.push(...child.getChildren(true, tweens, timelines));\n\t\t\t\t}\n\t\t\t}\n\t\t\tchild = child._next;\n\t\t}\n\t\treturn a;\n\t}\n\n\tgetById(id) {\n\t\tlet animations = this.getChildren(1, 1, 1),\n\t\t\ti = animations.length;\n\t\twhile(i--) {\n\t\t\tif (animations[i].vars.id === id) {\n\t\t\t\treturn animations[i];\n\t\t\t}\n\t\t}\n\t}\n\n\tremove(child) {\n\t\tif (_isString(child)) {\n\t\t\treturn this.removeLabel(child);\n\t\t}\n\t\tif (_isFunction(child)) {\n\t\t\treturn this.killTweensOf(child);\n\t\t}\n\t\tchild.parent === this && _removeLinkedListItem(this, child);\n\t\tif (child === this._recent) {\n\t\t\tthis._recent = this._last;\n\t\t}\n\t\treturn _uncache(this);\n\t}\n\n\ttotalTime(totalTime, suppressEvents) {\n\t\tif (!arguments.length) {\n\t\t\treturn this._tTime;\n\t\t}\n\t\tthis._forcing = 1;\n\t\tif (!this._dp && this._ts) { //special case for the global timeline (or any other that has no parent or detached parent).\n\t\t\tthis._start = _roundPrecise(_ticker.time - (this._ts > 0 ? totalTime / this._ts : (this.totalDuration() - totalTime) / -this._ts));\n\t\t}\n\t\tsuper.totalTime(totalTime, suppressEvents);\n\t\tthis._forcing = 0;\n\t\treturn this;\n\t}\n\n\taddLabel(label, position) {\n\t\tthis.labels[label] = _parsePosition(this, position);\n\t\treturn this;\n\t}\n\n\tremoveLabel(label) {\n\t\tdelete this.labels[label];\n\t\treturn this;\n\t}\n\n\taddPause(position, callback, params) {\n\t\tlet t = Tween.delayedCall(0, callback || _emptyFunc, params);\n\t\tt.data = \"isPause\";\n\t\tthis._hasPause = 1;\n\t\treturn _addToTimeline(this, t, _parsePosition(this, position));\n\t}\n\n\tremovePause(position) {\n\t\tlet child = this._first;\n\t\tposition = _parsePosition(this, position);\n\t\twhile (child) {\n\t\t\tif (child._start === position && child.data === \"isPause\") {\n\t\t\t\t_removeFromParent(child);\n\t\t\t}\n\t\t\tchild = child._next;\n\t\t}\n\t}\n\n\tkillTweensOf(targets, props, onlyActive) {\n\t\tlet tweens = this.getTweensOf(targets, onlyActive),\n\t\t\ti = tweens.length;\n\t\twhile (i--) {\n\t\t\t(_overwritingTween !== tweens[i]) && tweens[i].kill(targets, props);\n\t\t}\n\t\treturn this;\n\t}\n\n\tgetTweensOf(targets, onlyActive) {\n\t\tlet a = [],\n\t\t\tparsedTargets = toArray(targets),\n\t\t\tchild = this._first,\n\t\t\tisGlobalTime = _isNumber(onlyActive), // a number is interpreted as a global time. If the animation spans\n\t\t\tchildren;\n\t\twhile (child) {\n\t\t\tif (child instanceof Tween) {\n\t\t\t\tif (_arrayContainsAny(child._targets, parsedTargets) && (isGlobalTime ? (!_overwritingTween || (child._initted && child._ts)) && child.globalTime(0) <= onlyActive && child.globalTime(child.totalDuration()) > onlyActive : !onlyActive || child.isActive())) { // note: if this is for overwriting, it should only be for tweens that aren't paused and are initted.\n\t\t\t\t\ta.push(child);\n\t\t\t\t}\n\t\t\t} else if ((children = child.getTweensOf(parsedTargets, onlyActive)).length) {\n\t\t\t\ta.push(...children);\n\t\t\t}\n\t\t\tchild = child._next;\n\t\t}\n\t\treturn a;\n\t}\n\n\t// potential future feature - targets() on timelines\n\t// targets() {\n\t// \tlet result = [];\n\t// \tthis.getChildren(true, true, false).forEach(t => result.push(...t.targets()));\n\t// \treturn result.filter((v, i) => result.indexOf(v) === i);\n\t// }\n\n\ttweenTo(position, vars) {\n\t\tvars = vars || {};\n\t\tlet tl = this,\n\t\t\tendTime = _parsePosition(tl, position),\n\t\t\t{ startAt, onStart, onStartParams, immediateRender } = vars,\n\t\t\tinitted,\n\t\t\ttween = Tween.to(tl, _setDefaults({\n\t\t\t\tease: vars.ease || \"none\",\n\t\t\t\tlazy: false,\n\t\t\t\timmediateRender: false,\n\t\t\t\ttime: endTime,\n\t\t\t\toverwrite: \"auto\",\n\t\t\t\tduration: vars.duration || (Math.abs((endTime - ((startAt && \"time\" in startAt) ? startAt.time : tl._time)) / tl.timeScale())) || _tinyNum,\n\t\t\t\tonStart: () => {\n\t\t\t\t\ttl.pause();\n\t\t\t\t\tif (!initted) {\n\t\t\t\t\t\tlet duration = vars.duration || Math.abs((endTime - ((startAt && \"time\" in startAt) ? startAt.time : tl._time)) / tl.timeScale());\n\t\t\t\t\t\t(tween._dur !== duration) && _setDuration(tween, duration, 0, 1).render(tween._time, true, true);\n\t\t\t\t\t\tinitted = 1;\n\t\t\t\t\t}\n\t\t\t\t\tonStart && onStart.apply(tween, onStartParams || []); //in case the user had an onStart in the vars - we don't want to overwrite it.\n\t\t\t\t}\n\t\t\t}, vars));\n\t\treturn immediateRender ? tween.render(0) : tween;\n\t}\n\n\ttweenFromTo(fromPosition, toPosition, vars) {\n\t\treturn this.tweenTo(toPosition, _setDefaults({startAt:{time:_parsePosition(this, fromPosition)}}, vars));\n\t}\n\n\trecent() {\n\t\treturn this._recent;\n\t}\n\n\tnextLabel(afterTime = this._time) {\n\t\treturn _getLabelInDirection(this, _parsePosition(this, afterTime));\n\t}\n\n\tpreviousLabel(beforeTime = this._time) {\n\t\treturn _getLabelInDirection(this, _parsePosition(this, beforeTime), 1);\n\t}\n\n\tcurrentLabel(value) {\n\t\treturn arguments.length ? this.seek(value, true) : this.previousLabel(this._time + _tinyNum);\n\t}\n\n\tshiftChildren(amount, adjustLabels, ignoreBeforeTime = 0) {\n\t\tlet child = this._first,\n\t\t\tlabels = this.labels,\n\t\t\tp;\n\t\twhile (child) {\n\t\t\tif (child._start >= ignoreBeforeTime) {\n\t\t\t\tchild._start += amount;\n\t\t\t\tchild._end += amount;\n\t\t\t}\n\t\t\tchild = child._next;\n\t\t}\n\t\tif (adjustLabels) {\n\t\t\tfor (p in labels) {\n\t\t\t\tif (labels[p] >= ignoreBeforeTime) {\n\t\t\t\t\tlabels[p] += amount;\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\treturn _uncache(this);\n\t}\n\n\tinvalidate(soft) {\n\t\tlet child = this._first;\n\t\tthis._lock = 0;\n\t\twhile (child) {\n\t\t\tchild.invalidate(soft);\n\t\t\tchild = child._next;\n\t\t}\n\t\treturn super.invalidate(soft);\n\t}\n\n\tclear(includeLabels = true) {\n\t\tlet child = this._first,\n\t\t\tnext;\n\t\twhile (child) {\n\t\t\tnext = child._next;\n\t\t\tthis.remove(child);\n\t\t\tchild = next;\n\t\t}\n\t\tthis._dp && (this._time = this._tTime = this._pTime = 0);\n\t\tincludeLabels && (this.labels = {});\n\t\treturn _uncache(this);\n\t}\n\n\ttotalDuration(value) {\n\t\tlet max = 0,\n\t\t\tself = this,\n\t\t\tchild = self._last,\n\t\t\tprevStart = _bigNum,\n\t\t\tprev, start, parent;\n\t\tif (arguments.length) {\n\t\t\treturn self.timeScale((self._repeat < 0 ? self.duration() : self.totalDuration()) / (self.reversed() ? -value : value));\n\t\t}\n\t\tif (self._dirty) {\n\t\t\tparent = self.parent;\n\t\t\twhile (child) {\n\t\t\t\tprev = child._prev; //record it here in case the tween changes position in the sequence...\n\t\t\t\tchild._dirty && child.totalDuration(); //could change the tween._startTime, so make sure the animation's cache is clean before analyzing it.\n\t\t\t\tstart = child._start;\n\t\t\t\tif (start > prevStart && self._sort && child._ts && !self._lock) { //in case one of the tweens shifted out of order, it needs to be re-inserted into the correct position in the sequence\n\t\t\t\t\tself._lock = 1; //prevent endless recursive calls - there are methods that get triggered that check duration/totalDuration when we add().\n\t\t\t\t\t_addToTimeline(self, child, start - child._delay, 1)._lock = 0;\n\t\t\t\t} else {\n\t\t\t\t\tprevStart = start;\n\t\t\t\t}\n\t\t\t\tif (start < 0 && child._ts) { //children aren't allowed to have negative startTimes unless smoothChildTiming is true, so adjust here if one is found.\n\t\t\t\t\tmax -= start;\n\t\t\t\t\tif ((!parent && !self._dp) || (parent && parent.smoothChildTiming)) {\n\t\t\t\t\t\tself._start += start / self._ts;\n\t\t\t\t\t\tself._time -= start;\n\t\t\t\t\t\tself._tTime -= start;\n\t\t\t\t\t}\n\t\t\t\t\tself.shiftChildren(-start, false, -1e999);\n\t\t\t\t\tprevStart = 0;\n\t\t\t\t}\n\t\t\t\tchild._end > max && child._ts && (max = child._end);\n\t\t\t\tchild = prev;\n\t\t\t}\n\t\t\t_setDuration(self, (self === _globalTimeline && self._time > max) ? self._time : max, 1, 1);\n\t\t\tself._dirty = 0;\n\t\t}\n\t\treturn self._tDur;\n\t}\n\n\tstatic updateRoot(time) {\n\t\tif (_globalTimeline._ts) {\n\t\t\t_lazySafeRender(_globalTimeline, _parentToChildTotalTime(time, _globalTimeline));\n\t\t\t_lastRenderedFrame = _ticker.frame;\n\t\t}\n\t\tif (_ticker.frame >= _nextGCFrame) {\n\t\t\t_nextGCFrame += _config.autoSleep || 120;\n\t\t\tlet child = _globalTimeline._first;\n\t\t\tif (!child || !child._ts) if (_config.autoSleep && _ticker._listeners.length < 2) {\n\t\t\t\twhile (child && !child._ts) {\n\t\t\t\t\tchild = child._next;\n\t\t\t\t}\n\t\t\t\tchild || _ticker.sleep();\n\t\t\t}\n\t\t}\n\t}\n\n}\n\n_setDefaults(Timeline.prototype, {_lock:0, _hasPause:0, _forcing:0});\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\nlet _addComplexStringPropTween = function(target, prop, start, end, setter, stringFilter, funcParam) { //note: we call _addComplexStringPropTween.call(tweenInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus \"this\" would refer to the plugin.\n\t\tlet pt = new PropTween(this._pt, target, prop, 0, 1, _renderComplexString, null, setter),\n\t\t\tindex = 0,\n\t\t\tmatchIndex = 0,\n\t\t\tresult,\tstartNums, color, endNum, chunk, startNum, hasRandom, a;\n\t\tpt.b = start;\n\t\tpt.e = end;\n\t\tstart += \"\"; //ensure values are strings\n\t\tend += \"\";\n\t\tif ((hasRandom = ~end.indexOf(\"random(\"))) {\n\t\t\tend = _replaceRandom(end);\n\t\t}\n\t\tif (stringFilter) {\n\t\t\ta = [start, end];\n\t\t\tstringFilter(a, target, prop); //pass an array with the starting and ending values and let the filter do whatever it needs to the values.\n\t\t\tstart = a[0];\n\t\t\tend = a[1];\n\t\t}\n\t\tstartNums = start.match(_complexStringNumExp) || [];\n\t\twhile ((result = _complexStringNumExp.exec(end))) {\n\t\t\tendNum = result[0];\n\t\t\tchunk = end.substring(index, result.index);\n\t\t\tif (color) {\n\t\t\t\tcolor = (color + 1) % 5;\n\t\t\t} else if (chunk.substr(-5) === \"rgba(\") {\n\t\t\t\tcolor = 1;\n\t\t\t}\n\t\t\tif (endNum !== startNums[matchIndex++]) {\n\t\t\t\tstartNum = parseFloat(startNums[matchIndex-1]) || 0;\n\t\t\t\t//these nested PropTweens are handled in a special way - we'll never actually call a render or setter method on them. We'll just loop through them in the parent complex string PropTween's render method.\n\t\t\t\tpt._pt = {\n\t\t\t\t\t_next: pt._pt,\n\t\t\t\t\tp: (chunk || matchIndex === 1) ? chunk : \",\", //note: SVG spec allows omission of comma/space when a negative sign is wedged between two numbers, like 2.5-5.3 instead of 2.5,-5.3 but when tweening, the negative value may switch to positive, so we insert the comma just in case.\n\t\t\t\t\ts: startNum,\n\t\t\t\t\tc: endNum.charAt(1) === \"=\" ? _parseRelative(startNum, endNum) - startNum : parseFloat(endNum) - startNum,\n\t\t\t\t\tm: (color && color < 4) ? Math.round : 0\n\t\t\t\t};\n\t\t\t\tindex = _complexStringNumExp.lastIndex;\n\t\t\t}\n\t\t}\n\t\tpt.c = (index < end.length) ? end.substring(index, end.length) : \"\"; //we use the \"c\" of the PropTween to store the final part of the string (after the last number)\n\t\tpt.fp = funcParam;\n\t\tif (_relExp.test(end) || hasRandom) {\n\t\t\tpt.e = 0; //if the end string contains relative values or dynamic random(...) values, delete the end it so that on the final render we don't actually set it to the string with += or -= characters (forces it to use the calculated value).\n\t\t}\n\t\tthis._pt = pt; //start the linked list with this new PropTween. Remember, we call _addComplexStringPropTween.call(tweenInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus \"this\" would refer to the plugin.\n\t\treturn pt;\n\t},\n\t_addPropTween = function(target, prop, start, end, index, targets, modifier, stringFilter, funcParam, optional) {\n\t\t_isFunction(end) && (end = end(index || 0, target, targets));\n\t\tlet currentValue = target[prop],\n\t\t\tparsedStart = (start !== \"get\") ? start : !_isFunction(currentValue) ? currentValue : (funcParam ? target[(prop.indexOf(\"set\") || !_isFunction(target[\"get\" + prop.substr(3)])) ? prop : \"get\" + prop.substr(3)](funcParam) : target[prop]()),\n\t\t\tsetter = !_isFunction(currentValue) ? _setterPlain : funcParam ? _setterFuncWithParam : _setterFunc,\n\t\t\tpt;\n\t\tif (_isString(end)) {\n\t\t\tif (~end.indexOf(\"random(\")) {\n\t\t\t\tend = _replaceRandom(end);\n\t\t\t}\n\t\t\tif (end.charAt(1) === \"=\") {\n\t\t\t\tpt = _parseRelative(parsedStart, end) + (getUnit(parsedStart) || 0);\n\t\t\t\tif (pt || pt === 0) { // to avoid isNaN, like if someone passes in a value like \"!= whatever\"\n\t\t\t\t\tend = pt;\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tif (!optional || parsedStart !== end || _forceAllPropTweens) {\n\t\t\tif (!isNaN(parsedStart * end) && end !== \"\") { // fun fact: any number multiplied by \"\" is evaluated as the number 0!\n\t\t\t\tpt = new PropTween(this._pt, target, prop, +parsedStart || 0, end - (parsedStart || 0), typeof(currentValue) === \"boolean\" ? _renderBoolean : _renderPlain, 0, setter);\n\t\t\t\tfuncParam && (pt.fp = funcParam);\n\t\t\t\tmodifier && pt.modifier(modifier, this, target);\n\t\t\t\treturn (this._pt = pt);\n\t\t\t}\n\t\t\t!currentValue && !(prop in target) && _missingPlugin(prop, end);\n\t\t\treturn _addComplexStringPropTween.call(this, target, prop, parsedStart, end, setter, stringFilter || _config.stringFilter, funcParam);\n\t\t}\n\t},\n\t//creates a copy of the vars object and processes any function-based values (putting the resulting values directly into the copy) as well as strings with \"random()\" in them. It does NOT process relative values.\n\t_processVars = (vars, index, target, targets, tween) => {\n\t\t_isFunction(vars) && (vars = _parseFuncOrString(vars, tween, index, target, targets));\n\t\tif (!_isObject(vars) || (vars.style && vars.nodeType) || _isArray(vars) || _isTypedArray(vars)) {\n\t\t\treturn _isString(vars) ? _parseFuncOrString(vars, tween, index, target, targets) : vars;\n\t\t}\n\t\tlet copy = {},\n\t\t\tp;\n\t\tfor (p in vars) {\n\t\t\tcopy[p] = _parseFuncOrString(vars[p], tween, index, target, targets);\n\t\t}\n\t\treturn copy;\n\t},\n\t_checkPlugin = (property, vars, tween, index, target, targets) => {\n\t\tlet plugin, pt, ptLookup, i;\n\t\tif (_plugins[property] && (plugin = new _plugins[property]()).init(target, plugin.rawVars ? vars[property] : _processVars(vars[property], index, target, targets, tween), tween, index, targets) !== false) {\n\t\t\ttween._pt = pt = new PropTween(tween._pt, target, property, 0, 1, plugin.render, plugin, 0, plugin.priority);\n\t\t\tif (tween !== _quickTween) {\n\t\t\t\tptLookup = tween._ptLookup[tween._targets.indexOf(target)]; //note: we can't use tween._ptLookup[index] because for staggered tweens, the index from the fullTargets array won't match what it is in each individual tween that spawns from the stagger.\n\t\t\t\ti = plugin._props.length;\n\t\t\t\twhile (i--) {\n\t\t\t\t\tptLookup[plugin._props[i]] = pt;\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\treturn plugin;\n\t},\n\t_overwritingTween, //store a reference temporarily so we can avoid overwriting itself.\n\t_forceAllPropTweens,\n\t_initTween = (tween, time, tTime) => {\n\t\tlet vars = tween.vars,\n\t\t\t{ ease, startAt, immediateRender, lazy, onUpdate, runBackwards, yoyoEase, keyframes, autoRevert } = vars,\n\t\t\tdur = tween._dur,\n\t\t\tprevStartAt = tween._startAt,\n\t\t\ttargets = tween._targets,\n\t\t\tparent = tween.parent,\n\t\t\t//when a stagger (or function-based duration/delay) is on a Tween instance, we create a nested timeline which means that the \"targets\" of that tween don't reflect the parent. This function allows us to discern when it's a nested tween and in that case, return the full targets array so that function-based values get calculated properly. Also remember that if the tween has a stagger AND keyframes, it could be multiple levels deep which is why we store the targets Array in the vars of the timeline.\n\t\t\tfullTargets = (parent && parent.data === \"nested\") ? parent.vars.targets : targets,\n\t\t\tautoOverwrite = (tween._overwrite === \"auto\") && !_suppressOverwrites,\n\t\t\ttl = tween.timeline,\n\t\t\tcleanVars, i, p, pt, target, hasPriority, gsData, harness, plugin, ptLookup, index, harnessVars, overwritten;\n\t\ttl && (!keyframes || !ease) && (ease = \"none\");\n\t\ttween._ease = _parseEase(ease, _defaults.ease);\n\t\ttween._yEase = yoyoEase ? _invertEase(_parseEase(yoyoEase === true ? ease : yoyoEase, _defaults.ease)) : 0;\n\t\tif (yoyoEase && tween._yoyo && !tween._repeat) { //there must have been a parent timeline with yoyo:true that is currently in its yoyo phase, so flip the eases.\n\t\t\tyoyoEase = tween._yEase;\n\t\t\ttween._yEase = tween._ease;\n\t\t\ttween._ease = yoyoEase;\n\t\t}\n\t\ttween._from = !tl && !!vars.runBackwards; //nested timelines should never run backwards - the backwards-ness is in the child tweens.\n\t\tif (!tl || (keyframes && !vars.stagger)) { //if there's an internal timeline, skip all the parsing because we passed that task down the chain.\n\t\t\tharness = targets[0] ? _getCache(targets[0]).harness : 0;\n\t\t\tharnessVars = harness && vars[harness.prop]; //someone may need to specify CSS-specific values AND non-CSS values, like if the element has an \"x\" property plus it's a standard DOM element. We allow people to distinguish by wrapping plugin-specific stuff in a css:{} object for example.\n\t\t\tcleanVars = _copyExcluding(vars, _reservedProps);\n\t\t\tif (prevStartAt) {\n\t\t\t\tprevStartAt._zTime < 0 && prevStartAt.progress(1); // in case it's a lazy startAt that hasn't rendered yet.\n\t\t\t\t(time < 0 && runBackwards && immediateRender && !autoRevert) ? prevStartAt.render(-1, true) : prevStartAt.revert(runBackwards && dur ? _revertConfigNoKill : _startAtRevertConfig); // if it's a \"startAt\" (not \"from()\" or runBackwards: true), we only need to do a shallow revert (keep transforms cached in CSSPlugin)\n\t\t\t\t// don't just _removeFromParent(prevStartAt.render(-1, true)) because that'll leave inline styles. We're creating a new _startAt for \"startAt\" tweens that re-capture things to ensure that if the pre-tween values changed since the tween was created, they're recorded.\n\t\t\t\tprevStartAt._lazy = 0;\n\t\t\t}\n\t\t\tif (startAt) {\n\t\t\t\t_removeFromParent(tween._startAt = Tween.set(targets, _setDefaults({data: \"isStart\", overwrite: false, parent: parent, immediateRender: true, lazy: !prevStartAt && _isNotFalse(lazy), startAt: null, delay: 0, onUpdate: onUpdate && (() => _callback(tween, \"onUpdate\")), stagger: 0}, startAt))); //copy the properties/values into a new object to avoid collisions, like var to = {x:0}, from = {x:500}; timeline.fromTo(e, from, to).fromTo(e, to, from);\n\t\t\t\ttween._startAt._dp = 0; // don't allow it to get put back into root timeline! Like when revert() is called and totalTime() gets set.\n\t\t\t\ttween._startAt._sat = tween; // used in globalTime(). _sat stands for _startAtTween\n\t\t\t\t(time < 0 && (_reverting || (!immediateRender && !autoRevert))) && tween._startAt.revert(_revertConfigNoKill); // rare edge case, like if a render is forced in the negative direction of a non-initted tween.\n\t\t\t\tif (immediateRender) {\n\t\t\t\t\tif (dur && time <= 0 && tTime <= 0) { // check tTime here because in the case of a yoyo tween whose playhead gets pushed to the end like tween.progress(1), we should allow it through so that the onComplete gets fired properly.\n\t\t\t\t\t\ttime && (tween._zTime = time);\n\t\t\t\t\t\treturn; //we skip initialization here so that overwriting doesn't occur until the tween actually begins. Otherwise, if you create several immediateRender:true tweens of the same target/properties to drop into a Timeline, the last one created would overwrite the first ones because they didn't get placed into the timeline yet before the first render occurs and kicks in overwriting.\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t} else if (runBackwards && dur) {\n\t\t\t\t//from() tweens must be handled uniquely: their beginning values must be rendered but we don't want overwriting to occur yet (when time is still 0). Wait until the tween actually begins before doing all the routines like overwriting. At that time, we should render at the END of the tween to ensure that things initialize correctly (remember, from() tweens go backwards)\n\t\t\t\tif (!prevStartAt) {\n\t\t\t\t\ttime && (immediateRender = false); //in rare cases (like if a from() tween runs and then is invalidate()-ed), immediateRender could be true but the initial forced-render gets skipped, so there's no need to force the render in this context when the _time is greater than 0\n\t\t\t\t\tp = _setDefaults({\n\t\t\t\t\t\toverwrite: false,\n\t\t\t\t\t\tdata: \"isFromStart\", //we tag the tween with as \"isFromStart\" so that if [inside a plugin] we need to only do something at the very END of a tween, we have a way of identifying this tween as merely the one that's setting the beginning values for a \"from()\" tween. For example, clearProps in CSSPlugin should only get applied at the very END of a tween and without this tag, from(...{height:100, clearProps:\"height\", delay:1}) would wipe the height at the beginning of the tween and after 1 second, it'd kick back in.\n\t\t\t\t\t\tlazy: immediateRender && !prevStartAt && _isNotFalse(lazy),\n\t\t\t\t\t\timmediateRender: immediateRender, //zero-duration tweens render immediately by default, but if we're not specifically instructed to render this tween immediately, we should skip this and merely _init() to record the starting values (rendering them immediately would push them to completion which is wasteful in that case - we'd have to render(-1) immediately after)\n\t\t\t\t\t\tstagger: 0,\n\t\t\t\t\t\tparent: parent //ensures that nested tweens that had a stagger are handled properly, like gsap.from(\".class\", {y: gsap.utils.wrap([-100,100]), stagger: 0.5})\n\t\t\t\t\t}, cleanVars);\n\t\t\t\t\tharnessVars && (p[harness.prop] = harnessVars); // in case someone does something like .from(..., {css:{}})\n\t\t\t\t\t_removeFromParent(tween._startAt = Tween.set(targets, p));\n\t\t\t\t\ttween._startAt._dp = 0; // don't allow it to get put back into root timeline!\n\t\t\t\t\ttween._startAt._sat = tween; // used in globalTime()\n\t\t\t\t\t(time < 0) && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween._startAt.render(-1, true));\n\t\t\t\t\ttween._zTime = time;\n\t\t\t\t\tif (!immediateRender) {\n\t\t\t\t\t\t_initTween(tween._startAt, _tinyNum, _tinyNum); //ensures that the initial values are recorded\n\t\t\t\t\t} else if (!time) {\n\t\t\t\t\t\treturn;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\ttween._pt = tween._ptCache = 0;\n\t\t\tlazy = (dur && _isNotFalse(lazy)) || (lazy && !dur);\n\t\t\tfor (i = 0; i < targets.length; i++) {\n\t\t\t\ttarget = targets[i];\n\t\t\t\tgsData = target._gsap || _harness(targets)[i]._gsap;\n\t\t\t\ttween._ptLookup[i] = ptLookup = {};\n\t\t\t\t_lazyLookup[gsData.id] && _lazyTweens.length && _lazyRender(); //if other tweens of the same target have recently initted but haven't rendered yet, we've got to force the render so that the starting values are correct (imagine populating a timeline with a bunch of sequential tweens and then jumping to the end)\n\t\t\t\tindex = fullTargets === targets ? i : fullTargets.indexOf(target);\n\t\t\t\tif (harness && (plugin = new harness()).init(target, harnessVars || cleanVars, tween, index, fullTargets) !== false) {\n\t\t\t\t\ttween._pt = pt = new PropTween(tween._pt, target, plugin.name, 0, 1, plugin.render, plugin, 0, plugin.priority);\n\t\t\t\t\tplugin._props.forEach(name => {ptLookup[name] = pt;});\n\t\t\t\t\tplugin.priority && (hasPriority = 1);\n\t\t\t\t}\n\t\t\t\tif (!harness || harnessVars) {\n\t\t\t\t\tfor (p in cleanVars) {\n\t\t\t\t\t\tif (_plugins[p] && (plugin = _checkPlugin(p, cleanVars, tween, index, target, fullTargets))) {\n\t\t\t\t\t\t\tplugin.priority && (hasPriority = 1);\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tptLookup[p] = pt = _addPropTween.call(tween, target, p, \"get\", cleanVars[p], index, fullTargets, 0, vars.stringFilter);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\ttween._op && tween._op[i] && tween.kill(target, tween._op[i]);\n\t\t\t\tif (autoOverwrite && tween._pt) {\n\t\t\t\t\t_overwritingTween = tween;\n\t\t\t\t\t_globalTimeline.killTweensOf(target, ptLookup, tween.globalTime(time)); // make sure the overwriting doesn't overwrite THIS tween!!!\n\t\t\t\t\toverwritten = !tween.parent;\n\t\t\t\t\t_overwritingTween = 0;\n\t\t\t\t}\n\t\t\t\ttween._pt && lazy && (_lazyLookup[gsData.id] = 1);\n\t\t\t}\n\t\t\thasPriority && _sortPropTweensByPriority(tween);\n\t\t\ttween._onInit && tween._onInit(tween); //plugins like RoundProps must wait until ALL of the PropTweens are instantiated. In the plugin's init() function, it sets the _onInit on the tween instance. May not be pretty/intuitive, but it's fast and keeps file size down.\n\t\t}\n\t\ttween._onUpdate = onUpdate;\n\t\ttween._initted = (!tween._op || tween._pt) && !overwritten; // if overwrittenProps resulted in the entire tween being killed, do NOT flag it as initted or else it may render for one tick.\n\t\t(keyframes && time <= 0) && tl.render(_bigNum, true, true); // if there's a 0% keyframe, it'll render in the \"before\" state for any staggered/delayed animations thus when the following tween initializes, it'll use the \"before\" state instead of the \"after\" state as the initial values.\n\t},\n\t_updatePropTweens = (tween, property, value, start, startIsRelative, ratio, time, skipRecursion) => {\n\t\tlet ptCache = ((tween._pt && tween._ptCache) || (tween._ptCache = {}))[property],\n\t\t\tpt, rootPT, lookup, i;\n\t\tif (!ptCache) {\n\t\t\tptCache = tween._ptCache[property] = [];\n\t\t\tlookup = tween._ptLookup;\n\t\t\ti = tween._targets.length;\n\t\t\twhile (i--) {\n\t\t\t\tpt = lookup[i][property];\n\t\t\t\tif (pt && pt.d && pt.d._pt) { // it's a plugin, so find the nested PropTween\n\t\t\t\t\tpt = pt.d._pt;\n\t\t\t\t\twhile (pt && pt.p !== property && pt.fp !== property) { // \"fp\" is functionParam for things like setting CSS variables which require .setProperty(\"--var-name\", value)\n\t\t\t\t\t\tpt = pt._next;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (!pt) { // there is no PropTween associated with that property, so we must FORCE one to be created and ditch out of this\n\t\t\t\t\t// if the tween has other properties that already rendered at new positions, we'd normally have to rewind to put them back like tween.render(0, true) before forcing an _initTween(), but that can create another edge case like tweening a timeline's progress would trigger onUpdates to fire which could move other things around. It's better to just inform users that .resetTo() should ONLY be used for tweens that already have that property. For example, you can't gsap.to(...{ y: 0 }) and then tween.restTo(\"x\", 200) for example.\n\t\t\t\t\t_forceAllPropTweens = 1; // otherwise, when we _addPropTween() and it finds no change between the start and end values, it skips creating a PropTween (for efficiency...why tween when there's no difference?) but in this case we NEED that PropTween created so we can edit it.\n\t\t\t\t\ttween.vars[property] = \"+=0\";\n\t\t\t\t\t_initTween(tween, time);\n\t\t\t\t\t_forceAllPropTweens = 0;\n\t\t\t\t\treturn skipRecursion ? _warn(property + \" not eligible for reset\") : 1; // if someone tries to do a quickTo() on a special property like borderRadius which must get split into 4 different properties, that's not eligible for .resetTo().\n\t\t\t\t}\n\t\t\t\tptCache.push(pt);\n\t\t\t}\n\t\t}\n\t\ti = ptCache.length;\n\t\twhile (i--) {\n\t\t\trootPT = ptCache[i];\n\t\t\tpt = rootPT._pt || rootPT; // complex values may have nested PropTweens. We only accommodate the FIRST value.\n\t\t\tpt.s = (start || start === 0) && !startIsRelative ? start : pt.s + (start || 0) + ratio * pt.c;\n\t\t\tpt.c = value - pt.s;\n\t\t\trootPT.e && (rootPT.e = _round(value) + getUnit(rootPT.e)); // mainly for CSSPlugin (end value)\n\t\t\trootPT.b && (rootPT.b = pt.s + getUnit(rootPT.b)); // (beginning value)\n\t\t}\n\t},\n\t_addAliasesToVars = (targets, vars) => {\n\t\tlet harness = targets[0] ? _getCache(targets[0]).harness : 0,\n\t\t\tpropertyAliases = (harness && harness.aliases),\n\t\t\tcopy, p, i, aliases;\n\t\tif (!propertyAliases) {\n\t\t\treturn vars;\n\t\t}\n\t\tcopy = _merge({}, vars);\n\t\tfor (p in propertyAliases) {\n\t\t\tif (p in copy) {\n\t\t\t\taliases = propertyAliases[p].split(\",\");\n\t\t\t\ti = aliases.length;\n\t\t\t\twhile(i--) {\n\t\t\t\t\tcopy[aliases[i]] = copy[p];\n\t\t\t\t}\n\t\t\t}\n\n\t\t}\n\t\treturn copy;\n\t},\n\t// parses multiple formats, like {\"0%\": {x: 100}, {\"50%\": {x: -20}} and { x: {\"0%\": 100, \"50%\": -20} }, and an \"ease\" can be set on any object. We populate an \"allProps\" object with an Array for each property, like {x: [{}, {}], y:[{}, {}]} with data for each property tween. The objects have a \"t\" (time), \"v\", (value), and \"e\" (ease) property. This allows us to piece together a timeline later.\n\t_parseKeyframe = (prop, obj, allProps, easeEach) => {\n\t\tlet ease = obj.ease || easeEach || \"power1.inOut\",\n\t\t\tp, a;\n\t\tif (_isArray(obj)) {\n\t\t\ta = allProps[prop] || (allProps[prop] = []);\n\t\t\t// t = time (out of 100), v = value, e = ease\n\t\t\tobj.forEach((value, i) => a.push({t: i / (obj.length - 1) * 100, v: value, e: ease}));\n\t\t} else {\n\t\t\tfor (p in obj) {\n\t\t\t\ta = allProps[p] || (allProps[p] = []);\n\t\t\t\tp === \"ease\" || a.push({t: parseFloat(prop), v: obj[p], e: ease});\n\t\t\t}\n\t\t}\n\t},\n\t_parseFuncOrString = (value, tween, i, target, targets) => (_isFunction(value) ? value.call(tween, i, target, targets) : (_isString(value) && ~value.indexOf(\"random(\")) ? _replaceRandom(value) : value),\n\t_staggerTweenProps = _callbackNames + \"repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase,autoRevert\",\n\t_staggerPropsToSkip = {};\n_forEachName(_staggerTweenProps + \",id,stagger,delay,duration,paused,scrollTrigger\", name => _staggerPropsToSkip[name] = 1);\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * TWEEN\n * --------------------------------------------------------------------------------------\n */\n\nexport class Tween extends Animation {\n\n\tconstructor(targets, vars, position, skipInherit) {\n\t\tif (typeof(vars) === \"number\") {\n\t\t\tposition.duration = vars;\n\t\t\tvars = position;\n\t\t\tposition = null;\n\t\t}\n\t\tsuper(skipInherit ? vars : _inheritDefaults(vars));\n\t\tlet { duration, delay, immediateRender, stagger, overwrite, keyframes, defaults, scrollTrigger, yoyoEase } = this.vars,\n\t\t\tparent = vars.parent || _globalTimeline,\n\t\t\tparsedTargets = (_isArray(targets) || _isTypedArray(targets) ? _isNumber(targets[0]) : (\"length\" in vars)) ? [targets] : toArray(targets), // edge case: someone might try animating the \"length\" of an object with a \"length\" property that's initially set to 0 so don't interpret that as an empty Array-like object.\n\t\t\ttl, i, copy, l, p, curTarget, staggerFunc, staggerVarsToMerge;\n\t\tthis._targets = parsedTargets.length ? _harness(parsedTargets) : _warn(\"GSAP target \" + targets + \" not found. https://gsap.com\", !_config.nullTargetWarn) || [];\n\t\tthis._ptLookup = []; //PropTween lookup. An array containing an object for each target, having keys for each tweening property\n\t\tthis._overwrite = overwrite;\n\t\tif (keyframes || stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) {\n\t\t\tvars = this.vars;\n\t\t\ttl = this.timeline = new Timeline({data: \"nested\", defaults: defaults || {}, targets: parent && parent.data === \"nested\" ? parent.vars.targets : parsedTargets}); // we need to store the targets because for staggers and keyframes, we end up creating an individual tween for each but function-based values need to know the index and the whole Array of targets.\n\t\t\ttl.kill();\n\t\t\ttl.parent = tl._dp = this;\n\t\t\ttl._start = 0;\n\t\t\tif (stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) {\n\t\t\t\tl = parsedTargets.length;\n\t\t\t\tstaggerFunc = stagger && distribute(stagger);\n\t\t\t\tif (_isObject(stagger)) { //users can pass in callbacks like onStart/onComplete in the stagger object. These should fire with each individual tween.\n\t\t\t\t\tfor (p in stagger) {\n\t\t\t\t\t\tif (~_staggerTweenProps.indexOf(p)) {\n\t\t\t\t\t\t\tstaggerVarsToMerge || (staggerVarsToMerge = {});\n\t\t\t\t\t\t\tstaggerVarsToMerge[p] = stagger[p];\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tfor (i = 0; i < l; i++) {\n\t\t\t\t\tcopy = _copyExcluding(vars, _staggerPropsToSkip);\n\t\t\t\t\tcopy.stagger = 0;\n\t\t\t\t\tyoyoEase && (copy.yoyoEase = yoyoEase);\n\t\t\t\t\tstaggerVarsToMerge && _merge(copy, staggerVarsToMerge);\n\t\t\t\t\tcurTarget = parsedTargets[i];\n\t\t\t\t\t//don't just copy duration or delay because if they're a string or function, we'd end up in an infinite loop because _isFuncOrString() would evaluate as true in the child tweens, entering this loop, etc. So we parse the value straight from vars and default to 0.\n\t\t\t\t\tcopy.duration = +_parseFuncOrString(duration, this, i, curTarget, parsedTargets);\n\t\t\t\t\tcopy.delay = (+_parseFuncOrString(delay, this, i, curTarget, parsedTargets) || 0) - this._delay;\n\t\t\t\t\tif (!stagger && l === 1 && copy.delay) { // if someone does delay:\"random(1, 5)\", repeat:-1, for example, the delay shouldn't be inside the repeat.\n\t\t\t\t\t\tthis._delay = delay = copy.delay;\n\t\t\t\t\t\tthis._start += delay;\n\t\t\t\t\t\tcopy.delay = 0;\n\t\t\t\t\t}\n\t\t\t\t\ttl.to(curTarget, copy, staggerFunc ? staggerFunc(i, curTarget, parsedTargets) : 0);\n\t\t\t\t\ttl._ease = _easeMap.none;\n\t\t\t\t}\n\t\t\t\ttl.duration() ? (duration = delay = 0) : (this.timeline = 0); // if the timeline's duration is 0, we don't need a timeline internally!\n\t\t\t} else if (keyframes) {\n\t\t\t\t_inheritDefaults(_setDefaults(tl.vars.defaults, {ease:\"none\"}));\n\t\t\t\ttl._ease = _parseEase(keyframes.ease || vars.ease || \"none\");\n\t\t\t\tlet time = 0,\n\t\t\t\t\ta, kf, v;\n\t\t\t\tif (_isArray(keyframes)) {\n\t\t\t\t\tkeyframes.forEach(frame => tl.to(parsedTargets, frame, \">\"));\n\t\t\t\t\ttl.duration(); // to ensure tl._dur is cached because we tap into it for performance purposes in the render() method.\n\t\t\t\t} else {\n\t\t\t\t\tcopy = {};\n\t\t\t\t\tfor (p in keyframes) {\n\t\t\t\t\t\tp === \"ease\" || p === \"easeEach\" || _parseKeyframe(p, keyframes[p], copy, keyframes.easeEach);\n\t\t\t\t\t}\n\t\t\t\t\tfor (p in copy) {\n\t\t\t\t\t\ta = copy[p].sort((a, b) => a.t - b.t);\n\t\t\t\t\t\ttime = 0;\n\t\t\t\t\t\tfor (i = 0; i < a.length; i++) {\n\t\t\t\t\t\t\tkf = a[i];\n\t\t\t\t\t\t\tv = {ease: kf.e, duration: (kf.t - (i ? a[i - 1].t : 0)) / 100 * duration};\n\t\t\t\t\t\t\tv[p] = kf.v;\n\t\t\t\t\t\t\ttl.to(parsedTargets, v, time);\n\t\t\t\t\t\t\ttime += v.duration;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\ttl.duration() < duration && tl.to({}, {duration: duration - tl.duration()}); // in case keyframes didn't go to 100%\n\t\t\t\t}\n\t\t\t}\n\t\t\tduration || this.duration((duration = tl.duration()));\n\n\t\t} else {\n\t\t\tthis.timeline = 0; //speed optimization, faster lookups (no going up the prototype chain)\n\t\t}\n\n\t\tif (overwrite === true && !_suppressOverwrites) {\n\t\t\t_overwritingTween = this;\n\t\t\t_globalTimeline.killTweensOf(parsedTargets);\n\t\t\t_overwritingTween = 0;\n\t\t}\n\t\t_addToTimeline(parent, this, position);\n\t\tvars.reversed && this.reverse();\n\t\tvars.paused && this.paused(true);\n\t\tif (immediateRender || (!duration && !keyframes && this._start === _roundPrecise(parent._time) && _isNotFalse(immediateRender) && _hasNoPausedAncestors(this) && parent.data !== \"nested\")) {\n\t\t\tthis._tTime = -_tinyNum; //forces a render without having to set the render() \"force\" parameter to true because we want to allow lazying by default (using the \"force\" parameter always forces an immediate full render)\n\t\t\tthis.render(Math.max(0, -delay) || 0); //in case delay is negative\n\t\t}\n\t\tscrollTrigger && _scrollTrigger(this, scrollTrigger);\n\t}\n\n\trender(totalTime, suppressEvents, force) {\n\t\tlet prevTime = this._time,\n\t\t\ttDur = this._tDur,\n\t\t\tdur = this._dur,\n\t\t\tisNegative = totalTime < 0,\n\t\t\ttTime = (totalTime > tDur - _tinyNum && !isNegative) ? tDur : (totalTime < _tinyNum) ? 0 : totalTime,\n\t\t\ttime, pt, iteration, cycleDuration, prevIteration, isYoyo, ratio, timeline, yoyoEase;\n\t\tif (!dur) {\n\t\t\t_renderZeroDurationTween(this, totalTime, suppressEvents, force);\n\t\t} else if (tTime !== this._tTime || !totalTime || force || (!this._initted && this._tTime) || (this._startAt && (this._zTime < 0) !== isNegative) || this._lazy) { // this senses if we're crossing over the start time, in which case we must record _zTime and force the render, but we do it in this lengthy conditional way for performance reasons (usually we can skip the calculations): this._initted && (this._zTime < 0) !== (totalTime < 0)\n\t\t\ttime = tTime;\n\t\t\ttimeline = this.timeline;\n\t\t\tif (this._repeat) { //adjust the time for repeats and yoyos\n\t\t\t\tcycleDuration = dur + this._rDelay;\n\t\t\t\tif (this._repeat < -1 && isNegative) {\n\t\t\t\t\treturn this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force);\n\t\t\t\t}\n\t\t\t\ttime = _roundPrecise(tTime % cycleDuration); //round to avoid floating point errors. (4 % 0.8 should be 0 but some browsers report it as 0.79999999!)\n\t\t\t\tif (tTime === tDur) { // the tDur === tTime is for edge cases where there's a lengthy decimal on the duration and it may reach the very end but the time is rendered as not-quite-there (remember, tDur is rounded to 4 decimals whereas dur isn't)\n\t\t\t\t\titeration = this._repeat;\n\t\t\t\t\ttime = dur;\n\t\t\t\t} else {\n\t\t\t\t\tprevIteration = _roundPrecise(tTime / cycleDuration); // full decimal version of iterations, not the previous iteration (we're reusing prevIteration variable for efficiency)\n\t\t\t\t\titeration = ~~prevIteration;\n\t\t\t\t\tif (iteration && iteration === prevIteration) {\n\t\t\t\t\t\ttime = dur;\n\t\t\t\t\t\titeration--;\n\t\t\t\t\t} else if (time > dur) {\n\t\t\t\t\t\ttime = dur;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tisYoyo = this._yoyo && (iteration & 1);\n\t\t\t\tif (isYoyo) {\n\t\t\t\t\tyoyoEase = this._yEase;\n\t\t\t\t\ttime = dur - time;\n\t\t\t\t}\n\t\t\t\tprevIteration = _animationCycle(this._tTime, cycleDuration);\n\t\t\t\tif (time === prevTime && !force && this._initted && iteration === prevIteration) {\n\t\t\t\t\t//could be during the repeatDelay part. No need to render and fire callbacks.\n\t\t\t\t\tthis._tTime = tTime;\n\t\t\t\t\treturn this;\n\t\t\t\t}\n\t\t\t\tif (iteration !== prevIteration) {\n\t\t\t\t\ttimeline && this._yEase && _propagateYoyoEase(timeline, isYoyo);\n\t\t\t\t\t//repeatRefresh functionality\n\t\t\t\t\tif (this.vars.repeatRefresh && !isYoyo && !this._lock && time !== cycleDuration && this._initted) { // this._time will === cycleDuration when we render at EXACTLY the end of an iteration. Without this condition, it'd often do the repeatRefresh render TWICE (again on the very next tick).\n\t\t\t\t\t\tthis._lock = force = 1; //force, otherwise if lazy is true, the _attemptInitTween() will return and we'll jump out and get caught bouncing on each tick.\n\t\t\t\t\t\tthis.render(_roundPrecise(cycleDuration * iteration), true).invalidate()._lock = 0;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\n\t\t\tif (!this._initted) {\n\t\t\t\tif (_attemptInitTween(this, isNegative ? totalTime : time, force, suppressEvents, tTime)) {\n\t\t\t\t\tthis._tTime = 0; // in constructor if immediateRender is true, we set _tTime to -_tinyNum to have the playhead cross the starting point but we can't leave _tTime as a negative number.\n\t\t\t\t\treturn this;\n\t\t\t\t}\n\t\t\t\tif (prevTime !== this._time && !(force && this.vars.repeatRefresh && iteration !== prevIteration)) { // rare edge case - during initialization, an onUpdate in the _startAt (.fromTo()) might force this tween to render at a different spot in which case we should ditch this render() call so that it doesn't revert the values. But we also don't want to dump if we're doing a repeatRefresh render!\n\t\t\t\t\treturn this;\n\t\t\t\t}\n\t\t\t\tif (dur !== this._dur) { // while initting, a plugin like InertiaPlugin might alter the duration, so rerun from the start to ensure everything renders as it should.\n\t\t\t\t\treturn this.render(totalTime, suppressEvents, force);\n\t\t\t\t}\n\t\t\t}\n\n\t\t\tthis._tTime = tTime;\n\t\t\tthis._time = time;\n\n\t\t\tif (!this._act && this._ts) {\n\t\t\t\tthis._act = 1; //as long as it's not paused, force it to be active so that if the user renders independent of the parent timeline, it'll be forced to re-render on the next tick.\n\t\t\t\tthis._lazy = 0;\n\t\t\t}\n\n\t\t\tthis.ratio = ratio = (yoyoEase || this._ease)(time / dur);\n\t\t\tif (this._from) {\n\t\t\t\tthis.ratio = ratio = 1 - ratio;\n\t\t\t}\n\n\t\t\tif (time && !prevTime && !suppressEvents && !iteration) {\n\t\t\t\t_callback(this, \"onStart\");\n\t\t\t\tif (this._tTime !== tTime) { // in case the onStart triggered a render at a different spot, eject. Like if someone did animation.pause(0.5) or something inside the onStart.\n\t\t\t\t\treturn this;\n\t\t\t\t}\n\t\t\t}\n\t\t\tpt = this._pt;\n\t\t\twhile (pt) {\n\t\t\t\tpt.r(ratio, pt.d);\n\t\t\t\tpt = pt._next;\n\t\t\t}\n\t\t\t(timeline && timeline.render(totalTime < 0 ? totalTime : timeline._dur * timeline._ease(time / this._dur), suppressEvents, force)) || (this._startAt && (this._zTime = totalTime));\n\n\t\t\tif (this._onUpdate && !suppressEvents) {\n\t\t\t\tisNegative && _rewindStartAt(this, totalTime, suppressEvents, force); //note: for performance reasons, we tuck this conditional logic inside less traveled areas (most tweens don't have an onUpdate). We'd just have it at the end before the onComplete, but the values should be updated before any onUpdate is called, so we ALSO put it here and then if it's not called, we do so later near the onComplete.\n\t\t\t\t_callback(this, \"onUpdate\");\n\t\t\t}\n\n\t\t\tthis._repeat && iteration !== prevIteration && this.vars.onRepeat && !suppressEvents && this.parent && _callback(this, \"onRepeat\");\n\n\t\t\tif ((tTime === this._tDur || !tTime) && this._tTime === tTime) {\n\t\t\t\tisNegative && !this._onUpdate && _rewindStartAt(this, totalTime, true, true);\n\t\t\t\t(totalTime || !dur) && ((tTime === this._tDur && this._ts > 0) || (!tTime && this._ts < 0)) && _removeFromParent(this, 1); // don't remove if we're rendering at exactly a time of 0, as there could be autoRevert values that should get set on the next tick (if the playhead goes backward beyond the startTime, negative totalTime). Don't remove if the timeline is reversed and the playhead isn't at 0, otherwise tl.progress(1).reverse() won't work. Only remove if the playhead is at the end and timeScale is positive, or if the playhead is at 0 and the timeScale is negative.\n\t\t\t if (!suppressEvents && !(isNegative && !prevTime) && (tTime || prevTime || isYoyo)) { // if prevTime and tTime are zero, we shouldn't fire the onReverseComplete. This could happen if you gsap.to(... {paused:true}).play();\n\t\t\t\t\t_callback(this, (tTime === tDur ? \"onComplete\" : \"onReverseComplete\"), true);\n\t\t\t\t\tthis._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom();\n\t\t\t\t}\n\t\t\t}\n\n\t\t}\n\t\treturn this;\n\t}\n\n\ttargets() {\n\t\treturn this._targets;\n\t}\n\n\tinvalidate(soft) { // \"soft\" gives us a way to clear out everything EXCEPT the recorded pre-\"from\" portion of from() tweens. Otherwise, for example, if you tween.progress(1).render(0, true true).invalidate(), the \"from\" values would persist and then on the next render, the from() tweens would initialize and the current value would match the \"from\" values, thus animate from the same value to the same value (no animation). We tap into this in ScrollTrigger's refresh() where we must push a tween to completion and then back again but honor its init state in case the tween is dependent on another tween further up on the page.\n\t\t(!soft || !this.vars.runBackwards) && (this._startAt = 0)\n\t\tthis._pt = this._op = this._onUpdate = this._lazy = this.ratio = 0;\n\t\tthis._ptLookup = [];\n\t\tthis.timeline && this.timeline.invalidate(soft);\n\t\treturn super.invalidate(soft);\n\t}\n\n\tresetTo(property, value, start, startIsRelative, skipRecursion) {\n\t\t_tickerActive || _ticker.wake();\n\t\tthis._ts || this.play();\n\t\tlet time = Math.min(this._dur, (this._dp._time - this._start) * this._ts),\n\t\t\tratio;\n\t\tthis._initted || _initTween(this, time);\n\t\tratio = this._ease(time / this._dur); // don't just get tween.ratio because it may not have rendered yet.\n\t\t// possible future addition to allow an object with multiple values to update, like tween.resetTo({x: 100, y: 200}); At this point, it doesn't seem worth the added kb given the fact that most users will likely opt for the convenient gsap.quickTo() way of interacting with this method.\n\t\t// if (_isObject(property)) { // performance optimization\n\t\t// \tfor (p in property) {\n\t\t// \t\tif (_updatePropTweens(this, p, property[p], value ? value[p] : null, start, ratio, time)) {\n\t\t// \t\t\treturn this.resetTo(property, value, start, startIsRelative); // if a PropTween wasn't found for the property, it'll get forced with a re-initialization so we need to jump out and start over again.\n\t\t// \t\t}\n\t\t// \t}\n\t\t// } else {\n\t\t\tif (_updatePropTweens(this, property, value, start, startIsRelative, ratio, time, skipRecursion)) {\n\t\t\t\treturn this.resetTo(property, value, start, startIsRelative, 1); // if a PropTween wasn't found for the property, it'll get forced with a re-initialization so we need to jump out and start over again.\n\t\t\t}\n\t\t//}\n\t\t_alignPlayhead(this, 0);\n\t\tthis.parent || _addLinkedListItem(this._dp, this, \"_first\", \"_last\", this._dp._sort ? \"_start\" : 0);\n\t\treturn this.render(0);\n\t}\n\n\tkill(targets, vars = \"all\") {\n\t\tif (!targets && (!vars || vars === \"all\")) {\n\t\t\tthis._lazy = this._pt = 0;\n\t\t\tthis.parent ? _interrupt(this) : this.scrollTrigger && this.scrollTrigger.kill(!!_reverting);\n\t\t\treturn this;\n\t\t}\n\t\tif (this.timeline) {\n\t\t\tlet tDur = this.timeline.totalDuration();\n\t\t\tthis.timeline.killTweensOf(targets, vars, _overwritingTween && _overwritingTween.vars.overwrite !== true)._first || _interrupt(this); // if nothing is left tweening, interrupt.\n\t\t\tthis.parent && tDur !== this.timeline.totalDuration() && _setDuration(this, this._dur * this.timeline._tDur / tDur, 0, 1); // if a nested tween is killed that changes the duration, it should affect this tween's duration. We must use the ratio, though, because sometimes the internal timeline is stretched like for keyframes where they don't all add up to whatever the parent tween's duration was set to.\n\t\t\treturn this;\n\t\t}\n\t\tlet parsedTargets = this._targets,\n\t\t\tkillingTargets = targets ? toArray(targets) : parsedTargets,\n\t\t\tpropTweenLookup = this._ptLookup,\n\t\t\tfirstPT = this._pt,\n\t\t\toverwrittenProps, curLookup, curOverwriteProps, props, p, pt, i;\n\t\tif ((!vars || vars === \"all\") && _arraysMatch(parsedTargets, killingTargets)) {\n\t\t\tvars === \"all\" && (this._pt = 0);\n\t\t\treturn _interrupt(this);\n\t\t}\n\t\toverwrittenProps = this._op = this._op || [];\n\t\tif (vars !== \"all\") { //so people can pass in a comma-delimited list of property names\n\t\t\tif (_isString(vars)) {\n\t\t\t\tp = {};\n\t\t\t\t_forEachName(vars, name => p[name] = 1);\n\t\t\t\tvars = p;\n\t\t\t}\n\t\t\tvars = _addAliasesToVars(parsedTargets, vars);\n\t\t}\n\t\ti = parsedTargets.length;\n\t\twhile (i--) {\n\t\t\tif (~killingTargets.indexOf(parsedTargets[i])) {\n\t\t\t\tcurLookup = propTweenLookup[i];\n\t\t\t\tif (vars === \"all\") {\n\t\t\t\t\toverwrittenProps[i] = vars;\n\t\t\t\t\tprops = curLookup;\n\t\t\t\t\tcurOverwriteProps = {};\n\t\t\t\t} else {\n\t\t\t\t\tcurOverwriteProps = overwrittenProps[i] = overwrittenProps[i] || {};\n\t\t\t\t\tprops = vars;\n\t\t\t\t}\n\t\t\t\tfor (p in props) {\n\t\t\t\t\tpt = curLookup && curLookup[p];\n\t\t\t\t\tif (pt) {\n\t\t\t\t\t\tif (!(\"kill\" in pt.d) || pt.d.kill(p) === true) {\n\t\t\t\t\t\t\t_removeLinkedListItem(this, pt, \"_pt\");\n\t\t\t\t\t\t}\n\t\t\t\t\t\tdelete curLookup[p];\n\t\t\t\t\t}\n\t\t\t\t\tif (curOverwriteProps !== \"all\") {\n\t\t\t\t\t\tcurOverwriteProps[p] = 1;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tthis._initted && !this._pt && firstPT && _interrupt(this); //if all tweening properties are killed, kill the tween. Without this line, if there's a tween with multiple targets and then you killTweensOf() each target individually, the tween would technically still remain active and fire its onComplete even though there aren't any more properties tweening.\n\t\treturn this;\n\t}\n\n\n\tstatic to(targets, vars) {\n\t\treturn new Tween(targets, vars, arguments[2]);\n\t}\n\n\tstatic from(targets, vars) {\n\t\treturn _createTweenType(1, arguments);\n\t}\n\n\tstatic delayedCall(delay, callback, params, scope) {\n\t\treturn new Tween(callback, 0, {immediateRender:false, lazy:false, overwrite:false, delay:delay, onComplete:callback, onReverseComplete:callback, onCompleteParams:params, onReverseCompleteParams:params, callbackScope:scope}); // we must use onReverseComplete too for things like timeline.add(() => {...}) which should be triggered in BOTH directions (forward and reverse)\n\t}\n\n\tstatic fromTo(targets, fromVars, toVars) {\n\t\treturn _createTweenType(2, arguments);\n\t}\n\n\tstatic set(targets, vars) {\n\t\tvars.duration = 0;\n\t\tvars.repeatDelay || (vars.repeat = 0);\n\t\treturn new Tween(targets, vars);\n\t}\n\n\tstatic killTweensOf(targets, props, onlyActive) {\n\t\treturn _globalTimeline.killTweensOf(targets, props, onlyActive);\n\t}\n}\n\n_setDefaults(Tween.prototype, {_targets:[], _lazy:0, _startAt:0, _op:0, _onInit:0});\n\n//add the pertinent timeline methods to Tween instances so that users can chain conveniently and create a timeline automatically. (removed due to concerns that it'd ultimately add to more confusion especially for beginners)\n// _forEachName(\"to,from,fromTo,set,call,add,addLabel,addPause\", name => {\n// \tTween.prototype[name] = function() {\n// \t\tlet tl = new Timeline();\n// \t\treturn _addToTimeline(tl, this)[name].apply(tl, toArray(arguments));\n// \t}\n// });\n\n//for backward compatibility. Leverage the timeline calls.\n_forEachName(\"staggerTo,staggerFrom,staggerFromTo\", name => {\n\tTween[name] = function() {\n\t\tlet tl = new Timeline(),\n\t\t\tparams = _slice.call(arguments, 0);\n\t\tparams.splice(name === \"staggerFromTo\" ? 5 : 4, 0, 0);\n\t\treturn tl[name].apply(tl, params);\n\t}\n});\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * PROPTWEEN\n * --------------------------------------------------------------------------------------\n */\nlet _setterPlain = (target, property, value) => target[property] = value,\n\t_setterFunc = (target, property, value) => target[property](value),\n\t_setterFuncWithParam = (target, property, value, data) => target[property](data.fp, value),\n\t_setterAttribute = (target, property, value) => target.setAttribute(property, value),\n\t_getSetter = (target, property) => _isFunction(target[property]) ? _setterFunc : _isUndefined(target[property]) && target.setAttribute ? _setterAttribute : _setterPlain,\n\t_renderPlain = (ratio, data) => data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 1000000) / 1000000, data),\n\t_renderBoolean = (ratio, data) => data.set(data.t, data.p, !!(data.s + data.c * ratio), data),\n\t_renderComplexString = function(ratio, data) {\n\t\tlet pt = data._pt,\n\t\t\ts = \"\";\n\t\tif (!ratio && data.b) { //b = beginning string\n\t\t\ts = data.b;\n\t\t} else if (ratio === 1 && data.e) { //e = ending string\n\t\t\ts = data.e;\n\t\t} else {\n\t\t\twhile (pt) {\n\t\t\t\ts = pt.p + (pt.m ? pt.m(pt.s + pt.c * ratio) : (Math.round((pt.s + pt.c * ratio) * 10000) / 10000)) + s; //we use the \"p\" property for the text inbetween (like a suffix). And in the context of a complex string, the modifier (m) is typically just Math.round(), like for RGB colors.\n\t\t\t\tpt = pt._next;\n\t\t\t}\n\t\t\ts += data.c; //we use the \"c\" of the PropTween to store the final chunk of non-numeric text.\n\t\t}\n\t\tdata.set(data.t, data.p, s, data);\n\t},\n\t_renderPropTweens = function(ratio, data) {\n\t\tlet pt = data._pt;\n\t\twhile (pt) {\n\t\t\tpt.r(ratio, pt.d);\n\t\t\tpt = pt._next;\n\t\t}\n\t},\n\t_addPluginModifier = function(modifier, tween, target, property) {\n\t\tlet pt = this._pt,\n\t\t\tnext;\n\t\twhile (pt) {\n\t\t\tnext = pt._next;\n\t\t\tpt.p === property && pt.modifier(modifier, tween, target);\n\t\t\tpt = next;\n\t\t}\n\t},\n\t_killPropTweensOf = function(property) {\n\t\tlet pt = this._pt,\n\t\t\thasNonDependentRemaining, next;\n\t\twhile (pt) {\n\t\t\tnext = pt._next;\n\t\t\tif ((pt.p === property && !pt.op) || pt.op === property) {\n\t\t\t\t_removeLinkedListItem(this, pt, \"_pt\");\n\t\t\t} else if (!pt.dep) {\n\t\t\t\thasNonDependentRemaining = 1;\n\t\t\t}\n\t\t\tpt = next;\n\t\t}\n\t\treturn !hasNonDependentRemaining;\n\t},\n\t_setterWithModifier = (target, property, value, data) => {\n\t\tdata.mSet(target, property, data.m.call(data.tween, value, data.mt), data);\n\t},\n\t_sortPropTweensByPriority = parent => {\n\t\tlet pt = parent._pt,\n\t\t\tnext, pt2, first, last;\n\t\t//sorts the PropTween linked list in order of priority because some plugins need to do their work after ALL of the PropTweens were created (like RoundPropsPlugin and ModifiersPlugin)\n\t\twhile (pt) {\n\t\t\tnext = pt._next;\n\t\t\tpt2 = first;\n\t\t\twhile (pt2 && pt2.pr > pt.pr) {\n\t\t\t\tpt2 = pt2._next;\n\t\t\t}\n\t\t\tif ((pt._prev = pt2 ? pt2._prev : last)) {\n\t\t\t\tpt._prev._next = pt;\n\t\t\t} else {\n\t\t\t\tfirst = pt;\n\t\t\t}\n\t\t\tif ((pt._next = pt2)) {\n\t\t\t\tpt2._prev = pt;\n\t\t\t} else {\n\t\t\t\tlast = pt;\n\t\t\t}\n\t\t\tpt = next;\n\t\t}\n\t\tparent._pt = first;\n\t};\n\n//PropTween key: t = target, p = prop, r = renderer, d = data, s = start, c = change, op = overwriteProperty (ONLY populated when it's different than p), pr = priority, _next/_prev for the linked list siblings, set = setter, m = modifier, mSet = modifierSetter (the original setter, before a modifier was added)\nexport class PropTween {\n\n\tconstructor(next, target, prop, start, change, renderer, data, setter, priority) {\n\t\tthis.t = target;\n\t\tthis.s = start;\n\t\tthis.c = change;\n\t\tthis.p = prop;\n\t\tthis.r = renderer || _renderPlain;\n\t\tthis.d = data || this;\n\t\tthis.set = setter || _setterPlain;\n\t\tthis.pr = priority || 0;\n\t\tthis._next = next;\n\t\tif (next) {\n\t\t\tnext._prev = this;\n\t\t}\n\t}\n\n\tmodifier(func, tween, target) {\n\t\tthis.mSet = this.mSet || this.set; //in case it was already set (a PropTween can only have one modifier)\n\t\tthis.set = _setterWithModifier;\n\t\tthis.m = func;\n\t\tthis.mt = target; //modifier target\n\t\tthis.tween = tween;\n\t}\n}\n\n\n\n//Initialization tasks\n_forEachName(_callbackNames + \"parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger\", name => _reservedProps[name] = 1);\n_globals.TweenMax = _globals.TweenLite = Tween;\n_globals.TimelineLite = _globals.TimelineMax = Timeline;\n_globalTimeline = new Timeline({sortChildren: false, defaults: _defaults, autoRemoveChildren: true, id:\"root\", smoothChildTiming: true});\n_config.stringFilter = _colorStringFilter;\n\n\n\n\n\n\n\n\n\n\n\n\n\n\nlet _media = [],\n\t_listeners = {},\n\t_emptyArray = [],\n\t_lastMediaTime = 0,\n\t_contextID = 0,\n\t_dispatch = type => (_listeners[type] || _emptyArray).map(f => f()),\n\t_onMediaChange = () => {\n\t\tlet time = Date.now(),\n\t\t\tmatches = [];\n\t\tif (time - _lastMediaTime > 2) {\n\t\t\t_dispatch(\"matchMediaInit\");\n\t\t\t_media.forEach(c => {\n\t\t\t\tlet queries = c.queries,\n\t\t\t\t\tconditions = c.conditions,\n\t\t\t\t\tmatch, p, anyMatch, toggled;\n\t\t\t\tfor (p in queries) {\n\t\t\t\t\tmatch = _win.matchMedia(queries[p]).matches; // Firefox doesn't update the \"matches\" property of the MediaQueryList object correctly - it only does so as it calls its change handler - so we must re-create a media query here to ensure it's accurate.\n\t\t\t\t\tmatch && (anyMatch = 1);\n\t\t\t\t\tif (match !== conditions[p]) {\n\t\t\t\t\t\tconditions[p] = match;\n\t\t\t\t\t\ttoggled = 1;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (toggled) {\n\t\t\t\t\tc.revert();\n\t\t\t\t\tanyMatch && matches.push(c);\n\t\t\t\t}\n\t\t\t});\n\t\t\t_dispatch(\"matchMediaRevert\");\n\t\t\tmatches.forEach(c => c.onMatch(c, func => c.add(null, func)));\n\t\t\t_lastMediaTime = time;\n\t\t\t_dispatch(\"matchMedia\");\n\t\t}\n\t};\n\nclass Context {\n\tconstructor(func, scope) {\n\t\tthis.selector = scope && selector(scope);\n\t\tthis.data = [];\n\t\tthis._r = []; // returned/cleanup functions\n\t\tthis.isReverted = false;\n\t\tthis.id = _contextID++; // to work around issues that frameworks like Vue cause by making things into Proxies which make it impossible to do something like _media.indexOf(this) because \"this\" would no longer refer to the Context instance itself - it'd refer to a Proxy! We needed a way to identify the context uniquely\n\t\tfunc && this.add(func);\n\t}\n\tadd(name, func, scope) {\n\t\t// possible future addition if we need the ability to add() an animation to a context and for whatever reason cannot create that animation inside of a context.add(() => {...}) function.\n\t\t// if (name && _isFunction(name.revert)) {\n\t\t// \tthis.data.push(name);\n\t\t// \treturn (name._ctx = this);\n\t\t// }\n\t\tif (_isFunction(name)) {\n\t\t\tscope = func;\n\t\t\tfunc = name;\n\t\t\tname = _isFunction;\n\t\t}\n\t\tlet self = this,\n\t\t\tf = function() {\n\t\t\t\tlet prev = _context,\n\t\t\t\t\tprevSelector = self.selector,\n\t\t\t\t\tresult;\n\t\t\t\tprev && prev !== self && prev.data.push(self);\n\t\t\t\tscope && (self.selector = selector(scope));\n\t\t\t\t_context = self;\n\t\t\t\tresult = func.apply(self, arguments);\n\t\t\t\t_isFunction(result) && self._r.push(result);\n\t\t\t\t_context = prev;\n\t\t\t\tself.selector = prevSelector;\n\t\t\t\tself.isReverted = false;\n\t\t\t\treturn result;\n\t\t\t};\n\t\tself.last = f;\n\t\treturn name === _isFunction ? f(self, func => self.add(null, func)) : name ? (self[name] = f) : f;\n\t}\n\tignore(func) {\n\t\tlet prev = _context;\n\t\t_context = null;\n\t\tfunc(this);\n\t\t_context = prev;\n\t}\n\tgetTweens() {\n\t\tlet a = [];\n\t\tthis.data.forEach(e => (e instanceof Context) ? a.push(...e.getTweens()) : (e instanceof Tween) && !(e.parent && e.parent.data === \"nested\") && a.push(e));\n\t\treturn a;\n\t}\n\tclear() {\n\t\tthis._r.length = this.data.length = 0;\n\t}\n\tkill(revert, matchMedia) {\n\t\tif (revert) {\n\t\t\tlet tweens = this.getTweens(),\n\t\t\t\ti = this.data.length,\n\t\t\t\tt;\n\t\t\twhile (i--) { // Flip plugin tweens are very different in that they should actually be pushed to their end. The plugin replaces the timeline's .revert() method to do exactly that. But we also need to remove any of those nested tweens inside the flip timeline so that they don't get individually reverted.\n\t\t\t\tt = this.data[i];\n\t\t\t\tif (t.data === \"isFlip\") {\n\t\t\t\t\tt.revert();\n\t\t\t\t\tt.getChildren(true, true, false).forEach(tween => tweens.splice(tweens.indexOf(tween), 1));\n\t\t\t\t}\n\t\t\t}\n\t\t\t// save as an object so that we can cache the globalTime for each tween to optimize performance during the sort\n\t\t\ttweens.map(t => { return {g: t._dur || t._delay || (t._sat && !t._sat.vars.immediateRender) ? t.globalTime(0) : -Infinity, t}}).sort((a, b) => b.g - a.g || -Infinity).forEach(o => o.t.revert(revert)); // note: all of the _startAt tweens should be reverted in reverse order that they were created, and they'll all have the same globalTime (-1) so the \" || -1\" in the sort keeps the order properly.\n\t\t\ti = this.data.length;\n\t\t\twhile (i--) { // make sure we loop backwards so that, for example, SplitTexts that were created later on the same element get reverted first\n\t\t\t\tt = this.data[i];\n\t\t\t\tif (t instanceof Timeline) {\n\t\t\t\t\tif (t.data !== \"nested\") {\n\t\t\t\t\t\tt.scrollTrigger && t.scrollTrigger.revert();\n\t\t\t\t\t\tt.kill(); // don't revert() the timeline because that's duplicating efforts since we already reverted all the tweens\n\t\t\t\t\t}\n\t\t\t\t} else {\n\t\t\t\t\t!(t instanceof Tween) && t.revert && t.revert(revert)\n\t\t\t\t}\n\t\t\t}\n\t\t\tthis._r.forEach(f => f(revert, this));\n\t\t\tthis.isReverted = true;\n\t\t} else {\n\t\t\tthis.data.forEach(e => e.kill && e.kill());\n\t\t}\n\t\tthis.clear();\n\t\tif (matchMedia) {\n\t\t\tlet i = _media.length;\n\t\t\twhile (i--) { // previously, we checked _media.indexOf(this), but some frameworks like Vue enforce Proxy objects that make it impossible to get the proper result that way, so we must use a unique ID number instead.\n\t\t\t\t_media[i].id === this.id && _media.splice(i, 1);\n\t\t\t}\n\t\t}\n\t}\n\n\t// killWithCleanup() {\n\t// \tthis.kill();\n\t// \tthis._r.forEach(f => f(false, this));\n\t// }\n\n\trevert(config) {\n\t\tthis.kill(config || {});\n\t}\n}\n\n\n\n\nclass MatchMedia {\n\tconstructor(scope) {\n\t\tthis.contexts = [];\n\t\tthis.scope = scope;\n\t\t_context && _context.data.push(this);\n\t}\n\tadd(conditions, func, scope) {\n\t\t_isObject(conditions) || (conditions = {matches: conditions});\n\t\tlet context = new Context(0, scope || this.scope),\n\t\t\tcond = context.conditions = {},\n\t\t\tmq, p, active;\n\t\t_context && !context.selector && (context.selector = _context.selector); // in case a context is created inside a context. Like a gsap.matchMedia() that's inside a scoped gsap.context()\n\t\tthis.contexts.push(context);\n\t\tfunc = context.add(\"onMatch\", func);\n\t\tcontext.queries = conditions;\n\t\tfor (p in conditions) {\n\t\t\tif (p === \"all\") {\n\t\t\t\tactive = 1;\n\t\t\t} else {\n\t\t\t\tmq = _win.matchMedia(conditions[p]);\n\t\t\t\tif (mq) {\n\t\t\t\t\t_media.indexOf(context) < 0 && _media.push(context);\n\t\t\t\t\t(cond[p] = mq.matches) && (active = 1);\n\t\t\t\t\tmq.addListener ? mq.addListener(_onMediaChange) : mq.addEventListener(\"change\", _onMediaChange);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\tactive && func(context, f => context.add(null, f));\n\t\treturn this;\n\t}\n\t// refresh() {\n\t// \tlet time = _lastMediaTime,\n\t// \t\tmedia = _media;\n\t// \t_lastMediaTime = -1;\n\t// \t_media = this.contexts;\n\t// \t_onMediaChange();\n\t// \t_lastMediaTime = time;\n\t// \t_media = media;\n\t// }\n\trevert(config) {\n\t\tthis.kill(config || {});\n\t}\n\tkill(revert) {\n\t\tthis.contexts.forEach(c => c.kill(revert, true));\n\t}\n}\n\n\n\n/*\n * --------------------------------------------------------------------------------------\n * GSAP\n * --------------------------------------------------------------------------------------\n */\nconst _gsap = {\n\tregisterPlugin(...args) {\n\t\targs.forEach(config => _createPlugin(config));\n\t},\n\ttimeline(vars) {\n\t\treturn new Timeline(vars);\n\t},\n\tgetTweensOf(targets, onlyActive) {\n\t\treturn _globalTimeline.getTweensOf(targets, onlyActive);\n\t},\n\tgetProperty(target, property, unit, uncache) {\n\t\t_isString(target) && (target = toArray(target)[0]); //in case selector text or an array is passed in\n\t\tlet getter = _getCache(target || {}).get,\n\t\t\tformat = unit ? _passThrough : _numericIfPossible;\n\t\tunit === \"native\" && (unit = \"\");\n\t\treturn !target ? target : !property ? (property, unit, uncache) => format(((_plugins[property] && _plugins[property].get) || getter)(target, property, unit, uncache)) : format(((_plugins[property] && _plugins[property].get) || getter)(target, property, unit, uncache));\n\t},\n\tquickSetter(target, property, unit) {\n\t\ttarget = toArray(target);\n\t\tif (target.length > 1) {\n\t\t\tlet setters = target.map(t => gsap.quickSetter(t, property, unit)),\n\t\t\t\tl = setters.length;\n\t\t\treturn value => {\n\t\t\t\tlet i = l;\n\t\t\t\twhile(i--) {\n\t\t\t\t\tsetters[i](value);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\ttarget = target[0] || {};\n\t\tlet Plugin = _plugins[property],\n\t\t\tcache = _getCache(target),\n\t\t\tp = (cache.harness && (cache.harness.aliases || {})[property]) || property, // in case it's an alias, like \"rotate\" for \"rotation\".\n\t\t\tsetter = Plugin ? value => {\n\t\t\t\tlet p = new Plugin();\n\t\t\t\t_quickTween._pt = 0;\n\t\t\t\tp.init(target, unit ? value + unit : value, _quickTween, 0, [target]);\n\t\t\t\tp.render(1, p);\n\t\t\t\t_quickTween._pt && _renderPropTweens(1, _quickTween);\n\t\t\t} : cache.set(target, p);\n\t\treturn Plugin ? setter : value => setter(target, p, unit ? value + unit : value, cache, 1);\n\t},\n\tquickTo(target, property, vars) {\n\t\tlet tween = gsap.to(target, _setDefaults({[property]: \"+=0.1\", paused: true, stagger: 0}, vars || {})),\n\t\t\tfunc = (value, start, startIsRelative) => tween.resetTo(property, value, start, startIsRelative);\n\t\tfunc.tween = tween;\n\t\treturn func;\n\t},\n\tisTweening(targets) {\n\t\treturn _globalTimeline.getTweensOf(targets, true).length > 0;\n\t},\n\tdefaults(value) {\n\t\tvalue && value.ease && (value.ease = _parseEase(value.ease, _defaults.ease));\n\t\treturn _mergeDeep(_defaults, value || {});\n\t},\n\tconfig(value) {\n\t\treturn _mergeDeep(_config, value || {});\n\t},\n\tregisterEffect({name, effect, plugins, defaults, extendTimeline}) {\n\t\t(plugins || \"\").split(\",\").forEach(pluginName => pluginName && !_plugins[pluginName] && !_globals[pluginName] && _warn(name + \" effect requires \" + pluginName + \" plugin.\"));\n\t\t_effects[name] = (targets, vars, tl) => effect(toArray(targets), _setDefaults(vars || {}, defaults), tl);\n\t\tif (extendTimeline) {\n\t\t\tTimeline.prototype[name] = function(targets, vars, position) {\n\t\t\t\treturn this.add(_effects[name](targets, _isObject(vars) ? vars : (position = vars) && {}, this), position);\n\t\t\t};\n\t\t}\n\t},\n\tregisterEase(name, ease) {\n\t\t_easeMap[name] = _parseEase(ease);\n\t},\n\tparseEase(ease, defaultEase) {\n\t\treturn arguments.length ? _parseEase(ease, defaultEase) : _easeMap;\n\t},\n\tgetById(id) {\n\t\treturn _globalTimeline.getById(id);\n\t},\n\texportRoot(vars = {}, includeDelayedCalls) {\n\t\tlet tl = new Timeline(vars),\n\t\t\tchild, next;\n\t\ttl.smoothChildTiming = _isNotFalse(vars.smoothChildTiming);\n\t\t_globalTimeline.remove(tl);\n\t\ttl._dp = 0; //otherwise it'll get re-activated when adding children and be re-introduced into _globalTimeline's linked list (then added to itself).\n\t\ttl._time = tl._tTime = _globalTimeline._time;\n\t\tchild = _globalTimeline._first;\n\t\twhile (child) {\n\t\t\tnext = child._next;\n\t\t\tif (includeDelayedCalls || !(!child._dur && child instanceof Tween && child.vars.onComplete === child._targets[0])) {\n\t\t\t\t_addToTimeline(tl, child, child._start - child._delay);\n\t\t\t}\n\t\t\tchild = next;\n\t\t}\n\t\t_addToTimeline(_globalTimeline, tl, 0);\n\t\treturn tl;\n\t},\n\tcontext: (func, scope) => func ? new Context(func, scope) : _context,\n\tmatchMedia: scope => new MatchMedia(scope),\n\tmatchMediaRefresh: () => _media.forEach(c => {\n\t\tlet cond = c.conditions,\n\t\t\tfound, p;\n\t\tfor (p in cond) {\n\t\t\tif (cond[p]) {\n\t\t\t\tcond[p] = false;\n\t\t\t\tfound = 1;\n\t\t\t}\n\t\t}\n\t\tfound && c.revert();\n\t}) || _onMediaChange(),\n\taddEventListener(type, callback) {\n\t\tlet a = _listeners[type] || (_listeners[type] = []);\n\t\t~a.indexOf(callback) || a.push(callback);\n\t},\n\tremoveEventListener(type, callback) {\n\t\tlet a = _listeners[type],\n\t\t\ti = a && a.indexOf(callback);\n\t\ti >= 0 && a.splice(i, 1);\n\t},\n\tutils: { wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, selector, mapRange, pipe, unitize, interpolate, shuffle },\n\tinstall: _install,\n\teffects: _effects,\n\tticker: _ticker,\n\tupdateRoot: Timeline.updateRoot,\n\tplugins: _plugins,\n\tglobalTimeline: _globalTimeline,\n\tcore: {PropTween, globals: _addGlobal, Tween, Timeline, Animation, getCache: _getCache, _removeLinkedListItem, reverting: () => _reverting, context: toAdd => {if (toAdd && _context) { _context.data.push(toAdd); toAdd._ctx = _context} return _context; }, suppressOverwrites: value => _suppressOverwrites = value}\n};\n\n_forEachName(\"to,from,fromTo,delayedCall,set,killTweensOf\", name => _gsap[name] = Tween[name]);\n_ticker.add(Timeline.updateRoot);\n_quickTween = _gsap.to({}, {duration:0});\n\n\n\n\n// ---- EXTRA PLUGINS --------------------------------------------------------\n\n\nlet _getPluginPropTween = (plugin, prop) => {\n\t\tlet pt = plugin._pt;\n\t\twhile (pt && pt.p !== prop && pt.op !== prop && pt.fp !== prop) {\n\t\t\tpt = pt._next;\n\t\t}\n\t\treturn pt;\n\t},\n\t_addModifiers = (tween, modifiers) => {\n\t\t\tlet\ttargets = tween._targets,\n\t\t\t\tp, i, pt;\n\t\t\tfor (p in modifiers) {\n\t\t\t\ti = targets.length;\n\t\t\t\twhile (i--) {\n\t\t\t\t\tpt = tween._ptLookup[i][p];\n\t\t\t\t\tif (pt && (pt = pt.d)) {\n\t\t\t\t\t\tif (pt._pt) { // is a plugin\n\t\t\t\t\t\t\tpt = _getPluginPropTween(pt, p);\n\t\t\t\t\t\t}\n\t\t\t\t\t\tpt && pt.modifier && pt.modifier(modifiers[p], tween, targets[i], p);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t},\n\t_buildModifierPlugin = (name, modifier) => {\n\t\treturn {\n\t\t\tname: name,\n\t\t\trawVars: 1, //don't pre-process function-based values or \"random()\" strings.\n\t\t\tinit(target, vars, tween) {\n\t\t\t\ttween._onInit = tween => {\n\t\t\t\t\tlet temp, p;\n\t\t\t\t\tif (_isString(vars)) {\n\t\t\t\t\t\ttemp = {};\n\t\t\t\t\t\t_forEachName(vars, name => temp[name] = 1); //if the user passes in a comma-delimited list of property names to roundProps, like \"x,y\", we round to whole numbers.\n\t\t\t\t\t\tvars = temp;\n\t\t\t\t\t}\n\t\t\t\t\tif (modifier) {\n\t\t\t\t\t\ttemp = {};\n\t\t\t\t\t\tfor (p in vars) {\n\t\t\t\t\t\t\ttemp[p] = modifier(vars[p]);\n\t\t\t\t\t\t}\n\t\t\t\t\t\tvars = temp;\n\t\t\t\t\t}\n\t\t\t\t\t_addModifiers(tween, vars);\n\t\t\t\t};\n\t\t\t}\n\t\t};\n\t};\n\n//register core plugins\nexport const gsap = _gsap.registerPlugin({\n\t\tname:\"attr\",\n\t\tinit(target, vars, tween, index, targets) {\n\t\t\tlet p, pt, v;\n\t\t\tthis.tween = tween;\n\t\t\tfor (p in vars) {\n\t\t\t\tv = target.getAttribute(p) || \"\";\n\t\t\t\tpt = this.add(target, \"setAttribute\", (v || 0) + \"\", vars[p], index, targets, 0, 0, p);\n\t\t\t\tpt.op = p;\n\t\t\t\tpt.b = v; // record the beginning value so we can revert()\n\t\t\t\tthis._props.push(p);\n\t\t\t}\n\t\t},\n\t\trender(ratio, data) {\n\t\t\tlet pt = data._pt;\n\t\t\twhile (pt) {\n\t\t\t\t_reverting ? pt.set(pt.t, pt.p, pt.b, pt) : pt.r(ratio, pt.d); // if reverting, go back to the original (pt.b)\n\t\t\t\tpt = pt._next;\n\t\t\t}\n\t\t}\n\t}, {\n\t\tname:\"endArray\",\n\t\tinit(target, value) {\n\t\t\tlet i = value.length;\n\t\t\twhile (i--) {\n\t\t\t\tthis.add(target, i, target[i] || 0, value[i], 0, 0, 0, 0, 0, 1);\n\t\t\t}\n\t\t}\n\t},\n\t_buildModifierPlugin(\"roundProps\", _roundModifier),\n\t_buildModifierPlugin(\"modifiers\"),\n\t_buildModifierPlugin(\"snap\", snap)\n) || _gsap; //to prevent the core plugins from being dropped via aggressive tree shaking, we must include them in the variable declaration in this way.\n\nTween.version = Timeline.version = gsap.version = \"3.12.7\";\n_coreReady = 1;\n_windowExists() && _wake();\n\nexport const { Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ } = _easeMap;\nexport { Tween as TweenMax, Tween as TweenLite, Timeline as TimelineMax, Timeline as TimelineLite, gsap as default, wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, selector, mapRange, pipe, unitize, interpolate, shuffle };\n//export some internal methods/orojects for use in CSSPlugin so that we can externalize that file and allow custom builds that exclude it.\nexport { _getProperty, _numExp, _numWithUnitExp, _isString, _isUndefined, _renderComplexString, _relExp, _setDefaults, _removeLinkedListItem, _forEachName, _sortPropTweensByPriority, _colorStringFilter, _replaceRandom, _checkPlugin, _plugins, _ticker, _config, _roundModifier, _round, _missingPlugin, _getSetter, _getCache, _colorExp, _parseRelative }","/*!\n * CSSPlugin 3.12.7\n * https://gsap.com\n *\n * Copyright 2008-2025, GreenSock. All rights reserved.\n * Subject to the terms at https://gsap.com/standard-license or for\n * Club GSAP members, the agreement issued with that membership.\n * @author: Jack Doyle, jack@greensock.com\n*/\n/* eslint-disable */\n\nimport {gsap, _getProperty, _numExp, _numWithUnitExp, getUnit, _isString, _isUndefined, _renderComplexString, _relExp, _forEachName, _sortPropTweensByPriority, _colorStringFilter, _checkPlugin, _replaceRandom, _plugins, GSCache, PropTween, _config, _ticker, _round, _missingPlugin, _getSetter, _getCache, _colorExp, _parseRelative,\n\t_setDefaults, _removeLinkedListItem //for the commented-out className feature.\n} from \"./gsap-core.js\";\n\nlet _win, _doc, _docElement, _pluginInitted, _tempDiv, _tempDivStyler, _recentSetterPlugin, _reverting,\n\t_windowExists = () => typeof(window) !== \"undefined\",\n\t_transformProps = {},\n\t_RAD2DEG = 180 / Math.PI,\n\t_DEG2RAD = Math.PI / 180,\n\t_atan2 = Math.atan2,\n\t_bigNum = 1e8,\n\t_capsExp = /([A-Z])/g,\n\t_horizontalExp = /(left|right|width|margin|padding|x)/i,\n\t_complexExp = /[\\s,\\(]\\S/,\n\t_propertyAliases = {autoAlpha:\"opacity,visibility\", scale:\"scaleX,scaleY\", alpha:\"opacity\"},\n\t_renderCSSProp = (ratio, data) => data.set(data.t, data.p, (Math.round((data.s + data.c * ratio) * 10000) / 10000) + data.u, data),\n\t_renderPropWithEnd = (ratio, data) => data.set(data.t, data.p, ratio === 1 ? data.e : (Math.round((data.s + data.c * ratio) * 10000) / 10000) + data.u, data),\n\t_renderCSSPropWithBeginning = (ratio, data) => data.set(data.t, data.p, ratio ? (Math.round((data.s + data.c * ratio) * 10000) / 10000) + data.u : data.b, data), //if units change, we need a way to render the original unit/value when the tween goes all the way back to the beginning (ratio:0)\n\t_renderRoundedCSSProp = (ratio, data) => {\n\t\tlet value = data.s + data.c * ratio;\n\t\tdata.set(data.t, data.p, ~~(value + (value < 0 ? -.5 : .5)) + data.u, data);\n\t},\n\t_renderNonTweeningValue = (ratio, data) => data.set(data.t, data.p, ratio ? data.e : data.b, data),\n\t_renderNonTweeningValueOnlyAtEnd = (ratio, data) => data.set(data.t, data.p, ratio !== 1 ? data.b : data.e, data),\n\t_setterCSSStyle = (target, property, value) => target.style[property] = value,\n\t_setterCSSProp = (target, property, value) => target.style.setProperty(property, value),\n\t_setterTransform = (target, property, value) => target._gsap[property] = value,\n\t_setterScale = (target, property, value) => target._gsap.scaleX = target._gsap.scaleY = value,\n\t_setterScaleWithRender = (target, property, value, data, ratio) => {\n\t\tlet cache = target._gsap;\n\t\tcache.scaleX = cache.scaleY = value;\n\t\tcache.renderTransform(ratio, cache);\n\t},\n\t_setterTransformWithRender = (target, property, value, data, ratio) => {\n\t\tlet cache = target._gsap;\n\t\tcache[property] = value;\n\t\tcache.renderTransform(ratio, cache);\n\t},\n\t_transformProp = \"transform\",\n\t_transformOriginProp = _transformProp + \"Origin\",\n\t_saveStyle = function(property, isNotCSS) {\n\t\tlet target = this.target,\n\t\t\tstyle = target.style,\n\t\t\tcache = target._gsap;\n\t\tif ((property in _transformProps) && style) {\n\t\t\tthis.tfm = this.tfm || {};\n\t\t\tif (property !== \"transform\") {\n\t\t\t\tproperty = _propertyAliases[property] || property;\n\t\t\t\t~property.indexOf(\",\") ? property.split(\",\").forEach(a => this.tfm[a] = _get(target, a)) : (this.tfm[property] = cache.x ? cache[property] : _get(target, property)); // note: scale would map to \"scaleX,scaleY\", thus we loop and apply them both.\n\t\t\t\tproperty === _transformOriginProp && (this.tfm.zOrigin = cache.zOrigin);\n\t\t\t} else {\n\t\t\t\treturn _propertyAliases.transform.split(\",\").forEach(p => _saveStyle.call(this, p, isNotCSS));\n\t\t\t}\n\t\t\tif (this.props.indexOf(_transformProp) >= 0) { return; }\n\t\t\tif (cache.svg) {\n\t\t\t\tthis.svgo = target.getAttribute(\"data-svg-origin\");\n\t\t\t\tthis.props.push(_transformOriginProp, isNotCSS, \"\");\n\t\t\t}\n\t\t\tproperty = _transformProp;\n\t\t}\n\t\t(style || isNotCSS) && this.props.push(property, isNotCSS, style[property]);\n\t},\n\t_removeIndependentTransforms = style => {\n\t\tif (style.translate) {\n\t\t\tstyle.removeProperty(\"translate\");\n\t\t\tstyle.removeProperty(\"scale\");\n\t\t\tstyle.removeProperty(\"rotate\");\n\t\t}\n\t},\n\t_revertStyle = function() {\n\t\tlet props = this.props,\n\t\t\ttarget = this.target,\n\t\t\tstyle = target.style,\n\t\t\tcache = target._gsap,\n\t\t\ti, p;\n\t\tfor (i = 0; i < props.length; i+=3) { // stored like this: property, isNotCSS, value\n\t\t\tif (!props[i+1]) {\n\t\t\t\tprops[i+2] ? (style[props[i]] = props[i+2]) : style.removeProperty(props[i].substr(0,2) === \"--\" ? props[i] : props[i].replace(_capsExp, \"-$1\").toLowerCase());\n\t\t\t} else if (props[i+1] === 2) { // non-CSS value (function-based)\n\t\t\t\ttarget[props[i]](props[i+2]);\n\t\t\t} else { // non-CSS value (not function-based)\n\t\t\t\ttarget[props[i]] = props[i+2];\n\t\t\t}\n\t\t}\n\t\tif (this.tfm) {\n\t\t\tfor (p in this.tfm) {\n\t\t\t\tcache[p] = this.tfm[p];\n\t\t\t}\n\t\t\tif (cache.svg) {\n\t\t\t\tcache.renderTransform();\n\t\t\t\ttarget.setAttribute(\"data-svg-origin\", this.svgo || \"\");\n\t\t\t}\n\t\t\ti = _reverting();\n\t\t\tif ((!i || !i.isStart) && !style[_transformProp]) {\n\t\t\t\t_removeIndependentTransforms(style);\n\t\t\t\tif (cache.zOrigin && style[_transformOriginProp]) {\n\t\t\t\t\tstyle[_transformOriginProp] += \" \" + cache.zOrigin + \"px\"; // since we're uncaching, we must put the zOrigin back into the transformOrigin so that we can pull it out accurately when we parse again. Otherwise, we'd lose the z portion of the origin since we extract it to protect from Safari bugs.\n\t\t\t\t\tcache.zOrigin = 0;\n\t\t\t\t\tcache.renderTransform();\n\t\t\t\t}\n\t\t\t\tcache.uncache = 1; // if it's a startAt that's being reverted in the _initTween() of the core, we don't need to uncache transforms. This is purely a performance optimization.\n\t\t\t}\n\t\t}\n\t},\n\t_getStyleSaver = (target, properties) => {\n\t\tlet saver = {\n\t\t\ttarget,\n\t\t\tprops: [],\n\t\t\trevert: _revertStyle,\n\t\t\tsave: _saveStyle\n\t\t};\n\t\ttarget._gsap || gsap.core.getCache(target); // just make sure there's a _gsap cache defined because we read from it in _saveStyle() and it's more efficient to just check it here once.\n\t\tproperties && target.style && target.nodeType && properties.split(\",\").forEach(p => saver.save(p)); // make sure it's a DOM node too.\n\t\treturn saver;\n\t},\n\t_supports3D,\n\t_createElement = (type, ns) => {\n\t\tlet e = _doc.createElementNS ? _doc.createElementNS((ns || \"http://www.w3.org/1999/xhtml\").replace(/^https/, \"http\"), type) : _doc.createElement(type); //some servers swap in https for http in the namespace which can break things, making \"style\" inaccessible.\n\t\treturn e && e.style ? e : _doc.createElement(type); //some environments won't allow access to the element's style when created with a namespace in which case we default to the standard createElement() to work around the issue. Also note that when GSAP is embedded directly inside an SVG file, createElement() won't allow access to the style object in Firefox (see https://gsap.com/forums/topic/20215-problem-using-tweenmax-in-standalone-self-containing-svg-file-err-cannot-set-property-csstext-of-undefined/).\n\t},\n\t_getComputedProperty = (target, property, skipPrefixFallback) => {\n\t\tlet cs = getComputedStyle(target);\n\t\treturn cs[property] || cs.getPropertyValue(property.replace(_capsExp, \"-$1\").toLowerCase()) || cs.getPropertyValue(property) || (!skipPrefixFallback && _getComputedProperty(target, _checkPropPrefix(property) || property, 1)) || \"\"; //css variables may not need caps swapped out for dashes and lowercase.\n\t},\n\t_prefixes = \"O,Moz,ms,Ms,Webkit\".split(\",\"),\n\t_checkPropPrefix = (property, element, preferPrefix) => {\n\t\tlet e = element || _tempDiv,\n\t\t\ts = e.style,\n\t\t\ti = 5;\n\t\tif (property in s && !preferPrefix) {\n\t\t\treturn property;\n\t\t}\n\t\tproperty = property.charAt(0).toUpperCase() + property.substr(1);\n\t\twhile (i-- && !((_prefixes[i]+property) in s)) { }\n\t\treturn (i < 0) ? null : ((i === 3) ? \"ms\" : (i >= 0) ? _prefixes[i] : \"\") + property;\n\t},\n\t_initCore = () => {\n\t\tif (_windowExists() && window.document) {\n\t\t\t_win = window;\n\t\t\t_doc = _win.document;\n\t\t\t_docElement = _doc.documentElement;\n\t\t\t_tempDiv = _createElement(\"div\") || {style:{}};\n\t\t\t_tempDivStyler = _createElement(\"div\");\n\t\t\t_transformProp = _checkPropPrefix(_transformProp);\n\t\t\t_transformOriginProp = _transformProp + \"Origin\";\n\t\t\t_tempDiv.style.cssText = \"border-width:0;line-height:0;position:absolute;padding:0\"; //make sure to override certain properties that may contaminate measurements, in case the user has overreaching style sheets.\n\t\t\t_supports3D = !!_checkPropPrefix(\"perspective\");\n\t\t\t_reverting = gsap.core.reverting;\n\t\t\t_pluginInitted = 1;\n\t\t}\n\t},\n\t_getReparentedCloneBBox = target => { //works around issues in some browsers (like Firefox) that don't correctly report getBBox() on SVG elements inside a <defs> element and/or <mask>. We try creating an SVG, adding it to the documentElement and toss the element in there so that it's definitely part of the rendering tree, then grab the bbox and if it works, we actually swap out the original getBBox() method for our own that does these extra steps whenever getBBox is needed. This helps ensure that performance is optimal (only do all these extra steps when absolutely necessary...most elements don't need it).\n\t\tlet owner = target.ownerSVGElement,\n\t\t\tsvg = _createElement(\"svg\", (owner && owner.getAttribute(\"xmlns\")) || \"http://www.w3.org/2000/svg\"),\n\t\t\tclone = target.cloneNode(true),\n\t\t\tbbox;\n\t\tclone.style.display = \"block\";\n\t\tsvg.appendChild(clone);\n\t\t_docElement.appendChild(svg);\n\t\ttry {\n\t\t\tbbox = clone.getBBox();\n\t\t} catch (e) { }\n\t\tsvg.removeChild(clone);\n\t\t_docElement.removeChild(svg);\n\t\treturn bbox;\n\t},\n\t_getAttributeFallbacks = (target, attributesArray) => {\n\t\tlet i = attributesArray.length;\n\t\twhile (i--) {\n\t\t\tif (target.hasAttribute(attributesArray[i])) {\n\t\t\t\treturn target.getAttribute(attributesArray[i]);\n\t\t\t}\n\t\t}\n\t},\n\t_getBBox = target => {\n\t\tlet bounds, cloned;\n\t\ttry {\n\t\t\tbounds = target.getBBox(); //Firefox throws errors if you try calling getBBox() on an SVG element that's not rendered (like in a <symbol> or <defs>). https://bugzilla.mozilla.org/show_bug.cgi?id=612118\n\t\t} catch (error) {\n\t\t\tbounds = _getReparentedCloneBBox(target);\n\t\t\tcloned = 1;\n\t\t}\n\t\t(bounds && (bounds.width || bounds.height)) || cloned || (bounds = _getReparentedCloneBBox(target));\n\t\t//some browsers (like Firefox) misreport the bounds if the element has zero width and height (it just assumes it's at x:0, y:0), thus we need to manually grab the position in that case.\n\t\treturn (bounds && !bounds.width && !bounds.x && !bounds.y) ? {x: +_getAttributeFallbacks(target, [\"x\",\"cx\",\"x1\"]) || 0, y:+_getAttributeFallbacks(target, [\"y\",\"cy\",\"y1\"]) || 0, width:0, height:0} : bounds;\n\t},\n\t_isSVG = e => !!(e.getCTM && (!e.parentNode || e.ownerSVGElement) && _getBBox(e)), //reports if the element is an SVG on which getBBox() actually works\n\t_removeProperty = (target, property) => {\n\t\tif (property) {\n\t\t\tlet style = target.style,\n\t\t\t\tfirst2Chars;\n\t\t\tif (property in _transformProps && property !== _transformOriginProp) {\n\t\t\t\tproperty = _transformProp;\n\t\t\t}\n\t\t\tif (style.removeProperty) {\n\t\t\t\tfirst2Chars = property.substr(0,2);\n\t\t\t\tif (first2Chars === \"ms\" || property.substr(0,6) === \"webkit\") { //Microsoft and some Webkit browsers don't conform to the standard of capitalizing the first prefix character, so we adjust so that when we prefix the caps with a dash, it's correct (otherwise it'd be \"ms-transform\" instead of \"-ms-transform\" for IE9, for example)\n\t\t\t\t\tproperty = \"-\" + property;\n\t\t\t\t}\n\t\t\t\tstyle.removeProperty(first2Chars === \"--\" ? property : property.replace(_capsExp, \"-$1\").toLowerCase());\n\t\t\t} else { //note: old versions of IE use \"removeAttribute()\" instead of \"removeProperty()\"\n\t\t\t\tstyle.removeAttribute(property);\n\t\t\t}\n\t\t}\n\t},\n\t_addNonTweeningPT = (plugin, target, property, beginning, end, onlySetAtEnd) => {\n\t\tlet pt = new PropTween(plugin._pt, target, property, 0, 1, onlySetAtEnd ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue);\n\t\tplugin._pt = pt;\n\t\tpt.b = beginning;\n\t\tpt.e = end;\n\t\tplugin._props.push(property);\n\t\treturn pt;\n\t},\n\t_nonConvertibleUnits = {deg:1, rad:1, turn:1},\n\t_nonStandardLayouts = {grid:1, flex:1},\n\t//takes a single value like 20px and converts it to the unit specified, like \"%\", returning only the numeric amount.\n\t_convertToUnit = (target, property, value, unit) => {\n\t\tlet curValue = parseFloat(value) || 0,\n\t\t\tcurUnit = (value + \"\").trim().substr((curValue + \"\").length) || \"px\", // some browsers leave extra whitespace at the beginning of CSS variables, hence the need to trim()\n\t\t\tstyle = _tempDiv.style,\n\t\t\thorizontal = _horizontalExp.test(property),\n\t\t\tisRootSVG = target.tagName.toLowerCase() === \"svg\",\n\t\t\tmeasureProperty = (isRootSVG ? \"client\" : \"offset\") + (horizontal ? \"Width\" : \"Height\"),\n\t\t\tamount = 100,\n\t\t\ttoPixels = unit === \"px\",\n\t\t\ttoPercent = unit === \"%\",\n\t\t\tpx, parent, cache, isSVG;\n\t\tif (unit === curUnit || !curValue || _nonConvertibleUnits[unit] || _nonConvertibleUnits[curUnit]) {\n\t\t\treturn curValue;\n\t\t}\n\t\t(curUnit !== \"px\" && !toPixels) && (curValue = _convertToUnit(target, property, value, \"px\"));\n\t\tisSVG = target.getCTM && _isSVG(target);\n\t\tif ((toPercent || curUnit === \"%\") && (_transformProps[property] || ~property.indexOf(\"adius\"))) {\n\t\t\tpx = isSVG ? target.getBBox()[horizontal ? \"width\" : \"height\"] : target[measureProperty];\n\t\t\treturn _round(toPercent ? curValue / px * amount : curValue / 100 * px);\n\t\t}\n\t\tstyle[horizontal ? \"width\" : \"height\"] = amount + (toPixels ? curUnit : unit);\n\t\tparent = ((unit !== \"rem\" && ~property.indexOf(\"adius\")) || (unit === \"em\" && target.appendChild && !isRootSVG)) ? target : target.parentNode;\n\t\tif (isSVG) {\n\t\t\tparent = (target.ownerSVGElement || {}).parentNode;\n\t\t}\n\t\tif (!parent || parent === _doc || !parent.appendChild) {\n\t\t\tparent = _doc.body;\n\t\t}\n\t\tcache = parent._gsap;\n\t\tif (cache && toPercent && cache.width && horizontal && cache.time === _ticker.time && !cache.uncache) {\n\t\t\treturn _round(curValue / cache.width * amount);\n\t\t} else {\n\t\t\tif (toPercent && (property === \"height\" || property === \"width\")) { // if we're dealing with width/height that's inside a container with padding and/or it's a flexbox/grid container, we must apply it to the target itself rather than the _tempDiv in order to ensure complete accuracy, factoring in the parent's padding.\n\t\t\t\tlet v = target.style[property];\n\t\t\t\ttarget.style[property] = amount + unit;\n\t\t\t\tpx = target[measureProperty];\n\t\t\t\tv ? (target.style[property] = v) : _removeProperty(target, property);\n\t\t\t} else {\n\t\t\t\t(toPercent || curUnit === \"%\") && !_nonStandardLayouts[_getComputedProperty(parent, \"display\")] && (style.position = _getComputedProperty(target, \"position\"));\n\t\t\t\t(parent === target) && (style.position = \"static\"); // like for borderRadius, if it's a % we must have it relative to the target itself but that may not have position: relative or position: absolute in which case it'd go up the chain until it finds its offsetParent (bad). position: static protects against that.\n\t\t\t\tparent.appendChild(_tempDiv);\n\t\t\t\tpx = _tempDiv[measureProperty];\n\t\t\t\tparent.removeChild(_tempDiv);\n\t\t\t\tstyle.position = \"absolute\";\n\t\t\t}\n\t\t\tif (horizontal && toPercent) {\n\t\t\t\tcache = _getCache(parent);\n\t\t\t\tcache.time = _ticker.time;\n\t\t\t\tcache.width = parent[measureProperty];\n\t\t\t}\n\t\t}\n\t\treturn _round(toPixels ? px * curValue / amount : px && curValue ? amount / px * curValue : 0);\n\t},\n\t_get = (target, property, unit, uncache) => {\n\t\tlet value;\n\t\t_pluginInitted || _initCore();\n\t\tif ((property in _propertyAliases) && property !== \"transform\") {\n\t\t\tproperty = _propertyAliases[property];\n\t\t\tif (~property.indexOf(\",\")) {\n\t\t\t\tproperty = property.split(\",\")[0];\n\t\t\t}\n\t\t}\n\t\tif (_transformProps[property] && property !== \"transform\") {\n\t\t\tvalue = _parseTransform(target, uncache);\n\t\t\tvalue = (property !== \"transformOrigin\") ? value[property] : value.svg ? value.origin : _firstTwoOnly(_getComputedProperty(target, _transformOriginProp)) + \" \" + value.zOrigin + \"px\";\n\t\t} else {\n\t\t\tvalue = target.style[property];\n\t\t\tif (!value || value === \"auto\" || uncache || ~(value + \"\").indexOf(\"calc(\")) {\n\t\t\t\tvalue = (_specialProps[property] && _specialProps[property](target, property, unit)) || _getComputedProperty(target, property) || _getProperty(target, property) || (property === \"opacity\" ? 1 : 0); // note: some browsers, like Firefox, don't report borderRadius correctly! Instead, it only reports every corner like borderTopLeftRadius\n\t\t\t}\n\t\t}\n\t\treturn unit && !~(value + \"\").trim().indexOf(\" \") ? _convertToUnit(target, property, value, unit) + unit : value;\n\n\t},\n\t_tweenComplexCSSString = function(target, prop, start, end) { // note: we call _tweenComplexCSSString.call(pluginInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus \"this\" would refer to the plugin.\n\t\tif (!start || start === \"none\") { // some browsers like Safari actually PREFER the prefixed property and mis-report the unprefixed value like clipPath (BUG). In other words, even though clipPath exists in the style (\"clipPath\" in target.style) and it's set in the CSS properly (along with -webkit-clip-path), Safari reports clipPath as \"none\" whereas WebkitClipPath reports accurately like \"ellipse(100% 0% at 50% 0%)\", so in this case we must SWITCH to using the prefixed property instead. See https://gsap.com/forums/topic/18310-clippath-doesnt-work-on-ios/\n\t\t\tlet p = _checkPropPrefix(prop, target, 1),\n\t\t\t\ts = p && _getComputedProperty(target, p, 1);\n\t\t\tif (s && s !== start) {\n\t\t\t\tprop = p;\n\t\t\t\tstart = s;\n\t\t\t} else if (prop === \"borderColor\") {\n\t\t\t\tstart = _getComputedProperty(target, \"borderTopColor\"); // Firefox bug: always reports \"borderColor\" as \"\", so we must fall back to borderTopColor. See https://gsap.com/forums/topic/24583-how-to-return-colors-that-i-had-after-reverse/\n\t\t\t}\n\t\t}\n\t\tlet pt = new PropTween(this._pt, target.style, prop, 0, 1, _renderComplexString),\n\t\t\tindex = 0,\n\t\t\tmatchIndex = 0,\n\t\t\ta, result,\tstartValues, startNum, color, startValue, endValue, endNum, chunk, endUnit, startUnit, endValues;\n\t\tpt.b = start;\n\t\tpt.e = end;\n\t\tstart += \"\"; // ensure values are strings\n\t\tend += \"\";\n\t\tif (end === \"auto\") {\n\t\t\tstartValue = target.style[prop];\n\t\t\ttarget.style[prop] = end;\n\t\t\tend = _getComputedProperty(target, prop) || end;\n\t\t\tstartValue ? (target.style[prop] = startValue) : _removeProperty(target, prop);\n\t\t}\n\t\ta = [start, end];\n\t\t_colorStringFilter(a); // pass an array with the starting and ending values and let the filter do whatever it needs to the values. If colors are found, it returns true and then we must match where the color shows up order-wise because for things like boxShadow, sometimes the browser provides the computed values with the color FIRST, but the user provides it with the color LAST, so flip them if necessary. Same for drop-shadow().\n\t\tstart = a[0];\n\t\tend = a[1];\n\t\tstartValues = start.match(_numWithUnitExp) || [];\n\t\tendValues = end.match(_numWithUnitExp) || [];\n\t\tif (endValues.length) {\n\t\t\twhile ((result = _numWithUnitExp.exec(end))) {\n\t\t\t\tendValue = result[0];\n\t\t\t\tchunk = end.substring(index, result.index);\n\t\t\t\tif (color) {\n\t\t\t\t\tcolor = (color + 1) % 5;\n\t\t\t\t} else if (chunk.substr(-5) === \"rgba(\" || chunk.substr(-5) === \"hsla(\") {\n\t\t\t\t\tcolor = 1;\n\t\t\t\t}\n\t\t\t\tif (endValue !== (startValue = startValues[matchIndex++] || \"\")) {\n\t\t\t\t\tstartNum = parseFloat(startValue) || 0;\n\t\t\t\t\tstartUnit = startValue.substr((startNum + \"\").length);\n\t\t\t\t\t(endValue.charAt(1) === \"=\") && (endValue = _parseRelative(startNum, endValue) + startUnit);\n\t\t\t\t\tendNum = parseFloat(endValue);\n\t\t\t\t\tendUnit = endValue.substr((endNum + \"\").length);\n\t\t\t\t\tindex = _numWithUnitExp.lastIndex - endUnit.length;\n\t\t\t\t\tif (!endUnit) { //if something like \"perspective:300\" is passed in and we must add a unit to the end\n\t\t\t\t\t\tendUnit = endUnit || _config.units[prop] || startUnit;\n\t\t\t\t\t\tif (index === end.length) {\n\t\t\t\t\t\t\tend += endUnit;\n\t\t\t\t\t\t\tpt.e += endUnit;\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (startUnit !== endUnit) {\n\t\t\t\t\t\tstartNum = _convertToUnit(target, prop, startValue, endUnit) || 0;\n\t\t\t\t\t}\n\t\t\t\t\t// these nested PropTweens are handled in a special way - we'll never actually call a render or setter method on them. We'll just loop through them in the parent complex string PropTween's render method.\n\t\t\t\t\tpt._pt = {\n\t\t\t\t\t\t_next: pt._pt,\n\t\t\t\t\t\tp: (chunk || (matchIndex === 1)) ? chunk : \",\", //note: SVG spec allows omission of comma/space when a negative sign is wedged between two numbers, like 2.5-5.3 instead of 2.5,-5.3 but when tweening, the negative value may switch to positive, so we insert the comma just in case.\n\t\t\t\t\t\ts: startNum,\n\t\t\t\t\t\tc: endNum - startNum,\n\t\t\t\t\t\tm: (color && color < 4) || prop === \"zIndex\" ? Math.round : 0\n\t\t\t\t\t};\n\t\t\t\t}\n\t\t\t}\n\t\t\tpt.c = (index < end.length) ? end.substring(index, end.length) : \"\"; //we use the \"c\" of the PropTween to store the final part of the string (after the last number)\n\t\t} else {\n\t\t\tpt.r = prop === \"display\" && end === \"none\" ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue;\n\t\t}\n\t\t_relExp.test(end) && (pt.e = 0); //if the end string contains relative values or dynamic random(...) values, delete the end it so that on the final render we don't actually set it to the string with += or -= characters (forces it to use the calculated value).\n\t\tthis._pt = pt; //start the linked list with this new PropTween. Remember, we call _tweenComplexCSSString.call(pluginInstance...) to ensure that it's scoped properly. We may call it from within another plugin too, thus \"this\" would refer to the plugin.\n\t\treturn pt;\n\t},\n\t_keywordToPercent = {top:\"0%\", bottom:\"100%\", left:\"0%\", right:\"100%\", center:\"50%\"},\n\t_convertKeywordsToPercentages = value => {\n\t\tlet split = value.split(\" \"),\n\t\t\tx = split[0],\n\t\t\ty = split[1] || \"50%\";\n\t\tif (x === \"top\" || x === \"bottom\" || y === \"left\" || y === \"right\") { //the user provided them in the wrong order, so flip them\n\t\t\tvalue = x;\n\t\t\tx = y;\n\t\t\ty = value;\n\t\t}\n\t\tsplit[0] = _keywordToPercent[x] || x;\n\t\tsplit[1] = _keywordToPercent[y] || y;\n\t\treturn split.join(\" \");\n\t},\n\t_renderClearProps = (ratio, data) => {\n\t\tif (data.tween && data.tween._time === data.tween._dur) {\n\t\t\tlet target = data.t,\n\t\t\t\tstyle = target.style,\n\t\t\t\tprops = data.u,\n\t\t\t\tcache = target._gsap,\n\t\t\t\tprop, clearTransforms, i;\n\t\t\tif (props === \"all\" || props === true) {\n\t\t\t\tstyle.cssText = \"\";\n\t\t\t\tclearTransforms = 1;\n\t\t\t} else {\n\t\t\t\tprops = props.split(\",\");\n\t\t\t\ti = props.length;\n\t\t\t\twhile (--i > -1) {\n\t\t\t\t\tprop = props[i];\n\t\t\t\t\tif (_transformProps[prop]) {\n\t\t\t\t\t\tclearTransforms = 1;\n\t\t\t\t\t\tprop = (prop === \"transformOrigin\") ? _transformOriginProp : _transformProp;\n\t\t\t\t\t}\n\t\t\t\t\t_removeProperty(target, prop);\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (clearTransforms) {\n\t\t\t\t_removeProperty(target, _transformProp);\n\t\t\t\tif (cache) {\n\t\t\t\t\tcache.svg && target.removeAttribute(\"transform\");\n\t\t\t\t\tstyle.scale = style.rotate = style.translate = \"none\";\n\t\t\t\t\t_parseTransform(target, 1); // force all the cached values back to \"normal\"/identity, otherwise if there's another tween that's already set to render transforms on this element, it could display the wrong values.\n\t\t\t\t\tcache.uncache = 1;\n\t\t\t\t\t_removeIndependentTransforms(style);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t},\n\t// note: specialProps should return 1 if (and only if) they have a non-zero priority. It indicates we need to sort the linked list.\n\t_specialProps = {\n\t\tclearProps(plugin, target, property, endValue, tween) {\n\t\t\tif (tween.data !== \"isFromStart\") {\n\t\t\t\tlet pt = plugin._pt = new PropTween(plugin._pt, target, property, 0, 0, _renderClearProps);\n\t\t\t\tpt.u = endValue;\n\t\t\t\tpt.pr = -10;\n\t\t\t\tpt.tween = tween;\n\t\t\t\tplugin._props.push(property);\n\t\t\t\treturn 1;\n\t\t\t}\n\t\t}\n\t\t/* className feature (about 0.4kb gzipped).\n\t\t, className(plugin, target, property, endValue, tween) {\n\t\t\tlet _renderClassName = (ratio, data) => {\n\t\t\t\t\tdata.css.render(ratio, data.css);\n\t\t\t\t\tif (!ratio || ratio === 1) {\n\t\t\t\t\t\tlet inline = data.rmv,\n\t\t\t\t\t\t\ttarget = data.t,\n\t\t\t\t\t\t\tp;\n\t\t\t\t\t\ttarget.setAttribute(\"class\", ratio ? data.e : data.b);\n\t\t\t\t\t\tfor (p in inline) {\n\t\t\t\t\t\t\t_removeProperty(target, p);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t},\n\t\t\t\t_getAllStyles = (target) => {\n\t\t\t\t\tlet styles = {},\n\t\t\t\t\t\tcomputed = getComputedStyle(target),\n\t\t\t\t\t\tp;\n\t\t\t\t\tfor (p in computed) {\n\t\t\t\t\t\tif (isNaN(p) && p !== \"cssText\" && p !== \"length\") {\n\t\t\t\t\t\t\tstyles[p] = computed[p];\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\t_setDefaults(styles, _parseTransform(target, 1));\n\t\t\t\t\treturn styles;\n\t\t\t\t},\n\t\t\t\tstartClassList = target.getAttribute(\"class\"),\n\t\t\t\tstyle = target.style,\n\t\t\t\tcssText = style.cssText,\n\t\t\t\tcache = target._gsap,\n\t\t\t\tclassPT = cache.classPT,\n\t\t\t\tinlineToRemoveAtEnd = {},\n\t\t\t\tdata = {t:target, plugin:plugin, rmv:inlineToRemoveAtEnd, b:startClassList, e:(endValue.charAt(1) !== \"=\") ? endValue : startClassList.replace(new RegExp(\"(?:\\\\s|^)\" + endValue.substr(2) + \"(?![\\\\w-])\"), \"\") + ((endValue.charAt(0) === \"+\") ? \" \" + endValue.substr(2) : \"\")},\n\t\t\t\tchangingVars = {},\n\t\t\t\tstartVars = _getAllStyles(target),\n\t\t\t\ttransformRelated = /(transform|perspective)/i,\n\t\t\t\tendVars, p;\n\t\t\tif (classPT) {\n\t\t\t\tclassPT.r(1, classPT.d);\n\t\t\t\t_removeLinkedListItem(classPT.d.plugin, classPT, \"_pt\");\n\t\t\t}\n\t\t\ttarget.setAttribute(\"class\", data.e);\n\t\t\tendVars = _getAllStyles(target, true);\n\t\t\ttarget.setAttribute(\"class\", startClassList);\n\t\t\tfor (p in endVars) {\n\t\t\t\tif (endVars[p] !== startVars[p] && !transformRelated.test(p)) {\n\t\t\t\t\tchangingVars[p] = endVars[p];\n\t\t\t\t\tif (!style[p] && style[p] !== \"0\") {\n\t\t\t\t\t\tinlineToRemoveAtEnd[p] = 1;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\tcache.classPT = plugin._pt = new PropTween(plugin._pt, target, \"className\", 0, 0, _renderClassName, data, 0, -11);\n\t\t\tif (style.cssText !== cssText) { //only apply if things change. Otherwise, in cases like a background-image that's pulled dynamically, it could cause a refresh. See https://gsap.com/forums/topic/20368-possible-gsap-bug-switching-classnames-in-chrome/.\n\t\t\t\tstyle.cssText = cssText; //we recorded cssText before we swapped classes and ran _getAllStyles() because in cases when a className tween is overwritten, we remove all the related tweening properties from that class change (otherwise class-specific stuff can't override properties we've directly set on the target's style object due to specificity).\n\t\t\t}\n\t\t\t_parseTransform(target, true); //to clear the caching of transforms\n\t\t\tdata.css = new gsap.plugins.css();\n\t\t\tdata.css.init(target, changingVars, tween);\n\t\t\tplugin._props.push(...data.css._props);\n\t\t\treturn 1;\n\t\t}\n\t\t*/\n\t},\n\n\n\n\n\n\t/*\n\t * --------------------------------------------------------------------------------------\n\t * TRANSFORMS\n\t * --------------------------------------------------------------------------------------\n\t */\n\t_identity2DMatrix = [1,0,0,1,0,0],\n\t_rotationalProperties = {},\n\t_isNullTransform = value => (value === \"matrix(1, 0, 0, 1, 0, 0)\" || value === \"none\" || !value),\n\t_getComputedTransformMatrixAsArray = target => {\n\t\tlet matrixString = _getComputedProperty(target, _transformProp);\n\t\treturn _isNullTransform(matrixString) ? _identity2DMatrix : matrixString.substr(7).match(_numExp).map(_round);\n\t},\n\t_getMatrix = (target, force2D) => {\n\t\tlet cache = target._gsap || _getCache(target),\n\t\t\tstyle = target.style,\n\t\t\tmatrix = _getComputedTransformMatrixAsArray(target),\n\t\t\tparent, nextSibling, temp, addedToDOM;\n\t\tif (cache.svg && target.getAttribute(\"transform\")) {\n\t\t\ttemp = target.transform.baseVal.consolidate().matrix; //ensures that even complex values like \"translate(50,60) rotate(135,0,0)\" are parsed because it mashes it into a matrix.\n\t\t\tmatrix = [temp.a, temp.b, temp.c, temp.d, temp.e, temp.f];\n\t\t\treturn (matrix.join(\",\") === \"1,0,0,1,0,0\") ? _identity2DMatrix : matrix;\n\t\t} else if (matrix === _identity2DMatrix && !target.offsetParent && target !== _docElement && !cache.svg) { //note: if offsetParent is null, that means the element isn't in the normal document flow, like if it has display:none or one of its ancestors has display:none). Firefox returns null for getComputedStyle() if the element is in an iframe that has display:none. https://bugzilla.mozilla.org/show_bug.cgi?id=548397\n\t\t\t//browsers don't report transforms accurately unless the element is in the DOM and has a display value that's not \"none\". Firefox and Microsoft browsers have a partial bug where they'll report transforms even if display:none BUT not any percentage-based values like translate(-50%, 8px) will be reported as if it's translate(0, 8px).\n\t\t\ttemp = style.display;\n\t\t\tstyle.display = \"block\";\n\t\t\tparent = target.parentNode;\n\t\t\tif (!parent || (!target.offsetParent && !target.getBoundingClientRect().width)) { // note: in 3.3.0 we switched target.offsetParent to _doc.body.contains(target) to avoid [sometimes unnecessary] MutationObserver calls but that wasn't adequate because there are edge cases where nested position: fixed elements need to get reparented to accurately sense transforms. See https://github.com/greensock/GSAP/issues/388 and https://github.com/greensock/GSAP/issues/375. Note: position: fixed elements report a null offsetParent but they could also be invisible because they're in an ancestor with display: none, so we check getBoundingClientRect(). We only want to alter the DOM if we absolutely have to because it can cause iframe content to reload, like a Vimeo video.\n\t\t\t\taddedToDOM = 1; //flag\n\t\t\t\tnextSibling = target.nextElementSibling;\n\t\t\t\t_docElement.appendChild(target); //we must add it to the DOM in order to get values properly\n\t\t\t}\n\t\t\tmatrix = _getComputedTransformMatrixAsArray(target);\n\t\t\ttemp ? (style.display = temp) : _removeProperty(target, \"display\");\n\t\t\tif (addedToDOM) {\n\t\t\t\tnextSibling ? parent.insertBefore(target, nextSibling) : parent ? parent.appendChild(target) : _docElement.removeChild(target);\n\t\t\t}\n\t\t}\n\t\treturn (force2D && matrix.length > 6) ? [matrix[0], matrix[1], matrix[4], matrix[5], matrix[12], matrix[13]] : matrix;\n\t},\n\t_applySVGOrigin = (target, origin, originIsAbsolute, smooth, matrixArray, pluginToAddPropTweensTo) => {\n\t\tlet cache = target._gsap,\n\t\t\tmatrix = matrixArray || _getMatrix(target, true),\n\t\t\txOriginOld = cache.xOrigin || 0,\n\t\t\tyOriginOld = cache.yOrigin || 0,\n\t\t\txOffsetOld = cache.xOffset || 0,\n\t\t\tyOffsetOld = cache.yOffset || 0,\n\t\t\t[a, b, c, d, tx, ty] = matrix,\n\t\t\toriginSplit = origin.split(\" \"),\n\t\t\txOrigin = parseFloat(originSplit[0]) || 0,\n\t\t\tyOrigin = parseFloat(originSplit[1]) || 0,\n\t\t\tbounds, determinant, x, y;\n\t\tif (!originIsAbsolute) {\n\t\t\tbounds = _getBBox(target);\n\t\t\txOrigin = bounds.x + (~originSplit[0].indexOf(\"%\") ? xOrigin / 100 * bounds.width : xOrigin);\n\t\t\tyOrigin = bounds.y + (~((originSplit[1] || originSplit[0]).indexOf(\"%\")) ? yOrigin / 100 * bounds.height : yOrigin);\n\t\t\t// if (!(\"xOrigin\" in cache) && (xOrigin || yOrigin)) { // added in 3.12.3, reverted in 3.12.4; requires more exploration\n\t\t\t// \txOrigin -= bounds.x;\n\t\t\t// \tyOrigin -= bounds.y;\n\t\t\t// }\n\t\t} else if (matrix !== _identity2DMatrix && (determinant = (a * d - b * c))) { //if it's zero (like if scaleX and scaleY are zero), skip it to avoid errors with dividing by zero.\n\t\t\tx = xOrigin * (d / determinant) + yOrigin * (-c / determinant) + ((c * ty - d * tx) / determinant);\n\t\t\ty = xOrigin * (-b / determinant) + yOrigin * (a / determinant) - ((a * ty - b * tx) / determinant);\n\t\t\txOrigin = x;\n\t\t\tyOrigin = y;\n\t\t\t// theory: we only had to do this for smoothing and it assumes that the previous one was not originIsAbsolute.\n\t\t}\n\t\tif (smooth || (smooth !== false && cache.smooth)) {\n\t\t\ttx = xOrigin - xOriginOld;\n\t\t\tty = yOrigin - yOriginOld;\n\t\t\tcache.xOffset = xOffsetOld + (tx * a + ty * c) - tx;\n\t\t\tcache.yOffset = yOffsetOld + (tx * b + ty * d) - ty;\n\t\t} else {\n\t\t\tcache.xOffset = cache.yOffset = 0;\n\t\t}\n\t\tcache.xOrigin = xOrigin;\n\t\tcache.yOrigin = yOrigin;\n\t\tcache.smooth = !!smooth;\n\t\tcache.origin = origin;\n\t\tcache.originIsAbsolute = !!originIsAbsolute;\n\t\ttarget.style[_transformOriginProp] = \"0px 0px\"; //otherwise, if someone sets an origin via CSS, it will likely interfere with the SVG transform attribute ones (because remember, we're baking the origin into the matrix() value).\n\t\tif (pluginToAddPropTweensTo) {\n\t\t\t_addNonTweeningPT(pluginToAddPropTweensTo, cache, \"xOrigin\", xOriginOld, xOrigin);\n\t\t\t_addNonTweeningPT(pluginToAddPropTweensTo, cache, \"yOrigin\", yOriginOld, yOrigin);\n\t\t\t_addNonTweeningPT(pluginToAddPropTweensTo, cache, \"xOffset\", xOffsetOld, cache.xOffset);\n\t\t\t_addNonTweeningPT(pluginToAddPropTweensTo, cache, \"yOffset\", yOffsetOld, cache.yOffset);\n\t\t}\n\t\ttarget.setAttribute(\"data-svg-origin\", xOrigin + \" \" + yOrigin);\n\t},\n\t_parseTransform = (target, uncache) => {\n\t\tlet cache = target._gsap || new GSCache(target);\n\t\tif (\"x\" in cache && !uncache && !cache.uncache) {\n\t\t\treturn cache;\n\t\t}\n\t\tlet style = target.style,\n\t\t\tinvertedScaleX = cache.scaleX < 0,\n\t\t\tpx = \"px\",\n\t\t\tdeg = \"deg\",\n\t\t\tcs = getComputedStyle(target),\n\t\t\torigin = _getComputedProperty(target, _transformOriginProp) || \"0\",\n\t\t\tx, y, z, scaleX, scaleY, rotation, rotationX, rotationY, skewX, skewY, perspective, xOrigin, yOrigin,\n\t\t\tmatrix, angle, cos, sin, a, b, c, d, a12, a22, t1, t2, t3, a13, a23, a33, a42, a43, a32;\n\t\tx = y = z = rotation = rotationX = rotationY = skewX = skewY = perspective = 0;\n\t\tscaleX = scaleY = 1;\n\t\tcache.svg = !!(target.getCTM && _isSVG(target));\n\n\t\tif (cs.translate) { // accommodate independent transforms by combining them into normal ones.\n\t\t\tif (cs.translate !== \"none\" || cs.scale !== \"none\" || cs.rotate !== \"none\") {\n\t\t\t\tstyle[_transformProp] = (cs.translate !== \"none\" ? \"translate3d(\" + (cs.translate + \" 0 0\").split(\" \").slice(0, 3).join(\", \") + \") \" : \"\") + (cs.rotate !== \"none\" ? \"rotate(\" + cs.rotate + \") \" : \"\") + (cs.scale !== \"none\" ? \"scale(\" + cs.scale.split(\" \").join(\",\") + \") \" : \"\") + (cs[_transformProp] !== \"none\" ? cs[_transformProp] : \"\");\n\t\t\t}\n\t\t\tstyle.scale = style.rotate = style.translate = \"none\";\n\t\t}\n\n\t\tmatrix = _getMatrix(target, cache.svg);\n\t\tif (cache.svg) {\n\t\t\tif (cache.uncache) { // if cache.uncache is true (and maybe if origin is 0,0), we need to set element.style.transformOrigin = (cache.xOrigin - bbox.x) + \"px \" + (cache.yOrigin - bbox.y) + \"px\". Previously we let the data-svg-origin stay instead, but when introducing revert(), it complicated things.\n\t\t\t\tt2 = target.getBBox();\n\t\t\t\torigin = (cache.xOrigin - t2.x) + \"px \" + (cache.yOrigin - t2.y) + \"px\";\n\t\t\t\tt1 = \"\";\n\t\t\t} else {\n\t\t\t\tt1 = !uncache && target.getAttribute(\"data-svg-origin\"); // Remember, to work around browser inconsistencies we always force SVG elements' transformOrigin to 0,0 and offset the translation accordingly.\n\t\t\t}\n\t\t\t_applySVGOrigin(target, t1 || origin, !!t1 || cache.originIsAbsolute, cache.smooth !== false, matrix);\n\t\t}\n\t\txOrigin = cache.xOrigin || 0;\n\t\tyOrigin = cache.yOrigin || 0;\n\t\tif (matrix !== _identity2DMatrix) {\n\t\t\ta = matrix[0]; //a11\n\t\t\tb = matrix[1]; //a21\n\t\t\tc = matrix[2]; //a31\n\t\t\td = matrix[3]; //a41\n\t\t\tx = a12 = matrix[4];\n\t\t\ty = a22 = matrix[5];\n\n\t\t\t//2D matrix\n\t\t\tif (matrix.length === 6) {\n\t\t\t\tscaleX = Math.sqrt(a * a + b * b);\n\t\t\t\tscaleY = Math.sqrt(d * d + c * c);\n\t\t\t\trotation = (a || b) ? _atan2(b, a) * _RAD2DEG : 0; //note: if scaleX is 0, we cannot accurately measure rotation. Same for skewX with a scaleY of 0. Therefore, we default to the previously recorded value (or zero if that doesn't exist).\n\t\t\t\tskewX = (c || d) ? _atan2(c, d) * _RAD2DEG + rotation : 0;\n\t\t\t\tskewX && (scaleY *= Math.abs(Math.cos(skewX * _DEG2RAD)));\n\t\t\t\tif (cache.svg) {\n\t\t\t\t\tx -= xOrigin - (xOrigin * a + yOrigin * c);\n\t\t\t\t\ty -= yOrigin - (xOrigin * b + yOrigin * d);\n\t\t\t\t}\n\n\t\t\t//3D matrix\n\t\t\t} else {\n\t\t\t\ta32 = matrix[6];\n\t\t\t\ta42 = matrix[7];\n\t\t\t\ta13 = matrix[8];\n\t\t\t\ta23 = matrix[9];\n\t\t\t\ta33 = matrix[10];\n\t\t\t\ta43 = matrix[11];\n\t\t\t\tx = matrix[12];\n\t\t\t\ty = matrix[13];\n\t\t\t\tz = matrix[14];\n\n\t\t\t\tangle = _atan2(a32, a33);\n\t\t\t\trotationX = angle * _RAD2DEG;\n\t\t\t\t//rotationX\n\t\t\t\tif (angle) {\n\t\t\t\t\tcos = Math.cos(-angle);\n\t\t\t\t\tsin = Math.sin(-angle);\n\t\t\t\t\tt1 = a12*cos+a13*sin;\n\t\t\t\t\tt2 = a22*cos+a23*sin;\n\t\t\t\t\tt3 = a32*cos+a33*sin;\n\t\t\t\t\ta13 = a12*-sin+a13*cos;\n\t\t\t\t\ta23 = a22*-sin+a23*cos;\n\t\t\t\t\ta33 = a32*-sin+a33*cos;\n\t\t\t\t\ta43 = a42*-sin+a43*cos;\n\t\t\t\t\ta12 = t1;\n\t\t\t\t\ta22 = t2;\n\t\t\t\t\ta32 = t3;\n\t\t\t\t}\n\t\t\t\t//rotationY\n\t\t\t\tangle = _atan2(-c, a33);\n\t\t\t\trotationY = angle * _RAD2DEG;\n\t\t\t\tif (angle) {\n\t\t\t\t\tcos = Math.cos(-angle);\n\t\t\t\t\tsin = Math.sin(-angle);\n\t\t\t\t\tt1 = a*cos-a13*sin;\n\t\t\t\t\tt2 = b*cos-a23*sin;\n\t\t\t\t\tt3 = c*cos-a33*sin;\n\t\t\t\t\ta43 = d*sin+a43*cos;\n\t\t\t\t\ta = t1;\n\t\t\t\t\tb = t2;\n\t\t\t\t\tc = t3;\n\t\t\t\t}\n\t\t\t\t//rotationZ\n\t\t\t\tangle = _atan2(b, a);\n\t\t\t\trotation = angle * _RAD2DEG;\n\t\t\t\tif (angle) {\n\t\t\t\t\tcos = Math.cos(angle);\n\t\t\t\t\tsin = Math.sin(angle);\n\t\t\t\t\tt1 = a*cos+b*sin;\n\t\t\t\t\tt2 = a12*cos+a22*sin;\n\t\t\t\t\tb = b*cos-a*sin;\n\t\t\t\t\ta22 = a22*cos-a12*sin;\n\t\t\t\t\ta = t1;\n\t\t\t\t\ta12 = t2;\n\t\t\t\t}\n\n\t\t\t\tif (rotationX && Math.abs(rotationX) + Math.abs(rotation) > 359.9) { //when rotationY is set, it will often be parsed as 180 degrees different than it should be, and rotationX and rotation both being 180 (it looks the same), so we adjust for that here.\n\t\t\t\t\trotationX = rotation = 0;\n\t\t\t\t\trotationY = 180 - rotationY;\n\t\t\t\t}\n\t\t\t\tscaleX = _round(Math.sqrt(a * a + b * b + c * c));\n\t\t\t\tscaleY = _round(Math.sqrt(a22 * a22 + a32 * a32));\n\t\t\t\tangle = _atan2(a12, a22);\n\t\t\t\tskewX = (Math.abs(angle) > 0.0002) ? angle * _RAD2DEG : 0;\n\t\t\t\tperspective = a43 ? 1 / ((a43 < 0) ? -a43 : a43) : 0;\n\t\t\t}\n\n\t\t\tif (cache.svg) { //sense if there are CSS transforms applied on an SVG element in which case we must overwrite them when rendering. The transform attribute is more reliable cross-browser, but we can't just remove the CSS ones because they may be applied in a CSS rule somewhere (not just inline).\n\t\t\t\tt1 = target.getAttribute(\"transform\");\n\t\t\t\tcache.forceCSS = target.setAttribute(\"transform\", \"\") || (!_isNullTransform(_getComputedProperty(target, _transformProp)));\n\t\t\t\tt1 && target.setAttribute(\"transform\", t1);\n\t\t\t}\n\t\t}\n\n\t\tif (Math.abs(skewX) > 90 && Math.abs(skewX) < 270) {\n\t\t\tif (invertedScaleX) {\n\t\t\t\tscaleX *= -1;\n\t\t\t\tskewX += (rotation <= 0) ? 180 : -180;\n\t\t\t\trotation += (rotation <= 0) ? 180 : -180;\n\t\t\t} else {\n\t\t\t\tscaleY *= -1;\n\t\t\t\tskewX += (skewX <= 0) ? 180 : -180;\n\t\t\t}\n\t\t}\n\t\tuncache = uncache || cache.uncache;\n\t\tcache.x = x - ((cache.xPercent = x && ((!uncache && cache.xPercent) || (Math.round(target.offsetWidth / 2) === Math.round(-x) ? -50 : 0))) ? target.offsetWidth * cache.xPercent / 100 : 0) + px;\n\t\tcache.y = y - ((cache.yPercent = y && ((!uncache && cache.yPercent) || (Math.round(target.offsetHeight / 2) === Math.round(-y) ? -50 : 0))) ? target.offsetHeight * cache.yPercent / 100 : 0) + px;\n\t\tcache.z = z + px;\n\t\tcache.scaleX = _round(scaleX);\n\t\tcache.scaleY = _round(scaleY);\n\t\tcache.rotation = _round(rotation) + deg;\n\t\tcache.rotationX = _round(rotationX) + deg;\n\t\tcache.rotationY = _round(rotationY) + deg;\n\t\tcache.skewX = skewX + deg;\n\t\tcache.skewY = skewY + deg;\n\t\tcache.transformPerspective = perspective + px;\n\t\tif ((cache.zOrigin = parseFloat(origin.split(\" \")[2]) || (!uncache && cache.zOrigin) || 0)) {\n\t\t\tstyle[_transformOriginProp] = _firstTwoOnly(origin);\n\t\t}\n\t\tcache.xOffset = cache.yOffset = 0;\n\t\tcache.force3D = _config.force3D;\n\t\tcache.renderTransform = cache.svg ? _renderSVGTransforms : _supports3D ? _renderCSSTransforms : _renderNon3DTransforms;\n\t\tcache.uncache = 0;\n\t\treturn cache;\n\t},\n\t_firstTwoOnly = value => (value = value.split(\" \"))[0] + \" \" + value[1], //for handling transformOrigin values, stripping out the 3rd dimension\n\t_addPxTranslate = (target, start, value) => {\n\t\tlet unit = getUnit(start);\n\t\treturn _round(parseFloat(start) + parseFloat(_convertToUnit(target, \"x\", value + \"px\", unit))) + unit;\n\t},\n\t_renderNon3DTransforms = (ratio, cache) => {\n\t\tcache.z = \"0px\";\n\t\tcache.rotationY = cache.rotationX = \"0deg\";\n\t\tcache.force3D = 0;\n\t\t_renderCSSTransforms(ratio, cache);\n\t},\n\t_zeroDeg = \"0deg\",\n\t_zeroPx = \"0px\",\n\t_endParenthesis = \") \",\n\t_renderCSSTransforms = function(ratio, cache) {\n\t\tlet {xPercent, yPercent, x, y, z, rotation, rotationY, rotationX, skewX, skewY, scaleX, scaleY, transformPerspective, force3D, target, zOrigin} = cache || this,\n\t\t\ttransforms = \"\",\n\t\t\tuse3D = (force3D === \"auto\" && ratio && ratio !== 1) || force3D === true;\n\n\t\t// Safari has a bug that causes it not to render 3D transform-origin values properly, so we force the z origin to 0, record it in the cache, and then do the math here to offset the translate values accordingly (basically do the 3D transform-origin part manually)\n\t\tif (zOrigin && (rotationX !== _zeroDeg || rotationY !== _zeroDeg)) {\n\t\t\tlet angle = parseFloat(rotationY) * _DEG2RAD,\n\t\t\t\ta13 = Math.sin(angle),\n\t\t\t\ta33 = Math.cos(angle),\n\t\t\t\tcos;\n\t\t\tangle = parseFloat(rotationX) * _DEG2RAD;\n\t\t\tcos = Math.cos(angle);\n\t\t\tx = _addPxTranslate(target, x, a13 * cos * -zOrigin);\n\t\t\ty = _addPxTranslate(target, y, -Math.sin(angle) * -zOrigin);\n\t\t\tz = _addPxTranslate(target, z, a33 * cos * -zOrigin + zOrigin);\n\t\t}\n\n\t\tif (transformPerspective !== _zeroPx) {\n\t\t\ttransforms += \"perspective(\" + transformPerspective + _endParenthesis;\n\t\t}\n\t\tif (xPercent || yPercent) {\n\t\t\ttransforms += \"translate(\" + xPercent + \"%, \" + yPercent + \"%) \";\n\t\t}\n\t\tif (use3D || x !== _zeroPx || y !== _zeroPx || z !== _zeroPx) {\n\t\t\ttransforms += (z !== _zeroPx || use3D) ? \"translate3d(\" + x + \", \" + y + \", \" + z + \") \" : \"translate(\" + x + \", \" + y + _endParenthesis;\n\t\t}\n\t\tif (rotation !== _zeroDeg) {\n\t\t\ttransforms += \"rotate(\" + rotation + _endParenthesis;\n\t\t}\n\t\tif (rotationY !== _zeroDeg) {\n\t\t\ttransforms += \"rotateY(\" + rotationY + _endParenthesis;\n\t\t}\n\t\tif (rotationX !== _zeroDeg) {\n\t\t\ttransforms += \"rotateX(\" + rotationX + _endParenthesis;\n\t\t}\n\t\tif (skewX !== _zeroDeg || skewY !== _zeroDeg) {\n\t\t\ttransforms += \"skew(\" + skewX + \", \" + skewY + _endParenthesis;\n\t\t}\n\t\tif (scaleX !== 1 || scaleY !== 1) {\n\t\t\ttransforms += \"scale(\" + scaleX + \", \" + scaleY + _endParenthesis;\n\t\t}\n\t\ttarget.style[_transformProp] = transforms || \"translate(0, 0)\";\n\t},\n\t_renderSVGTransforms = function(ratio, cache) {\n\t\tlet {xPercent, yPercent, x, y, rotation, skewX, skewY, scaleX, scaleY, target, xOrigin, yOrigin, xOffset, yOffset, forceCSS} = cache || this,\n\t\t\ttx = parseFloat(x),\n\t\t\tty = parseFloat(y),\n\t\t\ta11, a21, a12, a22, temp;\n\t\trotation = parseFloat(rotation);\n\t\tskewX = parseFloat(skewX);\n\t\tskewY = parseFloat(skewY);\n\t\tif (skewY) { //for performance reasons, we combine all skewing into the skewX and rotation values. Remember, a skewY of 10 degrees looks the same as a rotation of 10 degrees plus a skewX of 10 degrees.\n\t\t\tskewY = parseFloat(skewY);\n\t\t\tskewX += skewY;\n\t\t\trotation += skewY;\n\t\t}\n\t\tif (rotation || skewX) {\n\t\t\trotation *= _DEG2RAD;\n\t\t\tskewX *= _DEG2RAD;\n\t\t\ta11 = Math.cos(rotation) * scaleX;\n\t\t\ta21 = Math.sin(rotation) * scaleX;\n\t\t\ta12 = Math.sin(rotation - skewX) * -scaleY;\n\t\t\ta22 = Math.cos(rotation - skewX) * scaleY;\n\t\t\tif (skewX) {\n\t\t\t\tskewY *= _DEG2RAD;\n\t\t\t\ttemp = Math.tan(skewX - skewY);\n\t\t\t\ttemp = Math.sqrt(1 + temp * temp);\n\t\t\t\ta12 *= temp;\n\t\t\t\ta22 *= temp;\n\t\t\t\tif (skewY) {\n\t\t\t\t\ttemp = Math.tan(skewY);\n\t\t\t\t\ttemp = Math.sqrt(1 + temp * temp);\n\t\t\t\t\ta11 *= temp;\n\t\t\t\t\ta21 *= temp;\n\t\t\t\t}\n\t\t\t}\n\t\t\ta11 = _round(a11);\n\t\t\ta21 = _round(a21);\n\t\t\ta12 = _round(a12);\n\t\t\ta22 = _round(a22);\n\t\t} else {\n\t\t\ta11 = scaleX;\n\t\t\ta22 = scaleY;\n\t\t\ta21 = a12 = 0;\n\t\t}\n\t\tif ((tx && !~(x + \"\").indexOf(\"px\")) || (ty && !~(y + \"\").indexOf(\"px\"))) {\n\t\t\ttx = _convertToUnit(target, \"x\", x, \"px\");\n\t\t\tty = _convertToUnit(target, \"y\", y, \"px\");\n\t\t}\n\t\tif (xOrigin || yOrigin || xOffset || yOffset) {\n\t\t\ttx = _round(tx + xOrigin - (xOrigin * a11 + yOrigin * a12) + xOffset);\n\t\t\tty = _round(ty + yOrigin - (xOrigin * a21 + yOrigin * a22) + yOffset);\n\t\t}\n\t\tif (xPercent || yPercent) {\n\t\t\t//The SVG spec doesn't support percentage-based translation in the \"transform\" attribute, so we merge it into the translation to simulate it.\n\t\t\ttemp = target.getBBox();\n\t\t\ttx = _round(tx + xPercent / 100 * temp.width);\n\t\t\tty = _round(ty + yPercent / 100 * temp.height);\n\t\t}\n\t\ttemp = \"matrix(\" + a11 + \",\" + a21 + \",\" + a12 + \",\" + a22 + \",\" + tx + \",\" + ty + \")\";\n\t\ttarget.setAttribute(\"transform\", temp);\n\t\tforceCSS && (target.style[_transformProp] = temp); //some browsers prioritize CSS transforms over the transform attribute. When we sense that the user has CSS transforms applied, we must overwrite them this way (otherwise some browser simply won't render the transform attribute changes!)\n\t},\n\t_addRotationalPropTween = function(plugin, target, property, startNum, endValue) {\n\t\tlet cap = 360,\n\t\t\tisString = _isString(endValue),\n\t\t\tendNum = parseFloat(endValue) * ((isString && ~endValue.indexOf(\"rad\")) ? _RAD2DEG : 1),\n\t\t\tchange = endNum - startNum,\n\t\t\tfinalValue = (startNum + change) + \"deg\",\n\t\t\tdirection, pt;\n\t\tif (isString) {\n\t\t\tdirection = endValue.split(\"_\")[1];\n\t\t\tif (direction === \"short\") {\n\t\t\t\tchange %= cap;\n\t\t\t\tif (change !== change % (cap / 2)) {\n\t\t\t\t\tchange += (change < 0) ? cap : -cap;\n\t\t\t\t}\n\t\t\t}\n\t\t\tif (direction === \"cw\" && change < 0) {\n\t\t\t\tchange = ((change + cap * _bigNum) % cap) - ~~(change / cap) * cap;\n\t\t\t} else if (direction === \"ccw\" && change > 0) {\n\t\t\t\tchange = ((change - cap * _bigNum) % cap) - ~~(change / cap) * cap;\n\t\t\t}\n\t\t}\n\t\tplugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd);\n\t\tpt.e = finalValue;\n\t\tpt.u = \"deg\";\n\t\tplugin._props.push(property);\n\t\treturn pt;\n\t},\n\t_assign = (target, source) => { // Internet Explorer doesn't have Object.assign(), so we recreate it here.\n\t\tfor (let p in source) {\n\t\t\ttarget[p] = source[p];\n\t\t}\n\t\treturn target;\n\t},\n\t_addRawTransformPTs = (plugin, transforms, target) => { //for handling cases where someone passes in a whole transform string, like transform: \"scale(2, 3) rotate(20deg) translateY(30em)\"\n\t\tlet startCache = _assign({}, target._gsap),\n\t\t\texclude = \"perspective,force3D,transformOrigin,svgOrigin\",\n\t\t\tstyle = target.style,\n\t\t\tendCache, p, startValue, endValue, startNum, endNum, startUnit, endUnit;\n\t\tif (startCache.svg) {\n\t\t\tstartValue = target.getAttribute(\"transform\");\n\t\t\ttarget.setAttribute(\"transform\", \"\");\n\t\t\tstyle[_transformProp] = transforms;\n\t\t\tendCache = _parseTransform(target, 1);\n\t\t\t_removeProperty(target, _transformProp);\n\t\t\ttarget.setAttribute(\"transform\", startValue);\n\t\t} else {\n\t\t\tstartValue = getComputedStyle(target)[_transformProp];\n\t\t\tstyle[_transformProp] = transforms;\n\t\t\tendCache = _parseTransform(target, 1);\n\t\t\tstyle[_transformProp] = startValue;\n\t\t}\n\t\tfor (p in _transformProps) {\n\t\t\tstartValue = startCache[p];\n\t\t\tendValue = endCache[p];\n\t\t\tif (startValue !== endValue && exclude.indexOf(p) < 0) { //tweening to no perspective gives very unintuitive results - just keep the same perspective in that case.\n\t\t\t\tstartUnit = getUnit(startValue);\n\t\t\t\tendUnit = getUnit(endValue);\n\t\t\t\tstartNum = (startUnit !== endUnit) ? _convertToUnit(target, p, startValue, endUnit) : parseFloat(startValue);\n\t\t\t\tendNum = parseFloat(endValue);\n\t\t\t\tplugin._pt = new PropTween(plugin._pt, endCache, p, startNum, endNum - startNum, _renderCSSProp);\n\t\t\t\tplugin._pt.u = endUnit || 0;\n\t\t\t\tplugin._props.push(p);\n\t\t\t}\n\t\t}\n\t\t_assign(endCache, startCache);\n\t};\n\n// handle splitting apart padding, margin, borderWidth, and borderRadius into their 4 components. Firefox, for example, won't report borderRadius correctly - it will only do borderTopLeftRadius and the other corners. We also want to handle paddingTop, marginLeft, borderRightWidth, etc.\n_forEachName(\"padding,margin,Width,Radius\", (name, index) => {\n\tlet t = \"Top\",\n\t\tr = \"Right\",\n\t\tb = \"Bottom\",\n\t\tl = \"Left\",\n\t\tprops = (index < 3 ? [t,r,b,l] : [t+l, t+r, b+r, b+l]).map(side => index < 2 ? name + side : \"border\" + side + name);\n\t_specialProps[(index > 1 ? \"border\" + name : name)] = function(plugin, target, property, endValue, tween) {\n\t\tlet a, vars;\n\t\tif (arguments.length < 4) { // getter, passed target, property, and unit (from _get())\n\t\t\ta = props.map(prop => _get(plugin, prop, property));\n\t\t\tvars = a.join(\" \");\n\t\t\treturn vars.split(a[0]).length === 5 ? a[0] : vars;\n\t\t}\n\t\ta = (endValue + \"\").split(\" \");\n\t\tvars = {};\n\t\tprops.forEach((prop, i) => vars[prop] = a[i] = a[i] || a[(((i - 1) / 2) | 0)]);\n\t\tplugin.init(target, vars, tween);\n\t}\n});\n\n\nexport const CSSPlugin = {\n\tname: \"css\",\n\tregister: _initCore,\n\ttargetTest(target) {\n\t\treturn target.style && target.nodeType;\n\t},\n\tinit(target, vars, tween, index, targets) {\n\t\tlet props = this._props,\n\t\t\tstyle = target.style,\n\t\t\tstartAt = tween.vars.startAt,\n\t\t\tstartValue, endValue, endNum, startNum, type, specialProp, p, startUnit, endUnit, relative, isTransformRelated, transformPropTween, cache, smooth, hasPriority, inlineProps;\n\t\t_pluginInitted || _initCore();\n\t\t// we may call init() multiple times on the same plugin instance, like when adding special properties, so make sure we don't overwrite the revert data or inlineProps\n\t\tthis.styles = this.styles || _getStyleSaver(target);\n\t\tinlineProps = this.styles.props;\n\t\tthis.tween = tween;\n\t\tfor (p in vars) {\n\t\t\tif (p === \"autoRound\") {\n\t\t\t\tcontinue;\n\t\t\t}\n\t\t\tendValue = vars[p];\n\t\t\tif (_plugins[p] && _checkPlugin(p, vars, tween, index, target, targets)) { // plugins\n\t\t\t\tcontinue;\n\t\t\t}\n\t\t\ttype = typeof(endValue);\n\t\t\tspecialProp = _specialProps[p];\n\t\t\tif (type === \"function\") {\n\t\t\t\tendValue = endValue.call(tween, index, target, targets);\n\t\t\t\ttype = typeof(endValue);\n\t\t\t}\n\t\t\tif (type === \"string\" && ~endValue.indexOf(\"random(\")) {\n\t\t\t\tendValue = _replaceRandom(endValue);\n\t\t\t}\n\t\t\tif (specialProp) {\n\t\t\t\tspecialProp(this, target, p, endValue, tween) && (hasPriority = 1);\n\t\t\t} else if (p.substr(0,2) === \"--\") { //CSS variable\n\t\t\t\tstartValue = (getComputedStyle(target).getPropertyValue(p) + \"\").trim();\n\t\t\t\tendValue += \"\";\n\t\t\t\t_colorExp.lastIndex = 0;\n\t\t\t\tif (!_colorExp.test(startValue)) { // colors don't have units\n\t\t\t\t\tstartUnit = getUnit(startValue);\n\t\t\t\t\tendUnit = getUnit(endValue);\n\t\t\t\t}\n\t\t\t\tendUnit ? startUnit !== endUnit && (startValue = _convertToUnit(target, p, startValue, endUnit) + endUnit) : startUnit && (endValue += startUnit);\n\t\t\t\tthis.add(style, \"setProperty\", startValue, endValue, index, targets, 0, 0, p);\n\t\t\t\tprops.push(p);\n\t\t\t\tinlineProps.push(p, 0, style[p]);\n\t\t\t} else if (type !== \"undefined\") {\n\t\t\t\tif (startAt && p in startAt) { // in case someone hard-codes a complex value as the start, like top: \"calc(2vh / 2)\". Without this, it'd use the computed value (always in px)\n\t\t\t\t\tstartValue = typeof(startAt[p]) === \"function\" ? startAt[p].call(tween, index, target, targets) : startAt[p];\n\t\t\t\t\t_isString(startValue) && ~startValue.indexOf(\"random(\") && (startValue = _replaceRandom(startValue));\n\t\t\t\t\tgetUnit(startValue + \"\") || startValue === \"auto\" || (startValue += _config.units[p] || getUnit(_get(target, p)) || \"\"); // for cases when someone passes in a unitless value like {x: 100}; if we try setting translate(100, 0px) it won't work.\n\t\t\t\t\t(startValue + \"\").charAt(1) === \"=\" && (startValue = _get(target, p)); // can't work with relative values\n\t\t\t\t} else {\n\t\t\t\t\tstartValue = _get(target, p);\n\t\t\t\t}\n\t\t\t\tstartNum = parseFloat(startValue);\n\t\t\t\trelative = (type === \"string\" && endValue.charAt(1) === \"=\") && endValue.substr(0, 2);\n\t\t\t\trelative && (endValue = endValue.substr(2));\n\t\t\t\tendNum = parseFloat(endValue);\n\t\t\t\tif (p in _propertyAliases) {\n\t\t\t\t\tif (p === \"autoAlpha\") { //special case where we control the visibility along with opacity. We still allow the opacity value to pass through and get tweened.\n\t\t\t\t\t\tif (startNum === 1 && _get(target, \"visibility\") === \"hidden\" && endNum) { //if visibility is initially set to \"hidden\", we should interpret that as intent to make opacity 0 (a convenience)\n\t\t\t\t\t\t\tstartNum = 0;\n\t\t\t\t\t\t}\n\t\t\t\t\t\tinlineProps.push(\"visibility\", 0, style.visibility);\n\t\t\t\t\t\t_addNonTweeningPT(this, style, \"visibility\", startNum ? \"inherit\" : \"hidden\", endNum ? \"inherit\" : \"hidden\", !endNum);\n\t\t\t\t\t}\n\t\t\t\t\tif (p !== \"scale\" && p !== \"transform\") {\n\t\t\t\t\t\tp = _propertyAliases[p];\n\t\t\t\t\t\t~p.indexOf(\",\") && (p = p.split(\",\")[0]);\n\t\t\t\t\t}\n\t\t\t\t}\n\n\t\t\t\tisTransformRelated = (p in _transformProps);\n\n\t\t\t\t//--- TRANSFORM-RELATED ---\n\t\t\t\tif (isTransformRelated) {\n\t\t\t\t\tthis.styles.save(p);\n\t\t\t\t\tif (!transformPropTween) {\n\t\t\t\t\t\tcache = target._gsap;\n\t\t\t\t\t\t(cache.renderTransform && !vars.parseTransform) || _parseTransform(target, vars.parseTransform); // if, for example, gsap.set(... {transform:\"translateX(50vw)\"}), the _get() call doesn't parse the transform, thus cache.renderTransform won't be set yet so force the parsing of the transform here.\n\t\t\t\t\t\tsmooth = (vars.smoothOrigin !== false && cache.smooth);\n\t\t\t\t\t\ttransformPropTween = this._pt = new PropTween(this._pt, style, _transformProp, 0, 1, cache.renderTransform, cache, 0, -1); //the first time through, create the rendering PropTween so that it runs LAST (in the linked list, we keep adding to the beginning)\n\t\t\t\t\t\ttransformPropTween.dep = 1; //flag it as dependent so that if things get killed/overwritten and this is the only PropTween left, we can safely kill the whole tween.\n\t\t\t\t\t}\n\t\t\t\t\tif (p === \"scale\") {\n\t\t\t\t\t\tthis._pt = new PropTween(this._pt, cache, \"scaleY\", cache.scaleY, ((relative ? _parseRelative(cache.scaleY, relative + endNum) : endNum) - cache.scaleY) || 0, _renderCSSProp);\n\t\t\t\t\t\tthis._pt.u = 0;\n\t\t\t\t\t\tprops.push(\"scaleY\", p);\n\t\t\t\t\t\tp += \"X\";\n\t\t\t\t\t} else if (p === \"transformOrigin\") {\n\t\t\t\t\t\tinlineProps.push(_transformOriginProp, 0, style[_transformOriginProp]);\n\t\t\t\t\t\tendValue = _convertKeywordsToPercentages(endValue); //in case something like \"left top\" or \"bottom right\" is passed in. Convert to percentages.\n\t\t\t\t\t\tif (cache.svg) {\n\t\t\t\t\t\t\t_applySVGOrigin(target, endValue, 0, smooth, 0, this);\n\t\t\t\t\t\t} else {\n\t\t\t\t\t\t\tendUnit = parseFloat(endValue.split(\" \")[2]) || 0; //handle the zOrigin separately!\n\t\t\t\t\t\t\tendUnit !== cache.zOrigin && _addNonTweeningPT(this, cache, \"zOrigin\", cache.zOrigin, endUnit);\n\t\t\t\t\t\t\t_addNonTweeningPT(this, style, p, _firstTwoOnly(startValue), _firstTwoOnly(endValue));\n\t\t\t\t\t\t}\n\t\t\t\t\t\tcontinue;\n\t\t\t\t\t} else if (p === \"svgOrigin\") {\n\t\t\t\t\t\t_applySVGOrigin(target, endValue, 1, smooth, 0, this);\n\t\t\t\t\t\tcontinue;\n\t\t\t\t\t} else if (p in _rotationalProperties) {\n\t\t\t\t\t\t_addRotationalPropTween(this, cache, p, startNum, relative ? _parseRelative(startNum, relative + endValue) : endValue);\n\t\t\t\t\t\tcontinue;\n\n\t\t\t\t\t} else if (p === \"smoothOrigin\") {\n\t\t\t\t\t\t_addNonTweeningPT(this, cache, \"smooth\", cache.smooth, endValue);\n\t\t\t\t\t\tcontinue;\n\t\t\t\t\t} else if (p === \"force3D\") {\n\t\t\t\t\t\tcache[p] = endValue;\n\t\t\t\t\t\tcontinue;\n\t\t\t\t\t} else if (p === \"transform\") {\n\t\t\t\t\t\t_addRawTransformPTs(this, endValue, target);\n\t\t\t\t\t\tcontinue;\n\t\t\t\t\t}\n\t\t\t\t} else if (!(p in style)) {\n\t\t\t\t\tp = _checkPropPrefix(p) || p;\n\t\t\t\t}\n\n\t\t\t\tif (isTransformRelated || ((endNum || endNum === 0) && (startNum || startNum === 0) && !_complexExp.test(endValue) && (p in style))) {\n\t\t\t\t\tstartUnit = (startValue + \"\").substr((startNum + \"\").length);\n\t\t\t\t\tendNum || (endNum = 0); // protect against NaN\n\t\t\t\t\tendUnit = getUnit(endValue) || ((p in _config.units) ? _config.units[p] : startUnit);\n\t\t\t\t\tstartUnit !== endUnit && (startNum = _convertToUnit(target, p, startValue, endUnit));\n\t\t\t\t\tthis._pt = new PropTween(this._pt, isTransformRelated ? cache : style, p, startNum, (relative ? _parseRelative(startNum, relative + endNum) : endNum) - startNum, (!isTransformRelated && (endUnit === \"px\" || p === \"zIndex\") && vars.autoRound !== false) ? _renderRoundedCSSProp : _renderCSSProp);\n\t\t\t\t\tthis._pt.u = endUnit || 0;\n\t\t\t\t\tif (startUnit !== endUnit && endUnit !== \"%\") { //when the tween goes all the way back to the beginning, we need to revert it to the OLD/ORIGINAL value (with those units). We record that as a \"b\" (beginning) property and point to a render method that handles that. (performance optimization)\n\t\t\t\t\t\tthis._pt.b = startValue;\n\t\t\t\t\t\tthis._pt.r = _renderCSSPropWithBeginning;\n\t\t\t\t\t}\n\t\t\t\t} else if (!(p in style)) {\n\t\t\t\t\tif (p in target) { //maybe it's not a style - it could be a property added directly to an element in which case we'll try to animate that.\n\t\t\t\t\t\tthis.add(target, p, startValue || target[p], relative ? relative + endValue : endValue, index, targets);\n\t\t\t\t\t} else if (p !== \"parseTransform\") {\n\t\t\t\t\t\t_missingPlugin(p, endValue);\n\t\t\t\t\t\tcontinue;\n\t\t\t\t\t}\n\t\t\t\t} else {\n\t\t\t\t\t_tweenComplexCSSString.call(this, target, p, startValue, relative ? relative + endValue : endValue);\n\t\t\t\t}\n\t\t\t\tisTransformRelated || (p in style ? inlineProps.push(p, 0, style[p]) : typeof(target[p]) === \"function\" ? inlineProps.push(p, 2, target[p]()) : inlineProps.push(p, 1, startValue || target[p]));\n\t\t\t\tprops.push(p);\n\t\t\t}\n\t\t}\n\t\thasPriority && _sortPropTweensByPriority(this);\n\n\t},\n\trender(ratio, data) {\n\t\tif (data.tween._time || !_reverting()) {\n\t\t\tlet pt = data._pt;\n\t\t\twhile (pt) {\n\t\t\t\tpt.r(ratio, pt.d);\n\t\t\t\tpt = pt._next;\n\t\t\t}\n\t\t} else {\n\t\t\tdata.styles.revert();\n\t\t}\n\t},\n\tget: _get,\n\taliases: _propertyAliases,\n\tgetSetter(target, property, plugin) { //returns a setter function that accepts target, property, value and applies it accordingly. Remember, properties like \"x\" aren't as simple as target.style.property = value because they've got to be applied to a proxy object and then merged into a transform string in a renderer.\n\t\tlet p = _propertyAliases[property];\n\t\t(p && p.indexOf(\",\") < 0) && (property = p);\n\t\treturn (property in _transformProps && property !== _transformOriginProp && (target._gsap.x || _get(target, \"x\"))) ? (plugin && _recentSetterPlugin === plugin ? (property === \"scale\" ? _setterScale : _setterTransform) : (_recentSetterPlugin = plugin || {}) && (property === \"scale\" ? _setterScaleWithRender : _setterTransformWithRender)) : target.style && !_isUndefined(target.style[property]) ? _setterCSSStyle : ~property.indexOf(\"-\") ? _setterCSSProp : _getSetter(target, property);\n\t},\n\tcore: { _removeProperty, _getMatrix }\n\n};\n\ngsap.utils.checkPrefix = _checkPropPrefix;\ngsap.core.getStyleSaver = _getStyleSaver;\n(function(positionAndScale, rotation, others, aliases) {\n\tlet all = _forEachName(positionAndScale + \",\" + rotation + \",\" + others, name => {_transformProps[name] = 1});\n\t_forEachName(rotation, name => {_config.units[name] = \"deg\"; _rotationalProperties[name] = 1});\n\t_propertyAliases[all[13]] = positionAndScale + \",\" + rotation;\n\t_forEachName(aliases, name => {\n\t\tlet split = name.split(\":\");\n\t\t_propertyAliases[split[1]] = all[split[0]];\n\t});\n})(\"x,y,z,scale,scaleX,scaleY,xPercent,yPercent\", \"rotation,rotationX,rotationY,skewX,skewY\", \"transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective\", \"0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY\");\n_forEachName(\"x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective\", name => {_config.units[name] = \"px\"});\n\ngsap.registerPlugin(CSSPlugin);\n\nexport { CSSPlugin as default, _getBBox, _createElement, _checkPropPrefix as checkPrefix };","import { gsap, Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ, TweenLite, TimelineLite, TimelineMax } from \"./gsap-core.js\";\nimport { CSSPlugin } from \"./CSSPlugin.js\";\n\nconst gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, // to protect from tree shaking\n\tTweenMaxWithCSS = gsapWithCSS.core.Tween;\n\nexport {\n\tgsapWithCSS as gsap,\n\tgsapWithCSS as default,\n\tCSSPlugin,\n\tTweenMaxWithCSS as TweenMax,\n\tTweenLite,\n\tTimelineMax,\n\tTimelineLite,\n\tPower0,\n\tPower1,\n\tPower2,\n\tPower3,\n\tPower4,\n\tLinear,\n\tQuad,\n\tCubic,\n\tQuart,\n\tQuint,\n\tStrong,\n\tElastic,\n\tBack,\n\tSteppedEase,\n\tBounce,\n\tSine,\n\tExpo,\n\tCirc\n};"],"names":["_isString","value","_isFunction","_isNumber","_isUndefined","_isObject","_isNotFalse","_windowExists","window","_isFuncOrString","_install","scope","_installScope","_merge","_globals","gsap","_missingPlugin","property","console","warn","_warn","message","suppress","_addGlobal","name","obj","_emptyFunc","_harness","targets","harnessPlugin","i","target","_gsap","harness","_harnessPlugins","length","targetTest","GSCache","splice","_getCache","toArray","_getProperty","v","getAttribute","_forEachName","names","func","split","forEach","_round","Math","round","_roundPrecise","_parseRelative","start","operator","charAt","end","parseFloat","substr","_arrayContainsAny","toSearch","toFind","l","indexOf","_lazyRender","tween","_lazyTweens","a","slice","_lazyLookup","_lazy","render","_lazySafeRender","animation","time","suppressEvents","force","_reverting","_initted","_startAt","_numericIfPossible","n","match","_delimitedValueExp","trim","_passThrough","p","_setDefaults","defaults","_mergeDeep","base","toMerge","_copyExcluding","excluding","copy","_inheritDefaults","vars","parent","_globalTimeline","keyframes","_setKeyframeDefaults","excludeDuration","_isArray","inherit","_dp","_addLinkedListItem","child","firstProp","lastProp","sortBy","t","prev","_prev","_next","_removeLinkedListItem","next","_removeFromParent","onlyIfParentHasAutoRemove","autoRemoveChildren","remove","_act","_uncache","_end","_dur","_start","_dirty","_rewindStartAt","totalTime","revert","_revertConfigNoKill","immediateRender","autoRevert","_elapsedCycleDuration","_repeat","_animationCycle","_tTime","duration","_rDelay","_parentToChildTotalTime","parentTime","_ts","totalDuration","_tDur","_setEnd","abs","_rts","_tinyNum","_alignPlayhead","smoothChildTiming","_time","_postAddChecks","timeline","add","rawTime","_clamp","_zTime","_addToTimeline","position","skipChecks","_parsePosition","_delay","timeScale","_sort","_isFromOrFromStart","_recent","_scrollTrigger","trigger","ScrollTrigger","create","_attemptInitTween","tTime","_initTween","_pt","lazy","_lastRenderedFrame","_ticker","frame","push","_setDuration","skipUncache","leavePlayhead","repeat","dur","totalProgress","_onUpdateTotalDuration","Timeline","_createTweenType","type","params","irVars","isLegacy","varsIndex","runBackwards","startAt","Tween","_conditionalReturn","getUnit","_unitExp","exec","_isArrayLike","nonEmpty","nodeType","_win","selector","el","current","nativeElement","querySelectorAll","_doc","createElement","shuffle","sort","random","distribute","each","ease","_parseEase","from","cache","isDecimal","ratios","isNaN","axis","ratioX","ratioY","center","edges","originX","originY","x","y","d","j","max","min","wrapAt","distances","grid","_bigNum","getBoundingClientRect","left","_sqrt","amount","b","u","_invertEase","_roundModifier","pow","raw","snap","snapTo","radius","is2D","isArray","values","increment","dx","dy","closest","roundingIncrement","returnFunction","floor","_wrapArray","wrapper","index","_replaceRandom","nums","s","_strictNumExp","_getLabelInDirection","fromTime","backward","distance","label","labels","_interrupt","scrollTrigger","kill","progress","_callback","_createPlugin","config","headless","isFunc","Plugin","init","_props","instanceDefaults","_renderPropTweens","_addPropTween","_killPropTweensOf","modifier","_addPluginModifier","rawVars","statics","get","getSetter","_getSetter","aliases","register","_wake","_plugins","prototype","prop","_reservedProps","toUpperCase","PropTween","_registerPluginQueue","_hue","h","m1","m2","_255","splitColor","toHSL","forceAlpha","r","g","wasHSL","_colorLookup","black","parseInt","_numExp","transparent","map","Number","_colorOrderData","c","_colorExp","_numWithUnitExp","_formatColors","orderMatchData","shell","result","colors","color","join","replace","shift","_colorStringFilter","combined","lastIndex","test","_hslExp","_configEaseFromString","_easeMap","apply","_parseObjectInString","val","parsedVal","key","lastIndexOf","_quotesExp","_valueInParentheses","open","close","nested","substring","_CE","_customEaseExp","_propagateYoyoEase","isYoyo","_first","yoyoEase","_yoyo","_ease","_yEase","_insertEase","easeIn","easeOut","easeInOut","lowercaseName","toLowerCase","_easeInOutFromOut","_configElastic","amplitude","period","p1","_sin","p3","p2","_2PI","asin","_configBack","overshoot","_suppressOverwrites","_context","_coreInitted","_coreReady","_quickTween","_tickerActive","_id","_req","_raf","_self","_delta","_i","_getTime","_lagThreshold","_adjustedLag","_startTime","_lastUpdate","_gap","_nextTime","_listeners","n1","_config","autoSleep","force3D","nullTargetWarn","units","lineHeight","_defaults","overwrite","delay","PI","_HALF_PI","_gsID","sqrt","_cos","cos","sin","_isTypedArray","ArrayBuffer","isView","Array","_complexStringNumExp","_relExp","_startAtRevertConfig","isStart","_revertConfig","_effects","_nextGCFrame","_callbackNames","cycleDuration","whole","data","_zeroPosition","endTime","percentAnimation","offset","isPercent","recent","clippedDuration","_slice","leaveStrings","_flatten","ar","accumulator","call","mapRange","inMin","inMax","outMin","outMax","inRange","outRange","executeLazyFirst","callback","prevContext","context","_ctx","callbackScope","aqua","lime","silver","maroon","teal","blue","navy","white","olive","yellow","orange","gray","purple","green","red","pink","cyan","RegExp","Date","now","tick","_tick","deltaRatio","fps","wake","document","gsapVersions","version","GreenSockGlobals","requestAnimationFrame","sleep","f","setTimeout","cancelAnimationFrame","clearTimeout","lagSmoothing","threshold","adjustedLag","Infinity","once","prioritize","defaultEase","overlap","dispatch","elapsed","manual","power","Linear","easeNone","none","SteppedEase","steps","immediateStart","id","this","set","Animation","startTime","arguments","_ptLookup","_pTime","iteration","_ps","_recacheAncestors","paused","includeRepeats","wrapRepeats","prevIsReverting","globalTime","_sat","repeatDelay","yoyo","seek","restart","includeDelay","play","reversed","reverse","pause","atTime","resume","invalidate","isActive","eventCallback","_onUpdate","then","onFulfilled","self","Promise","resolve","_resolve","_then","_prom","ratio","sortChildren","_this","to","fromTo","fromVars","toVars","delayedCall","staggerTo","stagger","onCompleteAll","onCompleteAllParams","onComplete","onCompleteParams","staggerFrom","staggerFromTo","prevPaused","pauseTween","prevStart","prevIteration","prevTime","tDur","crossingStart","_lock","rewinding","doesWrap","repeatRefresh","onRepeat","_hasPause","_forcing","_findNextPauseTween","_last","onUpdate","adjustedTime","_this2","addLabel","getChildren","tweens","timelines","ignoreBeforeTime","getById","animations","removeLabel","killTweensOf","addPause","removePause","props","onlyActive","getTweensOf","_overwritingTween","children","parsedTargets","isGlobalTime","_targets","tweenTo","initted","tl","onStart","onStartParams","tweenFromTo","fromPosition","toPosition","nextLabel","afterTime","previousLabel","beforeTime","currentLabel","shiftChildren","adjustLabels","soft","clear","includeLabels","updateRoot","_checkPlugin","plugin","pt","ptLookup","_processVars","_parseFuncOrString","style","priority","_parseKeyframe","allProps","easeEach","e","_forceAllPropTweens","stringFilter","funcParam","optional","currentValue","parsedStart","setter","_setterFuncWithParam","_setterFunc","_setterPlain","_addComplexStringPropTween","startNums","endNum","chunk","startNum","hasRandom","_renderComplexString","matchIndex","m","fp","_renderBoolean","_renderPlain","cleanVars","hasPriority","gsData","harnessVars","overwritten","prevStartAt","fullTargets","autoOverwrite","_overwrite","_from","_ptCache","_op","_sortPropTweensByPriority","_onInit","_staggerTweenProps","_staggerPropsToSkip","skipInherit","curTarget","staggerFunc","staggerVarsToMerge","_this3","kf","_hasNoPausedAncestors","isNegative","_renderZeroDurationTween","prevRatio","_parentPlayheadIsBeforeStart","resetTo","startIsRelative","skipRecursion","_updatePropTweens","rootPT","lookup","ptCache","overwrittenProps","curLookup","curOverwriteProps","killingTargets","propTweenLookup","firstPT","_arraysMatch","a1","a2","_addAliasesToVars","propertyAliases","onReverseComplete","onReverseCompleteParams","_setterAttribute","setAttribute","_setterWithModifier","mSet","mt","hasNonDependentRemaining","op","dep","pt2","first","last","pr","change","renderer","TweenMax","TweenLite","TimelineLite","TimelineMax","_dispatch","_emptyArray","_onMediaChange","matches","_lastMediaTime","_media","anyMatch","toggled","queries","conditions","matchMedia","onMatch","_contextID","Context","prevSelector","_r","isReverted","ignore","getTweens","_this4","o","MatchMedia","mq","active","cond","contexts","addListener","addEventListener","registerPlugin","args","getProperty","unit","uncache","getter","format","quickSetter","setters","quickTo","isTweening","registerEffect","effect","plugins","extendTimeline","pluginName","registerEase","parseEase","exportRoot","includeDelayedCalls","matchMediaRefresh","found","removeEventListener","utils","wrap","range","wrapYoyo","total","normalize","clamp","pipe","functions","reduce","unitize","interpolate","mutate","interpolators","il","isString","master","install","effects","ticker","globalTimeline","core","globals","getCache","reverting","toAdd","suppressOverwrites","_getPluginPropTween","_buildModifierPlugin","temp","_addModifiers","modifiers","_renderCSSProp","_renderPropWithEnd","_renderCSSPropWithBeginning","_renderRoundedCSSProp","_renderNonTweeningValue","_renderNonTweeningValueOnlyAtEnd","_setterCSSStyle","_setterCSSProp","setProperty","_setterTransform","_setterScale","scaleX","scaleY","_setterScaleWithRender","renderTransform","_setterTransformWithRender","_saveStyle","isNotCSS","_transformProps","tfm","_propertyAliases","transform","_get","_transformOriginProp","zOrigin","_transformProp","svg","svgo","_removeIndependentTransforms","translate","removeProperty","_revertStyle","_capsExp","_getStyleSaver","properties","saver","save","_createElement","ns","createElementNS","_getComputedProperty","skipPrefixFallback","cs","getComputedStyle","getPropertyValue","_checkPropPrefix","_initCore","_docElement","documentElement","_tempDiv","cssText","_supports3D","_pluginInitted","_getReparentedCloneBBox","bbox","owner","ownerSVGElement","clone","cloneNode","display","appendChild","getBBox","removeChild","_getAttributeFallbacks","attributesArray","hasAttribute","_getBBox","bounds","cloned","error","width","height","_isSVG","getCTM","parentNode","_removeProperty","first2Chars","removeAttribute","_addNonTweeningPT","beginning","onlySetAtEnd","_convertToUnit","px","isSVG","curValue","curUnit","horizontal","_horizontalExp","isRootSVG","tagName","measureProperty","toPixels","toPercent","_nonConvertibleUnits","body","_nonStandardLayouts","_tweenComplexCSSString","startValues","startValue","endValue","endUnit","startUnit","_convertKeywordsToPercentages","_keywordToPercent","_renderClearProps","clearTransforms","scale","rotate","_parseTransform","_isNullTransform","_getComputedTransformMatrixAsArray","matrixString","_identity2DMatrix","_getMatrix","force2D","nextSibling","addedToDOM","matrix","baseVal","consolidate","offsetParent","nextElementSibling","insertBefore","_applySVGOrigin","origin","originIsAbsolute","smooth","matrixArray","pluginToAddPropTweensTo","determinant","xOriginOld","xOrigin","yOriginOld","yOrigin","xOffsetOld","xOffset","yOffsetOld","yOffset","tx","ty","originSplit","_addPxTranslate","_addRotationalPropTween","direction","cap","_RAD2DEG","finalValue","_assign","source","_addRawTransformPTs","transforms","endCache","startCache","_recentSetterPlugin","Power0","Power1","Power2","Power3","Power4","Quad","Cubic","Quart","Quint","Strong","Elastic","Back","Bounce","Sine","Expo","Circ","_DEG2RAD","_atan2","atan2","_complexExp","autoAlpha","alpha","_prefixes","element","preferPrefix","deg","rad","turn","flex","_firstTwoOnly","_specialProps","top","bottom","right","clearProps","_rotationalProperties","z","rotation","rotationX","rotationY","skewX","skewY","perspective","angle","a12","a22","t1","t2","t3","a13","a23","a33","a42","a43","a32","invertedScaleX","forceCSS","xPercent","offsetWidth","yPercent","offsetHeight","transformPerspective","_renderSVGTransforms","_renderCSSTransforms","_renderNon3DTransforms","_zeroDeg","_zeroPx","_endParenthesis","use3D","a11","a21","tan","side","positionAndScale","all","CSSPlugin","specialProp","relative","isTransformRelated","transformPropTween","inlineProps","styles","visibility","parseTransform","smoothOrigin","autoRound","checkPrefix","getStyleSaver","gsapWithCSS","TweenMaxWithCSS"],"mappings":";;;;;;;;;ycAgCa,SAAZA,EAAYC,SAA2B,iBAAXA,EACd,SAAdC,EAAcD,SAA2B,mBAAXA,EAClB,SAAZE,EAAYF,SAA2B,iBAAXA,EACb,SAAfG,EAAeH,eAA2B,IAAXA,EACnB,SAAZI,EAAYJ,SAA2B,iBAAXA,EACd,SAAdK,EAAcL,UAAmB,IAAVA,EACP,SAAhBM,UAAyC,oBAAZC,OACX,SAAlBC,EAAkBR,UAASC,EAAYD,IAAUD,EAAUC,GAchD,SAAXS,EAAWC,UAAUC,EAAgBC,GAAOF,EAAOG,MAAcC,GAChD,SAAjBC,EAAkBC,EAAUhB,UAAUiB,QAAQC,KAAK,mBAAoBF,EAAU,SAAUhB,EAAO,yCAC1F,SAARmB,EAASC,EAASC,UAAcA,GAAYJ,QAAQC,KAAKE,GAC5C,SAAbE,EAAcC,EAAMC,UAASD,IAASV,GAASU,GAAQC,IAASb,IAAkBA,EAAcY,GAAQC,IAAUX,GACrG,SAAbY,WAAmB,EAaR,SAAXC,GAAWC,OAETC,EAAeC,EADZC,EAASH,EAAQ,MAErBvB,EAAU0B,IAAW7B,EAAY6B,KAAYH,EAAU,CAACA,MAClDC,GAAiBE,EAAOC,OAAS,IAAIC,SAAU,KACpDH,EAAII,GAAgBC,OACbL,MAAQI,GAAgBJ,GAAGM,WAAWL,KAC7CF,EAAgBK,GAAgBJ,OAEjCA,EAAIF,EAAQO,OACLL,KACLF,EAAQE,KAAOF,EAAQE,GAAGE,QAAUJ,EAAQE,GAAGE,MAAQ,IAAIK,GAAQT,EAAQE,GAAID,MAAqBD,EAAQU,OAAOR,EAAG,UAEjHF,EAEI,SAAZW,GAAYR,UAAUA,EAAOC,OAASL,GAASa,GAAQT,IAAS,GAAGC,MACpD,SAAfS,GAAgBV,EAAQd,EAAUyB,UAAOA,EAAIX,EAAOd,KAAcf,EAAYwC,GAAKX,EAAOd,KAAeb,EAAasC,IAAMX,EAAOY,cAAgBZ,EAAOY,aAAa1B,IAAcyB,EACtK,SAAfE,GAAgBC,EAAOC,UAAWD,EAAQA,EAAME,MAAM,MAAMC,QAAQF,IAAUD,EACrE,SAATI,GAAShD,UAASiD,KAAKC,MAAc,IAARlD,GAAkB,KAAU,EACzC,SAAhBmD,GAAgBnD,UAASiD,KAAKC,MAAc,IAARlD,GAAoB,KAAY,EACnD,SAAjBoD,GAAkBC,EAAOrD,OACpBsD,EAAWtD,EAAMuD,OAAO,GAC3BC,EAAMC,WAAWzD,EAAM0D,OAAO,WAC/BL,EAAQI,WAAWJ,GACC,MAAbC,EAAmBD,EAAQG,EAAmB,MAAbF,EAAmBD,EAAQG,EAAmB,MAAbF,EAAmBD,EAAQG,EAAMH,EAAQG,EAE/F,SAApBG,GAAqBC,EAAUC,WAC1BC,EAAID,EAAO3B,OACdL,EAAI,EACE+B,EAASG,QAAQF,EAAOhC,IAAM,KAAOA,EAAIiC,WACxCjC,EAAIiC,EAEC,SAAdE,SAGEnC,EAAGoC,EAFAH,EAAII,GAAYhC,OACnBiC,EAAID,GAAYE,MAAM,OAEvBC,GAAc,GAETxC,EADLqC,GAAYhC,OAAS,EACTL,EAAIiC,EAAGjC,KAClBoC,EAAQE,EAAEtC,KACDoC,EAAMK,QAAUL,EAAMM,OAAON,EAAMK,MAAM,GAAIL,EAAMK,MAAM,IAAI,GAAMA,MAAQ,GAGpE,SAAlBE,GAAmBC,EAAWC,EAAMC,EAAgBC,GACnDV,GAAYhC,SAAW2C,GAAcb,KACrCS,EAAUF,OAAOG,EAAMC,EAAgBC,GAAUC,GAAcH,EAAO,IAAMD,EAAUK,UAAYL,EAAUM,WAC5Gb,GAAYhC,SAAW2C,GAAcb,KAEjB,SAArBgB,GAAqBhF,OAChBiF,EAAIxB,WAAWzD,UACXiF,GAAW,IAANA,KAAajF,EAAQ,IAAIkF,MAAMC,IAAoBjD,OAAS,EAAI+C,EAAIlF,EAAUC,GAASA,EAAMoF,OAASpF,EAErG,SAAfqF,GAAeC,UAAKA,EACL,SAAfC,GAAgB/D,EAAKgE,OACf,IAAIF,KAAKE,EACZF,KAAK9D,IAASA,EAAI8D,GAAKE,EAASF,WAE3B9D,EAaK,SAAbiE,GAAcC,EAAMC,OACd,IAAIL,KAAKK,EACP,cAANL,GAA2B,gBAANA,GAA6B,cAANA,IAAsBI,EAAKJ,GAAKlF,EAAUuF,EAAQL,IAAMG,GAAWC,EAAKJ,KAAOI,EAAKJ,GAAK,IAAKK,EAAQL,IAAMK,EAAQL,WAE1JI,EAES,SAAjBE,GAAkBpE,EAAKqE,OAErBP,EADGQ,EAAO,OAENR,KAAK9D,EACR8D,KAAKO,IAAeC,EAAKR,GAAK9D,EAAI8D,WAE7BQ,EAEW,SAAnBC,GAAmBC,OACdC,EAASD,EAAKC,QAAUC,EAC3BrD,EAAOmD,EAAKG,UA3BS,SAAvBC,qBAAuBC,UAAmB,SAAC7E,EAAKgE,OAC1C,IAAIF,KAAKE,EACZF,KAAK9D,GAAe,aAAN8D,GAAoBe,GAA0B,SAANf,IAAiB9D,EAAI8D,GAAKE,EAASF,KAyBlEc,CAAqBE,EAASN,EAAKG,YAAcZ,MACtElF,EAAY2F,EAAKO,cACbN,GACNpD,EAAKmD,EAAMC,EAAOD,KAAKR,UACvBS,EAASA,EAAOA,QAAUA,EAAOO,WAG5BR,EAQa,SAArBS,GAAsBR,EAAQS,EAAOC,EAAsBC,EAAoBC,YAA1CF,IAAAA,EAAY,mBAAUC,IAAAA,EAAW,aAEpEE,EADGC,EAAOd,EAAOW,MAEdC,MACHC,EAAIJ,EAAMG,GACHE,GAAQA,EAAKF,GAAUC,GAC7BC,EAAOA,EAAKC,aAGVD,GACHL,EAAMO,MAAQF,EAAKE,MACnBF,EAAKE,MAAQP,IAEbA,EAAMO,MAAQhB,EAAOU,GACrBV,EAAOU,GAAaD,GAEjBA,EAAMO,MACTP,EAAMO,MAAMD,MAAQN,EAEpBT,EAAOW,GAAYF,EAEpBA,EAAMM,MAAQD,EACdL,EAAMT,OAASS,EAAMF,IAAMP,EACpBS,EAEgB,SAAxBQ,GAAyBjB,EAAQS,EAAOC,EAAsBC,YAAtBD,IAAAA,EAAY,mBAAUC,IAAAA,EAAW,aACpEG,EAAOL,EAAMM,MAChBG,EAAOT,EAAMO,MACVF,EACHA,EAAKE,MAAQE,EACHlB,EAAOU,KAAeD,IAChCT,EAAOU,GAAaQ,GAEjBA,EACHA,EAAKH,MAAQD,EACHd,EAAOW,KAAcF,IAC/BT,EAAOW,GAAYG,GAEpBL,EAAMO,MAAQP,EAAMM,MAAQN,EAAMT,OAAS,KAExB,SAApBmB,GAAqBV,EAAOW,GAC3BX,EAAMT,UAAYoB,GAA6BX,EAAMT,OAAOqB,qBAAuBZ,EAAMT,OAAOsB,QAAUb,EAAMT,OAAOsB,OAAOb,GAC9HA,EAAMc,KAAO,EAEH,SAAXC,GAAYhD,EAAWiC,MAClBjC,KAAeiC,GAASA,EAAMgB,KAAOjD,EAAUkD,MAAQjB,EAAMkB,OAAS,WACrEzD,EAAIM,EACDN,GACNA,EAAE0D,OAAS,EACX1D,EAAIA,EAAE8B,cAGDxB,EAWS,SAAjBqD,GAAkB7D,EAAO8D,EAAWpD,EAAgBC,UAAUX,EAAMc,WAAaF,EAAaZ,EAAMc,SAASiD,OAAOC,IAAwBhE,EAAM+B,KAAKkC,kBAAoBjE,EAAM+B,KAAKmC,YAAelE,EAAMc,SAASR,OAAOwD,GAAW,EAAMnD,IAEpN,SAAxBwD,GAAwB3D,UAAaA,EAAU4D,QAAUC,GAAgB7D,EAAU8D,OAAS9D,EAAYA,EAAU+D,WAAa/D,EAAUgE,SAAYhE,EAAY,EAMvI,SAA1BiE,GAA2BC,EAAYjC,UAAWiC,EAAajC,EAAMkB,QAAUlB,EAAMkC,KAAoB,GAAblC,EAAMkC,IAAW,EAAKlC,EAAMmB,OAASnB,EAAMmC,gBAAkBnC,EAAMoC,OACrJ,SAAVC,GAAUtE,UAAcA,EAAUiD,KAAOvE,GAAcsB,EAAUmD,QAAWnD,EAAUqE,MAAQ7F,KAAK+F,IAAIvE,EAAUmE,KAAOnE,EAAUwE,MAAQC,IAAc,IACvI,SAAjBC,GAAkB1E,EAAWsD,OACxB9B,EAASxB,EAAU+B,WACnBP,GAAUA,EAAOmD,mBAAqB3E,EAAUmE,MACnDnE,EAAUmD,OAASzE,GAAc8C,EAAOoD,OAAyB,EAAhB5E,EAAUmE,IAAUb,EAAYtD,EAAUmE,MAAQnE,EAAUoD,OAASpD,EAAUoE,gBAAkBpE,EAAUqE,OAASf,IAActD,EAAUmE,MAC7LG,GAAQtE,GACRwB,EAAO4B,QAAUJ,GAASxB,EAAQxB,IAE5BA,EAYS,SAAjB6E,GAAkBC,EAAU7C,OACvBI,MACAJ,EAAM2C,QAAW3C,EAAMiB,MAAQjB,EAAM5B,UAAc4B,EAAMkB,OAAS2B,EAASF,QAAU3C,EAAMiB,OAASjB,EAAM8C,QAC7G1C,EAAI4B,GAAwBa,EAASE,UAAW/C,KAC3CA,EAAMiB,MAAQ+B,GAAO,EAAGhD,EAAMmC,gBAAiB/B,GAAKJ,EAAM6B,OAASW,IACvExC,EAAMnC,OAAOuC,GAAG,IAIdW,GAAS8B,EAAU7C,GAAOF,KAAO+C,EAASzE,UAAYyE,EAASF,OAASE,EAAS5B,MAAQ4B,EAASX,IAAK,IAEtGW,EAAS5B,KAAO4B,EAASf,eAC5B1B,EAAIyC,EACGzC,EAAEN,KACQ,GAAfM,EAAE2C,WAAmB3C,EAAEiB,UAAUjB,EAAEyB,QACpCzB,EAAIA,EAAEN,IAGR+C,EAASI,QAAUT,GAGJ,SAAjBU,GAAkBL,EAAU7C,EAAOmD,EAAUC,UAC5CpD,EAAMT,QAAUmB,GAAkBV,GAClCA,EAAMkB,OAASzE,IAAejD,EAAU2J,GAAYA,EAAWA,GAAYN,IAAarD,EAAkB6D,GAAeR,EAAUM,EAAUnD,GAAS6C,EAASF,OAAS3C,EAAMsD,QAC9KtD,EAAMgB,KAAOvE,GAAcuD,EAAMkB,QAAWlB,EAAMmC,gBAAkB5F,KAAK+F,IAAItC,EAAMuD,cAAiB,IACpGxD,GAAmB8C,EAAU7C,EAAO,SAAU,QAAS6C,EAASW,MAAQ,SAAW,GACnFC,GAAmBzD,KAAW6C,EAASa,QAAU1D,GACjDoD,GAAcR,GAAeC,EAAU7C,GACvC6C,EAASX,IAAM,GAAKO,GAAeI,EAAUA,EAAShB,QAC/CgB,EAES,SAAjBc,GAAkB5F,EAAW6F,UAAazJ,GAAS0J,eAAiBxJ,EAAe,gBAAiBuJ,KAAazJ,GAAS0J,cAAcC,OAAOF,EAAS7F,GACpI,SAApBgG,GAAqBxG,EAAOS,EAAME,EAAOD,EAAgB+F,UACxDC,GAAW1G,EAAOS,EAAMgG,GACnBzG,EAAMa,UAGNF,GAASX,EAAM2G,MAAQ/F,IAAgBZ,EAAM0D,OAA4B,IAApB1D,EAAM+B,KAAK6E,OAAqB5G,EAAM0D,MAAQ1D,EAAM+B,KAAK6E,OAAUC,IAAuBC,GAAQC,OAC3J9G,GAAY+G,KAAKhH,GACjBA,EAAMK,MAAQ,CAACoG,EAAO/F,GACf,UALA,EA2EM,SAAfuG,GAAgBzG,EAAW+D,EAAU2C,EAAaC,OAC7CC,EAAS5G,EAAU4D,QACtBiD,EAAMnI,GAAcqF,IAAa,EACjC+C,EAAgB9G,EAAU8D,OAAS9D,EAAUqE,aAC9CyC,IAAkBH,IAAkB3G,EAAU4E,OAASiC,EAAM7G,EAAUkD,MACvElD,EAAUkD,KAAO2D,EACjB7G,EAAUqE,MAASuC,EAAeA,EAAS,EAAI,KAAOlI,GAAcmI,GAAOD,EAAS,GAAM5G,EAAUgE,QAAU4C,GAAlFC,EACZ,EAAhBC,IAAsBH,GAAiBjC,GAAe1E,EAAYA,EAAU8D,OAAS9D,EAAUqE,MAAQyC,GACvG9G,EAAUwB,QAAU8C,GAAQtE,GAC5B0G,GAAe1D,GAAShD,EAAUwB,OAAQxB,GACnCA,EAEiB,SAAzB+G,GAAyB/G,UAAcA,aAAqBgH,GAAYhE,GAAShD,GAAayG,GAAazG,EAAWA,EAAUkD,MA2B7G,SAAnB+D,GAAoBC,EAAMC,EAAQrC,OAIhCsC,EAAQ5F,EAHL6F,EAAW5L,EAAU0L,EAAO,IAC/BG,GAAaD,EAAW,EAAI,IAAMH,EAAO,EAAI,EAAI,GACjD3F,EAAO4F,EAAOG,MAEfD,IAAa9F,EAAKwC,SAAWoD,EAAO,IACpC5F,EAAKC,OAASsD,EACVoC,EAAM,KACTE,EAAS7F,EACTC,EAASsD,EACFtD,KAAY,oBAAqB4F,IACvCA,EAAS5F,EAAOD,KAAKR,UAAY,GACjCS,EAAS5F,EAAY4F,EAAOD,KAAKO,UAAYN,EAAOA,OAErDD,EAAKkC,gBAAkB7H,EAAYwL,EAAO3D,iBAC1CyD,EAAO,EAAK3F,EAAKgG,aAAe,EAAMhG,EAAKiG,QAAUL,EAAOG,EAAY,UAElE,IAAIG,GAAMN,EAAO,GAAI5F,EAAM4F,EAAmB,EAAZG,IAErB,SAArBI,GAAsBnM,EAAO6C,UAAS7C,GAAmB,IAAVA,EAAc6C,EAAK7C,GAAS6C,EAEjE,SAAVuJ,GAAWpM,EAAOyC,UAAO1C,EAAUC,KAAYyC,EAAI4J,GAASC,KAAKtM,IAAeyC,EAAE,GAAP,GAG5D,SAAf8J,GAAgBvM,EAAOwM,UAAaxM,GAAUI,EAAUJ,IAAU,WAAYA,KAAYwM,IAAaxM,EAAMkC,QAAalC,EAAMkC,OAAS,KAAMlC,GAASI,EAAUJ,EAAM,OAAUA,EAAMyM,UAAYzM,IAAU0M,EAInM,SAAXC,GAAW3M,UACVA,EAAQuC,GAAQvC,GAAO,IAAMmB,EAAM,kBAAoB,GAChD,SAAAsB,OACFmK,EAAK5M,EAAM6M,SAAW7M,EAAM8M,eAAiB9M,SAC1CuC,GAAQE,EAAGmK,EAAGG,iBAAmBH,EAAKA,IAAO5M,EAAQmB,EAAM,kBAAoB6L,EAAKC,cAAc,OAASjN,IAG1G,SAAVkN,GAAU/I,UAAKA,EAAEgJ,KAAK,iBAAM,GAAKlK,KAAKmK,WAEzB,SAAbC,GAAa5K,MACRxC,EAAYwC,UACRA,MAEJuD,EAAO5F,EAAUqC,GAAKA,EAAI,CAAC6K,KAAK7K,GACnC8K,EAAOC,GAAWxH,EAAKuH,MACvBE,EAAOzH,EAAKyH,MAAQ,EACpB/H,EAAOjC,WAAWuC,EAAKN,OAAS,EAChCgI,EAAQ,GACRC,EAAoB,EAAPF,GAAYA,EAAO,EAChCG,EAASC,MAAMJ,IAASE,EACxBG,EAAO9H,EAAK8H,KACZC,EAASN,EACTO,EAASP,SACN1N,EAAU0N,GACbM,EAASC,EAAS,CAACC,OAAO,GAAIC,MAAM,GAAI1K,IAAI,GAAGiK,IAAS,GAC7CE,GAAaC,IACxBG,EAASN,EAAK,GACdO,EAASP,EAAK,IAER,SAAC5L,EAAGC,EAAQqC,OAGjBgK,EAASC,EAASC,EAAGC,EAAGC,EAAGC,EAAGC,EAAKC,EAAKC,EAFrC7K,GAAKK,GAAK6B,GAAM9D,OACnB0M,EAAYlB,EAAM5J,OAEd8K,EAAW,MACfD,EAAwB,SAAd3I,EAAK6I,KAAmB,GAAK7I,EAAK6I,MAAQ,CAAC,EAAGC,IAAU,IACrD,KACZL,GAAOK,EACAL,GAAOA,EAAMtK,EAAEwK,KAAUI,wBAAwBC,OAASL,EAAS7K,IAC1E6K,EAAS7K,GAAK6K,QAEfC,EAAYlB,EAAM5J,GAAK,GACvBqK,EAAUP,EAAU3K,KAAKyL,IAAIC,EAAQ7K,GAAKiK,EAAU,GAAKN,EAAOkB,EAChEP,EAAUO,IAAWG,EAAU,EAAIlB,EAAS9J,EAAIkK,EAASW,EAAS,GAAMlB,EAAOkB,EAAU,EAEzFD,EAAMI,EACDN,EAFLC,EAAM,EAEMD,EAAI1K,EAAG0K,IAClBH,EAAKG,EAAIG,EAAUR,EACnBG,EAAIF,GAAYI,EAAIG,EAAU,GAC9BC,EAAUJ,GAAKD,EAAKT,EAA8B7K,KAAK+F,IAAc,MAAT8E,EAAgBQ,EAAID,GAArDY,EAAMZ,EAAIA,EAAIC,EAAIA,GACxCG,EAAJF,IAAaE,EAAMF,GACnBA,EAAIG,IAASA,EAAMH,GAEX,WAATd,GAAsBP,GAAQ0B,GAC/BA,EAAUH,IAAMA,EAAMC,EACtBE,EAAUF,IAAMA,EAChBE,EAAUnM,EAAIqB,GAAKL,WAAWuC,EAAKkJ,SAAYzL,WAAWuC,EAAKsH,OAAkBxJ,EAAT6K,EAAa7K,EAAI,EAAKgK,EAA+C,MAATA,EAAehK,EAAI6K,EAASA,EAA3D1L,KAAKwL,IAAIE,EAAQ7K,EAAI6K,KAAkD,IAAe,UAATlB,GAAoB,EAAI,GAC1MmB,EAAUO,EAAKrL,EAAI,EAAK4B,EAAO5B,EAAI4B,EACnCkJ,EAAUQ,EAAIhD,GAAQpG,EAAKkJ,QAAUlJ,EAAKsH,OAAS,EACnDC,EAAQA,GAAQzJ,EAAI,EAAKuL,GAAY9B,GAAQA,SAE9CzJ,GAAM8K,EAAU/M,GAAK+M,EAAUF,KAAOE,EAAUH,KAAQ,EACjDtL,GAAcyL,EAAUO,GAAK5B,EAAOA,EAAKzJ,GAAKA,GAAK8K,EAAUnM,GAAKmM,EAAUQ,GAGpE,SAAjBE,GAAiB7M,OACZ6C,EAAIrC,KAAKsM,IAAI,KAAM9M,EAAI,IAAIK,MAAM,KAAK,IAAM,IAAIZ,eAC7C,SAAAsN,OACFvK,EAAI9B,GAAcF,KAAKC,MAAMO,WAAW+L,GAAO/M,GAAKA,EAAI6C,UACpDL,EAAIA,EAAI,GAAKK,GAAKpF,EAAUsP,GAAO,EAAIpD,GAAQoD,KAGlD,SAAPC,GAAQC,EAAQ1P,OAEd2P,EAAQC,EADLC,EAAUvJ,EAASoJ,UAElBG,GAAWzP,EAAUsP,KACzBC,EAASE,EAAUH,EAAOC,QAAUb,EAChCY,EAAOI,QACVJ,EAASnN,GAAQmN,EAAOI,SACnBF,GAAQ1P,EAAUwP,EAAO,OAC7BC,GAAUA,IAGXD,EAASJ,GAAeI,EAAOK,YAG1B5D,GAAmBnM,EAAQ6P,EAAmC5P,EAAYyP,GAAU,SAAAF,UAAQI,EAAOF,EAAOF,GAAavM,KAAK+F,IAAI4G,EAAOJ,IAAQG,EAASC,EAAOJ,GAAS,SAAAA,WAM7KQ,EAAIC,EALD5B,EAAI5K,WAAWmM,EAAOJ,EAAInB,EAAImB,GACjClB,EAAI7K,WAAWmM,EAAOJ,EAAIlB,EAAI,GAC9BI,EAAMI,EACNoB,EAAU,EACVrO,EAAI6N,EAAOxN,OAELL,MAILmO,EAHGJ,GACHI,EAAKN,EAAO7N,GAAGwM,EAAIA,GAET2B,GADVC,EAAKP,EAAO7N,GAAGyM,EAAIA,GACC2B,EAEfhN,KAAK+F,IAAI0G,EAAO7N,GAAKwM,IAElBK,IACRA,EAAMsB,EACNE,EAAUrO,UAGZqO,GAAYP,GAAUjB,GAAOiB,EAAUD,EAAOQ,GAAWV,EACjDI,GAAQM,IAAYV,GAAOtP,EAAUsP,GAAQU,EAAUA,EAAU9D,GAAQoD,IArBtCF,GAAeI,IAwBnD,SAATtC,GAAUsB,EAAKD,EAAK0B,EAAmBC,UAAmBjE,GAAmB7F,EAASoI,IAAQD,GAA4B,IAAtB0B,KAAgCA,EAAoB,IAAMC,EAAgB,kBAAM9J,EAASoI,GAAOA,KAAOzL,KAAKmK,SAAWsB,EAAIxM,UAAYiO,EAAoBA,GAAqB,QAAUC,EAAiBD,EAAoB,WAAI,IAAQA,EAAoB,IAAIjO,OAAS,GAAK,IAAOe,KAAKoN,MAAMpN,KAAKC,OAAOwL,EAAMyB,EAAoB,EAAIlN,KAAKmK,UAAYqB,EAAMC,EAA0B,IAApByB,IAA4BA,GAAqBA,EAAoBC,GAAkBA,IAIxhB,SAAbE,GAAcnM,EAAGoM,EAASvQ,UAAUmM,GAAmBnM,EAAO,SAAAwQ,UAASrM,IAAIoM,EAAQC,MAalE,SAAjBC,GAAiBzQ,WAGf6B,EAAG6O,EAAMlN,EAAKqM,EAFX9I,EAAO,EACV4J,EAAI,KAEI9O,EAAI7B,EAAM+D,QAAQ,UAAWgD,KACrCvD,EAAMxD,EAAM+D,QAAQ,IAAKlC,GACzBgO,EAAkC,MAAxB7P,EAAMuD,OAAO1B,EAAI,GAC3B6O,EAAO1Q,EAAM0D,OAAO7B,EAAI,EAAG2B,EAAM3B,EAAI,GAAGqD,MAAM2K,EAAU1K,GAAqByL,IAC7ED,GAAK3Q,EAAM0D,OAAOqD,EAAMlF,EAAIkF,GAAQqG,GAAOyC,EAAUa,GAAQA,EAAK,GAAIb,EAAU,GAAKa,EAAK,IAAKA,EAAK,IAAM,MAC1G3J,EAAOvD,EAAM,SAEPmN,EAAI3Q,EAAM0D,OAAOqD,EAAM/G,EAAMkC,OAAS6E,GA4CvB,SAAvB8J,GAAwBtH,EAAUuH,EAAUC,OAG1CzL,EAAG0L,EAAUC,EAFVC,EAAS3H,EAAS2H,OACrBxC,EAAMI,MAEFxJ,KAAK4L,GACTF,EAAWE,EAAO5L,GAAKwL,GACP,KAASC,GAAYC,GAAYtC,GAAOsC,EAAW/N,KAAK+F,IAAIgI,MAC3EC,EAAQ3L,EACRoJ,EAAMsC,UAGDC,EAmBK,SAAbE,GAAa1M,UACZ2C,GAAkB3C,GAClBA,EAAU2M,eAAiB3M,EAAU2M,cAAcC,OAAOxM,GAC1DJ,EAAU6M,WAAa,GAAKC,GAAU9M,EAAW,eAC1CA,EAIQ,SAAhB+M,GAAgBC,MACVA,KACLA,GAAWA,EAAOlQ,MAAQkQ,WAAmBA,EACzCnR,KAAmBmR,EAAOC,SAAU,KACnCnQ,EAAOkQ,EAAOlQ,KACjBoQ,EAAS1R,EAAYwR,GACrBG,EAAUrQ,IAASoQ,GAAUF,EAAOI,KAAQ,gBACtCC,OAAS,IACXL,EACJM,EAAmB,CAACF,KAAMpQ,EAAY8C,OAAQyN,GAAmBxI,IAAKyI,GAAeZ,KAAMa,GAAmBC,SAAUC,GAAoBC,QAAS,GACrJC,EAAU,CAACnQ,WAAY,EAAGoQ,IAAK,EAAGC,UAAWC,GAAYC,QAAS,GAAIC,SAAU,MACjFC,KACInB,IAAWG,EAAQ,IAClBiB,GAAStR,UAGbgE,GAAaqM,EAAQrM,GAAaK,GAAe6L,EAAQM,GAAmBO,IAC5E1R,GAAOgR,EAAOkB,UAAWlS,GAAOmR,EAAkBnM,GAAe6L,EAAQa,KACzEO,GAAUjB,EAAOmB,KAAOxR,GAASqQ,EAC7BH,EAAOtP,aACVF,GAAgBgJ,KAAK2G,GACrBoB,GAAezR,GAAQ,GAExBA,GAAiB,QAATA,EAAiB,MAAQA,EAAKgC,OAAO,GAAG0P,cAAgB1R,EAAKmC,OAAO,IAAM,SAEnFpC,EAAWC,EAAMqQ,GACjBH,EAAOkB,UAAYlB,EAAOkB,SAAS7R,GAAM8Q,EAAQsB,SAEjDC,GAAqBlI,KAAKwG,GAkDrB,SAAP2B,GAAQC,EAAGC,EAAIC,UAEC,GADfF,GAAKA,EAAI,EAAI,EAAQ,EAAJA,GAAS,EAAI,GACX,EAAKC,GAAMC,EAAKD,GAAMD,EAAI,EAAIA,EAAI,GAAKE,EAAU,EAAJF,EAAQ,EAAKC,GAAMC,EAAKD,IAAO,EAAI,EAAID,GAAK,EAAIC,GAAME,GAAQ,GAAM,EAExH,SAAbC,GAAchR,EAAGiR,EAAOC,OAEtBC,EAAGC,EAAG1E,EAAGkE,EAAG1C,EAAG7M,EAAG2K,EAAKC,EAAKH,EAAGuF,EAD5B3P,EAAK1B,EAAyBvC,EAAUuC,GAAK,CAACA,GAAK,GAAKA,GAAK,EAAK+Q,GAAM/Q,EAAI+Q,IAAQ,EAA3EO,GAAaC,UAErB7P,EAAG,IACc,MAAjB1B,EAAEiB,QAAQ,KACbjB,EAAIA,EAAEiB,OAAO,EAAGjB,EAAEP,OAAS,IAExB6R,GAAatR,GAChB0B,EAAI4P,GAAatR,QACX,GAAoB,MAAhBA,EAAEc,OAAO,GAAY,IAC3Bd,EAAEP,OAAS,IAIdO,EAAI,KAHJmR,EAAInR,EAAEc,OAAO,IAGCqQ,GAFdC,EAAIpR,EAAEc,OAAO,IAESsQ,GADtB1E,EAAI1M,EAAEc,OAAO,IACiB4L,GAAkB,IAAb1M,EAAEP,OAAeO,EAAEc,OAAO,GAAKd,EAAEc,OAAO,GAAK,KAEhE,IAAbd,EAAEP,aAEE,EADPiC,EAAI8P,SAASxR,EAAEiB,OAAO,EAAG,GAAI,MAChB,GAAKS,GAAK,EAAKqP,GAAMrP,EAAIqP,GAAMS,SAASxR,EAAEiB,OAAO,GAAI,IAAM,KAGzES,EAAI,EADJ1B,EAAIwR,SAASxR,EAAEiB,OAAO,GAAI,MAChB,GAAKjB,GAAK,EAAK+Q,GAAM/Q,EAAI+Q,SAC7B,GAAuB,QAAnB/Q,EAAEiB,OAAO,EAAG,MACtBS,EAAI2P,EAASrR,EAAEyC,MAAM0L,IAChB8C,GAUE,IAAKjR,EAAEsB,QAAQ,YACrBI,EAAI1B,EAAEyC,MAAMgP,IACZP,GAAcxP,EAAEjC,OAAS,IAAMiC,EAAE,GAAK,GAC/BA,OAZPkP,GAAMlP,EAAE,GAAK,IAAO,IACpBwM,EAAKxM,EAAE,GAAK,IAGZyP,EAAQ,GAFR9P,EAAKK,EAAE,GAAK,MACZ0P,EAAK/P,GAAK,GAAMA,GAAK6M,EAAI,GAAK7M,EAAI6M,EAAI7M,EAAI6M,GAE/B,EAAXxM,EAAEjC,SAAeiC,EAAE,IAAM,GACzBA,EAAE,GAAKiP,GAAKC,EAAI,EAAI,EAAGO,EAAGC,GAC1B1P,EAAE,GAAKiP,GAAKC,EAAGO,EAAGC,GAClB1P,EAAE,GAAKiP,GAAKC,EAAI,EAAI,EAAGO,EAAGC,QAO3B1P,EAAI1B,EAAEyC,MAAM0L,KAAkBmD,GAAaI,YAE5ChQ,EAAIA,EAAEiQ,IAAIC,eAEPX,IAAUI,IACbF,EAAIzP,EAAE,GAAKqP,GACXK,EAAI1P,EAAE,GAAKqP,GACXrE,EAAIhL,EAAE,GAAKqP,GAGX1P,IAFA2K,EAAMxL,KAAKwL,IAAImF,EAAGC,EAAG1E,KACrBT,EAAMzL,KAAKyL,IAAIkF,EAAGC,EAAG1E,KACH,EACdV,IAAQC,EACX2E,EAAI1C,EAAI,GAERpC,EAAIE,EAAMC,EACViC,EAAQ,GAAJ7M,EAAUyK,GAAK,EAAIE,EAAMC,GAAOH,GAAKE,EAAMC,GAC/C2E,EAAI5E,IAAQmF,GAAKC,EAAI1E,GAAKZ,GAAKsF,EAAI1E,EAAI,EAAI,GAAKV,IAAQoF,GAAK1E,EAAIyE,GAAKrF,EAAI,GAAKqF,EAAIC,GAAKtF,EAAI,EAC5F8E,GAAK,IAENlP,EAAE,MAAQkP,EAAI,IACdlP,EAAE,MAAY,IAAJwM,EAAU,IACpBxM,EAAE,MAAY,IAAJL,EAAU,KAErB6P,GAAcxP,EAAEjC,OAAS,IAAMiC,EAAE,GAAK,GAC/BA,EAEU,SAAlBmQ,GAAkB7R,OACbqN,EAAS,GACZyE,EAAI,GACJ1S,GAAK,SACNY,EAAEK,MAAM0R,IAAWzR,QAAQ,SAAAN,OACtB0B,EAAI1B,EAAEyC,MAAMuP,KAAoB,GACpC3E,EAAO7E,WAAP6E,EAAe3L,GACfoQ,EAAEtJ,KAAKpJ,GAAKsC,EAAEjC,OAAS,KAExB4N,EAAOyE,EAAIA,EACJzE,EAEQ,SAAhB4E,GAAiB/D,EAAG+C,EAAOiB,OAKzBJ,EAAGK,EAAOrG,EAAGzK,EAJV+Q,EAAS,GACZC,GAAUnE,EAAIkE,GAAQ3P,MAAMsP,IAC5B7I,EAAO+H,EAAQ,QAAU,QACzB7R,EAAI,MAEAiT,SACGnE,KAERmE,EAASA,EAAOV,IAAI,SAAAW,UAAUA,EAAQtB,GAAWsB,EAAOrB,EAAO,KAAO/H,GAAQ+H,EAAQqB,EAAM,GAAK,IAAMA,EAAM,GAAK,KAAOA,EAAM,GAAK,KAAOA,EAAM,GAAKA,EAAMC,KAAK,MAAQ,MACrKL,IACHpG,EAAI+F,GAAgB3D,IACpB4D,EAAII,EAAeJ,GACbS,KAAKH,KAAYtG,EAAEgG,EAAES,KAAKH,QAE/B/Q,GADA8Q,EAAQjE,EAAEsE,QAAQT,GAAW,KAAK1R,MAAM2R,KAC9BvS,OAAS,EACZL,EAAIiC,EAAGjC,IACbgT,GAAUD,EAAM/S,KAAO0S,EAAExQ,QAAQlC,GAAKiT,EAAOI,SAAWvJ,EAAO,YAAc4C,EAAErM,OAASqM,EAAIuG,EAAO5S,OAAS4S,EAASH,GAAgBO,aAInIN,MAEJ9Q,GADA8Q,EAAQjE,EAAE7N,MAAM0R,KACNtS,OAAS,EACZL,EAAIiC,EAAGjC,IACbgT,GAAUD,EAAM/S,GAAKiT,EAAOjT,UAGvBgT,EAASD,EAAM9Q,GAWF,SAArBqR,GAAqBhR,OAEnBuP,EADG0B,EAAWjR,EAAE6Q,KAAK,QAEtBR,GAAUa,UAAY,EAClBb,GAAUc,KAAKF,UAClB1B,EAAQ6B,GAAQD,KAAKF,GACrBjR,EAAE,GAAKuQ,GAAcvQ,EAAE,GAAIuP,GAC3BvP,EAAE,GAAKuQ,GAAcvQ,EAAE,GAAIuP,EAAOY,GAAgBnQ,EAAE,MAC7C,EA2Je,SAAxBqR,GAAwBjU,OACnBuB,GAASvB,EAAO,IAAIuB,MAAM,KAC7ByK,EAAOkI,GAAS3S,EAAM,WACfyK,GAAuB,EAAfzK,EAAMZ,QAAcqL,EAAKkE,OAAUlE,EAAKkE,OAAOiE,MAAM,MAAOnU,EAAKwC,QAAQ,KAAO,CAzB1E,SAAvB4R,qBAAuB3V,WAMrBwQ,EAAOoF,EAAKC,EALTrU,EAAM,GACTsB,EAAQ9C,EAAM0D,OAAO,EAAG1D,EAAMkC,OAAO,GAAGY,MAAM,KAC9CgT,EAAMhT,EAAM,GACZjB,EAAI,EACJiC,EAAIhB,EAAMZ,OAEJL,EAAIiC,EAAGjC,IACb+T,EAAM9S,EAAMjB,GACZ2O,EAAQ3O,IAAMiC,EAAE,EAAI8R,EAAIG,YAAY,KAAOH,EAAI1T,OAC/C2T,EAAYD,EAAIlS,OAAO,EAAG8M,GAC1BhP,EAAIsU,GAAOjI,MAAMgI,GAAaA,EAAUZ,QAAQe,GAAY,IAAI5Q,QAAUyQ,EAC1EC,EAAMF,EAAIlS,OAAO8M,EAAM,GAAGpL,cAEpB5D,EAW0FmU,CAAqB7S,EAAM,KATvG,SAAtBmT,oBAAsBjW,OACjBkW,EAAOlW,EAAM+D,QAAQ,KAAO,EAC/BoS,EAAQnW,EAAM+D,QAAQ,KACtBqS,EAASpW,EAAM+D,QAAQ,IAAKmS,UACtBlW,EAAMqW,UAAUH,GAAOE,GAAUA,EAASD,EAAQnW,EAAM+D,QAAQ,IAAKoS,EAAQ,GAAKA,GAK0CF,CAAoB1U,GAAMuB,MAAM,KAAKsR,IAAIpP,KAAwByQ,GAASa,KAAOC,GAAejB,KAAK/T,GAASkU,GAASa,IAAI,GAAI/U,GAAQgM,EAItP,SAArBiJ,GAAsBjN,EAAUkN,WACFlJ,EAAzB7G,EAAQ6C,EAASmN,OACdhQ,GACFA,aAAiB+E,GACpB+K,GAAmB9P,EAAO+P,IAChB/P,EAAMV,KAAK2Q,UAAcjQ,EAAMkQ,OAAUlQ,EAAM2B,SAAY3B,EAAMkQ,QAAUH,IACjF/P,EAAM6C,SACTiN,GAAmB9P,EAAM6C,SAAUkN,IAEnClJ,EAAO7G,EAAMmQ,MACbnQ,EAAMmQ,MAAQnQ,EAAMoQ,OACpBpQ,EAAMoQ,OAASvJ,EACf7G,EAAMkQ,MAAQH,IAGhB/P,EAAQA,EAAMO,MAIF,SAAd8P,GAAenU,EAAOoU,EAAQC,EAAkCC,YAAlCD,IAAAA,EAAU,iBAAA3R,UAAK,EAAI0R,EAAO,EAAI1R,cAAI4R,IAAAA,EAAa,mBAAA5R,UAAKA,EAAI,GAAK0R,EAAW,EAAJ1R,GAAS,EAAI,EAAI0R,EAAiB,GAAT,EAAI1R,IAAU,QAEvI6R,EADG5J,EAAO,CAACyJ,OAAAA,EAAQC,QAAAA,EAASC,UAAAA,UAE7BvU,GAAaC,EAAO,SAAArB,OAGd,IAAI+D,KAFTmQ,GAASlU,GAAQV,GAASU,GAAQgM,EAClCkI,GAAU0B,EAAgB5V,EAAK6V,eAAkBH,EACnC1J,EACbkI,GAAS0B,GAAuB,WAAN7R,EAAiB,MAAc,YAANA,EAAkB,OAAS,WAAamQ,GAASlU,EAAO,IAAM+D,GAAKiI,EAAKjI,KAGtHiI,EAEY,SAApB8J,GAAoBJ,UAAY,SAAA3R,UAAKA,EAAI,IAAM,EAAI2R,EAAQ,EAAS,EAAJ3R,IAAW,EAAI,GAAK2R,EAAmB,GAAV3R,EAAI,KAAW,GAC3F,SAAjBgS,GAAkB3L,EAAM4L,EAAWC,GAIvB,SAAVP,GAAU3R,UAAW,IAANA,EAAU,EAAImS,WAAM,GAAO,GAAKnS,GAAMoS,GAAMpS,EAAIqS,GAAMC,GAAM,MAHxEH,EAAmB,GAAbF,EAAkBA,EAAY,EACvCK,GAAMJ,IAAW7L,EAAO,GAAK,OAAS4L,EAAY,EAAIA,EAAY,GAClEI,EAAKC,EAAKC,GAAQ5U,KAAK6U,KAAK,EAAIL,IAAO,GAEvClK,EAAiB,QAAT5B,EAAkBsL,GAAoB,OAATtL,EAAiB,SAAArG,UAAK,EAAI2R,GAAQ,EAAI3R,IAAK+R,GAAkBJ,WACnGW,EAAKC,EAAOD,EACZrK,EAAKkE,OAAS,SAAC8F,EAAWC,UAAWF,GAAe3L,EAAM4L,EAAWC,IAC9DjK,EAEM,SAAdwK,GAAepM,EAAMqM,GACN,SAAVf,GAAU3R,UAAKA,IAAQA,EAAKA,IAAM0S,EAAY,GAAK1S,EAAI0S,GAAa,EAAK,WADzDA,IAAAA,EAAY,aAE/BzK,EAAgB,QAAT5B,EAAiBsL,GAAmB,OAATtL,EAAgB,SAAArG,UAAK,EAAI2R,GAAQ,EAAI3R,IAAK+R,GAAkBJ,WAC/F1J,EAAKkE,OAAS,SAAAuG,UAAaD,GAAYpM,EAAMqM,IACtCzK,EAviCT,IAWC0K,EACApT,EAAYqT,EA0BZhS,EAAiBwG,EAAMyL,EAAcnL,EAErCrM,EACAyX,EAYAtN,EAilBAuN,EAyOAC,EAUEC,EAAKC,EAAMC,EAAMC,EAAOC,EAAQC,EAR7BC,EACHC,EACAC,EACAC,EACAC,EACAC,EACAC,EACAC,EAqMDnU,EACGoU,EA9jCDC,EAAU,CACZC,UAAW,IACXC,QAAS,OACTC,eAAgB,EAChBC,MAAO,CAACC,WAAW,KAEpBC,EAAY,CACXpR,SAAU,GACVqR,WAAW,EACXC,MAAO,GAIRhL,EAAU,IACV5F,EAAW,EAAI4F,EACf+I,EAAiB,EAAV5U,KAAK8W,GACZC,EAAWnC,EAAO,EAClBoC,EAAQ,EACRhL,EAAQhM,KAAKiX,KACbC,EAAOlX,KAAKmX,IACZ1C,EAAOzU,KAAKoX,IASZC,EAAwC,mBAAhBC,aAA8BA,YAAYC,QAAW,aAC7ElU,EAAWmU,MAAM5K,QACjBe,GAAgB,oBAChBsD,GAAU,mCACVO,GAAkB,8BAClBiG,GAAuB,mCACvBC,GAAU,gBACVxV,GAAqB,kBACrBkH,GAAW,wCAEXxL,GAAW,GAQX+Z,GAAuB,CAACjW,gBAAgB,EAAMkW,SAAS,EAAMxJ,MAAM,GACnEpJ,GAAsB,CAACtD,gBAAgB,EAAM0M,MAAM,GACnDyJ,GAAgB,CAACnW,gBAAgB,GACjCqO,GAAiB,GACjB9O,GAAc,GACdG,GAAc,GAEdwO,GAAW,GACXkI,GAAW,GACXC,GAAe,GACf/Y,GAAkB,GAClBgZ,GAAiB,GAiEjBra,GAAS,SAATA,OAAU8E,EAAMC,OACV,IAAIL,KAAKK,EACbD,EAAKJ,GAAKK,EAAQL,UAEZI,GAoGR4C,GAAkB,SAAlBA,gBAAmBoC,EAAOwQ,OACrBC,EAAQlY,KAAKoN,MAAM3F,EAAQvH,GAAcuH,EAAQwQ,WAC9CxQ,GAAUyQ,IAAUzQ,EAASyQ,EAAQ,EAAIA,GAmEjDhR,GAAqB,SAArBA,0BAAuBiR,IAAAA,WAAmB,gBAATA,GAAmC,YAATA,GA+E3DC,GAAgB,CAACzT,OAAO,EAAG0T,QAAQ7Z,EAAYoH,cAAcpH,GAC7DsI,GAAiB,SAAjBA,eAAkBtF,EAAWoF,EAAU0R,OAIrC1Z,EAAG2Z,EAAQC,EAHRvK,EAASzM,EAAUyM,OACtBwK,EAASjX,EAAU2F,SAAWiR,GAC9BM,EAAkBlX,EAAU+D,YAAcsG,EAAU4M,EAAOJ,SAAQ,GAAS7W,EAAUkD,YAEnF5H,EAAU8J,KAAcgE,MAAMhE,IAAcA,KAAYqH,IAC3DsK,EAAS3R,EAAStG,OAAO,GACzBkY,EAAoC,MAAxB5R,EAASnG,QAAQ,GAC7B7B,EAAIgI,EAAS9F,QAAQ,KACN,MAAXyX,GAA6B,MAAXA,GAChB,GAAL3Z,IAAWgI,EAAWA,EAASoL,QAAQ,IAAK,MACzB,MAAXuG,EAAiBE,EAAO9T,OAAS8T,EAAOJ,QAA0B,GAAlBI,EAAOrT,WAAkB5E,WAAWoG,EAASnG,OAAO,KAAO,IAAM+X,GAAa5Z,EAAI,EAAI6Z,EAASH,GAAkB1S,gBAAkB,IAAM,IAE9LhH,EAAI,GACNgI,KAAYqH,IAAYA,EAAOrH,GAAY8R,GACrCzK,EAAOrH,KAEf2R,EAAS/X,WAAWoG,EAAStG,OAAO1B,EAAE,GAAKgI,EAASnG,OAAO7B,EAAE,IACzD4Z,GAAaF,IAChBC,EAASA,EAAS,KAAOlV,EAASiV,GAAoBA,EAAiB,GAAKA,GAAkB1S,iBAEnF,EAAJhH,EAASkI,eAAetF,EAAWoF,EAASnG,OAAO,EAAG7B,EAAE,GAAI0Z,GAAoBC,EAASG,EAAkBH,IAEhG,MAAZ3R,EAAoB8R,GAAmB9R,GAsBhDH,GAAS,SAATA,OAAUgF,EAAKD,EAAKzO,UAAUA,EAAQ0O,EAAMA,EAAcD,EAARzO,EAAcyO,EAAMzO,GAGtE4b,GAAS,GAAGxX,MAIZ7B,GAAU,SAAVA,QAAWvC,EAAOU,EAAOmb,UAAiB3D,IAAaxX,GAASwX,EAASvL,SAAWuL,EAASvL,SAAS3M,IAASD,EAAUC,IAAW6b,IAAiB1D,GAAiBvF,KAAqEtM,EAAStG,GAFzO,SAAX8b,SAAYC,EAAIF,EAAcG,mBAAAA,IAAAA,EAAc,IAAOD,EAAGhZ,QAAQ,SAAA/C,UAAUD,EAAUC,KAAW6b,GAAiBtP,GAAavM,EAAO,GAAKgc,EAAY/Q,WAAZ+Q,EAAoBzZ,GAAQvC,IAAUgc,EAAY/Q,KAAKjL,MAAWgc,EAEoDF,CAAS9b,EAAO6b,GAAgBtP,GAAavM,GAAS4b,GAAOK,KAAKjc,EAAO,GAAKA,EAAQ,CAACA,GAAS,GAA5K4b,GAAOK,MAAMvb,GAASsM,GAAMD,iBAAiB/M,GAAQ,IA4ItOkc,GAAW,SAAXA,SAAYC,EAAOC,EAAOC,EAAQC,EAAQtc,OACrCuc,EAAUH,EAAQD,EACrBK,EAAWF,EAASD,SACdlQ,GAAmBnM,EAAO,SAAAA,UAASqc,IAAarc,EAAQmc,GAASI,EAAWC,GAAa,MAoDjGjL,GAAY,SAAZA,UAAa9M,EAAWkH,EAAM8Q,OAK5B7Q,EAAQlL,EAAOmU,EAJZpS,EAAIgC,EAAUuB,KACjB0W,EAAWja,EAAEkJ,GACbgR,EAAczE,EACd0E,EAAUnY,EAAUoY,QAEhBH,SAGL9Q,EAASnJ,EAAEkJ,EAAO,UAClBjL,EAAQ+B,EAAEqa,eAAiBrY,EAC3BgY,GAAoBvY,GAAYhC,QAAU8B,KAC1C4Y,IAAY1E,EAAW0E,GACvB/H,EAASjJ,EAAS8Q,EAAShH,MAAMhV,EAAOkL,GAAU8Q,EAAST,KAAKvb,GAChEwX,EAAWyE,EACJ9H,GASR1B,GAAuB,GAsDvBK,GAAO,IACPO,GAAe,CACdgJ,KAAK,CAAC,EAAEvJ,GAAKA,IACbwJ,KAAK,CAAC,EAAExJ,GAAK,GACbyJ,OAAO,CAAC,IAAI,IAAI,KAChBjJ,MAAM,CAAC,EAAE,EAAE,GACXkJ,OAAO,CAAC,IAAI,EAAE,GACdC,KAAK,CAAC,EAAE,IAAI,KACZC,KAAK,CAAC,EAAE,EAAE5J,IACV6J,KAAK,CAAC,EAAE,EAAE,KACVC,MAAM,CAAC9J,GAAKA,GAAKA,IACjB+J,MAAM,CAAC,IAAI,IAAI,GACfC,OAAO,CAAChK,GAAKA,GAAK,GAClBiK,OAAO,CAACjK,GAAK,IAAI,GACjBkK,KAAK,CAAC,IAAI,IAAI,KACdC,OAAO,CAAC,IAAI,EAAE,KACdC,MAAM,CAAC,EAAE,IAAI,GACbC,IAAI,CAACrK,GAAK,EAAE,GACZsK,KAAK,CAACtK,GAAK,IAAI,KACfuK,KAAK,CAAC,EAAEvK,GAAKA,IACbW,YAAY,CAACX,GAAKA,GAAKA,GAAK,IAqH7BgB,GAAa,eAEXlP,EADGqL,EAAI,6EAEHrL,KAAKyO,GACTpD,GAAK,IAAMrL,EAAI,aAET,IAAI0Y,OAAOrN,EAAI,IAAK,MANf,GAQb4E,GAAU,YAkCVxK,IACK8N,EAAWoF,KAAKC,IACnBpF,EAAgB,IAChBC,EAAe,GACfC,EAAaH,IACbI,EAAcD,EAEdG,EADAD,EAAO,IAAO,IA0BfR,EAAQ,CACPhU,KAAK,EACLsG,MAAM,EACNmT,qBACCC,IAAM,IAEPC,+BAAWC,UACH3F,GAAU,KAAQ2F,GAAO,MAEjCC,qBACKnG,KACED,GAAgB7X,MACpBoM,EAAOyL,EAAe5X,OACtByM,EAAON,EAAK8R,UAAY,GACxB3d,GAASC,KAAOA,IACf4L,EAAK+R,eAAiB/R,EAAK+R,aAAe,KAAKxT,KAAKnK,GAAK4d,SAC1Dje,EAASE,GAAiB+L,EAAKiS,mBAAsBjS,EAAK5L,MAAQ4L,GAAS,IAC3EyG,GAAqBpQ,QAAQyO,KAE9BiH,EAAyC,oBAA3BmG,uBAA0CA,sBACxDrG,GAAOG,EAAMmG,QACbrG,EAAOC,GAAS,SAAAqG,UAAKC,WAAWD,EAAI3F,EAAyB,IAAbT,EAAMhU,KAAc,EAAK,IACzE4T,EAAgB,EAChB8F,GAAM,KAGRS,wBACEpG,EAAOuG,qBAAuBC,cAAc1G,GAC7CD,EAAgB,EAChBE,EAAO/W,GAERyd,mCAAaC,EAAWC,GACvBtG,EAAgBqG,GAAaE,EAAAA,EAC7BtG,EAAe9V,KAAKyL,IAAI0Q,GAAe,GAAItG,IAE5CwF,iBAAIA,GACHpF,EAAO,KAAQoF,GAAO,KACtBnF,EAAyB,IAAbT,EAAMhU,KAAcwU,GAEjC1P,iBAAIkT,EAAU4C,EAAMC,OACf1c,EAAOyc,EAAO,SAACxY,EAAGyH,EAAGuQ,EAAGrc,GAAOia,EAAS5V,EAAGyH,EAAGuQ,EAAGrc,GAAIiW,EAAMnR,OAAO1E,IAAU6Z,SAChFhE,EAAMnR,OAAOmV,GACbtD,EAAWmG,EAAa,UAAY,QAAQ1c,GAC5C+P,KACO/P,GAER0E,uBAAOmV,EAAU7a,KACdA,EAAIuX,EAAWrV,QAAQ2Y,KAActD,EAAW/W,OAAOR,EAAG,IAAYA,GAAN+W,GAAWA,KAE9EQ,WAzEAA,EAAa,KA6EfxG,GAAQ,SAARA,eAAe0F,GAAiBvN,GAAQwT,QAoBxC9I,GAAW,GACXc,GAAiB,sBACjBP,GAAa,QA4Bb3G,GAAc,SAAdA,YAAc9B,UAAQ,SAAAjI,UAAK,EAAIiI,EAAK,EAAIjI,KAoBxCkI,GAAa,SAAbA,WAAcD,EAAMiS,UAAiBjS,IAAsBtN,EAAYsN,GAAQA,EAAOkI,GAASlI,IAASiI,GAAsBjI,KAAlFiS,GAjJlC,SAARpB,GAAQ3b,OAGNgd,EAASC,EAAUhb,EAAMsG,EAFtB2U,EAAU9G,IAAaI,EAC1B2G,GAAe,IAANnd,MAECqW,EAAV6G,GAA2BA,EAAU,KAAO3G,GAAc2G,EAAU5G,IAIvD,GADd0G,GADA/a,GADAuU,GAAe0G,GACM3G,GACJG,IACEyG,KAClB5U,IAAU0N,EAAM1N,MAChB2N,EAASjU,EAAoB,IAAbgU,EAAMhU,KACtBgU,EAAMhU,KAAOA,GAAc,IAC3ByU,GAAasG,GAAsBvG,GAAXuG,EAAkB,EAAIvG,EAAOuG,GACrDC,EAAW,GAEZE,IAAWrH,EAAMC,EAAK4F,KAClBsB,MACE9G,EAAK,EAAGA,EAAKQ,EAAWlX,OAAQ0W,IACpCQ,EAAWR,GAAIlU,EAAMiU,EAAQ3N,EAAOvI,GAqL9B,SAAVwU,GAAU3R,UAAMA,EAAI+T,EAAMpU,EAAIK,EAAIA,EAAKA,EAFlC,kBAE4CL,WAAKK,EAAI,IAEjD,KAF6D,GAAI,IAAOA,EAD5E,kBACsFL,GAAKK,GAAK,KAE5F,MAFwGA,EAAI,MAAQL,WAAKK,EAAI,MAE7H,KAF2I,GAAI,QAV1J3C,GAAa,uCAAwC,SAACpB,EAAMM,OACvDge,EAAQhe,EAAI,EAAIA,EAAI,EAAIA,EAC5BkV,GAAYxV,EAAO,UAAYse,EAAQ,GAAIhe,EAAI,SAAAyD,mBAAKA,EAAKua,IAAQ,SAAAva,UAAKA,GAAG,SAAAA,UAAK,WAAK,EAAIA,EAAMua,IAAO,SAAAva,UAAKA,EAAI,GAAKrC,SAAK,EAAJqC,EAAUua,GAAQ,EAAI,EAAI5c,SAAW,GAAT,EAAIqC,GAAWua,GAAQ,MAEvKpK,GAASqK,OAAOC,SAAWtK,GAASuK,KAAOvK,GAASqK,OAAO9I,OAC3DD,GAAY,UAAWO,GAAe,MAAOA,GAAe,OAAQA,MAClErS,EAMC,OALEoU,EAAK,EAKC,KADVtC,GAAY,SAAU,SAAAzR,UAAK,EAAI2R,GAAQ,EAAI3R,IAAI2R,IAEhDF,GAAY,OAAQ,SAAAzR,UAAKrC,SAAC,EAAM,IAAMqC,EAAI,IAAOA,EAAIA,EAAIA,EAAIA,EAAIA,EAAIA,EAAIA,GAAK,EAAEA,KAChFyR,GAAY,OAAQ,SAAAzR,WAAO2J,EAAM,EAAK3J,EAAIA,GAAM,KAChDyR,GAAY,OAAQ,SAAAzR,UAAW,IAANA,EAAU,EAA0B,EAArB6U,EAAK7U,EAAI0U,KACjDjD,GAAY,OAAQgB,GAAY,MAAOA,GAAY,OAAQA,MAC3DtC,GAASwK,YAAcxK,GAASyK,MAAQrf,GAASof,YAAc,CAC9DxO,uBAAOyO,EAAWC,YAAXD,IAAAA,EAAQ,OACVzI,EAAK,EAAIyI,EACZtI,EAAKsI,GAASC,EAAiB,EAAI,GACnCxI,EAAKwI,EAAiB,EAAI,SAEpB,SAAA7a,WAAQsS,EAAKlO,GAAO,EADpB,UAC4BpE,GAAM,GAAKqS,GAAMF,KAGtDmC,EAAUrM,KAAOkI,GAAS,YAG1B9S,GAAa,qEAAsE,SAAApB,UAAQ0Z,IAAkB1Z,EAAO,IAAMA,EAAO,mBAoBpHa,GAEZ,iBAAYN,EAAQE,QACdoe,GAAKnG,KACVnY,EAAOC,MAAQse,MACVve,OAASA,OACTE,QAAUA,OACVuQ,IAAMvQ,EAAUA,EAAQuQ,IAAM/P,QAC9B8d,IAAMte,EAAUA,EAAQwQ,UAAYC,IAyB9B8N,6BAmBZzG,MAAA,eAAM9Z,UACDA,GAAmB,IAAVA,QACPiG,QAAUoa,KAAKpa,OAAOmD,mBAAsBiX,KAAKG,UAAUH,KAAKzY,OAAS5H,EAAQqgB,KAAKrW,aACtFA,OAAShK,EACPqgB,MAEDA,KAAKrW,WAGbxB,SAAA,kBAASxI,UACDygB,UAAUve,OAASme,KAAKxX,cAA6B,EAAfwX,KAAKhY,QAAcrI,GAASA,EAAQqgB,KAAK5X,SAAW4X,KAAKhY,QAAUrI,GAASqgB,KAAKxX,iBAAmBwX,KAAK1Y,SAGvJkB,cAAA,uBAAc7I,UACRygB,UAAUve,aAGV2F,OAAS,EACPqD,GAAamV,KAAMA,KAAKhY,QAAU,EAAIrI,GAASA,EAASqgB,KAAKhY,QAAUgY,KAAK5X,UAAa4X,KAAKhY,QAAU,KAHvGgY,KAAKvX,UAMdf,UAAA,mBAAUA,EAAWpD,MACpBiO,MACK6N,UAAUve,cACPme,KAAK9X,WAETtC,EAASoa,KAAK7Z,OACdP,GAAUA,EAAOmD,mBAAqBiX,KAAKzX,IAAK,KACnDO,GAAekX,KAAMtY,IACpB9B,EAAOO,KAAOP,EAAOA,QAAUqD,GAAerD,EAAQoa,MAEhDpa,GAAUA,EAAOA,QACnBA,EAAOA,OAAOoD,QAAUpD,EAAO2B,QAAwB,GAAd3B,EAAO2C,IAAW3C,EAAOsC,OAAStC,EAAO2C,KAAO3C,EAAO4C,gBAAkB5C,EAAOsC,SAAWtC,EAAO2C,MAC9I3C,EAAO8B,UAAU9B,EAAOsC,QAAQ,GAEjCtC,EAASA,EAAOA,QAEZoa,KAAKpa,QAAUoa,KAAK7Z,IAAIc,qBAAmC,EAAX+Y,KAAKzX,KAAWb,EAAYsY,KAAKvX,OAAWuX,KAAKzX,IAAM,GAAiB,EAAZb,IAAoBsY,KAAKvX,QAAUf,IACnJ6B,GAAeyW,KAAK7Z,IAAK6Z,KAAMA,KAAKzY,OAASyY,KAAKrW,eAG1CqW,KAAK9X,SAAWR,IAAesY,KAAK1Y,OAAShD,GAAoB0b,KAAKvb,UAAY7B,KAAK+F,IAAIqX,KAAK1W,UAAYT,IAAenB,IAAcsY,KAAKvb,WAAaub,KAAK7W,KAAO6W,KAAKK,mBAC1K9X,MAAQyX,KAAKM,OAAS5Y,GAG1BvD,GAAgB6b,KAAMtY,EAAWpD,IAIlC0b,SAGR3b,KAAA,cAAK1E,EAAO2E,UACJ8b,UAAUve,OAASme,KAAKtY,UAAW9E,KAAKyL,IAAI2R,KAAKxX,gBAAiB7I,EAAQoI,GAAsBiY,QAAUA,KAAK1Y,KAAO0Y,KAAK5X,WAAczI,EAAQqgB,KAAK1Y,KAAO,GAAIhD,GAAkB0b,KAAKhX,UAGhMkC,cAAA,uBAAcvL,EAAO2E,UACb8b,UAAUve,OAASme,KAAKtY,UAAWsY,KAAKxX,gBAAkB7I,EAAO2E,GAAkB0b,KAAKxX,gBAAkB5F,KAAKyL,IAAI,EAAG2R,KAAK9X,OAAS8X,KAAKvX,OAA2B,GAAlBuX,KAAK5W,WAAkB4W,KAAKvb,SAAW,EAAI,MAGrMwM,SAAA,kBAAStR,EAAO2E,UACR8b,UAAUve,OAASme,KAAKtY,UAAWsY,KAAK7X,aAAc6X,KAAKzJ,OAA8B,EAAnByJ,KAAKO,YAA+B5gB,EAAZ,EAAIA,GAAiBoI,GAAsBiY,MAAO1b,GAAmB0b,KAAK7X,WAAavF,KAAKyL,IAAI,EAAG2R,KAAKhX,MAAQgX,KAAK1Y,MAAyB,EAAjB0Y,KAAK5W,UAAgB,EAAI,MAG5PmX,UAAA,mBAAU5gB,EAAO2E,OACZuW,EAAgBmF,KAAK7X,WAAa6X,KAAK5X,eACpCgY,UAAUve,OAASme,KAAKtY,UAAUsY,KAAKhX,OAASrJ,EAAQ,GAAKkb,EAAevW,GAAkB0b,KAAKhY,QAAUC,GAAgB+X,KAAK9X,OAAQ2S,GAAiB,EAAI,MAcvKjR,UAAA,mBAAUjK,EAAO2E,OACX8b,UAAUve,cACPme,KAAKpX,QAAUC,EAAW,EAAImX,KAAKpX,QAEvCoX,KAAKpX,OAASjJ,SACVqgB,SAEJ3V,EAAQ2V,KAAKpa,QAAUoa,KAAKzX,IAAMF,GAAwB2X,KAAKpa,OAAOoD,MAAOgX,MAAQA,KAAK9X,mBAMzFU,MAAQjJ,GAAS,OACjB4I,IAAOyX,KAAKQ,KAAO7gB,KAAWkJ,EAAY,EAAImX,KAAKpX,UACnDlB,UAAU2B,IAAQzG,KAAK+F,IAAIqX,KAAKrW,QAASqW,KAAKvX,MAAO4B,IAA2B,IAAnB/F,GAClEoE,GAAQsX,MAtiCW,SAApBS,kBAAoBrc,WACfwB,EAASxB,EAAUwB,OAChBA,GAAUA,EAAOA,QACvBA,EAAO4B,OAAS,EAChB5B,EAAO4C,gBACP5C,EAASA,EAAOA,cAEVxB,EAgiCAqc,CAAkBT,UAG1BU,OAAA,gBAAO/gB,UACDygB,UAAUve,QAKXme,KAAKQ,MAAQ7gB,UACX6gB,IAAM7gB,SAEL2gB,OAASN,KAAK9X,QAAUtF,KAAKwL,KAAK4R,KAAKrW,OAAQqW,KAAK5W,gBACpDb,IAAMyX,KAAK7Y,KAAO,IAEvBoL,UACKhK,IAAMyX,KAAKpX,UAEXlB,UAAUsY,KAAKpa,SAAWoa,KAAKpa,OAAOmD,kBAAoBiX,KAAK5W,UAAY4W,KAAK9X,QAAU8X,KAAKM,OAA6B,IAApBN,KAAK/O,YAAqBrO,KAAK+F,IAAIqX,KAAK1W,UAAYT,IAAamX,KAAK9X,QAAUW,MAGxLmX,MAhBCA,KAAKQ,QAmBdL,UAAA,mBAAUxgB,MACLygB,UAAUve,OAAQ,MAChB0F,OAAS5H,MACViG,EAASoa,KAAKpa,QAAUoa,KAAK7Z,WACjCP,IAAWA,EAAOiE,OAAUmW,KAAKpa,QAAW2D,GAAe3D,EAAQoa,KAAMrgB,EAAQqgB,KAAKrW,QAC/EqW,YAEDA,KAAKzY,WAGb0T,QAAA,iBAAQ0F,UACAX,KAAKzY,QAAUvH,EAAY2gB,GAAkBX,KAAKxX,gBAAkBwX,KAAK7X,YAAcvF,KAAK+F,IAAIqX,KAAKzX,KAAO,OAGpHa,QAAA,iBAAQwX,OACHhb,EAASoa,KAAKpa,QAAUoa,KAAK7Z,WACzBP,EAAwBgb,KAAiBZ,KAAKzX,KAAQyX,KAAKhY,SAAWgY,KAAKhX,OAASgX,KAAK9U,gBAAkB,GAAO8U,KAAK9X,QAAU8X,KAAK1Y,KAAO0Y,KAAK5X,SAAY4X,KAAKzX,IAAoBF,GAAwBzC,EAAOwD,QAAQwX,GAAcZ,MAAnEA,KAAK9X,OAArK8X,KAAK9X,WAGvBP,OAAA,gBAAOyJ,YAAAA,IAAAA,EAAQqJ,QACVoG,EAAkBrc,SACtBA,EAAa4M,GACT4O,KAAKvb,UAAYub,KAAKtb,iBACpBwE,UAAY8W,KAAK9W,SAASvB,OAAOyJ,QACjC1J,WAAW,IAAM0J,EAAO9M,iBAEhB,gBAATyW,OAAqC,IAAhB3J,EAAOJ,MAAkBgP,KAAKhP,OACxDxM,EAAaqc,EACNb,SAGRc,WAAA,oBAAW1X,WACNhF,EAAY4b,KACf3b,EAAO+b,UAAUve,OAASuH,EAAUhF,EAAUgF,UACxChF,GACNC,EAAOD,EAAUmD,OAASlD,GAAQzB,KAAK+F,IAAIvE,EAAUmE,MAAQ,GAC7DnE,EAAYA,EAAU+B,WAEf6Z,KAAKpa,QAAUoa,KAAKe,KAAOf,KAAKe,KAAKD,WAAW1X,GAAW/E,MAGpE2G,OAAA,gBAAOrL,UACFygB,UAAUve,aACRmG,QAAUrI,IAAUqf,EAAAA,GAAY,EAAIrf,EAClCwL,GAAuB6U,QAEN,IAAlBA,KAAKhY,QAAiBgX,EAAAA,EAAWgB,KAAKhY,YAG9CgZ,YAAA,qBAAYrhB,MACPygB,UAAUve,OAAQ,KACjBwC,EAAO2b,KAAKhX,kBACXZ,QAAUzI,EACfwL,GAAuB6U,MAChB3b,EAAO2b,KAAK3b,KAAKA,GAAQ2b,YAE1BA,KAAK5X,YAGb6Y,KAAA,cAAKthB,UACAygB,UAAUve,aACR0U,MAAQ5W,EACNqgB,MAEDA,KAAKzJ,UAGb2K,KAAA,cAAK1X,EAAUlF,UACP0b,KAAKtY,UAAUgC,GAAesW,KAAMxW,GAAWxJ,EAAYsE,QAGnE6c,QAAA,iBAAQC,EAAc9c,eAChB+c,OAAO3Z,UAAU0Z,GAAgBpB,KAAKrW,OAAS,EAAG3J,EAAYsE,SAC9DgD,OAAS0Y,KAAK1W,QAAUT,GACtBmX,SAGRqB,KAAA,cAAKjU,EAAM9I,UACF,MAAR8I,GAAgB4S,KAAKkB,KAAK9T,EAAM9I,GACzB0b,KAAKsB,UAAS,GAAOZ,QAAO,OAGpCa,QAAA,iBAAQnU,EAAM9I,UACL,MAAR8I,GAAgB4S,KAAKkB,KAAK9T,GAAQ4S,KAAKxX,gBAAiBlE,GACjD0b,KAAKsB,UAAS,GAAMZ,QAAO,OAGnCc,MAAA,eAAMC,EAAQnd,UACH,MAAVmd,GAAkBzB,KAAKkB,KAAKO,EAAQnd,GAC7B0b,KAAKU,QAAO,OAGpBgB,OAAA,yBACQ1B,KAAKU,QAAO,OAGpBY,SAAA,kBAAS3hB,UACJygB,UAAUve,UACXlC,IAAUqgB,KAAKsB,YAActB,KAAKpW,WAAWoW,KAAKpX,OAASjJ,GAASkJ,EAAW,IAC1EmX,MAEDA,KAAKpX,KAAO,MAGpB+Y,WAAA,kCACMld,SAAWub,KAAK7Y,KAAO,OACvBmC,QAAUT,EACRmX,SAGR4B,SAAA,wBAGExY,EAFGxD,EAASoa,KAAKpa,QAAUoa,KAAK7Z,IAChCnD,EAAQgd,KAAKzY,eAEH3B,KAAWoa,KAAKzX,KAAOyX,KAAKvb,UAAYmB,EAAOgc,aAAexY,EAAUxD,EAAOwD,SAAQ,KAAUpG,GAASoG,EAAU4W,KAAK/E,SAAQ,GAAQpS,QAGrJgZ,cAAA,uBAAcvW,EAAM+Q,EAAU9Q,OACzB5F,EAAOqa,KAAKra,YACO,EAAnBya,UAAUve,QACRwa,GAGJ1W,EAAK2F,GAAQ+Q,EACb9Q,IAAW5F,EAAK2F,EAAO,UAAYC,GAC1B,aAATD,IAAwB0U,KAAK8B,UAAYzF,WAJlC1W,EAAK2F,GAMN0U,MAEDra,EAAK2F,OAGbyW,KAAA,cAAKC,OACAC,EAAOjC,YACJ,IAAIkC,QAAQ,SAAAC,GAEN,SAAXC,SACKC,EAAQJ,EAAKF,KACjBE,EAAKF,KAAO,KACZniB,EAAY6e,KAAOA,EAAIA,EAAEwD,MAAWxD,EAAEsD,MAAQtD,IAAMwD,KAAUA,EAAKF,KAAOM,GAC1EF,EAAQ1D,GACRwD,EAAKF,KAAOM,MANV5D,EAAI7e,EAAYoiB,GAAeA,EAAchd,GAQ7Cid,EAAKxd,UAAsC,IAAzBwd,EAAK/W,iBAAqC,GAAZ+W,EAAK1Z,MAAe0Z,EAAK/Z,QAAU+Z,EAAK1Z,IAAM,EACjG6Z,KAEAH,EAAKK,MAAQF,SAKhBpR,KAAA,gBACCF,GAAWkP,qCAlSAra,QACNA,KAAOA,OACPgE,QAAUhE,EAAK8T,OAAS,GACxBuG,KAAKhY,QAAUrC,EAAKqF,SAAWgU,EAAAA,GAAY,EAAIrZ,EAAKqF,QAAU,UAC7D5C,QAAUzC,EAAKqb,aAAe,OAC9BzK,QAAU5Q,EAAKsb,QAAUtb,EAAK2Q,eAE/B/N,IAAM,EACXsC,GAAamV,MAAOra,EAAKwC,SAAU,EAAG,QACjC4S,KAAOpV,EAAKoV,KACblD,SACE2E,KAAO3E,GACHkD,KAAKnQ,KAAKoV,MAEpB/H,GAAiBvN,GAAQwT,OAyR3BhZ,GAAagb,GAAUzN,UAAW,CAACzJ,MAAM,EAAGzB,OAAO,EAAGF,KAAK,EAAGa,OAAO,EAAGO,MAAM,EAAGjB,OAAO,EAAGQ,QAAQ,EAAGuO,OAAM,EAAO3Q,OAAO,KAAMnB,UAAS,EAAO2D,QAAQ,EAAGG,IAAI,EAAGpC,IAAI,EAAGoc,MAAM,EAAGjZ,QAAQT,EAAUyZ,MAAM,EAAG9B,KAAI,EAAO5X,KAAK,QAyBhNwC,iCAEAzF,EAAW6D,yBAAX7D,IAAAA,EAAO,mBACZA,UACDkL,OAAS,KACT9H,oBAAsBpD,EAAKoD,oBAC3B9B,qBAAuBtB,EAAKsB,qBAC5B4C,MAAQ7J,EAAY2F,EAAK6c,cAC9B3c,GAAmB0D,GAAe5D,EAAKC,QAAUC,4BAAuB2D,GACxE7D,EAAK2b,UAAYmB,EAAKlB,UACtB5b,EAAK+a,QAAU+B,EAAK/B,QAAO,GAC3B/a,EAAKoL,eAAiB/G,6BAAqBrE,EAAKoL,8EAGjD2R,GAAA,YAAGphB,EAASqE,EAAM6D,UACjB6B,GAAiB,EAAG+U,UAAWJ,MACxBA,QAGR5S,KAAA,cAAK9L,EAASqE,EAAM6D,UACnB6B,GAAiB,EAAG+U,UAAWJ,MACxBA,QAGR2C,OAAA,gBAAOrhB,EAASshB,EAAUC,EAAQrZ,UACjC6B,GAAiB,EAAG+U,UAAWJ,MACxBA,QAGRC,IAAA,aAAI3e,EAASqE,EAAM6D,UAClB7D,EAAKwC,SAAW,EAChBxC,EAAKC,OAASoa,KACdta,GAAiBC,GAAMqb,cAAgBrb,EAAKqF,OAAS,GACrDrF,EAAKkC,kBAAoBlC,EAAKkC,oBAC1BgE,GAAMvK,EAASqE,EAAM+D,GAAesW,KAAMxW,GAAW,GAClDwW,QAGRpE,KAAA,cAAKS,EAAU9Q,EAAQ/B,UACfD,GAAeyW,KAAMnU,GAAMiX,YAAY,EAAGzG,EAAU9Q,GAAS/B,MAIrEuZ,UAAA,mBAAUzhB,EAAS6G,EAAUxC,EAAMqd,EAASxZ,EAAUyZ,EAAeC,UACpEvd,EAAKwC,SAAWA,EAChBxC,EAAKqd,QAAUrd,EAAKqd,SAAWA,EAC/Brd,EAAKwd,WAAaF,EAClBtd,EAAKyd,iBAAmBF,EACxBvd,EAAKC,OAASoa,SACVnU,GAAMvK,EAASqE,EAAM+D,GAAesW,KAAMxW,IACvCwW,QAGRqD,YAAA,qBAAY/hB,EAAS6G,EAAUxC,EAAMqd,EAASxZ,EAAUyZ,EAAeC,UACtEvd,EAAKgG,aAAe,EACpBjG,GAAiBC,GAAMkC,gBAAkB7H,EAAY2F,EAAKkC,iBACnDmY,KAAK+C,UAAUzhB,EAAS6G,EAAUxC,EAAMqd,EAASxZ,EAAUyZ,EAAeC,MAGlFI,cAAA,uBAAchiB,EAAS6G,EAAUya,EAAUC,EAAQG,EAASxZ,EAAUyZ,EAAeC,UACpFL,EAAOjX,QAAUgX,EACjBld,GAAiBmd,GAAQhb,gBAAkB7H,EAAY6iB,EAAOhb,iBACvDmY,KAAK+C,UAAUzhB,EAAS6G,EAAU0a,EAAQG,EAASxZ,EAAUyZ,EAAeC,MAGpFhf,OAAA,gBAAOwD,EAAWpD,EAAgBC,OAMhCF,EAAMgC,EAAOS,EAAMyZ,EAAW1F,EAAe0I,EAAYC,EAAY5Z,EAAW6Z,EAAWC,EAAezC,EAAM7K,EAL7GuN,EAAW3D,KAAKhX,MACnB4a,EAAO5D,KAAKxY,OAASwY,KAAKxX,gBAAkBwX,KAAKvX,MACjDwC,EAAM+U,KAAK1Y,KACX+C,EAAQ3C,GAAa,EAAI,EAAI5E,GAAc4E,GAC3Cmc,EAAiB7D,KAAK1W,OAAS,GAAQ5B,EAAY,IAAOsY,KAAKvb,WAAawG,aAEpEpF,GAA2B+d,EAARvZ,GAA6B,GAAb3C,IAAmB2C,EAAQuZ,GACnEvZ,IAAU2V,KAAK9X,QAAU3D,GAASsf,EAAe,IAChDF,IAAa3D,KAAKhX,OAASiC,IAC9BZ,GAAS2V,KAAKhX,MAAQ2a,EACtBjc,GAAasY,KAAKhX,MAAQ2a,GAE3Btf,EAAOgG,EACPoZ,EAAYzD,KAAKzY,OAEjBgc,IADA3Z,EAAYoW,KAAKzX,KAEbsb,IACH5Y,IAAQ0Y,EAAW3D,KAAK1W,SAEvB5B,GAAcpD,IAAoB0b,KAAK1W,OAAS5B,IAE9CsY,KAAKhY,QAAS,IACjBiZ,EAAOjB,KAAKzJ,MACZsE,EAAgB5P,EAAM+U,KAAK5X,QACvB4X,KAAKhY,SAAW,GAAKN,EAAY,SAC7BsY,KAAKtY,UAA0B,IAAhBmT,EAAsBnT,EAAWpD,EAAgBC,MAExEF,EAAOvB,GAAcuH,EAAQwQ,GACzBxQ,IAAUuZ,GACbrD,EAAYP,KAAKhY,QACjB3D,EAAO4G,KAGPsV,KADAmD,EAAgB5gB,GAAcuH,EAAQwQ,MAErB0F,IAAcmD,IAC9Brf,EAAO4G,EACPsV,KAEMtV,EAAP5G,IAAeA,EAAO4G,IAEvByY,EAAgBzb,GAAgB+X,KAAK9X,OAAQ2S,IAC5C8I,GAAY3D,KAAK9X,QAAUwb,IAAkBnD,GAAaP,KAAK9X,OAASwb,EAAgB7I,EAAgBmF,KAAK1Y,MAAQ,IAAMoc,EAAgBnD,GACxIU,GAAqB,EAAZV,IACZlc,EAAO4G,EAAM5G,EACb+R,EAAS,GAUNmK,IAAcmD,IAAkB1D,KAAK8D,MAAO,KAC3CC,EAAa9C,GAAyB,EAAhByC,EACzBM,EAAYD,KAAe9C,GAAqB,EAAZV,MACrCA,EAAYmD,IAAkBK,GAAaA,GAC3CJ,EAAWI,EAAY,EAAI1Z,EAAQY,EAAMA,EAAMZ,OAC1CyZ,MAAQ,OACR5f,OAAOyf,IAAavN,EAAS,EAAItT,GAAcyd,EAAY1F,IAAiBvW,GAAiB2G,GAAK6Y,MAAQ,OAC1G5b,OAASmC,GACb/F,GAAkB0b,KAAKpa,QAAUsL,GAAU8O,KAAM,iBAC7Cra,KAAKse,gBAAkB7N,IAAW4J,KAAK2B,aAAamC,MAAQ,GAC5DH,GAAYA,IAAa3D,KAAKhX,OAAUua,IAAgBvD,KAAKzX,KAAQyX,KAAKra,KAAKue,WAAalE,KAAKpa,SAAWoa,KAAK7Y,YAC9G6Y,QAER/U,EAAM+U,KAAK1Y,KACXsc,EAAO5D,KAAKvX,MACRub,SACEF,MAAQ,EACbH,EAAWI,EAAY9Y,GAAO,UACzB/G,OAAOyf,GAAU,QACjBhe,KAAKse,gBAAkB7N,GAAU4J,KAAK2B,mBAEvCmC,MAAQ,GACR9D,KAAKzX,MAAQgb,SACVvD,KAGR7J,GAAmB6J,KAAM5J,OAGvB4J,KAAKmE,YAAcnE,KAAKoE,UAAYpE,KAAK8D,MAAQ,IACpDN,EA3wCmB,SAAtBa,oBAAuBjgB,EAAWuf,EAAUtf,OACvCgC,KACOsd,EAAPtf,MACHgC,EAAQjC,EAAUiS,OACXhQ,GAASA,EAAMkB,QAAUlD,GAAM,IAClB,YAAfgC,EAAM0U,MAAsB1U,EAAMkB,OAASoc,SACvCtd,EAERA,EAAQA,EAAMO,eAGfP,EAAQjC,EAAUkgB,MACXje,GAASA,EAAMkB,QAAUlD,GAAM,IAClB,YAAfgC,EAAM0U,MAAsB1U,EAAMkB,OAASoc,SACvCtd,EAERA,EAAQA,EAAMM,OA2vCD0d,CAAoBrE,KAAMld,GAAc6gB,GAAW7gB,GAAcuB,OAE7EgG,GAAShG,GAAQA,EAAOmf,EAAWjc,cAIhCW,OAASmC,OACTrB,MAAQ3E,OACR8C,MAAQyC,EAERoW,KAAKvb,gBACJqd,UAAY9B,KAAKra,KAAK4e,cACtB9f,SAAW,OACX6E,OAAS5B,EACdic,EAAW,IAEPA,GAAYtf,IAASC,IAAmBic,IAC5CrP,GAAU8O,KAAM,WACZA,KAAK9X,SAAWmC,UACZ2V,QAGG2D,GAARtf,GAAiC,GAAbqD,MACvBrB,EAAQ2Z,KAAK3J,OACNhQ,GAAO,IACbS,EAAOT,EAAMO,OACRP,EAAMc,MAAQ9C,GAAQgC,EAAMkB,SAAWlB,EAAMkC,KAAOib,IAAend,EAAO,IAC1EA,EAAMT,SAAWoa,YACbA,KAAK9b,OAAOwD,EAAWpD,EAAgBC,MAE/C8B,EAAMnC,OAAmB,EAAZmC,EAAMkC,KAAWlE,EAAOgC,EAAMkB,QAAUlB,EAAMkC,KAAOlC,EAAMmB,OAASnB,EAAMmC,gBAAkBnC,EAAMoC,QAAUpE,EAAOgC,EAAMkB,QAAUlB,EAAMkC,IAAKjE,EAAgBC,GACvKF,IAAS2b,KAAKhX,QAAWgX,KAAKzX,MAAQgb,EAAa,CACtDC,EAAa,EACb1c,IAASuD,GAAU2V,KAAK1W,QAAUT,UAIpCxC,EAAQS,MAEH,CACNT,EAAQ2Z,KAAKsE,cACTE,EAAe9c,EAAY,EAAIA,EAAYrD,EACxCgC,GAAO,IACbS,EAAOT,EAAMM,OACRN,EAAMc,MAAQqd,GAAgBne,EAAMgB,OAAShB,EAAMkC,KAAOib,IAAend,EAAO,IAChFA,EAAMT,SAAWoa,YACbA,KAAK9b,OAAOwD,EAAWpD,EAAgBC,MAE/C8B,EAAMnC,OAAmB,EAAZmC,EAAMkC,KAAWic,EAAene,EAAMkB,QAAUlB,EAAMkC,KAAOlC,EAAMmB,OAASnB,EAAMmC,gBAAkBnC,EAAMoC,QAAU+b,EAAene,EAAMkB,QAAUlB,EAAMkC,IAAKjE,EAAgBC,GAAUC,IAAe6B,EAAM5B,UAAY4B,EAAM3B,WACxOL,IAAS2b,KAAKhX,QAAWgX,KAAKzX,MAAQgb,EAAa,CACtDC,EAAa,EACb1c,IAASuD,GAAU2V,KAAK1W,OAASkb,GAAgB3b,EAAWA,UAI9DxC,EAAQS,MAGN0c,IAAelf,SACbkd,QACLgC,EAAWtf,OAAeyf,GAARtf,EAAmB,GAAKwE,GAAUS,OAAiBqa,GAARtf,EAAmB,GAAK,EACjF2b,KAAKzX,iBACHhB,OAASkc,EACd/a,GAAQsX,MACDA,KAAK9b,OAAOwD,EAAWpD,EAAgBC,QAG3Cud,YAAcxd,GAAkB4M,GAAU8O,KAAM,YAAY,IAC5D3V,IAAUuZ,GAAQ5D,KAAK9X,QAAU8X,KAAKxX,kBAAsB6B,GAASsZ,KAAeF,IAAczD,KAAKzY,QAAU3E,KAAK+F,IAAIiB,KAAehH,KAAK+F,IAAIqX,KAAKzX,MAAWyX,KAAK8D,SAC1Kpc,GAAcuD,KAAUZ,IAAUuZ,GAAmB,EAAX5D,KAAKzX,MAAc8B,GAAS2V,KAAKzX,IAAM,IAAOxB,GAAkBiZ,KAAM,GAC5G1b,GAAoBoD,EAAY,IAAMic,IAActZ,IAASsZ,GAAaC,IAC9E1S,GAAU8O,KAAO3V,IAAUuZ,GAAqB,GAAblc,EAAiB,aAAe,qBAAsB,SACpF4a,OAAWjY,EAAQuZ,GAA2B,EAAnB5D,KAAKpW,aAAoBoW,KAAKsC,kBAI1DtC,QAGR7W,IAAA,aAAI9C,EAAOmD,iBACV3J,EAAU2J,KAAcA,EAAWE,GAAesW,KAAMxW,EAAUnD,MAC5DA,aAAiB6Z,IAAY,IAC9Bja,EAASI,UACZA,EAAM3D,QAAQ,SAAAvB,UAAOsjB,EAAKtb,IAAIhI,EAAKqI,KAC5BwW,QAEJtgB,EAAU2G,UACN2Z,KAAK0E,SAASre,EAAOmD,OAEzB5J,EAAYyG,UAGR2Z,KAFP3Z,EAAQwF,GAAMiX,YAAY,EAAGzc,UAKxB2Z,OAAS3Z,EAAQkD,GAAeyW,KAAM3Z,EAAOmD,GAAYwW,QAGjE2E,YAAA,qBAAY5O,EAAe6O,EAAeC,EAAkBC,YAAhD/O,IAAAA,GAAS,YAAM6O,IAAAA,GAAS,YAAMC,IAAAA,GAAY,YAAMC,IAAAA,GAAoBrW,WAC3E3K,EAAI,GACPuC,EAAQ2Z,KAAK3J,OACPhQ,GACFA,EAAMkB,QAAUud,IACfze,aAAiBwF,GACpB+Y,GAAU9gB,EAAE8G,KAAKvE,IAEjBwe,GAAa/gB,EAAE8G,KAAKvE,GACpB0P,GAAUjS,EAAE8G,WAAF9G,EAAUuC,EAAMse,aAAY,EAAMC,EAAQC,MAGtDxe,EAAQA,EAAMO,aAER9C,KAGRihB,QAAA,iBAAQhF,WACHiF,EAAahF,KAAK2E,YAAY,EAAG,EAAG,GACvCnjB,EAAIwjB,EAAWnjB,OACVL,QACDwjB,EAAWxjB,GAAGmE,KAAKoa,KAAOA,SACtBiF,EAAWxjB,MAKrB0F,OAAA,gBAAOb,UACF3G,EAAU2G,GACN2Z,KAAKiF,YAAY5e,GAErBzG,EAAYyG,GACR2Z,KAAKkF,aAAa7e,IAE1BA,EAAMT,SAAWoa,MAAQnZ,GAAsBmZ,KAAM3Z,GACjDA,IAAU2Z,KAAKjW,eACbA,QAAUiW,KAAKsE,OAEdld,GAAS4Y,UAGjBtY,UAAA,mBAAUA,EAAWpD,UACf8b,UAAUve,aAGVuiB,SAAW,GACXpE,KAAK7Z,KAAO6Z,KAAKzX,WAChBhB,OAASzE,GAAc4H,GAAQrG,MAAmB,EAAX2b,KAAKzX,IAAUb,EAAYsY,KAAKzX,KAAOyX,KAAKxX,gBAAkBd,IAAcsY,KAAKzX,mBAExHb,oBAAUA,EAAWpD,QACtB8f,SAAW,EACTpE,MARCA,KAAK9X,UAWdwc,SAAA,kBAAS9T,EAAOpH,eACVqH,OAAOD,GAASlH,GAAesW,KAAMxW,GACnCwW,QAGRiF,YAAA,qBAAYrU,iBACJoP,KAAKnP,OAAOD,GACZoP,QAGRmF,SAAA,kBAAS3b,EAAU6S,EAAU9Q,OACxB9E,EAAIoF,GAAMiX,YAAY,EAAGzG,GAAYjb,EAAYmK,UACrD9E,EAAEsU,KAAO,eACJoJ,UAAY,EACV5a,GAAeyW,KAAMvZ,EAAGiD,GAAesW,KAAMxW,OAGrD4b,YAAA,qBAAY5b,OACPnD,EAAQ2Z,KAAK3J,WACjB7M,EAAWE,GAAesW,KAAMxW,GACzBnD,GACFA,EAAMkB,SAAWiC,GAA2B,YAAfnD,EAAM0U,MACtChU,GAAkBV,GAEnBA,EAAQA,EAAMO,SAIhBse,aAAA,sBAAa5jB,EAAS+jB,EAAOC,WACxBV,EAAS5E,KAAKuF,YAAYjkB,EAASgkB,GACtC9jB,EAAIojB,EAAO/iB,OACLL,KACLgkB,KAAsBZ,EAAOpjB,IAAOojB,EAAOpjB,GAAGwP,KAAK1P,EAAS+jB,UAEvDrF,QAGRuF,YAAA,qBAAYjkB,EAASgkB,WAKnBG,EAJG3hB,EAAI,GACP4hB,EAAgBxjB,GAAQZ,GACxB+E,EAAQ2Z,KAAK3J,OACbsP,EAAe9lB,EAAUylB,GAEnBjf,GACFA,aAAiBwF,GAChBvI,GAAkB+C,EAAMuf,SAAUF,KAAmBC,IAAiBH,IAAsBnf,EAAM5B,UAAY4B,EAAMkC,MAASlC,EAAMya,WAAW,IAAMwE,GAAcjf,EAAMya,WAAWza,EAAMmC,iBAAmB8c,GAAcA,GAAcjf,EAAMub,aACjP9d,EAAE8G,KAAKvE,IAEGof,EAAWpf,EAAMkf,YAAYG,EAAeJ,IAAazjB,QACpEiC,EAAE8G,WAAF9G,EAAU2hB,GAEXpf,EAAQA,EAAMO,aAER9C,KAUR+hB,QAAA,iBAAQrc,EAAU7D,GACjBA,EAAOA,GAAQ,OAIdmgB,EAHGC,EAAK/F,KACR/E,EAAUvR,GAAeqc,EAAIvc,GAC3BoC,EAAqDjG,EAArDiG,QAASoa,EAA4CrgB,EAA5CqgB,QAASC,EAAmCtgB,EAAnCsgB,cAAepe,EAAoBlC,EAApBkC,gBAEnCjE,EAAQiI,GAAM6W,GAAGqD,EAAI7gB,GAAa,CACjCgI,KAAMvH,EAAKuH,MAAQ,OACnB1C,MAAM,EACN3C,iBAAiB,EACjBxD,KAAM4W,EACNzB,UAAW,OACXrR,SAAUxC,EAAKwC,UAAavF,KAAK+F,KAAKsS,GAAYrP,GAAW,SAAUA,EAAWA,EAAQvH,KAAO0hB,EAAG/c,QAAU+c,EAAGnc,cAAiBf,EAClImd,QAAS,sBACRD,EAAGvE,SACEsE,EAAS,KACT3d,EAAWxC,EAAKwC,UAAYvF,KAAK+F,KAAKsS,GAAYrP,GAAW,SAAUA,EAAWA,EAAQvH,KAAO0hB,EAAG/c,QAAU+c,EAAGnc,aACpHhG,EAAM0D,OAASa,GAAa0C,GAAajH,EAAOuE,EAAU,EAAG,GAAGjE,OAAON,EAAMoF,OAAO,GAAM,GAC3F8c,EAAU,EAEXE,GAAWA,EAAQ3Q,MAAMzR,EAAOqiB,GAAiB,MAEhDtgB,WACGkC,EAAkBjE,EAAMM,OAAO,GAAKN,KAG5CsiB,YAAA,qBAAYC,EAAcC,EAAYzgB,UAC9Bqa,KAAK6F,QAAQO,EAAYlhB,GAAa,CAAC0G,QAAQ,CAACvH,KAAKqF,GAAesW,KAAMmG,KAAiBxgB,OAGnG0V,OAAA,yBACQ2E,KAAKjW,WAGbsc,UAAA,mBAAUC,mBAAAA,IAAAA,EAAYtG,KAAKhX,OACnBwH,GAAqBwP,KAAMtW,GAAesW,KAAMsG,OAGxDC,cAAA,uBAAcC,mBAAAA,IAAAA,EAAaxG,KAAKhX,OACxBwH,GAAqBwP,KAAMtW,GAAesW,KAAMwG,GAAa,MAGrEC,aAAA,sBAAa9mB,UACLygB,UAAUve,OAASme,KAAKkB,KAAKvhB,GAAO,GAAQqgB,KAAKuG,cAAcvG,KAAKhX,MAAQH,MAGpF6d,cAAA,uBAAc7X,EAAQ8X,EAAc7B,YAAAA,IAAAA,EAAmB,WAGrD7f,EAFGoB,EAAQ2Z,KAAK3J,OAChBxF,EAASmP,KAAKnP,OAERxK,GACFA,EAAMkB,QAAUud,IACnBze,EAAMkB,QAAUsH,EAChBxI,EAAMgB,MAAQwH,GAEfxI,EAAQA,EAAMO,SAEX+f,MACE1hB,KAAK4L,EACLA,EAAO5L,IAAM6f,IAChBjU,EAAO5L,IAAM4J,UAITzH,GAAS4Y,SAGjB2B,WAAA,oBAAWiF,OACNvgB,EAAQ2Z,KAAK3J,gBACZyN,MAAQ,EACNzd,GACNA,EAAMsb,WAAWiF,GACjBvgB,EAAQA,EAAMO,yBAEF+a,qBAAWiF,MAGzBC,MAAA,eAAMC,YAAAA,IAAAA,GAAgB,WAEpBhgB,EADGT,EAAQ2Z,KAAK3J,OAEVhQ,GACNS,EAAOT,EAAMO,WACRM,OAAOb,GACZA,EAAQS,cAEJX,MAAQ6Z,KAAKhX,MAAQgX,KAAK9X,OAAS8X,KAAKM,OAAS,GACtDwG,IAAkB9G,KAAKnP,OAAS,IACzBzJ,GAAS4Y,SAGjBxX,cAAA,uBAAc7I,OAKZ+G,EAAM1D,EAAO4C,EAJVwI,EAAM,EACT6T,EAAOjC,KACP3Z,EAAQ4b,EAAKqC,MACbb,EAAYhV,KAET2R,UAAUve,cACNogB,EAAKrY,WAAWqY,EAAKja,QAAU,EAAIia,EAAK9Z,WAAa8Z,EAAKzZ,kBAAoByZ,EAAKX,YAAc3hB,EAAQA,OAE7GsiB,EAAKza,OAAQ,KAChB5B,EAASqc,EAAKrc,OACPS,GACNK,EAAOL,EAAMM,MACbN,EAAMmB,QAAUnB,EAAMmC,gBAEVib,GADZzgB,EAAQqD,EAAMkB,SACW0a,EAAKpY,OAASxD,EAAMkC,MAAQ0Z,EAAK6B,OACzD7B,EAAK6B,MAAQ,EACbva,GAAe0Y,EAAM5b,EAAOrD,EAAQqD,EAAMsD,OAAQ,GAAGma,MAAQ,GAE7DL,EAAYzgB,EAETA,EAAQ,GAAKqD,EAAMkC,MACtB6F,GAAOpL,IACD4C,IAAWqc,EAAK9b,KAASP,GAAUA,EAAOmD,qBAC/CkZ,EAAK1a,QAAUvE,EAAQif,EAAK1Z,IAC5B0Z,EAAKjZ,OAAShG,EACdif,EAAK/Z,QAAUlF,GAEhBif,EAAKyE,eAAe1jB,GAAO,GAAQ,UACnCygB,EAAY,GAEbpd,EAAMgB,KAAO+G,GAAO/H,EAAMkC,MAAQ6F,EAAM/H,EAAMgB,MAC9ChB,EAAQK,EAETmE,GAAaoX,EAAOA,IAASpc,GAAmBoc,EAAKjZ,MAAQoF,EAAO6T,EAAKjZ,MAAQoF,EAAK,EAAG,GACzF6T,EAAKza,OAAS,SAERya,EAAKxZ,gBAGNse,WAAP,oBAAkB1iB,MACbwB,EAAgB0C,MACnBpE,GAAgB0B,EAAiBwC,GAAwBhE,EAAMwB,IAC/D4E,EAAqBC,GAAQC,OAE1BD,GAAQC,OAASgQ,GAAc,CAClCA,IAAgB1B,EAAQC,WAAa,QACjC7S,EAAQR,EAAgBwQ,YACvBhQ,IAAUA,EAAMkC,MAAS0Q,EAAQC,WAAaxO,GAAQqO,WAAWlX,OAAS,EAAG,MAC1EwE,IAAUA,EAAMkC,KACtBlC,EAAQA,EAAMO,MAEfP,GAASqE,GAAQ8T,qBA3fS0B,IAkgB9Bhb,GAAakG,GAASqH,UAAW,CAACqR,MAAM,EAAGK,UAAU,EAAGC,SAAS,IA8GjD,SAAf4C,GAAgBrmB,EAAUgF,EAAM/B,EAAOuM,EAAO1O,EAAQH,OACjD2lB,EAAQC,EAAIC,EAAU3lB,KACtBgR,GAAS7R,KAAwL,KAA1KsmB,EAAS,IAAIzU,GAAS7R,IAAa6Q,KAAK/P,EAAQwlB,EAAOjV,QAAUrM,EAAKhF,GAdnF,SAAfymB,aAAgBzhB,EAAMwK,EAAO1O,EAAQH,EAASsC,MAC7ChE,EAAY+F,KAAUA,EAAO0hB,GAAmB1hB,EAAM/B,EAAOuM,EAAO1O,EAAQH,KACvEvB,EAAU4F,IAAUA,EAAK2hB,OAAS3hB,EAAKyG,UAAanG,EAASN,IAASsU,EAActU,UACjFjG,EAAUiG,GAAQ0hB,GAAmB1hB,EAAM/B,EAAOuM,EAAO1O,EAAQH,GAAWqE,MAGnFV,EADGQ,EAAO,OAENR,KAAKU,EACTF,EAAKR,GAAKoiB,GAAmB1hB,EAAKV,GAAIrB,EAAOuM,EAAO1O,EAAQH,UAEtDmE,EAIsG2hB,CAAazhB,EAAKhF,GAAWwP,EAAO1O,EAAQH,EAASsC,GAAQA,EAAOuM,EAAO7O,KACvLsC,EAAM2G,IAAM2c,EAAK,IAAIrU,GAAUjP,EAAM2G,IAAK9I,EAAQd,EAAU,EAAG,EAAGsmB,EAAO/iB,OAAQ+iB,EAAQ,EAAGA,EAAOM,UAC/F3jB,IAAUoU,OACbmP,EAAWvjB,EAAMyc,UAAUzc,EAAMgiB,SAASliB,QAAQjC,IAClDD,EAAIylB,EAAOxV,OAAO5P,OACXL,KACN2lB,EAASF,EAAOxV,OAAOjQ,IAAM0lB,SAIzBD,EAsKS,SAAjBO,GAAkB9U,EAAMvR,EAAKsmB,EAAUC,OAErCziB,EAAGnB,EADAoJ,EAAO/L,EAAI+L,MAAQwa,GAAY,kBAE/BzhB,EAAS9E,GACZ2C,EAAI2jB,EAAS/U,KAAU+U,EAAS/U,GAAQ,IAExCvR,EAAIuB,QAAQ,SAAC/C,EAAO6B,UAAMsC,EAAE8G,KAAK,CAACnE,EAAGjF,GAAKL,EAAIU,OAAS,GAAK,IAAKO,EAAGzC,EAAOgoB,EAAGza,eAEzEjI,KAAK9D,EACT2C,EAAI2jB,EAASxiB,KAAOwiB,EAASxiB,GAAK,IAC5B,SAANA,GAAgBnB,EAAE8G,KAAK,CAACnE,EAAGrD,WAAWsP,GAAOtQ,EAAGjB,EAAI8D,GAAI0iB,EAAGza,IArR/D,IAuGCsY,GACAoC,GAxDAhW,GAAgB,SAAhBA,cAAyBnQ,EAAQiR,EAAM1P,EAAOG,EAAKgN,EAAO7O,EAASwQ,EAAU+V,EAAcC,EAAWC,GACrGnoB,EAAYuD,KAASA,EAAMA,EAAIgN,GAAS,EAAG1O,EAAQH,QAIlD4lB,EAHGc,EAAevmB,EAAOiR,GACzBuV,EAAyB,QAAVjlB,EAAmBA,EAASpD,EAAYooB,GAAgCF,EAAYrmB,EAAQiR,EAAKhP,QAAQ,SAAW9D,EAAY6B,EAAO,MAAQiR,EAAKrP,OAAO,KAAQqP,EAAO,MAAQA,EAAKrP,OAAO,IAAIykB,GAAarmB,EAAOiR,KAA9JsV,EACvEE,EAAUtoB,EAAYooB,GAA+BF,EAAYK,GAAuBC,GAAlDC,MAEnC3oB,EAAUyD,MACRA,EAAIO,QAAQ,aAChBP,EAAMiN,GAAejN,IAEA,MAAlBA,EAAID,OAAO,OACdgkB,EAAKnkB,GAAeklB,EAAa9kB,IAAQ4I,GAAQkc,IAAgB,KAChD,IAAPf,IACT/jB,EAAM+jB,MAIJa,GAAYE,IAAgB9kB,GAAOykB,UAClCpa,MAAMya,EAAc9kB,IAAgB,KAARA,GAMhC6kB,GAAkBtV,KAAQjR,GAAWf,EAAegS,EAAMvP,GAxE7B,SAA7BmlB,2BAAsC7mB,EAAQiR,EAAM1P,EAAOG,EAAK+kB,EAAQL,EAAcC,OAIvFtT,EAAQ+T,EAAW7T,EAAO8T,EAAQC,EAAOC,EAAUC,EAAW7kB,EAH3DojB,EAAK,IAAIrU,GAAUmN,KAAKzV,IAAK9I,EAAQiR,EAAM,EAAG,EAAGkW,GAAsB,KAAMV,GAChF/X,EAAQ,EACR0Y,EAAa,MAEd3B,EAAGpY,EAAI9L,EACPkkB,EAAGS,EAAIxkB,EACPH,GAAS,IAEJ2lB,IADLxlB,GAAO,IACeO,QAAQ,cAC7BP,EAAMiN,GAAejN,IAElB0kB,IAEHA,EADA/jB,EAAI,CAACd,EAAOG,GACI1B,EAAQiR,GACxB1P,EAAQc,EAAE,GACVX,EAAMW,EAAE,IAETykB,EAAYvlB,EAAM6B,MAAMwV,KAAyB,GACzC7F,EAAS6F,GAAqBpO,KAAK9I,IAC1CqlB,EAAShU,EAAO,GAChBiU,EAAQtlB,EAAI6S,UAAU7F,EAAOqE,EAAOrE,OAChCuE,EACHA,GAASA,EAAQ,GAAK,EACS,UAArB+T,EAAMplB,QAAQ,KACxBqR,EAAQ,GAEL8T,IAAWD,EAAUM,OACxBH,EAAWtlB,WAAWmlB,EAAUM,EAAW,KAAO,EAElD3B,EAAG3c,IAAM,CACR3D,MAAOsgB,EAAG3c,IACVtF,EAAIwjB,GAAwB,IAAfI,EAAoBJ,EAAQ,IACzCnY,EAAGoY,EACHxU,EAAwB,MAArBsU,EAAOtlB,OAAO,GAAaH,GAAe2lB,EAAUF,GAAUE,EAAWtlB,WAAWolB,GAAUE,EACjGI,EAAIpU,GAASA,EAAQ,EAAK9R,KAAKC,MAAQ,GAExCsN,EAAQkK,GAAqBrF,kBAG/BkS,EAAGhT,EAAK/D,EAAQhN,EAAItB,OAAUsB,EAAI6S,UAAU7F,EAAOhN,EAAItB,QAAU,GACjEqlB,EAAG6B,GAAKjB,GACJxN,GAAQrF,KAAK9R,IAAQwlB,KACxBzB,EAAGS,EAAI,QAEHpd,IAAM2c,GA4BwBtL,KAAKoE,KAAMve,EAAQiR,EAAMuV,EAAa9kB,EAAK+kB,EAAQL,GAAgB5O,EAAQ4O,aAAcC,KAN1HZ,EAAK,IAAIrU,GAAUmN,KAAKzV,IAAK9I,EAAQiR,GAAOuV,GAAe,EAAG9kB,GAAO8kB,GAAe,GAA6B,kBAAlBD,EAA8BgB,GAAiBC,GAAc,EAAGf,GAC/JJ,IAAcZ,EAAG6B,GAAKjB,GACtBhW,GAAYoV,EAAGpV,SAASA,EAAUkO,KAAMve,GAChCue,KAAKzV,IAAM2c,IAmCtB5c,GAAa,SAAbA,WAAc1G,EAAOS,EAAMgG,OAWzB6e,EAAW1nB,EAAGyD,EAAGiiB,EAAIzlB,EAAQ0nB,EAAaC,EAAQznB,EAASslB,EAAQE,EAAUhX,EAAOkZ,EAAaC,EAV9F3jB,EAAO/B,EAAM+B,KACduH,EAAkGvH,EAAlGuH,KAAMtB,EAA4FjG,EAA5FiG,QAAS/D,EAAmFlC,EAAnFkC,gBAAiB2C,EAAkE7E,EAAlE6E,KAAM+Z,EAA4D5e,EAA5D4e,SAAU5Y,EAAkDhG,EAAlDgG,aAAc2K,EAAoC3Q,EAApC2Q,SAAUxQ,EAA0BH,EAA1BG,UAAWgC,EAAenC,EAAfmC,WACrFmD,EAAMrH,EAAM0D,KACZiiB,EAAc3lB,EAAMc,SACpBpD,EAAUsC,EAAMgiB,SAChBhgB,EAAShC,EAAMgC,OAEf4jB,EAAe5jB,GAA0B,WAAhBA,EAAOmV,KAAqBnV,EAAOD,KAAKrE,QAAUA,EAC3EmoB,EAAsC,SAArB7lB,EAAM8lB,aAA2B9R,EAClDmO,EAAKniB,EAAMsF,aAEZ6c,GAAQjgB,GAAcoH,IAAUA,EAAO,QACvCtJ,EAAM4S,MAAQrJ,GAAWD,EAAMqM,EAAUrM,MACzCtJ,EAAM6S,OAASH,EAAWtH,GAAY7B,IAAwB,IAAbmJ,EAAoBpJ,EAAOoJ,EAAUiD,EAAUrM,OAAS,EACrGoJ,GAAY1S,EAAM2S,QAAU3S,EAAMoE,UACrCsO,EAAW1S,EAAM6S,OACjB7S,EAAM6S,OAAS7S,EAAM4S,MACrB5S,EAAM4S,MAAQF,GAEf1S,EAAM+lB,OAAS5D,KAAQpgB,EAAKgG,cACvBoa,GAAOjgB,IAAcH,EAAKqd,QAAU,IAExCqG,GADA1nB,EAAUL,EAAQ,GAAKW,GAAUX,EAAQ,IAAIK,QAAU,IAC9BgE,EAAKhE,EAAQ+Q,MACtCwW,EAAY3jB,GAAeI,EAAMgN,IAC7B4W,IACHA,EAAYjgB,OAAS,GAAKigB,EAAYtY,SAAS,GAC9C5M,EAAO,GAAKsH,GAAgB9D,IAAoBC,EAAcyhB,EAAYrlB,QAAQ,GAAG,GAAQqlB,EAAY5hB,OAAOgE,GAAgBV,EAAMrD,GAAsB2S,IAE7JgP,EAAYtlB,MAAQ,GAEjB2H,MACH7E,GAAkBnD,EAAMc,SAAWmH,GAAMoU,IAAI3e,EAAS4D,GAAa,CAAC6V,KAAM,UAAWvB,WAAW,EAAO5T,OAAQA,EAAQiC,iBAAiB,EAAM2C,MAAO+e,GAAevpB,EAAYwK,GAAOoB,QAAS,KAAM6N,MAAO,EAAG8K,SAAUA,GAAa,kBAAMrT,GAAUtN,EAAO,aAAcof,QAAS,GAAIpX,KACzRhI,EAAMc,SAASyB,IAAM,EACrBvC,EAAMc,SAASqc,KAAOnd,EACrBS,EAAO,IAAMG,IAAgBqD,IAAoBC,IAAiBlE,EAAMc,SAASiD,OAAOC,IACrFC,GACCoD,GAAO5G,GAAQ,GAAKgG,GAAS,cAChChG,IAAST,EAAM0F,OAASjF,SAIpB,GAAIsH,GAAgBV,IAErBse,KACJllB,IAASwD,GAAkB,GAC3B5C,EAAIC,GAAa,CAChBsU,WAAW,EACXuB,KAAM,cACNvQ,KAAM3C,IAAoB0hB,GAAevpB,EAAYwK,GACrD3C,gBAAiBA,EACjBmb,QAAS,EACTpd,OAAQA,GACNsjB,GACHG,IAAgBpkB,EAAEtD,EAAQ+Q,MAAQ2W,GAClCtiB,GAAkBnD,EAAMc,SAAWmH,GAAMoU,IAAI3e,EAAS2D,IACtDrB,EAAMc,SAASyB,IAAM,EACrBvC,EAAMc,SAASqc,KAAOnd,EACrBS,EAAO,IAAOG,EAAaZ,EAAMc,SAASiD,OAAOC,IAAuBhE,EAAMc,SAASR,QAAQ,GAAG,IACnGN,EAAM0F,OAASjF,EACVwD,GAEE,IAAKxD,cADXiG,WAAW1G,EAAMc,SAAUmE,EAAUA,OAMxCjF,EAAM2G,IAAM3G,EAAMgmB,SAAW,EAC7Bpf,EAAQS,GAAOjL,EAAYwK,IAAWA,IAASS,EAC1CzJ,EAAI,EAAGA,EAAIF,EAAQO,OAAQL,IAAK,IAEpC4nB,GADA3nB,EAASH,EAAQE,IACDE,OAASL,GAASC,GAASE,GAAGE,MAC9CkC,EAAMyc,UAAU7e,GAAK2lB,EAAW,GAChCnjB,GAAYolB,EAAOrJ,KAAOlc,GAAYhC,QAAU8B,KAChDwM,EAAQqZ,IAAgBloB,EAAUE,EAAIgoB,EAAY9lB,QAAQjC,GACtDE,IAA0G,KAA9FslB,EAAS,IAAItlB,GAAW6P,KAAK/P,EAAQ4nB,GAAeH,EAAWtlB,EAAOuM,EAAOqZ,KAC5F5lB,EAAM2G,IAAM2c,EAAK,IAAIrU,GAAUjP,EAAM2G,IAAK9I,EAAQwlB,EAAO/lB,KAAM,EAAG,EAAG+lB,EAAO/iB,OAAQ+iB,EAAQ,EAAGA,EAAOM,UACtGN,EAAOxV,OAAO/O,QAAQ,SAAAxB,GAASimB,EAASjmB,GAAQgmB,IAChDD,EAAOM,WAAa4B,EAAc,KAE9BxnB,GAAW0nB,MACVpkB,KAAKikB,EACL1W,GAASvN,KAAOgiB,EAASD,GAAa/hB,EAAGikB,EAAWtlB,EAAOuM,EAAO1O,EAAQ+nB,IAC7EvC,EAAOM,WAAa4B,EAAc,GAElChC,EAASliB,GAAKiiB,EAAKtV,GAAcgK,KAAKhY,EAAOnC,EAAQwD,EAAG,MAAOikB,EAAUjkB,GAAIkL,EAAOqZ,EAAa,EAAG7jB,EAAKkiB,cAI5GjkB,EAAMimB,KAAOjmB,EAAMimB,IAAIroB,IAAMoC,EAAMoN,KAAKvP,EAAQmC,EAAMimB,IAAIroB,IACtDioB,GAAiB7lB,EAAM2G,MAC1Bib,GAAoB5hB,EACpBiC,EAAgBqf,aAAazjB,EAAQ0lB,EAAUvjB,EAAMkd,WAAWzc,IAChEilB,GAAe1lB,EAAMgC,OACrB4f,GAAoB,GAErB5hB,EAAM2G,KAAOC,IAASxG,GAAYolB,EAAOrJ,IAAM,GAEhDoJ,GAAeW,GAA0BlmB,GACzCA,EAAMmmB,SAAWnmB,EAAMmmB,QAAQnmB,GAEhCA,EAAMke,UAAYyC,EAClB3gB,EAAMa,WAAab,EAAMimB,KAAOjmB,EAAM2G,OAAS+e,EAC9CxjB,GAAazB,GAAQ,GAAM0hB,EAAG7hB,OAAOuK,GAAS,GAAM,IAyEtD4Y,GAAqB,SAArBA,mBAAsB1nB,EAAOiE,EAAOpC,EAAGC,EAAQH,UAAa1B,EAAYD,GAASA,EAAMic,KAAKhY,EAAOpC,EAAGC,EAAQH,GAAY5B,EAAUC,KAAWA,EAAM+D,QAAQ,WAAc0M,GAAezQ,GAASA,GACnMqqB,GAAqBpP,GAAiB,4DACtCqP,GAAsB,GACvB3nB,GAAa0nB,GAAqB,kDAAmD,SAAA9oB,UAAQ+oB,GAAoB/oB,GAAQ,QA8B5G2K,8BAEAvK,EAASqE,EAAM6D,EAAU0gB,SACf,iBAAVvkB,IACV6D,EAASrB,SAAWxC,EACpBA,EAAO6D,EACPA,EAAW,UAMXuc,EAAIvkB,EAAGiE,EAAMhC,EAAGwB,EAAGklB,EAAWC,EAAaC,mBAJtCH,EAAcvkB,EAAOD,GAAiBC,WACsEA,KAA5GwC,IAAAA,SAAUsR,IAAAA,MAAO5R,IAAAA,gBAAiBmb,IAAAA,QAASxJ,IAAAA,UAAW1T,IAAAA,UAAWX,IAAAA,SAAU4L,IAAAA,cAAeuF,IAAAA,SAC/F1Q,EAASD,EAAKC,QAAUC,EACxB6f,GAAiBzf,EAAS3E,IAAY2Y,EAAc3Y,GAAWzB,EAAUyB,EAAQ,IAAO,WAAYqE,GAAS,CAACrE,GAAWY,GAAQZ,QAE7HskB,SAAWF,EAAc7jB,OAASR,GAASqkB,GAAiB5kB,EAAM,eAAiBQ,EAAU,gCAAiC2X,EAAQG,iBAAmB,KACzJiH,UAAY,KACZqJ,WAAalQ,EACd1T,GAAakd,GAAW7iB,EAAgBgI,IAAahI,EAAgBsZ,GAAQ,IAChF9T,EAAO2kB,EAAK3kB,MACZogB,EAAKuE,EAAKphB,SAAW,IAAIkC,GAAS,CAAC2P,KAAM,SAAU5V,SAAUA,GAAY,GAAI7D,QAASsE,GAA0B,WAAhBA,EAAOmV,KAAoBnV,EAAOD,KAAKrE,QAAUokB,KAC9I1U,OACH+U,EAAGngB,OAASmgB,EAAG5f,8BACf4f,EAAGxe,OAAS,EACRyb,GAAW7iB,EAAgBgI,IAAahI,EAAgBsZ,GAAQ,IACnEhW,EAAIiiB,EAAc7jB,OAClBuoB,EAAcpH,GAAWhW,GAAWgW,GAChCjjB,EAAUijB,OACR/d,KAAK+d,GACJgH,GAAmBtmB,QAAQuB,MACRolB,EAAvBA,GAA4C,IACzBplB,GAAK+d,EAAQ/d,QAI9BzD,EAAI,EAAGA,EAAIiC,EAAGjC,KAClBiE,EAAOF,GAAeI,EAAMskB,KACvBjH,QAAU,EACf1M,IAAa7Q,EAAK6Q,SAAWA,GAC7B+T,GAAsB9pB,GAAOkF,EAAM4kB,GACnCF,EAAYzE,EAAclkB,GAE1BiE,EAAK0C,UAAYkf,GAAmBlf,4BAAgB3G,EAAG2oB,EAAWzE,GAClEjgB,EAAKgU,QAAU4N,GAAmB5N,4BAAajY,EAAG2oB,EAAWzE,IAAkB,GAAK4E,EAAK3gB,QACpFqZ,GAAiB,IAANvf,GAAWgC,EAAKgU,UAC1B9P,OAAS8P,EAAQhU,EAAKgU,QACtBlS,QAAUkS,EACfhU,EAAKgU,MAAQ,GAEdsM,EAAGrD,GAAGyH,EAAW1kB,EAAM2kB,EAAcA,EAAY5oB,EAAG2oB,EAAWzE,GAAiB,GAChFK,EAAGvP,MAAQpB,GAASuK,KAErBoG,EAAG5d,WAAcA,EAAWsR,EAAQ,EAAM6Q,EAAKphB,SAAW,OACpD,GAAIpD,EAAW,CACrBJ,GAAiBR,GAAa6gB,EAAGpgB,KAAKR,SAAU,CAAC+H,KAAK,UACtD6Y,EAAGvP,MAAQrJ,GAAWrH,EAAUoH,MAAQvH,EAAKuH,MAAQ,YAEpDpJ,EAAGymB,EAAInoB,EADJiC,EAAO,KAEP4B,EAASH,GACZA,EAAUpD,QAAQ,SAAAiI,UAASob,EAAGrD,GAAGgD,EAAe/a,EAAO,OACvDob,EAAG5d,eACG,KAEDlD,KADLQ,EAAO,GACGK,EACH,SAANb,GAAsB,aAANA,GAAoBuiB,GAAeviB,EAAGa,EAAUb,GAAIQ,EAAMK,EAAU4hB,cAEhFziB,KAAKQ,MACT3B,EAAI2B,EAAKR,GAAG6H,KAAK,SAAChJ,EAAGgL,UAAMhL,EAAE2C,EAAIqI,EAAErI,IAE9BjF,EADL6C,EAAO,EACK7C,EAAIsC,EAAEjC,OAAQL,KAEzBY,EAAI,CAAC8K,MADLqd,EAAKzmB,EAAEtC,IACOmmB,EAAGxf,UAAWoiB,EAAG9jB,GAAKjF,EAAIsC,EAAEtC,EAAI,GAAGiF,EAAI,IAAM,IAAM0B,IAC/DlD,GAAKslB,EAAGnoB,EACV2jB,EAAGrD,GAAGgD,EAAetjB,EAAGiC,GACxBA,GAAQjC,EAAE+F,SAGZ4d,EAAG5d,WAAaA,GAAY4d,EAAGrD,GAAG,GAAI,CAACva,SAAUA,EAAW4d,EAAG5d,cAGjEA,GAAYmiB,EAAKniB,SAAUA,EAAW4d,EAAG5d,mBAGpCe,SAAW,SAGC,IAAdsQ,GAAuB5B,IAC1B4N,6BACA3f,EAAgBqf,aAAaQ,GAC7BF,GAAoB,GAErBjc,GAAe3D,4BAAc4D,GAC7B7D,EAAK2b,UAAYgJ,EAAK/I,UACtB5b,EAAK+a,QAAU4J,EAAK5J,QAAO,IACvB7Y,IAAqBM,IAAarC,GAAawkB,EAAK/iB,SAAWzE,GAAc8C,EAAOoD,QAAUhJ,EAAY6H,IAxpEvF,SAAxB2iB,sBAAwBpmB,UAAcA,GAAcA,EAAUmE,KAAOiiB,sBAAsBpmB,EAAUwB,QAwpE8B4kB,6BAA+C,WAAhB5kB,EAAOmV,UAClK7S,QAAUW,IACV3E,OAAOtB,KAAKwL,IAAI,GAAIqL,IAAU,IAEpC1I,GAAiB/G,6BAAqB+G,4DAGvC7M,OAAA,gBAAOwD,EAAWpD,EAAgBC,OAMhCF,EAAM6iB,EAAI3G,EAAW1F,EAAe6I,EAAetN,EAAQmM,EAAOrZ,EAAUoN,EALzEqN,EAAW3D,KAAKhX,MACnB4a,EAAO5D,KAAKvX,MACZwC,EAAM+U,KAAK1Y,KACXmjB,EAAa/iB,EAAY,EACzB2C,EAAqBuZ,EAAO/a,EAAnBnB,IAAgC+iB,EAAc7G,EAAQlc,EAAYmB,EAAY,EAAInB,KAEvFuD,GAEE,GAAIZ,IAAU2V,KAAK9X,SAAWR,GAAanD,IAAWyb,KAAKvb,UAAYub,KAAK9X,QAAY8X,KAAKtb,UAAasb,KAAK1W,OAAS,GAAOmhB,GAAezK,KAAK/b,MAAO,IAChKI,EAAOgG,EACPnB,EAAW8W,KAAK9W,SACZ8W,KAAKhY,QAAS,IACjB6S,EAAgB5P,EAAM+U,KAAK5X,QACvB4X,KAAKhY,SAAW,GAAKyiB,SACjBzK,KAAKtY,UAA0B,IAAhBmT,EAAsBnT,EAAWpD,EAAgBC,MAExEF,EAAOvB,GAAcuH,EAAQwQ,GACzBxQ,IAAUuZ,GACbrD,EAAYP,KAAKhY,QACjB3D,EAAO4G,IAGPsV,KADAmD,EAAgB5gB,GAAcuH,EAAQwQ,MAErB0F,IAAcmD,GAC9Brf,EAAO4G,EACPsV,KACiBtV,EAAP5G,IACVA,EAAO4G,IAGTmL,EAAS4J,KAAKzJ,OAAsB,EAAZgK,KAEvBjK,EAAW0J,KAAKvJ,OAChBpS,EAAO4G,EAAM5G,GAEdqf,EAAgBzb,GAAgB+X,KAAK9X,OAAQ2S,GACzCxW,IAASsf,IAAapf,GAASyb,KAAKvb,UAAY8b,IAAcmD,cAE5Dxb,OAASmC,EACP2V,KAEJO,IAAcmD,IACjBxa,GAAY8W,KAAKvJ,QAAUN,GAAmBjN,EAAUkN,GAEpD4J,KAAKra,KAAKse,gBAAkB7N,IAAW4J,KAAK8D,OAASzf,IAASwW,GAAiBmF,KAAKvb,gBAClFqf,MAAQvf,EAAQ,OAChBL,OAAOpB,GAAc+X,EAAgB0F,IAAY,GAAMoB,aAAamC,MAAQ,QAK/E9D,KAAKvb,SAAU,IACf2F,GAAkB4V,KAAMyK,EAAa/iB,EAAYrD,EAAME,EAAOD,EAAgB+F,eAC5EnC,OAAS,EACP8X,UAEJ2D,IAAa3D,KAAKhX,OAAWzE,GAASyb,KAAKra,KAAKse,eAAiB1D,IAAcmD,UAC3E1D,QAEJ/U,IAAQ+U,KAAK1Y,YACT0Y,KAAK9b,OAAOwD,EAAWpD,EAAgBC,WAI3C2D,OAASmC,OACTrB,MAAQ3E,GAER2b,KAAK7Y,MAAQ6Y,KAAKzX,WACjBpB,KAAO,OACPlD,MAAQ,QAGTse,MAAQA,GAASjM,GAAY0J,KAAKxJ,OAAOnS,EAAO4G,GACjD+U,KAAK2J,aACHpH,MAAQA,EAAQ,EAAIA,GAGtBle,IAASsf,IAAarf,IAAmBic,IAC5CrP,GAAU8O,KAAM,WACZA,KAAK9X,SAAWmC,UACZ2V,SAGTkH,EAAKlH,KAAKzV,IACH2c,GACNA,EAAG3T,EAAEgP,EAAO2E,EAAGhZ,GACfgZ,EAAKA,EAAGtgB,MAERsC,GAAYA,EAAShF,OAAOwD,EAAY,EAAIA,EAAYwB,EAAS5B,KAAO4B,EAASsN,MAAMnS,EAAO2b,KAAK1Y,MAAOhD,EAAgBC,IAAYyb,KAAKtb,WAAasb,KAAK1W,OAAS5B,GAEnKsY,KAAK8B,YAAcxd,IACtBmmB,GAAchjB,GAAeuY,KAAMtY,EAAWpD,EAAgBC,GAC9D2M,GAAU8O,KAAM,kBAGZhY,SAAWuY,IAAcmD,GAAiB1D,KAAKra,KAAKue,WAAa5f,GAAkB0b,KAAKpa,QAAUsL,GAAU8O,KAAM,YAElH3V,IAAU2V,KAAKvX,OAAU4B,GAAU2V,KAAK9X,SAAWmC,IACvDogB,IAAezK,KAAK8B,WAAara,GAAeuY,KAAMtY,EAAW,GAAM,IACtEA,GAAcuD,KAAUZ,IAAU2V,KAAKvX,OAAoB,EAAXuX,KAAKzX,MAAc8B,GAAS2V,KAAKzX,IAAM,IAAOxB,GAAkBiZ,KAAM,GAC/G1b,GAAoBmmB,IAAe9G,KAActZ,GAASsZ,GAAYvN,KAC7ElF,GAAU8O,KAAO3V,IAAUuZ,EAAO,aAAe,qBAAsB,SAClEtB,OAAWjY,EAAQuZ,GAA2B,EAAnB5D,KAAKpW,aAAoBoW,KAAKsC,gBA7rEvC,SAA3BoI,yBAA4B9mB,EAAO8D,EAAWpD,EAAgBC,OAK5D2iB,EAAI3G,EAAWmD,EAJZiH,EAAY/mB,EAAM2e,MACrBA,EAAQ7a,EAAY,IAAOA,KAAgB9D,EAAM2D,QAJpB,SAA/BqjB,oCAAiChlB,IAAAA,cAAYA,GAAUA,EAAO2C,KAAO3C,EAAOnB,WAAamB,EAAOke,QAAUle,EAAOwD,UAAY,GAAKwhB,6BAA6BhlB,IAIlGglB,CAA6BhnB,KAAaA,EAAMa,WAAYqF,GAAmBlG,MAAcA,EAAM2E,IAAM,GAAK3E,EAAMuC,IAAIoC,IAAM,KAAOuB,GAAmBlG,IAAY,EAAI,EACnOod,EAAcpd,EAAMwE,QACpBiC,EAAQ,KAEL2W,GAAepd,EAAMoE,UACxBqC,EAAQhB,GAAO,EAAGzF,EAAM6E,MAAOf,GAC/B6Y,EAAYtY,GAAgBoC,EAAO2W,GACnCpd,EAAM2S,OAAsB,EAAZgK,IAAmBgC,EAAQ,EAAIA,GAC3ChC,IAActY,GAAgBrE,EAAMsE,OAAQ8Y,KAC/C2J,EAAY,EAAIpI,EAChB3e,EAAM+B,KAAKse,eAAiBrgB,EAAMa,UAAYb,EAAM+d,eAGlDY,IAAUoI,GAAanmB,GAAcD,GAASX,EAAM0F,SAAWT,IAAcnB,GAAa9D,EAAM0F,OAAS,KACvG1F,EAAMa,UAAY2F,GAAkBxG,EAAO8D,EAAWnD,EAAOD,EAAgB+F,cAGlFqZ,EAAgB9f,EAAM0F,OACtB1F,EAAM0F,OAAS5B,IAAcpD,EAAiBuE,EAAW,GACtCvE,EAAnBA,GAAoCoD,IAAcgc,EAClD9f,EAAM2e,MAAQA,EACd3e,EAAM+lB,QAAUpH,EAAQ,EAAIA,GAC5B3e,EAAMoF,MAAQ,EACdpF,EAAMsE,OAASmC,EACf6c,EAAKtjB,EAAM2G,IACJ2c,GACNA,EAAG3T,EAAEgP,EAAO2E,EAAGhZ,GACfgZ,EAAKA,EAAGtgB,MAETc,EAAY,GAAKD,GAAe7D,EAAO8D,EAAWpD,GAAgB,GAClEV,EAAMke,YAAcxd,GAAkB4M,GAAUtN,EAAO,YACvDyG,GAASzG,EAAMoE,UAAY1D,GAAkBV,EAAMgC,QAAUsL,GAAUtN,EAAO,aACzE8D,GAAa9D,EAAM6E,OAASf,EAAY,IAAM9D,EAAM2e,QAAUA,IAClEA,GAASxb,GAAkBnD,EAAO,GAC7BU,GAAmBE,IACvB0M,GAAUtN,EAAQ2e,EAAQ,aAAe,qBAAsB,GAC/D3e,EAAM0e,OAAS1e,EAAM0e,eAGZ1e,EAAM0F,SACjB1F,EAAM0F,OAAS5B,GAojEfgjB,CAAyB1K,KAAMtY,EAAWpD,EAAgBC,UAoGpDyb,QAGR1e,QAAA,0BACQ0e,KAAK4F,YAGbjE,WAAA,oBAAWiF,UACRA,GAAS5G,KAAKra,KAAKgG,eAAkBqU,KAAKtb,SAAW,QAClD6F,IAAMyV,KAAK6J,IAAM7J,KAAK8B,UAAY9B,KAAK/b,MAAQ+b,KAAKuC,MAAQ,OAC5DlC,UAAY,QACZnX,UAAY8W,KAAK9W,SAASyY,WAAWiF,eAC7BjF,qBAAWiF,MAGzBiE,QAAA,iBAAQlqB,EAAUhB,EAAOqD,EAAO8nB,EAAiBC,GAChD9S,GAAiBvN,GAAQwT,YACpB3V,KAAOyX,KAAKqB,WAEhBkB,EADGle,EAAOzB,KAAKyL,IAAI2R,KAAK1Y,MAAO0Y,KAAK7Z,IAAI6C,MAAQgX,KAAKzY,QAAUyY,KAAKzX,iBAEhE9D,UAAY6F,GAAW0V,KAAM3b,GAClCke,EAAQvC,KAAKxJ,MAAMnS,EAAO2b,KAAK1Y,MA5UZ,SAApB0jB,kBAAqBpnB,EAAOjD,EAAUhB,EAAOqD,EAAO8nB,EAAiBvI,EAAOle,EAAM0mB,OAEhF7D,EAAI+D,EAAQC,EAAQ1pB,EADjB2pB,GAAYvnB,EAAM2G,KAAO3G,EAAMgmB,WAAchmB,EAAMgmB,SAAW,KAAKjpB,OAElEwqB,MACJA,EAAUvnB,EAAMgmB,SAASjpB,GAAY,GACrCuqB,EAAStnB,EAAMyc,UACf7e,EAAIoC,EAAMgiB,SAAS/jB,OACZL,KAAK,KACX0lB,EAAKgE,EAAO1pB,GAAGb,KACLumB,EAAGhZ,GAAKgZ,EAAGhZ,EAAE3D,QACtB2c,EAAKA,EAAGhZ,EAAE3D,IACH2c,GAAMA,EAAGjiB,IAAMtE,GAAYumB,EAAG6B,KAAOpoB,GAC3CumB,EAAKA,EAAGtgB,UAGLsgB,SAEJU,GAAsB,EACtBhkB,EAAM+B,KAAKhF,GAAY,MACvB2J,GAAW1G,EAAOS,GAClBujB,GAAsB,EACfmD,EAAgBjqB,EAAMH,EAAW,2BAA6B,EAEtEwqB,EAAQvgB,KAAKsc,OAGf1lB,EAAI2pB,EAAQtpB,OACLL,MAEN0lB,GADA+D,EAASE,EAAQ3pB,IACL+I,KAAO0gB,GAChB3a,GAAKtN,GAAmB,IAAVA,GAAiB8nB,EAA0B5D,EAAG5W,GAAKtN,GAAS,GAAKuf,EAAQ2E,EAAGhT,EAAzClR,EACpDkkB,EAAGhT,EAAIvU,EAAQunB,EAAG5W,EAClB2a,EAAOtD,IAAMsD,EAAOtD,EAAIhlB,GAAOhD,GAASoM,GAAQkf,EAAOtD,IACvDsD,EAAOnc,IAAMmc,EAAOnc,EAAIoY,EAAG5W,EAAIvE,GAAQkf,EAAOnc,IAoT1Ckc,CAAkBhL,KAAMrf,EAAUhB,EAAOqD,EAAO8nB,EAAiBvI,EAAOle,EAAM0mB,GAC1E/K,KAAK6K,QAAQlqB,EAAUhB,EAAOqD,EAAO8nB,EAAiB,IAG/DhiB,GAAekX,KAAM,QAChBpa,QAAUQ,GAAmB4Z,KAAK7Z,IAAK6Z,KAAM,SAAU,QAASA,KAAK7Z,IAAI0D,MAAQ,SAAW,GAC1FmW,KAAK9b,OAAO,OAGpB8M,KAAA,cAAK1P,EAASqE,eAAAA,IAAAA,EAAO,SACfrE,GAAaqE,GAAiB,QAATA,eACpB1B,MAAQ+b,KAAKzV,IAAM,OACnB3E,OAASkL,GAAWkP,MAAQA,KAAKjP,eAAiBiP,KAAKjP,cAAcC,OAAOxM,GAC1Ewb,QAEJA,KAAK9W,SAAU,KACd0a,EAAO5D,KAAK9W,SAASV,4BACpBU,SAASgc,aAAa5jB,EAASqE,EAAM6f,KAA0D,IAArCA,GAAkB7f,KAAK6T,WAAoBnD,QAAUvF,GAAWkP,WAC1Hpa,QAAUge,IAAS5D,KAAK9W,SAASV,iBAAmBqC,GAAamV,KAAMA,KAAK1Y,KAAO0Y,KAAK9W,SAAST,MAAQmb,EAAM,EAAG,GAChH5D,SAMPoL,EAAkBC,EAAWC,EAAmBjG,EAAOpgB,EAAGiiB,EAAI1lB,EAJ3DkkB,EAAgB1F,KAAK4F,SACxB2F,EAAiBjqB,EAAUY,GAAQZ,GAAWokB,EAC9C8F,EAAkBxL,KAAKK,UACvBoL,EAAUzL,KAAKzV,SAEV5E,GAAiB,QAATA,IAz4EA,SAAf+lB,aAAgBC,EAAIC,WACfpqB,EAAImqB,EAAG9pB,OACVgD,EAAQrD,IAAMoqB,EAAG/pB,OACXgD,GAASrD,KAAOmqB,EAAGnqB,KAAOoqB,EAAGpqB,YAC7BA,EAAI,EAq4EsBkqB,CAAahG,EAAe6F,SACnD,QAAT5lB,IAAmBqa,KAAKzV,IAAM,GACvBuG,GAAWkP,UAEnBoL,EAAmBpL,KAAK6J,IAAM7J,KAAK6J,KAAO,GAC7B,QAATlkB,IACCjG,EAAUiG,KACbV,EAAI,GACJ3C,GAAaqD,EAAM,SAAAzE,UAAQ+D,EAAE/D,GAAQ,IACrCyE,EAAOV,GAERU,EAtVkB,SAApBkmB,kBAAqBvqB,EAASqE,OAG5BF,EAAMR,EAAGzD,EAAG6Q,EAFT1Q,EAAUL,EAAQ,GAAKW,GAAUX,EAAQ,IAAIK,QAAU,EAC1DmqB,EAAmBnqB,GAAWA,EAAQ0Q,YAElCyZ,SACGnmB,MAGHV,KADLQ,EAAOlF,GAAO,GAAIoF,GACRmmB,KACL7mB,KAAKQ,MAERjE,GADA6Q,EAAUyZ,EAAgB7mB,GAAGxC,MAAM,MACvBZ,OACNL,KACLiE,EAAK4M,EAAQ7Q,IAAMiE,EAAKR,UAKpBQ,EAoUComB,CAAkBnG,EAAe/f,IAEzCnE,EAAIkkB,EAAc7jB,OACXL,SACD+pB,EAAe7nB,QAAQgiB,EAAclkB,QAUpCyD,KATLomB,EAAYG,EAAgBhqB,GACf,QAATmE,GACHylB,EAAiB5pB,GAAKmE,EACtB0f,EAAQgG,EACRC,EAAoB,KAEpBA,EAAoBF,EAAiB5pB,GAAK4pB,EAAiB5pB,IAAM,GACjE6jB,EAAQ1f,GAEC0f,GACT6B,EAAKmE,GAAaA,EAAUpmB,MAErB,SAAUiiB,EAAGhZ,IAAuB,IAAjBgZ,EAAGhZ,EAAE8C,KAAK/L,IAClC4B,GAAsBmZ,KAAMkH,EAAI,cAE1BmE,EAAUpmB,IAEQ,QAAtBqmB,IACHA,EAAkBrmB,GAAK,eAKtBR,WAAaub,KAAKzV,KAAOkhB,GAAW3a,GAAWkP,MAC7CA,YAID0C,GAAP,YAAUphB,EAASqE,EAAnB,UACQ,IAAIkG,MAAMvK,EAASqE,EAD3B,UAIOyH,KAAP,cAAY9L,EAASqE,UACb0F,GAAiB,EAAG+U,kBAGrB0C,YAAP,qBAAmBrJ,EAAO4C,EAAU9Q,EAAQlL,UACpC,IAAIwL,MAAMwQ,EAAU,EAAG,CAACxU,iBAAgB,EAAO2C,MAAK,EAAOgP,WAAU,EAAOC,MAAMA,EAAO0J,WAAW9G,EAAU0P,kBAAkB1P,EAAU+G,iBAAiB7X,EAAQygB,wBAAwBzgB,EAAQkR,cAAcpc,WAGlNsiB,OAAP,gBAAcrhB,EAASshB,EAAUC,UACzBxX,GAAiB,EAAG+U,kBAGrBH,IAAP,aAAW3e,EAASqE,UACnBA,EAAKwC,SAAW,EAChBxC,EAAKqb,cAAgBrb,EAAKqF,OAAS,GAC5B,IAAIa,MAAMvK,EAASqE,UAGpBuf,aAAP,sBAAoB5jB,EAAS+jB,EAAOC,UAC5Bzf,EAAgBqf,aAAa5jB,EAAS+jB,EAAOC,WA1U3BpF,IA8U3Bhb,GAAa2G,GAAM4G,UAAW,CAACmT,SAAS,GAAI3hB,MAAM,EAAGS,SAAS,EAAGmlB,IAAI,EAAGE,QAAQ,IAWhFznB,GAAa,sCAAuC,SAAApB,GACnD2K,GAAM3K,GAAQ,eACT6kB,EAAK,IAAI3a,GACZG,EAASgQ,GAAOK,KAAKwE,UAAW,UACjC7U,EAAOvJ,OAAgB,kBAATd,EAA2B,EAAI,EAAG,EAAG,GAC5C6kB,EAAG7kB,GAAMmU,MAAM0Q,EAAIxa,MA2BR,SAAnB0gB,GAAoBxqB,EAAQd,EAAUhB,UAAU8B,EAAOyqB,aAAavrB,EAAUhB,GAkDxD,SAAtBwsB,GAAuB1qB,EAAQd,EAAUhB,EAAOob,GAC/CA,EAAKqR,KAAK3qB,EAAQd,EAAUoa,EAAK+N,EAAElN,KAAKb,EAAKnX,MAAOjE,EAAOob,EAAKsR,IAAKtR,GAtDvE,IAAIsN,GAAe,SAAfA,aAAgB5mB,EAAQd,EAAUhB,UAAU8B,EAAOd,GAAYhB,GAClEyoB,GAAc,SAAdA,YAAe3mB,EAAQd,EAAUhB,UAAU8B,EAAOd,GAAUhB,IAC5DwoB,GAAuB,SAAvBA,qBAAwB1mB,EAAQd,EAAUhB,EAAOob,UAAStZ,EAAOd,GAAUoa,EAAKgO,GAAIppB,IAEpFyS,GAAa,SAAbA,WAAc3Q,EAAQd,UAAaf,EAAY6B,EAAOd,IAAaynB,GAActoB,EAAa2B,EAAOd,KAAcc,EAAOyqB,aAAeD,GAAmB5D,IAC5JY,GAAe,SAAfA,aAAgB1G,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAGrC,KAAKC,MAAkC,KAA3BkY,EAAKzK,EAAIyK,EAAK7G,EAAIqO,IAAoB,IAASxH,IACpHiO,GAAiB,SAAjBA,eAAkBzG,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,KAAM8V,EAAKzK,EAAIyK,EAAK7G,EAAIqO,GAAQxH,IACxF6N,GAAuB,SAAvBA,qBAAgCrG,EAAOxH,OAClCmM,EAAKnM,EAAKxQ,IACb+F,EAAI,OACAiS,GAASxH,EAAKjM,EAClBwB,EAAIyK,EAAKjM,OACH,GAAc,IAAVyT,GAAexH,EAAK4M,EAC9BrX,EAAIyK,EAAK4M,MACH,MACCT,GACN5W,EAAI4W,EAAGjiB,GAAKiiB,EAAG4B,EAAI5B,EAAG4B,EAAE5B,EAAG5W,EAAI4W,EAAGhT,EAAIqO,GAAU3f,KAAKC,MAA8B,KAAvBqkB,EAAG5W,EAAI4W,EAAGhT,EAAIqO,IAAkB,KAAUjS,EACtG4W,EAAKA,EAAGtgB,MAET0J,GAAKyK,EAAK7G,EAEX6G,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAGqL,EAAGyK,IAE7BpJ,GAAoB,SAApBA,kBAA6B4Q,EAAOxH,WAC/BmM,EAAKnM,EAAKxQ,IACP2c,GACNA,EAAG3T,EAAEgP,EAAO2E,EAAGhZ,GACfgZ,EAAKA,EAAGtgB,OAGVmL,GAAqB,SAArBA,mBAA8BD,EAAUlO,EAAOnC,EAAQd,WAErDmG,EADGogB,EAAKlH,KAAKzV,IAEP2c,GACNpgB,EAAOogB,EAAGtgB,MACVsgB,EAAGjiB,IAAMtE,GAAYumB,EAAGpV,SAASA,EAAUlO,EAAOnC,GAClDylB,EAAKpgB,GAGP+K,GAAoB,SAApBA,kBAA6BlR,WAE3B2rB,EAA0BxlB,EADvBogB,EAAKlH,KAAKzV,IAEP2c,GACNpgB,EAAOogB,EAAGtgB,MACLsgB,EAAGjiB,IAAMtE,IAAaumB,EAAGqF,IAAOrF,EAAGqF,KAAO5rB,EAC9CkG,GAAsBmZ,KAAMkH,EAAI,OACrBA,EAAGsF,MACdF,EAA2B,GAE5BpF,EAAKpgB,SAEEwlB,GAKTxC,GAA4B,SAA5BA,0BAA4BlkB,WAE1BkB,EAAM2lB,EAAKC,EAAOC,EADfzF,EAAKthB,EAAO2E,IAGT2c,GAAI,KACVpgB,EAAOogB,EAAGtgB,MACV6lB,EAAMC,EACCD,GAAOA,EAAIG,GAAK1F,EAAG0F,IACzBH,EAAMA,EAAI7lB,OAENsgB,EAAGvgB,MAAQ8lB,EAAMA,EAAI9lB,MAAQgmB,GACjCzF,EAAGvgB,MAAMC,MAAQsgB,EAEjBwF,EAAQxF,GAEJA,EAAGtgB,MAAQ6lB,GACfA,EAAI9lB,MAAQugB,EAEZyF,EAAOzF,EAERA,EAAKpgB,EAENlB,EAAO2E,IAAMmiB,GAIF7Z,wBAiBZf,SAAA,kBAAStP,EAAMoB,EAAOnC,QAChB2qB,KAAOpM,KAAKoM,MAAQpM,KAAKC,SACzBA,IAAMkM,QACNrD,EAAItmB,OACJ6pB,GAAK5qB,OACLmC,MAAQA,iCApBFkD,EAAMrF,EAAQiR,EAAM1P,EAAO6pB,EAAQC,EAAU/R,EAAMmN,EAAQX,QACjE9gB,EAAIhF,OACJ6O,EAAItN,OACJkR,EAAI2Y,OACJ5nB,EAAIyN,OACJa,EAAIuZ,GAAY7D,QAChB/a,EAAI6M,GAAQiF,UACZC,IAAMiI,GAAUG,QAChBuE,GAAKrF,GAAY,QACjB3gB,MAAQE,KAEZA,EAAKH,MAAQqZ,MAgBhB1d,GAAasY,GAAiB,sOAAuO,SAAA1Z,UAAQyR,GAAezR,GAAQ,IACpSV,GAASusB,SAAWvsB,GAASwsB,UAAYnhB,GACzCrL,GAASysB,aAAezsB,GAAS0sB,YAAc9hB,GAC/CvF,EAAkB,IAAIuF,GAAS,CAACoX,cAAc,EAAOrd,SAAUoU,EAAWtS,oBAAoB,EAAM8Y,GAAG,OAAQhX,mBAAmB,IAClIkQ,EAAQ4O,aAAe/S,GAoBV,SAAZqY,GAAY7hB,UAASyN,GAAWzN,IAAS8hB,IAAarZ,IAAI,SAAA0K,UAAKA,MAC9C,SAAjB4O,SACKhpB,EAAOuZ,KAAKC,MACfyP,EAAU,GACiB,EAAxBjpB,EAAOkpB,KACVJ,GAAU,kBACVK,GAAO9qB,QAAQ,SAAAwR,OAGbrP,EAAOI,EAAGwoB,EAAUC,EAFjBC,EAAUzZ,EAAEyZ,QACfC,EAAa1Z,EAAE0Z,eAEX3oB,KAAK0oB,GACT9oB,EAAQwH,EAAKwhB,WAAWF,EAAQ1oB,IAAIqoB,WAC1BG,EAAW,GACjB5oB,IAAU+oB,EAAW3oB,KACxB2oB,EAAW3oB,GAAKJ,EAChB6oB,EAAU,GAGRA,IACHxZ,EAAEvM,SACF8lB,GAAYH,EAAQ1iB,KAAKsJ,MAG3BiZ,GAAU,oBACVG,EAAQ5qB,QAAQ,SAAAwR,UAAKA,EAAE4Z,QAAQ5Z,EAAG,SAAA1R,UAAQ0R,EAAE/K,IAAI,KAAM3G,OACtD+qB,GAAiBlpB,EACjB8oB,GAAU,eA/Bb,OAAIK,GAAS,GACZzU,GAAa,GACbqU,GAAc,GACdG,GAAiB,EACjBQ,GAAa,EA+BRC,2BASL7kB,IAAA,aAAIjI,EAAMsB,EAAMnC,GAYV,SAAJoe,SAGEjK,EAFG9N,EAAOmR,EACVoW,EAAehM,EAAK3V,gBAErB5F,GAAQA,IAASub,GAAQvb,EAAKqU,KAAKnQ,KAAKqX,GACxC5hB,IAAU4hB,EAAK3V,SAAWA,GAASjM,IACnCwX,EAAWoK,EACXzN,EAAShS,EAAK6S,MAAM4M,EAAM7B,WAC1BxgB,EAAY4U,IAAWyN,EAAKiM,GAAGtjB,KAAK4J,GACpCqD,EAAWnR,EACXub,EAAK3V,SAAW2hB,EAChBhM,EAAKkM,YAAa,EACX3Z,EAlBL5U,EAAYsB,KACfb,EAAQmC,EACRA,EAAOtB,EACPA,EAAOtB,OAEJqiB,EAAOjC,YAeXiC,EAAK0K,KAAOlO,GACLvd,IAAStB,EAAc6e,GAAEwD,EAAM,SAAAzf,UAAQyf,EAAK9Y,IAAI,KAAM3G,KAAStB,EAAQ+gB,EAAK/gB,GAAQud,GAAKA,OAEjG2P,OAAA,gBAAO5rB,OACFkE,EAAOmR,EACXA,EAAW,KACXrV,EAAKwd,MACLnI,EAAWnR,MAEZ2nB,UAAA,yBACKvqB,EAAI,eACHiX,KAAKrY,QAAQ,SAAAilB,UAAMA,aAAaqG,QAAWlqB,EAAE8G,WAAF9G,EAAU6jB,EAAE0G,aAAgB1G,aAAa9b,MAAY8b,EAAE/hB,QAA4B,WAAlB+hB,EAAE/hB,OAAOmV,OAAsBjX,EAAE8G,KAAK+c,KAChJ7jB,MAER+iB,MAAA,sBACMqH,GAAGrsB,OAASme,KAAKjF,KAAKlZ,OAAS,MAErCmP,KAAA,cAAKrJ,EAAQkmB,iBACRlmB,qBAGFlB,EAFGme,EAAS0J,EAAKD,YACjB7sB,EAAI8sB,EAAKvT,KAAKlZ,OAERL,KAES,YADfiF,EAAI6nB,EAAKvT,KAAKvZ,IACRuZ,OACLtU,EAAEkB,SACFlB,EAAEke,aAAY,GAAM,GAAM,GAAOjiB,QAAQ,SAAAkB,UAASghB,EAAO5iB,OAAO4iB,EAAOlhB,QAAQE,GAAQ,UAIzFghB,EAAO7Q,IAAI,SAAAtN,SAAc,CAAC+M,EAAG/M,EAAEa,MAAQb,EAAEkD,QAAWlD,EAAEsa,OAASta,EAAEsa,KAAKpb,KAAKkC,gBAAmBpB,EAAEqa,WAAW,IAAK,EAAA,EAAWra,EAAAA,KAAKqG,KAAK,SAAChJ,EAAGgL,UAAMA,EAAE0E,EAAI1P,EAAE0P,IAAK,EAAA,IAAW9Q,QAAQ,SAAA6rB,UAAKA,EAAE9nB,EAAEkB,OAAOA,KAC/LnG,EAAI8sB,EAAKvT,KAAKlZ,OACPL,MACNiF,EAAI6nB,EAAKvT,KAAKvZ,cACG4J,GACD,WAAX3E,EAAEsU,OACLtU,EAAEsK,eAAiBtK,EAAEsK,cAAcpJ,SACnClB,EAAEuK,QAGDvK,aAAaoF,KAAUpF,EAAEkB,QAAUlB,EAAEkB,OAAOA,GAGhD2mB,EAAKJ,GAAGxrB,QAAQ,SAAA+b,UAAKA,EAAE9W,EAAQ2mB,KAC/BA,EAAKH,YAAa,UAEbpT,KAAKrY,QAAQ,SAAAilB,UAAKA,EAAE3W,MAAQ2W,EAAE3W,cAE/B6V,QACDgH,UACCrsB,EAAIgsB,GAAO3rB,OACRL,KACNgsB,GAAOhsB,GAAGue,KAAOC,KAAKD,IAAMyN,GAAOxrB,OAAOR,EAAG,OAUhDmG,OAAA,gBAAOyJ,QACDJ,KAAKI,GAAU,+BAjGT5O,EAAMnC,QACZiM,SAAWjM,GAASiM,GAASjM,QAC7B0a,KAAO,QACPmT,GAAK,QACLC,YAAa,OACbpO,GAAKgO,KACVvrB,GAAQwd,KAAK7W,IAAI3G,UAkGbgsB,8BAMLrlB,IAAA,aAAIykB,EAAYprB,EAAMnC,GACrBN,EAAU6tB,KAAgBA,EAAa,CAACN,QAASM,QAGhDa,EAAIxpB,EAAGypB,EAFJnS,EAAU,IAAIyR,GAAQ,EAAG3tB,GAAS2f,KAAK3f,OAC1CsuB,EAAOpS,EAAQqR,WAAa,OAMxB3oB,KAJL4S,IAAa0E,EAAQjQ,WAAaiQ,EAAQjQ,SAAWuL,EAASvL,eACzDsiB,SAAShkB,KAAK2R,GACnB/Z,EAAO+Z,EAAQpT,IAAI,UAAW3G,GAC9B+Z,EAAQoR,QAAUC,EAEP,QAAN3oB,EACHypB,EAAS,GAETD,EAAKpiB,EAAKwhB,WAAWD,EAAW3oB,OAE/BuoB,GAAO9pB,QAAQ6Y,GAAW,GAAKiR,GAAO5iB,KAAK2R,IAC1CoS,EAAK1pB,GAAKwpB,EAAGnB,WAAaoB,EAAS,GACpCD,EAAGI,YAAcJ,EAAGI,YAAYxB,IAAkBoB,EAAGK,iBAAiB,SAAUzB,YAInFqB,GAAUlsB,EAAK+Z,EAAS,SAAAkC,UAAKlC,EAAQpT,IAAI,KAAMsV,KACxCuB,SAWRrY,OAAA,gBAAOyJ,QACDJ,KAAKI,GAAU,QAErBJ,KAAA,cAAKrJ,QACCinB,SAASlsB,QAAQ,SAAAwR,UAAKA,EAAElD,KAAKrJ,GAAQ,sCA1C/BtH,QACNuuB,SAAW,QACXvuB,MAAQA,EACbwX,GAAYA,EAASkD,KAAKnQ,KAAKoV,MAkDjC,IAAMte,GAAQ,CACbqtB,oEAAkBC,2BAAAA,kBACjBA,EAAKtsB,QAAQ,SAAA0O,UAAUD,GAAcC,MAEtClI,2BAASvD,UACD,IAAIyF,GAASzF,IAErB4f,iCAAYjkB,EAASgkB,UACbzf,EAAgB0f,YAAYjkB,EAASgkB,IAE7C2J,iCAAYxtB,EAAQd,EAAUuuB,EAAMC,GACnCzvB,EAAU+B,KAAYA,EAASS,GAAQT,GAAQ,QAC3C2tB,EAASntB,GAAUR,GAAU,IAAIyQ,IACpCmd,EAASH,EAAOlqB,GAAeL,SACvB,WAATuqB,IAAsBA,EAAO,IACrBztB,EAAmBd,EAA8I0uB,GAAS7c,GAAS7R,IAAa6R,GAAS7R,GAAUuR,KAAQkd,GAAQ3tB,EAAQd,EAAUuuB,EAAMC,IAA7N,SAACxuB,EAAUuuB,EAAMC,UAAYE,GAAS7c,GAAS7R,IAAa6R,GAAS7R,GAAUuR,KAAQkd,GAAQ3tB,EAAQd,EAAUuuB,EAAMC,KAA5I1tB,GAElB6tB,iCAAY7tB,EAAQd,EAAUuuB,MAET,GADpBztB,EAASS,GAAQT,IACNI,OAAY,KAClB0tB,EAAU9tB,EAAOsS,IAAI,SAAAtN,UAAKhG,GAAK6uB,YAAY7oB,EAAG9F,EAAUuuB,KAC3DzrB,EAAI8rB,EAAQ1tB,cACN,SAAAlC,WACF6B,EAAIiC,EACFjC,KACL+tB,EAAQ/tB,GAAG7B,IAId8B,EAASA,EAAO,IAAM,OAClB8P,EAASiB,GAAS7R,GACrB0M,EAAQpL,GAAUR,GAClBwD,EAAKoI,EAAM1L,UAAY0L,EAAM1L,QAAQ0Q,SAAW,IAAI1R,IAAcA,EAClEunB,EAAS3W,EAAS,SAAA5R,OACbsF,EAAI,IAAIsM,EACZyG,EAAYzN,IAAM,EAClBtF,EAAEuM,KAAK/P,EAAQytB,EAAOvvB,EAAQuvB,EAAOvvB,EAAOqY,EAAa,EAAG,CAACvW,IAC7DwD,EAAEf,OAAO,EAAGe,GACZ+S,EAAYzN,KAAOoH,GAAkB,EAAGqG,IACrC3K,EAAM4S,IAAIxe,EAAQwD,UAChBsM,EAAS2W,EAAS,SAAAvoB,UAASuoB,EAAOzmB,EAAQwD,EAAGiqB,EAAOvvB,EAAQuvB,EAAOvvB,EAAO0N,EAAO,KAEzFmiB,yBAAQ/tB,EAAQd,EAAUgF,GAEjB,SAAPnD,GAAQ7C,EAAOqD,EAAO8nB,UAAoBlnB,EAAMinB,QAAQlqB,EAAUhB,EAAOqD,EAAO8nB,SAD7ElnB,EAAQnD,GAAKiiB,GAAGjhB,EAAQyD,WAAevE,GAAW,UAAS+f,QAAQ,IAAMsC,QAAS,KAAIrd,GAAQ,YAElGnD,GAAKoB,MAAQA,EACNpB,IAERitB,+BAAWnuB,UACiD,EAApDuE,EAAgB0f,YAAYjkB,GAAS,GAAMO,QAEnDsD,2BAASxF,UACRA,GAASA,EAAMuN,OAASvN,EAAMuN,KAAOC,GAAWxN,EAAMuN,KAAMqM,EAAUrM,OAC/D9H,GAAWmU,EAAW5Z,GAAS,KAEvCyR,uBAAOzR,UACCyF,GAAW6T,EAAStZ,GAAS,KAErC+vB,8CAAgBxuB,IAAAA,KAAMyuB,IAAAA,OAAQC,IAAAA,QAASzqB,IAAAA,SAAU0qB,IAAAA,gBAC/CD,GAAW,IAAIntB,MAAM,KAAKC,QAAQ,SAAAotB,UAAcA,IAAetd,GAASsd,KAAgBtvB,GAASsvB,IAAehvB,EAAMI,EAAO,oBAAsB4uB,EAAa,cACjKpV,GAASxZ,GAAQ,SAACI,EAASqE,EAAMogB,UAAO4J,EAAOztB,GAAQZ,GAAU4D,GAAaS,GAAQ,GAAIR,GAAW4gB,IACjG8J,IACHzkB,GAASqH,UAAUvR,GAAQ,SAASI,EAASqE,EAAM6D,UAC3CwW,KAAK7W,IAAIuR,GAASxZ,GAAMI,EAASvB,EAAU4F,GAAQA,GAAQ6D,EAAW7D,IAAS,GAAIqa,MAAOxW,MAIpGumB,mCAAa7uB,EAAMgM,GAClBkI,GAASlU,GAAQiM,GAAWD,IAE7B8iB,6BAAU9iB,EAAMiS,UACRiB,UAAUve,OAASsL,GAAWD,EAAMiS,GAAe/J,IAE3D2P,yBAAQhF,UACAla,EAAgBkf,QAAQhF,IAEhCkQ,+BAAWtqB,EAAWuqB,YAAXvqB,IAAAA,EAAO,QAEhBU,EAAOS,EADJif,EAAK,IAAI3a,GAASzF,OAEtBogB,EAAGhd,kBAAoB/I,EAAY2F,EAAKoD,mBACxClD,EAAgBqB,OAAO6e,GACvBA,EAAG5f,IAAM,EACT4f,EAAG/c,MAAQ+c,EAAG7d,OAASrC,EAAgBmD,MACvC3C,EAAQR,EAAgBwQ,OACjBhQ,GACNS,EAAOT,EAAMO,OACTspB,IAA0B7pB,EAAMiB,MAAQjB,aAAiBwF,IAASxF,EAAMV,KAAKwd,aAAe9c,EAAMuf,SAAS,IAC9Grc,GAAewc,EAAI1f,EAAOA,EAAMkB,OAASlB,EAAMsD,QAEhDtD,EAAQS,SAETyC,GAAe1D,EAAiBkgB,EAAI,GAC7BA,GAERxJ,QAAS,iBAAC/Z,EAAMnC,UAAUmC,EAAO,IAAIwrB,GAAQxrB,EAAMnC,GAASwX,GAC5DgW,WAAY,oBAAAxtB,UAAS,IAAImuB,GAAWnuB,IACpC8vB,kBAAmB,oCAAM3C,GAAO9qB,QAAQ,SAAAwR,OAEtCkc,EAAOnrB,EADJ0pB,EAAOza,EAAE0Z,eAER3oB,KAAK0pB,EACLA,EAAK1pB,KACR0pB,EAAK1pB,IAAK,EACVmrB,EAAQ,GAGVA,GAASlc,EAAEvM,YACN0lB,MACNyB,2CAAiBxjB,EAAM+Q,OAClBvY,EAAIiV,GAAWzN,KAAUyN,GAAWzN,GAAQ,KAC/CxH,EAAEJ,QAAQ2Y,IAAavY,EAAE8G,KAAKyR,IAEhCgU,iDAAoB/kB,EAAM+Q,OACrBvY,EAAIiV,GAAWzN,GAClB9J,EAAIsC,GAAKA,EAAEJ,QAAQ2Y,GACf,GAAL7a,GAAUsC,EAAE9B,OAAOR,EAAG,IAEvB8uB,MAAO,CAAEC,KA3iFF,SAAPA,KAAgBliB,EAAKD,EAAKzO,OACrB6wB,EAAQpiB,EAAMC,SACXpI,EAASoI,GAAO4B,GAAW5B,EAAKkiB,KAAK,EAAGliB,EAAIxM,QAASuM,GAAOtC,GAAmBnM,EAAO,SAAAA,UAAW6wB,GAAS7wB,EAAQ0O,GAAOmiB,GAASA,EAASniB,KAyiFpIoiB,SAviFJ,SAAXA,SAAYpiB,EAAKD,EAAKzO,OACjB6wB,EAAQpiB,EAAMC,EACjBqiB,EAAgB,EAARF,SACFvqB,EAASoI,GAAO4B,GAAW5B,EAAKoiB,SAAS,EAAGpiB,EAAIxM,OAAS,GAAIuM,GAAOtC,GAAmBnM,EAAO,SAAAA,UAE7F0O,GAAgBmiB,GADvB7wB,GAAS+wB,GAAS/wB,EAAQ0O,GAAOqiB,GAASA,GAAS,GAClBA,EAAQ/wB,EAASA,MAkiF3BqN,WAAAA,GAAYD,OAAAA,GAAQqC,KAAAA,GAAMuhB,UA7iFvC,SAAZA,UAAatiB,EAAKD,EAAKzO,UAAUkc,GAASxN,EAAKD,EAAK,EAAG,EAAGzO,IA6iFIoM,QAAAA,GAAS6kB,MAnqF/D,SAARA,MAASviB,EAAKD,EAAKzO,UAAUmM,GAAmBnM,EAAO,SAAAyC,UAAKiH,GAAOgF,EAAKD,EAAKhM,MAmqFCgR,WAAAA,GAAYlR,QAAAA,GAASoK,SAAAA,GAAUuP,SAAAA,GAAUgV,KA/iFhH,SAAPA,kCAAWC,2BAAAA,yBAAc,SAAAnxB,UAASmxB,EAAUC,OAAO,SAAC3uB,EAAGqc,UAAMA,EAAErc,IAAIzC,KA+iF0DqxB,QA9iFnH,SAAVA,QAAWxuB,EAAM0sB,UAAS,SAAAvvB,UAAS6C,EAAKY,WAAWzD,KAAWuvB,GAAQnjB,GAAQpM,MA8iFwDsxB,YA7gFxH,SAAdA,YAAejuB,EAAOG,EAAK8N,EAAUigB,OAChC1uB,EAAOgL,MAAMxK,EAAQG,GAAO,EAAI,SAAA8B,UAAM,EAAIA,GAAKjC,EAAQiC,EAAI9B,OAC1DX,EAAM,KAGTyC,EAAGzD,EAAG2vB,EAAe1tB,EAAG2tB,EAFrBC,EAAW3xB,EAAUsD,GACxBsuB,EAAS,OAEG,IAAbrgB,IAAsBigB,EAAS,KAAOjgB,EAAW,MAC7CogB,EACHruB,EAAQ,CAACiC,EAAGjC,GACZG,EAAM,CAAC8B,EAAG9B,QAEJ,GAAI8C,EAASjD,KAAWiD,EAAS9C,GAAM,KAC7CguB,EAAgB,GAChB1tB,EAAIT,EAAMnB,OACVuvB,EAAK3tB,EAAI,EACJjC,EAAI,EAAGA,EAAIiC,EAAGjC,IAClB2vB,EAAcvmB,KAAKqmB,YAAYjuB,EAAMxB,EAAE,GAAIwB,EAAMxB,KAElDiC,IACAjB,EAAO,cAAAyC,GACNA,GAAKxB,MACDjC,EAAIoB,KAAKyL,IAAI+iB,IAAMnsB,UAChBksB,EAAc3vB,GAAGyD,EAAIzD,IAE7ByP,EAAW9N,OACA+tB,IACXluB,EAAQzC,GAAO0F,EAASjD,GAAS,GAAK,GAAIA,QAEtCmuB,EAAe,KACdlsB,KAAK9B,EACTyO,GAAcgK,KAAK0V,EAAQtuB,EAAOiC,EAAG,MAAO9B,EAAI8B,IAEjDzC,EAAO,cAAAyC,UAAK0M,GAAkB1M,EAAGqsB,KAAYD,EAAWruB,EAAMiC,EAAIjC,YAG7D8I,GAAmBmF,EAAUzO,IA0+E8GqK,QAAAA,IACnJ0kB,QAASnxB,EACToxB,QAAS9W,GACT+W,OAAQ/mB,GACRqc,WAAY3b,GAAS2b,WACrB6I,QAASpd,GACTkf,eAAgB7rB,EAChB8rB,KAAM,CAAC9e,UAAAA,GAAW+e,QAAS3wB,EAAY4K,MAAAA,GAAOT,SAAAA,GAAU8U,UAAAA,GAAW2R,SAAU5vB,GAAW4E,sBAAAA,GAAuBirB,UAAW,4BAAMttB,GAAY+X,QAAS,iBAAAwV,UAAcA,GAASla,IAAYA,EAASkD,KAAKnQ,KAAKmnB,GAAQA,EAAMvV,KAAO3E,GAAiBA,GAAama,mBAAoB,4BAAAryB,UAASiY,EAAsBjY,KAGlT2C,GAAa,8CAA+C,SAAApB,UAAQQ,GAAMR,GAAQ2K,GAAM3K,KACxFwJ,GAAQvB,IAAIiC,GAAS2b,YACrB/O,EAActW,GAAMghB,GAAG,GAAI,CAACva,SAAS,IAQX,SAAtB8pB,GAAuBhL,EAAQvU,WAC7BwU,EAAKD,EAAO1c,IACT2c,GAAMA,EAAGjiB,IAAMyN,GAAQwU,EAAGqF,KAAO7Z,GAAQwU,EAAG6B,KAAOrW,GACzDwU,EAAKA,EAAGtgB,aAEFsgB,EAkBe,SAAvBgL,GAAwBhxB,EAAM4Q,SACtB,CACN5Q,KAAMA,EACN8Q,QAAS,EACTR,mBAAK/P,EAAQkE,EAAM/B,GAClBA,EAAMmmB,QAAU,SAAAnmB,OACXuuB,EAAMltB,KACNvF,EAAUiG,KACbwsB,EAAO,GACP7vB,GAAaqD,EAAM,SAAAzE,UAAQixB,EAAKjxB,GAAQ,IACxCyE,EAAOwsB,GAEJrgB,EAAU,KAER7M,KADLktB,EAAO,GACGxsB,EACTwsB,EAAKltB,GAAK6M,EAASnM,EAAKV,IAEzBU,EAAOwsB,GAjCI,SAAhBC,cAAiBxuB,EAAOyuB,OAErBptB,EAAGzD,EAAG0lB,EADH5lB,EAAUsC,EAAMgiB,aAEf3gB,KAAKotB,MACT7wB,EAAIF,EAAQO,OACLL,MAEK0lB,GADXA,EAAKtjB,EAAMyc,UAAU7e,GAAGyD,KACRiiB,EAAGhZ,KACdgZ,EAAG3c,MACN2c,EAAK+K,GAAoB/K,EAAIjiB,IAE9BiiB,GAAMA,EAAGpV,UAAYoV,EAAGpV,SAASugB,EAAUptB,GAAIrB,EAAOtC,EAAQE,GAAIyD,IAwBnEmtB,CAAcxuB,EAAO+B,MA1C1B,IAiDalF,GAAOiB,GAAMqtB,eAAe,CACvC7tB,KAAK,OACLsQ,mBAAK/P,EAAQkE,EAAM/B,EAAOuM,EAAO7O,OAC5B2D,EAAGiiB,EAAI9kB,MAEN6C,UADArB,MAAQA,EACH+B,EACTvD,EAAIX,EAAOY,aAAa4C,IAAM,IAC9BiiB,EAAKlH,KAAK7W,IAAI1H,EAAQ,gBAAiBW,GAAK,GAAK,GAAIuD,EAAKV,GAAIkL,EAAO7O,EAAS,EAAG,EAAG2D,IACjFsnB,GAAKtnB,EACRiiB,EAAGpY,EAAI1M,OACFqP,OAAO7G,KAAK3F,IAGnBf,uBAAOqe,EAAOxH,WACTmM,EAAKnM,EAAKxQ,IACP2c,GACN1iB,EAAa0iB,EAAGjH,IAAIiH,EAAGzgB,EAAGygB,EAAGjiB,EAAGiiB,EAAGpY,EAAGoY,GAAMA,EAAG3T,EAAEgP,EAAO2E,EAAGhZ,GAC3DgZ,EAAKA,EAAGtgB,QAGR,CACF1F,KAAK,WACLsQ,mBAAK/P,EAAQ9B,WACR6B,EAAI7B,EAAMkC,OACPL,UACD2H,IAAI1H,EAAQD,EAAGC,EAAOD,IAAM,EAAG7B,EAAM6B,GAAI,EAAG,EAAG,EAAG,EAAG,EAAG,KAIhE0wB,GAAqB,aAAcjjB,IACnCijB,GAAqB,aACrBA,GAAqB,OAAQ9iB,MACzB1N,GAELmK,GAAMwS,QAAUjT,GAASiT,QAAU5d,GAAK4d,QAAU,SAClDtG,EAAa,EACb9X,KAAmBsS,KCpqGD,SAAjB+f,GAAkB/P,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAIrC,KAAKC,MAAkC,KAA3BkY,EAAKzK,EAAIyK,EAAK7G,EAAIqO,IAAkB,IAASxH,EAAKhM,EAAGgM,GACxG,SAArBwX,GAAsBhQ,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAa,IAAVsd,EAAcxH,EAAK4M,EAAK/kB,KAAKC,MAAkC,KAA3BkY,EAAKzK,EAAIyK,EAAK7G,EAAIqO,IAAkB,IAASxH,EAAKhM,EAAGgM,GAC1H,SAA9ByX,GAA+BjQ,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAGsd,EAAS3f,KAAKC,MAAkC,KAA3BkY,EAAKzK,EAAIyK,EAAK7G,EAAIqO,IAAkB,IAASxH,EAAKhM,EAAIgM,EAAKjM,EAAGiM,GACnI,SAAxB0X,GAAyBlQ,EAAOxH,OAC3Bpb,EAAQob,EAAKzK,EAAIyK,EAAK7G,EAAIqO,EAC9BxH,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,KAAMtF,GAASA,EAAQ,GAAK,GAAK,KAAOob,EAAKhM,EAAGgM,GAE7C,SAA1B2X,GAA2BnQ,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAGsd,EAAQxH,EAAK4M,EAAI5M,EAAKjM,EAAGiM,GAC1D,SAAnC4X,GAAoCpQ,EAAOxH,UAASA,EAAKkF,IAAIlF,EAAKtU,EAAGsU,EAAK9V,EAAa,IAAVsd,EAAcxH,EAAKjM,EAAIiM,EAAK4M,EAAG5M,GAC1F,SAAlB6X,GAAmBnxB,EAAQd,EAAUhB,UAAU8B,EAAO6lB,MAAM3mB,GAAYhB,EACvD,SAAjBkzB,GAAkBpxB,EAAQd,EAAUhB,UAAU8B,EAAO6lB,MAAMwL,YAAYnyB,EAAUhB,GAC9D,SAAnBozB,GAAoBtxB,EAAQd,EAAUhB,UAAU8B,EAAOC,MAAMf,GAAYhB,EAC1D,SAAfqzB,GAAgBvxB,EAAQd,EAAUhB,UAAU8B,EAAOC,MAAMuxB,OAASxxB,EAAOC,MAAMwxB,OAASvzB,EAC/D,SAAzBwzB,GAA0B1xB,EAAQd,EAAUhB,EAAOob,EAAMwH,OACpDlV,EAAQ5L,EAAOC,MACnB2L,EAAM4lB,OAAS5lB,EAAM6lB,OAASvzB,EAC9B0N,EAAM+lB,gBAAgB7Q,EAAOlV,GAED,SAA7BgmB,GAA8B5xB,EAAQd,EAAUhB,EAAOob,EAAMwH,OACxDlV,EAAQ5L,EAAOC,MACnB2L,EAAM1M,GAAYhB,EAClB0N,EAAM+lB,gBAAgB7Q,EAAOlV,GAIjB,SAAbimB,GAAsB3yB,EAAU4yB,cAC3B9xB,EAASue,KAAKve,OACjB6lB,EAAQ7lB,EAAO6lB,MACfja,EAAQ5L,EAAOC,SACXf,KAAY6yB,IAAoBlM,EAAO,SACtCmM,IAAMzT,KAAKyT,KAAO,GACN,cAAb9yB,SAKI+yB,GAAiBC,UAAUlxB,MAAM,KAAKC,QAAQ,SAAAuC,UAAKquB,GAAW1X,KAAK6G,EAAMxd,EAAGsuB,UAJnF5yB,EAAW+yB,GAAiB/yB,IAAaA,GAC/B+C,QAAQ,KAAO/C,EAAS8B,MAAM,KAAKC,QAAQ,SAAAoB,UAAK2e,EAAKgR,IAAI3vB,GAAK8vB,GAAKnyB,EAAQqC,KAAOkc,KAAKyT,IAAI9yB,GAAY0M,EAAMW,EAAIX,EAAM1M,GAAYizB,GAAKnyB,EAAQd,GAC1JA,IAAakzB,KAAyB7T,KAAKyT,IAAIK,QAAUzmB,EAAMymB,SAItB,GAAtC9T,KAAKqF,MAAM3hB,QAAQqwB,WACnB1mB,EAAM2mB,WACJC,KAAOxyB,EAAOY,aAAa,wBAC3BgjB,MAAMza,KAAKipB,GAAsBN,EAAU,KAEjD5yB,EAAWozB,IAEXzM,GAASiM,IAAavT,KAAKqF,MAAMza,KAAKjK,EAAU4yB,EAAUjM,EAAM3mB,IAEnC,SAA/BuzB,GAA+B5M,GAC1BA,EAAM6M,YACT7M,EAAM8M,eAAe,aACrB9M,EAAM8M,eAAe,SACrB9M,EAAM8M,eAAe,WAGR,SAAfC,SAKE7yB,EAAGyD,EAJAogB,EAAQrF,KAAKqF,MAChB5jB,EAASue,KAAKve,OACd6lB,EAAQ7lB,EAAO6lB,MACfja,EAAQ5L,EAAOC,UAEXF,EAAI,EAAGA,EAAI6jB,EAAMxjB,OAAQL,GAAG,EAC3B6jB,EAAM7jB,EAAE,GAEa,IAAf6jB,EAAM7jB,EAAE,GAClBC,EAAO4jB,EAAM7jB,IAAI6jB,EAAM7jB,EAAE,IAEzBC,EAAO4jB,EAAM7jB,IAAM6jB,EAAM7jB,EAAE,GAJ3B6jB,EAAM7jB,EAAE,GAAM8lB,EAAMjC,EAAM7jB,IAAM6jB,EAAM7jB,EAAE,GAAM8lB,EAAM8M,eAAwC,OAAzB/O,EAAM7jB,GAAG6B,OAAO,EAAE,GAAcgiB,EAAM7jB,GAAK6jB,EAAM7jB,GAAGoT,QAAQ0f,GAAU,OAAOvd,kBAO9IiJ,KAAKyT,IAAK,KACRxuB,KAAK+a,KAAKyT,IACdpmB,EAAMpI,GAAK+a,KAAKyT,IAAIxuB,GAEjBoI,EAAM2mB,MACT3mB,EAAM+lB,kBACN3xB,EAAOyqB,aAAa,kBAAmBlM,KAAKiU,MAAQ,MAErDzyB,EAAIgD,OACQhD,EAAEgZ,SAAa8M,EAAMyM,MAChCG,GAA6B5M,GACzBja,EAAMymB,SAAWxM,EAAMuM,MAC1BvM,EAAMuM,KAAyB,IAAMxmB,EAAMymB,QAAU,KACrDzmB,EAAMymB,QAAU,EAChBzmB,EAAM+lB,mBAEP/lB,EAAM8hB,QAAU,IAIF,SAAjBoF,GAAkB9yB,EAAQ+yB,OACrBC,EAAQ,CACXhzB,OAAAA,EACA4jB,MAAO,GACP1d,OAAQ0sB,GACRK,KAAMpB,WAEP7xB,EAAOC,OAASjB,GAAKkxB,KAAKE,SAASpwB,GACnC+yB,GAAc/yB,EAAO6lB,OAAS7lB,EAAO2K,UAAYooB,EAAW/xB,MAAM,KAAKC,QAAQ,SAAAuC,UAAKwvB,EAAMC,KAAKzvB,KACxFwvB,EAGS,SAAjBE,GAAkBrpB,EAAMspB,OACnBjN,EAAIhb,GAAKkoB,gBAAkBloB,GAAKkoB,iBAAiBD,GAAM,gCAAgChgB,QAAQ,SAAU,QAAStJ,GAAQqB,GAAKC,cAActB,UAC1Iqc,GAAKA,EAAEL,MAAQK,EAAIhb,GAAKC,cAActB,GAEvB,SAAvBwpB,GAAwBrzB,EAAQd,EAAUo0B,OACrCC,EAAKC,iBAAiBxzB,UACnBuzB,EAAGr0B,IAAaq0B,EAAGE,iBAAiBv0B,EAASiU,QAAQ0f,GAAU,OAAOvd,gBAAkBie,EAAGE,iBAAiBv0B,KAAeo0B,GAAsBD,GAAqBrzB,EAAQ0zB,GAAiBx0B,IAAaA,EAAU,IAAO,GAczN,SAAZy0B,MAnIgB,SAAhBn1B,sBAAyC,oBAAZC,QAoIxBD,IAAmBC,OAAOie,WAC7B9R,GAAOnM,OACPyM,GAAON,GAAK8R,SACZkX,GAAc1oB,GAAK2oB,gBACnBC,GAAWZ,GAAe,QAAU,CAACrN,MAAM,IAC1BqN,GAAe,OAChCZ,GAAiBoB,GAAiBpB,IAClCF,GAAuBE,GAAiB,SACxCwB,GAASjO,MAAMkO,QAAU,2DACzBC,KAAgBN,GAAiB,eACjC3wB,GAAa/D,GAAKkxB,KAAKG,UACvB4D,GAAiB,GAGO,SAA1BC,GAA0Bl0B,OAIxBm0B,EAHGC,EAAQp0B,EAAOq0B,gBAClB9B,EAAMW,GAAe,MAAQkB,GAASA,EAAMxzB,aAAa,UAAa,8BACtE0zB,EAAQt0B,EAAOu0B,WAAU,GAE1BD,EAAMzO,MAAM2O,QAAU,QACtBjC,EAAIkC,YAAYH,GAChBV,GAAYa,YAAYlC,OAEvB4B,EAAOG,EAAMI,UACZ,MAAOxO,WACTqM,EAAIoC,YAAYL,GAChBV,GAAYe,YAAYpC,GACjB4B,EAEiB,SAAzBS,GAA0B50B,EAAQ60B,WAC7B90B,EAAI80B,EAAgBz0B,OACjBL,QACFC,EAAO80B,aAAaD,EAAgB90B,WAChCC,EAAOY,aAAai0B,EAAgB90B,IAInC,SAAXg1B,GAAW/0B,OACNg1B,EAAQC,MAEXD,EAASh1B,EAAO00B,UACf,MAAOQ,GACRF,EAASd,GAAwBl0B,GACjCi1B,EAAS,SAETD,IAAWA,EAAOG,OAASH,EAAOI,SAAYH,IAAWD,EAASd,GAAwBl0B,KAEnFg1B,GAAWA,EAAOG,OAAUH,EAAOzoB,GAAMyoB,EAAOxoB,EAA8IwoB,EAAzI,CAACzoB,GAAIqoB,GAAuB50B,EAAQ,CAAC,IAAI,KAAK,QAAU,EAAGwM,GAAGooB,GAAuB50B,EAAQ,CAAC,IAAI,KAAK,QAAU,EAAGm1B,MAAM,EAAGC,OAAO,GAEzL,SAATC,GAASnP,YAAQA,EAAEoP,QAAYpP,EAAEqP,aAAcrP,EAAEmO,kBAAoBU,GAAS7O,IAC5D,SAAlBsP,GAAmBx1B,EAAQd,MACtBA,EAAU,KAEZu2B,EADG5P,EAAQ7lB,EAAO6lB,MAEf3mB,KAAY6yB,IAAmB7yB,IAAakzB,KAC/ClzB,EAAWozB,IAERzM,EAAM8M,gBAEW,QADpB8C,EAAcv2B,EAAS0C,OAAO,EAAE,KACqB,WAAzB1C,EAAS0C,OAAO,EAAE,KAC7C1C,EAAW,IAAMA,GAElB2mB,EAAM8M,eAA+B,OAAhB8C,EAAuBv2B,EAAWA,EAASiU,QAAQ0f,GAAU,OAAOvd,gBAEzFuQ,EAAM6P,gBAAgBx2B,IAIL,SAApBy2B,GAAqBnQ,EAAQxlB,EAAQd,EAAU02B,EAAWl0B,EAAKm0B,OAC1DpQ,EAAK,IAAIrU,GAAUoU,EAAO1c,IAAK9I,EAAQd,EAAU,EAAG,EAAG22B,EAAe3E,GAAmCD,WAC7GzL,EAAO1c,IAAM2c,GACVpY,EAAIuoB,EACPnQ,EAAGS,EAAIxkB,EACP8jB,EAAOxV,OAAO7G,KAAKjK,GACZumB,EAKS,SAAjBqQ,GAAkB91B,EAAQd,EAAUhB,EAAOuvB,OAUzCsI,EAAI5xB,EAAQyH,EAAOoqB,EAThBC,EAAWt0B,WAAWzD,IAAU,EACnCg4B,GAAWh4B,EAAQ,IAAIoF,OAAO1B,QAAQq0B,EAAW,IAAI71B,SAAW,KAChEylB,EAAQiO,GAASjO,MACjBsQ,EAAaC,GAAe5iB,KAAKtU,GACjCm3B,EAA6C,QAAjCr2B,EAAOs2B,QAAQhhB,cAC3BihB,GAAmBF,EAAY,SAAW,WAAaF,EAAa,QAAU,UAE9EK,EAAoB,OAAT/I,EACXgJ,EAAqB,MAAThJ,KAETA,IAASyI,IAAYD,GAAYS,GAAqBjJ,IAASiJ,GAAqBR,UAChFD,KAEK,OAAZC,GAAqBM,IAAcP,EAAWH,GAAe91B,EAAQd,EAAUhB,EAAO,OACvF83B,EAAQh2B,EAAOs1B,QAAUD,GAAOr1B,IAC3By2B,GAAyB,MAAZP,KAAqBnE,GAAgB7yB,KAAcA,EAAS+C,QAAQ,iBACrF8zB,EAAKC,EAAQh2B,EAAO00B,UAAUyB,EAAa,QAAU,UAAYn2B,EAAOu2B,GACjEr1B,GAAOu1B,EAAYR,EAAWF,EAX5B,IAW0CE,EAAW,IAAMF,MAErElQ,EAAMsQ,EAAa,QAAU,UAbnB,KAayCK,EAAWN,EAAUzI,GACxEtpB,EAAoB,QAATspB,IAAmBvuB,EAAS+C,QAAQ,UAAuB,OAATwrB,GAAiBztB,EAAOy0B,cAAgB4B,EAAcr2B,EAASA,EAAOu1B,WAC/HS,IACH7xB,GAAUnE,EAAOq0B,iBAAmB,IAAIkB,YAEpCpxB,GAAUA,IAAW+G,IAAS/G,EAAOswB,cACzCtwB,EAAS+G,GAAKyrB,OAEf/qB,EAAQzH,EAAOlE,QACFw2B,GAAa7qB,EAAMupB,OAASgB,GAAcvqB,EAAMhJ,OAASqG,GAAQrG,OAASgJ,EAAM8hB,eACrFxsB,GAAO+0B,EAAWrqB,EAAMupB,MAvBtB,SAyBLsB,GAA2B,WAAbv3B,GAAsC,UAAbA,GAMzCu3B,GAAyB,MAAZP,GAAqBU,GAAoBvD,GAAqBlvB,EAAQ,cAAgB0hB,EAAM9d,SAAWsrB,GAAqBrzB,EAAQ,aACjJmE,IAAWnE,IAAY6lB,EAAM9d,SAAW,UACzC5D,EAAOswB,YAAYX,IACnBiC,EAAKjC,GAASyC,GACdpyB,EAAOwwB,YAAYb,IACnBjO,EAAM9d,SAAW,eAXgD,KAC7DpH,EAAIX,EAAO6lB,MAAM3mB,GACrBc,EAAO6lB,MAAM3mB,GA3BL,IA2B0BuuB,EAClCsI,EAAK/1B,EAAOu2B,GACZ51B,EAAKX,EAAO6lB,MAAM3mB,GAAYyB,EAAK60B,GAAgBx1B,EAAQd,UASxDi3B,GAAcM,KACjB7qB,EAAQpL,GAAU2D,IACZvB,KAAOqG,GAAQrG,KACrBgJ,EAAMupB,MAAQhxB,EAAOoyB,IAGhBr1B,GAAOs1B,EAAWT,EAAKE,EA5CpB,IA4CwCF,GAAME,EA5C9C,IA4CkEF,EAAKE,EAAW,GAuBpE,SAAzBY,GAAkC72B,EAAQiR,EAAM1P,EAAOG,OACjDH,GAAmB,SAAVA,EAAkB,KAC3BiC,EAAIkwB,GAAiBziB,EAAMjR,EAAQ,GACtC6O,EAAIrL,GAAK6vB,GAAqBrzB,EAAQwD,EAAG,GACtCqL,GAAKA,IAAMtN,GACd0P,EAAOzN,EACPjC,EAAQsN,GACW,gBAAToC,IACV1P,EAAQ8xB,GAAqBrzB,EAAQ,uBAMtCqC,EAAG0Q,EAAQ+jB,EAAa7P,EAAUhU,EAAO8jB,EAAYC,EAAUjQ,EAAQC,EAAOiQ,EAASC,EAHpFzR,EAAK,IAAIrU,GAAUmN,KAAKzV,IAAK9I,EAAO6lB,MAAO5U,EAAM,EAAG,EAAGkW,IAC1DzY,EAAQ,EACR0Y,EAAa,KAEd3B,EAAGpY,EAAI9L,EACPkkB,EAAGS,EAAIxkB,EACPH,GAAS,GAEG,UADZG,GAAO,MAENq1B,EAAa/2B,EAAO6lB,MAAM5U,GAC1BjR,EAAO6lB,MAAM5U,GAAQvP,EACrBA,EAAM2xB,GAAqBrzB,EAAQiR,IAASvP,EAC5Cq1B,EAAc/2B,EAAO6lB,MAAM5U,GAAQ8lB,EAAcvB,GAAgBx1B,EAAQiR,IAG1EoC,GADAhR,EAAI,CAACd,EAAOG,IAGZA,EAAMW,EAAE,GACRy0B,GAFAv1B,EAAQc,EAAE,IAEUe,MAAMuP,KAAoB,IAClCjR,EAAI0B,MAAMuP,KAAoB,IAC5BvS,OAAQ,MACb2S,EAASJ,GAAgBnI,KAAK9I,IACrCs1B,EAAWjkB,EAAO,GAClBiU,EAAQtlB,EAAI6S,UAAU7F,EAAOqE,EAAOrE,OAChCuE,EACHA,GAASA,EAAQ,GAAK,EACS,UAArB+T,EAAMplB,QAAQ,IAAuC,UAArBolB,EAAMplB,QAAQ,KACxDqR,EAAQ,GAEL+jB,KAAcD,EAAaD,EAAY1P,MAAiB,MAC3DH,EAAWtlB,WAAWo1B,IAAe,EACrCG,EAAYH,EAAWn1B,QAAQqlB,EAAW,IAAI7mB,QACtB,MAAvB42B,EAASv1B,OAAO,KAAgBu1B,EAAW11B,GAAe2lB,EAAU+P,GAAYE,GACjFnQ,EAASplB,WAAWq1B,GACpBC,EAAUD,EAASp1B,QAAQmlB,EAAS,IAAI3mB,QACxCsO,EAAQiE,GAAgBY,UAAY0jB,EAAQ72B,OACvC62B,IACJA,EAAUA,GAAWzf,EAAQI,MAAM3G,IAASimB,EACxCxoB,IAAUhN,EAAItB,SACjBsB,GAAOu1B,EACPxR,EAAGS,GAAK+Q,IAGNC,IAAcD,IACjBhQ,EAAW6O,GAAe91B,EAAQiR,EAAM8lB,EAAYE,IAAY,GAGjExR,EAAG3c,IAAM,CACR3D,MAAOsgB,EAAG3c,IACVtF,EAAIwjB,GAAyB,IAAfI,EAAqBJ,EAAQ,IAC3CnY,EAAGoY,EACHxU,EAAGsU,EAASE,EACZI,EAAIpU,GAASA,EAAQ,GAAe,WAAThC,EAAoB9P,KAAKC,MAAQ,IAI/DqkB,EAAGhT,EAAK/D,EAAQhN,EAAItB,OAAUsB,EAAI6S,UAAU7F,EAAOhN,EAAItB,QAAU,QAEjEqlB,EAAG3T,EAAa,YAATb,GAA8B,SAARvP,EAAiBwvB,GAAmCD,UAElFpY,GAAQrF,KAAK9R,KAAS+jB,EAAGS,EAAI,QACxBpd,IAAM2c,EAIoB,SAAhC0R,GAAgCj5B,OAC3B8C,EAAQ9C,EAAM8C,MAAM,KACvBuL,EAAIvL,EAAM,GACVwL,EAAIxL,EAAM,IAAM,YACP,QAANuL,GAAqB,WAANA,GAAwB,SAANC,GAAsB,UAANA,IACpDtO,EAAQqO,EACRA,EAAIC,EACJA,EAAItO,GAEL8C,EAAM,GAAKo2B,GAAkB7qB,IAAMA,EACnCvL,EAAM,GAAKo2B,GAAkB5qB,IAAMA,EAC5BxL,EAAMkS,KAAK,KAEC,SAApBmkB,GAAqBvW,EAAOxH,MACvBA,EAAKnX,OAASmX,EAAKnX,MAAMoF,QAAU+R,EAAKnX,MAAM0D,KAAM,KAKtDoL,EAAMqmB,EAAiBv3B,EAJpBC,EAASsZ,EAAKtU,EACjB6gB,EAAQ7lB,EAAO6lB,MACfjC,EAAQtK,EAAKhM,EACb1B,EAAQ5L,EAAOC,SAEF,QAAV2jB,IAA6B,IAAVA,EACtBiC,EAAMkO,QAAU,GAChBuD,EAAkB,WAGlBv3B,GADA6jB,EAAQA,EAAM5iB,MAAM,MACVZ,QACI,IAALL,GACRkR,EAAO2S,EAAM7jB,GACTgyB,GAAgB9gB,KACnBqmB,EAAkB,EAClBrmB,EAAiB,oBAATA,EAA8BmhB,GAAuBE,IAE9DkD,GAAgBx1B,EAAQiR,GAGtBqmB,IACH9B,GAAgBx1B,EAAQsyB,IACpB1mB,IACHA,EAAM2mB,KAAOvyB,EAAO01B,gBAAgB,aACpC7P,EAAM0R,MAAQ1R,EAAM2R,OAAS3R,EAAM6M,UAAY,OAC/C+E,GAAgBz3B,EAAQ,GACxB4L,EAAM8hB,QAAU,EAChB+E,GAA6B5M,MA6Fd,SAAnB6R,GAAmBx5B,SAAoB,6BAAVA,GAAkD,SAAVA,IAAqBA,EACrD,SAArCy5B,GAAqC33B,OAChC43B,EAAevE,GAAqBrzB,EAAQsyB,WACzCoF,GAAiBE,GAAgBC,GAAoBD,EAAah2B,OAAO,GAAGwB,MAAMgP,IAASE,IAAIpR,IAE1F,SAAb42B,GAAc93B,EAAQ+3B,OAIpB5zB,EAAQ6zB,EAAatH,EAAMuH,EAHxBrsB,EAAQ5L,EAAOC,OAASO,GAAUR,GACrC6lB,EAAQ7lB,EAAO6lB,MACfqS,EAASP,GAAmC33B,UAEzC4L,EAAM2mB,KAAOvyB,EAAOY,aAAa,aAGP,iBAD7Bs3B,EAAS,EADTxH,EAAO1wB,EAAOkyB,UAAUiG,QAAQC,cAAcF,QAC/B71B,EAAGquB,EAAKrjB,EAAGqjB,EAAKje,EAAGie,EAAKjkB,EAAGikB,EAAKxK,EAAGwK,EAAK1T,IACxC9J,KAAK,KAA0B2kB,GAAoBK,GACxDA,IAAWL,IAAsB73B,EAAOq4B,cAAgBr4B,IAAW4zB,IAAgBhoB,EAAM2mB,MAEnG7B,EAAO7K,EAAM2O,QACb3O,EAAM2O,QAAU,SAChBrwB,EAASnE,EAAOu1B,cACCv1B,EAAOq4B,cAAiBr4B,EAAOiN,wBAAwBkoB,SACvE8C,EAAa,EACbD,EAAch4B,EAAOs4B,mBACrB1E,GAAYa,YAAYz0B,IAEzBk4B,EAASP,GAAmC33B,GAC5C0wB,EAAQ7K,EAAM2O,QAAU9D,EAAQ8E,GAAgBx1B,EAAQ,WACpDi4B,IACHD,EAAc7zB,EAAOo0B,aAAav4B,EAAQg4B,GAAe7zB,EAASA,EAAOswB,YAAYz0B,GAAU4zB,GAAYe,YAAY30B,KAGjH+3B,GAA2B,EAAhBG,EAAO93B,OAAc,CAAC83B,EAAO,GAAIA,EAAO,GAAIA,EAAO,GAAIA,EAAO,GAAIA,EAAO,IAAKA,EAAO,KAAOA,GAE9F,SAAlBM,GAAmBx4B,EAAQy4B,EAAQC,EAAkBC,EAAQC,EAAaC,OAWxE7D,EAAQ8D,EAAgBtsB,EAVrBZ,EAAQ5L,EAAOC,MAClBi4B,EAASU,GAAed,GAAW93B,GAAQ,GAC3C+4B,EAAantB,EAAMotB,SAAW,EAC9BC,EAAartB,EAAMstB,SAAW,EAC9BC,EAAavtB,EAAMwtB,SAAW,EAC9BC,EAAaztB,EAAM0tB,SAAW,EAC7Bj3B,EAAsB61B,KAAnB7qB,EAAmB6qB,KAAhBzlB,EAAgBylB,KAAbzrB,EAAayrB,KAAVqB,EAAUrB,KAANsB,EAAMtB,KACvBuB,EAAchB,EAAOz3B,MAAM,KAC3Bg4B,EAAUr3B,WAAW83B,EAAY,KAAO,EACxCP,EAAUv3B,WAAW83B,EAAY,KAAO,EAEpCf,EAQMR,IAAWL,KAAsBiB,EAAez2B,EAAIoK,EAAIY,EAAIoF,KAEtEjG,EAAIwsB,IAAY3rB,EAAIyrB,GAAeI,GAAW72B,EAAIy2B,IAAiBz2B,EAAIm3B,EAAKnsB,EAAIksB,GAAMT,EACtFE,EAFIA,GAAWvsB,EAAIqsB,GAAeI,IAAYzmB,EAAIqmB,IAAiBrmB,EAAI+mB,EAAK/sB,EAAI8sB,GAAMT,EAGtFI,EAAU1sB,IAVVwsB,GADAhE,EAASD,GAAS/0B,IACDuM,IAAMktB,EAAY,GAAGx3B,QAAQ,KAAO+2B,EAAU,IAAMhE,EAAOG,MAAQ6D,GACpFE,EAAUlE,EAAOxoB,KAAQitB,EAAY,IAAMA,EAAY,IAAIx3B,QAAQ,KAAQi3B,EAAU,IAAMlE,EAAOI,OAAS8D,IAYxGP,IAAsB,IAAXA,GAAoB/sB,EAAM+sB,QACxCY,EAAKP,EAAUD,EACfS,EAAKN,EAAUD,EACfrtB,EAAMwtB,QAAUD,GAAcI,EAAKl3B,EAAIm3B,EAAK/mB,GAAK8mB,EACjD3tB,EAAM0tB,QAAUD,GAAcE,EAAKlsB,EAAImsB,EAAK/sB,GAAK+sB,GAEjD5tB,EAAMwtB,QAAUxtB,EAAM0tB,QAAU,EAEjC1tB,EAAMotB,QAAUA,EAChBptB,EAAMstB,QAAUA,EAChBttB,EAAM+sB,SAAWA,EACjB/sB,EAAM6sB,OAASA,EACf7sB,EAAM8sB,mBAAqBA,EAC3B14B,EAAO6lB,MAAMuM,IAAwB,UACjCyG,IACHlD,GAAkBkD,EAAyBjtB,EAAO,UAAWmtB,EAAYC,GACzErD,GAAkBkD,EAAyBjtB,EAAO,UAAWqtB,EAAYC,GACzEvD,GAAkBkD,EAAyBjtB,EAAO,UAAWutB,EAAYvtB,EAAMwtB,SAC/EzD,GAAkBkD,EAAyBjtB,EAAO,UAAWytB,EAAYztB,EAAM0tB,UAEhFt5B,EAAOyqB,aAAa,kBAAmBuO,EAAU,IAAME,GAsKtC,SAAlBQ,GAAmB15B,EAAQuB,EAAOrD,OAC7BuvB,EAAOnjB,GAAQ/I,UACZL,GAAOS,WAAWJ,GAASI,WAAWm0B,GAAe91B,EAAQ,IAAK9B,EAAQ,KAAMuvB,KAAUA,EAmHxE,SAA1BkM,GAAmCnU,EAAQxlB,EAAQd,EAAU+nB,EAAU+P,OAMrE4C,EAAWnU,EALRoU,EAAM,IACTjK,EAAW3xB,EAAU+4B,GAErB5L,EADSzpB,WAAWq1B,IAAcpH,IAAaoH,EAAS/0B,QAAQ,OAAU63B,GAAW,GACnE7S,EAClB8S,EAAc9S,EAAWmE,EAAU,aAEhCwE,IAEe,WADlBgK,EAAY5C,EAASh2B,MAAM,KAAK,MAE/BoqB,GAAUyO,KACKzO,QACdA,GAAWA,EAAS,EAAKyO,GAAOA,GAGhB,OAAdD,GAAsBxO,EAAS,EAClCA,GAAWA,EAASyO,MAAiBA,KAAUzO,EAASyO,GAAOA,EACvC,QAAdD,GAAgC,EAATxO,IACjCA,GAAWA,EAASyO,MAAiBA,KAAUzO,EAASyO,GAAOA,IAGjErU,EAAO1c,IAAM2c,EAAK,IAAIrU,GAAUoU,EAAO1c,IAAK9I,EAAQd,EAAU+nB,EAAUmE,EAAQ0F,IAChFrL,EAAGS,EAAI6T,EACPtU,EAAGnY,EAAI,MACPkY,EAAOxV,OAAO7G,KAAKjK,GACZumB,EAEE,SAAVuU,GAAWh6B,EAAQi6B,OACb,IAAIz2B,KAAKy2B,EACbj6B,EAAOwD,GAAKy2B,EAAOz2B,UAEbxD,EAEc,SAAtBk6B,GAAuB1U,EAAQ2U,EAAYn6B,OAIzCo6B,EAAU52B,EAAGuzB,EAAYC,EAAU/P,EAAUF,EAAmBkQ,EAH7DoD,EAAaL,GAAQ,GAAIh6B,EAAOC,OAEnC4lB,EAAQ7lB,EAAO6lB,UAeXriB,KAbD62B,EAAW9H,KACdwE,EAAa/2B,EAAOY,aAAa,aACjCZ,EAAOyqB,aAAa,YAAa,IACjC5E,EAAMyM,IAAkB6H,EACxBC,EAAW3C,GAAgBz3B,EAAQ,GACnCw1B,GAAgBx1B,EAAQsyB,IACxBtyB,EAAOyqB,aAAa,YAAasM,KAEjCA,EAAavD,iBAAiBxzB,GAAQsyB,IACtCzM,EAAMyM,IAAkB6H,EACxBC,EAAW3C,GAAgBz3B,EAAQ,GACnC6lB,EAAMyM,IAAkByE,GAEfhF,IACTgF,EAAasD,EAAW72B,OACxBwzB,EAAWoD,EAAS52B,KAlBV,gDAmB6BvB,QAAQuB,GAAK,IAGnDyjB,EAFY3c,GAAQysB,MACpBE,EAAU3sB,GAAQ0sB,IACmBlB,GAAe91B,EAAQwD,EAAGuzB,EAAYE,GAAWt1B,WAAWo1B,GACjGhQ,EAASplB,WAAWq1B,GACpBxR,EAAO1c,IAAM,IAAIsI,GAAUoU,EAAO1c,IAAKsxB,EAAU52B,EAAGyjB,EAAUF,EAASE,EAAU4J,IACjFrL,EAAO1c,IAAIwE,EAAI2pB,GAAW,EAC1BzR,EAAOxV,OAAO7G,KAAK3F,IAGrBw2B,GAAQI,EAAUC,OA35BhBzvB,GAAMM,GAAM0oB,GAAaK,GAAgBH,GAA0BwG,GAAqBv3B,GA+G3FixB,GDkkGcuG,GAA4I5mB,GAA5I4mB,OAAQC,GAAoI7mB,GAApI6mB,OAAQC,GAA4H9mB,GAA5H8mB,OAAQC,GAAoH/mB,GAApH+mB,OAAQC,GAA4GhnB,GAA5GgnB,OAAQ3c,GAAoGrK,GAApGqK,OAAQ4c,GAA4FjnB,GAA5FinB,KAAMC,GAAsFlnB,GAAtFknB,MAAOC,GAA+EnnB,GAA/EmnB,MAAOC,GAAwEpnB,GAAxEonB,MAAOC,GAAiErnB,GAAjEqnB,OAAQC,GAAyDtnB,GAAzDsnB,QAASC,GAAgDvnB,GAAhDunB,KAAM/c,GAA0CxK,GAA1CwK,YAAagd,GAA6BxnB,GAA7BwnB,OAAQC,GAAqBznB,GAArBynB,KAAMC,GAAe1nB,GAAf0nB,KAAMC,GAAS3nB,GAAT2nB,KC/qGjJvJ,GAAkB,GAClB+H,GAAW,IAAM34B,KAAK8W,GACtBsjB,GAAWp6B,KAAK8W,GAAK,IACrBujB,GAASr6B,KAAKs6B,MAEd5I,GAAW,WACXuD,GAAiB,uCACjBsF,GAAc,YACdzJ,GAAmB,CAAC0J,UAAU,qBAAsBpE,MAAM,gBAAiBqE,MAAM,WAwBjFtJ,GAAiB,YACjBF,GAAuBE,GAAiB,SAqFxCuJ,GAAY,qBAAqB76B,MAAM,KACvC0yB,GAAmB,SAAnBA,iBAAoBx0B,EAAU48B,EAASC,OAErCltB,GADOitB,GAAWhI,IACZjO,MACN9lB,EAAI,KACDb,KAAY2P,IAAMktB,SACd78B,MAERA,EAAWA,EAASuC,OAAO,GAAG0P,cAAgBjS,EAAS0C,OAAO,GACvD7B,OAAU87B,GAAU97B,GAAGb,KAAa2P,YACnC9O,EAAI,EAAK,MAAe,IAANA,EAAW,KAAa,GAALA,EAAU87B,GAAU97B,GAAK,IAAMb,GA+E7Ew3B,GAAuB,CAACsF,IAAI,EAAGC,IAAI,EAAGC,KAAK,GAC3CtF,GAAsB,CAAC7pB,KAAK,EAAGovB,KAAK,GAuDpChK,GAAO,SAAPA,KAAQnyB,EAAQd,EAAUuuB,EAAMC,OAC3BxvB,SACJ+1B,IAAkBN,KACbz0B,KAAY+yB,IAAkC,cAAb/yB,KACrCA,EAAW+yB,GAAiB/yB,IACd+C,QAAQ,OACrB/C,EAAWA,EAAS8B,MAAM,KAAK,IAG7B+wB,GAAgB7yB,IAA0B,cAAbA,GAChChB,EAAQu5B,GAAgBz3B,EAAQ0tB,GAChCxvB,EAAsB,oBAAbgB,EAAkChB,EAAMgB,GAAYhB,EAAMq0B,IAAMr0B,EAAMu6B,OAAS2D,GAAc/I,GAAqBrzB,EAAQoyB,KAAyB,IAAMl0B,EAAMm0B,QAAU,OAElLn0B,EAAQ8B,EAAO6lB,MAAM3mB,KACG,SAAVhB,IAAoBwvB,MAAaxvB,EAAQ,IAAI+D,QAAQ,WAClE/D,EAASm+B,GAAcn9B,IAAam9B,GAAcn9B,GAAUc,EAAQd,EAAUuuB,IAAU4F,GAAqBrzB,EAAQd,IAAawB,GAAaV,EAAQd,KAA2B,YAAbA,EAAyB,EAAI,IAG7LuuB,MAAWvvB,EAAQ,IAAIoF,OAAOrB,QAAQ,KAAO6zB,GAAe91B,EAAQd,EAAUhB,EAAOuvB,GAAQA,EAAOvvB,GA8E5Gk5B,GAAoB,CAACkF,IAAI,KAAMC,OAAO,OAAQrvB,KAAK,KAAMsvB,MAAM,OAAQrwB,OAAO,OAiD9EkwB,GAAgB,CACfI,+BAAWjX,EAAQxlB,EAAQd,EAAU83B,EAAU70B,MAC3B,gBAAfA,EAAMmX,KAAwB,KAC7BmM,EAAKD,EAAO1c,IAAM,IAAIsI,GAAUoU,EAAO1c,IAAK9I,EAAQd,EAAU,EAAG,EAAGm4B,WACxE5R,EAAGnY,EAAI0pB,EACPvR,EAAG0F,IAAM,GACT1F,EAAGtjB,MAAQA,EACXqjB,EAAOxV,OAAO7G,KAAKjK,GACZ,KA6EV24B,GAAoB,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAC/B6E,GAAwB,GAkFxBjF,GAAkB,SAAlBA,gBAAmBz3B,EAAQ0tB,OACtB9hB,EAAQ5L,EAAOC,OAAS,IAAIK,GAAQN,MACpC,MAAO4L,IAAU8hB,IAAY9hB,EAAM8hB,eAC/B9hB,MAQPW,EAAGC,EAAGmwB,EAAGnL,EAAQC,EAAQmL,EAAUC,EAAWC,EAAWC,EAAOC,EAAOC,EAAajE,EAASE,EAC7FhB,EAAQgF,EAAO5kB,EAAKC,EAAKlW,EAAGgL,EAAGoF,EAAGhG,EAAG0wB,EAAKC,EAAKC,EAAIC,EAAIC,EAAIC,EAAKC,EAAKC,EAAKC,EAAKC,EAAKC,EAPjFhY,EAAQ7lB,EAAO6lB,MAClBiY,EAAiBlyB,EAAM4lB,OAAS,EAEhCwK,EAAM,MACNzI,EAAKC,iBAAiBxzB,GACtBy4B,EAASpF,GAAqBrzB,EAAQoyB,KAAyB,WAGhE7lB,EAAIC,EAAImwB,EAAIC,EAAWC,EAAYC,EAAYC,EAAQC,EAAQC,EAAc,EAC7EzL,EAASC,EAAS,EAClB7lB,EAAM2mB,OAASvyB,EAAOs1B,SAAUD,GAAOr1B,IAEnCuzB,EAAGb,YACe,SAAjBa,EAAGb,WAAqC,SAAba,EAAGgE,OAAkC,SAAdhE,EAAGiE,SACxD3R,EAAMyM,KAAoC,SAAjBiB,EAAGb,UAAuB,gBAAkBa,EAAGb,UAAY,QAAQ1xB,MAAM,KAAKsB,MAAM,EAAG,GAAG4Q,KAAK,MAAQ,KAAO,KAAqB,SAAdqgB,EAAGiE,OAAoB,UAAYjE,EAAGiE,OAAS,KAAO,KAAoB,SAAbjE,EAAGgE,MAAmB,SAAWhE,EAAGgE,MAAMv2B,MAAM,KAAKkS,KAAK,KAAO,KAAO,KAA8B,SAAvBqgB,EAAGjB,IAA6BiB,EAAGjB,IAAkB,KAEhVzM,EAAM0R,MAAQ1R,EAAM2R,OAAS3R,EAAM6M,UAAY,QAGhDwF,EAASJ,GAAW93B,EAAQ4L,EAAM2mB,KAC9B3mB,EAAM2mB,MAIR8K,EAHGzxB,EAAM8hB,SACT4P,EAAKt9B,EAAO00B,UACZ+D,EAAU7sB,EAAMotB,QAAUsE,EAAG/wB,EAAK,OAASX,EAAMstB,QAAUoE,EAAG9wB,GAAK,KAC9D,KAECkhB,GAAW1tB,EAAOY,aAAa,mBAEtC43B,GAAgBx4B,EAAQq9B,GAAM5E,IAAU4E,GAAMzxB,EAAM8sB,kBAAmC,IAAjB9sB,EAAM+sB,OAAkBT,IAE/Fc,EAAUptB,EAAMotB,SAAW,EAC3BE,EAAUttB,EAAMstB,SAAW,EACvBhB,IAAWL,KACdx1B,EAAI61B,EAAO,GACX7qB,EAAI6qB,EAAO,GACXzlB,EAAIylB,EAAO,GACXzrB,EAAIyrB,EAAO,GACX3rB,EAAI4wB,EAAMjF,EAAO,GACjB1rB,EAAI4wB,EAAMlF,EAAO,GAGK,IAAlBA,EAAO93B,QACVoxB,EAASrwB,KAAKiX,KAAK/V,EAAIA,EAAIgL,EAAIA,GAC/BokB,EAAStwB,KAAKiX,KAAK3L,EAAIA,EAAIgG,EAAIA,GAC/BmqB,EAAYv6B,GAAKgL,EAAKmuB,GAAOnuB,EAAGhL,GAAKy3B,GAAW,GAChDiD,EAAStqB,GAAKhG,EAAK+uB,GAAO/oB,EAAGhG,GAAKqtB,GAAW8C,EAAW,KAC9CnL,GAAUtwB,KAAK+F,IAAI/F,KAAKmX,IAAIykB,EAAQxB,MAC1C3vB,EAAM2mB,MACThmB,GAAKysB,GAAWA,EAAU32B,EAAI62B,EAAUzmB,GACxCjG,GAAK0sB,GAAWF,EAAU3rB,EAAI6rB,EAAUzsB,MAKzCoxB,EAAM3F,EAAO,GACbyF,EAAMzF,EAAO,GACbsF,EAAMtF,EAAO,GACbuF,EAAMvF,EAAO,GACbwF,EAAMxF,EAAO,IACb0F,EAAM1F,EAAO,IACb3rB,EAAI2rB,EAAO,IACX1rB,EAAI0rB,EAAO,IACXyE,EAAIzE,EAAO,IAGX2E,GADAK,EAAQ1B,GAAOqC,EAAKH,IACA5D,GAEhBoD,IAGHG,EAAKF,GAFL7kB,EAAMnX,KAAKmX,KAAK4kB,IAEHM,GADbjlB,EAAMpX,KAAKoX,KAAK2kB,IAEhBI,EAAKF,EAAI9kB,EAAImlB,EAAIllB,EACjBglB,EAAKM,EAAIvlB,EAAIolB,EAAInlB,EACjBilB,EAAML,GAAK5kB,EAAIilB,EAAIllB,EACnBmlB,EAAML,GAAK7kB,EAAIklB,EAAInlB,EACnBolB,EAAMG,GAAKtlB,EAAImlB,EAAIplB,EACnBslB,EAAMD,GAAKplB,EAAIqlB,EAAItlB,EACnB6kB,EAAME,EACND,EAAME,EACNO,EAAMN,GAIPT,GADAI,EAAQ1B,IAAQ/oB,EAAGirB,IACC5D,GAChBoD,IACH5kB,EAAMnX,KAAKmX,KAAK4kB,GAKhBU,EAAMnxB,GAJN8L,EAAMpX,KAAKoX,KAAK2kB,IAIJU,EAAItlB,EAChBjW,EAJAg7B,EAAKh7B,EAAEiW,EAAIklB,EAAIjlB,EAKflL,EAJAiwB,EAAKjwB,EAAEiL,EAAImlB,EAAIllB,EAKf9F,EAJA8qB,EAAK9qB,EAAE6F,EAAIolB,EAAInlB,GAQhBqkB,GADAM,EAAQ1B,GAAOnuB,EAAGhL,IACCy3B,GACfoD,IAGHG,EAAKh7B,GAFLiW,EAAMnX,KAAKmX,IAAI4kB,IAEJ7vB,GADXkL,EAAMpX,KAAKoX,IAAI2kB,IAEfI,EAAKH,EAAI7kB,EAAI8kB,EAAI7kB,EACjBlL,EAAIA,EAAEiL,EAAIjW,EAAEkW,EACZ6kB,EAAMA,EAAI9kB,EAAI6kB,EAAI5kB,EAClBlW,EAAIg7B,EACJF,EAAMG,GAGHT,GAAwD,MAA3C17B,KAAK+F,IAAI21B,GAAa17B,KAAK+F,IAAI01B,KAC/CC,EAAYD,EAAW,EACvBE,EAAY,IAAMA,GAEnBtL,EAAStwB,GAAOC,KAAKiX,KAAK/V,EAAIA,EAAIgL,EAAIA,EAAIoF,EAAIA,IAC9Cgf,EAASvwB,GAAOC,KAAKiX,KAAKglB,EAAMA,EAAMS,EAAMA,IAC5CX,EAAQ1B,GAAO2B,EAAKC,GACpBL,EAA2B,KAAlB57B,KAAK+F,IAAIg2B,GAAmBA,EAAQpD,GAAW,EACxDmD,EAAcW,EAAM,GAAMA,EAAM,GAAMA,EAAMA,GAAO,GAGhDhyB,EAAM2mB,MACT8K,EAAKr9B,EAAOY,aAAa,aACzBgL,EAAMmyB,SAAW/9B,EAAOyqB,aAAa,YAAa,MAASiN,GAAiBrE,GAAqBrzB,EAAQsyB,KACzG+K,GAAMr9B,EAAOyqB,aAAa,YAAa4S,KAInB,GAAlBl8B,KAAK+F,IAAI61B,IAAe57B,KAAK+F,IAAI61B,GAAS,MACzCe,GACHtM,IAAW,EACXuL,GAAUH,GAAY,EAAK,KAAO,IAClCA,GAAaA,GAAY,EAAK,KAAO,MAErCnL,IAAW,EACXsL,GAAUA,GAAS,EAAK,KAAO,MAGjCrP,EAAUA,GAAW9hB,EAAM8hB,QAC3B9hB,EAAMW,EAAIA,IAAMX,EAAMoyB,SAAWzxB,KAAQmhB,GAAW9hB,EAAMoyB,WAAc78B,KAAKC,MAAMpB,EAAOi+B,YAAc,KAAO98B,KAAKC,OAAOmL,IAAM,GAAK,KAAOvM,EAAOi+B,YAAcryB,EAAMoyB,SAAW,IAAM,GAxInL,KAyINpyB,EAAMY,EAAIA,IAAMZ,EAAMsyB,SAAW1xB,KAAQkhB,GAAW9hB,EAAMsyB,WAAc/8B,KAAKC,MAAMpB,EAAOm+B,aAAe,KAAOh9B,KAAKC,OAAOoL,IAAM,GAAK,KAAOxM,EAAOm+B,aAAevyB,EAAMsyB,SAAW,IAAM,GAzIrL,KA0INtyB,EAAM+wB,EAAIA,EA1IJ,KA2IN/wB,EAAM4lB,OAAStwB,GAAOswB,GACtB5lB,EAAM6lB,OAASvwB,GAAOuwB,GACtB7lB,EAAMgxB,SAAW17B,GAAO07B,GAAYZ,EACpCpwB,EAAMixB,UAAY37B,GAAO27B,GAAab,EACtCpwB,EAAMkxB,UAAY57B,GAAO47B,GAAad,EACtCpwB,EAAMmxB,MAAQA,EAAQf,EACtBpwB,EAAMoxB,MAAQA,EAAQhB,EACtBpwB,EAAMwyB,qBAAuBnB,EAlJvB,MAmJDrxB,EAAMymB,QAAU1wB,WAAW82B,EAAOz3B,MAAM,KAAK,MAAS0sB,GAAW9hB,EAAMymB,SAAY,KACvFxM,EAAMuM,IAAwBgK,GAAc3D,IAE7C7sB,EAAMwtB,QAAUxtB,EAAM0tB,QAAU,EAChC1tB,EAAM8L,QAAUF,EAAQE,QACxB9L,EAAM+lB,gBAAkB/lB,EAAM2mB,IAAM8L,GAAuBrK,GAAcsK,GAAuBC,GAChG3yB,EAAM8hB,QAAU,EACT9hB,GAERwwB,GAAgB,SAAhBA,cAAgBl+B,UAAUA,EAAQA,EAAM8C,MAAM,MAAM,GAAK,IAAM9C,EAAM,IAKrEqgC,GAAyB,SAAzBA,uBAA0Bzd,EAAOlV,GAChCA,EAAM+wB,EAAI,MACV/wB,EAAMkxB,UAAYlxB,EAAMixB,UAAY,OACpCjxB,EAAM8L,QAAU,EAChB4mB,GAAqBxd,EAAOlV,IAE7B4yB,GAAW,OACXC,GAAU,MACVC,GAAkB,KAClBJ,GAAuB,SAAvBA,qBAAgCxd,EAAOlV,SAC4GA,GAAS2S,KAAtJyf,IAAAA,SAAUE,IAAAA,SAAU3xB,IAAAA,EAAGC,IAAAA,EAAGmwB,IAAAA,EAAGC,IAAAA,SAAUE,IAAAA,UAAWD,IAAAA,UAAWE,IAAAA,MAAOC,IAAAA,MAAOxL,IAAAA,OAAQC,IAAAA,OAAQ2M,IAAAA,qBAAsB1mB,IAAAA,QAAS1X,IAAAA,OAAQqyB,IAAAA,QACtI8H,EAAa,GACbwE,EAAqB,SAAZjnB,GAAsBoJ,GAAmB,IAAVA,IAA4B,IAAZpJ,KAGrD2a,IAAYwK,IAAc2B,IAAY1B,IAAc0B,IAAW,KAIjElmB,EAHG4kB,EAAQv7B,WAAWm7B,GAAavB,GACnCiC,EAAMr8B,KAAKoX,IAAI2kB,GACfQ,EAAMv8B,KAAKmX,IAAI4kB,GAEhBA,EAAQv7B,WAAWk7B,GAAatB,GAChCjjB,EAAMnX,KAAKmX,IAAI4kB,GACf3wB,EAAImtB,GAAgB15B,EAAQuM,EAAGixB,EAAMllB,GAAO+Z,GAC5C7lB,EAAIktB,GAAgB15B,EAAQwM,GAAIrL,KAAKoX,IAAI2kB,IAAU7K,GACnDsK,EAAIjD,GAAgB15B,EAAQ28B,EAAGe,EAAMplB,GAAO+Z,EAAUA,GAGnD+L,IAAyBK,KAC5BtE,GAAc,eAAiBiE,EAAuBM,KAEnDV,GAAYE,KACf/D,GAAc,aAAe6D,EAAW,MAAQE,EAAW,QAExDS,GAASpyB,IAAMkyB,IAAWjyB,IAAMiyB,IAAW9B,IAAM8B,KACpDtE,GAAewC,IAAM8B,IAAWE,EAAS,eAAiBpyB,EAAI,KAAOC,EAAI,KAAOmwB,EAAI,KAAO,aAAepwB,EAAI,KAAOC,EAAIkyB,IAEtH9B,IAAa4B,KAChBrE,GAAc,UAAYyC,EAAW8B,IAElC5B,IAAc0B,KACjBrE,GAAc,WAAa2C,EAAY4B,IAEpC7B,IAAc2B,KACjBrE,GAAc,WAAa0C,EAAY6B,IAEpC3B,IAAUyB,IAAYxB,IAAUwB,KACnCrE,GAAc,QAAU4C,EAAQ,KAAOC,EAAQ0B,IAEjC,IAAXlN,GAA2B,IAAXC,IACnB0I,GAAc,SAAW3I,EAAS,KAAOC,EAASiN,IAEnD1+B,EAAO6lB,MAAMyM,IAAkB6H,GAAc,mBAE9CkE,GAAuB,SAAvBA,qBAAgCvd,EAAOlV,OAIrCgzB,EAAKC,EAAK1B,EAAKC,EAAK1M,IAH0G9kB,GAAS2S,KAAnIyf,IAAAA,SAAUE,IAAAA,SAAU3xB,IAAAA,EAAGC,IAAAA,EAAGowB,IAAAA,SAAUG,IAAAA,MAAOC,IAAAA,MAAOxL,IAAAA,OAAQC,IAAAA,OAAQzxB,IAAAA,OAAQg5B,IAAAA,QAASE,IAAAA,QAASE,IAAAA,QAASE,IAAAA,QAASyE,IAAAA,SAClHxE,EAAK53B,WAAW4K,GAChBitB,EAAK73B,WAAW6K,GAEjBowB,EAAWj7B,WAAWi7B,GACtBG,EAAQp7B,WAAWo7B,IACnBC,EAAQr7B,WAAWq7B,MAGlBD,GADAC,EAAQr7B,WAAWq7B,GAEnBJ,GAAYI,GAETJ,GAAYG,GACfH,GAAYrB,GACZwB,GAASxB,GACTqD,EAAMz9B,KAAKmX,IAAIskB,GAAYpL,EAC3BqN,EAAM19B,KAAKoX,IAAIqkB,GAAYpL,EAC3B2L,EAAMh8B,KAAKoX,IAAIqkB,EAAWG,IAAUtL,EACpC2L,EAAMj8B,KAAKmX,IAAIskB,EAAWG,GAAStL,EAC/BsL,IACHC,GAASzB,GACT7K,EAAOvvB,KAAK29B,IAAI/B,EAAQC,GAExBG,GADAzM,EAAOvvB,KAAKiX,KAAK,EAAIsY,EAAOA,GAE5B0M,GAAO1M,EACHsM,IACHtM,EAAOvvB,KAAK29B,IAAI9B,GAEhB4B,GADAlO,EAAOvvB,KAAKiX,KAAK,EAAIsY,EAAOA,GAE5BmO,GAAOnO,IAGTkO,EAAM19B,GAAO09B,GACbC,EAAM39B,GAAO29B,GACb1B,EAAMj8B,GAAOi8B,GACbC,EAAMl8B,GAAOk8B,KAEbwB,EAAMpN,EACN4L,EAAM3L,EACNoN,EAAM1B,EAAM,IAER5D,MAAShtB,EAAI,IAAItK,QAAQ,OAAWu3B,MAAShtB,EAAI,IAAIvK,QAAQ,SACjEs3B,EAAKzD,GAAe91B,EAAQ,IAAKuM,EAAG,MACpCitB,EAAK1D,GAAe91B,EAAQ,IAAKwM,EAAG,QAEjCwsB,GAAWE,GAAWE,GAAWE,KACpCC,EAAKr4B,GAAOq4B,EAAKP,GAAWA,EAAU4F,EAAM1F,EAAUiE,GAAO/D,GAC7DI,EAAKt4B,GAAOs4B,EAAKN,GAAWF,EAAU6F,EAAM3F,EAAUkE,GAAO9D,KAE1D0E,GAAYE,KAEfxN,EAAO1wB,EAAO00B,UACd6E,EAAKr4B,GAAOq4B,EAAKyE,EAAW,IAAMtN,EAAKyE,OACvCqE,EAAKt4B,GAAOs4B,EAAK0E,EAAW,IAAMxN,EAAK0E,SAExC1E,EAAO,UAAYkO,EAAM,IAAMC,EAAM,IAAM1B,EAAM,IAAMC,EAAM,IAAM7D,EAAK,IAAMC,EAAK,IACnFx5B,EAAOyqB,aAAa,YAAaiG,GACjCqN,IAAa/9B,EAAO6lB,MAAMyM,IAAkB5B,IAsE9C7vB,GAAa,8BAA+B,SAACpB,EAAMiP,OAEjDoD,EAAI,QACJzE,EAAI,SACJrL,EAAI,OACJ4hB,GAASlV,EAAQ,EAAI,CAJd,MAIiBoD,EAAEzE,EAAErL,GAAK,CAJ1B,MAI6BA,EAJ7B,MAIkC8P,EAAGzE,EAAEyE,EAAGzE,EAAErL,IAAIsQ,IAAI,SAAAysB,UAAQrwB,EAAQ,EAAIjP,EAAOs/B,EAAO,SAAWA,EAAOt/B,IAChH48B,GAAuB,EAAR3tB,EAAY,SAAWjP,EAAOA,GAAS,SAAS+lB,EAAQxlB,EAAQd,EAAU83B,EAAU70B,OAC9FE,EAAG6B,KACHya,UAAUve,OAAS,SACtBiC,EAAIuhB,EAAMtR,IAAI,SAAArB,UAAQkhB,GAAK3M,EAAQvU,EAAM/R,KAEN,KADnCgF,EAAO7B,EAAE6Q,KAAK,MACFlS,MAAMqB,EAAE,IAAIjC,OAAeiC,EAAE,GAAK6B,EAE/C7B,GAAK20B,EAAW,IAAIh2B,MAAM,KAC1BkD,EAAO,GACP0f,EAAM3iB,QAAQ,SAACgQ,EAAMlR,UAAMmE,EAAK+M,GAAQ5O,EAAEtC,GAAKsC,EAAEtC,IAAMsC,GAAKtC,EAAI,GAAK,EAAK,KAC1EylB,EAAOzV,KAAK/P,EAAQkE,EAAM/B,UAoLlB68B,GAAkBpC,GACvBqC,GAhLQC,GAAY,CACxBz/B,KAAM,MACNoR,SAAU8iB,GACVtzB,+BAAWL,UACHA,EAAO6lB,OAAS7lB,EAAO2K,UAE/BoF,mBAAK/P,EAAQkE,EAAM/B,EAAOuM,EAAO7O,OAI/Bk3B,EAAYC,EAAUjQ,EAAQE,EAAUpd,EAAMs1B,EAAa37B,EAAG0zB,EAAWD,EAASmI,EAAUC,EAAoBC,EAAoB1zB,EAAO+sB,EAAQjR,EAAa6X,EAH7J3b,EAAQrF,KAAKvO,OAChB6V,EAAQ7lB,EAAO6lB,MACf1b,EAAUhI,EAAM+B,KAAKiG,YAOjB3G,KALLywB,IAAkBN,UAEb6L,OAASjhB,KAAKihB,QAAU1M,GAAe9yB,GAC5Cu/B,EAAchhB,KAAKihB,OAAO5b,WACrBzhB,MAAQA,EACH+B,KACC,cAANV,IAGJwzB,EAAW9yB,EAAKV,IACZuN,GAASvN,KAAM+hB,GAAa/hB,EAAGU,EAAM/B,EAAOuM,EAAO1O,EAAQH,OAG/DgK,SAAcmtB,EACdmI,EAAc9C,GAAc74B,GACf,aAATqG,IAEHA,SADAmtB,EAAWA,EAAS7c,KAAKhY,EAAOuM,EAAO1O,EAAQH,KAGnC,WAATgK,IAAsBmtB,EAAS/0B,QAAQ,aAC1C+0B,EAAWroB,GAAeqoB,IAEvBmI,EACHA,EAAY5gB,KAAMve,EAAQwD,EAAGwzB,EAAU70B,KAAWulB,EAAc,QAC1D,GAAsB,OAAlBlkB,EAAE5B,OAAO,EAAE,GACrBm1B,GAAcvD,iBAAiBxzB,GAAQyzB,iBAAiBjwB,GAAK,IAAIF,OACjE0zB,GAAY,GACZtkB,GAAUa,UAAY,EACjBb,GAAUc,KAAKujB,KACnBG,EAAY5sB,GAAQysB,GACpBE,EAAU3sB,GAAQ0sB,IAEnBC,EAAUC,IAAcD,IAAYF,EAAajB,GAAe91B,EAAQwD,EAAGuzB,EAAYE,GAAWA,GAAWC,IAAcF,GAAYE,QAClIxvB,IAAIme,EAAO,cAAekR,EAAYC,EAAUtoB,EAAO7O,EAAS,EAAG,EAAG2D,GAC3EogB,EAAMza,KAAK3F,GACX+7B,EAAYp2B,KAAK3F,EAAG,EAAGqiB,EAAMriB,SACvB,GAAa,cAATqG,EAAsB,IAC5BM,GAAW3G,KAAK2G,GACnB4sB,EAAoC,mBAAhB5sB,EAAQ3G,GAAqB2G,EAAQ3G,GAAG2W,KAAKhY,EAAOuM,EAAO1O,EAAQH,GAAWsK,EAAQ3G,GAC1GvF,EAAU84B,KAAgBA,EAAW90B,QAAQ,aAAe80B,EAAapoB,GAAeooB,IACxFzsB,GAAQysB,EAAa,KAAsB,SAAfA,IAA0BA,GAAcvf,EAAQI,MAAMpU,IAAM8G,GAAQ6nB,GAAKnyB,EAAQwD,KAAO,IACpF,OAA/BuzB,EAAa,IAAIt1B,OAAO,KAAes1B,EAAa5E,GAAKnyB,EAAQwD,KAElEuzB,EAAa5E,GAAKnyB,EAAQwD,GAE3ByjB,EAAWtlB,WAAWo1B,IACtBqI,EAAqB,WAATv1B,GAA4C,MAAvBmtB,EAASv1B,OAAO,IAAeu1B,EAASp1B,OAAO,EAAG,MACtEo1B,EAAWA,EAASp1B,OAAO,IACxCmlB,EAASplB,WAAWq1B,GAChBxzB,KAAKyuB,KACE,cAANzuB,IACc,IAAbyjB,GAAiD,WAA/BkL,GAAKnyB,EAAQ,eAA8B+mB,IAChEE,EAAW,GAEZsY,EAAYp2B,KAAK,aAAc,EAAG0c,EAAM4Z,YACxC9J,GAAkBpX,KAAMsH,EAAO,aAAcoB,EAAW,UAAY,SAAUF,EAAS,UAAY,UAAWA,IAErG,UAANvjB,GAAuB,cAANA,KACpBA,EAAIyuB,GAAiBzuB,IAClBvB,QAAQ,OAASuB,EAAIA,EAAExC,MAAM,KAAK,KAIvCq+B,EAAsB77B,KAAKuuB,WAIrByN,OAAOvM,KAAKzvB,GACZ87B,KACJ1zB,EAAQ5L,EAAOC,OACR0xB,kBAAoBztB,EAAKw7B,gBAAmBjI,GAAgBz3B,EAAQkE,EAAKw7B,gBAChF/G,GAAgC,IAAtBz0B,EAAKy7B,cAA0B/zB,EAAM+sB,QAC/C2G,EAAqB/gB,KAAKzV,IAAM,IAAIsI,GAAUmN,KAAKzV,IAAK+c,EAAOyM,GAAgB,EAAG,EAAG1mB,EAAM+lB,gBAAiB/lB,EAAO,GAAI,IACpGmf,IAAM,GAEhB,UAANvnB,OACEsF,IAAM,IAAIsI,GAAUmN,KAAKzV,IAAK8C,EAAO,SAAUA,EAAM6lB,QAAU2N,EAAW99B,GAAesK,EAAM6lB,OAAQ2N,EAAWrY,GAAUA,GAAUnb,EAAM6lB,QAAW,EAAGZ,SAC1J/nB,IAAIwE,EAAI,EACbsW,EAAMza,KAAK,SAAU3F,GACrBA,GAAK,QACC,CAAA,GAAU,oBAANA,EAAyB,CACnC+7B,EAAYp2B,KAAKipB,GAAsB,EAAGvM,EAAMuM,KAChD4E,EAAWG,GAA8BH,GACrCprB,EAAM2mB,IACTiG,GAAgBx4B,EAAQg3B,EAAU,EAAG2B,EAAQ,EAAGpa,QAEhD0Y,EAAUt1B,WAAWq1B,EAASh2B,MAAM,KAAK,KAAO,KACpC4K,EAAMymB,SAAWsD,GAAkBpX,KAAM3S,EAAO,UAAWA,EAAMymB,QAAS4E,GACtFtB,GAAkBpX,KAAMsH,EAAOriB,EAAG44B,GAAcrF,GAAaqF,GAAcpF,cAGtE,GAAU,cAANxzB,EAAmB,CAC7Bg1B,GAAgBx4B,EAAQg3B,EAAU,EAAG2B,EAAQ,EAAGpa,eAE1C,GAAI/a,KAAKk5B,GAAuB,CACtC/C,GAAwBpb,KAAM3S,EAAOpI,EAAGyjB,EAAUmY,EAAW99B,GAAe2lB,EAAUmY,EAAWpI,GAAYA,YAGvG,GAAU,iBAANxzB,EAAsB,CAChCmyB,GAAkBpX,KAAM3S,EAAO,SAAUA,EAAM+sB,OAAQ3B,YAEjD,GAAU,YAANxzB,EAAiB,CAC3BoI,EAAMpI,GAAKwzB,WAEL,GAAU,cAANxzB,EAAmB,CAC7B02B,GAAoB3b,KAAMyY,EAAUh3B,kBAGzBwD,KAAKqiB,IACjBriB,EAAIkwB,GAAiBlwB,IAAMA,MAGxB67B,IAAwBtY,GAAqB,IAAXA,KAAkBE,GAAyB,IAAbA,KAAoByU,GAAYloB,KAAKwjB,IAAcxzB,KAAKqiB,EAEhHkB,EAAXA,GAAoB,GADpBmQ,GAAaH,EAAa,IAAIn1B,QAAQqlB,EAAW,IAAI7mB,YAErD62B,EAAU3sB,GAAQ0sB,KAAexzB,KAAKgU,EAAQI,MAASJ,EAAQI,MAAMpU,GAAK0zB,MAChDjQ,EAAW6O,GAAe91B,EAAQwD,EAAGuzB,EAAYE,SACtEnuB,IAAM,IAAIsI,GAAUmN,KAAKzV,IAAKu2B,EAAqBzzB,EAAQia,EAAOriB,EAAGyjB,GAAWmY,EAAW99B,GAAe2lB,EAAUmY,EAAWrY,GAAUA,GAAUE,EAAYoY,GAAmC,OAAZpI,GAA0B,WAANzzB,IAAsC,IAAnBU,EAAK07B,UAA+C/O,GAAxBG,SACzPloB,IAAIwE,EAAI2pB,GAAW,EACpBC,IAAcD,GAAuB,MAAZA,SACvBnuB,IAAIuE,EAAI0pB,OACRjuB,IAAIgJ,EAAIif,SAER,GAAMvtB,KAAKqiB,EAQjBgR,GAAuB1c,KAAKoE,KAAMve,EAAQwD,EAAGuzB,EAAYqI,EAAWA,EAAWpI,EAAWA,WAPtFxzB,KAAKxD,OACH0H,IAAI1H,EAAQwD,EAAGuzB,GAAc/2B,EAAOwD,GAAI47B,EAAWA,EAAWpI,EAAWA,EAAUtoB,EAAO7O,QACzF,GAAU,mBAAN2D,EAAwB,CAClCvE,EAAeuE,EAAGwzB,YAMpBqI,IAAuB77B,KAAKqiB,EAAQ0Z,EAAYp2B,KAAK3F,EAAG,EAAGqiB,EAAMriB,IAA4B,mBAAfxD,EAAOwD,GAAqB+7B,EAAYp2B,KAAK3F,EAAG,EAAGxD,EAAOwD,MAAQ+7B,EAAYp2B,KAAK3F,EAAG,EAAGuzB,GAAc/2B,EAAOwD,KAC5LogB,EAAMza,KAAK3F,GAGbkkB,GAAeW,GAA0B9J,OAG1C9b,uBAAOqe,EAAOxH,MACTA,EAAKnX,MAAMoF,QAAUxE,aACpB0iB,EAAKnM,EAAKxQ,IACP2c,GACNA,EAAG3T,EAAEgP,EAAO2E,EAAGhZ,GACfgZ,EAAKA,EAAGtgB,WAGTmU,EAAKkmB,OAAOt5B,UAGduK,IAAK0hB,GACLvhB,QAASqhB,GACTvhB,6BAAU1Q,EAAQd,EAAUsmB,OACvBhiB,EAAIyuB,GAAiB/yB,UACxBsE,GAAKA,EAAEvB,QAAQ,KAAO,IAAO/C,EAAWsE,GACjCtE,KAAY6yB,IAAmB7yB,IAAakzB,KAAyBpyB,EAAOC,MAAMsM,GAAK4lB,GAAKnyB,EAAQ,MAAUwlB,GAAU8U,KAAwB9U,EAAuB,UAAbtmB,EAAuBqyB,GAAeD,IAAqBgJ,GAAsB9U,GAAU,MAAqB,UAAbtmB,EAAuBwyB,GAAyBE,IAA+B5xB,EAAO6lB,QAAUxnB,EAAa2B,EAAO6lB,MAAM3mB,IAAaiyB,IAAmBjyB,EAAS+C,QAAQ,KAAOmvB,GAAiBzgB,GAAW3Q,EAAQd,IAE5dgxB,KAAM,CAAEsF,gBAAAA,GAAiBsC,WAAAA,KAI1B94B,GAAK6vB,MAAMgR,YAAcnM,GACzB10B,GAAKkxB,KAAK4P,cAAgBhN,GAErBmM,GAAMp+B,IADDm+B,GAQP,+CAPwC,KADfpC,GAQsB,4CAPU,iFAAc,SAAAn9B,GAASsyB,GAAgBtyB,GAAQ,IAC1GoB,GAAa+7B,GAAU,SAAAn9B,GAAS+X,EAAQI,MAAMnY,GAAQ,MAAOi9B,GAAsBj9B,GAAQ,IAC3FwyB,GAAiBgN,GAAI,KAAOD,GAAmB,IAAMpC,GACrD/7B,GAI8K,6FAJxJ,SAAApB,OACjBuB,EAAQvB,EAAKuB,MAAM,KACvBixB,GAAiBjxB,EAAM,IAAMi+B,GAAIj+B,EAAM,MAGzCH,GAAa,+EAAgF,SAAApB,GAAS+X,EAAQI,MAAMnY,GAAQ,OAE5HT,GAAKsuB,eAAe4R,QC1nCda,GAAc/gC,GAAKsuB,eAAe4R,KAAclgC,GACrDghC,GAAkBD,GAAY7P,KAAK9lB"} \ No newline at end of file diff --git a/node_modules/gsap/gsap-core.js b/node_modules/gsap/gsap-core.js new file mode 100644 index 0000000000000000000000000000000000000000..e5f5a1c2624417509e57fea6b106705fdebae8f6 --- /dev/null +++ b/node_modules/gsap/gsap-core.js @@ -0,0 +1,4502 @@ +function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } + +function _inheritsLoose(subClass, superClass) { subClass.prototype = Object.create(superClass.prototype); subClass.prototype.constructor = subClass; subClass.__proto__ = superClass; } + +/*! + * GSAP 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var _config = { + autoSleep: 120, + force3D: "auto", + nullTargetWarn: 1, + units: { + lineHeight: "" + } +}, + _defaults = { + duration: .5, + overwrite: false, + delay: 0 +}, + _suppressOverwrites, + _reverting, + _context, + _bigNum = 1e8, + _tinyNum = 1 / _bigNum, + _2PI = Math.PI * 2, + _HALF_PI = _2PI / 4, + _gsID = 0, + _sqrt = Math.sqrt, + _cos = Math.cos, + _sin = Math.sin, + _isString = function _isString(value) { + return typeof value === "string"; +}, + _isFunction = function _isFunction(value) { + return typeof value === "function"; +}, + _isNumber = function _isNumber(value) { + return typeof value === "number"; +}, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; +}, + _isObject = function _isObject(value) { + return typeof value === "object"; +}, + _isNotFalse = function _isNotFalse(value) { + return value !== false; +}, + _windowExists = function _windowExists() { + return typeof window !== "undefined"; +}, + _isFuncOrString = function _isFuncOrString(value) { + return _isFunction(value) || _isString(value); +}, + _isTypedArray = typeof ArrayBuffer === "function" && ArrayBuffer.isView || function () {}, + // note: IE10 has ArrayBuffer, but NOT ArrayBuffer.isView(). +_isArray = Array.isArray, + _strictNumExp = /(?:-?\.?\d|\.)+/gi, + //only numbers (including negatives and decimals) but NOT relative values. +_numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/g, + //finds any numbers, including ones that start with += or -=, negative numbers, and ones in scientific notation like 1e-8. +_numWithUnitExp = /[-+=.]*\d+[.e-]*\d*[a-z%]*/g, + _complexStringNumExp = /[-+=.]*\d+\.?\d*(?:e-|e\+)?\d*/gi, + //duplicate so that while we're looping through matches from exec(), it doesn't contaminate the lastIndex of _numExp which we use to search for colors too. +_relExp = /[+-]=-?[.\d]+/, + _delimitedValueExp = /[^,'"\[\]\s]+/gi, + // previously /[#\-+.]*\b[a-z\d\-=+%.]+/gi but didn't catch special characters. +_unitExp = /^[+\-=e\s\d]*\d+[.\d]*([a-z]*|%)\s*$/i, + _globalTimeline, + _win, + _coreInitted, + _doc, + _globals = {}, + _installScope = {}, + _coreReady, + _install = function _install(scope) { + return (_installScope = _merge(scope, _globals)) && gsap; +}, + _missingPlugin = function _missingPlugin(property, value) { + return console.warn("Invalid property", property, "set to", value, "Missing plugin? gsap.registerPlugin()"); +}, + _warn = function _warn(message, suppress) { + return !suppress && console.warn(message); +}, + _addGlobal = function _addGlobal(name, obj) { + return name && (_globals[name] = obj) && _installScope && (_installScope[name] = obj) || _globals; +}, + _emptyFunc = function _emptyFunc() { + return 0; +}, + _startAtRevertConfig = { + suppressEvents: true, + isStart: true, + kill: false +}, + _revertConfigNoKill = { + suppressEvents: true, + kill: false +}, + _revertConfig = { + suppressEvents: true +}, + _reservedProps = {}, + _lazyTweens = [], + _lazyLookup = {}, + _lastRenderedFrame, + _plugins = {}, + _effects = {}, + _nextGCFrame = 30, + _harnessPlugins = [], + _callbackNames = "", + _harness = function _harness(targets) { + var target = targets[0], + harnessPlugin, + i; + _isObject(target) || _isFunction(target) || (targets = [targets]); + + if (!(harnessPlugin = (target._gsap || {}).harness)) { + // find the first target with a harness. We assume targets passed into an animation will be of similar type, meaning the same kind of harness can be used for them all (performance optimization) + i = _harnessPlugins.length; + + while (i-- && !_harnessPlugins[i].targetTest(target)) {} + + harnessPlugin = _harnessPlugins[i]; + } + + i = targets.length; + + while (i--) { + targets[i] && (targets[i]._gsap || (targets[i]._gsap = new GSCache(targets[i], harnessPlugin))) || targets.splice(i, 1); + } + + return targets; +}, + _getCache = function _getCache(target) { + return target._gsap || _harness(toArray(target))[0]._gsap; +}, + _getProperty = function _getProperty(target, property, v) { + return (v = target[property]) && _isFunction(v) ? target[property]() : _isUndefined(v) && target.getAttribute && target.getAttribute(property) || v; +}, + _forEachName = function _forEachName(names, func) { + return (names = names.split(",")).forEach(func) || names; +}, + //split a comma-delimited list of names into an array, then run a forEach() function and return the split array (this is just a way to consolidate/shorten some code). +_round = function _round(value) { + return Math.round(value * 100000) / 100000 || 0; +}, + _roundPrecise = function _roundPrecise(value) { + return Math.round(value * 10000000) / 10000000 || 0; +}, + // increased precision mostly for timing values. +_parseRelative = function _parseRelative(start, value) { + var operator = value.charAt(0), + end = parseFloat(value.substr(2)); + start = parseFloat(start); + return operator === "+" ? start + end : operator === "-" ? start - end : operator === "*" ? start * end : start / end; +}, + _arrayContainsAny = function _arrayContainsAny(toSearch, toFind) { + //searches one array to find matches for any of the items in the toFind array. As soon as one is found, it returns true. It does NOT return all the matches; it's simply a boolean search. + var l = toFind.length, + i = 0; + + for (; toSearch.indexOf(toFind[i]) < 0 && ++i < l;) {} + + return i < l; +}, + _lazyRender = function _lazyRender() { + var l = _lazyTweens.length, + a = _lazyTweens.slice(0), + i, + tween; + + _lazyLookup = {}; + _lazyTweens.length = 0; + + for (i = 0; i < l; i++) { + tween = a[i]; + tween && tween._lazy && (tween.render(tween._lazy[0], tween._lazy[1], true)._lazy = 0); + } +}, + _lazySafeRender = function _lazySafeRender(animation, time, suppressEvents, force) { + _lazyTweens.length && !_reverting && _lazyRender(); + animation.render(time, suppressEvents, force || _reverting && time < 0 && (animation._initted || animation._startAt)); + _lazyTweens.length && !_reverting && _lazyRender(); //in case rendering caused any tweens to lazy-init, we should render them because typically when someone calls seek() or time() or progress(), they expect an immediate render. +}, + _numericIfPossible = function _numericIfPossible(value) { + var n = parseFloat(value); + return (n || n === 0) && (value + "").match(_delimitedValueExp).length < 2 ? n : _isString(value) ? value.trim() : value; +}, + _passThrough = function _passThrough(p) { + return p; +}, + _setDefaults = function _setDefaults(obj, defaults) { + for (var p in defaults) { + p in obj || (obj[p] = defaults[p]); + } + + return obj; +}, + _setKeyframeDefaults = function _setKeyframeDefaults(excludeDuration) { + return function (obj, defaults) { + for (var p in defaults) { + p in obj || p === "duration" && excludeDuration || p === "ease" || (obj[p] = defaults[p]); + } + }; +}, + _merge = function _merge(base, toMerge) { + for (var p in toMerge) { + base[p] = toMerge[p]; + } + + return base; +}, + _mergeDeep = function _mergeDeep(base, toMerge) { + for (var p in toMerge) { + p !== "__proto__" && p !== "constructor" && p !== "prototype" && (base[p] = _isObject(toMerge[p]) ? _mergeDeep(base[p] || (base[p] = {}), toMerge[p]) : toMerge[p]); + } + + return base; +}, + _copyExcluding = function _copyExcluding(obj, excluding) { + var copy = {}, + p; + + for (p in obj) { + p in excluding || (copy[p] = obj[p]); + } + + return copy; +}, + _inheritDefaults = function _inheritDefaults(vars) { + var parent = vars.parent || _globalTimeline, + func = vars.keyframes ? _setKeyframeDefaults(_isArray(vars.keyframes)) : _setDefaults; + + if (_isNotFalse(vars.inherit)) { + while (parent) { + func(vars, parent.vars.defaults); + parent = parent.parent || parent._dp; + } + } + + return vars; +}, + _arraysMatch = function _arraysMatch(a1, a2) { + var i = a1.length, + match = i === a2.length; + + while (match && i-- && a1[i] === a2[i]) {} + + return i < 0; +}, + _addLinkedListItem = function _addLinkedListItem(parent, child, firstProp, lastProp, sortBy) { + if (firstProp === void 0) { + firstProp = "_first"; + } + + if (lastProp === void 0) { + lastProp = "_last"; + } + + var prev = parent[lastProp], + t; + + if (sortBy) { + t = child[sortBy]; + + while (prev && prev[sortBy] > t) { + prev = prev._prev; + } + } + + if (prev) { + child._next = prev._next; + prev._next = child; + } else { + child._next = parent[firstProp]; + parent[firstProp] = child; + } + + if (child._next) { + child._next._prev = child; + } else { + parent[lastProp] = child; + } + + child._prev = prev; + child.parent = child._dp = parent; + return child; +}, + _removeLinkedListItem = function _removeLinkedListItem(parent, child, firstProp, lastProp) { + if (firstProp === void 0) { + firstProp = "_first"; + } + + if (lastProp === void 0) { + lastProp = "_last"; + } + + var prev = child._prev, + next = child._next; + + if (prev) { + prev._next = next; + } else if (parent[firstProp] === child) { + parent[firstProp] = next; + } + + if (next) { + next._prev = prev; + } else if (parent[lastProp] === child) { + parent[lastProp] = prev; + } + + child._next = child._prev = child.parent = null; // don't delete the _dp just so we can revert if necessary. But parent should be null to indicate the item isn't in a linked list. +}, + _removeFromParent = function _removeFromParent(child, onlyIfParentHasAutoRemove) { + child.parent && (!onlyIfParentHasAutoRemove || child.parent.autoRemoveChildren) && child.parent.remove && child.parent.remove(child); + child._act = 0; +}, + _uncache = function _uncache(animation, child) { + if (animation && (!child || child._end > animation._dur || child._start < 0)) { + // performance optimization: if a child animation is passed in we should only uncache if that child EXTENDS the animation (its end time is beyond the end) + var a = animation; + + while (a) { + a._dirty = 1; + a = a.parent; + } + } + + return animation; +}, + _recacheAncestors = function _recacheAncestors(animation) { + var parent = animation.parent; + + while (parent && parent.parent) { + //sometimes we must force a re-sort of all children and update the duration/totalDuration of all ancestor timelines immediately in case, for example, in the middle of a render loop, one tween alters another tween's timeScale which shoves its startTime before 0, forcing the parent timeline to shift around and shiftChildren() which could affect that next tween's render (startTime). Doesn't matter for the root timeline though. + parent._dirty = 1; + parent.totalDuration(); + parent = parent.parent; + } + + return animation; +}, + _rewindStartAt = function _rewindStartAt(tween, totalTime, suppressEvents, force) { + return tween._startAt && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween.vars.immediateRender && !tween.vars.autoRevert || tween._startAt.render(totalTime, true, force)); +}, + _hasNoPausedAncestors = function _hasNoPausedAncestors(animation) { + return !animation || animation._ts && _hasNoPausedAncestors(animation.parent); +}, + _elapsedCycleDuration = function _elapsedCycleDuration(animation) { + return animation._repeat ? _animationCycle(animation._tTime, animation = animation.duration() + animation._rDelay) * animation : 0; +}, + // feed in the totalTime and cycleDuration and it'll return the cycle (iteration minus 1) and if the playhead is exactly at the very END, it will NOT bump up to the next cycle. +_animationCycle = function _animationCycle(tTime, cycleDuration) { + var whole = Math.floor(tTime = _roundPrecise(tTime / cycleDuration)); + return tTime && whole === tTime ? whole - 1 : whole; +}, + _parentToChildTotalTime = function _parentToChildTotalTime(parentTime, child) { + return (parentTime - child._start) * child._ts + (child._ts >= 0 ? 0 : child._dirty ? child.totalDuration() : child._tDur); +}, + _setEnd = function _setEnd(animation) { + return animation._end = _roundPrecise(animation._start + (animation._tDur / Math.abs(animation._ts || animation._rts || _tinyNum) || 0)); +}, + _alignPlayhead = function _alignPlayhead(animation, totalTime) { + // adjusts the animation's _start and _end according to the provided totalTime (only if the parent's smoothChildTiming is true and the animation isn't paused). It doesn't do any rendering or forcing things back into parent timelines, etc. - that's what totalTime() is for. + var parent = animation._dp; + + if (parent && parent.smoothChildTiming && animation._ts) { + animation._start = _roundPrecise(parent._time - (animation._ts > 0 ? totalTime / animation._ts : ((animation._dirty ? animation.totalDuration() : animation._tDur) - totalTime) / -animation._ts)); + + _setEnd(animation); + + parent._dirty || _uncache(parent, animation); //for performance improvement. If the parent's cache is already dirty, it already took care of marking the ancestors as dirty too, so skip the function call here. + } + + return animation; +}, + +/* +_totalTimeToTime = (clampedTotalTime, duration, repeat, repeatDelay, yoyo) => { + let cycleDuration = duration + repeatDelay, + time = _round(clampedTotalTime % cycleDuration); + if (time > duration) { + time = duration; + } + return (yoyo && (~~(clampedTotalTime / cycleDuration) & 1)) ? duration - time : time; +}, +*/ +_postAddChecks = function _postAddChecks(timeline, child) { + var t; + + if (child._time || !child._dur && child._initted || child._start < timeline._time && (child._dur || !child.add)) { + // in case, for example, the _start is moved on a tween that has already rendered, or if it's being inserted into a timeline BEFORE where the playhead is currently. Imagine it's at its end state, then the startTime is moved WAY later (after the end of this timeline), it should render at its beginning. Special case: if it's a timeline (has .add() method) and no duration, we can skip rendering because the user may be populating it AFTER adding it to a parent timeline (unconventional, but possible, and we wouldn't want it to get removed if the parent's autoRemoveChildren is true). + t = _parentToChildTotalTime(timeline.rawTime(), child); + + if (!child._dur || _clamp(0, child.totalDuration(), t) - child._tTime > _tinyNum) { + child.render(t, true); + } + } //if the timeline has already ended but the inserted tween/timeline extends the duration, we should enable this timeline again so that it renders properly. We should also align the playhead with the parent timeline's when appropriate. + + + if (_uncache(timeline, child)._dp && timeline._initted && timeline._time >= timeline._dur && timeline._ts) { + //in case any of the ancestors had completed but should now be enabled... + if (timeline._dur < timeline.duration()) { + t = timeline; + + while (t._dp) { + t.rawTime() >= 0 && t.totalTime(t._tTime); //moves the timeline (shifts its startTime) if necessary, and also enables it. If it's currently zero, though, it may not be scheduled to render until later so there's no need to force it to align with the current playhead position. Only move to catch up with the playhead. + + t = t._dp; + } + } + + timeline._zTime = -_tinyNum; // helps ensure that the next render() will be forced (crossingStart = true in render()), even if the duration hasn't changed (we're adding a child which would need to get rendered). Definitely an edge case. Note: we MUST do this AFTER the loop above where the totalTime() might trigger a render() because this _addToTimeline() method gets called from the Animation constructor, BEFORE tweens even record their targets, etc. so we wouldn't want things to get triggered in the wrong order. + } +}, + _addToTimeline = function _addToTimeline(timeline, child, position, skipChecks) { + child.parent && _removeFromParent(child); + child._start = _roundPrecise((_isNumber(position) ? position : position || timeline !== _globalTimeline ? _parsePosition(timeline, position, child) : timeline._time) + child._delay); + child._end = _roundPrecise(child._start + (child.totalDuration() / Math.abs(child.timeScale()) || 0)); + + _addLinkedListItem(timeline, child, "_first", "_last", timeline._sort ? "_start" : 0); + + _isFromOrFromStart(child) || (timeline._recent = child); + skipChecks || _postAddChecks(timeline, child); + timeline._ts < 0 && _alignPlayhead(timeline, timeline._tTime); // if the timeline is reversed and the new child makes it longer, we may need to adjust the parent's _start (push it back) + + return timeline; +}, + _scrollTrigger = function _scrollTrigger(animation, trigger) { + return (_globals.ScrollTrigger || _missingPlugin("scrollTrigger", trigger)) && _globals.ScrollTrigger.create(trigger, animation); +}, + _attemptInitTween = function _attemptInitTween(tween, time, force, suppressEvents, tTime) { + _initTween(tween, time, tTime); + + if (!tween._initted) { + return 1; + } + + if (!force && tween._pt && !_reverting && (tween._dur && tween.vars.lazy !== false || !tween._dur && tween.vars.lazy) && _lastRenderedFrame !== _ticker.frame) { + _lazyTweens.push(tween); + + tween._lazy = [tTime, suppressEvents]; + return 1; + } +}, + _parentPlayheadIsBeforeStart = function _parentPlayheadIsBeforeStart(_ref) { + var parent = _ref.parent; + return parent && parent._ts && parent._initted && !parent._lock && (parent.rawTime() < 0 || _parentPlayheadIsBeforeStart(parent)); +}, + // check parent's _lock because when a timeline repeats/yoyos and does its artificial wrapping, we shouldn't force the ratio back to 0 +_isFromOrFromStart = function _isFromOrFromStart(_ref2) { + var data = _ref2.data; + return data === "isFromStart" || data === "isStart"; +}, + _renderZeroDurationTween = function _renderZeroDurationTween(tween, totalTime, suppressEvents, force) { + var prevRatio = tween.ratio, + ratio = totalTime < 0 || !totalTime && (!tween._start && _parentPlayheadIsBeforeStart(tween) && !(!tween._initted && _isFromOrFromStart(tween)) || (tween._ts < 0 || tween._dp._ts < 0) && !_isFromOrFromStart(tween)) ? 0 : 1, + // if the tween or its parent is reversed and the totalTime is 0, we should go to a ratio of 0. Edge case: if a from() or fromTo() stagger tween is placed later in a timeline, the "startAt" zero-duration tween could initially render at a time when the parent timeline's playhead is technically BEFORE where this tween is, so make sure that any "from" and "fromTo" startAt tweens are rendered the first time at a ratio of 1. + repeatDelay = tween._rDelay, + tTime = 0, + pt, + iteration, + prevIteration; + + if (repeatDelay && tween._repeat) { + // in case there's a zero-duration tween that has a repeat with a repeatDelay + tTime = _clamp(0, tween._tDur, totalTime); + iteration = _animationCycle(tTime, repeatDelay); + tween._yoyo && iteration & 1 && (ratio = 1 - ratio); + + if (iteration !== _animationCycle(tween._tTime, repeatDelay)) { + // if iteration changed + prevRatio = 1 - ratio; + tween.vars.repeatRefresh && tween._initted && tween.invalidate(); + } + } + + if (ratio !== prevRatio || _reverting || force || tween._zTime === _tinyNum || !totalTime && tween._zTime) { + if (!tween._initted && _attemptInitTween(tween, totalTime, force, suppressEvents, tTime)) { + // if we render the very beginning (time == 0) of a fromTo(), we must force the render (normal tweens wouldn't need to render at a time of 0 when the prevTime was also 0). This is also mandatory to make sure overwriting kicks in immediately. + return; + } + + prevIteration = tween._zTime; + tween._zTime = totalTime || (suppressEvents ? _tinyNum : 0); // when the playhead arrives at EXACTLY time 0 (right on top) of a zero-duration tween, we need to discern if events are suppressed so that when the playhead moves again (next time), it'll trigger the callback. If events are NOT suppressed, obviously the callback would be triggered in this render. Basically, the callback should fire either when the playhead ARRIVES or LEAVES this exact spot, not both. Imagine doing a timeline.seek(0) and there's a callback that sits at 0. Since events are suppressed on that seek() by default, nothing will fire, but when the playhead moves off of that position, the callback should fire. This behavior is what people intuitively expect. + + suppressEvents || (suppressEvents = totalTime && !prevIteration); // if it was rendered previously at exactly 0 (_zTime) and now the playhead is moving away, DON'T fire callbacks otherwise they'll seem like duplicates. + + tween.ratio = ratio; + tween._from && (ratio = 1 - ratio); + tween._time = 0; + tween._tTime = tTime; + pt = tween._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + totalTime < 0 && _rewindStartAt(tween, totalTime, suppressEvents, true); + tween._onUpdate && !suppressEvents && _callback(tween, "onUpdate"); + tTime && tween._repeat && !suppressEvents && tween.parent && _callback(tween, "onRepeat"); + + if ((totalTime >= tween._tDur || totalTime < 0) && tween.ratio === ratio) { + ratio && _removeFromParent(tween, 1); + + if (!suppressEvents && !_reverting) { + _callback(tween, ratio ? "onComplete" : "onReverseComplete", true); + + tween._prom && tween._prom(); + } + } + } else if (!tween._zTime) { + tween._zTime = totalTime; + } +}, + _findNextPauseTween = function _findNextPauseTween(animation, prevTime, time) { + var child; + + if (time > prevTime) { + child = animation._first; + + while (child && child._start <= time) { + if (child.data === "isPause" && child._start > prevTime) { + return child; + } + + child = child._next; + } + } else { + child = animation._last; + + while (child && child._start >= time) { + if (child.data === "isPause" && child._start < prevTime) { + return child; + } + + child = child._prev; + } + } +}, + _setDuration = function _setDuration(animation, duration, skipUncache, leavePlayhead) { + var repeat = animation._repeat, + dur = _roundPrecise(duration) || 0, + totalProgress = animation._tTime / animation._tDur; + totalProgress && !leavePlayhead && (animation._time *= dur / animation._dur); + animation._dur = dur; + animation._tDur = !repeat ? dur : repeat < 0 ? 1e10 : _roundPrecise(dur * (repeat + 1) + animation._rDelay * repeat); + totalProgress > 0 && !leavePlayhead && _alignPlayhead(animation, animation._tTime = animation._tDur * totalProgress); + animation.parent && _setEnd(animation); + skipUncache || _uncache(animation.parent, animation); + return animation; +}, + _onUpdateTotalDuration = function _onUpdateTotalDuration(animation) { + return animation instanceof Timeline ? _uncache(animation) : _setDuration(animation, animation._dur); +}, + _zeroPosition = { + _start: 0, + endTime: _emptyFunc, + totalDuration: _emptyFunc +}, + _parsePosition = function _parsePosition(animation, position, percentAnimation) { + var labels = animation.labels, + recent = animation._recent || _zeroPosition, + clippedDuration = animation.duration() >= _bigNum ? recent.endTime(false) : animation._dur, + //in case there's a child that infinitely repeats, users almost never intend for the insertion point of a new child to be based on a SUPER long value like that so we clip it and assume the most recently-added child's endTime should be used instead. + i, + offset, + isPercent; + + if (_isString(position) && (isNaN(position) || position in labels)) { + //if the string is a number like "1", check to see if there's a label with that name, otherwise interpret it as a number (absolute value). + offset = position.charAt(0); + isPercent = position.substr(-1) === "%"; + i = position.indexOf("="); + + if (offset === "<" || offset === ">") { + i >= 0 && (position = position.replace(/=/, "")); + return (offset === "<" ? recent._start : recent.endTime(recent._repeat >= 0)) + (parseFloat(position.substr(1)) || 0) * (isPercent ? (i < 0 ? recent : percentAnimation).totalDuration() / 100 : 1); + } + + if (i < 0) { + position in labels || (labels[position] = clippedDuration); + return labels[position]; + } + + offset = parseFloat(position.charAt(i - 1) + position.substr(i + 1)); + + if (isPercent && percentAnimation) { + offset = offset / 100 * (_isArray(percentAnimation) ? percentAnimation[0] : percentAnimation).totalDuration(); + } + + return i > 1 ? _parsePosition(animation, position.substr(0, i - 1), percentAnimation) + offset : clippedDuration + offset; + } + + return position == null ? clippedDuration : +position; +}, + _createTweenType = function _createTweenType(type, params, timeline) { + var isLegacy = _isNumber(params[1]), + varsIndex = (isLegacy ? 2 : 1) + (type < 2 ? 0 : 1), + vars = params[varsIndex], + irVars, + parent; + + isLegacy && (vars.duration = params[1]); + vars.parent = timeline; + + if (type) { + irVars = vars; + parent = timeline; + + while (parent && !("immediateRender" in irVars)) { + // inheritance hasn't happened yet, but someone may have set a default in an ancestor timeline. We could do vars.immediateRender = _isNotFalse(_inheritDefaults(vars).immediateRender) but that'd exact a slight performance penalty because _inheritDefaults() also runs in the Tween constructor. We're paying a small kb price here to gain speed. + irVars = parent.vars.defaults || {}; + parent = _isNotFalse(parent.vars.inherit) && parent.parent; + } + + vars.immediateRender = _isNotFalse(irVars.immediateRender); + type < 2 ? vars.runBackwards = 1 : vars.startAt = params[varsIndex - 1]; // "from" vars + } + + return new Tween(params[0], vars, params[varsIndex + 1]); +}, + _conditionalReturn = function _conditionalReturn(value, func) { + return value || value === 0 ? func(value) : func; +}, + _clamp = function _clamp(min, max, value) { + return value < min ? min : value > max ? max : value; +}, + getUnit = function getUnit(value, v) { + return !_isString(value) || !(v = _unitExp.exec(value)) ? "" : v[1]; +}, + // note: protect against padded numbers as strings, like "100.100". That shouldn't return "00" as the unit. If it's numeric, return no unit. +clamp = function clamp(min, max, value) { + return _conditionalReturn(value, function (v) { + return _clamp(min, max, v); + }); +}, + _slice = [].slice, + _isArrayLike = function _isArrayLike(value, nonEmpty) { + return value && _isObject(value) && "length" in value && (!nonEmpty && !value.length || value.length - 1 in value && _isObject(value[0])) && !value.nodeType && value !== _win; +}, + _flatten = function _flatten(ar, leaveStrings, accumulator) { + if (accumulator === void 0) { + accumulator = []; + } + + return ar.forEach(function (value) { + var _accumulator; + + return _isString(value) && !leaveStrings || _isArrayLike(value, 1) ? (_accumulator = accumulator).push.apply(_accumulator, toArray(value)) : accumulator.push(value); + }) || accumulator; +}, + //takes any value and returns an array. If it's a string (and leaveStrings isn't true), it'll use document.querySelectorAll() and convert that to an array. It'll also accept iterables like jQuery objects. +toArray = function toArray(value, scope, leaveStrings) { + return _context && !scope && _context.selector ? _context.selector(value) : _isString(value) && !leaveStrings && (_coreInitted || !_wake()) ? _slice.call((scope || _doc).querySelectorAll(value), 0) : _isArray(value) ? _flatten(value, leaveStrings) : _isArrayLike(value) ? _slice.call(value, 0) : value ? [value] : []; +}, + selector = function selector(value) { + value = toArray(value)[0] || _warn("Invalid scope") || {}; + return function (v) { + var el = value.current || value.nativeElement || value; + return toArray(v, el.querySelectorAll ? el : el === value ? _warn("Invalid scope") || _doc.createElement("div") : value); + }; +}, + shuffle = function shuffle(a) { + return a.sort(function () { + return .5 - Math.random(); + }); +}, + // alternative that's a bit faster and more reliably diverse but bigger: for (let j, v, i = a.length; i; j = (Math.random() * i) | 0, v = a[--i], a[i] = a[j], a[j] = v); return a; +//for distributing values across an array. Can accept a number, a function or (most commonly) a function which can contain the following properties: {base, amount, from, ease, grid, axis, length, each}. Returns a function that expects the following parameters: index, target, array. Recognizes the following +distribute = function distribute(v) { + if (_isFunction(v)) { + return v; + } + + var vars = _isObject(v) ? v : { + each: v + }, + //n:1 is just to indicate v was a number; we leverage that later to set v according to the length we get. If a number is passed in, we treat it like the old stagger value where 0.1, for example, would mean that things would be distributed with 0.1 between each element in the array rather than a total "amount" that's chunked out among them all. + ease = _parseEase(vars.ease), + from = vars.from || 0, + base = parseFloat(vars.base) || 0, + cache = {}, + isDecimal = from > 0 && from < 1, + ratios = isNaN(from) || isDecimal, + axis = vars.axis, + ratioX = from, + ratioY = from; + + if (_isString(from)) { + ratioX = ratioY = { + center: .5, + edges: .5, + end: 1 + }[from] || 0; + } else if (!isDecimal && ratios) { + ratioX = from[0]; + ratioY = from[1]; + } + + return function (i, target, a) { + var l = (a || vars).length, + distances = cache[l], + originX, + originY, + x, + y, + d, + j, + max, + min, + wrapAt; + + if (!distances) { + wrapAt = vars.grid === "auto" ? 0 : (vars.grid || [1, _bigNum])[1]; + + if (!wrapAt) { + max = -_bigNum; + + while (max < (max = a[wrapAt++].getBoundingClientRect().left) && wrapAt < l) {} + + wrapAt < l && wrapAt--; + } + + distances = cache[l] = []; + originX = ratios ? Math.min(wrapAt, l) * ratioX - .5 : from % wrapAt; + originY = wrapAt === _bigNum ? 0 : ratios ? l * ratioY / wrapAt - .5 : from / wrapAt | 0; + max = 0; + min = _bigNum; + + for (j = 0; j < l; j++) { + x = j % wrapAt - originX; + y = originY - (j / wrapAt | 0); + distances[j] = d = !axis ? _sqrt(x * x + y * y) : Math.abs(axis === "y" ? y : x); + d > max && (max = d); + d < min && (min = d); + } + + from === "random" && shuffle(distances); + distances.max = max - min; + distances.min = min; + distances.v = l = (parseFloat(vars.amount) || parseFloat(vars.each) * (wrapAt > l ? l - 1 : !axis ? Math.max(wrapAt, l / wrapAt) : axis === "y" ? l / wrapAt : wrapAt) || 0) * (from === "edges" ? -1 : 1); + distances.b = l < 0 ? base - l : base; + distances.u = getUnit(vars.amount || vars.each) || 0; //unit + + ease = ease && l < 0 ? _invertEase(ease) : ease; + } + + l = (distances[i] - distances.min) / distances.max || 0; + return _roundPrecise(distances.b + (ease ? ease(l) : l) * distances.v) + distances.u; //round in order to work around floating point errors + }; +}, + _roundModifier = function _roundModifier(v) { + //pass in 0.1 get a function that'll round to the nearest tenth, or 5 to round to the closest 5, or 0.001 to the closest 1000th, etc. + var p = Math.pow(10, ((v + "").split(".")[1] || "").length); //to avoid floating point math errors (like 24 * 0.1 == 2.4000000000000004), we chop off at a specific number of decimal places (much faster than toFixed()) + + return function (raw) { + var n = _roundPrecise(Math.round(parseFloat(raw) / v) * v * p); + + return (n - n % 1) / p + (_isNumber(raw) ? 0 : getUnit(raw)); // n - n % 1 replaces Math.floor() in order to handle negative values properly. For example, Math.floor(-150.00000000000003) is 151! + }; +}, + snap = function snap(snapTo, value) { + var isArray = _isArray(snapTo), + radius, + is2D; + + if (!isArray && _isObject(snapTo)) { + radius = isArray = snapTo.radius || _bigNum; + + if (snapTo.values) { + snapTo = toArray(snapTo.values); + + if (is2D = !_isNumber(snapTo[0])) { + radius *= radius; //performance optimization so we don't have to Math.sqrt() in the loop. + } + } else { + snapTo = _roundModifier(snapTo.increment); + } + } + + return _conditionalReturn(value, !isArray ? _roundModifier(snapTo) : _isFunction(snapTo) ? function (raw) { + is2D = snapTo(raw); + return Math.abs(is2D - raw) <= radius ? is2D : raw; + } : function (raw) { + var x = parseFloat(is2D ? raw.x : raw), + y = parseFloat(is2D ? raw.y : 0), + min = _bigNum, + closest = 0, + i = snapTo.length, + dx, + dy; + + while (i--) { + if (is2D) { + dx = snapTo[i].x - x; + dy = snapTo[i].y - y; + dx = dx * dx + dy * dy; + } else { + dx = Math.abs(snapTo[i] - x); + } + + if (dx < min) { + min = dx; + closest = i; + } + } + + closest = !radius || min <= radius ? snapTo[closest] : raw; + return is2D || closest === raw || _isNumber(raw) ? closest : closest + getUnit(raw); + }); +}, + random = function random(min, max, roundingIncrement, returnFunction) { + return _conditionalReturn(_isArray(min) ? !max : roundingIncrement === true ? !!(roundingIncrement = 0) : !returnFunction, function () { + return _isArray(min) ? min[~~(Math.random() * min.length)] : (roundingIncrement = roundingIncrement || 1e-5) && (returnFunction = roundingIncrement < 1 ? Math.pow(10, (roundingIncrement + "").length - 2) : 1) && Math.floor(Math.round((min - roundingIncrement / 2 + Math.random() * (max - min + roundingIncrement * .99)) / roundingIncrement) * roundingIncrement * returnFunction) / returnFunction; + }); +}, + pipe = function pipe() { + for (var _len = arguments.length, functions = new Array(_len), _key = 0; _key < _len; _key++) { + functions[_key] = arguments[_key]; + } + + return function (value) { + return functions.reduce(function (v, f) { + return f(v); + }, value); + }; +}, + unitize = function unitize(func, unit) { + return function (value) { + return func(parseFloat(value)) + (unit || getUnit(value)); + }; +}, + normalize = function normalize(min, max, value) { + return mapRange(min, max, 0, 1, value); +}, + _wrapArray = function _wrapArray(a, wrapper, value) { + return _conditionalReturn(value, function (index) { + return a[~~wrapper(index)]; + }); +}, + wrap = function wrap(min, max, value) { + // NOTE: wrap() CANNOT be an arrow function! A very odd compiling bug causes problems (unrelated to GSAP). + var range = max - min; + return _isArray(min) ? _wrapArray(min, wrap(0, min.length), max) : _conditionalReturn(value, function (value) { + return (range + (value - min) % range) % range + min; + }); +}, + wrapYoyo = function wrapYoyo(min, max, value) { + var range = max - min, + total = range * 2; + return _isArray(min) ? _wrapArray(min, wrapYoyo(0, min.length - 1), max) : _conditionalReturn(value, function (value) { + value = (total + (value - min) % total) % total || 0; + return min + (value > range ? total - value : value); + }); +}, + _replaceRandom = function _replaceRandom(value) { + //replaces all occurrences of random(...) in a string with the calculated random value. can be a range like random(-100, 100, 5) or an array like random([0, 100, 500]) + var prev = 0, + s = "", + i, + nums, + end, + isArray; + + while (~(i = value.indexOf("random(", prev))) { + end = value.indexOf(")", i); + isArray = value.charAt(i + 7) === "["; + nums = value.substr(i + 7, end - i - 7).match(isArray ? _delimitedValueExp : _strictNumExp); + s += value.substr(prev, i - prev) + random(isArray ? nums : +nums[0], isArray ? 0 : +nums[1], +nums[2] || 1e-5); + prev = end + 1; + } + + return s + value.substr(prev, value.length - prev); +}, + mapRange = function mapRange(inMin, inMax, outMin, outMax, value) { + var inRange = inMax - inMin, + outRange = outMax - outMin; + return _conditionalReturn(value, function (value) { + return outMin + ((value - inMin) / inRange * outRange || 0); + }); +}, + interpolate = function interpolate(start, end, progress, mutate) { + var func = isNaN(start + end) ? 0 : function (p) { + return (1 - p) * start + p * end; + }; + + if (!func) { + var isString = _isString(start), + master = {}, + p, + i, + interpolators, + l, + il; + + progress === true && (mutate = 1) && (progress = null); + + if (isString) { + start = { + p: start + }; + end = { + p: end + }; + } else if (_isArray(start) && !_isArray(end)) { + interpolators = []; + l = start.length; + il = l - 2; + + for (i = 1; i < l; i++) { + interpolators.push(interpolate(start[i - 1], start[i])); //build the interpolators up front as a performance optimization so that when the function is called many times, it can just reuse them. + } + + l--; + + func = function func(p) { + p *= l; + var i = Math.min(il, ~~p); + return interpolators[i](p - i); + }; + + progress = end; + } else if (!mutate) { + start = _merge(_isArray(start) ? [] : {}, start); + } + + if (!interpolators) { + for (p in end) { + _addPropTween.call(master, start, p, "get", end[p]); + } + + func = function func(p) { + return _renderPropTweens(p, master) || (isString ? start.p : start); + }; + } + } + + return _conditionalReturn(progress, func); +}, + _getLabelInDirection = function _getLabelInDirection(timeline, fromTime, backward) { + //used for nextLabel() and previousLabel() + var labels = timeline.labels, + min = _bigNum, + p, + distance, + label; + + for (p in labels) { + distance = labels[p] - fromTime; + + if (distance < 0 === !!backward && distance && min > (distance = Math.abs(distance))) { + label = p; + min = distance; + } + } + + return label; +}, + _callback = function _callback(animation, type, executeLazyFirst) { + var v = animation.vars, + callback = v[type], + prevContext = _context, + context = animation._ctx, + params, + scope, + result; + + if (!callback) { + return; + } + + params = v[type + "Params"]; + scope = v.callbackScope || animation; + executeLazyFirst && _lazyTweens.length && _lazyRender(); //in case rendering caused any tweens to lazy-init, we should render them because typically when a timeline finishes, users expect things to have rendered fully. Imagine an onUpdate on a timeline that reports/checks tweened values. + + context && (_context = context); + result = params ? callback.apply(scope, params) : callback.call(scope); + _context = prevContext; + return result; +}, + _interrupt = function _interrupt(animation) { + _removeFromParent(animation); + + animation.scrollTrigger && animation.scrollTrigger.kill(!!_reverting); + animation.progress() < 1 && _callback(animation, "onInterrupt"); + return animation; +}, + _quickTween, + _registerPluginQueue = [], + _createPlugin = function _createPlugin(config) { + if (!config) return; + config = !config.name && config["default"] || config; // UMD packaging wraps things oddly, so for example MotionPathHelper becomes {MotionPathHelper:MotionPathHelper, default:MotionPathHelper}. + + if (_windowExists() || config.headless) { + // edge case: some build tools may pass in a null/undefined value + var name = config.name, + isFunc = _isFunction(config), + Plugin = name && !isFunc && config.init ? function () { + this._props = []; + } : config, + //in case someone passes in an object that's not a plugin, like CustomEase + instanceDefaults = { + init: _emptyFunc, + render: _renderPropTweens, + add: _addPropTween, + kill: _killPropTweensOf, + modifier: _addPluginModifier, + rawVars: 0 + }, + statics = { + targetTest: 0, + get: 0, + getSetter: _getSetter, + aliases: {}, + register: 0 + }; + + _wake(); + + if (config !== Plugin) { + if (_plugins[name]) { + return; + } + + _setDefaults(Plugin, _setDefaults(_copyExcluding(config, instanceDefaults), statics)); //static methods + + + _merge(Plugin.prototype, _merge(instanceDefaults, _copyExcluding(config, statics))); //instance methods + + + _plugins[Plugin.prop = name] = Plugin; + + if (config.targetTest) { + _harnessPlugins.push(Plugin); + + _reservedProps[name] = 1; + } + + name = (name === "css" ? "CSS" : name.charAt(0).toUpperCase() + name.substr(1)) + "Plugin"; //for the global name. "motionPath" should become MotionPathPlugin + } + + _addGlobal(name, Plugin); + + config.register && config.register(gsap, Plugin, PropTween); + } else { + _registerPluginQueue.push(config); + } +}, + +/* + * -------------------------------------------------------------------------------------- + * COLORS + * -------------------------------------------------------------------------------------- + */ +_255 = 255, + _colorLookup = { + aqua: [0, _255, _255], + lime: [0, _255, 0], + silver: [192, 192, 192], + black: [0, 0, 0], + maroon: [128, 0, 0], + teal: [0, 128, 128], + blue: [0, 0, _255], + navy: [0, 0, 128], + white: [_255, _255, _255], + olive: [128, 128, 0], + yellow: [_255, _255, 0], + orange: [_255, 165, 0], + gray: [128, 128, 128], + purple: [128, 0, 128], + green: [0, 128, 0], + red: [_255, 0, 0], + pink: [_255, 192, 203], + cyan: [0, _255, _255], + transparent: [_255, _255, _255, 0] +}, + // possible future idea to replace the hard-coded color name values - put this in the ticker.wake() where we set the _doc: +// let ctx = _doc.createElement("canvas").getContext("2d"); +// _forEachName("aqua,lime,silver,black,maroon,teal,blue,navy,white,olive,yellow,orange,gray,purple,green,red,pink,cyan", color => {ctx.fillStyle = color; _colorLookup[color] = splitColor(ctx.fillStyle)}); +_hue = function _hue(h, m1, m2) { + h += h < 0 ? 1 : h > 1 ? -1 : 0; + return (h * 6 < 1 ? m1 + (m2 - m1) * h * 6 : h < .5 ? m2 : h * 3 < 2 ? m1 + (m2 - m1) * (2 / 3 - h) * 6 : m1) * _255 + .5 | 0; +}, + splitColor = function splitColor(v, toHSL, forceAlpha) { + var a = !v ? _colorLookup.black : _isNumber(v) ? [v >> 16, v >> 8 & _255, v & _255] : 0, + r, + g, + b, + h, + s, + l, + max, + min, + d, + wasHSL; + + if (!a) { + if (v.substr(-1) === ",") { + //sometimes a trailing comma is included and we should chop it off (typically from a comma-delimited list of values like a textShadow:"2px 2px 2px blue, 5px 5px 5px rgb(255,0,0)" - in this example "blue," has a trailing comma. We could strip it out inside parseComplex() but we'd need to do it to the beginning and ending values plus it wouldn't provide protection from other potential scenarios like if the user passes in a similar value. + v = v.substr(0, v.length - 1); + } + + if (_colorLookup[v]) { + a = _colorLookup[v]; + } else if (v.charAt(0) === "#") { + if (v.length < 6) { + //for shorthand like #9F0 or #9F0F (could have alpha) + r = v.charAt(1); + g = v.charAt(2); + b = v.charAt(3); + v = "#" + r + r + g + g + b + b + (v.length === 5 ? v.charAt(4) + v.charAt(4) : ""); + } + + if (v.length === 9) { + // hex with alpha, like #fd5e53ff + a = parseInt(v.substr(1, 6), 16); + return [a >> 16, a >> 8 & _255, a & _255, parseInt(v.substr(7), 16) / 255]; + } + + v = parseInt(v.substr(1), 16); + a = [v >> 16, v >> 8 & _255, v & _255]; + } else if (v.substr(0, 3) === "hsl") { + a = wasHSL = v.match(_strictNumExp); + + if (!toHSL) { + h = +a[0] % 360 / 360; + s = +a[1] / 100; + l = +a[2] / 100; + g = l <= .5 ? l * (s + 1) : l + s - l * s; + r = l * 2 - g; + a.length > 3 && (a[3] *= 1); //cast as number + + a[0] = _hue(h + 1 / 3, r, g); + a[1] = _hue(h, r, g); + a[2] = _hue(h - 1 / 3, r, g); + } else if (~v.indexOf("=")) { + //if relative values are found, just return the raw strings with the relative prefixes in place. + a = v.match(_numExp); + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + } + } else { + a = v.match(_strictNumExp) || _colorLookup.transparent; + } + + a = a.map(Number); + } + + if (toHSL && !wasHSL) { + r = a[0] / _255; + g = a[1] / _255; + b = a[2] / _255; + max = Math.max(r, g, b); + min = Math.min(r, g, b); + l = (max + min) / 2; + + if (max === min) { + h = s = 0; + } else { + d = max - min; + s = l > 0.5 ? d / (2 - max - min) : d / (max + min); + h = max === r ? (g - b) / d + (g < b ? 6 : 0) : max === g ? (b - r) / d + 2 : (r - g) / d + 4; + h *= 60; + } + + a[0] = ~~(h + .5); + a[1] = ~~(s * 100 + .5); + a[2] = ~~(l * 100 + .5); + } + + forceAlpha && a.length < 4 && (a[3] = 1); + return a; +}, + _colorOrderData = function _colorOrderData(v) { + // strips out the colors from the string, finds all the numeric slots (with units) and returns an array of those. The Array also has a "c" property which is an Array of the index values where the colors belong. This is to help work around issues where there's a mis-matched order of color/numeric data like drop-shadow(#f00 0px 1px 2px) and drop-shadow(0x 1px 2px #f00). This is basically a helper function used in _formatColors() + var values = [], + c = [], + i = -1; + v.split(_colorExp).forEach(function (v) { + var a = v.match(_numWithUnitExp) || []; + values.push.apply(values, a); + c.push(i += a.length + 1); + }); + values.c = c; + return values; +}, + _formatColors = function _formatColors(s, toHSL, orderMatchData) { + var result = "", + colors = (s + result).match(_colorExp), + type = toHSL ? "hsla(" : "rgba(", + i = 0, + c, + shell, + d, + l; + + if (!colors) { + return s; + } + + colors = colors.map(function (color) { + return (color = splitColor(color, toHSL, 1)) && type + (toHSL ? color[0] + "," + color[1] + "%," + color[2] + "%," + color[3] : color.join(",")) + ")"; + }); + + if (orderMatchData) { + d = _colorOrderData(s); + c = orderMatchData.c; + + if (c.join(result) !== d.c.join(result)) { + shell = s.replace(_colorExp, "1").split(_numWithUnitExp); + l = shell.length - 1; + + for (; i < l; i++) { + result += shell[i] + (~c.indexOf(i) ? colors.shift() || type + "0,0,0,0)" : (d.length ? d : colors.length ? colors : orderMatchData).shift()); + } + } + } + + if (!shell) { + shell = s.split(_colorExp); + l = shell.length - 1; + + for (; i < l; i++) { + result += shell[i] + colors[i]; + } + } + + return result + shell[l]; +}, + _colorExp = function () { + var s = "(?:\\b(?:(?:rgb|rgba|hsl|hsla)\\(.+?\\))|\\B#(?:[0-9a-f]{3,4}){1,2}\\b", + //we'll dynamically build this Regular Expression to conserve file size. After building it, it will be able to find rgb(), rgba(), # (hexadecimal), and named color values like red, blue, purple, etc., + p; + + for (p in _colorLookup) { + s += "|" + p + "\\b"; + } + + return new RegExp(s + ")", "gi"); +}(), + _hslExp = /hsl[a]?\(/, + _colorStringFilter = function _colorStringFilter(a) { + var combined = a.join(" "), + toHSL; + _colorExp.lastIndex = 0; + + if (_colorExp.test(combined)) { + toHSL = _hslExp.test(combined); + a[1] = _formatColors(a[1], toHSL); + a[0] = _formatColors(a[0], toHSL, _colorOrderData(a[1])); // make sure the order of numbers/colors match with the END value. + + return true; + } +}, + +/* + * -------------------------------------------------------------------------------------- + * TICKER + * -------------------------------------------------------------------------------------- + */ +_tickerActive, + _ticker = function () { + var _getTime = Date.now, + _lagThreshold = 500, + _adjustedLag = 33, + _startTime = _getTime(), + _lastUpdate = _startTime, + _gap = 1000 / 240, + _nextTime = _gap, + _listeners = [], + _id, + _req, + _raf, + _self, + _delta, + _i, + _tick = function _tick(v) { + var elapsed = _getTime() - _lastUpdate, + manual = v === true, + overlap, + dispatch, + time, + frame; + + (elapsed > _lagThreshold || elapsed < 0) && (_startTime += elapsed - _adjustedLag); + _lastUpdate += elapsed; + time = _lastUpdate - _startTime; + overlap = time - _nextTime; + + if (overlap > 0 || manual) { + frame = ++_self.frame; + _delta = time - _self.time * 1000; + _self.time = time = time / 1000; + _nextTime += overlap + (overlap >= _gap ? 4 : _gap - overlap); + dispatch = 1; + } + + manual || (_id = _req(_tick)); //make sure the request is made before we dispatch the "tick" event so that timing is maintained. Otherwise, if processing the "tick" requires a bunch of time (like 15ms) and we're using a setTimeout() that's based on 16.7ms, it'd technically take 31.7ms between frames otherwise. + + if (dispatch) { + for (_i = 0; _i < _listeners.length; _i++) { + // use _i and check _listeners.length instead of a variable because a listener could get removed during the loop, and if that happens to an element less than the current index, it'd throw things off in the loop. + _listeners[_i](time, _delta, frame, v); + } + } + }; + + _self = { + time: 0, + frame: 0, + tick: function tick() { + _tick(true); + }, + deltaRatio: function deltaRatio(fps) { + return _delta / (1000 / (fps || 60)); + }, + wake: function wake() { + if (_coreReady) { + if (!_coreInitted && _windowExists()) { + _win = _coreInitted = window; + _doc = _win.document || {}; + _globals.gsap = gsap; + (_win.gsapVersions || (_win.gsapVersions = [])).push(gsap.version); + + _install(_installScope || _win.GreenSockGlobals || !_win.gsap && _win || {}); + + _registerPluginQueue.forEach(_createPlugin); + } + + _raf = typeof requestAnimationFrame !== "undefined" && requestAnimationFrame; + _id && _self.sleep(); + + _req = _raf || function (f) { + return setTimeout(f, _nextTime - _self.time * 1000 + 1 | 0); + }; + + _tickerActive = 1; + + _tick(2); + } + }, + sleep: function sleep() { + (_raf ? cancelAnimationFrame : clearTimeout)(_id); + _tickerActive = 0; + _req = _emptyFunc; + }, + lagSmoothing: function lagSmoothing(threshold, adjustedLag) { + _lagThreshold = threshold || Infinity; // zero should be interpreted as basically unlimited + + _adjustedLag = Math.min(adjustedLag || 33, _lagThreshold); + }, + fps: function fps(_fps) { + _gap = 1000 / (_fps || 240); + _nextTime = _self.time * 1000 + _gap; + }, + add: function add(callback, once, prioritize) { + var func = once ? function (t, d, f, v) { + callback(t, d, f, v); + + _self.remove(func); + } : callback; + + _self.remove(callback); + + _listeners[prioritize ? "unshift" : "push"](func); + + _wake(); + + return func; + }, + remove: function remove(callback, i) { + ~(i = _listeners.indexOf(callback)) && _listeners.splice(i, 1) && _i >= i && _i--; + }, + _listeners: _listeners + }; + return _self; +}(), + _wake = function _wake() { + return !_tickerActive && _ticker.wake(); +}, + //also ensures the core classes are initialized. + +/* +* ------------------------------------------------- +* EASING +* ------------------------------------------------- +*/ +_easeMap = {}, + _customEaseExp = /^[\d.\-M][\d.\-,\s]/, + _quotesExp = /["']/g, + _parseObjectInString = function _parseObjectInString(value) { + //takes a string like "{wiggles:10, type:anticipate})" and turns it into a real object. Notice it ends in ")" and includes the {} wrappers. This is because we only use this function for parsing ease configs and prioritized optimization rather than reusability. + var obj = {}, + split = value.substr(1, value.length - 3).split(":"), + key = split[0], + i = 1, + l = split.length, + index, + val, + parsedVal; + + for (; i < l; i++) { + val = split[i]; + index = i !== l - 1 ? val.lastIndexOf(",") : val.length; + parsedVal = val.substr(0, index); + obj[key] = isNaN(parsedVal) ? parsedVal.replace(_quotesExp, "").trim() : +parsedVal; + key = val.substr(index + 1).trim(); + } + + return obj; +}, + _valueInParentheses = function _valueInParentheses(value) { + var open = value.indexOf("(") + 1, + close = value.indexOf(")"), + nested = value.indexOf("(", open); + return value.substring(open, ~nested && nested < close ? value.indexOf(")", close + 1) : close); +}, + _configEaseFromString = function _configEaseFromString(name) { + //name can be a string like "elastic.out(1,0.5)", and pass in _easeMap as obj and it'll parse it out and call the actual function like _easeMap.Elastic.easeOut.config(1,0.5). It will also parse custom ease strings as long as CustomEase is loaded and registered (internally as _easeMap._CE). + var split = (name + "").split("("), + ease = _easeMap[split[0]]; + return ease && split.length > 1 && ease.config ? ease.config.apply(null, ~name.indexOf("{") ? [_parseObjectInString(split[1])] : _valueInParentheses(name).split(",").map(_numericIfPossible)) : _easeMap._CE && _customEaseExp.test(name) ? _easeMap._CE("", name) : ease; +}, + _invertEase = function _invertEase(ease) { + return function (p) { + return 1 - ease(1 - p); + }; +}, + // allow yoyoEase to be set in children and have those affected when the parent/ancestor timeline yoyos. +_propagateYoyoEase = function _propagateYoyoEase(timeline, isYoyo) { + var child = timeline._first, + ease; + + while (child) { + if (child instanceof Timeline) { + _propagateYoyoEase(child, isYoyo); + } else if (child.vars.yoyoEase && (!child._yoyo || !child._repeat) && child._yoyo !== isYoyo) { + if (child.timeline) { + _propagateYoyoEase(child.timeline, isYoyo); + } else { + ease = child._ease; + child._ease = child._yEase; + child._yEase = ease; + child._yoyo = isYoyo; + } + } + + child = child._next; + } +}, + _parseEase = function _parseEase(ease, defaultEase) { + return !ease ? defaultEase : (_isFunction(ease) ? ease : _easeMap[ease] || _configEaseFromString(ease)) || defaultEase; +}, + _insertEase = function _insertEase(names, easeIn, easeOut, easeInOut) { + if (easeOut === void 0) { + easeOut = function easeOut(p) { + return 1 - easeIn(1 - p); + }; + } + + if (easeInOut === void 0) { + easeInOut = function easeInOut(p) { + return p < .5 ? easeIn(p * 2) / 2 : 1 - easeIn((1 - p) * 2) / 2; + }; + } + + var ease = { + easeIn: easeIn, + easeOut: easeOut, + easeInOut: easeInOut + }, + lowercaseName; + + _forEachName(names, function (name) { + _easeMap[name] = _globals[name] = ease; + _easeMap[lowercaseName = name.toLowerCase()] = easeOut; + + for (var p in ease) { + _easeMap[lowercaseName + (p === "easeIn" ? ".in" : p === "easeOut" ? ".out" : ".inOut")] = _easeMap[name + "." + p] = ease[p]; + } + }); + + return ease; +}, + _easeInOutFromOut = function _easeInOutFromOut(easeOut) { + return function (p) { + return p < .5 ? (1 - easeOut(1 - p * 2)) / 2 : .5 + easeOut((p - .5) * 2) / 2; + }; +}, + _configElastic = function _configElastic(type, amplitude, period) { + var p1 = amplitude >= 1 ? amplitude : 1, + //note: if amplitude is < 1, we simply adjust the period for a more natural feel. Otherwise the math doesn't work right and the curve starts at 1. + p2 = (period || (type ? .3 : .45)) / (amplitude < 1 ? amplitude : 1), + p3 = p2 / _2PI * (Math.asin(1 / p1) || 0), + easeOut = function easeOut(p) { + return p === 1 ? 1 : p1 * Math.pow(2, -10 * p) * _sin((p - p3) * p2) + 1; + }, + ease = type === "out" ? easeOut : type === "in" ? function (p) { + return 1 - easeOut(1 - p); + } : _easeInOutFromOut(easeOut); + + p2 = _2PI / p2; //precalculate to optimize + + ease.config = function (amplitude, period) { + return _configElastic(type, amplitude, period); + }; + + return ease; +}, + _configBack = function _configBack(type, overshoot) { + if (overshoot === void 0) { + overshoot = 1.70158; + } + + var easeOut = function easeOut(p) { + return p ? --p * p * ((overshoot + 1) * p + overshoot) + 1 : 0; + }, + ease = type === "out" ? easeOut : type === "in" ? function (p) { + return 1 - easeOut(1 - p); + } : _easeInOutFromOut(easeOut); + + ease.config = function (overshoot) { + return _configBack(type, overshoot); + }; + + return ease; +}; // a cheaper (kb and cpu) but more mild way to get a parameterized weighted ease by feeding in a value between -1 (easeIn) and 1 (easeOut) where 0 is linear. +// _weightedEase = ratio => { +// let y = 0.5 + ratio / 2; +// return p => (2 * (1 - p) * p * y + p * p); +// }, +// a stronger (but more expensive kb/cpu) parameterized weighted ease that lets you feed in a value between -1 (easeIn) and 1 (easeOut) where 0 is linear. +// _weightedEaseStrong = ratio => { +// ratio = .5 + ratio / 2; +// let o = 1 / 3 * (ratio < .5 ? ratio : 1 - ratio), +// b = ratio - o, +// c = ratio + o; +// return p => p === 1 ? p : 3 * b * (1 - p) * (1 - p) * p + 3 * c * (1 - p) * p * p + p * p * p; +// }; + + +_forEachName("Linear,Quad,Cubic,Quart,Quint,Strong", function (name, i) { + var power = i < 5 ? i + 1 : i; + + _insertEase(name + ",Power" + (power - 1), i ? function (p) { + return Math.pow(p, power); + } : function (p) { + return p; + }, function (p) { + return 1 - Math.pow(1 - p, power); + }, function (p) { + return p < .5 ? Math.pow(p * 2, power) / 2 : 1 - Math.pow((1 - p) * 2, power) / 2; + }); +}); + +_easeMap.Linear.easeNone = _easeMap.none = _easeMap.Linear.easeIn; + +_insertEase("Elastic", _configElastic("in"), _configElastic("out"), _configElastic()); + +(function (n, c) { + var n1 = 1 / c, + n2 = 2 * n1, + n3 = 2.5 * n1, + easeOut = function easeOut(p) { + return p < n1 ? n * p * p : p < n2 ? n * Math.pow(p - 1.5 / c, 2) + .75 : p < n3 ? n * (p -= 2.25 / c) * p + .9375 : n * Math.pow(p - 2.625 / c, 2) + .984375; + }; + + _insertEase("Bounce", function (p) { + return 1 - easeOut(1 - p); + }, easeOut); +})(7.5625, 2.75); + +_insertEase("Expo", function (p) { + return Math.pow(2, 10 * (p - 1)) * p + p * p * p * p * p * p * (1 - p); +}); // previously 2 ** (10 * (p - 1)) but that doesn't end up with the value quite at the right spot so we do a blended ease to ensure it lands where it should perfectly. + + +_insertEase("Circ", function (p) { + return -(_sqrt(1 - p * p) - 1); +}); + +_insertEase("Sine", function (p) { + return p === 1 ? 1 : -_cos(p * _HALF_PI) + 1; +}); + +_insertEase("Back", _configBack("in"), _configBack("out"), _configBack()); + +_easeMap.SteppedEase = _easeMap.steps = _globals.SteppedEase = { + config: function config(steps, immediateStart) { + if (steps === void 0) { + steps = 1; + } + + var p1 = 1 / steps, + p2 = steps + (immediateStart ? 0 : 1), + p3 = immediateStart ? 1 : 0, + max = 1 - _tinyNum; + return function (p) { + return ((p2 * _clamp(0, max, p) | 0) + p3) * p1; + }; + } +}; +_defaults.ease = _easeMap["quad.out"]; + +_forEachName("onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt", function (name) { + return _callbackNames += name + "," + name + "Params,"; +}); +/* + * -------------------------------------------------------------------------------------- + * CACHE + * -------------------------------------------------------------------------------------- + */ + + +export var GSCache = function GSCache(target, harness) { + this.id = _gsID++; + target._gsap = this; + this.target = target; + this.harness = harness; + this.get = harness ? harness.get : _getProperty; + this.set = harness ? harness.getSetter : _getSetter; +}; +/* + * -------------------------------------------------------------------------------------- + * ANIMATION + * -------------------------------------------------------------------------------------- + */ + +export var Animation = /*#__PURE__*/function () { + function Animation(vars) { + this.vars = vars; + this._delay = +vars.delay || 0; + + if (this._repeat = vars.repeat === Infinity ? -2 : vars.repeat || 0) { + // TODO: repeat: Infinity on a timeline's children must flag that timeline internally and affect its totalDuration, otherwise it'll stop in the negative direction when reaching the start. + this._rDelay = vars.repeatDelay || 0; + this._yoyo = !!vars.yoyo || !!vars.yoyoEase; + } + + this._ts = 1; + + _setDuration(this, +vars.duration, 1, 1); + + this.data = vars.data; + + if (_context) { + this._ctx = _context; + + _context.data.push(this); + } + + _tickerActive || _ticker.wake(); + } + + var _proto = Animation.prototype; + + _proto.delay = function delay(value) { + if (value || value === 0) { + this.parent && this.parent.smoothChildTiming && this.startTime(this._start + value - this._delay); + this._delay = value; + return this; + } + + return this._delay; + }; + + _proto.duration = function duration(value) { + return arguments.length ? this.totalDuration(this._repeat > 0 ? value + (value + this._rDelay) * this._repeat : value) : this.totalDuration() && this._dur; + }; + + _proto.totalDuration = function totalDuration(value) { + if (!arguments.length) { + return this._tDur; + } + + this._dirty = 0; + return _setDuration(this, this._repeat < 0 ? value : (value - this._repeat * this._rDelay) / (this._repeat + 1)); + }; + + _proto.totalTime = function totalTime(_totalTime, suppressEvents) { + _wake(); + + if (!arguments.length) { + return this._tTime; + } + + var parent = this._dp; + + if (parent && parent.smoothChildTiming && this._ts) { + _alignPlayhead(this, _totalTime); + + !parent._dp || parent.parent || _postAddChecks(parent, this); // edge case: if this is a child of a timeline that already completed, for example, we must re-activate the parent. + //in case any of the ancestor timelines had completed but should now be enabled, we should reset their totalTime() which will also ensure that they're lined up properly and enabled. Skip for animations that are on the root (wasteful). Example: a TimelineLite.exportRoot() is performed when there's a paused tween on the root, the export will not complete until that tween is unpaused, but imagine a child gets restarted later, after all [unpaused] tweens have completed. The start of that child would get pushed out, but one of the ancestors may have completed. + + while (parent && parent.parent) { + if (parent.parent._time !== parent._start + (parent._ts >= 0 ? parent._tTime / parent._ts : (parent.totalDuration() - parent._tTime) / -parent._ts)) { + parent.totalTime(parent._tTime, true); + } + + parent = parent.parent; + } + + if (!this.parent && this._dp.autoRemoveChildren && (this._ts > 0 && _totalTime < this._tDur || this._ts < 0 && _totalTime > 0 || !this._tDur && !_totalTime)) { + //if the animation doesn't have a parent, put it back into its last parent (recorded as _dp for exactly cases like this). Limit to parents with autoRemoveChildren (like globalTimeline) so that if the user manually removes an animation from a timeline and then alters its playhead, it doesn't get added back in. + _addToTimeline(this._dp, this, this._start - this._delay); + } + } + + if (this._tTime !== _totalTime || !this._dur && !suppressEvents || this._initted && Math.abs(this._zTime) === _tinyNum || !_totalTime && !this._initted && (this.add || this._ptLookup)) { + // check for _ptLookup on a Tween instance to ensure it has actually finished being instantiated, otherwise if this.reverse() gets called in the Animation constructor, it could trigger a render() here even though the _targets weren't populated, thus when _init() is called there won't be any PropTweens (it'll act like the tween is non-functional) + this._ts || (this._pTime = _totalTime); // otherwise, if an animation is paused, then the playhead is moved back to zero, then resumed, it'd revert back to the original time at the pause + //if (!this._lock) { // avoid endless recursion (not sure we need this yet or if it's worth the performance hit) + // this._lock = 1; + + _lazySafeRender(this, _totalTime, suppressEvents); // this._lock = 0; + //} + + } + + return this; + }; + + _proto.time = function time(value, suppressEvents) { + return arguments.length ? this.totalTime(Math.min(this.totalDuration(), value + _elapsedCycleDuration(this)) % (this._dur + this._rDelay) || (value ? this._dur : 0), suppressEvents) : this._time; // note: if the modulus results in 0, the playhead could be exactly at the end or the beginning, and we always defer to the END with a non-zero value, otherwise if you set the time() to the very end (duration()), it would render at the START! + }; + + _proto.totalProgress = function totalProgress(value, suppressEvents) { + return arguments.length ? this.totalTime(this.totalDuration() * value, suppressEvents) : this.totalDuration() ? Math.min(1, this._tTime / this._tDur) : this.rawTime() >= 0 && this._initted ? 1 : 0; + }; + + _proto.progress = function progress(value, suppressEvents) { + return arguments.length ? this.totalTime(this.duration() * (this._yoyo && !(this.iteration() & 1) ? 1 - value : value) + _elapsedCycleDuration(this), suppressEvents) : this.duration() ? Math.min(1, this._time / this._dur) : this.rawTime() > 0 ? 1 : 0; + }; + + _proto.iteration = function iteration(value, suppressEvents) { + var cycleDuration = this.duration() + this._rDelay; + + return arguments.length ? this.totalTime(this._time + (value - 1) * cycleDuration, suppressEvents) : this._repeat ? _animationCycle(this._tTime, cycleDuration) + 1 : 1; + } // potential future addition: + // isPlayingBackwards() { + // let animation = this, + // orientation = 1; // 1 = forward, -1 = backward + // while (animation) { + // orientation *= animation.reversed() || (animation.repeat() && !(animation.iteration() & 1)) ? -1 : 1; + // animation = animation.parent; + // } + // return orientation < 0; + // } + ; + + _proto.timeScale = function timeScale(value, suppressEvents) { + if (!arguments.length) { + return this._rts === -_tinyNum ? 0 : this._rts; // recorded timeScale. Special case: if someone calls reverse() on an animation with timeScale of 0, we assign it -_tinyNum to remember it's reversed. + } + + if (this._rts === value) { + return this; + } + + var tTime = this.parent && this._ts ? _parentToChildTotalTime(this.parent._time, this) : this._tTime; // make sure to do the parentToChildTotalTime() BEFORE setting the new _ts because the old one must be used in that calculation. + // future addition? Up side: fast and minimal file size. Down side: only works on this animation; if a timeline is reversed, for example, its childrens' onReverse wouldn't get called. + //(+value < 0 && this._rts >= 0) && _callback(this, "onReverse", true); + // prioritize rendering where the parent's playhead lines up instead of this._tTime because there could be a tween that's animating another tween's timeScale in the same rendering loop (same parent), thus if the timeScale tween renders first, it would alter _start BEFORE _tTime was set on that tick (in the rendering loop), effectively freezing it until the timeScale tween finishes. + + this._rts = +value || 0; + this._ts = this._ps || value === -_tinyNum ? 0 : this._rts; // _ts is the functional timeScale which would be 0 if the animation is paused. + + this.totalTime(_clamp(-Math.abs(this._delay), this._tDur, tTime), suppressEvents !== false); + + _setEnd(this); // if parent.smoothChildTiming was false, the end time didn't get updated in the _alignPlayhead() method, so do it here. + + + return _recacheAncestors(this); + }; + + _proto.paused = function paused(value) { + if (!arguments.length) { + return this._ps; + } // possible future addition - if an animation is removed from its parent and then .restart() or .play() or .resume() is called, perhaps we should force it back into the globalTimeline but be careful because what if it's already at its end? We don't want it to just persist forever and not get released for GC. + // !this.parent && !value && this._tTime < this._tDur && this !== _globalTimeline && _globalTimeline.add(this); + + + if (this._ps !== value) { + this._ps = value; + + if (value) { + this._pTime = this._tTime || Math.max(-this._delay, this.rawTime()); // if the pause occurs during the delay phase, make sure that's factored in when resuming. + + this._ts = this._act = 0; // _ts is the functional timeScale, so a paused tween would effectively have a timeScale of 0. We record the "real" timeScale as _rts (recorded time scale) + } else { + _wake(); + + this._ts = this._rts; //only defer to _pTime (pauseTime) if tTime is zero. Remember, someone could pause() an animation, then scrub the playhead and resume(). If the parent doesn't have smoothChildTiming, we render at the rawTime() because the startTime won't get updated. + + this.totalTime(this.parent && !this.parent.smoothChildTiming ? this.rawTime() : this._tTime || this._pTime, this.progress() === 1 && Math.abs(this._zTime) !== _tinyNum && (this._tTime -= _tinyNum)); // edge case: animation.progress(1).pause().play() wouldn't render again because the playhead is already at the end, but the call to totalTime() below will add it back to its parent...and not remove it again (since removing only happens upon rendering at a new time). Offsetting the _tTime slightly is done simply to cause the final render in totalTime() that'll pop it off its timeline (if autoRemoveChildren is true, of course). Check to make sure _zTime isn't -_tinyNum to avoid an edge case where the playhead is pushed to the end but INSIDE a tween/callback, the timeline itself is paused thus halting rendering and leaving a few unrendered. When resuming, it wouldn't render those otherwise. + } + } + + return this; + }; + + _proto.startTime = function startTime(value) { + if (arguments.length) { + this._start = value; + var parent = this.parent || this._dp; + parent && (parent._sort || !this.parent) && _addToTimeline(parent, this, value - this._delay); + return this; + } + + return this._start; + }; + + _proto.endTime = function endTime(includeRepeats) { + return this._start + (_isNotFalse(includeRepeats) ? this.totalDuration() : this.duration()) / Math.abs(this._ts || 1); + }; + + _proto.rawTime = function rawTime(wrapRepeats) { + var parent = this.parent || this._dp; // _dp = detached parent + + return !parent ? this._tTime : wrapRepeats && (!this._ts || this._repeat && this._time && this.totalProgress() < 1) ? this._tTime % (this._dur + this._rDelay) : !this._ts ? this._tTime : _parentToChildTotalTime(parent.rawTime(wrapRepeats), this); + }; + + _proto.revert = function revert(config) { + if (config === void 0) { + config = _revertConfig; + } + + var prevIsReverting = _reverting; + _reverting = config; + + if (this._initted || this._startAt) { + this.timeline && this.timeline.revert(config); + this.totalTime(-0.01, config.suppressEvents); + } + + this.data !== "nested" && config.kill !== false && this.kill(); + _reverting = prevIsReverting; + return this; + }; + + _proto.globalTime = function globalTime(rawTime) { + var animation = this, + time = arguments.length ? rawTime : animation.rawTime(); + + while (animation) { + time = animation._start + time / (Math.abs(animation._ts) || 1); + animation = animation._dp; + } + + return !this.parent && this._sat ? this._sat.globalTime(rawTime) : time; // the _startAt tweens for .fromTo() and .from() that have immediateRender should always be FIRST in the timeline (important for context.revert()). "_sat" stands for _startAtTween, referring to the parent tween that created the _startAt. We must discern if that tween had immediateRender so that we can know whether or not to prioritize it in revert(). + }; + + _proto.repeat = function repeat(value) { + if (arguments.length) { + this._repeat = value === Infinity ? -2 : value; + return _onUpdateTotalDuration(this); + } + + return this._repeat === -2 ? Infinity : this._repeat; + }; + + _proto.repeatDelay = function repeatDelay(value) { + if (arguments.length) { + var time = this._time; + this._rDelay = value; + + _onUpdateTotalDuration(this); + + return time ? this.time(time) : this; + } + + return this._rDelay; + }; + + _proto.yoyo = function yoyo(value) { + if (arguments.length) { + this._yoyo = value; + return this; + } + + return this._yoyo; + }; + + _proto.seek = function seek(position, suppressEvents) { + return this.totalTime(_parsePosition(this, position), _isNotFalse(suppressEvents)); + }; + + _proto.restart = function restart(includeDelay, suppressEvents) { + this.play().totalTime(includeDelay ? -this._delay : 0, _isNotFalse(suppressEvents)); + this._dur || (this._zTime = -_tinyNum); // ensures onComplete fires on a zero-duration animation that gets restarted. + + return this; + }; + + _proto.play = function play(from, suppressEvents) { + from != null && this.seek(from, suppressEvents); + return this.reversed(false).paused(false); + }; + + _proto.reverse = function reverse(from, suppressEvents) { + from != null && this.seek(from || this.totalDuration(), suppressEvents); + return this.reversed(true).paused(false); + }; + + _proto.pause = function pause(atTime, suppressEvents) { + atTime != null && this.seek(atTime, suppressEvents); + return this.paused(true); + }; + + _proto.resume = function resume() { + return this.paused(false); + }; + + _proto.reversed = function reversed(value) { + if (arguments.length) { + !!value !== this.reversed() && this.timeScale(-this._rts || (value ? -_tinyNum : 0)); // in case timeScale is zero, reversing would have no effect so we use _tinyNum. + + return this; + } + + return this._rts < 0; + }; + + _proto.invalidate = function invalidate() { + this._initted = this._act = 0; + this._zTime = -_tinyNum; + return this; + }; + + _proto.isActive = function isActive() { + var parent = this.parent || this._dp, + start = this._start, + rawTime; + return !!(!parent || this._ts && this._initted && parent.isActive() && (rawTime = parent.rawTime(true)) >= start && rawTime < this.endTime(true) - _tinyNum); + }; + + _proto.eventCallback = function eventCallback(type, callback, params) { + var vars = this.vars; + + if (arguments.length > 1) { + if (!callback) { + delete vars[type]; + } else { + vars[type] = callback; + params && (vars[type + "Params"] = params); + type === "onUpdate" && (this._onUpdate = callback); + } + + return this; + } + + return vars[type]; + }; + + _proto.then = function then(onFulfilled) { + var self = this; + return new Promise(function (resolve) { + var f = _isFunction(onFulfilled) ? onFulfilled : _passThrough, + _resolve = function _resolve() { + var _then = self.then; + self.then = null; // temporarily null the then() method to avoid an infinite loop (see https://github.com/greensock/GSAP/issues/322) + + _isFunction(f) && (f = f(self)) && (f.then || f === self) && (self.then = _then); + resolve(f); + self.then = _then; + }; + + if (self._initted && self.totalProgress() === 1 && self._ts >= 0 || !self._tTime && self._ts < 0) { + _resolve(); + } else { + self._prom = _resolve; + } + }); + }; + + _proto.kill = function kill() { + _interrupt(this); + }; + + return Animation; +}(); + +_setDefaults(Animation.prototype, { + _time: 0, + _start: 0, + _end: 0, + _tTime: 0, + _tDur: 0, + _dirty: 0, + _repeat: 0, + _yoyo: false, + parent: null, + _initted: false, + _rDelay: 0, + _ts: 1, + _dp: 0, + ratio: 0, + _zTime: -_tinyNum, + _prom: 0, + _ps: false, + _rts: 1 +}); +/* + * ------------------------------------------------- + * TIMELINE + * ------------------------------------------------- + */ + + +export var Timeline = /*#__PURE__*/function (_Animation) { + _inheritsLoose(Timeline, _Animation); + + function Timeline(vars, position) { + var _this; + + if (vars === void 0) { + vars = {}; + } + + _this = _Animation.call(this, vars) || this; + _this.labels = {}; + _this.smoothChildTiming = !!vars.smoothChildTiming; + _this.autoRemoveChildren = !!vars.autoRemoveChildren; + _this._sort = _isNotFalse(vars.sortChildren); + _globalTimeline && _addToTimeline(vars.parent || _globalTimeline, _assertThisInitialized(_this), position); + vars.reversed && _this.reverse(); + vars.paused && _this.paused(true); + vars.scrollTrigger && _scrollTrigger(_assertThisInitialized(_this), vars.scrollTrigger); + return _this; + } + + var _proto2 = Timeline.prototype; + + _proto2.to = function to(targets, vars, position) { + _createTweenType(0, arguments, this); + + return this; + }; + + _proto2.from = function from(targets, vars, position) { + _createTweenType(1, arguments, this); + + return this; + }; + + _proto2.fromTo = function fromTo(targets, fromVars, toVars, position) { + _createTweenType(2, arguments, this); + + return this; + }; + + _proto2.set = function set(targets, vars, position) { + vars.duration = 0; + vars.parent = this; + _inheritDefaults(vars).repeatDelay || (vars.repeat = 0); + vars.immediateRender = !!vars.immediateRender; + new Tween(targets, vars, _parsePosition(this, position), 1); + return this; + }; + + _proto2.call = function call(callback, params, position) { + return _addToTimeline(this, Tween.delayedCall(0, callback, params), position); + } //ONLY for backward compatibility! Maybe delete? + ; + + _proto2.staggerTo = function staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.duration = duration; + vars.stagger = vars.stagger || stagger; + vars.onComplete = onCompleteAll; + vars.onCompleteParams = onCompleteAllParams; + vars.parent = this; + new Tween(targets, vars, _parsePosition(this, position)); + return this; + }; + + _proto2.staggerFrom = function staggerFrom(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.runBackwards = 1; + _inheritDefaults(vars).immediateRender = _isNotFalse(vars.immediateRender); + return this.staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams); + }; + + _proto2.staggerFromTo = function staggerFromTo(targets, duration, fromVars, toVars, stagger, position, onCompleteAll, onCompleteAllParams) { + toVars.startAt = fromVars; + _inheritDefaults(toVars).immediateRender = _isNotFalse(toVars.immediateRender); + return this.staggerTo(targets, duration, toVars, stagger, position, onCompleteAll, onCompleteAllParams); + }; + + _proto2.render = function render(totalTime, suppressEvents, force) { + var prevTime = this._time, + tDur = this._dirty ? this.totalDuration() : this._tDur, + dur = this._dur, + tTime = totalTime <= 0 ? 0 : _roundPrecise(totalTime), + // if a paused timeline is resumed (or its _start is updated for another reason...which rounds it), that could result in the playhead shifting a **tiny** amount and a zero-duration child at that spot may get rendered at a different ratio, like its totalTime in render() may be 1e-17 instead of 0, for example. + crossingStart = this._zTime < 0 !== totalTime < 0 && (this._initted || !dur), + time, + child, + next, + iteration, + cycleDuration, + prevPaused, + pauseTween, + timeScale, + prevStart, + prevIteration, + yoyo, + isYoyo; + this !== _globalTimeline && tTime > tDur && totalTime >= 0 && (tTime = tDur); + + if (tTime !== this._tTime || force || crossingStart) { + if (prevTime !== this._time && dur) { + //if totalDuration() finds a child with a negative startTime and smoothChildTiming is true, things get shifted around internally so we need to adjust the time accordingly. For example, if a tween starts at -30 we must shift EVERYTHING forward 30 seconds and move this timeline's startTime backward by 30 seconds so that things align with the playhead (no jump). + tTime += this._time - prevTime; + totalTime += this._time - prevTime; + } + + time = tTime; + prevStart = this._start; + timeScale = this._ts; + prevPaused = !timeScale; + + if (crossingStart) { + dur || (prevTime = this._zTime); //when the playhead arrives at EXACTLY time 0 (right on top) of a zero-duration timeline, we need to discern if events are suppressed so that when the playhead moves again (next time), it'll trigger the callback. If events are NOT suppressed, obviously the callback would be triggered in this render. Basically, the callback should fire either when the playhead ARRIVES or LEAVES this exact spot, not both. Imagine doing a timeline.seek(0) and there's a callback that sits at 0. Since events are suppressed on that seek() by default, nothing will fire, but when the playhead moves off of that position, the callback should fire. This behavior is what people intuitively expect. + + (totalTime || !suppressEvents) && (this._zTime = totalTime); + } + + if (this._repeat) { + //adjust the time for repeats and yoyos + yoyo = this._yoyo; + cycleDuration = dur + this._rDelay; + + if (this._repeat < -1 && totalTime < 0) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + + time = _roundPrecise(tTime % cycleDuration); //round to avoid floating point errors. (4 % 0.8 should be 0 but some browsers report it as 0.79999999!) + + if (tTime === tDur) { + // the tDur === tTime is for edge cases where there's a lengthy decimal on the duration and it may reach the very end but the time is rendered as not-quite-there (remember, tDur is rounded to 4 decimals whereas dur isn't) + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); // full decimal version of iterations, not the previous iteration (we're reusing prevIteration variable for efficiency) + + iteration = ~~prevIteration; + + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } + + time > dur && (time = dur); + } + + prevIteration = _animationCycle(this._tTime, cycleDuration); + !prevTime && this._tTime && prevIteration !== iteration && this._tTime - prevIteration * cycleDuration - this._dur <= 0 && (prevIteration = iteration); // edge case - if someone does addPause() at the very beginning of a repeating timeline, that pause is technically at the same spot as the end which causes this._time to get set to 0 when the totalTime would normally place the playhead at the end. See https://gsap.com/forums/topic/23823-closing-nav-animation-not-working-on-ie-and-iphone-6-maybe-other-older-browser/?tab=comments#comment-113005 also, this._tTime - prevIteration * cycleDuration - this._dur <= 0 just checks to make sure it wasn't previously in the "repeatDelay" portion + + if (yoyo && iteration & 1) { + time = dur - time; + isYoyo = 1; + } + /* + make sure children at the end/beginning of the timeline are rendered properly. If, for example, + a 3-second long timeline rendered at 2.9 seconds previously, and now renders at 3.2 seconds (which + would get translated to 2.8 seconds if the timeline yoyos or 0.2 seconds if it just repeats), there + could be a callback or a short tween that's at 2.95 or 3 seconds in which wouldn't render. So + we need to push the timeline to the end (and/or beginning depending on its yoyo value). Also we must + ensure that zero-duration tweens at the very beginning or end of the Timeline work. + */ + + + if (iteration !== prevIteration && !this._lock) { + var rewinding = yoyo && prevIteration & 1, + doesWrap = rewinding === (yoyo && iteration & 1); + iteration < prevIteration && (rewinding = !rewinding); + prevTime = rewinding ? 0 : tTime % dur ? dur : tTime; // if the playhead is landing exactly at the end of an iteration, use that totalTime rather than only the duration, otherwise it'll skip the 2nd render since it's effectively at the same time. + + this._lock = 1; + this.render(prevTime || (isYoyo ? 0 : _roundPrecise(iteration * cycleDuration)), suppressEvents, !dur)._lock = 0; + this._tTime = tTime; // if a user gets the iteration() inside the onRepeat, for example, it should be accurate. + + !suppressEvents && this.parent && _callback(this, "onRepeat"); + this.vars.repeatRefresh && !isYoyo && (this.invalidate()._lock = 1); + + if (prevTime && prevTime !== this._time || prevPaused !== !this._ts || this.vars.onRepeat && !this.parent && !this._act) { + // if prevTime is 0 and we render at the very end, _time will be the end, thus won't match. So in this edge case, prevTime won't match _time but that's okay. If it gets killed in the onRepeat, eject as well. + return this; + } + + dur = this._dur; // in case the duration changed in the onRepeat + + tDur = this._tDur; + + if (doesWrap) { + this._lock = 2; + prevTime = rewinding ? dur : -0.0001; + this.render(prevTime, true); + this.vars.repeatRefresh && !isYoyo && this.invalidate(); + } + + this._lock = 0; + + if (!this._ts && !prevPaused) { + return this; + } //in order for yoyoEase to work properly when there's a stagger, we must swap out the ease in each sub-tween. + + + _propagateYoyoEase(this, isYoyo); + } + } + + if (this._hasPause && !this._forcing && this._lock < 2) { + pauseTween = _findNextPauseTween(this, _roundPrecise(prevTime), _roundPrecise(time)); + + if (pauseTween) { + tTime -= time - (time = pauseTween._start); + } + } + + this._tTime = tTime; + this._time = time; + this._act = !timeScale; //as long as it's not paused, force it to be active so that if the user renders independent of the parent timeline, it'll be forced to re-render on the next tick. + + if (!this._initted) { + this._onUpdate = this.vars.onUpdate; + this._initted = 1; + this._zTime = totalTime; + prevTime = 0; // upon init, the playhead should always go forward; someone could invalidate() a completed timeline and then if they restart(), that would make child tweens render in reverse order which could lock in the wrong starting values if they build on each other, like tl.to(obj, {x: 100}).to(obj, {x: 0}). + } + + if (!prevTime && time && !suppressEvents && !iteration) { + _callback(this, "onStart"); + + if (this._tTime !== tTime) { + // in case the onStart triggered a render at a different spot, eject. Like if someone did animation.pause(0.5) or something inside the onStart. + return this; + } + } + + if (time >= prevTime && totalTime >= 0) { + child = this._first; + + while (child) { + next = child._next; + + if ((child._act || time >= child._start) && child._ts && pauseTween !== child) { + if (child.parent !== this) { + // an extreme edge case - the child's render could do something like kill() the "next" one in the linked list, or reparent it. In that case we must re-initiate the whole render to be safe. + return this.render(totalTime, suppressEvents, force); + } + + child.render(child._ts > 0 ? (time - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (time - child._start) * child._ts, suppressEvents, force); + + if (time !== this._time || !this._ts && !prevPaused) { + //in case a tween pauses or seeks the timeline when rendering, like inside of an onUpdate/onComplete + pauseTween = 0; + next && (tTime += this._zTime = -_tinyNum); // it didn't finish rendering, so flag zTime as negative so that the next time render() is called it'll be forced (to render any remaining children) + + break; + } + } + + child = next; + } + } else { + child = this._last; + var adjustedTime = totalTime < 0 ? totalTime : time; //when the playhead goes backward beyond the start of this timeline, we must pass that information down to the child animations so that zero-duration tweens know whether to render their starting or ending values. + + while (child) { + next = child._prev; + + if ((child._act || adjustedTime <= child._end) && child._ts && pauseTween !== child) { + if (child.parent !== this) { + // an extreme edge case - the child's render could do something like kill() the "next" one in the linked list, or reparent it. In that case we must re-initiate the whole render to be safe. + return this.render(totalTime, suppressEvents, force); + } + + child.render(child._ts > 0 ? (adjustedTime - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (adjustedTime - child._start) * child._ts, suppressEvents, force || _reverting && (child._initted || child._startAt)); // if reverting, we should always force renders of initted tweens (but remember that .fromTo() or .from() may have a _startAt but not _initted yet). If, for example, a .fromTo() tween with a stagger (which creates an internal timeline) gets reverted BEFORE some of its child tweens render for the first time, it may not properly trigger them to revert. + + if (time !== this._time || !this._ts && !prevPaused) { + //in case a tween pauses or seeks the timeline when rendering, like inside of an onUpdate/onComplete + pauseTween = 0; + next && (tTime += this._zTime = adjustedTime ? -_tinyNum : _tinyNum); // it didn't finish rendering, so adjust zTime so that so that the next time render() is called it'll be forced (to render any remaining children) + + break; + } + } + + child = next; + } + } + + if (pauseTween && !suppressEvents) { + this.pause(); + pauseTween.render(time >= prevTime ? 0 : -_tinyNum)._zTime = time >= prevTime ? 1 : -1; + + if (this._ts) { + //the callback resumed playback! So since we may have held back the playhead due to where the pause is positioned, go ahead and jump to where it's SUPPOSED to be (if no pause happened). + this._start = prevStart; //if the pause was at an earlier time and the user resumed in the callback, it could reposition the timeline (changing its startTime), throwing things off slightly, so we make sure the _start doesn't shift. + + _setEnd(this); + + return this.render(totalTime, suppressEvents, force); + } + } + + this._onUpdate && !suppressEvents && _callback(this, "onUpdate", true); + if (tTime === tDur && this._tTime >= this.totalDuration() || !tTime && prevTime) if (prevStart === this._start || Math.abs(timeScale) !== Math.abs(this._ts)) if (!this._lock) { + // remember, a child's callback may alter this timeline's playhead or timeScale which is why we need to add some of these checks. + (totalTime || !dur) && (tTime === tDur && this._ts > 0 || !tTime && this._ts < 0) && _removeFromParent(this, 1); // don't remove if the timeline is reversed and the playhead isn't at 0, otherwise tl.progress(1).reverse() won't work. Only remove if the playhead is at the end and timeScale is positive, or if the playhead is at 0 and the timeScale is negative. + + if (!suppressEvents && !(totalTime < 0 && !prevTime) && (tTime || prevTime || !tDur)) { + _callback(this, tTime === tDur && totalTime >= 0 ? "onComplete" : "onReverseComplete", true); + + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + + return this; + }; + + _proto2.add = function add(child, position) { + var _this2 = this; + + _isNumber(position) || (position = _parsePosition(this, position, child)); + + if (!(child instanceof Animation)) { + if (_isArray(child)) { + child.forEach(function (obj) { + return _this2.add(obj, position); + }); + return this; + } + + if (_isString(child)) { + return this.addLabel(child, position); + } + + if (_isFunction(child)) { + child = Tween.delayedCall(0, child); + } else { + return this; + } + } + + return this !== child ? _addToTimeline(this, child, position) : this; //don't allow a timeline to be added to itself as a child! + }; + + _proto2.getChildren = function getChildren(nested, tweens, timelines, ignoreBeforeTime) { + if (nested === void 0) { + nested = true; + } + + if (tweens === void 0) { + tweens = true; + } + + if (timelines === void 0) { + timelines = true; + } + + if (ignoreBeforeTime === void 0) { + ignoreBeforeTime = -_bigNum; + } + + var a = [], + child = this._first; + + while (child) { + if (child._start >= ignoreBeforeTime) { + if (child instanceof Tween) { + tweens && a.push(child); + } else { + timelines && a.push(child); + nested && a.push.apply(a, child.getChildren(true, tweens, timelines)); + } + } + + child = child._next; + } + + return a; + }; + + _proto2.getById = function getById(id) { + var animations = this.getChildren(1, 1, 1), + i = animations.length; + + while (i--) { + if (animations[i].vars.id === id) { + return animations[i]; + } + } + }; + + _proto2.remove = function remove(child) { + if (_isString(child)) { + return this.removeLabel(child); + } + + if (_isFunction(child)) { + return this.killTweensOf(child); + } + + child.parent === this && _removeLinkedListItem(this, child); + + if (child === this._recent) { + this._recent = this._last; + } + + return _uncache(this); + }; + + _proto2.totalTime = function totalTime(_totalTime2, suppressEvents) { + if (!arguments.length) { + return this._tTime; + } + + this._forcing = 1; + + if (!this._dp && this._ts) { + //special case for the global timeline (or any other that has no parent or detached parent). + this._start = _roundPrecise(_ticker.time - (this._ts > 0 ? _totalTime2 / this._ts : (this.totalDuration() - _totalTime2) / -this._ts)); + } + + _Animation.prototype.totalTime.call(this, _totalTime2, suppressEvents); + + this._forcing = 0; + return this; + }; + + _proto2.addLabel = function addLabel(label, position) { + this.labels[label] = _parsePosition(this, position); + return this; + }; + + _proto2.removeLabel = function removeLabel(label) { + delete this.labels[label]; + return this; + }; + + _proto2.addPause = function addPause(position, callback, params) { + var t = Tween.delayedCall(0, callback || _emptyFunc, params); + t.data = "isPause"; + this._hasPause = 1; + return _addToTimeline(this, t, _parsePosition(this, position)); + }; + + _proto2.removePause = function removePause(position) { + var child = this._first; + position = _parsePosition(this, position); + + while (child) { + if (child._start === position && child.data === "isPause") { + _removeFromParent(child); + } + + child = child._next; + } + }; + + _proto2.killTweensOf = function killTweensOf(targets, props, onlyActive) { + var tweens = this.getTweensOf(targets, onlyActive), + i = tweens.length; + + while (i--) { + _overwritingTween !== tweens[i] && tweens[i].kill(targets, props); + } + + return this; + }; + + _proto2.getTweensOf = function getTweensOf(targets, onlyActive) { + var a = [], + parsedTargets = toArray(targets), + child = this._first, + isGlobalTime = _isNumber(onlyActive), + // a number is interpreted as a global time. If the animation spans + children; + + while (child) { + if (child instanceof Tween) { + if (_arrayContainsAny(child._targets, parsedTargets) && (isGlobalTime ? (!_overwritingTween || child._initted && child._ts) && child.globalTime(0) <= onlyActive && child.globalTime(child.totalDuration()) > onlyActive : !onlyActive || child.isActive())) { + // note: if this is for overwriting, it should only be for tweens that aren't paused and are initted. + a.push(child); + } + } else if ((children = child.getTweensOf(parsedTargets, onlyActive)).length) { + a.push.apply(a, children); + } + + child = child._next; + } + + return a; + } // potential future feature - targets() on timelines + // targets() { + // let result = []; + // this.getChildren(true, true, false).forEach(t => result.push(...t.targets())); + // return result.filter((v, i) => result.indexOf(v) === i); + // } + ; + + _proto2.tweenTo = function tweenTo(position, vars) { + vars = vars || {}; + + var tl = this, + endTime = _parsePosition(tl, position), + _vars = vars, + startAt = _vars.startAt, + _onStart = _vars.onStart, + onStartParams = _vars.onStartParams, + immediateRender = _vars.immediateRender, + initted, + tween = Tween.to(tl, _setDefaults({ + ease: vars.ease || "none", + lazy: false, + immediateRender: false, + time: endTime, + overwrite: "auto", + duration: vars.duration || Math.abs((endTime - (startAt && "time" in startAt ? startAt.time : tl._time)) / tl.timeScale()) || _tinyNum, + onStart: function onStart() { + tl.pause(); + + if (!initted) { + var duration = vars.duration || Math.abs((endTime - (startAt && "time" in startAt ? startAt.time : tl._time)) / tl.timeScale()); + tween._dur !== duration && _setDuration(tween, duration, 0, 1).render(tween._time, true, true); + initted = 1; + } + + _onStart && _onStart.apply(tween, onStartParams || []); //in case the user had an onStart in the vars - we don't want to overwrite it. + } + }, vars)); + + return immediateRender ? tween.render(0) : tween; + }; + + _proto2.tweenFromTo = function tweenFromTo(fromPosition, toPosition, vars) { + return this.tweenTo(toPosition, _setDefaults({ + startAt: { + time: _parsePosition(this, fromPosition) + } + }, vars)); + }; + + _proto2.recent = function recent() { + return this._recent; + }; + + _proto2.nextLabel = function nextLabel(afterTime) { + if (afterTime === void 0) { + afterTime = this._time; + } + + return _getLabelInDirection(this, _parsePosition(this, afterTime)); + }; + + _proto2.previousLabel = function previousLabel(beforeTime) { + if (beforeTime === void 0) { + beforeTime = this._time; + } + + return _getLabelInDirection(this, _parsePosition(this, beforeTime), 1); + }; + + _proto2.currentLabel = function currentLabel(value) { + return arguments.length ? this.seek(value, true) : this.previousLabel(this._time + _tinyNum); + }; + + _proto2.shiftChildren = function shiftChildren(amount, adjustLabels, ignoreBeforeTime) { + if (ignoreBeforeTime === void 0) { + ignoreBeforeTime = 0; + } + + var child = this._first, + labels = this.labels, + p; + + while (child) { + if (child._start >= ignoreBeforeTime) { + child._start += amount; + child._end += amount; + } + + child = child._next; + } + + if (adjustLabels) { + for (p in labels) { + if (labels[p] >= ignoreBeforeTime) { + labels[p] += amount; + } + } + } + + return _uncache(this); + }; + + _proto2.invalidate = function invalidate(soft) { + var child = this._first; + this._lock = 0; + + while (child) { + child.invalidate(soft); + child = child._next; + } + + return _Animation.prototype.invalidate.call(this, soft); + }; + + _proto2.clear = function clear(includeLabels) { + if (includeLabels === void 0) { + includeLabels = true; + } + + var child = this._first, + next; + + while (child) { + next = child._next; + this.remove(child); + child = next; + } + + this._dp && (this._time = this._tTime = this._pTime = 0); + includeLabels && (this.labels = {}); + return _uncache(this); + }; + + _proto2.totalDuration = function totalDuration(value) { + var max = 0, + self = this, + child = self._last, + prevStart = _bigNum, + prev, + start, + parent; + + if (arguments.length) { + return self.timeScale((self._repeat < 0 ? self.duration() : self.totalDuration()) / (self.reversed() ? -value : value)); + } + + if (self._dirty) { + parent = self.parent; + + while (child) { + prev = child._prev; //record it here in case the tween changes position in the sequence... + + child._dirty && child.totalDuration(); //could change the tween._startTime, so make sure the animation's cache is clean before analyzing it. + + start = child._start; + + if (start > prevStart && self._sort && child._ts && !self._lock) { + //in case one of the tweens shifted out of order, it needs to be re-inserted into the correct position in the sequence + self._lock = 1; //prevent endless recursive calls - there are methods that get triggered that check duration/totalDuration when we add(). + + _addToTimeline(self, child, start - child._delay, 1)._lock = 0; + } else { + prevStart = start; + } + + if (start < 0 && child._ts) { + //children aren't allowed to have negative startTimes unless smoothChildTiming is true, so adjust here if one is found. + max -= start; + + if (!parent && !self._dp || parent && parent.smoothChildTiming) { + self._start += start / self._ts; + self._time -= start; + self._tTime -= start; + } + + self.shiftChildren(-start, false, -1e999); + prevStart = 0; + } + + child._end > max && child._ts && (max = child._end); + child = prev; + } + + _setDuration(self, self === _globalTimeline && self._time > max ? self._time : max, 1, 1); + + self._dirty = 0; + } + + return self._tDur; + }; + + Timeline.updateRoot = function updateRoot(time) { + if (_globalTimeline._ts) { + _lazySafeRender(_globalTimeline, _parentToChildTotalTime(time, _globalTimeline)); + + _lastRenderedFrame = _ticker.frame; + } + + if (_ticker.frame >= _nextGCFrame) { + _nextGCFrame += _config.autoSleep || 120; + var child = _globalTimeline._first; + if (!child || !child._ts) if (_config.autoSleep && _ticker._listeners.length < 2) { + while (child && !child._ts) { + child = child._next; + } + + child || _ticker.sleep(); + } + } + }; + + return Timeline; +}(Animation); + +_setDefaults(Timeline.prototype, { + _lock: 0, + _hasPause: 0, + _forcing: 0 +}); + +var _addComplexStringPropTween = function _addComplexStringPropTween(target, prop, start, end, setter, stringFilter, funcParam) { + //note: we call _addComplexStringPropTween.call(tweenInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus "this" would refer to the plugin. + var pt = new PropTween(this._pt, target, prop, 0, 1, _renderComplexString, null, setter), + index = 0, + matchIndex = 0, + result, + startNums, + color, + endNum, + chunk, + startNum, + hasRandom, + a; + pt.b = start; + pt.e = end; + start += ""; //ensure values are strings + + end += ""; + + if (hasRandom = ~end.indexOf("random(")) { + end = _replaceRandom(end); + } + + if (stringFilter) { + a = [start, end]; + stringFilter(a, target, prop); //pass an array with the starting and ending values and let the filter do whatever it needs to the values. + + start = a[0]; + end = a[1]; + } + + startNums = start.match(_complexStringNumExp) || []; + + while (result = _complexStringNumExp.exec(end)) { + endNum = result[0]; + chunk = end.substring(index, result.index); + + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(") { + color = 1; + } + + if (endNum !== startNums[matchIndex++]) { + startNum = parseFloat(startNums[matchIndex - 1]) || 0; //these nested PropTweens are handled in a special way - we'll never actually call a render or setter method on them. We'll just loop through them in the parent complex string PropTween's render method. + + pt._pt = { + _next: pt._pt, + p: chunk || matchIndex === 1 ? chunk : ",", + //note: SVG spec allows omission of comma/space when a negative sign is wedged between two numbers, like 2.5-5.3 instead of 2.5,-5.3 but when tweening, the negative value may switch to positive, so we insert the comma just in case. + s: startNum, + c: endNum.charAt(1) === "=" ? _parseRelative(startNum, endNum) - startNum : parseFloat(endNum) - startNum, + m: color && color < 4 ? Math.round : 0 + }; + index = _complexStringNumExp.lastIndex; + } + } + + pt.c = index < end.length ? end.substring(index, end.length) : ""; //we use the "c" of the PropTween to store the final part of the string (after the last number) + + pt.fp = funcParam; + + if (_relExp.test(end) || hasRandom) { + pt.e = 0; //if the end string contains relative values or dynamic random(...) values, delete the end it so that on the final render we don't actually set it to the string with += or -= characters (forces it to use the calculated value). + } + + this._pt = pt; //start the linked list with this new PropTween. Remember, we call _addComplexStringPropTween.call(tweenInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus "this" would refer to the plugin. + + return pt; +}, + _addPropTween = function _addPropTween(target, prop, start, end, index, targets, modifier, stringFilter, funcParam, optional) { + _isFunction(end) && (end = end(index || 0, target, targets)); + var currentValue = target[prop], + parsedStart = start !== "get" ? start : !_isFunction(currentValue) ? currentValue : funcParam ? target[prop.indexOf("set") || !_isFunction(target["get" + prop.substr(3)]) ? prop : "get" + prop.substr(3)](funcParam) : target[prop](), + setter = !_isFunction(currentValue) ? _setterPlain : funcParam ? _setterFuncWithParam : _setterFunc, + pt; + + if (_isString(end)) { + if (~end.indexOf("random(")) { + end = _replaceRandom(end); + } + + if (end.charAt(1) === "=") { + pt = _parseRelative(parsedStart, end) + (getUnit(parsedStart) || 0); + + if (pt || pt === 0) { + // to avoid isNaN, like if someone passes in a value like "!= whatever" + end = pt; + } + } + } + + if (!optional || parsedStart !== end || _forceAllPropTweens) { + if (!isNaN(parsedStart * end) && end !== "") { + // fun fact: any number multiplied by "" is evaluated as the number 0! + pt = new PropTween(this._pt, target, prop, +parsedStart || 0, end - (parsedStart || 0), typeof currentValue === "boolean" ? _renderBoolean : _renderPlain, 0, setter); + funcParam && (pt.fp = funcParam); + modifier && pt.modifier(modifier, this, target); + return this._pt = pt; + } + + !currentValue && !(prop in target) && _missingPlugin(prop, end); + return _addComplexStringPropTween.call(this, target, prop, parsedStart, end, setter, stringFilter || _config.stringFilter, funcParam); + } +}, + //creates a copy of the vars object and processes any function-based values (putting the resulting values directly into the copy) as well as strings with "random()" in them. It does NOT process relative values. +_processVars = function _processVars(vars, index, target, targets, tween) { + _isFunction(vars) && (vars = _parseFuncOrString(vars, tween, index, target, targets)); + + if (!_isObject(vars) || vars.style && vars.nodeType || _isArray(vars) || _isTypedArray(vars)) { + return _isString(vars) ? _parseFuncOrString(vars, tween, index, target, targets) : vars; + } + + var copy = {}, + p; + + for (p in vars) { + copy[p] = _parseFuncOrString(vars[p], tween, index, target, targets); + } + + return copy; +}, + _checkPlugin = function _checkPlugin(property, vars, tween, index, target, targets) { + var plugin, pt, ptLookup, i; + + if (_plugins[property] && (plugin = new _plugins[property]()).init(target, plugin.rawVars ? vars[property] : _processVars(vars[property], index, target, targets, tween), tween, index, targets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, property, 0, 1, plugin.render, plugin, 0, plugin.priority); + + if (tween !== _quickTween) { + ptLookup = tween._ptLookup[tween._targets.indexOf(target)]; //note: we can't use tween._ptLookup[index] because for staggered tweens, the index from the fullTargets array won't match what it is in each individual tween that spawns from the stagger. + + i = plugin._props.length; + + while (i--) { + ptLookup[plugin._props[i]] = pt; + } + } + } + + return plugin; +}, + _overwritingTween, + //store a reference temporarily so we can avoid overwriting itself. +_forceAllPropTweens, + _initTween = function _initTween(tween, time, tTime) { + var vars = tween.vars, + ease = vars.ease, + startAt = vars.startAt, + immediateRender = vars.immediateRender, + lazy = vars.lazy, + onUpdate = vars.onUpdate, + runBackwards = vars.runBackwards, + yoyoEase = vars.yoyoEase, + keyframes = vars.keyframes, + autoRevert = vars.autoRevert, + dur = tween._dur, + prevStartAt = tween._startAt, + targets = tween._targets, + parent = tween.parent, + fullTargets = parent && parent.data === "nested" ? parent.vars.targets : targets, + autoOverwrite = tween._overwrite === "auto" && !_suppressOverwrites, + tl = tween.timeline, + cleanVars, + i, + p, + pt, + target, + hasPriority, + gsData, + harness, + plugin, + ptLookup, + index, + harnessVars, + overwritten; + tl && (!keyframes || !ease) && (ease = "none"); + tween._ease = _parseEase(ease, _defaults.ease); + tween._yEase = yoyoEase ? _invertEase(_parseEase(yoyoEase === true ? ease : yoyoEase, _defaults.ease)) : 0; + + if (yoyoEase && tween._yoyo && !tween._repeat) { + //there must have been a parent timeline with yoyo:true that is currently in its yoyo phase, so flip the eases. + yoyoEase = tween._yEase; + tween._yEase = tween._ease; + tween._ease = yoyoEase; + } + + tween._from = !tl && !!vars.runBackwards; //nested timelines should never run backwards - the backwards-ness is in the child tweens. + + if (!tl || keyframes && !vars.stagger) { + //if there's an internal timeline, skip all the parsing because we passed that task down the chain. + harness = targets[0] ? _getCache(targets[0]).harness : 0; + harnessVars = harness && vars[harness.prop]; //someone may need to specify CSS-specific values AND non-CSS values, like if the element has an "x" property plus it's a standard DOM element. We allow people to distinguish by wrapping plugin-specific stuff in a css:{} object for example. + + cleanVars = _copyExcluding(vars, _reservedProps); + + if (prevStartAt) { + prevStartAt._zTime < 0 && prevStartAt.progress(1); // in case it's a lazy startAt that hasn't rendered yet. + + time < 0 && runBackwards && immediateRender && !autoRevert ? prevStartAt.render(-1, true) : prevStartAt.revert(runBackwards && dur ? _revertConfigNoKill : _startAtRevertConfig); // if it's a "startAt" (not "from()" or runBackwards: true), we only need to do a shallow revert (keep transforms cached in CSSPlugin) + // don't just _removeFromParent(prevStartAt.render(-1, true)) because that'll leave inline styles. We're creating a new _startAt for "startAt" tweens that re-capture things to ensure that if the pre-tween values changed since the tween was created, they're recorded. + + prevStartAt._lazy = 0; + } + + if (startAt) { + _removeFromParent(tween._startAt = Tween.set(targets, _setDefaults({ + data: "isStart", + overwrite: false, + parent: parent, + immediateRender: true, + lazy: !prevStartAt && _isNotFalse(lazy), + startAt: null, + delay: 0, + onUpdate: onUpdate && function () { + return _callback(tween, "onUpdate"); + }, + stagger: 0 + }, startAt))); //copy the properties/values into a new object to avoid collisions, like var to = {x:0}, from = {x:500}; timeline.fromTo(e, from, to).fromTo(e, to, from); + + + tween._startAt._dp = 0; // don't allow it to get put back into root timeline! Like when revert() is called and totalTime() gets set. + + tween._startAt._sat = tween; // used in globalTime(). _sat stands for _startAtTween + + time < 0 && (_reverting || !immediateRender && !autoRevert) && tween._startAt.revert(_revertConfigNoKill); // rare edge case, like if a render is forced in the negative direction of a non-initted tween. + + if (immediateRender) { + if (dur && time <= 0 && tTime <= 0) { + // check tTime here because in the case of a yoyo tween whose playhead gets pushed to the end like tween.progress(1), we should allow it through so that the onComplete gets fired properly. + time && (tween._zTime = time); + return; //we skip initialization here so that overwriting doesn't occur until the tween actually begins. Otherwise, if you create several immediateRender:true tweens of the same target/properties to drop into a Timeline, the last one created would overwrite the first ones because they didn't get placed into the timeline yet before the first render occurs and kicks in overwriting. + } + } + } else if (runBackwards && dur) { + //from() tweens must be handled uniquely: their beginning values must be rendered but we don't want overwriting to occur yet (when time is still 0). Wait until the tween actually begins before doing all the routines like overwriting. At that time, we should render at the END of the tween to ensure that things initialize correctly (remember, from() tweens go backwards) + if (!prevStartAt) { + time && (immediateRender = false); //in rare cases (like if a from() tween runs and then is invalidate()-ed), immediateRender could be true but the initial forced-render gets skipped, so there's no need to force the render in this context when the _time is greater than 0 + + p = _setDefaults({ + overwrite: false, + data: "isFromStart", + //we tag the tween with as "isFromStart" so that if [inside a plugin] we need to only do something at the very END of a tween, we have a way of identifying this tween as merely the one that's setting the beginning values for a "from()" tween. For example, clearProps in CSSPlugin should only get applied at the very END of a tween and without this tag, from(...{height:100, clearProps:"height", delay:1}) would wipe the height at the beginning of the tween and after 1 second, it'd kick back in. + lazy: immediateRender && !prevStartAt && _isNotFalse(lazy), + immediateRender: immediateRender, + //zero-duration tweens render immediately by default, but if we're not specifically instructed to render this tween immediately, we should skip this and merely _init() to record the starting values (rendering them immediately would push them to completion which is wasteful in that case - we'd have to render(-1) immediately after) + stagger: 0, + parent: parent //ensures that nested tweens that had a stagger are handled properly, like gsap.from(".class", {y: gsap.utils.wrap([-100,100]), stagger: 0.5}) + + }, cleanVars); + harnessVars && (p[harness.prop] = harnessVars); // in case someone does something like .from(..., {css:{}}) + + _removeFromParent(tween._startAt = Tween.set(targets, p)); + + tween._startAt._dp = 0; // don't allow it to get put back into root timeline! + + tween._startAt._sat = tween; // used in globalTime() + + time < 0 && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween._startAt.render(-1, true)); + tween._zTime = time; + + if (!immediateRender) { + _initTween(tween._startAt, _tinyNum, _tinyNum); //ensures that the initial values are recorded + + } else if (!time) { + return; + } + } + } + + tween._pt = tween._ptCache = 0; + lazy = dur && _isNotFalse(lazy) || lazy && !dur; + + for (i = 0; i < targets.length; i++) { + target = targets[i]; + gsData = target._gsap || _harness(targets)[i]._gsap; + tween._ptLookup[i] = ptLookup = {}; + _lazyLookup[gsData.id] && _lazyTweens.length && _lazyRender(); //if other tweens of the same target have recently initted but haven't rendered yet, we've got to force the render so that the starting values are correct (imagine populating a timeline with a bunch of sequential tweens and then jumping to the end) + + index = fullTargets === targets ? i : fullTargets.indexOf(target); + + if (harness && (plugin = new harness()).init(target, harnessVars || cleanVars, tween, index, fullTargets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, plugin.name, 0, 1, plugin.render, plugin, 0, plugin.priority); + + plugin._props.forEach(function (name) { + ptLookup[name] = pt; + }); + + plugin.priority && (hasPriority = 1); + } + + if (!harness || harnessVars) { + for (p in cleanVars) { + if (_plugins[p] && (plugin = _checkPlugin(p, cleanVars, tween, index, target, fullTargets))) { + plugin.priority && (hasPriority = 1); + } else { + ptLookup[p] = pt = _addPropTween.call(tween, target, p, "get", cleanVars[p], index, fullTargets, 0, vars.stringFilter); + } + } + } + + tween._op && tween._op[i] && tween.kill(target, tween._op[i]); + + if (autoOverwrite && tween._pt) { + _overwritingTween = tween; + + _globalTimeline.killTweensOf(target, ptLookup, tween.globalTime(time)); // make sure the overwriting doesn't overwrite THIS tween!!! + + + overwritten = !tween.parent; + _overwritingTween = 0; + } + + tween._pt && lazy && (_lazyLookup[gsData.id] = 1); + } + + hasPriority && _sortPropTweensByPriority(tween); + tween._onInit && tween._onInit(tween); //plugins like RoundProps must wait until ALL of the PropTweens are instantiated. In the plugin's init() function, it sets the _onInit on the tween instance. May not be pretty/intuitive, but it's fast and keeps file size down. + } + + tween._onUpdate = onUpdate; + tween._initted = (!tween._op || tween._pt) && !overwritten; // if overwrittenProps resulted in the entire tween being killed, do NOT flag it as initted or else it may render for one tick. + + keyframes && time <= 0 && tl.render(_bigNum, true, true); // if there's a 0% keyframe, it'll render in the "before" state for any staggered/delayed animations thus when the following tween initializes, it'll use the "before" state instead of the "after" state as the initial values. +}, + _updatePropTweens = function _updatePropTweens(tween, property, value, start, startIsRelative, ratio, time, skipRecursion) { + var ptCache = (tween._pt && tween._ptCache || (tween._ptCache = {}))[property], + pt, + rootPT, + lookup, + i; + + if (!ptCache) { + ptCache = tween._ptCache[property] = []; + lookup = tween._ptLookup; + i = tween._targets.length; + + while (i--) { + pt = lookup[i][property]; + + if (pt && pt.d && pt.d._pt) { + // it's a plugin, so find the nested PropTween + pt = pt.d._pt; + + while (pt && pt.p !== property && pt.fp !== property) { + // "fp" is functionParam for things like setting CSS variables which require .setProperty("--var-name", value) + pt = pt._next; + } + } + + if (!pt) { + // there is no PropTween associated with that property, so we must FORCE one to be created and ditch out of this + // if the tween has other properties that already rendered at new positions, we'd normally have to rewind to put them back like tween.render(0, true) before forcing an _initTween(), but that can create another edge case like tweening a timeline's progress would trigger onUpdates to fire which could move other things around. It's better to just inform users that .resetTo() should ONLY be used for tweens that already have that property. For example, you can't gsap.to(...{ y: 0 }) and then tween.restTo("x", 200) for example. + _forceAllPropTweens = 1; // otherwise, when we _addPropTween() and it finds no change between the start and end values, it skips creating a PropTween (for efficiency...why tween when there's no difference?) but in this case we NEED that PropTween created so we can edit it. + + tween.vars[property] = "+=0"; + + _initTween(tween, time); + + _forceAllPropTweens = 0; + return skipRecursion ? _warn(property + " not eligible for reset") : 1; // if someone tries to do a quickTo() on a special property like borderRadius which must get split into 4 different properties, that's not eligible for .resetTo(). + } + + ptCache.push(pt); + } + } + + i = ptCache.length; + + while (i--) { + rootPT = ptCache[i]; + pt = rootPT._pt || rootPT; // complex values may have nested PropTweens. We only accommodate the FIRST value. + + pt.s = (start || start === 0) && !startIsRelative ? start : pt.s + (start || 0) + ratio * pt.c; + pt.c = value - pt.s; + rootPT.e && (rootPT.e = _round(value) + getUnit(rootPT.e)); // mainly for CSSPlugin (end value) + + rootPT.b && (rootPT.b = pt.s + getUnit(rootPT.b)); // (beginning value) + } +}, + _addAliasesToVars = function _addAliasesToVars(targets, vars) { + var harness = targets[0] ? _getCache(targets[0]).harness : 0, + propertyAliases = harness && harness.aliases, + copy, + p, + i, + aliases; + + if (!propertyAliases) { + return vars; + } + + copy = _merge({}, vars); + + for (p in propertyAliases) { + if (p in copy) { + aliases = propertyAliases[p].split(","); + i = aliases.length; + + while (i--) { + copy[aliases[i]] = copy[p]; + } + } + } + + return copy; +}, + // parses multiple formats, like {"0%": {x: 100}, {"50%": {x: -20}} and { x: {"0%": 100, "50%": -20} }, and an "ease" can be set on any object. We populate an "allProps" object with an Array for each property, like {x: [{}, {}], y:[{}, {}]} with data for each property tween. The objects have a "t" (time), "v", (value), and "e" (ease) property. This allows us to piece together a timeline later. +_parseKeyframe = function _parseKeyframe(prop, obj, allProps, easeEach) { + var ease = obj.ease || easeEach || "power1.inOut", + p, + a; + + if (_isArray(obj)) { + a = allProps[prop] || (allProps[prop] = []); // t = time (out of 100), v = value, e = ease + + obj.forEach(function (value, i) { + return a.push({ + t: i / (obj.length - 1) * 100, + v: value, + e: ease + }); + }); + } else { + for (p in obj) { + a = allProps[p] || (allProps[p] = []); + p === "ease" || a.push({ + t: parseFloat(prop), + v: obj[p], + e: ease + }); + } + } +}, + _parseFuncOrString = function _parseFuncOrString(value, tween, i, target, targets) { + return _isFunction(value) ? value.call(tween, i, target, targets) : _isString(value) && ~value.indexOf("random(") ? _replaceRandom(value) : value; +}, + _staggerTweenProps = _callbackNames + "repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase,autoRevert", + _staggerPropsToSkip = {}; + +_forEachName(_staggerTweenProps + ",id,stagger,delay,duration,paused,scrollTrigger", function (name) { + return _staggerPropsToSkip[name] = 1; +}); +/* + * -------------------------------------------------------------------------------------- + * TWEEN + * -------------------------------------------------------------------------------------- + */ + + +export var Tween = /*#__PURE__*/function (_Animation2) { + _inheritsLoose(Tween, _Animation2); + + function Tween(targets, vars, position, skipInherit) { + var _this3; + + if (typeof vars === "number") { + position.duration = vars; + vars = position; + position = null; + } + + _this3 = _Animation2.call(this, skipInherit ? vars : _inheritDefaults(vars)) || this; + var _this3$vars = _this3.vars, + duration = _this3$vars.duration, + delay = _this3$vars.delay, + immediateRender = _this3$vars.immediateRender, + stagger = _this3$vars.stagger, + overwrite = _this3$vars.overwrite, + keyframes = _this3$vars.keyframes, + defaults = _this3$vars.defaults, + scrollTrigger = _this3$vars.scrollTrigger, + yoyoEase = _this3$vars.yoyoEase, + parent = vars.parent || _globalTimeline, + parsedTargets = (_isArray(targets) || _isTypedArray(targets) ? _isNumber(targets[0]) : "length" in vars) ? [targets] : toArray(targets), + tl, + i, + copy, + l, + p, + curTarget, + staggerFunc, + staggerVarsToMerge; + _this3._targets = parsedTargets.length ? _harness(parsedTargets) : _warn("GSAP target " + targets + " not found. https://gsap.com", !_config.nullTargetWarn) || []; + _this3._ptLookup = []; //PropTween lookup. An array containing an object for each target, having keys for each tweening property + + _this3._overwrite = overwrite; + + if (keyframes || stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + vars = _this3.vars; + tl = _this3.timeline = new Timeline({ + data: "nested", + defaults: defaults || {}, + targets: parent && parent.data === "nested" ? parent.vars.targets : parsedTargets + }); // we need to store the targets because for staggers and keyframes, we end up creating an individual tween for each but function-based values need to know the index and the whole Array of targets. + + tl.kill(); + tl.parent = tl._dp = _assertThisInitialized(_this3); + tl._start = 0; + + if (stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + l = parsedTargets.length; + staggerFunc = stagger && distribute(stagger); + + if (_isObject(stagger)) { + //users can pass in callbacks like onStart/onComplete in the stagger object. These should fire with each individual tween. + for (p in stagger) { + if (~_staggerTweenProps.indexOf(p)) { + staggerVarsToMerge || (staggerVarsToMerge = {}); + staggerVarsToMerge[p] = stagger[p]; + } + } + } + + for (i = 0; i < l; i++) { + copy = _copyExcluding(vars, _staggerPropsToSkip); + copy.stagger = 0; + yoyoEase && (copy.yoyoEase = yoyoEase); + staggerVarsToMerge && _merge(copy, staggerVarsToMerge); + curTarget = parsedTargets[i]; //don't just copy duration or delay because if they're a string or function, we'd end up in an infinite loop because _isFuncOrString() would evaluate as true in the child tweens, entering this loop, etc. So we parse the value straight from vars and default to 0. + + copy.duration = +_parseFuncOrString(duration, _assertThisInitialized(_this3), i, curTarget, parsedTargets); + copy.delay = (+_parseFuncOrString(delay, _assertThisInitialized(_this3), i, curTarget, parsedTargets) || 0) - _this3._delay; + + if (!stagger && l === 1 && copy.delay) { + // if someone does delay:"random(1, 5)", repeat:-1, for example, the delay shouldn't be inside the repeat. + _this3._delay = delay = copy.delay; + _this3._start += delay; + copy.delay = 0; + } + + tl.to(curTarget, copy, staggerFunc ? staggerFunc(i, curTarget, parsedTargets) : 0); + tl._ease = _easeMap.none; + } + + tl.duration() ? duration = delay = 0 : _this3.timeline = 0; // if the timeline's duration is 0, we don't need a timeline internally! + } else if (keyframes) { + _inheritDefaults(_setDefaults(tl.vars.defaults, { + ease: "none" + })); + + tl._ease = _parseEase(keyframes.ease || vars.ease || "none"); + var time = 0, + a, + kf, + v; + + if (_isArray(keyframes)) { + keyframes.forEach(function (frame) { + return tl.to(parsedTargets, frame, ">"); + }); + tl.duration(); // to ensure tl._dur is cached because we tap into it for performance purposes in the render() method. + } else { + copy = {}; + + for (p in keyframes) { + p === "ease" || p === "easeEach" || _parseKeyframe(p, keyframes[p], copy, keyframes.easeEach); + } + + for (p in copy) { + a = copy[p].sort(function (a, b) { + return a.t - b.t; + }); + time = 0; + + for (i = 0; i < a.length; i++) { + kf = a[i]; + v = { + ease: kf.e, + duration: (kf.t - (i ? a[i - 1].t : 0)) / 100 * duration + }; + v[p] = kf.v; + tl.to(parsedTargets, v, time); + time += v.duration; + } + } + + tl.duration() < duration && tl.to({}, { + duration: duration - tl.duration() + }); // in case keyframes didn't go to 100% + } + } + + duration || _this3.duration(duration = tl.duration()); + } else { + _this3.timeline = 0; //speed optimization, faster lookups (no going up the prototype chain) + } + + if (overwrite === true && !_suppressOverwrites) { + _overwritingTween = _assertThisInitialized(_this3); + + _globalTimeline.killTweensOf(parsedTargets); + + _overwritingTween = 0; + } + + _addToTimeline(parent, _assertThisInitialized(_this3), position); + + vars.reversed && _this3.reverse(); + vars.paused && _this3.paused(true); + + if (immediateRender || !duration && !keyframes && _this3._start === _roundPrecise(parent._time) && _isNotFalse(immediateRender) && _hasNoPausedAncestors(_assertThisInitialized(_this3)) && parent.data !== "nested") { + _this3._tTime = -_tinyNum; //forces a render without having to set the render() "force" parameter to true because we want to allow lazying by default (using the "force" parameter always forces an immediate full render) + + _this3.render(Math.max(0, -delay) || 0); //in case delay is negative + + } + + scrollTrigger && _scrollTrigger(_assertThisInitialized(_this3), scrollTrigger); + return _this3; + } + + var _proto3 = Tween.prototype; + + _proto3.render = function render(totalTime, suppressEvents, force) { + var prevTime = this._time, + tDur = this._tDur, + dur = this._dur, + isNegative = totalTime < 0, + tTime = totalTime > tDur - _tinyNum && !isNegative ? tDur : totalTime < _tinyNum ? 0 : totalTime, + time, + pt, + iteration, + cycleDuration, + prevIteration, + isYoyo, + ratio, + timeline, + yoyoEase; + + if (!dur) { + _renderZeroDurationTween(this, totalTime, suppressEvents, force); + } else if (tTime !== this._tTime || !totalTime || force || !this._initted && this._tTime || this._startAt && this._zTime < 0 !== isNegative || this._lazy) { + // this senses if we're crossing over the start time, in which case we must record _zTime and force the render, but we do it in this lengthy conditional way for performance reasons (usually we can skip the calculations): this._initted && (this._zTime < 0) !== (totalTime < 0) + time = tTime; + timeline = this.timeline; + + if (this._repeat) { + //adjust the time for repeats and yoyos + cycleDuration = dur + this._rDelay; + + if (this._repeat < -1 && isNegative) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + + time = _roundPrecise(tTime % cycleDuration); //round to avoid floating point errors. (4 % 0.8 should be 0 but some browsers report it as 0.79999999!) + + if (tTime === tDur) { + // the tDur === tTime is for edge cases where there's a lengthy decimal on the duration and it may reach the very end but the time is rendered as not-quite-there (remember, tDur is rounded to 4 decimals whereas dur isn't) + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); // full decimal version of iterations, not the previous iteration (we're reusing prevIteration variable for efficiency) + + iteration = ~~prevIteration; + + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } else if (time > dur) { + time = dur; + } + } + + isYoyo = this._yoyo && iteration & 1; + + if (isYoyo) { + yoyoEase = this._yEase; + time = dur - time; + } + + prevIteration = _animationCycle(this._tTime, cycleDuration); + + if (time === prevTime && !force && this._initted && iteration === prevIteration) { + //could be during the repeatDelay part. No need to render and fire callbacks. + this._tTime = tTime; + return this; + } + + if (iteration !== prevIteration) { + timeline && this._yEase && _propagateYoyoEase(timeline, isYoyo); //repeatRefresh functionality + + if (this.vars.repeatRefresh && !isYoyo && !this._lock && time !== cycleDuration && this._initted) { + // this._time will === cycleDuration when we render at EXACTLY the end of an iteration. Without this condition, it'd often do the repeatRefresh render TWICE (again on the very next tick). + this._lock = force = 1; //force, otherwise if lazy is true, the _attemptInitTween() will return and we'll jump out and get caught bouncing on each tick. + + this.render(_roundPrecise(cycleDuration * iteration), true).invalidate()._lock = 0; + } + } + } + + if (!this._initted) { + if (_attemptInitTween(this, isNegative ? totalTime : time, force, suppressEvents, tTime)) { + this._tTime = 0; // in constructor if immediateRender is true, we set _tTime to -_tinyNum to have the playhead cross the starting point but we can't leave _tTime as a negative number. + + return this; + } + + if (prevTime !== this._time && !(force && this.vars.repeatRefresh && iteration !== prevIteration)) { + // rare edge case - during initialization, an onUpdate in the _startAt (.fromTo()) might force this tween to render at a different spot in which case we should ditch this render() call so that it doesn't revert the values. But we also don't want to dump if we're doing a repeatRefresh render! + return this; + } + + if (dur !== this._dur) { + // while initting, a plugin like InertiaPlugin might alter the duration, so rerun from the start to ensure everything renders as it should. + return this.render(totalTime, suppressEvents, force); + } + } + + this._tTime = tTime; + this._time = time; + + if (!this._act && this._ts) { + this._act = 1; //as long as it's not paused, force it to be active so that if the user renders independent of the parent timeline, it'll be forced to re-render on the next tick. + + this._lazy = 0; + } + + this.ratio = ratio = (yoyoEase || this._ease)(time / dur); + + if (this._from) { + this.ratio = ratio = 1 - ratio; + } + + if (time && !prevTime && !suppressEvents && !iteration) { + _callback(this, "onStart"); + + if (this._tTime !== tTime) { + // in case the onStart triggered a render at a different spot, eject. Like if someone did animation.pause(0.5) or something inside the onStart. + return this; + } + } + + pt = this._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + + timeline && timeline.render(totalTime < 0 ? totalTime : timeline._dur * timeline._ease(time / this._dur), suppressEvents, force) || this._startAt && (this._zTime = totalTime); + + if (this._onUpdate && !suppressEvents) { + isNegative && _rewindStartAt(this, totalTime, suppressEvents, force); //note: for performance reasons, we tuck this conditional logic inside less traveled areas (most tweens don't have an onUpdate). We'd just have it at the end before the onComplete, but the values should be updated before any onUpdate is called, so we ALSO put it here and then if it's not called, we do so later near the onComplete. + + _callback(this, "onUpdate"); + } + + this._repeat && iteration !== prevIteration && this.vars.onRepeat && !suppressEvents && this.parent && _callback(this, "onRepeat"); + + if ((tTime === this._tDur || !tTime) && this._tTime === tTime) { + isNegative && !this._onUpdate && _rewindStartAt(this, totalTime, true, true); + (totalTime || !dur) && (tTime === this._tDur && this._ts > 0 || !tTime && this._ts < 0) && _removeFromParent(this, 1); // don't remove if we're rendering at exactly a time of 0, as there could be autoRevert values that should get set on the next tick (if the playhead goes backward beyond the startTime, negative totalTime). Don't remove if the timeline is reversed and the playhead isn't at 0, otherwise tl.progress(1).reverse() won't work. Only remove if the playhead is at the end and timeScale is positive, or if the playhead is at 0 and the timeScale is negative. + + if (!suppressEvents && !(isNegative && !prevTime) && (tTime || prevTime || isYoyo)) { + // if prevTime and tTime are zero, we shouldn't fire the onReverseComplete. This could happen if you gsap.to(... {paused:true}).play(); + _callback(this, tTime === tDur ? "onComplete" : "onReverseComplete", true); + + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + + return this; + }; + + _proto3.targets = function targets() { + return this._targets; + }; + + _proto3.invalidate = function invalidate(soft) { + // "soft" gives us a way to clear out everything EXCEPT the recorded pre-"from" portion of from() tweens. Otherwise, for example, if you tween.progress(1).render(0, true true).invalidate(), the "from" values would persist and then on the next render, the from() tweens would initialize and the current value would match the "from" values, thus animate from the same value to the same value (no animation). We tap into this in ScrollTrigger's refresh() where we must push a tween to completion and then back again but honor its init state in case the tween is dependent on another tween further up on the page. + (!soft || !this.vars.runBackwards) && (this._startAt = 0); + this._pt = this._op = this._onUpdate = this._lazy = this.ratio = 0; + this._ptLookup = []; + this.timeline && this.timeline.invalidate(soft); + return _Animation2.prototype.invalidate.call(this, soft); + }; + + _proto3.resetTo = function resetTo(property, value, start, startIsRelative, skipRecursion) { + _tickerActive || _ticker.wake(); + this._ts || this.play(); + var time = Math.min(this._dur, (this._dp._time - this._start) * this._ts), + ratio; + this._initted || _initTween(this, time); + ratio = this._ease(time / this._dur); // don't just get tween.ratio because it may not have rendered yet. + // possible future addition to allow an object with multiple values to update, like tween.resetTo({x: 100, y: 200}); At this point, it doesn't seem worth the added kb given the fact that most users will likely opt for the convenient gsap.quickTo() way of interacting with this method. + // if (_isObject(property)) { // performance optimization + // for (p in property) { + // if (_updatePropTweens(this, p, property[p], value ? value[p] : null, start, ratio, time)) { + // return this.resetTo(property, value, start, startIsRelative); // if a PropTween wasn't found for the property, it'll get forced with a re-initialization so we need to jump out and start over again. + // } + // } + // } else { + + if (_updatePropTweens(this, property, value, start, startIsRelative, ratio, time, skipRecursion)) { + return this.resetTo(property, value, start, startIsRelative, 1); // if a PropTween wasn't found for the property, it'll get forced with a re-initialization so we need to jump out and start over again. + } //} + + + _alignPlayhead(this, 0); + + this.parent || _addLinkedListItem(this._dp, this, "_first", "_last", this._dp._sort ? "_start" : 0); + return this.render(0); + }; + + _proto3.kill = function kill(targets, vars) { + if (vars === void 0) { + vars = "all"; + } + + if (!targets && (!vars || vars === "all")) { + this._lazy = this._pt = 0; + this.parent ? _interrupt(this) : this.scrollTrigger && this.scrollTrigger.kill(!!_reverting); + return this; + } + + if (this.timeline) { + var tDur = this.timeline.totalDuration(); + this.timeline.killTweensOf(targets, vars, _overwritingTween && _overwritingTween.vars.overwrite !== true)._first || _interrupt(this); // if nothing is left tweening, interrupt. + + this.parent && tDur !== this.timeline.totalDuration() && _setDuration(this, this._dur * this.timeline._tDur / tDur, 0, 1); // if a nested tween is killed that changes the duration, it should affect this tween's duration. We must use the ratio, though, because sometimes the internal timeline is stretched like for keyframes where they don't all add up to whatever the parent tween's duration was set to. + + return this; + } + + var parsedTargets = this._targets, + killingTargets = targets ? toArray(targets) : parsedTargets, + propTweenLookup = this._ptLookup, + firstPT = this._pt, + overwrittenProps, + curLookup, + curOverwriteProps, + props, + p, + pt, + i; + + if ((!vars || vars === "all") && _arraysMatch(parsedTargets, killingTargets)) { + vars === "all" && (this._pt = 0); + return _interrupt(this); + } + + overwrittenProps = this._op = this._op || []; + + if (vars !== "all") { + //so people can pass in a comma-delimited list of property names + if (_isString(vars)) { + p = {}; + + _forEachName(vars, function (name) { + return p[name] = 1; + }); + + vars = p; + } + + vars = _addAliasesToVars(parsedTargets, vars); + } + + i = parsedTargets.length; + + while (i--) { + if (~killingTargets.indexOf(parsedTargets[i])) { + curLookup = propTweenLookup[i]; + + if (vars === "all") { + overwrittenProps[i] = vars; + props = curLookup; + curOverwriteProps = {}; + } else { + curOverwriteProps = overwrittenProps[i] = overwrittenProps[i] || {}; + props = vars; + } + + for (p in props) { + pt = curLookup && curLookup[p]; + + if (pt) { + if (!("kill" in pt.d) || pt.d.kill(p) === true) { + _removeLinkedListItem(this, pt, "_pt"); + } + + delete curLookup[p]; + } + + if (curOverwriteProps !== "all") { + curOverwriteProps[p] = 1; + } + } + } + } + + this._initted && !this._pt && firstPT && _interrupt(this); //if all tweening properties are killed, kill the tween. Without this line, if there's a tween with multiple targets and then you killTweensOf() each target individually, the tween would technically still remain active and fire its onComplete even though there aren't any more properties tweening. + + return this; + }; + + Tween.to = function to(targets, vars) { + return new Tween(targets, vars, arguments[2]); + }; + + Tween.from = function from(targets, vars) { + return _createTweenType(1, arguments); + }; + + Tween.delayedCall = function delayedCall(delay, callback, params, scope) { + return new Tween(callback, 0, { + immediateRender: false, + lazy: false, + overwrite: false, + delay: delay, + onComplete: callback, + onReverseComplete: callback, + onCompleteParams: params, + onReverseCompleteParams: params, + callbackScope: scope + }); // we must use onReverseComplete too for things like timeline.add(() => {...}) which should be triggered in BOTH directions (forward and reverse) + }; + + Tween.fromTo = function fromTo(targets, fromVars, toVars) { + return _createTweenType(2, arguments); + }; + + Tween.set = function set(targets, vars) { + vars.duration = 0; + vars.repeatDelay || (vars.repeat = 0); + return new Tween(targets, vars); + }; + + Tween.killTweensOf = function killTweensOf(targets, props, onlyActive) { + return _globalTimeline.killTweensOf(targets, props, onlyActive); + }; + + return Tween; +}(Animation); + +_setDefaults(Tween.prototype, { + _targets: [], + _lazy: 0, + _startAt: 0, + _op: 0, + _onInit: 0 +}); //add the pertinent timeline methods to Tween instances so that users can chain conveniently and create a timeline automatically. (removed due to concerns that it'd ultimately add to more confusion especially for beginners) +// _forEachName("to,from,fromTo,set,call,add,addLabel,addPause", name => { +// Tween.prototype[name] = function() { +// let tl = new Timeline(); +// return _addToTimeline(tl, this)[name].apply(tl, toArray(arguments)); +// } +// }); +//for backward compatibility. Leverage the timeline calls. + + +_forEachName("staggerTo,staggerFrom,staggerFromTo", function (name) { + Tween[name] = function () { + var tl = new Timeline(), + params = _slice.call(arguments, 0); + + params.splice(name === "staggerFromTo" ? 5 : 4, 0, 0); + return tl[name].apply(tl, params); + }; +}); +/* + * -------------------------------------------------------------------------------------- + * PROPTWEEN + * -------------------------------------------------------------------------------------- + */ + + +var _setterPlain = function _setterPlain(target, property, value) { + return target[property] = value; +}, + _setterFunc = function _setterFunc(target, property, value) { + return target[property](value); +}, + _setterFuncWithParam = function _setterFuncWithParam(target, property, value, data) { + return target[property](data.fp, value); +}, + _setterAttribute = function _setterAttribute(target, property, value) { + return target.setAttribute(property, value); +}, + _getSetter = function _getSetter(target, property) { + return _isFunction(target[property]) ? _setterFunc : _isUndefined(target[property]) && target.setAttribute ? _setterAttribute : _setterPlain; +}, + _renderPlain = function _renderPlain(ratio, data) { + return data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 1000000) / 1000000, data); +}, + _renderBoolean = function _renderBoolean(ratio, data) { + return data.set(data.t, data.p, !!(data.s + data.c * ratio), data); +}, + _renderComplexString = function _renderComplexString(ratio, data) { + var pt = data._pt, + s = ""; + + if (!ratio && data.b) { + //b = beginning string + s = data.b; + } else if (ratio === 1 && data.e) { + //e = ending string + s = data.e; + } else { + while (pt) { + s = pt.p + (pt.m ? pt.m(pt.s + pt.c * ratio) : Math.round((pt.s + pt.c * ratio) * 10000) / 10000) + s; //we use the "p" property for the text inbetween (like a suffix). And in the context of a complex string, the modifier (m) is typically just Math.round(), like for RGB colors. + + pt = pt._next; + } + + s += data.c; //we use the "c" of the PropTween to store the final chunk of non-numeric text. + } + + data.set(data.t, data.p, s, data); +}, + _renderPropTweens = function _renderPropTweens(ratio, data) { + var pt = data._pt; + + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } +}, + _addPluginModifier = function _addPluginModifier(modifier, tween, target, property) { + var pt = this._pt, + next; + + while (pt) { + next = pt._next; + pt.p === property && pt.modifier(modifier, tween, target); + pt = next; + } +}, + _killPropTweensOf = function _killPropTweensOf(property) { + var pt = this._pt, + hasNonDependentRemaining, + next; + + while (pt) { + next = pt._next; + + if (pt.p === property && !pt.op || pt.op === property) { + _removeLinkedListItem(this, pt, "_pt"); + } else if (!pt.dep) { + hasNonDependentRemaining = 1; + } + + pt = next; + } + + return !hasNonDependentRemaining; +}, + _setterWithModifier = function _setterWithModifier(target, property, value, data) { + data.mSet(target, property, data.m.call(data.tween, value, data.mt), data); +}, + _sortPropTweensByPriority = function _sortPropTweensByPriority(parent) { + var pt = parent._pt, + next, + pt2, + first, + last; //sorts the PropTween linked list in order of priority because some plugins need to do their work after ALL of the PropTweens were created (like RoundPropsPlugin and ModifiersPlugin) + + while (pt) { + next = pt._next; + pt2 = first; + + while (pt2 && pt2.pr > pt.pr) { + pt2 = pt2._next; + } + + if (pt._prev = pt2 ? pt2._prev : last) { + pt._prev._next = pt; + } else { + first = pt; + } + + if (pt._next = pt2) { + pt2._prev = pt; + } else { + last = pt; + } + + pt = next; + } + + parent._pt = first; +}; //PropTween key: t = target, p = prop, r = renderer, d = data, s = start, c = change, op = overwriteProperty (ONLY populated when it's different than p), pr = priority, _next/_prev for the linked list siblings, set = setter, m = modifier, mSet = modifierSetter (the original setter, before a modifier was added) + + +export var PropTween = /*#__PURE__*/function () { + function PropTween(next, target, prop, start, change, renderer, data, setter, priority) { + this.t = target; + this.s = start; + this.c = change; + this.p = prop; + this.r = renderer || _renderPlain; + this.d = data || this; + this.set = setter || _setterPlain; + this.pr = priority || 0; + this._next = next; + + if (next) { + next._prev = this; + } + } + + var _proto4 = PropTween.prototype; + + _proto4.modifier = function modifier(func, tween, target) { + this.mSet = this.mSet || this.set; //in case it was already set (a PropTween can only have one modifier) + + this.set = _setterWithModifier; + this.m = func; + this.mt = target; //modifier target + + this.tween = tween; + }; + + return PropTween; +}(); //Initialization tasks + +_forEachName(_callbackNames + "parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger", function (name) { + return _reservedProps[name] = 1; +}); + +_globals.TweenMax = _globals.TweenLite = Tween; +_globals.TimelineLite = _globals.TimelineMax = Timeline; +_globalTimeline = new Timeline({ + sortChildren: false, + defaults: _defaults, + autoRemoveChildren: true, + id: "root", + smoothChildTiming: true +}); +_config.stringFilter = _colorStringFilter; + +var _media = [], + _listeners = {}, + _emptyArray = [], + _lastMediaTime = 0, + _contextID = 0, + _dispatch = function _dispatch(type) { + return (_listeners[type] || _emptyArray).map(function (f) { + return f(); + }); +}, + _onMediaChange = function _onMediaChange() { + var time = Date.now(), + matches = []; + + if (time - _lastMediaTime > 2) { + _dispatch("matchMediaInit"); + + _media.forEach(function (c) { + var queries = c.queries, + conditions = c.conditions, + match, + p, + anyMatch, + toggled; + + for (p in queries) { + match = _win.matchMedia(queries[p]).matches; // Firefox doesn't update the "matches" property of the MediaQueryList object correctly - it only does so as it calls its change handler - so we must re-create a media query here to ensure it's accurate. + + match && (anyMatch = 1); + + if (match !== conditions[p]) { + conditions[p] = match; + toggled = 1; + } + } + + if (toggled) { + c.revert(); + anyMatch && matches.push(c); + } + }); + + _dispatch("matchMediaRevert"); + + matches.forEach(function (c) { + return c.onMatch(c, function (func) { + return c.add(null, func); + }); + }); + _lastMediaTime = time; + + _dispatch("matchMedia"); + } +}; + +var Context = /*#__PURE__*/function () { + function Context(func, scope) { + this.selector = scope && selector(scope); + this.data = []; + this._r = []; // returned/cleanup functions + + this.isReverted = false; + this.id = _contextID++; // to work around issues that frameworks like Vue cause by making things into Proxies which make it impossible to do something like _media.indexOf(this) because "this" would no longer refer to the Context instance itself - it'd refer to a Proxy! We needed a way to identify the context uniquely + + func && this.add(func); + } + + var _proto5 = Context.prototype; + + _proto5.add = function add(name, func, scope) { + // possible future addition if we need the ability to add() an animation to a context and for whatever reason cannot create that animation inside of a context.add(() => {...}) function. + // if (name && _isFunction(name.revert)) { + // this.data.push(name); + // return (name._ctx = this); + // } + if (_isFunction(name)) { + scope = func; + func = name; + name = _isFunction; + } + + var self = this, + f = function f() { + var prev = _context, + prevSelector = self.selector, + result; + prev && prev !== self && prev.data.push(self); + scope && (self.selector = selector(scope)); + _context = self; + result = func.apply(self, arguments); + _isFunction(result) && self._r.push(result); + _context = prev; + self.selector = prevSelector; + self.isReverted = false; + return result; + }; + + self.last = f; + return name === _isFunction ? f(self, function (func) { + return self.add(null, func); + }) : name ? self[name] = f : f; + }; + + _proto5.ignore = function ignore(func) { + var prev = _context; + _context = null; + func(this); + _context = prev; + }; + + _proto5.getTweens = function getTweens() { + var a = []; + this.data.forEach(function (e) { + return e instanceof Context ? a.push.apply(a, e.getTweens()) : e instanceof Tween && !(e.parent && e.parent.data === "nested") && a.push(e); + }); + return a; + }; + + _proto5.clear = function clear() { + this._r.length = this.data.length = 0; + }; + + _proto5.kill = function kill(revert, matchMedia) { + var _this4 = this; + + if (revert) { + (function () { + var tweens = _this4.getTweens(), + i = _this4.data.length, + t; + + while (i--) { + // Flip plugin tweens are very different in that they should actually be pushed to their end. The plugin replaces the timeline's .revert() method to do exactly that. But we also need to remove any of those nested tweens inside the flip timeline so that they don't get individually reverted. + t = _this4.data[i]; + + if (t.data === "isFlip") { + t.revert(); + t.getChildren(true, true, false).forEach(function (tween) { + return tweens.splice(tweens.indexOf(tween), 1); + }); + } + } // save as an object so that we can cache the globalTime for each tween to optimize performance during the sort + + + tweens.map(function (t) { + return { + g: t._dur || t._delay || t._sat && !t._sat.vars.immediateRender ? t.globalTime(0) : -Infinity, + t: t + }; + }).sort(function (a, b) { + return b.g - a.g || -Infinity; + }).forEach(function (o) { + return o.t.revert(revert); + }); // note: all of the _startAt tweens should be reverted in reverse order that they were created, and they'll all have the same globalTime (-1) so the " || -1" in the sort keeps the order properly. + + i = _this4.data.length; + + while (i--) { + // make sure we loop backwards so that, for example, SplitTexts that were created later on the same element get reverted first + t = _this4.data[i]; + + if (t instanceof Timeline) { + if (t.data !== "nested") { + t.scrollTrigger && t.scrollTrigger.revert(); + t.kill(); // don't revert() the timeline because that's duplicating efforts since we already reverted all the tweens + } + } else { + !(t instanceof Tween) && t.revert && t.revert(revert); + } + } + + _this4._r.forEach(function (f) { + return f(revert, _this4); + }); + + _this4.isReverted = true; + })(); + } else { + this.data.forEach(function (e) { + return e.kill && e.kill(); + }); + } + + this.clear(); + + if (matchMedia) { + var i = _media.length; + + while (i--) { + // previously, we checked _media.indexOf(this), but some frameworks like Vue enforce Proxy objects that make it impossible to get the proper result that way, so we must use a unique ID number instead. + _media[i].id === this.id && _media.splice(i, 1); + } + } + } // killWithCleanup() { + // this.kill(); + // this._r.forEach(f => f(false, this)); + // } + ; + + _proto5.revert = function revert(config) { + this.kill(config || {}); + }; + + return Context; +}(); + +var MatchMedia = /*#__PURE__*/function () { + function MatchMedia(scope) { + this.contexts = []; + this.scope = scope; + _context && _context.data.push(this); + } + + var _proto6 = MatchMedia.prototype; + + _proto6.add = function add(conditions, func, scope) { + _isObject(conditions) || (conditions = { + matches: conditions + }); + var context = new Context(0, scope || this.scope), + cond = context.conditions = {}, + mq, + p, + active; + _context && !context.selector && (context.selector = _context.selector); // in case a context is created inside a context. Like a gsap.matchMedia() that's inside a scoped gsap.context() + + this.contexts.push(context); + func = context.add("onMatch", func); + context.queries = conditions; + + for (p in conditions) { + if (p === "all") { + active = 1; + } else { + mq = _win.matchMedia(conditions[p]); + + if (mq) { + _media.indexOf(context) < 0 && _media.push(context); + (cond[p] = mq.matches) && (active = 1); + mq.addListener ? mq.addListener(_onMediaChange) : mq.addEventListener("change", _onMediaChange); + } + } + } + + active && func(context, function (f) { + return context.add(null, f); + }); + return this; + } // refresh() { + // let time = _lastMediaTime, + // media = _media; + // _lastMediaTime = -1; + // _media = this.contexts; + // _onMediaChange(); + // _lastMediaTime = time; + // _media = media; + // } + ; + + _proto6.revert = function revert(config) { + this.kill(config || {}); + }; + + _proto6.kill = function kill(revert) { + this.contexts.forEach(function (c) { + return c.kill(revert, true); + }); + }; + + return MatchMedia; +}(); +/* + * -------------------------------------------------------------------------------------- + * GSAP + * -------------------------------------------------------------------------------------- + */ + + +var _gsap = { + registerPlugin: function registerPlugin() { + for (var _len2 = arguments.length, args = new Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { + args[_key2] = arguments[_key2]; + } + + args.forEach(function (config) { + return _createPlugin(config); + }); + }, + timeline: function timeline(vars) { + return new Timeline(vars); + }, + getTweensOf: function getTweensOf(targets, onlyActive) { + return _globalTimeline.getTweensOf(targets, onlyActive); + }, + getProperty: function getProperty(target, property, unit, uncache) { + _isString(target) && (target = toArray(target)[0]); //in case selector text or an array is passed in + + var getter = _getCache(target || {}).get, + format = unit ? _passThrough : _numericIfPossible; + + unit === "native" && (unit = ""); + return !target ? target : !property ? function (property, unit, uncache) { + return format((_plugins[property] && _plugins[property].get || getter)(target, property, unit, uncache)); + } : format((_plugins[property] && _plugins[property].get || getter)(target, property, unit, uncache)); + }, + quickSetter: function quickSetter(target, property, unit) { + target = toArray(target); + + if (target.length > 1) { + var setters = target.map(function (t) { + return gsap.quickSetter(t, property, unit); + }), + l = setters.length; + return function (value) { + var i = l; + + while (i--) { + setters[i](value); + } + }; + } + + target = target[0] || {}; + + var Plugin = _plugins[property], + cache = _getCache(target), + p = cache.harness && (cache.harness.aliases || {})[property] || property, + // in case it's an alias, like "rotate" for "rotation". + setter = Plugin ? function (value) { + var p = new Plugin(); + _quickTween._pt = 0; + p.init(target, unit ? value + unit : value, _quickTween, 0, [target]); + p.render(1, p); + _quickTween._pt && _renderPropTweens(1, _quickTween); + } : cache.set(target, p); + + return Plugin ? setter : function (value) { + return setter(target, p, unit ? value + unit : value, cache, 1); + }; + }, + quickTo: function quickTo(target, property, vars) { + var _setDefaults2; + + var tween = gsap.to(target, _setDefaults((_setDefaults2 = {}, _setDefaults2[property] = "+=0.1", _setDefaults2.paused = true, _setDefaults2.stagger = 0, _setDefaults2), vars || {})), + func = function func(value, start, startIsRelative) { + return tween.resetTo(property, value, start, startIsRelative); + }; + + func.tween = tween; + return func; + }, + isTweening: function isTweening(targets) { + return _globalTimeline.getTweensOf(targets, true).length > 0; + }, + defaults: function defaults(value) { + value && value.ease && (value.ease = _parseEase(value.ease, _defaults.ease)); + return _mergeDeep(_defaults, value || {}); + }, + config: function config(value) { + return _mergeDeep(_config, value || {}); + }, + registerEffect: function registerEffect(_ref3) { + var name = _ref3.name, + effect = _ref3.effect, + plugins = _ref3.plugins, + defaults = _ref3.defaults, + extendTimeline = _ref3.extendTimeline; + (plugins || "").split(",").forEach(function (pluginName) { + return pluginName && !_plugins[pluginName] && !_globals[pluginName] && _warn(name + " effect requires " + pluginName + " plugin."); + }); + + _effects[name] = function (targets, vars, tl) { + return effect(toArray(targets), _setDefaults(vars || {}, defaults), tl); + }; + + if (extendTimeline) { + Timeline.prototype[name] = function (targets, vars, position) { + return this.add(_effects[name](targets, _isObject(vars) ? vars : (position = vars) && {}, this), position); + }; + } + }, + registerEase: function registerEase(name, ease) { + _easeMap[name] = _parseEase(ease); + }, + parseEase: function parseEase(ease, defaultEase) { + return arguments.length ? _parseEase(ease, defaultEase) : _easeMap; + }, + getById: function getById(id) { + return _globalTimeline.getById(id); + }, + exportRoot: function exportRoot(vars, includeDelayedCalls) { + if (vars === void 0) { + vars = {}; + } + + var tl = new Timeline(vars), + child, + next; + tl.smoothChildTiming = _isNotFalse(vars.smoothChildTiming); + + _globalTimeline.remove(tl); + + tl._dp = 0; //otherwise it'll get re-activated when adding children and be re-introduced into _globalTimeline's linked list (then added to itself). + + tl._time = tl._tTime = _globalTimeline._time; + child = _globalTimeline._first; + + while (child) { + next = child._next; + + if (includeDelayedCalls || !(!child._dur && child instanceof Tween && child.vars.onComplete === child._targets[0])) { + _addToTimeline(tl, child, child._start - child._delay); + } + + child = next; + } + + _addToTimeline(_globalTimeline, tl, 0); + + return tl; + }, + context: function context(func, scope) { + return func ? new Context(func, scope) : _context; + }, + matchMedia: function matchMedia(scope) { + return new MatchMedia(scope); + }, + matchMediaRefresh: function matchMediaRefresh() { + return _media.forEach(function (c) { + var cond = c.conditions, + found, + p; + + for (p in cond) { + if (cond[p]) { + cond[p] = false; + found = 1; + } + } + + found && c.revert(); + }) || _onMediaChange(); + }, + addEventListener: function addEventListener(type, callback) { + var a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); + }, + removeEventListener: function removeEventListener(type, callback) { + var a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); + }, + utils: { + wrap: wrap, + wrapYoyo: wrapYoyo, + distribute: distribute, + random: random, + snap: snap, + normalize: normalize, + getUnit: getUnit, + clamp: clamp, + splitColor: splitColor, + toArray: toArray, + selector: selector, + mapRange: mapRange, + pipe: pipe, + unitize: unitize, + interpolate: interpolate, + shuffle: shuffle + }, + install: _install, + effects: _effects, + ticker: _ticker, + updateRoot: Timeline.updateRoot, + plugins: _plugins, + globalTimeline: _globalTimeline, + core: { + PropTween: PropTween, + globals: _addGlobal, + Tween: Tween, + Timeline: Timeline, + Animation: Animation, + getCache: _getCache, + _removeLinkedListItem: _removeLinkedListItem, + reverting: function reverting() { + return _reverting; + }, + context: function context(toAdd) { + if (toAdd && _context) { + _context.data.push(toAdd); + + toAdd._ctx = _context; + } + + return _context; + }, + suppressOverwrites: function suppressOverwrites(value) { + return _suppressOverwrites = value; + } + } +}; + +_forEachName("to,from,fromTo,delayedCall,set,killTweensOf", function (name) { + return _gsap[name] = Tween[name]; +}); + +_ticker.add(Timeline.updateRoot); + +_quickTween = _gsap.to({}, { + duration: 0 +}); // ---- EXTRA PLUGINS -------------------------------------------------------- + +var _getPluginPropTween = function _getPluginPropTween(plugin, prop) { + var pt = plugin._pt; + + while (pt && pt.p !== prop && pt.op !== prop && pt.fp !== prop) { + pt = pt._next; + } + + return pt; +}, + _addModifiers = function _addModifiers(tween, modifiers) { + var targets = tween._targets, + p, + i, + pt; + + for (p in modifiers) { + i = targets.length; + + while (i--) { + pt = tween._ptLookup[i][p]; + + if (pt && (pt = pt.d)) { + if (pt._pt) { + // is a plugin + pt = _getPluginPropTween(pt, p); + } + + pt && pt.modifier && pt.modifier(modifiers[p], tween, targets[i], p); + } + } + } +}, + _buildModifierPlugin = function _buildModifierPlugin(name, modifier) { + return { + name: name, + rawVars: 1, + //don't pre-process function-based values or "random()" strings. + init: function init(target, vars, tween) { + tween._onInit = function (tween) { + var temp, p; + + if (_isString(vars)) { + temp = {}; + + _forEachName(vars, function (name) { + return temp[name] = 1; + }); //if the user passes in a comma-delimited list of property names to roundProps, like "x,y", we round to whole numbers. + + + vars = temp; + } + + if (modifier) { + temp = {}; + + for (p in vars) { + temp[p] = modifier(vars[p]); + } + + vars = temp; + } + + _addModifiers(tween, vars); + }; + } + }; +}; //register core plugins + + +export var gsap = _gsap.registerPlugin({ + name: "attr", + init: function init(target, vars, tween, index, targets) { + var p, pt, v; + this.tween = tween; + + for (p in vars) { + v = target.getAttribute(p) || ""; + pt = this.add(target, "setAttribute", (v || 0) + "", vars[p], index, targets, 0, 0, p); + pt.op = p; + pt.b = v; // record the beginning value so we can revert() + + this._props.push(p); + } + }, + render: function render(ratio, data) { + var pt = data._pt; + + while (pt) { + _reverting ? pt.set(pt.t, pt.p, pt.b, pt) : pt.r(ratio, pt.d); // if reverting, go back to the original (pt.b) + + pt = pt._next; + } + } +}, { + name: "endArray", + init: function init(target, value) { + var i = value.length; + + while (i--) { + this.add(target, i, target[i] || 0, value[i], 0, 0, 0, 0, 0, 1); + } + } +}, _buildModifierPlugin("roundProps", _roundModifier), _buildModifierPlugin("modifiers"), _buildModifierPlugin("snap", snap)) || _gsap; //to prevent the core plugins from being dropped via aggressive tree shaking, we must include them in the variable declaration in this way. + +Tween.version = Timeline.version = gsap.version = "3.12.7"; +_coreReady = 1; +_windowExists() && _wake(); +var Power0 = _easeMap.Power0, + Power1 = _easeMap.Power1, + Power2 = _easeMap.Power2, + Power3 = _easeMap.Power3, + Power4 = _easeMap.Power4, + Linear = _easeMap.Linear, + Quad = _easeMap.Quad, + Cubic = _easeMap.Cubic, + Quart = _easeMap.Quart, + Quint = _easeMap.Quint, + Strong = _easeMap.Strong, + Elastic = _easeMap.Elastic, + Back = _easeMap.Back, + SteppedEase = _easeMap.SteppedEase, + Bounce = _easeMap.Bounce, + Sine = _easeMap.Sine, + Expo = _easeMap.Expo, + Circ = _easeMap.Circ; +export { Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ }; +export { Tween as TweenMax, Tween as TweenLite, Timeline as TimelineMax, Timeline as TimelineLite, gsap as default, wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, selector, mapRange, pipe, unitize, interpolate, shuffle }; //export some internal methods/orojects for use in CSSPlugin so that we can externalize that file and allow custom builds that exclude it. + +export { _getProperty, _numExp, _numWithUnitExp, _isString, _isUndefined, _renderComplexString, _relExp, _setDefaults, _removeLinkedListItem, _forEachName, _sortPropTweensByPriority, _colorStringFilter, _replaceRandom, _checkPlugin, _plugins, _ticker, _config, _roundModifier, _round, _missingPlugin, _getSetter, _getCache, _colorExp, _parseRelative }; \ No newline at end of file diff --git a/node_modules/gsap/index.js b/node_modules/gsap/index.js new file mode 100644 index 0000000000000000000000000000000000000000..c94f3329f75ee88c9bab8ab93f1167199eac667a --- /dev/null +++ b/node_modules/gsap/index.js @@ -0,0 +1,6 @@ +import { gsap, Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ, TweenLite, TimelineLite, TimelineMax } from "./gsap-core.js"; +import { CSSPlugin } from "./CSSPlugin.js"; +var gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, + // to protect from tree shaking +TweenMaxWithCSS = gsapWithCSS.core.Tween; +export { gsapWithCSS as gsap, gsapWithCSS as default, CSSPlugin, TweenMaxWithCSS as TweenMax, TweenLite, TimelineMax, TimelineLite, Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ }; \ No newline at end of file diff --git a/node_modules/gsap/package.json b/node_modules/gsap/package.json new file mode 100644 index 0000000000000000000000000000000000000000..30e8cb6b98aa26eb378db8db465c4c1ea2ce76a7 --- /dev/null +++ b/node_modules/gsap/package.json @@ -0,0 +1,50 @@ +{ + "name": "gsap", + "version": "3.12.7", + "description": "GSAP is a framework-agnostic JavaScript animation library that turns developers into animation superheroes. Build high-performance animations that work in **every** major browser. Animate CSS, SVG, canvas, React, Vue, WebGL, colors, strings, motion paths, generic objects...anything JavaScript can touch! The ScrollTrigger plugin lets you create jaw-dropping scroll-based animations with minimal code. No other library delivers such advanced sequencing, reliability, and tight control while solving real-world problems on millions of sites. GSAP works around countless browser inconsistencies; your animations **just work**. At its core, GSAP is a high-speed property manipulator, updating values over time with extreme accuracy.", + "homepage": "https://gsap.com", + "module": "index.js", + "main": "dist/gsap.js", + "types": "types/index.d.ts", + "sideEffects": false, + "keywords": [ + "GSAP", + "GreenSock", + "animation", + "MotionPathPlugin", + "motion", + "motionPath", + "matchMedia", + "easing", + "ScrollTrigger", + "ScrollSmoother", + "Observer", + "JavaScript", + "PixiPlugin", + "CustomEase", + "Flip", + "SVG", + "3D", + "2D", + "transform", + "morph", + "morphing", + "tweening" + ], + "maintainers": [ + { + "name": "Jack Doyle", + "email": "jack@greensock.com", + "web": "https://gsap.com" + } + ], + "license": "Standard 'no charge' license: https://gsap.com/standard-license. Club GSAP members get more: https://gsap.com/licensing/. Why GreenSock doesn't employ an MIT license: https://gsap.com/why-license/", + "bugs": { + "url": "https://gsap.com/community/" + }, + "repository": { + "type": "git", + "url": "https://github.com/greensock/GSAP" + } + +} \ No newline at end of file diff --git a/node_modules/gsap/src/CSSPlugin.js b/node_modules/gsap/src/CSSPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..42a93954b2b011d6fab3acd1edbcf301bdcd2523 --- /dev/null +++ b/node_modules/gsap/src/CSSPlugin.js @@ -0,0 +1,1152 @@ +/*! + * CSSPlugin 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +import {gsap, _getProperty, _numExp, _numWithUnitExp, getUnit, _isString, _isUndefined, _renderComplexString, _relExp, _forEachName, _sortPropTweensByPriority, _colorStringFilter, _checkPlugin, _replaceRandom, _plugins, GSCache, PropTween, _config, _ticker, _round, _missingPlugin, _getSetter, _getCache, _colorExp, _parseRelative, + _setDefaults, _removeLinkedListItem //for the commented-out className feature. +} from "./gsap-core.js"; + +let _win, _doc, _docElement, _pluginInitted, _tempDiv, _tempDivStyler, _recentSetterPlugin, _reverting, + _windowExists = () => typeof(window) !== "undefined", + _transformProps = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _atan2 = Math.atan2, + _bigNum = 1e8, + _capsExp = /([A-Z])/g, + _horizontalExp = /(left|right|width|margin|padding|x)/i, + _complexExp = /[\s,\(]\S/, + _propertyAliases = {autoAlpha:"opacity,visibility", scale:"scaleX,scaleY", alpha:"opacity"}, + _renderCSSProp = (ratio, data) => data.set(data.t, data.p, (Math.round((data.s + data.c * ratio) * 10000) / 10000) + data.u, data), + _renderPropWithEnd = (ratio, data) => data.set(data.t, data.p, ratio === 1 ? data.e : (Math.round((data.s + data.c * ratio) * 10000) / 10000) + data.u, data), + _renderCSSPropWithBeginning = (ratio, data) => data.set(data.t, data.p, ratio ? (Math.round((data.s + data.c * ratio) * 10000) / 10000) + data.u : data.b, data), //if units change, we need a way to render the original unit/value when the tween goes all the way back to the beginning (ratio:0) + _renderRoundedCSSProp = (ratio, data) => { + let value = data.s + data.c * ratio; + data.set(data.t, data.p, ~~(value + (value < 0 ? -.5 : .5)) + data.u, data); + }, + _renderNonTweeningValue = (ratio, data) => data.set(data.t, data.p, ratio ? data.e : data.b, data), + _renderNonTweeningValueOnlyAtEnd = (ratio, data) => data.set(data.t, data.p, ratio !== 1 ? data.b : data.e, data), + _setterCSSStyle = (target, property, value) => target.style[property] = value, + _setterCSSProp = (target, property, value) => target.style.setProperty(property, value), + _setterTransform = (target, property, value) => target._gsap[property] = value, + _setterScale = (target, property, value) => target._gsap.scaleX = target._gsap.scaleY = value, + _setterScaleWithRender = (target, property, value, data, ratio) => { + let cache = target._gsap; + cache.scaleX = cache.scaleY = value; + cache.renderTransform(ratio, cache); + }, + _setterTransformWithRender = (target, property, value, data, ratio) => { + let cache = target._gsap; + cache[property] = value; + cache.renderTransform(ratio, cache); + }, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _saveStyle = function(property, isNotCSS) { + let target = this.target, + style = target.style, + cache = target._gsap; + if ((property in _transformProps) && style) { + this.tfm = this.tfm || {}; + if (property !== "transform") { + property = _propertyAliases[property] || property; + ~property.indexOf(",") ? property.split(",").forEach(a => this.tfm[a] = _get(target, a)) : (this.tfm[property] = cache.x ? cache[property] : _get(target, property)); // note: scale would map to "scaleX,scaleY", thus we loop and apply them both. + property === _transformOriginProp && (this.tfm.zOrigin = cache.zOrigin); + } else { + return _propertyAliases.transform.split(",").forEach(p => _saveStyle.call(this, p, isNotCSS)); + } + if (this.props.indexOf(_transformProp) >= 0) { return; } + if (cache.svg) { + this.svgo = target.getAttribute("data-svg-origin"); + this.props.push(_transformOriginProp, isNotCSS, ""); + } + property = _transformProp; + } + (style || isNotCSS) && this.props.push(property, isNotCSS, style[property]); + }, + _removeIndependentTransforms = style => { + if (style.translate) { + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } + }, + _revertStyle = function() { + let props = this.props, + target = this.target, + style = target.style, + cache = target._gsap, + i, p; + for (i = 0; i < props.length; i+=3) { // stored like this: property, isNotCSS, value + if (!props[i+1]) { + props[i+2] ? (style[props[i]] = props[i+2]) : style.removeProperty(props[i].substr(0,2) === "--" ? props[i] : props[i].replace(_capsExp, "-$1").toLowerCase()); + } else if (props[i+1] === 2) { // non-CSS value (function-based) + target[props[i]](props[i+2]); + } else { // non-CSS value (not function-based) + target[props[i]] = props[i+2]; + } + } + if (this.tfm) { + for (p in this.tfm) { + cache[p] = this.tfm[p]; + } + if (cache.svg) { + cache.renderTransform(); + target.setAttribute("data-svg-origin", this.svgo || ""); + } + i = _reverting(); + if ((!i || !i.isStart) && !style[_transformProp]) { + _removeIndependentTransforms(style); + if (cache.zOrigin && style[_transformOriginProp]) { + style[_transformOriginProp] += " " + cache.zOrigin + "px"; // since we're uncaching, we must put the zOrigin back into the transformOrigin so that we can pull it out accurately when we parse again. Otherwise, we'd lose the z portion of the origin since we extract it to protect from Safari bugs. + cache.zOrigin = 0; + cache.renderTransform(); + } + cache.uncache = 1; // if it's a startAt that's being reverted in the _initTween() of the core, we don't need to uncache transforms. This is purely a performance optimization. + } + } + }, + _getStyleSaver = (target, properties) => { + let saver = { + target, + props: [], + revert: _revertStyle, + save: _saveStyle + }; + target._gsap || gsap.core.getCache(target); // just make sure there's a _gsap cache defined because we read from it in _saveStyle() and it's more efficient to just check it here once. + properties && target.style && target.nodeType && properties.split(",").forEach(p => saver.save(p)); // make sure it's a DOM node too. + return saver; + }, + _supports3D, + _createElement = (type, ns) => { + let e = _doc.createElementNS ? _doc.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc.createElement(type); //some servers swap in https for http in the namespace which can break things, making "style" inaccessible. + return e && e.style ? e : _doc.createElement(type); //some environments won't allow access to the element's style when created with a namespace in which case we default to the standard createElement() to work around the issue. Also note that when GSAP is embedded directly inside an SVG file, createElement() won't allow access to the style object in Firefox (see https://gsap.com/forums/topic/20215-problem-using-tweenmax-in-standalone-self-containing-svg-file-err-cannot-set-property-csstext-of-undefined/). + }, + _getComputedProperty = (target, property, skipPrefixFallback) => { + let cs = getComputedStyle(target); + return cs[property] || cs.getPropertyValue(property.replace(_capsExp, "-$1").toLowerCase()) || cs.getPropertyValue(property) || (!skipPrefixFallback && _getComputedProperty(target, _checkPropPrefix(property) || property, 1)) || ""; //css variables may not need caps swapped out for dashes and lowercase. + }, + _prefixes = "O,Moz,ms,Ms,Webkit".split(","), + _checkPropPrefix = (property, element, preferPrefix) => { + let e = element || _tempDiv, + s = e.style, + i = 5; + if (property in s && !preferPrefix) { + return property; + } + property = property.charAt(0).toUpperCase() + property.substr(1); + while (i-- && !((_prefixes[i]+property) in s)) { } + return (i < 0) ? null : ((i === 3) ? "ms" : (i >= 0) ? _prefixes[i] : "") + property; + }, + _initCore = () => { + if (_windowExists() && window.document) { + _win = window; + _doc = _win.document; + _docElement = _doc.documentElement; + _tempDiv = _createElement("div") || {style:{}}; + _tempDivStyler = _createElement("div"); + _transformProp = _checkPropPrefix(_transformProp); + _transformOriginProp = _transformProp + "Origin"; + _tempDiv.style.cssText = "border-width:0;line-height:0;position:absolute;padding:0"; //make sure to override certain properties that may contaminate measurements, in case the user has overreaching style sheets. + _supports3D = !!_checkPropPrefix("perspective"); + _reverting = gsap.core.reverting; + _pluginInitted = 1; + } + }, + _getReparentedCloneBBox = target => { //works around issues in some browsers (like Firefox) that don't correctly report getBBox() on SVG elements inside a <defs> element and/or <mask>. We try creating an SVG, adding it to the documentElement and toss the element in there so that it's definitely part of the rendering tree, then grab the bbox and if it works, we actually swap out the original getBBox() method for our own that does these extra steps whenever getBBox is needed. This helps ensure that performance is optimal (only do all these extra steps when absolutely necessary...most elements don't need it). + let owner = target.ownerSVGElement, + svg = _createElement("svg", (owner && owner.getAttribute("xmlns")) || "http://www.w3.org/2000/svg"), + clone = target.cloneNode(true), + bbox; + clone.style.display = "block"; + svg.appendChild(clone); + _docElement.appendChild(svg); + try { + bbox = clone.getBBox(); + } catch (e) { } + svg.removeChild(clone); + _docElement.removeChild(svg); + return bbox; + }, + _getAttributeFallbacks = (target, attributesArray) => { + let i = attributesArray.length; + while (i--) { + if (target.hasAttribute(attributesArray[i])) { + return target.getAttribute(attributesArray[i]); + } + } + }, + _getBBox = target => { + let bounds, cloned; + try { + bounds = target.getBBox(); //Firefox throws errors if you try calling getBBox() on an SVG element that's not rendered (like in a <symbol> or <defs>). https://bugzilla.mozilla.org/show_bug.cgi?id=612118 + } catch (error) { + bounds = _getReparentedCloneBBox(target); + cloned = 1; + } + (bounds && (bounds.width || bounds.height)) || cloned || (bounds = _getReparentedCloneBBox(target)); + //some browsers (like Firefox) misreport the bounds if the element has zero width and height (it just assumes it's at x:0, y:0), thus we need to manually grab the position in that case. + return (bounds && !bounds.width && !bounds.x && !bounds.y) ? {x: +_getAttributeFallbacks(target, ["x","cx","x1"]) || 0, y:+_getAttributeFallbacks(target, ["y","cy","y1"]) || 0, width:0, height:0} : bounds; + }, + _isSVG = e => !!(e.getCTM && (!e.parentNode || e.ownerSVGElement) && _getBBox(e)), //reports if the element is an SVG on which getBBox() actually works + _removeProperty = (target, property) => { + if (property) { + let style = target.style, + first2Chars; + if (property in _transformProps && property !== _transformOriginProp) { + property = _transformProp; + } + if (style.removeProperty) { + first2Chars = property.substr(0,2); + if (first2Chars === "ms" || property.substr(0,6) === "webkit") { //Microsoft and some Webkit browsers don't conform to the standard of capitalizing the first prefix character, so we adjust so that when we prefix the caps with a dash, it's correct (otherwise it'd be "ms-transform" instead of "-ms-transform" for IE9, for example) + property = "-" + property; + } + style.removeProperty(first2Chars === "--" ? property : property.replace(_capsExp, "-$1").toLowerCase()); + } else { //note: old versions of IE use "removeAttribute()" instead of "removeProperty()" + style.removeAttribute(property); + } + } + }, + _addNonTweeningPT = (plugin, target, property, beginning, end, onlySetAtEnd) => { + let pt = new PropTween(plugin._pt, target, property, 0, 1, onlySetAtEnd ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue); + plugin._pt = pt; + pt.b = beginning; + pt.e = end; + plugin._props.push(property); + return pt; + }, + _nonConvertibleUnits = {deg:1, rad:1, turn:1}, + _nonStandardLayouts = {grid:1, flex:1}, + //takes a single value like 20px and converts it to the unit specified, like "%", returning only the numeric amount. + _convertToUnit = (target, property, value, unit) => { + let curValue = parseFloat(value) || 0, + curUnit = (value + "").trim().substr((curValue + "").length) || "px", // some browsers leave extra whitespace at the beginning of CSS variables, hence the need to trim() + style = _tempDiv.style, + horizontal = _horizontalExp.test(property), + isRootSVG = target.tagName.toLowerCase() === "svg", + measureProperty = (isRootSVG ? "client" : "offset") + (horizontal ? "Width" : "Height"), + amount = 100, + toPixels = unit === "px", + toPercent = unit === "%", + px, parent, cache, isSVG; + if (unit === curUnit || !curValue || _nonConvertibleUnits[unit] || _nonConvertibleUnits[curUnit]) { + return curValue; + } + (curUnit !== "px" && !toPixels) && (curValue = _convertToUnit(target, property, value, "px")); + isSVG = target.getCTM && _isSVG(target); + if ((toPercent || curUnit === "%") && (_transformProps[property] || ~property.indexOf("adius"))) { + px = isSVG ? target.getBBox()[horizontal ? "width" : "height"] : target[measureProperty]; + return _round(toPercent ? curValue / px * amount : curValue / 100 * px); + } + style[horizontal ? "width" : "height"] = amount + (toPixels ? curUnit : unit); + parent = ((unit !== "rem" && ~property.indexOf("adius")) || (unit === "em" && target.appendChild && !isRootSVG)) ? target : target.parentNode; + if (isSVG) { + parent = (target.ownerSVGElement || {}).parentNode; + } + if (!parent || parent === _doc || !parent.appendChild) { + parent = _doc.body; + } + cache = parent._gsap; + if (cache && toPercent && cache.width && horizontal && cache.time === _ticker.time && !cache.uncache) { + return _round(curValue / cache.width * amount); + } else { + if (toPercent && (property === "height" || property === "width")) { // if we're dealing with width/height that's inside a container with padding and/or it's a flexbox/grid container, we must apply it to the target itself rather than the _tempDiv in order to ensure complete accuracy, factoring in the parent's padding. + let v = target.style[property]; + target.style[property] = amount + unit; + px = target[measureProperty]; + v ? (target.style[property] = v) : _removeProperty(target, property); + } else { + (toPercent || curUnit === "%") && !_nonStandardLayouts[_getComputedProperty(parent, "display")] && (style.position = _getComputedProperty(target, "position")); + (parent === target) && (style.position = "static"); // like for borderRadius, if it's a % we must have it relative to the target itself but that may not have position: relative or position: absolute in which case it'd go up the chain until it finds its offsetParent (bad). position: static protects against that. + parent.appendChild(_tempDiv); + px = _tempDiv[measureProperty]; + parent.removeChild(_tempDiv); + style.position = "absolute"; + } + if (horizontal && toPercent) { + cache = _getCache(parent); + cache.time = _ticker.time; + cache.width = parent[measureProperty]; + } + } + return _round(toPixels ? px * curValue / amount : px && curValue ? amount / px * curValue : 0); + }, + _get = (target, property, unit, uncache) => { + let value; + _pluginInitted || _initCore(); + if ((property in _propertyAliases) && property !== "transform") { + property = _propertyAliases[property]; + if (~property.indexOf(",")) { + property = property.split(",")[0]; + } + } + if (_transformProps[property] && property !== "transform") { + value = _parseTransform(target, uncache); + value = (property !== "transformOrigin") ? value[property] : value.svg ? value.origin : _firstTwoOnly(_getComputedProperty(target, _transformOriginProp)) + " " + value.zOrigin + "px"; + } else { + value = target.style[property]; + if (!value || value === "auto" || uncache || ~(value + "").indexOf("calc(")) { + value = (_specialProps[property] && _specialProps[property](target, property, unit)) || _getComputedProperty(target, property) || _getProperty(target, property) || (property === "opacity" ? 1 : 0); // note: some browsers, like Firefox, don't report borderRadius correctly! Instead, it only reports every corner like borderTopLeftRadius + } + } + return unit && !~(value + "").trim().indexOf(" ") ? _convertToUnit(target, property, value, unit) + unit : value; + + }, + _tweenComplexCSSString = function(target, prop, start, end) { // note: we call _tweenComplexCSSString.call(pluginInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus "this" would refer to the plugin. + if (!start || start === "none") { // some browsers like Safari actually PREFER the prefixed property and mis-report the unprefixed value like clipPath (BUG). In other words, even though clipPath exists in the style ("clipPath" in target.style) and it's set in the CSS properly (along with -webkit-clip-path), Safari reports clipPath as "none" whereas WebkitClipPath reports accurately like "ellipse(100% 0% at 50% 0%)", so in this case we must SWITCH to using the prefixed property instead. See https://gsap.com/forums/topic/18310-clippath-doesnt-work-on-ios/ + let p = _checkPropPrefix(prop, target, 1), + s = p && _getComputedProperty(target, p, 1); + if (s && s !== start) { + prop = p; + start = s; + } else if (prop === "borderColor") { + start = _getComputedProperty(target, "borderTopColor"); // Firefox bug: always reports "borderColor" as "", so we must fall back to borderTopColor. See https://gsap.com/forums/topic/24583-how-to-return-colors-that-i-had-after-reverse/ + } + } + let pt = new PropTween(this._pt, target.style, prop, 0, 1, _renderComplexString), + index = 0, + matchIndex = 0, + a, result, startValues, startNum, color, startValue, endValue, endNum, chunk, endUnit, startUnit, endValues; + pt.b = start; + pt.e = end; + start += ""; // ensure values are strings + end += ""; + if (end === "auto") { + startValue = target.style[prop]; + target.style[prop] = end; + end = _getComputedProperty(target, prop) || end; + startValue ? (target.style[prop] = startValue) : _removeProperty(target, prop); + } + a = [start, end]; + _colorStringFilter(a); // pass an array with the starting and ending values and let the filter do whatever it needs to the values. If colors are found, it returns true and then we must match where the color shows up order-wise because for things like boxShadow, sometimes the browser provides the computed values with the color FIRST, but the user provides it with the color LAST, so flip them if necessary. Same for drop-shadow(). + start = a[0]; + end = a[1]; + startValues = start.match(_numWithUnitExp) || []; + endValues = end.match(_numWithUnitExp) || []; + if (endValues.length) { + while ((result = _numWithUnitExp.exec(end))) { + endValue = result[0]; + chunk = end.substring(index, result.index); + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(" || chunk.substr(-5) === "hsla(") { + color = 1; + } + if (endValue !== (startValue = startValues[matchIndex++] || "")) { + startNum = parseFloat(startValue) || 0; + startUnit = startValue.substr((startNum + "").length); + (endValue.charAt(1) === "=") && (endValue = _parseRelative(startNum, endValue) + startUnit); + endNum = parseFloat(endValue); + endUnit = endValue.substr((endNum + "").length); + index = _numWithUnitExp.lastIndex - endUnit.length; + if (!endUnit) { //if something like "perspective:300" is passed in and we must add a unit to the end + endUnit = endUnit || _config.units[prop] || startUnit; + if (index === end.length) { + end += endUnit; + pt.e += endUnit; + } + } + if (startUnit !== endUnit) { + startNum = _convertToUnit(target, prop, startValue, endUnit) || 0; + } + // these nested PropTweens are handled in a special way - we'll never actually call a render or setter method on them. We'll just loop through them in the parent complex string PropTween's render method. + pt._pt = { + _next: pt._pt, + p: (chunk || (matchIndex === 1)) ? chunk : ",", //note: SVG spec allows omission of comma/space when a negative sign is wedged between two numbers, like 2.5-5.3 instead of 2.5,-5.3 but when tweening, the negative value may switch to positive, so we insert the comma just in case. + s: startNum, + c: endNum - startNum, + m: (color && color < 4) || prop === "zIndex" ? Math.round : 0 + }; + } + } + pt.c = (index < end.length) ? end.substring(index, end.length) : ""; //we use the "c" of the PropTween to store the final part of the string (after the last number) + } else { + pt.r = prop === "display" && end === "none" ? _renderNonTweeningValueOnlyAtEnd : _renderNonTweeningValue; + } + _relExp.test(end) && (pt.e = 0); //if the end string contains relative values or dynamic random(...) values, delete the end it so that on the final render we don't actually set it to the string with += or -= characters (forces it to use the calculated value). + this._pt = pt; //start the linked list with this new PropTween. Remember, we call _tweenComplexCSSString.call(pluginInstance...) to ensure that it's scoped properly. We may call it from within another plugin too, thus "this" would refer to the plugin. + return pt; + }, + _keywordToPercent = {top:"0%", bottom:"100%", left:"0%", right:"100%", center:"50%"}, + _convertKeywordsToPercentages = value => { + let split = value.split(" "), + x = split[0], + y = split[1] || "50%"; + if (x === "top" || x === "bottom" || y === "left" || y === "right") { //the user provided them in the wrong order, so flip them + value = x; + x = y; + y = value; + } + split[0] = _keywordToPercent[x] || x; + split[1] = _keywordToPercent[y] || y; + return split.join(" "); + }, + _renderClearProps = (ratio, data) => { + if (data.tween && data.tween._time === data.tween._dur) { + let target = data.t, + style = target.style, + props = data.u, + cache = target._gsap, + prop, clearTransforms, i; + if (props === "all" || props === true) { + style.cssText = ""; + clearTransforms = 1; + } else { + props = props.split(","); + i = props.length; + while (--i > -1) { + prop = props[i]; + if (_transformProps[prop]) { + clearTransforms = 1; + prop = (prop === "transformOrigin") ? _transformOriginProp : _transformProp; + } + _removeProperty(target, prop); + } + } + if (clearTransforms) { + _removeProperty(target, _transformProp); + if (cache) { + cache.svg && target.removeAttribute("transform"); + style.scale = style.rotate = style.translate = "none"; + _parseTransform(target, 1); // force all the cached values back to "normal"/identity, otherwise if there's another tween that's already set to render transforms on this element, it could display the wrong values. + cache.uncache = 1; + _removeIndependentTransforms(style); + } + } + } + }, + // note: specialProps should return 1 if (and only if) they have a non-zero priority. It indicates we need to sort the linked list. + _specialProps = { + clearProps(plugin, target, property, endValue, tween) { + if (tween.data !== "isFromStart") { + let pt = plugin._pt = new PropTween(plugin._pt, target, property, 0, 0, _renderClearProps); + pt.u = endValue; + pt.pr = -10; + pt.tween = tween; + plugin._props.push(property); + return 1; + } + } + /* className feature (about 0.4kb gzipped). + , className(plugin, target, property, endValue, tween) { + let _renderClassName = (ratio, data) => { + data.css.render(ratio, data.css); + if (!ratio || ratio === 1) { + let inline = data.rmv, + target = data.t, + p; + target.setAttribute("class", ratio ? data.e : data.b); + for (p in inline) { + _removeProperty(target, p); + } + } + }, + _getAllStyles = (target) => { + let styles = {}, + computed = getComputedStyle(target), + p; + for (p in computed) { + if (isNaN(p) && p !== "cssText" && p !== "length") { + styles[p] = computed[p]; + } + } + _setDefaults(styles, _parseTransform(target, 1)); + return styles; + }, + startClassList = target.getAttribute("class"), + style = target.style, + cssText = style.cssText, + cache = target._gsap, + classPT = cache.classPT, + inlineToRemoveAtEnd = {}, + data = {t:target, plugin:plugin, rmv:inlineToRemoveAtEnd, b:startClassList, e:(endValue.charAt(1) !== "=") ? endValue : startClassList.replace(new RegExp("(?:\\s|^)" + endValue.substr(2) + "(?![\\w-])"), "") + ((endValue.charAt(0) === "+") ? " " + endValue.substr(2) : "")}, + changingVars = {}, + startVars = _getAllStyles(target), + transformRelated = /(transform|perspective)/i, + endVars, p; + if (classPT) { + classPT.r(1, classPT.d); + _removeLinkedListItem(classPT.d.plugin, classPT, "_pt"); + } + target.setAttribute("class", data.e); + endVars = _getAllStyles(target, true); + target.setAttribute("class", startClassList); + for (p in endVars) { + if (endVars[p] !== startVars[p] && !transformRelated.test(p)) { + changingVars[p] = endVars[p]; + if (!style[p] && style[p] !== "0") { + inlineToRemoveAtEnd[p] = 1; + } + } + } + cache.classPT = plugin._pt = new PropTween(plugin._pt, target, "className", 0, 0, _renderClassName, data, 0, -11); + if (style.cssText !== cssText) { //only apply if things change. Otherwise, in cases like a background-image that's pulled dynamically, it could cause a refresh. See https://gsap.com/forums/topic/20368-possible-gsap-bug-switching-classnames-in-chrome/. + style.cssText = cssText; //we recorded cssText before we swapped classes and ran _getAllStyles() because in cases when a className tween is overwritten, we remove all the related tweening properties from that class change (otherwise class-specific stuff can't override properties we've directly set on the target's style object due to specificity). + } + _parseTransform(target, true); //to clear the caching of transforms + data.css = new gsap.plugins.css(); + data.css.init(target, changingVars, tween); + plugin._props.push(...data.css._props); + return 1; + } + */ + }, + + + + + + /* + * -------------------------------------------------------------------------------------- + * TRANSFORMS + * -------------------------------------------------------------------------------------- + */ + _identity2DMatrix = [1,0,0,1,0,0], + _rotationalProperties = {}, + _isNullTransform = value => (value === "matrix(1, 0, 0, 1, 0, 0)" || value === "none" || !value), + _getComputedTransformMatrixAsArray = target => { + let matrixString = _getComputedProperty(target, _transformProp); + return _isNullTransform(matrixString) ? _identity2DMatrix : matrixString.substr(7).match(_numExp).map(_round); + }, + _getMatrix = (target, force2D) => { + let cache = target._gsap || _getCache(target), + style = target.style, + matrix = _getComputedTransformMatrixAsArray(target), + parent, nextSibling, temp, addedToDOM; + if (cache.svg && target.getAttribute("transform")) { + temp = target.transform.baseVal.consolidate().matrix; //ensures that even complex values like "translate(50,60) rotate(135,0,0)" are parsed because it mashes it into a matrix. + matrix = [temp.a, temp.b, temp.c, temp.d, temp.e, temp.f]; + return (matrix.join(",") === "1,0,0,1,0,0") ? _identity2DMatrix : matrix; + } else if (matrix === _identity2DMatrix && !target.offsetParent && target !== _docElement && !cache.svg) { //note: if offsetParent is null, that means the element isn't in the normal document flow, like if it has display:none or one of its ancestors has display:none). Firefox returns null for getComputedStyle() if the element is in an iframe that has display:none. https://bugzilla.mozilla.org/show_bug.cgi?id=548397 + //browsers don't report transforms accurately unless the element is in the DOM and has a display value that's not "none". Firefox and Microsoft browsers have a partial bug where they'll report transforms even if display:none BUT not any percentage-based values like translate(-50%, 8px) will be reported as if it's translate(0, 8px). + temp = style.display; + style.display = "block"; + parent = target.parentNode; + if (!parent || (!target.offsetParent && !target.getBoundingClientRect().width)) { // note: in 3.3.0 we switched target.offsetParent to _doc.body.contains(target) to avoid [sometimes unnecessary] MutationObserver calls but that wasn't adequate because there are edge cases where nested position: fixed elements need to get reparented to accurately sense transforms. See https://github.com/greensock/GSAP/issues/388 and https://github.com/greensock/GSAP/issues/375. Note: position: fixed elements report a null offsetParent but they could also be invisible because they're in an ancestor with display: none, so we check getBoundingClientRect(). We only want to alter the DOM if we absolutely have to because it can cause iframe content to reload, like a Vimeo video. + addedToDOM = 1; //flag + nextSibling = target.nextElementSibling; + _docElement.appendChild(target); //we must add it to the DOM in order to get values properly + } + matrix = _getComputedTransformMatrixAsArray(target); + temp ? (style.display = temp) : _removeProperty(target, "display"); + if (addedToDOM) { + nextSibling ? parent.insertBefore(target, nextSibling) : parent ? parent.appendChild(target) : _docElement.removeChild(target); + } + } + return (force2D && matrix.length > 6) ? [matrix[0], matrix[1], matrix[4], matrix[5], matrix[12], matrix[13]] : matrix; + }, + _applySVGOrigin = (target, origin, originIsAbsolute, smooth, matrixArray, pluginToAddPropTweensTo) => { + let cache = target._gsap, + matrix = matrixArray || _getMatrix(target, true), + xOriginOld = cache.xOrigin || 0, + yOriginOld = cache.yOrigin || 0, + xOffsetOld = cache.xOffset || 0, + yOffsetOld = cache.yOffset || 0, + [a, b, c, d, tx, ty] = matrix, + originSplit = origin.split(" "), + xOrigin = parseFloat(originSplit[0]) || 0, + yOrigin = parseFloat(originSplit[1]) || 0, + bounds, determinant, x, y; + if (!originIsAbsolute) { + bounds = _getBBox(target); + xOrigin = bounds.x + (~originSplit[0].indexOf("%") ? xOrigin / 100 * bounds.width : xOrigin); + yOrigin = bounds.y + (~((originSplit[1] || originSplit[0]).indexOf("%")) ? yOrigin / 100 * bounds.height : yOrigin); + // if (!("xOrigin" in cache) && (xOrigin || yOrigin)) { // added in 3.12.3, reverted in 3.12.4; requires more exploration + // xOrigin -= bounds.x; + // yOrigin -= bounds.y; + // } + } else if (matrix !== _identity2DMatrix && (determinant = (a * d - b * c))) { //if it's zero (like if scaleX and scaleY are zero), skip it to avoid errors with dividing by zero. + x = xOrigin * (d / determinant) + yOrigin * (-c / determinant) + ((c * ty - d * tx) / determinant); + y = xOrigin * (-b / determinant) + yOrigin * (a / determinant) - ((a * ty - b * tx) / determinant); + xOrigin = x; + yOrigin = y; + // theory: we only had to do this for smoothing and it assumes that the previous one was not originIsAbsolute. + } + if (smooth || (smooth !== false && cache.smooth)) { + tx = xOrigin - xOriginOld; + ty = yOrigin - yOriginOld; + cache.xOffset = xOffsetOld + (tx * a + ty * c) - tx; + cache.yOffset = yOffsetOld + (tx * b + ty * d) - ty; + } else { + cache.xOffset = cache.yOffset = 0; + } + cache.xOrigin = xOrigin; + cache.yOrigin = yOrigin; + cache.smooth = !!smooth; + cache.origin = origin; + cache.originIsAbsolute = !!originIsAbsolute; + target.style[_transformOriginProp] = "0px 0px"; //otherwise, if someone sets an origin via CSS, it will likely interfere with the SVG transform attribute ones (because remember, we're baking the origin into the matrix() value). + if (pluginToAddPropTweensTo) { + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOrigin", xOriginOld, xOrigin); + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOrigin", yOriginOld, yOrigin); + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "xOffset", xOffsetOld, cache.xOffset); + _addNonTweeningPT(pluginToAddPropTweensTo, cache, "yOffset", yOffsetOld, cache.yOffset); + } + target.setAttribute("data-svg-origin", xOrigin + " " + yOrigin); + }, + _parseTransform = (target, uncache) => { + let cache = target._gsap || new GSCache(target); + if ("x" in cache && !uncache && !cache.uncache) { + return cache; + } + let style = target.style, + invertedScaleX = cache.scaleX < 0, + px = "px", + deg = "deg", + cs = getComputedStyle(target), + origin = _getComputedProperty(target, _transformOriginProp) || "0", + x, y, z, scaleX, scaleY, rotation, rotationX, rotationY, skewX, skewY, perspective, xOrigin, yOrigin, + matrix, angle, cos, sin, a, b, c, d, a12, a22, t1, t2, t3, a13, a23, a33, a42, a43, a32; + x = y = z = rotation = rotationX = rotationY = skewX = skewY = perspective = 0; + scaleX = scaleY = 1; + cache.svg = !!(target.getCTM && _isSVG(target)); + + if (cs.translate) { // accommodate independent transforms by combining them into normal ones. + if (cs.translate !== "none" || cs.scale !== "none" || cs.rotate !== "none") { + style[_transformProp] = (cs.translate !== "none" ? "translate3d(" + (cs.translate + " 0 0").split(" ").slice(0, 3).join(", ") + ") " : "") + (cs.rotate !== "none" ? "rotate(" + cs.rotate + ") " : "") + (cs.scale !== "none" ? "scale(" + cs.scale.split(" ").join(",") + ") " : "") + (cs[_transformProp] !== "none" ? cs[_transformProp] : ""); + } + style.scale = style.rotate = style.translate = "none"; + } + + matrix = _getMatrix(target, cache.svg); + if (cache.svg) { + if (cache.uncache) { // if cache.uncache is true (and maybe if origin is 0,0), we need to set element.style.transformOrigin = (cache.xOrigin - bbox.x) + "px " + (cache.yOrigin - bbox.y) + "px". Previously we let the data-svg-origin stay instead, but when introducing revert(), it complicated things. + t2 = target.getBBox(); + origin = (cache.xOrigin - t2.x) + "px " + (cache.yOrigin - t2.y) + "px"; + t1 = ""; + } else { + t1 = !uncache && target.getAttribute("data-svg-origin"); // Remember, to work around browser inconsistencies we always force SVG elements' transformOrigin to 0,0 and offset the translation accordingly. + } + _applySVGOrigin(target, t1 || origin, !!t1 || cache.originIsAbsolute, cache.smooth !== false, matrix); + } + xOrigin = cache.xOrigin || 0; + yOrigin = cache.yOrigin || 0; + if (matrix !== _identity2DMatrix) { + a = matrix[0]; //a11 + b = matrix[1]; //a21 + c = matrix[2]; //a31 + d = matrix[3]; //a41 + x = a12 = matrix[4]; + y = a22 = matrix[5]; + + //2D matrix + if (matrix.length === 6) { + scaleX = Math.sqrt(a * a + b * b); + scaleY = Math.sqrt(d * d + c * c); + rotation = (a || b) ? _atan2(b, a) * _RAD2DEG : 0; //note: if scaleX is 0, we cannot accurately measure rotation. Same for skewX with a scaleY of 0. Therefore, we default to the previously recorded value (or zero if that doesn't exist). + skewX = (c || d) ? _atan2(c, d) * _RAD2DEG + rotation : 0; + skewX && (scaleY *= Math.abs(Math.cos(skewX * _DEG2RAD))); + if (cache.svg) { + x -= xOrigin - (xOrigin * a + yOrigin * c); + y -= yOrigin - (xOrigin * b + yOrigin * d); + } + + //3D matrix + } else { + a32 = matrix[6]; + a42 = matrix[7]; + a13 = matrix[8]; + a23 = matrix[9]; + a33 = matrix[10]; + a43 = matrix[11]; + x = matrix[12]; + y = matrix[13]; + z = matrix[14]; + + angle = _atan2(a32, a33); + rotationX = angle * _RAD2DEG; + //rotationX + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a12*cos+a13*sin; + t2 = a22*cos+a23*sin; + t3 = a32*cos+a33*sin; + a13 = a12*-sin+a13*cos; + a23 = a22*-sin+a23*cos; + a33 = a32*-sin+a33*cos; + a43 = a42*-sin+a43*cos; + a12 = t1; + a22 = t2; + a32 = t3; + } + //rotationY + angle = _atan2(-c, a33); + rotationY = angle * _RAD2DEG; + if (angle) { + cos = Math.cos(-angle); + sin = Math.sin(-angle); + t1 = a*cos-a13*sin; + t2 = b*cos-a23*sin; + t3 = c*cos-a33*sin; + a43 = d*sin+a43*cos; + a = t1; + b = t2; + c = t3; + } + //rotationZ + angle = _atan2(b, a); + rotation = angle * _RAD2DEG; + if (angle) { + cos = Math.cos(angle); + sin = Math.sin(angle); + t1 = a*cos+b*sin; + t2 = a12*cos+a22*sin; + b = b*cos-a*sin; + a22 = a22*cos-a12*sin; + a = t1; + a12 = t2; + } + + if (rotationX && Math.abs(rotationX) + Math.abs(rotation) > 359.9) { //when rotationY is set, it will often be parsed as 180 degrees different than it should be, and rotationX and rotation both being 180 (it looks the same), so we adjust for that here. + rotationX = rotation = 0; + rotationY = 180 - rotationY; + } + scaleX = _round(Math.sqrt(a * a + b * b + c * c)); + scaleY = _round(Math.sqrt(a22 * a22 + a32 * a32)); + angle = _atan2(a12, a22); + skewX = (Math.abs(angle) > 0.0002) ? angle * _RAD2DEG : 0; + perspective = a43 ? 1 / ((a43 < 0) ? -a43 : a43) : 0; + } + + if (cache.svg) { //sense if there are CSS transforms applied on an SVG element in which case we must overwrite them when rendering. The transform attribute is more reliable cross-browser, but we can't just remove the CSS ones because they may be applied in a CSS rule somewhere (not just inline). + t1 = target.getAttribute("transform"); + cache.forceCSS = target.setAttribute("transform", "") || (!_isNullTransform(_getComputedProperty(target, _transformProp))); + t1 && target.setAttribute("transform", t1); + } + } + + if (Math.abs(skewX) > 90 && Math.abs(skewX) < 270) { + if (invertedScaleX) { + scaleX *= -1; + skewX += (rotation <= 0) ? 180 : -180; + rotation += (rotation <= 0) ? 180 : -180; + } else { + scaleY *= -1; + skewX += (skewX <= 0) ? 180 : -180; + } + } + uncache = uncache || cache.uncache; + cache.x = x - ((cache.xPercent = x && ((!uncache && cache.xPercent) || (Math.round(target.offsetWidth / 2) === Math.round(-x) ? -50 : 0))) ? target.offsetWidth * cache.xPercent / 100 : 0) + px; + cache.y = y - ((cache.yPercent = y && ((!uncache && cache.yPercent) || (Math.round(target.offsetHeight / 2) === Math.round(-y) ? -50 : 0))) ? target.offsetHeight * cache.yPercent / 100 : 0) + px; + cache.z = z + px; + cache.scaleX = _round(scaleX); + cache.scaleY = _round(scaleY); + cache.rotation = _round(rotation) + deg; + cache.rotationX = _round(rotationX) + deg; + cache.rotationY = _round(rotationY) + deg; + cache.skewX = skewX + deg; + cache.skewY = skewY + deg; + cache.transformPerspective = perspective + px; + if ((cache.zOrigin = parseFloat(origin.split(" ")[2]) || (!uncache && cache.zOrigin) || 0)) { + style[_transformOriginProp] = _firstTwoOnly(origin); + } + cache.xOffset = cache.yOffset = 0; + cache.force3D = _config.force3D; + cache.renderTransform = cache.svg ? _renderSVGTransforms : _supports3D ? _renderCSSTransforms : _renderNon3DTransforms; + cache.uncache = 0; + return cache; + }, + _firstTwoOnly = value => (value = value.split(" "))[0] + " " + value[1], //for handling transformOrigin values, stripping out the 3rd dimension + _addPxTranslate = (target, start, value) => { + let unit = getUnit(start); + return _round(parseFloat(start) + parseFloat(_convertToUnit(target, "x", value + "px", unit))) + unit; + }, + _renderNon3DTransforms = (ratio, cache) => { + cache.z = "0px"; + cache.rotationY = cache.rotationX = "0deg"; + cache.force3D = 0; + _renderCSSTransforms(ratio, cache); + }, + _zeroDeg = "0deg", + _zeroPx = "0px", + _endParenthesis = ") ", + _renderCSSTransforms = function(ratio, cache) { + let {xPercent, yPercent, x, y, z, rotation, rotationY, rotationX, skewX, skewY, scaleX, scaleY, transformPerspective, force3D, target, zOrigin} = cache || this, + transforms = "", + use3D = (force3D === "auto" && ratio && ratio !== 1) || force3D === true; + + // Safari has a bug that causes it not to render 3D transform-origin values properly, so we force the z origin to 0, record it in the cache, and then do the math here to offset the translate values accordingly (basically do the 3D transform-origin part manually) + if (zOrigin && (rotationX !== _zeroDeg || rotationY !== _zeroDeg)) { + let angle = parseFloat(rotationY) * _DEG2RAD, + a13 = Math.sin(angle), + a33 = Math.cos(angle), + cos; + angle = parseFloat(rotationX) * _DEG2RAD; + cos = Math.cos(angle); + x = _addPxTranslate(target, x, a13 * cos * -zOrigin); + y = _addPxTranslate(target, y, -Math.sin(angle) * -zOrigin); + z = _addPxTranslate(target, z, a33 * cos * -zOrigin + zOrigin); + } + + if (transformPerspective !== _zeroPx) { + transforms += "perspective(" + transformPerspective + _endParenthesis; + } + if (xPercent || yPercent) { + transforms += "translate(" + xPercent + "%, " + yPercent + "%) "; + } + if (use3D || x !== _zeroPx || y !== _zeroPx || z !== _zeroPx) { + transforms += (z !== _zeroPx || use3D) ? "translate3d(" + x + ", " + y + ", " + z + ") " : "translate(" + x + ", " + y + _endParenthesis; + } + if (rotation !== _zeroDeg) { + transforms += "rotate(" + rotation + _endParenthesis; + } + if (rotationY !== _zeroDeg) { + transforms += "rotateY(" + rotationY + _endParenthesis; + } + if (rotationX !== _zeroDeg) { + transforms += "rotateX(" + rotationX + _endParenthesis; + } + if (skewX !== _zeroDeg || skewY !== _zeroDeg) { + transforms += "skew(" + skewX + ", " + skewY + _endParenthesis; + } + if (scaleX !== 1 || scaleY !== 1) { + transforms += "scale(" + scaleX + ", " + scaleY + _endParenthesis; + } + target.style[_transformProp] = transforms || "translate(0, 0)"; + }, + _renderSVGTransforms = function(ratio, cache) { + let {xPercent, yPercent, x, y, rotation, skewX, skewY, scaleX, scaleY, target, xOrigin, yOrigin, xOffset, yOffset, forceCSS} = cache || this, + tx = parseFloat(x), + ty = parseFloat(y), + a11, a21, a12, a22, temp; + rotation = parseFloat(rotation); + skewX = parseFloat(skewX); + skewY = parseFloat(skewY); + if (skewY) { //for performance reasons, we combine all skewing into the skewX and rotation values. Remember, a skewY of 10 degrees looks the same as a rotation of 10 degrees plus a skewX of 10 degrees. + skewY = parseFloat(skewY); + skewX += skewY; + rotation += skewY; + } + if (rotation || skewX) { + rotation *= _DEG2RAD; + skewX *= _DEG2RAD; + a11 = Math.cos(rotation) * scaleX; + a21 = Math.sin(rotation) * scaleX; + a12 = Math.sin(rotation - skewX) * -scaleY; + a22 = Math.cos(rotation - skewX) * scaleY; + if (skewX) { + skewY *= _DEG2RAD; + temp = Math.tan(skewX - skewY); + temp = Math.sqrt(1 + temp * temp); + a12 *= temp; + a22 *= temp; + if (skewY) { + temp = Math.tan(skewY); + temp = Math.sqrt(1 + temp * temp); + a11 *= temp; + a21 *= temp; + } + } + a11 = _round(a11); + a21 = _round(a21); + a12 = _round(a12); + a22 = _round(a22); + } else { + a11 = scaleX; + a22 = scaleY; + a21 = a12 = 0; + } + if ((tx && !~(x + "").indexOf("px")) || (ty && !~(y + "").indexOf("px"))) { + tx = _convertToUnit(target, "x", x, "px"); + ty = _convertToUnit(target, "y", y, "px"); + } + if (xOrigin || yOrigin || xOffset || yOffset) { + tx = _round(tx + xOrigin - (xOrigin * a11 + yOrigin * a12) + xOffset); + ty = _round(ty + yOrigin - (xOrigin * a21 + yOrigin * a22) + yOffset); + } + if (xPercent || yPercent) { + //The SVG spec doesn't support percentage-based translation in the "transform" attribute, so we merge it into the translation to simulate it. + temp = target.getBBox(); + tx = _round(tx + xPercent / 100 * temp.width); + ty = _round(ty + yPercent / 100 * temp.height); + } + temp = "matrix(" + a11 + "," + a21 + "," + a12 + "," + a22 + "," + tx + "," + ty + ")"; + target.setAttribute("transform", temp); + forceCSS && (target.style[_transformProp] = temp); //some browsers prioritize CSS transforms over the transform attribute. When we sense that the user has CSS transforms applied, we must overwrite them this way (otherwise some browser simply won't render the transform attribute changes!) + }, + _addRotationalPropTween = function(plugin, target, property, startNum, endValue) { + let cap = 360, + isString = _isString(endValue), + endNum = parseFloat(endValue) * ((isString && ~endValue.indexOf("rad")) ? _RAD2DEG : 1), + change = endNum - startNum, + finalValue = (startNum + change) + "deg", + direction, pt; + if (isString) { + direction = endValue.split("_")[1]; + if (direction === "short") { + change %= cap; + if (change !== change % (cap / 2)) { + change += (change < 0) ? cap : -cap; + } + } + if (direction === "cw" && change < 0) { + change = ((change + cap * _bigNum) % cap) - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = ((change - cap * _bigNum) % cap) - ~~(change / cap) * cap; + } + } + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + pt.u = "deg"; + plugin._props.push(property); + return pt; + }, + _assign = (target, source) => { // Internet Explorer doesn't have Object.assign(), so we recreate it here. + for (let p in source) { + target[p] = source[p]; + } + return target; + }, + _addRawTransformPTs = (plugin, transforms, target) => { //for handling cases where someone passes in a whole transform string, like transform: "scale(2, 3) rotate(20deg) translateY(30em)" + let startCache = _assign({}, target._gsap), + exclude = "perspective,force3D,transformOrigin,svgOrigin", + style = target.style, + endCache, p, startValue, endValue, startNum, endNum, startUnit, endUnit; + if (startCache.svg) { + startValue = target.getAttribute("transform"); + target.setAttribute("transform", ""); + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + _removeProperty(target, _transformProp); + target.setAttribute("transform", startValue); + } else { + startValue = getComputedStyle(target)[_transformProp]; + style[_transformProp] = transforms; + endCache = _parseTransform(target, 1); + style[_transformProp] = startValue; + } + for (p in _transformProps) { + startValue = startCache[p]; + endValue = endCache[p]; + if (startValue !== endValue && exclude.indexOf(p) < 0) { //tweening to no perspective gives very unintuitive results - just keep the same perspective in that case. + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + startNum = (startUnit !== endUnit) ? _convertToUnit(target, p, startValue, endUnit) : parseFloat(startValue); + endNum = parseFloat(endValue); + plugin._pt = new PropTween(plugin._pt, endCache, p, startNum, endNum - startNum, _renderCSSProp); + plugin._pt.u = endUnit || 0; + plugin._props.push(p); + } + } + _assign(endCache, startCache); + }; + +// handle splitting apart padding, margin, borderWidth, and borderRadius into their 4 components. Firefox, for example, won't report borderRadius correctly - it will only do borderTopLeftRadius and the other corners. We also want to handle paddingTop, marginLeft, borderRightWidth, etc. +_forEachName("padding,margin,Width,Radius", (name, index) => { + let t = "Top", + r = "Right", + b = "Bottom", + l = "Left", + props = (index < 3 ? [t,r,b,l] : [t+l, t+r, b+r, b+l]).map(side => index < 2 ? name + side : "border" + side + name); + _specialProps[(index > 1 ? "border" + name : name)] = function(plugin, target, property, endValue, tween) { + let a, vars; + if (arguments.length < 4) { // getter, passed target, property, and unit (from _get()) + a = props.map(prop => _get(plugin, prop, property)); + vars = a.join(" "); + return vars.split(a[0]).length === 5 ? a[0] : vars; + } + a = (endValue + "").split(" "); + vars = {}; + props.forEach((prop, i) => vars[prop] = a[i] = a[i] || a[(((i - 1) / 2) | 0)]); + plugin.init(target, vars, tween); + } +}); + + +export const CSSPlugin = { + name: "css", + register: _initCore, + targetTest(target) { + return target.style && target.nodeType; + }, + init(target, vars, tween, index, targets) { + let props = this._props, + style = target.style, + startAt = tween.vars.startAt, + startValue, endValue, endNum, startNum, type, specialProp, p, startUnit, endUnit, relative, isTransformRelated, transformPropTween, cache, smooth, hasPriority, inlineProps; + _pluginInitted || _initCore(); + // we may call init() multiple times on the same plugin instance, like when adding special properties, so make sure we don't overwrite the revert data or inlineProps + this.styles = this.styles || _getStyleSaver(target); + inlineProps = this.styles.props; + this.tween = tween; + for (p in vars) { + if (p === "autoRound") { + continue; + } + endValue = vars[p]; + if (_plugins[p] && _checkPlugin(p, vars, tween, index, target, targets)) { // plugins + continue; + } + type = typeof(endValue); + specialProp = _specialProps[p]; + if (type === "function") { + endValue = endValue.call(tween, index, target, targets); + type = typeof(endValue); + } + if (type === "string" && ~endValue.indexOf("random(")) { + endValue = _replaceRandom(endValue); + } + if (specialProp) { + specialProp(this, target, p, endValue, tween) && (hasPriority = 1); + } else if (p.substr(0,2) === "--") { //CSS variable + startValue = (getComputedStyle(target).getPropertyValue(p) + "").trim(); + endValue += ""; + _colorExp.lastIndex = 0; + if (!_colorExp.test(startValue)) { // colors don't have units + startUnit = getUnit(startValue); + endUnit = getUnit(endValue); + } + endUnit ? startUnit !== endUnit && (startValue = _convertToUnit(target, p, startValue, endUnit) + endUnit) : startUnit && (endValue += startUnit); + this.add(style, "setProperty", startValue, endValue, index, targets, 0, 0, p); + props.push(p); + inlineProps.push(p, 0, style[p]); + } else if (type !== "undefined") { + if (startAt && p in startAt) { // in case someone hard-codes a complex value as the start, like top: "calc(2vh / 2)". Without this, it'd use the computed value (always in px) + startValue = typeof(startAt[p]) === "function" ? startAt[p].call(tween, index, target, targets) : startAt[p]; + _isString(startValue) && ~startValue.indexOf("random(") && (startValue = _replaceRandom(startValue)); + getUnit(startValue + "") || startValue === "auto" || (startValue += _config.units[p] || getUnit(_get(target, p)) || ""); // for cases when someone passes in a unitless value like {x: 100}; if we try setting translate(100, 0px) it won't work. + (startValue + "").charAt(1) === "=" && (startValue = _get(target, p)); // can't work with relative values + } else { + startValue = _get(target, p); + } + startNum = parseFloat(startValue); + relative = (type === "string" && endValue.charAt(1) === "=") && endValue.substr(0, 2); + relative && (endValue = endValue.substr(2)); + endNum = parseFloat(endValue); + if (p in _propertyAliases) { + if (p === "autoAlpha") { //special case where we control the visibility along with opacity. We still allow the opacity value to pass through and get tweened. + if (startNum === 1 && _get(target, "visibility") === "hidden" && endNum) { //if visibility is initially set to "hidden", we should interpret that as intent to make opacity 0 (a convenience) + startNum = 0; + } + inlineProps.push("visibility", 0, style.visibility); + _addNonTweeningPT(this, style, "visibility", startNum ? "inherit" : "hidden", endNum ? "inherit" : "hidden", !endNum); + } + if (p !== "scale" && p !== "transform") { + p = _propertyAliases[p]; + ~p.indexOf(",") && (p = p.split(",")[0]); + } + } + + isTransformRelated = (p in _transformProps); + + //--- TRANSFORM-RELATED --- + if (isTransformRelated) { + this.styles.save(p); + if (!transformPropTween) { + cache = target._gsap; + (cache.renderTransform && !vars.parseTransform) || _parseTransform(target, vars.parseTransform); // if, for example, gsap.set(... {transform:"translateX(50vw)"}), the _get() call doesn't parse the transform, thus cache.renderTransform won't be set yet so force the parsing of the transform here. + smooth = (vars.smoothOrigin !== false && cache.smooth); + transformPropTween = this._pt = new PropTween(this._pt, style, _transformProp, 0, 1, cache.renderTransform, cache, 0, -1); //the first time through, create the rendering PropTween so that it runs LAST (in the linked list, we keep adding to the beginning) + transformPropTween.dep = 1; //flag it as dependent so that if things get killed/overwritten and this is the only PropTween left, we can safely kill the whole tween. + } + if (p === "scale") { + this._pt = new PropTween(this._pt, cache, "scaleY", cache.scaleY, ((relative ? _parseRelative(cache.scaleY, relative + endNum) : endNum) - cache.scaleY) || 0, _renderCSSProp); + this._pt.u = 0; + props.push("scaleY", p); + p += "X"; + } else if (p === "transformOrigin") { + inlineProps.push(_transformOriginProp, 0, style[_transformOriginProp]); + endValue = _convertKeywordsToPercentages(endValue); //in case something like "left top" or "bottom right" is passed in. Convert to percentages. + if (cache.svg) { + _applySVGOrigin(target, endValue, 0, smooth, 0, this); + } else { + endUnit = parseFloat(endValue.split(" ")[2]) || 0; //handle the zOrigin separately! + endUnit !== cache.zOrigin && _addNonTweeningPT(this, cache, "zOrigin", cache.zOrigin, endUnit); + _addNonTweeningPT(this, style, p, _firstTwoOnly(startValue), _firstTwoOnly(endValue)); + } + continue; + } else if (p === "svgOrigin") { + _applySVGOrigin(target, endValue, 1, smooth, 0, this); + continue; + } else if (p in _rotationalProperties) { + _addRotationalPropTween(this, cache, p, startNum, relative ? _parseRelative(startNum, relative + endValue) : endValue); + continue; + + } else if (p === "smoothOrigin") { + _addNonTweeningPT(this, cache, "smooth", cache.smooth, endValue); + continue; + } else if (p === "force3D") { + cache[p] = endValue; + continue; + } else if (p === "transform") { + _addRawTransformPTs(this, endValue, target); + continue; + } + } else if (!(p in style)) { + p = _checkPropPrefix(p) || p; + } + + if (isTransformRelated || ((endNum || endNum === 0) && (startNum || startNum === 0) && !_complexExp.test(endValue) && (p in style))) { + startUnit = (startValue + "").substr((startNum + "").length); + endNum || (endNum = 0); // protect against NaN + endUnit = getUnit(endValue) || ((p in _config.units) ? _config.units[p] : startUnit); + startUnit !== endUnit && (startNum = _convertToUnit(target, p, startValue, endUnit)); + this._pt = new PropTween(this._pt, isTransformRelated ? cache : style, p, startNum, (relative ? _parseRelative(startNum, relative + endNum) : endNum) - startNum, (!isTransformRelated && (endUnit === "px" || p === "zIndex") && vars.autoRound !== false) ? _renderRoundedCSSProp : _renderCSSProp); + this._pt.u = endUnit || 0; + if (startUnit !== endUnit && endUnit !== "%") { //when the tween goes all the way back to the beginning, we need to revert it to the OLD/ORIGINAL value (with those units). We record that as a "b" (beginning) property and point to a render method that handles that. (performance optimization) + this._pt.b = startValue; + this._pt.r = _renderCSSPropWithBeginning; + } + } else if (!(p in style)) { + if (p in target) { //maybe it's not a style - it could be a property added directly to an element in which case we'll try to animate that. + this.add(target, p, startValue || target[p], relative ? relative + endValue : endValue, index, targets); + } else if (p !== "parseTransform") { + _missingPlugin(p, endValue); + continue; + } + } else { + _tweenComplexCSSString.call(this, target, p, startValue, relative ? relative + endValue : endValue); + } + isTransformRelated || (p in style ? inlineProps.push(p, 0, style[p]) : typeof(target[p]) === "function" ? inlineProps.push(p, 2, target[p]()) : inlineProps.push(p, 1, startValue || target[p])); + props.push(p); + } + } + hasPriority && _sortPropTweensByPriority(this); + + }, + render(ratio, data) { + if (data.tween._time || !_reverting()) { + let pt = data._pt; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + } else { + data.styles.revert(); + } + }, + get: _get, + aliases: _propertyAliases, + getSetter(target, property, plugin) { //returns a setter function that accepts target, property, value and applies it accordingly. Remember, properties like "x" aren't as simple as target.style.property = value because they've got to be applied to a proxy object and then merged into a transform string in a renderer. + let p = _propertyAliases[property]; + (p && p.indexOf(",") < 0) && (property = p); + return (property in _transformProps && property !== _transformOriginProp && (target._gsap.x || _get(target, "x"))) ? (plugin && _recentSetterPlugin === plugin ? (property === "scale" ? _setterScale : _setterTransform) : (_recentSetterPlugin = plugin || {}) && (property === "scale" ? _setterScaleWithRender : _setterTransformWithRender)) : target.style && !_isUndefined(target.style[property]) ? _setterCSSStyle : ~property.indexOf("-") ? _setterCSSProp : _getSetter(target, property); + }, + core: { _removeProperty, _getMatrix } + +}; + +gsap.utils.checkPrefix = _checkPropPrefix; +gsap.core.getStyleSaver = _getStyleSaver; +(function(positionAndScale, rotation, others, aliases) { + let all = _forEachName(positionAndScale + "," + rotation + "," + others, name => {_transformProps[name] = 1}); + _forEachName(rotation, name => {_config.units[name] = "deg"; _rotationalProperties[name] = 1}); + _propertyAliases[all[13]] = positionAndScale + "," + rotation; + _forEachName(aliases, name => { + let split = name.split(":"); + _propertyAliases[split[1]] = all[split[0]]; + }); +})("x,y,z,scale,scaleX,scaleY,xPercent,yPercent", "rotation,rotationX,rotationY,skewX,skewY", "transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective", "0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY"); +_forEachName("x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective", name => {_config.units[name] = "px"}); + +gsap.registerPlugin(CSSPlugin); + +export { CSSPlugin as default, _getBBox, _createElement, _checkPropPrefix as checkPrefix }; \ No newline at end of file diff --git a/node_modules/gsap/src/CSSRulePlugin.js b/node_modules/gsap/src/CSSRulePlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..719dc3e0075ce6b545a55a1100fcdb423ca8e23c --- /dev/null +++ b/node_modules/gsap/src/CSSRulePlugin.js @@ -0,0 +1,107 @@ +/*! + * CSSRulePlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let gsap, _coreInitted, _win, _doc, CSSPlugin, + _windowExists = () => typeof(window) !== "undefined", + _getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _checkRegister = () => { + if (!_coreInitted) { + _initCore(); + if (!CSSPlugin) { + console.warn("Please gsap.registerPlugin(CSSPlugin, CSSRulePlugin)"); + } + } + return _coreInitted; + }, + _initCore = core => { + gsap = core || _getGSAP(); + if (_windowExists()) { + _win = window; + _doc = document; + } + if (gsap) { + CSSPlugin = gsap.plugins.css; + if (CSSPlugin) { + _coreInitted = 1; + } + } + }; + + +export const CSSRulePlugin = { + version: "3.12.7", + name: "cssRule", + init(target, value, tween, index, targets) { + if (!_checkRegister() || typeof(target.cssText) === "undefined") { + return false; + } + let div = target._gsProxy = target._gsProxy || _doc.createElement("div"); + this.ss = target; + this.style = div.style; + div.style.cssText = target.cssText; + CSSPlugin.prototype.init.call(this, div, value, tween, index, targets); //we just offload all the work to the regular CSSPlugin and then copy the cssText back over to the rule in the render() method. This allows us to have all of the updates to CSSPlugin automatically flow through to CSSRulePlugin instead of having to maintain both + }, + render(ratio, data) { + let pt = data._pt, + style = data.style, + ss = data.ss, + i; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + i = style.length; + while (--i > -1) { + ss[style[i]] = style[style[i]]; + } + }, + getRule(selector) { + _checkRegister(); + let ruleProp = _doc.all ? "rules" : "cssRules", + styleSheets = _doc.styleSheets, + i = styleSheets.length, + pseudo = (selector.charAt(0) === ":"), + j, curSS, cs, a; + selector = (pseudo ? "" : ",") + selector.split("::").join(":").toLowerCase() + ","; //note: old versions of IE report tag name selectors as upper case, so we just change everything to lowercase. + if (pseudo) { + a = []; + } + while (i--) { + //Firefox may throw insecure operation errors when css is loaded from other domains, so try/catch. + try { + curSS = styleSheets[i][ruleProp]; + if (!curSS) { + continue; + } + j = curSS.length; + } catch (e) { + console.warn(e); + continue; + } + while (--j > -1) { + cs = curSS[j]; + if (cs.selectorText && ("," + cs.selectorText.split("::").join(":").toLowerCase() + ",").indexOf(selector) !== -1) { //note: IE adds an extra ":" to pseudo selectors, so .myClass:after becomes .myClass::after, so we need to strip the extra one out. + if (pseudo) { + a.push(cs.style); + } else { + return cs.style; + } + } + } + } + return a; + }, + register: _initCore +}; + +_getGSAP() && gsap.registerPlugin(CSSRulePlugin); + +export { CSSRulePlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/CustomEase.js b/node_modules/gsap/src/CustomEase.js new file mode 100644 index 0000000000000000000000000000000000000000..0dbbc3e4272f788cbd2a8c85a42720127c723d63 --- /dev/null +++ b/node_modules/gsap/src/CustomEase.js @@ -0,0 +1,278 @@ +/*! + * CustomEase 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +import { stringToRawPath, rawPathToString, transformRawPath } from "./utils/paths.js"; + +let gsap, _coreInitted, + _getGSAP = () => gsap || (typeof(window) !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _initCore = () => { + gsap = _getGSAP(); + if (gsap) { + gsap.registerEase("_CE", CustomEase.create); + _coreInitted = 1; + } else { + console.warn("Please gsap.registerPlugin(CustomEase)"); + } + }, + _bigNum = 1e20, + _round = value => ~~(value * 1000 + (value < 0 ? -.5 : .5)) / 1000, + _bonusValidated = 1, //<name>CustomEase</name> + _numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/gi, //finds any numbers, including ones that start with += or -=, negative numbers, and ones in scientific notation like 1e-8. + _needsParsingExp = /[cLlsSaAhHvVtTqQ]/g, + _findMinimum = values => { + let l = values.length, + min = _bigNum, + i; + for (i = 1; i < l; i += 6) { + +values[i] < min && (min = +values[i]); + } + return min; + }, + //takes all the points and translates/scales them so that the x starts at 0 and ends at 1. + _normalize = (values, height, originY) => { + if (!originY && originY !== 0) { + originY = Math.max(+values[values.length-1], +values[1]); + } + let tx = +values[0] * -1, + ty = -originY, + l = values.length, + sx = 1 / (+values[l - 2] + tx), + sy = -height || ((Math.abs(+values[l - 1] - +values[1]) < 0.01 * (+values[l - 2] - +values[0])) ? _findMinimum(values) + ty : +values[l - 1] + ty), + i; + if (sy) { //typically y ends at 1 (so that the end values are reached) + sy = 1 / sy; + } else { //in case the ease returns to its beginning value, scale everything proportionally + sy = -sx; + } + for (i = 0; i < l; i += 2) { + values[i] = (+values[i] + tx) * sx; + values[i + 1] = (+values[i + 1] + ty) * sy; + } + }, + //note that this function returns point objects like {x, y} rather than working with segments which are arrays with alternating x, y values as in the similar function in paths.js + _bezierToPoints = function (x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) { + let x12 = (x1 + x2) / 2, + y12 = (y1 + y2) / 2, + x23 = (x2 + x3) / 2, + y23 = (y2 + y3) / 2, + x34 = (x3 + x4) / 2, + y34 = (y3 + y4) / 2, + x123 = (x12 + x23) / 2, + y123 = (y12 + y23) / 2, + x234 = (x23 + x34) / 2, + y234 = (y23 + y34) / 2, + x1234 = (x123 + x234) / 2, + y1234 = (y123 + y234) / 2, + dx = x4 - x1, + dy = y4 - y1, + d2 = Math.abs((x2 - x4) * dy - (y2 - y4) * dx), + d3 = Math.abs((x3 - x4) * dy - (y3 - y4) * dx), + length; + if (!points) { + points = [{x: x1, y: y1}, {x: x4, y: y4}]; + index = 1; + } + points.splice(index || points.length - 1, 0, {x: x1234, y: y1234}); + if ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) { + length = points.length; + _bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index); + _bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 1 + (points.length - length)); + } + return points; + }; + +export class CustomEase { + + constructor(id, data, config) { + _coreInitted || _initCore(); + this.id = id; + _bonusValidated && this.setData(data, config); + } + + setData(data, config) { + config = config || {}; + data = data || "0,0,1,1"; + let values = data.match(_numExp), + closest = 1, + points = [], + lookup = [], + precision = config.precision || 1, + fast = (precision <= 1), + l, a1, a2, i, inc, j, point, prevPoint, p; + this.data = data; + if (_needsParsingExp.test(data) || (~data.indexOf("M") && data.indexOf("C") < 0)) { + values = stringToRawPath(data)[0]; + } + l = values.length; + if (l === 4) { + values.unshift(0, 0); + values.push(1, 1); + l = 8; + } else if ((l - 2) % 6) { + throw "Invalid CustomEase"; + } + if (+values[0] !== 0 || +values[l - 2] !== 1) { + _normalize(values, config.height, config.originY); + } + this.segment = values; + for (i = 2; i < l; i += 6) { + a1 = {x: +values[i - 2], y: +values[i - 1]}; + a2 = {x: +values[i + 4], y: +values[i + 5]}; + points.push(a1, a2); + _bezierToPoints(a1.x, a1.y, +values[i], +values[i + 1], +values[i + 2], +values[i + 3], a2.x, a2.y, 1 / (precision * 200000), points, points.length - 1); + } + l = points.length; + for (i = 0; i < l; i++) { + point = points[i]; + prevPoint = points[i - 1] || point; + if ((point.x > prevPoint.x || (prevPoint.y !== point.y && prevPoint.x === point.x) || point === prevPoint) && point.x <= 1) { //if a point goes BACKWARD in time or is a duplicate, just drop it. Also it shouldn't go past 1 on the x axis, as could happen in a string like "M0,0 C0,0 0.12,0.68 0.18,0.788 0.195,0.845 0.308,1 0.32,1 0.403,1.005 0.398,1 0.5,1 0.602,1 0.816,1.005 0.9,1 0.91,1 0.948,0.69 0.962,0.615 1.003,0.376 1,0 1,0". + prevPoint.cx = point.x - prevPoint.x; //change in x between this point and the next point (performance optimization) + prevPoint.cy = point.y - prevPoint.y; + prevPoint.n = point; + prevPoint.nx = point.x; //next point's x value (performance optimization, making lookups faster in getRatio()). Remember, the lookup will always land on a spot where it's either this point or the very next one (never beyond that) + if (fast && i > 1 && Math.abs(prevPoint.cy / prevPoint.cx - points[i - 2].cy / points[i - 2].cx) > 2) { //if there's a sudden change in direction, prioritize accuracy over speed. Like a bounce ease - you don't want to risk the sampling chunks landing on each side of the bounce anchor and having it clipped off. + fast = 0; + } + if (prevPoint.cx < closest) { + if (!prevPoint.cx) { + prevPoint.cx = 0.001; //avoids math problems in getRatio() (dividing by zero) + if (i === l - 1) { //in case the final segment goes vertical RIGHT at the end, make sure we end at the end. + prevPoint.x -= 0.001; + closest = Math.min(closest, 0.001); + fast = 0; + } + } else { + closest = prevPoint.cx; + } + } + } else { + points.splice(i--, 1); + l--; + } + } + l = (1 / closest + 1) | 0; + inc = 1 / l; + j = 0; + point = points[0]; + if (fast) { + for (i = 0; i < l; i++) { //for fastest lookups, we just sample along the path at equal x (time) distance. Uses more memory and is slightly less accurate for anchors that don't land on the sampling points, but for the vast majority of eases it's excellent (and fast). + p = i * inc; + if (point.nx < p) { + point = points[++j]; + } + a1 = point.y + ((p - point.x) / point.cx) * point.cy; + lookup[i] = {x: p, cx: inc, y: a1, cy: 0, nx: 9}; + if (i) { + lookup[i - 1].cy = a1 - lookup[i - 1].y; + } + } + j = points[points.length - 1]; + lookup[l - 1].cy = j.y - a1; + lookup[l - 1].cx = j.x - lookup[lookup.length - 1].x; //make sure it lands EXACTLY where it should. Otherwise, it might be something like 0.9999999999 instead of 1. + } else { //this option is more accurate, ensuring that EVERY anchor is hit perfectly. Clipping across a bounce, for example, would never happen. + for (i = 0; i < l; i++) { //build a lookup table based on the smallest distance so that we can instantly find the appropriate point (well, it'll either be that point or the very next one). We'll look up based on the linear progress. So it's it's 0.5 and the lookup table has 100 elements, it'd be like lookup[Math.floor(0.5 * 100)] + if (point.nx < i * inc) { + point = points[++j]; + } + lookup[i] = point; + } + + if (j < points.length - 1) { + lookup[i-1] = points[points.length-2]; + } + } + //this._calcEnd = (points[points.length-1].y !== 1 || points[0].y !== 0); //ensures that we don't run into floating point errors. As long as we're starting at 0 and ending at 1, tell GSAP to skip the final calculation and use 0/1 as the factor. + + this.ease = p => { + let point = lookup[(p * l) | 0] || lookup[l - 1]; + if (point.nx < p) { + point = point.n; + } + return point.y + ((p - point.x) / point.cx) * point.cy; + }; + + this.ease.custom = this; + + this.id && gsap && gsap.registerEase(this.id, this.ease); + + return this; + } + + getSVGData(config) { + return CustomEase.getSVGData(this, config); + } + + static create(id, data, config) { + return (new CustomEase(id, data, config)).ease; + } + + static register(core) { + gsap = core; + _initCore(); + } + + static get(id) { + return gsap.parseEase(id); + } + + static getSVGData(ease, config) { + config = config || {}; + let width = config.width || 100, + height = config.height || 100, + x = config.x || 0, + y = (config.y || 0) + height, + e = gsap.utils.toArray(config.path)[0], + a, slope, i, inc, tx, ty, precision, threshold, prevX, prevY; + if (config.invert) { + height = -height; + y = 0; + } + if (typeof(ease) === "string") { + ease = gsap.parseEase(ease); + } + if (ease.custom) { + ease = ease.custom; + } + if (ease instanceof CustomEase) { + a = rawPathToString(transformRawPath([ease.segment], width, 0, 0, -height, x, y)); + } else { + a = [x, y]; + precision = Math.max(5, (config.precision || 1) * 200); + inc = 1 / precision; + precision += 2; + threshold = 5 / precision; + prevX = _round(x + inc * width); + prevY = _round(y + ease(inc) * -height); + slope = (prevY - y) / (prevX - x); + for (i = 2; i < precision; i++) { + tx = _round(x + i * inc * width); + ty = _round(y + ease(i * inc) * -height); + if (Math.abs((ty - prevY) / (tx - prevX) - slope) > threshold || i === precision - 1) { //only add points when the slope changes beyond the threshold + a.push(prevX, prevY); + slope = (ty - prevY) / (tx - prevX); + } + prevX = tx; + prevY = ty; + } + a = "M" + a.join(","); + } + e && e.setAttribute("d", a); + return a; + } + +} + +CustomEase.version = "3.12.7"; +CustomEase.headless = true; + +_getGSAP() && gsap.registerPlugin(CustomEase); + +export { CustomEase as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/Draggable.js b/node_modules/gsap/src/Draggable.js new file mode 100644 index 0000000000000000000000000000000000000000..db66a5ac8bda1640b59242366b613fc01316301f --- /dev/null +++ b/node_modules/gsap/src/Draggable.js @@ -0,0 +1,1941 @@ +/*! + * Draggable 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com + */ +/* eslint-disable */ + +import { getGlobalMatrix, Matrix2D } from "./utils/matrix.js"; + +let gsap, _win, _doc, _docElement, _body, _tempDiv, _placeholderDiv, _coreInitted, _checkPrefix, _toArray, _supportsPassive, _isTouchDevice, _touchEventLookup, _isMultiTouching, _isAndroid, InertiaPlugin, _defaultCursor, _supportsPointer, _context, _getStyleSaver, + _dragCount = 0, + _windowExists = () => typeof(window) !== "undefined", + _getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _isFunction = value => typeof(value) === "function", + _isObject = value => typeof(value) === "object", + _isUndefined = value => typeof(value) === "undefined", + _emptyFunc = () => false, + _transformProp = "transform", + _transformOriginProp = "transformOrigin", + _round = value => Math.round(value * 10000) / 10000, + _isArray = Array.isArray, + _createElement = (type, ns) => { + let e = _doc.createElementNS ? _doc.createElementNS((ns || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), type) : _doc.createElement(type); //some servers swap in https for http in the namespace which can break things, making "style" inaccessible. + return e.style ? e : _doc.createElement(type); //some environments won't allow access to the element's style when created with a namespace in which case we default to the standard createElement() to work around the issue. Also note that when GSAP is embedded directly inside an SVG file, createElement() won't allow access to the style object in Firefox (see https://gsap.com/forums/topic/20215-problem-using-tweenmax-in-standalone-self-containing-svg-file-err-cannot-set-property-csstext-of-undefined/). + }, + _RAD2DEG = 180 / Math.PI, + _bigNum = 1e20, + _identityMatrix = new Matrix2D(), + _getTime = Date.now || (() => new Date().getTime()), + _renderQueue = [], + _lookup = {}, //when a Draggable is created, the target gets a unique _gsDragID property that allows gets associated with the Draggable instance for quick lookups in Draggable.get(). This avoids circular references that could cause gc problems. + _lookupCount = 0, + _clickableTagExp = /^(?:a|input|textarea|button|select)$/i, + _lastDragTime = 0, + _temp1 = {}, // a simple object we reuse and populate (usually x/y properties) to conserve memory and improve performance. + _windowProxy = {}, //memory/performance optimization - we reuse this object during autoScroll to store window-related bounds/offsets. + _copy = (obj, factor) => { + let copy = {}, p; + for (p in obj) { + copy[p] = factor ? obj[p] * factor : obj[p]; + } + return copy; + }, + _extend = (obj, defaults) => { + for (let p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + return obj; + }, + _setTouchActionForAllDescendants = (elements, value) => { + let i = elements.length, + children; + while (i--) { + value ? (elements[i].style.touchAction = value) : elements[i].style.removeProperty("touch-action"); + children = elements[i].children; + children && children.length && _setTouchActionForAllDescendants(children, value); + } + }, + _renderQueueTick = () => _renderQueue.forEach(func => func()), + _addToRenderQueue = func => { + _renderQueue.push(func); + if (_renderQueue.length === 1) { + gsap.ticker.add(_renderQueueTick); + } + }, + _renderQueueTimeout = () => !_renderQueue.length && gsap.ticker.remove(_renderQueueTick), + _removeFromRenderQueue = func => { + let i = _renderQueue.length; + while (i--) { + if (_renderQueue[i] === func) { + _renderQueue.splice(i, 1); + } + } + gsap.to(_renderQueueTimeout, {overwrite:true, delay:15, duration:0, onComplete:_renderQueueTimeout, data:"_draggable"}); //remove the "tick" listener only after the render queue is empty for 15 seconds (to improve performance). Adding/removing it constantly for every click/touch wouldn't deliver optimal speed, and we also don't want the ticker to keep calling the render method when things are idle for long periods of time (we want to improve battery life on mobile devices). + }, + _setDefaults = (obj, defaults) => { + for (let p in defaults) { + if (!(p in obj)) { + obj[p] = defaults[p]; + } + } + return obj; + }, + _addListener = (element, type, func, capture) => { + if (element.addEventListener) { + let touchType = _touchEventLookup[type]; + capture = capture || (_supportsPassive ? {passive: false} : null); + element.addEventListener(touchType || type, func, capture); + (touchType && type !== touchType) && element.addEventListener(type, func, capture);//some browsers actually support both, so must we. But pointer events cover all. + } + }, + _removeListener = (element, type, func, capture) => { + if (element.removeEventListener) { + let touchType = _touchEventLookup[type]; + element.removeEventListener(touchType || type, func, capture); + (touchType && type !== touchType) && element.removeEventListener(type, func, capture); + } + }, + _preventDefault = event => { + event.preventDefault && event.preventDefault(); + event.preventManipulation && event.preventManipulation(); //for some Microsoft browsers + }, + _hasTouchID = (list, ID) => { + let i = list.length; + while (i--) { + if (list[i].identifier === ID) { + return true; + } + } + }, + _onMultiTouchDocumentEnd = event => { + _isMultiTouching = (event.touches && _dragCount < event.touches.length); + _removeListener(event.target, "touchend", _onMultiTouchDocumentEnd); + }, + + _onMultiTouchDocument = event => { + _isMultiTouching = (event.touches && _dragCount < event.touches.length); + _addListener(event.target, "touchend", _onMultiTouchDocumentEnd); + }, + _getDocScrollTop = doc => _win.pageYOffset || doc.scrollTop || doc.documentElement.scrollTop || doc.body.scrollTop || 0, + _getDocScrollLeft = doc => _win.pageXOffset || doc.scrollLeft || doc.documentElement.scrollLeft || doc.body.scrollLeft || 0, + _addScrollListener = (e, callback) => { + _addListener(e, "scroll", callback); + if (!_isRoot(e.parentNode)) { + _addScrollListener(e.parentNode, callback); + } + }, + _removeScrollListener = (e, callback) => { + _removeListener(e, "scroll", callback); + if (!_isRoot(e.parentNode)) { + _removeScrollListener(e.parentNode, callback); + } + }, + _isRoot = e => !!(!e || e === _docElement || e.nodeType === 9 || e === _doc.body || e === _win || !e.nodeType || !e.parentNode), + _getMaxScroll = (element, axis) => { + let dim = (axis === "x") ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return Math.max(0, _isRoot(element) ? Math.max(_docElement[scroll], _body[scroll]) - (_win["inner" + dim] || _docElement[client] || _body[client]) : element[scroll] - element[client]); + }, + _recordMaxScrolls = (e, skipCurrent) => { //records _gsMaxScrollX and _gsMaxScrollY properties for the element and all ancestors up the chain so that we can cap it, otherwise dragging beyond the edges with autoScroll on can endlessly scroll. + let x = _getMaxScroll(e, "x"), + y = _getMaxScroll(e, "y"); + if (_isRoot(e)) { + e = _windowProxy; + } else { + _recordMaxScrolls(e.parentNode, skipCurrent); + } + e._gsMaxScrollX = x; + e._gsMaxScrollY = y; + if (!skipCurrent) { + e._gsScrollX = e.scrollLeft || 0; + e._gsScrollY = e.scrollTop || 0; + } + }, + _setStyle = (element, property, value) => { + let style = element.style; + if (!style) { + return; + } + if (_isUndefined(style[property])) { + property = _checkPrefix(property, element) || property; + } + if (value == null) { + style.removeProperty && style.removeProperty(property.replace(/([A-Z])/g, "-$1").toLowerCase()); + } else { + style[property] = value; + } + }, + _getComputedStyle = element => _win.getComputedStyle((element instanceof Element) ? element : element.host || (element.parentNode || {}).host || element), //the "host" stuff helps to accommodate ShadowDom objects. + + _tempRect = {}, //reuse to reduce garbage collection tasks + _parseRect = e => { //accepts a DOM element, a mouse event, or a rectangle object and returns the corresponding rectangle with left, right, width, height, top, and bottom properties + if (e === _win) { + _tempRect.left = _tempRect.top = 0; + _tempRect.width = _tempRect.right = _docElement.clientWidth || e.innerWidth || _body.clientWidth || 0; + _tempRect.height = _tempRect.bottom = ((e.innerHeight || 0) - 20 < _docElement.clientHeight) ? _docElement.clientHeight : e.innerHeight || _body.clientHeight || 0; + return _tempRect; + } + let doc = e.ownerDocument || _doc, + r = !_isUndefined(e.pageX) ? {left: e.pageX - _getDocScrollLeft(doc), top: e.pageY - _getDocScrollTop(doc), right: e.pageX - _getDocScrollLeft(doc) + 1, bottom: e.pageY - _getDocScrollTop(doc) + 1} : (!e.nodeType && !_isUndefined(e.left) && !_isUndefined(e.top)) ? e : _toArray(e)[0].getBoundingClientRect(); + if (_isUndefined(r.right) && !_isUndefined(r.width)) { + r.right = r.left + r.width; + r.bottom = r.top + r.height; + } else if (_isUndefined(r.width)) { //some browsers don't include width and height properties. We can't just set them directly on r because some browsers throw errors, so create a new generic object. + r = {width: r.right - r.left, height: r.bottom - r.top, right: r.right, left: r.left, bottom: r.bottom, top: r.top}; + } + return r; + }, + + _dispatchEvent = (target, type, callbackName) => { + let vars = target.vars, + callback = vars[callbackName], + listeners = target._listeners[type], + result; + if (_isFunction(callback)) { + result = callback.apply(vars.callbackScope || target, vars[callbackName + "Params"] || [target.pointerEvent]); + } + if (listeners && target.dispatchEvent(type) === false) { + result = false; + } + return result; + }, + _getBounds = (target, context) => { //accepts any of the following: a DOM element, jQuery object, selector text, or an object defining bounds as {top, left, width, height} or {minX, maxX, minY, maxY}. Returns an object with left, top, width, and height properties. + let e = _toArray(target)[0], + top, left, offset; + if (!e.nodeType && e !== _win) { + if (!_isUndefined(target.left)) { + offset = {x:0, y:0}; //_getOffsetTransformOrigin(context); //the bounds should be relative to the origin + return {left: target.left - offset.x, top: target.top - offset.y, width: target.width, height: target.height}; + } + left = target.min || target.minX || target.minRotation || 0; + top = target.min || target.minY || 0; + return {left:left, top:top, width:(target.max || target.maxX || target.maxRotation || 0) - left, height:(target.max || target.maxY || 0) - top}; + } + return _getElementBounds(e, context); + }, + _point1 = {}, //we reuse to minimize garbage collection tasks. + _getElementBounds = (element, context) => { + context = _toArray(context)[0]; + let isSVG = (element.getBBox && element.ownerSVGElement), + doc = element.ownerDocument || _doc, + left, right, top, bottom, matrix, p1, p2, p3, p4, bbox, width, height, cs; + if (element === _win) { + top = _getDocScrollTop(doc); + left = _getDocScrollLeft(doc); + right = left + (doc.documentElement.clientWidth || element.innerWidth || doc.body.clientWidth || 0); + bottom = top + (((element.innerHeight || 0) - 20 < doc.documentElement.clientHeight) ? doc.documentElement.clientHeight : element.innerHeight || doc.body.clientHeight || 0); //some browsers (like Firefox) ignore absolutely positioned elements, and collapse the height of the documentElement, so it could be 8px, for example, if you have just an absolutely positioned div. In that case, we use the innerHeight to resolve this. + } else if (context === _win || _isUndefined(context)) { + return element.getBoundingClientRect(); + } else { + left = top = 0; + if (isSVG) { + bbox = element.getBBox(); + width = bbox.width; + height = bbox.height; + } else { + if (element.viewBox && (bbox = element.viewBox.baseVal)) { + left = bbox.x || 0; + top = bbox.y || 0; + width = bbox.width; + height = bbox.height; + } + if (!width) { + cs = _getComputedStyle(element); + bbox = cs.boxSizing === "border-box"; + width = (parseFloat(cs.width) || element.clientWidth || 0) + (bbox ? 0 : parseFloat(cs.borderLeftWidth) + parseFloat(cs.borderRightWidth)); + height = (parseFloat(cs.height) || element.clientHeight || 0) + (bbox ? 0 : parseFloat(cs.borderTopWidth) + parseFloat(cs.borderBottomWidth)); + } + } + right = width; + bottom = height; + } + if (element === context) { + return {left:left, top:top, width: right - left, height: bottom - top}; + } + matrix = getGlobalMatrix(context, true).multiply(getGlobalMatrix(element)); + p1 = matrix.apply({x:left, y:top}); + p2 = matrix.apply({x:right, y:top}); + p3 = matrix.apply({x:right, y:bottom}); + p4 = matrix.apply({x:left, y:bottom}); + left = Math.min(p1.x, p2.x, p3.x, p4.x); + top = Math.min(p1.y, p2.y, p3.y, p4.y); + return {left: left, top: top, width: Math.max(p1.x, p2.x, p3.x, p4.x) - left, height: Math.max(p1.y, p2.y, p3.y, p4.y) - top}; + }, + _parseInertia = (draggable, snap, max, min, factor, forceZeroVelocity) => { + let vars = {}, + a, i, l; + if (snap) { + if (factor !== 1 && snap instanceof Array) { //some data must be altered to make sense, like if the user passes in an array of rotational values in degrees, we must convert it to radians. Or for scrollLeft and scrollTop, we invert the values. + vars.end = a = []; + l = snap.length; + if (_isObject(snap[0])) { //if the array is populated with objects, like points ({x:100, y:200}), make copies before multiplying by the factor, otherwise we'll mess up the originals and the user may reuse it elsewhere. + for (i = 0; i < l; i++) { + a[i] = _copy(snap[i], factor); + } + } else { + for (i = 0; i < l; i++) { + a[i] = snap[i] * factor; + } + } + max += 1.1; //allow 1.1 pixels of wiggle room when snapping in order to work around some browser inconsistencies in the way bounds are reported which can make them roughly a pixel off. For example, if "snap:[-$('#menu').width(), 0]" was defined and #menu had a wrapper that was used as the bounds, some browsers would be one pixel off, making the minimum -752 for example when snap was [-753,0], thus instead of snapping to -753, it would snap to 0 since -753 was below the minimum. + min -= 1.1; + } else if (_isFunction(snap)) { + vars.end = value => { + let result = snap.call(draggable, value), + copy, p; + if (factor !== 1) { + if (_isObject(result)) { + copy = {}; + for (p in result) { + copy[p] = result[p] * factor; + } + result = copy; + } else { + result *= factor; + } + } + return result; //we need to ensure that we can scope the function call to the Draggable instance itself so that users can access important values like maxX, minX, maxY, minY, x, and y from within that function. + }; + } else { + vars.end = snap; + } + } + if (max || max === 0) { + vars.max = max; + } + if (min || min === 0) { + vars.min = min; + } + if (forceZeroVelocity) { + vars.velocity = 0; + } + return vars; + }, + _isClickable = element => { //sometimes it's convenient to mark an element as clickable by adding a data-clickable="true" attribute (in which case we won't preventDefault() the mouse/touch event). This method checks if the element is an <a>, <input>, or <button> or has the data-clickable or contentEditable attribute set to true (or any of its parent elements). + let data; + return (!element || !element.getAttribute || element === _body) ? false : ((data = element.getAttribute("data-clickable")) === "true" || (data !== "false" && (_clickableTagExp.test(element.nodeName + "") || element.getAttribute("contentEditable") === "true"))) ? true : _isClickable(element.parentNode); + }, + _setSelectable = (elements, selectable) => { + let i = elements.length, + e; + while (i--) { + e = elements[i]; + e.ondragstart = e.onselectstart = selectable ? null : _emptyFunc; + gsap.set(e, {lazy:true, userSelect: (selectable ? "text" : "none")}); + } + }, + _isFixed = element => { + if (_getComputedStyle(element).position === "fixed") { + return true; + } + element = element.parentNode; + if (element && element.nodeType === 1) { // avoid document fragments which will throw an error. + return _isFixed(element); + } + }, + _supports3D, _addPaddingBR, + + //The ScrollProxy class wraps an element's contents into another div (we call it "content") that we either add padding when necessary or apply a translate3d() transform in order to overscroll (scroll past the boundaries). This allows us to simply set the scrollTop/scrollLeft (or top/left for easier reverse-axis orientation, which is what we do in Draggable) and it'll do all the work for us. For example, if we tried setting scrollTop to -100 on a normal DOM element, it wouldn't work - it'd look the same as setting it to 0, but if we set scrollTop of a ScrollProxy to -100, it'll give the correct appearance by either setting paddingTop of the wrapper to 100 or applying a 100-pixel translateY. + ScrollProxy = function(element, vars) { + element = gsap.utils.toArray(element)[0]; + vars = vars || {}; + let content = document.createElement("div"), + style = content.style, + node = element.firstChild, + offsetTop = 0, + offsetLeft = 0, + prevTop = element.scrollTop, + prevLeft = element.scrollLeft, + scrollWidth = element.scrollWidth, + scrollHeight = element.scrollHeight, + extraPadRight = 0, + maxLeft = 0, + maxTop = 0, + elementWidth, elementHeight, contentHeight, nextNode, transformStart, transformEnd; + if (_supports3D && vars.force3D !== false) { + transformStart = "translate3d("; + transformEnd = "px,0px)"; + } else if (_transformProp) { + transformStart = "translate("; + transformEnd = "px)"; + } + this.scrollTop = function(value, force) { + if (!arguments.length) { + return -this.top(); + } + this.top(-value, force); + }; + this.scrollLeft = function(value, force) { + if (!arguments.length) { + return -this.left(); + } + this.left(-value, force); + }; + this.left = function(value, force) { + if (!arguments.length) { + return -(element.scrollLeft + offsetLeft); + } + let dif = element.scrollLeft - prevLeft, + oldOffset = offsetLeft; + if ((dif > 2 || dif < -2) && !force) { //if the user interacts with the scrollbar (or something else scrolls it, like the mouse wheel), we should kill any tweens of the ScrollProxy. + prevLeft = element.scrollLeft; + gsap.killTweensOf(this, {left:1, scrollLeft:1}); + this.left(-prevLeft); + if (vars.onKill) { + vars.onKill(); + } + return; + } + value = -value; //invert because scrolling works in the opposite direction + if (value < 0) { + offsetLeft = (value - 0.5) | 0; + value = 0; + } else if (value > maxLeft) { + offsetLeft = (value - maxLeft) | 0; + value = maxLeft; + } else { + offsetLeft = 0; + } + if (offsetLeft || oldOffset) { + if (!this._skip) { + style[_transformProp] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + if (offsetLeft + extraPadRight >= 0) { + style.paddingRight = offsetLeft + extraPadRight + "px"; + } + } + element.scrollLeft = value | 0; + prevLeft = element.scrollLeft; //don't merge this with the line above because some browsers adjust the scrollLeft after it's set, so in order to be 100% accurate in tracking it, we need to ask the browser to report it. + }; + this.top = function(value, force) { + if (!arguments.length) { + return -(element.scrollTop + offsetTop); + } + let dif = element.scrollTop - prevTop, + oldOffset = offsetTop; + if ((dif > 2 || dif < -2) && !force) { //if the user interacts with the scrollbar (or something else scrolls it, like the mouse wheel), we should kill any tweens of the ScrollProxy. + prevTop = element.scrollTop; + gsap.killTweensOf(this, {top:1, scrollTop:1}); + this.top(-prevTop); + if (vars.onKill) { + vars.onKill(); + } + return; + } + value = -value; //invert because scrolling works in the opposite direction + if (value < 0) { + offsetTop = (value - 0.5) | 0; + value = 0; + } else if (value > maxTop) { + offsetTop = (value - maxTop) | 0; + value = maxTop; + } else { + offsetTop = 0; + } + if (offsetTop || oldOffset) { + if (!this._skip) { + style[_transformProp] = transformStart + -offsetLeft + "px," + -offsetTop + transformEnd; + } + } + element.scrollTop = value | 0; + prevTop = element.scrollTop; + }; + + this.maxScrollTop = () => maxTop; + this.maxScrollLeft = () => maxLeft; + + this.disable = function() { + node = content.firstChild; + while (node) { + nextNode = node.nextSibling; + element.appendChild(node); + node = nextNode; + } + if (element === content.parentNode) { //in case disable() is called when it's already disabled. + element.removeChild(content); + } + }; + this.enable = function() { + node = element.firstChild; + if (node === content) { + return; + } + while (node) { + nextNode = node.nextSibling; + content.appendChild(node); + node = nextNode; + } + element.appendChild(content); + this.calibrate(); + }; + this.calibrate = function(force) { + let widthMatches = (element.clientWidth === elementWidth), + cs, x, y; + prevTop = element.scrollTop; + prevLeft = element.scrollLeft; + if (widthMatches && element.clientHeight === elementHeight && content.offsetHeight === contentHeight && scrollWidth === element.scrollWidth && scrollHeight === element.scrollHeight && !force) { + return; //no need to recalculate things if the width and height haven't changed. + } + if (offsetTop || offsetLeft) { + x = this.left(); + y = this.top(); + this.left(-element.scrollLeft); + this.top(-element.scrollTop); + } + cs = _getComputedStyle(element); + //first, we need to remove any width constraints to see how the content naturally flows so that we can see if it's wider than the containing element. If so, we've got to record the amount of overage so that we can apply that as padding in order for browsers to correctly handle things. Then we switch back to a width of 100% (without that, some browsers don't flow the content correctly) + if (!widthMatches || force) { + style.display = "block"; + style.width = "auto"; + style.paddingRight = "0px"; + extraPadRight = Math.max(0, element.scrollWidth - element.clientWidth); + //if the content is wider than the container, we need to add the paddingLeft and paddingRight in order for things to behave correctly. + if (extraPadRight) { + extraPadRight += parseFloat(cs.paddingLeft) + (_addPaddingBR ? parseFloat(cs.paddingRight) : 0); + } + } + style.display = "inline-block"; + style.position = "relative"; + style.overflow = "visible"; + style.verticalAlign = "top"; + style.boxSizing = "content-box"; + style.width = "100%"; + style.paddingRight = extraPadRight + "px"; + //some browsers neglect to factor in the bottom padding when calculating the scrollHeight, so we need to add that padding to the content when that happens. Allow a 2px margin for error + if (_addPaddingBR) { + style.paddingBottom = cs.paddingBottom; + } + elementWidth = element.clientWidth; + elementHeight = element.clientHeight; + scrollWidth = element.scrollWidth; + scrollHeight = element.scrollHeight; + maxLeft = element.scrollWidth - elementWidth; + maxTop = element.scrollHeight - elementHeight; + contentHeight = content.offsetHeight; + style.display = "block"; + if (x || y) { + this.left(x); + this.top(y); + } + }; + this.content = content; + this.element = element; + this._skip = false; + this.enable(); + }, + _initCore = required => { + if (_windowExists() && document.body) { + let nav = window && window.navigator; + _win = window; + _doc = document; + _docElement = _doc.documentElement; + _body = _doc.body; + _tempDiv = _createElement("div"); + _supportsPointer = !!window.PointerEvent; + _placeholderDiv = _createElement("div"); + _placeholderDiv.style.cssText = "visibility:hidden;height:1px;top:-1px;pointer-events:none;position:relative;clear:both;cursor:grab"; + _defaultCursor = _placeholderDiv.style.cursor === "grab" ? "grab" : "move"; + _isAndroid = (nav && nav.userAgent.toLowerCase().indexOf("android") !== -1); //Android handles touch events in an odd way and it's virtually impossible to "feature test" so we resort to UA sniffing + _isTouchDevice = (("ontouchstart" in _docElement) && ("orientation" in _win)) || (nav && (nav.MaxTouchPoints > 0 || nav.msMaxTouchPoints > 0)); + _addPaddingBR = (function() { //this function is in charge of analyzing browser behavior related to padding. It sets the _addPaddingBR to true if the browser doesn't normally factor in the bottom or right padding on the element inside the scrolling area, and it sets _addPaddingLeft to true if it's a browser that requires the extra offset (offsetLeft) to be added to the paddingRight (like Opera). + let div = _createElement("div"), + child = _createElement("div"), + childStyle = child.style, + parent = _body, + val; + childStyle.display = "inline-block"; + childStyle.position = "relative"; + div.style.cssText = "width:90px;height:40px;padding:10px;overflow:auto;visibility:hidden"; + div.appendChild(child); + parent.appendChild(div); + val = (child.offsetHeight + 18 > div.scrollHeight); //div.scrollHeight should be child.offsetHeight + 20 because of the 10px of padding on each side, but some browsers ignore one side. We allow a 2px margin of error. + parent.removeChild(div); + return val; + }()); + _touchEventLookup = (function(types) { //we create an object that makes it easy to translate touch event types into their "pointer" counterparts if we're in a browser that uses those instead. Like IE10 uses "MSPointerDown" instead of "touchstart", for example. + let standard = types.split(","), + converted = ("onpointerdown" in _tempDiv ? "pointerdown,pointermove,pointerup,pointercancel" : "onmspointerdown" in _tempDiv ? "MSPointerDown,MSPointerMove,MSPointerUp,MSPointerCancel" : types).split(","), + obj = {}, + i = 4; + while (--i > -1) { + obj[standard[i]] = converted[i]; + obj[converted[i]] = standard[i]; + } + //to avoid problems in iOS 9, test to see if the browser supports the "passive" option on addEventListener(). + try { + _docElement.addEventListener("test", null, Object.defineProperty({}, "passive", { + get: function () { + _supportsPassive = 1; + } + })); + } catch (e) {} + return obj; + }("touchstart,touchmove,touchend,touchcancel")); + _addListener(_doc, "touchcancel", _emptyFunc); //some older Android devices intermittently stop dispatching "touchmove" events if we don't listen for "touchcancel" on the document. Very strange indeed. + _addListener(_win, "touchmove", _emptyFunc); //works around Safari bugs that still allow the page to scroll even when we preventDefault() on the touchmove event. + _body && _body.addEventListener("touchstart", _emptyFunc); //works around Safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/ + _addListener(_doc, "contextmenu", function() { + for (let p in _lookup) { + if (_lookup[p].isPressed) { + _lookup[p].endDrag(); + } + } + }); + gsap = _coreInitted = _getGSAP(); + } + if (gsap) { + InertiaPlugin = gsap.plugins.inertia; + _context = gsap.core.context || function() {}; + _checkPrefix = gsap.utils.checkPrefix; + _transformProp = _checkPrefix(_transformProp); + _transformOriginProp = _checkPrefix(_transformOriginProp); + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + _supports3D = !!_checkPrefix("perspective"); + } else if (required) { + console.warn("Please gsap.registerPlugin(Draggable)"); + } + }; + + + + + + +class EventDispatcher { + + constructor(target) { + this._listeners = {}; + this.target = target || this; + } + + addEventListener(type, callback) { + let list = this._listeners[type] || (this._listeners[type] = []); + if (!~list.indexOf(callback)) { + list.push(callback); + } + } + + removeEventListener(type, callback) { + let list = this._listeners[type], + i = (list && list.indexOf(callback)); + (i >= 0) && list.splice(i, 1); + } + + dispatchEvent(type) { + let result; + (this._listeners[type] || []).forEach(callback => (callback.call(this, {type: type, target: this.target}) === false) && (result = false)); + return result; //if any of the callbacks return false, pass that along. + } +} + + + + + + + + + +export class Draggable extends EventDispatcher { + + constructor(target, vars) { + super(); + _coreInitted || _initCore(1); + target = _toArray(target)[0]; //in case the target is a selector object or selector text + this.styles = _getStyleSaver && _getStyleSaver(target, "transform,left,top"); + if (!InertiaPlugin) { + InertiaPlugin = gsap.plugins.inertia; + } + this.vars = vars = _copy(vars || {}); + this.target = target; + this.x = this.y = this.rotation = 0; + this.dragResistance = parseFloat(vars.dragResistance) || 0; + this.edgeResistance = isNaN(vars.edgeResistance) ? 1 : parseFloat(vars.edgeResistance) || 0; + this.lockAxis = vars.lockAxis; + this.autoScroll = vars.autoScroll || 0; + this.lockedAxis = null; + this.allowEventDefault = !!vars.allowEventDefault; + + gsap.getProperty(target, "x"); // to ensure that transforms are instantiated. + + let type = (vars.type || "x,y").toLowerCase(), + xyMode = (~type.indexOf("x") || ~type.indexOf("y")), + rotationMode = (type.indexOf("rotation") !== -1), + xProp = rotationMode ? "rotation" : xyMode ? "x" : "left", + yProp = xyMode ? "y" : "top", + allowX = !!(~type.indexOf("x") || ~type.indexOf("left") || type === "scroll"), + allowY = !!(~type.indexOf("y") || ~type.indexOf("top") || type === "scroll"), + minimumMovement = vars.minimumMovement || 2, + self = this, + triggers = _toArray(vars.trigger || vars.handle || target), + killProps = {}, + dragEndTime = 0, + checkAutoScrollBounds = false, + autoScrollMarginTop = vars.autoScrollMarginTop || 40, + autoScrollMarginRight = vars.autoScrollMarginRight || 40, + autoScrollMarginBottom = vars.autoScrollMarginBottom || 40, + autoScrollMarginLeft = vars.autoScrollMarginLeft || 40, + isClickable = vars.clickableTest || _isClickable, + clickTime = 0, + gsCache = target._gsap || gsap.core.getCache(target), + isFixed = _isFixed(target), + getPropAsNum = (property, unit) => parseFloat(gsCache.get(target, property, unit)), + ownerDoc = target.ownerDocument || _doc, + enabled, scrollProxy, startPointerX, startPointerY, startElementX, startElementY, hasBounds, hasDragCallback, hasMoveCallback, maxX, minX, maxY, minY, touch, touchID, rotationOrigin, dirty, old, snapX, snapY, snapXY, isClicking, touchEventTarget, matrix, interrupted, allowNativeTouchScrolling, touchDragAxis, isDispatching, clickDispatch, trustedClickDispatch, isPreventingDefault, innerMatrix, dragged, + + onContextMenu = e => { //used to prevent long-touch from triggering a context menu. + // (self.isPressed && e.which < 2) && self.endDrag() // previously ended drag when context menu was triggered, but instead we should just stop propagation and prevent the default event behavior. + _preventDefault(e); + e.stopImmediatePropagation && e.stopImmediatePropagation(); + return false; + }, + + //this method gets called on every tick of TweenLite.ticker which allows us to synchronize the renders to the core engine (which is typically synchronized with the display refresh via requestAnimationFrame). This is an optimization - it's better than applying the values inside the "mousemove" or "touchmove" event handler which may get called many times inbetween refreshes. + render = suppressEvents => { + if (self.autoScroll && self.isDragging && (checkAutoScrollBounds || dirty)) { + let e = target, + autoScrollFactor = self.autoScroll * 15, //multiplying by 15 just gives us a better "feel" speed-wise. + parent, isRoot, rect, pointerX, pointerY, changeX, changeY, gap; + checkAutoScrollBounds = false; + _windowProxy.scrollTop = ((_win.pageYOffset != null) ? _win.pageYOffset : (ownerDoc.documentElement.scrollTop != null) ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop); + _windowProxy.scrollLeft = ((_win.pageXOffset != null) ? _win.pageXOffset : (ownerDoc.documentElement.scrollLeft != null) ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft); + pointerX = self.pointerX - _windowProxy.scrollLeft; + pointerY = self.pointerY - _windowProxy.scrollTop; + while (e && !isRoot) { //walk up the chain and sense wherever the pointer is within 40px of an edge that's scrollable. + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + rect = isRoot ? {bottom:Math.max(_docElement.clientHeight, _win.innerHeight || 0), right: Math.max(_docElement.clientWidth, _win.innerWidth || 0), left:0, top:0} : parent.getBoundingClientRect(); + changeX = changeY = 0; + if (allowY) { + gap = parent._gsMaxScrollY - parent.scrollTop; + if (gap < 0) { + changeY = gap; + } else if (pointerY > rect.bottom - autoScrollMarginBottom && gap) { + checkAutoScrollBounds = true; + changeY = Math.min(gap, (autoScrollFactor * (1 - Math.max(0, (rect.bottom - pointerY)) / autoScrollMarginBottom)) | 0); + } else if (pointerY < rect.top + autoScrollMarginTop && parent.scrollTop) { + checkAutoScrollBounds = true; + changeY = -Math.min(parent.scrollTop, (autoScrollFactor * (1 - Math.max(0, (pointerY - rect.top)) / autoScrollMarginTop)) | 0); + } + if (changeY) { + parent.scrollTop += changeY; + } + } + if (allowX) { + gap = parent._gsMaxScrollX - parent.scrollLeft; + if (gap < 0) { + changeX = gap; + } else if (pointerX > rect.right - autoScrollMarginRight && gap) { + checkAutoScrollBounds = true; + changeX = Math.min(gap, (autoScrollFactor * (1 - Math.max(0, (rect.right - pointerX)) / autoScrollMarginRight)) | 0); + } else if (pointerX < rect.left + autoScrollMarginLeft && parent.scrollLeft) { + checkAutoScrollBounds = true; + changeX = -Math.min(parent.scrollLeft, (autoScrollFactor * (1 - Math.max(0, (pointerX - rect.left)) / autoScrollMarginLeft)) | 0); + } + if (changeX) { + parent.scrollLeft += changeX; + } + } + + if (isRoot && (changeX || changeY)) { + _win.scrollTo(parent.scrollLeft, parent.scrollTop); + setPointerPosition(self.pointerX + changeX, self.pointerY + changeY); + } + e = parent; + } + } + if (dirty) { + let {x, y} = self; + if (rotationMode) { + self.deltaX = x - parseFloat(gsCache.rotation); + self.rotation = x; + gsCache.rotation = x + "deg"; + gsCache.renderTransform(1, gsCache); + } else { + if (scrollProxy) { + if (allowY) { + self.deltaY = y - scrollProxy.top(); + scrollProxy.top(y); + } + if (allowX) { + self.deltaX = x - scrollProxy.left(); + scrollProxy.left(x); + } + } else if (xyMode) { + if (allowY) { + self.deltaY = y - parseFloat(gsCache.y); + gsCache.y = y + "px"; + } + if (allowX) { + self.deltaX = x - parseFloat(gsCache.x); + gsCache.x = x + "px"; + } + gsCache.renderTransform(1, gsCache); + } else { + if (allowY) { + self.deltaY = y - parseFloat(target.style.top || 0); + target.style.top = y + "px"; + } + if (allowX) { + self.deltaX = x - parseFloat(target.style.left || 0); + target.style.left = x + "px"; + } + } + } + if (hasDragCallback && !suppressEvents && !isDispatching) { + isDispatching = true; //in case onDrag has an update() call (avoid endless loop) + if (_dispatchEvent(self, "drag", "onDrag") === false) { + if (allowX) { + self.x -= self.deltaX; + } + if (allowY) { + self.y -= self.deltaY; + } + render(true); + } + isDispatching = false; + } + } + dirty = false; + }, + + //copies the x/y from the element (whether that be transforms, top/left, or ScrollProxy's top/left) to the Draggable's x and y (and rotation if necessary) properties so that they reflect reality and it also (optionally) applies any snapping necessary. This is used by the InertiaPlugin tween in an onUpdate to ensure things are synced and snapped. + syncXY = (skipOnUpdate, skipSnap) => { + let { x, y } = self, + snappedValue, cs; + if (!target._gsap) { //just in case the _gsap cache got wiped, like if the user called clearProps on the transform or something (very rare). + gsCache = gsap.core.getCache(target); + } + gsCache.uncache && gsap.getProperty(target, "x"); // trigger a re-cache + if (xyMode) { + self.x = parseFloat(gsCache.x); + self.y = parseFloat(gsCache.y); + } else if (rotationMode) { + self.x = self.rotation = parseFloat(gsCache.rotation); + } else if (scrollProxy) { + self.y = scrollProxy.top(); + self.x = scrollProxy.left(); + } else { + self.y = parseFloat(target.style.top || ((cs = _getComputedStyle(target)) && cs.top)) || 0; + self.x = parseFloat(target.style.left || (cs || {}).left) || 0; + } + if ((snapX || snapY || snapXY) && !skipSnap && (self.isDragging || self.isThrowing)) { + if (snapXY) { + _temp1.x = self.x; + _temp1.y = self.y; + snappedValue = snapXY(_temp1); + if (snappedValue.x !== self.x) { + self.x = snappedValue.x; + dirty = true; + } + if (snappedValue.y !== self.y) { + self.y = snappedValue.y; + dirty = true; + } + } + if (snapX) { + snappedValue = snapX(self.x); + if (snappedValue !== self.x) { + self.x = snappedValue; + if (rotationMode) { + self.rotation = snappedValue; + } + dirty = true; + } + } + if (snapY) { + snappedValue = snapY(self.y); + if (snappedValue !== self.y) { + self.y = snappedValue; + } + dirty = true; + } + } + dirty && render(true); + if (!skipOnUpdate) { + self.deltaX = self.x - x; + self.deltaY = self.y - y; + _dispatchEvent(self, "throwupdate", "onThrowUpdate"); + } + }, + + buildSnapFunc = (snap, min, max, factor) => { + if (min == null) { + min = -_bigNum; + } + if (max == null) { + max = _bigNum; + } + if (_isFunction(snap)) { + return n => { + let edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance; //if we're tweening, disable the edgeTolerance because it's already factored into the tweening values (we don't want to apply it multiple times) + return snap.call(self, (n > max ? max + (n - max) * edgeTolerance : (n < min) ? min + (n - min) * edgeTolerance : n) * factor) * factor; + }; + } + if (_isArray(snap)) { + return n => { + let i = snap.length, + closest = 0, + absDif = _bigNum, + val, dif; + while (--i > -1) { + val = snap[i]; + dif = val - n; + if (dif < 0) { + dif = -dif; + } + if (dif < absDif && val >= min && val <= max) { + closest = i; + absDif = dif; + } + } + return snap[closest]; + }; + } + return isNaN(snap) ? n => n : () => snap * factor; + }, + + buildPointSnapFunc = (snap, minX, maxX, minY, maxY, radius, factor) => { + radius = (radius && radius < _bigNum) ? radius * radius : _bigNum; //so we don't have to Math.sqrt() in the functions. Performance optimization. + if (_isFunction(snap)) { + return point => { + let edgeTolerance = !self.isPressed ? 1 : 1 - self.edgeResistance, + x = point.x, + y = point.y, + result, dx, dy; //if we're tweening, disable the edgeTolerance because it's already factored into the tweening values (we don't want to apply it multiple times) + point.x = x = (x > maxX ? maxX + (x - maxX) * edgeTolerance : (x < minX) ? minX + (x - minX) * edgeTolerance : x); + point.y = y = (y > maxY ? maxY + (y - maxY) * edgeTolerance : (y < minY) ? minY + (y - minY) * edgeTolerance : y); + result = snap.call(self, point); + if (result !== point) { + point.x = result.x; + point.y = result.y; + } + if (factor !== 1) { + point.x *= factor; + point.y *= factor; + } + if (radius < _bigNum) { + dx = point.x - x; + dy = point.y - y; + if (dx * dx + dy * dy > radius) { + point.x = x; + point.y = y; + } + } + return point; + }; + } + if (_isArray(snap)) { + return p => { + let i = snap.length, + closest = 0, + minDist = _bigNum, + x, y, point, dist; + while (--i > -1) { + point = snap[i]; + x = point.x - p.x; + y = point.y - p.y; + dist = x * x + y * y; + if (dist < minDist) { + closest = i; + minDist = dist; + } + } + return (minDist <= radius) ? snap[closest] : p; + }; + } + return n => n; + }, + + calculateBounds = () => { + let bounds, targetBounds, snap, snapIsRaw; + hasBounds = false; + if (scrollProxy) { + scrollProxy.calibrate(); + self.minX = minX = -scrollProxy.maxScrollLeft(); + self.minY = minY = -scrollProxy.maxScrollTop(); + self.maxX = maxX = self.maxY = maxY = 0; + hasBounds = true; + } else if (!!vars.bounds) { + bounds = _getBounds(vars.bounds, target.parentNode); //could be a selector/jQuery object or a DOM element or a generic object like {top:0, left:100, width:1000, height:800} or {minX:100, maxX:1100, minY:0, maxY:800} + if (rotationMode) { + self.minX = minX = bounds.left; + self.maxX = maxX = bounds.left + bounds.width; + self.minY = minY = self.maxY = maxY = 0; + } else if (!_isUndefined(vars.bounds.maxX) || !_isUndefined(vars.bounds.maxY)) { + bounds = vars.bounds; + self.minX = minX = bounds.minX; + self.minY = minY = bounds.minY; + self.maxX = maxX = bounds.maxX; + self.maxY = maxY = bounds.maxY; + } else { + targetBounds = _getBounds(target, target.parentNode); + self.minX = minX = Math.round(getPropAsNum(xProp, "px") + bounds.left - targetBounds.left); + self.minY = minY = Math.round(getPropAsNum(yProp, "px") + bounds.top - targetBounds.top); + self.maxX = maxX = Math.round(minX + (bounds.width - targetBounds.width)); + self.maxY = maxY = Math.round(minY + (bounds.height - targetBounds.height)); + } + if (minX > maxX) { + self.minX = maxX; + self.maxX = maxX = minX; + minX = self.minX; + } + if (minY > maxY) { + self.minY = maxY; + self.maxY = maxY = minY; + minY = self.minY; + } + if (rotationMode) { + self.minRotation = minX; + self.maxRotation = maxX; + } + hasBounds = true; + } + if (vars.liveSnap) { + snap = (vars.liveSnap === true) ? (vars.snap || {}) : vars.liveSnap; + snapIsRaw = (_isArray(snap) || _isFunction(snap)); + if (rotationMode) { + snapX = buildSnapFunc((snapIsRaw ? snap : snap.rotation), minX, maxX, 1); + snapY = null; + } else { + if (snap.points) { + snapXY = buildPointSnapFunc((snapIsRaw ? snap : snap.points), minX, maxX, minY, maxY, snap.radius, scrollProxy ? -1 : 1); + } else { + if (allowX) { + snapX = buildSnapFunc((snapIsRaw ? snap : snap.x || snap.left || snap.scrollLeft), minX, maxX, scrollProxy ? -1 : 1); + } + if (allowY) { + snapY = buildSnapFunc((snapIsRaw ? snap : snap.y || snap.top || snap.scrollTop), minY, maxY, scrollProxy ? -1 : 1); + } + } + } + } + }, + + onThrowComplete = () => { + self.isThrowing = false; + _dispatchEvent(self, "throwcomplete", "onThrowComplete"); + }, + onThrowInterrupt = () => { + self.isThrowing = false; + }, + + animate = (inertia, forceZeroVelocity) => { + let snap, snapIsRaw, tween, overshootTolerance; + if (inertia && InertiaPlugin) { + if (inertia === true) { + snap = vars.snap || vars.liveSnap || {}; + snapIsRaw = (_isArray(snap) || _isFunction(snap)); + inertia = {resistance:(vars.throwResistance || vars.resistance || 1000) / (rotationMode ? 10 : 1)}; + if (rotationMode) { + inertia.rotation = _parseInertia(self, snapIsRaw ? snap : snap.rotation, maxX, minX, 1, forceZeroVelocity); + } else { + if (allowX) { + inertia[xProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.x || snap.left, maxX, minX, scrollProxy ? -1 : 1, forceZeroVelocity || (self.lockedAxis === "x")); + } + if (allowY) { + inertia[yProp] = _parseInertia(self, snapIsRaw ? snap : snap.points || snap.y || snap.top, maxY, minY, scrollProxy ? -1 : 1, forceZeroVelocity || (self.lockedAxis === "y")); + } + if (snap.points || (_isArray(snap) && _isObject(snap[0]))) { + inertia.linkedProps = xProp + "," + yProp; + inertia.radius = snap.radius; //note: we also disable liveSnapping while throwing if there's a "radius" defined, otherwise it looks weird to have the item thrown past a snapping point but live-snapping mid-tween. We do this by altering the onUpdateParams so that "skipSnap" parameter is true for syncXY. + } + } + } + self.isThrowing = true; + overshootTolerance = (!isNaN(vars.overshootTolerance)) ? vars.overshootTolerance : (vars.edgeResistance === 1) ? 0 : (1 - self.edgeResistance) + 0.2; + if (!inertia.duration) { + inertia.duration = {max: Math.max(vars.minDuration || 0, ("maxDuration" in vars) ? vars.maxDuration : 2), min: (!isNaN(vars.minDuration) ? vars.minDuration : (overshootTolerance === 0 || (_isObject(inertia) && inertia.resistance > 1000)) ? 0 : 0.5), overshoot: overshootTolerance}; + } + self.tween = tween = gsap.to(scrollProxy || target, { + inertia: inertia, + data: "_draggable", + inherit: false, + onComplete: onThrowComplete, + onInterrupt: onThrowInterrupt, + onUpdate: (vars.fastMode ? _dispatchEvent : syncXY), + onUpdateParams: (vars.fastMode ? [self, "onthrowupdate", "onThrowUpdate"] : (snap && snap.radius) ? [false, true] : []) + }); + if (!vars.fastMode) { + if (scrollProxy) { + scrollProxy._skip = true; // Microsoft browsers have a bug that causes them to briefly render the position incorrectly (it flashes to the end state when we seek() the tween even though we jump right back to the current position, and this only seems to happen when we're affecting both top and left), so we set a _suspendTransforms flag to prevent it from actually applying the values in the ScrollProxy. + } + tween.render(1e9, true, true); // force to the end. Remember, the duration will likely change upon initting because that's when InertiaPlugin calculates it. + syncXY(true, true); + self.endX = self.x; + self.endY = self.y; + if (rotationMode) { + self.endRotation = self.x; + } + tween.play(0); + syncXY(true, true); + if (scrollProxy) { + scrollProxy._skip = false; //Microsoft browsers have a bug that causes them to briefly render the position incorrectly (it flashes to the end state when we seek() the tween even though we jump right back to the current position, and this only seems to happen when we're affecting both top and left), so we set a _suspendTransforms flag to prevent it from actually applying the values in the ScrollProxy. + } + } + } else if (hasBounds) { + self.applyBounds(); + } + }, + + updateMatrix = shiftStart => { + let start = matrix, + p; + matrix = getGlobalMatrix(target.parentNode, true); + if (shiftStart && self.isPressed && !matrix.equals(start || new Matrix2D())) { //if the matrix changes WHILE the element is pressed, we must adjust the startPointerX and startPointerY accordingly, so we invert the original matrix and figure out where the pointerX and pointerY were in the global space, then apply the new matrix to get the updated coordinates. + p = start.inverse().apply({x:startPointerX, y:startPointerY}); + matrix.apply(p, p); + startPointerX = p.x; + startPointerY = p.y; + } + if (matrix.equals(_identityMatrix)) { //if there are no transforms, we can optimize performance by not factoring in the matrix + matrix = null; + } + }, + + recordStartPositions = () => { + let edgeTolerance = 1 - self.edgeResistance, + offsetX = isFixed ? _getDocScrollLeft(ownerDoc) : 0, + offsetY = isFixed ? _getDocScrollTop(ownerDoc) : 0, + parsedOrigin, x, y; + if (xyMode) { // in case the user set it as a different unit, like animating the x to "100%". We must convert it back to px! + gsCache.x = getPropAsNum(xProp, "px") + "px"; + gsCache.y = getPropAsNum(yProp, "px") + "px"; + gsCache.renderTransform(); + } + updateMatrix(false); + _point1.x = self.pointerX - offsetX; + _point1.y = self.pointerY - offsetY; + matrix && matrix.apply(_point1, _point1); + startPointerX = _point1.x; //translate to local coordinate system + startPointerY = _point1.y; + if (dirty) { + setPointerPosition(self.pointerX, self.pointerY); + render(true); + } + innerMatrix = getGlobalMatrix(target); + if (scrollProxy) { + calculateBounds(); + startElementY = scrollProxy.top(); + startElementX = scrollProxy.left(); + } else { + //if the element is in the process of tweening, don't force snapping to occur because it could make it jump. Imagine the user throwing, then before it's done, clicking on the element in its inbetween state. + if (isTweening()) { + syncXY(true, true); + calculateBounds(); + } else { + self.applyBounds(); + } + if (rotationMode) { + parsedOrigin = target.ownerSVGElement ? [gsCache.xOrigin - target.getBBox().x, gsCache.yOrigin - target.getBBox().y] : (_getComputedStyle(target)[_transformOriginProp] || "0 0").split(" "); + rotationOrigin = self.rotationOrigin = getGlobalMatrix(target).apply({x: parseFloat(parsedOrigin[0]) || 0, y: parseFloat(parsedOrigin[1]) || 0}); + syncXY(true, true); + x = self.pointerX - rotationOrigin.x - offsetX; + y = rotationOrigin.y - self.pointerY + offsetY; + startElementX = self.x; //starting rotation (x always refers to rotation in type:"rotation", measured in degrees) + startElementY = self.y = Math.atan2(y, x) * _RAD2DEG; + } else { + //parent = !isFixed && target.parentNode; + //startScrollTop = parent ? parent.scrollTop || 0 : 0; + //startScrollLeft = parent ? parent.scrollLeft || 0 : 0; + startElementY = getPropAsNum(yProp, "px"); //record the starting top and left values so that we can just add the mouse's movement to them later. + startElementX = getPropAsNum(xProp, "px"); + } + } + + if (hasBounds && edgeTolerance) { + if (startElementX > maxX) { + startElementX = maxX + (startElementX - maxX) / edgeTolerance; + } else if (startElementX < minX) { + startElementX = minX - (minX - startElementX) / edgeTolerance; + } + if (!rotationMode) { + if (startElementY > maxY) { + startElementY = maxY + (startElementY - maxY) / edgeTolerance; + } else if (startElementY < minY) { + startElementY = minY - (minY - startElementY) / edgeTolerance; + } + } + } + self.startX = startElementX = _round(startElementX); + self.startY = startElementY = _round(startElementY); + }, + + isTweening = () => self.tween && self.tween.isActive(), + + removePlaceholder = () => { + if (_placeholderDiv.parentNode && !isTweening() && !self.isDragging) { //_placeholderDiv just props open auto-scrolling containers so they don't collapse as the user drags left/up. We remove it after dragging (and throwing, if necessary) finishes. + _placeholderDiv.parentNode.removeChild(_placeholderDiv); + } + }, + + //called when the mouse is pressed (or touch starts) + onPress = (e, force) => { + let i; + if (!enabled || self.isPressed || !e || ((e.type === "mousedown" || e.type === "pointerdown") && !force && _getTime() - clickTime < 30 && _touchEventLookup[self.pointerEvent.type])) { //when we DON'T preventDefault() in order to accommodate touch-scrolling and the user just taps, many browsers also fire a mousedown/mouseup sequence AFTER the touchstart/touchend sequence, thus it'd result in two quick "click" events being dispatched. This line senses that condition and halts it on the subsequent mousedown. + isPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchstart, pointerdown, mousedown. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary. + return; + } + interrupted = isTweening(); + dragged = false; // we need to track whether or not it was dragged in this interaction so that if, for example, the user calls .endDrag() to FORCE it to stop and then they keep the mouse pressed down and eventually release, that would normally cause an onClick but we have to skip it in that case if there was dragging that occurred. + self.pointerEvent = e; + if (_touchEventLookup[e.type]) { //note: on iOS, BOTH touchmove and mousemove are dispatched, but the mousemove has pageY and pageX of 0 which would mess up the calculations and needlessly hurt performance. + touchEventTarget = ~e.type.indexOf("touch") ? (e.currentTarget || e.target) : ownerDoc; //pointer-based touches (for Microsoft browsers) don't remain locked to the original target like other browsers, so we must use the document instead. The event type would be "MSPointerDown" or "pointerdown". + _addListener(touchEventTarget, "touchend", onRelease); + _addListener(touchEventTarget, "touchmove", onMove); // possible future change if PointerEvents are more standardized: https://developer.mozilla.org/en-US/docs/Web/API/Element/setPointerCapture + _addListener(touchEventTarget, "touchcancel", onRelease); + _addListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + touchEventTarget = null; + _addListener(ownerDoc, "mousemove", onMove); //attach these to the document instead of the box itself so that if the user's mouse moves too quickly (and off of the box), things still work. + } + touchDragAxis = null; + if (!_supportsPointer || !touchEventTarget) { + _addListener(ownerDoc, "mouseup", onRelease); + e && e.target && _addListener(e.target, "mouseup", onRelease); //we also have to listen directly on the element because some browsers don't bubble up the event to the _doc on elements with contentEditable="true" + } + isClicking = (isClickable.call(self, e.target) && vars.dragClickables === false && !force); + if (isClicking) { + _addListener(e.target, "change", onRelease); //in some browsers, when you mousedown on a <select> element, no mouseup gets dispatched! So we listen for a "change" event instead. + _dispatchEvent(self, "pressInit", "onPressInit"); + _dispatchEvent(self, "press", "onPress"); + _setSelectable(triggers, true); //accommodates things like inputs and elements with contentEditable="true" (otherwise user couldn't drag to select text) + isPreventingDefault = false; + return; + } + allowNativeTouchScrolling = (!touchEventTarget || allowX === allowY || self.vars.allowNativeTouchScrolling === false || (self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2))) ? false : allowX ? "y" : "x"; //note: in Chrome, right-clicking (for a context menu) fires onPress and it doesn't have the event.which set properly, so we must look for event.ctrlKey. If the user wants to allow context menus we should of course sense it here and not allow native touch scrolling. + isPreventingDefault = !allowNativeTouchScrolling && !self.allowEventDefault; + if (isPreventingDefault) { + _preventDefault(e); + _addListener(_win, "touchforcechange", _preventDefault); //works around safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/ + } + if (e.changedTouches) { //touch events store the data slightly differently + e = touch = e.changedTouches[0]; + touchID = e.identifier; + } else if (e.pointerId) { + touchID = e.pointerId; //for some Microsoft browsers + } else { + touch = touchID = null; + } + _dragCount++; + _addToRenderQueue(render); //causes the Draggable to render on each "tick" of gsap.ticker (performance optimization - updating values in a mousemove can cause them to happen too frequently, like multiple times between frame redraws which is wasteful, and it also prevents values from updating properly in IE8) + startPointerY = self.pointerY = e.pageY; //record the starting x and y so that we can calculate the movement from the original in _onMouseMove + startPointerX = self.pointerX = e.pageX; + _dispatchEvent(self, "pressInit", "onPressInit"); + if (allowNativeTouchScrolling || self.autoScroll) { + _recordMaxScrolls(target.parentNode); + } + if (target.parentNode && self.autoScroll && !scrollProxy && !rotationMode && target.parentNode._gsMaxScrollX && !_placeholderDiv.parentNode && !target.getBBox) { //add a placeholder div to prevent the parent container from collapsing when the user drags the element left. + _placeholderDiv.style.width = target.parentNode.scrollWidth + "px"; + target.parentNode.appendChild(_placeholderDiv); + } + recordStartPositions(); + self.tween && self.tween.kill(); + self.isThrowing = false; + gsap.killTweensOf(scrollProxy || target, killProps, true); //in case the user tries to drag it before the last tween is done. + scrollProxy && gsap.killTweensOf(target, {scrollTo:1}, true); //just in case the original target's scroll position is being tweened somewhere else. + self.tween = self.lockedAxis = null; + if (vars.zIndexBoost || (!rotationMode && !scrollProxy && vars.zIndexBoost !== false)) { + target.style.zIndex = Draggable.zIndex++; + } + self.isPressed = true; + hasDragCallback = !!(vars.onDrag || self._listeners.drag); + hasMoveCallback = !!(vars.onMove || self._listeners.move); + if (vars.cursor !== false || vars.activeCursor) { + i = triggers.length; + while (--i > -1) { + gsap.set(triggers[i], {cursor: vars.activeCursor || vars.cursor || (_defaultCursor === "grab" ? "grabbing" : _defaultCursor)}); + } + } + _dispatchEvent(self, "press", "onPress"); + }, + + //called every time the mouse/touch moves + onMove = e => { + let originalEvent = e, + touches, pointerX, pointerY, i, dx, dy; + if (!enabled || _isMultiTouching || !self.isPressed || !e) { + isPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchmove, pointermove, mousemove. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary. + return; + } + self.pointerEvent = e; + touches = e.changedTouches; + if (touches) { //touch events store the data slightly differently + e = touches[0]; + if (e !== touch && e.identifier !== touchID) { //Usually changedTouches[0] will be what we're looking for, but in case it's not, look through the rest of the array...(and Android browsers don't reuse the event like iOS) + i = touches.length; + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} // Some Android devices dispatch a touchstart AND pointerdown initially, and then only pointermove thus the touchID may not match because it was grabbed from the touchstart event whereas the pointer event is the one that the browser dispatches for move, so if the event target matches this Draggable's target, let it through. + if (i < 0) { + return; + } + } + } else if (e.pointerId && touchID && e.pointerId !== touchID) { //for some Microsoft browsers, we must attach the listener to the doc rather than the trigger so that when the finger moves outside the bounds of the trigger, things still work. So if the event we're receiving has a pointerId that doesn't match the touchID, ignore it (for multi-touch) + return; + } + + if (touchEventTarget && allowNativeTouchScrolling && !touchDragAxis) { //Android browsers force us to decide on the first "touchmove" event if we should allow the default (scrolling) behavior or preventDefault(). Otherwise, a "touchcancel" will be fired and then no "touchmove" or "touchend" will fire during the scrolling (no good). + _point1.x = e.pageX - (isFixed ? _getDocScrollLeft(ownerDoc) : 0); + _point1.y = e.pageY - (isFixed ? _getDocScrollTop(ownerDoc) : 0); + matrix && matrix.apply(_point1, _point1); + pointerX = _point1.x; + pointerY = _point1.y; + dx = Math.abs(pointerX - startPointerX); + dy = Math.abs(pointerY - startPointerY); + if ((dx !== dy && (dx > minimumMovement || dy > minimumMovement)) || (_isAndroid && allowNativeTouchScrolling === touchDragAxis)) { + touchDragAxis = (dx > dy && allowX) ? "x" : "y"; + if (allowNativeTouchScrolling && touchDragAxis !== allowNativeTouchScrolling) { + _addListener(_win, "touchforcechange", _preventDefault); // prevents native touch scrolling from taking over if the user started dragging in the other direction in iOS Safari + } + if (self.vars.lockAxisOnTouchScroll !== false && allowX && allowY) { + self.lockedAxis = (touchDragAxis === "x") ? "y" : "x"; + _isFunction(self.vars.onLockAxis) && self.vars.onLockAxis.call(self, originalEvent); + } + if (_isAndroid && allowNativeTouchScrolling === touchDragAxis) { + onRelease(originalEvent); + return; + } + } + } + if (!self.allowEventDefault && (!allowNativeTouchScrolling || (touchDragAxis && allowNativeTouchScrolling !== touchDragAxis)) && originalEvent.cancelable !== false) { + _preventDefault(originalEvent); + isPreventingDefault = true; + } else if (isPreventingDefault) { + isPreventingDefault = false; + } + + if (self.autoScroll) { + checkAutoScrollBounds = true; + } + setPointerPosition(e.pageX, e.pageY, hasMoveCallback); + }, + + setPointerPosition = (pointerX, pointerY, invokeOnMove) => { + let dragTolerance = 1 - self.dragResistance, + edgeTolerance = 1 - self.edgeResistance, + prevPointerX = self.pointerX, + prevPointerY = self.pointerY, + prevStartElementY = startElementY, + prevX = self.x, + prevY = self.y, + prevEndX = self.endX, + prevEndY = self.endY, + prevEndRotation = self.endRotation, + prevDirty = dirty, + xChange, yChange, x, y, dif, temp; + self.pointerX = pointerX; + self.pointerY = pointerY; + if (isFixed) { + pointerX -= _getDocScrollLeft(ownerDoc); + pointerY -= _getDocScrollTop(ownerDoc); + } + if (rotationMode) { + y = Math.atan2(rotationOrigin.y - pointerY, pointerX - rotationOrigin.x) * _RAD2DEG; + dif = self.y - y; + if (dif > 180) { + startElementY -= 360; + self.y = y; + } else if (dif < -180) { + startElementY += 360; + self.y = y; + } + if (self.x !== startElementX || Math.max(Math.abs(startPointerX - pointerX), Math.abs(startPointerY - pointerY)) > minimumMovement) { + self.y = y; + x = startElementX + (startElementY - y) * dragTolerance; + } else { + x = startElementX; + } + + } else { + if (matrix) { + temp = pointerX * matrix.a + pointerY * matrix.c + matrix.e; + pointerY = pointerX * matrix.b + pointerY * matrix.d + matrix.f; + pointerX = temp; + } + yChange = (pointerY - startPointerY); + xChange = (pointerX - startPointerX); + if (yChange < minimumMovement && yChange > -minimumMovement) { + yChange = 0; + } + if (xChange < minimumMovement && xChange > -minimumMovement) { + xChange = 0; + } + if ((self.lockAxis || self.lockedAxis) && (xChange || yChange)) { + temp = self.lockedAxis; + if (!temp) { + self.lockedAxis = temp = (allowX && Math.abs(xChange) > Math.abs(yChange)) ? "y" : allowY ? "x" : null; + if (temp && _isFunction(self.vars.onLockAxis)) { + self.vars.onLockAxis.call(self, self.pointerEvent); + } + } + if (temp === "y") { + yChange = 0; + } else if (temp === "x") { + xChange = 0; + } + } + x = _round(startElementX + xChange * dragTolerance); + y = _round(startElementY + yChange * dragTolerance); + } + + if ((snapX || snapY || snapXY) && (self.x !== x || (self.y !== y && !rotationMode))) { + if (snapXY) { + _temp1.x = x; + _temp1.y = y; + temp = snapXY(_temp1); + x = _round(temp.x); + y = _round(temp.y); + } + if (snapX) { + x = _round(snapX(x)); + } + if (snapY) { + y = _round(snapY(y)); + } + } + if (hasBounds) { + if (x > maxX) { + x = maxX + Math.round((x - maxX) * edgeTolerance); + } else if (x < minX) { + x = minX + Math.round((x - minX) * edgeTolerance); + } + if (!rotationMode) { + if (y > maxY) { + y = Math.round(maxY + (y - maxY) * edgeTolerance); + } else if (y < minY) { + y = Math.round(minY + (y - minY) * edgeTolerance); + } + } + } + if (self.x !== x || (self.y !== y && !rotationMode)) { + if (rotationMode) { + self.endRotation = self.x = self.endX = x; + dirty = true; + } else { + if (allowY) { + self.y = self.endY = y; + dirty = true; //a flag that indicates we need to render the target next time the TweenLite.ticker dispatches a "tick" event (typically on a requestAnimationFrame) - this is a performance optimization (we shouldn't render on every move because sometimes many move events can get dispatched between screen refreshes, and that'd be wasteful to render every time) + } + if (allowX) { + self.x = self.endX = x; + dirty = true; + } + } + if (!invokeOnMove || _dispatchEvent(self, "move", "onMove") !== false) { + if (!self.isDragging && self.isPressed) { + self.isDragging = dragged = true; + _dispatchEvent(self, "dragstart", "onDragStart"); + } + } else { //revert because the onMove returned false! + self.pointerX = prevPointerX; + self.pointerY = prevPointerY; + startElementY = prevStartElementY; + self.x = prevX; + self.y = prevY; + self.endX = prevEndX; + self.endY = prevEndY; + self.endRotation = prevEndRotation; + dirty = prevDirty; + } + } + }, + + //called when the mouse/touch is released + onRelease = (e, force) => { + if (!enabled || !self.isPressed || (e && touchID != null && !force && ((e.pointerId && e.pointerId !== touchID && e.target !== target) || (e.changedTouches && !_hasTouchID(e.changedTouches, touchID))))) { //for some Microsoft browsers, we must attach the listener to the doc rather than the trigger so that when the finger moves outside the bounds of the trigger, things still work. So if the event we're receiving has a pointerId that doesn't match the touchID, ignore it (for multi-touch) + isPreventingDefault && e && enabled && _preventDefault(e); // in some browsers, we must listen for multiple event types like touchend, pointerup, mouseup. The first time this function is called, we record whether or not we _preventDefault() so that on duplicate calls, we can do the same if necessary. + return; + } + self.isPressed = false; + let originalEvent = e, + wasDragging = self.isDragging, + isContextMenuRelease = (self.vars.allowContextMenu && e && (e.ctrlKey || e.which > 2)), + placeholderDelayedCall = gsap.delayedCall(0.001, removePlaceholder), + touches, i, syntheticEvent, eventTarget, syntheticClick; + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchend", onRelease); + _removeListener(touchEventTarget, "touchmove", onMove); + _removeListener(touchEventTarget, "touchcancel", onRelease); + _removeListener(ownerDoc, "touchstart", _onMultiTouchDocument); + } else { + _removeListener(ownerDoc, "mousemove", onMove); + } + _removeListener(_win, "touchforcechange", _preventDefault); + if (!_supportsPointer || !touchEventTarget) { + _removeListener(ownerDoc, "mouseup", onRelease); + e && e.target && _removeListener(e.target, "mouseup", onRelease); + } + dirty = false; + if (wasDragging) { + dragEndTime = _lastDragTime = _getTime(); + self.isDragging = false; + } + _removeFromRenderQueue(render); + if (isClicking && !isContextMenuRelease) { + if (e) { + _removeListener(e.target, "change", onRelease); + self.pointerEvent = originalEvent; + } + _setSelectable(triggers, false); + _dispatchEvent(self, "release", "onRelease"); + _dispatchEvent(self, "click", "onClick"); + isClicking = false; + return; + } + i = triggers.length; + while (--i > -1) { + _setStyle(triggers[i], "cursor", vars.cursor || (vars.cursor !== false ? _defaultCursor : null)); + } + _dragCount--; + if (e) { + touches = e.changedTouches; + if (touches) { //touch events store the data slightly differently + e = touches[0]; + if (e !== touch && e.identifier !== touchID) { //Usually changedTouches[0] will be what we're looking for, but in case it's not, look through the rest of the array...(and Android browsers don't reuse the event like iOS) + i = touches.length; + while (--i > -1 && (e = touches[i]).identifier !== touchID && e.target !== target) {} + if (i < 0 && !force) { + return; + } + } + } + self.pointerEvent = originalEvent; + self.pointerX = e.pageX; + self.pointerY = e.pageY; + } + if (isContextMenuRelease && originalEvent) { + _preventDefault(originalEvent); + isPreventingDefault = true; + _dispatchEvent(self, "release", "onRelease"); + } else if (originalEvent && !wasDragging) { + isPreventingDefault = false; + if (interrupted && (vars.snap || vars.bounds)) { //otherwise, if the user clicks on the object while it's animating to a snapped position, and then releases without moving 3 pixels, it will just stay there (it should animate/snap) + animate(vars.inertia || vars.throwProps); + } + _dispatchEvent(self, "release", "onRelease"); + if ((!_isAndroid || originalEvent.type !== "touchmove") && originalEvent.type.indexOf("cancel") === -1) { //to accommodate native scrolling on Android devices, we have to immediately call onRelease() on the first touchmove event, but that shouldn't trigger a "click". + _dispatchEvent(self, "click", "onClick"); + if (_getTime() - clickTime < 300) { + _dispatchEvent(self, "doubleclick", "onDoubleClick"); + } + eventTarget = originalEvent.target || target; //old IE uses srcElement + clickTime = _getTime(); + syntheticClick = () => { // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular "click" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the "real"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the "real" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched. + if (clickTime !== clickDispatch && self.enabled() && !self.isPressed && !originalEvent.defaultPrevented) { + if (eventTarget.click) { //some browsers (like mobile Safari) don't properly trigger the click event + eventTarget.click(); + } else if (ownerDoc.createEvent) { + syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win, 1, self.pointerEvent.screenX, self.pointerEvent.screenY, self.pointerX, self.pointerY, false, false, false, false, 0, null); + eventTarget.dispatchEvent(syntheticEvent); + } + } + }; + if (!_isAndroid && !originalEvent.defaultPrevented) { //iOS Safari requires the synthetic click to happen immediately or else it simply won't work, but Android doesn't play nice. + gsap.delayedCall(0.05, syntheticClick); //in addition to the iOS bug workaround, there's a Firefox issue with clicking on things like a video to play, so we must fake a click event in a slightly delayed fashion. Previously, we listened for the "click" event with "capture" false which solved the video-click-to-play issue, but it would allow the "click" event to be dispatched twice like if you were using a jQuery.click() because that was handled in the capture phase, thus we had to switch to the capture phase to avoid the double-dispatching, but do the delayed synthetic click. Don't fire it too fast (like 0.00001) because we want to give the native event a chance to fire first as it's "trusted". + } + } + } else { + animate(vars.inertia || vars.throwProps); //will skip if inertia/throwProps isn't defined or InertiaPlugin isn't loaded. + if (!self.allowEventDefault && originalEvent && (vars.dragClickables !== false || !isClickable.call(self, originalEvent.target)) && wasDragging && (!allowNativeTouchScrolling || (touchDragAxis && allowNativeTouchScrolling === touchDragAxis)) && originalEvent.cancelable !== false) { + isPreventingDefault = true; + _preventDefault(originalEvent); + } else { + isPreventingDefault = false; + } + _dispatchEvent(self, "release", "onRelease"); + } + isTweening() && placeholderDelayedCall.duration( self.tween.duration() ); //sync the timing so that the placeholder DIV gets + wasDragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return true; + }, + + updateScroll = e => { + if (e && self.isDragging && !scrollProxy) { + let parent = e.target || target.parentNode, + deltaX = parent.scrollLeft - parent._gsScrollX, + deltaY = parent.scrollTop - parent._gsScrollY; + if (deltaX || deltaY) { + if (matrix) { + startPointerX -= deltaX * matrix.a + deltaY * matrix.c; + startPointerY -= deltaY * matrix.d + deltaX * matrix.b; + } else { + startPointerX -= deltaX; + startPointerY -= deltaY; + } + parent._gsScrollX += deltaX; + parent._gsScrollY += deltaY; + setPointerPosition(self.pointerX, self.pointerY); + } + } + }, + + onClick = e => { //this was a huge pain in the neck to align all the various browsers and their behaviors. Chrome, Firefox, Safari, Opera, Android, and Microsoft Edge all handle events differently! Some will only trigger native behavior (like checkbox toggling) from trusted events. Others don't even support isTrusted, but require 2 events to flow through before triggering native behavior. Edge treats everything as trusted but also mandates that 2 flow through to trigger the correct native behavior. + let time = _getTime(), + recentlyClicked = (time - clickTime < 100), + recentlyDragged = (time - dragEndTime < 50), + alreadyDispatched = (recentlyClicked && clickDispatch === clickTime), + defaultPrevented = (self.pointerEvent && self.pointerEvent.defaultPrevented), + alreadyDispatchedTrusted = (recentlyClicked && trustedClickDispatch === clickTime), + trusted = e.isTrusted || (e.isTrusted == null && recentlyClicked && alreadyDispatched); //note: Safari doesn't support isTrusted, and it won't properly execute native behavior (like toggling checkboxes) on the first synthetic "click" event - we must wait for the 2nd and treat it as trusted (but stop propagation at that point). Confusing, I know. Don't you love cross-browser compatibility challenges? + if ((alreadyDispatched || (recentlyDragged && self.vars.suppressClickOnDrag !== false) ) && e.stopImmediatePropagation) { + e.stopImmediatePropagation(); + } + if (recentlyClicked && !(self.pointerEvent && self.pointerEvent.defaultPrevented) && (!alreadyDispatched || (trusted && !alreadyDispatchedTrusted))) { //let the first click pass through unhindered. Let the next one only if it's trusted, then no more (stop quick-succession ones) + if (trusted && alreadyDispatched) { + trustedClickDispatch = clickTime; + } + clickDispatch = clickTime; + return; + } + if (self.isPressed || recentlyDragged || recentlyClicked) { + if (!trusted || !e.detail || !recentlyClicked || defaultPrevented) { + _preventDefault(e); + } + } + if (!recentlyClicked && !recentlyDragged && !dragged) { // for script-triggered event dispatches, like element.click() + e && e.target && (self.pointerEvent = e); + _dispatchEvent(self, "click", "onClick"); + } + }, + + localizePoint = p => matrix ? {x:p.x * matrix.a + p.y * matrix.c + matrix.e, y:p.x * matrix.b + p.y * matrix.d + matrix.f} : {x:p.x, y:p.y}; + + old = Draggable.get(target); + old && old.kill(); // avoids duplicates (an element can only be controlled by one Draggable) + + //give the user access to start/stop dragging... + this.startDrag = (event, align) => { + let r1, r2, p1, p2; + onPress(event || self.pointerEvent, true); + //if the pointer isn't on top of the element, adjust things accordingly + if (align && !self.hitTest(event || self.pointerEvent)) { + r1 = _parseRect(event || self.pointerEvent); + r2 = _parseRect(target); + p1 = localizePoint({x:r1.left + r1.width / 2, y:r1.top + r1.height / 2}); + p2 = localizePoint({x:r2.left + r2.width / 2, y:r2.top + r2.height / 2}); + startPointerX -= p1.x - p2.x; + startPointerY -= p1.y - p2.y; + } + if (!self.isDragging) { + self.isDragging = dragged = true; + _dispatchEvent(self, "dragstart", "onDragStart"); + } + }; + this.drag = onMove; + this.endDrag = e => onRelease(e || self.pointerEvent, true); + this.timeSinceDrag = () => self.isDragging ? 0 : (_getTime() - dragEndTime) / 1000; + this.timeSinceClick = () => (_getTime() - clickTime) / 1000; + this.hitTest = (target, threshold) => Draggable.hitTest(self.target, target, threshold); + + this.getDirection = (from, diagonalThreshold) => { //from can be "start" (default), "velocity", or an element + let mode = (from === "velocity" && InertiaPlugin) ? from : (_isObject(from) && !rotationMode) ? "element" : "start", + xChange, yChange, ratio, direction, r1, r2; + if (mode === "element") { + r1 = _parseRect(self.target); + r2 = _parseRect(from); + } + xChange = (mode === "start") ? self.x - startElementX : (mode === "velocity") ? InertiaPlugin.getVelocity(target, xProp) : (r1.left + r1.width / 2) - (r2.left + r2.width / 2); + if (rotationMode) { + return xChange < 0 ? "counter-clockwise" : "clockwise"; + } else { + diagonalThreshold = diagonalThreshold || 2; + yChange = (mode === "start") ? self.y - startElementY : (mode === "velocity") ? InertiaPlugin.getVelocity(target, yProp) : (r1.top + r1.height / 2) - (r2.top + r2.height / 2); + ratio = Math.abs(xChange / yChange); + direction = (ratio < 1 / diagonalThreshold) ? "" : (xChange < 0) ? "left" : "right"; + if (ratio < diagonalThreshold) { + if (direction !== "") { + direction += "-"; + } + direction += (yChange < 0) ? "up" : "down"; + } + } + return direction; + }; + + this.applyBounds = (newBounds, sticky) => { + let x, y, forceZeroVelocity, e, parent, isRoot; + if (newBounds && vars.bounds !== newBounds) { + vars.bounds = newBounds; + return self.update(true, sticky); + } + syncXY(true); + calculateBounds(); + if (hasBounds && !isTweening()) { + x = self.x; + y = self.y; + if (x > maxX) { + x = maxX; + } else if (x < minX) { + x = minX; + } + if (y > maxY) { + y = maxY; + } else if (y < minY) { + y = minY; + } + if (self.x !== x || self.y !== y) { + forceZeroVelocity = true; + self.x = self.endX = x; + if (rotationMode) { + self.endRotation = x; + } else { + self.y = self.endY = y; + } + dirty = true; + render(true); + if (self.autoScroll && !self.isDragging) { + _recordMaxScrolls(target.parentNode); + e = target; + _windowProxy.scrollTop = ((_win.pageYOffset != null) ? _win.pageYOffset : (ownerDoc.documentElement.scrollTop != null) ? ownerDoc.documentElement.scrollTop : ownerDoc.body.scrollTop); + _windowProxy.scrollLeft = ((_win.pageXOffset != null) ? _win.pageXOffset : (ownerDoc.documentElement.scrollLeft != null) ? ownerDoc.documentElement.scrollLeft : ownerDoc.body.scrollLeft); + while (e && !isRoot) { //walk up the chain and sense wherever the scrollTop/scrollLeft exceeds the maximum. + isRoot = _isRoot(e.parentNode); + parent = isRoot ? _windowProxy : e.parentNode; + if (allowY && parent.scrollTop > parent._gsMaxScrollY) { + parent.scrollTop = parent._gsMaxScrollY; + } + if (allowX && parent.scrollLeft > parent._gsMaxScrollX) { + parent.scrollLeft = parent._gsMaxScrollX; + } + e = parent; + } + } + } + if (self.isThrowing && (forceZeroVelocity || self.endX > maxX || self.endX < minX || self.endY > maxY || self.endY < minY)) { + animate(vars.inertia || vars.throwProps, forceZeroVelocity); + } + } + return self; + }; + + this.update = (applyBounds, sticky, ignoreExternalChanges) => { + if (sticky && self.isPressed) { // in case the element was repositioned in the document flow, thus its x/y may be identical but its position is actually quite different. + let m = getGlobalMatrix(target), + p = innerMatrix.apply({x: self.x - startElementX, y: self.y - startElementY}), + m2 = getGlobalMatrix(target.parentNode, true); + m2.apply({x: m.e - p.x, y: m.f - p.y}, p); + self.x -= p.x - m2.e; + self.y -= p.y - m2.f; + render(true); + recordStartPositions(); + } + let { x, y } = self; + updateMatrix(!sticky); + if (applyBounds) { + self.applyBounds(); + } else { + dirty && ignoreExternalChanges && render(true); + syncXY(true); + } + if (sticky) { + setPointerPosition(self.pointerX, self.pointerY); + dirty && render(true); + } + if (self.isPressed && !sticky && ((allowX && Math.abs(x - self.x) > 0.01) || (allowY && (Math.abs(y - self.y) > 0.01 && !rotationMode)))) { + recordStartPositions(); + } + if (self.autoScroll) { + _recordMaxScrolls(target.parentNode, self.isDragging); + checkAutoScrollBounds = self.isDragging; + render(true); + //in case reparenting occurred. + _removeScrollListener(target, updateScroll); + _addScrollListener(target, updateScroll); + } + return self; + }; + + this.enable = type => { + let setVars = {lazy: true}, + id, i, trigger; + if (vars.cursor !== false) { + setVars.cursor = vars.cursor || _defaultCursor; + } + if (gsap.utils.checkPrefix("touchCallout")) { + setVars.touchCallout = "none"; + } + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, (allowX === allowY) ? "none" : (vars.allowNativeTouchScrolling && (target.scrollHeight === target.clientHeight) === (target.scrollWidth === target.clientHeight)) || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x"); // Some browsers like Internet Explorer will fire a pointercancel event when the user attempts to drag when touchAction is "manipulate" because it's perceived as a pan. If the element has scrollable content in only one direction, we should use pan-x or pan-y accordingly so that the pointercancel doesn't prevent dragging. + i = triggers.length; + while (--i > -1) { + trigger = triggers[i]; + _supportsPointer || _addListener(trigger, "mousedown", onPress); + _addListener(trigger, "touchstart", onPress); + _addListener(trigger, "click", onClick, true); // note: used to pass true for capture but it prevented click-to-play-video functionality in Firefox. + gsap.set(trigger, setVars); + if (trigger.getBBox && trigger.ownerSVGElement && allowX !== allowY) { // a bug in chrome doesn't respect touch-action on SVG elements - it only works if we set it on the parent SVG. + gsap.set(trigger.ownerSVGElement, {touchAction: vars.allowNativeTouchScrolling || vars.allowEventDefault ? "manipulation" : allowX ? "pan-y" : "pan-x"}); + } + vars.allowContextMenu || _addListener(trigger, "contextmenu", onContextMenu); + } + _setSelectable(triggers, false); + } + _addScrollListener(target, updateScroll); + enabled = true; + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.track(scrollProxy || target, (xyMode ? "x,y" : rotationMode ? "rotation" : "top,left")); + } + target._gsDragID = id = target._gsDragID || ("d" + (_lookupCount++)); + _lookup[id] = self; + if (scrollProxy) { + scrollProxy.enable(); + scrollProxy.element._gsDragID = id; + } + (vars.bounds || rotationMode) && recordStartPositions(); + vars.bounds && self.applyBounds(); + return self; + }; + + this.disable = type => { + let dragging = self.isDragging, + i = triggers.length, + trigger; + while (--i > -1) { + _setStyle(triggers[i], "cursor", null); + } + if (type !== "soft") { + _setTouchActionForAllDescendants(triggers, null); + i = triggers.length; + while (--i > -1) { + trigger = triggers[i]; + _setStyle(trigger, "touchCallout", null); + _removeListener(trigger, "mousedown", onPress); + _removeListener(trigger, "touchstart", onPress); + _removeListener(trigger, "click", onClick, true); + _removeListener(trigger, "contextmenu", onContextMenu); + } + _setSelectable(triggers, true); + if (touchEventTarget) { + _removeListener(touchEventTarget, "touchcancel", onRelease); + _removeListener(touchEventTarget, "touchend", onRelease); + _removeListener(touchEventTarget, "touchmove", onMove); + } + _removeListener(ownerDoc, "mouseup", onRelease); + _removeListener(ownerDoc, "mousemove", onMove); + } + _removeScrollListener(target, updateScroll); + enabled = false; + if (InertiaPlugin && type !== "soft") { + InertiaPlugin.untrack(scrollProxy || target, (xyMode ? "x,y" : rotationMode ? "rotation" : "top,left")); + self.tween && self.tween.kill(); + } + scrollProxy && scrollProxy.disable(); + _removeFromRenderQueue(render); + self.isDragging = self.isPressed = isClicking = false; + dragging && _dispatchEvent(self, "dragend", "onDragEnd"); + return self; + }; + + this.enabled = function(value, type) { + return arguments.length ? (value ? self.enable(type) : self.disable(type)) : enabled; + }; + + this.kill = function() { + self.isThrowing = false; + self.tween && self.tween.kill(); + self.disable(); + gsap.set(triggers, {clearProps:"userSelect"}); + delete _lookup[target._gsDragID]; + return self; + }; + + this.revert = function() { + this.kill(); + this.styles && this.styles.revert(); + }; + + if (~type.indexOf("scroll")) { + scrollProxy = this.scrollProxy = new ScrollProxy(target, _extend({onKill:function() { //ScrollProxy's onKill() gets called if/when the ScrollProxy senses that the user interacted with the scroll position manually (like using the scrollbar). IE9 doesn't fire the "mouseup" properly when users drag the scrollbar of an element, so this works around that issue. + self.isPressed && onRelease(null); + }}, vars)); + //a bug in many Android devices' stock browser causes scrollTop to get forced back to 0 after it is altered via JS, so we set overflow to "hidden" on mobile/touch devices (they hide the scroll bar anyway). That works around the bug. (This bug is discussed at https://code.google.com/p/android/issues/detail?id=19625) + target.style.overflowY = (allowY && !_isTouchDevice) ? "auto" : "hidden"; + target.style.overflowX = (allowX && !_isTouchDevice) ? "auto" : "hidden"; + target = scrollProxy.content; + } + + if (rotationMode) { + killProps.rotation = 1; + } else { + if (allowX) { + killProps[xProp] = 1; + } + if (allowY) { + killProps[yProp] = 1; + } + } + + gsCache.force3D = ("force3D" in vars) ? vars.force3D : true; //otherwise, normal dragging would be in 2D and then as soon as it's released and there's an inertia tween, it'd jump to 3D which can create an initial jump due to the work the browser must to do layerize it. + + _context(this); + this.enable(); + } + + + + + static register(core) { + gsap = core; + _initCore(); + } + + static create(targets, vars) { + _coreInitted || _initCore(true); + return _toArray(targets).map(target => new Draggable(target, vars)); + } + + static get(target) { + return _lookup[(_toArray(target)[0] || {})._gsDragID]; + } + + static timeSinceDrag() { + return (_getTime() - _lastDragTime) / 1000; + } + + static hitTest(obj1, obj2, threshold) { + if (obj1 === obj2) { + return false; + } + let r1 = _parseRect(obj1), + r2 = _parseRect(obj2), + { top, left, right, bottom, width, height } = r1, + isOutside = (r2.left > right || r2.right < left || r2.top > bottom || r2.bottom < top), + overlap, area, isRatio; + if (isOutside || !threshold) { + return !isOutside; + } + isRatio = ((threshold + "").indexOf("%") !== -1); + threshold = parseFloat(threshold) || 0; + overlap = {left: Math.max(left, r2.left), top: Math.max(top, r2.top)}; + overlap.width = Math.min(right, r2.right) - overlap.left; + overlap.height = Math.min(bottom, r2.bottom) - overlap.top; + if (overlap.width < 0 || overlap.height < 0) { + return false; + } + if (isRatio) { + threshold *= 0.01; + area = overlap.width * overlap.height; + return (area >= width * height * threshold || area >= r2.width * r2.height * threshold); + } + return (overlap.width > threshold && overlap.height > threshold); + } + +} + +_setDefaults(Draggable.prototype, {pointerX:0, pointerY: 0, startX: 0, startY: 0, deltaX: 0, deltaY: 0, isDragging: false, isPressed: false}); + +Draggable.zIndex = 1000; +Draggable.version = "3.12.7"; + +_getGSAP() && gsap.registerPlugin(Draggable); + +export { Draggable as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/EasePack.js b/node_modules/gsap/src/EasePack.js new file mode 100644 index 0000000000000000000000000000000000000000..0aca6d45d3726830764502b7e77fe25d7995d7e0 --- /dev/null +++ b/node_modules/gsap/src/EasePack.js @@ -0,0 +1,163 @@ +/*! + * EasePack 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let gsap, _coreInitted, _registerEase, + _getGSAP = () => gsap || (typeof(window) !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _boolean = (value, defaultValue) => !!(typeof(value) === "undefined" ? defaultValue : value && !~((value + "").indexOf("false"))), + _initCore = core => { + gsap = core || _getGSAP(); + if (gsap) { + _registerEase = gsap.registerEase; + //add weighted ease capabilities to standard eases so users can do "power2.inOut(0.8)" for example to push everything toward the "out", or (-0.8) to push it toward the "in" (0 is neutral) + let eases = gsap.parseEase(), + createConfig = ease => ratio => { + let y = 0.5 + ratio / 2; + ease.config = p => ease(2 * (1 - p) * p * y + p * p); + }, + p; + for (p in eases) { + if (!eases[p].config) { + createConfig(eases[p]); + } + } + _registerEase("slow", SlowMo); + _registerEase("expoScale", ExpoScaleEase); + _registerEase("rough", RoughEase); + for (p in EasePack) { + p !== "version" && gsap.core.globals(p, EasePack[p]); + } + _coreInitted = 1; + } + }, + _createSlowMo = (linearRatio, power, yoyoMode) => { + linearRatio = Math.min(1, linearRatio || 0.7); + let pow = linearRatio < 1 ? ((power || power === 0) ? power : 0.7) : 0, + p1 = (1 - linearRatio) / 2, + p3 = p1 + linearRatio, + calcEnd = _boolean(yoyoMode); + return p => { + let r = p + (0.5 - p) * pow; + return (p < p1) ? (calcEnd ? 1 - ((p = 1 - (p / p1)) * p) : r - ((p = 1 - (p / p1)) * p * p * p * r)) : (p > p3) ? (calcEnd ? (p === 1 ? 0 : 1 - (p = (p - p3) / p1) * p) : r + ((p - r) * (p = (p - p3) / p1) * p * p * p)) : (calcEnd ? 1 : r); + } + }, + _createExpoScale = (start, end, ease) => { + let p1 = Math.log(end / start), + p2 = end - start; + ease && (ease = gsap.parseEase(ease)); + return p => (start * Math.exp(p1 * (ease ? ease(p) : p)) - start) / p2; + }, + EasePoint = function(time, value, next) { + this.t = time; + this.v = value; + if (next) { + this.next = next; + next.prev = this; + this.c = next.v - value; + this.gap = next.t - time; + } + }, + _createRoughEase = vars => { + if (typeof(vars) !== "object") { //users may pass in via a string, like "rough(30)" + vars = {points: +vars || 20}; + } + let taper = vars.taper || "none", + a = [], + cnt = 0, + points = (+vars.points || 20) | 0, + i = points, + randomize = _boolean(vars.randomize, true), + clamp = _boolean(vars.clamp), + template = gsap ? gsap.parseEase(vars.template) : 0, + strength = (+vars.strength || 1) * 0.4, + x, y, bump, invX, obj, pnt, recent; + while (--i > -1) { + x = randomize ? Math.random() : (1 / points) * i; + y = template ? template(x) : x; + if (taper === "none") { + bump = strength; + } else if (taper === "out") { + invX = 1 - x; + bump = invX * invX * strength; + } else if (taper === "in") { + bump = x * x * strength; + } else if (x < 0.5) { //"both" (start) + invX = x * 2; + bump = invX * invX * 0.5 * strength; + } else { //"both" (end) + invX = (1 - x) * 2; + bump = invX * invX * 0.5 * strength; + } + if (randomize) { + y += (Math.random() * bump) - (bump * 0.5); + } else if (i % 2) { + y += bump * 0.5; + } else { + y -= bump * 0.5; + } + if (clamp) { + if (y > 1) { + y = 1; + } else if (y < 0) { + y = 0; + } + } + a[cnt++] = {x:x, y:y}; + } + a.sort((a, b) => a.x - b.x); + pnt = new EasePoint(1, 1, null); + i = points; + while (i--) { + obj = a[i]; + pnt = new EasePoint(obj.x, obj.y, pnt); + } + recent = new EasePoint(0, 0, pnt.t ? pnt : pnt.next); + return p => { + let pnt = recent; + if (p > pnt.t) { + while (pnt.next && p >= pnt.t) { + pnt = pnt.next; + } + pnt = pnt.prev; + } else { + while (pnt.prev && p <= pnt.t) { + pnt = pnt.prev; + } + } + recent = pnt; + return pnt.v + ((p - pnt.t) / pnt.gap) * pnt.c; + }; + }; + +export const SlowMo = _createSlowMo(0.7); +SlowMo.ease = SlowMo; //for backward compatibility +SlowMo.config = _createSlowMo; + +export const ExpoScaleEase = _createExpoScale(1, 2); +ExpoScaleEase.config = _createExpoScale; + +export const RoughEase = _createRoughEase(); +RoughEase.ease = RoughEase; //for backward compatibility +RoughEase.config = _createRoughEase; + +export const EasePack = { + SlowMo: SlowMo, + RoughEase: RoughEase, + ExpoScaleEase: ExpoScaleEase +}; + +for (let p in EasePack) { + EasePack[p].register = _initCore; + EasePack[p].version = "3.12.7"; +} + +_getGSAP() && gsap.registerPlugin(SlowMo); + +export { EasePack as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/EaselPlugin.js b/node_modules/gsap/src/EaselPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..c2ab93ed8e272e5fe319402bb084675ec3126931 --- /dev/null +++ b/node_modules/gsap/src/EaselPlugin.js @@ -0,0 +1,282 @@ +/*! + * EaselPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let gsap, _coreInitted, _win, _createJS, _ColorFilter, _ColorMatrixFilter, + _colorProps = "redMultiplier,greenMultiplier,blueMultiplier,alphaMultiplier,redOffset,greenOffset,blueOffset,alphaOffset".split(","), + _windowExists = () => typeof(window) !== "undefined", + _getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _getCreateJS = () => _createJS || (_win && _win.createjs) || _win || {}, + _warn = message => console.warn(message), + _cache = target => { + let b = target.getBounds && target.getBounds(); + if (!b) { + b = target.nominalBounds || {x:0, y:0, width: 100, height: 100}; + target.setBounds && target.setBounds(b.x, b.y, b.width, b.height); + } + target.cache && target.cache(b.x, b.y, b.width, b.height); + _warn("EaselPlugin: for filters to display in EaselJS, you must call the object's cache() method first. GSAP attempted to use the target's getBounds() for the cache but that may not be completely accurate. " + target); + }, + _parseColorFilter = (target, v, plugin) => { + if (!_ColorFilter) { + _ColorFilter = _getCreateJS().ColorFilter; + if (!_ColorFilter) { + _warn("EaselPlugin error: The EaselJS ColorFilter JavaScript file wasn't loaded."); + } + } + let filters = target.filters || [], + i = filters.length, + c, s, e, a, p, pt; + while (i--) { + if (filters[i] instanceof _ColorFilter) { + s = filters[i]; + break; + } + } + if (!s) { + s = new _ColorFilter(); + filters.push(s); + target.filters = filters; + } + e = s.clone(); + if (v.tint != null) { + c = gsap.utils.splitColor(v.tint); + a = (v.tintAmount != null) ? +v.tintAmount : 1; + e.redOffset = +c[0] * a; + e.greenOffset = +c[1] * a; + e.blueOffset = +c[2] * a; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - a; + } else { + for (p in v) { + if (p !== "exposure") if (p !== "brightness") { + e[p] = +v[p]; + } + } + } + if (v.exposure != null) { + e.redOffset = e.greenOffset = e.blueOffset = 255 * (+v.exposure - 1); + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1; + } else if (v.brightness != null) { + a = +v.brightness - 1; + e.redOffset = e.greenOffset = e.blueOffset = (a > 0) ? a * 255 : 0; + e.redMultiplier = e.greenMultiplier = e.blueMultiplier = 1 - Math.abs(a); + } + i = 8; + while (i--) { + p = _colorProps[i]; + if (s[p] !== e[p]) { + pt = plugin.add(s, p, s[p], e[p], 0, 0, 0, 0, 0, 1); + if (pt) { + pt.op = "easel_colorFilter"; + } + } + } + plugin._props.push("easel_colorFilter"); + if (!target.cacheID) { + _cache(target); + } + }, + + _idMatrix = [1,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,0,1,0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + + _applyMatrix = (m, m2) => { + if (!(m instanceof Array) || !(m2 instanceof Array)) { + return m2; + } + let temp = [], + i = 0, + z = 0, + y, x; + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = (x === 4) ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i+1] * m2[x + 5] + m[i+2] * m2[x + 10] + m[i+3] * m2[x + 15] + z; + } + i += 5; + } + return temp; + }, + + _setSaturation = (m, n) => { + if (isNaN(n)) { + return m; + } + let inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); + }, + + _colorize = (m, color, amount) => { + if (isNaN(amount)) { + amount = 1; + } + let c = gsap.utils.splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); + }, + + _setHue = (m, n) => { + if (isNaN(n)) { + return m; + } + n *= Math.PI / 180; + let c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([(_lumR + (c * (1 - _lumR))) + (s * (-_lumR)), (_lumG + (c * (-_lumG))) + (s * (-_lumG)), (_lumB + (c * (-_lumB))) + (s * (1 - _lumB)), 0, 0, (_lumR + (c * (-_lumR))) + (s * 0.143), (_lumG + (c * (1 - _lumG))) + (s * 0.14), (_lumB + (c * (-_lumB))) + (s * -0.283), 0, 0, (_lumR + (c * (-_lumR))) + (s * (-(1 - _lumR))), (_lumG + (c * (-_lumG))) + (s * _lumG), (_lumB + (c * (1 - _lumB))) + (s * _lumB), 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); + }, + + _setContrast = (m, n) => { + if (isNaN(n)) { + return m; + } + n += 0.01; + return _applyMatrix([n,0,0,0,128 * (1 - n), 0,n,0,0,128 * (1 - n), 0,0,n,0,128 * (1 - n), 0,0,0,1,0], m); + }, + + _parseColorMatrixFilter = (target, v, plugin) => { + if (!_ColorMatrixFilter) { + _ColorMatrixFilter = _getCreateJS().ColorMatrixFilter; + if (!_ColorMatrixFilter) { + _warn("EaselPlugin: The EaselJS ColorMatrixFilter JavaScript file wasn't loaded."); + } + } + let filters = target.filters || [], + i = filters.length, + matrix, startMatrix, s, pg; + while (--i > -1) { + if (filters[i] instanceof _ColorMatrixFilter) { + s = filters[i]; + break; + } + } + if (!s) { + s = new _ColorMatrixFilter(_idMatrix.slice()); + filters.push(s); + target.filters = filters; + } + startMatrix = s.matrix; + matrix = _idMatrix.slice(); + if (v.colorize != null) { + matrix = _colorize(matrix, v.colorize, Number(v.colorizeAmount)); + } + if (v.contrast != null) { + matrix = _setContrast(matrix, Number(v.contrast)); + } + if (v.hue != null) { + matrix = _setHue(matrix, Number(v.hue)); + } + if (v.saturation != null) { + matrix = _setSaturation(matrix, Number(v.saturation)); + } + + i = matrix.length; + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg = plugin.add(startMatrix, i, startMatrix[i], matrix[i], 0, 0, 0, 0, 0, 1); + if (pg) { + pg.op = "easel_colorMatrixFilter"; + } + } + } + + plugin._props.push("easel_colorMatrixFilter"); + if (!target.cacheID) { + _cache(); + } + + plugin._matrix = startMatrix; + }, + + _initCore = core => { + gsap = core || _getGSAP(); + if (_windowExists()) { + _win = window; + } + if (gsap) { + + _coreInitted = 1; + } + }; + + +export const EaselPlugin = { + version: "3.12.7", + name: "easel", + init(target, value, tween, index, targets) { + if (!_coreInitted) { + _initCore(); + if (!gsap) { + _warn("Please gsap.registerPlugin(EaselPlugin)"); + } + } + this.target = target; + let p, pt, tint, colorMatrix, end, labels, i; + for (p in value) { + + end = value[p]; + if (p === "colorFilter" || p === "tint" || p === "tintAmount" || p === "exposure" || p === "brightness") { + if (!tint) { + _parseColorFilter(target, value.colorFilter || value, this); + tint = true; + } + + } else if (p === "saturation" || p === "contrast" || p === "hue" || p === "colorize" || p === "colorizeAmount") { + if (!colorMatrix) { + _parseColorMatrixFilter(target, value.colorMatrixFilter || value, this); + colorMatrix = true; + } + + } else if (p === "frame") { + if (typeof(end) === "string" && end.charAt(1) !== "=" && (labels = target.labels)) { + for (i = 0; i < labels.length; i++) { + if (labels[i].label === end) { + end = labels[i].position; + } + } + } + pt = this.add(target, "gotoAndStop", target.currentFrame, end, index, targets, Math.round, 0, 0, 1); + if (pt) { + pt.op = p; + } + + } else if (target[p] != null) { + this.add(target, p, "get", end); + } + + } + }, + render(ratio, data) { + let pt = data._pt; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + if (data.target.cacheID) { + data.target.updateCache(); + } + }, + register: _initCore +}; + +EaselPlugin.registerCreateJS = createjs => { + _createJS = createjs; +}; + +_getGSAP() && gsap.registerPlugin(EaselPlugin); + +export { EaselPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/Flip.js b/node_modules/gsap/src/Flip.js new file mode 100644 index 0000000000000000000000000000000000000000..0b147c55d2ed626ed53ae3f6168e261070b729c9 --- /dev/null +++ b/node_modules/gsap/src/Flip.js @@ -0,0 +1,1033 @@ +/*! + * Flip 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +import { getGlobalMatrix, _getDocScrollTop, _getDocScrollLeft, Matrix2D, _setDoc, _getCTM } from "./utils/matrix.js"; + +let _id = 1, + _toArray, gsap, _batch, _batchAction, _body, _closestTenth, _getStyleSaver, + _forEachBatch = (batch, name) => batch.actions.forEach(a => a.vars[name] && a.vars[name](a)), + _batchLookup = {}, + _RAD2DEG = 180 / Math.PI, + _DEG2RAD = Math.PI / 180, + _emptyObj = {}, + _dashedNameLookup = {}, + _memoizedRemoveProps = {}, + _listToArray = list => typeof(list) === "string" ? list.split(" ").join("").split(",") : list, // removes extra spaces contaminating the names, returns an Array. + _callbacks = _listToArray("onStart,onUpdate,onComplete,onReverseComplete,onInterrupt"), + _removeProps = _listToArray("transform,transformOrigin,width,height,position,top,left,opacity,zIndex,maxWidth,maxHeight,minWidth,minHeight"), + _getEl = target => _toArray(target)[0] || console.warn("Element not found:", target), + _round = value => Math.round(value * 10000) / 10000 || 0, + _toggleClass = (targets, className, action) => targets.forEach(el => el.classList[action](className)), + _reserved = {zIndex:1, kill:1, simple:1, spin:1, clearProps:1, targets:1, toggleClass:1, onComplete:1, onUpdate:1, onInterrupt:1, onStart:1, delay:1, repeat:1, repeatDelay:1, yoyo:1, scale:1, fade:1, absolute:1, props:1, onEnter:1, onLeave:1, custom:1, paused:1, nested:1, prune:1, absoluteOnLeave: 1}, + _fitReserved = {zIndex:1, simple:1, clearProps:1, scale:1, absolute:1, fitChild:1, getVars:1, props:1}, + _camelToDashed = p => p.replace(/([A-Z])/g, "-$1").toLowerCase(), + _copy = (obj, exclude) => { + let result = {}, p; + for (p in obj) { + exclude[p] || (result[p] = obj[p]); + } + return result; + }, + _memoizedProps = {}, + _memoizeProps = props => { + let p = _memoizedProps[props] = _listToArray(props); + _memoizedRemoveProps[props] = p.concat(_removeProps); + return p; + }, + _getInverseGlobalMatrix = el => { // integrates caching for improved performance + let cache = el._gsap || gsap.core.getCache(el); + if (cache.gmCache === gsap.ticker.frame) { + return cache.gMatrix; + } + cache.gmCache = gsap.ticker.frame; + return (cache.gMatrix = getGlobalMatrix(el, true, false, true)); + }, + _getDOMDepth = (el, invert, level = 0) => { // In invert is true, the sibling depth is increments of 1, and parent/nesting depth is increments of 1000. This lets us order elements in an Array to reflect document flow. + let parent = el.parentNode, + inc = 1000 * (10 ** level) * (invert ? -1 : 1), + l = invert ? -inc * 900 : 0; + while (el) { + l += inc; + el = el.previousSibling; + } + return parent ? l + _getDOMDepth(parent, invert, level + 1) : l; + }, + _orderByDOMDepth = (comps, invert, isElStates) => { + comps.forEach(comp => comp.d = _getDOMDepth(isElStates ? comp.element : comp.t, invert)); + comps.sort((c1, c2) => c1.d - c2.d); + return comps; + }, + _recordInlineStyles = (elState, props) => { // records the current inline CSS properties into an Array in alternating name/value pairs that's stored in a "css" property on the state object so that we can revert later. + let style = elState.element.style, + a = elState.css = elState.css || [], + i = props.length, + p, v; + while (i--) { + p = props[i]; + v = style[p] || style.getPropertyValue(p); + a.push(v ? p : _dashedNameLookup[p] || (_dashedNameLookup[p] = _camelToDashed(p)), v); + } + return style; + }, + _applyInlineStyles = state => { + let css = state.css, + style = state.element.style, + i = 0; + state.cache.uncache = 1; + for (; i < css.length; i+=2) { + css[i+1] ? (style[css[i]] = css[i+1]) : style.removeProperty(css[i]); + } + if (!css[css.indexOf("transform")+1] && style.translate) { // CSSPlugin adds scale, translate, and rotate inline CSS as "none" in order to keep CSS rules from contaminating transforms. + style.removeProperty("translate"); + style.removeProperty("scale"); + style.removeProperty("rotate"); + } + }, + _setFinalStates = (comps, onlyTransforms) => { + comps.forEach(c => c.a.cache.uncache = 1); + onlyTransforms || comps.finalStates.forEach(_applyInlineStyles); + }, + _absoluteProps = "paddingTop,paddingRight,paddingBottom,paddingLeft,gridArea,transition".split(","), // properties that we must record just + _makeAbsolute = (elState, fallbackNode, ignoreBatch) => { + let { element, width, height, uncache, getProp } = elState, + style = element.style, + i = 4, + result, displayIsNone, cs; + (typeof(fallbackNode) !== "object") && (fallbackNode = elState); + if (_batch && ignoreBatch !== 1) { + _batch._abs.push({t: element, b: elState, a: elState, sd: 0}); + _batch._final.push(() => (elState.cache.uncache = 1) && _applyInlineStyles(elState)); + return element; + } + displayIsNone = getProp("display") === "none"; + + if (!elState.isVisible || displayIsNone) { + displayIsNone && (_recordInlineStyles(elState, ["display"]).display = fallbackNode.display); + elState.matrix = fallbackNode.matrix; + elState.width = width = elState.width || fallbackNode.width; + elState.height = height = elState.height || fallbackNode.height; + } + + _recordInlineStyles(elState, _absoluteProps); + cs = window.getComputedStyle(element); + while (i--) { + style[_absoluteProps[i]] = cs[_absoluteProps[i]]; // record paddings as px-based because if removed from grid, percentage-based ones could be altered. + } + style.gridArea = "1 / 1 / 1 / 1"; + style.transition = "none"; + + style.position = "absolute"; + style.width = width + "px"; + style.height = height + "px"; + style.top || (style.top = "0px"); + style.left || (style.left = "0px"); + if (uncache) { + result = new ElementState(element); + } else { // better performance + result = _copy(elState, _emptyObj); + result.position = "absolute"; + if (elState.simple) { + let bounds = element.getBoundingClientRect(); + result.matrix = new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()); + } else { + result.matrix = getGlobalMatrix(element, false, false, true); + } + } + result = _fit(result, elState, true); + elState.x = _closestTenth(result.x, 0.01); + elState.y = _closestTenth(result.y, 0.01); + return element; + }, + _filterComps = (comps, targets) => { + if (targets !== true) { + targets = _toArray(targets); + comps = comps.filter(c => { + if (targets.indexOf((c.sd < 0 ? c.b : c.a).element) !== -1) { + return true; + } else { + c.t._gsap.renderTransform(1); // we must force transforms to render on anything that isn't being made position: absolute, otherwise the absolute position happens and then when animation begins it applies transforms which can create a new stacking context, throwing off positioning! + if (c.b.isVisible) { + c.t.style.width = c.b.width + "px"; // otherwise things can collapse when contents are made position: absolute. + c.t.style.height = c.b.height + "px"; + } + } + }); + } + return comps; + }, + _makeCompsAbsolute = comps => _orderByDOMDepth(comps, true).forEach(c => (c.a.isVisible || c.b.isVisible) && _makeAbsolute(c.sd < 0 ? c.b : c.a, c.b, 1)), + _findElStateInState = (state, other) => (other && state.idLookup[_parseElementState(other).id]) || state.elementStates[0], + _parseElementState = (elOrNode, props, simple, other) => elOrNode instanceof ElementState ? elOrNode : elOrNode instanceof FlipState ? _findElStateInState(elOrNode, other) : new ElementState(typeof(elOrNode) === "string" ? _getEl(elOrNode) || console.warn(elOrNode + " not found") : elOrNode, props, simple), + _recordProps = (elState, props) => { + let getProp = gsap.getProperty(elState.element, null, "native"), + obj = elState.props = {}, + i = props.length; + while (i--) { + obj[props[i]] = (getProp(props[i]) + "").trim(); + } + obj.zIndex && (obj.zIndex = parseFloat(obj.zIndex) || 0); + return elState; + }, + _applyProps = (element, props) => { + let style = element.style || element, // could pass in a vars object. + p; + for (p in props) { + style[p] = props[p]; + } + }, + _getID = el => { + let id = el.getAttribute("data-flip-id"); + id || el.setAttribute("data-flip-id", (id = "auto-" + _id++)); + return id; + }, + _elementsFromElementStates = elStates => elStates.map(elState => elState.element), + _handleCallback = (callback, elStates, tl) => callback && elStates.length && tl.add(callback(_elementsFromElementStates(elStates), tl, new FlipState(elStates, 0, true)), 0), + + _fit = (fromState, toState, scale, applyProps, fitChild, vars) => { + let { element, cache, parent, x, y } = fromState, + { width, height, scaleX, scaleY, rotation, bounds } = toState, + styles = vars && _getStyleSaver && _getStyleSaver(element, "transform,width,height"), // requires at least 3.11.5 + dimensionState = fromState, + {e, f} = toState.matrix, + deep = fromState.bounds.width !== bounds.width || fromState.bounds.height !== bounds.height || fromState.scaleX !== scaleX || fromState.scaleY !== scaleY || fromState.rotation !== rotation, + simple = !deep && fromState.simple && toState.simple && !fitChild, + skewX, fromPoint, toPoint, getProp, parentMatrix, matrix, bbox; + if (simple || !parent) { + scaleX = scaleY = 1; + rotation = skewX = 0; + } else { + parentMatrix = _getInverseGlobalMatrix(parent); + matrix = parentMatrix.clone().multiply(toState.ctm ? toState.matrix.clone().multiply(toState.ctm) : toState.matrix); // root SVG elements have a ctm that we must factor out (for example, viewBox:"0 0 94 94" with a width of 200px would scale the internals by 2.127 but when we're matching the size of the root <svg> element itself, that scaling shouldn't factor in!) + rotation = _round(Math.atan2(matrix.b, matrix.a) * _RAD2DEG); + skewX = _round(Math.atan2(matrix.c, matrix.d) * _RAD2DEG + rotation) % 360; // in very rare cases, minor rounding might end up with 360 which should be 0. + scaleX = Math.sqrt(matrix.a ** 2 + matrix.b ** 2); + scaleY = Math.sqrt(matrix.c ** 2 + matrix.d ** 2) * Math.cos(skewX * _DEG2RAD); + if (fitChild) { + fitChild = _toArray(fitChild)[0]; + getProp = gsap.getProperty(fitChild); + bbox = fitChild.getBBox && typeof(fitChild.getBBox) === "function" && fitChild.getBBox(); + dimensionState = {scaleX: getProp("scaleX"), scaleY: getProp("scaleY"), width: bbox ? bbox.width : Math.ceil(parseFloat(getProp("width", "px"))), height: bbox ? bbox.height : parseFloat(getProp("height", "px")) }; + } + cache.rotation = rotation + "deg"; + cache.skewX = skewX + "deg"; + } + if (scale) { + scaleX *= width === dimensionState.width || !dimensionState.width ? 1 : width / dimensionState.width; // note if widths are both 0, we should make scaleX 1 - some elements have box-sizing that incorporates padding, etc. and we don't want it to collapse in that case. + scaleY *= height === dimensionState.height || !dimensionState.height ? 1 : height / dimensionState.height; + cache.scaleX = scaleX; + cache.scaleY = scaleY; + } else { + width = _closestTenth(width * scaleX / dimensionState.scaleX, 0); + height = _closestTenth(height * scaleY / dimensionState.scaleY, 0); + element.style.width = width + "px"; + element.style.height = height + "px"; + } + // if (fromState.isFixed) { // commented out because it's now taken care of in getGlobalMatrix() with a flag at the end. + // e -= _getDocScrollLeft(); + // f -= _getDocScrollTop(); + // } + applyProps && _applyProps(element, toState.props); + if (simple || !parent) { + x += e - fromState.matrix.e; + y += f - fromState.matrix.f; + } else if (deep || parent !== toState.parent) { + cache.renderTransform(1, cache); + matrix = getGlobalMatrix(fitChild || element, false, false, true); + fromPoint = parentMatrix.apply({x: matrix.e, y: matrix.f}); + toPoint = parentMatrix.apply({x: e, y: f}); + x += toPoint.x - fromPoint.x; + y += toPoint.y - fromPoint.y; + } else { // use a faster/cheaper algorithm if we're just moving x/y + parentMatrix.e = parentMatrix.f = 0; + toPoint = parentMatrix.apply({x: e - fromState.matrix.e, y: f - fromState.matrix.f}); + x += toPoint.x; + y += toPoint.y; + } + x = _closestTenth(x, 0.02); + y = _closestTenth(y, 0.02); + if (vars && !(vars instanceof ElementState)) { // revert + styles && styles.revert(); + } else { // or apply the transform immediately + cache.x = x + "px"; + cache.y = y + "px"; + cache.renderTransform(1, cache); + } + if (vars) { + vars.x = x; + vars.y = y; + vars.rotation = rotation; + vars.skewX = skewX; + if (scale) { + vars.scaleX = scaleX; + vars.scaleY = scaleY; + } else { + vars.width = width; + vars.height = height; + } + } + return vars || cache; + }, + + _parseState = (targetsOrState, vars) => targetsOrState instanceof FlipState ? targetsOrState : new FlipState(targetsOrState, vars), + _getChangingElState = (toState, fromState, id) => { + let to1 = toState.idLookup[id], + to2 = toState.alt[id]; + return to2.isVisible && (!(fromState.getElementState(to2.element) || to2).isVisible || !to1.isVisible) ? to2 : to1; + }, + _bodyMetrics = [], _bodyProps = "width,height,overflowX,overflowY".split(","), _bodyLocked, + _lockBodyScroll = lock => { // if there's no scrollbar, we should lock that so that measurements don't get affected by temporary repositioning, like if something is centered in the window. + if (lock !== _bodyLocked) { + let s = _body.style, + w = _body.clientWidth === window.outerWidth, + h = _body.clientHeight === window.outerHeight, + i = 4; + if (lock && (w || h)) { + while (i--) { + _bodyMetrics[i] = s[_bodyProps[i]]; + } + if (w) { + s.width = _body.clientWidth + "px"; + s.overflowY = "hidden"; + } + if (h) { + s.height = _body.clientHeight + "px"; + s.overflowX = "hidden"; + } + _bodyLocked= lock; + } else if (_bodyLocked) { + while (i--) { + _bodyMetrics[i] ? (s[_bodyProps[i]] = _bodyMetrics[i]) : s.removeProperty(_camelToDashed(_bodyProps[i])); + } + _bodyLocked = lock; + } + } + }, + + _fromTo = (fromState, toState, vars, relative) => { // relative is -1 if "from()", and 1 if "to()" + (fromState instanceof FlipState && toState instanceof FlipState) || console.warn("Not a valid state object."); + vars = vars || {}; + let { clearProps, onEnter, onLeave, absolute, absoluteOnLeave, custom, delay, paused, repeat, repeatDelay, yoyo, toggleClass, nested, zIndex, scale, fade, stagger, spin, prune } = vars, + props = ("props" in vars ? vars : fromState).props, + tweenVars = _copy(vars, _reserved), + animation = gsap.timeline({ delay, paused, repeat, repeatDelay, yoyo, data: "isFlip" }), + remainingProps = tweenVars, + entering = [], + leaving = [], + comps = [], + swapOutTargets = [], + spinNum = spin === true ? 1 : spin || 0, + spinFunc = typeof(spin) === "function" ? spin : () => spinNum, + interrupted = fromState.interrupted || toState.interrupted, + addFunc = animation[relative !== 1 ? "to" : "from"], + v, p, endTime, i, el, comp, state, targets, finalStates, fromNode, toNode, run, a, b; + //relative || (toState = (new FlipState(toState.targets, {props: props})).fit(toState, scale)); + for (p in toState.idLookup) { + toNode = !toState.alt[p] ? toState.idLookup[p] : _getChangingElState(toState, fromState, p); + el = toNode.element; + fromNode = fromState.idLookup[p]; + fromState.alt[p] && el === fromNode.element && (fromState.alt[p].isVisible || !toNode.isVisible) && (fromNode = fromState.alt[p]); + if (fromNode) { + comp = {t: el, b: fromNode, a: toNode, sd: fromNode.element === el ? 0 : toNode.isVisible ? 1 : -1}; + comps.push(comp); + if (comp.sd) { + if (comp.sd < 0) { + comp.b = toNode; + comp.a = fromNode; + } + // for swapping elements that got interrupted, we must re-record the inline styles to ensure they're not tainted. Remember, .batch() permits getState() not to force in-progress flips to their end state. + interrupted && _recordInlineStyles(comp.b, props ? _memoizedRemoveProps[props] : _removeProps); + fade && comps.push(comp.swap = {t: fromNode.element, b: comp.b, a: comp.a, sd: -comp.sd, swap: comp}); + } + el._flip = fromNode.element._flip = _batch ? _batch.timeline : animation; + } else if (toNode.isVisible) { + comps.push({t: el, b: _copy(toNode, {isVisible:1}), a: toNode, sd: 0, entering: 1}); // to include it in the "entering" Array and do absolute positioning if necessary + el._flip = _batch ? _batch.timeline : animation; + } + } + + props && (_memoizedProps[props] || _memoizeProps(props)).forEach(p => tweenVars[p] = i => comps[i].a.props[p]); + comps.finalStates = finalStates = []; + + run = () => { + _orderByDOMDepth(comps); + _lockBodyScroll(true); // otherwise, measurements may get thrown off when things get fit. + // TODO: cache the matrix, especially for parent because it'll probably get reused quite a bit, but lock it to a particular cycle(?). + for (i = 0; i < comps.length; i++) { + comp = comps[i]; + a = comp.a; + b = comp.b; + if (prune && !a.isDifferent(b) && !comp.entering) { // only flip if things changed! Don't omit it from comps initially because that'd prevent the element from being positioned absolutely (if necessary) + comps.splice(i--, 1); + } else { + el = comp.t; + nested && !(comp.sd < 0) && i && (a.matrix = getGlobalMatrix(el, false, false, true)); // moving a parent affects the position of children + if (b.isVisible && a.isVisible) { + if (comp.sd < 0) { // swapping OUT (swap direction of -1 is out) + state = new ElementState(el, props, fromState.simple); + _fit(state, a, scale, 0, 0, state); + state.matrix = getGlobalMatrix(el, false, false, true); + state.css = comp.b.css; + comp.a = a = state; + fade && (el.style.opacity = interrupted ? b.opacity : a.opacity); + stagger && swapOutTargets.push(el); + } else if (comp.sd > 0 && fade) { // swapping IN (swap direction of 1 is in) + el.style.opacity = interrupted ? a.opacity - b.opacity : "0"; + } + _fit(a, b, scale, props); + + } else if (b.isVisible !== a.isVisible) { // either entering or leaving (one side is invisible) + if (!b.isVisible) { // entering + a.isVisible && entering.push(a); + comps.splice(i--, 1); + } else if (!a.isVisible) { // leaving + b.css = a.css; + leaving.push(b); + comps.splice(i--, 1); + absolute && nested && _fit(a, b, scale, props); + } + } + if (!scale) { + el.style.maxWidth = Math.max(a.width, b.width) + "px"; + el.style.maxHeight = Math.max(a.height, b.height) + "px"; + el.style.minWidth = Math.min(a.width, b.width) + "px"; + el.style.minHeight = Math.min(a.height, b.height) + "px"; + } + nested && toggleClass && el.classList.add(toggleClass); + } + finalStates.push(a); + } + let classTargets; + if (toggleClass) { + classTargets = finalStates.map(s => s.element); + nested && classTargets.forEach(e => e.classList.remove(toggleClass)); // there could be a delay, so don't leave the classes applied (we'll do it in a timeline callback) + } + + _lockBodyScroll(false); + + if (scale) { + tweenVars.scaleX = i => comps[i].a.scaleX; + tweenVars.scaleY = i => comps[i].a.scaleY; + } else { + tweenVars.width = i => comps[i].a.width + "px"; + tweenVars.height = i => comps[i].a.height + "px"; + tweenVars.autoRound = vars.autoRound || false; + } + tweenVars.x = i => comps[i].a.x + "px"; + tweenVars.y = i => comps[i].a.y + "px"; + tweenVars.rotation = i => comps[i].a.rotation + (spin ? spinFunc(i, targets[i], targets) * 360 : 0); + tweenVars.skewX = i => comps[i].a.skewX; + + targets = comps.map(c => c.t); + + if (zIndex || zIndex === 0) { + tweenVars.modifiers = {zIndex: () => zIndex}; + tweenVars.zIndex = zIndex; + tweenVars.immediateRender = vars.immediateRender !== false; + } + + fade && (tweenVars.opacity = i => comps[i].sd < 0 ? 0 : comps[i].sd > 0 ? comps[i].a.opacity : "+=0"); + + if (swapOutTargets.length) { + stagger = gsap.utils.distribute(stagger); + let dummyArray = targets.slice(swapOutTargets.length); + tweenVars.stagger = (i, el) => stagger(~swapOutTargets.indexOf(el) ? targets.indexOf(comps[i].swap.t) : i, el, dummyArray); + } + + // // for testing... + // gsap.delayedCall(vars.data ? 50 : 1, function() { + // animation.eventCallback("onComplete", () => _setFinalStates(comps, !clearProps)); + // addFunc.call(animation, targets, tweenVars, 0).play(); + // }); + // return; + + _callbacks.forEach(name => vars[name] && animation.eventCallback(name, vars[name], vars[name + "Params"])); // apply callbacks to the timeline, not tweens (because "custom" timing can make multiple tweens) + + if (custom && targets.length) { // bust out the custom properties as their own tweens so they can use different eases, durations, etc. + remainingProps = _copy(tweenVars, _reserved); + if ("scale" in custom) { + custom.scaleX = custom.scaleY = custom.scale; + delete custom.scale; + } + for (p in custom) { + v = _copy(custom[p], _fitReserved); + v[p] = tweenVars[p]; + !("duration" in v) && ("duration" in tweenVars) && (v.duration = tweenVars.duration); + v.stagger = tweenVars.stagger; + addFunc.call(animation, targets, v, 0); + delete remainingProps[p]; + } + } + if (targets.length || leaving.length || entering.length) { + toggleClass && animation.add(() => _toggleClass(classTargets, toggleClass, animation._zTime < 0 ? "remove" : "add"), 0) && !paused && _toggleClass(classTargets, toggleClass, "add"); + targets.length && addFunc.call(animation, targets, remainingProps, 0); + } + + _handleCallback(onEnter, entering, animation); + _handleCallback(onLeave, leaving, animation); + + let batchTl = _batch && _batch.timeline; + + if (batchTl) { + batchTl.add(animation, 0); + _batch._final.push(() => _setFinalStates(comps, !clearProps)); + } + + endTime = animation.duration(); + animation.call(() => { + let forward = animation.time() >= endTime; + forward && !batchTl && _setFinalStates(comps, !clearProps); + toggleClass && _toggleClass(classTargets, toggleClass, forward ? "remove" : "add"); + }); + }; + + absoluteOnLeave && (absolute = comps.filter(comp => !comp.sd && !comp.a.isVisible && comp.b.isVisible).map(comp => comp.a.element)); + if (_batch) { + absolute && _batch._abs.push(..._filterComps(comps, absolute)); + _batch._run.push(run); + } else { + absolute && _makeCompsAbsolute(_filterComps(comps, absolute)); // when making absolute, we must go in a very particular order so that document flow changes don't affect things. Don't make it visible if both the before and after states are invisible! There's no point, and it could make things appear visible during the flip that shouldn't be. + run(); + } + + let anim = _batch ? _batch.timeline : animation; + anim.revert = () => _killFlip(anim, 1, 1); // a Flip timeline should behave very different when reverting - it should actually jump to the end so that styles get cleared out. + + return anim; + }, + _interrupt = tl => { + tl.vars.onInterrupt && tl.vars.onInterrupt.apply(tl, tl.vars.onInterruptParams || []); + tl.getChildren(true, false, true).forEach(_interrupt); + }, + _killFlip = (tl, action, force) => { // action: 0 = nothing, 1 = complete, 2 = only kill (don't complete) + if (tl && tl.progress() < 1 && (!tl.paused() || force)) { + if (action) { + _interrupt(tl); + action < 2 && tl.progress(1); // we should also kill it in case it was added to a parent timeline. + tl.kill(); + } + return true; + } + }, + _createLookup = state => { + let lookup = state.idLookup = {}, + alt = state.alt = {}, + elStates = state.elementStates, + i = elStates.length, + elState; + while (i--) { + elState = elStates[i]; + lookup[elState.id] ? (alt[elState.id] = elState) : (lookup[elState.id] = elState); + } + }; + + + + + + +class FlipState { + + constructor(targets, vars, targetsAreElementStates) { + this.props = vars && vars.props; + this.simple = !!(vars && vars.simple); + if (targetsAreElementStates) { + this.targets = _elementsFromElementStates(targets); + this.elementStates = targets; + _createLookup(this); + } else { + this.targets = _toArray(targets); + let soft = vars && (vars.kill === false || (vars.batch && !vars.kill)); + _batch && !soft && _batch._kill.push(this); + this.update(soft || !!_batch); // when batching, don't force in-progress flips to their end; we need to do that AFTER all getStates() are called. + } + } + + update(soft) { + this.elementStates = this.targets.map(el => new ElementState(el, this.props, this.simple)); + _createLookup(this); + this.interrupt(soft); + this.recordInlineStyles(); + return this; + } + + clear() { + this.targets.length = this.elementStates.length = 0; + _createLookup(this); + return this; + } + + fit(state, scale, nested) { + let elStatesInOrder = _orderByDOMDepth(this.elementStates.slice(0), false, true), + toElStates = (state || this).idLookup, + i = 0, + fromNode, toNode; + for (; i < elStatesInOrder.length; i++) { + fromNode = elStatesInOrder[i]; + nested && (fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true)); // moving a parent affects the position of children + toNode = toElStates[fromNode.id]; + toNode && _fit(fromNode, toNode, scale, true, 0, fromNode); + fromNode.matrix = getGlobalMatrix(fromNode.element, false, false, true); + } + return this; + } + + getProperty(element, property) { + let es = this.getElementState(element) || _emptyObj; + return (property in es ? es : es.props || _emptyObj)[property]; + } + + add(state) { + let i = state.targets.length, + lookup = this.idLookup, + alt = this.alt, + index, es, es2; + while (i--) { + es = state.elementStates[i]; + es2 = lookup[es.id]; + if (es2 && (es.element === es2.element || (alt[es.id] && alt[es.id].element === es.element))) { // if the flip id is already in this FlipState, replace it! + index = this.elementStates.indexOf(es.element === es2.element ? es2 : alt[es.id]); + this.targets.splice(index, 1, state.targets[i]); + this.elementStates.splice(index, 1, es); + } else { + this.targets.push(state.targets[i]); + this.elementStates.push(es); + } + } + state.interrupted && (this.interrupted = true); + state.simple || (this.simple = false); + _createLookup(this); + return this; + } + + compare(state) { + let l1 = state.idLookup, + l2 = this.idLookup, + unchanged = [], + changed = [], + enter = [], + leave = [], + targets = [], + a1 = state.alt, + a2 = this.alt, + place = (s1, s2, el) => (s1.isVisible !== s2.isVisible ? (s1.isVisible ? enter : leave) : s1.isVisible ? changed : unchanged).push(el) && targets.push(el), + placeIfDoesNotExist = (s1, s2, el) => targets.indexOf(el) < 0 && place(s1, s2, el), + s1, s2, p, el, s1Alt, s2Alt, c1, c2; + for (p in l1) { + s1Alt = a1[p]; + s2Alt = a2[p]; + s1 = !s1Alt ? l1[p] : _getChangingElState(state, this, p); + el = s1.element; + s2 = l2[p]; + if (s2Alt) { + c2 = s2.isVisible || (!s2Alt.isVisible && el === s2.element) ? s2 : s2Alt; + c1 = s1Alt && !s1.isVisible && !s1Alt.isVisible && c2.element === s1Alt.element ? s1Alt : s1; + //c1.element !== c2.element && c1.element === s2.element && (c2 = s2); + if (c1.isVisible && c2.isVisible && c1.element !== c2.element) { // swapping, so force into "changed" array + (c1.isDifferent(c2) ? changed : unchanged).push(c1.element, c2.element); + targets.push(c1.element, c2.element); + } else { + place(c1, c2, c1.element); + } + s1Alt && c1.element === s1Alt.element && (s1Alt = l1[p]); + placeIfDoesNotExist(c1.element !== s2.element && s1Alt ? s1Alt : c1, s2, s2.element); + placeIfDoesNotExist(s1Alt && s1Alt.element === s2Alt.element ? s1Alt : c1, s2Alt, s2Alt.element); + s1Alt && placeIfDoesNotExist(s1Alt, s2Alt.element === s1Alt.element ? s2Alt : s2, s1Alt.element); + } else { + !s2 ? enter.push(el) : !s2.isDifferent(s1) ? unchanged.push(el) : place(s1, s2, el); + s1Alt && placeIfDoesNotExist(s1Alt, s2, s1Alt.element); + } + } + for (p in l2) { + if (!l1[p]) { + leave.push(l2[p].element); + a2[p] && leave.push(a2[p].element); + } + } + return {changed, unchanged, enter, leave}; + } + + recordInlineStyles() { + let props = _memoizedRemoveProps[this.props] || _removeProps, + i = this.elementStates.length; + while (i--) { + _recordInlineStyles(this.elementStates[i], props); + } + } + + interrupt(soft) { // soft = DON'T force in-progress flip animations to completion (like when running a batch, we can't immediately kill flips when getting states because it could contaminate positioning and other .getState() calls that will run in the batch (we kill AFTER all the .getState() calls complete). + let timelines = []; + this.targets.forEach(t => { + let tl = t._flip, + foundInProgress = _killFlip(tl, soft ? 0 : 1); + soft && foundInProgress && timelines.indexOf(tl) < 0 && tl.add(() => this.updateVisibility()); + foundInProgress && timelines.push(tl); + }); + !soft && timelines.length && this.updateVisibility(); // if we found an in-progress Flip animation, we must record all the values in their current state at that point BUT we should update the isVisible value AFTER pushing that flip to completion so that elements that are entering or leaving will populate those Arrays properly. + this.interrupted || (this.interrupted = !!timelines.length); + } + + updateVisibility() { + this.elementStates.forEach(es => { + let b = es.element.getBoundingClientRect(); + es.isVisible = !!(b.width || b.height || b.top || b.left); + es.uncache = 1; + }); + } + + getElementState(element) { + return this.elementStates[this.targets.indexOf(_getEl(element))]; + } + + makeAbsolute() { + return _orderByDOMDepth(this.elementStates.slice(0), true, true).map(_makeAbsolute); + } + +} + + + +class ElementState { + + constructor(element, props, simple) { + this.element = element; + this.update(props, simple); + } + + isDifferent(state) { + let b1 = this.bounds, + b2 = state.bounds; + return b1.top !== b2.top || b1.left !== b2.left || b1.width !== b2.width || b1.height !== b2.height || !this.matrix.equals(state.matrix) || this.opacity !== state.opacity || (this.props && state.props && JSON.stringify(this.props) !== JSON.stringify(state.props)); + } + + update(props, simple) { + let self = this, + element = self.element, + getProp = gsap.getProperty(element), + cache = gsap.core.getCache(element), + bounds = element.getBoundingClientRect(), + bbox = element.getBBox && typeof(element.getBBox) === "function" && element.nodeName.toLowerCase() !== "svg" && element.getBBox(), + m = simple ? new Matrix2D(1, 0, 0, 1, bounds.left + _getDocScrollLeft(), bounds.top + _getDocScrollTop()) : getGlobalMatrix(element, false, false, true); + self.getProp = getProp; + self.element = element; + self.id = _getID(element); + self.matrix = m; + self.cache = cache; + self.bounds = bounds; + self.isVisible = !!(bounds.width || bounds.height || bounds.left || bounds.top); + self.display = getProp("display"); + self.position = getProp("position"); + self.parent = element.parentNode; + self.x = getProp("x"); + self.y = getProp("y"); + self.scaleX = cache.scaleX; + self.scaleY = cache.scaleY; + self.rotation = getProp("rotation"); + self.skewX = getProp("skewX"); + self.opacity = getProp("opacity"); + self.width = bbox ? bbox.width : _closestTenth(getProp("width", "px"), 0.04); // round up to the closest 0.1 so that text doesn't wrap. + self.height = bbox ? bbox.height : _closestTenth(getProp("height", "px"), 0.04); + props && _recordProps(self, _memoizedProps[props] || _memoizeProps(props)); + self.ctm = element.getCTM && element.nodeName.toLowerCase() === "svg" && _getCTM(element).inverse(); + self.simple = simple || (_round(m.a) === 1 && !_round(m.b) && !_round(m.c) && _round(m.d) === 1); // allows us to speed through some other tasks if it's not scale/rotated + self.uncache = 0; + } + +} + +class FlipAction { + constructor(vars, batch) { + this.vars = vars; + this.batch = batch; + this.states = []; + this.timeline = batch.timeline; + } + + getStateById(id) { + let i = this.states.length; + while (i--) { + if (this.states[i].idLookup[id]) { + return this.states[i]; + } + } + } + + kill() { + this.batch.remove(this); + } +} + +class FlipBatch { + constructor(id) { + this.id = id; + this.actions = []; + this._kill = []; + this._final = []; + this._abs = []; + this._run = []; + this.data = {}; + this.state = new FlipState(); + this.timeline = gsap.timeline(); + } + + add(config) { + let result = this.actions.filter(action => action.vars === config); + if (result.length) { + return result[0]; + } + result = new FlipAction(typeof(config) === "function" ? {animate: config} : config, this); + this.actions.push(result); + return result; + } + + remove(action) { + let i = this.actions.indexOf(action); + i >= 0 && this.actions.splice(i, 1); + return this; + } + + getState(merge) { + let prevBatch = _batch, + prevAction = _batchAction; + _batch = this; + this.state.clear(); + this._kill.length = 0; + this.actions.forEach(action => { + if (action.vars.getState) { + action.states.length = 0; + _batchAction = action; + action.state = action.vars.getState(action); + } + merge && action.states.forEach(s => this.state.add(s)); + }); + _batchAction = prevAction; + _batch = prevBatch; + this.killConflicts(); + return this; + } + + animate() { + let prevBatch = _batch, + tl = this.timeline, + i = this.actions.length, + finalStates, endTime; + _batch = this; + tl.clear(); + this._abs.length = this._final.length = this._run.length = 0; + this.actions.forEach(a => { + a.vars.animate && a.vars.animate(a); + let onEnter = a.vars.onEnter, + onLeave = a.vars.onLeave, + targets = a.targets, s, result; + if (targets && targets.length && (onEnter || onLeave)) { + s = new FlipState(); + a.states.forEach(state => s.add(state)); + result = s.compare(Flip.getState(targets)); + result.enter.length && onEnter && onEnter(result.enter); + result.leave.length && onLeave && onLeave(result.leave); + } + }); + _makeCompsAbsolute(this._abs); + this._run.forEach(f => f()); + endTime = tl.duration(); + finalStates = this._final.slice(0); + tl.add(() => { + if (endTime <= tl.time()) { // only call if moving forward in the timeline (in case it's nested in a timeline that gets reversed) + finalStates.forEach(f => f()); + _forEachBatch(this, "onComplete"); + } + }); + _batch = prevBatch; + while (i--) { + this.actions[i].vars.once && this.actions[i].kill(); + } + _forEachBatch(this, "onStart"); + tl.restart(); + return this; + } + + loadState(done) { + done || (done = () => 0); + let queue = []; + this.actions.forEach(c => { + if (c.vars.loadState) { + let i, f = targets => { + targets && (c.targets = targets); + i = queue.indexOf(f); + if (~i) { + queue.splice(i, 1); + queue.length || done(); + } + }; + queue.push(f); + c.vars.loadState(f); + } + }); + queue.length || done(); + return this; + } + + setState() { + this.actions.forEach(c => c.targets = c.vars.setState && c.vars.setState(c)); + return this; + } + + killConflicts(soft) { + this.state.interrupt(soft); + this._kill.forEach(state => state.interrupt(soft)); + return this; + } + + run(skipGetState, merge) { + if (this !== _batch) { + skipGetState || this.getState(merge); + this.loadState(() => { + if (!this._killed) { + this.setState(); + this.animate(); + } + }); + } + return this; + } + + clear(stateOnly) { + this.state.clear(); + stateOnly || (this.actions.length = 0); + } + + getStateById(id) { + let i = this.actions.length, + s; + while (i--) { + s = this.actions[i].getStateById(id); + if (s) { + return s; + } + } + return this.state.idLookup[id] && this.state; + } + + kill() { + this._killed = 1; + this.clear(); + delete _batchLookup[this.id]; + } +} + + +export class Flip { + + static getState(targets, vars) { + let state = _parseState(targets, vars); + _batchAction && _batchAction.states.push(state); + vars && vars.batch && Flip.batch(vars.batch).state.add(state); + return state; + } + + static from(state, vars) { + vars = vars || {}; + ("clearProps" in vars) || (vars.clearProps = true); + return _fromTo(state, _parseState(vars.targets || state.targets, {props: vars.props || state.props, simple: vars.simple, kill: !!vars.kill}), vars, -1); + } + + static to(state, vars) { + return _fromTo(state, _parseState(vars.targets || state.targets, {props: vars.props || state.props, simple: vars.simple, kill: !!vars.kill}), vars, 1); + } + + static fromTo(fromState, toState, vars) { + return _fromTo(fromState, toState, vars); + } + + static fit(fromEl, toEl, vars) { + let v = vars ? _copy(vars, _fitReserved) : {}, + {absolute, scale, getVars, props, runBackwards, onComplete, simple} = vars || v, + fitChild = vars && vars.fitChild && _getEl(vars.fitChild), + before = _parseElementState(toEl, props, simple, fromEl), + after = _parseElementState(fromEl, 0, simple, before), + inlineProps = props ? _memoizedRemoveProps[props] : _removeProps, + ctx = gsap.context(); + props && _applyProps(v, before.props); + _recordInlineStyles(after, inlineProps); + if (runBackwards) { + ("immediateRender" in v) || (v.immediateRender = true); + v.onComplete = function() { + _applyInlineStyles(after); + onComplete && onComplete.apply(this, arguments); + }; + } + absolute && _makeAbsolute(after, before); + v = _fit(after, before, scale || fitChild, props, fitChild, v.duration || getVars ? v : 0); + typeof(vars) === "object" && "zIndex" in vars && (v.zIndex = vars.zIndex); + ctx && !getVars && ctx.add(() => () => _applyInlineStyles(after)); + return getVars ? v : v.duration ? gsap.to(after.element, v) : null; + } + + static makeAbsolute(targetsOrStates, vars) { + return (targetsOrStates instanceof FlipState ? targetsOrStates : new FlipState(targetsOrStates, vars)).makeAbsolute(); + } + + static batch(id) { + id || (id = "default"); + return _batchLookup[id] || (_batchLookup[id] = new FlipBatch(id)); + } + + static killFlipsOf(targets, complete) { + (targets instanceof FlipState ? targets.targets : _toArray(targets)).forEach(t => t && _killFlip(t._flip, complete !== false ? 1 : 2)); + } + + static isFlipping(target) { + let f = Flip.getByTarget(target); + return !!f && f.isActive(); + } + + static getByTarget(target) { + return (_getEl(target) || _emptyObj)._flip; + } + + static getElementState(target, props) { + return new ElementState(_getEl(target), props); + } + + static convertCoordinates(fromElement, toElement, point) { + let m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + } + + + static register(core) { + _body = typeof(document) !== "undefined" && document.body; + if (_body) { + gsap = core; + _setDoc(_body); + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + let snap = gsap.utils.snap(0.1); + _closestTenth = (value, add) => snap(parseFloat(value) + add); + } + } +} + +Flip.version = "3.12.7"; + +// function whenImagesLoad(el, func) { +// let pending = [], +// onLoad = e => { +// pending.splice(pending.indexOf(e.target), 1); +// e.target.removeEventListener("load", onLoad); +// pending.length || func(); +// }; +// gsap.utils.toArray(el.tagName.toLowerCase() === "img" ? el : el.querySelectorAll("img")).forEach(img => img.complete || img.addEventListener("load", onLoad) || pending.push(img)); +// pending.length || func(); +// } + +typeof(window) !== "undefined" && window.gsap && window.gsap.registerPlugin(Flip); + +export { Flip as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/MotionPathPlugin.js b/node_modules/gsap/src/MotionPathPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..bc0e17333906cf9d8e3d70ce0855e1d43ca230d2 --- /dev/null +++ b/node_modules/gsap/src/MotionPathPlugin.js @@ -0,0 +1,271 @@ +/*! + * MotionPathPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +import { getRawPath, cacheRawPathMeasurements, getPositionOnPath, pointsToSegment, flatPointsToSegment, sliceRawPath, stringToRawPath, rawPathToString, transformRawPath, convertToPath } from "./utils/paths.js"; +import { getGlobalMatrix } from "./utils/matrix.js"; + +let _xProps = "x,translateX,left,marginLeft,xPercent".split(","), + _yProps = "y,translateY,top,marginTop,yPercent".split(","), + _DEG2RAD = Math.PI / 180, + gsap, PropTween, _getUnit, _toArray, _getStyleSaver, _reverting, + _getGSAP = () => gsap || (typeof(window) !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _populateSegmentFromArray = (segment, values, property, mode) => { //mode: 0 = x but don't fill y yet, 1 = y, 2 = x and fill y with 0. + let l = values.length, + si = mode === 2 ? 0 : mode, + i = 0, + v; + for (; i < l; i++) { + segment[si] = v = parseFloat(values[i][property]); + mode === 2 && (segment[si+1] = 0); + si += 2; + } + return segment; + }, + _getPropNum = (target, prop, unit) => parseFloat(target._gsap.get(target, prop, unit || "px")) || 0, + _relativize = segment => { + let x = segment[0], + y = segment[1], + i; + for (i = 2; i < segment.length; i+=2) { + x = (segment[i] += x); + y = (segment[i+1] += y); + } + }, + // feed in an array of quadratic bezier points like [{x: 0, y: 0}, ...] and it'll convert it to cubic bezier + // _quadToCubic = points => { + // let cubic = [], + // l = points.length - 1, + // i = 1, + // a, b, c; + // for (; i < l; i+=2) { + // a = points[i-1]; + // b = points[i]; + // c = points[i+1]; + // cubic.push(a, {x: (2 * b.x + a.x) / 3, y: (2 * b.y + a.y) / 3}, {x: (2 * b.x + c.x) / 3, y: (2 * b.y + c.y) / 3}); + // } + // cubic.push(points[l]); + // return cubic; + // }, + _segmentToRawPath = (plugin, segment, target, x, y, slicer, vars, unitX, unitY) => { + if (vars.type === "cubic") { + segment = [segment]; + } else { + vars.fromCurrent !== false && segment.unshift(_getPropNum(target, x, unitX), y ? _getPropNum(target, y, unitY) : 0); + vars.relative && _relativize(segment); + let pointFunc = y ? pointsToSegment : flatPointsToSegment; + segment = [pointFunc(segment, vars.curviness)]; + } + segment = slicer(_align(segment, target, vars)); + _addDimensionalPropTween(plugin, target, x, segment, "x", unitX); + y && _addDimensionalPropTween(plugin, target, y, segment, "y", unitY); + return cacheRawPathMeasurements(segment, vars.resolution || (vars.curviness === 0 ? 20 : 12)); //when curviness is 0, it creates control points right on top of the anchors which makes it more sensitive to resolution, thus we change the default accordingly. + }, + _emptyFunc = v => v, + _numExp = /[-+\.]*\d+\.?(?:e-|e\+)?\d*/g, + _originToPoint = (element, origin, parentMatrix) => { // origin is an array of normalized values (0-1) in relation to the width/height, so [0.5, 0.5] would be the center. It can also be "auto" in which case it will be the top left unless it's a <path>, when it will start at the beginning of the path itself. + let m = getGlobalMatrix(element), + x = 0, + y = 0, + svg; + if ((element.tagName + "").toLowerCase() === "svg") { + svg = element.viewBox.baseVal; + svg.width || (svg = {width: +element.getAttribute("width"), height: +element.getAttribute("height")}); + } else { + svg = origin && element.getBBox && element.getBBox(); + } + if (origin && origin !== "auto") { + x = origin.push ? origin[0] * (svg ? svg.width : element.offsetWidth || 0) : origin.x; + y = origin.push ? origin[1] * (svg ? svg.height : element.offsetHeight || 0) : origin.y; + } + return parentMatrix.apply( x || y ? m.apply({x: x, y: y}) : {x: m.e, y: m.f} ); + }, + _getAlignMatrix = (fromElement, toElement, fromOrigin, toOrigin) => { + let parentMatrix = getGlobalMatrix(fromElement.parentNode, true, true), + m = parentMatrix.clone().multiply(getGlobalMatrix(toElement)), + fromPoint = _originToPoint(fromElement, fromOrigin, parentMatrix), + {x, y} = _originToPoint(toElement, toOrigin, parentMatrix), + p; + m.e = m.f = 0; + if (toOrigin === "auto" && toElement.getTotalLength && toElement.tagName.toLowerCase() === "path") { + p = toElement.getAttribute("d").match(_numExp) || []; + p = m.apply({x:+p[0], y:+p[1]}); + x += p.x; + y += p.y; + } + //if (p || (toElement.getBBox && fromElement.getBBox && toElement.ownerSVGElement === fromElement.ownerSVGElement)) { + if (p) { + p = m.apply(toElement.getBBox()); + x -= p.x; + y -= p.y; + } + m.e = x - fromPoint.x; + m.f = y - fromPoint.y; + return m; + }, + _align = (rawPath, target, {align, matrix, offsetX, offsetY, alignOrigin}) => { + let x = rawPath[0][0], + y = rawPath[0][1], + curX = _getPropNum(target, "x"), + curY = _getPropNum(target, "y"), + alignTarget, m, p; + if (!rawPath || !rawPath.length) { + return getRawPath("M0,0L0,0"); + } + if (align) { + if (align === "self" || ((alignTarget = _toArray(align)[0] || target) === target)) { + transformRawPath(rawPath, 1, 0, 0, 1, curX - x, curY - y); + } else { + if (alignOrigin && alignOrigin[2] !== false) { + gsap.set(target, {transformOrigin:(alignOrigin[0] * 100) + "% " + (alignOrigin[1] * 100) + "%"}); + } else { + alignOrigin = [_getPropNum(target, "xPercent") / -100, _getPropNum(target, "yPercent") / -100]; + } + m = _getAlignMatrix(target, alignTarget, alignOrigin, "auto"); + p = m.apply({x: x, y: y}); + transformRawPath(rawPath, m.a, m.b, m.c, m.d, curX + m.e - (p.x - m.e), curY + m.f - (p.y - m.f)); + } + } + if (matrix) { + transformRawPath(rawPath, matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f); + } else if (offsetX || offsetY) { + transformRawPath(rawPath, 1, 0, 0, 1, offsetX || 0, offsetY || 0); + } + return rawPath; + }, + _addDimensionalPropTween = (plugin, target, property, rawPath, pathProperty, forceUnit) => { + let cache = target._gsap, + harness = cache.harness, + alias = (harness && harness.aliases && harness.aliases[property]), + prop = alias && alias.indexOf(",") < 0 ? alias : property, + pt = plugin._pt = new PropTween(plugin._pt, target, prop, 0, 0, _emptyFunc, 0, cache.set(target, prop, plugin)); + pt.u = _getUnit(cache.get(target, prop, forceUnit)) || 0; + pt.path = rawPath; + pt.pp = pathProperty; + plugin._props.push(prop); + }, + _sliceModifier = (start, end) => rawPath => (start || end !== 1) ? sliceRawPath(rawPath, start, end) : rawPath; + + +export const MotionPathPlugin = { + version: "3.12.7", + name: "motionPath", + register(core, Plugin, propTween) { + gsap = core; + _getUnit = gsap.utils.getUnit; + _toArray = gsap.utils.toArray; + _getStyleSaver = gsap.core.getStyleSaver; + _reverting = gsap.core.reverting || function() {}; + PropTween = propTween; + }, + init(target, vars, tween) { + if (!gsap) { + console.warn("Please gsap.registerPlugin(MotionPathPlugin)"); + return false; + } + if (!(typeof(vars) === "object" && !vars.style) || !vars.path) { + vars = {path:vars}; + } + let rawPaths = [], + {path, autoRotate, unitX, unitY, x, y} = vars, + firstObj = path[0], + slicer = _sliceModifier(vars.start, ("end" in vars) ? vars.end : 1), + rawPath, p; + this.rawPaths = rawPaths; + this.target = target; + this.tween = tween; + this.styles = _getStyleSaver && _getStyleSaver(target, "transform"); + if ((this.rotate = (autoRotate || autoRotate === 0))) { //get the rotational data FIRST so that the setTransform() method is called in the correct order in the render() loop - rotation gets set last. + this.rOffset = parseFloat(autoRotate) || 0; + this.radians = !!vars.useRadians; + this.rProp = vars.rotation || "rotation"; // rotation property + this.rSet = target._gsap.set(target, this.rProp, this); // rotation setter + this.ru = _getUnit(target._gsap.get(target, this.rProp)) || 0; // rotation units + } + if (Array.isArray(path) && !("closed" in path) && typeof(firstObj) !== "number") { + for (p in firstObj) { + if (!x && ~_xProps.indexOf(p)) { + x = p; + } else if (!y && ~_yProps.indexOf(p)) { + y = p; + } + } + if (x && y) { //correlated values + rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray(_populateSegmentFromArray([], path, x, 0), path, y, 1), target, x, y, slicer, vars, unitX || _getUnit(path[0][x]), unitY || _getUnit(path[0][y]))); + } else { + x = y = 0; + } + for (p in firstObj) { + p !== x && p !== y && rawPaths.push(_segmentToRawPath(this, _populateSegmentFromArray([], path, p, 2), target, p, 0, slicer, vars, _getUnit(path[0][p]))); + } + } else { + rawPath = slicer(_align(getRawPath(vars.path), target, vars)); + cacheRawPathMeasurements(rawPath, vars.resolution); + rawPaths.push(rawPath); + _addDimensionalPropTween(this, target, vars.x || "x", rawPath, "x", vars.unitX || "px"); + _addDimensionalPropTween(this, target, vars.y || "y", rawPath, "y", vars.unitY || "px"); + } + tween.vars.immediateRender && this.render(tween.progress(), this); + }, + render(ratio, data) { + let rawPaths = data.rawPaths, + i = rawPaths.length, + pt = data._pt; + if (data.tween._time || !_reverting()) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + while (i--) { + getPositionOnPath(rawPaths[i], ratio, !i && data.rotate, rawPaths[i]); + } + while (pt) { + pt.set(pt.t, pt.p, pt.path[pt.pp] + pt.u, pt.d, ratio); + pt = pt._next; + } + data.rotate && data.rSet(data.target, data.rProp, rawPaths[0].angle * (data.radians ? _DEG2RAD : 1) + data.rOffset + data.ru, data, ratio); + } else { + data.styles.revert(); + } + }, + getLength(path) { + return cacheRawPathMeasurements(getRawPath(path)).totalLength; + }, + sliceRawPath, + getRawPath, + pointsToSegment, + stringToRawPath, + rawPathToString, + transformRawPath, + getGlobalMatrix, + getPositionOnPath, + cacheRawPathMeasurements, + convertToPath: (targets, swap) => _toArray(targets).map(target => convertToPath(target, swap !== false)), + convertCoordinates(fromElement, toElement, point) { + let m = getGlobalMatrix(toElement, true, true).multiply(getGlobalMatrix(fromElement)); + return point ? m.apply(point) : m; + }, + getAlignMatrix: _getAlignMatrix, + getRelativePosition(fromElement, toElement, fromOrigin, toOrigin) { + let m =_getAlignMatrix(fromElement, toElement, fromOrigin, toOrigin); + return {x: m.e, y: m.f}; + }, + arrayToRawPath(value, vars) { + vars = vars || {}; + let segment = _populateSegmentFromArray(_populateSegmentFromArray([], value, vars.x || "x", 0), value, vars.y || "y", 1); + vars.relative && _relativize(segment); + return [(vars.type === "cubic") ? segment : pointsToSegment(segment, vars.curviness)]; + } +}; + +_getGSAP() && gsap.registerPlugin(MotionPathPlugin); + +export { MotionPathPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/Observer.js b/node_modules/gsap/src/Observer.js new file mode 100644 index 0000000000000000000000000000000000000000..0e7f73cf99decd81ff0c9096d8c8e8af540ca300 --- /dev/null +++ b/node_modules/gsap/src/Observer.js @@ -0,0 +1,431 @@ +/*! + * Observer 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let gsap, _coreInitted, _clamp, _win, _doc, _docEl, _body, _isTouch, _pointerType, ScrollTrigger, _root, _normalizer, _eventTypes, _context, + _getGSAP = () => gsap || (typeof(window) !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _startup = 1, + _observers = [], + _scrollers = [], + _proxies = [], + _getTime = Date.now, + _bridge = (name, value) => value, + _integrate = () => { + let core = ScrollTrigger.core, + data = core.bridge || {}, + scrollers = core._scrollers, + proxies = core._proxies; + scrollers.push(..._scrollers); + proxies.push(..._proxies); + _scrollers = scrollers; + _proxies = proxies; + _bridge = (name, value) => data[name](value); + }, + _getProxyProp = (element, property) => ~_proxies.indexOf(element) && _proxies[_proxies.indexOf(element) + 1][property], + _isViewport = el => !!~_root.indexOf(el), + _addListener = (element, type, func, passive, capture) => element.addEventListener(type, func, {passive: passive !== false, capture: !!capture}), + _removeListener = (element, type, func, capture) => element.removeEventListener(type, func, !!capture), + _scrollLeft = "scrollLeft", + _scrollTop = "scrollTop", + _onScroll = () => (_normalizer && _normalizer.isPressed) || _scrollers.cache++, + _scrollCacheFunc = (f, doNotCache) => { + let cachingFunc = value => { // since reading the scrollTop/scrollLeft/pageOffsetY/pageOffsetX can trigger a layout, this function allows us to cache the value so it only gets read fresh after a "scroll" event fires (or while we're refreshing because that can lengthen the page and alter the scroll position). when "soft" is true, that means don't actually set the scroll, but cache the new value instead (useful in ScrollSmoother) + if (value || value === 0) { + _startup && (_win.history.scrollRestoration = "manual"); // otherwise the new position will get overwritten by the browser onload. + let isNormalizing = _normalizer && _normalizer.isPressed; + value = cachingFunc.v = Math.round(value) || (_normalizer && _normalizer.iOS ? 1 : 0); //TODO: iOS Bug: if you allow it to go to 0, Safari can start to report super strange (wildly inaccurate) touch positions! + f(value); + cachingFunc.cacheID = _scrollers.cache; + isNormalizing && _bridge("ss", value); // set scroll (notify ScrollTrigger so it can dispatch a "scrollStart" event if necessary + } else if (doNotCache || _scrollers.cache !== cachingFunc.cacheID || _bridge("ref")) { + cachingFunc.cacheID = _scrollers.cache; + cachingFunc.v = f(); + } + return cachingFunc.v + cachingFunc.offset; + }; + cachingFunc.offset = 0; + return f && cachingFunc; + }, + _horizontal = {s: _scrollLeft, p: "left", p2: "Left", os: "right", os2: "Right", d: "width", d2: "Width", a: "x", sc: _scrollCacheFunc(function(value) { return arguments.length ? _win.scrollTo(value, _vertical.sc()) : _win.pageXOffset || _doc[_scrollLeft] || _docEl[_scrollLeft] || _body[_scrollLeft] || 0})}, + _vertical = {s: _scrollTop, p: "top", p2: "Top", os: "bottom", os2: "Bottom", d: "height", d2: "Height", a: "y", op: _horizontal, sc: _scrollCacheFunc(function(value) { return arguments.length ? _win.scrollTo(_horizontal.sc(), value) : _win.pageYOffset || _doc[_scrollTop] || _docEl[_scrollTop] || _body[_scrollTop] || 0})}, + _getTarget = (t, self) => ((self && self._ctx && self._ctx.selector) || gsap.utils.toArray)(t)[0] || (typeof(t) === "string" && gsap.config().nullTargetWarn !== false ? console.warn("Element not found:", t) : null), + + _getScrollFunc = (element, {s, sc}) => { // we store the scroller functions in an alternating sequenced Array like [element, verticalScrollFunc, horizontalScrollFunc, ...] so that we can minimize memory, maximize performance, and we also record the last position as a ".rec" property in order to revert to that after refreshing to ensure things don't shift around. + _isViewport(element) && (element = _doc.scrollingElement || _docEl); + let i = _scrollers.indexOf(element), + offset = sc === _vertical.sc ? 1 : 2; + !~i && (i = _scrollers.push(element) - 1); + _scrollers[i + offset] || _addListener(element, "scroll", _onScroll); // clear the cache when a scroll occurs + let prev = _scrollers[i + offset], + func = prev || (_scrollers[i + offset] = _scrollCacheFunc(_getProxyProp(element, s), true) || (_isViewport(element) ? sc : _scrollCacheFunc(function(value) { return arguments.length ? (element[s] = value) : element[s]; }))); + func.target = element; + prev || (func.smooth = gsap.getProperty(element, "scrollBehavior") === "smooth"); // only set it the first time (don't reset every time a scrollFunc is requested because perhaps it happens during a refresh() when it's disabled in ScrollTrigger. + return func; + }, + _getVelocityProp = (value, minTimeRefresh, useDelta) => { + let v1 = value, + v2 = value, + t1 = _getTime(), + t2 = t1, + min = minTimeRefresh || 50, + dropToZeroTime = Math.max(500, min * 3), + update = (value, force) => { + let t = _getTime(); + if (force || t - t1 > min) { + v2 = v1; + v1 = value; + t2 = t1; + t1 = t; + } else if (useDelta) { + v1 += value; + } else { // not totally necessary, but makes it a bit more accurate by adjusting the v1 value according to the new slope. This way we're not just ignoring the incoming data. Removing for now because it doesn't seem to make much practical difference and it's probably not worth the kb. + v1 = v2 + (value - v2) / (t - t2) * (t1 - t2); + } + }, + reset = () => { v2 = v1 = useDelta ? 0 : v1; t2 = t1 = 0; }, + getVelocity = latestValue => { + let tOld = t2, + vOld = v2, + t = _getTime(); + (latestValue || latestValue === 0) && latestValue !== v1 && update(latestValue); + return (t1 === t2 || t - t2 > dropToZeroTime) ? 0 : (v1 + (useDelta ? vOld : -vOld)) / ((useDelta ? t : t1) - tOld) * 1000; + }; + return {update, reset, getVelocity}; + }, + _getEvent = (e, preventDefault) => { + preventDefault && !e._gsapAllow && e.preventDefault(); + return e.changedTouches ? e.changedTouches[0] : e; + }, + _getAbsoluteMax = a => { + let max = Math.max(...a), + min = Math.min(...a); + return Math.abs(max) >= Math.abs(min) ? max : min; + }, + _setScrollTrigger = () => { + ScrollTrigger = gsap.core.globals().ScrollTrigger; + ScrollTrigger && ScrollTrigger.core && _integrate(); + }, + _initCore = core => { + gsap = core || _getGSAP(); + if (!_coreInitted && gsap && typeof(document) !== "undefined" && document.body) { + _win = window; + _doc = document; + _docEl = _doc.documentElement; + _body = _doc.body; + _root = [_win, _doc, _docEl, _body]; + _clamp = gsap.utils.clamp; + _context = gsap.core.context || function() {}; + _pointerType = "onpointerenter" in _body ? "pointer" : "mouse"; + // isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer. + _isTouch = Observer.isTouch = _win.matchMedia && _win.matchMedia("(hover: none), (pointer: coarse)").matches ? 1 : ("ontouchstart" in _win || navigator.maxTouchPoints > 0 || navigator.msMaxTouchPoints > 0) ? 2 : 0; + _eventTypes = Observer.eventTypes = ("ontouchstart" in _docEl ? "touchstart,touchmove,touchcancel,touchend" : !("onpointerdown" in _docEl) ? "mousedown,mousemove,mouseup,mouseup" : "pointerdown,pointermove,pointercancel,pointerup").split(","); + setTimeout(() => _startup = 0, 500); + _setScrollTrigger(); + _coreInitted = 1; + } + return _coreInitted; + }; + +_horizontal.op = _vertical; +_scrollers.cache = 0; + +export class Observer { + constructor(vars) { + this.init(vars); + } + + init(vars) { + _coreInitted || _initCore(gsap) || console.warn("Please gsap.registerPlugin(Observer)"); + ScrollTrigger || _setScrollTrigger(); + let {tolerance, dragMinimum, type, target, lineHeight, debounce, preventDefault, onStop, onStopDelay, ignore, wheelSpeed, event, onDragStart, onDragEnd, onDrag, onPress, onRelease, onRight, onLeft, onUp, onDown, onChangeX, onChangeY, onChange, onToggleX, onToggleY, onHover, onHoverEnd, onMove, ignoreCheck, isNormalizer, onGestureStart, onGestureEnd, onWheel, onEnable, onDisable, onClick, scrollSpeed, capture, allowClicks, lockAxis, onLockAxis} = vars; + this.target = target = _getTarget(target) || _docEl; + this.vars = vars; + ignore && (ignore = gsap.utils.toArray(ignore)); + tolerance = tolerance || 1e-9; + dragMinimum = dragMinimum || 0; + wheelSpeed = wheelSpeed || 1; + scrollSpeed = scrollSpeed || 1; + type = type || "wheel,touch,pointer"; + debounce = debounce !== false; + lineHeight || (lineHeight = parseFloat(_win.getComputedStyle(_body).lineHeight) || 22); // note: browser may report "normal", so default to 22. + let id, onStopDelayedCall, dragged, moved, wheeled, locked, axis, + self = this, + prevDeltaX = 0, + prevDeltaY = 0, + passive = vars.passive || (!preventDefault && vars.passive !== false), + scrollFuncX = _getScrollFunc(target, _horizontal), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollX = scrollFuncX(), + scrollY = scrollFuncY(), + limitToTouch = ~type.indexOf("touch") && !~type.indexOf("pointer") && _eventTypes[0] === "pointerdown", // for devices that accommodate mouse events and touch events, we need to distinguish. + isViewport = _isViewport(target), + ownerDoc = target.ownerDocument || _doc, + deltaX = [0, 0, 0], // wheel, scroll, pointer/touch + deltaY = [0, 0, 0], + onClickTime = 0, + clickCapture = () => onClickTime = _getTime(), + _ignoreCheck = (e, isPointerOrTouch) => (self.event = e) && (ignore && ~ignore.indexOf(e.target)) || (isPointerOrTouch && limitToTouch && e.pointerType !== "touch") || (ignoreCheck && ignoreCheck(e, isPointerOrTouch)), + onStopFunc = () => { + self._vx.reset(); + self._vy.reset(); + onStopDelayedCall.pause(); + onStop && onStop(self); + }, + update = () => { + let dx = self.deltaX = _getAbsoluteMax(deltaX), + dy = self.deltaY = _getAbsoluteMax(deltaY), + changedX = Math.abs(dx) >= tolerance, + changedY = Math.abs(dy) >= tolerance; + onChange && (changedX || changedY) && onChange(self, dx, dy, deltaX, deltaY); // in ScrollTrigger.normalizeScroll(), we need to know if it was touch/pointer so we need access to the deltaX/deltaY Arrays before we clear them out. + if (changedX) { + onRight && self.deltaX > 0 && onRight(self); + onLeft && self.deltaX < 0 && onLeft(self); + onChangeX && onChangeX(self); + onToggleX && ((self.deltaX < 0) !== (prevDeltaX < 0)) && onToggleX(self); + prevDeltaX = self.deltaX; + deltaX[0] = deltaX[1] = deltaX[2] = 0 + } + if (changedY) { + onDown && self.deltaY > 0 && onDown(self); + onUp && self.deltaY < 0 && onUp(self); + onChangeY && onChangeY(self); + onToggleY && ((self.deltaY < 0) !== (prevDeltaY < 0)) && onToggleY(self); + prevDeltaY = self.deltaY; + deltaY[0] = deltaY[1] = deltaY[2] = 0 + } + if (moved || dragged) { + onMove && onMove(self); + if (dragged) { + onDragStart && dragged === 1 && onDragStart(self); + onDrag && onDrag(self); + dragged = 0; + } + moved = false; + } + locked && !(locked = false) && onLockAxis && onLockAxis(self); + if (wheeled) { + onWheel(self); + wheeled = false; + } + id = 0; + }, + onDelta = (x, y, index) => { + deltaX[index] += x; + deltaY[index] += y; + self._vx.update(x); + self._vy.update(y); + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + onTouchOrPointerDelta = (x, y) => { + if (lockAxis && !axis) { + self.axis = axis = Math.abs(x) > Math.abs(y) ? "x" : "y"; + locked = true; + } + if (axis !== "y") { + deltaX[2] += x; + self._vx.update(x, true); // update the velocity as frequently as possible instead of in the debounced function so that very quick touch-scrolls (flicks) feel natural. If it's the mouse/touch/pointer, force it so that we get snappy/accurate momentum scroll. + } + if (axis !== "x") { + deltaY[2] += y; + self._vy.update(y, true); + } + debounce ? id || (id = requestAnimationFrame(update)) : update(); + }, + _onDrag = e => { + if (_ignoreCheck(e, 1)) {return;} + e = _getEvent(e, preventDefault); + let x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y, + isDragging = self.isDragging; + self.x = x; + self.y = y; + if (isDragging || ((dx || dy) && (Math.abs(self.startX - x) >= dragMinimum || Math.abs(self.startY - y) >= dragMinimum))) { + dragged = isDragging ? 2 : 1; // dragged: 0 = not dragging, 1 = first drag, 2 = normal drag + isDragging || (self.isDragging = true); + onTouchOrPointerDelta(dx, dy); + } + }, + _onPress = self.onPress = e => { + if (_ignoreCheck(e, 1) || (e && e.button)) {return;} + self.axis = axis = null; + onStopDelayedCall.pause(); + self.isPressed = true; + e = _getEvent(e); // note: may need to preventDefault(?) Won't side-scroll on iOS Safari if we do, though. + prevDeltaX = prevDeltaY = 0; + self.startX = self.x = e.clientX; + self.startY = self.y = e.clientY; + self._vx.reset(); // otherwise the t2 may be stale if the user touches and flicks super fast and releases in less than 2 requestAnimationFrame ticks, causing velocity to be 0. + self._vy.reset(); + _addListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, passive, true); + self.deltaX = self.deltaY = 0; + onPress && onPress(self); + }, + _onRelease = self.onRelease = e => { + if (_ignoreCheck(e, 1)) {return;} + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + let isTrackingDrag = !isNaN(self.y - self.startY), + wasDragging = self.isDragging, + isDragNotClick = wasDragging && (Math.abs(self.x - self.startX) > 3 || Math.abs(self.y - self.startY) > 3), // some touch devices need some wiggle room in terms of sensing clicks - the finger may move a few pixels. + eventData = _getEvent(e); + if (!isDragNotClick && isTrackingDrag) { + self._vx.reset(); + self._vy.reset(); + //if (preventDefault && allowClicks && self.isPressed) { // check isPressed because in a rare edge case, the inputObserver in ScrollTrigger may stopPropagation() on the press/drag, so the onRelease may get fired without the onPress/onDrag ever getting called, thus it could trigger a click to occur on a link after scroll-dragging it. + if (preventDefault && allowClicks) { + gsap.delayedCall(0.08, () => { // some browsers (like Firefox) won't trust script-generated clicks, so if the user tries to click on a video to play it, for example, it simply won't work. Since a regular "click" event will most likely be generated anyway (one that has its isTrusted flag set to true), we must slightly delay our script-generated click so that the "real"/trusted one is prioritized. Remember, when there are duplicate events in quick succession, we suppress all but the first one. Some browsers don't even trigger the "real" one at all, so our synthetic one is a safety valve that ensures that no matter what, a click event does get dispatched. + if (_getTime() - onClickTime > 300 && !e.defaultPrevented) { + if (e.target.click) { //some browsers (like mobile Safari) don't properly trigger the click event + e.target.click(); + } else if (ownerDoc.createEvent) { + let syntheticEvent = ownerDoc.createEvent("MouseEvents"); + syntheticEvent.initMouseEvent("click", true, true, _win, 1, eventData.screenX, eventData.screenY, eventData.clientX, eventData.clientY, false, false, false, false, 0, null); + e.target.dispatchEvent(syntheticEvent); + } + } + }); + } + } + self.isDragging = self.isGesturing = self.isPressed = false; + onStop && wasDragging && !isNormalizer && onStopDelayedCall.restart(true); + dragged && update(); // in case debouncing, we don't want onDrag to fire AFTER onDragEnd(). + onDragEnd && wasDragging && onDragEnd(self); + onRelease && onRelease(self, isDragNotClick); + }, + _onGestureStart = e => e.touches && e.touches.length > 1 && (self.isGesturing = true) && onGestureStart(e, self.isDragging), + _onGestureEnd = () => (self.isGesturing = false) || onGestureEnd(self), + onScroll = e => { + if (_ignoreCheck(e)) {return;} + let x = scrollFuncX(), + y = scrollFuncY(); + onDelta((x - scrollX) * scrollSpeed, (y - scrollY) * scrollSpeed, 1); + scrollX = x; + scrollY = y; + onStop && onStopDelayedCall.restart(true); + }, + _onWheel = e => { + if (_ignoreCheck(e)) {return;} + e = _getEvent(e, preventDefault); + onWheel && (wheeled = true); + let multiplier = (e.deltaMode === 1 ? lineHeight : e.deltaMode === 2 ? _win.innerHeight : 1) * wheelSpeed; + onDelta(e.deltaX * multiplier, e.deltaY * multiplier, 0); + onStop && !isNormalizer && onStopDelayedCall.restart(true); + }, + _onMove = e => { + if (_ignoreCheck(e)) {return;} + let x = e.clientX, + y = e.clientY, + dx = x - self.x, + dy = y - self.y; + self.x = x; + self.y = y; + moved = true; + onStop && onStopDelayedCall.restart(true); + (dx || dy) && onTouchOrPointerDelta(dx, dy); + }, + _onHover = e => {self.event = e; onHover(self);}, + _onHoverEnd = e => {self.event = e; onHoverEnd(self);}, + _onClick = e => _ignoreCheck(e) || (_getEvent(e, preventDefault) && onClick(self)); + + onStopDelayedCall = self._dc = gsap.delayedCall(onStopDelay || 0.25, onStopFunc).pause(); + + self.deltaX = self.deltaY = 0; + self._vx = _getVelocityProp(0, 50, true); + self._vy = _getVelocityProp(0, 50, true); + self.scrollX = scrollFuncX; + self.scrollY = scrollFuncY; + self.isDragging = self.isGesturing = self.isPressed = false; + _context(this); + self.enable = e => { + if (!self.isEnabled) { + _addListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + type.indexOf("scroll") >= 0 && _addListener(isViewport ? ownerDoc : target, "scroll", onScroll, passive, capture); + type.indexOf("wheel") >= 0 && _addListener(target, "wheel", _onWheel, passive, capture); + if ((type.indexOf("touch") >= 0 && _isTouch) || type.indexOf("pointer") >= 0) { + _addListener(target, _eventTypes[0], _onPress, passive, capture); + _addListener(ownerDoc, _eventTypes[2], _onRelease); + _addListener(ownerDoc, _eventTypes[3], _onRelease); + allowClicks && _addListener(target, "click", clickCapture, true, true); + onClick && _addListener(target, "click", _onClick); + onGestureStart && _addListener(ownerDoc, "gesturestart", _onGestureStart); + onGestureEnd && _addListener(ownerDoc, "gestureend", _onGestureEnd); + onHover && _addListener(target, _pointerType + "enter", _onHover); + onHoverEnd && _addListener(target, _pointerType + "leave", _onHoverEnd); + onMove && _addListener(target, _pointerType + "move", _onMove); + } + self.isEnabled = true; + self.isDragging = self.isGesturing = self.isPressed = moved = dragged = false; + self._vx.reset(); + self._vy.reset(); + scrollX = scrollFuncX(); + scrollY = scrollFuncY(); + e && e.type && _onPress(e); + onEnable && onEnable(self); + } + return self; + }; + self.disable = () => { + if (self.isEnabled) { + // only remove the _onScroll listener if there aren't any others that rely on the functionality. + _observers.filter(o => o !== self && _isViewport(o.target)).length || _removeListener(isViewport ? ownerDoc : target, "scroll", _onScroll); + if (self.isPressed) { + self._vx.reset(); + self._vy.reset(); + _removeListener(isNormalizer ? target : ownerDoc, _eventTypes[1], _onDrag, true); + } + _removeListener(isViewport ? ownerDoc : target, "scroll", onScroll, capture); + _removeListener(target, "wheel", _onWheel, capture); + _removeListener(target, _eventTypes[0], _onPress, capture); + _removeListener(ownerDoc, _eventTypes[2], _onRelease); + _removeListener(ownerDoc, _eventTypes[3], _onRelease); + _removeListener(target, "click", clickCapture, true); + _removeListener(target, "click", _onClick); + _removeListener(ownerDoc, "gesturestart", _onGestureStart); + _removeListener(ownerDoc, "gestureend", _onGestureEnd); + _removeListener(target, _pointerType + "enter", _onHover); + _removeListener(target, _pointerType + "leave", _onHoverEnd); + _removeListener(target, _pointerType + "move", _onMove); + self.isEnabled = self.isPressed = self.isDragging = false; + onDisable && onDisable(self); + } + }; + + self.kill = self.revert = () => { + self.disable(); + let i = _observers.indexOf(self); + i >= 0 && _observers.splice(i, 1); + _normalizer === self && (_normalizer = 0); + } + + _observers.push(self); + isNormalizer && _isViewport(target) && (_normalizer = self); + + self.enable(event); + } + + get velocityX() { + return this._vx.getVelocity(); + } + get velocityY() { + return this._vy.getVelocity(); + } + +} + +Observer.version = "3.12.7"; +Observer.create = vars => new Observer(vars); +Observer.register = _initCore; +Observer.getAll = () => _observers.slice(); +Observer.getById = id => _observers.filter(o => o.vars.id === id)[0]; + +_getGSAP() && gsap.registerPlugin(Observer); + +export { Observer as default, _isViewport, _scrollers, _getScrollFunc, _getProxyProp, _proxies, _getVelocityProp, _vertical, _horizontal, _getTarget }; \ No newline at end of file diff --git a/node_modules/gsap/src/PixiPlugin.js b/node_modules/gsap/src/PixiPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..e1b10bf1572ad94fb8ab206aaab2c4cf90cd75cc --- /dev/null +++ b/node_modules/gsap/src/PixiPlugin.js @@ -0,0 +1,340 @@ +/*! + * PixiPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let gsap, _splitColor, _coreInitted, _PIXI, PropTween, _getSetter, _isV4, _isV8Plus, + _windowExists = () => typeof(window) !== "undefined", + _getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _isFunction = value => typeof(value) === "function", + _warn = message => console.warn(message), + _idMatrix = [1,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,0,1,0], + _lumR = 0.212671, + _lumG = 0.715160, + _lumB = 0.072169, + _filterClass = name => _isFunction(_PIXI[name]) ? _PIXI[name] : _PIXI.filters[name], // in PIXI 7.1, filters moved from PIXI.filters to just PIXI + _applyMatrix = (m, m2) => { + let temp = [], + i = 0, + z = 0, + y, x; + for (y = 0; y < 4; y++) { + for (x = 0; x < 5; x++) { + z = (x === 4) ? m[i + 4] : 0; + temp[i + x] = m[i] * m2[x] + m[i+1] * m2[x + 5] + m[i+2] * m2[x + 10] + m[i+3] * m2[x + 15] + z; + } + i += 5; + } + return temp; + }, + _setSaturation = (m, n) => { + let inv = 1 - n, + r = inv * _lumR, + g = inv * _lumG, + b = inv * _lumB; + return _applyMatrix([r + n, g, b, 0, 0, r, g + n, b, 0, 0, r, g, b + n, 0, 0, 0, 0, 0, 1, 0], m); + }, + _colorize = (m, color, amount) => { + let c = _splitColor(color), + r = c[0] / 255, + g = c[1] / 255, + b = c[2] / 255, + inv = 1 - amount; + return _applyMatrix([inv + amount * r * _lumR, amount * r * _lumG, amount * r * _lumB, 0, 0, amount * g * _lumR, inv + amount * g * _lumG, amount * g * _lumB, 0, 0, amount * b * _lumR, amount * b * _lumG, inv + amount * b * _lumB, 0, 0, 0, 0, 0, 1, 0], m); + }, + _setHue = (m, n) => { + n *= Math.PI / 180; + let c = Math.cos(n), + s = Math.sin(n); + return _applyMatrix([(_lumR + (c * (1 - _lumR))) + (s * (-_lumR)), (_lumG + (c * (-_lumG))) + (s * (-_lumG)), (_lumB + (c * (-_lumB))) + (s * (1 - _lumB)), 0, 0, (_lumR + (c * (-_lumR))) + (s * 0.143), (_lumG + (c * (1 - _lumG))) + (s * 0.14), (_lumB + (c * (-_lumB))) + (s * -0.283), 0, 0, (_lumR + (c * (-_lumR))) + (s * (-(1 - _lumR))), (_lumG + (c * (-_lumG))) + (s * _lumG), (_lumB + (c * (1 - _lumB))) + (s * _lumB), 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], m); + }, + _setContrast = (m, n) => _applyMatrix([n,0,0,0,0.5 * (1 - n), 0,n,0,0,0.5 * (1 - n), 0,0,n,0,0.5 * (1 - n), 0,0,0,1,0], m), + _getFilter = (target, type) => { + let filterClass = _filterClass(type), + filters = target.filters || [], + i = filters.length, + filter; + filterClass || _warn(type + " not found. PixiPlugin.registerPIXI(PIXI)"); + while (--i > -1) { + if (filters[i] instanceof filterClass) { + return filters[i]; + } + } + filter = new filterClass(); + if (type === "BlurFilter") { + filter.blur = 0; + } + filters.push(filter); + target.filters = filters; + return filter; + }, + _addColorMatrixFilterCacheTween = (p, plugin, cache, vars) => { //we cache the ColorMatrixFilter components in a _gsColorMatrixFilter object attached to the target object so that it's easy to grab the current value at any time. + plugin.add(cache, p, cache[p], vars[p]); + plugin._props.push(p); + }, + _applyBrightnessToMatrix = (brightness, matrix) => { + let filterClass = _filterClass("ColorMatrixFilter"), + temp = new filterClass(); + temp.matrix = matrix; + temp.brightness(brightness, true); + return temp.matrix; + }, + _copy = obj => { + let copy = {}, + p; + for (p in obj) { + copy[p] = obj[p]; + } + return copy; + }, + _CMFdefaults = {contrast:1, saturation:1, colorizeAmount:0, colorize:"rgb(255,255,255)", hue:0, brightness:1}, + _parseColorMatrixFilter = (target, v, pg) => { + let filter = _getFilter(target, "ColorMatrixFilter"), + cache = target._gsColorMatrixFilter = target._gsColorMatrixFilter || _copy(_CMFdefaults), + combine = v.combineCMF && !("colorMatrixFilter" in v && !v.colorMatrixFilter), + i, matrix, startMatrix; + startMatrix = filter.matrix; + if (v.resolution) { + filter.resolution = v.resolution; + } + if (v.matrix && v.matrix.length === startMatrix.length) { + matrix = v.matrix; + if (cache.contrast !== 1) { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + if (cache.hue) { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + if (cache.brightness !== 1) { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + if (cache.colorizeAmount) { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + if (cache.saturation !== 1) { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + + } else { + matrix = _idMatrix.slice(); + if (v.contrast != null) { + matrix = _setContrast(matrix, +v.contrast); + _addColorMatrixFilterCacheTween("contrast", pg, cache, v); + } else if (cache.contrast !== 1) { + if (combine) { + matrix = _setContrast(matrix, cache.contrast); + } else { + _addColorMatrixFilterCacheTween("contrast", pg, cache, _CMFdefaults); + } + } + if (v.hue != null) { + matrix = _setHue(matrix, +v.hue); + _addColorMatrixFilterCacheTween("hue", pg, cache, v); + } else if (cache.hue) { + if (combine) { + matrix = _setHue(matrix, cache.hue); + } else { + _addColorMatrixFilterCacheTween("hue", pg, cache, _CMFdefaults); + } + } + if (v.brightness != null) { + matrix = _applyBrightnessToMatrix(+v.brightness, matrix); + _addColorMatrixFilterCacheTween("brightness", pg, cache, v); + } else if (cache.brightness !== 1) { + if (combine) { + matrix = _applyBrightnessToMatrix(cache.brightness, matrix); + } else { + _addColorMatrixFilterCacheTween("brightness", pg, cache, _CMFdefaults); + } + } + if (v.colorize != null) { + v.colorizeAmount = ("colorizeAmount" in v) ? +v.colorizeAmount : 1; + matrix = _colorize(matrix, v.colorize, v.colorizeAmount); + _addColorMatrixFilterCacheTween("colorize", pg, cache, v); + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, v); + } else if (cache.colorizeAmount) { + if (combine) { + matrix = _colorize(matrix, cache.colorize, cache.colorizeAmount); + } else { + _addColorMatrixFilterCacheTween("colorize", pg, cache, _CMFdefaults); + _addColorMatrixFilterCacheTween("colorizeAmount", pg, cache, _CMFdefaults); + } + } + if (v.saturation != null) { + matrix = _setSaturation(matrix, +v.saturation); + _addColorMatrixFilterCacheTween("saturation", pg, cache, v); + } else if (cache.saturation !== 1) { + if (combine) { + matrix = _setSaturation(matrix, cache.saturation); + } else { + _addColorMatrixFilterCacheTween("saturation", pg, cache, _CMFdefaults); + } + } + } + i = matrix.length; + while (--i > -1) { + if (matrix[i] !== startMatrix[i]) { + pg.add(startMatrix, i, startMatrix[i], matrix[i], "colorMatrixFilter"); + } + } + pg._props.push("colorMatrixFilter"); + }, + _renderColor = (ratio, {t, p, color, set}) => { + set(t, p, color[0] << 16 | color[1] << 8 | color[2]); + }, + _renderDirtyCache = (ratio, {g}) => { + if (_isV8Plus) { + g.fill(); + g.stroke(); + } else if (g) { // in order for PixiJS to actually redraw GraphicsData, we've gotta increment the "dirty" and "clearDirty" values. If we don't do this, the values will be tween properly, but not rendered. + g.dirty++; + g.clearDirty++; + } + }, + _renderAutoAlpha = (ratio, data) => { + data.t.visible = !!data.t.alpha; + }, + _addColorTween = (target, p, value, plugin) => { + let currentValue = target[p], + startColor = _splitColor(_isFunction(currentValue) ? target[ ((p.indexOf("set") || !_isFunction(target["get" + p.substr(3)])) ? p : "get" + p.substr(3)) ]() : currentValue), + endColor = _splitColor(value); + plugin._pt = new PropTween(plugin._pt, target, p, 0, 0, _renderColor, {t:target, p:p, color:startColor, set:_getSetter(target, p)}); + plugin.add(startColor, 0, startColor[0], endColor[0]); + plugin.add(startColor, 1, startColor[1], endColor[1]); + plugin.add(startColor, 2, startColor[2], endColor[2]); + }, + + _colorProps = {tint:1, lineColor:1, fillColor:1, strokeColor:1}, + _xyContexts = "position,scale,skew,pivot,anchor,tilePosition,tileScale".split(","), + _contexts = {x:"position", y:"position", tileX:"tilePosition", tileY:"tilePosition"}, + _colorMatrixFilterProps = {colorMatrixFilter:1, saturation:1, contrast:1, hue:1, colorize:1, colorizeAmount:1, brightness:1, combineCMF:1}, + _DEG2RAD = Math.PI / 180, + _isString = value => typeof(value) === "string", + _degreesToRadians = value => (_isString(value) && value.charAt(1) === "=") ? value.substr(0, 2) + (parseFloat(value.substr(2)) * _DEG2RAD) : value * _DEG2RAD, + _renderPropWithEnd = (ratio, data) => data.set(data.t, data.p, ratio === 1 ? data.e : (Math.round((data.s + data.c * ratio) * 100000) / 100000), data), + _addRotationalPropTween = (plugin, target, property, startNum, endValue, radians) => { + let cap = 360 * (radians ? _DEG2RAD : 1), + isString = _isString(endValue), + relative = (isString && endValue.charAt(1) === "=") ? +(endValue.charAt(0) + "1") : 0, + endNum = parseFloat(relative ? endValue.substr(2) : endValue) * (radians ? _DEG2RAD : 1), + change = relative ? endNum * relative : endNum - startNum, + finalValue = startNum + change, + direction, pt; + if (isString) { + direction = endValue.split("_")[1]; + if (direction === "short") { + change %= cap; + if (change !== change % (cap / 2)) { + change += (change < 0) ? cap : -cap; + } + } + if (direction === "cw" && change < 0) { + change = ((change + cap * 1e10) % cap) - ~~(change / cap) * cap; + } else if (direction === "ccw" && change > 0) { + change = ((change - cap * 1e10) % cap) - ~~(change / cap) * cap; + } + } + plugin._pt = pt = new PropTween(plugin._pt, target, property, startNum, change, _renderPropWithEnd); + pt.e = finalValue; + return pt; + }, + _initCore = () => { + if (!_coreInitted) { + gsap = _getGSAP(); + _PIXI = _coreInitted = _PIXI || (_windowExists() && window.PIXI); + let version = (_PIXI && _PIXI.VERSION && parseFloat(_PIXI.VERSION.split(".")[0])) || 0; + _isV4 = version === 4; + _isV8Plus = version >= 8; + _splitColor = color => gsap.utils.splitColor((color + "").substr(0,2) === "0x" ? "#" + color.substr(2) : color); // some colors in PIXI are reported as "0xFF4421" instead of "#FF4421". + } + }, i, p; + +//context setup... +for (i = 0; i < _xyContexts.length; i++) { + p = _xyContexts[i]; + _contexts[p + "X"] = p; + _contexts[p + "Y"] = p; +} + + +export const PixiPlugin = { + version: "3.12.7", + name: "pixi", + register(core, Plugin, propTween) { + gsap = core; + PropTween = propTween; + _getSetter = Plugin.getSetter; + _initCore(); + }, + headless: true, // doesn't need window + registerPIXI(pixi) { + _PIXI = pixi; + }, + init(target, values, tween, index, targets) { + _PIXI || _initCore(); + if (!_PIXI) { + _warn("PIXI was not found. PixiPlugin.registerPIXI(PIXI);"); + return false; + } + let context, axis, value, colorMatrix, filter, p, padding, i, data, subProp; + for (p in values) { + context = _contexts[p]; + value = values[p]; + if (context) { + axis = ~p.charAt(p.length-1).toLowerCase().indexOf("x") ? "x" : "y"; + this.add(target[context], axis, target[context][axis], (context === "skew") ? _degreesToRadians(value) : value, 0, 0, 0, 0, 0, 1); + } else if (p === "scale" || p === "anchor" || p === "pivot" || p === "tileScale") { + this.add(target[p], "x", target[p].x, value); + this.add(target[p], "y", target[p].y, value); + } else if (p === "rotation" || p === "angle") { //PIXI expects rotation in radians, but as a convenience we let folks define it in degrees and we do the conversion. + _addRotationalPropTween(this, target, p, target[p], value, p === "rotation"); + } else if (_colorMatrixFilterProps[p]) { + if (!colorMatrix) { + _parseColorMatrixFilter(target, values.colorMatrixFilter || values, this); + colorMatrix = true; + } + } else if (p === "blur" || p === "blurX" || p === "blurY" || p === "blurPadding") { + filter = _getFilter(target, "BlurFilter"); + this.add(filter, p, filter[p], value); + if (values.blurPadding !== 0) { + padding = values.blurPadding || Math.max(filter[p], value) * 2; + i = target.filters.length; + while (--i > -1) { + target.filters[i].padding = Math.max(target.filters[i].padding, padding); //if we don't expand the padding on all the filters, it can look clipped. + } + } + } else if (_colorProps[p]) { + if ((p === "lineColor" || p === "fillColor" || p === "strokeColor") && target instanceof _PIXI.Graphics) { + data = "fillStyle" in target ? [target] : (target.geometry || target).graphicsData; //"geometry" was introduced in PIXI version 5 + subProp = p.substr(0, p.length - 5); + _isV8Plus && subProp === "line" && (subProp = "stroke"); // in v8, lineColor became strokeColor. + this._pt = new PropTween(this._pt, target, p, 0, 0, _renderDirtyCache, {g: target.geometry || target}); + i = data.length; + while (--i > -1) { + _addColorTween(_isV4 ? data[i] : data[i][subProp + "Style"], _isV4 ? p : "color", value, this); + } + } else { + _addColorTween(target, p, value, this); + } + } else if (p === "autoAlpha") { + this._pt = new PropTween(this._pt, target, "visible", 0, 0, _renderAutoAlpha); + this.add(target, "alpha", target.alpha, value); + this._props.push("alpha", "visible"); + } else if (p !== "resolution") { + this.add(target, p, "get", value); + } + this._props.push(p); + } + } +}; + +_getGSAP() && gsap.registerPlugin(PixiPlugin); + +export { PixiPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/ScrollToPlugin.js b/node_modules/gsap/src/ScrollToPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..33e5762b8fd70cf4f403be1260a1fcce2e51987a --- /dev/null +++ b/node_modules/gsap/src/ScrollToPlugin.js @@ -0,0 +1,197 @@ +/*! + * ScrollToPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let gsap, _coreInitted, _window, _docEl, _body, _toArray, _config, ScrollTrigger, + _windowExists = () => typeof(window) !== "undefined", + _getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _isString = value => typeof(value) === "string", + _isFunction = value => typeof(value) === "function", + _max = (element, axis) => { + let dim = (axis === "x") ? "Width" : "Height", + scroll = "scroll" + dim, + client = "client" + dim; + return (element === _window || element === _docEl || element === _body) ? Math.max(_docEl[scroll], _body[scroll]) - (_window["inner" + dim] || _docEl[client] || _body[client]) : element[scroll] - element["offset" + dim]; + }, + _buildGetter = (e, axis) => { //pass in an element and an axis ("x" or "y") and it'll return a getter function for the scroll position of that element (like scrollTop or scrollLeft, although if the element is the window, it'll use the pageXOffset/pageYOffset or the documentElement's scrollTop/scrollLeft or document.body's. Basically this streamlines things and makes a very fast getter across browsers. + let p = "scroll" + ((axis === "x") ? "Left" : "Top"); + if (e === _window) { + if (e.pageXOffset != null) { + p = "page" + axis.toUpperCase() + "Offset"; + } else { + e = _docEl[p] != null ? _docEl : _body; + } + } + return () => e[p]; + }, + _clean = (value, index, target, targets) => { + _isFunction(value) && (value = value(index, target, targets)); + if (typeof(value) !== "object") { + return _isString(value) && value !== "max" && value.charAt(1) !== "=" ? {x: value, y: value} : {y: value}; //if we don't receive an object as the parameter, assume the user intends "y". + } else if (value.nodeType) { + return {y: value, x: value}; + } else { + let result = {}, p; + for (p in value) { + result[p] = p !== "onAutoKill" && _isFunction(value[p]) ? value[p](index, target, targets) : value[p]; + } + return result; + } + }, + _getOffset = (element, container) => { + element = _toArray(element)[0]; + if (!element || !element.getBoundingClientRect) { + return console.warn("scrollTo target doesn't exist. Using 0") || {x:0, y:0}; + } + let rect = element.getBoundingClientRect(), + isRoot = (!container || container === _window || container === _body), + cRect = isRoot ? {top:_docEl.clientTop - (_window.pageYOffset || _docEl.scrollTop || _body.scrollTop || 0), left:_docEl.clientLeft - (_window.pageXOffset || _docEl.scrollLeft || _body.scrollLeft || 0)} : container.getBoundingClientRect(), + offsets = {x: rect.left - cRect.left, y: rect.top - cRect.top}; + if (!isRoot && container) { //only add the current scroll position if it's not the window/body. + offsets.x += _buildGetter(container, "x")(); + offsets.y += _buildGetter(container, "y")(); + } + return offsets; + }, + _parseVal = (value, target, axis, currentVal, offset) => !isNaN(value) && typeof(value) !== "object" ? parseFloat(value) - offset : (_isString(value) && value.charAt(1) === "=") ? parseFloat(value.substr(2)) * (value.charAt(0) === "-" ? -1 : 1) + currentVal - offset : (value === "max") ? _max(target, axis) - offset : Math.min(_max(target, axis), _getOffset(value, target)[axis] - offset), + _initCore = () => { + gsap = _getGSAP(); + if (_windowExists() && gsap && typeof(document) !== "undefined" && document.body) { + _window = window; + _body = document.body; + _docEl = document.documentElement; + _toArray = gsap.utils.toArray; + gsap.config({autoKillThreshold:7}); + _config = gsap.config(); + _coreInitted = 1; + } + }; + + +export const ScrollToPlugin = { + version: "3.12.7", + name: "scrollTo", + rawVars: 1, + register(core) { + gsap = core; + _initCore(); + }, + init(target, value, tween, index, targets) { + _coreInitted || _initCore(); + let data = this, + snapType = gsap.getProperty(target, "scrollSnapType"); + data.isWin = (target === _window); + data.target = target; + data.tween = tween; + value = _clean(value, index, target, targets); + data.vars = value; + data.autoKill = !!("autoKill" in value ? value : _config).autoKill; + data.getX = _buildGetter(target, "x"); + data.getY = _buildGetter(target, "y"); + data.x = data.xPrev = data.getX(); + data.y = data.yPrev = data.getY(); + ScrollTrigger || (ScrollTrigger = gsap.core.globals().ScrollTrigger); + gsap.getProperty(target, "scrollBehavior") === "smooth" && gsap.set(target, {scrollBehavior: "auto"}); + if (snapType && snapType !== "none") { // disable scroll snapping to avoid strange behavior + data.snap = 1; + data.snapInline = target.style.scrollSnapType; + target.style.scrollSnapType = "none"; + } + if (value.x != null) { + data.add(data, "x", data.x, _parseVal(value.x, target, "x", data.x, value.offsetX || 0), index, targets); + data._props.push("scrollTo_x"); + } else { + data.skipX = 1; + } + if (value.y != null) { + data.add(data, "y", data.y, _parseVal(value.y, target, "y", data.y, value.offsetY || 0), index, targets); + data._props.push("scrollTo_y"); + } else { + data.skipY = 1; + } + }, + render(ratio, data) { + let pt = data._pt, + { target, tween, autoKill, xPrev, yPrev, isWin, snap, snapInline } = data, + x, y, yDif, xDif, threshold; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + x = (isWin || !data.skipX) ? data.getX() : xPrev; + y = (isWin || !data.skipY) ? data.getY() : yPrev; + yDif = y - yPrev; + xDif = x - xPrev; + threshold = _config.autoKillThreshold; + if (data.x < 0) { //can't scroll to a position less than 0! Might happen if someone uses a Back.easeOut or Elastic.easeOut when scrolling back to the top of the page (for example) + data.x = 0; + } + if (data.y < 0) { + data.y = 0; + } + if (autoKill) { + //note: iOS has a bug that throws off the scroll by several pixels, so we need to check if it's within 7 pixels of the previous one that we set instead of just looking for an exact match. + if (!data.skipX && (xDif > threshold || xDif < -threshold) && x < _max(target, "x")) { + data.skipX = 1; //if the user scrolls separately, we should stop tweening! + } + if (!data.skipY && (yDif > threshold || yDif < -threshold) && y < _max(target, "y")) { + data.skipY = 1; //if the user scrolls separately, we should stop tweening! + } + if (data.skipX && data.skipY) { + tween.kill(); + data.vars.onAutoKill && data.vars.onAutoKill.apply(tween, data.vars.onAutoKillParams || []); + } + } + if (isWin) { + _window.scrollTo((!data.skipX) ? data.x : x, (!data.skipY) ? data.y : y); + } else { + data.skipY || (target.scrollTop = data.y); + data.skipX || (target.scrollLeft = data.x); + } + if (snap && (ratio === 1 || ratio === 0)) { + y = target.scrollTop; + x = target.scrollLeft; + snapInline ? (target.style.scrollSnapType = snapInline) : target.style.removeProperty("scroll-snap-type"); + target.scrollTop = y + 1; // bug in Safari causes the element to totally reset its scroll position when scroll-snap-type changes, so we need to set it to a slightly different value and then back again to work around this bug. + target.scrollLeft = x + 1; + target.scrollTop = y; + target.scrollLeft = x; + } + data.xPrev = data.x; + data.yPrev = data.y; + ScrollTrigger && ScrollTrigger.update(); + }, + kill(property) { + let both = (property === "scrollTo"), + i = this._props.indexOf(property); + if (both || property === "scrollTo_x") { + this.skipX = 1; + } + if (both || property === "scrollTo_y") { + this.skipY = 1; + } + i > -1 && this._props.splice(i, 1); + return !this._props.length; + } +}; + +ScrollToPlugin.max = _max; +ScrollToPlugin.getOffset = _getOffset; +ScrollToPlugin.buildGetter = _buildGetter; +ScrollToPlugin.config = vars => { + _config || _initCore() || (_config = gsap.config()); // in case the window hasn't been defined yet. + for (let p in vars) { + _config[p] = vars[p]; + } +} + +_getGSAP() && gsap.registerPlugin(ScrollToPlugin); + +export { ScrollToPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/ScrollTrigger.js b/node_modules/gsap/src/ScrollTrigger.js new file mode 100644 index 0000000000000000000000000000000000000000..98767bb5eddf873ab81d80b75d6c78bf338a37fc --- /dev/null +++ b/node_modules/gsap/src/ScrollTrigger.js @@ -0,0 +1,1783 @@ +/*! + * ScrollTrigger 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +import { Observer, _getTarget, _vertical, _horizontal, _scrollers, _proxies, _getScrollFunc, _getProxyProp, _getVelocityProp } from "./Observer.js"; + +let gsap, _coreInitted, _win, _doc, _docEl, _body, _root, _resizeDelay, _toArray, _clamp, _time2, _syncInterval, _refreshing, _pointerIsDown, _transformProp, _i, _prevWidth, _prevHeight, _autoRefresh, _sort, _suppressOverwrites, _ignoreResize, _normalizer, _ignoreMobileResize, _baseScreenHeight, _baseScreenWidth, _fixIOSBug, _context, _scrollRestoration, _div100vh, _100vh, _isReverted, _clampingMax, + _limitCallbacks, // if true, we'll only trigger callbacks if the active state toggles, so if you scroll immediately past both the start and end positions of a ScrollTrigger (thus inactive to inactive), neither its onEnter nor onLeave will be called. This is useful during startup. + _startup = 1, + _getTime = Date.now, + _time1 = _getTime(), + _lastScrollTime = 0, + _enabled = 0, + _parseClamp = (value, type, self) => { + let clamp = (_isString(value) && (value.substr(0, 6) === "clamp(" || value.indexOf("max") > -1)); + self["_" + type + "Clamp"] = clamp; + return clamp ? value.substr(6, value.length - 7) : value; + }, + _keepClamp = (value, clamp) => clamp && (!_isString(value) || value.substr(0, 6) !== "clamp(") ? "clamp(" + value + ")" : value, + _rafBugFix = () => _enabled && requestAnimationFrame(_rafBugFix), // in some browsers (like Firefox), screen repaints weren't consistent unless we had SOMETHING queued up in requestAnimationFrame()! So this just creates a super simple loop to keep it alive and smooth out repaints. + _pointerDownHandler = () => _pointerIsDown = 1, + _pointerUpHandler = () => _pointerIsDown = 0, + _passThrough = v => v, + _round = value => Math.round(value * 100000) / 100000 || 0, + _windowExists = () => typeof(window) !== "undefined", + _getGSAP = () => gsap || (_windowExists() && (gsap = window.gsap) && gsap.registerPlugin && gsap), + _isViewport = e => !!~_root.indexOf(e), + _getViewportDimension = dimensionProperty => (dimensionProperty === "Height" ? _100vh : _win["inner" + dimensionProperty]) || _docEl["client" + dimensionProperty] || _body["client" + dimensionProperty], + _getBoundsFunc = element => _getProxyProp(element, "getBoundingClientRect") || (_isViewport(element) ? () => {_winOffsets.width = _win.innerWidth; _winOffsets.height = _100vh; return _winOffsets;} : () => _getBounds(element)), + _getSizeFunc = (scroller, isViewport, {d, d2, a}) => (a = _getProxyProp(scroller, "getBoundingClientRect")) ? () => a()[d] : () => (isViewport ? _getViewportDimension(d2) : scroller["client" + d2]) || 0, + _getOffsetsFunc = (element, isViewport) => !isViewport || ~_proxies.indexOf(element) ? _getBoundsFunc(element) : () => _winOffsets, + _maxScroll = (element, {s, d2, d, a}) => Math.max(0, (s = "scroll" + d2) && (a = _getProxyProp(element, s)) ? a() - _getBoundsFunc(element)()[d] : _isViewport(element) ? (_docEl[s] || _body[s]) - _getViewportDimension(d2) : element[s] - element["offset" + d2]), + _iterateAutoRefresh = (func, events) => { + for (let i = 0; i < _autoRefresh.length; i += 3) { + (!events || ~events.indexOf(_autoRefresh[i+1])) && func(_autoRefresh[i], _autoRefresh[i+1], _autoRefresh[i+2]); + } + }, + _isString = value => typeof(value) === "string", + _isFunction = value => typeof(value) === "function", + _isNumber = value => typeof(value) === "number", + _isObject = value => typeof(value) === "object", + _endAnimation = (animation, reversed, pause) => animation && animation.progress(reversed ? 0 : 1) && pause && animation.pause(), + _callback = (self, func) => { + if (self.enabled) { + let result = self._ctx ? self._ctx.add(() => func(self)) : func(self); + result && result.totalTime && (self.callbackAnimation = result); + } + }, + _abs = Math.abs, + _left = "left", + _top = "top", + _right = "right", + _bottom = "bottom", + _width = "width", + _height = "height", + _Right = "Right", + _Left = "Left", + _Top = "Top", + _Bottom = "Bottom", + _padding = "padding", + _margin = "margin", + _Width = "Width", + _Height = "Height", + _px = "px", + _getComputedStyle = element => _win.getComputedStyle(element), + _makePositionable = element => { // if the element already has position: absolute or fixed, leave that, otherwise make it position: relative + let position = _getComputedStyle(element).position; + element.style.position = (position === "absolute" || position === "fixed") ? position : "relative"; + }, + _setDefaults = (obj, defaults) => { + for (let p in defaults) { + (p in obj) || (obj[p] = defaults[p]); + } + return obj; + }, + _getBounds = (element, withoutTransforms) => { + let tween = withoutTransforms && _getComputedStyle(element)[_transformProp] !== "matrix(1, 0, 0, 1, 0, 0)" && gsap.to(element, {x: 0, y: 0, xPercent: 0, yPercent: 0, rotation: 0, rotationX: 0, rotationY: 0, scale: 1, skewX: 0, skewY: 0}).progress(1), + bounds = element.getBoundingClientRect(); + tween && tween.progress(0).kill(); + return bounds; + }, + _getSize = (element, {d2}) => element["offset" + d2] || element["client" + d2] || 0, + _getLabelRatioArray = timeline => { + let a = [], + labels = timeline.labels, + duration = timeline.duration(), + p; + for (p in labels) { + a.push(labels[p] / duration); + } + return a; + }, + _getClosestLabel = animation => value => gsap.utils.snap(_getLabelRatioArray(animation), value), + _snapDirectional = snapIncrementOrArray => { + let snap = gsap.utils.snap(snapIncrementOrArray), + a = Array.isArray(snapIncrementOrArray) && snapIncrementOrArray.slice(0).sort((a, b) => a - b); + return a ? (value, direction, threshold= 1e-3) => { + let i; + if (!direction) { + return snap(value); + } + if (direction > 0) { + value -= threshold; // to avoid rounding errors. If we're too strict, it might snap forward, then immediately again, and again. + for (i = 0; i < a.length; i++) { + if (a[i] >= value) { + return a[i]; + } + } + return a[i-1]; + } else { + i = a.length; + value += threshold; + while (i--) { + if (a[i] <= value) { + return a[i]; + } + } + } + return a[0]; + } : (value, direction, threshold= 1e-3) => { + let snapped = snap(value); + return !direction || Math.abs(snapped - value) < threshold || ((snapped - value < 0) === direction < 0) ? snapped : snap(direction < 0 ? value - snapIncrementOrArray : value + snapIncrementOrArray); + }; + }, + _getLabelAtDirection = timeline => (value, st) => _snapDirectional(_getLabelRatioArray(timeline))(value, st.direction), + _multiListener = (func, element, types, callback) => types.split(",").forEach(type => func(element, type, callback)), + _addListener = (element, type, func, nonPassive, capture) => element.addEventListener(type, func, {passive: !nonPassive, capture: !!capture}), + _removeListener = (element, type, func, capture) => element.removeEventListener(type, func, !!capture), + _wheelListener = (func, el, scrollFunc) => { + scrollFunc = scrollFunc && scrollFunc.wheelHandler + if (scrollFunc) { + func(el, "wheel", scrollFunc); + func(el, "touchmove", scrollFunc); + } + }, + _markerDefaults = {startColor: "green", endColor: "red", indent: 0, fontSize: "16px", fontWeight:"normal"}, + _defaults = {toggleActions: "play", anticipatePin: 0}, + _keywords = {top: 0, left: 0, center: 0.5, bottom: 1, right: 1}, + _offsetToPx = (value, size) => { + if (_isString(value)) { + let eqIndex = value.indexOf("="), + relative = ~eqIndex ? +(value.charAt(eqIndex-1) + 1) * parseFloat(value.substr(eqIndex + 1)) : 0; + if (~eqIndex) { + (value.indexOf("%") > eqIndex) && (relative *= size / 100); + value = value.substr(0, eqIndex-1); + } + value = relative + ((value in _keywords) ? _keywords[value] * size : ~value.indexOf("%") ? parseFloat(value) * size / 100 : parseFloat(value) || 0); + } + return value; + }, + _createMarker = (type, name, container, direction, {startColor, endColor, fontSize, indent, fontWeight}, offset, matchWidthEl, containerAnimation) => { + let e = _doc.createElement("div"), + useFixedPosition = _isViewport(container) || _getProxyProp(container, "pinType") === "fixed", + isScroller = type.indexOf("scroller") !== -1, + parent = useFixedPosition ? _body : container, + isStart = type.indexOf("start") !== -1, + color = isStart ? startColor : endColor, + css = "border-color:" + color + ";font-size:" + fontSize + ";color:" + color + ";font-weight:" + fontWeight + ";pointer-events:none;white-space:nowrap;font-family:sans-serif,Arial;z-index:1000;padding:4px 8px;border-width:0;border-style:solid;"; + css += "position:" + ((isScroller || containerAnimation) && useFixedPosition ? "fixed;" : "absolute;"); + (isScroller || containerAnimation || !useFixedPosition) && (css += (direction === _vertical ? _right : _bottom) + ":" + (offset + parseFloat(indent)) + "px;"); + matchWidthEl && (css += "box-sizing:border-box;text-align:left;width:" + matchWidthEl.offsetWidth + "px;"); + e._isStart = isStart; + e.setAttribute("class", "gsap-marker-" + type + (name ? " marker-" + name : "")); + e.style.cssText = css; + e.innerText = name || name === 0 ? type + "-" + name : type; + parent.children[0] ? parent.insertBefore(e, parent.children[0]) : parent.appendChild(e); + e._offset = e["offset" + direction.op.d2]; + _positionMarker(e, 0, direction, isStart); + return e; + }, + _positionMarker = (marker, start, direction, flipped) => { + let vars = {display: "block"}, + side = direction[flipped ? "os2" : "p2"], + oppositeSide = direction[flipped ? "p2" : "os2"]; + marker._isFlipped = flipped; + vars[direction.a + "Percent"] = flipped ? -100 : 0; + vars[direction.a] = flipped ? "1px" : 0; + vars["border" + side + _Width] = 1; + vars["border" + oppositeSide + _Width] = 0; + vars[direction.p] = start + "px"; + gsap.set(marker, vars); + }, + _triggers = [], + _ids = {}, + _rafID, + _sync = () => _getTime() - _lastScrollTime > 34 && (_rafID || (_rafID = requestAnimationFrame(_updateAll))), + _onScroll = () => { // previously, we tried to optimize performance by batching/deferring to the next requestAnimationFrame(), but discovered that Safari has a few bugs that make this unworkable (especially on iOS). See https://codepen.io/GreenSock/pen/16c435b12ef09c38125204818e7b45fc?editors=0010 and https://codepen.io/GreenSock/pen/JjOxYpQ/3dd65ccec5a60f1d862c355d84d14562?editors=0010 and https://codepen.io/GreenSock/pen/ExbrPNa/087cef197dc35445a0951e8935c41503?editors=0010 + if (!_normalizer || !_normalizer.isPressed || _normalizer.startX > _body.clientWidth) { // if the user is dragging the scrollbar, allow it. + _scrollers.cache++; + if (_normalizer) { + _rafID || (_rafID = requestAnimationFrame(_updateAll)); + } else { + _updateAll(); // Safari in particular (on desktop) NEEDS the immediate update rather than waiting for a requestAnimationFrame() whereas iOS seems to benefit from waiting for the requestAnimationFrame() tick, at least when normalizing. See https://codepen.io/GreenSock/pen/qBYozqO?editors=0110 + } + _lastScrollTime || _dispatch("scrollStart"); + _lastScrollTime = _getTime(); + } + }, + _setBaseDimensions = () => { + _baseScreenWidth = _win.innerWidth; + _baseScreenHeight = _win.innerHeight; + }, + _onResize = (force) => { + _scrollers.cache++; + (force === true || (!_refreshing && !_ignoreResize && !_doc.fullscreenElement && !_doc.webkitFullscreenElement && (!_ignoreMobileResize || _baseScreenWidth !== _win.innerWidth || Math.abs(_win.innerHeight - _baseScreenHeight) > _win.innerHeight * 0.25))) && _resizeDelay.restart(true); + }, // ignore resizes triggered by refresh() + _listeners = {}, + _emptyArray = [], + _softRefresh = () => _removeListener(ScrollTrigger, "scrollEnd", _softRefresh) || _refreshAll(true), + _dispatch = type => (_listeners[type] && _listeners[type].map(f => f())) || _emptyArray, + _savedStyles = [], // when ScrollTrigger.saveStyles() is called, the inline styles are recorded in this Array in a sequential format like [element, cssText, gsCache, media]. This keeps it very memory-efficient and fast to iterate through. + _revertRecorded = media => { + for (let i = 0; i < _savedStyles.length; i+=5) { + if (!media || _savedStyles[i+4] && _savedStyles[i+4].query === media) { + _savedStyles[i].style.cssText = _savedStyles[i+1]; + _savedStyles[i].getBBox && _savedStyles[i].setAttribute("transform", _savedStyles[i+2] || ""); + _savedStyles[i+3].uncache = 1; + } + } + }, + _revertAll = (kill, media) => { + let trigger; + for (_i = 0; _i < _triggers.length; _i++) { + trigger = _triggers[_i]; + if (trigger && (!media || trigger._ctx === media)) { + if (kill) { + trigger.kill(1); + } else { + trigger.revert(true, true); + } + } + } + _isReverted = true; + media && _revertRecorded(media); + media || _dispatch("revert"); + }, + _clearScrollMemory = (scrollRestoration, force) => { // zero-out all the recorded scroll positions. Don't use _triggers because if, for example, .matchMedia() is used to create some ScrollTriggers and then the user resizes and it removes ALL ScrollTriggers, and then go back to a size where there are ScrollTriggers, it would have kept the position(s) saved from the initial state. + _scrollers.cache++; + (force || !_refreshingAll) && _scrollers.forEach(obj => _isFunction(obj) && obj.cacheID++ && (obj.rec = 0)); + _isString(scrollRestoration) && (_win.history.scrollRestoration = _scrollRestoration = scrollRestoration); + }, + _refreshingAll, + _refreshID = 0, + _queueRefreshID, + _queueRefreshAll = () => { // we don't want to call _refreshAll() every time we create a new ScrollTrigger (for performance reasons) - it's better to batch them. Some frameworks dynamically load content and we can't rely on the window's "load" or "DOMContentLoaded" events to trigger it. + if (_queueRefreshID !== _refreshID) { + let id = _queueRefreshID = _refreshID; + requestAnimationFrame(() => id === _refreshID && _refreshAll(true)); + } + }, + _refresh100vh = () => { + _body.appendChild(_div100vh); + _100vh = (!_normalizer && _div100vh.offsetHeight) || _win.innerHeight; + _body.removeChild(_div100vh); + }, + _hideAllMarkers = hide => _toArray(".gsap-marker-start, .gsap-marker-end, .gsap-marker-scroller-start, .gsap-marker-scroller-end").forEach(el => el.style.display = hide ? "none" : "block"), + _refreshAll = (force, skipRevert) => { + _docEl = _doc.documentElement; // some frameworks like Astro may cache the <body> and replace it during routing, so we'll just re-record the _docEl and _body for safety (otherwise, the markers may not get added properly). + _body = _doc.body; + _root = [_win, _doc, _docEl, _body]; + if (_lastScrollTime && !force && !_isReverted) { + _addListener(ScrollTrigger, "scrollEnd", _softRefresh); + return; + } + _refresh100vh(); + _refreshingAll = ScrollTrigger.isRefreshing = true; + _scrollers.forEach(obj => _isFunction(obj) && ++obj.cacheID && (obj.rec = obj())); // force the clearing of the cache because some browsers take a little while to dispatch the "scroll" event and the user may have changed the scroll position and then called ScrollTrigger.refresh() right away + let refreshInits = _dispatch("refreshInit"); + _sort && ScrollTrigger.sort(); + skipRevert || _revertAll(); + _scrollers.forEach(obj => { + if (_isFunction(obj)) { + obj.smooth && (obj.target.style.scrollBehavior = "auto"); // smooth scrolling interferes + obj(0); + } + }); + _triggers.slice(0).forEach(t => t.refresh()) // don't loop with _i because during a refresh() someone could call ScrollTrigger.update() which would iterate through _i resulting in a skip. + _isReverted = false; + _triggers.forEach((t) => { // nested pins (pinnedContainer) with pinSpacing may expand the container, so we must accommodate that here. + if (t._subPinOffset && t.pin) { + let prop = t.vars.horizontal ? "offsetWidth" : "offsetHeight", + original = t.pin[prop]; + t.revert(true, 1); + t.adjustPinSpacing(t.pin[prop] - original); + t.refresh(); + } + }); + _clampingMax = 1; // pinSpacing might be propping a page open, thus when we .setPositions() to clamp a ScrollTrigger's end we should leave the pinSpacing alone. That's what this flag is for. + _hideAllMarkers(true); + _triggers.forEach(t => { // the scroller's max scroll position may change after all the ScrollTriggers refreshed (like pinning could push it down), so we need to loop back and correct any with end: "max". Same for anything with a clamped end + let max = _maxScroll(t.scroller, t._dir), + endClamp = t.vars.end === "max" || (t._endClamp && t.end > max), + startClamp = t._startClamp && t.start >= max; + (endClamp || startClamp) && t.setPositions(startClamp ? max - 1 : t.start, endClamp ? Math.max(startClamp ? max : t.start + 1, max) : t.end, true); + }); + _hideAllMarkers(false); + _clampingMax = 0; + refreshInits.forEach(result => result && result.render && result.render(-1)); // if the onRefreshInit() returns an animation (typically a gsap.set()), revert it. This makes it easy to put things in a certain spot before refreshing for measurement purposes, and then put things back. + _scrollers.forEach(obj => { + if (_isFunction(obj)) { + obj.smooth && requestAnimationFrame(() => obj.target.style.scrollBehavior = "smooth"); + obj.rec && obj(obj.rec); + } + }); + _clearScrollMemory(_scrollRestoration, 1); + _resizeDelay.pause(); + _refreshID++; + _refreshingAll = 2; + _updateAll(2); + _triggers.forEach(t => _isFunction(t.vars.onRefresh) && t.vars.onRefresh(t)); + _refreshingAll = ScrollTrigger.isRefreshing = false; + _dispatch("refresh"); + }, + _lastScroll = 0, + _direction = 1, + _primary, + _updateAll = (force) => { + if (force === 2 || (!_refreshingAll && !_isReverted)) { // _isReverted could be true if, for example, a matchMedia() is in the process of executing. We don't want to update during the time everything is reverted. + ScrollTrigger.isUpdating = true; + _primary && _primary.update(0); // ScrollSmoother uses refreshPriority -9999 to become the primary that gets updated before all others because it affects the scroll position. + let l = _triggers.length, + time = _getTime(), + recordVelocity = time - _time1 >= 50, + scroll = l && _triggers[0].scroll(); + _direction = _lastScroll > scroll ? -1 : 1; + _refreshingAll || (_lastScroll = scroll); + if (recordVelocity) { + if (_lastScrollTime && !_pointerIsDown && time - _lastScrollTime > 200) { + _lastScrollTime = 0; + _dispatch("scrollEnd"); + } + _time2 = _time1; + _time1 = time; + } + if (_direction < 0) { + _i = l; + while (_i-- > 0) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + _direction = 1; + } else { + for (_i = 0; _i < l; _i++) { + _triggers[_i] && _triggers[_i].update(0, recordVelocity); + } + } + ScrollTrigger.isUpdating = false; + } + _rafID = 0; + }, + _propNamesToCopy = [_left, _top, _bottom, _right, _margin + _Bottom, _margin + _Right, _margin + _Top, _margin + _Left, "display", "flexShrink", "float", "zIndex", "gridColumnStart", "gridColumnEnd", "gridRowStart", "gridRowEnd", "gridArea", "justifySelf", "alignSelf", "placeSelf", "order"], + _stateProps = _propNamesToCopy.concat([_width, _height, "boxSizing", "max" + _Width, "max" + _Height, "position", _margin, _padding, _padding + _Top, _padding + _Right, _padding + _Bottom, _padding + _Left]), + _swapPinOut = (pin, spacer, state) => { + _setState(state); + let cache = pin._gsap; + if (cache.spacerIsNative) { + _setState(cache.spacerState); + } else if (pin._gsap.swappedIn) { + let parent = spacer.parentNode; + if (parent) { + parent.insertBefore(pin, spacer); + parent.removeChild(spacer); + } + } + pin._gsap.swappedIn = false; + }, + _swapPinIn = (pin, spacer, cs, spacerState) => { + if (!pin._gsap.swappedIn) { + let i = _propNamesToCopy.length, + spacerStyle = spacer.style, + pinStyle = pin.style, + p; + while (i--) { + p = _propNamesToCopy[i]; + spacerStyle[p] = cs[p]; + } + spacerStyle.position = cs.position === "absolute" ? "absolute" : "relative"; + (cs.display === "inline") && (spacerStyle.display = "inline-block"); + pinStyle[_bottom] = pinStyle[_right] = "auto"; + spacerStyle.flexBasis = cs.flexBasis || "auto"; + spacerStyle.overflow = "visible"; + spacerStyle.boxSizing = "border-box"; + spacerStyle[_width] = _getSize(pin, _horizontal) + _px; + spacerStyle[_height] = _getSize(pin, _vertical) + _px; + spacerStyle[_padding] = pinStyle[_margin] = pinStyle[_top] = pinStyle[_left] = "0"; + _setState(spacerState); + pinStyle[_width] = pinStyle["max" + _Width] = cs[_width]; + pinStyle[_height] = pinStyle["max" + _Height] = cs[_height]; + pinStyle[_padding] = cs[_padding]; + if (pin.parentNode !== spacer) { + pin.parentNode.insertBefore(spacer, pin); + spacer.appendChild(pin); + } + pin._gsap.swappedIn = true; + } + }, + _capsExp = /([A-Z])/g, + _setState = state => { + if (state) { + let style = state.t.style, + l = state.length, + i = 0, + p, value; + (state.t._gsap || gsap.core.getCache(state.t)).uncache = 1; // otherwise transforms may be off + for (; i < l; i +=2) { + value = state[i+1]; + p = state[i]; + if (value) { + style[p] = value; + } else if (style[p]) { + style.removeProperty(p.replace(_capsExp, "-$1").toLowerCase()); + } + } + } + }, + _getState = element => { // returns an Array with alternating values like [property, value, property, value] and a "t" property pointing to the target (element). Makes it fast and cheap. + let l = _stateProps.length, + style = element.style, + state = [], + i = 0; + for (; i < l; i++) { + state.push(_stateProps[i], style[_stateProps[i]]); + } + state.t = element; + return state; + }, + _copyState = (state, override, omitOffsets) => { + let result = [], + l = state.length, + i = omitOffsets ? 8 : 0, // skip top, left, right, bottom if omitOffsets is true + p; + for (; i < l; i += 2) { + p = state[i]; + result.push(p, (p in override) ? override[p] : state[i+1]); + } + result.t = state.t; + return result; + }, + _winOffsets = {left:0, top:0}, + // // potential future feature (?) Allow users to calculate where a trigger hits (scroll position) like getScrollPosition("#id", "top bottom") + // _getScrollPosition = (trigger, position, {scroller, containerAnimation, horizontal}) => { + // scroller = _getTarget(scroller || _win); + // let direction = horizontal ? _horizontal : _vertical, + // isViewport = _isViewport(scroller); + // _getSizeFunc(scroller, isViewport, direction); + // return _parsePosition(position, _getTarget(trigger), _getSizeFunc(scroller, isViewport, direction)(), direction, _getScrollFunc(scroller, direction)(), 0, 0, 0, _getOffsetsFunc(scroller, isViewport)(), isViewport ? 0 : parseFloat(_getComputedStyle(scroller)["border" + direction.p2 + _Width]) || 0, 0, containerAnimation ? containerAnimation.duration() : _maxScroll(scroller), containerAnimation); + // }, + _parsePosition = (value, trigger, scrollerSize, direction, scroll, marker, markerScroller, self, scrollerBounds, borderWidth, useFixedPosition, scrollerMax, containerAnimation, clampZeroProp) => { + _isFunction(value) && (value = value(self)); + if (_isString(value) && value.substr(0,3) === "max") { + value = scrollerMax + (value.charAt(4) === "=" ? _offsetToPx("0" + value.substr(3), scrollerSize) : 0); + } + let time = containerAnimation ? containerAnimation.time() : 0, + p1, p2, element; + containerAnimation && containerAnimation.seek(0); + isNaN(value) || (value = +value); // convert a string number like "45" to an actual number + if (!_isNumber(value)) { + _isFunction(trigger) && (trigger = trigger(self)); + let offsets = (value || "0").split(" "), + bounds, localOffset, globalOffset, display; + element = _getTarget(trigger, self) || _body; + bounds = _getBounds(element) || {}; + if ((!bounds || (!bounds.left && !bounds.top)) && _getComputedStyle(element).display === "none") { // if display is "none", it won't report getBoundingClientRect() properly + display = element.style.display; + element.style.display = "block"; + bounds = _getBounds(element); + display ? (element.style.display = display) : element.style.removeProperty("display"); + } + localOffset = _offsetToPx(offsets[0], bounds[direction.d]); + globalOffset = _offsetToPx(offsets[1] || "0", scrollerSize); + value = bounds[direction.p] - scrollerBounds[direction.p] - borderWidth + localOffset + scroll - globalOffset; + markerScroller && _positionMarker(markerScroller, globalOffset, direction, (scrollerSize - globalOffset < 20 || (markerScroller._isStart && globalOffset > 20))); + scrollerSize -= scrollerSize - globalOffset; // adjust for the marker + } else { + containerAnimation && (value = gsap.utils.mapRange(containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, 0, scrollerMax, value)); + markerScroller && _positionMarker(markerScroller, scrollerSize, direction, true); + } + if (clampZeroProp) { + self[clampZeroProp] = value || -0.001; + value < 0 && (value = 0); + } + if (marker) { + let position = value + scrollerSize, + isStart = marker._isStart; + p1 = "scroll" + direction.d2; + _positionMarker(marker, position, direction, (isStart && position > 20) || (!isStart && (useFixedPosition ? Math.max(_body[p1], _docEl[p1]) : marker.parentNode[p1]) <= position + 1)); + if (useFixedPosition) { + scrollerBounds = _getBounds(markerScroller); + useFixedPosition && (marker.style[direction.op.p] = (scrollerBounds[direction.op.p] - direction.op.m - marker._offset) + _px); + } + } + if (containerAnimation && element) { + p1 = _getBounds(element); + containerAnimation.seek(scrollerMax); + p2 = _getBounds(element); + containerAnimation._caScrollDist = p1[direction.p] - p2[direction.p]; + value = value / (containerAnimation._caScrollDist) * scrollerMax; + } + containerAnimation && containerAnimation.seek(time); + return containerAnimation ? value : Math.round(value); + }, + _prefixExp = /(webkit|moz|length|cssText|inset)/i, + _reparent = (element, parent, top, left) => { + if (element.parentNode !== parent) { + let style = element.style, + p, cs; + if (parent === _body) { + element._stOrig = style.cssText; // record original inline styles so we can revert them later + cs = _getComputedStyle(element); + for (p in cs) { // must copy all relevant styles to ensure that nothing changes visually when we reparent to the <body>. Skip the vendor prefixed ones. + if (!+p && !_prefixExp.test(p) && cs[p] && typeof style[p] === "string" && p !== "0") { + style[p] = cs[p]; + } + } + style.top = top; + style.left = left; + } else { + style.cssText = element._stOrig; + } + gsap.core.getCache(element).uncache = 1; + parent.appendChild(element); + } + }, + _interruptionTracker = (getValueFunc, initialValue, onInterrupt) => { + let last1 = initialValue, + last2 = last1; + return value => { + let current = Math.round(getValueFunc()); // round because in some [very uncommon] Windows environments, scroll can get reported with decimals even though it was set without. + if (current !== last1 && current !== last2 && Math.abs(current - last1) > 3 && Math.abs(current - last2) > 3) { // if the user scrolls, kill the tween. iOS Safari intermittently misreports the scroll position, it may be the most recently-set one or the one before that! When Safari is zoomed (CMD-+), it often misreports as 1 pixel off too! So if we set the scroll position to 125, for example, it'll actually report it as 124. + value = current; + onInterrupt && onInterrupt(); + } + last2 = last1; + last1 = Math.round(value); + return last1; + }; + }, + _shiftMarker = (marker, direction, value) => { + let vars = {}; + vars[direction.p] = "+=" + value; + gsap.set(marker, vars); + }, + // _mergeAnimations = animations => { + // let tl = gsap.timeline({smoothChildTiming: true}).startTime(Math.min(...animations.map(a => a.globalTime(0)))); + // animations.forEach(a => {let time = a.totalTime(); tl.add(a); a.totalTime(time); }); + // tl.smoothChildTiming = false; + // return tl; + // }, + + // returns a function that can be used to tween the scroll position in the direction provided, and when doing so it'll add a .tween property to the FUNCTION itself, and remove it when the tween completes or gets killed. This gives us a way to have multiple ScrollTriggers use a central function for any given scroller and see if there's a scroll tween running (which would affect if/how things get updated) + _getTweenCreator = (scroller, direction) => { + let getScroll = _getScrollFunc(scroller, direction), + prop = "_scroll" + direction.p2, // add a tweenable property to the scroller that's a getter/setter function, like _scrollTop or _scrollLeft. This way, if someone does gsap.killTweensOf(scroller) it'll kill the scroll tween. + getTween = (scrollTo, vars, initialValue, change1, change2) => { + let tween = getTween.tween, + onComplete = vars.onComplete, + modifiers = {}; + initialValue = initialValue || getScroll(); + let checkForInterruption = _interruptionTracker(getScroll, initialValue, () => { + tween.kill(); + getTween.tween = 0; + }); + change2 = (change1 && change2) || 0; // if change1 is 0, we set that to the difference and ignore change2. Otherwise, there would be a compound effect. + change1 = change1 || (scrollTo - initialValue); + tween && tween.kill(); + vars[prop] = scrollTo; + vars.inherit = false; + vars.modifiers = modifiers; + modifiers[prop] = () => checkForInterruption(initialValue + change1 * tween.ratio + change2 * tween.ratio * tween.ratio); + vars.onUpdate = () => { + _scrollers.cache++; + getTween.tween && _updateAll(); // if it was interrupted/killed, like in a context.revert(), don't force an updateAll() + }; + vars.onComplete = () => { + getTween.tween = 0; + onComplete && onComplete.call(tween); + }; + tween = getTween.tween = gsap.to(scroller, vars); + return tween; + }; + scroller[prop] = getScroll; + getScroll.wheelHandler = () => getTween.tween && getTween.tween.kill() && (getTween.tween = 0); + _addListener(scroller, "wheel", getScroll.wheelHandler); // Windows machines handle mousewheel scrolling in chunks (like "3 lines per scroll") meaning the typical strategy for cancelling the scroll isn't as sensitive. It's much more likely to match one of the previous 2 scroll event positions. So we kill any snapping as soon as there's a wheel event. + ScrollTrigger.isTouch && _addListener(scroller, "touchmove", getScroll.wheelHandler); + return getTween; + }; + + + + +export class ScrollTrigger { + + constructor(vars, animation) { + _coreInitted || ScrollTrigger.register(gsap) || console.warn("Please gsap.registerPlugin(ScrollTrigger)"); + _context(this); + this.init(vars, animation); + } + + init(vars, animation) { + this.progress = this.start = 0; + this.vars && this.kill(true, true); // in case it's being initted again + if (!_enabled) { + this.update = this.refresh = this.kill = _passThrough; + return; + } + vars = _setDefaults((_isString(vars) || _isNumber(vars) || vars.nodeType) ? {trigger: vars} : vars, _defaults); + let {onUpdate, toggleClass, id, onToggle, onRefresh, scrub, trigger, pin, pinSpacing, invalidateOnRefresh, anticipatePin, onScrubComplete, onSnapComplete, once, snap, pinReparent, pinSpacer, containerAnimation, fastScrollEnd, preventOverlaps} = vars, + direction = vars.horizontal || (vars.containerAnimation && vars.horizontal !== false) ? _horizontal : _vertical, + isToggle = !scrub && scrub !== 0, + scroller = _getTarget(vars.scroller || _win), + scrollerCache = gsap.core.getCache(scroller), + isViewport = _isViewport(scroller), + useFixedPosition = ("pinType" in vars ? vars.pinType : _getProxyProp(scroller, "pinType") || (isViewport && "fixed")) === "fixed", + callbacks = [vars.onEnter, vars.onLeave, vars.onEnterBack, vars.onLeaveBack], + toggleActions = isToggle && vars.toggleActions.split(" "), + markers = "markers" in vars ? vars.markers : _defaults.markers, + borderWidth = isViewport ? 0 : parseFloat(_getComputedStyle(scroller)["border" + direction.p2 + _Width]) || 0, + self = this, + onRefreshInit = vars.onRefreshInit && (() => vars.onRefreshInit(self)), + getScrollerSize = _getSizeFunc(scroller, isViewport, direction), + getScrollerOffsets = _getOffsetsFunc(scroller, isViewport), + lastSnap = 0, + lastRefresh = 0, + prevProgress = 0, + scrollFunc = _getScrollFunc(scroller, direction), + tweenTo, pinCache, snapFunc, scroll1, scroll2, start, end, markerStart, markerEnd, markerStartTrigger, markerEndTrigger, markerVars, executingOnRefresh, + change, pinOriginalState, pinActiveState, pinState, spacer, offset, pinGetter, pinSetter, pinStart, pinChange, spacingStart, spacerState, markerStartSetter, pinMoves, + markerEndSetter, cs, snap1, snap2, scrubTween, scrubSmooth, snapDurClamp, snapDelayedCall, prevScroll, prevAnimProgress, caMarkerSetter, customRevertReturn; + + // for the sake of efficiency, _startClamp/_endClamp serve like a truthy value indicating that clamping was enabled on the start/end, and ALSO store the actual pre-clamped numeric value. We tap into that in ScrollSmoother for speed effects. So for example, if start="clamp(top bottom)" results in a start of -100 naturally, it would get clamped to 0 but -100 would be stored in _startClamp. + self._startClamp = self._endClamp = false; + self._dir = direction; + anticipatePin *= 45; + self.scroller = scroller; + self.scroll = containerAnimation ? containerAnimation.time.bind(containerAnimation) : scrollFunc; + scroll1 = scrollFunc(); + self.vars = vars; + animation = animation || vars.animation; + if ("refreshPriority" in vars) { + _sort = 1; + vars.refreshPriority === -9999 && (_primary = self); // used by ScrollSmoother + } + scrollerCache.tweenScroll = scrollerCache.tweenScroll || { + top: _getTweenCreator(scroller, _vertical), + left: _getTweenCreator(scroller, _horizontal) + }; + self.tweenTo = tweenTo = scrollerCache.tweenScroll[direction.p]; + self.scrubDuration = value => { + scrubSmooth = _isNumber(value) && value; + if (!scrubSmooth) { + scrubTween && scrubTween.progress(1).kill(); + scrubTween = 0; + } else { + scrubTween ? scrubTween.duration(value) : (scrubTween = gsap.to(animation, {ease: "expo", totalProgress: "+=0", inherit: false, duration: scrubSmooth, paused: true, onComplete: () => onScrubComplete && onScrubComplete(self)})); + } + }; + if (animation) { + animation.vars.lazy = false; + (animation._initted && !self.isReverted) || (animation.vars.immediateRender !== false && vars.immediateRender !== false && animation.duration() && animation.render(0, true, true)); // special case: if this ScrollTrigger gets re-initted, a from() tween with a stagger could get initted initially and then reverted on the re-init which means it'll need to get rendered again here to properly display things. Otherwise, See https://gsap.com/forums/topic/36777-scrollsmoother-splittext-nextjs/ and https://codepen.io/GreenSock/pen/eYPyPpd?editors=0010 + self.animation = animation.pause(); + animation.scrollTrigger = self; + self.scrubDuration(scrub); + snap1 = 0; + id || (id = animation.vars.id); + } + + if (snap) { + // TODO: potential idea: use legitimate CSS scroll snapping by pushing invisible elements into the DOM that serve as snap positions, and toggle the document.scrollingElement.style.scrollSnapType onToggle. See https://codepen.io/GreenSock/pen/JjLrgWM for a quick proof of concept. + if (!_isObject(snap) || snap.push) { + snap = {snapTo: snap}; + } + ("scrollBehavior" in _body.style) && gsap.set(isViewport ? [_body, _docEl] : scroller, {scrollBehavior: "auto"}); // smooth scrolling doesn't work with snap. + _scrollers.forEach(o => _isFunction(o) && o.target === (isViewport ? _doc.scrollingElement || _docEl : scroller) && (o.smooth = false)); // note: set smooth to false on both the vertical and horizontal scroll getters/setters + snapFunc = _isFunction(snap.snapTo) ? snap.snapTo : snap.snapTo === "labels" ? _getClosestLabel(animation) : snap.snapTo === "labelsDirectional" ? _getLabelAtDirection(animation) : snap.directional !== false ? (value, st) => _snapDirectional(snap.snapTo)(value, _getTime() - lastRefresh < 500 ? 0 : st.direction) : gsap.utils.snap(snap.snapTo); + snapDurClamp = snap.duration || {min: 0.1, max: 2}; + snapDurClamp = _isObject(snapDurClamp) ? _clamp(snapDurClamp.min, snapDurClamp.max) : _clamp(snapDurClamp, snapDurClamp); + snapDelayedCall = gsap.delayedCall(snap.delay || (scrubSmooth / 2) || 0.1, () => { + let scroll = scrollFunc(), + refreshedRecently = _getTime() - lastRefresh < 500, + tween = tweenTo.tween; + if ((refreshedRecently || Math.abs(self.getVelocity()) < 10) && !tween && !_pointerIsDown && lastSnap !== scroll) { + let progress = (scroll - start) / change, // don't use self.progress because this might run between the refresh() and when the scroll position updates and self.progress is set properly in the update() method. + totalProgress = animation && !isToggle ? animation.totalProgress() : progress, + velocity = refreshedRecently ? 0 : ((totalProgress - snap2) / (_getTime() - _time2) * 1000) || 0, + change1 = gsap.utils.clamp(-progress, 1 - progress, _abs(velocity / 2) * velocity / 0.185), + naturalEnd = progress + (snap.inertia === false ? 0 : change1), + endValue, endScroll, + { onStart, onInterrupt, onComplete } = snap; + endValue = snapFunc(naturalEnd, self); + _isNumber(endValue) || (endValue = naturalEnd); // in case the function didn't return a number, fall back to using the naturalEnd + endScroll = Math.max(0, Math.round(start + endValue * change)); + if (scroll <= end && scroll >= start && endScroll !== scroll) { + if (tween && !tween._initted && tween.data <= _abs(endScroll - scroll)) { // there's an overlapping snap! So we must figure out which one is closer and let that tween live. + return; + } + if (snap.inertia === false) { + change1 = endValue - progress; + } + tweenTo(endScroll, { + duration: snapDurClamp(_abs( (Math.max(_abs(naturalEnd - totalProgress), _abs(endValue - totalProgress)) * 0.185 / velocity / 0.05) || 0)), + ease: snap.ease || "power3", + data: _abs(endScroll - scroll), // record the distance so that if another snap tween occurs (conflict) we can prioritize the closest snap. + onInterrupt: () => snapDelayedCall.restart(true) && onInterrupt && onInterrupt(self), + onComplete() { + self.update(); + lastSnap = scrollFunc(); + if (animation && !isToggle) { // the resolution of the scrollbar is limited, so we should correct the scrubbed animation's playhead at the end to match EXACTLY where it was supposed to snap + scrubTween ? scrubTween.resetTo("totalProgress", endValue, animation._tTime / animation._tDur) : animation.progress(endValue); + } + snap1 = snap2 = animation && !isToggle ? animation.totalProgress() : self.progress; + onSnapComplete && onSnapComplete(self); + onComplete && onComplete(self); + } + }, scroll, change1 * change, endScroll - scroll - change1 * change); + onStart && onStart(self, tweenTo.tween); + } + } else if (self.isActive && lastSnap !== scroll) { + snapDelayedCall.restart(true); + } + }).pause(); + } + id && (_ids[id] = self); + trigger = self.trigger = _getTarget(trigger || (pin !== true && pin)); + + // if a trigger has some kind of scroll-related effect applied that could contaminate the "y" or "x" position (like a ScrollSmoother effect), we needed a way to temporarily revert it, so we use the stRevert property of the gsCache. It can return another function that we'll call at the end so it can return to its normal state. + customRevertReturn = trigger && trigger._gsap && trigger._gsap.stRevert; + customRevertReturn && (customRevertReturn = customRevertReturn(self)); + + pin = pin === true ? trigger : _getTarget(pin); + _isString(toggleClass) && (toggleClass = {targets: trigger, className: toggleClass}); + if (pin) { + (pinSpacing === false || pinSpacing === _margin) || (pinSpacing = !pinSpacing && pin.parentNode && pin.parentNode.style && _getComputedStyle(pin.parentNode).display === "flex" ? false : _padding); // if the parent is display: flex, don't apply pinSpacing by default. We should check that pin.parentNode is an element (not shadow dom window) + self.pin = pin; + pinCache = gsap.core.getCache(pin); + if (!pinCache.spacer) { // record the spacer and pinOriginalState on the cache in case someone tries pinning the same element with MULTIPLE ScrollTriggers - we don't want to have multiple spacers or record the "original" pin state after it has already been affected by another ScrollTrigger. + if (pinSpacer) { + pinSpacer = _getTarget(pinSpacer); + pinSpacer && !pinSpacer.nodeType && (pinSpacer = pinSpacer.current || pinSpacer.nativeElement); // for React & Angular + pinCache.spacerIsNative = !!pinSpacer; + pinSpacer && (pinCache.spacerState = _getState(pinSpacer)); + } + pinCache.spacer = spacer = pinSpacer || _doc.createElement("div"); + spacer.classList.add("pin-spacer"); + id && spacer.classList.add("pin-spacer-" + id); + pinCache.pinState = pinOriginalState = _getState(pin); + } else { + pinOriginalState = pinCache.pinState; + } + vars.force3D !== false && gsap.set(pin, {force3D: true}); + self.spacer = spacer = pinCache.spacer; + cs = _getComputedStyle(pin); + spacingStart = cs[pinSpacing + direction.os2]; + pinGetter = gsap.getProperty(pin); + pinSetter = gsap.quickSetter(pin, direction.a, _px); + // pin.firstChild && !_maxScroll(pin, direction) && (pin.style.overflow = "hidden"); // protects from collapsing margins, but can have unintended consequences as demonstrated here: https://codepen.io/GreenSock/pen/1e42c7a73bfa409d2cf1e184e7a4248d so it was removed in favor of just telling people to set up their CSS to avoid the collapsing margins (overflow: hidden | auto is just one option. Another is border-top: 1px solid transparent). + _swapPinIn(pin, spacer, cs); + pinState = _getState(pin); + } + if (markers) { + markerVars = _isObject(markers) ? _setDefaults(markers, _markerDefaults) : _markerDefaults; + markerStartTrigger = _createMarker("scroller-start", id, scroller, direction, markerVars, 0); + markerEndTrigger = _createMarker("scroller-end", id, scroller, direction, markerVars, 0, markerStartTrigger); + offset = markerStartTrigger["offset" + direction.op.d2]; + let content = _getTarget(_getProxyProp(scroller, "content") || scroller); + markerStart = this.markerStart = _createMarker("start", id, content, direction, markerVars, offset, 0, containerAnimation); + markerEnd = this.markerEnd = _createMarker("end", id, content, direction, markerVars, offset, 0, containerAnimation); + containerAnimation && (caMarkerSetter = gsap.quickSetter([markerStart, markerEnd], direction.a, _px)); + if ((!useFixedPosition && !(_proxies.length && _getProxyProp(scroller, "fixedMarkers") === true))) { + _makePositionable(isViewport ? _body : scroller); + gsap.set([markerStartTrigger, markerEndTrigger], {force3D: true}); + markerStartSetter = gsap.quickSetter(markerStartTrigger, direction.a, _px); + markerEndSetter = gsap.quickSetter(markerEndTrigger, direction.a, _px); + } + } + + if (containerAnimation) { + let oldOnUpdate = containerAnimation.vars.onUpdate, + oldParams = containerAnimation.vars.onUpdateParams; + containerAnimation.eventCallback("onUpdate", () => { + self.update(0, 0, 1); + oldOnUpdate && oldOnUpdate.apply(containerAnimation, oldParams || []); + }); + } + + self.previous = () => _triggers[_triggers.indexOf(self) - 1]; + self.next = () => _triggers[_triggers.indexOf(self) + 1]; + + self.revert = (revert, temp) => { + if (!temp) { return self.kill(true); } // for compatibility with gsap.context() and gsap.matchMedia() which call revert() + let r = revert !== false || !self.enabled, + prevRefreshing = _refreshing; + if (r !== self.isReverted) { + if (r) { + prevScroll = Math.max(scrollFunc(), self.scroll.rec || 0); // record the scroll so we can revert later (repositioning/pinning things can affect scroll position). In the static refresh() method, we first record all the scroll positions as a reference. + prevProgress = self.progress; + prevAnimProgress = animation && animation.progress(); + } + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(m => m.style.display = r ? "none" : "block"); + if (r) { + _refreshing = self; + self.update(r); // make sure the pin is back in its original position so that all the measurements are correct. do this BEFORE swapping the pin out + } + if (pin && (!pinReparent || !self.isActive)) { + if (r) { + _swapPinOut(pin, spacer, pinOriginalState); + } else { + _swapPinIn(pin, spacer, _getComputedStyle(pin), spacerState); + } + } + r || self.update(r); // when we're restoring, the update should run AFTER swapping the pin into its pin-spacer. + _refreshing = prevRefreshing; // restore. We set it to true during the update() so that things fire properly in there. + self.isReverted = r; + } + } + + self.refresh = (soft, force, position, pinOffset) => { // position is typically only defined if it's coming from setPositions() - it's a way to skip the normal parsing. pinOffset is also only from setPositions() and is mostly related to fancy stuff we need to do in ScrollSmoother with effects + if ((_refreshing || !self.enabled) && !force) { + return; + } + if (pin && soft && _lastScrollTime) { + _addListener(ScrollTrigger, "scrollEnd", _softRefresh); + return; + } + !_refreshingAll && onRefreshInit && onRefreshInit(self); + _refreshing = self; + if (tweenTo.tween && !position) { // we skip this if a position is passed in because typically that's from .setPositions() and it's best to allow in-progress snapping to continue. + tweenTo.tween.kill(); + tweenTo.tween = 0; + } + scrubTween && scrubTween.pause(); + invalidateOnRefresh && animation && animation.revert({kill: false}).invalidate(); + self.isReverted || self.revert(true, true); + self._subPinOffset = false; // we'll set this to true in the sub-pins if we find any + let size = getScrollerSize(), + scrollerBounds = getScrollerOffsets(), + max = containerAnimation ? containerAnimation.duration() : _maxScroll(scroller, direction), + isFirstRefresh = change <= 0.01, + offset = 0, + otherPinOffset = pinOffset || 0, + parsedEnd = _isObject(position) ? position.end : vars.end, + parsedEndTrigger = vars.endTrigger || trigger, + parsedStart = _isObject(position) ? position.start : (vars.start || (vars.start === 0 || !trigger ? 0 : (pin ? "0 0" : "0 100%"))), + pinnedContainer = self.pinnedContainer = vars.pinnedContainer && _getTarget(vars.pinnedContainer, self), + triggerIndex = (trigger && Math.max(0, _triggers.indexOf(self))) || 0, + i = triggerIndex, + cs, bounds, scroll, isVertical, override, curTrigger, curPin, oppositeScroll, initted, revertedPins, forcedOverflow, markerStartOffset, markerEndOffset; + if (markers && _isObject(position)) { // if we alter the start/end positions with .setPositions(), it generally feeds in absolute NUMBERS which don't convey information about where to line up the markers, so to keep it intuitive, we record how far the trigger positions shift after applying the new numbers and then offset by that much in the opposite direction. We do the same to the associated trigger markers too of course. + markerStartOffset = gsap.getProperty(markerStartTrigger, direction.p); + markerEndOffset = gsap.getProperty(markerEndTrigger, direction.p); + } + while (i-- > 0) { // user might try to pin the same element more than once, so we must find any prior triggers with the same pin, revert them, and determine how long they're pinning so that we can offset things appropriately. Make sure we revert from last to first so that things "rewind" properly. + curTrigger = _triggers[i]; + curTrigger.end || curTrigger.refresh(0, 1) || (_refreshing = self); // if it's a timeline-based trigger that hasn't been fully initialized yet because it's waiting for 1 tick, just force the refresh() here, otherwise if it contains a pin that's supposed to affect other ScrollTriggers further down the page, they won't be adjusted properly. + curPin = curTrigger.pin; + if (curPin && (curPin === trigger || curPin === pin || curPin === pinnedContainer) && !curTrigger.isReverted) { + revertedPins || (revertedPins = []); + revertedPins.unshift(curTrigger); // we'll revert from first to last to make sure things reach their end state properly + curTrigger.revert(true, true); + } + if (curTrigger !== _triggers[i]) { // in case it got removed. + triggerIndex--; + i--; + } + } + _isFunction(parsedStart) && (parsedStart = parsedStart(self)); + parsedStart = _parseClamp(parsedStart, "start", self); + start = _parsePosition(parsedStart, trigger, size, direction, scrollFunc(), markerStart, markerStartTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._startClamp && "_startClamp") || (pin ? -0.001 : 0); + _isFunction(parsedEnd) && (parsedEnd = parsedEnd(self)); + if (_isString(parsedEnd) && !parsedEnd.indexOf("+=")) { + if (~parsedEnd.indexOf(" ")) { + parsedEnd = (_isString(parsedStart) ? parsedStart.split(" ")[0] : "") + parsedEnd; + } else { + offset = _offsetToPx(parsedEnd.substr(2), size); + parsedEnd = _isString(parsedStart) ? parsedStart : (containerAnimation ? gsap.utils.mapRange(0, containerAnimation.duration(), containerAnimation.scrollTrigger.start, containerAnimation.scrollTrigger.end, start) : start) + offset; // _parsePosition won't factor in the offset if the start is a number, so do it here. + parsedEndTrigger = trigger; + } + } + parsedEnd = _parseClamp(parsedEnd, "end", self); + end = Math.max(start, _parsePosition(parsedEnd || (parsedEndTrigger ? "100% 0" : max), parsedEndTrigger, size, direction, scrollFunc() + offset, markerEnd, markerEndTrigger, self, scrollerBounds, borderWidth, useFixedPosition, max, containerAnimation, self._endClamp && "_endClamp")) || -0.001; + + offset = 0; + i = triggerIndex; + while (i--) { + curTrigger = _triggers[i]; + curPin = curTrigger.pin; + if (curPin && curTrigger.start - curTrigger._pinPush <= start && !containerAnimation && curTrigger.end > 0) { + cs = curTrigger.end - (self._startClamp ? Math.max(0, curTrigger.start) : curTrigger.start); + if (((curPin === trigger && curTrigger.start - curTrigger._pinPush < start) || curPin === pinnedContainer) && isNaN(parsedStart)) { // numeric start values shouldn't be offset at all - treat them as absolute + offset += cs * (1 - curTrigger.progress); + } + curPin === pin && (otherPinOffset += cs); + } + } + start += offset; + end += offset; + self._startClamp && (self._startClamp += offset); + + if (self._endClamp && !_refreshingAll) { + self._endClamp = end || -0.001; + end = Math.min(end, _maxScroll(scroller, direction)); + } + change = (end - start) || ((start -= 0.01) && 0.001); + + if (isFirstRefresh) { // on the very first refresh(), the prevProgress couldn't have been accurate yet because the start/end were never calculated, so we set it here. Before 3.11.5, it could lead to an inaccurate scroll position restoration with snapping. + prevProgress = gsap.utils.clamp(0, 1, gsap.utils.normalize(start, end, prevScroll)); + } + self._pinPush = otherPinOffset; + if (markerStart && offset) { // offset the markers if necessary + cs = {}; + cs[direction.a] = "+=" + offset; + pinnedContainer && (cs[direction.p] = "-=" + scrollFunc()); + gsap.set([markerStart, markerEnd], cs); + } + + if (pin && !(_clampingMax && self.end >= _maxScroll(scroller, direction))) { + cs = _getComputedStyle(pin); + isVertical = direction === _vertical; + scroll = scrollFunc(); // recalculate because the triggers can affect the scroll + pinStart = parseFloat(pinGetter(direction.a)) + otherPinOffset; + if (!max && end > 1) { // makes sure the scroller has a scrollbar, otherwise if something has width: 100%, for example, it would be too big (exclude the scrollbar). See https://gsap.com/forums/topic/25182-scrolltrigger-width-of-page-increase-where-markers-are-set-to-false/ + forcedOverflow = (isViewport ? (_doc.scrollingElement || _docEl) : scroller).style; + forcedOverflow = {style: forcedOverflow, value: forcedOverflow["overflow" + direction.a.toUpperCase()]}; + if (isViewport && _getComputedStyle(_body)["overflow" + direction.a.toUpperCase()] !== "scroll") { // avoid an extra scrollbar if BOTH <html> and <body> have overflow set to "scroll" + forcedOverflow.style["overflow" + direction.a.toUpperCase()] = "scroll"; + } + } + _swapPinIn(pin, spacer, cs); + pinState = _getState(pin); + // transforms will interfere with the top/left/right/bottom placement, so remove them temporarily. getBoundingClientRect() factors in transforms. + bounds = _getBounds(pin, true); + oppositeScroll = useFixedPosition && _getScrollFunc(scroller, isVertical ? _horizontal : _vertical)(); + if (pinSpacing) { + spacerState = [pinSpacing + direction.os2, change + otherPinOffset + _px]; + spacerState.t = spacer; + i = (pinSpacing === _padding) ? _getSize(pin, direction) + change + otherPinOffset : 0; + if (i) { + spacerState.push(direction.d, i + _px); // for box-sizing: border-box (must include padding). + spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + _setState(spacerState); + if (pinnedContainer) { // in ScrollTrigger.refresh(), we need to re-evaluate the pinContainer's size because this pinSpacing may stretch it out, but we can't just add the exact distance because depending on layout, it may not push things down or it may only do so partially. + _triggers.forEach(t => { + if (t.pin === pinnedContainer && t.vars.pinSpacing !== false) { + t._subPinOffset = true; + } + }); + } + useFixedPosition && scrollFunc(prevScroll); + } else { + i = _getSize(pin, direction); + i && spacer.style.flexBasis !== "auto" && (spacer.style.flexBasis = i + _px); + } + if (useFixedPosition) { + override = { + top: (bounds.top + (isVertical ? scroll - start : oppositeScroll)) + _px, + left: (bounds.left + (isVertical ? oppositeScroll : scroll - start)) + _px, + boxSizing: "border-box", + position: "fixed" + }; + override[_width] = override["max" + _Width] = Math.ceil(bounds.width) + _px; + override[_height] = override["max" + _Height] = Math.ceil(bounds.height) + _px; + override[_margin] = override[_margin + _Top] = override[_margin + _Right] = override[_margin + _Bottom] = override[_margin + _Left] = "0"; + override[_padding] = cs[_padding]; + override[_padding + _Top] = cs[_padding + _Top]; + override[_padding + _Right] = cs[_padding + _Right]; + override[_padding + _Bottom] = cs[_padding + _Bottom]; + override[_padding + _Left] = cs[_padding + _Left]; + pinActiveState = _copyState(pinOriginalState, override, pinReparent); + _refreshingAll && scrollFunc(0); + } + if (animation) { // the animation might be affecting the transform, so we must jump to the end, check the value, and compensate accordingly. Otherwise, when it becomes unpinned, the pinSetter() will get set to a value that doesn't include whatever the animation did. + initted = animation._initted; // if not, we must invalidate() after this step, otherwise it could lock in starting values prematurely. + _suppressOverwrites(1); + animation.render(animation.duration(), true, true); + pinChange = pinGetter(direction.a) - pinStart + change + otherPinOffset; + pinMoves = Math.abs(change - pinChange) > 1; + useFixedPosition && pinMoves && pinActiveState.splice(pinActiveState.length - 2, 2); // transform is the last property/value set in the state Array. Since the animation is controlling that, we should omit it. + animation.render(0, true, true); + initted || animation.invalidate(true); + animation.parent || animation.totalTime(animation.totalTime()); // if, for example, a toggleAction called play() and then refresh() happens and when we render(1) above, it would cause the animation to complete and get removed from its parent, so this makes sure it gets put back in. + _suppressOverwrites(0); + } else { + pinChange = change + } + forcedOverflow && (forcedOverflow.value ? (forcedOverflow.style["overflow" + direction.a.toUpperCase()] = forcedOverflow.value) : forcedOverflow.style.removeProperty("overflow-" + direction.a)); + } else if (trigger && scrollFunc() && !containerAnimation) { // it may be INSIDE a pinned element, so walk up the tree and look for any elements with _pinOffset to compensate because anything with pinSpacing that's already scrolled would throw off the measurements in getBoundingClientRect() + bounds = trigger.parentNode; + while (bounds && bounds !== _body) { + if (bounds._pinOffset) { + start -= bounds._pinOffset; + end -= bounds._pinOffset; + } + bounds = bounds.parentNode; + } + } + revertedPins && revertedPins.forEach(t => t.revert(false, true)); + self.start = start; + self.end = end; + scroll1 = scroll2 = _refreshingAll ? prevScroll : scrollFunc(); // reset velocity + if (!containerAnimation && !_refreshingAll) { + scroll1 < prevScroll && scrollFunc(prevScroll); + self.scroll.rec = 0; + } + self.revert(false, true); + lastRefresh = _getTime(); + if (snapDelayedCall) { + lastSnap = -1; // just so snapping gets re-enabled, clear out any recorded last value + // self.isActive && scrollFunc(start + change * prevProgress); // previously this line was here to ensure that when snapping kicks in, it's from the previous progress but in some cases that's not desirable, like an all-page ScrollTrigger when new content gets added to the page, that'd totally change the progress. + snapDelayedCall.restart(true); + } + _refreshing = 0; + animation && isToggle && (animation._initted || prevAnimProgress) && animation.progress() !== prevAnimProgress && animation.progress(prevAnimProgress || 0, true).render(animation.time(), true, true); // must force a re-render because if saveStyles() was used on the target(s), the styles could have been wiped out during the refresh(). + if (isFirstRefresh || prevProgress !== self.progress || containerAnimation || invalidateOnRefresh || (animation && !animation._initted)) { // ensures that the direction is set properly (when refreshing, progress is set back to 0 initially, then back again to wherever it needs to be) and that callbacks are triggered. + animation && !isToggle && animation.totalProgress(containerAnimation && start < -0.001 && !prevProgress ? gsap.utils.normalize(start, end, 0) : prevProgress, true); // to avoid issues where animation callbacks like onStart aren't triggered. + self.progress = isFirstRefresh || ((scroll1 - start) / change === prevProgress) ? 0 : prevProgress; + } + pin && pinSpacing && (spacer._pinOffset = Math.round(self.progress * pinChange)); + scrubTween && scrubTween.invalidate(); + + if (!isNaN(markerStartOffset)) { // numbers were passed in for the position which are absolute, so instead of just putting the markers at the very bottom of the viewport, we figure out how far they shifted down (it's safe to assume they were originally positioned in closer relation to the trigger element with values like "top", "center", a percentage or whatever, so we offset that much in the opposite direction to basically revert them to the relative position thy were at previously. + markerStartOffset -= gsap.getProperty(markerStartTrigger, direction.p); + markerEndOffset -= gsap.getProperty(markerEndTrigger, direction.p); + _shiftMarker(markerStartTrigger, direction, markerStartOffset); + _shiftMarker(markerStart, direction, markerStartOffset - (pinOffset || 0)); + _shiftMarker(markerEndTrigger, direction, markerEndOffset); + _shiftMarker(markerEnd, direction, markerEndOffset - (pinOffset || 0)); + } + + isFirstRefresh && !_refreshingAll && self.update(); // edge case - when you reload a page when it's already scrolled down, some browsers fire a "scroll" event before DOMContentLoaded, triggering an updateAll(). If we don't update the self.progress as part of refresh(), then when it happens next, it may record prevProgress as 0 when it really shouldn't, potentially causing a callback in an animation to fire again. + + if (onRefresh && !_refreshingAll && !executingOnRefresh) { // when refreshing all, we do extra work to correct pinnedContainer sizes and ensure things don't exceed the maxScroll, so we should do all the refreshes at the end after all that work so that the start/end values are corrected. + executingOnRefresh = true; + onRefresh(self); + executingOnRefresh = false; + } + }; + + self.getVelocity = () => ((scrollFunc() - scroll2) / (_getTime() - _time2) * 1000) || 0; + + self.endAnimation = () => { + _endAnimation(self.callbackAnimation); + if (animation) { + scrubTween ? scrubTween.progress(1) : (!animation.paused() ? _endAnimation(animation, animation.reversed()) : isToggle || _endAnimation(animation, self.direction < 0, 1)); + } + }; + + self.labelToScroll = label => animation && animation.labels && ((start || self.refresh() || start) + (animation.labels[label] / animation.duration()) * change) || 0; + + self.getTrailing = name => { + let i = _triggers.indexOf(self), + a = self.direction > 0 ? _triggers.slice(0, i).reverse() : _triggers.slice(i+1); + return (_isString(name) ? a.filter(t => t.vars.preventOverlaps === name) : a).filter(t => self.direction > 0 ? t.end <= start : t.start >= end); + }; + + + self.update = (reset, recordVelocity, forceFake) => { + if (containerAnimation && !forceFake && !reset) { + return; + } + let scroll = _refreshingAll === true ? prevScroll : self.scroll(), + p = reset ? 0 : (scroll - start) / change, + clipped = p < 0 ? 0 : p > 1 ? 1 : p || 0, + prevProgress = self.progress, + isActive, wasActive, toggleState, action, stateChanged, toggled, isAtMax, isTakingAction; + if (recordVelocity) { + scroll2 = scroll1; + scroll1 = containerAnimation ? scrollFunc() : scroll; + if (snap) { + snap2 = snap1; + snap1 = animation && !isToggle ? animation.totalProgress() : clipped; + } + } + // anticipate the pinning a few ticks ahead of time based on velocity to avoid a visual glitch due to the fact that most browsers do scrolling on a separate thread (not synced with requestAnimationFrame). + if (anticipatePin && pin && !_refreshing && !_startup && _lastScrollTime) { + if (!clipped && start < scroll + ((scroll - scroll2) / (_getTime() - _time2)) * anticipatePin) { + clipped = 0.0001; + } else if (clipped === 1 && end > scroll + ((scroll - scroll2) / (_getTime() - _time2)) * anticipatePin) { + clipped = 0.9999; + } + } + if (clipped !== prevProgress && self.enabled) { + isActive = self.isActive = !!clipped && clipped < 1; + wasActive = !!prevProgress && prevProgress < 1; + toggled = isActive !== wasActive; + stateChanged = toggled || !!clipped !== !!prevProgress; // could go from start all the way to end, thus it didn't toggle but it did change state in a sense (may need to fire a callback) + self.direction = clipped > prevProgress ? 1 : -1; + self.progress = clipped; + + if (stateChanged && !_refreshing) { + toggleState = clipped && !prevProgress ? 0 : clipped === 1 ? 1 : prevProgress === 1 ? 2 : 3; // 0 = enter, 1 = leave, 2 = enterBack, 3 = leaveBack (we prioritize the FIRST encounter, thus if you scroll really fast past the onEnter and onLeave in one tick, it'd prioritize onEnter. + if (isToggle) { + action = (!toggled && toggleActions[toggleState + 1] !== "none" && toggleActions[toggleState + 1]) || toggleActions[toggleState]; // if it didn't toggle, that means it shot right past and since we prioritize the "enter" action, we should switch to the "leave" in this case (but only if one is defined) + isTakingAction = animation && (action === "complete" || action === "reset" || action in animation); + } + } + + preventOverlaps && (toggled || isTakingAction) && (isTakingAction || scrub || !animation) && (_isFunction(preventOverlaps) ? preventOverlaps(self) : self.getTrailing(preventOverlaps).forEach(t => t.endAnimation())); + + if (!isToggle) { + if (scrubTween && !_refreshing && !_startup) { + (scrubTween._dp._time - scrubTween._start !== scrubTween._time) && scrubTween.render(scrubTween._dp._time - scrubTween._start); // if there's a scrub on both the container animation and this one (or a ScrollSmoother), the update order would cause this one not to have rendered yet, so it wouldn't make any progress before we .restart() it heading toward the new progress so it'd appear stuck thus we force a render here. + if (scrubTween.resetTo) { + scrubTween.resetTo("totalProgress", clipped, animation._tTime / animation._tDur); + } else { // legacy support (courtesy), before 3.10.0 + scrubTween.vars.totalProgress = clipped; + scrubTween.invalidate().restart(); + } + } else if (animation) { + animation.totalProgress(clipped, !!(_refreshing && (lastRefresh || reset))); + } + } + if (pin) { + reset && pinSpacing && (spacer.style[pinSpacing + direction.os2] = spacingStart); + if (!useFixedPosition) { + pinSetter(_round(pinStart + pinChange * clipped)); + } else if (stateChanged) { + isAtMax = !reset && clipped > prevProgress && end + 1 > scroll && scroll + 1 >= _maxScroll(scroller, direction); // if it's at the VERY end of the page, don't switch away from position: fixed because it's pointless and it could cause a brief flash when the user scrolls back up (when it gets pinned again) + if (pinReparent) { + if (!reset && (isActive || isAtMax)) { + let bounds = _getBounds(pin, true), + offset = scroll - start; + _reparent(pin, _body, (bounds.top + (direction === _vertical ? offset : 0)) + _px, (bounds.left + (direction === _vertical ? 0 : offset)) + _px); + } else { + _reparent(pin, spacer); + } + } + _setState(isActive || isAtMax ? pinActiveState : pinState); + (pinMoves && clipped < 1 && isActive) || pinSetter(pinStart + (clipped === 1 && !isAtMax ? pinChange : 0)); + } + } + snap && !tweenTo.tween && !_refreshing && !_startup && snapDelayedCall.restart(true); + toggleClass && (toggled || (once && clipped && (clipped < 1 || !_limitCallbacks))) && _toArray(toggleClass.targets).forEach(el => el.classList[isActive || once ? "add" : "remove"](toggleClass.className)); // classes could affect positioning, so do it even if reset or refreshing is true. + onUpdate && !isToggle && !reset && onUpdate(self); + if (stateChanged && !_refreshing) { + if (isToggle) { + if (isTakingAction) { + if (action === "complete") { + animation.pause().totalProgress(1); + } else if (action === "reset") { + animation.restart(true).pause(); + } else if (action === "restart") { + animation.restart(true); + } else { + animation[action](); + } + } + onUpdate && onUpdate(self); + } + if (toggled || !_limitCallbacks) { // on startup, the page could be scrolled and we don't want to fire callbacks that didn't toggle. For example onEnter shouldn't fire if the ScrollTrigger isn't actually entered. + onToggle && toggled && _callback(self, onToggle); + callbacks[toggleState] && _callback(self, callbacks[toggleState]); + once && (clipped === 1 ? self.kill(false, 1) : (callbacks[toggleState] = 0)); // a callback shouldn't be called again if once is true. + if (!toggled) { // it's possible to go completely past, like from before the start to after the end (or vice-versa) in which case BOTH callbacks should be fired in that order + toggleState = clipped === 1 ? 1 : 3; + callbacks[toggleState] && _callback(self, callbacks[toggleState]); + } + } + if (fastScrollEnd && !isActive && Math.abs(self.getVelocity()) > (_isNumber(fastScrollEnd) ? fastScrollEnd : 2500)) { + _endAnimation(self.callbackAnimation); + scrubTween ? scrubTween.progress(1) : _endAnimation(animation, action === "reverse" ? 1 : !clipped, 1); + } + } else if (isToggle && onUpdate && !_refreshing) { + onUpdate(self); + } + } + // update absolutely-positioned markers (only if the scroller isn't the viewport) + if (markerEndSetter) { + let n = containerAnimation ? scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0) : scroll; + markerStartSetter(n + (markerStartTrigger._isFlipped ? 1 : 0)); + markerEndSetter(n); + } + caMarkerSetter && caMarkerSetter(-scroll / containerAnimation.duration() * (containerAnimation._caScrollDist || 0)); + }; + + self.enable = (reset, refresh) => { + if (!self.enabled) { + self.enabled = true; + _addListener(scroller, "resize", _onResize); + isViewport || _addListener(scroller, "scroll", _onScroll); + onRefreshInit && _addListener(ScrollTrigger, "refreshInit", onRefreshInit); + if (reset !== false) { + self.progress = prevProgress = 0; + scroll1 = scroll2 = lastSnap = scrollFunc(); + } + refresh !== false && self.refresh(); + } + }; + + self.getTween = snap => snap && tweenTo ? tweenTo.tween : scrubTween; + + self.setPositions = (newStart, newEnd, keepClamp, pinOffset) => { // doesn't persist after refresh()! Intended to be a way to override values that were set during refresh(), like you could set it in onRefresh() + if (containerAnimation) { // convert ratios into scroll positions. Remember, start/end values on ScrollTriggers that have a containerAnimation refer to the time (in seconds), NOT scroll positions. + let st = containerAnimation.scrollTrigger, + duration = containerAnimation.duration(), + change = st.end - st.start; + newStart = st.start + change * newStart / duration; + newEnd = st.start + change * newEnd / duration; + } + self.refresh(false, false, {start: _keepClamp(newStart, keepClamp && !!self._startClamp), end: _keepClamp(newEnd, keepClamp && !!self._endClamp)}, pinOffset); + self.update(); + }; + + self.adjustPinSpacing = amount => { + if (spacerState && amount) { + let i = spacerState.indexOf(direction.d) + 1; + spacerState[i] = (parseFloat(spacerState[i]) + amount) + _px; + spacerState[1] = (parseFloat(spacerState[1]) + amount) + _px; + _setState(spacerState); + } + }; + + self.disable = (reset, allowAnimation) => { + if (self.enabled) { + reset !== false && self.revert(true, true); + self.enabled = self.isActive = false; + allowAnimation || (scrubTween && scrubTween.pause()); + prevScroll = 0; + pinCache && (pinCache.uncache = 1); + onRefreshInit && _removeListener(ScrollTrigger, "refreshInit", onRefreshInit); + if (snapDelayedCall) { + snapDelayedCall.pause(); + tweenTo.tween && tweenTo.tween.kill() && (tweenTo.tween = 0); + } + if (!isViewport) { + let i = _triggers.length; + while (i--) { + if (_triggers[i].scroller === scroller && _triggers[i] !== self) { + return; //don't remove the listeners if there are still other triggers referencing it. + } + } + _removeListener(scroller, "resize", _onResize); + isViewport || _removeListener(scroller, "scroll", _onScroll); + } + } + }; + + self.kill = (revert, allowAnimation) => { + self.disable(revert, allowAnimation); + scrubTween && !allowAnimation && scrubTween.kill(); + id && (delete _ids[id]); + let i = _triggers.indexOf(self); + i >= 0 && _triggers.splice(i, 1); + i === _i && _direction > 0 && _i--; // if we're in the middle of a refresh() or update(), splicing would cause skips in the index, so adjust... + + // if no other ScrollTrigger instances of the same scroller are found, wipe out any recorded scroll position. Otherwise, in a single page application, for example, it could maintain scroll position when it really shouldn't. + i = 0; + _triggers.forEach(t => t.scroller === self.scroller && (i = 1)); + i || _refreshingAll || (self.scroll.rec = 0); + + if (animation) { + animation.scrollTrigger = null; + revert && animation.revert({kill: false}); + allowAnimation || animation.kill(); + } + markerStart && [markerStart, markerEnd, markerStartTrigger, markerEndTrigger].forEach(m => m.parentNode && m.parentNode.removeChild(m)); + _primary === self && (_primary = 0); + if (pin) { + pinCache && (pinCache.uncache = 1); + i = 0; + _triggers.forEach(t => t.pin === pin && i++); + i || (pinCache.spacer = 0); // if there aren't any more ScrollTriggers with the same pin, remove the spacer, otherwise it could be contaminated with old/stale values if the user re-creates a ScrollTrigger for the same element. + } + vars.onKill && vars.onKill(self); + }; + + _triggers.push(self); + self.enable(false, false); + customRevertReturn && customRevertReturn(self); + + if (animation && animation.add && !change) { // if the animation is a timeline, it may not have been populated yet, so it wouldn't render at the proper place on the first refresh(), thus we should schedule one for the next tick. If "change" is defined, we know it must be re-enabling, thus we can refresh() right away. + let updateFunc = self.update; // some browsers may fire a scroll event BEFORE a tick elapses and/or the DOMContentLoaded fires. So there's a chance update() will be called BEFORE a refresh() has happened on a Timeline-attached ScrollTrigger which means the start/end won't be calculated yet. We don't want to add conditional logic inside the update() method (like check to see if end is defined and if not, force a refresh()) because that's a function that gets hit a LOT (performance). So we swap out the real update() method for this one that'll re-attach it the first time it gets called and of course forces a refresh(). + self.update = () => { + self.update = updateFunc; + _scrollers.cache++; // otherwise a cached scroll position may get used in the refresh() in a very rare scenario, like if ScrollTriggers are created inside a DOMContentLoaded event and the queued requestAnimationFrame() fires beforehand. See https://gsap.com/community/forums/topic/41267-scrolltrigger-breaks-on-refresh-when-using-domcontentloaded/ + start || end || self.refresh(); + }; + gsap.delayedCall(0.01, self.update); + change = 0.01; + start = end = 0; + } else { + self.refresh(); + } + pin && _queueRefreshAll(); // pinning could affect the positions of other things, so make sure we queue a full refresh() + } + + + static register(core) { + if (!_coreInitted) { + gsap = core || _getGSAP(); + _windowExists() && window.document && ScrollTrigger.enable(); + _coreInitted = _enabled; + } + return _coreInitted; + } + + static defaults(config) { + if (config) { + for (let p in config) { + _defaults[p] = config[p]; + } + } + return _defaults; + } + + static disable(reset, kill) { + _enabled = 0; + _triggers.forEach(trigger => trigger[kill ? "kill" : "disable"](reset)); + _removeListener(_win, "wheel", _onScroll); + _removeListener(_doc, "scroll", _onScroll); + clearInterval(_syncInterval); + _removeListener(_doc, "touchcancel", _passThrough); + _removeListener(_body, "touchstart", _passThrough); + _multiListener(_removeListener, _doc, "pointerdown,touchstart,mousedown", _pointerDownHandler); + _multiListener(_removeListener, _doc, "pointerup,touchend,mouseup", _pointerUpHandler); + _resizeDelay.kill(); + _iterateAutoRefresh(_removeListener); + for (let i = 0; i < _scrollers.length; i+=3) { + _wheelListener(_removeListener, _scrollers[i], _scrollers[i+1]); + _wheelListener(_removeListener, _scrollers[i], _scrollers[i+2]); + } + } + + static enable() { + _win = window; + _doc = document; + _docEl = _doc.documentElement; + _body = _doc.body; + if (gsap) { + _toArray = gsap.utils.toArray; + _clamp = gsap.utils.clamp; + _context = gsap.core.context || _passThrough; + _suppressOverwrites = gsap.core.suppressOverwrites || _passThrough; + _scrollRestoration = _win.history.scrollRestoration || "auto"; + _lastScroll = _win.pageYOffset || 0; + gsap.core.globals("ScrollTrigger", ScrollTrigger); // must register the global manually because in Internet Explorer, functions (classes) don't have a "name" property. + if (_body) { + _enabled = 1; + _div100vh = document.createElement("div"); // to solve mobile browser address bar show/hide resizing, we shouldn't rely on window.innerHeight. Instead, use a <div> with its height set to 100vh and measure that since that's what the scrolling is based on anyway and it's not affected by address bar showing/hiding. + _div100vh.style.height = "100vh"; + _div100vh.style.position = "absolute"; + _refresh100vh(); + _rafBugFix(); + Observer.register(gsap); + // isTouch is 0 if no touch, 1 if ONLY touch, and 2 if it can accommodate touch but also other types like mouse/pointer. + ScrollTrigger.isTouch = Observer.isTouch; + _fixIOSBug = Observer.isTouch && /(iPad|iPhone|iPod|Mac)/g.test(navigator.userAgent); // since 2017, iOS has had a bug that causes event.clientX/Y to be inaccurate when a scroll occurs, thus we must alternate ignoring every other touchmove event to work around it. See https://bugs.webkit.org/show_bug.cgi?id=181954 and https://codepen.io/GreenSock/pen/ExbrPNa/087cef197dc35445a0951e8935c41503 + _ignoreMobileResize = Observer.isTouch === 1; + _addListener(_win, "wheel", _onScroll); // mostly for 3rd party smooth scrolling libraries. + _root = [_win, _doc, _docEl, _body]; + if (gsap.matchMedia) { + ScrollTrigger.matchMedia = vars => { + let mm = gsap.matchMedia(), + p; + for (p in vars) { + mm.add(p, vars[p]); + } + return mm; + }; + gsap.addEventListener("matchMediaInit", () => _revertAll()); + gsap.addEventListener("matchMediaRevert", () => _revertRecorded()); + gsap.addEventListener("matchMedia", () => { + _refreshAll(0, 1); + _dispatch("matchMedia"); + }); + gsap.matchMedia().add("(orientation: portrait)", () => { // when orientation changes, we should take new base measurements for the ignoreMobileResize feature. + _setBaseDimensions(); + return _setBaseDimensions; + }); + } else { + console.warn("Requires GSAP 3.11.0 or later"); + } + _setBaseDimensions(); + _addListener(_doc, "scroll", _onScroll); // some browsers (like Chrome), the window stops dispatching scroll events on the window if you scroll really fast, but it's consistent on the document! + let bodyHasStyle = _body.hasAttribute("style"), + bodyStyle = _body.style, + border = bodyStyle.borderTopStyle, + AnimationProto = gsap.core.Animation.prototype, + bounds, i; + AnimationProto.revert || Object.defineProperty(AnimationProto, "revert", { value: function() { return this.time(-0.01, true); }}); // only for backwards compatibility (Animation.revert() was added after 3.10.4) + bodyStyle.borderTopStyle = "solid"; // works around an issue where a margin of a child element could throw off the bounds of the _body, making it seem like there's a margin when there actually isn't. The border ensures that the bounds are accurate. + bounds = _getBounds(_body); + _vertical.m = Math.round(bounds.top + _vertical.sc()) || 0; // accommodate the offset of the <body> caused by margins and/or padding + _horizontal.m = Math.round(bounds.left + _horizontal.sc()) || 0; + border ? (bodyStyle.borderTopStyle = border) : bodyStyle.removeProperty("border-top-style"); + if (!bodyHasStyle) { // SSR frameworks like Next.js complain if this attribute gets added. + _body.setAttribute("style", ""); // it's not enough to just removeAttribute() - we must first set it to empty, otherwise Next.js complains. + _body.removeAttribute("style"); + } + // TODO: (?) maybe move to leveraging the velocity mechanism in Observer and skip intervals. + _syncInterval = setInterval(_sync, 250); + gsap.delayedCall(0.5, () => _startup = 0); + _addListener(_doc, "touchcancel", _passThrough); // some older Android devices intermittently stop dispatching "touchmove" events if we don't listen for "touchcancel" on the document. + _addListener(_body, "touchstart", _passThrough); //works around Safari bug: https://gsap.com/forums/topic/21450-draggable-in-iframe-on-mobile-is-buggy/ + _multiListener(_addListener, _doc, "pointerdown,touchstart,mousedown", _pointerDownHandler); + _multiListener(_addListener, _doc, "pointerup,touchend,mouseup", _pointerUpHandler); + _transformProp = gsap.utils.checkPrefix("transform"); + _stateProps.push(_transformProp); + _coreInitted = _getTime(); + _resizeDelay = gsap.delayedCall(0.2, _refreshAll).pause(); + _autoRefresh = [_doc, "visibilitychange", () => { + let w = _win.innerWidth, + h = _win.innerHeight; + if (_doc.hidden) { + _prevWidth = w; + _prevHeight = h; + } else if (_prevWidth !== w || _prevHeight !== h) { + _onResize(); + } + }, _doc, "DOMContentLoaded", _refreshAll, _win, "load", _refreshAll, _win, "resize", _onResize]; + _iterateAutoRefresh(_addListener); + _triggers.forEach(trigger => trigger.enable(0, 1)); + for (i = 0; i < _scrollers.length; i+=3) { + _wheelListener(_removeListener, _scrollers[i], _scrollers[i+1]); + _wheelListener(_removeListener, _scrollers[i], _scrollers[i+2]); + } + } + } + } + + static config(vars) { + ("limitCallbacks" in vars) && (_limitCallbacks = !!vars.limitCallbacks); + let ms = vars.syncInterval; + ms && clearInterval(_syncInterval) || ((_syncInterval = ms) && setInterval(_sync, ms)); + ("ignoreMobileResize" in vars) && (_ignoreMobileResize = ScrollTrigger.isTouch === 1 && vars.ignoreMobileResize); + if ("autoRefreshEvents" in vars) { + _iterateAutoRefresh(_removeListener) || _iterateAutoRefresh(_addListener, vars.autoRefreshEvents || "none"); + _ignoreResize = (vars.autoRefreshEvents + "").indexOf("resize") === -1; + } + } + + static scrollerProxy(target, vars) { + let t = _getTarget(target), + i = _scrollers.indexOf(t), + isViewport = _isViewport(t); + if (~i) { + _scrollers.splice(i, isViewport ? 6 : 2); + } + if (vars) { + isViewport ? _proxies.unshift(_win, vars, _body, vars, _docEl, vars) : _proxies.unshift(t, vars); + } + } + + static clearMatchMedia(query) { + _triggers.forEach(t => t._ctx && t._ctx.query === query && t._ctx.kill(true, true)); + } + + static isInViewport(element, ratio, horizontal) { + let bounds = (_isString(element) ? _getTarget(element) : element).getBoundingClientRect(), + offset = bounds[horizontal ? _width : _height] * ratio || 0; + return horizontal ? bounds.right - offset > 0 && bounds.left + offset < _win.innerWidth : bounds.bottom - offset > 0 && bounds.top + offset < _win.innerHeight; + } + + static positionInViewport(element, referencePoint, horizontal) { + _isString(element) && (element = _getTarget(element)); + let bounds = element.getBoundingClientRect(), + size = bounds[horizontal ? _width : _height], + offset = referencePoint == null ? size / 2 : ((referencePoint in _keywords) ? _keywords[referencePoint] * size : ~referencePoint.indexOf("%") ? parseFloat(referencePoint) * size / 100 : parseFloat(referencePoint) || 0); + return horizontal ? (bounds.left + offset) / _win.innerWidth : (bounds.top + offset) / _win.innerHeight; + } + + static killAll(allowListeners) { + _triggers.slice(0).forEach(t => t.vars.id !== "ScrollSmoother" && t.kill()); + if (allowListeners !== true) { + let listeners = _listeners.killAll || []; + _listeners = {}; + listeners.forEach(f => f()); + } + } + +} + +ScrollTrigger.version = "3.12.7"; +ScrollTrigger.saveStyles = targets => targets ? _toArray(targets).forEach(target => { // saved styles are recorded in a consecutive alternating Array, like [element, cssText, transform attribute, cache, matchMedia, ...] + if (target && target.style) { + let i = _savedStyles.indexOf(target); + i >= 0 && _savedStyles.splice(i, 5); + _savedStyles.push(target, target.style.cssText, target.getBBox && target.getAttribute("transform"), gsap.core.getCache(target), _context()); + } +}) : _savedStyles; +ScrollTrigger.revert = (soft, media) => _revertAll(!soft, media); +ScrollTrigger.create = (vars, animation) => new ScrollTrigger(vars, animation); +ScrollTrigger.refresh = safe => safe ? _onResize(true) : (_coreInitted || ScrollTrigger.register()) && _refreshAll(true); +ScrollTrigger.update = force => ++_scrollers.cache && _updateAll(force === true ? 2 : 0); +ScrollTrigger.clearScrollMemory = _clearScrollMemory; +ScrollTrigger.maxScroll = (element, horizontal) => _maxScroll(element, horizontal ? _horizontal : _vertical); +ScrollTrigger.getScrollFunc = (element, horizontal) => _getScrollFunc(_getTarget(element), horizontal ? _horizontal : _vertical); +ScrollTrigger.getById = id => _ids[id]; +ScrollTrigger.getAll = () => _triggers.filter(t => t.vars.id !== "ScrollSmoother"); // it's common for people to ScrollTrigger.getAll(t => t.kill()) on page routes, for example, and we don't want it to ruin smooth scrolling by killing the main ScrollSmoother one. +ScrollTrigger.isScrolling = () => !!_lastScrollTime; +ScrollTrigger.snapDirectional = _snapDirectional; +ScrollTrigger.addEventListener = (type, callback) => { + let a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); +}; +ScrollTrigger.removeEventListener = (type, callback) => { + let a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); +}; +ScrollTrigger.batch = (targets, vars) => { + let result = [], + varsCopy = {}, + interval = vars.interval || 0.016, + batchMax = vars.batchMax || 1e9, + proxyCallback = (type, callback) => { + let elements = [], + triggers = [], + delay = gsap.delayedCall(interval, () => {callback(elements, triggers); elements = []; triggers = [];}).pause(); + return self => { + elements.length || delay.restart(true); + elements.push(self.trigger); + triggers.push(self); + batchMax <= elements.length && delay.progress(1); + }; + }, + p; + for (p in vars) { + varsCopy[p] = (p.substr(0, 2) === "on" && _isFunction(vars[p]) && p !== "onRefreshInit") ? proxyCallback(p, vars[p]) : vars[p]; + } + if (_isFunction(batchMax)) { + batchMax = batchMax(); + _addListener(ScrollTrigger, "refresh", () => batchMax = vars.batchMax()); + } + _toArray(targets).forEach(target => { + let config = {}; + for (p in varsCopy) { + config[p] = varsCopy[p]; + } + config.trigger = target; + result.push(ScrollTrigger.create(config)); + }); + return result; +} + + +// to reduce file size. clamps the scroll and also returns a duration multiplier so that if the scroll gets chopped shorter, the duration gets curtailed as well (otherwise if you're very close to the top of the page, for example, and swipe up really fast, it'll suddenly slow down and take a long time to reach the top). +let _clampScrollAndGetDurationMultiplier = (scrollFunc, current, end, max) => { + current > max ? scrollFunc(max) : current < 0 && scrollFunc(0); + return end > max ? (max - current) / (end - current) : end < 0 ? current / (current - end) : 1; + }, + _allowNativePanning = (target, direction) => { + if (direction === true) { + target.style.removeProperty("touch-action"); + } else { + target.style.touchAction = direction === true ? "auto" : direction ? "pan-" + direction + (Observer.isTouch ? " pinch-zoom" : "") : "none"; // note: Firefox doesn't support it pinch-zoom properly, at least in addition to a pan-x or pan-y. + } + target === _docEl && _allowNativePanning(_body, direction); + }, + _overflow = {auto: 1, scroll: 1}, + _nestedScroll = ({event, target, axis}) => { + let node = (event.changedTouches ? event.changedTouches[0] : event).target, + cache = node._gsap || gsap.core.getCache(node), + time = _getTime(), cs; + if (!cache._isScrollT || time - cache._isScrollT > 2000) { // cache for 2 seconds to improve performance. + while (node && node !== _body && ((node.scrollHeight <= node.clientHeight && node.scrollWidth <= node.clientWidth) || !(_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]))) node = node.parentNode; + cache._isScroll = node && node !== target && !_isViewport(node) && (_overflow[(cs = _getComputedStyle(node)).overflowY] || _overflow[cs.overflowX]); + cache._isScrollT = time; + } + if (cache._isScroll || axis === "x") { + event.stopPropagation(); + event._gsapAllow = true; + } + }, + // capture events on scrollable elements INSIDE the <body> and allow those by calling stopPropagation() when we find a scrollable ancestor + _inputObserver = (target, type, inputs, nested) => Observer.create({ + target: target, + capture: true, + debounce: false, + lockAxis: true, + type: type, + onWheel: (nested = nested && _nestedScroll), + onPress: nested, + onDrag: nested, + onScroll: nested, + onEnable: () => inputs && _addListener(_doc, Observer.eventTypes[0], _captureInputs, false, true), + onDisable: () => _removeListener(_doc, Observer.eventTypes[0], _captureInputs, true) + }), + _inputExp = /(input|label|select|textarea)/i, + _inputIsFocused, + _captureInputs = e => { + let isInput = _inputExp.test(e.target.tagName); + if (isInput || _inputIsFocused) { + e._gsapAllow = true; + _inputIsFocused = isInput; + } + }, + _getScrollNormalizer = vars => { + _isObject(vars) || (vars = {}); + vars.preventDefault = vars.isNormalizer = vars.allowClicks = true; + vars.type || (vars.type = "wheel,touch"); + vars.debounce = !!vars.debounce; + vars.id = vars.id || "normalizer"; + let {normalizeScrollX, momentum, allowNestedScroll, onRelease} = vars, + self, maxY, + target = _getTarget(vars.target) || _docEl, + smoother = gsap.core.globals().ScrollSmoother, + smootherInstance = smoother && smoother.get(), + content = _fixIOSBug && ((vars.content && _getTarget(vars.content)) || (smootherInstance && vars.content !== false && !smootherInstance.smooth() && smootherInstance.content())), + scrollFuncY = _getScrollFunc(target, _vertical), + scrollFuncX = _getScrollFunc(target, _horizontal), + scale = 1, + initialScale = (Observer.isTouch && _win.visualViewport ? _win.visualViewport.scale * _win.visualViewport.width : _win.outerWidth) / _win.innerWidth, + wheelRefresh = 0, + resolveMomentumDuration = _isFunction(momentum) ? () => momentum(self) : () => momentum || 2.8, + lastRefreshID, skipTouchMove, + inputObserver = _inputObserver(target, vars.type, true, allowNestedScroll), + resumeTouchMove = () => skipTouchMove = false, + scrollClampX = _passThrough, + scrollClampY = _passThrough, + updateClamps = () => { + maxY = _maxScroll(target, _vertical); + scrollClampY = _clamp(_fixIOSBug ? 1 : 0, maxY); + normalizeScrollX && (scrollClampX = _clamp(0, _maxScroll(target, _horizontal))); + lastRefreshID = _refreshID; + }, + removeContentOffset = () => { + content._gsap.y = _round(parseFloat(content._gsap.y) + scrollFuncY.offset) + "px"; + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + parseFloat(content._gsap.y) + ", 0, 1)"; + scrollFuncY.offset = scrollFuncY.cacheID = 0; + }, + ignoreDrag = () => { + if (skipTouchMove) { + requestAnimationFrame(resumeTouchMove); + let offset = _round(self.deltaY / 2), + scroll = scrollClampY(scrollFuncY.v - offset); + if (content && scroll !== scrollFuncY.v + scrollFuncY.offset) { + scrollFuncY.offset = scroll - scrollFuncY.v; + let y = _round((parseFloat(content && content._gsap.y) || 0) - scrollFuncY.offset); + content.style.transform = "matrix3d(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, " + y + ", 0, 1)"; + content._gsap.y = y + "px"; + scrollFuncY.cacheID = _scrollers.cache; + _updateAll(); + } + return true; + } + scrollFuncY.offset && removeContentOffset(); + skipTouchMove = true; + }, + tween, startScrollX, startScrollY, onStopDelayedCall, + onResize = () => { // if the window resizes, like on an iPhone which Apple FORCES the address bar to show/hide even if we event.preventDefault(), it may be scrolling too far now that the address bar is showing, so we must dynamically adjust the momentum tween. + updateClamps(); + if (tween.isActive() && tween.vars.scrollY > maxY) { + scrollFuncY() > maxY ? tween.progress(1) && scrollFuncY(maxY) : tween.resetTo("scrollY", maxY); + } + }; + content && gsap.set(content, {y: "+=0"}); // to ensure there's a cache (element._gsap) + vars.ignoreCheck = e => (_fixIOSBug && e.type === "touchmove" && ignoreDrag(e)) || (scale > 1.05 && e.type !== "touchstart") || self.isGesturing || (e.touches && e.touches.length > 1); + vars.onPress = () => { + skipTouchMove = false; + let prevScale = scale; + scale = _round(((_win.visualViewport && _win.visualViewport.scale) || 1) / initialScale); + tween.pause(); + prevScale !== scale && _allowNativePanning(target, scale > 1.01 ? true : normalizeScrollX ? false : "x"); + startScrollX = scrollFuncX(); + startScrollY = scrollFuncY(); + updateClamps(); + lastRefreshID = _refreshID; + } + vars.onRelease = vars.onGestureStart = (self, wasDragging) => { + scrollFuncY.offset && removeContentOffset(); + if (!wasDragging) { + onStopDelayedCall.restart(true); + } else { + _scrollers.cache++; // make sure we're pulling the non-cached value + // alternate algorithm: durX = Math.min(6, Math.abs(self.velocityX / 800)), dur = Math.max(durX, Math.min(6, Math.abs(self.velocityY / 800))); dur = dur * (0.4 + (1 - _power4In(dur / 6)) * 0.6)) * (momentumSpeed || 1) + let dur = resolveMomentumDuration(), + currentScroll, endScroll; + if (normalizeScrollX) { + currentScroll = scrollFuncX(); + endScroll = currentScroll + (dur * 0.05 * -self.velocityX) / 0.227; // the constant .227 is from power4(0.05). velocity is inverted because scrolling goes in the opposite direction. + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncX, currentScroll, endScroll, _maxScroll(target, _horizontal)); + tween.vars.scrollX = scrollClampX(endScroll); + } + currentScroll = scrollFuncY(); + endScroll = currentScroll + (dur * 0.05 * -self.velocityY) / 0.227; // the constant .227 is from power4(0.05) + dur *= _clampScrollAndGetDurationMultiplier(scrollFuncY, currentScroll, endScroll, _maxScroll(target, _vertical)); + tween.vars.scrollY = scrollClampY(endScroll); + tween.invalidate().duration(dur).play(0.01); + if (_fixIOSBug && tween.vars.scrollY >= maxY || currentScroll >= maxY-1) { // iOS bug: it'll show the address bar but NOT fire the window "resize" event until the animation is done but we must protect against overshoot so we leverage an onUpdate to do so. + gsap.to({}, {onUpdate: onResize, duration: dur}); + } + } + onRelease && onRelease(self); + }; + vars.onWheel = () => { + tween._ts && tween.pause(); + if (_getTime() - wheelRefresh > 1000) { // after 1 second, refresh the clamps otherwise that'll only happen when ScrollTrigger.refresh() is called or for touch-scrolling. + lastRefreshID = 0; + wheelRefresh = _getTime(); + } + }; + vars.onChange = (self, dx, dy, xArray, yArray) => { + _refreshID !== lastRefreshID && updateClamps(); + dx && normalizeScrollX && scrollFuncX(scrollClampX(xArray[2] === dx ? startScrollX + (self.startX - self.x) : scrollFuncX() + dx - xArray[1])); // for more precision, we track pointer/touch movement from the start, otherwise it'll drift. + if (dy) { + scrollFuncY.offset && removeContentOffset(); + let isTouch = yArray[2] === dy, + y = isTouch ? startScrollY + self.startY - self.y : scrollFuncY() + dy - yArray[1], + yClamped = scrollClampY(y); + isTouch && y !== yClamped && (startScrollY += yClamped - y); + scrollFuncY(yClamped); + } + (dy || dx) && _updateAll(); + }; + vars.onEnable = () => { + _allowNativePanning(target, normalizeScrollX ? false : "x"); + ScrollTrigger.addEventListener("refresh", onResize); + _addListener(_win, "resize", onResize); + if (scrollFuncY.smooth) { + scrollFuncY.target.style.scrollBehavior = "auto"; + scrollFuncY.smooth = scrollFuncX.smooth = false; + } + inputObserver.enable(); + }; + vars.onDisable = () => { + _allowNativePanning(target, true); + _removeListener(_win, "resize", onResize); + ScrollTrigger.removeEventListener("refresh", onResize); + inputObserver.kill(); + }; + vars.lockAxis = vars.lockAxis !== false; + self = new Observer(vars); + self.iOS = _fixIOSBug; // used in the Observer getCachedScroll() function to work around an iOS bug that wreaks havoc with TouchEvent.clientY if we allow scroll to go all the way back to 0. + _fixIOSBug && !scrollFuncY() && scrollFuncY(1); // iOS bug causes event.clientY values to freak out (wildly inaccurate) if the scroll position is exactly 0. + _fixIOSBug && gsap.ticker.add(_passThrough); // prevent the ticker from sleeping + onStopDelayedCall = self._dc; + tween = gsap.to(self, {ease: "power4", paused: true, inherit: false, scrollX: normalizeScrollX ? "+=0.1" : "+=0", scrollY: "+=0.1", modifiers: {scrollY: _interruptionTracker(scrollFuncY, scrollFuncY(), () => tween.pause()) }, onUpdate: _updateAll, onComplete: onStopDelayedCall.vars.onComplete}); // we need the modifier to sense if the scroll position is altered outside of the momentum tween (like with a scrollTo tween) so we can pause() it to prevent conflicts. + return self; + }; + +ScrollTrigger.sort = func => { + if (_isFunction(func)) { + return _triggers.sort(func); + } + let scroll = _win.pageYOffset || 0; + ScrollTrigger.getAll().forEach(t => t._sortY = t.trigger ? scroll + t.trigger.getBoundingClientRect().top : t.start + _win.innerHeight); + return _triggers.sort(func || ((a, b) => (a.vars.refreshPriority || 0) * -1e6 + (a.vars.containerAnimation ? 1e6 : a._sortY) - ((b.vars.containerAnimation ? 1e6 : b._sortY) + (b.vars.refreshPriority || 0) * -1e6))); // anything with a containerAnimation should refresh last. +} +ScrollTrigger.observe = vars => new Observer(vars); +ScrollTrigger.normalizeScroll = vars => { + if (typeof(vars) === "undefined") { + return _normalizer; + } + if (vars === true && _normalizer) { + return _normalizer.enable(); + } + if (vars === false) { + _normalizer && _normalizer.kill(); + _normalizer = vars; + return; + } + let normalizer = vars instanceof Observer ? vars : _getScrollNormalizer(vars); + _normalizer && _normalizer.target === normalizer.target && _normalizer.kill(); + _isViewport(normalizer.target) && (_normalizer = normalizer); + return normalizer; +}; + + +ScrollTrigger.core = { // smaller file size way to leverage in ScrollSmoother and Observer + _getVelocityProp, + _inputObserver, + _scrollers, + _proxies, + bridge: { + // when normalizeScroll sets the scroll position (ss = setScroll) + ss: () => { + _lastScrollTime || _dispatch("scrollStart"); + _lastScrollTime = _getTime(); + }, + // a way to get the _refreshing value in Observer + ref: () => _refreshing + } +}; + +_getGSAP() && gsap.registerPlugin(ScrollTrigger); + +export { ScrollTrigger as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/TextPlugin.js b/node_modules/gsap/src/TextPlugin.js new file mode 100644 index 0000000000000000000000000000000000000000..ef62935b1fe56794c34d16c1bc769eecc451e024 --- /dev/null +++ b/node_modules/gsap/src/TextPlugin.js @@ -0,0 +1,121 @@ +/*! + * TextPlugin 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +import { emojiSafeSplit, getText, splitInnerHTML } from "./utils/strings.js"; + +let gsap, _tempDiv, + _getGSAP = () => gsap || (typeof(window) !== "undefined" && (gsap = window.gsap) && gsap.registerPlugin && gsap); + + +export const TextPlugin = { + version:"3.12.7", + name:"text", + init(target, value, tween) { + typeof(value) !== "object" && (value = {value:value}); + let i = target.nodeName.toUpperCase(), + data = this, + { newClass, oldClass, preserveSpaces, rtl } = value, + delimiter = data.delimiter = value.delimiter || "", + fillChar = data.fillChar = value.fillChar || (value.padSpace ? " " : ""), + short, text, original, j, condensedText, condensedOriginal, aggregate, s; + data.svg = (target.getBBox && (i === "TEXT" || i === "TSPAN")); + if (!("innerHTML" in target) && !data.svg) { + return false; + } + data.target = target; + if (!("value" in value)) { + data.text = data.original = [""]; + return; + } + original = splitInnerHTML(target, delimiter, false, preserveSpaces, data.svg); + _tempDiv || (_tempDiv = document.createElement("div")); + _tempDiv.innerHTML = value.value; + text = splitInnerHTML(_tempDiv, delimiter, false, preserveSpaces, data.svg); + data.from = tween._from; + if ((data.from || rtl) && !(rtl && data.from)) { // right-to-left or "from()" tweens should invert things (but if it's BOTH .from() and rtl, inverting twice equals not inverting at all :) + i = original; + original = text; + text = i; + } + data.hasClass = !!(newClass || oldClass); + data.newClass = rtl ? oldClass : newClass; + data.oldClass = rtl ? newClass : oldClass; + i = original.length - text.length; + short = i < 0 ? original : text; + if (i < 0) { + i = -i; + } + while (--i > -1) { + short.push(fillChar); + } + if (value.type === "diff") { + j = 0; + condensedText = []; + condensedOriginal = []; + aggregate = ""; + for (i = 0; i < text.length; i++) { + s = text[i]; + if (s === original[i]) { + aggregate += s; + } else { + condensedText[j] = aggregate + s; + condensedOriginal[j++] = aggregate + original[i]; + aggregate = ""; + } + } + text = condensedText; + original = condensedOriginal; + if (aggregate) { + text.push(aggregate); + original.push(aggregate); + } + } + value.speed && tween.duration(Math.min(0.05 / value.speed * short.length, value.maxDuration || 9999)); + data.rtl = rtl; + data.original = original; + data.text = text; + data._props.push("text"); + }, + render(ratio, data) { + if (ratio > 1) { + ratio = 1; + } else if (ratio < 0) { + ratio = 0; + } + if (data.from) { + ratio = 1 - ratio; + } + let { text, hasClass, newClass, oldClass, delimiter, target, fillChar, original, rtl } = data, + l = text.length, + i = ((rtl ? 1 - ratio : ratio) * l + 0.5) | 0, + applyNew, applyOld, str; + if (hasClass && ratio) { + applyNew = (newClass && i); + applyOld = (oldClass && i !== l); + str = (applyNew ? "<span class='" + newClass + "'>" : "") + text.slice(0, i).join(delimiter) + (applyNew ? "</span>" : "") + (applyOld ? "<span class='" + oldClass + "'>" : "") + delimiter + original.slice(i).join(delimiter) + (applyOld ? "</span>" : ""); + } else { + str = text.slice(0, i).join(delimiter) + delimiter + original.slice(i).join(delimiter); + } + if (data.svg) { //SVG text elements don't have an "innerHTML" in Microsoft browsers. + target.textContent = str; + } else { + target.innerHTML = (fillChar === " " && ~str.indexOf(" ")) ? str.split(" ").join(" ") : str; + } + } +}; + +TextPlugin.splitInnerHTML = splitInnerHTML; +TextPlugin.emojiSafeSplit = emojiSafeSplit; +TextPlugin.getText = getText; + +_getGSAP() && gsap.registerPlugin(TextPlugin); + +export { TextPlugin as default }; \ No newline at end of file diff --git a/node_modules/gsap/src/all.js b/node_modules/gsap/src/all.js new file mode 100644 index 0000000000000000000000000000000000000000..fd745081a96e386fb6415a27d5fdb54565ec8880 --- /dev/null +++ b/node_modules/gsap/src/all.js @@ -0,0 +1,34 @@ +import gsap from "./gsap-core.js"; +import CSSPlugin from "./CSSPlugin.js"; +const gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, // to protect from tree shaking + TweenMaxWithCSS = gsapWithCSS.core.Tween; + +export { gsapWithCSS as gsap, gsapWithCSS as default, TweenMaxWithCSS as TweenMax, CSSPlugin }; + +export { TweenLite, TimelineMax, TimelineLite, Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ, wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, mapRange, pipe, unitize, interpolate, shuffle, selector } from "./gsap-core.js"; +export * from "./CustomEase.js"; +export * from "./Draggable.js"; +export * from "./CSSRulePlugin.js"; +export * from "./EaselPlugin.js"; +export * from "./EasePack.js"; +export * from "./Flip.js"; +export * from "./MotionPathPlugin.js"; +export * from "./Observer.js"; +export * from "./PixiPlugin.js"; +export * from "./ScrollToPlugin.js"; +export * from "./ScrollTrigger.js"; +export * from "./TextPlugin.js"; + +//BONUS EXPORTS +// export * from "./DrawSVGPlugin.js"; +// export * from "./Physics2DPlugin.js"; +// export * from "./PhysicsPropsPlugin.js"; +// export * from "./ScrambleTextPlugin.js"; +// export * from "./CustomBounce.js"; +// export * from "./CustomWiggle.js"; +// export * from "./GSDevTools.js"; +// export * from "./InertiaPlugin.js"; +// export * from "./MorphSVGPlugin.js"; +// export * from "./MotionPathHelper.js"; +// export * from "./ScrollSmoother.js"; +// export * from "./SplitText.js"; \ No newline at end of file diff --git a/node_modules/gsap/src/gsap-core.js b/node_modules/gsap/src/gsap-core.js new file mode 100644 index 0000000000000000000000000000000000000000..a80b3f0cb88cf62fe2c82663b0d20e2fb240dcb5 --- /dev/null +++ b/node_modules/gsap/src/gsap-core.js @@ -0,0 +1,3268 @@ +/*! + * GSAP 3.12.7 + * https://gsap.com + * + * @license Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let _config = { + autoSleep: 120, + force3D: "auto", + nullTargetWarn: 1, + units: {lineHeight:""} + }, + _defaults = { + duration: .5, + overwrite: false, + delay: 0 + }, + _suppressOverwrites, + _reverting, _context, + _bigNum = 1e8, + _tinyNum = 1 / _bigNum, + _2PI = Math.PI * 2, + _HALF_PI = _2PI / 4, + _gsID = 0, + _sqrt = Math.sqrt, + _cos = Math.cos, + _sin = Math.sin, + _isString = value => typeof(value) === "string", + _isFunction = value => typeof(value) === "function", + _isNumber = value => typeof(value) === "number", + _isUndefined = value => typeof(value) === "undefined", + _isObject = value => typeof(value) === "object", + _isNotFalse = value => value !== false, + _windowExists = () => typeof(window) !== "undefined", + _isFuncOrString = value => _isFunction(value) || _isString(value), + _isTypedArray = (typeof ArrayBuffer === "function" && ArrayBuffer.isView) || function() {}, // note: IE10 has ArrayBuffer, but NOT ArrayBuffer.isView(). + _isArray = Array.isArray, + _strictNumExp = /(?:-?\.?\d|\.)+/gi, //only numbers (including negatives and decimals) but NOT relative values. + _numExp = /[-+=.]*\d+[.e\-+]*\d*[e\-+]*\d*/g, //finds any numbers, including ones that start with += or -=, negative numbers, and ones in scientific notation like 1e-8. + _numWithUnitExp = /[-+=.]*\d+[.e-]*\d*[a-z%]*/g, + _complexStringNumExp = /[-+=.]*\d+\.?\d*(?:e-|e\+)?\d*/gi, //duplicate so that while we're looping through matches from exec(), it doesn't contaminate the lastIndex of _numExp which we use to search for colors too. + _relExp = /[+-]=-?[.\d]+/, + _delimitedValueExp = /[^,'"\[\]\s]+/gi, // previously /[#\-+.]*\b[a-z\d\-=+%.]+/gi but didn't catch special characters. + _unitExp = /^[+\-=e\s\d]*\d+[.\d]*([a-z]*|%)\s*$/i, + _globalTimeline, _win, _coreInitted, _doc, + _globals = {}, + _installScope = {}, + _coreReady, + _install = scope => (_installScope = _merge(scope, _globals)) && gsap, + _missingPlugin = (property, value) => console.warn("Invalid property", property, "set to", value, "Missing plugin? gsap.registerPlugin()"), + _warn = (message, suppress) => !suppress && console.warn(message), + _addGlobal = (name, obj) => (name && (_globals[name] = obj) && (_installScope && (_installScope[name] = obj))) || _globals, + _emptyFunc = () => 0, + _startAtRevertConfig = {suppressEvents: true, isStart: true, kill: false}, + _revertConfigNoKill = {suppressEvents: true, kill: false}, + _revertConfig = {suppressEvents: true}, + _reservedProps = {}, + _lazyTweens = [], + _lazyLookup = {}, + _lastRenderedFrame, + _plugins = {}, + _effects = {}, + _nextGCFrame = 30, + _harnessPlugins = [], + _callbackNames = "", + _harness = targets => { + let target = targets[0], + harnessPlugin, i; + _isObject(target) || _isFunction(target) || (targets = [targets]); + if (!(harnessPlugin = (target._gsap || {}).harness)) { // find the first target with a harness. We assume targets passed into an animation will be of similar type, meaning the same kind of harness can be used for them all (performance optimization) + i = _harnessPlugins.length; + while (i-- && !_harnessPlugins[i].targetTest(target)) { } + harnessPlugin = _harnessPlugins[i]; + } + i = targets.length; + while (i--) { + (targets[i] && (targets[i]._gsap || (targets[i]._gsap = new GSCache(targets[i], harnessPlugin)))) || targets.splice(i, 1); + } + return targets; + }, + _getCache = target => target._gsap || _harness(toArray(target))[0]._gsap, + _getProperty = (target, property, v) => (v = target[property]) && _isFunction(v) ? target[property]() : (_isUndefined(v) && target.getAttribute && target.getAttribute(property)) || v, + _forEachName = (names, func) => ((names = names.split(",")).forEach(func)) || names, //split a comma-delimited list of names into an array, then run a forEach() function and return the split array (this is just a way to consolidate/shorten some code). + _round = value => Math.round(value * 100000) / 100000 || 0, + _roundPrecise = value => Math.round(value * 10000000) / 10000000 || 0, // increased precision mostly for timing values. + _parseRelative = (start, value) => { + let operator = value.charAt(0), + end = parseFloat(value.substr(2)); + start = parseFloat(start); + return operator === "+" ? start + end : operator === "-" ? start - end : operator === "*" ? start * end : start / end; + }, + _arrayContainsAny = (toSearch, toFind) => { //searches one array to find matches for any of the items in the toFind array. As soon as one is found, it returns true. It does NOT return all the matches; it's simply a boolean search. + let l = toFind.length, + i = 0; + for (; toSearch.indexOf(toFind[i]) < 0 && ++i < l;) { } + return (i < l); + }, + _lazyRender = () => { + let l = _lazyTweens.length, + a = _lazyTweens.slice(0), + i, tween; + _lazyLookup = {}; + _lazyTweens.length = 0; + for (i = 0; i < l; i++) { + tween = a[i]; + tween && tween._lazy && (tween.render(tween._lazy[0], tween._lazy[1], true)._lazy = 0); + } + }, + _lazySafeRender = (animation, time, suppressEvents, force) => { + _lazyTweens.length && !_reverting && _lazyRender(); + animation.render(time, suppressEvents, force || (_reverting && time < 0 && (animation._initted || animation._startAt))); + _lazyTweens.length && !_reverting && _lazyRender(); //in case rendering caused any tweens to lazy-init, we should render them because typically when someone calls seek() or time() or progress(), they expect an immediate render. + }, + _numericIfPossible = value => { + let n = parseFloat(value); + return (n || n === 0) && (value + "").match(_delimitedValueExp).length < 2 ? n : _isString(value) ? value.trim() : value; + }, + _passThrough = p => p, + _setDefaults = (obj, defaults) => { + for (let p in defaults) { + (p in obj) || (obj[p] = defaults[p]); + } + return obj; + }, + _setKeyframeDefaults = excludeDuration => (obj, defaults) => { + for (let p in defaults) { + (p in obj) || (p === "duration" && excludeDuration) || p === "ease" || (obj[p] = defaults[p]); + } + }, + _merge = (base, toMerge) => { + for (let p in toMerge) { + base[p] = toMerge[p]; + } + return base; + }, + _mergeDeep = (base, toMerge) => { + for (let p in toMerge) { + p !== "__proto__" && p !== "constructor" && p !== "prototype" && (base[p] = _isObject(toMerge[p]) ? _mergeDeep(base[p] || (base[p] = {}), toMerge[p]) : toMerge[p]); + } + return base; + }, + _copyExcluding = (obj, excluding) => { + let copy = {}, + p; + for (p in obj) { + (p in excluding) || (copy[p] = obj[p]); + } + return copy; + }, + _inheritDefaults = vars => { + let parent = vars.parent || _globalTimeline, + func = vars.keyframes ? _setKeyframeDefaults(_isArray(vars.keyframes)) : _setDefaults; + if (_isNotFalse(vars.inherit)) { + while (parent) { + func(vars, parent.vars.defaults); + parent = parent.parent || parent._dp; + } + } + return vars; + }, + _arraysMatch = (a1, a2) => { + let i = a1.length, + match = i === a2.length; + while (match && i-- && a1[i] === a2[i]) { } + return i < 0; + }, + _addLinkedListItem = (parent, child, firstProp = "_first", lastProp = "_last", sortBy) => { + let prev = parent[lastProp], + t; + if (sortBy) { + t = child[sortBy]; + while (prev && prev[sortBy] > t) { + prev = prev._prev; + } + } + if (prev) { + child._next = prev._next; + prev._next = child; + } else { + child._next = parent[firstProp]; + parent[firstProp] = child; + } + if (child._next) { + child._next._prev = child; + } else { + parent[lastProp] = child; + } + child._prev = prev; + child.parent = child._dp = parent; + return child; + }, + _removeLinkedListItem = (parent, child, firstProp = "_first", lastProp = "_last") => { + let prev = child._prev, + next = child._next; + if (prev) { + prev._next = next; + } else if (parent[firstProp] === child) { + parent[firstProp] = next; + } + if (next) { + next._prev = prev; + } else if (parent[lastProp] === child) { + parent[lastProp] = prev; + } + child._next = child._prev = child.parent = null; // don't delete the _dp just so we can revert if necessary. But parent should be null to indicate the item isn't in a linked list. + }, + _removeFromParent = (child, onlyIfParentHasAutoRemove) => { + child.parent && (!onlyIfParentHasAutoRemove || child.parent.autoRemoveChildren) && child.parent.remove && child.parent.remove(child); + child._act = 0; + }, + _uncache = (animation, child) => { + if (animation && (!child || child._end > animation._dur || child._start < 0)) { // performance optimization: if a child animation is passed in we should only uncache if that child EXTENDS the animation (its end time is beyond the end) + let a = animation; + while (a) { + a._dirty = 1; + a = a.parent; + } + } + return animation; + }, + _recacheAncestors = animation => { + let parent = animation.parent; + while (parent && parent.parent) { //sometimes we must force a re-sort of all children and update the duration/totalDuration of all ancestor timelines immediately in case, for example, in the middle of a render loop, one tween alters another tween's timeScale which shoves its startTime before 0, forcing the parent timeline to shift around and shiftChildren() which could affect that next tween's render (startTime). Doesn't matter for the root timeline though. + parent._dirty = 1; + parent.totalDuration(); + parent = parent.parent; + } + return animation; + }, + _rewindStartAt = (tween, totalTime, suppressEvents, force) => tween._startAt && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : (tween.vars.immediateRender && !tween.vars.autoRevert) || tween._startAt.render(totalTime, true, force)), + _hasNoPausedAncestors = animation => !animation || (animation._ts && _hasNoPausedAncestors(animation.parent)), + _elapsedCycleDuration = animation => animation._repeat ? _animationCycle(animation._tTime, (animation = animation.duration() + animation._rDelay)) * animation : 0, + // feed in the totalTime and cycleDuration and it'll return the cycle (iteration minus 1) and if the playhead is exactly at the very END, it will NOT bump up to the next cycle. + _animationCycle = (tTime, cycleDuration) => { + let whole = Math.floor(tTime = _roundPrecise(tTime / cycleDuration)); + return tTime && (whole === tTime) ? whole - 1 : whole; + }, + _parentToChildTotalTime = (parentTime, child) => (parentTime - child._start) * child._ts + (child._ts >= 0 ? 0 : (child._dirty ? child.totalDuration() : child._tDur)), + _setEnd = animation => (animation._end = _roundPrecise(animation._start + ((animation._tDur / Math.abs(animation._ts || animation._rts || _tinyNum)) || 0))), + _alignPlayhead = (animation, totalTime) => { // adjusts the animation's _start and _end according to the provided totalTime (only if the parent's smoothChildTiming is true and the animation isn't paused). It doesn't do any rendering or forcing things back into parent timelines, etc. - that's what totalTime() is for. + let parent = animation._dp; + if (parent && parent.smoothChildTiming && animation._ts) { + animation._start = _roundPrecise(parent._time - (animation._ts > 0 ? totalTime / animation._ts : ((animation._dirty ? animation.totalDuration() : animation._tDur) - totalTime) / -animation._ts)); + _setEnd(animation); + parent._dirty || _uncache(parent, animation); //for performance improvement. If the parent's cache is already dirty, it already took care of marking the ancestors as dirty too, so skip the function call here. + } + return animation; + }, + /* + _totalTimeToTime = (clampedTotalTime, duration, repeat, repeatDelay, yoyo) => { + let cycleDuration = duration + repeatDelay, + time = _round(clampedTotalTime % cycleDuration); + if (time > duration) { + time = duration; + } + return (yoyo && (~~(clampedTotalTime / cycleDuration) & 1)) ? duration - time : time; + }, + */ + _postAddChecks = (timeline, child) => { + let t; + if (child._time || (!child._dur && child._initted) || (child._start < timeline._time && (child._dur || !child.add))) { // in case, for example, the _start is moved on a tween that has already rendered, or if it's being inserted into a timeline BEFORE where the playhead is currently. Imagine it's at its end state, then the startTime is moved WAY later (after the end of this timeline), it should render at its beginning. Special case: if it's a timeline (has .add() method) and no duration, we can skip rendering because the user may be populating it AFTER adding it to a parent timeline (unconventional, but possible, and we wouldn't want it to get removed if the parent's autoRemoveChildren is true). + t = _parentToChildTotalTime(timeline.rawTime(), child); + if (!child._dur || _clamp(0, child.totalDuration(), t) - child._tTime > _tinyNum) { + child.render(t, true); + } + } + //if the timeline has already ended but the inserted tween/timeline extends the duration, we should enable this timeline again so that it renders properly. We should also align the playhead with the parent timeline's when appropriate. + if (_uncache(timeline, child)._dp && timeline._initted && timeline._time >= timeline._dur && timeline._ts) { + //in case any of the ancestors had completed but should now be enabled... + if (timeline._dur < timeline.duration()) { + t = timeline; + while (t._dp) { + (t.rawTime() >= 0) && t.totalTime(t._tTime); //moves the timeline (shifts its startTime) if necessary, and also enables it. If it's currently zero, though, it may not be scheduled to render until later so there's no need to force it to align with the current playhead position. Only move to catch up with the playhead. + t = t._dp; + } + } + timeline._zTime = -_tinyNum; // helps ensure that the next render() will be forced (crossingStart = true in render()), even if the duration hasn't changed (we're adding a child which would need to get rendered). Definitely an edge case. Note: we MUST do this AFTER the loop above where the totalTime() might trigger a render() because this _addToTimeline() method gets called from the Animation constructor, BEFORE tweens even record their targets, etc. so we wouldn't want things to get triggered in the wrong order. + } + }, + _addToTimeline = (timeline, child, position, skipChecks) => { + child.parent && _removeFromParent(child); + child._start = _roundPrecise((_isNumber(position) ? position : position || timeline !== _globalTimeline ? _parsePosition(timeline, position, child) : timeline._time) + child._delay); + child._end = _roundPrecise(child._start + ((child.totalDuration() / Math.abs(child.timeScale())) || 0)); + _addLinkedListItem(timeline, child, "_first", "_last", timeline._sort ? "_start" : 0); + _isFromOrFromStart(child) || (timeline._recent = child); + skipChecks || _postAddChecks(timeline, child); + timeline._ts < 0 && _alignPlayhead(timeline, timeline._tTime); // if the timeline is reversed and the new child makes it longer, we may need to adjust the parent's _start (push it back) + return timeline; + }, + _scrollTrigger = (animation, trigger) => (_globals.ScrollTrigger || _missingPlugin("scrollTrigger", trigger)) && _globals.ScrollTrigger.create(trigger, animation), + _attemptInitTween = (tween, time, force, suppressEvents, tTime) => { + _initTween(tween, time, tTime); + if (!tween._initted) { + return 1; + } + if (!force && tween._pt && !_reverting && ((tween._dur && tween.vars.lazy !== false) || (!tween._dur && tween.vars.lazy)) && _lastRenderedFrame !== _ticker.frame) { + _lazyTweens.push(tween); + tween._lazy = [tTime, suppressEvents]; + return 1; + } + }, + _parentPlayheadIsBeforeStart = ({parent}) => parent && parent._ts && parent._initted && !parent._lock && (parent.rawTime() < 0 || _parentPlayheadIsBeforeStart(parent)), // check parent's _lock because when a timeline repeats/yoyos and does its artificial wrapping, we shouldn't force the ratio back to 0 + _isFromOrFromStart = ({data}) => data === "isFromStart" || data === "isStart", + _renderZeroDurationTween = (tween, totalTime, suppressEvents, force) => { + let prevRatio = tween.ratio, + ratio = totalTime < 0 || (!totalTime && ((!tween._start && _parentPlayheadIsBeforeStart(tween) && !(!tween._initted && _isFromOrFromStart(tween))) || ((tween._ts < 0 || tween._dp._ts < 0) && !_isFromOrFromStart(tween)))) ? 0 : 1, // if the tween or its parent is reversed and the totalTime is 0, we should go to a ratio of 0. Edge case: if a from() or fromTo() stagger tween is placed later in a timeline, the "startAt" zero-duration tween could initially render at a time when the parent timeline's playhead is technically BEFORE where this tween is, so make sure that any "from" and "fromTo" startAt tweens are rendered the first time at a ratio of 1. + repeatDelay = tween._rDelay, + tTime = 0, + pt, iteration, prevIteration; + if (repeatDelay && tween._repeat) { // in case there's a zero-duration tween that has a repeat with a repeatDelay + tTime = _clamp(0, tween._tDur, totalTime); + iteration = _animationCycle(tTime, repeatDelay); + tween._yoyo && (iteration & 1) && (ratio = 1 - ratio); + if (iteration !== _animationCycle(tween._tTime, repeatDelay)) { // if iteration changed + prevRatio = 1 - ratio; + tween.vars.repeatRefresh && tween._initted && tween.invalidate(); + } + } + if (ratio !== prevRatio || _reverting || force || tween._zTime === _tinyNum || (!totalTime && tween._zTime)) { + if (!tween._initted && _attemptInitTween(tween, totalTime, force, suppressEvents, tTime)) { // if we render the very beginning (time == 0) of a fromTo(), we must force the render (normal tweens wouldn't need to render at a time of 0 when the prevTime was also 0). This is also mandatory to make sure overwriting kicks in immediately. + return; + } + prevIteration = tween._zTime; + tween._zTime = totalTime || (suppressEvents ? _tinyNum : 0); // when the playhead arrives at EXACTLY time 0 (right on top) of a zero-duration tween, we need to discern if events are suppressed so that when the playhead moves again (next time), it'll trigger the callback. If events are NOT suppressed, obviously the callback would be triggered in this render. Basically, the callback should fire either when the playhead ARRIVES or LEAVES this exact spot, not both. Imagine doing a timeline.seek(0) and there's a callback that sits at 0. Since events are suppressed on that seek() by default, nothing will fire, but when the playhead moves off of that position, the callback should fire. This behavior is what people intuitively expect. + suppressEvents || (suppressEvents = totalTime && !prevIteration); // if it was rendered previously at exactly 0 (_zTime) and now the playhead is moving away, DON'T fire callbacks otherwise they'll seem like duplicates. + tween.ratio = ratio; + tween._from && (ratio = 1 - ratio); + tween._time = 0; + tween._tTime = tTime; + pt = tween._pt; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + totalTime < 0 && _rewindStartAt(tween, totalTime, suppressEvents, true); + tween._onUpdate && !suppressEvents && _callback(tween, "onUpdate"); + tTime && tween._repeat && !suppressEvents && tween.parent && _callback(tween, "onRepeat"); + if ((totalTime >= tween._tDur || totalTime < 0) && tween.ratio === ratio) { + ratio && _removeFromParent(tween, 1); + if (!suppressEvents && !_reverting) { + _callback(tween, (ratio ? "onComplete" : "onReverseComplete"), true); + tween._prom && tween._prom(); + } + } + } else if (!tween._zTime) { + tween._zTime = totalTime; + } + }, + _findNextPauseTween = (animation, prevTime, time) => { + let child; + if (time > prevTime) { + child = animation._first; + while (child && child._start <= time) { + if (child.data === "isPause" && child._start > prevTime) { + return child; + } + child = child._next; + } + } else { + child = animation._last; + while (child && child._start >= time) { + if (child.data === "isPause" && child._start < prevTime) { + return child; + } + child = child._prev; + } + } + }, + _setDuration = (animation, duration, skipUncache, leavePlayhead) => { + let repeat = animation._repeat, + dur = _roundPrecise(duration) || 0, + totalProgress = animation._tTime / animation._tDur; + totalProgress && !leavePlayhead && (animation._time *= dur / animation._dur); + animation._dur = dur; + animation._tDur = !repeat ? dur : repeat < 0 ? 1e10 : _roundPrecise(dur * (repeat + 1) + (animation._rDelay * repeat)); + totalProgress > 0 && !leavePlayhead && _alignPlayhead(animation, (animation._tTime = animation._tDur * totalProgress)); + animation.parent && _setEnd(animation); + skipUncache || _uncache(animation.parent, animation); + return animation; + }, + _onUpdateTotalDuration = animation => (animation instanceof Timeline) ? _uncache(animation) : _setDuration(animation, animation._dur), + _zeroPosition = {_start:0, endTime:_emptyFunc, totalDuration:_emptyFunc}, + _parsePosition = (animation, position, percentAnimation) => { + let labels = animation.labels, + recent = animation._recent || _zeroPosition, + clippedDuration = animation.duration() >= _bigNum ? recent.endTime(false) : animation._dur, //in case there's a child that infinitely repeats, users almost never intend for the insertion point of a new child to be based on a SUPER long value like that so we clip it and assume the most recently-added child's endTime should be used instead. + i, offset, isPercent; + if (_isString(position) && (isNaN(position) || (position in labels))) { //if the string is a number like "1", check to see if there's a label with that name, otherwise interpret it as a number (absolute value). + offset = position.charAt(0); + isPercent = position.substr(-1) === "%"; + i = position.indexOf("="); + if (offset === "<" || offset === ">") { + i >= 0 && (position = position.replace(/=/, "")); + return (offset === "<" ? recent._start : recent.endTime(recent._repeat >= 0)) + (parseFloat(position.substr(1)) || 0) * (isPercent ? (i < 0 ? recent : percentAnimation).totalDuration() / 100 : 1); + } + if (i < 0) { + (position in labels) || (labels[position] = clippedDuration); + return labels[position]; + } + offset = parseFloat(position.charAt(i-1) + position.substr(i+1)); + if (isPercent && percentAnimation) { + offset = offset / 100 * (_isArray(percentAnimation) ? percentAnimation[0] : percentAnimation).totalDuration(); + } + return (i > 1) ? _parsePosition(animation, position.substr(0, i-1), percentAnimation) + offset : clippedDuration + offset; + } + return (position == null) ? clippedDuration : +position; + }, + _createTweenType = (type, params, timeline) => { + let isLegacy = _isNumber(params[1]), + varsIndex = (isLegacy ? 2 : 1) + (type < 2 ? 0 : 1), + vars = params[varsIndex], + irVars, parent; + isLegacy && (vars.duration = params[1]); + vars.parent = timeline; + if (type) { + irVars = vars; + parent = timeline; + while (parent && !("immediateRender" in irVars)) { // inheritance hasn't happened yet, but someone may have set a default in an ancestor timeline. We could do vars.immediateRender = _isNotFalse(_inheritDefaults(vars).immediateRender) but that'd exact a slight performance penalty because _inheritDefaults() also runs in the Tween constructor. We're paying a small kb price here to gain speed. + irVars = parent.vars.defaults || {}; + parent = _isNotFalse(parent.vars.inherit) && parent.parent; + } + vars.immediateRender = _isNotFalse(irVars.immediateRender); + type < 2 ? (vars.runBackwards = 1) : (vars.startAt = params[varsIndex - 1]); // "from" vars + } + return new Tween(params[0], vars, params[varsIndex + 1]); + }, + _conditionalReturn = (value, func) => value || value === 0 ? func(value) : func, + _clamp = (min, max, value) => value < min ? min : value > max ? max : value, + getUnit = (value, v) => !_isString(value) || !(v = _unitExp.exec(value)) ? "" : v[1], // note: protect against padded numbers as strings, like "100.100". That shouldn't return "00" as the unit. If it's numeric, return no unit. + clamp = (min, max, value) => _conditionalReturn(value, v => _clamp(min, max, v)), + _slice = [].slice, + _isArrayLike = (value, nonEmpty) => value && (_isObject(value) && "length" in value && ((!nonEmpty && !value.length) || ((value.length - 1) in value && _isObject(value[0]))) && !value.nodeType && value !== _win), + _flatten = (ar, leaveStrings, accumulator = []) => ar.forEach(value => (_isString(value) && !leaveStrings) || _isArrayLike(value, 1) ? accumulator.push(...toArray(value)) : accumulator.push(value)) || accumulator, + //takes any value and returns an array. If it's a string (and leaveStrings isn't true), it'll use document.querySelectorAll() and convert that to an array. It'll also accept iterables like jQuery objects. + toArray = (value, scope, leaveStrings) => _context && !scope && _context.selector ? _context.selector(value) : _isString(value) && !leaveStrings && (_coreInitted || !_wake()) ? _slice.call((scope || _doc).querySelectorAll(value), 0) : _isArray(value) ? _flatten(value, leaveStrings) : _isArrayLike(value) ? _slice.call(value, 0) : value ? [value] : [], + selector = value => { + value = toArray(value)[0] || _warn("Invalid scope") || {}; + return v => { + let el = value.current || value.nativeElement || value; + return toArray(v, el.querySelectorAll ? el : el === value ? _warn("Invalid scope") || _doc.createElement("div") : value); + }; + }, + shuffle = a => a.sort(() => .5 - Math.random()), // alternative that's a bit faster and more reliably diverse but bigger: for (let j, v, i = a.length; i; j = (Math.random() * i) | 0, v = a[--i], a[i] = a[j], a[j] = v); return a; + //for distributing values across an array. Can accept a number, a function or (most commonly) a function which can contain the following properties: {base, amount, from, ease, grid, axis, length, each}. Returns a function that expects the following parameters: index, target, array. Recognizes the following + distribute = v => { + if (_isFunction(v)) { + return v; + } + let vars = _isObject(v) ? v : {each:v}, //n:1 is just to indicate v was a number; we leverage that later to set v according to the length we get. If a number is passed in, we treat it like the old stagger value where 0.1, for example, would mean that things would be distributed with 0.1 between each element in the array rather than a total "amount" that's chunked out among them all. + ease = _parseEase(vars.ease), + from = vars.from || 0, + base = parseFloat(vars.base) || 0, + cache = {}, + isDecimal = (from > 0 && from < 1), + ratios = isNaN(from) || isDecimal, + axis = vars.axis, + ratioX = from, + ratioY = from; + if (_isString(from)) { + ratioX = ratioY = {center:.5, edges:.5, end:1}[from] || 0; + } else if (!isDecimal && ratios) { + ratioX = from[0]; + ratioY = from[1]; + } + return (i, target, a) => { + let l = (a || vars).length, + distances = cache[l], + originX, originY, x, y, d, j, max, min, wrapAt; + if (!distances) { + wrapAt = (vars.grid === "auto") ? 0 : (vars.grid || [1, _bigNum])[1]; + if (!wrapAt) { + max = -_bigNum; + while (max < (max = a[wrapAt++].getBoundingClientRect().left) && wrapAt < l) { } + wrapAt < l && wrapAt--; + } + distances = cache[l] = []; + originX = ratios ? (Math.min(wrapAt, l) * ratioX) - .5 : from % wrapAt; + originY = wrapAt === _bigNum ? 0 : ratios ? l * ratioY / wrapAt - .5 : (from / wrapAt) | 0; + max = 0; + min = _bigNum; + for (j = 0; j < l; j++) { + x = (j % wrapAt) - originX; + y = originY - ((j / wrapAt) | 0); + distances[j] = d = !axis ? _sqrt(x * x + y * y) : Math.abs((axis === "y") ? y : x); + (d > max) && (max = d); + (d < min) && (min = d); + } + (from === "random") && shuffle(distances); + distances.max = max - min; + distances.min = min; + distances.v = l = (parseFloat(vars.amount) || (parseFloat(vars.each) * (wrapAt > l ? l - 1 : !axis ? Math.max(wrapAt, l / wrapAt) : axis === "y" ? l / wrapAt : wrapAt)) || 0) * (from === "edges" ? -1 : 1); + distances.b = (l < 0) ? base - l : base; + distances.u = getUnit(vars.amount || vars.each) || 0; //unit + ease = (ease && l < 0) ? _invertEase(ease) : ease; + } + l = ((distances[i] - distances.min) / distances.max) || 0; + return _roundPrecise(distances.b + (ease ? ease(l) : l) * distances.v) + distances.u; //round in order to work around floating point errors + }; + }, + _roundModifier = v => { //pass in 0.1 get a function that'll round to the nearest tenth, or 5 to round to the closest 5, or 0.001 to the closest 1000th, etc. + let p = Math.pow(10, ((v + "").split(".")[1] || "").length); //to avoid floating point math errors (like 24 * 0.1 == 2.4000000000000004), we chop off at a specific number of decimal places (much faster than toFixed()) + return raw => { + let n = _roundPrecise(Math.round(parseFloat(raw) / v) * v * p); + return (n - n % 1) / p + (_isNumber(raw) ? 0 : getUnit(raw)); // n - n % 1 replaces Math.floor() in order to handle negative values properly. For example, Math.floor(-150.00000000000003) is 151! + }; + }, + snap = (snapTo, value) => { + let isArray = _isArray(snapTo), + radius, is2D; + if (!isArray && _isObject(snapTo)) { + radius = isArray = snapTo.radius || _bigNum; + if (snapTo.values) { + snapTo = toArray(snapTo.values); + if ((is2D = !_isNumber(snapTo[0]))) { + radius *= radius; //performance optimization so we don't have to Math.sqrt() in the loop. + } + } else { + snapTo = _roundModifier(snapTo.increment); + } + } + return _conditionalReturn(value, !isArray ? _roundModifier(snapTo) : _isFunction(snapTo) ? raw => {is2D = snapTo(raw); return Math.abs(is2D - raw) <= radius ? is2D : raw; } : raw => { + let x = parseFloat(is2D ? raw.x : raw), + y = parseFloat(is2D ? raw.y : 0), + min = _bigNum, + closest = 0, + i = snapTo.length, + dx, dy; + while (i--) { + if (is2D) { + dx = snapTo[i].x - x; + dy = snapTo[i].y - y; + dx = dx * dx + dy * dy; + } else { + dx = Math.abs(snapTo[i] - x); + } + if (dx < min) { + min = dx; + closest = i; + } + } + closest = (!radius || min <= radius) ? snapTo[closest] : raw; + return (is2D || closest === raw || _isNumber(raw)) ? closest : closest + getUnit(raw); + }); + }, + random = (min, max, roundingIncrement, returnFunction) => _conditionalReturn(_isArray(min) ? !max : roundingIncrement === true ? !!(roundingIncrement = 0) : !returnFunction, () => _isArray(min) ? min[~~(Math.random() * min.length)] : (roundingIncrement = roundingIncrement || 1e-5) && (returnFunction = roundingIncrement < 1 ? 10 ** ((roundingIncrement + "").length - 2) : 1) && (Math.floor(Math.round((min - roundingIncrement / 2 + Math.random() * (max - min + roundingIncrement * .99)) / roundingIncrement) * roundingIncrement * returnFunction) / returnFunction)), + pipe = (...functions) => value => functions.reduce((v, f) => f(v), value), + unitize = (func, unit) => value => func(parseFloat(value)) + (unit || getUnit(value)), + normalize = (min, max, value) => mapRange(min, max, 0, 1, value), + _wrapArray = (a, wrapper, value) => _conditionalReturn(value, index => a[~~wrapper(index)]), + wrap = function(min, max, value) { // NOTE: wrap() CANNOT be an arrow function! A very odd compiling bug causes problems (unrelated to GSAP). + let range = max - min; + return _isArray(min) ? _wrapArray(min, wrap(0, min.length), max) : _conditionalReturn(value, value => ((range + (value - min) % range) % range) + min); + }, + wrapYoyo = (min, max, value) => { + let range = max - min, + total = range * 2; + return _isArray(min) ? _wrapArray(min, wrapYoyo(0, min.length - 1), max) : _conditionalReturn(value, value => { + value = (total + (value - min) % total) % total || 0; + return min + ((value > range) ? (total - value) : value); + }); + }, + _replaceRandom = value => { //replaces all occurrences of random(...) in a string with the calculated random value. can be a range like random(-100, 100, 5) or an array like random([0, 100, 500]) + let prev = 0, + s = "", + i, nums, end, isArray; + while (~(i = value.indexOf("random(", prev))) { + end = value.indexOf(")", i); + isArray = value.charAt(i + 7) === "["; + nums = value.substr(i + 7, end - i - 7).match(isArray ? _delimitedValueExp : _strictNumExp); + s += value.substr(prev, i - prev) + random(isArray ? nums : +nums[0], isArray ? 0 : +nums[1], +nums[2] || 1e-5); + prev = end + 1; + } + return s + value.substr(prev, value.length - prev); + }, + mapRange = (inMin, inMax, outMin, outMax, value) => { + let inRange = inMax - inMin, + outRange = outMax - outMin; + return _conditionalReturn(value, value => outMin + ((((value - inMin) / inRange) * outRange) || 0)); + }, + interpolate = (start, end, progress, mutate) => { + let func = isNaN(start + end) ? 0 : p => (1 - p) * start + p * end; + if (!func) { + let isString = _isString(start), + master = {}, + p, i, interpolators, l, il; + progress === true && (mutate = 1) && (progress = null); + if (isString) { + start = {p: start}; + end = {p: end}; + + } else if (_isArray(start) && !_isArray(end)) { + interpolators = []; + l = start.length; + il = l - 2; + for (i = 1; i < l; i++) { + interpolators.push(interpolate(start[i-1], start[i])); //build the interpolators up front as a performance optimization so that when the function is called many times, it can just reuse them. + } + l--; + func = p => { + p *= l; + let i = Math.min(il, ~~p); + return interpolators[i](p - i); + }; + progress = end; + } else if (!mutate) { + start = _merge(_isArray(start) ? [] : {}, start); + } + if (!interpolators) { + for (p in end) { + _addPropTween.call(master, start, p, "get", end[p]); + } + func = p => _renderPropTweens(p, master) || (isString ? start.p : start); + } + } + return _conditionalReturn(progress, func); + }, + _getLabelInDirection = (timeline, fromTime, backward) => { //used for nextLabel() and previousLabel() + let labels = timeline.labels, + min = _bigNum, + p, distance, label; + for (p in labels) { + distance = labels[p] - fromTime; + if ((distance < 0) === !!backward && distance && min > (distance = Math.abs(distance))) { + label = p; + min = distance; + } + } + return label; + }, + _callback = (animation, type, executeLazyFirst) => { + let v = animation.vars, + callback = v[type], + prevContext = _context, + context = animation._ctx, + params, scope, result; + if (!callback) { + return; + } + params = v[type + "Params"]; + scope = v.callbackScope || animation; + executeLazyFirst && _lazyTweens.length && _lazyRender(); //in case rendering caused any tweens to lazy-init, we should render them because typically when a timeline finishes, users expect things to have rendered fully. Imagine an onUpdate on a timeline that reports/checks tweened values. + context && (_context = context); + result = params ? callback.apply(scope, params) : callback.call(scope); + _context = prevContext; + return result; + }, + _interrupt = animation => { + _removeFromParent(animation); + animation.scrollTrigger && animation.scrollTrigger.kill(!!_reverting); + animation.progress() < 1 && _callback(animation, "onInterrupt"); + return animation; + }, + _quickTween, + _registerPluginQueue = [], + _createPlugin = config => { + if (!config) return; + config = (!config.name && config.default) || config; // UMD packaging wraps things oddly, so for example MotionPathHelper becomes {MotionPathHelper:MotionPathHelper, default:MotionPathHelper}. + if (_windowExists() || config.headless) { // edge case: some build tools may pass in a null/undefined value + let name = config.name, + isFunc = _isFunction(config), + Plugin = (name && !isFunc && config.init) ? function () { + this._props = []; + } : config, //in case someone passes in an object that's not a plugin, like CustomEase + instanceDefaults = {init: _emptyFunc, render: _renderPropTweens, add: _addPropTween, kill: _killPropTweensOf, modifier: _addPluginModifier, rawVars: 0}, + statics = {targetTest: 0, get: 0, getSetter: _getSetter, aliases: {}, register: 0}; + _wake(); + if (config !== Plugin) { + if (_plugins[name]) { + return; + } + _setDefaults(Plugin, _setDefaults(_copyExcluding(config, instanceDefaults), statics)); //static methods + _merge(Plugin.prototype, _merge(instanceDefaults, _copyExcluding(config, statics))); //instance methods + _plugins[(Plugin.prop = name)] = Plugin; + if (config.targetTest) { + _harnessPlugins.push(Plugin); + _reservedProps[name] = 1; + } + name = (name === "css" ? "CSS" : name.charAt(0).toUpperCase() + name.substr(1)) + "Plugin"; //for the global name. "motionPath" should become MotionPathPlugin + } + _addGlobal(name, Plugin); + config.register && config.register(gsap, Plugin, PropTween); + } else { + _registerPluginQueue.push(config); + } + }, + + + + + + + + + + + + + + + + +/* + * -------------------------------------------------------------------------------------- + * COLORS + * -------------------------------------------------------------------------------------- + */ + + _255 = 255, + _colorLookup = { + aqua:[0,_255,_255], + lime:[0,_255,0], + silver:[192,192,192], + black:[0,0,0], + maroon:[128,0,0], + teal:[0,128,128], + blue:[0,0,_255], + navy:[0,0,128], + white:[_255,_255,_255], + olive:[128,128,0], + yellow:[_255,_255,0], + orange:[_255,165,0], + gray:[128,128,128], + purple:[128,0,128], + green:[0,128,0], + red:[_255,0,0], + pink:[_255,192,203], + cyan:[0,_255,_255], + transparent:[_255,_255,_255,0] + }, + // possible future idea to replace the hard-coded color name values - put this in the ticker.wake() where we set the _doc: + // let ctx = _doc.createElement("canvas").getContext("2d"); + // _forEachName("aqua,lime,silver,black,maroon,teal,blue,navy,white,olive,yellow,orange,gray,purple,green,red,pink,cyan", color => {ctx.fillStyle = color; _colorLookup[color] = splitColor(ctx.fillStyle)}); + _hue = (h, m1, m2) => { + h += h < 0 ? 1 : h > 1 ? -1 : 0; + return ((((h * 6 < 1) ? m1 + (m2 - m1) * h * 6 : h < .5 ? m2 : (h * 3 < 2) ? m1 + (m2 - m1) * (2 / 3 - h) * 6 : m1) * _255) + .5) | 0; + }, + splitColor = (v, toHSL, forceAlpha) => { + let a = !v ? _colorLookup.black : _isNumber(v) ? [v >> 16, (v >> 8) & _255, v & _255] : 0, + r, g, b, h, s, l, max, min, d, wasHSL; + if (!a) { + if (v.substr(-1) === ",") { //sometimes a trailing comma is included and we should chop it off (typically from a comma-delimited list of values like a textShadow:"2px 2px 2px blue, 5px 5px 5px rgb(255,0,0)" - in this example "blue," has a trailing comma. We could strip it out inside parseComplex() but we'd need to do it to the beginning and ending values plus it wouldn't provide protection from other potential scenarios like if the user passes in a similar value. + v = v.substr(0, v.length - 1); + } + if (_colorLookup[v]) { + a = _colorLookup[v]; + } else if (v.charAt(0) === "#") { + if (v.length < 6) { //for shorthand like #9F0 or #9F0F (could have alpha) + r = v.charAt(1); + g = v.charAt(2); + b = v.charAt(3); + v = "#" + r + r + g + g + b + b + (v.length === 5 ? v.charAt(4) + v.charAt(4) : ""); + } + if (v.length === 9) { // hex with alpha, like #fd5e53ff + a = parseInt(v.substr(1, 6), 16); + return [a >> 16, (a >> 8) & _255, a & _255, parseInt(v.substr(7), 16) / 255]; + } + v = parseInt(v.substr(1), 16); + a = [v >> 16, (v >> 8) & _255, v & _255]; + } else if (v.substr(0, 3) === "hsl") { + a = wasHSL = v.match(_strictNumExp); + if (!toHSL) { + h = (+a[0] % 360) / 360; + s = +a[1] / 100; + l = +a[2] / 100; + g = (l <= .5) ? l * (s + 1) : l + s - l * s; + r = l * 2 - g; + a.length > 3 && (a[3] *= 1); //cast as number + a[0] = _hue(h + 1 / 3, r, g); + a[1] = _hue(h, r, g); + a[2] = _hue(h - 1 / 3, r, g); + } else if (~v.indexOf("=")) { //if relative values are found, just return the raw strings with the relative prefixes in place. + a = v.match(_numExp); + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + } + } else { + a = v.match(_strictNumExp) || _colorLookup.transparent; + } + a = a.map(Number); + } + if (toHSL && !wasHSL) { + r = a[0] / _255; + g = a[1] / _255; + b = a[2] / _255; + max = Math.max(r, g, b); + min = Math.min(r, g, b); + l = (max + min) / 2; + if (max === min) { + h = s = 0; + } else { + d = max - min; + s = l > 0.5 ? d / (2 - max - min) : d / (max + min); + h = max === r ? (g - b) / d + (g < b ? 6 : 0) : max === g ? (b - r) / d + 2 : (r - g) / d + 4; + h *= 60; + } + a[0] = ~~(h + .5); + a[1] = ~~(s * 100 + .5); + a[2] = ~~(l * 100 + .5); + } + forceAlpha && a.length < 4 && (a[3] = 1); + return a; + }, + _colorOrderData = v => { // strips out the colors from the string, finds all the numeric slots (with units) and returns an array of those. The Array also has a "c" property which is an Array of the index values where the colors belong. This is to help work around issues where there's a mis-matched order of color/numeric data like drop-shadow(#f00 0px 1px 2px) and drop-shadow(0x 1px 2px #f00). This is basically a helper function used in _formatColors() + let values = [], + c = [], + i = -1; + v.split(_colorExp).forEach(v => { + let a = v.match(_numWithUnitExp) || []; + values.push(...a); + c.push(i += a.length + 1); + }); + values.c = c; + return values; + }, + _formatColors = (s, toHSL, orderMatchData) => { + let result = "", + colors = (s + result).match(_colorExp), + type = toHSL ? "hsla(" : "rgba(", + i = 0, + c, shell, d, l; + if (!colors) { + return s; + } + colors = colors.map(color => (color = splitColor(color, toHSL, 1)) && type + (toHSL ? color[0] + "," + color[1] + "%," + color[2] + "%," + color[3] : color.join(",")) + ")"); + if (orderMatchData) { + d = _colorOrderData(s); + c = orderMatchData.c; + if (c.join(result) !== d.c.join(result)) { + shell = s.replace(_colorExp, "1").split(_numWithUnitExp); + l = shell.length - 1; + for (; i < l; i++) { + result += shell[i] + (~c.indexOf(i) ? colors.shift() || type + "0,0,0,0)" : (d.length ? d : colors.length ? colors : orderMatchData).shift()); + } + } + } + if (!shell) { + shell = s.split(_colorExp); + l = shell.length - 1; + for (; i < l; i++) { + result += shell[i] + colors[i]; + } + } + return result + shell[l]; + }, + _colorExp = (function() { + let s = "(?:\\b(?:(?:rgb|rgba|hsl|hsla)\\(.+?\\))|\\B#(?:[0-9a-f]{3,4}){1,2}\\b", //we'll dynamically build this Regular Expression to conserve file size. After building it, it will be able to find rgb(), rgba(), # (hexadecimal), and named color values like red, blue, purple, etc., + p; + for (p in _colorLookup) { + s += "|" + p + "\\b"; + } + return new RegExp(s + ")", "gi"); + })(), + _hslExp = /hsl[a]?\(/, + _colorStringFilter = a => { + let combined = a.join(" "), + toHSL; + _colorExp.lastIndex = 0; + if (_colorExp.test(combined)) { + toHSL = _hslExp.test(combined); + a[1] = _formatColors(a[1], toHSL); + a[0] = _formatColors(a[0], toHSL, _colorOrderData(a[1])); // make sure the order of numbers/colors match with the END value. + return true; + } + }, + + + + + + + + + + + + + + + + +/* + * -------------------------------------------------------------------------------------- + * TICKER + * -------------------------------------------------------------------------------------- + */ + _tickerActive, + _ticker = (function() { + let _getTime = Date.now, + _lagThreshold = 500, + _adjustedLag = 33, + _startTime = _getTime(), + _lastUpdate = _startTime, + _gap = 1000 / 240, + _nextTime = _gap, + _listeners = [], + _id, _req, _raf, _self, _delta, _i, + _tick = v => { + let elapsed = _getTime() - _lastUpdate, + manual = v === true, + overlap, dispatch, time, frame; + (elapsed > _lagThreshold || elapsed < 0) && (_startTime += elapsed - _adjustedLag); + _lastUpdate += elapsed; + time = _lastUpdate - _startTime; + overlap = time - _nextTime; + if (overlap > 0 || manual) { + frame = ++_self.frame; + _delta = time - _self.time * 1000; + _self.time = time = time / 1000; + _nextTime += overlap + (overlap >= _gap ? 4 : _gap - overlap); + dispatch = 1; + } + manual || (_id = _req(_tick)); //make sure the request is made before we dispatch the "tick" event so that timing is maintained. Otherwise, if processing the "tick" requires a bunch of time (like 15ms) and we're using a setTimeout() that's based on 16.7ms, it'd technically take 31.7ms between frames otherwise. + if (dispatch) { + for (_i = 0; _i < _listeners.length; _i++) { // use _i and check _listeners.length instead of a variable because a listener could get removed during the loop, and if that happens to an element less than the current index, it'd throw things off in the loop. + _listeners[_i](time, _delta, frame, v); + } + } + }; + _self = { + time:0, + frame:0, + tick() { + _tick(true); + }, + deltaRatio(fps) { + return _delta / (1000 / (fps || 60)); + }, + wake() { + if (_coreReady) { + if (!_coreInitted && _windowExists()) { + _win = _coreInitted = window; + _doc = _win.document || {}; + _globals.gsap = gsap; + (_win.gsapVersions || (_win.gsapVersions = [])).push(gsap.version); + _install(_installScope || _win.GreenSockGlobals || (!_win.gsap && _win) || {}); + _registerPluginQueue.forEach(_createPlugin); + } + _raf = typeof(requestAnimationFrame) !== "undefined" && requestAnimationFrame; + _id && _self.sleep(); + _req = _raf || (f => setTimeout(f, (_nextTime - _self.time * 1000 + 1) | 0)); + _tickerActive = 1; + _tick(2); + } + }, + sleep() { + (_raf ? cancelAnimationFrame : clearTimeout)(_id); + _tickerActive = 0; + _req = _emptyFunc; + }, + lagSmoothing(threshold, adjustedLag) { + _lagThreshold = threshold || Infinity; // zero should be interpreted as basically unlimited + _adjustedLag = Math.min(adjustedLag || 33, _lagThreshold); + }, + fps(fps) { + _gap = 1000 / (fps || 240); + _nextTime = _self.time * 1000 + _gap; + }, + add(callback, once, prioritize) { + let func = once ? (t, d, f, v) => {callback(t, d, f, v); _self.remove(func);} : callback; + _self.remove(callback); + _listeners[prioritize ? "unshift" : "push"](func); + _wake(); + return func; + }, + remove(callback, i) { + ~(i = _listeners.indexOf(callback)) && _listeners.splice(i, 1) && _i >= i && _i--; + }, + _listeners:_listeners + }; + return _self; + })(), + _wake = () => !_tickerActive && _ticker.wake(), //also ensures the core classes are initialized. + + + + + + + + + + + + + + +/* +* ------------------------------------------------- +* EASING +* ------------------------------------------------- +*/ + _easeMap = {}, + _customEaseExp = /^[\d.\-M][\d.\-,\s]/, + _quotesExp = /["']/g, + _parseObjectInString = value => { //takes a string like "{wiggles:10, type:anticipate})" and turns it into a real object. Notice it ends in ")" and includes the {} wrappers. This is because we only use this function for parsing ease configs and prioritized optimization rather than reusability. + let obj = {}, + split = value.substr(1, value.length-3).split(":"), + key = split[0], + i = 1, + l = split.length, + index, val, parsedVal; + for (; i < l; i++) { + val = split[i]; + index = i !== l-1 ? val.lastIndexOf(",") : val.length; + parsedVal = val.substr(0, index); + obj[key] = isNaN(parsedVal) ? parsedVal.replace(_quotesExp, "").trim() : +parsedVal; + key = val.substr(index+1).trim(); + } + return obj; + }, + _valueInParentheses = value => { + let open = value.indexOf("(") + 1, + close = value.indexOf(")"), + nested = value.indexOf("(", open); + return value.substring(open, ~nested && nested < close ? value.indexOf(")", close + 1) : close); + }, + _configEaseFromString = name => { //name can be a string like "elastic.out(1,0.5)", and pass in _easeMap as obj and it'll parse it out and call the actual function like _easeMap.Elastic.easeOut.config(1,0.5). It will also parse custom ease strings as long as CustomEase is loaded and registered (internally as _easeMap._CE). + let split = (name + "").split("("), + ease = _easeMap[split[0]]; + return (ease && split.length > 1 && ease.config) ? ease.config.apply(null, ~name.indexOf("{") ? [_parseObjectInString(split[1])] : _valueInParentheses(name).split(",").map(_numericIfPossible)) : (_easeMap._CE && _customEaseExp.test(name)) ? _easeMap._CE("", name) : ease; + }, + _invertEase = ease => p => 1 - ease(1 - p), + // allow yoyoEase to be set in children and have those affected when the parent/ancestor timeline yoyos. + _propagateYoyoEase = (timeline, isYoyo) => { + let child = timeline._first, ease; + while (child) { + if (child instanceof Timeline) { + _propagateYoyoEase(child, isYoyo); + } else if (child.vars.yoyoEase && (!child._yoyo || !child._repeat) && child._yoyo !== isYoyo) { + if (child.timeline) { + _propagateYoyoEase(child.timeline, isYoyo); + } else { + ease = child._ease; + child._ease = child._yEase; + child._yEase = ease; + child._yoyo = isYoyo; + } + } + child = child._next; + } + }, + _parseEase = (ease, defaultEase) => !ease ? defaultEase : (_isFunction(ease) ? ease : _easeMap[ease] || _configEaseFromString(ease)) || defaultEase, + _insertEase = (names, easeIn, easeOut = p => 1 - easeIn(1 - p), easeInOut = (p => p < .5 ? easeIn(p * 2) / 2 : 1 - easeIn((1 - p) * 2) / 2)) => { + let ease = {easeIn, easeOut, easeInOut}, + lowercaseName; + _forEachName(names, name => { + _easeMap[name] = _globals[name] = ease; + _easeMap[(lowercaseName = name.toLowerCase())] = easeOut; + for (let p in ease) { + _easeMap[lowercaseName + (p === "easeIn" ? ".in" : p === "easeOut" ? ".out" : ".inOut")] = _easeMap[name + "." + p] = ease[p]; + } + }); + return ease; + }, + _easeInOutFromOut = easeOut => (p => p < .5 ? (1 - easeOut(1 - (p * 2))) / 2 : .5 + easeOut((p - .5) * 2) / 2), + _configElastic = (type, amplitude, period) => { + let p1 = (amplitude >= 1) ? amplitude : 1, //note: if amplitude is < 1, we simply adjust the period for a more natural feel. Otherwise the math doesn't work right and the curve starts at 1. + p2 = (period || (type ? .3 : .45)) / (amplitude < 1 ? amplitude : 1), + p3 = p2 / _2PI * (Math.asin(1 / p1) || 0), + easeOut = p => p === 1 ? 1 : p1 * (2 ** (-10 * p)) * _sin((p - p3) * p2) + 1, + ease = (type === "out") ? easeOut : (type === "in") ? p => 1 - easeOut(1 - p) : _easeInOutFromOut(easeOut); + p2 = _2PI / p2; //precalculate to optimize + ease.config = (amplitude, period) => _configElastic(type, amplitude, period); + return ease; + }, + _configBack = (type, overshoot = 1.70158) => { + let easeOut = p => p ? ((--p) * p * ((overshoot + 1) * p + overshoot) + 1) : 0, + ease = type === "out" ? easeOut : type === "in" ? p => 1 - easeOut(1 - p) : _easeInOutFromOut(easeOut); + ease.config = overshoot => _configBack(type, overshoot); + return ease; + }; + // a cheaper (kb and cpu) but more mild way to get a parameterized weighted ease by feeding in a value between -1 (easeIn) and 1 (easeOut) where 0 is linear. + // _weightedEase = ratio => { + // let y = 0.5 + ratio / 2; + // return p => (2 * (1 - p) * p * y + p * p); + // }, + // a stronger (but more expensive kb/cpu) parameterized weighted ease that lets you feed in a value between -1 (easeIn) and 1 (easeOut) where 0 is linear. + // _weightedEaseStrong = ratio => { + // ratio = .5 + ratio / 2; + // let o = 1 / 3 * (ratio < .5 ? ratio : 1 - ratio), + // b = ratio - o, + // c = ratio + o; + // return p => p === 1 ? p : 3 * b * (1 - p) * (1 - p) * p + 3 * c * (1 - p) * p * p + p * p * p; + // }; + +_forEachName("Linear,Quad,Cubic,Quart,Quint,Strong", (name, i) => { + let power = i < 5 ? i + 1 : i; + _insertEase(name + ",Power" + (power - 1), i ? p => p ** power : p => p, p => 1 - (1 - p) ** power, p => p < .5 ? (p * 2) ** power / 2 : 1 - ((1 - p) * 2) ** power / 2); +}); +_easeMap.Linear.easeNone = _easeMap.none = _easeMap.Linear.easeIn; +_insertEase("Elastic", _configElastic("in"), _configElastic("out"), _configElastic()); +((n, c) => { + let n1 = 1 / c, + n2 = 2 * n1, + n3 = 2.5 * n1, + easeOut = p => (p < n1) ? n * p * p : (p < n2) ? n * (p - 1.5 / c) ** 2 + .75 : (p < n3) ? n * (p -= 2.25 / c) * p + .9375 : n * (p - 2.625 / c) ** 2 + .984375; + _insertEase("Bounce", p => 1 - easeOut(1 - p), easeOut); +})(7.5625, 2.75); +_insertEase("Expo", p => (2 ** (10 * (p - 1))) * p + p * p * p * p * p * p * (1-p)); // previously 2 ** (10 * (p - 1)) but that doesn't end up with the value quite at the right spot so we do a blended ease to ensure it lands where it should perfectly. +_insertEase("Circ", p => -(_sqrt(1 - (p * p)) - 1)); +_insertEase("Sine", p => p === 1 ? 1 : -_cos(p * _HALF_PI) + 1); +_insertEase("Back", _configBack("in"), _configBack("out"), _configBack()); +_easeMap.SteppedEase = _easeMap.steps = _globals.SteppedEase = { + config(steps = 1, immediateStart) { + let p1 = 1 / steps, + p2 = steps + (immediateStart ? 0 : 1), + p3 = immediateStart ? 1 : 0, + max = 1 - _tinyNum; + return p => (((p2 * _clamp(0, max, p)) | 0) + p3) * p1; + } +}; +_defaults.ease = _easeMap["quad.out"]; + + +_forEachName("onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt", name => _callbackNames += name + "," + name + "Params,"); + + + + + + + + + + + + + + +/* + * -------------------------------------------------------------------------------------- + * CACHE + * -------------------------------------------------------------------------------------- + */ +export class GSCache { + + constructor(target, harness) { + this.id = _gsID++; + target._gsap = this; + this.target = target; + this.harness = harness; + this.get = harness ? harness.get : _getProperty; + this.set = harness ? harness.getSetter : _getSetter; + } + +} + + + + + + + + + + + + + + + +/* + * -------------------------------------------------------------------------------------- + * ANIMATION + * -------------------------------------------------------------------------------------- + */ + +export class Animation { + + constructor(vars) { + this.vars = vars; + this._delay = +vars.delay || 0; + if ((this._repeat = vars.repeat === Infinity ? -2 : vars.repeat || 0)) { // TODO: repeat: Infinity on a timeline's children must flag that timeline internally and affect its totalDuration, otherwise it'll stop in the negative direction when reaching the start. + this._rDelay = vars.repeatDelay || 0; + this._yoyo = !!vars.yoyo || !!vars.yoyoEase; + } + this._ts = 1; + _setDuration(this, +vars.duration, 1, 1); + this.data = vars.data; + if (_context) { + this._ctx = _context; + _context.data.push(this); + } + _tickerActive || _ticker.wake(); + } + + delay(value) { + if (value || value === 0) { + this.parent && this.parent.smoothChildTiming && (this.startTime(this._start + value - this._delay)); + this._delay = value; + return this; + } + return this._delay; + } + + duration(value) { + return arguments.length ? this.totalDuration(this._repeat > 0 ? value + (value + this._rDelay) * this._repeat : value) : this.totalDuration() && this._dur; + } + + totalDuration(value) { + if (!arguments.length) { + return this._tDur; + } + this._dirty = 0; + return _setDuration(this, this._repeat < 0 ? value : (value - (this._repeat * this._rDelay)) / (this._repeat + 1)); + } + + totalTime(totalTime, suppressEvents) { + _wake(); + if (!arguments.length) { + return this._tTime; + } + let parent = this._dp; + if (parent && parent.smoothChildTiming && this._ts) { + _alignPlayhead(this, totalTime); + !parent._dp || parent.parent || _postAddChecks(parent, this); // edge case: if this is a child of a timeline that already completed, for example, we must re-activate the parent. + //in case any of the ancestor timelines had completed but should now be enabled, we should reset their totalTime() which will also ensure that they're lined up properly and enabled. Skip for animations that are on the root (wasteful). Example: a TimelineLite.exportRoot() is performed when there's a paused tween on the root, the export will not complete until that tween is unpaused, but imagine a child gets restarted later, after all [unpaused] tweens have completed. The start of that child would get pushed out, but one of the ancestors may have completed. + while (parent && parent.parent) { + if (parent.parent._time !== parent._start + (parent._ts >= 0 ? parent._tTime / parent._ts : (parent.totalDuration() - parent._tTime) / -parent._ts)) { + parent.totalTime(parent._tTime, true); + } + parent = parent.parent; + } + if (!this.parent && this._dp.autoRemoveChildren && ((this._ts > 0 && totalTime < this._tDur) || (this._ts < 0 && totalTime > 0) || (!this._tDur && !totalTime) )) { //if the animation doesn't have a parent, put it back into its last parent (recorded as _dp for exactly cases like this). Limit to parents with autoRemoveChildren (like globalTimeline) so that if the user manually removes an animation from a timeline and then alters its playhead, it doesn't get added back in. + _addToTimeline(this._dp, this, this._start - this._delay); + } + } + if (this._tTime !== totalTime || (!this._dur && !suppressEvents) || (this._initted && Math.abs(this._zTime) === _tinyNum) || (!totalTime && !this._initted && (this.add || this._ptLookup))) { // check for _ptLookup on a Tween instance to ensure it has actually finished being instantiated, otherwise if this.reverse() gets called in the Animation constructor, it could trigger a render() here even though the _targets weren't populated, thus when _init() is called there won't be any PropTweens (it'll act like the tween is non-functional) + this._ts || (this._pTime = totalTime); // otherwise, if an animation is paused, then the playhead is moved back to zero, then resumed, it'd revert back to the original time at the pause + //if (!this._lock) { // avoid endless recursion (not sure we need this yet or if it's worth the performance hit) + // this._lock = 1; + _lazySafeRender(this, totalTime, suppressEvents); + // this._lock = 0; + //} + } + return this; + } + + time(value, suppressEvents) { + return arguments.length ? this.totalTime((Math.min(this.totalDuration(), value + _elapsedCycleDuration(this)) % (this._dur + this._rDelay)) || (value ? this._dur : 0), suppressEvents) : this._time; // note: if the modulus results in 0, the playhead could be exactly at the end or the beginning, and we always defer to the END with a non-zero value, otherwise if you set the time() to the very end (duration()), it would render at the START! + } + + totalProgress(value, suppressEvents) { + return arguments.length ? this.totalTime( this.totalDuration() * value, suppressEvents) : this.totalDuration() ? Math.min(1, this._tTime / this._tDur) : this.rawTime() >= 0 && this._initted ? 1 : 0; + } + + progress(value, suppressEvents) { + return arguments.length ? this.totalTime( this.duration() * (this._yoyo && !(this.iteration() & 1) ? 1 - value : value) + _elapsedCycleDuration(this), suppressEvents) : (this.duration() ? Math.min(1, this._time / this._dur) : this.rawTime() > 0 ? 1 : 0); + } + + iteration(value, suppressEvents) { + let cycleDuration = this.duration() + this._rDelay; + return arguments.length ? this.totalTime(this._time + (value - 1) * cycleDuration, suppressEvents) : this._repeat ? _animationCycle(this._tTime, cycleDuration) + 1 : 1; + } + + // potential future addition: + // isPlayingBackwards() { + // let animation = this, + // orientation = 1; // 1 = forward, -1 = backward + // while (animation) { + // orientation *= animation.reversed() || (animation.repeat() && !(animation.iteration() & 1)) ? -1 : 1; + // animation = animation.parent; + // } + // return orientation < 0; + // } + + timeScale(value, suppressEvents) { + if (!arguments.length) { + return this._rts === -_tinyNum ? 0 : this._rts; // recorded timeScale. Special case: if someone calls reverse() on an animation with timeScale of 0, we assign it -_tinyNum to remember it's reversed. + } + if (this._rts === value) { + return this; + } + let tTime = this.parent && this._ts ? _parentToChildTotalTime(this.parent._time, this) : this._tTime; // make sure to do the parentToChildTotalTime() BEFORE setting the new _ts because the old one must be used in that calculation. + + // future addition? Up side: fast and minimal file size. Down side: only works on this animation; if a timeline is reversed, for example, its childrens' onReverse wouldn't get called. + //(+value < 0 && this._rts >= 0) && _callback(this, "onReverse", true); + + // prioritize rendering where the parent's playhead lines up instead of this._tTime because there could be a tween that's animating another tween's timeScale in the same rendering loop (same parent), thus if the timeScale tween renders first, it would alter _start BEFORE _tTime was set on that tick (in the rendering loop), effectively freezing it until the timeScale tween finishes. + this._rts = +value || 0; + this._ts = (this._ps || value === -_tinyNum) ? 0 : this._rts; // _ts is the functional timeScale which would be 0 if the animation is paused. + this.totalTime(_clamp(-Math.abs(this._delay), this._tDur, tTime), suppressEvents !== false); + _setEnd(this); // if parent.smoothChildTiming was false, the end time didn't get updated in the _alignPlayhead() method, so do it here. + return _recacheAncestors(this); + } + + paused(value) { + if (!arguments.length) { + return this._ps; + } + // possible future addition - if an animation is removed from its parent and then .restart() or .play() or .resume() is called, perhaps we should force it back into the globalTimeline but be careful because what if it's already at its end? We don't want it to just persist forever and not get released for GC. + // !this.parent && !value && this._tTime < this._tDur && this !== _globalTimeline && _globalTimeline.add(this); + if (this._ps !== value) { + this._ps = value; + if (value) { + this._pTime = this._tTime || Math.max(-this._delay, this.rawTime()); // if the pause occurs during the delay phase, make sure that's factored in when resuming. + this._ts = this._act = 0; // _ts is the functional timeScale, so a paused tween would effectively have a timeScale of 0. We record the "real" timeScale as _rts (recorded time scale) + } else { + _wake(); + this._ts = this._rts; + //only defer to _pTime (pauseTime) if tTime is zero. Remember, someone could pause() an animation, then scrub the playhead and resume(). If the parent doesn't have smoothChildTiming, we render at the rawTime() because the startTime won't get updated. + this.totalTime(this.parent && !this.parent.smoothChildTiming ? this.rawTime() : this._tTime || this._pTime, (this.progress() === 1) && Math.abs(this._zTime) !== _tinyNum && (this._tTime -= _tinyNum)); // edge case: animation.progress(1).pause().play() wouldn't render again because the playhead is already at the end, but the call to totalTime() below will add it back to its parent...and not remove it again (since removing only happens upon rendering at a new time). Offsetting the _tTime slightly is done simply to cause the final render in totalTime() that'll pop it off its timeline (if autoRemoveChildren is true, of course). Check to make sure _zTime isn't -_tinyNum to avoid an edge case where the playhead is pushed to the end but INSIDE a tween/callback, the timeline itself is paused thus halting rendering and leaving a few unrendered. When resuming, it wouldn't render those otherwise. + } + } + return this; + } + + startTime(value) { + if (arguments.length) { + this._start = value; + let parent = this.parent || this._dp; + parent && (parent._sort || !this.parent) && _addToTimeline(parent, this, value - this._delay); + return this; + } + return this._start; + } + + endTime(includeRepeats) { + return this._start + (_isNotFalse(includeRepeats) ? this.totalDuration() : this.duration()) / Math.abs(this._ts || 1); + } + + rawTime(wrapRepeats) { + let parent = this.parent || this._dp; // _dp = detached parent + return !parent ? this._tTime : (wrapRepeats && (!this._ts || (this._repeat && this._time && this.totalProgress() < 1))) ? this._tTime % (this._dur + this._rDelay) : !this._ts ? this._tTime : _parentToChildTotalTime(parent.rawTime(wrapRepeats), this); + } + + revert(config= _revertConfig) { + let prevIsReverting = _reverting; + _reverting = config; + if (this._initted || this._startAt) { + this.timeline && this.timeline.revert(config); + this.totalTime(-0.01, config.suppressEvents); + } + this.data !== "nested" && config.kill !== false && this.kill(); + _reverting = prevIsReverting; + return this; + } + + globalTime(rawTime) { + let animation = this, + time = arguments.length ? rawTime : animation.rawTime(); + while (animation) { + time = animation._start + time / (Math.abs(animation._ts) || 1); + animation = animation._dp; + } + return !this.parent && this._sat ? this._sat.globalTime(rawTime) : time; // the _startAt tweens for .fromTo() and .from() that have immediateRender should always be FIRST in the timeline (important for context.revert()). "_sat" stands for _startAtTween, referring to the parent tween that created the _startAt. We must discern if that tween had immediateRender so that we can know whether or not to prioritize it in revert(). + } + + repeat(value) { + if (arguments.length) { + this._repeat = value === Infinity ? -2 : value; + return _onUpdateTotalDuration(this); + } + return this._repeat === -2 ? Infinity : this._repeat; + } + + repeatDelay(value) { + if (arguments.length) { + let time = this._time; + this._rDelay = value; + _onUpdateTotalDuration(this); + return time ? this.time(time) : this; + } + return this._rDelay; + } + + yoyo(value) { + if (arguments.length) { + this._yoyo = value; + return this; + } + return this._yoyo; + } + + seek(position, suppressEvents) { + return this.totalTime(_parsePosition(this, position), _isNotFalse(suppressEvents)); + } + + restart(includeDelay, suppressEvents) { + this.play().totalTime(includeDelay ? -this._delay : 0, _isNotFalse(suppressEvents)); + this._dur || (this._zTime = -_tinyNum); // ensures onComplete fires on a zero-duration animation that gets restarted. + return this; + } + + play(from, suppressEvents) { + from != null && this.seek(from, suppressEvents); + return this.reversed(false).paused(false); + } + + reverse(from, suppressEvents) { + from != null && this.seek(from || this.totalDuration(), suppressEvents); + return this.reversed(true).paused(false); + } + + pause(atTime, suppressEvents) { + atTime != null && this.seek(atTime, suppressEvents); + return this.paused(true); + } + + resume() { + return this.paused(false); + } + + reversed(value) { + if (arguments.length) { + !!value !== this.reversed() && this.timeScale(-this._rts || (value ? -_tinyNum : 0)); // in case timeScale is zero, reversing would have no effect so we use _tinyNum. + return this; + } + return this._rts < 0; + } + + invalidate() { + this._initted = this._act = 0; + this._zTime = -_tinyNum; + return this; + } + + isActive() { + let parent = this.parent || this._dp, + start = this._start, + rawTime; + return !!(!parent || (this._ts && this._initted && parent.isActive() && (rawTime = parent.rawTime(true)) >= start && rawTime < this.endTime(true) - _tinyNum)); + } + + eventCallback(type, callback, params) { + let vars = this.vars; + if (arguments.length > 1) { + if (!callback) { + delete vars[type]; + } else { + vars[type] = callback; + params && (vars[type + "Params"] = params); + type === "onUpdate" && (this._onUpdate = callback); + } + return this; + } + return vars[type]; + } + + then(onFulfilled) { + let self = this; + return new Promise(resolve => { + let f = _isFunction(onFulfilled) ? onFulfilled : _passThrough, + _resolve = () => { + let _then = self.then; + self.then = null; // temporarily null the then() method to avoid an infinite loop (see https://github.com/greensock/GSAP/issues/322) + _isFunction(f) && (f = f(self)) && (f.then || f === self) && (self.then = _then); + resolve(f); + self.then = _then; + }; + if (self._initted && (self.totalProgress() === 1 && self._ts >= 0) || (!self._tTime && self._ts < 0)) { + _resolve(); + } else { + self._prom = _resolve; + } + }); + } + + kill() { + _interrupt(this); + } + +} + +_setDefaults(Animation.prototype, {_time:0, _start:0, _end:0, _tTime:0, _tDur:0, _dirty:0, _repeat:0, _yoyo:false, parent:null, _initted:false, _rDelay:0, _ts:1, _dp:0, ratio:0, _zTime:-_tinyNum, _prom:0, _ps:false, _rts:1}); + + + + + + + + + + + + + + + + + + +/* + * ------------------------------------------------- + * TIMELINE + * ------------------------------------------------- + */ + +export class Timeline extends Animation { + + constructor(vars = {}, position) { + super(vars); + this.labels = {}; + this.smoothChildTiming = !!vars.smoothChildTiming; + this.autoRemoveChildren = !!vars.autoRemoveChildren; + this._sort = _isNotFalse(vars.sortChildren); + _globalTimeline && _addToTimeline(vars.parent || _globalTimeline, this, position); + vars.reversed && this.reverse(); + vars.paused && this.paused(true); + vars.scrollTrigger && _scrollTrigger(this, vars.scrollTrigger); + } + + to(targets, vars, position) { + _createTweenType(0, arguments, this); + return this; + } + + from(targets, vars, position) { + _createTweenType(1, arguments, this); + return this; + } + + fromTo(targets, fromVars, toVars, position) { + _createTweenType(2, arguments, this); + return this; + } + + set(targets, vars, position) { + vars.duration = 0; + vars.parent = this; + _inheritDefaults(vars).repeatDelay || (vars.repeat = 0); + vars.immediateRender = !!vars.immediateRender; + new Tween(targets, vars, _parsePosition(this, position), 1); + return this; + } + + call(callback, params, position) { + return _addToTimeline(this, Tween.delayedCall(0, callback, params), position); + } + + //ONLY for backward compatibility! Maybe delete? + staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.duration = duration; + vars.stagger = vars.stagger || stagger; + vars.onComplete = onCompleteAll; + vars.onCompleteParams = onCompleteAllParams; + vars.parent = this; + new Tween(targets, vars, _parsePosition(this, position)); + return this; + } + + staggerFrom(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams) { + vars.runBackwards = 1; + _inheritDefaults(vars).immediateRender = _isNotFalse(vars.immediateRender); + return this.staggerTo(targets, duration, vars, stagger, position, onCompleteAll, onCompleteAllParams); + } + + staggerFromTo(targets, duration, fromVars, toVars, stagger, position, onCompleteAll, onCompleteAllParams) { + toVars.startAt = fromVars; + _inheritDefaults(toVars).immediateRender = _isNotFalse(toVars.immediateRender); + return this.staggerTo(targets, duration, toVars, stagger, position, onCompleteAll, onCompleteAllParams); + } + + render(totalTime, suppressEvents, force) { + let prevTime = this._time, + tDur = this._dirty ? this.totalDuration() : this._tDur, + dur = this._dur, + tTime = totalTime <= 0 ? 0 : _roundPrecise(totalTime), // if a paused timeline is resumed (or its _start is updated for another reason...which rounds it), that could result in the playhead shifting a **tiny** amount and a zero-duration child at that spot may get rendered at a different ratio, like its totalTime in render() may be 1e-17 instead of 0, for example. + crossingStart = (this._zTime < 0) !== (totalTime < 0) && (this._initted || !dur), + time, child, next, iteration, cycleDuration, prevPaused, pauseTween, timeScale, prevStart, prevIteration, yoyo, isYoyo; + this !== _globalTimeline && tTime > tDur && totalTime >= 0 && (tTime = tDur); + if (tTime !== this._tTime || force || crossingStart) { + if (prevTime !== this._time && dur) { //if totalDuration() finds a child with a negative startTime and smoothChildTiming is true, things get shifted around internally so we need to adjust the time accordingly. For example, if a tween starts at -30 we must shift EVERYTHING forward 30 seconds and move this timeline's startTime backward by 30 seconds so that things align with the playhead (no jump). + tTime += this._time - prevTime; + totalTime += this._time - prevTime; + } + time = tTime; + prevStart = this._start; + timeScale = this._ts; + prevPaused = !timeScale; + if (crossingStart) { + dur || (prevTime = this._zTime); + //when the playhead arrives at EXACTLY time 0 (right on top) of a zero-duration timeline, we need to discern if events are suppressed so that when the playhead moves again (next time), it'll trigger the callback. If events are NOT suppressed, obviously the callback would be triggered in this render. Basically, the callback should fire either when the playhead ARRIVES or LEAVES this exact spot, not both. Imagine doing a timeline.seek(0) and there's a callback that sits at 0. Since events are suppressed on that seek() by default, nothing will fire, but when the playhead moves off of that position, the callback should fire. This behavior is what people intuitively expect. + (totalTime || !suppressEvents) && (this._zTime = totalTime); + } + if (this._repeat) { //adjust the time for repeats and yoyos + yoyo = this._yoyo; + cycleDuration = dur + this._rDelay; + if (this._repeat < -1 && totalTime < 0) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + time = _roundPrecise(tTime % cycleDuration); //round to avoid floating point errors. (4 % 0.8 should be 0 but some browsers report it as 0.79999999!) + if (tTime === tDur) { // the tDur === tTime is for edge cases where there's a lengthy decimal on the duration and it may reach the very end but the time is rendered as not-quite-there (remember, tDur is rounded to 4 decimals whereas dur isn't) + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); // full decimal version of iterations, not the previous iteration (we're reusing prevIteration variable for efficiency) + iteration = ~~prevIteration; + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } + time > dur && (time = dur); + } + prevIteration = _animationCycle(this._tTime, cycleDuration); + !prevTime && this._tTime && prevIteration !== iteration && this._tTime - prevIteration * cycleDuration - this._dur <= 0 && (prevIteration = iteration); // edge case - if someone does addPause() at the very beginning of a repeating timeline, that pause is technically at the same spot as the end which causes this._time to get set to 0 when the totalTime would normally place the playhead at the end. See https://gsap.com/forums/topic/23823-closing-nav-animation-not-working-on-ie-and-iphone-6-maybe-other-older-browser/?tab=comments#comment-113005 also, this._tTime - prevIteration * cycleDuration - this._dur <= 0 just checks to make sure it wasn't previously in the "repeatDelay" portion + if (yoyo && (iteration & 1)) { + time = dur - time; + isYoyo = 1; + } + /* + make sure children at the end/beginning of the timeline are rendered properly. If, for example, + a 3-second long timeline rendered at 2.9 seconds previously, and now renders at 3.2 seconds (which + would get translated to 2.8 seconds if the timeline yoyos or 0.2 seconds if it just repeats), there + could be a callback or a short tween that's at 2.95 or 3 seconds in which wouldn't render. So + we need to push the timeline to the end (and/or beginning depending on its yoyo value). Also we must + ensure that zero-duration tweens at the very beginning or end of the Timeline work. + */ + if (iteration !== prevIteration && !this._lock) { + let rewinding = (yoyo && (prevIteration & 1)), + doesWrap = (rewinding === (yoyo && (iteration & 1))); + iteration < prevIteration && (rewinding = !rewinding); + prevTime = rewinding ? 0 : tTime % dur ? dur : tTime; // if the playhead is landing exactly at the end of an iteration, use that totalTime rather than only the duration, otherwise it'll skip the 2nd render since it's effectively at the same time. + this._lock = 1; + this.render(prevTime || (isYoyo ? 0 : _roundPrecise(iteration * cycleDuration)), suppressEvents, !dur)._lock = 0; + this._tTime = tTime; // if a user gets the iteration() inside the onRepeat, for example, it should be accurate. + !suppressEvents && this.parent && _callback(this, "onRepeat"); + this.vars.repeatRefresh && !isYoyo && (this.invalidate()._lock = 1); + if ((prevTime && prevTime !== this._time) || prevPaused !== !this._ts || (this.vars.onRepeat && !this.parent && !this._act)) { // if prevTime is 0 and we render at the very end, _time will be the end, thus won't match. So in this edge case, prevTime won't match _time but that's okay. If it gets killed in the onRepeat, eject as well. + return this; + } + dur = this._dur; // in case the duration changed in the onRepeat + tDur = this._tDur; + if (doesWrap) { + this._lock = 2; + prevTime = rewinding ? dur : -0.0001; + this.render(prevTime, true); + this.vars.repeatRefresh && !isYoyo && this.invalidate(); + } + this._lock = 0; + if (!this._ts && !prevPaused) { + return this; + } + //in order for yoyoEase to work properly when there's a stagger, we must swap out the ease in each sub-tween. + _propagateYoyoEase(this, isYoyo); + } + } + if (this._hasPause && !this._forcing && this._lock < 2) { + pauseTween = _findNextPauseTween(this, _roundPrecise(prevTime), _roundPrecise(time)); + if (pauseTween) { + tTime -= time - (time = pauseTween._start); + } + } + + this._tTime = tTime; + this._time = time; + this._act = !timeScale; //as long as it's not paused, force it to be active so that if the user renders independent of the parent timeline, it'll be forced to re-render on the next tick. + + if (!this._initted) { + this._onUpdate = this.vars.onUpdate; + this._initted = 1; + this._zTime = totalTime; + prevTime = 0; // upon init, the playhead should always go forward; someone could invalidate() a completed timeline and then if they restart(), that would make child tweens render in reverse order which could lock in the wrong starting values if they build on each other, like tl.to(obj, {x: 100}).to(obj, {x: 0}). + } + if (!prevTime && time && !suppressEvents && !iteration) { + _callback(this, "onStart"); + if (this._tTime !== tTime) { // in case the onStart triggered a render at a different spot, eject. Like if someone did animation.pause(0.5) or something inside the onStart. + return this; + } + } + if (time >= prevTime && totalTime >= 0) { + child = this._first; + while (child) { + next = child._next; + if ((child._act || time >= child._start) && child._ts && pauseTween !== child) { + if (child.parent !== this) { // an extreme edge case - the child's render could do something like kill() the "next" one in the linked list, or reparent it. In that case we must re-initiate the whole render to be safe. + return this.render(totalTime, suppressEvents, force); + } + child.render(child._ts > 0 ? (time - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (time - child._start) * child._ts, suppressEvents, force); + if (time !== this._time || (!this._ts && !prevPaused)) { //in case a tween pauses or seeks the timeline when rendering, like inside of an onUpdate/onComplete + pauseTween = 0; + next && (tTime += (this._zTime = -_tinyNum)); // it didn't finish rendering, so flag zTime as negative so that the next time render() is called it'll be forced (to render any remaining children) + break; + } + } + child = next; + } + } else { + child = this._last; + let adjustedTime = totalTime < 0 ? totalTime : time; //when the playhead goes backward beyond the start of this timeline, we must pass that information down to the child animations so that zero-duration tweens know whether to render their starting or ending values. + while (child) { + next = child._prev; + if ((child._act || adjustedTime <= child._end) && child._ts && pauseTween !== child) { + if (child.parent !== this) { // an extreme edge case - the child's render could do something like kill() the "next" one in the linked list, or reparent it. In that case we must re-initiate the whole render to be safe. + return this.render(totalTime, suppressEvents, force); + } + child.render(child._ts > 0 ? (adjustedTime - child._start) * child._ts : (child._dirty ? child.totalDuration() : child._tDur) + (adjustedTime - child._start) * child._ts, suppressEvents, force || (_reverting && (child._initted || child._startAt))); // if reverting, we should always force renders of initted tweens (but remember that .fromTo() or .from() may have a _startAt but not _initted yet). If, for example, a .fromTo() tween with a stagger (which creates an internal timeline) gets reverted BEFORE some of its child tweens render for the first time, it may not properly trigger them to revert. + if (time !== this._time || (!this._ts && !prevPaused)) { //in case a tween pauses or seeks the timeline when rendering, like inside of an onUpdate/onComplete + pauseTween = 0; + next && (tTime += (this._zTime = adjustedTime ? -_tinyNum : _tinyNum)); // it didn't finish rendering, so adjust zTime so that so that the next time render() is called it'll be forced (to render any remaining children) + break; + } + } + child = next; + } + } + if (pauseTween && !suppressEvents) { + this.pause(); + pauseTween.render(time >= prevTime ? 0 : -_tinyNum)._zTime = time >= prevTime ? 1 : -1; + if (this._ts) { //the callback resumed playback! So since we may have held back the playhead due to where the pause is positioned, go ahead and jump to where it's SUPPOSED to be (if no pause happened). + this._start = prevStart; //if the pause was at an earlier time and the user resumed in the callback, it could reposition the timeline (changing its startTime), throwing things off slightly, so we make sure the _start doesn't shift. + _setEnd(this); + return this.render(totalTime, suppressEvents, force); + } + } + this._onUpdate && !suppressEvents && _callback(this, "onUpdate", true); + if ((tTime === tDur && this._tTime >= this.totalDuration()) || (!tTime && prevTime)) if (prevStart === this._start || Math.abs(timeScale) !== Math.abs(this._ts)) if (!this._lock) { // remember, a child's callback may alter this timeline's playhead or timeScale which is why we need to add some of these checks. + (totalTime || !dur) && ((tTime === tDur && this._ts > 0) || (!tTime && this._ts < 0)) && _removeFromParent(this, 1); // don't remove if the timeline is reversed and the playhead isn't at 0, otherwise tl.progress(1).reverse() won't work. Only remove if the playhead is at the end and timeScale is positive, or if the playhead is at 0 and the timeScale is negative. + if (!suppressEvents && !(totalTime < 0 && !prevTime) && (tTime || prevTime || !tDur)) { + _callback(this, (tTime === tDur && totalTime >= 0 ? "onComplete" : "onReverseComplete"), true); + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + } + return this; + } + + add(child, position) { + _isNumber(position) || (position = _parsePosition(this, position, child)); + if (!(child instanceof Animation)) { + if (_isArray(child)) { + child.forEach(obj => this.add(obj, position)); + return this; + } + if (_isString(child)) { + return this.addLabel(child, position); + } + if (_isFunction(child)) { + child = Tween.delayedCall(0, child); + } else { + return this; + } + } + return this !== child ? _addToTimeline(this, child, position) : this; //don't allow a timeline to be added to itself as a child! + } + + getChildren(nested = true, tweens = true, timelines = true, ignoreBeforeTime = -_bigNum) { + let a = [], + child = this._first; + while (child) { + if (child._start >= ignoreBeforeTime) { + if (child instanceof Tween) { + tweens && a.push(child); + } else { + timelines && a.push(child); + nested && a.push(...child.getChildren(true, tweens, timelines)); + } + } + child = child._next; + } + return a; + } + + getById(id) { + let animations = this.getChildren(1, 1, 1), + i = animations.length; + while(i--) { + if (animations[i].vars.id === id) { + return animations[i]; + } + } + } + + remove(child) { + if (_isString(child)) { + return this.removeLabel(child); + } + if (_isFunction(child)) { + return this.killTweensOf(child); + } + child.parent === this && _removeLinkedListItem(this, child); + if (child === this._recent) { + this._recent = this._last; + } + return _uncache(this); + } + + totalTime(totalTime, suppressEvents) { + if (!arguments.length) { + return this._tTime; + } + this._forcing = 1; + if (!this._dp && this._ts) { //special case for the global timeline (or any other that has no parent or detached parent). + this._start = _roundPrecise(_ticker.time - (this._ts > 0 ? totalTime / this._ts : (this.totalDuration() - totalTime) / -this._ts)); + } + super.totalTime(totalTime, suppressEvents); + this._forcing = 0; + return this; + } + + addLabel(label, position) { + this.labels[label] = _parsePosition(this, position); + return this; + } + + removeLabel(label) { + delete this.labels[label]; + return this; + } + + addPause(position, callback, params) { + let t = Tween.delayedCall(0, callback || _emptyFunc, params); + t.data = "isPause"; + this._hasPause = 1; + return _addToTimeline(this, t, _parsePosition(this, position)); + } + + removePause(position) { + let child = this._first; + position = _parsePosition(this, position); + while (child) { + if (child._start === position && child.data === "isPause") { + _removeFromParent(child); + } + child = child._next; + } + } + + killTweensOf(targets, props, onlyActive) { + let tweens = this.getTweensOf(targets, onlyActive), + i = tweens.length; + while (i--) { + (_overwritingTween !== tweens[i]) && tweens[i].kill(targets, props); + } + return this; + } + + getTweensOf(targets, onlyActive) { + let a = [], + parsedTargets = toArray(targets), + child = this._first, + isGlobalTime = _isNumber(onlyActive), // a number is interpreted as a global time. If the animation spans + children; + while (child) { + if (child instanceof Tween) { + if (_arrayContainsAny(child._targets, parsedTargets) && (isGlobalTime ? (!_overwritingTween || (child._initted && child._ts)) && child.globalTime(0) <= onlyActive && child.globalTime(child.totalDuration()) > onlyActive : !onlyActive || child.isActive())) { // note: if this is for overwriting, it should only be for tweens that aren't paused and are initted. + a.push(child); + } + } else if ((children = child.getTweensOf(parsedTargets, onlyActive)).length) { + a.push(...children); + } + child = child._next; + } + return a; + } + + // potential future feature - targets() on timelines + // targets() { + // let result = []; + // this.getChildren(true, true, false).forEach(t => result.push(...t.targets())); + // return result.filter((v, i) => result.indexOf(v) === i); + // } + + tweenTo(position, vars) { + vars = vars || {}; + let tl = this, + endTime = _parsePosition(tl, position), + { startAt, onStart, onStartParams, immediateRender } = vars, + initted, + tween = Tween.to(tl, _setDefaults({ + ease: vars.ease || "none", + lazy: false, + immediateRender: false, + time: endTime, + overwrite: "auto", + duration: vars.duration || (Math.abs((endTime - ((startAt && "time" in startAt) ? startAt.time : tl._time)) / tl.timeScale())) || _tinyNum, + onStart: () => { + tl.pause(); + if (!initted) { + let duration = vars.duration || Math.abs((endTime - ((startAt && "time" in startAt) ? startAt.time : tl._time)) / tl.timeScale()); + (tween._dur !== duration) && _setDuration(tween, duration, 0, 1).render(tween._time, true, true); + initted = 1; + } + onStart && onStart.apply(tween, onStartParams || []); //in case the user had an onStart in the vars - we don't want to overwrite it. + } + }, vars)); + return immediateRender ? tween.render(0) : tween; + } + + tweenFromTo(fromPosition, toPosition, vars) { + return this.tweenTo(toPosition, _setDefaults({startAt:{time:_parsePosition(this, fromPosition)}}, vars)); + } + + recent() { + return this._recent; + } + + nextLabel(afterTime = this._time) { + return _getLabelInDirection(this, _parsePosition(this, afterTime)); + } + + previousLabel(beforeTime = this._time) { + return _getLabelInDirection(this, _parsePosition(this, beforeTime), 1); + } + + currentLabel(value) { + return arguments.length ? this.seek(value, true) : this.previousLabel(this._time + _tinyNum); + } + + shiftChildren(amount, adjustLabels, ignoreBeforeTime = 0) { + let child = this._first, + labels = this.labels, + p; + while (child) { + if (child._start >= ignoreBeforeTime) { + child._start += amount; + child._end += amount; + } + child = child._next; + } + if (adjustLabels) { + for (p in labels) { + if (labels[p] >= ignoreBeforeTime) { + labels[p] += amount; + } + } + } + return _uncache(this); + } + + invalidate(soft) { + let child = this._first; + this._lock = 0; + while (child) { + child.invalidate(soft); + child = child._next; + } + return super.invalidate(soft); + } + + clear(includeLabels = true) { + let child = this._first, + next; + while (child) { + next = child._next; + this.remove(child); + child = next; + } + this._dp && (this._time = this._tTime = this._pTime = 0); + includeLabels && (this.labels = {}); + return _uncache(this); + } + + totalDuration(value) { + let max = 0, + self = this, + child = self._last, + prevStart = _bigNum, + prev, start, parent; + if (arguments.length) { + return self.timeScale((self._repeat < 0 ? self.duration() : self.totalDuration()) / (self.reversed() ? -value : value)); + } + if (self._dirty) { + parent = self.parent; + while (child) { + prev = child._prev; //record it here in case the tween changes position in the sequence... + child._dirty && child.totalDuration(); //could change the tween._startTime, so make sure the animation's cache is clean before analyzing it. + start = child._start; + if (start > prevStart && self._sort && child._ts && !self._lock) { //in case one of the tweens shifted out of order, it needs to be re-inserted into the correct position in the sequence + self._lock = 1; //prevent endless recursive calls - there are methods that get triggered that check duration/totalDuration when we add(). + _addToTimeline(self, child, start - child._delay, 1)._lock = 0; + } else { + prevStart = start; + } + if (start < 0 && child._ts) { //children aren't allowed to have negative startTimes unless smoothChildTiming is true, so adjust here if one is found. + max -= start; + if ((!parent && !self._dp) || (parent && parent.smoothChildTiming)) { + self._start += start / self._ts; + self._time -= start; + self._tTime -= start; + } + self.shiftChildren(-start, false, -1e999); + prevStart = 0; + } + child._end > max && child._ts && (max = child._end); + child = prev; + } + _setDuration(self, (self === _globalTimeline && self._time > max) ? self._time : max, 1, 1); + self._dirty = 0; + } + return self._tDur; + } + + static updateRoot(time) { + if (_globalTimeline._ts) { + _lazySafeRender(_globalTimeline, _parentToChildTotalTime(time, _globalTimeline)); + _lastRenderedFrame = _ticker.frame; + } + if (_ticker.frame >= _nextGCFrame) { + _nextGCFrame += _config.autoSleep || 120; + let child = _globalTimeline._first; + if (!child || !child._ts) if (_config.autoSleep && _ticker._listeners.length < 2) { + while (child && !child._ts) { + child = child._next; + } + child || _ticker.sleep(); + } + } + } + +} + +_setDefaults(Timeline.prototype, {_lock:0, _hasPause:0, _forcing:0}); + + + + + + + + + + + + + + + + + + + + +let _addComplexStringPropTween = function(target, prop, start, end, setter, stringFilter, funcParam) { //note: we call _addComplexStringPropTween.call(tweenInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus "this" would refer to the plugin. + let pt = new PropTween(this._pt, target, prop, 0, 1, _renderComplexString, null, setter), + index = 0, + matchIndex = 0, + result, startNums, color, endNum, chunk, startNum, hasRandom, a; + pt.b = start; + pt.e = end; + start += ""; //ensure values are strings + end += ""; + if ((hasRandom = ~end.indexOf("random("))) { + end = _replaceRandom(end); + } + if (stringFilter) { + a = [start, end]; + stringFilter(a, target, prop); //pass an array with the starting and ending values and let the filter do whatever it needs to the values. + start = a[0]; + end = a[1]; + } + startNums = start.match(_complexStringNumExp) || []; + while ((result = _complexStringNumExp.exec(end))) { + endNum = result[0]; + chunk = end.substring(index, result.index); + if (color) { + color = (color + 1) % 5; + } else if (chunk.substr(-5) === "rgba(") { + color = 1; + } + if (endNum !== startNums[matchIndex++]) { + startNum = parseFloat(startNums[matchIndex-1]) || 0; + //these nested PropTweens are handled in a special way - we'll never actually call a render or setter method on them. We'll just loop through them in the parent complex string PropTween's render method. + pt._pt = { + _next: pt._pt, + p: (chunk || matchIndex === 1) ? chunk : ",", //note: SVG spec allows omission of comma/space when a negative sign is wedged between two numbers, like 2.5-5.3 instead of 2.5,-5.3 but when tweening, the negative value may switch to positive, so we insert the comma just in case. + s: startNum, + c: endNum.charAt(1) === "=" ? _parseRelative(startNum, endNum) - startNum : parseFloat(endNum) - startNum, + m: (color && color < 4) ? Math.round : 0 + }; + index = _complexStringNumExp.lastIndex; + } + } + pt.c = (index < end.length) ? end.substring(index, end.length) : ""; //we use the "c" of the PropTween to store the final part of the string (after the last number) + pt.fp = funcParam; + if (_relExp.test(end) || hasRandom) { + pt.e = 0; //if the end string contains relative values or dynamic random(...) values, delete the end it so that on the final render we don't actually set it to the string with += or -= characters (forces it to use the calculated value). + } + this._pt = pt; //start the linked list with this new PropTween. Remember, we call _addComplexStringPropTween.call(tweenInstance...) to ensure that it's scoped properly. We may call it from within a plugin too, thus "this" would refer to the plugin. + return pt; + }, + _addPropTween = function(target, prop, start, end, index, targets, modifier, stringFilter, funcParam, optional) { + _isFunction(end) && (end = end(index || 0, target, targets)); + let currentValue = target[prop], + parsedStart = (start !== "get") ? start : !_isFunction(currentValue) ? currentValue : (funcParam ? target[(prop.indexOf("set") || !_isFunction(target["get" + prop.substr(3)])) ? prop : "get" + prop.substr(3)](funcParam) : target[prop]()), + setter = !_isFunction(currentValue) ? _setterPlain : funcParam ? _setterFuncWithParam : _setterFunc, + pt; + if (_isString(end)) { + if (~end.indexOf("random(")) { + end = _replaceRandom(end); + } + if (end.charAt(1) === "=") { + pt = _parseRelative(parsedStart, end) + (getUnit(parsedStart) || 0); + if (pt || pt === 0) { // to avoid isNaN, like if someone passes in a value like "!= whatever" + end = pt; + } + } + } + if (!optional || parsedStart !== end || _forceAllPropTweens) { + if (!isNaN(parsedStart * end) && end !== "") { // fun fact: any number multiplied by "" is evaluated as the number 0! + pt = new PropTween(this._pt, target, prop, +parsedStart || 0, end - (parsedStart || 0), typeof(currentValue) === "boolean" ? _renderBoolean : _renderPlain, 0, setter); + funcParam && (pt.fp = funcParam); + modifier && pt.modifier(modifier, this, target); + return (this._pt = pt); + } + !currentValue && !(prop in target) && _missingPlugin(prop, end); + return _addComplexStringPropTween.call(this, target, prop, parsedStart, end, setter, stringFilter || _config.stringFilter, funcParam); + } + }, + //creates a copy of the vars object and processes any function-based values (putting the resulting values directly into the copy) as well as strings with "random()" in them. It does NOT process relative values. + _processVars = (vars, index, target, targets, tween) => { + _isFunction(vars) && (vars = _parseFuncOrString(vars, tween, index, target, targets)); + if (!_isObject(vars) || (vars.style && vars.nodeType) || _isArray(vars) || _isTypedArray(vars)) { + return _isString(vars) ? _parseFuncOrString(vars, tween, index, target, targets) : vars; + } + let copy = {}, + p; + for (p in vars) { + copy[p] = _parseFuncOrString(vars[p], tween, index, target, targets); + } + return copy; + }, + _checkPlugin = (property, vars, tween, index, target, targets) => { + let plugin, pt, ptLookup, i; + if (_plugins[property] && (plugin = new _plugins[property]()).init(target, plugin.rawVars ? vars[property] : _processVars(vars[property], index, target, targets, tween), tween, index, targets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, property, 0, 1, plugin.render, plugin, 0, plugin.priority); + if (tween !== _quickTween) { + ptLookup = tween._ptLookup[tween._targets.indexOf(target)]; //note: we can't use tween._ptLookup[index] because for staggered tweens, the index from the fullTargets array won't match what it is in each individual tween that spawns from the stagger. + i = plugin._props.length; + while (i--) { + ptLookup[plugin._props[i]] = pt; + } + } + } + return plugin; + }, + _overwritingTween, //store a reference temporarily so we can avoid overwriting itself. + _forceAllPropTweens, + _initTween = (tween, time, tTime) => { + let vars = tween.vars, + { ease, startAt, immediateRender, lazy, onUpdate, runBackwards, yoyoEase, keyframes, autoRevert } = vars, + dur = tween._dur, + prevStartAt = tween._startAt, + targets = tween._targets, + parent = tween.parent, + //when a stagger (or function-based duration/delay) is on a Tween instance, we create a nested timeline which means that the "targets" of that tween don't reflect the parent. This function allows us to discern when it's a nested tween and in that case, return the full targets array so that function-based values get calculated properly. Also remember that if the tween has a stagger AND keyframes, it could be multiple levels deep which is why we store the targets Array in the vars of the timeline. + fullTargets = (parent && parent.data === "nested") ? parent.vars.targets : targets, + autoOverwrite = (tween._overwrite === "auto") && !_suppressOverwrites, + tl = tween.timeline, + cleanVars, i, p, pt, target, hasPriority, gsData, harness, plugin, ptLookup, index, harnessVars, overwritten; + tl && (!keyframes || !ease) && (ease = "none"); + tween._ease = _parseEase(ease, _defaults.ease); + tween._yEase = yoyoEase ? _invertEase(_parseEase(yoyoEase === true ? ease : yoyoEase, _defaults.ease)) : 0; + if (yoyoEase && tween._yoyo && !tween._repeat) { //there must have been a parent timeline with yoyo:true that is currently in its yoyo phase, so flip the eases. + yoyoEase = tween._yEase; + tween._yEase = tween._ease; + tween._ease = yoyoEase; + } + tween._from = !tl && !!vars.runBackwards; //nested timelines should never run backwards - the backwards-ness is in the child tweens. + if (!tl || (keyframes && !vars.stagger)) { //if there's an internal timeline, skip all the parsing because we passed that task down the chain. + harness = targets[0] ? _getCache(targets[0]).harness : 0; + harnessVars = harness && vars[harness.prop]; //someone may need to specify CSS-specific values AND non-CSS values, like if the element has an "x" property plus it's a standard DOM element. We allow people to distinguish by wrapping plugin-specific stuff in a css:{} object for example. + cleanVars = _copyExcluding(vars, _reservedProps); + if (prevStartAt) { + prevStartAt._zTime < 0 && prevStartAt.progress(1); // in case it's a lazy startAt that hasn't rendered yet. + (time < 0 && runBackwards && immediateRender && !autoRevert) ? prevStartAt.render(-1, true) : prevStartAt.revert(runBackwards && dur ? _revertConfigNoKill : _startAtRevertConfig); // if it's a "startAt" (not "from()" or runBackwards: true), we only need to do a shallow revert (keep transforms cached in CSSPlugin) + // don't just _removeFromParent(prevStartAt.render(-1, true)) because that'll leave inline styles. We're creating a new _startAt for "startAt" tweens that re-capture things to ensure that if the pre-tween values changed since the tween was created, they're recorded. + prevStartAt._lazy = 0; + } + if (startAt) { + _removeFromParent(tween._startAt = Tween.set(targets, _setDefaults({data: "isStart", overwrite: false, parent: parent, immediateRender: true, lazy: !prevStartAt && _isNotFalse(lazy), startAt: null, delay: 0, onUpdate: onUpdate && (() => _callback(tween, "onUpdate")), stagger: 0}, startAt))); //copy the properties/values into a new object to avoid collisions, like var to = {x:0}, from = {x:500}; timeline.fromTo(e, from, to).fromTo(e, to, from); + tween._startAt._dp = 0; // don't allow it to get put back into root timeline! Like when revert() is called and totalTime() gets set. + tween._startAt._sat = tween; // used in globalTime(). _sat stands for _startAtTween + (time < 0 && (_reverting || (!immediateRender && !autoRevert))) && tween._startAt.revert(_revertConfigNoKill); // rare edge case, like if a render is forced in the negative direction of a non-initted tween. + if (immediateRender) { + if (dur && time <= 0 && tTime <= 0) { // check tTime here because in the case of a yoyo tween whose playhead gets pushed to the end like tween.progress(1), we should allow it through so that the onComplete gets fired properly. + time && (tween._zTime = time); + return; //we skip initialization here so that overwriting doesn't occur until the tween actually begins. Otherwise, if you create several immediateRender:true tweens of the same target/properties to drop into a Timeline, the last one created would overwrite the first ones because they didn't get placed into the timeline yet before the first render occurs and kicks in overwriting. + } + } + } else if (runBackwards && dur) { + //from() tweens must be handled uniquely: their beginning values must be rendered but we don't want overwriting to occur yet (when time is still 0). Wait until the tween actually begins before doing all the routines like overwriting. At that time, we should render at the END of the tween to ensure that things initialize correctly (remember, from() tweens go backwards) + if (!prevStartAt) { + time && (immediateRender = false); //in rare cases (like if a from() tween runs and then is invalidate()-ed), immediateRender could be true but the initial forced-render gets skipped, so there's no need to force the render in this context when the _time is greater than 0 + p = _setDefaults({ + overwrite: false, + data: "isFromStart", //we tag the tween with as "isFromStart" so that if [inside a plugin] we need to only do something at the very END of a tween, we have a way of identifying this tween as merely the one that's setting the beginning values for a "from()" tween. For example, clearProps in CSSPlugin should only get applied at the very END of a tween and without this tag, from(...{height:100, clearProps:"height", delay:1}) would wipe the height at the beginning of the tween and after 1 second, it'd kick back in. + lazy: immediateRender && !prevStartAt && _isNotFalse(lazy), + immediateRender: immediateRender, //zero-duration tweens render immediately by default, but if we're not specifically instructed to render this tween immediately, we should skip this and merely _init() to record the starting values (rendering them immediately would push them to completion which is wasteful in that case - we'd have to render(-1) immediately after) + stagger: 0, + parent: parent //ensures that nested tweens that had a stagger are handled properly, like gsap.from(".class", {y: gsap.utils.wrap([-100,100]), stagger: 0.5}) + }, cleanVars); + harnessVars && (p[harness.prop] = harnessVars); // in case someone does something like .from(..., {css:{}}) + _removeFromParent(tween._startAt = Tween.set(targets, p)); + tween._startAt._dp = 0; // don't allow it to get put back into root timeline! + tween._startAt._sat = tween; // used in globalTime() + (time < 0) && (_reverting ? tween._startAt.revert(_revertConfigNoKill) : tween._startAt.render(-1, true)); + tween._zTime = time; + if (!immediateRender) { + _initTween(tween._startAt, _tinyNum, _tinyNum); //ensures that the initial values are recorded + } else if (!time) { + return; + } + } + } + tween._pt = tween._ptCache = 0; + lazy = (dur && _isNotFalse(lazy)) || (lazy && !dur); + for (i = 0; i < targets.length; i++) { + target = targets[i]; + gsData = target._gsap || _harness(targets)[i]._gsap; + tween._ptLookup[i] = ptLookup = {}; + _lazyLookup[gsData.id] && _lazyTweens.length && _lazyRender(); //if other tweens of the same target have recently initted but haven't rendered yet, we've got to force the render so that the starting values are correct (imagine populating a timeline with a bunch of sequential tweens and then jumping to the end) + index = fullTargets === targets ? i : fullTargets.indexOf(target); + if (harness && (plugin = new harness()).init(target, harnessVars || cleanVars, tween, index, fullTargets) !== false) { + tween._pt = pt = new PropTween(tween._pt, target, plugin.name, 0, 1, plugin.render, plugin, 0, plugin.priority); + plugin._props.forEach(name => {ptLookup[name] = pt;}); + plugin.priority && (hasPriority = 1); + } + if (!harness || harnessVars) { + for (p in cleanVars) { + if (_plugins[p] && (plugin = _checkPlugin(p, cleanVars, tween, index, target, fullTargets))) { + plugin.priority && (hasPriority = 1); + } else { + ptLookup[p] = pt = _addPropTween.call(tween, target, p, "get", cleanVars[p], index, fullTargets, 0, vars.stringFilter); + } + } + } + tween._op && tween._op[i] && tween.kill(target, tween._op[i]); + if (autoOverwrite && tween._pt) { + _overwritingTween = tween; + _globalTimeline.killTweensOf(target, ptLookup, tween.globalTime(time)); // make sure the overwriting doesn't overwrite THIS tween!!! + overwritten = !tween.parent; + _overwritingTween = 0; + } + tween._pt && lazy && (_lazyLookup[gsData.id] = 1); + } + hasPriority && _sortPropTweensByPriority(tween); + tween._onInit && tween._onInit(tween); //plugins like RoundProps must wait until ALL of the PropTweens are instantiated. In the plugin's init() function, it sets the _onInit on the tween instance. May not be pretty/intuitive, but it's fast and keeps file size down. + } + tween._onUpdate = onUpdate; + tween._initted = (!tween._op || tween._pt) && !overwritten; // if overwrittenProps resulted in the entire tween being killed, do NOT flag it as initted or else it may render for one tick. + (keyframes && time <= 0) && tl.render(_bigNum, true, true); // if there's a 0% keyframe, it'll render in the "before" state for any staggered/delayed animations thus when the following tween initializes, it'll use the "before" state instead of the "after" state as the initial values. + }, + _updatePropTweens = (tween, property, value, start, startIsRelative, ratio, time, skipRecursion) => { + let ptCache = ((tween._pt && tween._ptCache) || (tween._ptCache = {}))[property], + pt, rootPT, lookup, i; + if (!ptCache) { + ptCache = tween._ptCache[property] = []; + lookup = tween._ptLookup; + i = tween._targets.length; + while (i--) { + pt = lookup[i][property]; + if (pt && pt.d && pt.d._pt) { // it's a plugin, so find the nested PropTween + pt = pt.d._pt; + while (pt && pt.p !== property && pt.fp !== property) { // "fp" is functionParam for things like setting CSS variables which require .setProperty("--var-name", value) + pt = pt._next; + } + } + if (!pt) { // there is no PropTween associated with that property, so we must FORCE one to be created and ditch out of this + // if the tween has other properties that already rendered at new positions, we'd normally have to rewind to put them back like tween.render(0, true) before forcing an _initTween(), but that can create another edge case like tweening a timeline's progress would trigger onUpdates to fire which could move other things around. It's better to just inform users that .resetTo() should ONLY be used for tweens that already have that property. For example, you can't gsap.to(...{ y: 0 }) and then tween.restTo("x", 200) for example. + _forceAllPropTweens = 1; // otherwise, when we _addPropTween() and it finds no change between the start and end values, it skips creating a PropTween (for efficiency...why tween when there's no difference?) but in this case we NEED that PropTween created so we can edit it. + tween.vars[property] = "+=0"; + _initTween(tween, time); + _forceAllPropTweens = 0; + return skipRecursion ? _warn(property + " not eligible for reset") : 1; // if someone tries to do a quickTo() on a special property like borderRadius which must get split into 4 different properties, that's not eligible for .resetTo(). + } + ptCache.push(pt); + } + } + i = ptCache.length; + while (i--) { + rootPT = ptCache[i]; + pt = rootPT._pt || rootPT; // complex values may have nested PropTweens. We only accommodate the FIRST value. + pt.s = (start || start === 0) && !startIsRelative ? start : pt.s + (start || 0) + ratio * pt.c; + pt.c = value - pt.s; + rootPT.e && (rootPT.e = _round(value) + getUnit(rootPT.e)); // mainly for CSSPlugin (end value) + rootPT.b && (rootPT.b = pt.s + getUnit(rootPT.b)); // (beginning value) + } + }, + _addAliasesToVars = (targets, vars) => { + let harness = targets[0] ? _getCache(targets[0]).harness : 0, + propertyAliases = (harness && harness.aliases), + copy, p, i, aliases; + if (!propertyAliases) { + return vars; + } + copy = _merge({}, vars); + for (p in propertyAliases) { + if (p in copy) { + aliases = propertyAliases[p].split(","); + i = aliases.length; + while(i--) { + copy[aliases[i]] = copy[p]; + } + } + + } + return copy; + }, + // parses multiple formats, like {"0%": {x: 100}, {"50%": {x: -20}} and { x: {"0%": 100, "50%": -20} }, and an "ease" can be set on any object. We populate an "allProps" object with an Array for each property, like {x: [{}, {}], y:[{}, {}]} with data for each property tween. The objects have a "t" (time), "v", (value), and "e" (ease) property. This allows us to piece together a timeline later. + _parseKeyframe = (prop, obj, allProps, easeEach) => { + let ease = obj.ease || easeEach || "power1.inOut", + p, a; + if (_isArray(obj)) { + a = allProps[prop] || (allProps[prop] = []); + // t = time (out of 100), v = value, e = ease + obj.forEach((value, i) => a.push({t: i / (obj.length - 1) * 100, v: value, e: ease})); + } else { + for (p in obj) { + a = allProps[p] || (allProps[p] = []); + p === "ease" || a.push({t: parseFloat(prop), v: obj[p], e: ease}); + } + } + }, + _parseFuncOrString = (value, tween, i, target, targets) => (_isFunction(value) ? value.call(tween, i, target, targets) : (_isString(value) && ~value.indexOf("random(")) ? _replaceRandom(value) : value), + _staggerTweenProps = _callbackNames + "repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase,autoRevert", + _staggerPropsToSkip = {}; +_forEachName(_staggerTweenProps + ",id,stagger,delay,duration,paused,scrollTrigger", name => _staggerPropsToSkip[name] = 1); + + + + + + + + + + + + + + + + + + + + + + + +/* + * -------------------------------------------------------------------------------------- + * TWEEN + * -------------------------------------------------------------------------------------- + */ + +export class Tween extends Animation { + + constructor(targets, vars, position, skipInherit) { + if (typeof(vars) === "number") { + position.duration = vars; + vars = position; + position = null; + } + super(skipInherit ? vars : _inheritDefaults(vars)); + let { duration, delay, immediateRender, stagger, overwrite, keyframes, defaults, scrollTrigger, yoyoEase } = this.vars, + parent = vars.parent || _globalTimeline, + parsedTargets = (_isArray(targets) || _isTypedArray(targets) ? _isNumber(targets[0]) : ("length" in vars)) ? [targets] : toArray(targets), // edge case: someone might try animating the "length" of an object with a "length" property that's initially set to 0 so don't interpret that as an empty Array-like object. + tl, i, copy, l, p, curTarget, staggerFunc, staggerVarsToMerge; + this._targets = parsedTargets.length ? _harness(parsedTargets) : _warn("GSAP target " + targets + " not found. https://gsap.com", !_config.nullTargetWarn) || []; + this._ptLookup = []; //PropTween lookup. An array containing an object for each target, having keys for each tweening property + this._overwrite = overwrite; + if (keyframes || stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + vars = this.vars; + tl = this.timeline = new Timeline({data: "nested", defaults: defaults || {}, targets: parent && parent.data === "nested" ? parent.vars.targets : parsedTargets}); // we need to store the targets because for staggers and keyframes, we end up creating an individual tween for each but function-based values need to know the index and the whole Array of targets. + tl.kill(); + tl.parent = tl._dp = this; + tl._start = 0; + if (stagger || _isFuncOrString(duration) || _isFuncOrString(delay)) { + l = parsedTargets.length; + staggerFunc = stagger && distribute(stagger); + if (_isObject(stagger)) { //users can pass in callbacks like onStart/onComplete in the stagger object. These should fire with each individual tween. + for (p in stagger) { + if (~_staggerTweenProps.indexOf(p)) { + staggerVarsToMerge || (staggerVarsToMerge = {}); + staggerVarsToMerge[p] = stagger[p]; + } + } + } + for (i = 0; i < l; i++) { + copy = _copyExcluding(vars, _staggerPropsToSkip); + copy.stagger = 0; + yoyoEase && (copy.yoyoEase = yoyoEase); + staggerVarsToMerge && _merge(copy, staggerVarsToMerge); + curTarget = parsedTargets[i]; + //don't just copy duration or delay because if they're a string or function, we'd end up in an infinite loop because _isFuncOrString() would evaluate as true in the child tweens, entering this loop, etc. So we parse the value straight from vars and default to 0. + copy.duration = +_parseFuncOrString(duration, this, i, curTarget, parsedTargets); + copy.delay = (+_parseFuncOrString(delay, this, i, curTarget, parsedTargets) || 0) - this._delay; + if (!stagger && l === 1 && copy.delay) { // if someone does delay:"random(1, 5)", repeat:-1, for example, the delay shouldn't be inside the repeat. + this._delay = delay = copy.delay; + this._start += delay; + copy.delay = 0; + } + tl.to(curTarget, copy, staggerFunc ? staggerFunc(i, curTarget, parsedTargets) : 0); + tl._ease = _easeMap.none; + } + tl.duration() ? (duration = delay = 0) : (this.timeline = 0); // if the timeline's duration is 0, we don't need a timeline internally! + } else if (keyframes) { + _inheritDefaults(_setDefaults(tl.vars.defaults, {ease:"none"})); + tl._ease = _parseEase(keyframes.ease || vars.ease || "none"); + let time = 0, + a, kf, v; + if (_isArray(keyframes)) { + keyframes.forEach(frame => tl.to(parsedTargets, frame, ">")); + tl.duration(); // to ensure tl._dur is cached because we tap into it for performance purposes in the render() method. + } else { + copy = {}; + for (p in keyframes) { + p === "ease" || p === "easeEach" || _parseKeyframe(p, keyframes[p], copy, keyframes.easeEach); + } + for (p in copy) { + a = copy[p].sort((a, b) => a.t - b.t); + time = 0; + for (i = 0; i < a.length; i++) { + kf = a[i]; + v = {ease: kf.e, duration: (kf.t - (i ? a[i - 1].t : 0)) / 100 * duration}; + v[p] = kf.v; + tl.to(parsedTargets, v, time); + time += v.duration; + } + } + tl.duration() < duration && tl.to({}, {duration: duration - tl.duration()}); // in case keyframes didn't go to 100% + } + } + duration || this.duration((duration = tl.duration())); + + } else { + this.timeline = 0; //speed optimization, faster lookups (no going up the prototype chain) + } + + if (overwrite === true && !_suppressOverwrites) { + _overwritingTween = this; + _globalTimeline.killTweensOf(parsedTargets); + _overwritingTween = 0; + } + _addToTimeline(parent, this, position); + vars.reversed && this.reverse(); + vars.paused && this.paused(true); + if (immediateRender || (!duration && !keyframes && this._start === _roundPrecise(parent._time) && _isNotFalse(immediateRender) && _hasNoPausedAncestors(this) && parent.data !== "nested")) { + this._tTime = -_tinyNum; //forces a render without having to set the render() "force" parameter to true because we want to allow lazying by default (using the "force" parameter always forces an immediate full render) + this.render(Math.max(0, -delay) || 0); //in case delay is negative + } + scrollTrigger && _scrollTrigger(this, scrollTrigger); + } + + render(totalTime, suppressEvents, force) { + let prevTime = this._time, + tDur = this._tDur, + dur = this._dur, + isNegative = totalTime < 0, + tTime = (totalTime > tDur - _tinyNum && !isNegative) ? tDur : (totalTime < _tinyNum) ? 0 : totalTime, + time, pt, iteration, cycleDuration, prevIteration, isYoyo, ratio, timeline, yoyoEase; + if (!dur) { + _renderZeroDurationTween(this, totalTime, suppressEvents, force); + } else if (tTime !== this._tTime || !totalTime || force || (!this._initted && this._tTime) || (this._startAt && (this._zTime < 0) !== isNegative) || this._lazy) { // this senses if we're crossing over the start time, in which case we must record _zTime and force the render, but we do it in this lengthy conditional way for performance reasons (usually we can skip the calculations): this._initted && (this._zTime < 0) !== (totalTime < 0) + time = tTime; + timeline = this.timeline; + if (this._repeat) { //adjust the time for repeats and yoyos + cycleDuration = dur + this._rDelay; + if (this._repeat < -1 && isNegative) { + return this.totalTime(cycleDuration * 100 + totalTime, suppressEvents, force); + } + time = _roundPrecise(tTime % cycleDuration); //round to avoid floating point errors. (4 % 0.8 should be 0 but some browsers report it as 0.79999999!) + if (tTime === tDur) { // the tDur === tTime is for edge cases where there's a lengthy decimal on the duration and it may reach the very end but the time is rendered as not-quite-there (remember, tDur is rounded to 4 decimals whereas dur isn't) + iteration = this._repeat; + time = dur; + } else { + prevIteration = _roundPrecise(tTime / cycleDuration); // full decimal version of iterations, not the previous iteration (we're reusing prevIteration variable for efficiency) + iteration = ~~prevIteration; + if (iteration && iteration === prevIteration) { + time = dur; + iteration--; + } else if (time > dur) { + time = dur; + } + } + isYoyo = this._yoyo && (iteration & 1); + if (isYoyo) { + yoyoEase = this._yEase; + time = dur - time; + } + prevIteration = _animationCycle(this._tTime, cycleDuration); + if (time === prevTime && !force && this._initted && iteration === prevIteration) { + //could be during the repeatDelay part. No need to render and fire callbacks. + this._tTime = tTime; + return this; + } + if (iteration !== prevIteration) { + timeline && this._yEase && _propagateYoyoEase(timeline, isYoyo); + //repeatRefresh functionality + if (this.vars.repeatRefresh && !isYoyo && !this._lock && time !== cycleDuration && this._initted) { // this._time will === cycleDuration when we render at EXACTLY the end of an iteration. Without this condition, it'd often do the repeatRefresh render TWICE (again on the very next tick). + this._lock = force = 1; //force, otherwise if lazy is true, the _attemptInitTween() will return and we'll jump out and get caught bouncing on each tick. + this.render(_roundPrecise(cycleDuration * iteration), true).invalidate()._lock = 0; + } + } + } + + if (!this._initted) { + if (_attemptInitTween(this, isNegative ? totalTime : time, force, suppressEvents, tTime)) { + this._tTime = 0; // in constructor if immediateRender is true, we set _tTime to -_tinyNum to have the playhead cross the starting point but we can't leave _tTime as a negative number. + return this; + } + if (prevTime !== this._time && !(force && this.vars.repeatRefresh && iteration !== prevIteration)) { // rare edge case - during initialization, an onUpdate in the _startAt (.fromTo()) might force this tween to render at a different spot in which case we should ditch this render() call so that it doesn't revert the values. But we also don't want to dump if we're doing a repeatRefresh render! + return this; + } + if (dur !== this._dur) { // while initting, a plugin like InertiaPlugin might alter the duration, so rerun from the start to ensure everything renders as it should. + return this.render(totalTime, suppressEvents, force); + } + } + + this._tTime = tTime; + this._time = time; + + if (!this._act && this._ts) { + this._act = 1; //as long as it's not paused, force it to be active so that if the user renders independent of the parent timeline, it'll be forced to re-render on the next tick. + this._lazy = 0; + } + + this.ratio = ratio = (yoyoEase || this._ease)(time / dur); + if (this._from) { + this.ratio = ratio = 1 - ratio; + } + + if (time && !prevTime && !suppressEvents && !iteration) { + _callback(this, "onStart"); + if (this._tTime !== tTime) { // in case the onStart triggered a render at a different spot, eject. Like if someone did animation.pause(0.5) or something inside the onStart. + return this; + } + } + pt = this._pt; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + (timeline && timeline.render(totalTime < 0 ? totalTime : timeline._dur * timeline._ease(time / this._dur), suppressEvents, force)) || (this._startAt && (this._zTime = totalTime)); + + if (this._onUpdate && !suppressEvents) { + isNegative && _rewindStartAt(this, totalTime, suppressEvents, force); //note: for performance reasons, we tuck this conditional logic inside less traveled areas (most tweens don't have an onUpdate). We'd just have it at the end before the onComplete, but the values should be updated before any onUpdate is called, so we ALSO put it here and then if it's not called, we do so later near the onComplete. + _callback(this, "onUpdate"); + } + + this._repeat && iteration !== prevIteration && this.vars.onRepeat && !suppressEvents && this.parent && _callback(this, "onRepeat"); + + if ((tTime === this._tDur || !tTime) && this._tTime === tTime) { + isNegative && !this._onUpdate && _rewindStartAt(this, totalTime, true, true); + (totalTime || !dur) && ((tTime === this._tDur && this._ts > 0) || (!tTime && this._ts < 0)) && _removeFromParent(this, 1); // don't remove if we're rendering at exactly a time of 0, as there could be autoRevert values that should get set on the next tick (if the playhead goes backward beyond the startTime, negative totalTime). Don't remove if the timeline is reversed and the playhead isn't at 0, otherwise tl.progress(1).reverse() won't work. Only remove if the playhead is at the end and timeScale is positive, or if the playhead is at 0 and the timeScale is negative. + if (!suppressEvents && !(isNegative && !prevTime) && (tTime || prevTime || isYoyo)) { // if prevTime and tTime are zero, we shouldn't fire the onReverseComplete. This could happen if you gsap.to(... {paused:true}).play(); + _callback(this, (tTime === tDur ? "onComplete" : "onReverseComplete"), true); + this._prom && !(tTime < tDur && this.timeScale() > 0) && this._prom(); + } + } + + } + return this; + } + + targets() { + return this._targets; + } + + invalidate(soft) { // "soft" gives us a way to clear out everything EXCEPT the recorded pre-"from" portion of from() tweens. Otherwise, for example, if you tween.progress(1).render(0, true true).invalidate(), the "from" values would persist and then on the next render, the from() tweens would initialize and the current value would match the "from" values, thus animate from the same value to the same value (no animation). We tap into this in ScrollTrigger's refresh() where we must push a tween to completion and then back again but honor its init state in case the tween is dependent on another tween further up on the page. + (!soft || !this.vars.runBackwards) && (this._startAt = 0) + this._pt = this._op = this._onUpdate = this._lazy = this.ratio = 0; + this._ptLookup = []; + this.timeline && this.timeline.invalidate(soft); + return super.invalidate(soft); + } + + resetTo(property, value, start, startIsRelative, skipRecursion) { + _tickerActive || _ticker.wake(); + this._ts || this.play(); + let time = Math.min(this._dur, (this._dp._time - this._start) * this._ts), + ratio; + this._initted || _initTween(this, time); + ratio = this._ease(time / this._dur); // don't just get tween.ratio because it may not have rendered yet. + // possible future addition to allow an object with multiple values to update, like tween.resetTo({x: 100, y: 200}); At this point, it doesn't seem worth the added kb given the fact that most users will likely opt for the convenient gsap.quickTo() way of interacting with this method. + // if (_isObject(property)) { // performance optimization + // for (p in property) { + // if (_updatePropTweens(this, p, property[p], value ? value[p] : null, start, ratio, time)) { + // return this.resetTo(property, value, start, startIsRelative); // if a PropTween wasn't found for the property, it'll get forced with a re-initialization so we need to jump out and start over again. + // } + // } + // } else { + if (_updatePropTweens(this, property, value, start, startIsRelative, ratio, time, skipRecursion)) { + return this.resetTo(property, value, start, startIsRelative, 1); // if a PropTween wasn't found for the property, it'll get forced with a re-initialization so we need to jump out and start over again. + } + //} + _alignPlayhead(this, 0); + this.parent || _addLinkedListItem(this._dp, this, "_first", "_last", this._dp._sort ? "_start" : 0); + return this.render(0); + } + + kill(targets, vars = "all") { + if (!targets && (!vars || vars === "all")) { + this._lazy = this._pt = 0; + this.parent ? _interrupt(this) : this.scrollTrigger && this.scrollTrigger.kill(!!_reverting); + return this; + } + if (this.timeline) { + let tDur = this.timeline.totalDuration(); + this.timeline.killTweensOf(targets, vars, _overwritingTween && _overwritingTween.vars.overwrite !== true)._first || _interrupt(this); // if nothing is left tweening, interrupt. + this.parent && tDur !== this.timeline.totalDuration() && _setDuration(this, this._dur * this.timeline._tDur / tDur, 0, 1); // if a nested tween is killed that changes the duration, it should affect this tween's duration. We must use the ratio, though, because sometimes the internal timeline is stretched like for keyframes where they don't all add up to whatever the parent tween's duration was set to. + return this; + } + let parsedTargets = this._targets, + killingTargets = targets ? toArray(targets) : parsedTargets, + propTweenLookup = this._ptLookup, + firstPT = this._pt, + overwrittenProps, curLookup, curOverwriteProps, props, p, pt, i; + if ((!vars || vars === "all") && _arraysMatch(parsedTargets, killingTargets)) { + vars === "all" && (this._pt = 0); + return _interrupt(this); + } + overwrittenProps = this._op = this._op || []; + if (vars !== "all") { //so people can pass in a comma-delimited list of property names + if (_isString(vars)) { + p = {}; + _forEachName(vars, name => p[name] = 1); + vars = p; + } + vars = _addAliasesToVars(parsedTargets, vars); + } + i = parsedTargets.length; + while (i--) { + if (~killingTargets.indexOf(parsedTargets[i])) { + curLookup = propTweenLookup[i]; + if (vars === "all") { + overwrittenProps[i] = vars; + props = curLookup; + curOverwriteProps = {}; + } else { + curOverwriteProps = overwrittenProps[i] = overwrittenProps[i] || {}; + props = vars; + } + for (p in props) { + pt = curLookup && curLookup[p]; + if (pt) { + if (!("kill" in pt.d) || pt.d.kill(p) === true) { + _removeLinkedListItem(this, pt, "_pt"); + } + delete curLookup[p]; + } + if (curOverwriteProps !== "all") { + curOverwriteProps[p] = 1; + } + } + } + } + this._initted && !this._pt && firstPT && _interrupt(this); //if all tweening properties are killed, kill the tween. Without this line, if there's a tween with multiple targets and then you killTweensOf() each target individually, the tween would technically still remain active and fire its onComplete even though there aren't any more properties tweening. + return this; + } + + + static to(targets, vars) { + return new Tween(targets, vars, arguments[2]); + } + + static from(targets, vars) { + return _createTweenType(1, arguments); + } + + static delayedCall(delay, callback, params, scope) { + return new Tween(callback, 0, {immediateRender:false, lazy:false, overwrite:false, delay:delay, onComplete:callback, onReverseComplete:callback, onCompleteParams:params, onReverseCompleteParams:params, callbackScope:scope}); // we must use onReverseComplete too for things like timeline.add(() => {...}) which should be triggered in BOTH directions (forward and reverse) + } + + static fromTo(targets, fromVars, toVars) { + return _createTweenType(2, arguments); + } + + static set(targets, vars) { + vars.duration = 0; + vars.repeatDelay || (vars.repeat = 0); + return new Tween(targets, vars); + } + + static killTweensOf(targets, props, onlyActive) { + return _globalTimeline.killTweensOf(targets, props, onlyActive); + } +} + +_setDefaults(Tween.prototype, {_targets:[], _lazy:0, _startAt:0, _op:0, _onInit:0}); + +//add the pertinent timeline methods to Tween instances so that users can chain conveniently and create a timeline automatically. (removed due to concerns that it'd ultimately add to more confusion especially for beginners) +// _forEachName("to,from,fromTo,set,call,add,addLabel,addPause", name => { +// Tween.prototype[name] = function() { +// let tl = new Timeline(); +// return _addToTimeline(tl, this)[name].apply(tl, toArray(arguments)); +// } +// }); + +//for backward compatibility. Leverage the timeline calls. +_forEachName("staggerTo,staggerFrom,staggerFromTo", name => { + Tween[name] = function() { + let tl = new Timeline(), + params = _slice.call(arguments, 0); + params.splice(name === "staggerFromTo" ? 5 : 4, 0, 0); + return tl[name].apply(tl, params); + } +}); + + + + + + + + + + + + + + + + +/* + * -------------------------------------------------------------------------------------- + * PROPTWEEN + * -------------------------------------------------------------------------------------- + */ +let _setterPlain = (target, property, value) => target[property] = value, + _setterFunc = (target, property, value) => target[property](value), + _setterFuncWithParam = (target, property, value, data) => target[property](data.fp, value), + _setterAttribute = (target, property, value) => target.setAttribute(property, value), + _getSetter = (target, property) => _isFunction(target[property]) ? _setterFunc : _isUndefined(target[property]) && target.setAttribute ? _setterAttribute : _setterPlain, + _renderPlain = (ratio, data) => data.set(data.t, data.p, Math.round((data.s + data.c * ratio) * 1000000) / 1000000, data), + _renderBoolean = (ratio, data) => data.set(data.t, data.p, !!(data.s + data.c * ratio), data), + _renderComplexString = function(ratio, data) { + let pt = data._pt, + s = ""; + if (!ratio && data.b) { //b = beginning string + s = data.b; + } else if (ratio === 1 && data.e) { //e = ending string + s = data.e; + } else { + while (pt) { + s = pt.p + (pt.m ? pt.m(pt.s + pt.c * ratio) : (Math.round((pt.s + pt.c * ratio) * 10000) / 10000)) + s; //we use the "p" property for the text inbetween (like a suffix). And in the context of a complex string, the modifier (m) is typically just Math.round(), like for RGB colors. + pt = pt._next; + } + s += data.c; //we use the "c" of the PropTween to store the final chunk of non-numeric text. + } + data.set(data.t, data.p, s, data); + }, + _renderPropTweens = function(ratio, data) { + let pt = data._pt; + while (pt) { + pt.r(ratio, pt.d); + pt = pt._next; + } + }, + _addPluginModifier = function(modifier, tween, target, property) { + let pt = this._pt, + next; + while (pt) { + next = pt._next; + pt.p === property && pt.modifier(modifier, tween, target); + pt = next; + } + }, + _killPropTweensOf = function(property) { + let pt = this._pt, + hasNonDependentRemaining, next; + while (pt) { + next = pt._next; + if ((pt.p === property && !pt.op) || pt.op === property) { + _removeLinkedListItem(this, pt, "_pt"); + } else if (!pt.dep) { + hasNonDependentRemaining = 1; + } + pt = next; + } + return !hasNonDependentRemaining; + }, + _setterWithModifier = (target, property, value, data) => { + data.mSet(target, property, data.m.call(data.tween, value, data.mt), data); + }, + _sortPropTweensByPriority = parent => { + let pt = parent._pt, + next, pt2, first, last; + //sorts the PropTween linked list in order of priority because some plugins need to do their work after ALL of the PropTweens were created (like RoundPropsPlugin and ModifiersPlugin) + while (pt) { + next = pt._next; + pt2 = first; + while (pt2 && pt2.pr > pt.pr) { + pt2 = pt2._next; + } + if ((pt._prev = pt2 ? pt2._prev : last)) { + pt._prev._next = pt; + } else { + first = pt; + } + if ((pt._next = pt2)) { + pt2._prev = pt; + } else { + last = pt; + } + pt = next; + } + parent._pt = first; + }; + +//PropTween key: t = target, p = prop, r = renderer, d = data, s = start, c = change, op = overwriteProperty (ONLY populated when it's different than p), pr = priority, _next/_prev for the linked list siblings, set = setter, m = modifier, mSet = modifierSetter (the original setter, before a modifier was added) +export class PropTween { + + constructor(next, target, prop, start, change, renderer, data, setter, priority) { + this.t = target; + this.s = start; + this.c = change; + this.p = prop; + this.r = renderer || _renderPlain; + this.d = data || this; + this.set = setter || _setterPlain; + this.pr = priority || 0; + this._next = next; + if (next) { + next._prev = this; + } + } + + modifier(func, tween, target) { + this.mSet = this.mSet || this.set; //in case it was already set (a PropTween can only have one modifier) + this.set = _setterWithModifier; + this.m = func; + this.mt = target; //modifier target + this.tween = tween; + } +} + + + +//Initialization tasks +_forEachName(_callbackNames + "parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger", name => _reservedProps[name] = 1); +_globals.TweenMax = _globals.TweenLite = Tween; +_globals.TimelineLite = _globals.TimelineMax = Timeline; +_globalTimeline = new Timeline({sortChildren: false, defaults: _defaults, autoRemoveChildren: true, id:"root", smoothChildTiming: true}); +_config.stringFilter = _colorStringFilter; + + + + + + + + + + + + + + +let _media = [], + _listeners = {}, + _emptyArray = [], + _lastMediaTime = 0, + _contextID = 0, + _dispatch = type => (_listeners[type] || _emptyArray).map(f => f()), + _onMediaChange = () => { + let time = Date.now(), + matches = []; + if (time - _lastMediaTime > 2) { + _dispatch("matchMediaInit"); + _media.forEach(c => { + let queries = c.queries, + conditions = c.conditions, + match, p, anyMatch, toggled; + for (p in queries) { + match = _win.matchMedia(queries[p]).matches; // Firefox doesn't update the "matches" property of the MediaQueryList object correctly - it only does so as it calls its change handler - so we must re-create a media query here to ensure it's accurate. + match && (anyMatch = 1); + if (match !== conditions[p]) { + conditions[p] = match; + toggled = 1; + } + } + if (toggled) { + c.revert(); + anyMatch && matches.push(c); + } + }); + _dispatch("matchMediaRevert"); + matches.forEach(c => c.onMatch(c, func => c.add(null, func))); + _lastMediaTime = time; + _dispatch("matchMedia"); + } + }; + +class Context { + constructor(func, scope) { + this.selector = scope && selector(scope); + this.data = []; + this._r = []; // returned/cleanup functions + this.isReverted = false; + this.id = _contextID++; // to work around issues that frameworks like Vue cause by making things into Proxies which make it impossible to do something like _media.indexOf(this) because "this" would no longer refer to the Context instance itself - it'd refer to a Proxy! We needed a way to identify the context uniquely + func && this.add(func); + } + add(name, func, scope) { + // possible future addition if we need the ability to add() an animation to a context and for whatever reason cannot create that animation inside of a context.add(() => {...}) function. + // if (name && _isFunction(name.revert)) { + // this.data.push(name); + // return (name._ctx = this); + // } + if (_isFunction(name)) { + scope = func; + func = name; + name = _isFunction; + } + let self = this, + f = function() { + let prev = _context, + prevSelector = self.selector, + result; + prev && prev !== self && prev.data.push(self); + scope && (self.selector = selector(scope)); + _context = self; + result = func.apply(self, arguments); + _isFunction(result) && self._r.push(result); + _context = prev; + self.selector = prevSelector; + self.isReverted = false; + return result; + }; + self.last = f; + return name === _isFunction ? f(self, func => self.add(null, func)) : name ? (self[name] = f) : f; + } + ignore(func) { + let prev = _context; + _context = null; + func(this); + _context = prev; + } + getTweens() { + let a = []; + this.data.forEach(e => (e instanceof Context) ? a.push(...e.getTweens()) : (e instanceof Tween) && !(e.parent && e.parent.data === "nested") && a.push(e)); + return a; + } + clear() { + this._r.length = this.data.length = 0; + } + kill(revert, matchMedia) { + if (revert) { + let tweens = this.getTweens(), + i = this.data.length, + t; + while (i--) { // Flip plugin tweens are very different in that they should actually be pushed to their end. The plugin replaces the timeline's .revert() method to do exactly that. But we also need to remove any of those nested tweens inside the flip timeline so that they don't get individually reverted. + t = this.data[i]; + if (t.data === "isFlip") { + t.revert(); + t.getChildren(true, true, false).forEach(tween => tweens.splice(tweens.indexOf(tween), 1)); + } + } + // save as an object so that we can cache the globalTime for each tween to optimize performance during the sort + tweens.map(t => { return {g: t._dur || t._delay || (t._sat && !t._sat.vars.immediateRender) ? t.globalTime(0) : -Infinity, t}}).sort((a, b) => b.g - a.g || -Infinity).forEach(o => o.t.revert(revert)); // note: all of the _startAt tweens should be reverted in reverse order that they were created, and they'll all have the same globalTime (-1) so the " || -1" in the sort keeps the order properly. + i = this.data.length; + while (i--) { // make sure we loop backwards so that, for example, SplitTexts that were created later on the same element get reverted first + t = this.data[i]; + if (t instanceof Timeline) { + if (t.data !== "nested") { + t.scrollTrigger && t.scrollTrigger.revert(); + t.kill(); // don't revert() the timeline because that's duplicating efforts since we already reverted all the tweens + } + } else { + !(t instanceof Tween) && t.revert && t.revert(revert) + } + } + this._r.forEach(f => f(revert, this)); + this.isReverted = true; + } else { + this.data.forEach(e => e.kill && e.kill()); + } + this.clear(); + if (matchMedia) { + let i = _media.length; + while (i--) { // previously, we checked _media.indexOf(this), but some frameworks like Vue enforce Proxy objects that make it impossible to get the proper result that way, so we must use a unique ID number instead. + _media[i].id === this.id && _media.splice(i, 1); + } + } + } + + // killWithCleanup() { + // this.kill(); + // this._r.forEach(f => f(false, this)); + // } + + revert(config) { + this.kill(config || {}); + } +} + + + + +class MatchMedia { + constructor(scope) { + this.contexts = []; + this.scope = scope; + _context && _context.data.push(this); + } + add(conditions, func, scope) { + _isObject(conditions) || (conditions = {matches: conditions}); + let context = new Context(0, scope || this.scope), + cond = context.conditions = {}, + mq, p, active; + _context && !context.selector && (context.selector = _context.selector); // in case a context is created inside a context. Like a gsap.matchMedia() that's inside a scoped gsap.context() + this.contexts.push(context); + func = context.add("onMatch", func); + context.queries = conditions; + for (p in conditions) { + if (p === "all") { + active = 1; + } else { + mq = _win.matchMedia(conditions[p]); + if (mq) { + _media.indexOf(context) < 0 && _media.push(context); + (cond[p] = mq.matches) && (active = 1); + mq.addListener ? mq.addListener(_onMediaChange) : mq.addEventListener("change", _onMediaChange); + } + } + } + active && func(context, f => context.add(null, f)); + return this; + } + // refresh() { + // let time = _lastMediaTime, + // media = _media; + // _lastMediaTime = -1; + // _media = this.contexts; + // _onMediaChange(); + // _lastMediaTime = time; + // _media = media; + // } + revert(config) { + this.kill(config || {}); + } + kill(revert) { + this.contexts.forEach(c => c.kill(revert, true)); + } +} + + + +/* + * -------------------------------------------------------------------------------------- + * GSAP + * -------------------------------------------------------------------------------------- + */ +const _gsap = { + registerPlugin(...args) { + args.forEach(config => _createPlugin(config)); + }, + timeline(vars) { + return new Timeline(vars); + }, + getTweensOf(targets, onlyActive) { + return _globalTimeline.getTweensOf(targets, onlyActive); + }, + getProperty(target, property, unit, uncache) { + _isString(target) && (target = toArray(target)[0]); //in case selector text or an array is passed in + let getter = _getCache(target || {}).get, + format = unit ? _passThrough : _numericIfPossible; + unit === "native" && (unit = ""); + return !target ? target : !property ? (property, unit, uncache) => format(((_plugins[property] && _plugins[property].get) || getter)(target, property, unit, uncache)) : format(((_plugins[property] && _plugins[property].get) || getter)(target, property, unit, uncache)); + }, + quickSetter(target, property, unit) { + target = toArray(target); + if (target.length > 1) { + let setters = target.map(t => gsap.quickSetter(t, property, unit)), + l = setters.length; + return value => { + let i = l; + while(i--) { + setters[i](value); + } + } + } + target = target[0] || {}; + let Plugin = _plugins[property], + cache = _getCache(target), + p = (cache.harness && (cache.harness.aliases || {})[property]) || property, // in case it's an alias, like "rotate" for "rotation". + setter = Plugin ? value => { + let p = new Plugin(); + _quickTween._pt = 0; + p.init(target, unit ? value + unit : value, _quickTween, 0, [target]); + p.render(1, p); + _quickTween._pt && _renderPropTweens(1, _quickTween); + } : cache.set(target, p); + return Plugin ? setter : value => setter(target, p, unit ? value + unit : value, cache, 1); + }, + quickTo(target, property, vars) { + let tween = gsap.to(target, _setDefaults({[property]: "+=0.1", paused: true, stagger: 0}, vars || {})), + func = (value, start, startIsRelative) => tween.resetTo(property, value, start, startIsRelative); + func.tween = tween; + return func; + }, + isTweening(targets) { + return _globalTimeline.getTweensOf(targets, true).length > 0; + }, + defaults(value) { + value && value.ease && (value.ease = _parseEase(value.ease, _defaults.ease)); + return _mergeDeep(_defaults, value || {}); + }, + config(value) { + return _mergeDeep(_config, value || {}); + }, + registerEffect({name, effect, plugins, defaults, extendTimeline}) { + (plugins || "").split(",").forEach(pluginName => pluginName && !_plugins[pluginName] && !_globals[pluginName] && _warn(name + " effect requires " + pluginName + " plugin.")); + _effects[name] = (targets, vars, tl) => effect(toArray(targets), _setDefaults(vars || {}, defaults), tl); + if (extendTimeline) { + Timeline.prototype[name] = function(targets, vars, position) { + return this.add(_effects[name](targets, _isObject(vars) ? vars : (position = vars) && {}, this), position); + }; + } + }, + registerEase(name, ease) { + _easeMap[name] = _parseEase(ease); + }, + parseEase(ease, defaultEase) { + return arguments.length ? _parseEase(ease, defaultEase) : _easeMap; + }, + getById(id) { + return _globalTimeline.getById(id); + }, + exportRoot(vars = {}, includeDelayedCalls) { + let tl = new Timeline(vars), + child, next; + tl.smoothChildTiming = _isNotFalse(vars.smoothChildTiming); + _globalTimeline.remove(tl); + tl._dp = 0; //otherwise it'll get re-activated when adding children and be re-introduced into _globalTimeline's linked list (then added to itself). + tl._time = tl._tTime = _globalTimeline._time; + child = _globalTimeline._first; + while (child) { + next = child._next; + if (includeDelayedCalls || !(!child._dur && child instanceof Tween && child.vars.onComplete === child._targets[0])) { + _addToTimeline(tl, child, child._start - child._delay); + } + child = next; + } + _addToTimeline(_globalTimeline, tl, 0); + return tl; + }, + context: (func, scope) => func ? new Context(func, scope) : _context, + matchMedia: scope => new MatchMedia(scope), + matchMediaRefresh: () => _media.forEach(c => { + let cond = c.conditions, + found, p; + for (p in cond) { + if (cond[p]) { + cond[p] = false; + found = 1; + } + } + found && c.revert(); + }) || _onMediaChange(), + addEventListener(type, callback) { + let a = _listeners[type] || (_listeners[type] = []); + ~a.indexOf(callback) || a.push(callback); + }, + removeEventListener(type, callback) { + let a = _listeners[type], + i = a && a.indexOf(callback); + i >= 0 && a.splice(i, 1); + }, + utils: { wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, selector, mapRange, pipe, unitize, interpolate, shuffle }, + install: _install, + effects: _effects, + ticker: _ticker, + updateRoot: Timeline.updateRoot, + plugins: _plugins, + globalTimeline: _globalTimeline, + core: {PropTween, globals: _addGlobal, Tween, Timeline, Animation, getCache: _getCache, _removeLinkedListItem, reverting: () => _reverting, context: toAdd => {if (toAdd && _context) { _context.data.push(toAdd); toAdd._ctx = _context} return _context; }, suppressOverwrites: value => _suppressOverwrites = value} +}; + +_forEachName("to,from,fromTo,delayedCall,set,killTweensOf", name => _gsap[name] = Tween[name]); +_ticker.add(Timeline.updateRoot); +_quickTween = _gsap.to({}, {duration:0}); + + + + +// ---- EXTRA PLUGINS -------------------------------------------------------- + + +let _getPluginPropTween = (plugin, prop) => { + let pt = plugin._pt; + while (pt && pt.p !== prop && pt.op !== prop && pt.fp !== prop) { + pt = pt._next; + } + return pt; + }, + _addModifiers = (tween, modifiers) => { + let targets = tween._targets, + p, i, pt; + for (p in modifiers) { + i = targets.length; + while (i--) { + pt = tween._ptLookup[i][p]; + if (pt && (pt = pt.d)) { + if (pt._pt) { // is a plugin + pt = _getPluginPropTween(pt, p); + } + pt && pt.modifier && pt.modifier(modifiers[p], tween, targets[i], p); + } + } + } + }, + _buildModifierPlugin = (name, modifier) => { + return { + name: name, + rawVars: 1, //don't pre-process function-based values or "random()" strings. + init(target, vars, tween) { + tween._onInit = tween => { + let temp, p; + if (_isString(vars)) { + temp = {}; + _forEachName(vars, name => temp[name] = 1); //if the user passes in a comma-delimited list of property names to roundProps, like "x,y", we round to whole numbers. + vars = temp; + } + if (modifier) { + temp = {}; + for (p in vars) { + temp[p] = modifier(vars[p]); + } + vars = temp; + } + _addModifiers(tween, vars); + }; + } + }; + }; + +//register core plugins +export const gsap = _gsap.registerPlugin({ + name:"attr", + init(target, vars, tween, index, targets) { + let p, pt, v; + this.tween = tween; + for (p in vars) { + v = target.getAttribute(p) || ""; + pt = this.add(target, "setAttribute", (v || 0) + "", vars[p], index, targets, 0, 0, p); + pt.op = p; + pt.b = v; // record the beginning value so we can revert() + this._props.push(p); + } + }, + render(ratio, data) { + let pt = data._pt; + while (pt) { + _reverting ? pt.set(pt.t, pt.p, pt.b, pt) : pt.r(ratio, pt.d); // if reverting, go back to the original (pt.b) + pt = pt._next; + } + } + }, { + name:"endArray", + init(target, value) { + let i = value.length; + while (i--) { + this.add(target, i, target[i] || 0, value[i], 0, 0, 0, 0, 0, 1); + } + } + }, + _buildModifierPlugin("roundProps", _roundModifier), + _buildModifierPlugin("modifiers"), + _buildModifierPlugin("snap", snap) +) || _gsap; //to prevent the core plugins from being dropped via aggressive tree shaking, we must include them in the variable declaration in this way. + +Tween.version = Timeline.version = gsap.version = "3.12.7"; +_coreReady = 1; +_windowExists() && _wake(); + +export const { Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ } = _easeMap; +export { Tween as TweenMax, Tween as TweenLite, Timeline as TimelineMax, Timeline as TimelineLite, gsap as default, wrap, wrapYoyo, distribute, random, snap, normalize, getUnit, clamp, splitColor, toArray, selector, mapRange, pipe, unitize, interpolate, shuffle }; +//export some internal methods/orojects for use in CSSPlugin so that we can externalize that file and allow custom builds that exclude it. +export { _getProperty, _numExp, _numWithUnitExp, _isString, _isUndefined, _renderComplexString, _relExp, _setDefaults, _removeLinkedListItem, _forEachName, _sortPropTweensByPriority, _colorStringFilter, _replaceRandom, _checkPlugin, _plugins, _ticker, _config, _roundModifier, _round, _missingPlugin, _getSetter, _getCache, _colorExp, _parseRelative } \ No newline at end of file diff --git a/node_modules/gsap/src/index.js b/node_modules/gsap/src/index.js new file mode 100644 index 0000000000000000000000000000000000000000..8cc4f165fd3a7ff08cc1025437ea31975b1b43d5 --- /dev/null +++ b/node_modules/gsap/src/index.js @@ -0,0 +1,33 @@ +import { gsap, Power0, Power1, Power2, Power3, Power4, Linear, Quad, Cubic, Quart, Quint, Strong, Elastic, Back, SteppedEase, Bounce, Sine, Expo, Circ, TweenLite, TimelineLite, TimelineMax } from "./gsap-core.js"; +import { CSSPlugin } from "./CSSPlugin.js"; + +const gsapWithCSS = gsap.registerPlugin(CSSPlugin) || gsap, // to protect from tree shaking + TweenMaxWithCSS = gsapWithCSS.core.Tween; + +export { + gsapWithCSS as gsap, + gsapWithCSS as default, + CSSPlugin, + TweenMaxWithCSS as TweenMax, + TweenLite, + TimelineMax, + TimelineLite, + Power0, + Power1, + Power2, + Power3, + Power4, + Linear, + Quad, + Cubic, + Quart, + Quint, + Strong, + Elastic, + Back, + SteppedEase, + Bounce, + Sine, + Expo, + Circ +}; \ No newline at end of file diff --git a/node_modules/gsap/src/utils/matrix.js b/node_modules/gsap/src/utils/matrix.js new file mode 100644 index 0000000000000000000000000000000000000000..90b598949d8336ad17852977d745b8acb855dc0f --- /dev/null +++ b/node_modules/gsap/src/utils/matrix.js @@ -0,0 +1,324 @@ +/*! + * matrix 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let _doc, _win, _docElement, _body, _divContainer, _svgContainer, _identityMatrix, _gEl, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _hasOffsetBug, + _setDoc = element => { + let doc = element.ownerDocument || element; + if (!(_transformProp in element.style) && "msTransform" in element.style) { //to improve compatibility with old Microsoft browsers + _transformProp = "msTransform"; + _transformOriginProp = _transformProp + "Origin"; + } + while (doc.parentNode && (doc = doc.parentNode)) { } + _win = window; + _identityMatrix = new Matrix2D(); + if (doc) { + _doc = doc; + _docElement = doc.documentElement; + _body = doc.body; + _gEl = _doc.createElementNS("http://www.w3.org/2000/svg", "g"); + // prevent any existing CSS from transforming it + _gEl.style.transform = "none"; + // now test for the offset reporting bug. Use feature detection instead of browser sniffing to make things more bulletproof and future-proof. Hopefully Safari will fix their bug soon. + let d1 = doc.createElement("div"), + d2 = doc.createElement("div"), + root = doc && (doc.body || doc.firstElementChild); + if (root && root.appendChild) { + root.appendChild(d1); + d1.appendChild(d2); + d1.setAttribute("style", "position:static;transform:translate3d(0,0,1px)"); + _hasOffsetBug = (d2.offsetParent !== d1); + root.removeChild(d1); + } + } + return doc; + }, + _forceNonZeroScale = e => { // walks up the element's ancestors and finds any that had their scale set to 0 via GSAP, and changes them to 0.0001 to ensure that measurements work. Firefox has a bug that causes it to incorrectly report getBoundingClientRect() when scale is 0. + let a, cache; + while (e && e !== _body) { + cache = e._gsap; + cache && cache.uncache && cache.get(e, "x"); // force re-parsing of transforms if necessary + if (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) { + cache.scaleX = cache.scaleY = 1e-4; + cache.renderTransform(1, cache); + a ? a.push(cache) : (a = [cache]); + } + e = e.parentNode; + } + return a; + }, + // possible future addition: pass an element to _forceDisplay() and it'll walk up all its ancestors and make sure anything with display: none is set to display: block, and if there's no parentNode, it'll add it to the body. It returns an Array that you can then feed to _revertDisplay() to have it revert all the changes it made. + // _forceDisplay = e => { + // let a = [], + // parent; + // while (e && e !== _body) { + // parent = e.parentNode; + // (_win.getComputedStyle(e).display === "none" || !parent) && a.push(e, e.style.display, parent) && (e.style.display = "block"); + // parent || _body.appendChild(e); + // e = parent; + // } + // return a; + // }, + // _revertDisplay = a => { + // for (let i = 0; i < a.length; i+=3) { + // a[i+1] ? (a[i].style.display = a[i+1]) : a[i].style.removeProperty("display"); + // a[i+2] || a[i].parentNode.removeChild(a[i]); + // } + // }, + _svgTemps = [], //we create 3 elements for SVG, and 3 for other DOM elements and cache them for performance reasons. They get nested in _divContainer and _svgContainer so that just one element is added to the DOM on each successive attempt. Again, performance is key. + _divTemps = [], + _getDocScrollTop = () => _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0, + _getDocScrollLeft = () => _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0, + _svgOwner = element => element.ownerSVGElement || ((element.tagName + "").toLowerCase() === "svg" ? element : null), + _isFixed = element => { + if (_win.getComputedStyle(element).position === "fixed") { + return true; + } + element = element.parentNode; + if (element && element.nodeType === 1) { // avoid document fragments which will throw an error. + return _isFixed(element); + } + }, + _createSibling = (element, i) => { + if (element.parentNode && (_doc || _setDoc(element))) { + let svg = _svgOwner(element), + ns = svg ? (svg.getAttribute("xmlns") || "http://www.w3.org/2000/svg") : "http://www.w3.org/1999/xhtml", + type = svg ? (i ? "rect" : "g") : "div", + x = i !== 2 ? 0 : 100, + y = i === 3 ? 100 : 0, + css = "position:absolute;display:block;pointer-events:none;margin:0;padding:0;", + e = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, "http"), type) : _doc.createElement(type); + if (i) { + if (!svg) { + if (!_divContainer) { + _divContainer = _createSibling(element); + _divContainer.style.cssText = css; + } + e.style.cssText = css + "width:0.1px;height:0.1px;top:" + y + "px;left:" + x + "px"; + _divContainer.appendChild(e); + + } else { + _svgContainer || (_svgContainer = _createSibling(element)); + e.setAttribute("width", 0.01); + e.setAttribute("height", 0.01); + e.setAttribute("transform", "translate(" + x + "," + y + ")"); + _svgContainer.appendChild(e); + } + } + return e; + } + throw "Need document and parent."; + }, + _consolidate = m => { // replaces SVGTransformList.consolidate() because a bug in Firefox causes it to break pointer events. See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800 + let c = new Matrix2D(), + i = 0; + for (; i < m.numberOfItems; i++) { + c.multiply(m.getItem(i).matrix); + } + return c; + }, + _getCTM = svg => { + let m = svg.getCTM(), + transform; + if (!m) { // Firefox returns null for getCTM() on root <svg> elements, so this is a workaround using a <g> that we temporarily append. + transform = svg.style[_transformProp]; + svg.style[_transformProp] = "none"; // a bug in Firefox causes css transforms to contaminate the getCTM() + svg.appendChild(_gEl); + m = _gEl.getCTM(); + svg.removeChild(_gEl); + transform ? (svg.style[_transformProp] = transform) : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, "-$1").toLowerCase()); + } + return m || _identityMatrix.clone(); // Firefox will still return null if the <svg> has a width/height of 0 in the browser. + }, + _placeSiblings = (element, adjustGOffset) => { + let svg = _svgOwner(element), + isRootSVG = element === svg, + siblings = svg ? _svgTemps : _divTemps, + parent = element.parentNode, + container, m, b, x, y, cs; + if (element === _win) { + return element; + } + siblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3)); + container = svg ? _svgContainer : _divContainer; + if (svg) { + if (isRootSVG) { + b = _getCTM(element); + x = -b.e / b.a; + y = -b.f / b.d; + m = _identityMatrix; + } else if (element.getBBox) { + b = element.getBBox(); + m = element.transform ? element.transform.baseVal : {}; // IE11 doesn't follow the spec. + m = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; // don't call m.consolidate().matrix because a bug in Firefox makes pointer events not work when consolidate() is called on the same tick as getBoundingClientRect()! See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800 + x = m.a * b.x + m.c * b.y; + y = m.b * b.x + m.d * b.y; + } else { // may be a <mask> which has no getBBox() so just use defaults instead of throwing errors. + m = new Matrix2D(); + x = y = 0; + } + if (adjustGOffset && element.tagName.toLowerCase() === "g") { + x = y = 0; + } + (isRootSVG ? svg : parent).appendChild(container); + container.setAttribute("transform", "matrix(" + m.a + "," + m.b + "," + m.c + "," + m.d + "," + (m.e + x) + "," + (m.f + y) + ")"); + } else { + x = y = 0; + if (_hasOffsetBug) { // some browsers (like Safari) have a bug that causes them to misreport offset values. When an ancestor element has a transform applied, it's supposed to treat it as if it's position: relative (new context). Safari botches this, so we need to find the closest ancestor (between the element and its offsetParent) that has a transform applied and if one is found, grab its offsetTop/Left and subtract them to compensate. + m = element.offsetParent; + b = element; + while (b && (b = b.parentNode) && b !== m && b.parentNode) { + if ((_win.getComputedStyle(b)[_transformProp] + "").length > 4) { + x = b.offsetLeft; + y = b.offsetTop; + b = 0; + } + } + } + cs = _win.getComputedStyle(element); + if (cs.position !== "absolute" && cs.position !== "fixed") { + m = element.offsetParent; + while (parent && parent !== m) { // if there's an ancestor element between the element and its offsetParent that's scrolled, we must factor that in. + x += parent.scrollLeft || 0; + y += parent.scrollTop || 0; + parent = parent.parentNode; + } + } + b = container.style; + b.top = (element.offsetTop - y) + "px"; + b.left = (element.offsetLeft - x) + "px"; + b[_transformProp] = cs[_transformProp]; + b[_transformOriginProp] = cs[_transformOriginProp]; + // b.border = m.border; + // b.borderLeftStyle = m.borderLeftStyle; + // b.borderTopStyle = m.borderTopStyle; + // b.borderLeftWidth = m.borderLeftWidth; + // b.borderTopWidth = m.borderTopWidth; + b.position = cs.position === "fixed" ? "fixed" : "absolute"; + element.parentNode.appendChild(container); + } + return container; + }, + _setMatrix = (m, a, b, c, d, e, f) => { + m.a = a; + m.b = b; + m.c = c; + m.d = d; + m.e = e; + m.f = f; + return m; + }; + +export class Matrix2D { + constructor(a=1, b=0, c=0, d=1, e=0, f=0) { + _setMatrix(this, a, b, c, d, e, f); + } + + inverse() { + let {a, b, c, d, e, f} = this, + determinant = (a * d - b * c) || 1e-10; + return _setMatrix( + this, + d / determinant, + -b / determinant, + -c / determinant, + a / determinant, + (c * f - d * e) / determinant, + -(a * f - b * e) / determinant + ); + } + + multiply(matrix) { + let {a, b, c, d, e, f} = this, + a2 = matrix.a, + b2 = matrix.c, + c2 = matrix.b, + d2 = matrix.d, + e2 = matrix.e, + f2 = matrix.f; + return _setMatrix(this, + a2 * a + c2 * c, + a2 * b + c2 * d, + b2 * a + d2 * c, + b2 * b + d2 * d, + e + e2 * a + f2 * c, + f + e2 * b + f2 * d); + } + + clone() { + return new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f); + } + + equals(matrix) { + let {a, b, c, d, e, f} = this; + return (a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f); + } + + apply(point, decoratee={}) { + let {x, y} = point, + {a, b, c, d, e, f} = this; + decoratee.x = (x * a + y * c + e) || 0; + decoratee.y = (x * b + y * d + f) || 0; + return decoratee; + } + +} + +// Feed in an element and it'll return a 2D matrix (optionally inverted) so that you can translate between coordinate spaces. +// Inverting lets you translate a global point into a local coordinate space. No inverting lets you go the other way. +// We needed this to work around various browser bugs, like Firefox doesn't accurately report getScreenCTM() when there +// are transforms applied to ancestor elements. +// The matrix math to convert any x/y coordinate is as follows, which is wrapped in a convenient apply() method of Matrix2D above: +// tx = m.a * x + m.c * y + m.e +// ty = m.b * x + m.d * y + m.f +export function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { // adjustGOffset is typically used only when grabbing an element's PARENT's global matrix, and it ignores the x/y offset of any SVG <g> elements because they behave in a special way. + if (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) { + return new Matrix2D(); + } + let zeroScales = _forceNonZeroScale(element), + svg = _svgOwner(element), + temps = svg ? _svgTemps : _divTemps, + container = _placeSiblings(element, adjustGOffset), + b1 = temps[0].getBoundingClientRect(), + b2 = temps[1].getBoundingClientRect(), + b3 = temps[2].getBoundingClientRect(), + parent = container.parentNode, + isFixed = !includeScrollInFixed && _isFixed(element), + m = new Matrix2D( + (b2.left - b1.left) / 100, + (b2.top - b1.top) / 100, + (b3.left - b1.left) / 100, + (b3.top - b1.top) / 100, + b1.left + (isFixed ? 0 : _getDocScrollLeft()), + b1.top + (isFixed ? 0 : _getDocScrollTop()) + ); + parent.removeChild(container); + if (zeroScales) { + b1 = zeroScales.length; + while (b1--) { + b2 = zeroScales[b1]; + b2.scaleX = b2.scaleY = 0; + b2.renderTransform(1, b2); + } + } + return inverse ? m.inverse() : m; +} + +export { _getDocScrollTop, _getDocScrollLeft, _setDoc, _isFixed, _getCTM }; + +// export function getMatrix(element) { +// _doc || _setDoc(element); +// let m = (_win.getComputedStyle(element)[_transformProp] + "").substr(7).match(/[-.]*\d+[.e\-+]*\d*[e\-\+]*\d*/g), +// is2D = m && m.length === 6; +// return !m || m.length < 6 ? new Matrix2D() : new Matrix2D(+m[0], +m[1], +m[is2D ? 2 : 4], +m[is2D ? 3 : 5], +m[is2D ? 4 : 12], +m[is2D ? 5 : 13]); +// } \ No newline at end of file diff --git a/node_modules/gsap/src/utils/paths.js b/node_modules/gsap/src/utils/paths.js new file mode 100644 index 0000000000000000000000000000000000000000..9137448754776ccc80b1add002402b39b59a3566 --- /dev/null +++ b/node_modules/gsap/src/utils/paths.js @@ -0,0 +1,1247 @@ +/*! + * paths 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _numbersExp = /(?:(-)?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + _selectorExp = /(^[#\.][a-z]|[a-y][a-z])/i, + _DEG2RAD = Math.PI / 180, + _RAD2DEG = 180 / Math.PI, + _sin = Math.sin, + _cos = Math.cos, + _abs = Math.abs, + _sqrt = Math.sqrt, + _atan2 = Math.atan2, + _largeNum = 1e8, + _isString = value => typeof(value) === "string", + _isNumber = value => typeof(value) === "number", + _isUndefined = value => typeof(value) === "undefined", + _temp = {}, + _temp2 = {}, + _roundingNum = 1e5, + _wrapProgress = progress => (Math.round((progress + _largeNum) % 1 * _roundingNum) / _roundingNum) || ((progress < 0) ? 0 : 1), //if progress lands on 1, the % will make it 0 which is why we || 1, but not if it's negative because it makes more sense for motion to end at 0 in that case. + _round = value => (Math.round(value * _roundingNum) / _roundingNum) || 0, + _roundPrecise = value => (Math.round(value * 1e10) / 1e10) || 0, + _splitSegment = (rawPath, segIndex, i, t) => { + let segment = rawPath[segIndex], + shift = t === 1 ? 6 : subdivideSegment(segment, i, t); + if ((shift || !t) && shift + i + 2 < segment.length) { + rawPath.splice(segIndex, 0, segment.slice(0, i + shift + 2)); + segment.splice(0, i + shift); + return 1; + } + }, + _getSampleIndex = (samples, length, progress) => { + // slightly slower way than doing this (when there's no lookup): segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0; + let l = samples.length, + i = ~~(progress * l); + if (samples[i] > length) { + while (--i && samples[i] > length) {} + i < 0 && (i = 0); + } else { + while (samples[++i] < length && i < l) {} + } + return i < l ? i : l - 1; + }, + _reverseRawPath = (rawPath, skipOuter) => { + let i = rawPath.length; + skipOuter || rawPath.reverse(); + while (i--) { + rawPath[i].reversed || reverseSegment(rawPath[i]); + } + }, + _copyMetaData = (source, copy) => { + copy.totalLength = source.totalLength; + if (source.samples) { //segment + copy.samples = source.samples.slice(0); + copy.lookup = source.lookup.slice(0); + copy.minLength = source.minLength; + copy.resolution = source.resolution; + } else if (source.totalPoints) { //rawPath + copy.totalPoints = source.totalPoints; + } + return copy; + }, + //pushes a new segment into a rawPath, but if its starting values match the ending values of the last segment, it'll merge it into that same segment (to reduce the number of segments) + _appendOrMerge = (rawPath, segment) => { + let index = rawPath.length, + prevSeg = rawPath[index - 1] || [], + l = prevSeg.length; + if (index && segment[0] === prevSeg[l-2] && segment[1] === prevSeg[l-1]) { + segment = prevSeg.concat(segment.slice(2)); + index--; + } + rawPath[index] = segment; + }, + _bestDistance; + +/* TERMINOLOGY + - RawPath - an array of arrays, one for each Segment. A single RawPath could have multiple "M" commands, defining Segments (paths aren't always connected). + - Segment - an array containing a sequence of Cubic Bezier coordinates in alternating x, y, x, y format. Starting anchor, then control point 1, control point 2, and ending anchor, then the next control point 1, control point 2, anchor, etc. Uses less memory than an array with a bunch of {x, y} points. + - Bezier - a single cubic Bezier with a starting anchor, two control points, and an ending anchor. + - the variable "t" is typically the position along an individual Bezier path (time) and it's NOT linear, meaning it could accelerate/decelerate based on the control points whereas the "p" or "progress" value is linearly mapped to the whole path, so it shouldn't really accelerate/decelerate based on control points. So a progress of 0.2 would be almost exactly 20% along the path. "t" is ONLY in an individual Bezier piece. + */ + +//accepts basic selector text, a path instance, a RawPath instance, or a Segment and returns a RawPath (makes it easy to homogenize things). If an element or selector text is passed in, it'll also cache the value so that if it's queried again, it'll just take the path data from there instead of parsing it all over again (as long as the path data itself hasn't changed - it'll check). +export function getRawPath(value) { + value = (_isString(value) && _selectorExp.test(value)) ? document.querySelector(value) || value : value; + let e = value.getAttribute ? value : 0, + rawPath; + if (e && (value = value.getAttribute("d"))) { + //implements caching + if (!e._gsPath) { + e._gsPath = {}; + } + rawPath = e._gsPath[value]; + return (rawPath && !rawPath._dirty) ? rawPath : (e._gsPath[value] = stringToRawPath(value)); + } + return !value ? console.warn("Expecting a <path> element or an SVG path data string") : _isString(value) ? stringToRawPath(value) : (_isNumber(value[0])) ? [value] : value; +} + +//copies a RawPath WITHOUT the length meta data (for speed) +export function copyRawPath(rawPath) { + let a = [], + i = 0; + for (; i < rawPath.length; i++) { + a[i] = _copyMetaData(rawPath[i], rawPath[i].slice(0)); + } + return _copyMetaData(rawPath, a); +} + +export function reverseSegment(segment) { + let i = 0, + y; + segment.reverse(); //this will invert the order y, x, y, x so we must flip it back. + for (; i < segment.length; i += 2) { + y = segment[i]; + segment[i] = segment[i+1]; + segment[i+1] = y; + } + segment.reversed = !segment.reversed; +} + + + +let _createPath = (e, ignore) => { + let path = document.createElementNS("http://www.w3.org/2000/svg", "path"), + attr = [].slice.call(e.attributes), + i = attr.length, + name; + ignore = "," + ignore + ","; + while (--i > -1) { + name = attr[i].nodeName.toLowerCase(); //in Microsoft Edge, if you don't set the attribute with a lowercase name, it doesn't render correctly! Super weird. + if (ignore.indexOf("," + name + ",") < 0) { + path.setAttributeNS(null, name, attr[i].nodeValue); + } + } + return path; + }, + _typeAttrs = { + rect:"rx,ry,x,y,width,height", + circle:"r,cx,cy", + ellipse:"rx,ry,cx,cy", + line:"x1,x2,y1,y2" + }, + _attrToObj = (e, attrs) => { + let props = attrs ? attrs.split(",") : [], + obj = {}, + i = props.length; + while (--i > -1) { + obj[props[i]] = +e.getAttribute(props[i]) || 0; + } + return obj; + }; + +//converts an SVG shape like <circle>, <rect>, <polygon>, <polyline>, <ellipse>, etc. to a <path>, swapping it in and copying the attributes to match. +export function convertToPath(element, swap) { + let type = element.tagName.toLowerCase(), + circ = 0.552284749831, + data, x, y, r, ry, path, rcirc, rycirc, points, w, h, x2, x3, x4, x5, x6, y2, y3, y4, y5, y6, attr; + if (type === "path" || !element.getBBox) { + return element; + } + path = _createPath(element, "x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points"); + attr = _attrToObj(element, _typeAttrs[type]); + if (type === "rect") { + r = attr.rx; + ry = attr.ry || r; + x = attr.x; + y = attr.y; + w = attr.width - r * 2; + h = attr.height - ry * 2; + if (r || ry) { //if there are rounded corners, render cubic beziers + x2 = x + r * (1 - circ); + x3 = x + r; + x4 = x3 + w; + x5 = x4 + r * circ; + x6 = x4 + r; + y2 = y + ry * (1 - circ); + y3 = y + ry; + y4 = y3 + h; + y5 = y4 + ry * circ; + y6 = y4 + ry; + data = "M" + x6 + "," + y3 + " V" + y4 + " C" + [x6, y5, x5, y6, x4, y6, x4 - (x4 - x3) / 3, y6, x3 + (x4 - x3) / 3, y6, x3, y6, x2, y6, x, y5, x, y4, x, y4 - (y4 - y3) / 3, x, y3 + (y4 - y3) / 3, x, y3, x, y2, x2, y, x3, y, x3 + (x4 - x3) / 3, y, x4 - (x4 - x3) / 3, y, x4, y, x5, y, x6, y2, x6, y3].join(",") + "z"; + } else { + data = "M" + (x + w) + "," + y + " v" + h + " h" + (-w) + " v" + (-h) + " h" + w + "z"; + } + + } else if (type === "circle" || type === "ellipse") { + if (type === "circle") { + r = ry = attr.r; + rycirc = r * circ; + } else { + r = attr.rx; + ry = attr.ry; + rycirc = ry * circ; + } + x = attr.cx; + y = attr.cy; + rcirc = r * circ; + data = "M" + (x+r) + "," + y + " C" + [x+r, y + rycirc, x + rcirc, y + ry, x, y + ry, x - rcirc, y + ry, x - r, y + rycirc, x - r, y, x - r, y - rycirc, x - rcirc, y - ry, x, y - ry, x + rcirc, y - ry, x + r, y - rycirc, x + r, y].join(",") + "z"; + } else if (type === "line") { + data = "M" + attr.x1 + "," + attr.y1 + " L" + attr.x2 + "," + attr.y2; //previously, we just converted to "Mx,y Lx,y" but Safari has bugs that cause that not to render properly when using a stroke-dasharray that's not fully visible! Using a cubic bezier fixes that issue. + } else if (type === "polyline" || type === "polygon") { + points = (element.getAttribute("points") + "").match(_numbersExp) || []; + x = points.shift(); + y = points.shift(); + data = "M" + x + "," + y + " L" + points.join(","); + if (type === "polygon") { + data += "," + x + "," + y + "z"; + } + } + path.setAttribute("d", rawPathToString(path._gsRawPath = stringToRawPath(data))); + if (swap && element.parentNode) { + element.parentNode.insertBefore(path, element); + element.parentNode.removeChild(element); + } + return path; +} + + + +//returns the rotation (in degrees) at a particular progress on a rawPath (the slope of the tangent) +export function getRotationAtProgress(rawPath, progress) { + let d = getProgressData(rawPath, progress >= 1 ? 1 - 1e-9 : progress ? progress : 1e-9); + return getRotationAtBezierT(d.segment, d.i, d.t); +} + +function getRotationAtBezierT(segment, i, t) { + let a = segment[i], + b = segment[i+2], + c = segment[i+4], + x; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + x = b + ((c + (segment[i+6] - c) * t) - b) * t - a; + a = segment[i+1]; + b = segment[i+3]; + c = segment[i+5]; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + return _round(_atan2(b + ((c + (segment[i+7] - c) * t) - b) * t - a, x) * _RAD2DEG); +} + +export function sliceRawPath(rawPath, start, end) { + end = _isUndefined(end) ? 1 : _roundPrecise(end) || 0; // we must round to avoid issues like 4.15 / 8 = 0.8300000000000001 instead of 0.83 or 2.8 / 5 = 0.5599999999999999 instead of 0.56 and if someone is doing a loop like start: 2.8 / 0.5, end: 2.8 / 0.5 + 1. + start = _roundPrecise(start) || 0; + let loops = Math.max(0, ~~(_abs(end - start) - 1e-8)), + path = copyRawPath(rawPath); + if (start > end) { + start = 1 - start; + end = 1 - end; + _reverseRawPath(path); + path.totalLength = 0; + } + if (start < 0 || end < 0) { + let offset = Math.abs(~~Math.min(start, end)) + 1; + start += offset; + end += offset; + } + path.totalLength || cacheRawPathMeasurements(path); + let wrap = (end > 1), + s = getProgressData(path, start, _temp, true), + e = getProgressData(path, end, _temp2), + eSeg = e.segment, + sSeg = s.segment, + eSegIndex = e.segIndex, + sSegIndex = s.segIndex, + ei = e.i, + si = s.i, + sameSegment = (sSegIndex === eSegIndex), + sameBezier = (ei === si && sameSegment), + wrapsBehind, sShift, eShift, i, copy, totalSegments, l, j; + if (wrap || loops) { + wrapsBehind = eSegIndex < sSegIndex || (sameSegment && ei < si) || (sameBezier && e.t < s.t); + if (_splitSegment(path, sSegIndex, si, s.t)) { + sSegIndex++; + if (!wrapsBehind) { + eSegIndex++; + if (sameBezier) { + e.t = (e.t - s.t) / (1 - s.t); + ei = 0; + } else if (sameSegment) { + ei -= si; + } + } + } + if (Math.abs(1 - (end - start)) < 1e-5) { + eSegIndex = sSegIndex - 1; + } else if (!e.t && eSegIndex) { + eSegIndex--; + } else if (_splitSegment(path, eSegIndex, ei, e.t) && wrapsBehind) { + sSegIndex++; + } + if (s.t === 1) { + sSegIndex = (sSegIndex + 1) % path.length; + } + copy = []; + totalSegments = path.length; + l = 1 + totalSegments * loops; + j = sSegIndex; + l += ((totalSegments - sSegIndex) + eSegIndex) % totalSegments; + for (i = 0; i < l; i++) { + _appendOrMerge(copy, path[j++ % totalSegments]); + } + path = copy; + } else { + eShift = e.t === 1 ? 6 : subdivideSegment(eSeg, ei, e.t); + if (start !== end) { + sShift = subdivideSegment(sSeg, si, sameBezier ? s.t / e.t : s.t); + sameSegment && (eShift += sShift); + eSeg.splice(ei + eShift + 2); + (sShift || si) && sSeg.splice(0, si + sShift); + i = path.length; + while (i--) { + //chop off any extra segments + (i < sSegIndex || i > eSegIndex) && path.splice(i, 1); + } + } else { + eSeg.angle = getRotationAtBezierT(eSeg, ei + eShift, 0); //record the value before we chop because it'll be impossible to determine the angle after its length is 0! + ei += eShift; + s = eSeg[ei]; + e = eSeg[ei+1]; + eSeg.length = eSeg.totalLength = 0; + eSeg.totalPoints = path.totalPoints = 8; + eSeg.push(s, e, s, e, s, e, s, e); + } + } + path.totalLength = 0; + return path; +} + +//measures a Segment according to its resolution (so if segment.resolution is 6, for example, it'll take 6 samples equally across each Bezier) and create/populate a "samples" Array that has the length up to each of those sample points (always increasing from the start) as well as a "lookup" array that's broken up according to the smallest distance between 2 samples. This gives us a very fast way of looking up a progress position rather than looping through all the points/Beziers. You can optionally have it only measure a subset, starting at startIndex and going for a specific number of beziers (remember, there are 3 x/y pairs each, for a total of 6 elements for each Bezier). It will also populate a "totalLength" property, but that's not generally super accurate because by default it'll only take 6 samples per Bezier. But for performance reasons, it's perfectly adequate for measuring progress values along the path. If you need a more accurate totalLength, either increase the resolution or use the more advanced bezierToPoints() method which keeps adding points until they don't deviate by more than a certain precision value. +function measureSegment(segment, startIndex, bezierQty) { + startIndex = startIndex || 0; + if (!segment.samples) { + segment.samples = []; + segment.lookup = []; + } + let resolution = ~~segment.resolution || 12, + inc = 1 / resolution, + endIndex = bezierQty ? startIndex + bezierQty * 6 + 1 : segment.length, + x1 = segment[startIndex], + y1 = segment[startIndex + 1], + samplesIndex = startIndex ? (startIndex / 6) * resolution : 0, + samples = segment.samples, + lookup = segment.lookup, + min = (startIndex ? segment.minLength : _largeNum) || _largeNum, + prevLength = samples[samplesIndex + bezierQty * resolution - 1], + length = startIndex ? samples[samplesIndex-1] : 0, + i, j, x4, x3, x2, xd, xd1, y4, y3, y2, yd, yd1, inv, t, lengthIndex, l, segLength; + samples.length = lookup.length = 0; + for (j = startIndex + 2; j < endIndex; j += 6) { + x4 = segment[j + 4] - x1; + x3 = segment[j + 2] - x1; + x2 = segment[j] - x1; + y4 = segment[j + 5] - y1; + y3 = segment[j + 3] - y1; + y2 = segment[j + 1] - y1; + xd = xd1 = yd = yd1 = 0; + if (_abs(x4) < .01 && _abs(y4) < .01 && _abs(x2) + _abs(y2) < .01) { //dump points that are sufficiently close (basically right on top of each other, making a bezier super tiny or 0 length) + if (segment.length > 8) { + segment.splice(j, 6); + j -= 6; + endIndex -= 6; + } + } else { + for (i = 1; i <= resolution; i++) { + t = inc * i; + inv = 1 - t; + xd = xd1 - (xd1 = (t * t * x4 + 3 * inv * (t * x3 + inv * x2)) * t); + yd = yd1 - (yd1 = (t * t * y4 + 3 * inv * (t * y3 + inv * y2)) * t); + l = _sqrt(yd * yd + xd * xd); + if (l < min) { + min = l; + } + length += l; + samples[samplesIndex++] = length; + } + } + x1 += x4; + y1 += y4; + } + if (prevLength) { + prevLength -= length; + for (; samplesIndex < samples.length; samplesIndex++) { + samples[samplesIndex] += prevLength; + } + } + if (samples.length && min) { + segment.totalLength = segLength = samples[samples.length-1] || 0; + segment.minLength = min; + if (segLength / min < 9999) { // if the lookup would require too many values (memory problem), we skip this and instead we use a loop to lookup values directly in the samples Array + l = lengthIndex = 0; + for (i = 0; i < segLength; i += min) { + lookup[l++] = (samples[lengthIndex] < i) ? ++lengthIndex : lengthIndex; + } + } + } else { + segment.totalLength = samples[0] = 0; + } + return startIndex ? length - samples[startIndex / 2 - 1] : length; +} + +export function cacheRawPathMeasurements(rawPath, resolution) { + let pathLength, points, i; + for (i = pathLength = points = 0; i < rawPath.length; i++) { + rawPath[i].resolution = ~~resolution || 12; //steps per Bezier curve (anchor, 2 control points, to anchor) + points += rawPath[i].length; + pathLength += measureSegment(rawPath[i]); + } + rawPath.totalPoints = points; + rawPath.totalLength = pathLength; + return rawPath; +} + +//divide segment[i] at position t (value between 0 and 1, progress along that particular cubic bezier segment that starts at segment[i]). Returns how many elements were spliced into the segment array (either 0 or 6) +export function subdivideSegment(segment, i, t) { + if (t <= 0 || t >= 1) { + return 0; + } + let ax = segment[i], + ay = segment[i+1], + cp1x = segment[i+2], + cp1y = segment[i+3], + cp2x = segment[i+4], + cp2y = segment[i+5], + bx = segment[i+6], + by = segment[i+7], + x1a = ax + (cp1x - ax) * t, + x2 = cp1x + (cp2x - cp1x) * t, + y1a = ay + (cp1y - ay) * t, + y2 = cp1y + (cp2y - cp1y) * t, + x1 = x1a + (x2 - x1a) * t, + y1 = y1a + (y2 - y1a) * t, + x2a = cp2x + (bx - cp2x) * t, + y2a = cp2y + (by - cp2y) * t; + x2 += (x2a - x2) * t; + y2 += (y2a - y2) * t; + segment.splice(i + 2, 4, + _round(x1a), //first control point + _round(y1a), + _round(x1), //second control point + _round(y1), + _round(x1 + (x2 - x1) * t), //new fabricated anchor on line + _round(y1 + (y2 - y1) * t), + _round(x2), //third control point + _round(y2), + _round(x2a), //fourth control point + _round(y2a) + ); + segment.samples && segment.samples.splice(((i / 6) * segment.resolution) | 0, 0, 0, 0, 0, 0, 0, 0); + return 6; +} + +// returns an object {path, segment, segIndex, i, t} +function getProgressData(rawPath, progress, decoratee, pushToNextIfAtEnd) { + decoratee = decoratee || {}; + rawPath.totalLength || cacheRawPathMeasurements(rawPath); + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + let segIndex = 0, + segment = rawPath[0], + samples, resolution, length, min, max, i, t; + if (!progress) { + t = i = segIndex = 0; + segment = rawPath[0]; + } else if (progress === 1) { + t = 1; + segIndex = rawPath.length - 1; + segment = rawPath[segIndex]; + i = segment.length - 8; + } else { + if (rawPath.length > 1) { //speed optimization: most of the time, there's only one segment so skip the recursion. + length = rawPath.totalLength * progress; + max = i = 0; + while ((max += rawPath[i++].totalLength) < length) { + segIndex = i; + } + segment = rawPath[segIndex]; + min = max - segment.totalLength; + progress = ((length - min) / (max - min)) || 0; + } + samples = segment.samples; + resolution = segment.resolution; //how many samples per cubic bezier chunk + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[~~(length / segment.minLength)] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i-1] : 0; + max = samples[i]; + if (max < length) { + min = max; + max = samples[++i]; + } + t = (1 / resolution) * (((length - min) / (max - min)) + ((i % resolution))); + i = ~~(i / resolution) * 6; + if (pushToNextIfAtEnd && t === 1) { + if (i + 6 < segment.length) { + i += 6; + t = 0; + } else if (segIndex + 1 < rawPath.length) { + i = t = 0; + segment = rawPath[++segIndex]; + } + } + } + decoratee.t = t; + decoratee.i = i; + decoratee.path = rawPath; + decoratee.segment = segment; + decoratee.segIndex = segIndex; + return decoratee; +} + +export function getPositionOnPath(rawPath, progress, includeAngle, point) { + let segment = rawPath[0], + result = point || {}, + samples, resolution, length, min, max, i, t, a, inv; + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + segment.lookup || cacheRawPathMeasurements(rawPath); + if (rawPath.length > 1) { //speed optimization: most of the time, there's only one segment so skip the recursion. + length = rawPath.totalLength * progress; + max = i = 0; + while ((max += rawPath[i++].totalLength) < length) { + segment = rawPath[i]; + } + min = max - segment.totalLength; + progress = ((length - min) / (max - min)) || 0; + } + samples = segment.samples; + resolution = segment.resolution; + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i-1] : 0; + max = samples[i]; + if (max < length) { + min = max; + max = samples[++i]; + } + t = ((1 / resolution) * (((length - min) / (max - min)) + ((i % resolution)))) || 0; + inv = 1 - t; + i = ~~(i / resolution) * 6; + a = segment[i]; + result.x = _round((t * t * (segment[i + 6] - a) + 3 * inv * (t * (segment[i + 4] - a) + inv * (segment[i + 2] - a))) * t + a); + result.y = _round((t * t * (segment[i + 7] - (a = segment[i+1])) + 3 * inv * (t * (segment[i + 5] - a) + inv * (segment[i + 3] - a))) * t + a); + if (includeAngle) { + result.angle = segment.totalLength ? getRotationAtBezierT(segment, i, t >= 1 ? 1 - 1e-9 : t ? t : 1e-9) : segment.angle || 0; + } + return result; +} + + + +//applies a matrix transform to RawPath (or a segment in a RawPath) and returns whatever was passed in (it transforms the values in the array(s), not a copy). +export function transformRawPath(rawPath, a, b, c, d, tx, ty) { + let j = rawPath.length, + segment, l, i, x, y; + while (--j > -1) { + segment = rawPath[j]; + l = segment.length; + for (i = 0; i < l; i += 2) { + x = segment[i]; + y = segment[i+1]; + segment[i] = x * a + y * c + tx; + segment[i+1] = x * b + y * d + ty; + } + } + rawPath._dirty = 1; + return rawPath; +} + + + +// translates SVG arc data into a segment (cubic beziers). Angle is in degrees. +function arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) { + if (lastX === x && lastY === y) { + return; + } + rx = _abs(rx); + ry = _abs(ry); + let angleRad = (angle % 360) * _DEG2RAD, + cosAngle = _cos(angleRad), + sinAngle = _sin(angleRad), + PI = Math.PI, + TWOPI = PI * 2, + dx2 = (lastX - x) / 2, + dy2 = (lastY - y) / 2, + x1 = (cosAngle * dx2 + sinAngle * dy2), + y1 = (-sinAngle * dx2 + cosAngle * dy2), + x1_sq = x1 * x1, + y1_sq = y1 * y1, + radiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry); + if (radiiCheck > 1) { + rx = _sqrt(radiiCheck) * rx; + ry = _sqrt(radiiCheck) * ry; + } + let rx_sq = rx * rx, + ry_sq = ry * ry, + sq = ((rx_sq * ry_sq) - (rx_sq * y1_sq) - (ry_sq * x1_sq)) / ((rx_sq * y1_sq) + (ry_sq * x1_sq)); + if (sq < 0) { + sq = 0; + } + let coef = ((largeArcFlag === sweepFlag) ? -1 : 1) * _sqrt(sq), + cx1 = coef * ((rx * y1) / ry), + cy1 = coef * -((ry * x1) / rx), + sx2 = (lastX + x) / 2, + sy2 = (lastY + y) / 2, + cx = sx2 + (cosAngle * cx1 - sinAngle * cy1), + cy = sy2 + (sinAngle * cx1 + cosAngle * cy1), + ux = (x1 - cx1) / rx, + uy = (y1 - cy1) / ry, + vx = (-x1 - cx1) / rx, + vy = (-y1 - cy1) / ry, + temp = ux * ux + uy * uy, + angleStart = ((uy < 0) ? -1 : 1) * Math.acos(ux / _sqrt(temp)), + angleExtent = ((ux * vy - uy * vx < 0) ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt(temp * (vx * vx + vy * vy))); + isNaN(angleExtent) && (angleExtent = PI); //rare edge case. Math.cos(-1) is NaN. + if (!sweepFlag && angleExtent > 0) { + angleExtent -= TWOPI; + } else if (sweepFlag && angleExtent < 0) { + angleExtent += TWOPI; + } + angleStart %= TWOPI; + angleExtent %= TWOPI; + let segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)), + rawPath = [], + angleIncrement = angleExtent / segments, + controlLength = 4 / 3 * _sin(angleIncrement / 2) / (1 + _cos(angleIncrement / 2)), + ma = cosAngle * rx, + mb = sinAngle * rx, + mc = sinAngle * -ry, + md = cosAngle * ry, + i; + for (i = 0; i < segments; i++) { + angle = angleStart + i * angleIncrement; + x1 = _cos(angle); + y1 = _sin(angle); + ux = _cos(angle += angleIncrement); + uy = _sin(angle); + rawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy); + } + //now transform according to the actual size of the ellipse/arc (the beziers were noramlized, between 0 and 1 on a circle). + for (i = 0; i < rawPath.length; i+=2) { + x1 = rawPath[i]; + y1 = rawPath[i+1]; + rawPath[i] = x1 * ma + y1 * mc + cx; + rawPath[i+1] = x1 * mb + y1 * md + cy; + } + rawPath[i-2] = x; //always set the end to exactly where it's supposed to be + rawPath[i-1] = y; + return rawPath; +} + +//Spits back a RawPath with absolute coordinates. Each segment starts with a "moveTo" command (x coordinate, then y) and then 2 control points (x, y, x, y), then anchor. The goal is to minimize memory and maximize speed. +export function stringToRawPath(d) { + let a = (d + "").replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp) || [], //some authoring programs spit out very small numbers in scientific notation like "1e-5", so make sure we round that down to 0 first. + path = [], + relativeX = 0, + relativeY = 0, + twoThirds = 2 / 3, + elements = a.length, + points = 0, + errorMessage = "ERROR: malformed path: " + d, + i, j, x, y, command, isRelative, segment, startX, startY, difX, difY, beziers, prevCommand, flag1, flag2, + line = function(sx, sy, ex, ey) { + difX = (ex - sx) / 3; + difY = (ey - sy) / 3; + segment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey); + }; + if (!d || !isNaN(a[0]) || isNaN(a[1])) { + console.log(errorMessage); + return path; + } + for (i = 0; i < elements; i++) { + prevCommand = command; + if (isNaN(a[i])) { + command = a[i].toUpperCase(); + isRelative = (command !== a[i]); //lower case means relative + } else { //commands like "C" can be strung together without any new command characters between. + i--; + } + x = +a[i + 1]; + y = +a[i + 2]; + if (isRelative) { + x += relativeX; + y += relativeY; + } + if (!i) { + startX = x; + startY = y; + } + + // "M" (move) + if (command === "M") { + if (segment) { + if (segment.length < 8) { //if the path data was funky and just had a M with no actual drawing anywhere, skip it. + path.length -= 1; + } else { + points += segment.length; + } + } + relativeX = startX = x; + relativeY = startY = y; + segment = [x, y]; + path.push(segment); + i += 2; + command = "L"; //an "M" with more than 2 values gets interpreted as "lineTo" commands ("L"). + + // "C" (cubic bezier) + } else if (command === "C") { + if (!segment) { + segment = [0, 0]; + } + if (!isRelative) { + relativeX = relativeY = 0; + } + //note: "*1" is just a fast/short way to cast the value as a Number. WAAAY faster in Chrome, slightly slower in Firefox. + segment.push(x, y, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, (relativeX += a[i + 5] * 1), (relativeY += a[i + 6] * 1)); + i += 6; + + // "S" (continuation of cubic bezier) + } else if (command === "S") { + difX = relativeX; + difY = relativeY; + if (prevCommand === "C" || prevCommand === "S") { + difX += relativeX - segment[segment.length - 4]; + difY += relativeY - segment[segment.length - 3]; + } + if (!isRelative) { + relativeX = relativeY = 0; + } + segment.push(difX, difY, x, y, (relativeX += a[i + 3] * 1), (relativeY += a[i + 4] * 1)); + i += 4; + + // "Q" (quadratic bezier) + } else if (command === "Q") { + difX = relativeX + (x - relativeX) * twoThirds; + difY = relativeY + (y - relativeY) * twoThirds; + if (!isRelative) { + relativeX = relativeY = 0; + } + relativeX += a[i + 3] * 1; + relativeY += a[i + 4] * 1; + segment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY); + i += 4; + + // "T" (continuation of quadratic bezier) + } else if (command === "T") { + difX = relativeX - segment[segment.length - 4]; + difY = relativeY - segment[segment.length - 3]; + segment.push(relativeX + difX, relativeY + difY, x + ((relativeX + difX * 1.5) - x) * twoThirds, y + ((relativeY + difY * 1.5) - y) * twoThirds, (relativeX = x), (relativeY = y)); + i += 2; + + // "H" (horizontal line) + } else if (command === "H") { + line(relativeX, relativeY, (relativeX = x), relativeY); + i += 1; + + // "V" (vertical line) + } else if (command === "V") { + //adjust values because the first (and only one) isn't x in this case, it's y. + line(relativeX, relativeY, relativeX, (relativeY = x + (isRelative ? relativeY - relativeX : 0))); + i += 1; + + // "L" (line) or "Z" (close) + } else if (command === "L" || command === "Z") { + if (command === "Z") { + x = startX; + y = startY; + segment.closed = true; + } + if (command === "L" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) { + line(relativeX, relativeY, x, y); + if (command === "L") { + i += 2; + } + } + relativeX = x; + relativeY = y; + + // "A" (arc) + } else if (command === "A") { + flag1 = a[i+4]; + flag2 = a[i+5]; + difX = a[i+6]; + difY = a[i+7]; + j = 7; + if (flag1.length > 1) { // for cases when the flags are merged, like "a8 8 0 018 8" (the 0 and 1 flags are WITH the x value of 8, but it could also be "a8 8 0 01-8 8" so it may include x or not) + if (flag1.length < 3) { + difY = difX; + difX = flag2; + j--; + } else { + difY = flag2; + difX = flag1.substr(2); + j-=2; + } + flag2 = flag1.charAt(1); + flag1 = flag1.charAt(0); + } + beziers = arcToSegment(relativeX, relativeY, +a[i+1], +a[i+2], +a[i+3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX*1, (isRelative ? relativeY : 0) + difY*1); + i += j; + if (beziers) { + for (j = 0; j < beziers.length; j++) { + segment.push(beziers[j]); + } + } + relativeX = segment[segment.length-2]; + relativeY = segment[segment.length-1]; + + } else { + console.log(errorMessage); + } + } + i = segment.length; + if (i < 6) { //in case there's odd SVG like a M0,0 command at the very end. + path.pop(); + i = 0; + } else if (segment[0] === segment[i-2] && segment[1] === segment[i-1]) { + segment.closed = true; + } + path.totalPoints = points + i; + return path; +} + +//populates the points array in alternating x/y values (like [x, y, x, y...] instead of individual point objects [{x, y}, {x, y}...] to conserve memory and stay in line with how we're handling segment arrays +export function bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) { + let x12 = (x1 + x2) / 2, + y12 = (y1 + y2) / 2, + x23 = (x2 + x3) / 2, + y23 = (y2 + y3) / 2, + x34 = (x3 + x4) / 2, + y34 = (y3 + y4) / 2, + x123 = (x12 + x23) / 2, + y123 = (y12 + y23) / 2, + x234 = (x23 + x34) / 2, + y234 = (y23 + y34) / 2, + x1234 = (x123 + x234) / 2, + y1234 = (y123 + y234) / 2, + dx = x4 - x1, + dy = y4 - y1, + d2 = _abs((x2 - x4) * dy - (y2 - y4) * dx), + d3 = _abs((x3 - x4) * dy - (y3 - y4) * dx), + length; + if (!points) { + points = [x1, y1, x4, y4]; + index = 2; + } + points.splice(index || points.length - 2, 0, x1234, y1234); + if ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) { + length = points.length; + bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index); + bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 2 + (points.length - length)); + } + return points; +} + +/* +function getAngleBetweenPoints(x0, y0, x1, y1, x2, y2) { //angle between 3 points in radians + var dx1 = x1 - x0, + dy1 = y1 - y0, + dx2 = x2 - x1, + dy2 = y2 - y1, + dx3 = x2 - x0, + dy3 = y2 - y0, + a = dx1 * dx1 + dy1 * dy1, + b = dx2 * dx2 + dy2 * dy2, + c = dx3 * dx3 + dy3 * dy3; + return Math.acos( (a + b - c) / _sqrt(4 * a * b) ); +}, +*/ + +//pointsToSegment() doesn't handle flat coordinates (where y is always 0) the way we need (the resulting control points are always right on top of the anchors), so this function basically makes the control points go directly up and down, varying in length based on the curviness (more curvy, further control points) +export function flatPointsToSegment(points, curviness=1) { + let x = points[0], + y = 0, + segment = [x, y], + i = 2; + for (; i < points.length; i+=2) { + segment.push( + x, + y, + points[i], + (y = (points[i] - x) * curviness / 2), + (x = points[i]), + -y + ); + } + return segment; +} + +//points is an array of x/y points, like [x, y, x, y, x, y] +export function pointsToSegment(points, curviness) { + //points = simplifyPoints(points, tolerance); + _abs(points[0] - points[2]) < 1e-4 && _abs(points[1] - points[3]) < 1e-4 && (points = points.slice(2)); // if the first two points are super close, dump the first one. + let l = points.length-2, + x = +points[0], + y = +points[1], + nextX = +points[2], + nextY = +points[3], + segment = [x, y, x, y], + dx2 = nextX - x, + dy2 = nextY - y, + closed = Math.abs(points[l] - x) < 0.001 && Math.abs(points[l+1] - y) < 0.001, + prevX, prevY, i, dx1, dy1, r1, r2, r3, tl, mx1, mx2, mxm, my1, my2, mym; + if (closed) { // if the start and end points are basically on top of each other, close the segment by adding the 2nd point to the end, and the 2nd-to-last point to the beginning (we'll remove them at the end, but this allows the curvature to look perfect) + points.push(nextX, nextY); + nextX = x; + nextY = y; + x = points[l-2]; + y = points[l-1]; + points.unshift(x, y); + l+=4; + } + curviness = (curviness || curviness === 0) ? +curviness : 1; + for (i = 2; i < l; i+=2) { + prevX = x; + prevY = y; + x = nextX; + y = nextY; + nextX = +points[i+2]; + nextY = +points[i+3]; + if (x === nextX && y === nextY) { + continue; + } + dx1 = dx2; + dy1 = dy2; + dx2 = nextX - x; + dy2 = nextY - y; + r1 = _sqrt(dx1 * dx1 + dy1 * dy1); // r1, r2, and r3 correlate x and y (and z in the future). Basically 2D or 3D hypotenuse + r2 = _sqrt(dx2 * dx2 + dy2 * dy2); + r3 = _sqrt((dx2 / r2 + dx1 / r1) ** 2 + (dy2 / r2 + dy1 / r1) ** 2); + tl = ((r1 + r2) * curviness * 0.25) / r3; + mx1 = x - (x - prevX) * (r1 ? tl / r1 : 0); + mx2 = x + (nextX - x) * (r2 ? tl / r2 : 0); + mxm = x - (mx1 + (((mx2 - mx1) * ((r1 * 3 / (r1 + r2)) + 0.5) / 4) || 0)); + my1 = y - (y - prevY) * (r1 ? tl / r1 : 0); + my2 = y + (nextY - y) * (r2 ? tl / r2 : 0); + mym = y - (my1 + (((my2 - my1) * ((r1 * 3 / (r1 + r2)) + 0.5) / 4) || 0)); + if (x !== prevX || y !== prevY) { + segment.push( + _round(mx1 + mxm), // first control point + _round(my1 + mym), + _round(x), // anchor + _round(y), + _round(mx2 + mxm), // second control point + _round(my2 + mym) + ); + } + } + x !== nextX || y !== nextY || segment.length < 4 ? segment.push(_round(nextX), _round(nextY), _round(nextX), _round(nextY)) : (segment.length -= 2); + if (segment.length === 2) { // only one point! + segment.push(x, y, x, y, x, y); + } else if (closed) { + segment.splice(0, 6); + segment.length = segment.length - 6; + } + return segment; +} + +//returns the squared distance between an x/y coordinate and a segment between x1/y1 and x2/y2 +function pointToSegDist(x, y, x1, y1, x2, y2) { + let dx = x2 - x1, + dy = y2 - y1, + t; + if (dx || dy) { + t = ((x - x1) * dx + (y - y1) * dy) / (dx * dx + dy * dy); + if (t > 1) { + x1 = x2; + y1 = y2; + } else if (t > 0) { + x1 += dx * t; + y1 += dy * t; + } + } + return (x - x1) ** 2 + (y - y1) ** 2; +} + +function simplifyStep(points, first, last, tolerance, simplified) { + let maxSqDist = tolerance, + firstX = points[first], + firstY = points[first+1], + lastX = points[last], + lastY = points[last+1], + index, i, d; + for (i = first + 2; i < last; i += 2) { + d = pointToSegDist(points[i], points[i+1], firstX, firstY, lastX, lastY); + if (d > maxSqDist) { + index = i; + maxSqDist = d; + } + } + if (maxSqDist > tolerance) { + index - first > 2 && simplifyStep(points, first, index, tolerance, simplified); + simplified.push(points[index], points[index+1]); + last - index > 2 && simplifyStep(points, index, last, tolerance, simplified); + } +} + +//points is an array of x/y values like [x, y, x, y, x, y] +export function simplifyPoints(points, tolerance) { + let prevX = parseFloat(points[0]), + prevY = parseFloat(points[1]), + temp = [prevX, prevY], + l = points.length - 2, + i, x, y, dx, dy, result, last; + tolerance = (tolerance || 1) ** 2; + for (i = 2; i < l; i += 2) { + x = parseFloat(points[i]); + y = parseFloat(points[i+1]); + dx = prevX - x; + dy = prevY - y; + if (dx * dx + dy * dy > tolerance) { + temp.push(x, y); + prevX = x; + prevY = y; + } + } + temp.push(parseFloat(points[l]), parseFloat(points[l+1])); + last = temp.length - 2; + result = [temp[0], temp[1]]; + simplifyStep(temp, 0, last, tolerance, result); + result.push(temp[last], temp[last+1]); + return result; +} + +function getClosestProgressOnBezier(iterations, px, py, start, end, slices, x0, y0, x1, y1, x2, y2, x3, y3) { + let inc = (end - start) / slices, + best = 0, + t = start, + x, y, d, dx, dy, inv; + _bestDistance = _largeNum; + while (t <= end) { + inv = 1 - t; + x = inv * inv * inv * x0 + 3 * inv * inv * t * x1 + 3 * inv * t * t * x2 + t * t * t * x3; + y = inv * inv * inv * y0 + 3 * inv * inv * t * y1 + 3 * inv * t * t * y2 + t * t * t * y3; + dx = x - px; + dy = y - py; + d = dx * dx + dy * dy; + if (d < _bestDistance) { + _bestDistance = d; + best = t; + } + t += inc; + } + return (iterations > 1) ? getClosestProgressOnBezier(iterations - 1, px, py, Math.max(best - inc, 0), Math.min(best + inc, 1), slices, x0, y0, x1, y1, x2, y2, x3, y3) : best; +} + +export function getClosestData(rawPath, x, y, slices) { //returns an object with the closest j, i, and t (j is the segment index, i is the index of the point in that segment, and t is the time/progress along that bezier) + let closest = {j:0, i:0, t:0}, + bestDistance = _largeNum, + i, j, t, segment; + for (j = 0; j < rawPath.length; j++) { + segment = rawPath[j]; + for (i = 0; i < segment.length; i+=6) { + t = getClosestProgressOnBezier(1, x, y, 0, 1, slices || 20, segment[i], segment[i+1], segment[i+2], segment[i+3], segment[i+4], segment[i+5], segment[i+6], segment[i+7]); + if (bestDistance > _bestDistance) { + bestDistance = _bestDistance; + closest.j = j; + closest.i = i; + closest.t = t; + } + } + } + return closest; +} + +//subdivide a Segment closest to a specific x,y coordinate +export function subdivideSegmentNear(x, y, segment, slices, iterations) { + let l = segment.length, + bestDistance = _largeNum, + bestT = 0, + bestSegmentIndex = 0, + t, i; + slices = slices || 20; + iterations = iterations || 3; + for (i = 0; i < l; i += 6) { + t = getClosestProgressOnBezier(1, x, y, 0, 1, slices, segment[i], segment[i+1], segment[i+2], segment[i+3], segment[i+4], segment[i+5], segment[i+6], segment[i+7]); + if (bestDistance > _bestDistance) { + bestDistance = _bestDistance; + bestT = t; + bestSegmentIndex = i; + } + } + t = getClosestProgressOnBezier(iterations, x, y, bestT - 0.05, bestT + 0.05, slices, segment[bestSegmentIndex], segment[bestSegmentIndex+1], segment[bestSegmentIndex+2], segment[bestSegmentIndex+3], segment[bestSegmentIndex+4], segment[bestSegmentIndex+5], segment[bestSegmentIndex+6], segment[bestSegmentIndex+7]); + subdivideSegment(segment, bestSegmentIndex, t); + return bestSegmentIndex + 6; +} + +/* +Takes any of the following and converts it to an all Cubic Bezier SVG data string: +- A <path> data string like "M0,0 L2,4 v20,15 H100" +- A RawPath, like [[x, y, x, y, x, y, x, y][[x, y, x, y, x, y, x, y]] +- A Segment, like [x, y, x, y, x, y, x, y] + +Note: all numbers are rounded down to the closest 0.001 to minimize memory, maximize speed, and avoid odd numbers like 1e-13 +*/ +export function rawPathToString(rawPath) { + if (_isNumber(rawPath[0])) { //in case a segment is passed in instead + rawPath = [rawPath]; + } + let result = "", + l = rawPath.length, + sl, s, i, segment; + for (s = 0; s < l; s++) { + segment = rawPath[s]; + result += "M" + _round(segment[0]) + "," + _round(segment[1]) + " C"; + sl = segment.length; + for (i = 2; i < sl; i++) { + result += _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i]) + " "; + } + if (segment.closed) { + result += "z"; + } + } + return result; +} + +/* +// takes a segment with coordinates [x, y, x, y, ...] and converts the control points into angles and lengths [x, y, angle, length, angle, length, x, y, angle, length, ...] so that it animates more cleanly and avoids odd breaks/kinks. For example, if you animate from 1 o'clock to 6 o'clock, it'd just go directly/linearly rather than around. So the length would be very short in the middle of the tween. +export function cpCoordsToAngles(segment, copy) { + var result = copy ? segment.slice(0) : segment, + x, y, i; + for (i = 0; i < segment.length; i+=6) { + x = segment[i+2] - segment[i]; + y = segment[i+3] - segment[i+1]; + result[i+2] = Math.atan2(y, x); + result[i+3] = Math.sqrt(x * x + y * y); + x = segment[i+6] - segment[i+4]; + y = segment[i+7] - segment[i+5]; + result[i+4] = Math.atan2(y, x); + result[i+5] = Math.sqrt(x * x + y * y); + } + return result; +} + +// takes a segment that was converted with cpCoordsToAngles() to have angles and lengths instead of coordinates for the control points, and converts it BACK into coordinates. +export function cpAnglesToCoords(segment, copy) { + var result = copy ? segment.slice(0) : segment, + length = segment.length, + rnd = 1000, + angle, l, i, j; + for (i = 0; i < length; i+=6) { + angle = segment[i+2]; + l = segment[i+3]; //length + result[i+2] = (((segment[i] + Math.cos(angle) * l) * rnd) | 0) / rnd; + result[i+3] = (((segment[i+1] + Math.sin(angle) * l) * rnd) | 0) / rnd; + angle = segment[i+4]; + l = segment[i+5]; //length + result[i+4] = (((segment[i+6] - Math.cos(angle) * l) * rnd) | 0) / rnd; + result[i+5] = (((segment[i+7] - Math.sin(angle) * l) * rnd) | 0) / rnd; + } + return result; +} + +//adds an "isSmooth" array to each segment and populates it with a boolean value indicating whether or not it's smooth (the control points have basically the same slope). For any smooth control points, it converts the coordinates into angle (x, in radians) and length (y) and puts them into the same index value in a smoothData array. +export function populateSmoothData(rawPath) { + let j = rawPath.length, + smooth, segment, x, y, x2, y2, i, l, a, a2, isSmooth, smoothData; + while (--j > -1) { + segment = rawPath[j]; + isSmooth = segment.isSmooth = segment.isSmooth || [0, 0, 0, 0]; + smoothData = segment.smoothData = segment.smoothData || [0, 0, 0, 0]; + isSmooth.length = 4; + l = segment.length - 2; + for (i = 6; i < l; i += 6) { + x = segment[i] - segment[i - 2]; + y = segment[i + 1] - segment[i - 1]; + x2 = segment[i + 2] - segment[i]; + y2 = segment[i + 3] - segment[i + 1]; + a = _atan2(y, x); + a2 = _atan2(y2, x2); + smooth = (Math.abs(a - a2) < 0.09); + if (smooth) { + smoothData[i - 2] = a; + smoothData[i + 2] = a2; + smoothData[i - 1] = _sqrt(x * x + y * y); + smoothData[i + 3] = _sqrt(x2 * x2 + y2 * y2); + } + isSmooth.push(smooth, smooth, 0, 0, smooth, smooth); + } + //if the first and last points are identical, check to see if there's a smooth transition. We must handle this a bit differently due to their positions in the array. + if (segment[l] === segment[0] && segment[l+1] === segment[1]) { + x = segment[0] - segment[l-2]; + y = segment[1] - segment[l-1]; + x2 = segment[2] - segment[0]; + y2 = segment[3] - segment[1]; + a = _atan2(y, x); + a2 = _atan2(y2, x2); + if (Math.abs(a - a2) < 0.09) { + smoothData[l-2] = a; + smoothData[2] = a2; + smoothData[l-1] = _sqrt(x * x + y * y); + smoothData[3] = _sqrt(x2 * x2 + y2 * y2); + isSmooth[l-2] = isSmooth[l-1] = true; //don't change indexes 2 and 3 because we'll trigger everything from the END, and this will optimize file size a bit. + } + } + } + return rawPath; +} +export function pointToScreen(svgElement, point) { + if (arguments.length < 2) { //by default, take the first set of coordinates in the path as the point + let rawPath = getRawPath(svgElement); + point = svgElement.ownerSVGElement.createSVGPoint(); + point.x = rawPath[0][0]; + point.y = rawPath[0][1]; + } + return point.matrixTransform(svgElement.getScreenCTM()); +} +// takes a <path> and normalizes all of its coordinates to values between 0 and 1 +export function normalizePath(path) { + path = gsap.utils.toArray(path); + if (!path[0].hasAttribute("d")) { + path = gsap.utils.toArray(path[0].children); + } + if (path.length > 1) { + path.forEach(normalizePath); + return path; + } + let _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + d = path[0].getAttribute("d"), + a = d.replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp), + nums = a.filter(n => !isNaN(n)).map(n => +n), + normalize = gsap.utils.normalize(Math.min(...nums), Math.max(...nums)), + finals = a.map(val => isNaN(val) ? val : normalize(+val)), + s = "", + prevWasCommand; + finals.forEach((value, i) => { + let isCommand = isNaN(value) + s += (isCommand && i ? " " : prevWasCommand || !i ? "" : ",") + value; + prevWasCommand = isCommand; + }); + path[0].setAttribute("d", s); +} +*/ \ No newline at end of file diff --git a/node_modules/gsap/src/utils/strings.js b/node_modules/gsap/src/utils/strings.js new file mode 100644 index 0000000000000000000000000000000000000000..4dc95890b01001c1db1e3bf76fc59c3815eec961 --- /dev/null +++ b/node_modules/gsap/src/utils/strings.js @@ -0,0 +1,93 @@ +/*! + * strings: 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ +/* eslint-disable */ + +let _trimExp = /(?:^\s+|\s+$)/g; + +export const emojiExp = /([\uD800-\uDBFF][\uDC00-\uDFFF](?:[\u200D\uFE0F][\uD800-\uDBFF][\uDC00-\uDFFF]){2,}|\uD83D\uDC69(?:\u200D(?:(?:\uD83D\uDC69\u200D)?\uD83D\uDC67|(?:\uD83D\uDC69\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2642\u2640]\uFE0F|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDD27\uDCBC\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC6F\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3C-\uDD3E\uDDD6-\uDDDF])\u200D[\u2640\u2642]\uFE0F|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|(?:\u26F9|\uD83C[\uDFCC\uDFCB]|\uD83D\uDD75)(?:\uFE0F\u200D[\u2640\u2642]|(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642])\uFE0F|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\uD83D\uDC69\u200D[\u2695\u2696\u2708]|\uD83D\uDC68(?:(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708]))\uFE0F|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83D\uDC69\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|[#\*0-9]\uFE0F\u20E3|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|\uD83D\uDC68(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]))|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uD83C[\uDFFB-\uDFFF])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270A-\u270D]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC70\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDCAA\uDD74\uDD7A\uDD90\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD36\uDDD1-\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\u200D(?:(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC69\uDC6E\uDC70-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD26\uDD30-\uDD39\uDD3D\uDD3E\uDDD1-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])?|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDEEB\uDEEC\uDEF4-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u2660\u2663\u2665\u2666\u2668\u267B\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])\uFE0F)/; + +export function getText(e) { + let type = e.nodeType, + result = ""; + if (type === 1 || type === 9 || type === 11) { + if (typeof(e.textContent) === "string") { + return e.textContent; + } else { + for (e = e.firstChild; e; e = e.nextSibling ) { + result += getText(e); + } + } + } else if (type === 3 || type === 4) { + return e.nodeValue; + } + return result; +} + +export function splitInnerHTML(element, delimiter, trim, preserveSpaces, unescapedCharCodes) { + let node = element.firstChild, + result = [], s; + while (node) { + if (node.nodeType === 3) { + s = (node.nodeValue + "").replace(/^\n+/g, ""); + if (!preserveSpaces) { + s = s.replace(/\s+/g, " "); + } + result.push(...emojiSafeSplit(s, delimiter, trim, preserveSpaces, unescapedCharCodes)); + } else if ((node.nodeName + "").toLowerCase() === "br") { + result[result.length-1] += "<br>"; + } else { + result.push(node.outerHTML); + } + node = node.nextSibling; + } + if (!unescapedCharCodes) { + s = result.length; + while (s--) { + result[s] === "&" && result.splice(s, 1, "&"); + } + } + return result; +} + +/* +//smaller kb version that only handles the simpler emoji's, which is often perfectly adequate. + +let _emoji = "[\uE000-\uF8FF]|\uD83C[\uDC00-\uDFFF]|\uD83D[\uDC00-\uDFFF]|[\u2694-\u2697]|\uD83E[\uDD10-\uDD5D]|[\uD800-\uDBFF][\uDC00-\uDFFF]", + _emojiExp = new RegExp(_emoji), + _emojiAndCharsExp = new RegExp(_emoji + "|.", "g"), + _emojiSafeSplit = (text, delimiter, trim) => { + if (trim) { + text = text.replace(_trimExp, ""); + } + return ((delimiter === "" || !delimiter) && _emojiExp.test(text)) ? text.match(_emojiAndCharsExp) : text.split(delimiter || ""); + }; + */ +export function emojiSafeSplit(text, delimiter, trim, preserveSpaces, unescapedCharCodes) { + text += ""; // make sure it's cast as a string. Someone may pass in a number. + trim && (text = text.trim ? text.trim() : text.replace(_trimExp, "")); // IE9 and earlier compatibility + if (delimiter && delimiter !== "") { + return text.replace(/>/g, ">").replace(/</g, "<").split(delimiter); + } + let result = [], + l = text.length, + i = 0, + j, character; + for (; i < l; i++) { + character = text.charAt(i); + if ((character.charCodeAt(0) >= 0xD800 && character.charCodeAt(0) <= 0xDBFF) || (text.charCodeAt(i+1) >= 0xFE00 && text.charCodeAt(i+1) <= 0xFE0F)) { //special emoji characters use 2 or 4 unicode characters that we must keep together. + j = ((text.substr(i, 12).split(emojiExp) || [])[1] || "").length || 2; + character = text.substr(i, j); + result.emoji = 1; + i += j - 1; + } + result.push(unescapedCharCodes ? character : character === ">" ? ">" : (character === "<") ? "<" : preserveSpaces && character === " " && (text.charAt(i-1) === " " || text.charAt(i+1) === " ") ? " " : character); + } + return result; +} \ No newline at end of file diff --git a/node_modules/gsap/types/animation.d.ts b/node_modules/gsap/types/animation.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..b4154f9818283f5c52d57a9c54ac57fe08f6cd5e --- /dev/null +++ b/node_modules/gsap/types/animation.d.ts @@ -0,0 +1,639 @@ +declare namespace gsap.core { + + // Added to TypeScript 3.5 + type Omit<T, K extends keyof any> = Pick<T, Exclude<keyof T, K>>; + + class Animation { + + static readonly version: string; + + data: any; + readonly parent: Timeline | null; + readonly scrollTrigger?: ScrollTrigger; + + constructor(vars?: object, time?: number); + + /** + * Sets the delay before the start of the animation. + * + * ```js + * anim.delay(1); + * ``` + * + * @param {number} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/delay() + */ + delay(value: number): this; + /** + * Gets the delay before the start of the animation. + * + * ```js + * anim.delay(); + * ``` + * + * @returns {number} The delay value + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/delay() + */ + delay(): number; + + /** + * Sets the duration of the animation. + * + * ```js + * anim.duration(1); + * ``` + * + * @param {number} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/duration() + */ + duration(value: number): this; + /** + * Gets the duration of the animation. + * + * ```js + * anim.duration(); + * ``` + * + * @returns {number} The duration + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/duration() + */ + duration(): number; + + /** + * Returns the time at which the animation will finish according to the parent timeline's local time. + * + * ```js + * anim.endTime() // the time, e.g. something like 17.854 + * ``` + * + * @returns {number} The end time + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/endTime() + */ + endTime(includeRepeats?: boolean): number; + + /** + * Sets a callback of the animation. + * + * ```js + * anim.eventCallback("onComplete", myCompleteCallback); + * ``` + * + * @param {CallbackType} type + * @param {Callback | null} callback + * @param {any[]} [params] + * @param {object} [scope] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/eventCallback() + */ + eventCallback(type: CallbackType, callback: Callback | null, params?: any[], scope?: object): this; + /** + * Gets the requested callback function of the animation. + * + * ```js + * anim.eventCallback("onComplete"); // function or undefined + * ``` + * + * @param {CallbackType} type + * @returns {Callback} The callback + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/eventCallback() + */ + eventCallback(type: CallbackType): Callback; + + /** + * Forces new starting & ending values based on the current state. + * + * ```js + * anim.invalidate(); + * ``` + * + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/invalidate() + */ + invalidate(): this; + + /** + * Returns true or false based on the active state of the animation. + * Being active means that the virtual playhead is actively moving across this instance's time span and it is not paused, nor are any of its ancestor timelines. + * + * ```js + * anim.isActive(); + * ``` + * + * @returns {boolean} The active state + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/isActive() + */ + isActive(): boolean; + + /** + * Sets the current iteration of the animation. + * + * ```js + * anim.iteration(1); // set the state back to the start in this case + * ``` + * + * @param {number} value + * @param {boolean} [suppressEvents] + * @returns {Animation} The tween + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/iteration() + */ + iteration(value: number, suppressEvents?: boolean): this; + /** + * Gets the current iteration of the animation. + * + * ```js + * anim.iteration(); // getter + * ``` + * + * @returns {number} The iteration + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/iteration() + */ + iteration(): number; + + /** + * Kills the animation entirely. + * To kill means to immediately stop the animation, remove it from its parent timeline, and release it for garbage collection. + * + * ```js + * anim.kill(); + * ``` + * + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/kill() + */ + kill(): this; + + /** + * Pauses the animation, optionally at the given time. + * + * ```js + * anim.pause(); // pause immediately + * anim.pause(1.5); // pause but seek to this time in the animation + * ``` + * + * @param {number} [atTime] + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/pause() + */ + pause(atTime?: number | string, suppressEvents?: boolean): this; + + /** + * Sets the paused state of the animation. + * + * ```js + * anim.paused(true); // pause immediately + * ``` + * + * @param {boolean} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/paused() + */ + paused(value: boolean): this; + /** + * Gets the paused state of the animation. + * + * ```js + * anim.paused(); // returns true or false + * ``` + * + * @returns {boolean} The paused state + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/paused() + */ + paused(): boolean; + + /** + * Plays the animation, optionally from the given start time. + * + * ```js + * anim.play(true); // play from current point + * anim.play(1.5); // play from the 1.5 second mark + * ``` + * + * @param {number} [from] + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/play() + */ + play(from?: number | string | null, suppressEvents?: boolean): this; + + /** + * Sets the progress of the animation (between 0 and 1). + * + * ```js + * anim.progress(0.5); // go to the half way point + * ``` + * + * @param {number} value + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/progress() + */ + progress(value: number, suppressEvents?: boolean): this; + /** + * Gets the progress of the animation. + * + * ```js + * anim.progress(); // returns the progress like 0.5 + * ``` + * + * @returns {number} The progress + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/progress() + */ + progress(): number; + + rawTime(wrapRepeats?: boolean): number; + + /** + * Forces things to render at a certain time WITHOUT adjusting the animation's position in its parent timeline + * + * ```js + * anim.render(1); + * ``` + * + * @param {number} totalTime + * @param {boolean} [suppressEvents] + * @param {boolean} [force] + * @returns {Animation} The animation + * @memberof Animation + */ + render(totalTime: number, suppressEvents?: boolean, force?: boolean): this; + + /** + * Sets the number of repeats of the animation. + * + * ```js + * anim.repeat(1); // sets repeat to 1 for a total iteration count of 2 + * ``` + * + * @param {number} [value] + * @returns {Animation} The animation + * @memberof Animation + */ + repeat(value: number): this; + /** + * Gets the number of repeats of the animation. + * + * ```js + * anim.repeat(); + * ``` + * + * @returns {number} The repeat value + * @memberof Animation + */ + repeat(): number; + + /** + * Sets the repeat delay (time between iterations) of the animation. + * + * ```js + * anim.repeatDelay(1); + * ``` + * + * @param {number} [value] + * @returns {Animation} The animation + * @memberof Animation + */ + repeatDelay(value: number): this; + /** + * Gets the repeat delay (time between iterations) of the animation. + * + * ```js + * anim.repeatDelay(); + * ``` + * + * @returns {number} The repeatDelay value + * @memberof Animation + */ + repeatDelay(): number; + + /** + * Begins playing the animation forward from the beginning, optionally including the starting delay. + * + * ```js + * anim.restart(); // repeats the animation NOT including the starting delay + * anim.restart(true); // repeats the animation including the starting delay + * ``` + * + * @param {number} [includeDelay] + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/restart() + */ + restart(includeDelay?: boolean, suppressEvents?: boolean): this; + + /** + * Continues a paused animation in the direction it was headed (forwards or reverse), optionally from the given time. + * + * ```js + * anim.resume(); // continues the animation + * anim.resume(1); // continues the animation from the 1 second mark + * ``` + * + * @param {number} [from] + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/resume() + */ + resume(from?: number | string, suppressEvents?: boolean): this; + + /** + * Plays an animation in the reverse direction, optionally from the given time. + * + * ```js + * anim.reverse(); // plays the animation in reverse + * anim.reverse(1); // plays the animation in reverse from the 1 second mark + * ``` + * + * @param {number} [from] + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/reverse() + */ + reverse(from?: number | string, suppressEvents?: boolean): this; + + /** + * Sets the reversed state of the animation. + * + * ```js + * anim.reversed(true); // plays the animation in reverse + * ``` + * + * @param {boolean} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/reversed() + */ + reversed(value: boolean): this; + /** + * Gets the reversed state of the animation. + * + * ```js + * anim.reversed(); // true or false + * ``` + * + * @returns {boolean} The reversed state + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/reversed() + */ + reversed(): boolean; + + /** + * Reverts the animation, returning the targets to their pre-animation state including the removal of inline styles added by the animation. + * + * ```js + * anim.revert(); + * ``` + * + * @param {object} [config] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/revert() + */ + revert(config?: object): this; + + /** + * Sets the start time of the animation in reference to its parent timeline (not including any delay). + * + * ```js + * anim.startTime(1); // plays the animation at the 1 second mark of the parent timeline + * ``` + * + * @param {number} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/startTime() + */ + startTime(value: number): this; + + /** + * Gets the start time of the animation in reference to its parent timeline (not including any delay). + * + * ```js + * anim.startTime(); // the current start time, something like 1.0 + * ``` + * + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/startTime() + */ + startTime(): number; + + /** + * Sets the current time of the given animation using a time or a label. + * + * ```js + * anim.seek(1); // moves the playhead to the 1 second mark + * ``` + * + * @param {number| string} time + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + */ + seek(time: number | string, suppressEvents?: boolean): this; + + /** + * Returns a promise for the given animation. + * + * ```js + * anim.then(yourFunction).then(...); + * ``` + * + * @param {Function} [onFulfilled] + * @returns {Promise} A promise for the completion of the animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/then() + */ + then(onFulfilled?: (result: Omit<this, "then">) => any): Promise<this>; + + /** + * Sets the current time of the given animation. + * + * ```js + * anim.time(1); // moves the playhead to the 1 second mark + * ``` + * + * @param {number} value + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/time() + */ + time(value: number, suppressEvents?: boolean): this; + /** + * Gets the current time of the given animation. + * + * ```js + * anim.time(); // the current time, e.g. something like 1.0 + * ``` + * + * @returns {number} The current time + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/time() + */ + time(): number; + + /** + * Sets the time scale of the given animation. + * + * ```js + * anim.timeScale(2.0); // makes the animation go at twice the normal speed + * ``` + * + * @param {number} value + * @param {boolean} suppressEvents + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/timeScale() + */ + timeScale(value: number, suppressEvents?: boolean): this; + + /** + * Gets the time scale of the given animation. + * + * ```js + * anim.timeScale(); // the + * ``` + * + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/timeScale() + */ + timeScale(): number; + + /** + * Sets the total duration (including repeats) of the given animation by time scaling the animation. + * + * ```js + * anim.totalDuration(8); + * ``` + * + * @param {number} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/totalDuration() + */ + totalDuration(value: number): this; + + /** + * Gets the total duration (including repeats) of the given animation. + * + * ```js + * anim.totalDuration(); // the total duration, e.g. something like 5.7 + * ``` + * + * @returns {number} The total duration + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/totalDuration() + */ + totalDuration(): number; + + /** + * Sets the total progress (including repeats) of the given animation. + * + * ```js + * anim.totalProgress(0.5); // move the playhead to half way through the animation (including repeats) + * ``` + * + * @param {number} value + * @param {boolean} suppressEvents + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/totalProgress() + */ + totalProgress(value: number, suppressEvents?: boolean): this; + + /** + * Gets the total progress (including repeats) of the given animation. + * + * ```js + * anim.totalProgress(); // the total progress, e.g. something like 0.5 + * ``` + * + * @returns {number} The total progress + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/totalProgress() + */ + totalProgress(): number; + + /** + * Sets the total time (meaning where the playhead is, including repeats) of the given animation. + * + * ```js + * anim.totalTime(5.7); // move the playhead to the 5.7 mark of the animation (including repeats) + * ``` + * + * @param {number} value + * @param {boolean} [suppressEvents] + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/totalTime() + */ + totalTime(value: number, suppressEvents?: boolean): this; + + /** + * Gets the total time (meaning where the playhead is, including repeats) of the given animation. + * + * ```js + * anim.totalTime(); // the total time, e.g. something like 5.7 + * ``` + * + * @returns {number} The total time + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/totalTime() + */ + totalTime(): number; + + /** + * Sets the yoyo value of the given animation. + * Setting yoyo to true means that each time the animation repeats it should switch its direction (forwards or reverse). + * + * ```js + * anim.yoyo(true); + * ``` + * + * @param {boolean} value + * @returns {Animation} The animation + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/yoyo() + */ + yoyo(value: boolean): this; + + /** + * Gets the yoyo value of the given animation. + * If yoyo is true that means that each time the animation repeats it should switch its direction (forwards or reverse). + * + * ```js + * anim.yoyo(); // true or false + * ``` + * + * @returns {boolean} The yoyo value + * @memberof Animation + * @link https://greensock.com/docs/v3/GSAP/Tween/yoyo() + */ + yoyo(): boolean; + } +} diff --git a/node_modules/gsap/types/css-plugin.d.ts b/node_modules/gsap/types/css-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..6d1c09208b818cd4720af38c188ddd89158739cc --- /dev/null +++ b/node_modules/gsap/types/css-plugin.d.ts @@ -0,0 +1,589 @@ +declare namespace gsap { + + // GSAP specific + interface CSSProperties { + [key: string]: any; + alpha: TweenValue; + autoAlpha: TweenValue; + rotate: TweenValue; + rotateX: TweenValue; + rotateY: TweenValue; + rotateZ: TweenValue; + rotation: TweenValue; + rotationX: TweenValue; + rotationY: TweenValue; + rotationZ: TweenValue; + scale: TweenValue; + scaleX: TweenValue; + scaleY: TweenValue; + skew: TweenValue; + skewX: TweenValue; + skewY: TweenValue; + smoothOrigin: BooleanValue; + svgOrigin: TweenValue; + translateX: TweenValue; + translateY: TweenValue; + translateZ: TweenValue; + x: TweenValue; + xPercent: TweenValue; + y: TweenValue; + yPercent: TweenValue; + z: TweenValue; + } + + interface CSSProperties { + alignContent: TweenValue; + alignItems: TweenValue; + alignSelf: TweenValue; + alignmentBaseline: TweenValue; + animation: TweenValue; + animationDelay: TweenValue; + animationDirection: TweenValue; + animationDuration: TweenValue; + animationFillMode: TweenValue; + animationIterationCount: TweenValue; + animationName: TweenValue; + animationPlayState: TweenValue; + animationTimingFunction: TweenValue; + backfaceVisibility: TweenValue; + background: TweenValue; + backgroundAttachment: TweenValue; + backgroundClip: TweenValue; + backgroundColor: TweenValue; + backgroundImage: TweenValue; + backgroundOrigin: TweenValue; + backgroundPosition: TweenValue; + backgroundPositionX: TweenValue; + backgroundPositionY: TweenValue; + backgroundRepeat: TweenValue; + backgroundSize: TweenValue; + baselineShift: TweenValue; + border: TweenValue; + borderBottom: TweenValue; + borderBottomColor: TweenValue; + borderBottomLeftRadius: TweenValue; + borderBottomRightRadius: TweenValue; + borderBottomStyle: TweenValue; + borderBottomWidth: TweenValue; + borderCollapse: TweenValue; + borderColor: TweenValue; + borderImage: TweenValue; + borderImageOutset: TweenValue; + borderImageRepeat: TweenValue; + borderImageSlice: TweenValue; + borderImageSource: TweenValue; + borderImageWidth: TweenValue; + borderLeft: TweenValue; + borderLeftColor: TweenValue; + borderLeftStyle: TweenValue; + borderLeftWidth: TweenValue; + borderRadius: TweenValue; + borderRight: TweenValue; + borderRightColor: TweenValue; + borderRightStyle: TweenValue; + borderRightWidth: TweenValue; + borderSpacing: TweenValue; + borderStyle: TweenValue; + borderTop: TweenValue; + borderTopColor: TweenValue; + borderTopLeftRadius: TweenValue; + borderTopRightRadius: TweenValue; + borderTopStyle: TweenValue; + borderTopWidth: TweenValue; + borderWidth: TweenValue; + bottom: TweenValue; + boxShadow: TweenValue; + boxSizing: TweenValue; + breakAfter: TweenValue; + breakBefore: TweenValue; + breakInside: TweenValue; + captionSide: TweenValue; + caretColor: TweenValue; + clear: TweenValue; + clip: TweenValue; + clipPath: TweenValue; + clipRule: TweenValue; + color: TweenValue; + colorInterpolationFilters: TweenValue; + columnCount: TweenValue; + columnFill: TweenValue; + columnGap: TweenValue; + columnRule: TweenValue; + columnRuleColor: TweenValue; + columnRuleStyle: TweenValue; + columnRuleWidth: TweenValue; + columnSpan: TweenValue; + columnWidth: TweenValue; + columns: TweenValue; + content: TweenValue; + counterIncrement: TweenValue; + counterReset: TweenValue; + cssFloat: TweenValue; + cssText: TweenValue; + cursor: TweenValue; + direction: TweenValue; + display: TweenValue; + dominantBaseline: TweenValue; + emptyCells: TweenValue; + enableBackground: TweenValue; + fill: TweenValue; + fillOpacity: TweenValue; + fillRule: TweenValue; + filter: TweenValue; + flex: TweenValue; + flexBasis: TweenValue; + flexDirection: TweenValue; + flexFlow: TweenValue; + flexGrow: TweenValue; + flexShrink: TweenValue; + flexWrap: TweenValue; + floodColor: TweenValue; + floodOpacity: TweenValue; + font: TweenValue; + fontFamily: TweenValue; + fontFeatureSettings: TweenValue; + fontKerning: TweenValue; + fontSize: TweenValue; + fontSizeAdjust: TweenValue; + fontStretch: TweenValue; + fontStyle: TweenValue; + fontSynthesis: TweenValue; + fontVariant: TweenValue; + fontVariantCaps: TweenValue; + fontVariantEastAsian: TweenValue; + fontVariantLigatures: TweenValue; + fontVariantNumeric: TweenValue; + fontVariantPosition: TweenValue; + fontWeight: TweenValue; + gap: TweenValue; + glyphOrientationHorizontal: TweenValue; + glyphOrientationVertical: TweenValue; + grid: TweenValue; + gridArea: TweenValue; + gridAutoColumns: TweenValue; + gridAutoFlow: TweenValue; + gridAutoRows: TweenValue; + gridColumn: TweenValue; + gridColumnEnd: TweenValue; + gridColumnGap: TweenValue; + gridColumnStart: TweenValue; + gridGap: TweenValue; + gridRow: TweenValue; + gridRowEnd: TweenValue; + gridRowGap: TweenValue; + gridRowStart: TweenValue; + gridTemplate: TweenValue; + gridTemplateAreas: TweenValue; + gridTemplateColumns: TweenValue; + gridTemplateRows: TweenValue; + height: TweenValue; + hyphens: TweenValue; + imageOrientation: TweenValue; + imageRendering: TweenValue; + imeMode: TweenValue; + justifyContent: TweenValue; + justifyItems: TweenValue; + justifySelf: TweenValue; + kerning: TweenValue; + layoutGrid: TweenValue; + layoutGridChar: TweenValue; + layoutGridLine: TweenValue; + layoutGridMode: TweenValue; + layoutGridType: TweenValue; + left: TweenValue; + letterSpacing: TweenValue; + lightingColor: TweenValue; + lineBreak: TweenValue; + lineHeight: TweenValue; + listStyle: TweenValue; + listStyleImage: TweenValue; + listStylePosition: TweenValue; + listStyleType: TweenValue; + margin: TweenValue; + marginBottom: TweenValue; + marginLeft: TweenValue; + marginRight: TweenValue; + marginTop: TweenValue; + marker: TweenValue; + markerEnd: TweenValue; + markerMid: TweenValue; + markerStart: TweenValue; + mask: TweenValue; + maskComposite: TweenValue; + maskImage: TweenValue; + maskPosition: TweenValue; + maskRepeat: TweenValue; + maskSize: TweenValue; + maskType: TweenValue; + maxHeight: TweenValue; + maxWidth: TweenValue; + minHeight: TweenValue; + minWidth: TweenValue; + msContentZoomChaining: TweenValue; + msContentZoomLimit: TweenValue; + msContentZoomLimitMax: any; + msContentZoomLimitMin: any; + msContentZoomSnap: TweenValue; + msContentZoomSnapPoints: TweenValue; + msContentZoomSnapType: TweenValue; + msContentZooming: TweenValue; + msFlowFrom: TweenValue; + msFlowInto: TweenValue; + msFontFeatureSettings: TweenValue; + msGridColumn: any; + msGridColumnAlign: TweenValue; + msGridColumnSpan: any; + msGridColumns: TweenValue; + msGridRow: any; + msGridRowAlign: TweenValue; + msGridRowSpan: any; + msGridRows: TweenValue; + msHighContrastAdjust: TweenValue; + msHyphenateLimitChars: TweenValue; + msHyphenateLimitLines: any; + msHyphenateLimitZone: any; + msHyphens: TweenValue; + msImeAlign: TweenValue; + msOverflowStyle: TweenValue; + msScrollChaining: TweenValue; + msScrollLimit: TweenValue; + msScrollLimitXMax: any; + msScrollLimitXMin: any; + msScrollLimitYMax: any; + msScrollLimitYMin: any; + msScrollRails: TweenValue; + msScrollSnapPointsX: TweenValue; + msScrollSnapPointsY: TweenValue; + msScrollSnapType: TweenValue; + msScrollSnapX: TweenValue; + msScrollSnapY: TweenValue; + msScrollTranslation: TweenValue; + msTextCombineHorizontal: TweenValue; + msTextSizeAdjust: any; + msTouchAction: TweenValue; + msTouchSelect: TweenValue; + msUserSelect: TweenValue; + msWrapFlow: TweenValue; + msWrapMargin: any; + msWrapThrough: TweenValue; + objectFit: TweenValue; + objectPosition: TweenValue; + opacity: TweenValue; + order: TweenValue; + orphans: TweenValue; + outline: TweenValue; + outlineColor: TweenValue; + outlineOffset: TweenValue; + outlineStyle: TweenValue; + outlineWidth: TweenValue; + overflow: TweenValue; + overflowAnchor: TweenValue; + overflowWrap: TweenValue; + overflowX: TweenValue; + overflowY: TweenValue; + padding: TweenValue; + paddingBottom: TweenValue; + paddingLeft: TweenValue; + paddingRight: TweenValue; + paddingTop: TweenValue; + pageBreakAfter: TweenValue; + pageBreakBefore: TweenValue; + pageBreakInside: TweenValue; + penAction: TweenValue; + perspective: TweenValue; + perspectiveOrigin: TweenValue; + placeContent: TweenValue; + placeItems: TweenValue; + placeSelf: TweenValue; + pointerEvents: TweenValue; + position: TweenValue; + quotes: TweenValue; + resize: TweenValue; + right: TweenValue; + rotate: TweenValue; + rowGap: TweenValue; + rubyAlign: TweenValue; + rubyOverhang: TweenValue; + rubyPosition: TweenValue; + scale: TweenValue; + scrollBehavior: TweenValue; + stopColor: TweenValue; + stopOpacity: TweenValue; + stroke: TweenValue; + strokeDasharray: TweenValue; + strokeDashoffset: TweenValue; + strokeLinecap: TweenValue; + strokeLinejoin: TweenValue; + strokeMiterlimit: TweenValue; + strokeOpacity: TweenValue; + strokeWidth: TweenValue; + tabSize: TweenValue; + tableLayout: TweenValue; + textAlign: TweenValue; + textAlignLast: TweenValue; + textAnchor: TweenValue; + textCombineUpright: TweenValue; + textDecoration: TweenValue; + textDecorationColor: TweenValue; + textDecorationLine: TweenValue; + textDecorationStyle: TweenValue; + textEmphasis: TweenValue; + textEmphasisColor: TweenValue; + textEmphasisPosition: TweenValue; + textEmphasisStyle: TweenValue; + textIndent: TweenValue; + textJustify: TweenValue; + textKashida: TweenValue; + textKashidaSpace: TweenValue; + textOrientation: TweenValue; + textOverflow: TweenValue; + textShadow: TweenValue; + textTransform: TweenValue; + textUnderlinePosition: TweenValue; + top: TweenValue; + touchAction: TweenValue; + transform: TweenValue; + transformBox: TweenValue; + transformOrigin: TweenValue; + transformStyle: TweenValue; + transition: TweenValue; + transitionDelay: TweenValue; + transitionDuration: TweenValue; + transitionProperty: TweenValue; + transitionTimingFunction: TweenValue; + translate: TweenValue; + unicodeBidi: TweenValue; + userSelect: TweenValue; + verticalAlign: TweenValue; + visibility: TweenValue; + /** @deprecated */ + webkitAlignContent: TweenValue; + /** @deprecated */ + webkitAlignItems: TweenValue; + /** @deprecated */ + webkitAlignSelf: TweenValue; + /** @deprecated */ + webkitAnimation: TweenValue; + /** @deprecated */ + webkitAnimationDelay: TweenValue; + /** @deprecated */ + webkitAnimationDirection: TweenValue; + /** @deprecated */ + webkitAnimationDuration: TweenValue; + /** @deprecated */ + webkitAnimationFillMode: TweenValue; + /** @deprecated */ + webkitAnimationIterationCount: TweenValue; + /** @deprecated */ + webkitAnimationName: TweenValue; + /** @deprecated */ + webkitAnimationPlayState: TweenValue; + /** @deprecated */ + webkitAnimationTimingFunction: TweenValue; + /** @deprecated */ + webkitAppearance: TweenValue; + /** @deprecated */ + webkitBackfaceVisibility: TweenValue; + /** @deprecated */ + webkitBackgroundClip: TweenValue; + /** @deprecated */ + webkitBackgroundOrigin: TweenValue; + /** @deprecated */ + webkitBackgroundSize: TweenValue; + /** @deprecated */ + webkitBorderBottomLeftRadius: TweenValue; + /** @deprecated */ + webkitBorderBottomRightRadius: TweenValue; + webkitBorderImage: TweenValue; + /** @deprecated */ + webkitBorderRadius: TweenValue; + /** @deprecated */ + webkitBorderTopLeftRadius: TweenValue; + /** @deprecated */ + webkitBorderTopRightRadius: TweenValue; + /** @deprecated */ + webkitBoxAlign: TweenValue; + webkitBoxDirection: TweenValue; + /** @deprecated */ + webkitBoxFlex: TweenValue; + /** @deprecated */ + webkitBoxOrdinalGroup: TweenValue; + webkitBoxOrient: TweenValue; + /** @deprecated */ + webkitBoxPack: TweenValue; + /** @deprecated */ + webkitBoxShadow: TweenValue; + /** @deprecated */ + webkitBoxSizing: TweenValue; + webkitColumnBreakAfter: TweenValue; + webkitColumnBreakBefore: TweenValue; + webkitColumnBreakInside: TweenValue; + webkitColumnCount: any; + webkitColumnGap: any; + webkitColumnRule: TweenValue; + webkitColumnRuleColor: any; + webkitColumnRuleStyle: TweenValue; + webkitColumnRuleWidth: any; + webkitColumnSpan: TweenValue; + webkitColumnWidth: any; + webkitColumns: TweenValue; + /** @deprecated */ + webkitFilter: TweenValue; + /** @deprecated */ + webkitFlex: TweenValue; + /** @deprecated */ + webkitFlexBasis: TweenValue; + /** @deprecated */ + webkitFlexDirection: TweenValue; + /** @deprecated */ + webkitFlexFlow: TweenValue; + /** @deprecated */ + webkitFlexGrow: TweenValue; + /** @deprecated */ + webkitFlexShrink: TweenValue; + /** @deprecated */ + webkitFlexWrap: TweenValue; + /** @deprecated */ + webkitJustifyContent: TweenValue; + webkitLineClamp: TweenValue; + /** @deprecated */ + webkitMask: TweenValue; + /** @deprecated */ + webkitMaskBoxImage: TweenValue; + /** @deprecated */ + webkitMaskBoxImageOutset: TweenValue; + /** @deprecated */ + webkitMaskBoxImageRepeat: TweenValue; + /** @deprecated */ + webkitMaskBoxImageSlice: TweenValue; + /** @deprecated */ + webkitMaskBoxImageSource: TweenValue; + /** @deprecated */ + webkitMaskBoxImageWidth: TweenValue; + /** @deprecated */ + webkitMaskClip: TweenValue; + /** @deprecated */ + webkitMaskComposite: TweenValue; + /** @deprecated */ + webkitMaskImage: TweenValue; + /** @deprecated */ + webkitMaskOrigin: TweenValue; + /** @deprecated */ + webkitMaskPosition: TweenValue; + /** @deprecated */ + webkitMaskRepeat: TweenValue; + /** @deprecated */ + webkitMaskSize: TweenValue; + /** @deprecated */ + webkitOrder: TweenValue; + /** @deprecated */ + webkitPerspective: TweenValue; + /** @deprecated */ + webkitPerspectiveOrigin: TweenValue; + webkitTapHighlightColor: TweenValue; + /** @deprecated */ + webkitTextFillColor: TweenValue; + /** @deprecated */ + webkitTextSizeAdjust: TweenValue; + /** @deprecated */ + webkitTextStroke: TweenValue; + /** @deprecated */ + webkitTextStrokeColor: TweenValue; + /** @deprecated */ + webkitTextStrokeWidth: TweenValue; + /** @deprecated */ + webkitTransform: TweenValue; + /** @deprecated */ + webkitTransformOrigin: TweenValue; + /** @deprecated */ + webkitTransformStyle: TweenValue; + /** @deprecated */ + webkitTransition: TweenValue; + /** @deprecated */ + webkitTransitionDelay: TweenValue; + /** @deprecated */ + webkitTransitionDuration: TweenValue; + /** @deprecated */ + webkitTransitionProperty: TweenValue; + /** @deprecated */ + webkitTransitionTimingFunction: TweenValue; + webkitUserModify: TweenValue; + webkitUserSelect: TweenValue; + webkitWritingMode: TweenValue; + whiteSpace: TweenValue; + widows: TweenValue; + width: TweenValue; + willChange: TweenValue; + wordBreak: TweenValue; + wordSpacing: TweenValue; + wordWrap: TweenValue; + writingMode: TweenValue; + zIndex: TweenValue; + zoom: TweenValue; + } + + interface CSSVars extends Partial<CSSProperties> { } + + interface TweenVars extends CSSVars { + css?: CSSVars; + } + + // TODO: Add types + interface GSCache { + [key: string]: any; + } +} + +declare namespace gsap.plugins { + + interface CSSPlugin extends Plugin {} + + interface CSSPluginClass extends CSSPlugin { + new(): PluginScope & CSSPlugin; + prototype: PluginScope & CSSPlugin; + } + + const css: CSSPluginClass; +} + +interface Element { + _gsap: gsap.GSCache; +} + +declare const CSSPlugin: gsap.plugins.CSSPlugin; + +declare module "gsap/CSSPlugin" { + export const CSSPlugin: gsap.plugins.CSSPlugin; + export { CSSPlugin as default }; +} + +declare module "gsap/src/CSSPlugin" { + export * from "gsap/CSSPlugin"; + export { CSSPlugin as default } from "gsap/CSSPlugin"; +} + +declare module "gsap" { + export * from "gsap/CSSPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/CSSPlugin"; +} + +declare module "gsap-trial/CSSPlugin" { + export * from "gsap/CSSPlugin"; + export { CSSPlugin as default } from "gsap/CSSPlugin"; +} + +declare module "gsap-trial/src/CSSPlugin" { + export * from "gsap/CSSPlugin"; + export { CSSPlugin as default } from "gsap/CSSPlugin"; +} + +declare module "gsap-trial" { + export * from "gsap/CSSPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/CSSPlugin"; +} diff --git a/node_modules/gsap/types/css-rule-plugin.d.ts b/node_modules/gsap/types/css-rule-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..099c0150c6b96e866a10a8ce30ae7a1f36390d0d --- /dev/null +++ b/node_modules/gsap/types/css-rule-plugin.d.ts @@ -0,0 +1,74 @@ +declare namespace gsap { + + interface TweenVars { + cssRule?: object; // TODO make more specific + } +} + +declare namespace gsap.plugins { + + interface CSSRulePlugin extends Plugin { + /** + * Gets the style sheet object associated with a particular selector. + * + * ```js + * var rule = CSSRulePlugin.getRule(".myClass::before"); + * // Then do what you want with it, such as: + * gsap.to(rule, { duration: 3, cssRule: { color: "#0000FF" } }); + * ``` + * + * @param {string} selector + * @returns {CSSRule} The CSSRule + * @memberof CSSRulePlugin + * @link https://greensock.com/docs/v3/Plugins/CSSRulePlugin + */ + getRule(selector: string): CSSRule; + } + + interface CSSRulePluginClass extends CSSRulePlugin { + new(): PluginScope & CSSRulePlugin; + prototype: PluginScope & CSSRulePlugin; + } + + const cssRule: CSSRulePluginClass; +} + +declare const CSSRulePlugin: gsap.plugins.CSSRulePlugin; + +declare module "gsap/CSSRulePlugin" { + export const CSSRulePlugin: gsap.plugins.CSSRulePlugin; + export { CSSRulePlugin as default }; +} + +declare module "gsap/src/CSSRulePlugin" { + export * from "gsap/CSSRulePlugin"; + export { CSSRulePlugin as default } from "gsap/CSSRulePlugin"; +} + +declare module "gsap/dist/CSSRulePlugin" { + export * from "gsap/CSSRulePlugin"; + export { CSSRulePlugin as default } from "gsap/CSSRulePlugin"; +} + +declare module "gsap/all" { + export * from "gsap/CSSRulePlugin"; +} + +declare module "gsap-trial/CSSRulePlugin" { + export * from "gsap/CSSRulePlugin"; + export { CSSRulePlugin as default } from "gsap/CSSRulePlugin"; +} + +declare module "gsap-trial/src/CSSRulePlugin" { + export * from "gsap/CSSRulePlugin"; + export { CSSRulePlugin as default } from "gsap/CSSRulePlugin"; +} + +declare module "gsap-trial/dist/CSSRulePlugin" { + export * from "gsap/CSSRulePlugin"; + export { CSSRulePlugin as default } from "gsap/CSSRulePlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/CSSRulePlugin"; +} diff --git a/node_modules/gsap/types/custom-bounce.d.ts b/node_modules/gsap/types/custom-bounce.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..5da52a39f93928638767f307805c8b01b0bcad8b --- /dev/null +++ b/node_modules/gsap/types/custom-bounce.d.ts @@ -0,0 +1,42 @@ +// TODO +declare const CustomBounce: any; + +declare module "gsap/CustomBounce" { + + // TODO + export const CustomBounce: any; + export { CustomBounce as default }; +} + +declare module "gsap/dist/CustomBounce" { + export * from "gsap/CustomBounce"; + export { CustomBounce as default } from "gsap/CustomBounce"; +} + +declare module "gsap/src/CustomBounce" { + export * from "gsap/CustomBounce"; + export { CustomBounce as default } from "gsap/CustomBounce"; +} + +declare module "gsap/all" { + export * from "gsap/CustomBounce"; +} + +declare module "gsap-trial/CustomBounce" { + export * from "gsap/CustomBounce"; + export { CustomBounce as default } from "gsap/CustomBounce"; +} + +declare module "gsap-trial/dist/CustomBounce" { + export * from "gsap/CustomBounce"; + export { CustomBounce as default } from "gsap/CustomBounce"; +} + +declare module "gsap-trial/src/CustomBounce" { + export * from "gsap/CustomBounce"; + export { CustomBounce as default } from "gsap/CustomBounce"; +} + +declare module "gsap-trial/all" { + export * from "gsap/CustomBounce"; +} diff --git a/node_modules/gsap/types/custom-ease.d.ts b/node_modules/gsap/types/custom-ease.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..3e3c15b26215efee8d984a10882a5d168c2fa99e --- /dev/null +++ b/node_modules/gsap/types/custom-ease.d.ts @@ -0,0 +1,44 @@ +// TODO +declare const CustomEase: any; + +declare module "gsap/CustomEase" { + + // TODO + export const CustomEase: any; + export { CustomEase as default }; +} + +declare module "gsap/dist/CustomEase" { + export * from "gsap/CustomEase"; + export { CustomEase as default } from "gsap/CustomEase"; +} + +declare module "gsap/src/CustomEase" { + export * from "gsap/CustomEase"; + export { CustomEase as default } from "gsap/CustomEase"; +} + +declare module "gsap/all" { + export * from "gsap/CustomEase"; +} + +declare module "gsap-trial/CustomEase" { + + // TODO + export const CustomEase: any; + export { CustomEase as default }; +} + +declare module "gsap-trial/dist/CustomEase" { + export * from "gsap/CustomEase"; + export { CustomEase as default } from "gsap/CustomEase"; +} + +declare module "gsap-trial/src/CustomEase" { + export * from "gsap/CustomEase"; + export { CustomEase as default } from "gsap/CustomEase"; +} + +declare module "gsap-trial/all" { + export * from "gsap/CustomEase"; +} diff --git a/node_modules/gsap/types/custom-wiggle.d.ts b/node_modules/gsap/types/custom-wiggle.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..5d189bc34177a3be267482e12d822d1baaff08fa --- /dev/null +++ b/node_modules/gsap/types/custom-wiggle.d.ts @@ -0,0 +1,42 @@ +// TODO +declare const CustomWiggle: any; + +declare module "gsap/CustomWiggle" { + + // TODO + export const CustomWiggle: any; + export { CustomWiggle as default }; +} + +declare module "gsap/dist/CustomWiggle" { + export * from "gsap/CustomWiggle"; + export { CustomWiggle as default } from "gsap/CustomWiggle"; +} + +declare module "gsap/src/CustomWiggle" { + export * from "gsap/CustomWiggle"; + export { CustomWiggle as default } from "gsap/CustomWiggle"; +} + +declare module "gsap/all" { + export * from "gsap/CustomWiggle"; +} + +declare module "gsap-trial/CustomWiggle" { + export * from "gsap/CustomWiggle"; + export { CustomWiggle as default } from "gsap/CustomWiggle"; +} + +declare module "gsap-trial/dist/CustomWiggle" { + export * from "gsap/CustomWiggle"; + export { CustomWiggle as default } from "gsap/CustomWiggle"; +} + +declare module "gsap-trial/src/CustomWiggle" { + export * from "gsap/CustomWiggle"; + export { CustomWiggle as default } from "gsap/CustomWiggle"; +} + +declare module "gsap-trial/all" { + export * from "gsap/CustomWiggle"; +} diff --git a/node_modules/gsap/types/draggable.d.ts b/node_modules/gsap/types/draggable.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..3e95c64ea4443bc52aa3d9511389e146c666a984 --- /dev/null +++ b/node_modules/gsap/types/draggable.d.ts @@ -0,0 +1,455 @@ +declare class Draggable { + + static version: string; + static zIndex: number; + + readonly autoScroll: number; + readonly deltaX: number; + readonly deltaY: number; + readonly endRotation: number; + readonly endX: number; + readonly endY: number; + readonly isDragging: boolean; + readonly isPressed: boolean; + readonly isThrowing: boolean; + readonly lockAxis: boolean; + readonly maxRotation: number; + readonly maxX: number; + readonly maxY: number; + readonly minX: number; + readonly minY: number; + readonly minRotation: number; + readonly pointerEvent: TouchEvent | PointerEvent; + readonly pointerX: number; + readonly pointerY: number; + readonly rotation: number; + readonly scrollProxy: any; // TODO: Create interface + readonly startX: number; + readonly startY: number; + readonly target: HTMLElement | SVGElement; + readonly tween: gsap.core.Tween; + readonly vars: Draggable.Vars; + readonly x: number; + readonly y: number; + + constructor(target: gsap.DOMTarget, vars?: Draggable.Vars); + + /** + * A more flexible way to create Draggable instances than the constructor. + * + * ```js + * Draggable.create(".myClass", {type: "x,y"}); + * ``` + * + * @param {gsap.DOMTarget} target + * @param {Draggable.Vars} [vars] + * @returns {Draggable[]} Array of Draggables + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/static.create() + */ + static create(target: gsap.DOMTarget, vars?: Draggable.Vars): Draggable[]; + + /** + * Get the Draggable instance that's associated with a particular DOM element. + * + * ```js + * var draggable = Draggable.get("#myId"); + * ``` + * + * @param {gsap.DOMTarget} target + * @returns {Draggable} The Draggable + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/static.get() + */ + static get(target: gsap.DOMTarget): Draggable; + + /** + * Test whether or not the target element overlaps with a particular element or the mouse position, optionally including a threshold. + * + * ```js + * Draggable.hitTest(element1, element2, 20) + * ``` + * + * @param {Draggable.TestObject} testObject1 + * @param {Draggable.TestObject} testObject2 + * @param {number | string} [threshold] + * @returns {boolean} If the hit threshhold is met or not + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/static.hitTest() + */ + static hitTest(testObject1: Draggable.TestObject, testObject2: Draggable.TestObject, threshold?: number | string): boolean; + + /** + * Returns the time (in seconds) that has elapsed since the last drag ended. + * + * ```js + * Draggable.timeSinceDrag(); + * ``` + * + * @returns {number} The time since the last drag ended + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/static.timeSinceDrag() + */ + static timeSinceDrag(): number; + + + /** + * Registers a function that should be called each time a particular type of event occurs. + * + * ```js + * draggable.addEventListener("press", myPressFunction); + * ``` + * + * @param {Draggable.CallbackType} type + * @param {gsap.Callback} callback + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/addEventListener() + */ + addEventListener(type: Draggable.CallbackType, callback: gsap.Callback): void; + + /** + * Registers a function that should be called each time a particular type of event occurs. + * + * ```js + * draggable.applyBounds("#dragContainer"); + * draggable.applyBounds({top: 100, left: 0, width: 1000, height: 800}); + * draggable.applyBounds({minX: 10, maxX: 300, minY: 50, maxY: 500}); + * draggable.applyBounds({minRotation: 0, maxRotation: 270}); + * ``` + * + * @param {gsap.DOMTarget | Draggable.BoundsMinMax | Draggable.BoundsRectangle | Draggable.BoundsRotation} bounds + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/applyBounds() + */ + applyBounds(bounds: gsap.DOMTarget | Draggable.BoundsMinMax | Draggable.BoundsRectangle | Draggable.BoundsRotation): void; + + /** + * Disables the Draggable instance so that it cannot be dragged anymore. + * + * ```js + * draggable.disable(); + * ``` + * + * @param {string | object} [type] + * @returns {Draggable} The Draggable instance + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/disable() + */ + disable(type?: string | object): this; + + dispatchEvent(type: Draggable.CallbackType): boolean; + + /** + * Enables the Draggable instance so that it can be dragged. + * + * ```js + * draggable.enable(); + * ``` + * + * @param {string | object} [type] + * @returns {Draggable} The Draggable instance + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/enable() + */ + enable(type?: string | object): this; + + /** + * Sets the enabled state of the Draggable. + * + * ```js + * draggable.enabled(true); + * ``` + * + * @param {boolean} value + * @returns {Draggable} The Draggable + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/enabled() + */ + enabled(value: boolean): this; + /** + * Gets the enabled state of the Draggable. + * + * ```js + * draggable.enabled(); + * ``` + * + * @returns {boolean} The enabled state + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/enabled() + */ + enabled(): boolean; + + /** + * Force the Draggable to immediately stop interactively dragging. + * You must pass it the original mouse or touch event that initiated the stop. + * + * ```js + * draggable.endDrag(e); + * ``` + * + * @param {Event} event + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/endDrag() + */ + endDrag(event: Event): void; + + /** + * Returns the direction, velocity, or proximity to another object. + * + * ```js + * draggable.getDirection("start"); + * draggable.getDirection("velocity"); + * draggable.getDirection(refElem); + * ``` + * + * @param {"start" | "velocity" | gsap.DOMTarget} from + * @returns {Draggable.Direction} The direction + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/getDirection() + */ + getDirection(from: "start" | "velocity" | gsap.DOMTarget): Draggable.Direction; + + /** + * Test whether or not the target element overlaps with a particular element or the mouse position, optionally including a threshold. + * + * ```js + * draggable.hitTest(otherElem, 20); + * ``` + * + * @param {Draggable.TestObject} testObject + * @param {number | string} [threshold] + * @returns {boolean} If the hit threshhold is met or not + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/static.hitTest() + */ + hitTest(testObject: Draggable.TestObject, threshold?: number | string): boolean; + + /** + * Disables the Draggable instance and frees it for garbage collection + * so that it cannot be dragged anymore. + * + * ```js + * draggable.kill(); + * ``` + * + * @returns {Draggable} The Draggable instance + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/kill() + */ + kill(): this; + + removeEventListener(type: Draggable.CallbackType, callback: gsap.Callback): void; + + /** + * Force the Draggable to start interactively dragging. + * You must pass it the original mouse or touch event that initiated the start. + * + * ```js + * draggable.startDrag(e); + * ``` + * + * @param {Event} event + * @param {boolean} align + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/startDrag() + */ + startDrag(event: Event, align?: boolean): void; + + /** + * Returns the time (in seconds) that has elapsed since the last drag ended. + * + * ```js + * draggable.timeSinceDrag(); + * ``` + * + * @returns {number} The time since the last drag ended + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/static.timeSinceDrag() + */ + timeSinceDrag(): number; + + /** + * Updates the Draggable's x/y properties to reflect the target element's current position. + * + * ```js + * Draggable.update(); + * ``` + * + * @param {boolean} [applyBounds] + * @param {boolean} [sticky] + * @returns {Draggable} The Draggable instance + * @memberof Draggable + * @link https://greensock.com/docs/v3/Plugins/Draggable/update() + */ + update(applyBounds?: boolean, sticky?: boolean): this; +} + +declare namespace Draggable { + + type CallbackType = + | "click" + | "drag" + | "dragend" + | "dragstart" + | "move" + | "press" + | "release" + | "throwcomplete" + | "throwupdate"; + + type Direction = + | "down" + | "left" + | "left-down" + | "left-up" + | "up" + | "right" + | "right-down" + | "right-up"; + + type DraggableType = + | "left" + | "left,top" + | "rotation" + | "scroll" + | "scrollLeft" + | "scrollTop" + | "top" + | "top,left" + | "x" + | "x,y" + | "y" + | "y,x"; + + type SnapValue = number[] | ((value: number) => number); + type TestObject = gsap.DOMTarget | Event | Rectangle; + + interface BoundsMinMax { + minX?: number; + minY?: number; + maxX?: number; + maxY?: number; + } + + interface BoundsRectangle { + height?: number; + left?: number; + top?: number; + width?: number; + } + + interface BoundsRotation { + minRotation?: number; + maxRotation?: number; + } + + interface Rectangle { + bottom: number; + left: number; + right: number; + top: number; + } + + interface SnapObject { + left?: SnapValue; + points?: gsap.Point2D[] | ((point: gsap.Point2D) => gsap.Point2D); + radius?: number; + rotation?: SnapValue; + top?: SnapValue; + x?: SnapValue; + y?: SnapValue; + } + + interface Vars { + [key: string]: any; + activeCursor?: string; + allowContextMenu?: boolean; + allowEventDefault?: boolean; + allowNativeTouchScrolling?: boolean; + autoScroll?: number; + bounds?: gsap.DOMTarget | BoundsMinMax | BoundsRectangle | BoundsRotation; + callbackScope?: object; + clickableTest?: (this: Draggable, element: HTMLElement | SVGElement) => void; + cursor?: string; + dragClickables?: boolean; + dragResistance?: number; + edgeResistance?: number; + force3D?: "auto" | boolean; + inertia?: boolean | gsap.InertiaVars; + liveSnap?: boolean | SnapValue | SnapObject; + lockAxis?: boolean; + maxDuration?: number; + minDuration?: number; + minimumMovement?: number; + onClick?: gsap.Callback; + onClickParams?: any[]; + onDrag?: gsap.Callback; + onDragParams?: any[]; + onDragStart?: gsap.Callback; + onDragStartParams?: any[]; + onDragEnd?: gsap.Callback; + onDragEndParams?: any[]; + onLockAxis?: (this: Draggable, event: Event) => void; + onMove?: gsap.Callback; + onMoveParams?: any[]; + onPress?: gsap.Callback; + onPressParams?: any[]; + onPressInit?: gsap.Callback; + onPressInitParams?: any[]; + onRelease?: gsap.Callback; + onReleaseParams?: any[]; + onThrowComplete?: gsap.Callback; + onThrowCompleteParams?: any[]; + onThrowUpdate?: gsap.Callback; + onThrowUpdateParams?: any[]; + overshootTolerance?: number; + resistance?: number; + snap?: SnapValue | SnapObject; + throwProps?: boolean | gsap.InertiaVars; + throwResistance?: number; + trigger?: gsap.DOMTarget; + type?: DraggableType; + zIndexBoost?: boolean; + } +} + +declare module "gsap/Draggable" { + class _Draggable extends Draggable {} + export { + _Draggable as Draggable, + _Draggable as default + } +} + +declare module "gsap/src/Draggable" { + export * from "gsap/Draggable"; + export { Draggable as default } from "gsap/Draggable"; +} + +declare module "gsap/dist/Draggable" { + export * from "gsap/Draggable"; + export { Draggable as default } from "gsap/Draggable"; +} + +declare module "gsap/all" { + export * from "gsap/Draggable"; +} + +declare module "gsap-trial/Draggable" { + export * from "gsap/Draggable"; + export { Draggable as default } from "gsap/Draggable"; +} + +declare module "gsap-trial/src/Draggable" { + export * from "gsap/Draggable"; + export { Draggable as default } from "gsap/Draggable"; +} + +declare module "gsap-trial/dist/Draggable" { + export * from "gsap/Draggable"; + export { Draggable as default } from "gsap/Draggable"; +} + +declare module "gsap-trial/all" { + export * from "gsap/Draggable"; +} diff --git a/node_modules/gsap/types/draw-svg-plugin.d.ts b/node_modules/gsap/types/draw-svg-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..7939afe055074d2506f11f7595a98fff98bbd707 --- /dev/null +++ b/node_modules/gsap/types/draw-svg-plugin.d.ts @@ -0,0 +1,89 @@ +declare namespace gsap { + + type DrawSVGTarget = string | SVGPrimitive | SVGPathElement; + + interface TweenVars { + drawSVG?: number | BooleanValue | DrawSVGTarget; + } +} + +declare namespace gsap.plugins { + + interface DrawSVGPlugin extends Plugin { + + /** + * Get the length of an SVG element's stroke. + * + * ```js + * DrawSVGPlugin.getLength(element); + * ``` + * + * @param {DrawSVGTarget} element + * @returns {number} The stroke length + * @memberof DrawSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/DrawSVGPlugin/static.getLength() + */ + getLength(element: DrawSVGTarget): number; + + /** + * Get the current position of the DrawSVG in array form. + * + * ```js + * DrawSVGPlugin.getPosition(element); + * ``` + * + * @param {DrawSVGTarget} element + * @returns {number[]} The position array + * @memberof DrawSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/DrawSVGPlugin/static.getPosition() + */ + getPosition(element: DrawSVGTarget): number[]; + } + + interface DrawSVGPluginClass extends DrawSVGPlugin { + new(): PluginScope & DrawSVGPlugin; + prototype: PluginScope & DrawSVGPlugin; + } + + const drawSVG: DrawSVGPluginClass; +} + +declare const DrawSVGPlugin: gsap.plugins.DrawSVGPlugin; + +declare module "gsap/DrawSVGPlugin" { + export const DrawSVGPlugin: gsap.plugins.DrawSVGPlugin; + export { DrawSVGPlugin as default }; +} + +declare module "gsap/src/DrawSVGPlugin" { + export * from "gsap/DrawSVGPlugin"; + export { DrawSVGPlugin as default } from "gsap/DrawSVGPlugin"; +} + +declare module "gsap/dist/DrawSVGPlugin" { + export * from "gsap/DrawSVGPlugin"; + export { DrawSVGPlugin as default } from "gsap/DrawSVGPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/DrawSVGPlugin"; +} + +declare module "gsap-trial/DrawSVGPlugin" { + export * from "gsap/DrawSVGPlugin"; + export { DrawSVGPlugin as default } from "gsap/DrawSVGPlugin"; +} + +declare module "gsap-trial/src/DrawSVGPlugin" { + export * from "gsap/DrawSVGPlugin"; + export { DrawSVGPlugin as default } from "gsap/DrawSVGPlugin"; +} + +declare module "gsap-trial/dist/DrawSVGPlugin" { + export * from "gsap/DrawSVGPlugin"; + export { DrawSVGPlugin as default } from "gsap/DrawSVGPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/DrawSVGPlugin"; +} diff --git a/node_modules/gsap/types/ease.d.ts b/node_modules/gsap/types/ease.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..56de1184552e01022be00a9eca2fabb6ce28e0d2 --- /dev/null +++ b/node_modules/gsap/types/ease.d.ts @@ -0,0 +1,171 @@ +declare namespace gsap { + + interface EaseFunction { + (progress: number): number; + } + + interface Ease { + easeIn: EaseFunction; + easeOut: EaseFunction; + easeInOut: EaseFunction; + } + + interface BackConfig extends EaseFunction { + config(overshoot: number): EaseFunction; + } + + interface Back { + easeIn: BackConfig; + easeOut: BackConfig; + easeInOut: BackConfig; + } + + interface EasePack { + SlowMo: gsap.SlowMo; + ExpoScaleEase: gsap.ExpoScaleEase; + RoughEase: gsap.RoughEase; + } + + interface ElasticConfig extends EaseFunction { + config(amplitude: number, period?: number): EaseFunction; + } + + interface Elastic { + easeIn: ElasticConfig; + easeOut: ElasticConfig; + easeInOut: ElasticConfig; + } + + interface ExpoScaleEase { + config(startingScale: number, endingScale: number, ease?: string | EaseFunction): EaseFunction; + } + + interface Linear extends Ease { + easeNone: EaseFunction; + } + + interface RoughEaseVars { + clamp?: boolean; + points?: number; + randomize?: boolean; + strength?: number; + taper?: "in" | "out" | "both" | "none"; + template?: string | EaseFunction + } + + interface RoughEaseEase extends EaseFunction { + config: RoughEaseConfig; + } + + interface RoughEaseConfig extends EaseFunction { + (config?: RoughEaseVars): EaseFunction; + } + + interface RoughEase extends EaseFunction { + config: RoughEaseConfig; + ease: RoughEaseEase; + } + + interface SlowMoEase extends EaseFunction { + config: SlowMoConfig; + } + + interface SlowMoConfig extends EaseFunction { + (linearRatio: number, power?: number, yoyoMode?: boolean): EaseFunction; + } + + interface SlowMo extends EaseFunction { + config: SlowMoConfig; + ease: SlowMoEase; + } + + interface SteppedEase { + config(steps: number): EaseFunction; + } +} + +declare const Back: gsap.Back; +declare const Bounce: gsap.Ease; +declare const Circ: gsap.Ease; +declare const Cubic: gsap.Ease; +declare const Elastic: gsap.Elastic; +declare const Expo: gsap.Ease; +declare const Linear: gsap.Linear; +declare const Power0: gsap.Linear; +declare const Power1: gsap.Ease; +declare const Power2: gsap.Ease; +declare const Power3: gsap.Ease; +declare const Power4: gsap.Ease; +declare const Quad: gsap.Ease; +declare const Quart: gsap.Ease; +declare const Quint: gsap.Ease; +declare const Sine: gsap.Ease; +declare const SteppedEase: gsap.SteppedEase; +declare const Strong: gsap.Ease; + +declare const EasePack: gsap.EasePack; +declare const ExpoScaleEase: gsap.ExpoScaleEase; +declare const RoughEase: gsap.RoughEase; +declare const SlowMo: gsap.SlowMo; + +declare module "gsap/gsap-core" { + export const Back: gsap.Back; + export const Bounce: gsap.Ease; + export const Circ: gsap.Ease; + export const Cubic: gsap.Ease; + export const Elastic: gsap.Elastic; + export const Expo: gsap.Ease; + export const Linear: gsap.Linear; + export const Power0: gsap.Linear; + export const Power1: gsap.Ease; + export const Power2: gsap.Ease; + export const Power3: gsap.Ease; + export const Power4: gsap.Ease; + export const Quad: gsap.Ease; + export const Quart: gsap.Ease; + export const Quint: gsap.Ease; + export const Sine: gsap.Ease; + export const SteppedEase: gsap.SteppedEase; + export const Strong: gsap.Ease; +} + +declare module "gsap/EasePack" { + export const EasePack: gsap.EasePack; + export const ExpoScaleEase: gsap.ExpoScaleEase; + export const SlowMo: gsap.SlowMo; + export const RoughEase: gsap.RoughEase; + export { EasePack as default }; +} + +declare module "gsap/src/EasePack" { + export * from "gsap/EasePack"; + export { EasePack as default } from "gsap/EasePack"; +} + +declare module "gsap/dist/EasePack" { + export * from "gsap/EasePack"; + export { EasePack as default } from "gsap/EasePack"; +} + +declare module "gsap/all" { + export * from "gsap/EasePack"; +} + +declare module "gsap-trial/EasePack" { + export * from "gsap/EasePack"; + export { EasePack as default } from "gsap/EasePack"; +} + +declare module "gsap-trial/src/EasePack" { + export * from "gsap/EasePack"; + export { EasePack as default } from "gsap/EasePack"; +} + +declare module "gsap-trial/dist/EasePack" { + export * from "gsap/EasePack"; + export { EasePack as default } from "gsap/EasePack"; +} + +declare module "gsap-trial/all" { + export * from "gsap/EasePack"; +} diff --git a/node_modules/gsap/types/easel-plugin.d.ts b/node_modules/gsap/types/easel-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..7145b859c447ff1bfc5bf153bbe552eae500a831 --- /dev/null +++ b/node_modules/gsap/types/easel-plugin.d.ts @@ -0,0 +1,66 @@ +declare namespace EaselPlugin { + interface Vars { + [key: string]: any; + } +} + +declare namespace gsap { + + interface TweenVars { + easel?: EaselPlugin.Vars; + } +} + +declare namespace gsap.plugins { + + interface EaselPlugin extends Plugin { + + } + + interface EaselPluginClass extends EaselPlugin { + new(): PluginScope & EaselPlugin; + prototype: PluginScope & EaselPlugin; + } + + const easel: EaselPluginClass; +} + +declare const EaselPlugin: gsap.plugins.EaselPlugin; + +declare module "gsap/EaselPlugin" { + export const EaselPlugin: gsap.plugins.EaselPlugin; + export { EaselPlugin as default }; +} + +declare module "gsap/src/EaselPlugin" { + export * from "gsap/EaselPlugin"; + export { EaselPlugin as default } from "gsap/EaselPlugin"; +} + +declare module "gsap/dist/EaselPlugin" { + export * from "gsap/EaselPlugin"; + export { EaselPlugin as default } from "gsap/EaselPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/EaselPlugin"; +} + +declare module "gsap-trial/EaselPlugin" { + export * from "gsap/EaselPlugin"; + export { EaselPlugin as default } from "gsap/EaselPlugin"; +} + +declare module "gsap-trial/src/EaselPlugin" { + export * from "gsap/EaselPlugin"; + export { EaselPlugin as default } from "gsap/EaselPlugin"; +} + +declare module "gsap-trial/dist/EaselPlugin" { + export * from "gsap/EaselPlugin"; + export { EaselPlugin as default } from "gsap/EaselPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/EaselPlugin"; +} diff --git a/node_modules/gsap/types/flip.d.ts b/node_modules/gsap/types/flip.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..f6eaa1c0eca49db92dfd37863b51d97b6cef2fa2 --- /dev/null +++ b/node_modules/gsap/types/flip.d.ts @@ -0,0 +1,551 @@ +declare class Flip { + + static readonly version: string; + + /** + * Gets the FlipBatch associated with the provided id ("default" by default); if one hasn't be created/registered yet, a new one is returned and registered. + * + * ```js + * let batch = Flip.batch("id"); + * ``` + * + * @static + * @param {string} [id] + * @returns {FlipBatch} the FlipBatch (if one isn't registered, a new one is created/registered and returned) + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.batch() + */ + static batch(id?: string): FlipBatch; + + /** + * Gets the matrix to convert points from one element's local coordinates into a + * different element's local coordinate system. + * + * ```js + * Flip.convertCoordinates(fromElement, toElement); + * ``` + * + * @static + * @param {Element} fromElement + * @param {Element} toElement + * @returns {gsap.plugins.Matrix2D} A matrix to convert from one element's coordinate system to another's + * @memberof Flip + */ + static convertCoordinates(fromElement: Element, toElement: Element): gsap.plugins.Matrix2D; + + /** + * Converts a point from one element's local coordinates into a + * different element's local coordinate system. + * + * ```js + * Flip.convertCoordinates(fromElement, toElement, point); + * ``` + * + * @static + * @param {Element} fromElement + * @param {Element} toElement + * @param {gsap.Point2D} point + * @returns {gsap.Point2D} A point to convert from one element's coordinate system to another's + * @memberof Flip + */ + static convertCoordinates(fromElement: Element, toElement: Element, point: gsap.Point2D): gsap.Point2D; + + /** + * Changes the x/y/rotation/skewX transforms (and width/height or scaleX/scaleY) to fit one element exactly into the the position/size/rotation of another element. + * + * ```js + * Flip.fit("#el1", "#el2", { + * scale: true, + * absolute: true, + * duration: 1, + * ease: "power2" + * }); + * ``` + * + * @static + * @param {gsap.DOMTarget} fromElement + * @param {(gsap.DOMTarget | Flip.FlipState)} toElement + * @param {Flip.FitVars} [vars] + * @returns {(gsap.core.Tween | object | null)} + * @memberof Flip + */ + static fit(fromElement: gsap.DOMTarget, toElement: gsap.DOMTarget | Flip.FlipState, vars?: Flip.FitVars): gsap.core.Tween | object | null; + + /** + * Animates the targets from the provided state to their current state (position/size). + * + * ```js + * Flip.from(state, { + * duration: 1, + * ease: "power1.inOut", + * stagger: 0.1, + * onComplete: () => console.log("done") + * }); + * ``` + * + * @static + * @param {Flip.FlipState} state + * @param {Flip.FromToVars} [vars] + * @returns {gsap.core.Timeline} Flip timeline + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.from() + */ + static from(state: Flip.FlipState, vars?: Flip.FromToVars): gsap.core.Timeline; + + /** + * Captures information about the current state of the targets so that they can be flipped later. + * + * ```js + * let state = Flip.getState(".my-class, .another-class", {props: "backgroundColor,color", simple: true}); + * ``` + * + * @static + * @param {gsap.DOMTarget} targets + * @param {(Flip.FlipStateVars | string)} [vars] + * @returns {Flip.FlipState} The resulting state object + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.getState() + */ + static getState(targets: gsap.DOMTarget, vars?: Flip.FlipStateVars | string): Flip.FlipState; + + /** + * Gets the timeline for the most recently-created flip animation associated with the provided element + * + * ```js + * let tl = Flip.getByTarget("#elementID"); + * ``` + * + * @static + * @param {Element | string} target + * @returns {core.Timeline | null} The timeline for the most recently-created flip animation associated with the provided element + * @memberof Flip + */ + static getByTarget(target: Element | string): gsap.core.Timeline | null; + + /** + * Determines whether or not a particular element is actively flipping (has an active flip animation) + * + * ```js + * if (!Flip.isFlipping("#elementID")) { + * // do stuff + * } + * ``` + * + * @static + * @param {gsap.DOMTarget} target + * @returns {boolean} whether or not the target element is actively flipping + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.isFlipping() + */ + static isFlipping(target: gsap.DOMTarget): boolean; + + /** + * Immediately kills any Flip animations that are running on the target(s) provided, completing them as well (unless "complete" parameter is explicitly false). + * + * ```js + * Flip.killFlipsOf(".box"); + * ``` + * + * @static + * @param {gsap.DOMTarget} targets + * @param {boolean} complete + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.killFlipsOf() + */ + static killFlipsOf(targets: gsap.DOMTarget, complete?: boolean): void; + + /** + * Sets all of the provided target elements to position: absolute while retaining their current positioning. + * + * ```js + * Flip.makeAbsolute(".my-class"); + * ``` + * + * @static + * @param {Element | string | null | ArrayLike<Element | string>} targets + * @returns {Element[]} An Array containing the Elements that were affected + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.makeAbsolute() + */ + static makeAbsolute(targets: gsap.DOMTarget | Flip.FlipState[]): Element[]; + + /** + * Animates the targets from the current state to the provided state. + * + * ```js + * Flip.to(state, { + * duration: 1, + * ease: "power1.inOut", + * stagger: 0.1, + * onComplete: () => console.log("done") + * }); + * ``` + * + * @static + * @param {Flip.FlipState} state + * @param {Flip.FromToVars} [vars] + * @returns {gsap.core.Timeline} Flip timeline + * @memberof Flip + * @link https://greensock.com/docs/v3/Plugins/Flip/static.to() + */ + static to(state: Flip.FlipState, vars?: Flip.FromToVars): gsap.core.Timeline; + + /** + * Registers Flip with gsap + * + * @static + * @param {typeof gsap} core + * @memberof Flip + */ + static register(core: typeof gsap): void; +} + +declare namespace Flip { + + type OverrideProps<M, N> = { [P in keyof M]: P extends keyof N ? N[P] : M[P] }; + + type EnterOrLeaveCallback = (elements: Element[]) => any; + type SpinFunction = (index: number, element: Element, targets: Element[]) => number; + type LoadStateFunction = (load: Function) => any; + type BatchActionFunction = (self: FlipBatchAction) => any; + + interface TweenVarOverrides { + scale?: boolean; + } + + interface AnimationVars extends OverrideProps<gsap.TweenVars, TweenVarOverrides> { + absolute?: boolean | gsap.DOMTarget; + simple?: boolean; + props?: string; + } + + interface FlipStateVars { + simple?: boolean; + props?: string; + } + + interface StateCompare { + changed: Element[]; + unchanged: Element[]; + enter: Element[]; + leave: Element[]; + } + + interface FromToVars extends AnimationVars { + absoluteOnLeave?: boolean; + prune?: boolean; + fade?: boolean; + nested?: boolean; + onEnter?: EnterOrLeaveCallback; + onLeave?: EnterOrLeaveCallback; + spin?: number | boolean | SpinFunction; + targets?: gsap.DOMTarget; + toggleClass?: string; + zIndex?: number; + } + + interface FitReturnVars { + width?: number; + height?: number; + rotation: number; + scaleX?: number; + scaleY?: number; + skewX: number; + x: number; + y: number; + [key: string]: any; + } + interface FitVars extends AnimationVars { + fitChild?: gsap.DOMTarget; + getVars?: boolean; + } + + interface BatchActionConfig { + getState?: BatchActionFunction; + loadState?: LoadStateFunction; + setState?: BatchActionFunction; + animate?: BatchActionFunction; + onEnter?: EnterOrLeaveCallback; + onLeave?: EnterOrLeaveCallback; + onStart?: BatchActionFunction; + onComplete?: BatchActionFunction; + once?: boolean; + } + + class ElementState { + readonly bounds: DOMRect; + readonly cache: object; + readonly display: string; + readonly element: Element; + readonly getProp: Function; + readonly height: number; + readonly id: string; + readonly isVisible: boolean; + readonly matrix: gsap.plugins.Matrix2D; + readonly opacity: number; + readonly parent: Element | null; + readonly position: string; + readonly rotation: number; + readonly scaleX: number; + readonly scaleY: number; + readonly simple: boolean; + readonly skewX: number; + readonly width: number; + readonly x: number; + readonly y: number; + + isDifferent(elState: ElementState): boolean; + } + + class FlipState { + readonly alt: object; + readonly elementStates: ElementState[]; + readonly idLookup: object; + readonly props: string | null; + readonly simple: boolean; + readonly targets: Element[]; + + add(state: FlipState): FlipState; + clear(): FlipState; + compare(state: FlipState): StateCompare; + update(soft?: boolean): FlipState; + fit(state: FlipState, scale?: boolean, nested?: boolean): this; + recordInlineStyles(): void; + interrupt(soft?: boolean): void; + getProperty(element: string | Element, property: string): any; + getElementState(element: Element): ElementState; + makeAbsolute(): Element[]; + } +} + +declare class FlipBatchAction { + readonly batch: FlipBatch; + readonly state: any; + readonly states: Flip.FlipState[]; + readonly timeline: gsap.core.Timeline; + readonly targets: any; + readonly vars: Flip.BatchActionConfig; + + /** + * Searches the state objects that were captured inside the action's getState() on its most recent call, and returns the first one it finds that matches the provided data-flip-id value. + * + * ```js + * let state = action.getStateById("box1"); + * ``` + * @param {string} id + * @memberof FlipBatchAction + */ + getStateById(id: string): Flip.FlipState | null; + + /** + * Kills the batch action, removing it from its batch. + * + * @memberof FlipBatchAction + */ + kill(): FlipBatchAction; +} + +declare class FlipBatch { + readonly actions: FlipBatchAction[]; + readonly state: Flip.FlipState; + readonly timeline: gsap.core.Timeline; + readonly id: string; + data: any; + + /** + * Adds a Flip action to the batch so that MULTIPLE Flips can be combined and run each of their steps together (getState(), loadState(), setState(), animate()) + * + * ```js + * batch.add({ + * getState: self => Flip.getState(targets), + * loadState: done => done(), + * setState: self => app.classList.toggle("active"), + * animate: self => { + * Flip.from(self.state, {ease: "power1.inOut"}); + * }, + * onStart: startCallback, + * onComplete: completeCallback, + * onEnter: elements => console.log("entering", elements), + * onLeave: elements => console.log("leaving", elements), + * once: true + * }); + * ``` + * + * @param {BatchActionConfig | Function} config + * @returns {FlipBatchAction} A FlipBatchAction + * @memberof FlipBatch + */ + add(config: Flip.BatchActionConfig | Function): FlipBatchAction; + + + /** + * Flushes the batch.state (merged) object and removes all actions (unless stateOnly parameter is true) + * + * ```js + * batch.clear(true); + * ``` + * + * @param {boolean} stateOnly + * @returns {FlipBatch} self + * @memberof FlipBatch + */ + clear(stateOnly?: boolean): FlipBatch; + + + /** + * Calls getState() on all actions in this batch (any that are defined at least), optionally merging the results into batch.state + * + * ```js + * batch.getState(true); + * ``` + * + * @param {boolean} merge (false by default) + * @returns {FlipBatch} self + * @memberof FlipBatch + */ + getState(merge?: boolean): FlipBatch; + + /** + * Searches the state objects that were captured inside ANY of this batch actions' most recent getState() call, and returns the first one it finds that matches the provided data-flip-id value. + * + * ```js + * let state = batch.getStateById("box1"); + * ``` + * @param {string} id + * @memberof FlipBatch + */ + getStateById(id: string): Flip.FlipState | null; + + /** + * Kills the batch, unregistering it internally and making it available for garbage collection. Also clears all actions and flushes the batch.state (merged) object. + * + * @memberof FlipBatch + */ + kill(): FlipBatchAction; + + /** + * Removes a particular action from the batch. + * + * ```js + * batch.remove(action); + * ``` + * + * @param {FlipBatchAction} action + * @returns {FlipBatch} self + * @memberof FlipBatch + */ + remove(action: FlipBatchAction): FlipBatch; + + /** + * Executes all actions in the batch in the proper order: getState() (unless skipGetState is true), loadState(), setState(), and animate() + * + * ```js + * batch.run(true); + * ``` + * + * @param {boolean} skipGetState + * @param {boolean} merge + * @returns {FlipBatch} self + * @memberof FlipBatch + */ + run(skipGetState?: boolean, merge?: boolean): FlipBatch; + +} + +declare namespace gsap { + + /** + * @deprecated since 3.7.0 + * @see Flip.ElementState + */ + type ElementState = any; + + /** + * @deprecated since 3.7.0 + * @see Flip.EnterOrLeaveCallback + */ + type EnterOrLeaveCallback = Flip.EnterOrLeaveCallback; + + /** + * @deprecated since 3.7.0 + * @see Flip.FitVars + */ + type FitVars = Flip.FitVars; + + /** + * @deprecated since 3.7.0 + * @see Flip.FitReturnVars + */ + type FitReturnVars = Flip.FitReturnVars; + + /** + * @deprecated since 3.7.0 + * @see Flip + */ + type Flip = any; + + /** + * @deprecated since 3.7.0 + * @see Flip.FlipState + */ + type FlipState = any; + + /** + * @deprecated since 3.7.0 + * @see Flip.FlipStateVars + */ + type FlipStateVars = Flip.FlipStateVars; + + /** + * @deprecated since 3.7.0 + * @see Flip.FromToVars + */ + type FlipToFromVars = Flip.FromToVars; + + /** + * @deprecated since 3.7.0 + * @see Flip.SpinFunction + */ + type SpinFunction = Flip.SpinFunction; +} + +declare module "gsap/Flip" { + class _Flip extends Flip { } + export { + _Flip as Flip, + _Flip as default + } +} + +declare module "gsap/dist/Flip" { + export * from "gsap/Flip"; + export { Flip as default } from "gsap/Flip"; +} + +declare module "gsap/src/Flip" { + export * from "gsap/Flip"; + export { Flip as default } from "gsap/Flip"; +} + +declare module "gsap/all" { + export * from "gsap/Flip"; +} + +declare module "gsap-trial/Flip" { + export * from "gsap/Flip"; + export { Flip as default } from "gsap/Flip"; +} + +declare module "gsap-trial/dist/Flip" { + export * from "gsap/Flip"; + export { Flip as default } from "gsap/Flip"; +} + +declare module "gsap-trial/src/Flip" { + export * from "gsap/Flip"; + export { Flip as default } from "gsap/Flip"; +} + +declare module "gsap-trial/all" { + export * from "gsap/Flip"; +} diff --git a/node_modules/gsap/types/gs-dev-tools.d.ts b/node_modules/gsap/types/gs-dev-tools.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..81a24c589bc4b95de2d84ca863da050c6fcbbd8d --- /dev/null +++ b/node_modules/gsap/types/gs-dev-tools.d.ts @@ -0,0 +1,106 @@ +declare class GSDevTools { + + constructor(target: gsap.DOMTarget, vars?: GSDevTools.Vars); + + /** + * Create a GSDevTools instance. + * + * ```js + * GSDevTools.create({animation: tl}); + * ``` + * + * @param {GSDevTools.Vars} vars + * @returns {GSDevTools} The GSDevTools instance + * @memberof GSDevTools + * @link https://greensock.com/docs/v3/Plugins/GSDevTools/static.create() + */ + static create(vars?: GSDevTools.Vars): GSDevTools; + + /** + * Returns the GSDevTools instance associated with the provided id. + * + * ```js + * GSDevTools.getById("my-id"); + * ``` + * + * @param {string} id + * @returns {GSDevTools} The GSDevTools instance + * @memberof GSDevTools + * @link https://greensock.com/docs/v3/Plugins/GSDevTools/static.getById() + */ + static getById(id: string): GSDevTools | null; + + /** + * Kills a GSDevTools instance + * + * ```js + * tool.kill(); + * ``` + * + * @memberof GSDevTools + * @link https://greensock.com/docs/v3/Plugins/GSDevTools/kill() + */ + kill(): void +} + +declare namespace GSDevTools { + interface Vars { + [key: string]: any; + animation?: string | gsap.core.Animation; + container?: string | Element; + css?: object | string; + globalSync?: boolean; + hideGlobalTimeline?: boolean; + id?: string; + inTime?: number | string; + keyboard?: boolean; + loop?: boolean; + minimal?: boolean; + outTime?: number | string; + paused?: boolean; + persist?: boolean; + timeScale?: number; + visibility?: string; + } +} + +declare module "gsap/GSDevTools" { + class _GSDevTools extends GSDevTools {} + export { + _GSDevTools as GSDevTools, + _GSDevTools as default + } +} + +declare module "gsap/src/GSDevTools" { + export * from "gsap/GSDevTools"; + export { GSDevTools as default } from "gsap/GSDevTools"; +} + +declare module "gsap/dist/GSDevTools" { + export * from "gsap/GSDevTools"; + export { GSDevTools as default } from "gsap/GSDevTools"; +} + +declare module "gsap/all" { + export * from "gsap/GSDevTools"; +} + +declare module "gsap-trial/GSDevTools" { + export * from "gsap/GSDevTools"; + export { GSDevTools as default } from "gsap/GSDevTools"; +} + +declare module "gsap-trial/src/GSDevTools" { + export * from "gsap/GSDevTools"; + export { GSDevTools as default } from "gsap/GSDevTools"; +} + +declare module "gsap-trial/dist/GSDevTools" { + export * from "gsap/GSDevTools"; + export { GSDevTools as default } from "gsap/GSDevTools"; +} + +declare module "gsap-trial/all" { + export * from "gsap/GSDevTools"; +} diff --git a/node_modules/gsap/types/gsap-core.d.ts b/node_modules/gsap/types/gsap-core.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..1bdc0dcf547fc562b5339aa2af09b608a4aeecc4 --- /dev/null +++ b/node_modules/gsap/types/gsap-core.d.ts @@ -0,0 +1,731 @@ +declare namespace gsap { + + type RegisterablePlugins = + | Ease + | EasePack + | ExpoScaleEase + | Plugin + | RoughEase + | SteppedEase + | VelocityTracker + | typeof core.Animation + | typeof core.Tween + | typeof core.Timeline + | typeof Draggable + | typeof GSDevTools + | typeof MotionPathHelper + | typeof SplitText + | typeof Flip + | typeof ScrollTrigger + | typeof Observer + | typeof ScrollSmoother; + + // querySelector returns type Element | null + type DOMTarget = Element | string | null | Window | ArrayLike<Element | string | Window | null>; + type TweenTarget = string | object | null; + + type Callback = (...args: any[]) => void | null; + type ContextSafeFunc = (func: Function) => Function; + type ContextFunc = (context: Context, contextSafe?: ContextSafeFunc) => Function | any | void; + type CallbackType = "onComplete" | "onInterrupt" | "onRepeat" | "onReverseComplete" | "onStart" | "onUpdate"; + type TickerCallback = (time: number, deltaTime: number, frame: number, elapsed: number) => void | null; + + type Point2D = { x: number, y: number }; + type Position = number | string; + + type FunctionBasedValue<T> = (index: number, target: any, targets: any[]) => T; + type ArrayValue = any[] | FunctionBasedValue<any[]>; + type BooleanValue = boolean | FunctionBasedValue<boolean>; + type NumberValue = number | FunctionBasedValue<number>; + type StringValue = string | FunctionBasedValue<string>; + type ElementValue = Element | FunctionBasedValue<Element>; + type TweenValue = NumberValue | StringValue; + type QuickToFunc = { + (value: number, start?: number, startIsRelative?: boolean): core.Tween; + tween: core.Tween; + } + + type SVGPathValue = string | SVGPathElement; + type SVGPathTarget = SVGPathValue | ArrayLike<SVGPathValue>; + type SVGPrimitive = SVGCircleElement | SVGRectElement | SVGEllipseElement | SVGPolygonElement | SVGPolylineElement | SVGLineElement; + + interface Conditions { + [key: string]: boolean; + } + interface Context { + [key: string]: Function | any; + selector?: Function; + isReverted: boolean; + conditions?: Conditions; + queries?: object; + add(methodName: string, func: Function, scope?: Element | string | object): Function; + add<T extends (...args: any[]) => any>(func: T, scope?: Element | string | object): ReturnType<T>; + ignore(func: Function): void; + kill(revert?: boolean): void; + revert(config?: object): void; + clear(): void; + } + + interface MatchMedia { + contexts: Context[]; + add(conditions: string | object, func: ContextFunc, scope?: Element | string | object): MatchMedia; + revert(config?: object): void; + kill(revert?: boolean):void; + } + + interface AnimationVars extends CallbackVars { + [key: string]: any; + data?: any; + id?: string | number; + inherit?: boolean; + paused?: boolean; + repeat?: number; + repeatDelay?: number; + repeatRefresh?: boolean; + reversed?: boolean; + yoyo?: boolean; + } + + interface CallbackVars { + callbackScope?: object; + onComplete?: Callback; + onCompleteParams?: any[]; + onRepeat?: Callback; + onRepeatParams?: any[]; + onReverseComplete?: Callback; + onReverseCompleteParams?: any[]; + onStart?: Callback; + onStartParams?: any[]; + onUpdate?: Callback; + onUpdateParams?: any[]; + } + + interface EaseMap { + [key: string]: EaseFunction; + } + + interface EffectsMap { + [key: string]: any; + } + + interface GSAPConfig { + autoKillThreshold?: number; + autoSleep?: number; + force3D?: "auto" | boolean; + nullTargetWarn?: boolean; + resistance?: number; + stringFilter?: Callback; // TODO: Find out signature + unitFactors?: { time?: number, totalTime?: number }; + units?: GSAPUnits + } + + type GSAPUnits = { + bottom?: string + fontSize?: string + height?: string + left?: string + lineHeight?: string + margin?: string + padding?: string + perspective?: string + right?: string + rotation?: string + rotationX?: string + rotationY?: string + skewX?: string + skewY?: string + top?: string + width?: string + x?: string + y?: string + z?: string + } & { + [key: string]: string + } + + interface StaggerVars extends CallbackVars, utils.DistributeConfig { + repeat?: number; + repeatDelay?: number; + repeatRefresh?: boolean; + yoyo?: boolean; + yoyoEase?: boolean | string | EaseFunction; + } + + interface Ticker { + add(callback: TickerCallback, once?: boolean, prioritize?: boolean): Callback; + fps(fps: number): void; + frame: number; + lagSmoothing(threshold: number | boolean, adjustedLag?: number): void; + remove(callback: Callback): void; + sleep(): void; + tick(): void; + time: number; + deltaRatio(fps?: number): number; + wake(): void; + } + + interface TimelineVars extends AnimationVars { + autoRemoveChildren?: boolean; + defaults?: TweenVars; + delay?: number; + smoothChildTiming?: boolean; + } + + type EaseString = "none" + | "power1" | "power1.in" | "power1.out" | "power1.inOut" + | "power2" | "power2.in" | "power2.out" | "power2.inOut" + | "power3" | "power3.in" | "power3.out" | "power3.inOut" + | "power4" | "power4.in" | "power4.out" | "power4.inOut" + | "back" | "back.in" | "back.out" | "back.inOut" + | "bounce" | "bounce.in" | "bounce.out" | "bounce.inOut" + | "circ" | "circ.in" | "circ.out" | "circ.inOut" + | "elastic" | "elastic.in" | "elastic.out" | "elastic.inOut" + | "expo" | "expo.in" | "expo.out" | "expo.inOut" + | "sine" | "sine.in" | "sine.out" | "sine.inOut" | ({} & string); + + interface TweenVars extends AnimationVars { + delay?: TweenValue; + duration?: TweenValue; + ease?: EaseString | EaseFunction; + endArray?: any[]; + immediateRender?: boolean; + lazy?: boolean; + keyframes?: TweenVars[] | object; + onInterrupt?: Callback; + onInterruptParams?: any[]; + overwrite?: "auto" | boolean; + runBackwards?: boolean; + stagger?: NumberValue | StaggerVars; + startAt?: TweenVars; + yoyoEase?: boolean | string | EaseFunction; + } + + const effects: EffectsMap; + + const globalTimeline: core.Timeline; + + const ticker: Ticker; + + const version: string; + + /** + * Gets or sets GSAP's global configuration settings. + * + * Options: autoSleep, force3D, nullTargetWarn, and units + * + * ```js + * gsap.config({force3D: false}); + * ``` + * + * @param {GSAPConfig} [config] + * @returns {GSAPConfig} Configuration object + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.config() + */ + function config(config?: GSAPConfig): GSAPConfig; + + /** + * Creates a Context object for recording/reverting any GSAP animations and/or ScrollTriggers that are in the provided function + * + * ```js + * let ctx = gsap.context((self) => { + * gsap.to(".box", {x: 100}); + * }, myElement); + * + * // then later + * ctx.revert(); + * ``` + * + * @param {ContextFunc} [func] + * @param {Element | string | object} [scope] + * @returns {Context} Context object + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.context() + */ + function context(func?: ContextFunc, scope?: Element | string | object): Context; + + /** + * Gets or sets GSAP's global defaults. These will be inherited by every tween. + * + * ```js + * gsap.defaults({ease: "none", duration: 1}); + * ``` + * + * @param {TweenVars} [defaults] + * @returns {TweenVars} Defaults object + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.defaults() + */ + function defaults(defaults?: TweenVars): TweenVars; + + /** + * Delays the call of a function by the specified amount. + * + * ```js + * let delayTween = gsap.delayedCall(1, myFunc); + * ``` + * + * @param {number} delay + * @param {Function} callback + * @param {any[]} [params] + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.delayedCall() + */ + function delayedCall(delay: number, callback: Function, params?: any[]): core.Tween; + + /** + * Transfers all tweens, timelines, and (optionally) delayed calls from the root timeline into a new timeline. + * + * ```js + * let exportedTL = gsap.exportRoot(); + * ``` + * + * @param {TimelineVars} [vars] + * @param {boolean} [includeDelayedCalls] + * @returns {Timeline} Timeline instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.exportRoot() + */ + function exportRoot(vars?: TimelineVars, includeDelayedCalls?: boolean): core.Timeline; + + /** + * Creates a tween coming FROM the given values. + * + * ```js + * gsap.from(".class", {x: 100}); + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.from() + */ + function from(targets: TweenTarget, vars: TweenVars): core.Tween; + /** + * **Deprecated method signature.** Use the `duration` property instead. + * + * ```js + * gsap.from(".class", 1, {x: 100}); + * ``` + * @deprecated since 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.from() + */ + function from(targets: TweenTarget, duration: number, vars:TweenVars): core.Tween; + + /** + * Creates a tween coming FROM the first set of values going TO the second set of values. + * + * ```js + * gsap.fromTo(".class", {x: 0}, {x: 100}); + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} fromVars + * @param {TweenVars} toVars + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.fromTo() + */ + function fromTo(targets: TweenTarget, fromVars: TweenVars, toVars: TweenVars): core.Tween; + /** + * **Deprecated method signature.** Use the `duration` property instead. + * + * ```js + * gsap.fromTo(".class", 1, {x: 0}, {x: 100}); + * ``` + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {TweenVars} fromVars + * @param {TweenVars} toVars + * @returns {Tween} Tween instance + * @link https://greensock.com/docs/v3/GSAP/gsap.fromTo() + */ + function fromTo(targets: TweenTarget, duration: number, fromVars: TweenVars, toVars: TweenVars): core.Tween; + + /** + * Gets the tween or timeline with the specified ID if it exists. + * + * ```js + * gsap.to(obj, {id: "myTween", x: 100}); + * + * // later + * let tween = gsap.getById("myTween"); + * ``` + * + * @param {string | number} id + * @returns {Tween | undefined} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.getById() + */ + function getById<T extends core.Animation>(id: string | number): T | undefined; + + /** + * Gets the specified property of the target (or first of the targets) if it exists. + * + * ```js + * gsap.getProperty(element, "x"); + * ``` + * + * @param {TweenTarget} target + * @param {string} property + * @param {string} [unit] + * @returns {string | number} Value + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.getProperty() + */ + function getProperty(target: TweenTarget, property: string, unit?: string): string | number; + function getProperty(target: TweenTarget): (property: string, unit?: string) => string | number; + + /** + * Gets all of the tweens whose targets include the specified target or group of targets. + * + * ```js + * gsap.getTweensOf(element); + * ``` + * + * @param {TweenTarget} targets + * @param {boolean} [onlyActive] + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.getTweensOf() + */ + function getTweensOf(targets: TweenTarget, onlyActive?: boolean): core.Tween[]; + + /** + * Used to add all the GSAP globals to a particular tween object. + * + * ```js + * gsap.install(myTween); + * ``` + * + * @param {object} targets + * @returns {gsap} The gsap object + * @memberof gsap + */ + function install(targets: object): typeof gsap; + + /** + * Reports whether or not a particular object is actively animating. + * + * ```js + * gsap.isTweening("#id"); + * ``` + * + * @param {TweenTarget} targets + * @returns {boolean} Status + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.isTweening() + */ + function isTweening(targets: TweenTarget): boolean; + + /** + * Kills all the tweens (or specific tweening properties) of a particular object or the delayedCalls to a particular function. + * + * ```js + * gsap.killTweensOf(".myClass"); + * gsap.killTweensOf(myObject, "opacity,x"); + * ``` + * + * @param {TweenTarget} targets + * @param {object | string} [properties] + * @param {boolean} [onlyActive] + * @returns {void} Void + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.killTweensOf() + */ + function killTweensOf(targets: TweenTarget, properties?: object | string, onlyActive?: boolean): void; + + /** + * Creates a MatchMedia object for adding functions that run when a media query matches + * + * ```js + * let mm = gsap.matchMedia(myElement); + * mm.add("(max-width: 500px)", (context) => { + * gsap.to(".box", {x: 100}); + * }); + * ``` + * + * @param {Element | string | object} [scope] + * @returns {MatchMedia} MatchMedia object + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.matchMedia() + */ + function matchMedia(scope?: Element | string | object): MatchMedia; + + /** + * Immediately reverts all active/matching MatchMedia objects and then runs any that currently match. + * + * ```js + * gsap.matchMediaRefresh(); + * ``` + * + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.matchMediaRefresh() + */ + function matchMediaRefresh(): void; + + /** + * Returns the corresponding easing function for the given easing string. + * + * ```js + * let ease = gsap.parseEase("power1"); + * ``` + * + * @param {EaseString | EaseFunction} ease + * @returns {EaseFunction} Ease function + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.parseEase() + */ + function parseEase(ease: EaseString | EaseFunction): EaseFunction; + function parseEase(): EaseMap; + + /** + * Returns a function that acts as a simpler alternative of gsap.set() that is more performant but less versatile. + * + * ```js + * let setX = gsap.quickSetter("#id", "x", "px"); + * + * // later + * setX(100); + * ``` + * + * @param {TweenTarget} targets + * @param {string} property + * @param {string} [unit] + * @returns {Function} Setter function + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.quickSetter() + */ + function quickSetter(targets: TweenTarget, property: string, unit?: string): Function; + + /** + * Returns a reusable function that performantly redirects a specific property to a new value, restarting the animation each time you feed in a new number. + * + * ```js + * let xTo = gsap.quickTo("#id", "x", {duration: 0.8, ease: "power3"}); + * + * // later + * xTo(100); + * ``` + * + * @param {TweenTarget} target + * @param {string} property + * @param {TweenVars} vars + * @returns {QuickToFunc} Setter function + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.quickTo() + */ + function quickTo(target: TweenTarget, property: string, vars?: TweenVars): QuickToFunc; + + /** + * Register custom easing functions with GSAP, giving it a name so it can be referenced in any tweens. + * + * ```js + * gsap.registerEase("myEaseName", function(progress) { + * return progress; //linear + * }); + * ``` + * + * @param {string} name + * @param {EaseFunction} ease + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.registerEase() + */ + function registerEase(name: string, ease: EaseFunction): void; + + // TODO: Create interface for effect + /** + * Registers custom effects (named tweens) for reuse with optional arguments. + * + * ```js + * // register the effect with GSAP: + * gsap.registerEffect({ + * name: "fade", + * effect: (targets, config) => { + * return gsap.to(targets, {duration: config.duration, opacity: 0}); + * }, + * defaults: {duration: 2}, //defaults get applied to any "config" object passed to the effect + * extendTimeline: true, //now you can call the effect directly on any GSAP timeline to have the result immediately inserted in the position you define (default is sequenced at the end) + * }); + * + * // now we can use it like this: + * gsap.effects.fade(".box"); + * // or + * tl.fade(".box", {duration: 3}) + * ``` + * + * @param {object} effect + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.registerEffect() + */ + function registerEffect(effect: object): void; + + /** + * Installs the specified GSAP plugins, provided they have been loaded already. + * + * ```js + * gsap.registerPlugin(MorphSVPlugin, MotionPathPlugin); + * ``` + * + * @param {RegisterablePlugins[]} args + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.registerPlugin() + */ + function registerPlugin(...args: object[]): void; + + /** + * Immediately sets properties of the target(s) to the properties specified. + * + * ```js + * gsap.set(".class", {x: 100, y: 50, opacity: 0}); + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.set() + */ + function set(targets: TweenTarget, vars: TweenVars): core.Tween; + + /** + * Creates a new timeline, used to compose sequences of tweens. + * + * @param {TimelineVars} [vars] + * @returns {Timeline} Timeline instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.timeline() + */ + function timeline(vars?: TimelineVars): core.Timeline; + + /** + * Creates a tween going TO the given values. + * + * ```js + * gsap.to(".class", {x: 100}); + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.to() + */ + function to(targets: TweenTarget, vars: TweenVars): core.Tween; + /** + * **Deprecated method signature.** Use the `duration` property instead. + * + * ```js + * gsap.to(".class", 1, {x: 100}); + * ``` + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.to() + */ + function to(targets: TweenTarget, duration: number, vars: TweenVars): core.Tween; + + /** + * Manually update the root (global) timeline. Make sure to unhook GSAP's default ticker. + * + * ```js + * // unhooks the GSAP ticker + * gsap.ticker.remove(gsap.updateRoot); + * + * // sets the root time to 20 seconds manually + * gsap.updateRoot(20); + * ``` + * + * @param {number} number + * @returns {void} Void + * @memberof gsap + * @link https://greensock.com/docs/v3/GSAP/gsap.updateRoot() + */ + function updateRoot(time: number): void; +} + +// TODO: Move to files where declared +/** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ +declare class TweenLite extends gsap.core.Tween {} + +/** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ +declare class TweenMax extends gsap.core.Tween {} + +/** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ +declare class TimelineLite extends gsap.core.Timeline {} + +/** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ +declare class TimelineMax extends gsap.core.Timeline {} + +declare module "gsap/gsap-core" { + + const _gsap: typeof gsap; + + // TODO: Move to files where declared + /** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ + export class TweenLite extends gsap.core.Tween {} + + /** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ + export class TweenMax extends gsap.core.Tween {} + + /** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ + export class TimelineLite extends gsap.core.Timeline {} + + /** + * @deprecated since 3.0.0 + * @link https://greensock.com/3-release-notes/ + */ + export class TimelineMax extends gsap.core.Timeline {} + + export { + _gsap as gsap, + _gsap as default + } +} + +declare module "gsap/src/gsap-core" { + export * from "gsap/gsap-core"; + export { gsap as default } from "gsap/gsap-core"; +} + +declare module "gsap-trial/gsap-core" { + export * from "gsap/gsap-core"; + export { gsap as default } from "gsap/gsap-core"; +} + +declare module "gsap-trial/src/gsap-core" { + export * from "gsap/gsap-core"; + export { gsap as default } from "gsap/gsap-core"; +} diff --git a/node_modules/gsap/types/gsap-plugins.d.ts b/node_modules/gsap/types/gsap-plugins.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..870c10d587ab8780609044156c100790af95666f --- /dev/null +++ b/node_modules/gsap/types/gsap-plugins.d.ts @@ -0,0 +1,107 @@ +declare namespace gsap { + + type PluginInit = ( + this: PluginScope, + target: object, + endValue: any, + tween: core.Tween, + index: number, + targets: object[] + ) => void; + + type PluginRender = (this: PropTween, progress: number, data: PluginScope) => void; + type PluginKill = (this: PluginScope) => void; + + interface PluginAliases { + [key: string]: string; + } + + // TODO: Incomplete + interface PropTween { + _next: PropTween; + _prev: PropTween; + d: PluginScope; + r: PluginRender; + t: object; + modifier(...args: any[]): any; // TODO: Add signature + } + + interface PluginScope { + [key: string]: any; + _props: string[]; + _pt: PropTween; + add(...args: any[]): PropTween; // TODO: Add signature + name: string; + init: PluginInit; + kill: PluginKill; + render: PluginRender; + } + + interface PluginStatic { + [key: string]: any; + targetTest(...args: any[]): any; // TODO: Add signature + get(target: object, prop: string): any; // TODO: Add signature + getSetter(...args: any[]): any; // TODO: Add signature + aliases: PluginAliases; + register(core: typeof gsap): void; + } + + interface Plugin extends Partial<PluginStatic> { + name: string; + init: PluginInit; + kill?: PluginKill; + render?: PluginRender; + } + + interface AttrVars { + [key: string]: ArrayValue | TweenValue; + } + + interface ModifiersVars { + [key: string]: (value: any, target: any) => any; + } + + interface SnapVars { + [key: string]: number | number[] | { values: number[], radius?: number }; + } + + interface TweenVars { + attr?: AttrVars; + modifiers?: ModifiersVars; + snap?: string | SnapVars; + } +} + +declare namespace gsap.plugins { + + interface AttrPlugin extends Plugin {} + interface ModifiersPlugin extends Plugin {} + interface SnapPlugin extends Plugin {} + + interface AttrPluginClass extends AttrPlugin { + new(): PluginScope & AttrPlugin; + prototype: PluginScope & AttrPlugin; + } + + interface ModifiersPluginClass extends ModifiersPlugin { + new(): PluginScope & ModifiersPlugin; + prototype: PluginScope & ModifiersPlugin; + } + + interface SnapPluginClass extends SnapPlugin { + new(): PluginScope & SnapPlugin; + prototype: PluginScope & SnapPlugin; + } + + const attr: AttrPluginClass; + const modifiers: ModifiersPluginClass; + const snap: SnapPluginClass; + + // Data types shared between plugins + type RawPath = number[][]; + type Matrix2D = { a: number, b: number, c: number, d: number, e: number, f: number}; +} + +declare const AttrPlugin: gsap.plugins.AttrPlugin; +declare const ModifiersPlugin: gsap.plugins.ModifiersPlugin; +declare const SnapPlugin: gsap.plugins.SnapPlugin; diff --git a/node_modules/gsap/types/gsap-utils.d.ts b/node_modules/gsap/types/gsap-utils.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..3106f4ae32a309b9bd619253ca47882201b8791f --- /dev/null +++ b/node_modules/gsap/types/gsap-utils.d.ts @@ -0,0 +1,508 @@ +declare namespace gsap.utils { + + interface DistributeConfig { + amount?: number; + axis?: "x" | "y"; + base?: number; + each?: number; + ease?: string | EaseFunction; + from?: "start" | "center" | "end" | "edges" | "random" | number | [number, number]; + grid?: "auto" | [number, number]; + } + + interface SnapNumberConfig { + increment?: number; + values?: number[]; + radius: number; + } + + interface SnapPoint2DConfig { + values: Point2D[]; + radius: number; + } + + interface SelectorFunc { + <K extends keyof HTMLElementTagNameMap>(selectorText: string): Array<HTMLElementTagNameMap[K]>; + <K extends keyof SVGElementTagNameMap>(selectorText: string): Array<SVGElementTagNameMap[K]>; + <E extends Element = Element>(selectorText: string): Array<E>; + } + + /** + * Prefixes the provided CSS property if necessary. Returns null if the property isn't supported at all. + * + * ```js + * // The following may return "filter", "WebkitFilter", or "MozFilter" depending on the browser + * let filterProperty = gsap.utils.checkPrefix("filter"); + * ``` + * + * @param {string} property + * @returns {string | null} The appropriately prefixed property + * @memberof gsap.utils + */ + function checkPrefix(property: string): string; + + /** + * Clamps a number between a given minimum and maximum. + * + * ```js + * gsap.utils.clamp(0, 100, 105); // returns 100 + * + * const clamper = gsap.utils.clamp(0, 100); // no value = reusable function + * console.log(clamper(105)); // returns 100 + * ``` + * + * @param {number} minimum + * @param {number} maximum + * @param {number} [valueToClamp] + * @returns {number | Function} The clamped number or function to clamp to given range + * @memberof gsap.utils + */ + function clamp(minimum: number, maximum: number, valueToClamp: number): number; + function clamp(minimum: number, maximum: number): (valueToClamp: number) => number; + + /** + * Returns a function to distribute an array of values based on the inputs that you give it. + * + * ```js + * gsap.utils.distribute({ + * base: 50, + * amount: 100, + * from: "center", + * grid: "auto", + * axis: "y", + * ease: "power1.inOut" + * }); + * ``` + * + * @param {DistributeConfig} config + * @returns {FunctionBasedValue<number>} The clamped number or function to clamp to given range + * @memberof gsap.utils + */ + function distribute(config: DistributeConfig): FunctionBasedValue<number>; + + /** + * Returns unit of a given string where the number comes first, then the unit. + * + * ```js + * gsap.utils.getUnit("50%"); // "%" + * ``` + * + * @param {string} value + * @returns {string} The unit + * @memberof gsap.utils + */ + function getUnit(value: string): string; + + /** + * Linearly interpolates between any two values of a similar type. + * + * ```js + * gsap.utils.interpolate(0, 500, 0.5); // 250 + * + * const interp = gsap.utils.interpolate(0, 100); // no value = reusable function + * console.log( interp(0.5) ); // 50 + * ``` + * + * @param {T} startValue + * @param {T} endValue + * @param {number} [number] + * @returns {T | Function<number>} The interpolated value or interpolate function + * @memberof gsap.utils + */ + function interpolate<T>(startValue: T, endValue: T, progress: number): T; + function interpolate<T>(startValue: T, endValue: T): (progress: number) => T; + /** + * Linearly interpolates between any two values of a similar type. + * + * ```js + * gsap.utils.interpolate([100, 50, 500], 0.5); // 50 + * + * c interp = gsap.utils.interpolate([100, 50, 500]); // no value = reusable function + * console.log( interp(0.5) ); // 50 + * ``` + * + * @param {T[]} array + * @param {number} progress + * @returns {T | Function} The interpolated value or interpolate function + * @memberof gsap.utils + */ + function interpolate<T>(array: T[], progress: number): T; + function interpolate<T>(array: T[]): (progress: number) => T; + + /** + * Maps a number's relative placement within one range to the equivalent position in another range. + * + * ```js + * gsap.utils.mapRange(-10, 10, 100, 200, 0); // 150 + * + * const mapper = gsap.utils.mapRange(0, 100, 0, 250); // no value = reusable function + * console.log( mapper(50) ); // 250 + * ``` + * + * @param {number} inMin + * @param {number} inMax + * @param {number} outMin + * @param {number} outMax + * @param {number} [value] + * @returns {number | Function} The mapped value or map function + * @memberof gsap.utils + */ + function mapRange(inMin: number, inMax: number, outMin: number, outMax: number, value: number): number; + function mapRange(inMin: number, inMax: number, outMin: number, outMax: number): (value: number) => number; + + /** + * Maps a value within a provided range to the corresponding position in the range between 0 and 1. + * + * ```js + * gsap.utils.normalize(-10, 10, 0); // 0.5 + * + * const clamper = gsap.utils.normalize(0, 100); // no value = reusable function + * console.log( clamper(50) ); // 0.5 + * ``` + * + * @param {number} inMin + * @param {number} inMax + * @param {number} [value] + * @returns {number | Function} The normalized value or normalizer function + * @memberof gsap.utils + */ + function normalize(inMin: number, inMax: number, value: number): number; + function normalize(inMin: number, inMax: number): (value: number) => number; + + /** + * Strings together multiple function calls, passing the result from one to the next. + * You can pass in as many function references as you'd like! + * + * ```js + * const transfrom = gsap.utils.pipe(func1, func2, func3); // reusable function + * const output = transform(input); + * ``` + * + * @param {Function} ab + * @param {Function} bc + * @param {Function} [cd] + * @returns {Function} The function that pipes values from function to function given + * @memberof gsap.utils + */ + function pipe<A extends Array<unknown>, B>( + ab: (...a: A) => B + ): (...a: A) => B; + function pipe<A extends Array<unknown>, B, C>( + ab: (...a: A) => B, + bc: (b: B) => C + ): (...a: A) => C + function pipe<A extends Array<unknown>, B, C, D>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D + ): (...a: A) => D; + function pipe<A extends Array<unknown>, B, C, D, E>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D, + de: (d: D) => E + ): (...a: A) => E; + function pipe<A extends Array<unknown>, B, C, D, E, F>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D, + de: (d: D) => E, + ef: (e: E) => F + ): (...a: A) => F; + function pipe<A extends Array<unknown>, B, C, D, E, F, G>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D, + de: (d: D) => E, + ef: (e: E) => F, + fg: (f: F) => G + ): (...a: A) => G; + function pipe<A extends Array<unknown>, B, C, D, E, F, G, H>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D, + de: (d: D) => E, + ef: (e: E) => F, + fg: (f: F) => G, + gh: (g: G) => H + ): (...a: A) => H; + function pipe<A extends Array<unknown>, B, C, D, E, F, G, H, I>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D, + de: (d: D) => E, + ef: (e: E) => F, + fg: (f: F) => G, + gh: (g: G) => H, + hi: (h: H) => I + ): (...a: A) => I; + function pipe<A extends Array<unknown>, B, C, D, E, F, G, H, I, J>( + ab: (...a: A) => B, + bc: (b: B) => C, + cd: (c: C) => D, + de: (d: D) => E, + ef: (e: E) => F, + fg: (f: F) => G, + gh: (g: G) => H, + hi: (h: H) => I, + ij: (i: I) => J + ): (...a: A) => J; + + /** + * Get a random number within a range, optionally rounding to an increment you provide. + * + * ```js + * gsap.utils.random(-100, 100); + * gsap.utils.random(0, 500, 5); // snapped to the nearest value of 5 + * + * const random = gsap.utils.random(-200, 500, 10, true); // reusable function + * console.log( random() ); + * ``` + * + * @param {number} minValue + * @param {number} maxValue + * @param {number} [snapIncrement] + * @param {boolean} [returnFunction] + * @returns {number | Function} The random number or random number generator function + * @memberof gsap.utils + */ + function random(minValue: number, maxValue: number, snapIncrement?: number): number; + function random<T extends boolean>(minValue: number, maxValue: number, returnFunction?: T): T extends true ? () => number : number; + function random<T extends boolean>(minValue: number, maxValue: number, snapIncrement: number, returnFunction?: T): T extends true ? () => number : number; + /** + * Get a random random element in an array. + * + * ```js + * gsap.utils.random(["red", "blue", "green"]); //"red", "blue", or "green" + * + * const random = gsap.utils.random([0, 100, 200], true); + * console.log( random() ); // 0, 100, or 200 (randomly selected) + * ``` + * + * @param {T[]} array + * @param {boolean} [returnFunction] + * @returns {number | Function} The random number or random number generator function + * @memberof gsap.utils + */ + function random<T>(array: T[]): T; + function random<T, U extends boolean>(array: T[], returnFunction?: U): U extends true ? () => T : T; + + /** + * Returns a selector function that is scoped to a particular Element. + * + * ```js + * const q = gsap.utils.selector("#id"); + * const q = gsap.utils.selector(myElement); + * gsap.to(q(".class"), {x: 100}); + * ``` + * + * @param {Element | object | string} scope + * @returns {SelectorFunc} A selector function + * @memberof gsap.utils + */ + function selector(scope: Element | object | string | null): SelectorFunc; + + /** + * Takes an array and randomly shuffles it, returning the same (but shuffled) array. + * + * ```js + * gsap.utils.shuffle(array); + * ``` + * + * @param {T[]} array + * @returns {T[]} The same shuffled array + * @memberof gsap.utils + */ + function shuffle<T>(array: T[]): T[]; + + /** + * Snaps a value to the nearest increment of the number provided. + * Or snaps to a value in the given array. + * Or snaps to a value within the given radius (if an object is provided). + * Or returns a function that does the above (if the second value is not provided). + * + * ```js + * gsap.utils.snap(10, 23.5); // 20 + * gsap.utils.snap([100, 50, 500], 65); // 50 + * gsap.utils.snap({values:[0, 100, 300], radius:20}, 30.5); // 30.5 + * gsap.utils.snap({increment:500, radius:150}, 310); // 310 + * + * const snap = gsap.utils.snap(5); // no value = reusable function + * console.log( snap(0.5) ); // 0 + * ``` + * + * @param {SnapNumberConfig} snapConfig + * @param {number} [valueToSnap] + * @returns {number | Function} The snapped number or snap function + * @memberof gsap.utils + */ + function snap(snapConfig: number | number[] | SnapNumberConfig, valueToSnap: number): number; + function snap(snapConfig: number | number[] | SnapNumberConfig): (valueToSnap: number) => number; + /** + * Snaps a value if within the given radius of a points (objects with "x" and "y" properties). + * Or returns a function that does the above (if the second value is not provided). + * + * ```js + * + * gsap.utils.snap({values:[0, 100, 300], radius:20}, 85); // 100 + * + * const snap = gsap.utils.snap({values:[{x:0, y:0}, {x:10, y:10}, {x:20, y:20}], radius:5}); // no value = reusable function + * console.log( snap({x:8, y:8}) ); // {x:10, y:10} + * ``` + * + * @param {SnapPoint2DConfig} snapConfig + * @param {number} [valueToSnap] + * @returns {Point2D | Function} The snapped number or snap function + * @memberof gsap.utils + */ + function snap(snapConfig: SnapPoint2DConfig, valueToSnap: Point2D): Point2D; + function snap(snapConfig: SnapPoint2DConfig): (valueToSnap: Point2D) => Point2D; + + /** + * Converts a string-based color value into an array consisting of RGB(A) or HSL values. + * + * ```js + * gsap.utils.splitColor("red"); // [255, 0, 0] + * gsap.utils.splitColor("rgba(204, 153, 51, 0.5)"); // [204, 153, 51, 0.5] + * + * gsap.utils.splitColor("#6fb936", true); // [94, 55, 47] - HSL value + * ``` + * + * @param {string} color + * @param {boolean} [hsl] + * @returns {[number, number, number] | [number, number, number, number]} The converted color array + * @memberof gsap.utils + */ + function splitColor(color: string, hsl?: boolean): [number, number, number] | [number, number, number, number]; + + /** + * Converts almost anything into a flat Array. + * + * ```js + * const targets = gsap.utils.toArray(".class"); + * const targets = gsap.utils.toArray(myElement); + * const targets = gsap.utils.toArray($(".class")); + * const targets = gsap.utils.toArray([".class1", ".class2"]); + * ``` + * + * @param {string | object | Element | null} value + * @param {object} [scope] + * @param {boolean} [leaveStrings] + * @returns {T[]} The converted Array + * @memberof gsap.utils + */ + function toArray<T>(value: string | object | Element | null, scope?: object | null, leaveStrings?: boolean): T[]; + + /** + * Ensures that a specific unit gets applied. + * + * ```js + * const clamp = gsap.utils.unitize( gsap.utils.clamp(0, 100), "px"); + * clamp(132); // "100px" + * + * gsap.to(".class", { + * x: 1000, + * modifiers: { + * x: gsap.utils.unitize( gsap.utils.wrap(0, window.innerWidth), "px") + * } + * }); + * ``` + * + * @param {Function} fn + * @param {string} [unit] + * @returns {string} The value with unit added + * @memberof gsap.utils + */ + function unitize<T extends Array<unknown>>(fn: (...args: T) => unknown, unit?: string): (...args: T) => string; + + /** + * Returns the next number in a range after the given index, jumping to the start after the end has been reached. + * + * ```js + * let color = gsap.utils.wrap(["red", "green", "yellow"], 5); // "yellow" + * + * let wrapper = gsap.utils.wrap(["red", "green", "yellow"]); // no value = reusable function + * let color = wrapper(5) // "yellow" + * ``` + * + * @param {number} value1 + * @param {number} value2 + * @param {number} [index] + * @returns {string} The wrapped value or wrap function + * @memberof gsap.utils + */ + function wrap(value1: number, value2: number, index: number): number; + function wrap(value1: number, value2: number): (index: number) => number; + /** + * Returns the next item in an array after the given index, jumping to the start after the end has been reached. + * + * ```js + * let color = gsap.utils.wrap(["red", "green", "yellow"], 5); // "yellow" + * + * let wrapper = gsap.utils.wrap(["red", "green", "yellow"]); // no value = reusable function + * let color = wrapper(5) // "yellow" + * ``` + * + * @param {T[]} values + * @param {number} [index] + * @returns {string} The wrapper value or wrap function + * @memberof gsap.utils + */ + function wrap<T>(values: T[], index: number): T; + function wrap<T>(values: T[]): (index: number) => T; + + /** + * Returns the next number in a range after the given index, wrapping backwards towards the start after the end has been reached. + * + * ```js + * let color = gsap.utils.wrap(["red", "green", "yellow"], 5); // "yellow" + * + * let wrapper = gsap.utils.wrap(["red", "green", "yellow"]); // no value = reusable function + * let color = wrapper(5) // "yellow" + * ``` + * + * @param {number} value1 + * @param {number} value2 + * @param {number} [index] + * @returns {string} The wrapped value or wrap function + * @memberof gsap.utils + */ + function wrapYoyo(value1: number, value2: number, index: number): number; + function wrapYoyo(value1: number, value2: number): (index: number) => number; + /** + * Returns the next item in an array after the given index, wrapping backwards towards the start after the end has been reached. + * + * ```js + * let color = gsap.utils.wrap(["red", "green", "yellow"], 5); // "yellow" + * + * let wrapper = gsap.utils.wrap(["red", "green", "yellow"]); // no value = reusable function + * let color = wrapper(5) // "yellow" + * ``` + * + * @param {T[]} values + * @param {number} [index] + * @returns {string} The wrapper value or wrap function + * @memberof gsap.utils + */ + function wrapYoyo<T>(values: T[], index: number): T; + function wrapYoyo<T>(values: T[]): (index: number) => T; +} + +declare module "gsap/gsap-core" { + export const clamp: typeof gsap.utils.clamp; + export const distribute: typeof gsap.utils.distribute; + export const getUnit: typeof gsap.utils.getUnit; + export const interpolate: typeof gsap.utils.interpolate; + export const mapRange: typeof gsap.utils.mapRange; + export const normalize: typeof gsap.utils.normalize; + export const pipe: typeof gsap.utils.pipe; + export const random: typeof gsap.utils.random; + export const selector: typeof gsap.utils.selector; + export const shuffle: typeof gsap.utils.shuffle; + export const snap: typeof gsap.utils.snap; + export const splitColor: typeof gsap.utils.splitColor; + export const toArray: typeof gsap.utils.toArray; + export const unitize: typeof gsap.utils.unitize; + export const wrap: typeof gsap.utils.wrap; + export const wrapYoyo: typeof gsap.utils.wrapYoyo; +} diff --git a/node_modules/gsap/types/index.d.ts b/node_modules/gsap/types/index.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..6d81c2ce1784ab46afee9a775fe5bce1b45f12ee --- /dev/null +++ b/node_modules/gsap/types/index.d.ts @@ -0,0 +1,128 @@ +// Type definitions for gsap 3 +// Project: https://gsap.com/ +// Definitions by: Jack Doyle <https://github.com/jackdoyle> +// Blake Bowen <https://github.com/OSUblake> +// Pedro Tavares <https://github.com/dipscom> +// Zach Saucier <https://github.com/ZachSaucier> +// Definitions: https://github.com/greensock/GSAP + +/// <reference path="animation.d.ts"/> +/// <reference path="custom-bounce.d.ts"/> +/// <reference path="custom-ease.d.ts"/> +/// <reference path="custom-wiggle.d.ts"/> +/// <reference path="css-plugin.d.ts"/> +/// <reference path="css-rule-plugin.d.ts"/> +/// <reference path="draggable.d.ts"/> +/// <reference path="draw-svg-plugin.d.ts"/> +/// <reference path="ease.d.ts"/> +/// <reference path="easel-plugin.d.ts"/> +/// <reference path="flip.d.ts"/> +/// <reference path="gs-dev-tools.d.ts"/> +/// <reference path="gsap-plugins.d.ts"/> +/// <reference path="gsap-utils.d.ts"/> +/// <reference path="inertia-plugin.d.ts"/> +/// <reference path="morph-svg-plugin.d.ts"/> +/// <reference path="motion-path-plugin.d.ts"/> +/// <reference path="motion-path-helper.d.ts"/> +/// <reference path="observer.d.ts"/> +/// <reference path="physics-2d-plugin.d.ts"/> +/// <reference path="physics-props-plugin.d.ts"/> +/// <reference path="pixi-plugin.d.ts"/> +/// <reference path="scramble-text-plugin.d.ts"/> +/// <reference path="scroll-to-plugin.d.ts"/> +/// <reference path="scroll-trigger.d.ts"/> +/// <reference path="scroll-smoother.d.ts"/> +/// <reference path="split-text.d.ts"/> +/// <reference path="text-plugin.d.ts"/> +/// <reference path="timeline.d.ts"/> +/// <reference path="tween.d.ts"/> +/// <reference path="utils/velocity-tracker.d.ts"/> +/// <reference path="gsap-core.d.ts"/> + +// Global types +type GSAPDraggableVars = Draggable.Vars; +type GSAPAnimation = gsap.core.Animation; +type GSAPCallback = gsap.Callback; +type GSAPDistributeConfig = gsap.utils.DistributeConfig; +type GSAPPlugin = gsap.Plugin; +type GSAPPluginScope = gsap.PluginScope; +type GSAPPluginStatic = gsap.PluginStatic; +type GSAPStaggerVars = gsap.StaggerVars; +type GSAPTickerCallback = gsap.TickerCallback; +type GSAPTimeline = gsap.core.Timeline; +type GSAPTimelineVars = gsap.TimelineVars; +type GSAPTween = gsap.core.Tween; +type GSAPTweenTarget = gsap.TweenTarget; +type GSAPTweenVars = gsap.TweenVars; + +type GSAP = typeof gsap; + +declare module "gsap" { + export * from "gsap/gsap-core"; + export { gsap as default } from "gsap/gsap-core"; +} + +declare module "gsap/src" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap/src/index" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap/dist" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap/dist/gsap" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap/all" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap/src/all" { + export * from "gsap/all"; + export { gsap as default } from "gsap/all"; +} + +declare module "gsap-trial" { + export * from "gsap/gsap-core"; + export { gsap as default } from "gsap/gsap-core"; +} + +declare module "gsap-trial/src" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap-trial/src/index" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap-trial/dist" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap-trial/dist/gsap" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap-trial/all" { + export * from "gsap"; + export { gsap as default } from "gsap"; +} + +declare module "gsap-trial/src/all" { + export * from "gsap/all"; + export { gsap as default } from "gsap/all"; +} diff --git a/node_modules/gsap/types/inertia-plugin.d.ts b/node_modules/gsap/types/inertia-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..4e912b79a9129af22e09e28e02906e69f26fd1a6 --- /dev/null +++ b/node_modules/gsap/types/inertia-plugin.d.ts @@ -0,0 +1,105 @@ +declare namespace gsap { + + type InertiaEndFunction<T> = (value: T) => T; + + interface InertiaDuration { + min?: number; + max?: number; + overshoot?: number; + } + + interface InertiaLinkedProps { + [key: string]: number; + } + + interface InertiaObject { + min?: number; + max?: number; + end?: number | number[] | InertiaEndFunction<any>; + // end?: number | number[] | InertiaEndFunction<number> | InertiaEndFunction<InertiaLinkedProps>; + velocity?: number | "auto"; + } + + type InertiaVars = { + duration?: number | InertiaDuration, + linkedProps?: string, + resistance?: number + } & { + [key: string]: TweenValue | InertiaObject + }; + + interface TweenVars { + inertia?: InertiaVars; + } +} + +declare namespace gsap.plugins { + + interface InertiaPlugin extends Plugin, VelocityTrackerStatic { + // TODO add missing methods + // TODO improve docs on site as well + + /** + * Returns the current velocity of the given property and target object (only works if you started tracking the property using the InertiaPlugin.track() method). + * + * ```js + * InertiaPlugin.getVelocity(obj, "x,y"); + * ``` + * + * @param {Element} target + * @param {string} props + * @returns {number} The current velocity + * @memberof InertiaPlugin + * @link https://greensock.com/docs/v3/Plugins/InertiaPlugin/static.getVelocity() + */ + getVelocity(target: Element, props: string): number; + } + + interface InertiaPluginClass extends InertiaPlugin { + new(): PluginScope & InertiaPlugin; + prototype: PluginScope & InertiaPlugin; + } + + const inertia: InertiaPluginClass; +} + +declare const InertiaPlugin: gsap.plugins.InertiaPlugin; + +declare module "gsap/InertiaPlugin" { + export * from "gsap/utils/VelocityTracker"; + export const InertiaPlugin: gsap.plugins.InertiaPlugin; + export { InertiaPlugin as default }; +} + +declare module "gsap/dist/InertiaPlugin" { + export * from "gsap/InertiaPlugin"; + export { InertiaPlugin as default } from "gsap/InertiaPlugin"; +} + +declare module "gsap/src/InertiaPlugin" { + export * from "gsap/InertiaPlugin"; + export { InertiaPlugin as default } from "gsap/InertiaPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/InertiaPlugin"; +} + +declare module "gsap-trial/InertiaPlugin" { + export * from "gsap/InertiaPlugin"; + export { InertiaPlugin as default } from "gsap/InertiaPlugin"; +} + +declare module "gsap-trial/dist/InertiaPlugin" { + export * from "gsap/InertiaPlugin"; + export { InertiaPlugin as default } from "gsap/InertiaPlugin"; +} + +declare module "gsap-trial/src/InertiaPlugin" { + export * from "gsap/InertiaPlugin"; + export { InertiaPlugin as default } from "gsap/InertiaPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/InertiaPlugin"; +} diff --git a/node_modules/gsap/types/morph-svg-plugin.d.ts b/node_modules/gsap/types/morph-svg-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..525a5be72a9df51ba46b3414594aceda0b337c6b --- /dev/null +++ b/node_modules/gsap/types/morph-svg-plugin.d.ts @@ -0,0 +1,152 @@ +declare namespace gsap { + + interface TweenVars { + morphSVG?: SVGPathValue | gsap.plugins.MorphSVGVars; + } +} + +declare namespace gsap.plugins { + + interface MorphSVGPlugin extends Plugin { + + defaultRender?: Function; + defaultType?: String; + defaultUpdateTarget?: Boolean; + + /** + * Converts SVG shapes into <path>s. + * + * ```js + * MorphSVGPlugin.convertToPath("circle"); + * ``` + * + * @param {DOMTarget} shape + * @param {boolean} [swap] + * @returns {SVGPathElement[]} The converted paths + * @memberof MorphSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/MorphSVGPlugin/static.convertToPath() + */ + convertToPath(shape: string | SVGPrimitive | (string | SVGPrimitive)[], swap?: boolean): SVGPathElement[]; + + /** + * Returns a RawPath associated with whatever is passed in (path data string, selector text, <path> element, or a RawPath) + * + * ```js + * MorphSVGPlugin.getRawPath("#my-path"); + * ``` + * + * @param {SVGPathValue} path + * @returns {array} The RawPath + * @memberof MorphSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/MorphSVGPlugin/static.getRawPath() + */ + getRawPath(path: SVGPathValue): string[]; + + /** + * Accepts two strings representing SVG path data and matches the number of points between them, returning an Array with the edited path data strings [shape1, shape2]. + * + * ```js + * MorphSVGPlugin.normalizeStrings(shape1, shape2, {map: "complexity"}); + * ``` + * + * @param {string} shape1 + * @param {string} shape2 + * @param {NormalizeVars} config + * @returns {string[]} An Array containing the converted string data with matching numbers of points, like [shape1, shape2] + * @memberof MorphSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/MorphSVGPlugin/static.normalizeStrings() + */ + normalizeStrings(shape1: string, shape2: string, config?: NormalizeVars): string[]; + + /** + * Converts a RawPath into a string of path data. + * + * ```js + * MorphSVGPlugin.rawPathToString(myRawPath); + * ``` + * + * @param {RawPath} rawPath + * @returns {string} The converted path data + * @memberof MorphSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/MorphSVGPlugin/static.rawPathToString() + */ + rawPathToString(rawPath: RawPath): string; + + /** + * Converts a string of path data into a RawPath. + * + * ```js + * MorphSVGPlugin.stringToRawPath("M0,0 C100,20 300,50 400,0..."); + * ``` + * + * @param {string} data + * @returns {RawPath} The converted RawPath + * @memberof MorphSVGPlugin + * @link https://greensock.com/docs/v3/Plugins/MorphSVGPlugin/static.stringToRawPath() + */ + stringToRawPath(data: string): RawPath; + } + + interface NormalizeVars { + shapeIndex?: number | "auto" | number[]; + map?: "complexity" | "position" | "size"; + } + + interface MorphSVGPluginClass extends MorphSVGPlugin { + new(): PluginScope & MorphSVGPlugin; + prototype: PluginScope & MorphSVGPlugin; + } + + interface MorphSVGVars { + shape: SVGPathValue; + type?: "rotational" | "linear"; + origin?: string; + shapeIndex?: number | "auto" | number[]; + precompile?: "log" | string[]; + map?: "size" | "position" | "complexity"; + render?: Function; + updateTarget?: boolean; + } + + const morphSVG: MorphSVGPluginClass; +} + +declare const MorphSVGPlugin: gsap.plugins.MorphSVGPlugin; + +declare module "gsap/MorphSVGPlugin" { + export const MorphSVGPlugin: gsap.plugins.MorphSVGPlugin; + export { MorphSVGPlugin as default }; +} + +declare module "gsap/dist/MorphSVGPlugin" { + export * from "gsap/MorphSVGPlugin"; + export { MorphSVGPlugin as default } from "gsap/MorphSVGPlugin"; +} + +declare module "gsap/src/MorphSVGPlugin" { + export * from "gsap/MorphSVGPlugin"; + export { MorphSVGPlugin as default } from "gsap/MorphSVGPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/MorphSVGPlugin"; +} + +declare module "gsap-trial/MorphSVGPlugin" { + export * from "gsap/MorphSVGPlugin"; + export { MorphSVGPlugin as default } from "gsap/MorphSVGPlugin"; +} + +declare module "gsap-trial/dist/MorphSVGPlugin" { + export * from "gsap/MorphSVGPlugin"; + export { MorphSVGPlugin as default } from "gsap/MorphSVGPlugin"; +} + +declare module "gsap-trial/src/MorphSVGPlugin" { + export * from "gsap/MorphSVGPlugin"; + export { MorphSVGPlugin as default } from "gsap/MorphSVGPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/MorphSVGPlugin"; +} diff --git a/node_modules/gsap/types/motion-path-helper.d.ts b/node_modules/gsap/types/motion-path-helper.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..535b57762ce6747caed2221045cbd7191bd10fb2 --- /dev/null +++ b/node_modules/gsap/types/motion-path-helper.d.ts @@ -0,0 +1,120 @@ +declare class MotionPathHelper { + + constructor(target: gsap.DOMTarget, vars?: MotionPathHelper.Vars); + + /** + * Kills the MotionPathHelper instance, removing the editing elements from the DOM. + * + * ```js + * myHelper.kill(); + * ``` + * + * @memberof MotionPathHelper + * @link https://greensock.com/docs/v3/Plugins/MotionPathHelper/kill() + */ + kill(): void + + /** + * Create a MotionPathHelper instance. + * + * ```js + * MotionPathHelper.create(".myClass"); + * ``` + * + * @param {gsap.DOMTarget} target + * @param {MotionPathHelper.Vars} [vars] + * @returns {MotionPathHelper} The MotionPathHelper instance + * @memberof MotionPathHelper + * @link https://greensock.com/docs/v3/Plugins/MotionPathHelper + */ + static create(target: gsap.DOMTarget, vars?: MotionPathHelper.Vars): MotionPathHelper; + + /** + * Makes an SVG <path> editable in the browser. + * + * ```js + * MotionPathHelper.editPath(".myClass", { + * onPress: () => console.log("press"), + * onRelease: () => console.log("release"), + * onUpdate: () => console.log("update") + * }); + * ``` + * + * @param {gsap.DOMTarget} target + * @param {MotionPathHelper.EditPathVars} [vars] + * @returns {object} A PathEditor instance + * @memberof MotionPathHelper + * @link https://greensock.com/docs/v3/Plugins/MotionPathHelper/static.editPath() + */ + static editPath(target: gsap.DOMTarget, vars?: MotionPathHelper.EditPathVars): MotionPathHelper; +} + +declare namespace MotionPathHelper { + interface Vars { + [key: string]: any; + ease?: string | gsap.EaseFunction; + end?: number; + duration?: number; + path?: gsap.DOMTarget; + pathColor?: gsap.TweenValue; + pathWidth?: number; + pathOpacity?: number; + selected?: boolean; + start?: number; + } + + interface EditPathVars { + [key: string]: any; + anchorSnap?: Function; + callbackScope?: object; + draggable?: boolean; + handleSize?: number; + handleSnap?: Function; + onDeleteAnchor?: Function; + onPress?: Function; + onRelease?: Function; + onUpdate?: Function; + selected?: boolean; + } +} + +declare module "gsap/MotionPathHelper" { + class _MotionPathHelper extends MotionPathHelper {} + export { + _MotionPathHelper as MotionPathHelper, + _MotionPathHelper as default + } +} + +declare module "gsap/src/MotionPathHelper" { + export * from "gsap/MotionPathHelper"; + export { MotionPathHelper as default } from "gsap/MotionPathHelper"; +} + +declare module "gsap/dist/MotionPathHelper" { + export * from "gsap/MotionPathHelper"; + export { MotionPathHelper as default } from "gsap/MotionPathHelper"; +} + +declare module "gsap/all" { + export * from "gsap/MotionPathHelper"; +} + +declare module "gsap-trial/MotionPathHelper" { + export * from "gsap/MotionPathHelper"; + export { MotionPathHelper as default } from "gsap/MotionPathHelper"; +} + +declare module "gsap-trial/src/MotionPathHelper" { + export * from "gsap/MotionPathHelper"; + export { MotionPathHelper as default } from "gsap/MotionPathHelper"; +} + +declare module "gsap-trial/dist/MotionPathHelper" { + export * from "gsap/MotionPathHelper"; + export { MotionPathHelper as default } from "gsap/MotionPathHelper"; +} + +declare module "gsap-trial/all" { + export * from "gsap/MotionPathHelper"; +} diff --git a/node_modules/gsap/types/motion-path-plugin.d.ts b/node_modules/gsap/types/motion-path-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..8b32dbc6e57f27d63ce33475662d14cb87552c48 --- /dev/null +++ b/node_modules/gsap/types/motion-path-plugin.d.ts @@ -0,0 +1,314 @@ +declare namespace gsap { + + interface PathObject { + [propName: string]: number | string; + } + + interface TweenVars { + motionPath?: SVGPathValue | TweenValue | Point2D[]| PathObject[] | MotionPath.Vars; + } +} + +declare namespace gsap.plugins { + + interface ArrayToRawPathObject { + curviness?: number; + relative?: boolean; + type?: string; + x?: string; + y?: string; + } + + interface getRelativePositionObject extends gsap.Point2D { + angle: number; + } + + interface MotionPathPlugin extends Plugin { + + /** + * Takes an array of coordinates and plots a curve through them. + * + * ```js + * MotionPathPlugin.arrayToRawPath(anchors, {curviness:0.5}) + * ``` + * + * @param {Point2D[]} values + * @param {ArrayToRawPathObject} vars + * @returns {RawPath} The converted rawPath + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.arrayToRawPath() + */ + arrayToRawPath(values: Point2D[], vars?: ArrayToRawPathObject): RawPath; + + /** + * Measures the path and caches the values on the RawPath itself for fast and accurate subsequent processing. + * + * ```js + * MotionPathPlugin.cacheRawPathMeasurements(rawPath); + * ``` + * + * @param {RawPath} rawPath + * @param {number} resolution + * @returns {RawPath} the RawPath that was passed in + * @memberof MotionPathPlugin + */ + cacheRawPathMeasurements(rawPath: RawPath, resolution?: number): RawPath; + + /** + * Gets the matrix to convert points from one element's local coordinates into a + * different element's local coordinate system. + * + * ```js + * MotionPathPlugin.convertCoordinates(fromElement, toElement); + * ``` + * + * @param {Element} fromElement + * @param {Element} toElement + * @returns {Matrix2D} A matrix to convert from one element's coordinate system to another's + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.convertCoordinates() + */ + convertCoordinates(fromElement: Element, toElement: Element): Matrix2D; + /** + * Converts a point from one element's local coordinates into a + * different element's local coordinate system. + * + * ```js + * MotionPathPlugin.convertCoordinates(fromElement, toElement, point); + * ``` + * + * @param {Element} fromElement + * @param {Element} toElement + * @param {Point2D} point + * @returns {Point2D} the converted point + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.convertCoordinates() + */ + convertCoordinates(fromElement: Element, toElement: Element, point: Point2D): Point2D; + + /** + * Converts SVG shapes into <path>s. + * + * ```js + * MotionPathPlugin.convertToPath("circle"); + * ``` + * + * @param {DOMTarget} shape + * @param {boolean} [swap] + * @returns {SVGPathElement[]} The converted paths + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.convertToPath() + */ + convertToPath(shape: SVGPathTarget, swap?: boolean): SVGPathElement[]; + + /** + * Gets the matrix to convert points from one element's local coordinates into a + * different element's local coordinate system. + * + * ```js + * MotionPathPlugin.getAlignMatrix(fromElement, toElement); + * ``` + * + * @param {Element} fromElement + * @param {Element} toElement + * @param {number[] | Point2D} [fromOrigin] + * @param {number[] | Point2D | "auto"} [toOrigin] + * @returns {Matrix2D} A matrix to convert from one element's coordinate system to another's + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.getAlignMatrix() + */ + getAlignMatrix(fromElement: Element, toElement: Element, fromOrigin?: number[] | Point2D, toOrigin?: number[] | Point2D | "auto"): Matrix2D; + + /** + * Gets the Matrix2D that would be used to convert the element's local coordinate + * space into the global coordinate space. + * + * ```js + * MotionPathPlugin.getGlobalMatrix(element); + * ``` + * + * @param {Element} element + * @param {Boolean} [inverse] + * @param {Boolean} [adjustGOffset] + * @returns {Matrix2D} A matrix to convert from one element's coordinate system to another's + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.getGlobalMatrix() + */ + getGlobalMatrix(element: Element, inverse?: boolean, adjustGOffset?: boolean): Matrix2D; + + /** + * Calculates the x/y position (and optionally the angle) corresponding to a + * particular progress value along the RawPath. + * + * ```js + * MotionPathPlugin.getPositionOnPath(rawPath, 0.5); + * ``` + * + * @param {RawPath} rawPath + * @param {Number} progress + * @param {Boolean} [includeAngle] + * @returns {Matrix2D} A matrix to convert from one element's coordinate system to another's + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.getPositionOnPath() + */ + getPositionOnPath(rawPath: RawPath, progress: number, includeAngle?: boolean): Point2D | getRelativePositionObject; + + /** + * Gets the RawPath for the provided element or raw SVG <path> data. + * + * ```js + * MotionPathPlugin.getRawPath(element); + * ``` + * + * @param {DOMTarget} value + * @returns {RawPath} The rawPath + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.getRawPath() + */ + getRawPath(value: SVGPathValue): RawPath; + + /** + * Gets the x and y distances between two elements regardless of nested transforms. + * + * ```js + * MotionPathPlugin.getRelativePosition(dot, inner, [0.5, 0.5], [0.5, 0.5]); + * ``` + * + * @param {Element} fromElement + * @param {Element} toElement + * @param {number[] | Point2D[]} [fromOrigin] + * @param {number[] | Point2D[] | "auto"} [toOrigin] + * @returns {Point2D} The x and y between the references given + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.getRelativePosition() + */ + getRelativePosition(fromElement: Element, toElement: Element, fromOrigin?: number[] | Point2D, toOrigin?: number[] | Point2D | "auto"): Point2D; + + /** + * Gets the x and y distances between two elements regardless of nested transforms. + * + * ```js + * MotionPathPlugin.pointsToSegment([0,0, 10,10, ...], 0.5); + * ``` + * + * @param {number[]} points + * @param {number} [curviness] + * @returns {number[]} Cubic Bezier data in alternating x, y, x, y format + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.pointsToSegment() + */ + pointsToSegment(points: number[], curviness?: number): number[]; + + /** + * Converts a RawPath to a path string. + * + * ```js + * MotionPathPlugin.rawPathToString(rawPath); + * ``` + * + * @param {RawPath} rawPath + * @returns {string} The converted path + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.rawPathToString() + */ + rawPathToString(rawPath: RawPath): string; + + /** + * Slices a RawPath into a smaller RawPath. + * + * ```js + * MotionPathPlugin.sliceRawPath(rawPath, 0, 3); + * ``` + * + * @param {RawPath} rawPath + * @param {number} start + * @param {number} end + * @returns {RawPath} The sliced RawPath + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.sliceRawPath() + */ + sliceRawPath(rawPath: RawPath, start: number, end: number): RawPath; + + /** + * Converts a string of path data into a rawPath. + * + * ```js + * MotionPathPlugin.stringToRawPath("M0,0 C100,20 300,50 400,0..."); + * ``` + * + * @param {string} data + * @returns {RawPath} The converted RawPath + * @memberof MotionPathPlugin + * @link https://greensock.com/docs/v3/Plugins/MotionPathPlugin/static.stringToRawPath() + */ + stringToRawPath(data: string): RawPath; + } + + interface MotionPathPluginClass extends MotionPathPlugin { + new(): PluginScope & MotionPathPlugin; + prototype: PluginScope & MotionPathPlugin; + } + + const motionPath: MotionPathPluginClass; +} + +declare namespace MotionPath { + + type NumFunc = (i: number, target: object | Element) => number; + interface Vars { + align?: string | Element; + alignOrigin?: number[]; + autoRotate?: boolean | number; + curviness?: number; + end?: number | NumFunc; + offsetX?: number; + offsetY?: number; + path?: gsap.SVGPathValue | gsap.TweenValue | gsap.Point2D[] | gsap.PathObject[]; + relative?: boolean; + resolution?: number; + start?: number | NumFunc; + type?: string; + useRadians?: boolean; + fromCurrent?: boolean; + } +} + +declare const MotionPathPlugin: gsap.plugins.MotionPathPlugin; + +declare module "gsap/MotionPathPlugin" { + export const MotionPathPlugin: gsap.plugins.MotionPathPlugin; + export { MotionPathPlugin as default }; +} + +declare module "gsap/dist/MotionPathPlugin" { + export * from "gsap/MotionPathPlugin"; + export { MotionPathPlugin as default } from "gsap/MotionPathPlugin"; +} + +declare module "gsap/src/MotionPathPlugin" { + export * from "gsap/MotionPathPlugin"; + export { MotionPathPlugin as default } from "gsap/MotionPathPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/MotionPathPlugin"; +} + +declare module "gsap-trial/MotionPathPlugin" { + export const MotionPathPlugin: gsap.plugins.MotionPathPlugin; + export { MotionPathPlugin as default }; +} + +declare module "gsap-trial/dist/MotionPathPlugin" { + export * from "gsap/MotionPathPlugin"; + export { MotionPathPlugin as default } from "gsap/MotionPathPlugin"; +} + +declare module "gsap-trial/src/MotionPathPlugin" { + export * from "gsap/MotionPathPlugin"; + export { MotionPathPlugin as default } from "gsap/MotionPathPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/MotionPathPlugin"; +} diff --git a/node_modules/gsap/types/observer.d.ts b/node_modules/gsap/types/observer.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..348bb5d31ea08dd9987c43699170d7d801455949 --- /dev/null +++ b/node_modules/gsap/types/observer.d.ts @@ -0,0 +1,231 @@ +declare class Observer { + static readonly isTouch: number; + static readonly eventTypes: string[]; + static readonly version: string; + + readonly deltaX: number; + readonly deltaY: number; + readonly event: Event; + readonly isDragging: boolean; + readonly isEnabled: boolean; + readonly isPressed: boolean; + readonly startX?: number; + readonly startY?: number; + readonly target: Element; + readonly vars: Observer.ObserverVars; + readonly velocityX: number; + readonly velocityY: number; + readonly x?: number; + readonly y?: number; + readonly axis?: string | null; + + /** + * Creates a new Observer + * + * ```js + * Observer.create({ + * target: "#id", + * onUp: () => console.log("up"), + * onDown: () => console.log("down"), + * onPress: () => console.log("press") + * }); + * ``` + * + * @static + * @param {Observer.ObserverVars} vars + * @returns {Observer} The Observer + * @memberof Observer + * @link https://greensock.com/docs/v3/Plugins/Observer/static.create() + */ + static create(vars: Observer.ObserverVars): Observer; + + /** + * Gets all Observers (that haven't been killed) + * + * ```js + * Observer.getAll().forEach(o => o.kill()); + * ``` + * + * @static + * @returns {Observer[]} An Array of Observers + * @memberof Observer + * @link https://greensock.com/docs/v3/Plugins/Observer/static.getAll() + */ + static getAll(): Observer[]; + + /** + * Gets the observer with the id provided. + * + * ```js + * let o = Observer.getById("my-id"); + * ``` + * + * @static + * @param {string} id + * @returns {Observer | undefined} The Observer with the supplied id (if one exists) + * @memberof Observer + * @link https://greensock.com/docs/v3/Plugins/Observer/static.getAll() + */ + static getById(id: string): Observer | undefined; + + /** + * Disables a Observer instance. + * + * ```js + * observer.disable(); + * ``` + */ + disable(): void; + + /** + * Re-enables a disabled Observer instance. + * + * ```js + * observer.enable(); + * ``` + */ + enable(): this; + + /** + * Kills a Observer instance (same as disabling, but typically permanent). + * + * ```js + * observer.kill(); + * ``` + */ + kill(): void; + + /** + * Gets the horizontal scroll position of the target (typically scrollLeft). + * + * ```js + * observer.scrollX(); + * ``` + * + * @returns {number} The horizontal scroll position of the target + */ + scrollX(): number; + + /** + * Sets the horizontal scroll position of the target (typically scrollTop). + * + * ```js + * observer.scrollX(100); + * ``` + * + * @param {number} position + */ + scrollX(position: number): void; + + /** + * Gets the vertical scroll position of the target (typically scrollTop). + * + * ```js + * observer.scrollY(); + * ``` + * + * @returns {number} The vertical scroll position of the target + */ + scrollY(): number; + + /** + * Sets the vertical scroll position of the target (typically scrollTop). + * + * ```js + * observer.scrollY(100); + * ``` + * + * @param {number} position + */ + scrollY(position: number): void; + +} + +declare namespace Observer { + + type ObserverCallback = (self: Observer) => any; + type IgnoreCheckCallback = (event: Event, isTouchOrPointer: boolean) => boolean; + + interface ObserverVars { + allowClicks?: boolean; + capture?: boolean; + debounce?: boolean; + dragMinimum?: number; + event?: Event; + id?: string; + ignore?: gsap.DOMTarget; + ignoreCheck?: IgnoreCheckCallback; + lineHeight?: number; + lockAxis?: boolean; + onLockAxis?: ObserverCallback; + onDown?: ObserverCallback; + onUp?: ObserverCallback; + onLeft?: ObserverCallback; + onRight?: ObserverCallback; + onDisable?: ObserverCallback; + onDrag?: ObserverCallback; + onDragStart?: ObserverCallback; + onDragEnd?: ObserverCallback; + onEnable?: ObserverCallback; + onHover?: ObserverCallback; + onHoverEnd?: ObserverCallback; + onToggleY?: ObserverCallback; + onToggleX?: ObserverCallback; + onChangeX?: ObserverCallback; + onChangeY?: ObserverCallback; + onChange?: ObserverCallback; + onClick?: ObserverCallback; + onPress?: ObserverCallback; + onRelease?: ObserverCallback; + onMove?: ObserverCallback; + onWheel?: ObserverCallback; + onStop?: ObserverCallback; + onStopDelay?: number; + preventDefault?: boolean; + target?: gsap.DOMTarget | Window | Document; + tolerance?: number; + type?: string; + wheelSpeed?: number; + } +} + +declare module "gsap/Observer" { + class _Observer extends Observer { } + export { + _Observer as Observer, + _Observer as default + } +} + +declare module "gsap/dist/Observer" { + export * from "gsap/Observer"; + export { Observer as default } from "gsap/Observer"; +} + +declare module "gsap/src/Observer" { + export * from "gsap/Observer"; + export { Observer as default } from "gsap/Observer"; +} + +declare module "gsap/all" { + export * from "gsap/Observer"; +} + +declare module "gsap-trial/Observer" { + export * from "gsap/Observer"; + export { Observer as default } from "gsap/Observer"; +} + +declare module "gsap-trial/dist/Observer" { + export * from "gsap/Observer"; + export { Observer as default } from "gsap/Observer"; +} + +declare module "gsap-trial/src/Observer" { + export * from "gsap/Observer"; + export { Observer as default } from "gsap/Observer"; +} + +declare module "gsap-trial/all" { + export * from "gsap/Observer"; +} diff --git a/node_modules/gsap/types/physics-2d-plugin.d.ts b/node_modules/gsap/types/physics-2d-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..b90af8cf175d4f3e674521b456e0dc535743e2de --- /dev/null +++ b/node_modules/gsap/types/physics-2d-plugin.d.ts @@ -0,0 +1,72 @@ +declare namespace Physics2DPlugin { + interface Vars { + acceleration?: gsap.TweenValue; + accelerationAngle?: gsap.TweenValue; + angle?: gsap.TweenValue; + friction?: gsap.TweenValue; + gravity?: gsap.TweenValue; + velocity?: gsap.TweenValue; + xProp?: string; + yProp?: string; + } +} + +declare namespace gsap { + + interface TweenVars { + physics2D?: Physics2DPlugin.Vars; + } +} + +declare namespace gsap.plugins { + interface Physics2DPlugin extends Plugin { + + } + + interface Physics2DPluginClass extends Physics2DPlugin { + new(): PluginScope & Physics2DPlugin; + prototype: PluginScope & Physics2DPlugin; + } + + const physics2D: Physics2DPluginClass; +} + +declare const Physics2DPlugin: gsap.plugins.Physics2DPlugin; + +declare module "gsap/Physics2DPlugin" { + export const Physics2DPlugin: gsap.plugins.Physics2DPlugin; + export { Physics2DPlugin as default }; +} + +declare module "gsap/src/Physics2DPlugin" { + export * from "gsap/Physics2DPlugin"; + export { Physics2DPlugin as default } from "gsap/Physics2DPlugin"; +} + +declare module "gsap/dist/Physics2DPlugin" { + export * from "gsap/Physics2DPlugin"; + export { Physics2DPlugin as default } from "gsap/Physics2DPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/Physics2DPlugin"; +} + +declare module "gsap-trial/Physics2DPlugin" { + export * from "gsap/Physics2DPlugin"; + export { Physics2DPlugin as default } from "gsap/Physics2DPlugin"; +} + +declare module "gsap-trial/src/Physics2DPlugin" { + export * from "gsap/Physics2DPlugin"; + export { Physics2DPlugin as default } from "gsap/Physics2DPlugin"; +} + +declare module "gsap-trial/dist/Physics2DPlugin" { + export * from "gsap/Physics2DPlugin"; + export { Physics2DPlugin as default } from "gsap/Physics2DPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/Physics2DPlugin"; +} diff --git a/node_modules/gsap/types/physics-props-plugin.d.ts b/node_modules/gsap/types/physics-props-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..98dbc6dbf7d8907c88a9bbd4e2bdda141c456f1d --- /dev/null +++ b/node_modules/gsap/types/physics-props-plugin.d.ts @@ -0,0 +1,71 @@ +declare namespace PhysicsPropsPlugin { + interface Vars { + [key: string]: Values; + } + + interface Values { + acceleration?: gsap.TweenValue; + friction?: gsap.TweenValue; + velocity?: gsap.TweenValue; + } +} + +declare namespace gsap { + + interface TweenVars { + physicsProps?: PhysicsPropsPlugin.Vars; + } +} + +declare namespace gsap.plugins { + interface PhysicsPropsPlugin extends Plugin { + + } + + interface PhysicsPropsPluginClass extends PhysicsPropsPlugin { + new(): PluginScope & PhysicsPropsPlugin; + prototype: PluginScope & PhysicsPropsPlugin; + } + + const physicsProps: PhysicsPropsPluginClass; +} + +declare const PhysicsPropsPlugin: gsap.plugins.PhysicsPropsPlugin; + +declare module "gsap/PhysicsPropsPlugin" { + export const PhysicsPropsPlugin: gsap.plugins.PhysicsPropsPlugin; + export { PhysicsPropsPlugin as default }; +} + +declare module "gsap/src/PhysicsPropsPlugin" { + export * from "gsap/PhysicsPropsPlugin"; + export { PhysicsPropsPlugin as default } from "gsap/PhysicsPropsPlugin"; +} + +declare module "gsap/dist/PhysicsPropsPlugin" { + export * from "gsap/PhysicsPropsPlugin"; + export { PhysicsPropsPlugin as default } from "gsap/PhysicsPropsPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/PhysicsPropsPlugin"; +} + +declare module "gsap-trial/PhysicsPropsPlugin" { + export * from "gsap/PhysicsPropsPlugin"; + export { PhysicsPropsPlugin as default } from "gsap/PhysicsPropsPlugin"; +} + +declare module "gsap-trial/src/PhysicsPropsPlugin" { + export * from "gsap/PhysicsPropsPlugin"; + export { PhysicsPropsPlugin as default } from "gsap/PhysicsPropsPlugin"; +} + +declare module "gsap-trial/dist/PhysicsPropsPlugin" { + export * from "gsap/PhysicsPropsPlugin"; + export { PhysicsPropsPlugin as default } from "gsap/PhysicsPropsPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/PhysicsPropsPlugin"; +} diff --git a/node_modules/gsap/types/pixi-plugin.d.ts b/node_modules/gsap/types/pixi-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..dcb6698c9678fca381e1a44d30451a2c9413b302 --- /dev/null +++ b/node_modules/gsap/types/pixi-plugin.d.ts @@ -0,0 +1,138 @@ +declare namespace PixiPlugin { + interface PixiMatrix { + a: number; + b: number; + c: number; + d: number; + tx: number; + ty: number; + array?: number[]; + } + + interface Vars { + [key: string]: any; + alpha?: number | string; + anchor?: number; + anchorX?: number | string; + anchorY?: number | string; + angle?: number | string; + autoAlpha?: number; + blur?: number; + blurX?: number; + blurY?: number; + blurPadding?: number; + brightness?: number; + colorize?: string | number; + colorizeAmount?: number; + colorMatrixFilter?: object; // TODO + combineCMF?: boolean; + contrast?: number; + fillColor?: string | number; + height?: number | string; + hue?: number; + lineColor?: string | number; + matrix?: PixiMatrix; + pivot?: number; + pivotX?: number | string; + pivotY?: number | string; + position?: number | string; + positionX?: number | string; + positionY?: number | string; + resolution?: number; + rotation?: number | string; + saturation?: number; + scale?: number | string; + scaleX?: number | string; + scaleY?: number | string; + skew?: number | string; + skewX?: number | string; + skewY?: number | string; + tilePosition?: number; + tilePositionX?: number | string; + tilePositionY?: number | string; + tileScale?: number; + tileScaleX?: number | string; + tileScaleY?: number | string; + tileX?: number | string; + tileY?: number | string; + tint?: string | number; + width?: number | string; + x?: number | string; + y?: number | string; + zIndex?: number | string; + } +} + +declare namespace gsap { + + interface TweenVars { + pixi?: PixiPlugin.Vars; + } +} + +declare namespace gsap.plugins { + + interface PixiPlugin extends Plugin { + + /** + * Registers the main PIXI library object with the PixiPlugin so that it can find the + * necessary classes/objects. You only need to register it once. + * + * ```js + * PixiPlugin.registerPIXI(PIXI); + * ``` + * + * @param {object} pixi + * @memberof PixiPlugin + * @link https://greensock.com/docs/v3/Plugins/PixiPlugin/static.registerPIXI() + */ + registerPIXI(pixi: object): void; + } + + interface PixiPluginClass extends PixiPlugin { + new(): PluginScope & PixiPlugin; + prototype: PluginScope & PixiPlugin; + } + + const pixi: PixiPluginClass; +} + +declare const PixiPlugin: gsap.plugins.PixiPlugin; + +declare module "gsap/PixiPlugin" { + export const PixiPlugin: gsap.plugins.PixiPlugin; + export { PixiPlugin as default }; +} + +declare module "gsap/src/PixiPlugin" { + export * from "gsap/PixiPlugin"; + export { PixiPlugin as default } from "gsap/PixiPlugin"; +} + +declare module "gsap/dist/PixiPlugin" { + export * from "gsap/PixiPlugin"; + export { PixiPlugin as default } from "gsap/PixiPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/PixiPlugin"; +} + +declare module "gsap-trial/PixiPlugin" { + export * from "gsap/PixiPlugin"; + export { PixiPlugin as default } from "gsap/PixiPlugin"; +} + +declare module "gsap-trial/src/PixiPlugin" { + export * from "gsap/PixiPlugin"; + export { PixiPlugin as default } from "gsap/PixiPlugin"; +} + +declare module "gsap-trial/dist/PixiPlugin" { + export * from "gsap/PixiPlugin"; + export { PixiPlugin as default } from "gsap/PixiPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/PixiPlugin"; +} diff --git a/node_modules/gsap/types/scramble-text-plugin.d.ts b/node_modules/gsap/types/scramble-text-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..5451377772653c3c01981b00ba1bd30b9c7ac90f --- /dev/null +++ b/node_modules/gsap/types/scramble-text-plugin.d.ts @@ -0,0 +1,73 @@ +declare namespace ScrambleTextPlugin { + interface Vars { + text: string; + chars?: string; + speed?: number; + delimiter?: string; + tweenLength?: boolean; + newClass?: string; + oldClass?: string; + revealDelay?: number; + rightToLeft?: boolean; + } +} + +declare namespace gsap { + + interface TweenVars { + scrambleText?: string | ScrambleTextPlugin.Vars; + } +} + +declare namespace gsap.plugins { + interface ScrambleTextPlugin extends Plugin { + + } + + interface ScrambleTextPluginClass extends ScrambleTextPlugin { + new(): PluginScope & ScrambleTextPlugin; + prototype: PluginScope & ScrambleTextPlugin; + } + + const scrambleText: ScrambleTextPluginClass; +} + +declare const ScrambleTextPlugin: gsap.plugins.ScrambleTextPlugin; + +declare module "gsap/ScrambleTextPlugin" { + export const ScrambleTextPlugin: gsap.plugins.ScrambleTextPlugin; + export { ScrambleTextPlugin as default }; +} + +declare module "gsap/src/ScrambleTextPlugin" { + export * from "gsap/ScrambleTextPlugin"; + export { ScrambleTextPlugin as default } from "gsap/ScrambleTextPlugin"; +} + +declare module "gsap/dist/ScrambleTextPlugin" { + export * from "gsap/ScrambleTextPlugin"; + export { ScrambleTextPlugin as default } from "gsap/ScrambleTextPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/ScrambleTextPlugin"; +} + +declare module "gsap-trial/ScrambleTextPlugin" { + export * from "gsap/ScrambleTextPlugin"; + export { ScrambleTextPlugin as default } from "gsap/ScrambleTextPlugin"; +} + +declare module "gsap-trial/src/ScrambleTextPlugin" { + export * from "gsap/ScrambleTextPlugin"; + export { ScrambleTextPlugin as default } from "gsap/ScrambleTextPlugin"; +} + +declare module "gsap-trial/dist/ScrambleTextPlugin" { + export * from "gsap/ScrambleTextPlugin"; + export { ScrambleTextPlugin as default } from "gsap/ScrambleTextPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/ScrambleTextPlugin"; +} diff --git a/node_modules/gsap/types/scroll-smoother.d.ts b/node_modules/gsap/types/scroll-smoother.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..b258806d43d1bb595357c4685d09c4f4b0a3377e --- /dev/null +++ b/node_modules/gsap/types/scroll-smoother.d.ts @@ -0,0 +1,408 @@ +declare class ScrollSmoother { + + static readonly version: string; + + readonly scrollTrigger: ScrollTrigger; + readonly progress: number; + readonly normalizer?: Observer; + + /** + * Creates an instance of ScrollSmoother. + * @param {ScrollSmoother.Vars} vars + * @memberof ScrollSmoother + */ + constructor(vars: ScrollSmoother.Vars); + + /** + * Create a ScrollSmoother instance to smooth the scrolling of the page (only one can exist at any time) + * + * ```js + * ScrollSmoother.create({ + * content: "#smooth-content", + * wrapper: "#smooth-wrapper", + * smooth: 1.5, + * effects: true + * }); + * ``` + * + * @static + * @param {ScrollSmoother.Vars} vars + * @returns {ScrollSmoother} The ScrollSmoother + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/static.create() + */ + static create(vars: ScrollSmoother.Vars): ScrollSmoother; + + /** + * Returns the ScrollSmoother instance (if one has been created). Only one is allowed at any given time. + * + * ```js + * let smoother = ScrollSmoother.get(); + * ``` + * + * @static + * @returns {ScrollSmoother} The ScrollSmoother + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/static.get() + */ + static get(): ScrollSmoother | undefined; + + /** + * Refreshes all ScrollTriggers (same as ScrollTrigger.refresh()) + * + * ```js + * ScrollSmoother.refresh(); + * ``` + * + * @param {boolean} safe + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/static.refresh() + */ + static refresh(safe?: boolean): void; + + /** + * Sets the content element (the element that moves up and down when scrolling) + * + * ```js + * smoother.content("#content"); + * ``` + * + * @param {gsap.DOMTarget} element + * @returns {ScrollSmoother} The ScrollSmoother instance (to make chaining easier) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/content() + */ + content(element: gsap.DOMTarget): this; + + /** + * Gets the content element (the element that moves up and down when scrolling) + * + * ```js + * let el = smoother.content(); + * ``` + * + * @returns {HTMLElement} The content Element + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/content() + */ + content(): HTMLElement; + + /** + * Applies "speed" and/or "lag" effects to the supplied targets (instead of using HTML attributes like data-speed and data-lag) + * + * ```js + * scroller.effects(".box", { + * speed: (i, el) => 0.5 + i * 0.1, + * lag: 0.5 + * }); + * ``` + * + * @param {gsap.DOMTarget} targets + * @param {ScrollSmoother.EffectsVars} vars + * @returns {ScrollTrigger[]} An Array of ScrollTrigger instances that were created to handle the effects + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/effects() + */ + effects(targets: gsap.DOMTarget, vars?: ScrollSmoother.EffectsVars | null): ScrollTrigger[]; + + /** + * Gets the ScrollTrigger instances that are managing the effects (like "speed" and/or "lag") + * + * ```js + * let effectTriggers = scroller.effects(); + * ``` + * + * @returns {ScrollTrigger[]} An Array of ScrollTrigger instances that were created to handle the effects + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/effects() + */ + effects(): ScrollTrigger[]; + + /** + * Returns the velocity of the vertical scrolling in pixels per second + * + * ```js + * let velocity = smoother.getVelocity() + * ``` + * + * @returns {number} The velocity of the vertical scrolling (in pixels per second) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/getVelocity() + */ + getVelocity(): number; + + /** + * Kills the ScrollSmoother instance, reverting the inline CSS of the content and wrapper, removing listeners, etc. This is permanent but you can ScrollSmoother.create() a new one. + * + * ```js + * scrollSmoother.kill(); + * ``` + * + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/kill() + */ + kill(): void; + + /** + * Gets the numeric offset (scroll position) associated with a particular element. + * + * ```js + * let offset = smoother.offset("#id", "center center"); + * ``` + * + * @param {gsap.DOMTarget} target + * @param {string} position - like "top center" or "50% bottom-=50px" + * @param {boolean} ignoreSpeed + * @returns {number} The numeric offset (scroll position) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/offset() + */ + offset(target: gsap.DOMTarget, position?: string, ignoreSpeed?: boolean): number; + + /** + * Sets the paused state - if true, nothing will scroll (except via .scrollTop() or .scrollTo() on this instance). Serves as a getter and setter function + * + * ```js + * smoother.paused(true); + * ``` + * + * @param {boolean} value + * @returns {ScrollSmoother} The ScrollSmoother instance (for easier chaining) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/paused() + */ + paused(value: boolean): this; + + /** + * Gets the paused state. Serves as a getter and setter function. + * + * ```js + * if (!smoother.paused()) { + * ... + * } + * ``` + * + * @returns {boolean} The paused state (true or false) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/paused() + */ + paused(): boolean; + + /** + * Refreshes only the main page's smoothing ScrollTrigger + * + * ```js + * smoother.refresh(); + * ``` + * + * @param {boolean} soft + * @param {boolean} force + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/refresh() + */ + refresh(soft?: boolean, force?: boolean): void; + + /** + * Scrolls to a particular position or target immediately or in a smooth manner. + * + * ```js + * smoother.scrollTo("#id", true, "center center"); + * ``` + * + * @param {gsap.DOMTarget | number} target + * @param {boolean} smooth + * @param {string} position + * @returns {void} + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/scrollTo() + */ + scrollTo(target: gsap.DOMTarget | number, smooth?: boolean, position?: string): void; + + /** + * Immediately scrolls to a particular numeric scroll position + * + * ```js + * smoother.scrollTop(500); + * ``` + * + * @param {number} position + * @returns {ScrollSmoother} Returns the instance itself for easier chaining + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/scrollTop() + */ + scrollTop(position: number): this; + + /** + * Gets the scroll position (numeric offset) + * + * ```js + * let offset = smoother.scrollTop(); + * ``` + * + * @returns {number} the numeric offset + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/scrollTop() + */ + scrollTop(): number; + // + // /** + // * Sets up ScrollTriggers to handle hiding elements (sections) when they're sufficiently outside the viewport in order to improve performance in some situations. + // * + // * ```js + // * smoother.sections("[data-section]"); + // * ``` + // * + // * @param {gsap.DOMTarget} targets + // * @param {ScrollSmoother.SectionVars} vars + // * @returns {ScrollTrigger[]} An Array of ScrollTrigger instances that were created to handle the sections + // * @memberof ScrollSmoother + // * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/sections() + // */ + // sections(targets: gsap.DOMTarget, vars?: ScrollSmoother.SectionVars | null): ScrollTrigger[]; + // + // /** + // * Gets the ScrollTrigger instances that are managing the sections + // * + // * ```js + // * let sectionTriggers = smoother.sections(); + // * ``` + // * + // * @returns {ScrollTrigger[]} An Array of ScrollTrigger instances that were created to handle the sections + // * @memberof ScrollSmoother + // * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/sections() + // */ + // sections(): ScrollTrigger[]; + + /** + * Sets the number of seconds it takes to catch up to the scroll position (smoothing). + * + * ```js + * smoother.smooth(1.5); + * ``` + * + * @param {number} value + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/smooth() + */ + smooth(value: number): void; + + /** + * Gets the number of seconds it takes to catch up to the scroll position (smoothing). + * + * ```js + * let duration = smoother.smooth(); + * ``` + * + * @returns {number} The amount of smoothing applied (in seconds) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/smooth() + */ + smooth(): number; + + /** + * Sets the wrapper element which serves as the viewport (scrolls the content) + * + * ```js + * smoother.wrapper("#wrapper"); + * ``` + * + * @param {gsap.DOMTarget} element + * @returns {ScrollSmoother} The ScrollSmoother instance (to make chaining easier) + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/wrapper() + */ + wrapper(element: gsap.DOMTarget): this; + + /** + * Gets the wrapper element which serves as the viewport (scrolls the content) + * + * ```js + * let el = smoother.wrapper(); + * ``` + * + * @returns {HTMLElement} The wrapper Element + * @memberof ScrollSmoother + * @link https://greensock.com/docs/v3/Plugins/ScrollSmoother/wrapper() + */ + wrapper(): HTMLElement; + +} + +declare namespace ScrollSmoother { + + type Callback = (self: ScrollSmoother) => any; + type EventCallback = (self: ScrollSmoother, event: Event) => any; + type EffectFunc = (index: number, element: Element) => number | string; + + interface EffectsVars { + speed?: number | string | EffectFunc; + lag?: number | EffectFunc; + effectsPadding?: number | string | EffectFunc; + } + // + // interface SectionVars { + // add?: boolean; + // } + + interface Vars { + autoResize?: boolean; + content?: gsap.DOMTarget; + ease?: string | Function; + effects?: boolean | gsap.DOMTarget; + effectsPrefix?: string; + effectsPadding?: number; + ignoreMobileResize?: boolean; + normalizeScroll?: boolean | ScrollTrigger.NormalizeVars; + onFocusIn?: EventCallback; + onUpdate?: Callback; + onStop?: Callback; + // sections?: boolean | gsap.DOMTarget; + smooth?: boolean | number; + smoothTouch?: boolean | number; + speed?: number; + wrapper?: gsap.DOMTarget; + } + +} + + +declare module "gsap/ScrollSmoother" { + class _ScrollSmoother extends ScrollSmoother { } + export { + _ScrollSmoother as ScrollSmoother, + _ScrollSmoother as default + } +} + +declare module "gsap/dist/ScrollSmoother" { + export * from "gsap/ScrollSmoother"; + export { ScrollSmoother as default } from "gsap/ScrollSmoother"; +} + +declare module "gsap/src/ScrollSmoother" { + export * from "gsap/ScrollSmoother"; + export { ScrollSmoother as default } from "gsap/ScrollSmoother"; +} + +declare module "gsap/all" { + export * from "gsap/ScrollSmoother"; +} + +declare module "gsap-trial/ScrollSmoother" { + export * from "gsap/ScrollSmoother"; + export { ScrollSmoother as default } from "gsap/ScrollSmoother"; +} + +declare module "gsap-trial/dist/ScrollSmoother" { + export * from "gsap/ScrollSmoother"; + export { ScrollSmoother as default } from "gsap/ScrollSmoother"; +} + +declare module "gsap-trial/src/ScrollSmoother" { + export * from "gsap/ScrollSmoother"; + export { ScrollSmoother as default } from "gsap/ScrollSmoother"; +} + +declare module "gsap-trial/all" { + export * from "gsap/ScrollSmoother"; +} diff --git a/node_modules/gsap/types/scroll-to-plugin.d.ts b/node_modules/gsap/types/scroll-to-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..c2fecc10d1a71e5c01fe2c177f2671dd35808222 --- /dev/null +++ b/node_modules/gsap/types/scroll-to-plugin.d.ts @@ -0,0 +1,114 @@ +declare class ScrollToPlugin { + + /** + * Configure ScrollToPlugin + * + * ```js + * ScrollToPlugin.config({ + * autoKill: true + * }); + * ``` + * + * @static + * @param {ScrollToPlugin.ConfigVars} vars + * @memberof ScrollToPlugin + * @link https://greensock.com/docs/v3/Plugins/ScrollToPlugin/static.config() + */ + static config(vars: ScrollToPlugin.ConfigVars): void; + + + /** + * Returns the maximum scroll value for the given Element + * + * ```js + * ScrollToPlugin.max(window); + * ``` + * + * @static + * @param {gsap.DOMTarget} element + * @returns {number} maximum scroll value in pixels + * @memberof ScrollToPlugin + * @link https://greensock.com/docs/v3/Plugins/ScrollToPlugin/static.max() + */ + static max(element: gsap.DOMTarget): number; + + /** + * Returns the maximum scroll value for the given Element + * + * ```js + * ScrollToPlugin.offset("#target", window); + * ``` + * + * @static + * @param {gsap.DOMTarget} element + * @param {gsap.DOMTarget} container + * @returns {number} offset value + * @memberof ScrollToPlugin + * @link https://greensock.com/docs/v3/Plugins/ScrollToPlugin/static.offset() + */ + static offset(element: gsap.DOMTarget, container?: gsap.DOMTarget): number; +} + +declare namespace ScrollToPlugin { + interface Vars { + x?: number | string | Element; + y?: number | string | Element; + offsetX?: number; + offsetY?: number; + autoKill?: boolean; + onAutoKill?: Function; + } + + interface ConfigVars { + autoKill?: boolean; + autoKillThreshold?: number; + } +} + +declare namespace gsap { + + interface TweenVars { + scrollTo?: number | string | Element | Function | ScrollToPlugin.Vars; + } +} + +declare module "gsap/ScrollToPlugin" { + class _ScrollToPlugin extends ScrollToPlugin { } + export { + _ScrollToPlugin as ScrollToPlugin, + _ScrollToPlugin as default + } +} + +declare module "gsap/src/ScrollToPlugin" { + export * from "gsap/ScrollToPlugin"; + export { ScrollToPlugin as default } from "gsap/ScrollToPlugin"; +} + +declare module "gsap/dist/ScrollToPlugin" { + export * from "gsap/ScrollToPlugin"; + export { ScrollToPlugin as default } from "gsap/ScrollToPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/ScrollToPlugin"; +} + +declare module "gsap-trial/ScrollToPlugin" { + export * from "gsap/ScrollToPlugin"; + export { ScrollToPlugin as default } from "gsap/ScrollToPlugin"; +} + +declare module "gsap-trial/src/ScrollToPlugin" { + export * from "gsap/ScrollToPlugin"; + export { ScrollToPlugin as default } from "gsap/ScrollToPlugin"; +} + +declare module "gsap-trial/dist/ScrollToPlugin" { + export * from "gsap/ScrollToPlugin"; + export { ScrollToPlugin as default } from "gsap/ScrollToPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/ScrollToPlugin"; +} diff --git a/node_modules/gsap/types/scroll-trigger.d.ts b/node_modules/gsap/types/scroll-trigger.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..105b862199c031b8477e6b47da0f6dd1a7d06b0a --- /dev/null +++ b/node_modules/gsap/types/scroll-trigger.d.ts @@ -0,0 +1,923 @@ +declare namespace gsap { + + interface AnimationVars { + scrollTrigger?: gsap.DOMTarget | ScrollTrigger.Vars; + } +} + +declare class ScrollTrigger { + + static readonly version: string; + static readonly isTouch: number; + + readonly animation?: gsap.core.Animation | undefined; + readonly callbackAnimation?: gsap.core.Animation | undefined; + readonly direction: number; + readonly end: number; + readonly isActive: boolean; + readonly pin?: Element; + readonly progress: number; + readonly scroller: Element | Window; + readonly start: number; + readonly trigger?: Element; + readonly vars: ScrollTrigger.Vars; + + /** + * Creates an instance of ScrollTrigger. + * @param {ScrollTrigger.StaticVars} vars + * @param {gsap.core.Animation} [animation] + * @memberof ScrollTrigger + */ + constructor(vars: ScrollTrigger.StaticVars, animation?: gsap.core.Animation); + + /** + * Attach a new event listener to a ScrollTrigger event. + * + * ```js + * ScrollTrigger.addEventListener("scrollStart", myFunc); + * ``` + * + * @static + * @param {"scrollStart" | "scrollEnd" | "refreshInit" | "refresh" | "revert"} event + * @param {gsap.Callback} callback + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.addEventListener() + */ + static addEventListener(event: "scrollStart" | "scrollEnd" | "refreshInit" | "refresh" | "matchMedia" | "revert", callback: gsap.Callback): void; + + /** + * Creates a coordinated group of ScrollTriggers (one for each target element) that batch their callbacks within a certain interval + * + * ```js + * ScrollTrigger.batch(".class", { + * interval: 0.1, + * batchMax: 3, + * onEnter: (elements, triggers) => gsap.to(elements, {opacity: 1, stagger: 0.15, overwrite: true}), + * onLeave: (elements, triggers) => gsap.set(elements, {opacity: 0, overwrite: true}), + * onEnterBack: (elements, triggers) => gsap.to(elements, {opacity: 1, stagger: 0.15, overwrite: true}), + * onLeaveBack: (elements, triggers) => gsap.set(elements, {opacity: 0, overwrite: true}) + * }); + * ``` + * + * @static + * @param {gsap.DOMTarget} targets + * @param {ScrollTrigger.BatchVars} vars + * @returns {ScrollTriggerInstance[]} An Array of the resulting ScrollTrigger instances + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.batch() + */ + static batch(targets: gsap.DOMTarget, vars: ScrollTrigger.BatchVars): ScrollTrigger[]; + + /** + * Un-registers .matchMedia() break points (or just one). + * + * ```js + * ScrollTrigger.clearMatchMedia(); + * ``` + * + * @static + * @param {string} [name] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.clearMatchMedia() + */ + static clearMatchMedia(name?: string): void; + + /** + * Clears any recorded scroll position data. + * + * ```js + * ScrollTrigger.clearScrollMemory(); + * ``` + * + * @static + * @param {string} [scrollRestoration] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.clearScrollMemory() + */ + static clearScrollMemory(scrollRestoration?: string): void; + + /** + * Configure ScrollTrigger + * + * ```js + * ScrollTrigger.config({ + * limitCallbacks: true, + * autoRefreshEvents: "resize,load,visibilitychange,DOMContentLoaded" + * }); + * ``` + * + * @static + * @param {ScrollTrigger.ConfigVars} vars + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.config() + */ + static config(vars: ScrollTrigger.ConfigVars): void; + + /** + * Create scroll triggers that aren't directly connected to a tween or timeline. + * + * ```js + * ScrollTrigger.create({ + * trigger: "#id", + * start: "top top", + * end: "bottom 50%+=100px" + * }); + * ``` + * + * @static + * @param {ScrollTrigger.StaticVars} vars + * @returns {ScrollTrigger} The ScrollTrigger + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.create() + */ + static create(vars: ScrollTrigger.StaticVars): ScrollTrigger; + + /** + * Set the default values that apply to every ScrollTrigger upon creation. + * + * ```js + * ScrollTrigger.defaults({ + * toggleActions: "restart pause resume none", + * markers: {startColor: "white", endColor: "white", fontSize: "18px", indent: 10} + * }); + * ``` + * + * @static + * @param {ScrollTrigger.StaticVars} vars + * @returns {ScrollTrigger.StaticVars} The config object + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.defaults() + */ + static defaults(vars: ScrollTrigger.StaticVars): ScrollTrigger.StaticVars; + + /** + * Returns all ScrollTriggers that exist. + * + * ```js + * ScrollTrigger.getAll("myID"); + * ``` + * + * @static + * @returns {ScrollTrigger[]} The ScrollTrigger + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.getAll() + */ + static getAll(): ScrollTrigger[]; + + /** + * Disables ALL ScrollTrigger functionality. + * + * ```js + * ScrollTrigger.disable(); + * ``` + * @static + * @param {boolean} reset + * @param {boolean} kill + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.disable() + */ + static disable(reset?: boolean, kill?: boolean): void; + + + /** + * Enables all ScrollTrigger functionality again after ScrollTrigger.disable() was called. + * + * ```js + * ScrollTrigger.enable(); + * ``` + * @static + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.enable() + */ + static enable(): void; + + /** + * Returns the ScrollTrigger that was assigned the corresponding id. + * + * ```js + * ScrollTrigger.getById("myID"); + * ``` + * + * @static + * @param {string} id + * @returns {ScrollTriggerInstance} The ScrollTrigger + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.getById() + */ + static getById(id: string): ScrollTrigger | undefined; + + /** + * Returns a function to control the scroll position of a particular element + * + * ```js + * let setScroll = ScrollTrigger.getScrollFunc(window); + * setScroll(250); + * ``` + * + * @static + * @param {(gsap.DOMTarget | Window)} element + * @param {boolean} [horizontal] + * @returns {ScrollTrigger.ScrollFunc} + * @memberof ScrollTrigger + */ + static getScrollFunc(element: gsap.DOMTarget | Window, horizontal?: boolean): ScrollTrigger.ScrollFunc; + + /** + * Checks if the element is in the viewport. + * + * ```js + * if (ScrollTrigger.isInViewport(element, 0.2)) {...}; + * ``` + * + * @static + * @param {Element | string} element + * @param {number} [ratio] + * @param {boolean} [horizontal] + * @returns {boolean} Boolean + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.isInViewport() + */ + static isInViewport(element: Element | string, ratio?: number, horizontal?: boolean): boolean; + + /** + * Find out if a ScrollTrigger-related scroller is currently scrolling. + * + * ```js + * ScrollTrigger.isScrolling(); + * ``` + * + * @static + * @returns {boolean} Whether or not any scroller is scrolling + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.isScrolling() + */ + static isScrolling(): boolean; + + /** + * Kills all ScrollTriggers (except the main ScrollSmoother one, if it exists) + * + * ```js + * ScrollTrigger.killAll(); + * ``` + * + * @static + * @param {boolean} [allowListeners] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.killAll() + */ + static killAll(allowListeners?: boolean): void; + + /** + * Set up ScrollTriggers that only apply to certain viewport sizes using media queries. Deprecated in favor of gsap.matchMedia() in version 3.11.0+ + * + * ```js + * ScrollTrigger.matchMedia({ + * "(min-width: 800px)": function() { }, + * "(max-width: 799px)": function() { }, + * "all": function() { } + * }); + * ``` + * @deprecated + * @static + * @param {ScrollTrigger.MatchMediaObject} vars + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.matchMedia() + */ + static matchMedia(vars: ScrollTrigger.MatchMediaObject): void; + + /** + * Get the maximum scroll value for any given element. + * + * ```js + * ScrollTrigger.maxScroll(window); + * ``` + * + * @static + * @param {(HTMLElement | Window)} target + * @param {boolean} [horizontal] + * @returns {number} The max distance the element can scroll + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.maxScroll() + */ + static maxScroll(target: HTMLElement | Window, horizontal?: boolean): number; + + /** + * Forces scrolling to be done on the JavaScript thread, ensuring it is synchronized and the address bar doesn't show/hide on [most] mobile devices. + * + * ```js + * ScrollTrigger.normalizeScroll(true); + * ``` + * @static + * @param {boolean | ScrollTrigger.NormalizeVars | Observer} enable + * @returns {Observer | undefined} a new Observer instance (if true) or undefined (if false) + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.normalizeScroll() + */ + static normalizeScroll(enable?: boolean | ScrollTrigger.NormalizeVars | Observer): Observer | undefined; + + /** + * Returns the Observer instance that is currently normalizing scroll behavior (if one exists). + * + * ```js + * let normalizer = ScrollTrigger.normalizeScroll(); + * ``` + * @static + * @returns {Observer | undefined} the Observer instance normalizing scroll (if one exists) or undefined (if false) + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.normalizeScroll() + */ + static normalizeScroll(): Observer | undefined; + + /** + * Creates an Observer that senses "scroll-like" behavior like a mouse wheel spin, finger swipe on a touch device, scrollbar drag or even a press/drag of the pointer. + * + * ```js + * ScrollTrigger.observe({ + * target: ".box", + * onUp: self => console.log("up", self.deltaY), + * onDown: self => console.log("down", self.deltaY) + * }); + * ``` + * @static + * @param {Observer.ObserverVars} vars + * @returns {Observer} a new Observer instance + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.observe() + */ + static observe(vars:Observer.ObserverVars): Observer; + + /** + * Returns the position of the Element in the viewport as a normalized value (0-1) where 0 is top/left and 1 is bottom/right. + * + * ```js + * if (ScrollTrigger.positionInViewport(element, "top")) {...}; + * ``` + * + * @static + * @param {Element | string} element + * @param {number} [referencePoint] - a number in pixels from top, percent like "20%" from top or keyword like "top"/"center"/"bottom" + * @param {boolean} [horizontal] + * @returns {number} normalized value (0-1) where 0 is top/left and 1 is bottom/right + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.positionInViewport() + */ + static positionInViewport(element: Element | string, referencePoint?: string | number, horizontal?: boolean): number; + + /** + * Recalculates the positioning of all of the ScrollTriggers on the page. + * + * ```js + * ScrollTrigger.refresh(); + * ``` + * + * @static + * @param {boolean} [safe] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.refresh() + */ + static refresh(safe?: boolean): void; + + /** + * Registers ScrollTrigger with gsap + * + * @static + * @param {typeof gsap} core + * @memberof ScrollTrigger + */ + static register(core: typeof gsap): void; + + /** + * Removes an event listener for a ScrollTrigger event. + * + * ```js + * ScrollTrigger.removeEventListener("scrollStart", myFunc); + * ``` + * + * @static + * @param {"scrollStart" | "scrollEnd" | "refreshInit" | "refresh" | "matchMedia"} event + * @param {gsap.Callback} callback + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.removeEventListener() + */ + static removeEventListener(event: "scrollStart" | "scrollEnd" | "refreshInit" | "refresh" | "matchMedia", callback: gsap.Callback): void; + + /** + * Records the current inline CSS styles for the given element(s) so they can be reverted later. + * + * ```js + * ScrollTrigger.saveStyles(".panel, #logo"); + * ``` + * + * @static + * @param {gsap.DOMTarget} targets + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.targets() + */ + static saveStyles(targets: gsap.DOMTarget): void; + + /** + * Sets up proxy methods for a particular scroller so that you can do advanced effects like integrate with a 3rd party smooth scrolling library. + * + * ```js + * ScrollTrigger.scrollerProxy(".container", { + * scrollTop(value) { + * return arguments.length ? locoScroll.scrollTo(value, 0, 0) : locoScroll.scroll.instance.scroll.y; + * }, + * getBoundingClientRect() { + * return {top: 0, left: 0, width: window.innerWidth, height: window.innerHeight}; + * }, + * pinType: document.querySelector(".container").style.transform ? "transform" : "fixed" + * }); + * ``` + * + * @static + * @param {gsap.DOMTarget} scroller + * @param {ScrollTrigger.ScrollerProxyVars} vars + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.scrollerProxy() + */ + static scrollerProxy(scroller: gsap.DOMTarget, vars?: ScrollTrigger.ScrollerProxyVars): void; + + /** + * Returns a function that will snap in a given direction where 1 is positive and -1 is negative. It will accept an increment or Array of numbers + * + * ```js + * let snap = ScrollTrigger.snapDirectional(5); + * snap(2, 1); // 5 + * snap(8, -1); // 5 + * snap(51, 1) // 55 + * ``` + * + * @static + * @param {number | number[]} incrementOrArray + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.snapDirectional() + */ + static snapDirectional(incrementOrArray: number | number[]): ScrollTrigger.SnapDirectionalFunc; + + /** + * Sorts the internal Array of ScrollTriggers by "refreshPriority" first, then by their "start" positions (or by a custom function you provide). + * + * ```js + * ScrollTrigger.sort(); + * ``` + * + * @static + * @param {Function} [func] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.sort() + */ + static sort(func?: Function): ScrollTrigger[]; + + /** + * Checks where the scrollbar is and updates all ScrollTrigger instances' progress and direction values accordingly, controls the animation (if necessary) and fires the appropriate callbacks. + * + * ```js + * ScrollTrigger.update(); + * ``` + * + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/static.update() + */ + static update(): void; + + /** + * Stops all of the ScrollTrigger's callbacks and removes any added markup and padding caused by pinning. + * + * ```js + * scrollTrigger.disable(); + * scrollTrigger.disable(true); + * ``` + * + * @param {boolean} [revert] + * @param {boolean} [allowAnimation] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/disable() + */ + disable(revert?: boolean, allowAnimation?: boolean): void; + + /** + * Re-enables a disabled ScrollTrigger instance. + * + * ```js + * scrollTrigger.enable(); + * ``` + * @param {boolean} [reset] + * @param {boolean} [refresh] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/enable() + */ + enable(reset?: boolean, refresh?: boolean): void; + + /** + * Forces any associated animation (including the callbackAnimation) to its natural end state immediately which is progress(1) if + * direction is 1 (forward) and progress(0) if direction is -1 (backward). + * + * ```js + * preventOverlaps: self => self.getTrailing().forEach(t => t.endAnimation()); + * ``` + * + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/endAnimation() + */ + endAnimation(): void; + + /** + * Gets the scrub tween associated with the ScrollTrigger instance (if scrub was defined), or getTween(true) will get the snap tween (assuming snap was defined). + * + * ```js + * let scrub = scrollTrigger.getTween(); + * scrub.progress(1); // immediately finish the scrub + * ``` + * + * @param {boolean} [snap] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/getTween() + */ + getTween(snap?: boolean): gsap.core.Tween; + + /** + * Returns an Array of all ScrollTriggers that precede this one in the updating order according to the current scroll direction. + * + * ```js + * preventOverlaps: self => self.getTrailing().forEach(t => t.endAnimation()); + * ``` + * + * @param {string | boolean} [name] optional preventOverlaps name to filter + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/getTrailing() + */ + getTrailing(name?: string | boolean | null): ScrollTrigger[]; + + /** + * Gets the current velocity of the element's scroll on which the ScrollTrigger is attached to (in pixels per second). + * + * ```js + * scrollTrigger.getVelocity(); + * ``` + * + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/getVelocity() + */ + getVelocity(): number; + + /** + * Removes all added markup, stops all callbacks, and frees it for GC. + * + * ```js + * scrollTrigger.kill(); + * ``` + * + * @param {boolean} [reset] + * @param {boolean} [allowAnimation] + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/kill() + */ + kill(reset?: boolean, allowAnimation?: boolean): void; + + /** + * Converts a timeline's label to the associated scroll position (in px) + * + * ```js + * scrollTrigger.labelToScroll("label-1"); + * ``` + * + * @param {string} label + * @returns {number} The associated scroll position + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/labelToScroll() + */ + labelToScroll(label: string): number; + + /** + * Gets the ScrollTrigger instance that's immediately after this one in the refresh order (if any) + * + * ```js + * scrollTrigger.next(); + * ``` + * + * @memberof ScrollTrigger + * @returns {ScrollTrigger | undefined} The next ScrollTrigger (if one exists) + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/next() + */ + next(): ScrollTrigger | undefined; + + /** + * Gets the ScrollTrigger instance that's immediately before this one in the refresh order (if any) + * + * ```js + * scrollTrigger.previous(); + * ``` + * + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/previous() + */ + previous(): ScrollTrigger | undefined; + + /** + * Forces the ScrollTrigger instance to re-calculate its start and end values (the scroll positions where it'll be activated). + * + * ```js + * scrollTrigger.refresh(); + * ``` + * + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/refresh() + */ + refresh(): void; + + /** + * Gets the scroll position of the ScrollTrigger's scroller. + * + * ```js + * scrollTrigger.scroll(); + * ``` + * + * @returns {number} The scroll position of the scroller + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/scroll() + */ + scroll(): number; + + /** + * Sets the scroll position of the ScrollTrigger's scroller. + * + * ```js + * scrollTrigger.scroll(100); + * ``` + * + * @param {number} position + * @memberof ScrollTrigger + * @link https://greensock.com/docs/v3/Plugins/ScrollTrigger/scroll() + */ + scroll(position: number): void; + + /** + * Animates the scroll position of the ScrollTrigger's scroller. + * + * ```js + * scrollTrigger.tweenTo(100); + * ``` + * + * @param {number} position + * @returns {gsap.core.Tween} Tween + * @memberof ScrollTrigger + */ + tweenTo(position: number): gsap.core.Tween; + + update(reset?: boolean, recordVelocity?: boolean, forceFake?: boolean): void; +} + +declare namespace ScrollTrigger { + + interface RectObj { + top: number; + left: number; + width: number; + height: number; + } + + interface MatchMediaObject { + [key: string]: Function; + } + + type Callback = (self: ScrollTrigger) => any; + type BatchCallback = (targets: Element[], triggers: ScrollTrigger[]) => any; + type NumFunc = () => number; + type SnapFunc = (value: number, self?: ScrollTrigger) => number; + type SnapDirectionalFunc = (value: number, direction?: number, threshold?: number) => number; + type GetterSetterNumFunc = (value?: number) => number | void; + type GetterRectFunc = () => RectObj; + type StartEndFunc = (self: ScrollTrigger) => string | number; + type ScrollFunc = (position: number) => void; + + interface MarkersVars { + endColor?: string; + fontSize?: string; + fontWeight?: string; + indent?: number; + startColor?: string; + } + + interface ToggleClassVars { + className: string; + targets?: gsap.DOMTarget; + } + + interface SnapVars { + delay?: number; + duration?: number | RangeObject; + inertia?: boolean; + ease?: string | gsap.EaseFunction; + snapTo?: number | number[] | "labels" | "labelsDirectional" | SnapFunc; + directional?: boolean; + onInterrupt?: Callback; + onStart?: Callback; + onComplete?: Callback; + } + + interface RangeObject { + min?: number; + max?: number; + } + + interface Vars { + anticipatePin?: number; + containerAnimation?: gsap.core.Animation; + end?: string | number | StartEndFunc; + endTrigger?: gsap.DOMTarget; + fastScrollEnd?: boolean | number; + horizontal?: boolean; + id?: string; + immediateRender?: boolean; + invalidateOnRefresh?: boolean; + markers?: boolean | MarkersVars; + once?: boolean; + onEnter?: Callback; + onEnterBack?: Callback; + onKill?: Callback; + onLeave?: Callback; + onLeaveBack?: Callback; + onRefresh?: Callback; + onRefreshInit?: Callback; + onSnapComplete?: Callback; + onScrubComplete?: Callback; + onUpdate?: Callback; + onToggle?: Callback; + pin?: boolean | gsap.DOMTarget; + pinnedContainer?: gsap.DOMTarget; + pinReparent?: boolean; + pinSpacing?: boolean | string; + pinSpacer?: gsap.DOMTarget; + pinType?: "fixed" | "transform"; + preventOverlaps?: boolean | string | Callback; + refreshPriority?: number; + scroller?: gsap.DOMTarget | Window; + scrub?: boolean | number; + snap?: number | number[] | "labels" | "labelsDirectional" | SnapFunc | SnapVars; + start?: string | number | StartEndFunc; + toggleActions?: string; + toggleClass?: string | ToggleClassVars; + trigger?: gsap.DOMTarget; + } + + interface StaticVars extends Vars { + animation?: gsap.core.Animation; + } + + interface BatchVars { + interval?: number; + batchMax?: number | NumFunc; + anticipatePin?: number; + end?: string | number | StartEndFunc; + fastScrollEnd?: boolean | number; + horizontal?: boolean; + once?: boolean; + onEnter?: BatchCallback; + onEnterBack?: BatchCallback; + onLeave?: BatchCallback; + onLeaveBack?: BatchCallback; + onRefresh?: BatchCallback; + onRefreshInit?: Callback; + onUpdate?: BatchCallback; + onToggle?: BatchCallback; + pin?: boolean | gsap.DOMTarget; + pinReparent?: boolean; + pinSpacing?: boolean | string; + pinSpacer?: gsap.DOMTarget; + pinType?: "fixed" | "transform"; + preventOverlaps?: boolean | string | Callback; + scroller?: gsap.DOMTarget | Window; + start?: string | number | StartEndFunc; + toggleClass?: string | ToggleClassVars; + } + + interface ConfigVars { + limitCallbacks?: boolean; + syncInterval?: number; + autoRefreshEvents?: string; + ignoreMobileResize?: boolean; + } + + interface ScrollerProxyVars { + scrollTop?: GetterSetterNumFunc; + scrollLeft?: GetterSetterNumFunc; + scrollWidth?: GetterSetterNumFunc; + scrollHeight?: GetterSetterNumFunc; + fixedMarkers?: boolean; + getBoundingClientRect?: GetterRectFunc; + pinType?: "fixed" | "transform"; + content?: gsap.DOMTarget; + } + interface NormalizeVars extends Observer.ObserverVars { + momentum?: number | Function; + content?: gsap.DOMTarget; + allowNestedScroll?: boolean; + } + +} + + +declare namespace gsap.plugins { + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.ScrollerProxyVars + */ + type ScrollerProxyVars = ScrollTrigger.ScrollerProxyVars; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger + */ + type ScrollTrigger = any; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.BatchVars + */ + type ScrollTriggerBatchVars = ScrollTrigger.BatchVars; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.ConfigVars + */ + type ScrollTriggerConfigVars = ScrollTrigger.ConfigVars; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger + */ + class ScrollTriggerInstance extends ScrollTrigger {} + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.Vars + */ + type ScrollTriggerInstanceVars = ScrollTrigger.Vars; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger + */ + class ScrollTriggerStatic extends ScrollTrigger {} + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.StaticVars + */ + type ScrollTriggerStaticVars = ScrollTrigger.StaticVars; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.SnapVars; + */ + type SnapVars = ScrollTrigger.SnapVars; + + /** + * @deprecated since 3.7.0 + * @see ScrollTrigger.ToggleClassVars + */ + type ToggleClassVars = ScrollTrigger.ToggleClassVars; +} + +declare module "gsap/ScrollTrigger" { + class _ScrollTrigger extends ScrollTrigger { } + export { + _ScrollTrigger as ScrollTrigger, + _ScrollTrigger as default + } +} + +declare module "gsap/dist/ScrollTrigger" { + export * from "gsap/ScrollTrigger"; + export { ScrollTrigger as default } from "gsap/ScrollTrigger"; +} + +declare module "gsap/src/ScrollTrigger" { + export * from "gsap/ScrollTrigger"; + export { ScrollTrigger as default } from "gsap/ScrollTrigger"; +} + +declare module "gsap/all" { + export * from "gsap/ScrollTrigger"; +} + +declare module "gsap-trial/ScrollTrigger" { + export * from "gsap/ScrollTrigger"; + export { ScrollTrigger as default } from "gsap/ScrollTrigger"; +} + +declare module "gsap-trial/dist/ScrollTrigger" { + export * from "gsap/ScrollTrigger"; + export { ScrollTrigger as default } from "gsap/ScrollTrigger"; +} + +declare module "gsap-trial/src/ScrollTrigger" { + export * from "gsap/ScrollTrigger"; + export { ScrollTrigger as default } from "gsap/ScrollTrigger"; +} + +declare module "gsap-trial/all" { + export * from "gsap/ScrollTrigger"; +} diff --git a/node_modules/gsap/types/split-text.d.ts b/node_modules/gsap/types/split-text.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..f5187709a38daa86023c97f79eb7982e49b38f05 --- /dev/null +++ b/node_modules/gsap/types/split-text.d.ts @@ -0,0 +1,92 @@ +declare class SplitText { + readonly chars: Element[]; + readonly lines: Element[]; + readonly words: Element[]; + readonly elements: Element[]; + readonly selector: string | Function; + + constructor(target: gsap.DOMTarget, vars?: SplitText.Vars); + + + /** + * Reverts the innerHTML to the original content. + * + * ```js + * split.revert(); + * ``` + * + * @memberof SplitText + * @link https://greensock.com/docs/v3/Plugins/SplitText/revert() + */ + revert(): void; + + /** + * Re-splits a SplitText according to the vars provided. It will automatically call revert() first if necessary. Useful if you want to change the way the text is split after the SplitText instance is created. + * + * ```js + * split.split({type: "lines,chars"}); + * ``` + * + * @param {SplitText.Vars} vars + * @returns {SplitText} The SplitText object created + * @memberof SplitText + * @link https://greensock.com/docs/v3/Plugins/SplitText/split() + */ + split(vars: SplitText.Vars): SplitText; +} + +declare namespace SplitText { + interface Vars { + [key: string]: any; + type?: string; + charsClass?: string; + wordsClass?: string; + linesClass?: string; + position?: string; + lineThreshold?: number; + reduceWhiteSpace?: boolean; + specialChars?: string[] | Function; + wordDelimiter?: string; + } +} + +declare module "gsap/SplitText" { + class _SplitText extends SplitText {} + export { + _SplitText as SplitText, + _SplitText as default + } +} + +declare module "gsap/src/SplitText" { + export * from "gsap/SplitText"; + export { SplitText as default } from "gsap/SplitText"; +} + +declare module "gsap/dist/SplitText" { + export * from "gsap/SplitText"; + export { SplitText as default } from "gsap/SplitText"; +} + +declare module "gsap/all" { + export * from "gsap/SplitText"; +} + +declare module "gsap-trial/SplitText" { + export * from "gsap/SplitText"; + export { SplitText as default } from "gsap/SplitText"; +} + +declare module "gsap-trial/src/SplitText" { + export * from "gsap/SplitText"; + export { SplitText as default } from "gsap/SplitText"; +} + +declare module "gsap-trial/dist/SplitText" { + export * from "gsap/SplitText"; + export { SplitText as default } from "gsap/SplitText"; +} + +declare module "gsap-trial/all" { + export * from "gsap/SplitText"; +} diff --git a/node_modules/gsap/types/text-plugin.d.ts b/node_modules/gsap/types/text-plugin.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..bbe7abd0a876ea011b5867fe144bf5fe47f6051b --- /dev/null +++ b/node_modules/gsap/types/text-plugin.d.ts @@ -0,0 +1,73 @@ +declare namespace TextPlugin { + interface Vars { + value: string; + type?: string; + rtl?: boolean; + speed?: number; + delimiter?: string; + padSpace?: boolean; + newClass?: string; + oldClass?: string; + preserveSpaces?: boolean; + } +} + +declare namespace gsap { + + interface TweenVars { + text?: string | TextPlugin.Vars; + } +} + +declare namespace gsap.plugins { + interface TextPlugin extends Plugin { + + } + + interface TextPluginClass extends TextPlugin { + new(): PluginScope & TextPlugin; + prototype: PluginScope & TextPlugin; + } + + const text: TextPluginClass; +} + +declare const TextPlugin: gsap.plugins.TextPlugin; + +declare module "gsap/TextPlugin" { + export const TextPlugin: gsap.plugins.TextPlugin; + export { TextPlugin as default }; +} + +declare module "gsap/src/TextPlugin" { + export * from "gsap/TextPlugin"; + export { TextPlugin as default } from "gsap/TextPlugin"; +} + +declare module "gsap/dist/TextPlugin" { + export * from "gsap/TextPlugin"; + export { TextPlugin as default } from "gsap/TextPlugin"; +} + +declare module "gsap/all" { + export * from "gsap/TextPlugin"; +} + +declare module "gsap-trial/TextPlugin" { + export * from "gsap/TextPlugin"; + export { TextPlugin as default } from "gsap/TextPlugin"; +} + +declare module "gsap-trial/src/TextPlugin" { + export * from "gsap/TextPlugin"; + export { TextPlugin as default } from "gsap/TextPlugin"; +} + +declare module "gsap-trial/dist/TextPlugin" { + export * from "gsap/TextPlugin"; + export { TextPlugin as default } from "gsap/TextPlugin"; +} + +declare module "gsap-trial/all" { + export * from "gsap/TextPlugin"; +} diff --git a/node_modules/gsap/types/timeline.d.ts b/node_modules/gsap/types/timeline.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..bb0daf6633f46d261ca6bdcfa375c8af41c630fc --- /dev/null +++ b/node_modules/gsap/types/timeline.d.ts @@ -0,0 +1,511 @@ +declare namespace gsap.core { + + type TimelineChild = string | Animation | Callback | Array<string | Animation | Callback>; + + interface Labels { + [key: string]: number; + } + + class Timeline extends Animation { + + autoRemoveChildren: boolean; + labels: Labels; + smoothChildTiming: boolean; + vars: TimelineVars; + [key: string]: any; // for gsap.registerEffect({... extendTimeline: true}) + + constructor(vars?: TimelineVars, time?: number); + + static updateRoot(time: number): void; + + /** + * Adds a label, tween, timeline, or an array of those values to the timeline, optionally at the specified time. + * + * ```js + * tl.add("myLabel"); // add a label at the end of the timeline + * tl.add(myTween, 1); // add a tween at the 1 second mark + * tl.add(myTimeline, "-=1"); // add a timeline 1 second before the end of the timeline + * tl.add(["myLabel", myTween, myTimeline], "<"); // add a label, tween, and timeline at the start of the previous tween + * ``` + * + * @param {TimelineChild} child + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/add() + */ + add(child: TimelineChild, position?: Position): this; + + /** + * Adds a label to the timeline, optionally at the specified time. + * + * ```js + * tl.addLabel("myLabel", 1); // add a label at the 1 second mark + * ``` + * + * @param {string} label + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/addLabel() + */ + addLabel(label: string, position?: Position): this; + + /** + * Adds a pause to the timeline, optionally at the specified time. + * + * ```js + * tl.addPause(); // add a pause at the end of the timeline + * tl.addPause(1, myCallback); // add a pause at the 1 second mark with a callback + * ``` + * + * @param {Position} [position] + * @param {Callback} [callback] + * @param {any[]} [params] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/addPause() + */ + addPause(position?: Position, callback?: Callback, params?: any[]): this; + + /** + * Call a function, optionally at the specified time. + * + * ```js + * tl.call(myCallback); // add a function call at the end of the timeline + * tl.call(myCallback, ["param"], 1); // add a function call at the 1 second mark with a parameter passed in + * ``` + * + * @param {Callback} callback + * @param {any[]} [params] + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/call() + */ + call(callback: Callback, params?: any[], position?: Position): this; + + /** + * Empties the timeline of all tweens, timelines, callbacks, and optionally labels. + * + * ```js + * tl.clear(); // empty the timeline not including labels + * tl.clear(true); // empty the timeline including labels + * ``` + * + * @param {boolean} labels + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/clear() + */ + clear(labels?: boolean): this; + + /** + * Makes the timeline's progress jump to the provided label. + * + * ```js + * tl.currentLabel("myLabel"); + * ``` + * + * @param {string} value + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/currentLabel() + */ + currentLabel(value: string): this; + + /** + * Gets the closest label that is at or before the current time. + * + * ```js + * tl.currentLabel(); + * ``` + * + * @returns {string} The nearest label + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/currentLabel() + */ + currentLabel(): string; + + /** + * Creates a tween coming FROM the given values. + * + * ```js + * tl.from(".class", { x: 100 }, "+=1"); // adds the tween one second after the end of the timeline + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/from() + */ + from(targets: TweenTarget, vars: TweenVars, position?: Position): this; + + /** + * **Deprecated method signature.** Use the `duration` property instead. + * + * ```js + * tl.from(".class", 1, { x: 100 }, "+=1"); // adds the tween one second after the end of the timeline + * ``` + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {TweenVars} vars + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/from() + */ + from(targets: TweenTarget, duration: number, vars: TweenVars, position?: Position): this; + + /** + * Creates a tween coming FROM the first set of values going TO the second set of values. + * + * ```js + * tl.fromTo(".class", {x: 0}, { x: 100 }, "+=1"); // adds the tween one second after the end of the timeline + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} fromVars + * @param {TweenVars} toVars + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/fromTo() + */ + fromTo(targets: TweenTarget, fromVars: TweenVars, toVars: TweenVars, position?: Position): this; + + /** + * **Deprecated method signature.** Use the `duration` property instead. + * + * ```js + * tl.fromTo(".class", 1, {x: 0}, { x: 100 }, "+=1"); // adds the tween one second after the end of the timeline + * ``` + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {TweenVars} fromVars + * @param {TweenVars} toVars + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/fromTo() + */ + fromTo(targets: TweenTarget, duration: number, fromVars: TweenVars, toVars: TweenVars, position?: Position): this; + + /** + * Returns the tween or timeline associated with the provided ID. + * + * ```js + * tl.getById("myTween"); + * ``` + * + * @param {string} id + * @returns {Tween | Timeline} + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/getById() + */ + getById(id: string): Tween | Timeline; + + /** + * Returns an array containing all the tweens and/or timelines nested in this timeline. + * + * ```js + * tl.getChildren(); + * tl.getChildren(true, true, true, 0.5); + * ``` + * + * @param {boolean} [nested] + * @param {boolean} [tweens] + * @param {boolean} [timelines] + * @param {number} [ignoreBeforeTime] + * @returns {(Tween | Timeline)[]} Array of tweens and timelines + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/getChildren() + */ + getChildren(nested?: boolean, tweens?: boolean, timelines?: boolean, ignoreBeforeTime?: number): (Tween | Timeline)[]; + + /** + * Returns the tweens of a particular object that are inside this timeline. + * + * ```js + * tl.getTweensOf(".myClass"); + * tl.getTweensOf(myElem, true); + * ``` + * + * @param {TweenTarget} targets + * @param {boolean} [onlyActive] + * @returns {Tween[]} Array of tweens + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/getTweensOf() + */ + getTweensOf(targets: TweenTarget, onlyActive?: boolean): Tween[]; + + /** + * Returns the next label in the timeline, optionally from the provided time. + * + * ```js + * tl.nextLabel(); + * tl.nextLabel(2); + * ``` + * + * @param {number} [time] + * @returns {string} The next label + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/nextLabel() + */ + nextLabel(time?: number): string; + + /** + * Returns the previous label in the timeline, optionally from the provided time. + * + * ```js + * tl.previousLabel(); + * tl.previousLabel(2); + * ``` + * + * @param {number} [time] + * @returns {string} The previous label + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/previousLabel() + */ + previousLabel(time?: number): string; + + /** + * Returns the most recently added child tween, timeline, or callback regardless of its position in the timeline. + * + * ```js + * tl.recent(); + * ``` + * + * @returns {Tween | Timeline | Function} The most recent tween, timeline, or callback + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/recent() + */ + recent(): Tween | Timeline | Function; + + /** + * Removes a tween, timeline, callback, label, or array of those values from the timeline. + * + * ```js + * tl.remove(myTween); + * tl.remove([myTween, mySubTimeline, "myLabel"]); + * ``` + * + * @param {TimelineChild} value + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/remove() + */ + remove(value: TimelineChild): this; + + /** + * Removes a label from the timeline and returns the time of that label. + * + * ```js + * tl.removeLabel("myLabel"); // returns the label time like 1.0 + * ``` + * + * @param {string} label + * @returns {number} The time of the removed label + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/removeLabel() + */ + removeLabel(label: string): number; + + /** + * Removes pauses that were added to a timeline via its .addPause() method. + * + * ```js + * tl.removePause(1); // returns the pause at time 1 + * ``` + * + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/removePause() + */ + removePause(position: Position): this; + + /** + * Sets properties of the target(s) to the properties specified at the time of the set call. + * + * ```js + * tl.set(".class", {x: 100, y: 50, opacity: 0}, 1); + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @param {Position} [position] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/set() + */ + set(targets: TweenTarget, vars: TweenVars, position?: Position): this; + + /** + * Shifts the startTime of the timeline's children by a certain amount and optionally adjusts labels too. + * + * ```js + * tl.shiftChildren(1); // shift the child tweens, timelines, and callbacks by 1 second + * ``` + * + * @param {number} amount + * @param {boolean} [adjustLabels] + * @param {number} [ignoreBeforeTime] + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/shiftChildren() + */ + shiftChildren(amount: number, adjustLabels?: boolean, ignoreBeforeTime?: number): this; + + /** + * **Deprecated method.** Use the `.from()` method instead. + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/from() + */ + staggerFrom(targets: TweenTarget, vars: TweenVars, position?: Position): this; + + /** + * **Deprecated method.** Use the `.from()` method instead. + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {Timeline} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/from() + */ + staggerFrom(targets: TweenTarget, duration: number, vars: TweenVars, position?: Position): this; + + /** + * **Deprecated method.** Use the `.fromTo()` method instead. + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/fromTo() + */ + staggerFromTo(targets: TweenTarget, fromVars: TweenVars, toVars: TweenVars, position?: Position): this; + + /** + * **Deprecated method.** Use the `.fromTo()` method instead. + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {Timeline} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/fromTo() + */ + staggerFromTo(targets: TweenTarget, duration: number, fromVars: TweenVars, toVars: TweenVars, position?: Position): this; + + /** + * **Deprecated method.** Use the `.to()` method instead. + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/to() + */ + staggerTo(targets: TweenTarget, vars: TweenVars, position?: Position): this; + + /** + * **Deprecated method.** Use the `.to()` method instead. + * + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {Timeline} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/to() + */ + staggerTo(targets: TweenTarget, duration: number, vars: TweenVars, position?: Position): this; + + /** + * Creates a tween going TO the given values. + * + * ```js + * tl.to(".class", {x: 100}, 1); + * ``` + * + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/to() + */ + to(targets: TweenTarget, vars: TweenVars, position?: Position): this; + + /** + * **Deprecated method signature.** Use the `duration` property instead. + * + * ```js + * tl.to(".class", 1, {x: 100}, 1); + * ``` + * @deprecated since version 3.0.0 + * @param {TweenTarget} targets + * @param {number} duration - The duration parameter is deprecated. Use the `duration` property instead. + * @param {Timeline} vars + * @param {Position} position + * @returns {Timeline} The timeline + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/to() + */ + to(targets: TweenTarget, duration: number, vars: TweenVars, position?: Position): this; + + /** + * Tween linearly from a particular time or label to another time or label and then stops. + * + * ```js + * tl.tweenFromTo("myLabel", 5); // tween from myLabel to the 5 second mark + * ``` + * + * @param {Position} fromPosition + * @param {Position} toPosition + * @param {TweenVars} [vars] + * @returns {Tween} The tweenFromTo tween + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/tweenFromTo() + */ + tweenFromTo(fromPosition: Position, toPosition: Position, vars?: TweenVars): Tween; + + /** + * Tween linearly to a particular time and then stops. + * + * ```js + * tl.tweenTo("myLabel"); // tween to myLabel + * ``` + * + * @param {Position} position + * @param {TweenVars} [vars] + * @returns {Tween} The tweenTo tween + * @memberof Timeline + * @link https://greensock.com/docs/v3/GSAP/Timeline/tweenTo() + */ + tweenTo(position: Position, vars?: TweenVars): Tween; + } +} diff --git a/node_modules/gsap/types/tween.d.ts b/node_modules/gsap/types/tween.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..ea7713076cba63da3db42d6c1e3443b9dd97810e --- /dev/null +++ b/node_modules/gsap/types/tween.d.ts @@ -0,0 +1,247 @@ +declare namespace gsap.core { + + class Tween extends Animation { + + data: any; + vars: TweenVars; + ratio: number; + + constructor(targets: TweenTarget, vars: TweenVars, time?: number); + constructor(targets: TweenTarget, duration: number, vars: TweenVars); + + /** + * **Deprecated method.** Use `gsap.to()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {number} duration + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.to() + */ + static to(targets: TweenTarget, duration: number, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.to()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.to() + */ + static to(targets: TweenTarget, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.from()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {number} duration + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.from() + */ + static from(targets: TweenTarget, duration: number, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.from()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.from() + */ + static from(targets: TweenTarget, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.fromTo()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {number} duration + * @param {TweenVars} fromVars + * @param {TweenVars} toVars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.fromTo() + */ + static fromTo(targets: TweenTarget, duration: number, fromVars: TweenVars, toVars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.fromTo()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} fromVars + * @param {TweenVars} toVars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.fromTo() + */ + static fromTo(targets: TweenTarget, fromVars: TweenVars, toVars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.set()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.set() + */ + static set(targets: TweenTarget, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.from()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {number} duration + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.from() + */ + static staggerFrom(targets: TweenTarget, duration: number, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.from()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.from() + */ + static staggerFrom(targets: TweenTarget, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.fromTo()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {number} duration + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.fromTo() + */ + static staggerFromTo(targets: TweenTarget, duration: number, fromVars: TweenVars, toVars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.fromTo()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.fromTo() + */ + static staggerFromTo(targets: TweenTarget, fromVars: TweenVars, toVars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.to()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {number} duration + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.to() + */ + static staggerTo(targets: TweenTarget, duration: number, vars: TweenVars): Tween; + + /** + * **Deprecated method.** Use `gsap.to()` instead. + * + * @deprecated since 3.0.0 + * @static + * @param {TweenTarget} targets + * @param {TweenVars} vars + * @returns {Tween} Tween instance + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/gsap.to() + */ + static staggerTo(targets: TweenTarget, vars: TweenVars): Tween; + + /** + * Kills the parts of the tween specified. + * To kill means to immediately stop the tween, remove it from its parent timeline, and release it for garbage collection. + * + * ```js + * // kills the entire tween + * tween.kill(); + * + * // kill all parts of the tween related to the target "myObject" (if the tween has multiple targets, the others will not be affected): + * tween.kill(myObject); + * + * // kill only the "x" and "y" properties of the tween (all targets): + * tween.kill(null, "x,y"); + * + * // kill only the "x" and "y" properties of tween of the target "myObject": + * tween.kill(myObject, "x,y"); + * + * // kill only the "opacity" properties of the tween of the targets "myObject1" and "myObject2": + * tween.kill([myObject1, myObject2], "opacity"); + * ``` + * + * @returns {Tween} The tween + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/Tween/kill() + */ + kill(target?: object, propertiesList?: string): this; + + /** + * Redirects a property [that's already being animated by the tween] to a new value and restarts the tween in a performant way. + * A more common technique is to use gsap.quickTo() which uses resetTo() under the hood. + * + * ```js + * tween.resetTo("x", 200); + * ``` + * + * @param {string} property + * @param {number} value + * @param {number} start + * @param {boolean} startIsRelative + * @returns {Tween} The tween + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/Tween/resetTo() + */ + resetTo(property: string, value: number, start?: number, startIsRelative?: boolean): this; + + /** + * Returns an array of all of the tween's targets. + * + * ```js + * tween.targets(); + * ``` + * + * @returns {T[]} The array of targets + * @memberof Tween + * @link https://greensock.com/docs/v3/GSAP/Tween/targets() + */ + targets<T>(): T[]; + } +} diff --git a/node_modules/gsap/types/utils/velocity-tracker.d.ts b/node_modules/gsap/types/utils/velocity-tracker.d.ts new file mode 100644 index 0000000000000000000000000000000000000000..14fd0c97ddfe9558b2e80e8f9e6707bf8d21affc --- /dev/null +++ b/node_modules/gsap/types/utils/velocity-tracker.d.ts @@ -0,0 +1,53 @@ +declare namespace gsap { + + type VelocityType = "num" | "deg" | "rad"; + + interface VelocityMap { + [key: string]: number; + } + + interface VelocityTrackerInstance { + readonly target: object; + add(property: string, type?: VelocityType): void; + kill(shallow?: boolean): void; + remove(property: string): void; + getAll(): VelocityMap; + get(property: string): number; + } + + interface VelocityTrackerStatic { + getByTarget(target: TweenTarget): VelocityTrackerInstance; + getVelocity(target: TweenTarget, property: string): number; + isTracking(target: TweenTarget, property?: string): boolean; + track(target: TweenTarget, properties: string, type?: VelocityType): VelocityTrackerInstance[]; + untrack(target: TweenTarget, properties?: string): void; + } + + interface VelocityTracker extends VelocityTrackerStatic { + new(target: TweenTarget, properties?: string, type?: VelocityType, next?: VelocityTrackerInstance): VelocityTrackerInstance; + prototype: VelocityTrackerInstance; + register(core: typeof gsap): void; + } +} + +declare const VelocityTracker: gsap.VelocityTracker; + +declare module "gsap/utils/VelocityTracker" { + export const VelocityTracker: gsap.VelocityTracker; + export { VelocityTracker as default }; +} + +declare module "gsap/src/utils/VelocityTracker" { + export * from "gsap/utils/VelocityTracker"; + export { VelocityTracker as default } from "gsap/utils/VelocityTracker"; +} + +declare module "gsap-trial/utils/VelocityTracker" { + export * from "gsap/utils/VelocityTracker"; + export { VelocityTracker as default } from "gsap/utils/VelocityTracker"; +} + +declare module "gsap-trial/src/utils/VelocityTracker" { + export * from "gsap/utils/VelocityTracker"; + export { VelocityTracker as default } from "gsap/utils/VelocityTracker"; +} diff --git a/node_modules/gsap/utils/matrix.js b/node_modules/gsap/utils/matrix.js new file mode 100644 index 0000000000000000000000000000000000000000..ddf594e32ede52a1e878088adf0367a9b3616e30 --- /dev/null +++ b/node_modules/gsap/utils/matrix.js @@ -0,0 +1,420 @@ +/*! + * matrix 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var _doc, + _win, + _docElement, + _body, + _divContainer, + _svgContainer, + _identityMatrix, + _gEl, + _transformProp = "transform", + _transformOriginProp = _transformProp + "Origin", + _hasOffsetBug, + _setDoc = function _setDoc(element) { + var doc = element.ownerDocument || element; + + if (!(_transformProp in element.style) && "msTransform" in element.style) { + //to improve compatibility with old Microsoft browsers + _transformProp = "msTransform"; + _transformOriginProp = _transformProp + "Origin"; + } + + while (doc.parentNode && (doc = doc.parentNode)) {} + + _win = window; + _identityMatrix = new Matrix2D(); + + if (doc) { + _doc = doc; + _docElement = doc.documentElement; + _body = doc.body; + _gEl = _doc.createElementNS("http://www.w3.org/2000/svg", "g"); // prevent any existing CSS from transforming it + + _gEl.style.transform = "none"; // now test for the offset reporting bug. Use feature detection instead of browser sniffing to make things more bulletproof and future-proof. Hopefully Safari will fix their bug soon. + + var d1 = doc.createElement("div"), + d2 = doc.createElement("div"), + root = doc && (doc.body || doc.firstElementChild); + + if (root && root.appendChild) { + root.appendChild(d1); + d1.appendChild(d2); + d1.setAttribute("style", "position:static;transform:translate3d(0,0,1px)"); + _hasOffsetBug = d2.offsetParent !== d1; + root.removeChild(d1); + } + } + + return doc; +}, + _forceNonZeroScale = function _forceNonZeroScale(e) { + // walks up the element's ancestors and finds any that had their scale set to 0 via GSAP, and changes them to 0.0001 to ensure that measurements work. Firefox has a bug that causes it to incorrectly report getBoundingClientRect() when scale is 0. + var a, cache; + + while (e && e !== _body) { + cache = e._gsap; + cache && cache.uncache && cache.get(e, "x"); // force re-parsing of transforms if necessary + + if (cache && !cache.scaleX && !cache.scaleY && cache.renderTransform) { + cache.scaleX = cache.scaleY = 1e-4; + cache.renderTransform(1, cache); + a ? a.push(cache) : a = [cache]; + } + + e = e.parentNode; + } + + return a; +}, + // possible future addition: pass an element to _forceDisplay() and it'll walk up all its ancestors and make sure anything with display: none is set to display: block, and if there's no parentNode, it'll add it to the body. It returns an Array that you can then feed to _revertDisplay() to have it revert all the changes it made. +// _forceDisplay = e => { +// let a = [], +// parent; +// while (e && e !== _body) { +// parent = e.parentNode; +// (_win.getComputedStyle(e).display === "none" || !parent) && a.push(e, e.style.display, parent) && (e.style.display = "block"); +// parent || _body.appendChild(e); +// e = parent; +// } +// return a; +// }, +// _revertDisplay = a => { +// for (let i = 0; i < a.length; i+=3) { +// a[i+1] ? (a[i].style.display = a[i+1]) : a[i].style.removeProperty("display"); +// a[i+2] || a[i].parentNode.removeChild(a[i]); +// } +// }, +_svgTemps = [], + //we create 3 elements for SVG, and 3 for other DOM elements and cache them for performance reasons. They get nested in _divContainer and _svgContainer so that just one element is added to the DOM on each successive attempt. Again, performance is key. +_divTemps = [], + _getDocScrollTop = function _getDocScrollTop() { + return _win.pageYOffset || _doc.scrollTop || _docElement.scrollTop || _body.scrollTop || 0; +}, + _getDocScrollLeft = function _getDocScrollLeft() { + return _win.pageXOffset || _doc.scrollLeft || _docElement.scrollLeft || _body.scrollLeft || 0; +}, + _svgOwner = function _svgOwner(element) { + return element.ownerSVGElement || ((element.tagName + "").toLowerCase() === "svg" ? element : null); +}, + _isFixed = function _isFixed(element) { + if (_win.getComputedStyle(element).position === "fixed") { + return true; + } + + element = element.parentNode; + + if (element && element.nodeType === 1) { + // avoid document fragments which will throw an error. + return _isFixed(element); + } +}, + _createSibling = function _createSibling(element, i) { + if (element.parentNode && (_doc || _setDoc(element))) { + var svg = _svgOwner(element), + ns = svg ? svg.getAttribute("xmlns") || "http://www.w3.org/2000/svg" : "http://www.w3.org/1999/xhtml", + type = svg ? i ? "rect" : "g" : "div", + x = i !== 2 ? 0 : 100, + y = i === 3 ? 100 : 0, + css = "position:absolute;display:block;pointer-events:none;margin:0;padding:0;", + e = _doc.createElementNS ? _doc.createElementNS(ns.replace(/^https/, "http"), type) : _doc.createElement(type); + + if (i) { + if (!svg) { + if (!_divContainer) { + _divContainer = _createSibling(element); + _divContainer.style.cssText = css; + } + + e.style.cssText = css + "width:0.1px;height:0.1px;top:" + y + "px;left:" + x + "px"; + + _divContainer.appendChild(e); + } else { + _svgContainer || (_svgContainer = _createSibling(element)); + e.setAttribute("width", 0.01); + e.setAttribute("height", 0.01); + e.setAttribute("transform", "translate(" + x + "," + y + ")"); + + _svgContainer.appendChild(e); + } + } + + return e; + } + + throw "Need document and parent."; +}, + _consolidate = function _consolidate(m) { + // replaces SVGTransformList.consolidate() because a bug in Firefox causes it to break pointer events. See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800 + var c = new Matrix2D(), + i = 0; + + for (; i < m.numberOfItems; i++) { + c.multiply(m.getItem(i).matrix); + } + + return c; +}, + _getCTM = function _getCTM(svg) { + var m = svg.getCTM(), + transform; + + if (!m) { + // Firefox returns null for getCTM() on root <svg> elements, so this is a workaround using a <g> that we temporarily append. + transform = svg.style[_transformProp]; + svg.style[_transformProp] = "none"; // a bug in Firefox causes css transforms to contaminate the getCTM() + + svg.appendChild(_gEl); + m = _gEl.getCTM(); + svg.removeChild(_gEl); + transform ? svg.style[_transformProp] = transform : svg.style.removeProperty(_transformProp.replace(/([A-Z])/g, "-$1").toLowerCase()); + } + + return m || _identityMatrix.clone(); // Firefox will still return null if the <svg> has a width/height of 0 in the browser. +}, + _placeSiblings = function _placeSiblings(element, adjustGOffset) { + var svg = _svgOwner(element), + isRootSVG = element === svg, + siblings = svg ? _svgTemps : _divTemps, + parent = element.parentNode, + container, + m, + b, + x, + y, + cs; + + if (element === _win) { + return element; + } + + siblings.length || siblings.push(_createSibling(element, 1), _createSibling(element, 2), _createSibling(element, 3)); + container = svg ? _svgContainer : _divContainer; + + if (svg) { + if (isRootSVG) { + b = _getCTM(element); + x = -b.e / b.a; + y = -b.f / b.d; + m = _identityMatrix; + } else if (element.getBBox) { + b = element.getBBox(); + m = element.transform ? element.transform.baseVal : {}; // IE11 doesn't follow the spec. + + m = !m.numberOfItems ? _identityMatrix : m.numberOfItems > 1 ? _consolidate(m) : m.getItem(0).matrix; // don't call m.consolidate().matrix because a bug in Firefox makes pointer events not work when consolidate() is called on the same tick as getBoundingClientRect()! See https://gsap.com/forums/topic/23248-touch-is-not-working-on-draggable-in-firefox-windows-v324/?tab=comments#comment-109800 + + x = m.a * b.x + m.c * b.y; + y = m.b * b.x + m.d * b.y; + } else { + // may be a <mask> which has no getBBox() so just use defaults instead of throwing errors. + m = new Matrix2D(); + x = y = 0; + } + + if (adjustGOffset && element.tagName.toLowerCase() === "g") { + x = y = 0; + } + + (isRootSVG ? svg : parent).appendChild(container); + container.setAttribute("transform", "matrix(" + m.a + "," + m.b + "," + m.c + "," + m.d + "," + (m.e + x) + "," + (m.f + y) + ")"); + } else { + x = y = 0; + + if (_hasOffsetBug) { + // some browsers (like Safari) have a bug that causes them to misreport offset values. When an ancestor element has a transform applied, it's supposed to treat it as if it's position: relative (new context). Safari botches this, so we need to find the closest ancestor (between the element and its offsetParent) that has a transform applied and if one is found, grab its offsetTop/Left and subtract them to compensate. + m = element.offsetParent; + b = element; + + while (b && (b = b.parentNode) && b !== m && b.parentNode) { + if ((_win.getComputedStyle(b)[_transformProp] + "").length > 4) { + x = b.offsetLeft; + y = b.offsetTop; + b = 0; + } + } + } + + cs = _win.getComputedStyle(element); + + if (cs.position !== "absolute" && cs.position !== "fixed") { + m = element.offsetParent; + + while (parent && parent !== m) { + // if there's an ancestor element between the element and its offsetParent that's scrolled, we must factor that in. + x += parent.scrollLeft || 0; + y += parent.scrollTop || 0; + parent = parent.parentNode; + } + } + + b = container.style; + b.top = element.offsetTop - y + "px"; + b.left = element.offsetLeft - x + "px"; + b[_transformProp] = cs[_transformProp]; + b[_transformOriginProp] = cs[_transformOriginProp]; // b.border = m.border; + // b.borderLeftStyle = m.borderLeftStyle; + // b.borderTopStyle = m.borderTopStyle; + // b.borderLeftWidth = m.borderLeftWidth; + // b.borderTopWidth = m.borderTopWidth; + + b.position = cs.position === "fixed" ? "fixed" : "absolute"; + element.parentNode.appendChild(container); + } + + return container; +}, + _setMatrix = function _setMatrix(m, a, b, c, d, e, f) { + m.a = a; + m.b = b; + m.c = c; + m.d = d; + m.e = e; + m.f = f; + return m; +}; + +export var Matrix2D = /*#__PURE__*/function () { + function Matrix2D(a, b, c, d, e, f) { + if (a === void 0) { + a = 1; + } + + if (b === void 0) { + b = 0; + } + + if (c === void 0) { + c = 0; + } + + if (d === void 0) { + d = 1; + } + + if (e === void 0) { + e = 0; + } + + if (f === void 0) { + f = 0; + } + + _setMatrix(this, a, b, c, d, e, f); + } + + var _proto = Matrix2D.prototype; + + _proto.inverse = function inverse() { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + determinant = a * d - b * c || 1e-10; + return _setMatrix(this, d / determinant, -b / determinant, -c / determinant, a / determinant, (c * f - d * e) / determinant, -(a * f - b * e) / determinant); + }; + + _proto.multiply = function multiply(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f, + a2 = matrix.a, + b2 = matrix.c, + c2 = matrix.b, + d2 = matrix.d, + e2 = matrix.e, + f2 = matrix.f; + return _setMatrix(this, a2 * a + c2 * c, a2 * b + c2 * d, b2 * a + d2 * c, b2 * b + d2 * d, e + e2 * a + f2 * c, f + e2 * b + f2 * d); + }; + + _proto.clone = function clone() { + return new Matrix2D(this.a, this.b, this.c, this.d, this.e, this.f); + }; + + _proto.equals = function equals(matrix) { + var a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + return a === matrix.a && b === matrix.b && c === matrix.c && d === matrix.d && e === matrix.e && f === matrix.f; + }; + + _proto.apply = function apply(point, decoratee) { + if (decoratee === void 0) { + decoratee = {}; + } + + var x = point.x, + y = point.y, + a = this.a, + b = this.b, + c = this.c, + d = this.d, + e = this.e, + f = this.f; + decoratee.x = x * a + y * c + e || 0; + decoratee.y = x * b + y * d + f || 0; + return decoratee; + }; + + return Matrix2D; +}(); // Feed in an element and it'll return a 2D matrix (optionally inverted) so that you can translate between coordinate spaces. +// Inverting lets you translate a global point into a local coordinate space. No inverting lets you go the other way. +// We needed this to work around various browser bugs, like Firefox doesn't accurately report getScreenCTM() when there +// are transforms applied to ancestor elements. +// The matrix math to convert any x/y coordinate is as follows, which is wrapped in a convenient apply() method of Matrix2D above: +// tx = m.a * x + m.c * y + m.e +// ty = m.b * x + m.d * y + m.f + +export function getGlobalMatrix(element, inverse, adjustGOffset, includeScrollInFixed) { + // adjustGOffset is typically used only when grabbing an element's PARENT's global matrix, and it ignores the x/y offset of any SVG <g> elements because they behave in a special way. + if (!element || !element.parentNode || (_doc || _setDoc(element)).documentElement === element) { + return new Matrix2D(); + } + + var zeroScales = _forceNonZeroScale(element), + svg = _svgOwner(element), + temps = svg ? _svgTemps : _divTemps, + container = _placeSiblings(element, adjustGOffset), + b1 = temps[0].getBoundingClientRect(), + b2 = temps[1].getBoundingClientRect(), + b3 = temps[2].getBoundingClientRect(), + parent = container.parentNode, + isFixed = !includeScrollInFixed && _isFixed(element), + m = new Matrix2D((b2.left - b1.left) / 100, (b2.top - b1.top) / 100, (b3.left - b1.left) / 100, (b3.top - b1.top) / 100, b1.left + (isFixed ? 0 : _getDocScrollLeft()), b1.top + (isFixed ? 0 : _getDocScrollTop())); + + parent.removeChild(container); + + if (zeroScales) { + b1 = zeroScales.length; + + while (b1--) { + b2 = zeroScales[b1]; + b2.scaleX = b2.scaleY = 0; + b2.renderTransform(1, b2); + } + } + + return inverse ? m.inverse() : m; +} +export { _getDocScrollTop, _getDocScrollLeft, _setDoc, _isFixed, _getCTM }; // export function getMatrix(element) { +// _doc || _setDoc(element); +// let m = (_win.getComputedStyle(element)[_transformProp] + "").substr(7).match(/[-.]*\d+[.e\-+]*\d*[e\-\+]*\d*/g), +// is2D = m && m.length === 6; +// return !m || m.length < 6 ? new Matrix2D() : new Matrix2D(+m[0], +m[1], +m[is2D ? 2 : 4], +m[is2D ? 3 : 5], +m[is2D ? 4 : 12], +m[is2D ? 5 : 13]); +// } \ No newline at end of file diff --git a/node_modules/gsap/utils/paths.js b/node_modules/gsap/utils/paths.js new file mode 100644 index 0000000000000000000000000000000000000000..046395a3ab68ac3355f87f8ed4386bb62d90711b --- /dev/null +++ b/node_modules/gsap/utils/paths.js @@ -0,0 +1,1512 @@ +/*! + * paths 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _numbersExp = /(?:(-)?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + _selectorExp = /(^[#\.][a-z]|[a-y][a-z])/i, + _DEG2RAD = Math.PI / 180, + _RAD2DEG = 180 / Math.PI, + _sin = Math.sin, + _cos = Math.cos, + _abs = Math.abs, + _sqrt = Math.sqrt, + _atan2 = Math.atan2, + _largeNum = 1e8, + _isString = function _isString(value) { + return typeof value === "string"; +}, + _isNumber = function _isNumber(value) { + return typeof value === "number"; +}, + _isUndefined = function _isUndefined(value) { + return typeof value === "undefined"; +}, + _temp = {}, + _temp2 = {}, + _roundingNum = 1e5, + _wrapProgress = function _wrapProgress(progress) { + return Math.round((progress + _largeNum) % 1 * _roundingNum) / _roundingNum || (progress < 0 ? 0 : 1); +}, + //if progress lands on 1, the % will make it 0 which is why we || 1, but not if it's negative because it makes more sense for motion to end at 0 in that case. +_round = function _round(value) { + return Math.round(value * _roundingNum) / _roundingNum || 0; +}, + _roundPrecise = function _roundPrecise(value) { + return Math.round(value * 1e10) / 1e10 || 0; +}, + _splitSegment = function _splitSegment(rawPath, segIndex, i, t) { + var segment = rawPath[segIndex], + shift = t === 1 ? 6 : subdivideSegment(segment, i, t); + + if ((shift || !t) && shift + i + 2 < segment.length) { + rawPath.splice(segIndex, 0, segment.slice(0, i + shift + 2)); + segment.splice(0, i + shift); + return 1; + } +}, + _getSampleIndex = function _getSampleIndex(samples, length, progress) { + // slightly slower way than doing this (when there's no lookup): segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0; + var l = samples.length, + i = ~~(progress * l); + + if (samples[i] > length) { + while (--i && samples[i] > length) {} + + i < 0 && (i = 0); + } else { + while (samples[++i] < length && i < l) {} + } + + return i < l ? i : l - 1; +}, + _reverseRawPath = function _reverseRawPath(rawPath, skipOuter) { + var i = rawPath.length; + skipOuter || rawPath.reverse(); + + while (i--) { + rawPath[i].reversed || reverseSegment(rawPath[i]); + } +}, + _copyMetaData = function _copyMetaData(source, copy) { + copy.totalLength = source.totalLength; + + if (source.samples) { + //segment + copy.samples = source.samples.slice(0); + copy.lookup = source.lookup.slice(0); + copy.minLength = source.minLength; + copy.resolution = source.resolution; + } else if (source.totalPoints) { + //rawPath + copy.totalPoints = source.totalPoints; + } + + return copy; +}, + //pushes a new segment into a rawPath, but if its starting values match the ending values of the last segment, it'll merge it into that same segment (to reduce the number of segments) +_appendOrMerge = function _appendOrMerge(rawPath, segment) { + var index = rawPath.length, + prevSeg = rawPath[index - 1] || [], + l = prevSeg.length; + + if (index && segment[0] === prevSeg[l - 2] && segment[1] === prevSeg[l - 1]) { + segment = prevSeg.concat(segment.slice(2)); + index--; + } + + rawPath[index] = segment; +}, + _bestDistance; +/* TERMINOLOGY + - RawPath - an array of arrays, one for each Segment. A single RawPath could have multiple "M" commands, defining Segments (paths aren't always connected). + - Segment - an array containing a sequence of Cubic Bezier coordinates in alternating x, y, x, y format. Starting anchor, then control point 1, control point 2, and ending anchor, then the next control point 1, control point 2, anchor, etc. Uses less memory than an array with a bunch of {x, y} points. + - Bezier - a single cubic Bezier with a starting anchor, two control points, and an ending anchor. + - the variable "t" is typically the position along an individual Bezier path (time) and it's NOT linear, meaning it could accelerate/decelerate based on the control points whereas the "p" or "progress" value is linearly mapped to the whole path, so it shouldn't really accelerate/decelerate based on control points. So a progress of 0.2 would be almost exactly 20% along the path. "t" is ONLY in an individual Bezier piece. + */ +//accepts basic selector text, a path instance, a RawPath instance, or a Segment and returns a RawPath (makes it easy to homogenize things). If an element or selector text is passed in, it'll also cache the value so that if it's queried again, it'll just take the path data from there instead of parsing it all over again (as long as the path data itself hasn't changed - it'll check). + + +export function getRawPath(value) { + value = _isString(value) && _selectorExp.test(value) ? document.querySelector(value) || value : value; + var e = value.getAttribute ? value : 0, + rawPath; + + if (e && (value = value.getAttribute("d"))) { + //implements caching + if (!e._gsPath) { + e._gsPath = {}; + } + + rawPath = e._gsPath[value]; + return rawPath && !rawPath._dirty ? rawPath : e._gsPath[value] = stringToRawPath(value); + } + + return !value ? console.warn("Expecting a <path> element or an SVG path data string") : _isString(value) ? stringToRawPath(value) : _isNumber(value[0]) ? [value] : value; +} //copies a RawPath WITHOUT the length meta data (for speed) + +export function copyRawPath(rawPath) { + var a = [], + i = 0; + + for (; i < rawPath.length; i++) { + a[i] = _copyMetaData(rawPath[i], rawPath[i].slice(0)); + } + + return _copyMetaData(rawPath, a); +} +export function reverseSegment(segment) { + var i = 0, + y; + segment.reverse(); //this will invert the order y, x, y, x so we must flip it back. + + for (; i < segment.length; i += 2) { + y = segment[i]; + segment[i] = segment[i + 1]; + segment[i + 1] = y; + } + + segment.reversed = !segment.reversed; +} + +var _createPath = function _createPath(e, ignore) { + var path = document.createElementNS("http://www.w3.org/2000/svg", "path"), + attr = [].slice.call(e.attributes), + i = attr.length, + name; + ignore = "," + ignore + ","; + + while (--i > -1) { + name = attr[i].nodeName.toLowerCase(); //in Microsoft Edge, if you don't set the attribute with a lowercase name, it doesn't render correctly! Super weird. + + if (ignore.indexOf("," + name + ",") < 0) { + path.setAttributeNS(null, name, attr[i].nodeValue); + } + } + + return path; +}, + _typeAttrs = { + rect: "rx,ry,x,y,width,height", + circle: "r,cx,cy", + ellipse: "rx,ry,cx,cy", + line: "x1,x2,y1,y2" +}, + _attrToObj = function _attrToObj(e, attrs) { + var props = attrs ? attrs.split(",") : [], + obj = {}, + i = props.length; + + while (--i > -1) { + obj[props[i]] = +e.getAttribute(props[i]) || 0; + } + + return obj; +}; //converts an SVG shape like <circle>, <rect>, <polygon>, <polyline>, <ellipse>, etc. to a <path>, swapping it in and copying the attributes to match. + + +export function convertToPath(element, swap) { + var type = element.tagName.toLowerCase(), + circ = 0.552284749831, + data, + x, + y, + r, + ry, + path, + rcirc, + rycirc, + points, + w, + h, + x2, + x3, + x4, + x5, + x6, + y2, + y3, + y4, + y5, + y6, + attr; + + if (type === "path" || !element.getBBox) { + return element; + } + + path = _createPath(element, "x,y,width,height,cx,cy,rx,ry,r,x1,x2,y1,y2,points"); + attr = _attrToObj(element, _typeAttrs[type]); + + if (type === "rect") { + r = attr.rx; + ry = attr.ry || r; + x = attr.x; + y = attr.y; + w = attr.width - r * 2; + h = attr.height - ry * 2; + + if (r || ry) { + //if there are rounded corners, render cubic beziers + x2 = x + r * (1 - circ); + x3 = x + r; + x4 = x3 + w; + x5 = x4 + r * circ; + x6 = x4 + r; + y2 = y + ry * (1 - circ); + y3 = y + ry; + y4 = y3 + h; + y5 = y4 + ry * circ; + y6 = y4 + ry; + data = "M" + x6 + "," + y3 + " V" + y4 + " C" + [x6, y5, x5, y6, x4, y6, x4 - (x4 - x3) / 3, y6, x3 + (x4 - x3) / 3, y6, x3, y6, x2, y6, x, y5, x, y4, x, y4 - (y4 - y3) / 3, x, y3 + (y4 - y3) / 3, x, y3, x, y2, x2, y, x3, y, x3 + (x4 - x3) / 3, y, x4 - (x4 - x3) / 3, y, x4, y, x5, y, x6, y2, x6, y3].join(",") + "z"; + } else { + data = "M" + (x + w) + "," + y + " v" + h + " h" + -w + " v" + -h + " h" + w + "z"; + } + } else if (type === "circle" || type === "ellipse") { + if (type === "circle") { + r = ry = attr.r; + rycirc = r * circ; + } else { + r = attr.rx; + ry = attr.ry; + rycirc = ry * circ; + } + + x = attr.cx; + y = attr.cy; + rcirc = r * circ; + data = "M" + (x + r) + "," + y + " C" + [x + r, y + rycirc, x + rcirc, y + ry, x, y + ry, x - rcirc, y + ry, x - r, y + rycirc, x - r, y, x - r, y - rycirc, x - rcirc, y - ry, x, y - ry, x + rcirc, y - ry, x + r, y - rycirc, x + r, y].join(",") + "z"; + } else if (type === "line") { + data = "M" + attr.x1 + "," + attr.y1 + " L" + attr.x2 + "," + attr.y2; //previously, we just converted to "Mx,y Lx,y" but Safari has bugs that cause that not to render properly when using a stroke-dasharray that's not fully visible! Using a cubic bezier fixes that issue. + } else if (type === "polyline" || type === "polygon") { + points = (element.getAttribute("points") + "").match(_numbersExp) || []; + x = points.shift(); + y = points.shift(); + data = "M" + x + "," + y + " L" + points.join(","); + + if (type === "polygon") { + data += "," + x + "," + y + "z"; + } + } + + path.setAttribute("d", rawPathToString(path._gsRawPath = stringToRawPath(data))); + + if (swap && element.parentNode) { + element.parentNode.insertBefore(path, element); + element.parentNode.removeChild(element); + } + + return path; +} //returns the rotation (in degrees) at a particular progress on a rawPath (the slope of the tangent) + +export function getRotationAtProgress(rawPath, progress) { + var d = getProgressData(rawPath, progress >= 1 ? 1 - 1e-9 : progress ? progress : 1e-9); + return getRotationAtBezierT(d.segment, d.i, d.t); +} + +function getRotationAtBezierT(segment, i, t) { + var a = segment[i], + b = segment[i + 2], + c = segment[i + 4], + x; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + x = b + (c + (segment[i + 6] - c) * t - b) * t - a; + a = segment[i + 1]; + b = segment[i + 3]; + c = segment[i + 5]; + a += (b - a) * t; + b += (c - b) * t; + a += (b - a) * t; + return _round(_atan2(b + (c + (segment[i + 7] - c) * t - b) * t - a, x) * _RAD2DEG); +} + +export function sliceRawPath(rawPath, start, end) { + end = _isUndefined(end) ? 1 : _roundPrecise(end) || 0; // we must round to avoid issues like 4.15 / 8 = 0.8300000000000001 instead of 0.83 or 2.8 / 5 = 0.5599999999999999 instead of 0.56 and if someone is doing a loop like start: 2.8 / 0.5, end: 2.8 / 0.5 + 1. + + start = _roundPrecise(start) || 0; + var loops = Math.max(0, ~~(_abs(end - start) - 1e-8)), + path = copyRawPath(rawPath); + + if (start > end) { + start = 1 - start; + end = 1 - end; + + _reverseRawPath(path); + + path.totalLength = 0; + } + + if (start < 0 || end < 0) { + var offset = Math.abs(~~Math.min(start, end)) + 1; + start += offset; + end += offset; + } + + path.totalLength || cacheRawPathMeasurements(path); + var wrap = end > 1, + s = getProgressData(path, start, _temp, true), + e = getProgressData(path, end, _temp2), + eSeg = e.segment, + sSeg = s.segment, + eSegIndex = e.segIndex, + sSegIndex = s.segIndex, + ei = e.i, + si = s.i, + sameSegment = sSegIndex === eSegIndex, + sameBezier = ei === si && sameSegment, + wrapsBehind, + sShift, + eShift, + i, + copy, + totalSegments, + l, + j; + + if (wrap || loops) { + wrapsBehind = eSegIndex < sSegIndex || sameSegment && ei < si || sameBezier && e.t < s.t; + + if (_splitSegment(path, sSegIndex, si, s.t)) { + sSegIndex++; + + if (!wrapsBehind) { + eSegIndex++; + + if (sameBezier) { + e.t = (e.t - s.t) / (1 - s.t); + ei = 0; + } else if (sameSegment) { + ei -= si; + } + } + } + + if (Math.abs(1 - (end - start)) < 1e-5) { + eSegIndex = sSegIndex - 1; + } else if (!e.t && eSegIndex) { + eSegIndex--; + } else if (_splitSegment(path, eSegIndex, ei, e.t) && wrapsBehind) { + sSegIndex++; + } + + if (s.t === 1) { + sSegIndex = (sSegIndex + 1) % path.length; + } + + copy = []; + totalSegments = path.length; + l = 1 + totalSegments * loops; + j = sSegIndex; + l += (totalSegments - sSegIndex + eSegIndex) % totalSegments; + + for (i = 0; i < l; i++) { + _appendOrMerge(copy, path[j++ % totalSegments]); + } + + path = copy; + } else { + eShift = e.t === 1 ? 6 : subdivideSegment(eSeg, ei, e.t); + + if (start !== end) { + sShift = subdivideSegment(sSeg, si, sameBezier ? s.t / e.t : s.t); + sameSegment && (eShift += sShift); + eSeg.splice(ei + eShift + 2); + (sShift || si) && sSeg.splice(0, si + sShift); + i = path.length; + + while (i--) { + //chop off any extra segments + (i < sSegIndex || i > eSegIndex) && path.splice(i, 1); + } + } else { + eSeg.angle = getRotationAtBezierT(eSeg, ei + eShift, 0); //record the value before we chop because it'll be impossible to determine the angle after its length is 0! + + ei += eShift; + s = eSeg[ei]; + e = eSeg[ei + 1]; + eSeg.length = eSeg.totalLength = 0; + eSeg.totalPoints = path.totalPoints = 8; + eSeg.push(s, e, s, e, s, e, s, e); + } + } + + path.totalLength = 0; + return path; +} //measures a Segment according to its resolution (so if segment.resolution is 6, for example, it'll take 6 samples equally across each Bezier) and create/populate a "samples" Array that has the length up to each of those sample points (always increasing from the start) as well as a "lookup" array that's broken up according to the smallest distance between 2 samples. This gives us a very fast way of looking up a progress position rather than looping through all the points/Beziers. You can optionally have it only measure a subset, starting at startIndex and going for a specific number of beziers (remember, there are 3 x/y pairs each, for a total of 6 elements for each Bezier). It will also populate a "totalLength" property, but that's not generally super accurate because by default it'll only take 6 samples per Bezier. But for performance reasons, it's perfectly adequate for measuring progress values along the path. If you need a more accurate totalLength, either increase the resolution or use the more advanced bezierToPoints() method which keeps adding points until they don't deviate by more than a certain precision value. + +function measureSegment(segment, startIndex, bezierQty) { + startIndex = startIndex || 0; + + if (!segment.samples) { + segment.samples = []; + segment.lookup = []; + } + + var resolution = ~~segment.resolution || 12, + inc = 1 / resolution, + endIndex = bezierQty ? startIndex + bezierQty * 6 + 1 : segment.length, + x1 = segment[startIndex], + y1 = segment[startIndex + 1], + samplesIndex = startIndex ? startIndex / 6 * resolution : 0, + samples = segment.samples, + lookup = segment.lookup, + min = (startIndex ? segment.minLength : _largeNum) || _largeNum, + prevLength = samples[samplesIndex + bezierQty * resolution - 1], + length = startIndex ? samples[samplesIndex - 1] : 0, + i, + j, + x4, + x3, + x2, + xd, + xd1, + y4, + y3, + y2, + yd, + yd1, + inv, + t, + lengthIndex, + l, + segLength; + samples.length = lookup.length = 0; + + for (j = startIndex + 2; j < endIndex; j += 6) { + x4 = segment[j + 4] - x1; + x3 = segment[j + 2] - x1; + x2 = segment[j] - x1; + y4 = segment[j + 5] - y1; + y3 = segment[j + 3] - y1; + y2 = segment[j + 1] - y1; + xd = xd1 = yd = yd1 = 0; + + if (_abs(x4) < .01 && _abs(y4) < .01 && _abs(x2) + _abs(y2) < .01) { + //dump points that are sufficiently close (basically right on top of each other, making a bezier super tiny or 0 length) + if (segment.length > 8) { + segment.splice(j, 6); + j -= 6; + endIndex -= 6; + } + } else { + for (i = 1; i <= resolution; i++) { + t = inc * i; + inv = 1 - t; + xd = xd1 - (xd1 = (t * t * x4 + 3 * inv * (t * x3 + inv * x2)) * t); + yd = yd1 - (yd1 = (t * t * y4 + 3 * inv * (t * y3 + inv * y2)) * t); + l = _sqrt(yd * yd + xd * xd); + + if (l < min) { + min = l; + } + + length += l; + samples[samplesIndex++] = length; + } + } + + x1 += x4; + y1 += y4; + } + + if (prevLength) { + prevLength -= length; + + for (; samplesIndex < samples.length; samplesIndex++) { + samples[samplesIndex] += prevLength; + } + } + + if (samples.length && min) { + segment.totalLength = segLength = samples[samples.length - 1] || 0; + segment.minLength = min; + + if (segLength / min < 9999) { + // if the lookup would require too many values (memory problem), we skip this and instead we use a loop to lookup values directly in the samples Array + l = lengthIndex = 0; + + for (i = 0; i < segLength; i += min) { + lookup[l++] = samples[lengthIndex] < i ? ++lengthIndex : lengthIndex; + } + } + } else { + segment.totalLength = samples[0] = 0; + } + + return startIndex ? length - samples[startIndex / 2 - 1] : length; +} + +export function cacheRawPathMeasurements(rawPath, resolution) { + var pathLength, points, i; + + for (i = pathLength = points = 0; i < rawPath.length; i++) { + rawPath[i].resolution = ~~resolution || 12; //steps per Bezier curve (anchor, 2 control points, to anchor) + + points += rawPath[i].length; + pathLength += measureSegment(rawPath[i]); + } + + rawPath.totalPoints = points; + rawPath.totalLength = pathLength; + return rawPath; +} //divide segment[i] at position t (value between 0 and 1, progress along that particular cubic bezier segment that starts at segment[i]). Returns how many elements were spliced into the segment array (either 0 or 6) + +export function subdivideSegment(segment, i, t) { + if (t <= 0 || t >= 1) { + return 0; + } + + var ax = segment[i], + ay = segment[i + 1], + cp1x = segment[i + 2], + cp1y = segment[i + 3], + cp2x = segment[i + 4], + cp2y = segment[i + 5], + bx = segment[i + 6], + by = segment[i + 7], + x1a = ax + (cp1x - ax) * t, + x2 = cp1x + (cp2x - cp1x) * t, + y1a = ay + (cp1y - ay) * t, + y2 = cp1y + (cp2y - cp1y) * t, + x1 = x1a + (x2 - x1a) * t, + y1 = y1a + (y2 - y1a) * t, + x2a = cp2x + (bx - cp2x) * t, + y2a = cp2y + (by - cp2y) * t; + x2 += (x2a - x2) * t; + y2 += (y2a - y2) * t; + segment.splice(i + 2, 4, _round(x1a), //first control point + _round(y1a), _round(x1), //second control point + _round(y1), _round(x1 + (x2 - x1) * t), //new fabricated anchor on line + _round(y1 + (y2 - y1) * t), _round(x2), //third control point + _round(y2), _round(x2a), //fourth control point + _round(y2a)); + segment.samples && segment.samples.splice(i / 6 * segment.resolution | 0, 0, 0, 0, 0, 0, 0, 0); + return 6; +} // returns an object {path, segment, segIndex, i, t} + +function getProgressData(rawPath, progress, decoratee, pushToNextIfAtEnd) { + decoratee = decoratee || {}; + rawPath.totalLength || cacheRawPathMeasurements(rawPath); + + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + + var segIndex = 0, + segment = rawPath[0], + samples, + resolution, + length, + min, + max, + i, + t; + + if (!progress) { + t = i = segIndex = 0; + segment = rawPath[0]; + } else if (progress === 1) { + t = 1; + segIndex = rawPath.length - 1; + segment = rawPath[segIndex]; + i = segment.length - 8; + } else { + if (rawPath.length > 1) { + //speed optimization: most of the time, there's only one segment so skip the recursion. + length = rawPath.totalLength * progress; + max = i = 0; + + while ((max += rawPath[i++].totalLength) < length) { + segIndex = i; + } + + segment = rawPath[segIndex]; + min = max - segment.totalLength; + progress = (length - min) / (max - min) || 0; + } + + samples = segment.samples; + resolution = segment.resolution; //how many samples per cubic bezier chunk + + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[~~(length / segment.minLength)] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i - 1] : 0; + max = samples[i]; + + if (max < length) { + min = max; + max = samples[++i]; + } + + t = 1 / resolution * ((length - min) / (max - min) + i % resolution); + i = ~~(i / resolution) * 6; + + if (pushToNextIfAtEnd && t === 1) { + if (i + 6 < segment.length) { + i += 6; + t = 0; + } else if (segIndex + 1 < rawPath.length) { + i = t = 0; + segment = rawPath[++segIndex]; + } + } + } + + decoratee.t = t; + decoratee.i = i; + decoratee.path = rawPath; + decoratee.segment = segment; + decoratee.segIndex = segIndex; + return decoratee; +} + +export function getPositionOnPath(rawPath, progress, includeAngle, point) { + var segment = rawPath[0], + result = point || {}, + samples, + resolution, + length, + min, + max, + i, + t, + a, + inv; + + if (progress < 0 || progress > 1) { + progress = _wrapProgress(progress); + } + + segment.lookup || cacheRawPathMeasurements(rawPath); + + if (rawPath.length > 1) { + //speed optimization: most of the time, there's only one segment so skip the recursion. + length = rawPath.totalLength * progress; + max = i = 0; + + while ((max += rawPath[i++].totalLength) < length) { + segment = rawPath[i]; + } + + min = max - segment.totalLength; + progress = (length - min) / (max - min) || 0; + } + + samples = segment.samples; + resolution = segment.resolution; + length = segment.totalLength * progress; + i = segment.lookup.length ? segment.lookup[progress < 1 ? ~~(length / segment.minLength) : segment.lookup.length - 1] || 0 : _getSampleIndex(samples, length, progress); + min = i ? samples[i - 1] : 0; + max = samples[i]; + + if (max < length) { + min = max; + max = samples[++i]; + } + + t = 1 / resolution * ((length - min) / (max - min) + i % resolution) || 0; + inv = 1 - t; + i = ~~(i / resolution) * 6; + a = segment[i]; + result.x = _round((t * t * (segment[i + 6] - a) + 3 * inv * (t * (segment[i + 4] - a) + inv * (segment[i + 2] - a))) * t + a); + result.y = _round((t * t * (segment[i + 7] - (a = segment[i + 1])) + 3 * inv * (t * (segment[i + 5] - a) + inv * (segment[i + 3] - a))) * t + a); + + if (includeAngle) { + result.angle = segment.totalLength ? getRotationAtBezierT(segment, i, t >= 1 ? 1 - 1e-9 : t ? t : 1e-9) : segment.angle || 0; + } + + return result; +} //applies a matrix transform to RawPath (or a segment in a RawPath) and returns whatever was passed in (it transforms the values in the array(s), not a copy). + +export function transformRawPath(rawPath, a, b, c, d, tx, ty) { + var j = rawPath.length, + segment, + l, + i, + x, + y; + + while (--j > -1) { + segment = rawPath[j]; + l = segment.length; + + for (i = 0; i < l; i += 2) { + x = segment[i]; + y = segment[i + 1]; + segment[i] = x * a + y * c + tx; + segment[i + 1] = x * b + y * d + ty; + } + } + + rawPath._dirty = 1; + return rawPath; +} // translates SVG arc data into a segment (cubic beziers). Angle is in degrees. + +function arcToSegment(lastX, lastY, rx, ry, angle, largeArcFlag, sweepFlag, x, y) { + if (lastX === x && lastY === y) { + return; + } + + rx = _abs(rx); + ry = _abs(ry); + + var angleRad = angle % 360 * _DEG2RAD, + cosAngle = _cos(angleRad), + sinAngle = _sin(angleRad), + PI = Math.PI, + TWOPI = PI * 2, + dx2 = (lastX - x) / 2, + dy2 = (lastY - y) / 2, + x1 = cosAngle * dx2 + sinAngle * dy2, + y1 = -sinAngle * dx2 + cosAngle * dy2, + x1_sq = x1 * x1, + y1_sq = y1 * y1, + radiiCheck = x1_sq / (rx * rx) + y1_sq / (ry * ry); + + if (radiiCheck > 1) { + rx = _sqrt(radiiCheck) * rx; + ry = _sqrt(radiiCheck) * ry; + } + + var rx_sq = rx * rx, + ry_sq = ry * ry, + sq = (rx_sq * ry_sq - rx_sq * y1_sq - ry_sq * x1_sq) / (rx_sq * y1_sq + ry_sq * x1_sq); + + if (sq < 0) { + sq = 0; + } + + var coef = (largeArcFlag === sweepFlag ? -1 : 1) * _sqrt(sq), + cx1 = coef * (rx * y1 / ry), + cy1 = coef * -(ry * x1 / rx), + sx2 = (lastX + x) / 2, + sy2 = (lastY + y) / 2, + cx = sx2 + (cosAngle * cx1 - sinAngle * cy1), + cy = sy2 + (sinAngle * cx1 + cosAngle * cy1), + ux = (x1 - cx1) / rx, + uy = (y1 - cy1) / ry, + vx = (-x1 - cx1) / rx, + vy = (-y1 - cy1) / ry, + temp = ux * ux + uy * uy, + angleStart = (uy < 0 ? -1 : 1) * Math.acos(ux / _sqrt(temp)), + angleExtent = (ux * vy - uy * vx < 0 ? -1 : 1) * Math.acos((ux * vx + uy * vy) / _sqrt(temp * (vx * vx + vy * vy))); + + isNaN(angleExtent) && (angleExtent = PI); //rare edge case. Math.cos(-1) is NaN. + + if (!sweepFlag && angleExtent > 0) { + angleExtent -= TWOPI; + } else if (sweepFlag && angleExtent < 0) { + angleExtent += TWOPI; + } + + angleStart %= TWOPI; + angleExtent %= TWOPI; + + var segments = Math.ceil(_abs(angleExtent) / (TWOPI / 4)), + rawPath = [], + angleIncrement = angleExtent / segments, + controlLength = 4 / 3 * _sin(angleIncrement / 2) / (1 + _cos(angleIncrement / 2)), + ma = cosAngle * rx, + mb = sinAngle * rx, + mc = sinAngle * -ry, + md = cosAngle * ry, + i; + + for (i = 0; i < segments; i++) { + angle = angleStart + i * angleIncrement; + x1 = _cos(angle); + y1 = _sin(angle); + ux = _cos(angle += angleIncrement); + uy = _sin(angle); + rawPath.push(x1 - controlLength * y1, y1 + controlLength * x1, ux + controlLength * uy, uy - controlLength * ux, ux, uy); + } //now transform according to the actual size of the ellipse/arc (the beziers were noramlized, between 0 and 1 on a circle). + + + for (i = 0; i < rawPath.length; i += 2) { + x1 = rawPath[i]; + y1 = rawPath[i + 1]; + rawPath[i] = x1 * ma + y1 * mc + cx; + rawPath[i + 1] = x1 * mb + y1 * md + cy; + } + + rawPath[i - 2] = x; //always set the end to exactly where it's supposed to be + + rawPath[i - 1] = y; + return rawPath; +} //Spits back a RawPath with absolute coordinates. Each segment starts with a "moveTo" command (x coordinate, then y) and then 2 control points (x, y, x, y), then anchor. The goal is to minimize memory and maximize speed. + + +export function stringToRawPath(d) { + var a = (d + "").replace(_scientific, function (m) { + var n = +m; + return n < 0.0001 && n > -0.0001 ? 0 : n; + }).match(_svgPathExp) || [], + //some authoring programs spit out very small numbers in scientific notation like "1e-5", so make sure we round that down to 0 first. + path = [], + relativeX = 0, + relativeY = 0, + twoThirds = 2 / 3, + elements = a.length, + points = 0, + errorMessage = "ERROR: malformed path: " + d, + i, + j, + x, + y, + command, + isRelative, + segment, + startX, + startY, + difX, + difY, + beziers, + prevCommand, + flag1, + flag2, + line = function line(sx, sy, ex, ey) { + difX = (ex - sx) / 3; + difY = (ey - sy) / 3; + segment.push(sx + difX, sy + difY, ex - difX, ey - difY, ex, ey); + }; + + if (!d || !isNaN(a[0]) || isNaN(a[1])) { + console.log(errorMessage); + return path; + } + + for (i = 0; i < elements; i++) { + prevCommand = command; + + if (isNaN(a[i])) { + command = a[i].toUpperCase(); + isRelative = command !== a[i]; //lower case means relative + } else { + //commands like "C" can be strung together without any new command characters between. + i--; + } + + x = +a[i + 1]; + y = +a[i + 2]; + + if (isRelative) { + x += relativeX; + y += relativeY; + } + + if (!i) { + startX = x; + startY = y; + } // "M" (move) + + + if (command === "M") { + if (segment) { + if (segment.length < 8) { + //if the path data was funky and just had a M with no actual drawing anywhere, skip it. + path.length -= 1; + } else { + points += segment.length; + } + } + + relativeX = startX = x; + relativeY = startY = y; + segment = [x, y]; + path.push(segment); + i += 2; + command = "L"; //an "M" with more than 2 values gets interpreted as "lineTo" commands ("L"). + // "C" (cubic bezier) + } else if (command === "C") { + if (!segment) { + segment = [0, 0]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } //note: "*1" is just a fast/short way to cast the value as a Number. WAAAY faster in Chrome, slightly slower in Firefox. + + + segment.push(x, y, relativeX + a[i + 3] * 1, relativeY + a[i + 4] * 1, relativeX += a[i + 5] * 1, relativeY += a[i + 6] * 1); + i += 6; // "S" (continuation of cubic bezier) + } else if (command === "S") { + difX = relativeX; + difY = relativeY; + + if (prevCommand === "C" || prevCommand === "S") { + difX += relativeX - segment[segment.length - 4]; + difY += relativeY - segment[segment.length - 3]; + } + + if (!isRelative) { + relativeX = relativeY = 0; + } + + segment.push(difX, difY, x, y, relativeX += a[i + 3] * 1, relativeY += a[i + 4] * 1); + i += 4; // "Q" (quadratic bezier) + } else if (command === "Q") { + difX = relativeX + (x - relativeX) * twoThirds; + difY = relativeY + (y - relativeY) * twoThirds; + + if (!isRelative) { + relativeX = relativeY = 0; + } + + relativeX += a[i + 3] * 1; + relativeY += a[i + 4] * 1; + segment.push(difX, difY, relativeX + (x - relativeX) * twoThirds, relativeY + (y - relativeY) * twoThirds, relativeX, relativeY); + i += 4; // "T" (continuation of quadratic bezier) + } else if (command === "T") { + difX = relativeX - segment[segment.length - 4]; + difY = relativeY - segment[segment.length - 3]; + segment.push(relativeX + difX, relativeY + difY, x + (relativeX + difX * 1.5 - x) * twoThirds, y + (relativeY + difY * 1.5 - y) * twoThirds, relativeX = x, relativeY = y); + i += 2; // "H" (horizontal line) + } else if (command === "H") { + line(relativeX, relativeY, relativeX = x, relativeY); + i += 1; // "V" (vertical line) + } else if (command === "V") { + //adjust values because the first (and only one) isn't x in this case, it's y. + line(relativeX, relativeY, relativeX, relativeY = x + (isRelative ? relativeY - relativeX : 0)); + i += 1; // "L" (line) or "Z" (close) + } else if (command === "L" || command === "Z") { + if (command === "Z") { + x = startX; + y = startY; + segment.closed = true; + } + + if (command === "L" || _abs(relativeX - x) > 0.5 || _abs(relativeY - y) > 0.5) { + line(relativeX, relativeY, x, y); + + if (command === "L") { + i += 2; + } + } + + relativeX = x; + relativeY = y; // "A" (arc) + } else if (command === "A") { + flag1 = a[i + 4]; + flag2 = a[i + 5]; + difX = a[i + 6]; + difY = a[i + 7]; + j = 7; + + if (flag1.length > 1) { + // for cases when the flags are merged, like "a8 8 0 018 8" (the 0 and 1 flags are WITH the x value of 8, but it could also be "a8 8 0 01-8 8" so it may include x or not) + if (flag1.length < 3) { + difY = difX; + difX = flag2; + j--; + } else { + difY = flag2; + difX = flag1.substr(2); + j -= 2; + } + + flag2 = flag1.charAt(1); + flag1 = flag1.charAt(0); + } + + beziers = arcToSegment(relativeX, relativeY, +a[i + 1], +a[i + 2], +a[i + 3], +flag1, +flag2, (isRelative ? relativeX : 0) + difX * 1, (isRelative ? relativeY : 0) + difY * 1); + i += j; + + if (beziers) { + for (j = 0; j < beziers.length; j++) { + segment.push(beziers[j]); + } + } + + relativeX = segment[segment.length - 2]; + relativeY = segment[segment.length - 1]; + } else { + console.log(errorMessage); + } + } + + i = segment.length; + + if (i < 6) { + //in case there's odd SVG like a M0,0 command at the very end. + path.pop(); + i = 0; + } else if (segment[0] === segment[i - 2] && segment[1] === segment[i - 1]) { + segment.closed = true; + } + + path.totalPoints = points + i; + return path; +} //populates the points array in alternating x/y values (like [x, y, x, y...] instead of individual point objects [{x, y}, {x, y}...] to conserve memory and stay in line with how we're handling segment arrays + +export function bezierToPoints(x1, y1, x2, y2, x3, y3, x4, y4, threshold, points, index) { + var x12 = (x1 + x2) / 2, + y12 = (y1 + y2) / 2, + x23 = (x2 + x3) / 2, + y23 = (y2 + y3) / 2, + x34 = (x3 + x4) / 2, + y34 = (y3 + y4) / 2, + x123 = (x12 + x23) / 2, + y123 = (y12 + y23) / 2, + x234 = (x23 + x34) / 2, + y234 = (y23 + y34) / 2, + x1234 = (x123 + x234) / 2, + y1234 = (y123 + y234) / 2, + dx = x4 - x1, + dy = y4 - y1, + d2 = _abs((x2 - x4) * dy - (y2 - y4) * dx), + d3 = _abs((x3 - x4) * dy - (y3 - y4) * dx), + length; + + if (!points) { + points = [x1, y1, x4, y4]; + index = 2; + } + + points.splice(index || points.length - 2, 0, x1234, y1234); + + if ((d2 + d3) * (d2 + d3) > threshold * (dx * dx + dy * dy)) { + length = points.length; + bezierToPoints(x1, y1, x12, y12, x123, y123, x1234, y1234, threshold, points, index); + bezierToPoints(x1234, y1234, x234, y234, x34, y34, x4, y4, threshold, points, index + 2 + (points.length - length)); + } + + return points; +} +/* +function getAngleBetweenPoints(x0, y0, x1, y1, x2, y2) { //angle between 3 points in radians + var dx1 = x1 - x0, + dy1 = y1 - y0, + dx2 = x2 - x1, + dy2 = y2 - y1, + dx3 = x2 - x0, + dy3 = y2 - y0, + a = dx1 * dx1 + dy1 * dy1, + b = dx2 * dx2 + dy2 * dy2, + c = dx3 * dx3 + dy3 * dy3; + return Math.acos( (a + b - c) / _sqrt(4 * a * b) ); +}, +*/ +//pointsToSegment() doesn't handle flat coordinates (where y is always 0) the way we need (the resulting control points are always right on top of the anchors), so this function basically makes the control points go directly up and down, varying in length based on the curviness (more curvy, further control points) + +export function flatPointsToSegment(points, curviness) { + if (curviness === void 0) { + curviness = 1; + } + + var x = points[0], + y = 0, + segment = [x, y], + i = 2; + + for (; i < points.length; i += 2) { + segment.push(x, y, points[i], y = (points[i] - x) * curviness / 2, x = points[i], -y); + } + + return segment; +} //points is an array of x/y points, like [x, y, x, y, x, y] + +export function pointsToSegment(points, curviness) { + //points = simplifyPoints(points, tolerance); + _abs(points[0] - points[2]) < 1e-4 && _abs(points[1] - points[3]) < 1e-4 && (points = points.slice(2)); // if the first two points are super close, dump the first one. + + var l = points.length - 2, + x = +points[0], + y = +points[1], + nextX = +points[2], + nextY = +points[3], + segment = [x, y, x, y], + dx2 = nextX - x, + dy2 = nextY - y, + closed = Math.abs(points[l] - x) < 0.001 && Math.abs(points[l + 1] - y) < 0.001, + prevX, + prevY, + i, + dx1, + dy1, + r1, + r2, + r3, + tl, + mx1, + mx2, + mxm, + my1, + my2, + mym; + + if (closed) { + // if the start and end points are basically on top of each other, close the segment by adding the 2nd point to the end, and the 2nd-to-last point to the beginning (we'll remove them at the end, but this allows the curvature to look perfect) + points.push(nextX, nextY); + nextX = x; + nextY = y; + x = points[l - 2]; + y = points[l - 1]; + points.unshift(x, y); + l += 4; + } + + curviness = curviness || curviness === 0 ? +curviness : 1; + + for (i = 2; i < l; i += 2) { + prevX = x; + prevY = y; + x = nextX; + y = nextY; + nextX = +points[i + 2]; + nextY = +points[i + 3]; + + if (x === nextX && y === nextY) { + continue; + } + + dx1 = dx2; + dy1 = dy2; + dx2 = nextX - x; + dy2 = nextY - y; + r1 = _sqrt(dx1 * dx1 + dy1 * dy1); // r1, r2, and r3 correlate x and y (and z in the future). Basically 2D or 3D hypotenuse + + r2 = _sqrt(dx2 * dx2 + dy2 * dy2); + r3 = _sqrt(Math.pow(dx2 / r2 + dx1 / r1, 2) + Math.pow(dy2 / r2 + dy1 / r1, 2)); + tl = (r1 + r2) * curviness * 0.25 / r3; + mx1 = x - (x - prevX) * (r1 ? tl / r1 : 0); + mx2 = x + (nextX - x) * (r2 ? tl / r2 : 0); + mxm = x - (mx1 + ((mx2 - mx1) * (r1 * 3 / (r1 + r2) + 0.5) / 4 || 0)); + my1 = y - (y - prevY) * (r1 ? tl / r1 : 0); + my2 = y + (nextY - y) * (r2 ? tl / r2 : 0); + mym = y - (my1 + ((my2 - my1) * (r1 * 3 / (r1 + r2) + 0.5) / 4 || 0)); + + if (x !== prevX || y !== prevY) { + segment.push(_round(mx1 + mxm), // first control point + _round(my1 + mym), _round(x), // anchor + _round(y), _round(mx2 + mxm), // second control point + _round(my2 + mym)); + } + } + + x !== nextX || y !== nextY || segment.length < 4 ? segment.push(_round(nextX), _round(nextY), _round(nextX), _round(nextY)) : segment.length -= 2; + + if (segment.length === 2) { + // only one point! + segment.push(x, y, x, y, x, y); + } else if (closed) { + segment.splice(0, 6); + segment.length = segment.length - 6; + } + + return segment; +} //returns the squared distance between an x/y coordinate and a segment between x1/y1 and x2/y2 + +function pointToSegDist(x, y, x1, y1, x2, y2) { + var dx = x2 - x1, + dy = y2 - y1, + t; + + if (dx || dy) { + t = ((x - x1) * dx + (y - y1) * dy) / (dx * dx + dy * dy); + + if (t > 1) { + x1 = x2; + y1 = y2; + } else if (t > 0) { + x1 += dx * t; + y1 += dy * t; + } + } + + return Math.pow(x - x1, 2) + Math.pow(y - y1, 2); +} + +function simplifyStep(points, first, last, tolerance, simplified) { + var maxSqDist = tolerance, + firstX = points[first], + firstY = points[first + 1], + lastX = points[last], + lastY = points[last + 1], + index, + i, + d; + + for (i = first + 2; i < last; i += 2) { + d = pointToSegDist(points[i], points[i + 1], firstX, firstY, lastX, lastY); + + if (d > maxSqDist) { + index = i; + maxSqDist = d; + } + } + + if (maxSqDist > tolerance) { + index - first > 2 && simplifyStep(points, first, index, tolerance, simplified); + simplified.push(points[index], points[index + 1]); + last - index > 2 && simplifyStep(points, index, last, tolerance, simplified); + } +} //points is an array of x/y values like [x, y, x, y, x, y] + + +export function simplifyPoints(points, tolerance) { + var prevX = parseFloat(points[0]), + prevY = parseFloat(points[1]), + temp = [prevX, prevY], + l = points.length - 2, + i, + x, + y, + dx, + dy, + result, + last; + tolerance = Math.pow(tolerance || 1, 2); + + for (i = 2; i < l; i += 2) { + x = parseFloat(points[i]); + y = parseFloat(points[i + 1]); + dx = prevX - x; + dy = prevY - y; + + if (dx * dx + dy * dy > tolerance) { + temp.push(x, y); + prevX = x; + prevY = y; + } + } + + temp.push(parseFloat(points[l]), parseFloat(points[l + 1])); + last = temp.length - 2; + result = [temp[0], temp[1]]; + simplifyStep(temp, 0, last, tolerance, result); + result.push(temp[last], temp[last + 1]); + return result; +} + +function getClosestProgressOnBezier(iterations, px, py, start, end, slices, x0, y0, x1, y1, x2, y2, x3, y3) { + var inc = (end - start) / slices, + best = 0, + t = start, + x, + y, + d, + dx, + dy, + inv; + _bestDistance = _largeNum; + + while (t <= end) { + inv = 1 - t; + x = inv * inv * inv * x0 + 3 * inv * inv * t * x1 + 3 * inv * t * t * x2 + t * t * t * x3; + y = inv * inv * inv * y0 + 3 * inv * inv * t * y1 + 3 * inv * t * t * y2 + t * t * t * y3; + dx = x - px; + dy = y - py; + d = dx * dx + dy * dy; + + if (d < _bestDistance) { + _bestDistance = d; + best = t; + } + + t += inc; + } + + return iterations > 1 ? getClosestProgressOnBezier(iterations - 1, px, py, Math.max(best - inc, 0), Math.min(best + inc, 1), slices, x0, y0, x1, y1, x2, y2, x3, y3) : best; +} + +export function getClosestData(rawPath, x, y, slices) { + //returns an object with the closest j, i, and t (j is the segment index, i is the index of the point in that segment, and t is the time/progress along that bezier) + var closest = { + j: 0, + i: 0, + t: 0 + }, + bestDistance = _largeNum, + i, + j, + t, + segment; + + for (j = 0; j < rawPath.length; j++) { + segment = rawPath[j]; + + for (i = 0; i < segment.length; i += 6) { + t = getClosestProgressOnBezier(1, x, y, 0, 1, slices || 20, segment[i], segment[i + 1], segment[i + 2], segment[i + 3], segment[i + 4], segment[i + 5], segment[i + 6], segment[i + 7]); + + if (bestDistance > _bestDistance) { + bestDistance = _bestDistance; + closest.j = j; + closest.i = i; + closest.t = t; + } + } + } + + return closest; +} //subdivide a Segment closest to a specific x,y coordinate + +export function subdivideSegmentNear(x, y, segment, slices, iterations) { + var l = segment.length, + bestDistance = _largeNum, + bestT = 0, + bestSegmentIndex = 0, + t, + i; + slices = slices || 20; + iterations = iterations || 3; + + for (i = 0; i < l; i += 6) { + t = getClosestProgressOnBezier(1, x, y, 0, 1, slices, segment[i], segment[i + 1], segment[i + 2], segment[i + 3], segment[i + 4], segment[i + 5], segment[i + 6], segment[i + 7]); + + if (bestDistance > _bestDistance) { + bestDistance = _bestDistance; + bestT = t; + bestSegmentIndex = i; + } + } + + t = getClosestProgressOnBezier(iterations, x, y, bestT - 0.05, bestT + 0.05, slices, segment[bestSegmentIndex], segment[bestSegmentIndex + 1], segment[bestSegmentIndex + 2], segment[bestSegmentIndex + 3], segment[bestSegmentIndex + 4], segment[bestSegmentIndex + 5], segment[bestSegmentIndex + 6], segment[bestSegmentIndex + 7]); + subdivideSegment(segment, bestSegmentIndex, t); + return bestSegmentIndex + 6; +} +/* +Takes any of the following and converts it to an all Cubic Bezier SVG data string: +- A <path> data string like "M0,0 L2,4 v20,15 H100" +- A RawPath, like [[x, y, x, y, x, y, x, y][[x, y, x, y, x, y, x, y]] +- A Segment, like [x, y, x, y, x, y, x, y] + +Note: all numbers are rounded down to the closest 0.001 to minimize memory, maximize speed, and avoid odd numbers like 1e-13 +*/ + +export function rawPathToString(rawPath) { + if (_isNumber(rawPath[0])) { + //in case a segment is passed in instead + rawPath = [rawPath]; + } + + var result = "", + l = rawPath.length, + sl, + s, + i, + segment; + + for (s = 0; s < l; s++) { + segment = rawPath[s]; + result += "M" + _round(segment[0]) + "," + _round(segment[1]) + " C"; + sl = segment.length; + + for (i = 2; i < sl; i++) { + result += _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i++]) + " " + _round(segment[i++]) + "," + _round(segment[i]) + " "; + } + + if (segment.closed) { + result += "z"; + } + } + + return result; +} +/* +// takes a segment with coordinates [x, y, x, y, ...] and converts the control points into angles and lengths [x, y, angle, length, angle, length, x, y, angle, length, ...] so that it animates more cleanly and avoids odd breaks/kinks. For example, if you animate from 1 o'clock to 6 o'clock, it'd just go directly/linearly rather than around. So the length would be very short in the middle of the tween. +export function cpCoordsToAngles(segment, copy) { + var result = copy ? segment.slice(0) : segment, + x, y, i; + for (i = 0; i < segment.length; i+=6) { + x = segment[i+2] - segment[i]; + y = segment[i+3] - segment[i+1]; + result[i+2] = Math.atan2(y, x); + result[i+3] = Math.sqrt(x * x + y * y); + x = segment[i+6] - segment[i+4]; + y = segment[i+7] - segment[i+5]; + result[i+4] = Math.atan2(y, x); + result[i+5] = Math.sqrt(x * x + y * y); + } + return result; +} + +// takes a segment that was converted with cpCoordsToAngles() to have angles and lengths instead of coordinates for the control points, and converts it BACK into coordinates. +export function cpAnglesToCoords(segment, copy) { + var result = copy ? segment.slice(0) : segment, + length = segment.length, + rnd = 1000, + angle, l, i, j; + for (i = 0; i < length; i+=6) { + angle = segment[i+2]; + l = segment[i+3]; //length + result[i+2] = (((segment[i] + Math.cos(angle) * l) * rnd) | 0) / rnd; + result[i+3] = (((segment[i+1] + Math.sin(angle) * l) * rnd) | 0) / rnd; + angle = segment[i+4]; + l = segment[i+5]; //length + result[i+4] = (((segment[i+6] - Math.cos(angle) * l) * rnd) | 0) / rnd; + result[i+5] = (((segment[i+7] - Math.sin(angle) * l) * rnd) | 0) / rnd; + } + return result; +} + +//adds an "isSmooth" array to each segment and populates it with a boolean value indicating whether or not it's smooth (the control points have basically the same slope). For any smooth control points, it converts the coordinates into angle (x, in radians) and length (y) and puts them into the same index value in a smoothData array. +export function populateSmoothData(rawPath) { + let j = rawPath.length, + smooth, segment, x, y, x2, y2, i, l, a, a2, isSmooth, smoothData; + while (--j > -1) { + segment = rawPath[j]; + isSmooth = segment.isSmooth = segment.isSmooth || [0, 0, 0, 0]; + smoothData = segment.smoothData = segment.smoothData || [0, 0, 0, 0]; + isSmooth.length = 4; + l = segment.length - 2; + for (i = 6; i < l; i += 6) { + x = segment[i] - segment[i - 2]; + y = segment[i + 1] - segment[i - 1]; + x2 = segment[i + 2] - segment[i]; + y2 = segment[i + 3] - segment[i + 1]; + a = _atan2(y, x); + a2 = _atan2(y2, x2); + smooth = (Math.abs(a - a2) < 0.09); + if (smooth) { + smoothData[i - 2] = a; + smoothData[i + 2] = a2; + smoothData[i - 1] = _sqrt(x * x + y * y); + smoothData[i + 3] = _sqrt(x2 * x2 + y2 * y2); + } + isSmooth.push(smooth, smooth, 0, 0, smooth, smooth); + } + //if the first and last points are identical, check to see if there's a smooth transition. We must handle this a bit differently due to their positions in the array. + if (segment[l] === segment[0] && segment[l+1] === segment[1]) { + x = segment[0] - segment[l-2]; + y = segment[1] - segment[l-1]; + x2 = segment[2] - segment[0]; + y2 = segment[3] - segment[1]; + a = _atan2(y, x); + a2 = _atan2(y2, x2); + if (Math.abs(a - a2) < 0.09) { + smoothData[l-2] = a; + smoothData[2] = a2; + smoothData[l-1] = _sqrt(x * x + y * y); + smoothData[3] = _sqrt(x2 * x2 + y2 * y2); + isSmooth[l-2] = isSmooth[l-1] = true; //don't change indexes 2 and 3 because we'll trigger everything from the END, and this will optimize file size a bit. + } + } + } + return rawPath; +} +export function pointToScreen(svgElement, point) { + if (arguments.length < 2) { //by default, take the first set of coordinates in the path as the point + let rawPath = getRawPath(svgElement); + point = svgElement.ownerSVGElement.createSVGPoint(); + point.x = rawPath[0][0]; + point.y = rawPath[0][1]; + } + return point.matrixTransform(svgElement.getScreenCTM()); +} +// takes a <path> and normalizes all of its coordinates to values between 0 and 1 +export function normalizePath(path) { + path = gsap.utils.toArray(path); + if (!path[0].hasAttribute("d")) { + path = gsap.utils.toArray(path[0].children); + } + if (path.length > 1) { + path.forEach(normalizePath); + return path; + } + let _svgPathExp = /[achlmqstvz]|(-?\d*\.?\d*(?:e[\-+]?\d+)?)[0-9]/ig, + _scientific = /[\+\-]?\d*\.?\d+e[\+\-]?\d+/ig, + d = path[0].getAttribute("d"), + a = d.replace(_scientific, m => { let n = +m; return (n < 0.0001 && n > -0.0001) ? 0 : n; }).match(_svgPathExp), + nums = a.filter(n => !isNaN(n)).map(n => +n), + normalize = gsap.utils.normalize(Math.min(...nums), Math.max(...nums)), + finals = a.map(val => isNaN(val) ? val : normalize(+val)), + s = "", + prevWasCommand; + finals.forEach((value, i) => { + let isCommand = isNaN(value) + s += (isCommand && i ? " " : prevWasCommand || !i ? "" : ",") + value; + prevWasCommand = isCommand; + }); + path[0].setAttribute("d", s); +} +*/ \ No newline at end of file diff --git a/node_modules/gsap/utils/strings.js b/node_modules/gsap/utils/strings.js new file mode 100644 index 0000000000000000000000000000000000000000..a5aaa62eeb271d2d733dc2d600989787f6660b17 --- /dev/null +++ b/node_modules/gsap/utils/strings.js @@ -0,0 +1,109 @@ +/*! + * strings: 3.12.7 + * https://gsap.com + * + * Copyright 2008-2025, GreenSock. All rights reserved. + * Subject to the terms at https://gsap.com/standard-license or for + * Club GSAP members, the agreement issued with that membership. + * @author: Jack Doyle, jack@greensock.com +*/ + +/* eslint-disable */ +var _trimExp = /(?:^\s+|\s+$)/g; +export var emojiExp = /([\uD800-\uDBFF][\uDC00-\uDFFF](?:[\u200D\uFE0F][\uD800-\uDBFF][\uDC00-\uDFFF]){2,}|\uD83D\uDC69(?:\u200D(?:(?:\uD83D\uDC69\u200D)?\uD83D\uDC67|(?:\uD83D\uDC69\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D(?:\uD83D\uDC69\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2642\u2640]\uFE0F|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDD27\uDCBC\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC6F\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3C-\uDD3E\uDDD6-\uDDDF])\u200D[\u2640\u2642]\uFE0F|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|(?:\u26F9|\uD83C[\uDFCC\uDFCB]|\uD83D\uDD75)(?:\uFE0F\u200D[\u2640\u2642]|(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642])\uFE0F|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83D\uDC69(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\uD83D\uDC69\u200D[\u2695\u2696\u2708]|\uD83D\uDC68(?:(?:\uD83C[\uDFFB-\uDFFF])\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708]))\uFE0F|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83D\uDC69\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|[#\*0-9]\uFE0F\u20E3|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|\uD83D\uDC68(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66\u200D\uD83D\uDC66|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92])|(?:\uD83C[\uDFFB-\uDFFF])\u200D(?:\uD83C[\uDF3E\uDF73\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]))|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uD83C[\uDFFB-\uDFFF])|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD37-\uDD39\uDD3D\uDD3E\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270A-\u270D]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC70\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDCAA\uDD74\uDD7A\uDD90\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD36\uDDD1-\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\u200D(?:(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC67|(?:(?:\uD83D[\uDC68\uDC69])\u200D)?\uD83D\uDC66)|\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC69\uDC6E\uDC70-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD18-\uDD1C\uDD1E\uDD1F\uDD26\uDD30-\uDD39\uDD3D\uDD3E\uDDD1-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])?|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDEEB\uDEEC\uDEF4-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u2660\u2663\u2665\u2666\u2668\u267B\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEF8]|\uD83E[\uDD10-\uDD3A\uDD3C-\uDD3E\uDD40-\uDD45\uDD47-\uDD4C\uDD50-\uDD6B\uDD80-\uDD97\uDDC0\uDDD0-\uDDE6])\uFE0F)/; +export function getText(e) { + var type = e.nodeType, + result = ""; + + if (type === 1 || type === 9 || type === 11) { + if (typeof e.textContent === "string") { + return e.textContent; + } else { + for (e = e.firstChild; e; e = e.nextSibling) { + result += getText(e); + } + } + } else if (type === 3 || type === 4) { + return e.nodeValue; + } + + return result; +} +export function splitInnerHTML(element, delimiter, trim, preserveSpaces, unescapedCharCodes) { + var node = element.firstChild, + result = [], + s; + + while (node) { + if (node.nodeType === 3) { + s = (node.nodeValue + "").replace(/^\n+/g, ""); + + if (!preserveSpaces) { + s = s.replace(/\s+/g, " "); + } + + result.push.apply(result, emojiSafeSplit(s, delimiter, trim, preserveSpaces, unescapedCharCodes)); + } else if ((node.nodeName + "").toLowerCase() === "br") { + result[result.length - 1] += "<br>"; + } else { + result.push(node.outerHTML); + } + + node = node.nextSibling; + } + + if (!unescapedCharCodes) { + s = result.length; + + while (s--) { + result[s] === "&" && result.splice(s, 1, "&"); + } + } + + return result; +} +/* +//smaller kb version that only handles the simpler emoji's, which is often perfectly adequate. + +let _emoji = "[\uE000-\uF8FF]|\uD83C[\uDC00-\uDFFF]|\uD83D[\uDC00-\uDFFF]|[\u2694-\u2697]|\uD83E[\uDD10-\uDD5D]|[\uD800-\uDBFF][\uDC00-\uDFFF]", + _emojiExp = new RegExp(_emoji), + _emojiAndCharsExp = new RegExp(_emoji + "|.", "g"), + _emojiSafeSplit = (text, delimiter, trim) => { + if (trim) { + text = text.replace(_trimExp, ""); + } + return ((delimiter === "" || !delimiter) && _emojiExp.test(text)) ? text.match(_emojiAndCharsExp) : text.split(delimiter || ""); + }; + */ + +export function emojiSafeSplit(text, delimiter, trim, preserveSpaces, unescapedCharCodes) { + text += ""; // make sure it's cast as a string. Someone may pass in a number. + + trim && (text = text.trim ? text.trim() : text.replace(_trimExp, "")); // IE9 and earlier compatibility + + if (delimiter && delimiter !== "") { + return text.replace(/>/g, ">").replace(/</g, "<").split(delimiter); + } + + var result = [], + l = text.length, + i = 0, + j, + character; + + for (; i < l; i++) { + character = text.charAt(i); + + if (character.charCodeAt(0) >= 0xD800 && character.charCodeAt(0) <= 0xDBFF || text.charCodeAt(i + 1) >= 0xFE00 && text.charCodeAt(i + 1) <= 0xFE0F) { + //special emoji characters use 2 or 4 unicode characters that we must keep together. + j = ((text.substr(i, 12).split(emojiExp) || [])[1] || "").length || 2; + character = text.substr(i, j); + result.emoji = 1; + i += j - 1; + } + + result.push(unescapedCharCodes ? character : character === ">" ? ">" : character === "<" ? "<" : preserveSpaces && character === " " && (text.charAt(i - 1) === " " || text.charAt(i + 1) === " ") ? " " : character); + } + + return result; +} \ No newline at end of file diff --git a/package-lock.json b/package-lock.json new file mode 100644 index 0000000000000000000000000000000000000000..a185f1504d28b0b4a1abd98b329c9aae749e16a8 --- /dev/null +++ b/package-lock.json @@ -0,0 +1,18 @@ +{ + "name": "Personal-Portfolio", + "lockfileVersion": 3, + "requires": true, + "packages": { + "": { + "dependencies": { + "gsap": "^3.12.7" + } + }, + "node_modules/gsap": { + "version": "3.12.7", + "resolved": "https://registry.npmjs.org/gsap/-/gsap-3.12.7.tgz", + "integrity": "sha512-V4GsyVamhmKefvcAKaoy0h6si0xX7ogwBoBSs2CTJwt7luW0oZzC0LhdkyuKV8PJAXr7Yaj8pMjCKD4GJ+eEMg==", + "license": "Standard 'no charge' license: https://gsap.com/standard-license. Club GSAP members get more: https://gsap.com/licensing/. Why GreenSock doesn't employ an MIT license: https://gsap.com/why-license/" + } + } +} diff --git a/package.json b/package.json new file mode 100644 index 0000000000000000000000000000000000000000..f109e84601565f16839efb88479d5f775a487961 --- /dev/null +++ b/package.json @@ -0,0 +1,5 @@ +{ + "dependencies": { + "gsap": "^3.12.7" + } +} diff --git a/projects.html b/projects.html index e170bf283c5cbc12b77fd4142178a09896c8370e..c16ebba1a999bd60be498ad19678b6f1c32a19d3 100644 --- a/projects.html +++ b/projects.html @@ -7,6 +7,8 @@ <link rel="stylesheet" href="css/styles-projects.css" /> </head> <body> + + <!-- Full-screen hero section --> <section class="hero" id="hero"> <h1 class="projects-header">Projects</h1> @@ -63,6 +65,13 @@ </article> </section> + <div class="svg-container"> + <svg width="1150" height="6473" viewBox="0 0 1150 6473" fill="none" xmlns="http://www.w3.org/2000/svg"> + <path d="M564.5 0.5C660.973 180.081 660.973 342.919 564.5 522.5C459.381 718.174 177.342 615.601 75.4998 813C-116.257 1184.68 927.238 1635.1 1058.5 1238C1087.5 1150.26 1092.29 1094.27 1081.5 1002.5C1033.28 592.43 297.791 1072.13 121.5 1445.5C57.7801 1580.45 -15.1503 1682.06 42.9998 1819.5C85.7648 1920.58 152.632 1959.82 251 2008.5C507.881 2135.62 704.455 1605.11 943 1764C1092.06 1863.29 1165.15 1996.87 1146.5 2175C1129.15 2340.68 1041.38 2437.9 897 2521C671.716 2650.66 466.749 2275.78 241.5 2405.5C25.6333 2529.82 21.542 2835.18 1.49992 2996.5C-23.2263 3195.52 657 3319.5 657 3319.5C657 3319.5 969.856 3346.62 1026.5 3665.5C1048.58 3789.81 1074.56 3911.79 971 3984C853.999 4065.58 766.945 3875.88 624.5 3868.5C392.207 3856.47 186.633 4303.84 75.4998 4099.5C22.1364 4001.38 52.2727 3922.75 75.4998 3813.5C172.67 3356.46 1058.44 4148 971 4607C900.591 4976.59 127.318 4793.87 186 5165.5C245.763 5543.98 1072.45 5045.57 1132.5 5424C1194.58 5815.27 2.02861 5593.4 241.5 5909C334.743 6031.89 509.176 5972.06 583 6107.5C651.123 6232.49 583 6472 583 6472" stroke="white" stroke-width="5"/> + </svg> + + </div> + <!-- Intersection Observer to trigger animation on scroll --> <script> // Select all project articles @@ -89,15 +98,9 @@ }); </script> - <!-- Optional scroll effect similar to home page --> - <script> - document.addEventListener("scroll", function() { - const hero = document.getElementById("hero"); - const scrollPos = window.scrollY; - const maxScroll = 300; - const scale = 1 - Math.min((scrollPos / maxScroll) * 0.2, 0.2); - hero.style.transform = `scale(${scale})`; - }); - </script> + <script src="https://cdn.jsdelivr.net/npm/gsap@3.12.5/dist/gsap.min.js"></script> + <script src="https://cdn.jsdelivr.net/npm/gsap@3.12.5/dist/ScrollTrigger.min.js"></script> + <script src="js/projects.js"></script> + </body> </html>